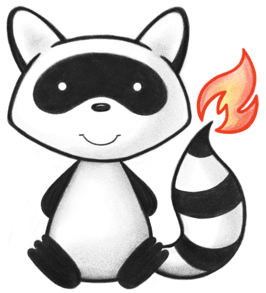
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * An action that is or was performed on or for a patient. This can be a 047 * physical intervention like an operation, or less invasive like long term 048 * services, counseling, or hypnotherapy. 049 */ 050@ResourceDef(name = "Procedure", profile = "http://hl7.org/fhir/StructureDefinition/Procedure") 051public class Procedure extends DomainResource { 052 053 public enum ProcedureStatus { 054 /** 055 * The core event has not started yet, but some staging activities have begun 056 * (e.g. surgical suite preparation). Preparation stages may be tracked for 057 * billing purposes. 058 */ 059 PREPARATION, 060 /** 061 * The event is currently occurring. 062 */ 063 INPROGRESS, 064 /** 065 * The event was terminated prior to any activity beyond preparation. I.e. The 066 * 'main' activity has not yet begun. The boundary between preparatory and the 067 * 'main' activity is context-specific. 068 */ 069 NOTDONE, 070 /** 071 * The event has been temporarily stopped but is expected to resume in the 072 * future. 073 */ 074 ONHOLD, 075 /** 076 * The event was terminated prior to the full completion of the intended 077 * activity but after at least some of the 'main' activity (beyond preparation) 078 * has occurred. 079 */ 080 STOPPED, 081 /** 082 * The event has now concluded. 083 */ 084 COMPLETED, 085 /** 086 * This electronic record should never have existed, though it is possible that 087 * real-world decisions were based on it. (If real-world activity has occurred, 088 * the status should be "stopped" rather than "entered-in-error".). 089 */ 090 ENTEREDINERROR, 091 /** 092 * The authoring/source system does not know which of the status values 093 * currently applies for this event. Note: This concept is not to be used for 094 * "other" - one of the listed statuses is presumed to apply, but the 095 * authoring/source system does not know which. 096 */ 097 UNKNOWN, 098 /** 099 * added to help the parsers with the generic types 100 */ 101 NULL; 102 103 public static ProcedureStatus fromCode(String codeString) throws FHIRException { 104 if (codeString == null || "".equals(codeString)) 105 return null; 106 if ("preparation".equals(codeString)) 107 return PREPARATION; 108 if ("in-progress".equals(codeString)) 109 return INPROGRESS; 110 if ("not-done".equals(codeString)) 111 return NOTDONE; 112 if ("on-hold".equals(codeString)) 113 return ONHOLD; 114 if ("stopped".equals(codeString)) 115 return STOPPED; 116 if ("completed".equals(codeString)) 117 return COMPLETED; 118 if ("entered-in-error".equals(codeString)) 119 return ENTEREDINERROR; 120 if ("unknown".equals(codeString)) 121 return UNKNOWN; 122 if (Configuration.isAcceptInvalidEnums()) 123 return null; 124 else 125 throw new FHIRException("Unknown ProcedureStatus code '" + codeString + "'"); 126 } 127 128 public String toCode() { 129 switch (this) { 130 case PREPARATION: 131 return "preparation"; 132 case INPROGRESS: 133 return "in-progress"; 134 case NOTDONE: 135 return "not-done"; 136 case ONHOLD: 137 return "on-hold"; 138 case STOPPED: 139 return "stopped"; 140 case COMPLETED: 141 return "completed"; 142 case ENTEREDINERROR: 143 return "entered-in-error"; 144 case UNKNOWN: 145 return "unknown"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 153 public String getSystem() { 154 switch (this) { 155 case PREPARATION: 156 return "http://hl7.org/fhir/event-status"; 157 case INPROGRESS: 158 return "http://hl7.org/fhir/event-status"; 159 case NOTDONE: 160 return "http://hl7.org/fhir/event-status"; 161 case ONHOLD: 162 return "http://hl7.org/fhir/event-status"; 163 case STOPPED: 164 return "http://hl7.org/fhir/event-status"; 165 case COMPLETED: 166 return "http://hl7.org/fhir/event-status"; 167 case ENTEREDINERROR: 168 return "http://hl7.org/fhir/event-status"; 169 case UNKNOWN: 170 return "http://hl7.org/fhir/event-status"; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178 public String getDefinition() { 179 switch (this) { 180 case PREPARATION: 181 return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 182 case INPROGRESS: 183 return "The event is currently occurring."; 184 case NOTDONE: 185 return "The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific."; 186 case ONHOLD: 187 return "The event has been temporarily stopped but is expected to resume in the future."; 188 case STOPPED: 189 return "The event was terminated prior to the full completion of the intended activity but after at least some of the 'main' activity (beyond preparation) has occurred."; 190 case COMPLETED: 191 return "The event has now concluded."; 192 case ENTEREDINERROR: 193 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".)."; 194 case UNKNOWN: 195 return "The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 196 case NULL: 197 return null; 198 default: 199 return "?"; 200 } 201 } 202 203 public String getDisplay() { 204 switch (this) { 205 case PREPARATION: 206 return "Preparation"; 207 case INPROGRESS: 208 return "In Progress"; 209 case NOTDONE: 210 return "Not Done"; 211 case ONHOLD: 212 return "On Hold"; 213 case STOPPED: 214 return "Stopped"; 215 case COMPLETED: 216 return "Completed"; 217 case ENTEREDINERROR: 218 return "Entered in Error"; 219 case UNKNOWN: 220 return "Unknown"; 221 case NULL: 222 return null; 223 default: 224 return "?"; 225 } 226 } 227 } 228 229 public static class ProcedureStatusEnumFactory implements EnumFactory<ProcedureStatus> { 230 public ProcedureStatus fromCode(String codeString) throws IllegalArgumentException { 231 if (codeString == null || "".equals(codeString)) 232 if (codeString == null || "".equals(codeString)) 233 return null; 234 if ("preparation".equals(codeString)) 235 return ProcedureStatus.PREPARATION; 236 if ("in-progress".equals(codeString)) 237 return ProcedureStatus.INPROGRESS; 238 if ("not-done".equals(codeString)) 239 return ProcedureStatus.NOTDONE; 240 if ("on-hold".equals(codeString)) 241 return ProcedureStatus.ONHOLD; 242 if ("stopped".equals(codeString)) 243 return ProcedureStatus.STOPPED; 244 if ("completed".equals(codeString)) 245 return ProcedureStatus.COMPLETED; 246 if ("entered-in-error".equals(codeString)) 247 return ProcedureStatus.ENTEREDINERROR; 248 if ("unknown".equals(codeString)) 249 return ProcedureStatus.UNKNOWN; 250 throw new IllegalArgumentException("Unknown ProcedureStatus code '" + codeString + "'"); 251 } 252 253 public Enumeration<ProcedureStatus> fromType(PrimitiveType<?> code) throws FHIRException { 254 if (code == null) 255 return null; 256 if (code.isEmpty()) 257 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.NULL, code); 258 String codeString = code.asStringValue(); 259 if (codeString == null || "".equals(codeString)) 260 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.NULL, code); 261 if ("preparation".equals(codeString)) 262 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.PREPARATION, code); 263 if ("in-progress".equals(codeString)) 264 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.INPROGRESS, code); 265 if ("not-done".equals(codeString)) 266 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.NOTDONE, code); 267 if ("on-hold".equals(codeString)) 268 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.ONHOLD, code); 269 if ("stopped".equals(codeString)) 270 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.STOPPED, code); 271 if ("completed".equals(codeString)) 272 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.COMPLETED, code); 273 if ("entered-in-error".equals(codeString)) 274 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.ENTEREDINERROR, code); 275 if ("unknown".equals(codeString)) 276 return new Enumeration<ProcedureStatus>(this, ProcedureStatus.UNKNOWN, code); 277 throw new FHIRException("Unknown ProcedureStatus code '" + codeString + "'"); 278 } 279 280 public String toCode(ProcedureStatus code) { 281 if (code == ProcedureStatus.NULL) 282 return null; 283 if (code == ProcedureStatus.PREPARATION) 284 return "preparation"; 285 if (code == ProcedureStatus.INPROGRESS) 286 return "in-progress"; 287 if (code == ProcedureStatus.NOTDONE) 288 return "not-done"; 289 if (code == ProcedureStatus.ONHOLD) 290 return "on-hold"; 291 if (code == ProcedureStatus.STOPPED) 292 return "stopped"; 293 if (code == ProcedureStatus.COMPLETED) 294 return "completed"; 295 if (code == ProcedureStatus.ENTEREDINERROR) 296 return "entered-in-error"; 297 if (code == ProcedureStatus.UNKNOWN) 298 return "unknown"; 299 return "?"; 300 } 301 302 public String toSystem(ProcedureStatus code) { 303 return code.getSystem(); 304 } 305 } 306 307 @Block() 308 public static class ProcedurePerformerComponent extends BackboneElement implements IBaseBackboneElement { 309 /** 310 * Distinguishes the type of involvement of the performer in the procedure. For 311 * example, surgeon, anaesthetist, endoscopist. 312 */ 313 @Child(name = "function", type = { 314 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 315 @Description(shortDefinition = "Type of performance", formalDefinition = "Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist.") 316 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/performer-role") 317 protected CodeableConcept function; 318 319 /** 320 * The practitioner who was involved in the procedure. 321 */ 322 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 323 RelatedPerson.class, Device.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 324 @Description(shortDefinition = "The reference to the practitioner", formalDefinition = "The practitioner who was involved in the procedure.") 325 protected Reference actor; 326 327 /** 328 * The actual object that is the target of the reference (The practitioner who 329 * was involved in the procedure.) 330 */ 331 protected Resource actorTarget; 332 333 /** 334 * The organization the device or practitioner was acting on behalf of. 335 */ 336 @Child(name = "onBehalfOf", type = { 337 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 338 @Description(shortDefinition = "Organization the device or practitioner was acting for", formalDefinition = "The organization the device or practitioner was acting on behalf of.") 339 protected Reference onBehalfOf; 340 341 /** 342 * The actual object that is the target of the reference (The organization the 343 * device or practitioner was acting on behalf of.) 344 */ 345 protected Organization onBehalfOfTarget; 346 347 private static final long serialVersionUID = -997772724L; 348 349 /** 350 * Constructor 351 */ 352 public ProcedurePerformerComponent() { 353 super(); 354 } 355 356 /** 357 * Constructor 358 */ 359 public ProcedurePerformerComponent(Reference actor) { 360 super(); 361 this.actor = actor; 362 } 363 364 /** 365 * @return {@link #function} (Distinguishes the type of involvement of the 366 * performer in the procedure. For example, surgeon, anaesthetist, 367 * endoscopist.) 368 */ 369 public CodeableConcept getFunction() { 370 if (this.function == null) 371 if (Configuration.errorOnAutoCreate()) 372 throw new Error("Attempt to auto-create ProcedurePerformerComponent.function"); 373 else if (Configuration.doAutoCreate()) 374 this.function = new CodeableConcept(); // cc 375 return this.function; 376 } 377 378 public boolean hasFunction() { 379 return this.function != null && !this.function.isEmpty(); 380 } 381 382 /** 383 * @param value {@link #function} (Distinguishes the type of involvement of the 384 * performer in the procedure. For example, surgeon, anaesthetist, 385 * endoscopist.) 386 */ 387 public ProcedurePerformerComponent setFunction(CodeableConcept value) { 388 this.function = value; 389 return this; 390 } 391 392 /** 393 * @return {@link #actor} (The practitioner who was involved in the procedure.) 394 */ 395 public Reference getActor() { 396 if (this.actor == null) 397 if (Configuration.errorOnAutoCreate()) 398 throw new Error("Attempt to auto-create ProcedurePerformerComponent.actor"); 399 else if (Configuration.doAutoCreate()) 400 this.actor = new Reference(); // cc 401 return this.actor; 402 } 403 404 public boolean hasActor() { 405 return this.actor != null && !this.actor.isEmpty(); 406 } 407 408 /** 409 * @param value {@link #actor} (The practitioner who was involved in the 410 * procedure.) 411 */ 412 public ProcedurePerformerComponent setActor(Reference value) { 413 this.actor = value; 414 return this; 415 } 416 417 /** 418 * @return {@link #actor} The actual object that is the target of the reference. 419 * The reference library doesn't populate this, but you can use it to 420 * hold the resource if you resolve it. (The practitioner who was 421 * involved in the procedure.) 422 */ 423 public Resource getActorTarget() { 424 return this.actorTarget; 425 } 426 427 /** 428 * @param value {@link #actor} The actual object that is the target of the 429 * reference. The reference library doesn't use these, but you can 430 * use it to hold the resource if you resolve it. (The practitioner 431 * who was involved in the procedure.) 432 */ 433 public ProcedurePerformerComponent setActorTarget(Resource value) { 434 this.actorTarget = value; 435 return this; 436 } 437 438 /** 439 * @return {@link #onBehalfOf} (The organization the device or practitioner was 440 * acting on behalf of.) 441 */ 442 public Reference getOnBehalfOf() { 443 if (this.onBehalfOf == null) 444 if (Configuration.errorOnAutoCreate()) 445 throw new Error("Attempt to auto-create ProcedurePerformerComponent.onBehalfOf"); 446 else if (Configuration.doAutoCreate()) 447 this.onBehalfOf = new Reference(); // cc 448 return this.onBehalfOf; 449 } 450 451 public boolean hasOnBehalfOf() { 452 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 453 } 454 455 /** 456 * @param value {@link #onBehalfOf} (The organization the device or practitioner 457 * was acting on behalf of.) 458 */ 459 public ProcedurePerformerComponent setOnBehalfOf(Reference value) { 460 this.onBehalfOf = value; 461 return this; 462 } 463 464 /** 465 * @return {@link #onBehalfOf} The actual object that is the target of the 466 * reference. The reference library doesn't populate this, but you can 467 * use it to hold the resource if you resolve it. (The organization the 468 * device or practitioner was acting on behalf of.) 469 */ 470 public Organization getOnBehalfOfTarget() { 471 if (this.onBehalfOfTarget == null) 472 if (Configuration.errorOnAutoCreate()) 473 throw new Error("Attempt to auto-create ProcedurePerformerComponent.onBehalfOf"); 474 else if (Configuration.doAutoCreate()) 475 this.onBehalfOfTarget = new Organization(); // aa 476 return this.onBehalfOfTarget; 477 } 478 479 /** 480 * @param value {@link #onBehalfOf} The actual object that is the target of the 481 * reference. The reference library doesn't use these, but you can 482 * use it to hold the resource if you resolve it. (The organization 483 * the device or practitioner was acting on behalf of.) 484 */ 485 public ProcedurePerformerComponent setOnBehalfOfTarget(Organization value) { 486 this.onBehalfOfTarget = value; 487 return this; 488 } 489 490 protected void listChildren(List<Property> children) { 491 super.listChildren(children); 492 children.add(new Property("function", "CodeableConcept", 493 "Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist.", 494 0, 1, function)); 495 children.add( 496 new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 497 "The practitioner who was involved in the procedure.", 0, 1, actor)); 498 children.add(new Property("onBehalfOf", "Reference(Organization)", 499 "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 500 } 501 502 @Override 503 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 504 switch (_hash) { 505 case 1380938712: 506 /* function */ return new Property("function", "CodeableConcept", 507 "Distinguishes the type of involvement of the performer in the procedure. For example, surgeon, anaesthetist, endoscopist.", 508 0, 1, function); 509 case 92645877: 510 /* actor */ return new Property("actor", 511 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 512 "The practitioner who was involved in the procedure.", 0, 1, actor); 513 case -14402964: 514 /* onBehalfOf */ return new Property("onBehalfOf", "Reference(Organization)", 515 "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 516 default: 517 return super.getNamedProperty(_hash, _name, _checkValid); 518 } 519 520 } 521 522 @Override 523 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 524 switch (hash) { 525 case 1380938712: 526 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 527 case 92645877: 528 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 529 case -14402964: 530 /* onBehalfOf */ return this.onBehalfOf == null ? new Base[0] : new Base[] { this.onBehalfOf }; // Reference 531 default: 532 return super.getProperty(hash, name, checkValid); 533 } 534 535 } 536 537 @Override 538 public Base setProperty(int hash, String name, Base value) throws FHIRException { 539 switch (hash) { 540 case 1380938712: // function 541 this.function = castToCodeableConcept(value); // CodeableConcept 542 return value; 543 case 92645877: // actor 544 this.actor = castToReference(value); // Reference 545 return value; 546 case -14402964: // onBehalfOf 547 this.onBehalfOf = castToReference(value); // Reference 548 return value; 549 default: 550 return super.setProperty(hash, name, value); 551 } 552 553 } 554 555 @Override 556 public Base setProperty(String name, Base value) throws FHIRException { 557 if (name.equals("function")) { 558 this.function = castToCodeableConcept(value); // CodeableConcept 559 } else if (name.equals("actor")) { 560 this.actor = castToReference(value); // Reference 561 } else if (name.equals("onBehalfOf")) { 562 this.onBehalfOf = castToReference(value); // Reference 563 } else 564 return super.setProperty(name, value); 565 return value; 566 } 567 568 @Override 569 public void removeChild(String name, Base value) throws FHIRException { 570 if (name.equals("function")) { 571 this.function = null; 572 } else if (name.equals("actor")) { 573 this.actor = null; 574 } else if (name.equals("onBehalfOf")) { 575 this.onBehalfOf = null; 576 } else 577 super.removeChild(name, value); 578 579 } 580 581 @Override 582 public Base makeProperty(int hash, String name) throws FHIRException { 583 switch (hash) { 584 case 1380938712: 585 return getFunction(); 586 case 92645877: 587 return getActor(); 588 case -14402964: 589 return getOnBehalfOf(); 590 default: 591 return super.makeProperty(hash, name); 592 } 593 594 } 595 596 @Override 597 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 598 switch (hash) { 599 case 1380938712: 600 /* function */ return new String[] { "CodeableConcept" }; 601 case 92645877: 602 /* actor */ return new String[] { "Reference" }; 603 case -14402964: 604 /* onBehalfOf */ return new String[] { "Reference" }; 605 default: 606 return super.getTypesForProperty(hash, name); 607 } 608 609 } 610 611 @Override 612 public Base addChild(String name) throws FHIRException { 613 if (name.equals("function")) { 614 this.function = new CodeableConcept(); 615 return this.function; 616 } else if (name.equals("actor")) { 617 this.actor = new Reference(); 618 return this.actor; 619 } else if (name.equals("onBehalfOf")) { 620 this.onBehalfOf = new Reference(); 621 return this.onBehalfOf; 622 } else 623 return super.addChild(name); 624 } 625 626 public ProcedurePerformerComponent copy() { 627 ProcedurePerformerComponent dst = new ProcedurePerformerComponent(); 628 copyValues(dst); 629 return dst; 630 } 631 632 public void copyValues(ProcedurePerformerComponent dst) { 633 super.copyValues(dst); 634 dst.function = function == null ? null : function.copy(); 635 dst.actor = actor == null ? null : actor.copy(); 636 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 637 } 638 639 @Override 640 public boolean equalsDeep(Base other_) { 641 if (!super.equalsDeep(other_)) 642 return false; 643 if (!(other_ instanceof ProcedurePerformerComponent)) 644 return false; 645 ProcedurePerformerComponent o = (ProcedurePerformerComponent) other_; 646 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true) 647 && compareDeep(onBehalfOf, o.onBehalfOf, true); 648 } 649 650 @Override 651 public boolean equalsShallow(Base other_) { 652 if (!super.equalsShallow(other_)) 653 return false; 654 if (!(other_ instanceof ProcedurePerformerComponent)) 655 return false; 656 ProcedurePerformerComponent o = (ProcedurePerformerComponent) other_; 657 return true; 658 } 659 660 public boolean isEmpty() { 661 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor, onBehalfOf); 662 } 663 664 public String fhirType() { 665 return "Procedure.performer"; 666 667 } 668 669 } 670 671 @Block() 672 public static class ProcedureFocalDeviceComponent extends BackboneElement implements IBaseBackboneElement { 673 /** 674 * The kind of change that happened to the device during the procedure. 675 */ 676 @Child(name = "action", type = { 677 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 678 @Description(shortDefinition = "Kind of change to device", formalDefinition = "The kind of change that happened to the device during the procedure.") 679 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-action") 680 protected CodeableConcept action; 681 682 /** 683 * The device that was manipulated (changed) during the procedure. 684 */ 685 @Child(name = "manipulated", type = { 686 Device.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 687 @Description(shortDefinition = "Device that was changed", formalDefinition = "The device that was manipulated (changed) during the procedure.") 688 protected Reference manipulated; 689 690 /** 691 * The actual object that is the target of the reference (The device that was 692 * manipulated (changed) during the procedure.) 693 */ 694 protected Device manipulatedTarget; 695 696 private static final long serialVersionUID = 1779937807L; 697 698 /** 699 * Constructor 700 */ 701 public ProcedureFocalDeviceComponent() { 702 super(); 703 } 704 705 /** 706 * Constructor 707 */ 708 public ProcedureFocalDeviceComponent(Reference manipulated) { 709 super(); 710 this.manipulated = manipulated; 711 } 712 713 /** 714 * @return {@link #action} (The kind of change that happened to the device 715 * during the procedure.) 716 */ 717 public CodeableConcept getAction() { 718 if (this.action == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.action"); 721 else if (Configuration.doAutoCreate()) 722 this.action = new CodeableConcept(); // cc 723 return this.action; 724 } 725 726 public boolean hasAction() { 727 return this.action != null && !this.action.isEmpty(); 728 } 729 730 /** 731 * @param value {@link #action} (The kind of change that happened to the device 732 * during the procedure.) 733 */ 734 public ProcedureFocalDeviceComponent setAction(CodeableConcept value) { 735 this.action = value; 736 return this; 737 } 738 739 /** 740 * @return {@link #manipulated} (The device that was manipulated (changed) 741 * during the procedure.) 742 */ 743 public Reference getManipulated() { 744 if (this.manipulated == null) 745 if (Configuration.errorOnAutoCreate()) 746 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.manipulated"); 747 else if (Configuration.doAutoCreate()) 748 this.manipulated = new Reference(); // cc 749 return this.manipulated; 750 } 751 752 public boolean hasManipulated() { 753 return this.manipulated != null && !this.manipulated.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #manipulated} (The device that was manipulated (changed) 758 * during the procedure.) 759 */ 760 public ProcedureFocalDeviceComponent setManipulated(Reference value) { 761 this.manipulated = value; 762 return this; 763 } 764 765 /** 766 * @return {@link #manipulated} The actual object that is the target of the 767 * reference. The reference library doesn't populate this, but you can 768 * use it to hold the resource if you resolve it. (The device that was 769 * manipulated (changed) during the procedure.) 770 */ 771 public Device getManipulatedTarget() { 772 if (this.manipulatedTarget == null) 773 if (Configuration.errorOnAutoCreate()) 774 throw new Error("Attempt to auto-create ProcedureFocalDeviceComponent.manipulated"); 775 else if (Configuration.doAutoCreate()) 776 this.manipulatedTarget = new Device(); // aa 777 return this.manipulatedTarget; 778 } 779 780 /** 781 * @param value {@link #manipulated} The actual object that is the target of the 782 * reference. The reference library doesn't use these, but you can 783 * use it to hold the resource if you resolve it. (The device that 784 * was manipulated (changed) during the procedure.) 785 */ 786 public ProcedureFocalDeviceComponent setManipulatedTarget(Device value) { 787 this.manipulatedTarget = value; 788 return this; 789 } 790 791 protected void listChildren(List<Property> children) { 792 super.listChildren(children); 793 children.add(new Property("action", "CodeableConcept", 794 "The kind of change that happened to the device during the procedure.", 0, 1, action)); 795 children.add(new Property("manipulated", "Reference(Device)", 796 "The device that was manipulated (changed) during the procedure.", 0, 1, manipulated)); 797 } 798 799 @Override 800 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 801 switch (_hash) { 802 case -1422950858: 803 /* action */ return new Property("action", "CodeableConcept", 804 "The kind of change that happened to the device during the procedure.", 0, 1, action); 805 case 947372650: 806 /* manipulated */ return new Property("manipulated", "Reference(Device)", 807 "The device that was manipulated (changed) during the procedure.", 0, 1, manipulated); 808 default: 809 return super.getNamedProperty(_hash, _name, _checkValid); 810 } 811 812 } 813 814 @Override 815 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 816 switch (hash) { 817 case -1422950858: 818 /* action */ return this.action == null ? new Base[0] : new Base[] { this.action }; // CodeableConcept 819 case 947372650: 820 /* manipulated */ return this.manipulated == null ? new Base[0] : new Base[] { this.manipulated }; // Reference 821 default: 822 return super.getProperty(hash, name, checkValid); 823 } 824 825 } 826 827 @Override 828 public Base setProperty(int hash, String name, Base value) throws FHIRException { 829 switch (hash) { 830 case -1422950858: // action 831 this.action = castToCodeableConcept(value); // CodeableConcept 832 return value; 833 case 947372650: // manipulated 834 this.manipulated = castToReference(value); // Reference 835 return value; 836 default: 837 return super.setProperty(hash, name, value); 838 } 839 840 } 841 842 @Override 843 public Base setProperty(String name, Base value) throws FHIRException { 844 if (name.equals("action")) { 845 this.action = castToCodeableConcept(value); // CodeableConcept 846 } else if (name.equals("manipulated")) { 847 this.manipulated = castToReference(value); // Reference 848 } else 849 return super.setProperty(name, value); 850 return value; 851 } 852 853 @Override 854 public void removeChild(String name, Base value) throws FHIRException { 855 if (name.equals("action")) { 856 this.action = null; 857 } else if (name.equals("manipulated")) { 858 this.manipulated = null; 859 } else 860 super.removeChild(name, value); 861 862 } 863 864 @Override 865 public Base makeProperty(int hash, String name) throws FHIRException { 866 switch (hash) { 867 case -1422950858: 868 return getAction(); 869 case 947372650: 870 return getManipulated(); 871 default: 872 return super.makeProperty(hash, name); 873 } 874 875 } 876 877 @Override 878 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 879 switch (hash) { 880 case -1422950858: 881 /* action */ return new String[] { "CodeableConcept" }; 882 case 947372650: 883 /* manipulated */ return new String[] { "Reference" }; 884 default: 885 return super.getTypesForProperty(hash, name); 886 } 887 888 } 889 890 @Override 891 public Base addChild(String name) throws FHIRException { 892 if (name.equals("action")) { 893 this.action = new CodeableConcept(); 894 return this.action; 895 } else if (name.equals("manipulated")) { 896 this.manipulated = new Reference(); 897 return this.manipulated; 898 } else 899 return super.addChild(name); 900 } 901 902 public ProcedureFocalDeviceComponent copy() { 903 ProcedureFocalDeviceComponent dst = new ProcedureFocalDeviceComponent(); 904 copyValues(dst); 905 return dst; 906 } 907 908 public void copyValues(ProcedureFocalDeviceComponent dst) { 909 super.copyValues(dst); 910 dst.action = action == null ? null : action.copy(); 911 dst.manipulated = manipulated == null ? null : manipulated.copy(); 912 } 913 914 @Override 915 public boolean equalsDeep(Base other_) { 916 if (!super.equalsDeep(other_)) 917 return false; 918 if (!(other_ instanceof ProcedureFocalDeviceComponent)) 919 return false; 920 ProcedureFocalDeviceComponent o = (ProcedureFocalDeviceComponent) other_; 921 return compareDeep(action, o.action, true) && compareDeep(manipulated, o.manipulated, true); 922 } 923 924 @Override 925 public boolean equalsShallow(Base other_) { 926 if (!super.equalsShallow(other_)) 927 return false; 928 if (!(other_ instanceof ProcedureFocalDeviceComponent)) 929 return false; 930 ProcedureFocalDeviceComponent o = (ProcedureFocalDeviceComponent) other_; 931 return true; 932 } 933 934 public boolean isEmpty() { 935 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, manipulated); 936 } 937 938 public String fhirType() { 939 return "Procedure.focalDevice"; 940 941 } 942 943 } 944 945 /** 946 * Business identifiers assigned to this procedure by the performer or other 947 * systems which remain constant as the resource is updated and is propagated 948 * from server to server. 949 */ 950 @Child(name = "identifier", type = { 951 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 952 @Description(shortDefinition = "External Identifiers for this procedure", formalDefinition = "Business identifiers assigned to this procedure by the performer or other systems which remain constant as the resource is updated and is propagated from server to server.") 953 protected List<Identifier> identifier; 954 955 /** 956 * The URL pointing to a FHIR-defined protocol, guideline, order set or other 957 * definition that is adhered to in whole or in part by this Procedure. 958 */ 959 @Child(name = "instantiatesCanonical", type = { 960 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 961 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.") 962 protected List<CanonicalType> instantiatesCanonical; 963 964 /** 965 * The URL pointing to an externally maintained protocol, guideline, order set 966 * or other definition that is adhered to in whole or in part by this Procedure. 967 */ 968 @Child(name = "instantiatesUri", type = { 969 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 970 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.") 971 protected List<UriType> instantiatesUri; 972 973 /** 974 * A reference to a resource that contains details of the request for this 975 * procedure. 976 */ 977 @Child(name = "basedOn", type = { CarePlan.class, 978 ServiceRequest.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 979 @Description(shortDefinition = "A request for this procedure", formalDefinition = "A reference to a resource that contains details of the request for this procedure.") 980 protected List<Reference> basedOn; 981 /** 982 * The actual objects that are the target of the reference (A reference to a 983 * resource that contains details of the request for this procedure.) 984 */ 985 protected List<Resource> basedOnTarget; 986 987 /** 988 * A larger event of which this particular procedure is a component or step. 989 */ 990 @Child(name = "partOf", type = { Procedure.class, Observation.class, 991 MedicationAdministration.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 992 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular procedure is a component or step.") 993 protected List<Reference> partOf; 994 /** 995 * The actual objects that are the target of the reference (A larger event of 996 * which this particular procedure is a component or step.) 997 */ 998 protected List<Resource> partOfTarget; 999 1000 /** 1001 * A code specifying the state of the procedure. Generally, this will be the 1002 * in-progress or completed state. 1003 */ 1004 @Child(name = "status", type = { CodeType.class }, order = 5, min = 1, max = 1, modifier = true, summary = true) 1005 @Description(shortDefinition = "preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", formalDefinition = "A code specifying the state of the procedure. Generally, this will be the in-progress or completed state.") 1006 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-status") 1007 protected Enumeration<ProcedureStatus> status; 1008 1009 /** 1010 * Captures the reason for the current state of the procedure. 1011 */ 1012 @Child(name = "statusReason", type = { 1013 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1014 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the procedure.") 1015 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-not-performed-reason") 1016 protected CodeableConcept statusReason; 1017 1018 /** 1019 * A code that classifies the procedure for searching, sorting and display 1020 * purposes (e.g. "Surgical Procedure"). 1021 */ 1022 @Child(name = "category", type = { 1023 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1024 @Description(shortDefinition = "Classification of the procedure", formalDefinition = "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").") 1025 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-category") 1026 protected CodeableConcept category; 1027 1028 /** 1029 * The specific procedure that is performed. Use text if the exact nature of the 1030 * procedure cannot be coded (e.g. "Laparoscopic Appendectomy"). 1031 */ 1032 @Child(name = "code", type = { CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1033 @Description(shortDefinition = "Identification of the procedure", formalDefinition = "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").") 1034 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-code") 1035 protected CodeableConcept code; 1036 1037 /** 1038 * The person, animal or group on which the procedure was performed. 1039 */ 1040 @Child(name = "subject", type = { Patient.class, 1041 Group.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 1042 @Description(shortDefinition = "Who the procedure was performed on", formalDefinition = "The person, animal or group on which the procedure was performed.") 1043 protected Reference subject; 1044 1045 /** 1046 * The actual object that is the target of the reference (The person, animal or 1047 * group on which the procedure was performed.) 1048 */ 1049 protected Resource subjectTarget; 1050 1051 /** 1052 * The Encounter during which this Procedure was created or performed or to 1053 * which the creation of this record is tightly associated. 1054 */ 1055 @Child(name = "encounter", type = { Encounter.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1056 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated.") 1057 protected Reference encounter; 1058 1059 /** 1060 * The actual object that is the target of the reference (The Encounter during 1061 * which this Procedure was created or performed or to which the creation of 1062 * this record is tightly associated.) 1063 */ 1064 protected Encounter encounterTarget; 1065 1066 /** 1067 * Estimated or actual date, date-time, period, or age when the procedure was 1068 * performed. Allows a period to support complex procedures that span more than 1069 * one date, and also allows for the length of the procedure to be captured. 1070 */ 1071 @Child(name = "performed", type = { DateTimeType.class, Period.class, StringType.class, Age.class, 1072 Range.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1073 @Description(shortDefinition = "When the procedure was performed", formalDefinition = "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.") 1074 protected Type performed; 1075 1076 /** 1077 * Individual who recorded the record and takes responsibility for its content. 1078 */ 1079 @Child(name = "recorder", type = { Patient.class, RelatedPerson.class, Practitioner.class, 1080 PractitionerRole.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1081 @Description(shortDefinition = "Who recorded the procedure", formalDefinition = "Individual who recorded the record and takes responsibility for its content.") 1082 protected Reference recorder; 1083 1084 /** 1085 * The actual object that is the target of the reference (Individual who 1086 * recorded the record and takes responsibility for its content.) 1087 */ 1088 protected Resource recorderTarget; 1089 1090 /** 1091 * Individual who is making the procedure statement. 1092 */ 1093 @Child(name = "asserter", type = { Patient.class, RelatedPerson.class, Practitioner.class, 1094 PractitionerRole.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1095 @Description(shortDefinition = "Person who asserts this procedure", formalDefinition = "Individual who is making the procedure statement.") 1096 protected Reference asserter; 1097 1098 /** 1099 * The actual object that is the target of the reference (Individual who is 1100 * making the procedure statement.) 1101 */ 1102 protected Resource asserterTarget; 1103 1104 /** 1105 * Limited to "real" people rather than equipment. 1106 */ 1107 @Child(name = "performer", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1108 @Description(shortDefinition = "The people who performed the procedure", formalDefinition = "Limited to \"real\" people rather than equipment.") 1109 protected List<ProcedurePerformerComponent> performer; 1110 1111 /** 1112 * The location where the procedure actually happened. E.g. a newborn at home, a 1113 * tracheostomy at a restaurant. 1114 */ 1115 @Child(name = "location", type = { Location.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 1116 @Description(shortDefinition = "Where the procedure happened", formalDefinition = "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.") 1117 protected Reference location; 1118 1119 /** 1120 * The actual object that is the target of the reference (The location where the 1121 * procedure actually happened. E.g. a newborn at home, a tracheostomy at a 1122 * restaurant.) 1123 */ 1124 protected Location locationTarget; 1125 1126 /** 1127 * The coded reason why the procedure was performed. This may be a coded entity 1128 * of some type, or may simply be present as text. 1129 */ 1130 @Child(name = "reasonCode", type = { 1131 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1132 @Description(shortDefinition = "Coded reason procedure performed", formalDefinition = "The coded reason why the procedure was performed. This may be a coded entity of some type, or may simply be present as text.") 1133 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-reason") 1134 protected List<CodeableConcept> reasonCode; 1135 1136 /** 1137 * The justification of why the procedure was performed. 1138 */ 1139 @Child(name = "reasonReference", type = { Condition.class, Observation.class, Procedure.class, DiagnosticReport.class, 1140 DocumentReference.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1141 @Description(shortDefinition = "The justification that the procedure was performed", formalDefinition = "The justification of why the procedure was performed.") 1142 protected List<Reference> reasonReference; 1143 /** 1144 * The actual objects that are the target of the reference (The justification of 1145 * why the procedure was performed.) 1146 */ 1147 protected List<Resource> reasonReferenceTarget; 1148 1149 /** 1150 * Detailed and structured anatomical location information. Multiple locations 1151 * are allowed - e.g. multiple punch biopsies of a lesion. 1152 */ 1153 @Child(name = "bodySite", type = { 1154 CodeableConcept.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1155 @Description(shortDefinition = "Target body sites", formalDefinition = "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.") 1156 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 1157 protected List<CodeableConcept> bodySite; 1158 1159 /** 1160 * The outcome of the procedure - did it resolve the reasons for the procedure 1161 * being performed? 1162 */ 1163 @Child(name = "outcome", type = { 1164 CodeableConcept.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 1165 @Description(shortDefinition = "The result of procedure", formalDefinition = "The outcome of the procedure - did it resolve the reasons for the procedure being performed?") 1166 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-outcome") 1167 protected CodeableConcept outcome; 1168 1169 /** 1170 * This could be a histology result, pathology report, surgical report, etc. 1171 */ 1172 @Child(name = "report", type = { DiagnosticReport.class, DocumentReference.class, 1173 Composition.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1174 @Description(shortDefinition = "Any report resulting from the procedure", formalDefinition = "This could be a histology result, pathology report, surgical report, etc.") 1175 protected List<Reference> report; 1176 /** 1177 * The actual objects that are the target of the reference (This could be a 1178 * histology result, pathology report, surgical report, etc.) 1179 */ 1180 protected List<Resource> reportTarget; 1181 1182 /** 1183 * Any complications that occurred during the procedure, or in the immediate 1184 * post-performance period. These are generally tracked separately from the 1185 * notes, which will typically describe the procedure itself rather than any 1186 * 'post procedure' issues. 1187 */ 1188 @Child(name = "complication", type = { 1189 CodeableConcept.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1190 @Description(shortDefinition = "Complication following the procedure", formalDefinition = "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.") 1191 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 1192 protected List<CodeableConcept> complication; 1193 1194 /** 1195 * Any complications that occurred during the procedure, or in the immediate 1196 * post-performance period. 1197 */ 1198 @Child(name = "complicationDetail", type = { 1199 Condition.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1200 @Description(shortDefinition = "A condition that is a result of the procedure", formalDefinition = "Any complications that occurred during the procedure, or in the immediate post-performance period.") 1201 protected List<Reference> complicationDetail; 1202 /** 1203 * The actual objects that are the target of the reference (Any complications 1204 * that occurred during the procedure, or in the immediate post-performance 1205 * period.) 1206 */ 1207 protected List<Condition> complicationDetailTarget; 1208 1209 /** 1210 * If the procedure required specific follow up - e.g. removal of sutures. The 1211 * follow up may be represented as a simple note or could potentially be more 1212 * complex, in which case the CarePlan resource can be used. 1213 */ 1214 @Child(name = "followUp", type = { 1215 CodeableConcept.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1216 @Description(shortDefinition = "Instructions for follow up", formalDefinition = "If the procedure required specific follow up - e.g. removal of sutures. The follow up may be represented as a simple note or could potentially be more complex, in which case the CarePlan resource can be used.") 1217 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-followup") 1218 protected List<CodeableConcept> followUp; 1219 1220 /** 1221 * Any other notes and comments about the procedure. 1222 */ 1223 @Child(name = "note", type = { 1224 Annotation.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1225 @Description(shortDefinition = "Additional information about the procedure", formalDefinition = "Any other notes and comments about the procedure.") 1226 protected List<Annotation> note; 1227 1228 /** 1229 * A device that is implanted, removed or otherwise manipulated (calibration, 1230 * battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a 1231 * focal portion of the Procedure. 1232 */ 1233 @Child(name = "focalDevice", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1234 @Description(shortDefinition = "Manipulated, implanted, or removed device", formalDefinition = "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.") 1235 protected List<ProcedureFocalDeviceComponent> focalDevice; 1236 1237 /** 1238 * Identifies medications, devices and any other substance used as part of the 1239 * procedure. 1240 */ 1241 @Child(name = "usedReference", type = { Device.class, Medication.class, 1242 Substance.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1243 @Description(shortDefinition = "Items used during procedure", formalDefinition = "Identifies medications, devices and any other substance used as part of the procedure.") 1244 protected List<Reference> usedReference; 1245 /** 1246 * The actual objects that are the target of the reference (Identifies 1247 * medications, devices and any other substance used as part of the procedure.) 1248 */ 1249 protected List<Resource> usedReferenceTarget; 1250 1251 /** 1252 * Identifies coded items that were used as part of the procedure. 1253 */ 1254 @Child(name = "usedCode", type = { 1255 CodeableConcept.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1256 @Description(shortDefinition = "Coded items used during the procedure", formalDefinition = "Identifies coded items that were used as part of the procedure.") 1257 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-kind") 1258 protected List<CodeableConcept> usedCode; 1259 1260 private static final long serialVersionUID = -29072720L; 1261 1262 /** 1263 * Constructor 1264 */ 1265 public Procedure() { 1266 super(); 1267 } 1268 1269 /** 1270 * Constructor 1271 */ 1272 public Procedure(Enumeration<ProcedureStatus> status, Reference subject) { 1273 super(); 1274 this.status = status; 1275 this.subject = subject; 1276 } 1277 1278 /** 1279 * @return {@link #identifier} (Business identifiers assigned to this procedure 1280 * by the performer or other systems which remain constant as the 1281 * resource is updated and is propagated from server to server.) 1282 */ 1283 public List<Identifier> getIdentifier() { 1284 if (this.identifier == null) 1285 this.identifier = new ArrayList<Identifier>(); 1286 return this.identifier; 1287 } 1288 1289 /** 1290 * @return Returns a reference to <code>this</code> for easy method chaining 1291 */ 1292 public Procedure setIdentifier(List<Identifier> theIdentifier) { 1293 this.identifier = theIdentifier; 1294 return this; 1295 } 1296 1297 public boolean hasIdentifier() { 1298 if (this.identifier == null) 1299 return false; 1300 for (Identifier item : this.identifier) 1301 if (!item.isEmpty()) 1302 return true; 1303 return false; 1304 } 1305 1306 public Identifier addIdentifier() { // 3 1307 Identifier t = new Identifier(); 1308 if (this.identifier == null) 1309 this.identifier = new ArrayList<Identifier>(); 1310 this.identifier.add(t); 1311 return t; 1312 } 1313 1314 public Procedure addIdentifier(Identifier t) { // 3 1315 if (t == null) 1316 return this; 1317 if (this.identifier == null) 1318 this.identifier = new ArrayList<Identifier>(); 1319 this.identifier.add(t); 1320 return this; 1321 } 1322 1323 /** 1324 * @return The first repetition of repeating field {@link #identifier}, creating 1325 * it if it does not already exist 1326 */ 1327 public Identifier getIdentifierFirstRep() { 1328 if (getIdentifier().isEmpty()) { 1329 addIdentifier(); 1330 } 1331 return getIdentifier().get(0); 1332 } 1333 1334 /** 1335 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1336 * protocol, guideline, order set or other definition that is adhered to 1337 * in whole or in part by this Procedure.) 1338 */ 1339 public List<CanonicalType> getInstantiatesCanonical() { 1340 if (this.instantiatesCanonical == null) 1341 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1342 return this.instantiatesCanonical; 1343 } 1344 1345 /** 1346 * @return Returns a reference to <code>this</code> for easy method chaining 1347 */ 1348 public Procedure setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1349 this.instantiatesCanonical = theInstantiatesCanonical; 1350 return this; 1351 } 1352 1353 public boolean hasInstantiatesCanonical() { 1354 if (this.instantiatesCanonical == null) 1355 return false; 1356 for (CanonicalType item : this.instantiatesCanonical) 1357 if (!item.isEmpty()) 1358 return true; 1359 return false; 1360 } 1361 1362 /** 1363 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1364 * protocol, guideline, order set or other definition that is adhered to 1365 * in whole or in part by this Procedure.) 1366 */ 1367 public CanonicalType addInstantiatesCanonicalElement() {// 2 1368 CanonicalType t = new CanonicalType(); 1369 if (this.instantiatesCanonical == null) 1370 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1371 this.instantiatesCanonical.add(t); 1372 return t; 1373 } 1374 1375 /** 1376 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1377 * FHIR-defined protocol, guideline, order set or other definition 1378 * that is adhered to in whole or in part by this Procedure.) 1379 */ 1380 public Procedure addInstantiatesCanonical(String value) { // 1 1381 CanonicalType t = new CanonicalType(); 1382 t.setValue(value); 1383 if (this.instantiatesCanonical == null) 1384 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1385 this.instantiatesCanonical.add(t); 1386 return this; 1387 } 1388 1389 /** 1390 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1391 * FHIR-defined protocol, guideline, order set or other definition 1392 * that is adhered to in whole or in part by this Procedure.) 1393 */ 1394 public boolean hasInstantiatesCanonical(String value) { 1395 if (this.instantiatesCanonical == null) 1396 return false; 1397 for (CanonicalType v : this.instantiatesCanonical) 1398 if (v.getValue().equals(value)) // canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire) 1399 return true; 1400 return false; 1401 } 1402 1403 /** 1404 * @return {@link #instantiatesUri} (The URL pointing to an externally 1405 * maintained protocol, guideline, order set or other definition that is 1406 * adhered to in whole or in part by this Procedure.) 1407 */ 1408 public List<UriType> getInstantiatesUri() { 1409 if (this.instantiatesUri == null) 1410 this.instantiatesUri = new ArrayList<UriType>(); 1411 return this.instantiatesUri; 1412 } 1413 1414 /** 1415 * @return Returns a reference to <code>this</code> for easy method chaining 1416 */ 1417 public Procedure setInstantiatesUri(List<UriType> theInstantiatesUri) { 1418 this.instantiatesUri = theInstantiatesUri; 1419 return this; 1420 } 1421 1422 public boolean hasInstantiatesUri() { 1423 if (this.instantiatesUri == null) 1424 return false; 1425 for (UriType item : this.instantiatesUri) 1426 if (!item.isEmpty()) 1427 return true; 1428 return false; 1429 } 1430 1431 /** 1432 * @return {@link #instantiatesUri} (The URL pointing to an externally 1433 * maintained protocol, guideline, order set or other definition that is 1434 * adhered to in whole or in part by this Procedure.) 1435 */ 1436 public UriType addInstantiatesUriElement() {// 2 1437 UriType t = new UriType(); 1438 if (this.instantiatesUri == null) 1439 this.instantiatesUri = new ArrayList<UriType>(); 1440 this.instantiatesUri.add(t); 1441 return t; 1442 } 1443 1444 /** 1445 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1446 * maintained protocol, guideline, order set or other definition 1447 * that is adhered to in whole or in part by this Procedure.) 1448 */ 1449 public Procedure addInstantiatesUri(String value) { // 1 1450 UriType t = new UriType(); 1451 t.setValue(value); 1452 if (this.instantiatesUri == null) 1453 this.instantiatesUri = new ArrayList<UriType>(); 1454 this.instantiatesUri.add(t); 1455 return this; 1456 } 1457 1458 /** 1459 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1460 * maintained protocol, guideline, order set or other definition 1461 * that is adhered to in whole or in part by this Procedure.) 1462 */ 1463 public boolean hasInstantiatesUri(String value) { 1464 if (this.instantiatesUri == null) 1465 return false; 1466 for (UriType v : this.instantiatesUri) 1467 if (v.getValue().equals(value)) // uri 1468 return true; 1469 return false; 1470 } 1471 1472 /** 1473 * @return {@link #basedOn} (A reference to a resource that contains details of 1474 * the request for this procedure.) 1475 */ 1476 public List<Reference> getBasedOn() { 1477 if (this.basedOn == null) 1478 this.basedOn = new ArrayList<Reference>(); 1479 return this.basedOn; 1480 } 1481 1482 /** 1483 * @return Returns a reference to <code>this</code> for easy method chaining 1484 */ 1485 public Procedure setBasedOn(List<Reference> theBasedOn) { 1486 this.basedOn = theBasedOn; 1487 return this; 1488 } 1489 1490 public boolean hasBasedOn() { 1491 if (this.basedOn == null) 1492 return false; 1493 for (Reference item : this.basedOn) 1494 if (!item.isEmpty()) 1495 return true; 1496 return false; 1497 } 1498 1499 public Reference addBasedOn() { // 3 1500 Reference t = new Reference(); 1501 if (this.basedOn == null) 1502 this.basedOn = new ArrayList<Reference>(); 1503 this.basedOn.add(t); 1504 return t; 1505 } 1506 1507 public Procedure addBasedOn(Reference t) { // 3 1508 if (t == null) 1509 return this; 1510 if (this.basedOn == null) 1511 this.basedOn = new ArrayList<Reference>(); 1512 this.basedOn.add(t); 1513 return this; 1514 } 1515 1516 /** 1517 * @return The first repetition of repeating field {@link #basedOn}, creating it 1518 * if it does not already exist 1519 */ 1520 public Reference getBasedOnFirstRep() { 1521 if (getBasedOn().isEmpty()) { 1522 addBasedOn(); 1523 } 1524 return getBasedOn().get(0); 1525 } 1526 1527 /** 1528 * @deprecated Use Reference#setResource(IBaseResource) instead 1529 */ 1530 @Deprecated 1531 public List<Resource> getBasedOnTarget() { 1532 if (this.basedOnTarget == null) 1533 this.basedOnTarget = new ArrayList<Resource>(); 1534 return this.basedOnTarget; 1535 } 1536 1537 /** 1538 * @return {@link #partOf} (A larger event of which this particular procedure is 1539 * a component or step.) 1540 */ 1541 public List<Reference> getPartOf() { 1542 if (this.partOf == null) 1543 this.partOf = new ArrayList<Reference>(); 1544 return this.partOf; 1545 } 1546 1547 /** 1548 * @return Returns a reference to <code>this</code> for easy method chaining 1549 */ 1550 public Procedure setPartOf(List<Reference> thePartOf) { 1551 this.partOf = thePartOf; 1552 return this; 1553 } 1554 1555 public boolean hasPartOf() { 1556 if (this.partOf == null) 1557 return false; 1558 for (Reference item : this.partOf) 1559 if (!item.isEmpty()) 1560 return true; 1561 return false; 1562 } 1563 1564 public Reference addPartOf() { // 3 1565 Reference t = new Reference(); 1566 if (this.partOf == null) 1567 this.partOf = new ArrayList<Reference>(); 1568 this.partOf.add(t); 1569 return t; 1570 } 1571 1572 public Procedure addPartOf(Reference t) { // 3 1573 if (t == null) 1574 return this; 1575 if (this.partOf == null) 1576 this.partOf = new ArrayList<Reference>(); 1577 this.partOf.add(t); 1578 return this; 1579 } 1580 1581 /** 1582 * @return The first repetition of repeating field {@link #partOf}, creating it 1583 * if it does not already exist 1584 */ 1585 public Reference getPartOfFirstRep() { 1586 if (getPartOf().isEmpty()) { 1587 addPartOf(); 1588 } 1589 return getPartOf().get(0); 1590 } 1591 1592 /** 1593 * @deprecated Use Reference#setResource(IBaseResource) instead 1594 */ 1595 @Deprecated 1596 public List<Resource> getPartOfTarget() { 1597 if (this.partOfTarget == null) 1598 this.partOfTarget = new ArrayList<Resource>(); 1599 return this.partOfTarget; 1600 } 1601 1602 /** 1603 * @return {@link #status} (A code specifying the state of the procedure. 1604 * Generally, this will be the in-progress or completed state.). This is 1605 * the underlying object with id, value and extensions. The accessor 1606 * "getStatus" gives direct access to the value 1607 */ 1608 public Enumeration<ProcedureStatus> getStatusElement() { 1609 if (this.status == null) 1610 if (Configuration.errorOnAutoCreate()) 1611 throw new Error("Attempt to auto-create Procedure.status"); 1612 else if (Configuration.doAutoCreate()) 1613 this.status = new Enumeration<ProcedureStatus>(new ProcedureStatusEnumFactory()); // bb 1614 return this.status; 1615 } 1616 1617 public boolean hasStatusElement() { 1618 return this.status != null && !this.status.isEmpty(); 1619 } 1620 1621 public boolean hasStatus() { 1622 return this.status != null && !this.status.isEmpty(); 1623 } 1624 1625 /** 1626 * @param value {@link #status} (A code specifying the state of the procedure. 1627 * Generally, this will be the in-progress or completed state.). 1628 * This is the underlying object with id, value and extensions. The 1629 * accessor "getStatus" gives direct access to the value 1630 */ 1631 public Procedure setStatusElement(Enumeration<ProcedureStatus> value) { 1632 this.status = value; 1633 return this; 1634 } 1635 1636 /** 1637 * @return A code specifying the state of the procedure. Generally, this will be 1638 * the in-progress or completed state. 1639 */ 1640 public ProcedureStatus getStatus() { 1641 return this.status == null ? null : this.status.getValue(); 1642 } 1643 1644 /** 1645 * @param value A code specifying the state of the procedure. Generally, this 1646 * will be the in-progress or completed state. 1647 */ 1648 public Procedure setStatus(ProcedureStatus value) { 1649 if (this.status == null) 1650 this.status = new Enumeration<ProcedureStatus>(new ProcedureStatusEnumFactory()); 1651 this.status.setValue(value); 1652 return this; 1653 } 1654 1655 /** 1656 * @return {@link #statusReason} (Captures the reason for the current state of 1657 * the procedure.) 1658 */ 1659 public CodeableConcept getStatusReason() { 1660 if (this.statusReason == null) 1661 if (Configuration.errorOnAutoCreate()) 1662 throw new Error("Attempt to auto-create Procedure.statusReason"); 1663 else if (Configuration.doAutoCreate()) 1664 this.statusReason = new CodeableConcept(); // cc 1665 return this.statusReason; 1666 } 1667 1668 public boolean hasStatusReason() { 1669 return this.statusReason != null && !this.statusReason.isEmpty(); 1670 } 1671 1672 /** 1673 * @param value {@link #statusReason} (Captures the reason for the current state 1674 * of the procedure.) 1675 */ 1676 public Procedure setStatusReason(CodeableConcept value) { 1677 this.statusReason = value; 1678 return this; 1679 } 1680 1681 /** 1682 * @return {@link #category} (A code that classifies the procedure for 1683 * searching, sorting and display purposes (e.g. "Surgical Procedure").) 1684 */ 1685 public CodeableConcept getCategory() { 1686 if (this.category == null) 1687 if (Configuration.errorOnAutoCreate()) 1688 throw new Error("Attempt to auto-create Procedure.category"); 1689 else if (Configuration.doAutoCreate()) 1690 this.category = new CodeableConcept(); // cc 1691 return this.category; 1692 } 1693 1694 public boolean hasCategory() { 1695 return this.category != null && !this.category.isEmpty(); 1696 } 1697 1698 /** 1699 * @param value {@link #category} (A code that classifies the procedure for 1700 * searching, sorting and display purposes (e.g. "Surgical 1701 * Procedure").) 1702 */ 1703 public Procedure setCategory(CodeableConcept value) { 1704 this.category = value; 1705 return this; 1706 } 1707 1708 /** 1709 * @return {@link #code} (The specific procedure that is performed. Use text if 1710 * the exact nature of the procedure cannot be coded (e.g. "Laparoscopic 1711 * Appendectomy").) 1712 */ 1713 public CodeableConcept getCode() { 1714 if (this.code == null) 1715 if (Configuration.errorOnAutoCreate()) 1716 throw new Error("Attempt to auto-create Procedure.code"); 1717 else if (Configuration.doAutoCreate()) 1718 this.code = new CodeableConcept(); // cc 1719 return this.code; 1720 } 1721 1722 public boolean hasCode() { 1723 return this.code != null && !this.code.isEmpty(); 1724 } 1725 1726 /** 1727 * @param value {@link #code} (The specific procedure that is performed. Use 1728 * text if the exact nature of the procedure cannot be coded (e.g. 1729 * "Laparoscopic Appendectomy").) 1730 */ 1731 public Procedure setCode(CodeableConcept value) { 1732 this.code = value; 1733 return this; 1734 } 1735 1736 /** 1737 * @return {@link #subject} (The person, animal or group on which the procedure 1738 * was performed.) 1739 */ 1740 public Reference getSubject() { 1741 if (this.subject == null) 1742 if (Configuration.errorOnAutoCreate()) 1743 throw new Error("Attempt to auto-create Procedure.subject"); 1744 else if (Configuration.doAutoCreate()) 1745 this.subject = new Reference(); // cc 1746 return this.subject; 1747 } 1748 1749 public boolean hasSubject() { 1750 return this.subject != null && !this.subject.isEmpty(); 1751 } 1752 1753 /** 1754 * @param value {@link #subject} (The person, animal or group on which the 1755 * procedure was performed.) 1756 */ 1757 public Procedure setSubject(Reference value) { 1758 this.subject = value; 1759 return this; 1760 } 1761 1762 /** 1763 * @return {@link #subject} The actual object that is the target of the 1764 * reference. The reference library doesn't populate this, but you can 1765 * use it to hold the resource if you resolve it. (The person, animal or 1766 * group on which the procedure was performed.) 1767 */ 1768 public Resource getSubjectTarget() { 1769 return this.subjectTarget; 1770 } 1771 1772 /** 1773 * @param value {@link #subject} The actual object that is the target of the 1774 * reference. The reference library doesn't use these, but you can 1775 * use it to hold the resource if you resolve it. (The person, 1776 * animal or group on which the procedure was performed.) 1777 */ 1778 public Procedure setSubjectTarget(Resource value) { 1779 this.subjectTarget = value; 1780 return this; 1781 } 1782 1783 /** 1784 * @return {@link #encounter} (The Encounter during which this Procedure was 1785 * created or performed or to which the creation of this record is 1786 * tightly associated.) 1787 */ 1788 public Reference getEncounter() { 1789 if (this.encounter == null) 1790 if (Configuration.errorOnAutoCreate()) 1791 throw new Error("Attempt to auto-create Procedure.encounter"); 1792 else if (Configuration.doAutoCreate()) 1793 this.encounter = new Reference(); // cc 1794 return this.encounter; 1795 } 1796 1797 public boolean hasEncounter() { 1798 return this.encounter != null && !this.encounter.isEmpty(); 1799 } 1800 1801 /** 1802 * @param value {@link #encounter} (The Encounter during which this Procedure 1803 * was created or performed or to which the creation of this record 1804 * is tightly associated.) 1805 */ 1806 public Procedure setEncounter(Reference value) { 1807 this.encounter = value; 1808 return this; 1809 } 1810 1811 /** 1812 * @return {@link #encounter} The actual object that is the target of the 1813 * reference. The reference library doesn't populate this, but you can 1814 * use it to hold the resource if you resolve it. (The Encounter during 1815 * which this Procedure was created or performed or to which the 1816 * creation of this record is tightly associated.) 1817 */ 1818 public Encounter getEncounterTarget() { 1819 if (this.encounterTarget == null) 1820 if (Configuration.errorOnAutoCreate()) 1821 throw new Error("Attempt to auto-create Procedure.encounter"); 1822 else if (Configuration.doAutoCreate()) 1823 this.encounterTarget = new Encounter(); // aa 1824 return this.encounterTarget; 1825 } 1826 1827 /** 1828 * @param value {@link #encounter} The actual object that is the target of the 1829 * reference. The reference library doesn't use these, but you can 1830 * use it to hold the resource if you resolve it. (The Encounter 1831 * during which this Procedure was created or performed or to which 1832 * the creation of this record is tightly associated.) 1833 */ 1834 public Procedure setEncounterTarget(Encounter value) { 1835 this.encounterTarget = value; 1836 return this; 1837 } 1838 1839 /** 1840 * @return {@link #performed} (Estimated or actual date, date-time, period, or 1841 * age when the procedure was performed. Allows a period to support 1842 * complex procedures that span more than one date, and also allows for 1843 * the length of the procedure to be captured.) 1844 */ 1845 public Type getPerformed() { 1846 return this.performed; 1847 } 1848 1849 /** 1850 * @return {@link #performed} (Estimated or actual date, date-time, period, or 1851 * age when the procedure was performed. Allows a period to support 1852 * complex procedures that span more than one date, and also allows for 1853 * the length of the procedure to be captured.) 1854 */ 1855 public DateTimeType getPerformedDateTimeType() throws FHIRException { 1856 if (this.performed == null) 1857 this.performed = new DateTimeType(); 1858 if (!(this.performed instanceof DateTimeType)) 1859 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1860 + this.performed.getClass().getName() + " was encountered"); 1861 return (DateTimeType) this.performed; 1862 } 1863 1864 public boolean hasPerformedDateTimeType() { 1865 return this != null && this.performed instanceof DateTimeType; 1866 } 1867 1868 /** 1869 * @return {@link #performed} (Estimated or actual date, date-time, period, or 1870 * age when the procedure was performed. Allows a period to support 1871 * complex procedures that span more than one date, and also allows for 1872 * the length of the procedure to be captured.) 1873 */ 1874 public Period getPerformedPeriod() throws FHIRException { 1875 if (this.performed == null) 1876 this.performed = new Period(); 1877 if (!(this.performed instanceof Period)) 1878 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.performed.getClass().getName() 1879 + " was encountered"); 1880 return (Period) this.performed; 1881 } 1882 1883 public boolean hasPerformedPeriod() { 1884 return this != null && this.performed instanceof Period; 1885 } 1886 1887 /** 1888 * @return {@link #performed} (Estimated or actual date, date-time, period, or 1889 * age when the procedure was performed. Allows a period to support 1890 * complex procedures that span more than one date, and also allows for 1891 * the length of the procedure to be captured.) 1892 */ 1893 public StringType getPerformedStringType() throws FHIRException { 1894 if (this.performed == null) 1895 this.performed = new StringType(); 1896 if (!(this.performed instanceof StringType)) 1897 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1898 + this.performed.getClass().getName() + " was encountered"); 1899 return (StringType) this.performed; 1900 } 1901 1902 public boolean hasPerformedStringType() { 1903 return this != null && this.performed instanceof StringType; 1904 } 1905 1906 /** 1907 * @return {@link #performed} (Estimated or actual date, date-time, period, or 1908 * age when the procedure was performed. Allows a period to support 1909 * complex procedures that span more than one date, and also allows for 1910 * the length of the procedure to be captured.) 1911 */ 1912 public Age getPerformedAge() throws FHIRException { 1913 if (this.performed == null) 1914 this.performed = new Age(); 1915 if (!(this.performed instanceof Age)) 1916 throw new FHIRException( 1917 "Type mismatch: the type Age was expected, but " + this.performed.getClass().getName() + " was encountered"); 1918 return (Age) this.performed; 1919 } 1920 1921 public boolean hasPerformedAge() { 1922 return this != null && this.performed instanceof Age; 1923 } 1924 1925 /** 1926 * @return {@link #performed} (Estimated or actual date, date-time, period, or 1927 * age when the procedure was performed. Allows a period to support 1928 * complex procedures that span more than one date, and also allows for 1929 * the length of the procedure to be captured.) 1930 */ 1931 public Range getPerformedRange() throws FHIRException { 1932 if (this.performed == null) 1933 this.performed = new Range(); 1934 if (!(this.performed instanceof Range)) 1935 throw new FHIRException("Type mismatch: the type Range was expected, but " + this.performed.getClass().getName() 1936 + " was encountered"); 1937 return (Range) this.performed; 1938 } 1939 1940 public boolean hasPerformedRange() { 1941 return this != null && this.performed instanceof Range; 1942 } 1943 1944 public boolean hasPerformed() { 1945 return this.performed != null && !this.performed.isEmpty(); 1946 } 1947 1948 /** 1949 * @param value {@link #performed} (Estimated or actual date, date-time, period, 1950 * or age when the procedure was performed. Allows a period to 1951 * support complex procedures that span more than one date, and 1952 * also allows for the length of the procedure to be captured.) 1953 */ 1954 public Procedure setPerformed(Type value) { 1955 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof StringType 1956 || value instanceof Age || value instanceof Range)) 1957 throw new Error("Not the right type for Procedure.performed[x]: " + value.fhirType()); 1958 this.performed = value; 1959 return this; 1960 } 1961 1962 /** 1963 * @return {@link #recorder} (Individual who recorded the record and takes 1964 * responsibility for its content.) 1965 */ 1966 public Reference getRecorder() { 1967 if (this.recorder == null) 1968 if (Configuration.errorOnAutoCreate()) 1969 throw new Error("Attempt to auto-create Procedure.recorder"); 1970 else if (Configuration.doAutoCreate()) 1971 this.recorder = new Reference(); // cc 1972 return this.recorder; 1973 } 1974 1975 public boolean hasRecorder() { 1976 return this.recorder != null && !this.recorder.isEmpty(); 1977 } 1978 1979 /** 1980 * @param value {@link #recorder} (Individual who recorded the record and takes 1981 * responsibility for its content.) 1982 */ 1983 public Procedure setRecorder(Reference value) { 1984 this.recorder = value; 1985 return this; 1986 } 1987 1988 /** 1989 * @return {@link #recorder} The actual object that is the target of the 1990 * reference. The reference library doesn't populate this, but you can 1991 * use it to hold the resource if you resolve it. (Individual who 1992 * recorded the record and takes responsibility for its content.) 1993 */ 1994 public Resource getRecorderTarget() { 1995 return this.recorderTarget; 1996 } 1997 1998 /** 1999 * @param value {@link #recorder} The actual object that is the target of the 2000 * reference. The reference library doesn't use these, but you can 2001 * use it to hold the resource if you resolve it. (Individual who 2002 * recorded the record and takes responsibility for its content.) 2003 */ 2004 public Procedure setRecorderTarget(Resource value) { 2005 this.recorderTarget = value; 2006 return this; 2007 } 2008 2009 /** 2010 * @return {@link #asserter} (Individual who is making the procedure statement.) 2011 */ 2012 public Reference getAsserter() { 2013 if (this.asserter == null) 2014 if (Configuration.errorOnAutoCreate()) 2015 throw new Error("Attempt to auto-create Procedure.asserter"); 2016 else if (Configuration.doAutoCreate()) 2017 this.asserter = new Reference(); // cc 2018 return this.asserter; 2019 } 2020 2021 public boolean hasAsserter() { 2022 return this.asserter != null && !this.asserter.isEmpty(); 2023 } 2024 2025 /** 2026 * @param value {@link #asserter} (Individual who is making the procedure 2027 * statement.) 2028 */ 2029 public Procedure setAsserter(Reference value) { 2030 this.asserter = value; 2031 return this; 2032 } 2033 2034 /** 2035 * @return {@link #asserter} The actual object that is the target of the 2036 * reference. The reference library doesn't populate this, but you can 2037 * use it to hold the resource if you resolve it. (Individual who is 2038 * making the procedure statement.) 2039 */ 2040 public Resource getAsserterTarget() { 2041 return this.asserterTarget; 2042 } 2043 2044 /** 2045 * @param value {@link #asserter} The actual object that is the target of the 2046 * reference. The reference library doesn't use these, but you can 2047 * use it to hold the resource if you resolve it. (Individual who 2048 * is making the procedure statement.) 2049 */ 2050 public Procedure setAsserterTarget(Resource value) { 2051 this.asserterTarget = value; 2052 return this; 2053 } 2054 2055 /** 2056 * @return {@link #performer} (Limited to "real" people rather than equipment.) 2057 */ 2058 public List<ProcedurePerformerComponent> getPerformer() { 2059 if (this.performer == null) 2060 this.performer = new ArrayList<ProcedurePerformerComponent>(); 2061 return this.performer; 2062 } 2063 2064 /** 2065 * @return Returns a reference to <code>this</code> for easy method chaining 2066 */ 2067 public Procedure setPerformer(List<ProcedurePerformerComponent> thePerformer) { 2068 this.performer = thePerformer; 2069 return this; 2070 } 2071 2072 public boolean hasPerformer() { 2073 if (this.performer == null) 2074 return false; 2075 for (ProcedurePerformerComponent item : this.performer) 2076 if (!item.isEmpty()) 2077 return true; 2078 return false; 2079 } 2080 2081 public ProcedurePerformerComponent addPerformer() { // 3 2082 ProcedurePerformerComponent t = new ProcedurePerformerComponent(); 2083 if (this.performer == null) 2084 this.performer = new ArrayList<ProcedurePerformerComponent>(); 2085 this.performer.add(t); 2086 return t; 2087 } 2088 2089 public Procedure addPerformer(ProcedurePerformerComponent t) { // 3 2090 if (t == null) 2091 return this; 2092 if (this.performer == null) 2093 this.performer = new ArrayList<ProcedurePerformerComponent>(); 2094 this.performer.add(t); 2095 return this; 2096 } 2097 2098 /** 2099 * @return The first repetition of repeating field {@link #performer}, creating 2100 * it if it does not already exist 2101 */ 2102 public ProcedurePerformerComponent getPerformerFirstRep() { 2103 if (getPerformer().isEmpty()) { 2104 addPerformer(); 2105 } 2106 return getPerformer().get(0); 2107 } 2108 2109 /** 2110 * @return {@link #location} (The location where the procedure actually 2111 * happened. E.g. a newborn at home, a tracheostomy at a restaurant.) 2112 */ 2113 public Reference getLocation() { 2114 if (this.location == null) 2115 if (Configuration.errorOnAutoCreate()) 2116 throw new Error("Attempt to auto-create Procedure.location"); 2117 else if (Configuration.doAutoCreate()) 2118 this.location = new Reference(); // cc 2119 return this.location; 2120 } 2121 2122 public boolean hasLocation() { 2123 return this.location != null && !this.location.isEmpty(); 2124 } 2125 2126 /** 2127 * @param value {@link #location} (The location where the procedure actually 2128 * happened. E.g. a newborn at home, a tracheostomy at a 2129 * restaurant.) 2130 */ 2131 public Procedure setLocation(Reference value) { 2132 this.location = value; 2133 return this; 2134 } 2135 2136 /** 2137 * @return {@link #location} The actual object that is the target of the 2138 * reference. The reference library doesn't populate this, but you can 2139 * use it to hold the resource if you resolve it. (The location where 2140 * the procedure actually happened. E.g. a newborn at home, a 2141 * tracheostomy at a restaurant.) 2142 */ 2143 public Location getLocationTarget() { 2144 if (this.locationTarget == null) 2145 if (Configuration.errorOnAutoCreate()) 2146 throw new Error("Attempt to auto-create Procedure.location"); 2147 else if (Configuration.doAutoCreate()) 2148 this.locationTarget = new Location(); // aa 2149 return this.locationTarget; 2150 } 2151 2152 /** 2153 * @param value {@link #location} The actual object that is the target of the 2154 * reference. The reference library doesn't use these, but you can 2155 * use it to hold the resource if you resolve it. (The location 2156 * where the procedure actually happened. E.g. a newborn at home, a 2157 * tracheostomy at a restaurant.) 2158 */ 2159 public Procedure setLocationTarget(Location value) { 2160 this.locationTarget = value; 2161 return this; 2162 } 2163 2164 /** 2165 * @return {@link #reasonCode} (The coded reason why the procedure was 2166 * performed. This may be a coded entity of some type, or may simply be 2167 * present as text.) 2168 */ 2169 public List<CodeableConcept> getReasonCode() { 2170 if (this.reasonCode == null) 2171 this.reasonCode = new ArrayList<CodeableConcept>(); 2172 return this.reasonCode; 2173 } 2174 2175 /** 2176 * @return Returns a reference to <code>this</code> for easy method chaining 2177 */ 2178 public Procedure setReasonCode(List<CodeableConcept> theReasonCode) { 2179 this.reasonCode = theReasonCode; 2180 return this; 2181 } 2182 2183 public boolean hasReasonCode() { 2184 if (this.reasonCode == null) 2185 return false; 2186 for (CodeableConcept item : this.reasonCode) 2187 if (!item.isEmpty()) 2188 return true; 2189 return false; 2190 } 2191 2192 public CodeableConcept addReasonCode() { // 3 2193 CodeableConcept t = new CodeableConcept(); 2194 if (this.reasonCode == null) 2195 this.reasonCode = new ArrayList<CodeableConcept>(); 2196 this.reasonCode.add(t); 2197 return t; 2198 } 2199 2200 public Procedure addReasonCode(CodeableConcept t) { // 3 2201 if (t == null) 2202 return this; 2203 if (this.reasonCode == null) 2204 this.reasonCode = new ArrayList<CodeableConcept>(); 2205 this.reasonCode.add(t); 2206 return this; 2207 } 2208 2209 /** 2210 * @return The first repetition of repeating field {@link #reasonCode}, creating 2211 * it if it does not already exist 2212 */ 2213 public CodeableConcept getReasonCodeFirstRep() { 2214 if (getReasonCode().isEmpty()) { 2215 addReasonCode(); 2216 } 2217 return getReasonCode().get(0); 2218 } 2219 2220 /** 2221 * @return {@link #reasonReference} (The justification of why the procedure was 2222 * performed.) 2223 */ 2224 public List<Reference> getReasonReference() { 2225 if (this.reasonReference == null) 2226 this.reasonReference = new ArrayList<Reference>(); 2227 return this.reasonReference; 2228 } 2229 2230 /** 2231 * @return Returns a reference to <code>this</code> for easy method chaining 2232 */ 2233 public Procedure setReasonReference(List<Reference> theReasonReference) { 2234 this.reasonReference = theReasonReference; 2235 return this; 2236 } 2237 2238 public boolean hasReasonReference() { 2239 if (this.reasonReference == null) 2240 return false; 2241 for (Reference item : this.reasonReference) 2242 if (!item.isEmpty()) 2243 return true; 2244 return false; 2245 } 2246 2247 public Reference addReasonReference() { // 3 2248 Reference t = new Reference(); 2249 if (this.reasonReference == null) 2250 this.reasonReference = new ArrayList<Reference>(); 2251 this.reasonReference.add(t); 2252 return t; 2253 } 2254 2255 public Procedure addReasonReference(Reference t) { // 3 2256 if (t == null) 2257 return this; 2258 if (this.reasonReference == null) 2259 this.reasonReference = new ArrayList<Reference>(); 2260 this.reasonReference.add(t); 2261 return this; 2262 } 2263 2264 /** 2265 * @return The first repetition of repeating field {@link #reasonReference}, 2266 * creating it if it does not already exist 2267 */ 2268 public Reference getReasonReferenceFirstRep() { 2269 if (getReasonReference().isEmpty()) { 2270 addReasonReference(); 2271 } 2272 return getReasonReference().get(0); 2273 } 2274 2275 /** 2276 * @deprecated Use Reference#setResource(IBaseResource) instead 2277 */ 2278 @Deprecated 2279 public List<Resource> getReasonReferenceTarget() { 2280 if (this.reasonReferenceTarget == null) 2281 this.reasonReferenceTarget = new ArrayList<Resource>(); 2282 return this.reasonReferenceTarget; 2283 } 2284 2285 /** 2286 * @return {@link #bodySite} (Detailed and structured anatomical location 2287 * information. Multiple locations are allowed - e.g. multiple punch 2288 * biopsies of a lesion.) 2289 */ 2290 public List<CodeableConcept> getBodySite() { 2291 if (this.bodySite == null) 2292 this.bodySite = new ArrayList<CodeableConcept>(); 2293 return this.bodySite; 2294 } 2295 2296 /** 2297 * @return Returns a reference to <code>this</code> for easy method chaining 2298 */ 2299 public Procedure setBodySite(List<CodeableConcept> theBodySite) { 2300 this.bodySite = theBodySite; 2301 return this; 2302 } 2303 2304 public boolean hasBodySite() { 2305 if (this.bodySite == null) 2306 return false; 2307 for (CodeableConcept item : this.bodySite) 2308 if (!item.isEmpty()) 2309 return true; 2310 return false; 2311 } 2312 2313 public CodeableConcept addBodySite() { // 3 2314 CodeableConcept t = new CodeableConcept(); 2315 if (this.bodySite == null) 2316 this.bodySite = new ArrayList<CodeableConcept>(); 2317 this.bodySite.add(t); 2318 return t; 2319 } 2320 2321 public Procedure addBodySite(CodeableConcept t) { // 3 2322 if (t == null) 2323 return this; 2324 if (this.bodySite == null) 2325 this.bodySite = new ArrayList<CodeableConcept>(); 2326 this.bodySite.add(t); 2327 return this; 2328 } 2329 2330 /** 2331 * @return The first repetition of repeating field {@link #bodySite}, creating 2332 * it if it does not already exist 2333 */ 2334 public CodeableConcept getBodySiteFirstRep() { 2335 if (getBodySite().isEmpty()) { 2336 addBodySite(); 2337 } 2338 return getBodySite().get(0); 2339 } 2340 2341 /** 2342 * @return {@link #outcome} (The outcome of the procedure - did it resolve the 2343 * reasons for the procedure being performed?) 2344 */ 2345 public CodeableConcept getOutcome() { 2346 if (this.outcome == null) 2347 if (Configuration.errorOnAutoCreate()) 2348 throw new Error("Attempt to auto-create Procedure.outcome"); 2349 else if (Configuration.doAutoCreate()) 2350 this.outcome = new CodeableConcept(); // cc 2351 return this.outcome; 2352 } 2353 2354 public boolean hasOutcome() { 2355 return this.outcome != null && !this.outcome.isEmpty(); 2356 } 2357 2358 /** 2359 * @param value {@link #outcome} (The outcome of the procedure - did it resolve 2360 * the reasons for the procedure being performed?) 2361 */ 2362 public Procedure setOutcome(CodeableConcept value) { 2363 this.outcome = value; 2364 return this; 2365 } 2366 2367 /** 2368 * @return {@link #report} (This could be a histology result, pathology report, 2369 * surgical report, etc.) 2370 */ 2371 public List<Reference> getReport() { 2372 if (this.report == null) 2373 this.report = new ArrayList<Reference>(); 2374 return this.report; 2375 } 2376 2377 /** 2378 * @return Returns a reference to <code>this</code> for easy method chaining 2379 */ 2380 public Procedure setReport(List<Reference> theReport) { 2381 this.report = theReport; 2382 return this; 2383 } 2384 2385 public boolean hasReport() { 2386 if (this.report == null) 2387 return false; 2388 for (Reference item : this.report) 2389 if (!item.isEmpty()) 2390 return true; 2391 return false; 2392 } 2393 2394 public Reference addReport() { // 3 2395 Reference t = new Reference(); 2396 if (this.report == null) 2397 this.report = new ArrayList<Reference>(); 2398 this.report.add(t); 2399 return t; 2400 } 2401 2402 public Procedure addReport(Reference t) { // 3 2403 if (t == null) 2404 return this; 2405 if (this.report == null) 2406 this.report = new ArrayList<Reference>(); 2407 this.report.add(t); 2408 return this; 2409 } 2410 2411 /** 2412 * @return The first repetition of repeating field {@link #report}, creating it 2413 * if it does not already exist 2414 */ 2415 public Reference getReportFirstRep() { 2416 if (getReport().isEmpty()) { 2417 addReport(); 2418 } 2419 return getReport().get(0); 2420 } 2421 2422 /** 2423 * @deprecated Use Reference#setResource(IBaseResource) instead 2424 */ 2425 @Deprecated 2426 public List<Resource> getReportTarget() { 2427 if (this.reportTarget == null) 2428 this.reportTarget = new ArrayList<Resource>(); 2429 return this.reportTarget; 2430 } 2431 2432 /** 2433 * @return {@link #complication} (Any complications that occurred during the 2434 * procedure, or in the immediate post-performance period. These are 2435 * generally tracked separately from the notes, which will typically 2436 * describe the procedure itself rather than any 'post procedure' 2437 * issues.) 2438 */ 2439 public List<CodeableConcept> getComplication() { 2440 if (this.complication == null) 2441 this.complication = new ArrayList<CodeableConcept>(); 2442 return this.complication; 2443 } 2444 2445 /** 2446 * @return Returns a reference to <code>this</code> for easy method chaining 2447 */ 2448 public Procedure setComplication(List<CodeableConcept> theComplication) { 2449 this.complication = theComplication; 2450 return this; 2451 } 2452 2453 public boolean hasComplication() { 2454 if (this.complication == null) 2455 return false; 2456 for (CodeableConcept item : this.complication) 2457 if (!item.isEmpty()) 2458 return true; 2459 return false; 2460 } 2461 2462 public CodeableConcept addComplication() { // 3 2463 CodeableConcept t = new CodeableConcept(); 2464 if (this.complication == null) 2465 this.complication = new ArrayList<CodeableConcept>(); 2466 this.complication.add(t); 2467 return t; 2468 } 2469 2470 public Procedure addComplication(CodeableConcept t) { // 3 2471 if (t == null) 2472 return this; 2473 if (this.complication == null) 2474 this.complication = new ArrayList<CodeableConcept>(); 2475 this.complication.add(t); 2476 return this; 2477 } 2478 2479 /** 2480 * @return The first repetition of repeating field {@link #complication}, 2481 * creating it if it does not already exist 2482 */ 2483 public CodeableConcept getComplicationFirstRep() { 2484 if (getComplication().isEmpty()) { 2485 addComplication(); 2486 } 2487 return getComplication().get(0); 2488 } 2489 2490 /** 2491 * @return {@link #complicationDetail} (Any complications that occurred during 2492 * the procedure, or in the immediate post-performance period.) 2493 */ 2494 public List<Reference> getComplicationDetail() { 2495 if (this.complicationDetail == null) 2496 this.complicationDetail = new ArrayList<Reference>(); 2497 return this.complicationDetail; 2498 } 2499 2500 /** 2501 * @return Returns a reference to <code>this</code> for easy method chaining 2502 */ 2503 public Procedure setComplicationDetail(List<Reference> theComplicationDetail) { 2504 this.complicationDetail = theComplicationDetail; 2505 return this; 2506 } 2507 2508 public boolean hasComplicationDetail() { 2509 if (this.complicationDetail == null) 2510 return false; 2511 for (Reference item : this.complicationDetail) 2512 if (!item.isEmpty()) 2513 return true; 2514 return false; 2515 } 2516 2517 public Reference addComplicationDetail() { // 3 2518 Reference t = new Reference(); 2519 if (this.complicationDetail == null) 2520 this.complicationDetail = new ArrayList<Reference>(); 2521 this.complicationDetail.add(t); 2522 return t; 2523 } 2524 2525 public Procedure addComplicationDetail(Reference t) { // 3 2526 if (t == null) 2527 return this; 2528 if (this.complicationDetail == null) 2529 this.complicationDetail = new ArrayList<Reference>(); 2530 this.complicationDetail.add(t); 2531 return this; 2532 } 2533 2534 /** 2535 * @return The first repetition of repeating field {@link #complicationDetail}, 2536 * creating it if it does not already exist 2537 */ 2538 public Reference getComplicationDetailFirstRep() { 2539 if (getComplicationDetail().isEmpty()) { 2540 addComplicationDetail(); 2541 } 2542 return getComplicationDetail().get(0); 2543 } 2544 2545 /** 2546 * @deprecated Use Reference#setResource(IBaseResource) instead 2547 */ 2548 @Deprecated 2549 public List<Condition> getComplicationDetailTarget() { 2550 if (this.complicationDetailTarget == null) 2551 this.complicationDetailTarget = new ArrayList<Condition>(); 2552 return this.complicationDetailTarget; 2553 } 2554 2555 /** 2556 * @deprecated Use Reference#setResource(IBaseResource) instead 2557 */ 2558 @Deprecated 2559 public Condition addComplicationDetailTarget() { 2560 Condition r = new Condition(); 2561 if (this.complicationDetailTarget == null) 2562 this.complicationDetailTarget = new ArrayList<Condition>(); 2563 this.complicationDetailTarget.add(r); 2564 return r; 2565 } 2566 2567 /** 2568 * @return {@link #followUp} (If the procedure required specific follow up - 2569 * e.g. removal of sutures. The follow up may be represented as a simple 2570 * note or could potentially be more complex, in which case the CarePlan 2571 * resource can be used.) 2572 */ 2573 public List<CodeableConcept> getFollowUp() { 2574 if (this.followUp == null) 2575 this.followUp = new ArrayList<CodeableConcept>(); 2576 return this.followUp; 2577 } 2578 2579 /** 2580 * @return Returns a reference to <code>this</code> for easy method chaining 2581 */ 2582 public Procedure setFollowUp(List<CodeableConcept> theFollowUp) { 2583 this.followUp = theFollowUp; 2584 return this; 2585 } 2586 2587 public boolean hasFollowUp() { 2588 if (this.followUp == null) 2589 return false; 2590 for (CodeableConcept item : this.followUp) 2591 if (!item.isEmpty()) 2592 return true; 2593 return false; 2594 } 2595 2596 public CodeableConcept addFollowUp() { // 3 2597 CodeableConcept t = new CodeableConcept(); 2598 if (this.followUp == null) 2599 this.followUp = new ArrayList<CodeableConcept>(); 2600 this.followUp.add(t); 2601 return t; 2602 } 2603 2604 public Procedure addFollowUp(CodeableConcept t) { // 3 2605 if (t == null) 2606 return this; 2607 if (this.followUp == null) 2608 this.followUp = new ArrayList<CodeableConcept>(); 2609 this.followUp.add(t); 2610 return this; 2611 } 2612 2613 /** 2614 * @return The first repetition of repeating field {@link #followUp}, creating 2615 * it if it does not already exist 2616 */ 2617 public CodeableConcept getFollowUpFirstRep() { 2618 if (getFollowUp().isEmpty()) { 2619 addFollowUp(); 2620 } 2621 return getFollowUp().get(0); 2622 } 2623 2624 /** 2625 * @return {@link #note} (Any other notes and comments about the procedure.) 2626 */ 2627 public List<Annotation> getNote() { 2628 if (this.note == null) 2629 this.note = new ArrayList<Annotation>(); 2630 return this.note; 2631 } 2632 2633 /** 2634 * @return Returns a reference to <code>this</code> for easy method chaining 2635 */ 2636 public Procedure setNote(List<Annotation> theNote) { 2637 this.note = theNote; 2638 return this; 2639 } 2640 2641 public boolean hasNote() { 2642 if (this.note == null) 2643 return false; 2644 for (Annotation item : this.note) 2645 if (!item.isEmpty()) 2646 return true; 2647 return false; 2648 } 2649 2650 public Annotation addNote() { // 3 2651 Annotation t = new Annotation(); 2652 if (this.note == null) 2653 this.note = new ArrayList<Annotation>(); 2654 this.note.add(t); 2655 return t; 2656 } 2657 2658 public Procedure addNote(Annotation t) { // 3 2659 if (t == null) 2660 return this; 2661 if (this.note == null) 2662 this.note = new ArrayList<Annotation>(); 2663 this.note.add(t); 2664 return this; 2665 } 2666 2667 /** 2668 * @return The first repetition of repeating field {@link #note}, creating it if 2669 * it does not already exist 2670 */ 2671 public Annotation getNoteFirstRep() { 2672 if (getNote().isEmpty()) { 2673 addNote(); 2674 } 2675 return getNote().get(0); 2676 } 2677 2678 /** 2679 * @return {@link #focalDevice} (A device that is implanted, removed or 2680 * otherwise manipulated (calibration, battery replacement, fitting a 2681 * prosthesis, attaching a wound-vac, etc.) as a focal portion of the 2682 * Procedure.) 2683 */ 2684 public List<ProcedureFocalDeviceComponent> getFocalDevice() { 2685 if (this.focalDevice == null) 2686 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2687 return this.focalDevice; 2688 } 2689 2690 /** 2691 * @return Returns a reference to <code>this</code> for easy method chaining 2692 */ 2693 public Procedure setFocalDevice(List<ProcedureFocalDeviceComponent> theFocalDevice) { 2694 this.focalDevice = theFocalDevice; 2695 return this; 2696 } 2697 2698 public boolean hasFocalDevice() { 2699 if (this.focalDevice == null) 2700 return false; 2701 for (ProcedureFocalDeviceComponent item : this.focalDevice) 2702 if (!item.isEmpty()) 2703 return true; 2704 return false; 2705 } 2706 2707 public ProcedureFocalDeviceComponent addFocalDevice() { // 3 2708 ProcedureFocalDeviceComponent t = new ProcedureFocalDeviceComponent(); 2709 if (this.focalDevice == null) 2710 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2711 this.focalDevice.add(t); 2712 return t; 2713 } 2714 2715 public Procedure addFocalDevice(ProcedureFocalDeviceComponent t) { // 3 2716 if (t == null) 2717 return this; 2718 if (this.focalDevice == null) 2719 this.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 2720 this.focalDevice.add(t); 2721 return this; 2722 } 2723 2724 /** 2725 * @return The first repetition of repeating field {@link #focalDevice}, 2726 * creating it if it does not already exist 2727 */ 2728 public ProcedureFocalDeviceComponent getFocalDeviceFirstRep() { 2729 if (getFocalDevice().isEmpty()) { 2730 addFocalDevice(); 2731 } 2732 return getFocalDevice().get(0); 2733 } 2734 2735 /** 2736 * @return {@link #usedReference} (Identifies medications, devices and any other 2737 * substance used as part of the procedure.) 2738 */ 2739 public List<Reference> getUsedReference() { 2740 if (this.usedReference == null) 2741 this.usedReference = new ArrayList<Reference>(); 2742 return this.usedReference; 2743 } 2744 2745 /** 2746 * @return Returns a reference to <code>this</code> for easy method chaining 2747 */ 2748 public Procedure setUsedReference(List<Reference> theUsedReference) { 2749 this.usedReference = theUsedReference; 2750 return this; 2751 } 2752 2753 public boolean hasUsedReference() { 2754 if (this.usedReference == null) 2755 return false; 2756 for (Reference item : this.usedReference) 2757 if (!item.isEmpty()) 2758 return true; 2759 return false; 2760 } 2761 2762 public Reference addUsedReference() { // 3 2763 Reference t = new Reference(); 2764 if (this.usedReference == null) 2765 this.usedReference = new ArrayList<Reference>(); 2766 this.usedReference.add(t); 2767 return t; 2768 } 2769 2770 public Procedure addUsedReference(Reference t) { // 3 2771 if (t == null) 2772 return this; 2773 if (this.usedReference == null) 2774 this.usedReference = new ArrayList<Reference>(); 2775 this.usedReference.add(t); 2776 return this; 2777 } 2778 2779 /** 2780 * @return The first repetition of repeating field {@link #usedReference}, 2781 * creating it if it does not already exist 2782 */ 2783 public Reference getUsedReferenceFirstRep() { 2784 if (getUsedReference().isEmpty()) { 2785 addUsedReference(); 2786 } 2787 return getUsedReference().get(0); 2788 } 2789 2790 /** 2791 * @deprecated Use Reference#setResource(IBaseResource) instead 2792 */ 2793 @Deprecated 2794 public List<Resource> getUsedReferenceTarget() { 2795 if (this.usedReferenceTarget == null) 2796 this.usedReferenceTarget = new ArrayList<Resource>(); 2797 return this.usedReferenceTarget; 2798 } 2799 2800 /** 2801 * @return {@link #usedCode} (Identifies coded items that were used as part of 2802 * the procedure.) 2803 */ 2804 public List<CodeableConcept> getUsedCode() { 2805 if (this.usedCode == null) 2806 this.usedCode = new ArrayList<CodeableConcept>(); 2807 return this.usedCode; 2808 } 2809 2810 /** 2811 * @return Returns a reference to <code>this</code> for easy method chaining 2812 */ 2813 public Procedure setUsedCode(List<CodeableConcept> theUsedCode) { 2814 this.usedCode = theUsedCode; 2815 return this; 2816 } 2817 2818 public boolean hasUsedCode() { 2819 if (this.usedCode == null) 2820 return false; 2821 for (CodeableConcept item : this.usedCode) 2822 if (!item.isEmpty()) 2823 return true; 2824 return false; 2825 } 2826 2827 public CodeableConcept addUsedCode() { // 3 2828 CodeableConcept t = new CodeableConcept(); 2829 if (this.usedCode == null) 2830 this.usedCode = new ArrayList<CodeableConcept>(); 2831 this.usedCode.add(t); 2832 return t; 2833 } 2834 2835 public Procedure addUsedCode(CodeableConcept t) { // 3 2836 if (t == null) 2837 return this; 2838 if (this.usedCode == null) 2839 this.usedCode = new ArrayList<CodeableConcept>(); 2840 this.usedCode.add(t); 2841 return this; 2842 } 2843 2844 /** 2845 * @return The first repetition of repeating field {@link #usedCode}, creating 2846 * it if it does not already exist 2847 */ 2848 public CodeableConcept getUsedCodeFirstRep() { 2849 if (getUsedCode().isEmpty()) { 2850 addUsedCode(); 2851 } 2852 return getUsedCode().get(0); 2853 } 2854 2855 protected void listChildren(List<Property> children) { 2856 super.listChildren(children); 2857 children.add(new Property("identifier", "Identifier", 2858 "Business identifiers assigned to this procedure by the performer or other systems which remain constant as the resource is updated and is propagated from server to server.", 2859 0, java.lang.Integer.MAX_VALUE, identifier)); 2860 children.add(new Property("instantiatesCanonical", 2861 "canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire)", 2862 "The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.", 2863 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2864 children.add(new Property("instantiatesUri", "uri", 2865 "The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.", 2866 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2867 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest)", 2868 "A reference to a resource that contains details of the request for this procedure.", 0, 2869 java.lang.Integer.MAX_VALUE, basedOn)); 2870 children.add(new Property("partOf", "Reference(Procedure|Observation|MedicationAdministration)", 2871 "A larger event of which this particular procedure is a component or step.", 0, java.lang.Integer.MAX_VALUE, 2872 partOf)); 2873 children.add(new Property("status", "code", 2874 "A code specifying the state of the procedure. Generally, this will be the in-progress or completed state.", 0, 2875 1, status)); 2876 children.add(new Property("statusReason", "CodeableConcept", 2877 "Captures the reason for the current state of the procedure.", 0, 1, statusReason)); 2878 children.add(new Property("category", "CodeableConcept", 2879 "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 2880 0, 1, category)); 2881 children.add(new Property("code", "CodeableConcept", 2882 "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").", 2883 0, 1, code)); 2884 children.add(new Property("subject", "Reference(Patient|Group)", 2885 "The person, animal or group on which the procedure was performed.", 0, 1, subject)); 2886 children.add(new Property("encounter", "Reference(Encounter)", 2887 "The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated.", 2888 0, 1, encounter)); 2889 children.add(new Property("performed[x]", "dateTime|Period|string|Age|Range", 2890 "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 2891 0, 1, performed)); 2892 children.add(new Property("recorder", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", 2893 "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder)); 2894 children.add(new Property("asserter", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", 2895 "Individual who is making the procedure statement.", 0, 1, asserter)); 2896 children.add(new Property("performer", "", "Limited to \"real\" people rather than equipment.", 0, 2897 java.lang.Integer.MAX_VALUE, performer)); 2898 children.add(new Property("location", "Reference(Location)", 2899 "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.", 2900 0, 1, location)); 2901 children.add(new Property("reasonCode", "CodeableConcept", 2902 "The coded reason why the procedure was performed. This may be a coded entity of some type, or may simply be present as text.", 2903 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2904 children.add( 2905 new Property("reasonReference", "Reference(Condition|Observation|Procedure|DiagnosticReport|DocumentReference)", 2906 "The justification of why the procedure was performed.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2907 children.add(new Property("bodySite", "CodeableConcept", 2908 "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.", 2909 0, java.lang.Integer.MAX_VALUE, bodySite)); 2910 children.add(new Property("outcome", "CodeableConcept", 2911 "The outcome of the procedure - did it resolve the reasons for the procedure being performed?", 0, 1, outcome)); 2912 children.add(new Property("report", "Reference(DiagnosticReport|DocumentReference|Composition)", 2913 "This could be a histology result, pathology report, surgical report, etc.", 0, java.lang.Integer.MAX_VALUE, 2914 report)); 2915 children.add(new Property("complication", "CodeableConcept", 2916 "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.", 2917 0, java.lang.Integer.MAX_VALUE, complication)); 2918 children.add(new Property("complicationDetail", "Reference(Condition)", 2919 "Any complications that occurred during the procedure, or in the immediate post-performance period.", 0, 2920 java.lang.Integer.MAX_VALUE, complicationDetail)); 2921 children.add(new Property("followUp", "CodeableConcept", 2922 "If the procedure required specific follow up - e.g. removal of sutures. The follow up may be represented as a simple note or could potentially be more complex, in which case the CarePlan resource can be used.", 2923 0, java.lang.Integer.MAX_VALUE, followUp)); 2924 children.add(new Property("note", "Annotation", "Any other notes and comments about the procedure.", 0, 2925 java.lang.Integer.MAX_VALUE, note)); 2926 children.add(new Property("focalDevice", "", 2927 "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.", 2928 0, java.lang.Integer.MAX_VALUE, focalDevice)); 2929 children.add(new Property("usedReference", "Reference(Device|Medication|Substance)", 2930 "Identifies medications, devices and any other substance used as part of the procedure.", 0, 2931 java.lang.Integer.MAX_VALUE, usedReference)); 2932 children.add(new Property("usedCode", "CodeableConcept", 2933 "Identifies coded items that were used as part of the procedure.", 0, java.lang.Integer.MAX_VALUE, usedCode)); 2934 } 2935 2936 @Override 2937 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2938 switch (_hash) { 2939 case -1618432855: 2940 /* identifier */ return new Property("identifier", "Identifier", 2941 "Business identifiers assigned to this procedure by the performer or other systems which remain constant as the resource is updated and is propagated from server to server.", 2942 0, java.lang.Integer.MAX_VALUE, identifier); 2943 case 8911915: 2944 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 2945 "canonical(PlanDefinition|ActivityDefinition|Measure|OperationDefinition|Questionnaire)", 2946 "The URL pointing to a FHIR-defined protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.", 2947 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2948 case -1926393373: 2949 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 2950 "The URL pointing to an externally maintained protocol, guideline, order set or other definition that is adhered to in whole or in part by this Procedure.", 2951 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2952 case -332612366: 2953 /* basedOn */ return new Property("basedOn", "Reference(CarePlan|ServiceRequest)", 2954 "A reference to a resource that contains details of the request for this procedure.", 0, 2955 java.lang.Integer.MAX_VALUE, basedOn); 2956 case -995410646: 2957 /* partOf */ return new Property("partOf", "Reference(Procedure|Observation|MedicationAdministration)", 2958 "A larger event of which this particular procedure is a component or step.", 0, java.lang.Integer.MAX_VALUE, 2959 partOf); 2960 case -892481550: 2961 /* status */ return new Property("status", "code", 2962 "A code specifying the state of the procedure. Generally, this will be the in-progress or completed state.", 2963 0, 1, status); 2964 case 2051346646: 2965 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2966 "Captures the reason for the current state of the procedure.", 0, 1, statusReason); 2967 case 50511102: 2968 /* category */ return new Property("category", "CodeableConcept", 2969 "A code that classifies the procedure for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 2970 0, 1, category); 2971 case 3059181: 2972 /* code */ return new Property("code", "CodeableConcept", 2973 "The specific procedure that is performed. Use text if the exact nature of the procedure cannot be coded (e.g. \"Laparoscopic Appendectomy\").", 2974 0, 1, code); 2975 case -1867885268: 2976 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2977 "The person, animal or group on which the procedure was performed.", 0, 1, subject); 2978 case 1524132147: 2979 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2980 "The Encounter during which this Procedure was created or performed or to which the creation of this record is tightly associated.", 2981 0, 1, encounter); 2982 case 1355984064: 2983 /* performed[x] */ return new Property("performed[x]", "dateTime|Period|string|Age|Range", 2984 "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 2985 0, 1, performed); 2986 case 481140672: 2987 /* performed */ return new Property("performed[x]", "dateTime|Period|string|Age|Range", 2988 "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 2989 0, 1, performed); 2990 case 1118270331: 2991 /* performedDateTime */ return new Property("performed[x]", "dateTime|Period|string|Age|Range", 2992 "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 2993 0, 1, performed); 2994 case 1622094241: 2995 /* performedPeriod */ return new Property("performed[x]", "dateTime|Period|string|Age|Range", 2996 "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 2997 0, 1, performed); 2998 case 1721834481: 2999 /* performedString */ return new Property("performed[x]", "dateTime|Period|string|Age|Range", 3000 "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 3001 0, 1, performed); 3002 case 1355958559: 3003 /* performedAge */ return new Property("performed[x]", "dateTime|Period|string|Age|Range", 3004 "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 3005 0, 1, performed); 3006 case 1716617565: 3007 /* performedRange */ return new Property("performed[x]", "dateTime|Period|string|Age|Range", 3008 "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 3009 0, 1, performed); 3010 case -799233858: 3011 /* recorder */ return new Property("recorder", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", 3012 "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder); 3013 case -373242253: 3014 /* asserter */ return new Property("asserter", "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole)", 3015 "Individual who is making the procedure statement.", 0, 1, asserter); 3016 case 481140686: 3017 /* performer */ return new Property("performer", "", "Limited to \"real\" people rather than equipment.", 0, 3018 java.lang.Integer.MAX_VALUE, performer); 3019 case 1901043637: 3020 /* location */ return new Property("location", "Reference(Location)", 3021 "The location where the procedure actually happened. E.g. a newborn at home, a tracheostomy at a restaurant.", 3022 0, 1, location); 3023 case 722137681: 3024 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 3025 "The coded reason why the procedure was performed. This may be a coded entity of some type, or may simply be present as text.", 3026 0, java.lang.Integer.MAX_VALUE, reasonCode); 3027 case -1146218137: 3028 /* reasonReference */ return new Property("reasonReference", 3029 "Reference(Condition|Observation|Procedure|DiagnosticReport|DocumentReference)", 3030 "The justification of why the procedure was performed.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 3031 case 1702620169: 3032 /* bodySite */ return new Property("bodySite", "CodeableConcept", 3033 "Detailed and structured anatomical location information. Multiple locations are allowed - e.g. multiple punch biopsies of a lesion.", 3034 0, java.lang.Integer.MAX_VALUE, bodySite); 3035 case -1106507950: 3036 /* outcome */ return new Property("outcome", "CodeableConcept", 3037 "The outcome of the procedure - did it resolve the reasons for the procedure being performed?", 0, 1, 3038 outcome); 3039 case -934521548: 3040 /* report */ return new Property("report", "Reference(DiagnosticReport|DocumentReference|Composition)", 3041 "This could be a histology result, pathology report, surgical report, etc.", 0, java.lang.Integer.MAX_VALUE, 3042 report); 3043 case -1644401602: 3044 /* complication */ return new Property("complication", "CodeableConcept", 3045 "Any complications that occurred during the procedure, or in the immediate post-performance period. These are generally tracked separately from the notes, which will typically describe the procedure itself rather than any 'post procedure' issues.", 3046 0, java.lang.Integer.MAX_VALUE, complication); 3047 case -1685272017: 3048 /* complicationDetail */ return new Property("complicationDetail", "Reference(Condition)", 3049 "Any complications that occurred during the procedure, or in the immediate post-performance period.", 0, 3050 java.lang.Integer.MAX_VALUE, complicationDetail); 3051 case 301801004: 3052 /* followUp */ return new Property("followUp", "CodeableConcept", 3053 "If the procedure required specific follow up - e.g. removal of sutures. The follow up may be represented as a simple note or could potentially be more complex, in which case the CarePlan resource can be used.", 3054 0, java.lang.Integer.MAX_VALUE, followUp); 3055 case 3387378: 3056 /* note */ return new Property("note", "Annotation", "Any other notes and comments about the procedure.", 0, 3057 java.lang.Integer.MAX_VALUE, note); 3058 case -1129235173: 3059 /* focalDevice */ return new Property("focalDevice", "", 3060 "A device that is implanted, removed or otherwise manipulated (calibration, battery replacement, fitting a prosthesis, attaching a wound-vac, etc.) as a focal portion of the Procedure.", 3061 0, java.lang.Integer.MAX_VALUE, focalDevice); 3062 case -504932338: 3063 /* usedReference */ return new Property("usedReference", "Reference(Device|Medication|Substance)", 3064 "Identifies medications, devices and any other substance used as part of the procedure.", 0, 3065 java.lang.Integer.MAX_VALUE, usedReference); 3066 case -279910582: 3067 /* usedCode */ return new Property("usedCode", "CodeableConcept", 3068 "Identifies coded items that were used as part of the procedure.", 0, java.lang.Integer.MAX_VALUE, usedCode); 3069 default: 3070 return super.getNamedProperty(_hash, _name, _checkValid); 3071 } 3072 3073 } 3074 3075 @Override 3076 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3077 switch (hash) { 3078 case -1618432855: 3079 /* identifier */ return this.identifier == null ? new Base[0] 3080 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3081 case 8911915: 3082 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 3083 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 3084 case -1926393373: 3085 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 3086 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 3087 case -332612366: 3088 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3089 case -995410646: 3090 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 3091 case -892481550: 3092 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ProcedureStatus> 3093 case 2051346646: 3094 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 3095 case 50511102: 3096 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 3097 case 3059181: 3098 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 3099 case -1867885268: 3100 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 3101 case 1524132147: 3102 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 3103 case 481140672: 3104 /* performed */ return this.performed == null ? new Base[0] : new Base[] { this.performed }; // Type 3105 case -799233858: 3106 /* recorder */ return this.recorder == null ? new Base[0] : new Base[] { this.recorder }; // Reference 3107 case -373242253: 3108 /* asserter */ return this.asserter == null ? new Base[0] : new Base[] { this.asserter }; // Reference 3109 case 481140686: 3110 /* performer */ return this.performer == null ? new Base[0] 3111 : this.performer.toArray(new Base[this.performer.size()]); // ProcedurePerformerComponent 3112 case 1901043637: 3113 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 3114 case 722137681: 3115 /* reasonCode */ return this.reasonCode == null ? new Base[0] 3116 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 3117 case -1146218137: 3118 /* reasonReference */ return this.reasonReference == null ? new Base[0] 3119 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 3120 case 1702620169: 3121 /* bodySite */ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 3122 case -1106507950: 3123 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // CodeableConcept 3124 case -934521548: 3125 /* report */ return this.report == null ? new Base[0] : this.report.toArray(new Base[this.report.size()]); // Reference 3126 case -1644401602: 3127 /* complication */ return this.complication == null ? new Base[0] 3128 : this.complication.toArray(new Base[this.complication.size()]); // CodeableConcept 3129 case -1685272017: 3130 /* complicationDetail */ return this.complicationDetail == null ? new Base[0] 3131 : this.complicationDetail.toArray(new Base[this.complicationDetail.size()]); // Reference 3132 case 301801004: 3133 /* followUp */ return this.followUp == null ? new Base[0] : this.followUp.toArray(new Base[this.followUp.size()]); // CodeableConcept 3134 case 3387378: 3135 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3136 case -1129235173: 3137 /* focalDevice */ return this.focalDevice == null ? new Base[0] 3138 : this.focalDevice.toArray(new Base[this.focalDevice.size()]); // ProcedureFocalDeviceComponent 3139 case -504932338: 3140 /* usedReference */ return this.usedReference == null ? new Base[0] 3141 : this.usedReference.toArray(new Base[this.usedReference.size()]); // Reference 3142 case -279910582: 3143 /* usedCode */ return this.usedCode == null ? new Base[0] : this.usedCode.toArray(new Base[this.usedCode.size()]); // CodeableConcept 3144 default: 3145 return super.getProperty(hash, name, checkValid); 3146 } 3147 3148 } 3149 3150 @Override 3151 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3152 switch (hash) { 3153 case -1618432855: // identifier 3154 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3155 return value; 3156 case 8911915: // instantiatesCanonical 3157 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 3158 return value; 3159 case -1926393373: // instantiatesUri 3160 this.getInstantiatesUri().add(castToUri(value)); // UriType 3161 return value; 3162 case -332612366: // basedOn 3163 this.getBasedOn().add(castToReference(value)); // Reference 3164 return value; 3165 case -995410646: // partOf 3166 this.getPartOf().add(castToReference(value)); // Reference 3167 return value; 3168 case -892481550: // status 3169 value = new ProcedureStatusEnumFactory().fromType(castToCode(value)); 3170 this.status = (Enumeration) value; // Enumeration<ProcedureStatus> 3171 return value; 3172 case 2051346646: // statusReason 3173 this.statusReason = castToCodeableConcept(value); // CodeableConcept 3174 return value; 3175 case 50511102: // category 3176 this.category = castToCodeableConcept(value); // CodeableConcept 3177 return value; 3178 case 3059181: // code 3179 this.code = castToCodeableConcept(value); // CodeableConcept 3180 return value; 3181 case -1867885268: // subject 3182 this.subject = castToReference(value); // Reference 3183 return value; 3184 case 1524132147: // encounter 3185 this.encounter = castToReference(value); // Reference 3186 return value; 3187 case 481140672: // performed 3188 this.performed = castToType(value); // Type 3189 return value; 3190 case -799233858: // recorder 3191 this.recorder = castToReference(value); // Reference 3192 return value; 3193 case -373242253: // asserter 3194 this.asserter = castToReference(value); // Reference 3195 return value; 3196 case 481140686: // performer 3197 this.getPerformer().add((ProcedurePerformerComponent) value); // ProcedurePerformerComponent 3198 return value; 3199 case 1901043637: // location 3200 this.location = castToReference(value); // Reference 3201 return value; 3202 case 722137681: // reasonCode 3203 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 3204 return value; 3205 case -1146218137: // reasonReference 3206 this.getReasonReference().add(castToReference(value)); // Reference 3207 return value; 3208 case 1702620169: // bodySite 3209 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 3210 return value; 3211 case -1106507950: // outcome 3212 this.outcome = castToCodeableConcept(value); // CodeableConcept 3213 return value; 3214 case -934521548: // report 3215 this.getReport().add(castToReference(value)); // Reference 3216 return value; 3217 case -1644401602: // complication 3218 this.getComplication().add(castToCodeableConcept(value)); // CodeableConcept 3219 return value; 3220 case -1685272017: // complicationDetail 3221 this.getComplicationDetail().add(castToReference(value)); // Reference 3222 return value; 3223 case 301801004: // followUp 3224 this.getFollowUp().add(castToCodeableConcept(value)); // CodeableConcept 3225 return value; 3226 case 3387378: // note 3227 this.getNote().add(castToAnnotation(value)); // Annotation 3228 return value; 3229 case -1129235173: // focalDevice 3230 this.getFocalDevice().add((ProcedureFocalDeviceComponent) value); // ProcedureFocalDeviceComponent 3231 return value; 3232 case -504932338: // usedReference 3233 this.getUsedReference().add(castToReference(value)); // Reference 3234 return value; 3235 case -279910582: // usedCode 3236 this.getUsedCode().add(castToCodeableConcept(value)); // CodeableConcept 3237 return value; 3238 default: 3239 return super.setProperty(hash, name, value); 3240 } 3241 3242 } 3243 3244 @Override 3245 public Base setProperty(String name, Base value) throws FHIRException { 3246 if (name.equals("identifier")) { 3247 this.getIdentifier().add(castToIdentifier(value)); 3248 } else if (name.equals("instantiatesCanonical")) { 3249 this.getInstantiatesCanonical().add(castToCanonical(value)); 3250 } else if (name.equals("instantiatesUri")) { 3251 this.getInstantiatesUri().add(castToUri(value)); 3252 } else if (name.equals("basedOn")) { 3253 this.getBasedOn().add(castToReference(value)); 3254 } else if (name.equals("partOf")) { 3255 this.getPartOf().add(castToReference(value)); 3256 } else if (name.equals("status")) { 3257 value = new ProcedureStatusEnumFactory().fromType(castToCode(value)); 3258 this.status = (Enumeration) value; // Enumeration<ProcedureStatus> 3259 } else if (name.equals("statusReason")) { 3260 this.statusReason = castToCodeableConcept(value); // CodeableConcept 3261 } else if (name.equals("category")) { 3262 this.category = castToCodeableConcept(value); // CodeableConcept 3263 } else if (name.equals("code")) { 3264 this.code = castToCodeableConcept(value); // CodeableConcept 3265 } else if (name.equals("subject")) { 3266 this.subject = castToReference(value); // Reference 3267 } else if (name.equals("encounter")) { 3268 this.encounter = castToReference(value); // Reference 3269 } else if (name.equals("performed[x]")) { 3270 this.performed = castToType(value); // Type 3271 } else if (name.equals("recorder")) { 3272 this.recorder = castToReference(value); // Reference 3273 } else if (name.equals("asserter")) { 3274 this.asserter = castToReference(value); // Reference 3275 } else if (name.equals("performer")) { 3276 this.getPerformer().add((ProcedurePerformerComponent) value); 3277 } else if (name.equals("location")) { 3278 this.location = castToReference(value); // Reference 3279 } else if (name.equals("reasonCode")) { 3280 this.getReasonCode().add(castToCodeableConcept(value)); 3281 } else if (name.equals("reasonReference")) { 3282 this.getReasonReference().add(castToReference(value)); 3283 } else if (name.equals("bodySite")) { 3284 this.getBodySite().add(castToCodeableConcept(value)); 3285 } else if (name.equals("outcome")) { 3286 this.outcome = castToCodeableConcept(value); // CodeableConcept 3287 } else if (name.equals("report")) { 3288 this.getReport().add(castToReference(value)); 3289 } else if (name.equals("complication")) { 3290 this.getComplication().add(castToCodeableConcept(value)); 3291 } else if (name.equals("complicationDetail")) { 3292 this.getComplicationDetail().add(castToReference(value)); 3293 } else if (name.equals("followUp")) { 3294 this.getFollowUp().add(castToCodeableConcept(value)); 3295 } else if (name.equals("note")) { 3296 this.getNote().add(castToAnnotation(value)); 3297 } else if (name.equals("focalDevice")) { 3298 this.getFocalDevice().add((ProcedureFocalDeviceComponent) value); 3299 } else if (name.equals("usedReference")) { 3300 this.getUsedReference().add(castToReference(value)); 3301 } else if (name.equals("usedCode")) { 3302 this.getUsedCode().add(castToCodeableConcept(value)); 3303 } else 3304 return super.setProperty(name, value); 3305 return value; 3306 } 3307 3308 @Override 3309 public void removeChild(String name, Base value) throws FHIRException { 3310 if (name.equals("identifier")) { 3311 this.getIdentifier().remove(castToIdentifier(value)); 3312 } else if (name.equals("instantiatesCanonical")) { 3313 this.getInstantiatesCanonical().remove(castToCanonical(value)); 3314 } else if (name.equals("instantiatesUri")) { 3315 this.getInstantiatesUri().remove(castToUri(value)); 3316 } else if (name.equals("basedOn")) { 3317 this.getBasedOn().remove(castToReference(value)); 3318 } else if (name.equals("partOf")) { 3319 this.getPartOf().remove(castToReference(value)); 3320 } else if (name.equals("status")) { 3321 this.status = null; 3322 } else if (name.equals("statusReason")) { 3323 this.statusReason = null; 3324 } else if (name.equals("category")) { 3325 this.category = null; 3326 } else if (name.equals("code")) { 3327 this.code = null; 3328 } else if (name.equals("subject")) { 3329 this.subject = null; 3330 } else if (name.equals("encounter")) { 3331 this.encounter = null; 3332 } else if (name.equals("performed[x]")) { 3333 this.performed = null; 3334 } else if (name.equals("recorder")) { 3335 this.recorder = null; 3336 } else if (name.equals("asserter")) { 3337 this.asserter = null; 3338 } else if (name.equals("performer")) { 3339 this.getPerformer().remove((ProcedurePerformerComponent) value); 3340 } else if (name.equals("location")) { 3341 this.location = null; 3342 } else if (name.equals("reasonCode")) { 3343 this.getReasonCode().remove(castToCodeableConcept(value)); 3344 } else if (name.equals("reasonReference")) { 3345 this.getReasonReference().remove(castToReference(value)); 3346 } else if (name.equals("bodySite")) { 3347 this.getBodySite().remove(castToCodeableConcept(value)); 3348 } else if (name.equals("outcome")) { 3349 this.outcome = null; 3350 } else if (name.equals("report")) { 3351 this.getReport().remove(castToReference(value)); 3352 } else if (name.equals("complication")) { 3353 this.getComplication().remove(castToCodeableConcept(value)); 3354 } else if (name.equals("complicationDetail")) { 3355 this.getComplicationDetail().remove(castToReference(value)); 3356 } else if (name.equals("followUp")) { 3357 this.getFollowUp().remove(castToCodeableConcept(value)); 3358 } else if (name.equals("note")) { 3359 this.getNote().remove(castToAnnotation(value)); 3360 } else if (name.equals("focalDevice")) { 3361 this.getFocalDevice().remove((ProcedureFocalDeviceComponent) value); 3362 } else if (name.equals("usedReference")) { 3363 this.getUsedReference().remove(castToReference(value)); 3364 } else if (name.equals("usedCode")) { 3365 this.getUsedCode().remove(castToCodeableConcept(value)); 3366 } else 3367 super.removeChild(name, value); 3368 3369 } 3370 3371 @Override 3372 public Base makeProperty(int hash, String name) throws FHIRException { 3373 switch (hash) { 3374 case -1618432855: 3375 return addIdentifier(); 3376 case 8911915: 3377 return addInstantiatesCanonicalElement(); 3378 case -1926393373: 3379 return addInstantiatesUriElement(); 3380 case -332612366: 3381 return addBasedOn(); 3382 case -995410646: 3383 return addPartOf(); 3384 case -892481550: 3385 return getStatusElement(); 3386 case 2051346646: 3387 return getStatusReason(); 3388 case 50511102: 3389 return getCategory(); 3390 case 3059181: 3391 return getCode(); 3392 case -1867885268: 3393 return getSubject(); 3394 case 1524132147: 3395 return getEncounter(); 3396 case 1355984064: 3397 return getPerformed(); 3398 case 481140672: 3399 return getPerformed(); 3400 case -799233858: 3401 return getRecorder(); 3402 case -373242253: 3403 return getAsserter(); 3404 case 481140686: 3405 return addPerformer(); 3406 case 1901043637: 3407 return getLocation(); 3408 case 722137681: 3409 return addReasonCode(); 3410 case -1146218137: 3411 return addReasonReference(); 3412 case 1702620169: 3413 return addBodySite(); 3414 case -1106507950: 3415 return getOutcome(); 3416 case -934521548: 3417 return addReport(); 3418 case -1644401602: 3419 return addComplication(); 3420 case -1685272017: 3421 return addComplicationDetail(); 3422 case 301801004: 3423 return addFollowUp(); 3424 case 3387378: 3425 return addNote(); 3426 case -1129235173: 3427 return addFocalDevice(); 3428 case -504932338: 3429 return addUsedReference(); 3430 case -279910582: 3431 return addUsedCode(); 3432 default: 3433 return super.makeProperty(hash, name); 3434 } 3435 3436 } 3437 3438 @Override 3439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3440 switch (hash) { 3441 case -1618432855: 3442 /* identifier */ return new String[] { "Identifier" }; 3443 case 8911915: 3444 /* instantiatesCanonical */ return new String[] { "canonical" }; 3445 case -1926393373: 3446 /* instantiatesUri */ return new String[] { "uri" }; 3447 case -332612366: 3448 /* basedOn */ return new String[] { "Reference" }; 3449 case -995410646: 3450 /* partOf */ return new String[] { "Reference" }; 3451 case -892481550: 3452 /* status */ return new String[] { "code" }; 3453 case 2051346646: 3454 /* statusReason */ return new String[] { "CodeableConcept" }; 3455 case 50511102: 3456 /* category */ return new String[] { "CodeableConcept" }; 3457 case 3059181: 3458 /* code */ return new String[] { "CodeableConcept" }; 3459 case -1867885268: 3460 /* subject */ return new String[] { "Reference" }; 3461 case 1524132147: 3462 /* encounter */ return new String[] { "Reference" }; 3463 case 481140672: 3464 /* performed */ return new String[] { "dateTime", "Period", "string", "Age", "Range" }; 3465 case -799233858: 3466 /* recorder */ return new String[] { "Reference" }; 3467 case -373242253: 3468 /* asserter */ return new String[] { "Reference" }; 3469 case 481140686: 3470 /* performer */ return new String[] {}; 3471 case 1901043637: 3472 /* location */ return new String[] { "Reference" }; 3473 case 722137681: 3474 /* reasonCode */ return new String[] { "CodeableConcept" }; 3475 case -1146218137: 3476 /* reasonReference */ return new String[] { "Reference" }; 3477 case 1702620169: 3478 /* bodySite */ return new String[] { "CodeableConcept" }; 3479 case -1106507950: 3480 /* outcome */ return new String[] { "CodeableConcept" }; 3481 case -934521548: 3482 /* report */ return new String[] { "Reference" }; 3483 case -1644401602: 3484 /* complication */ return new String[] { "CodeableConcept" }; 3485 case -1685272017: 3486 /* complicationDetail */ return new String[] { "Reference" }; 3487 case 301801004: 3488 /* followUp */ return new String[] { "CodeableConcept" }; 3489 case 3387378: 3490 /* note */ return new String[] { "Annotation" }; 3491 case -1129235173: 3492 /* focalDevice */ return new String[] {}; 3493 case -504932338: 3494 /* usedReference */ return new String[] { "Reference" }; 3495 case -279910582: 3496 /* usedCode */ return new String[] { "CodeableConcept" }; 3497 default: 3498 return super.getTypesForProperty(hash, name); 3499 } 3500 3501 } 3502 3503 @Override 3504 public Base addChild(String name) throws FHIRException { 3505 if (name.equals("identifier")) { 3506 return addIdentifier(); 3507 } else if (name.equals("instantiatesCanonical")) { 3508 throw new FHIRException("Cannot call addChild on a singleton property Procedure.instantiatesCanonical"); 3509 } else if (name.equals("instantiatesUri")) { 3510 throw new FHIRException("Cannot call addChild on a singleton property Procedure.instantiatesUri"); 3511 } else if (name.equals("basedOn")) { 3512 return addBasedOn(); 3513 } else if (name.equals("partOf")) { 3514 return addPartOf(); 3515 } else if (name.equals("status")) { 3516 throw new FHIRException("Cannot call addChild on a singleton property Procedure.status"); 3517 } else if (name.equals("statusReason")) { 3518 this.statusReason = new CodeableConcept(); 3519 return this.statusReason; 3520 } else if (name.equals("category")) { 3521 this.category = new CodeableConcept(); 3522 return this.category; 3523 } else if (name.equals("code")) { 3524 this.code = new CodeableConcept(); 3525 return this.code; 3526 } else if (name.equals("subject")) { 3527 this.subject = new Reference(); 3528 return this.subject; 3529 } else if (name.equals("encounter")) { 3530 this.encounter = new Reference(); 3531 return this.encounter; 3532 } else if (name.equals("performedDateTime")) { 3533 this.performed = new DateTimeType(); 3534 return this.performed; 3535 } else if (name.equals("performedPeriod")) { 3536 this.performed = new Period(); 3537 return this.performed; 3538 } else if (name.equals("performedString")) { 3539 this.performed = new StringType(); 3540 return this.performed; 3541 } else if (name.equals("performedAge")) { 3542 this.performed = new Age(); 3543 return this.performed; 3544 } else if (name.equals("performedRange")) { 3545 this.performed = new Range(); 3546 return this.performed; 3547 } else if (name.equals("recorder")) { 3548 this.recorder = new Reference(); 3549 return this.recorder; 3550 } else if (name.equals("asserter")) { 3551 this.asserter = new Reference(); 3552 return this.asserter; 3553 } else if (name.equals("performer")) { 3554 return addPerformer(); 3555 } else if (name.equals("location")) { 3556 this.location = new Reference(); 3557 return this.location; 3558 } else if (name.equals("reasonCode")) { 3559 return addReasonCode(); 3560 } else if (name.equals("reasonReference")) { 3561 return addReasonReference(); 3562 } else if (name.equals("bodySite")) { 3563 return addBodySite(); 3564 } else if (name.equals("outcome")) { 3565 this.outcome = new CodeableConcept(); 3566 return this.outcome; 3567 } else if (name.equals("report")) { 3568 return addReport(); 3569 } else if (name.equals("complication")) { 3570 return addComplication(); 3571 } else if (name.equals("complicationDetail")) { 3572 return addComplicationDetail(); 3573 } else if (name.equals("followUp")) { 3574 return addFollowUp(); 3575 } else if (name.equals("note")) { 3576 return addNote(); 3577 } else if (name.equals("focalDevice")) { 3578 return addFocalDevice(); 3579 } else if (name.equals("usedReference")) { 3580 return addUsedReference(); 3581 } else if (name.equals("usedCode")) { 3582 return addUsedCode(); 3583 } else 3584 return super.addChild(name); 3585 } 3586 3587 public String fhirType() { 3588 return "Procedure"; 3589 3590 } 3591 3592 public Procedure copy() { 3593 Procedure dst = new Procedure(); 3594 copyValues(dst); 3595 return dst; 3596 } 3597 3598 public void copyValues(Procedure dst) { 3599 super.copyValues(dst); 3600 if (identifier != null) { 3601 dst.identifier = new ArrayList<Identifier>(); 3602 for (Identifier i : identifier) 3603 dst.identifier.add(i.copy()); 3604 } 3605 ; 3606 if (instantiatesCanonical != null) { 3607 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 3608 for (CanonicalType i : instantiatesCanonical) 3609 dst.instantiatesCanonical.add(i.copy()); 3610 } 3611 ; 3612 if (instantiatesUri != null) { 3613 dst.instantiatesUri = new ArrayList<UriType>(); 3614 for (UriType i : instantiatesUri) 3615 dst.instantiatesUri.add(i.copy()); 3616 } 3617 ; 3618 if (basedOn != null) { 3619 dst.basedOn = new ArrayList<Reference>(); 3620 for (Reference i : basedOn) 3621 dst.basedOn.add(i.copy()); 3622 } 3623 ; 3624 if (partOf != null) { 3625 dst.partOf = new ArrayList<Reference>(); 3626 for (Reference i : partOf) 3627 dst.partOf.add(i.copy()); 3628 } 3629 ; 3630 dst.status = status == null ? null : status.copy(); 3631 dst.statusReason = statusReason == null ? null : statusReason.copy(); 3632 dst.category = category == null ? null : category.copy(); 3633 dst.code = code == null ? null : code.copy(); 3634 dst.subject = subject == null ? null : subject.copy(); 3635 dst.encounter = encounter == null ? null : encounter.copy(); 3636 dst.performed = performed == null ? null : performed.copy(); 3637 dst.recorder = recorder == null ? null : recorder.copy(); 3638 dst.asserter = asserter == null ? null : asserter.copy(); 3639 if (performer != null) { 3640 dst.performer = new ArrayList<ProcedurePerformerComponent>(); 3641 for (ProcedurePerformerComponent i : performer) 3642 dst.performer.add(i.copy()); 3643 } 3644 ; 3645 dst.location = location == null ? null : location.copy(); 3646 if (reasonCode != null) { 3647 dst.reasonCode = new ArrayList<CodeableConcept>(); 3648 for (CodeableConcept i : reasonCode) 3649 dst.reasonCode.add(i.copy()); 3650 } 3651 ; 3652 if (reasonReference != null) { 3653 dst.reasonReference = new ArrayList<Reference>(); 3654 for (Reference i : reasonReference) 3655 dst.reasonReference.add(i.copy()); 3656 } 3657 ; 3658 if (bodySite != null) { 3659 dst.bodySite = new ArrayList<CodeableConcept>(); 3660 for (CodeableConcept i : bodySite) 3661 dst.bodySite.add(i.copy()); 3662 } 3663 ; 3664 dst.outcome = outcome == null ? null : outcome.copy(); 3665 if (report != null) { 3666 dst.report = new ArrayList<Reference>(); 3667 for (Reference i : report) 3668 dst.report.add(i.copy()); 3669 } 3670 ; 3671 if (complication != null) { 3672 dst.complication = new ArrayList<CodeableConcept>(); 3673 for (CodeableConcept i : complication) 3674 dst.complication.add(i.copy()); 3675 } 3676 ; 3677 if (complicationDetail != null) { 3678 dst.complicationDetail = new ArrayList<Reference>(); 3679 for (Reference i : complicationDetail) 3680 dst.complicationDetail.add(i.copy()); 3681 } 3682 ; 3683 if (followUp != null) { 3684 dst.followUp = new ArrayList<CodeableConcept>(); 3685 for (CodeableConcept i : followUp) 3686 dst.followUp.add(i.copy()); 3687 } 3688 ; 3689 if (note != null) { 3690 dst.note = new ArrayList<Annotation>(); 3691 for (Annotation i : note) 3692 dst.note.add(i.copy()); 3693 } 3694 ; 3695 if (focalDevice != null) { 3696 dst.focalDevice = new ArrayList<ProcedureFocalDeviceComponent>(); 3697 for (ProcedureFocalDeviceComponent i : focalDevice) 3698 dst.focalDevice.add(i.copy()); 3699 } 3700 ; 3701 if (usedReference != null) { 3702 dst.usedReference = new ArrayList<Reference>(); 3703 for (Reference i : usedReference) 3704 dst.usedReference.add(i.copy()); 3705 } 3706 ; 3707 if (usedCode != null) { 3708 dst.usedCode = new ArrayList<CodeableConcept>(); 3709 for (CodeableConcept i : usedCode) 3710 dst.usedCode.add(i.copy()); 3711 } 3712 ; 3713 } 3714 3715 protected Procedure typedCopy() { 3716 return copy(); 3717 } 3718 3719 @Override 3720 public boolean equalsDeep(Base other_) { 3721 if (!super.equalsDeep(other_)) 3722 return false; 3723 if (!(other_ instanceof Procedure)) 3724 return false; 3725 Procedure o = (Procedure) other_; 3726 return compareDeep(identifier, o.identifier, true) 3727 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 3728 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 3729 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 3730 && compareDeep(statusReason, o.statusReason, true) && compareDeep(category, o.category, true) 3731 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 3732 && compareDeep(encounter, o.encounter, true) && compareDeep(performed, o.performed, true) 3733 && compareDeep(recorder, o.recorder, true) && compareDeep(asserter, o.asserter, true) 3734 && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) 3735 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3736 && compareDeep(bodySite, o.bodySite, true) && compareDeep(outcome, o.outcome, true) 3737 && compareDeep(report, o.report, true) && compareDeep(complication, o.complication, true) 3738 && compareDeep(complicationDetail, o.complicationDetail, true) && compareDeep(followUp, o.followUp, true) 3739 && compareDeep(note, o.note, true) && compareDeep(focalDevice, o.focalDevice, true) 3740 && compareDeep(usedReference, o.usedReference, true) && compareDeep(usedCode, o.usedCode, true); 3741 } 3742 3743 @Override 3744 public boolean equalsShallow(Base other_) { 3745 if (!super.equalsShallow(other_)) 3746 return false; 3747 if (!(other_ instanceof Procedure)) 3748 return false; 3749 Procedure o = (Procedure) other_; 3750 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true); 3751 } 3752 3753 public boolean isEmpty() { 3754 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 3755 basedOn, partOf, status, statusReason, category, code, subject, encounter, performed, recorder, asserter, 3756 performer, location, reasonCode, reasonReference, bodySite, outcome, report, complication, complicationDetail, 3757 followUp, note, focalDevice, usedReference, usedCode); 3758 } 3759 3760 @Override 3761 public ResourceType getResourceType() { 3762 return ResourceType.Procedure; 3763 } 3764 3765 /** 3766 * Search parameter: <b>date</b> 3767 * <p> 3768 * Description: <b>When the procedure was performed</b><br> 3769 * Type: <b>date</b><br> 3770 * Path: <b>Procedure.performed[x]</b><br> 3771 * </p> 3772 */ 3773 @SearchParamDefinition(name = "date", path = "Procedure.performed", description = "When the procedure was performed", type = "date") 3774 public static final String SP_DATE = "date"; 3775 /** 3776 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3777 * <p> 3778 * Description: <b>When the procedure was performed</b><br> 3779 * Type: <b>date</b><br> 3780 * Path: <b>Procedure.performed[x]</b><br> 3781 * </p> 3782 */ 3783 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3784 SP_DATE); 3785 3786 /** 3787 * Search parameter: <b>identifier</b> 3788 * <p> 3789 * Description: <b>A unique identifier for a procedure</b><br> 3790 * Type: <b>token</b><br> 3791 * Path: <b>Procedure.identifier</b><br> 3792 * </p> 3793 */ 3794 @SearchParamDefinition(name = "identifier", path = "Procedure.identifier", description = "A unique identifier for a procedure", type = "token") 3795 public static final String SP_IDENTIFIER = "identifier"; 3796 /** 3797 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3798 * <p> 3799 * Description: <b>A unique identifier for a procedure</b><br> 3800 * Type: <b>token</b><br> 3801 * Path: <b>Procedure.identifier</b><br> 3802 * </p> 3803 */ 3804 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3805 SP_IDENTIFIER); 3806 3807 /** 3808 * Search parameter: <b>code</b> 3809 * <p> 3810 * Description: <b>A code to identify a procedure</b><br> 3811 * Type: <b>token</b><br> 3812 * Path: <b>Procedure.code</b><br> 3813 * </p> 3814 */ 3815 @SearchParamDefinition(name = "code", path = "Procedure.code", description = "A code to identify a procedure", type = "token") 3816 public static final String SP_CODE = "code"; 3817 /** 3818 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3819 * <p> 3820 * Description: <b>A code to identify a procedure</b><br> 3821 * Type: <b>token</b><br> 3822 * Path: <b>Procedure.code</b><br> 3823 * </p> 3824 */ 3825 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3826 SP_CODE); 3827 3828 /** 3829 * Search parameter: <b>performer</b> 3830 * <p> 3831 * Description: <b>The reference to the practitioner</b><br> 3832 * Type: <b>reference</b><br> 3833 * Path: <b>Procedure.performer.actor</b><br> 3834 * </p> 3835 */ 3836 @SearchParamDefinition(name = "performer", path = "Procedure.performer.actor", description = "The reference to the practitioner", type = "reference", providesMembershipIn = { 3837 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3838 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3839 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3840 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3841 public static final String SP_PERFORMER = "performer"; 3842 /** 3843 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3844 * <p> 3845 * Description: <b>The reference to the practitioner</b><br> 3846 * Type: <b>reference</b><br> 3847 * Path: <b>Procedure.performer.actor</b><br> 3848 * </p> 3849 */ 3850 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3851 SP_PERFORMER); 3852 3853 /** 3854 * Constant for fluent queries to be used to add include statements. Specifies 3855 * the path value of "<b>Procedure:performer</b>". 3856 */ 3857 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 3858 "Procedure:performer").toLocked(); 3859 3860 /** 3861 * Search parameter: <b>subject</b> 3862 * <p> 3863 * Description: <b>Search by subject</b><br> 3864 * Type: <b>reference</b><br> 3865 * Path: <b>Procedure.subject</b><br> 3866 * </p> 3867 */ 3868 @SearchParamDefinition(name = "subject", path = "Procedure.subject", description = "Search by subject", type = "reference", target = { 3869 Group.class, Patient.class }) 3870 public static final String SP_SUBJECT = "subject"; 3871 /** 3872 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3873 * <p> 3874 * Description: <b>Search by subject</b><br> 3875 * Type: <b>reference</b><br> 3876 * Path: <b>Procedure.subject</b><br> 3877 * </p> 3878 */ 3879 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3880 SP_SUBJECT); 3881 3882 /** 3883 * Constant for fluent queries to be used to add include statements. Specifies 3884 * the path value of "<b>Procedure:subject</b>". 3885 */ 3886 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3887 "Procedure:subject").toLocked(); 3888 3889 /** 3890 * Search parameter: <b>instantiates-canonical</b> 3891 * <p> 3892 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3893 * Type: <b>reference</b><br> 3894 * Path: <b>Procedure.instantiatesCanonical</b><br> 3895 * </p> 3896 */ 3897 @SearchParamDefinition(name = "instantiates-canonical", path = "Procedure.instantiatesCanonical", description = "Instantiates FHIR protocol or definition", type = "reference", target = { 3898 ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class }) 3899 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 3900 /** 3901 * <b>Fluent Client</b> search parameter constant for 3902 * <b>instantiates-canonical</b> 3903 * <p> 3904 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3905 * Type: <b>reference</b><br> 3906 * Path: <b>Procedure.instantiatesCanonical</b><br> 3907 * </p> 3908 */ 3909 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3910 SP_INSTANTIATES_CANONICAL); 3911 3912 /** 3913 * Constant for fluent queries to be used to add include statements. Specifies 3914 * the path value of "<b>Procedure:instantiates-canonical</b>". 3915 */ 3916 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 3917 "Procedure:instantiates-canonical").toLocked(); 3918 3919 /** 3920 * Search parameter: <b>part-of</b> 3921 * <p> 3922 * Description: <b>Part of referenced event</b><br> 3923 * Type: <b>reference</b><br> 3924 * Path: <b>Procedure.partOf</b><br> 3925 * </p> 3926 */ 3927 @SearchParamDefinition(name = "part-of", path = "Procedure.partOf", description = "Part of referenced event", type = "reference", target = { 3928 MedicationAdministration.class, Observation.class, Procedure.class }) 3929 public static final String SP_PART_OF = "part-of"; 3930 /** 3931 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 3932 * <p> 3933 * Description: <b>Part of referenced event</b><br> 3934 * Type: <b>reference</b><br> 3935 * Path: <b>Procedure.partOf</b><br> 3936 * </p> 3937 */ 3938 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3939 SP_PART_OF); 3940 3941 /** 3942 * Constant for fluent queries to be used to add include statements. Specifies 3943 * the path value of "<b>Procedure:part-of</b>". 3944 */ 3945 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 3946 "Procedure:part-of").toLocked(); 3947 3948 /** 3949 * Search parameter: <b>encounter</b> 3950 * <p> 3951 * Description: <b>Encounter created as part of</b><br> 3952 * Type: <b>reference</b><br> 3953 * Path: <b>Procedure.encounter</b><br> 3954 * </p> 3955 */ 3956 @SearchParamDefinition(name = "encounter", path = "Procedure.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 3957 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 3958 public static final String SP_ENCOUNTER = "encounter"; 3959 /** 3960 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3961 * <p> 3962 * Description: <b>Encounter created as part of</b><br> 3963 * Type: <b>reference</b><br> 3964 * Path: <b>Procedure.encounter</b><br> 3965 * </p> 3966 */ 3967 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3968 SP_ENCOUNTER); 3969 3970 /** 3971 * Constant for fluent queries to be used to add include statements. Specifies 3972 * the path value of "<b>Procedure:encounter</b>". 3973 */ 3974 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3975 "Procedure:encounter").toLocked(); 3976 3977 /** 3978 * Search parameter: <b>reason-code</b> 3979 * <p> 3980 * Description: <b>Coded reason procedure performed</b><br> 3981 * Type: <b>token</b><br> 3982 * Path: <b>Procedure.reasonCode</b><br> 3983 * </p> 3984 */ 3985 @SearchParamDefinition(name = "reason-code", path = "Procedure.reasonCode", description = "Coded reason procedure performed", type = "token") 3986 public static final String SP_REASON_CODE = "reason-code"; 3987 /** 3988 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 3989 * <p> 3990 * Description: <b>Coded reason procedure performed</b><br> 3991 * Type: <b>token</b><br> 3992 * Path: <b>Procedure.reasonCode</b><br> 3993 * </p> 3994 */ 3995 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3996 SP_REASON_CODE); 3997 3998 /** 3999 * Search parameter: <b>based-on</b> 4000 * <p> 4001 * Description: <b>A request for this procedure</b><br> 4002 * Type: <b>reference</b><br> 4003 * Path: <b>Procedure.basedOn</b><br> 4004 * </p> 4005 */ 4006 @SearchParamDefinition(name = "based-on", path = "Procedure.basedOn", description = "A request for this procedure", type = "reference", target = { 4007 CarePlan.class, ServiceRequest.class }) 4008 public static final String SP_BASED_ON = "based-on"; 4009 /** 4010 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 4011 * <p> 4012 * Description: <b>A request for this procedure</b><br> 4013 * Type: <b>reference</b><br> 4014 * Path: <b>Procedure.basedOn</b><br> 4015 * </p> 4016 */ 4017 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4018 SP_BASED_ON); 4019 4020 /** 4021 * Constant for fluent queries to be used to add include statements. Specifies 4022 * the path value of "<b>Procedure:based-on</b>". 4023 */ 4024 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 4025 "Procedure:based-on").toLocked(); 4026 4027 /** 4028 * Search parameter: <b>patient</b> 4029 * <p> 4030 * Description: <b>Search by subject - a patient</b><br> 4031 * Type: <b>reference</b><br> 4032 * Path: <b>Procedure.subject</b><br> 4033 * </p> 4034 */ 4035 @SearchParamDefinition(name = "patient", path = "Procedure.subject.where(resolve() is Patient)", description = "Search by subject - a patient", type = "reference", providesMembershipIn = { 4036 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 4037 public static final String SP_PATIENT = "patient"; 4038 /** 4039 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4040 * <p> 4041 * Description: <b>Search by subject - a patient</b><br> 4042 * Type: <b>reference</b><br> 4043 * Path: <b>Procedure.subject</b><br> 4044 * </p> 4045 */ 4046 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4047 SP_PATIENT); 4048 4049 /** 4050 * Constant for fluent queries to be used to add include statements. Specifies 4051 * the path value of "<b>Procedure:patient</b>". 4052 */ 4053 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4054 "Procedure:patient").toLocked(); 4055 4056 /** 4057 * Search parameter: <b>reason-reference</b> 4058 * <p> 4059 * Description: <b>The justification that the procedure was performed</b><br> 4060 * Type: <b>reference</b><br> 4061 * Path: <b>Procedure.reasonReference</b><br> 4062 * </p> 4063 */ 4064 @SearchParamDefinition(name = "reason-reference", path = "Procedure.reasonReference", description = "The justification that the procedure was performed", type = "reference", target = { 4065 Condition.class, DiagnosticReport.class, DocumentReference.class, Observation.class, Procedure.class }) 4066 public static final String SP_REASON_REFERENCE = "reason-reference"; 4067 /** 4068 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 4069 * <p> 4070 * Description: <b>The justification that the procedure was performed</b><br> 4071 * Type: <b>reference</b><br> 4072 * Path: <b>Procedure.reasonReference</b><br> 4073 * </p> 4074 */ 4075 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4076 SP_REASON_REFERENCE); 4077 4078 /** 4079 * Constant for fluent queries to be used to add include statements. Specifies 4080 * the path value of "<b>Procedure:reason-reference</b>". 4081 */ 4082 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include( 4083 "Procedure:reason-reference").toLocked(); 4084 4085 /** 4086 * Search parameter: <b>location</b> 4087 * <p> 4088 * Description: <b>Where the procedure happened</b><br> 4089 * Type: <b>reference</b><br> 4090 * Path: <b>Procedure.location</b><br> 4091 * </p> 4092 */ 4093 @SearchParamDefinition(name = "location", path = "Procedure.location", description = "Where the procedure happened", type = "reference", target = { 4094 Location.class }) 4095 public static final String SP_LOCATION = "location"; 4096 /** 4097 * <b>Fluent Client</b> search parameter constant for <b>location</b> 4098 * <p> 4099 * Description: <b>Where the procedure happened</b><br> 4100 * Type: <b>reference</b><br> 4101 * Path: <b>Procedure.location</b><br> 4102 * </p> 4103 */ 4104 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4105 SP_LOCATION); 4106 4107 /** 4108 * Constant for fluent queries to be used to add include statements. Specifies 4109 * the path value of "<b>Procedure:location</b>". 4110 */ 4111 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 4112 "Procedure:location").toLocked(); 4113 4114 /** 4115 * Search parameter: <b>instantiates-uri</b> 4116 * <p> 4117 * Description: <b>Instantiates external protocol or definition</b><br> 4118 * Type: <b>uri</b><br> 4119 * Path: <b>Procedure.instantiatesUri</b><br> 4120 * </p> 4121 */ 4122 @SearchParamDefinition(name = "instantiates-uri", path = "Procedure.instantiatesUri", description = "Instantiates external protocol or definition", type = "uri") 4123 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 4124 /** 4125 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 4126 * <p> 4127 * Description: <b>Instantiates external protocol or definition</b><br> 4128 * Type: <b>uri</b><br> 4129 * Path: <b>Procedure.instantiatesUri</b><br> 4130 * </p> 4131 */ 4132 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 4133 SP_INSTANTIATES_URI); 4134 4135 /** 4136 * Search parameter: <b>category</b> 4137 * <p> 4138 * Description: <b>Classification of the procedure</b><br> 4139 * Type: <b>token</b><br> 4140 * Path: <b>Procedure.category</b><br> 4141 * </p> 4142 */ 4143 @SearchParamDefinition(name = "category", path = "Procedure.category", description = "Classification of the procedure", type = "token") 4144 public static final String SP_CATEGORY = "category"; 4145 /** 4146 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4147 * <p> 4148 * Description: <b>Classification of the procedure</b><br> 4149 * Type: <b>token</b><br> 4150 * Path: <b>Procedure.category</b><br> 4151 * </p> 4152 */ 4153 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4154 SP_CATEGORY); 4155 4156 /** 4157 * Search parameter: <b>status</b> 4158 * <p> 4159 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | 4160 * completed | entered-in-error | unknown</b><br> 4161 * Type: <b>token</b><br> 4162 * Path: <b>Procedure.status</b><br> 4163 * </p> 4164 */ 4165 @SearchParamDefinition(name = "status", path = "Procedure.status", description = "preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", type = "token") 4166 public static final String SP_STATUS = "status"; 4167 /** 4168 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4169 * <p> 4170 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | 4171 * completed | entered-in-error | unknown</b><br> 4172 * Type: <b>token</b><br> 4173 * Path: <b>Procedure.status</b><br> 4174 * </p> 4175 */ 4176 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4177 SP_STATUS); 4178 4179}