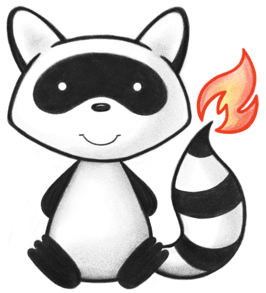
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * The shelf-life and storage information for a medicinal product item or 045 * container can be described using this class. 046 */ 047@DatatypeDef(name = "ProductShelfLife") 048public class ProductShelfLife extends BackboneType implements ICompositeType { 049 050 /** 051 * Unique identifier for the packaged Medicinal Product. 052 */ 053 @Child(name = "identifier", type = { 054 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 055 @Description(shortDefinition = "Unique identifier for the packaged Medicinal Product", formalDefinition = "Unique identifier for the packaged Medicinal Product.") 056 protected Identifier identifier; 057 058 /** 059 * This describes the shelf life, taking into account various scenarios such as 060 * shelf life of the packaged Medicinal Product itself, shelf life after 061 * transformation where necessary and shelf life after the first opening of a 062 * bottle, etc. The shelf life type shall be specified using an appropriate 063 * controlled vocabulary The controlled term and the controlled term identifier 064 * shall be specified. 065 */ 066 @Child(name = "type", type = { CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 067 @Description(shortDefinition = "This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified", formalDefinition = "This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.") 068 protected CodeableConcept type; 069 070 /** 071 * The shelf life time period can be specified using a numerical value for the 072 * period of time and its unit of time measurement The unit of measurement shall 073 * be specified in accordance with ISO 11240 and the resulting terminology The 074 * symbol and the symbol identifier shall be used. 075 */ 076 @Child(name = "period", type = { Quantity.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 077 @Description(shortDefinition = "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used", formalDefinition = "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.") 078 protected Quantity period; 079 080 /** 081 * Special precautions for storage, if any, can be specified using an 082 * appropriate controlled vocabulary The controlled term and the controlled term 083 * identifier shall be specified. 084 */ 085 @Child(name = "specialPrecautionsForStorage", type = { 086 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 087 @Description(shortDefinition = "Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified", formalDefinition = "Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.") 088 protected List<CodeableConcept> specialPrecautionsForStorage; 089 090 private static final long serialVersionUID = -1561196410L; 091 092 /** 093 * Constructor 094 */ 095 public ProductShelfLife() { 096 super(); 097 } 098 099 /** 100 * Constructor 101 */ 102 public ProductShelfLife(CodeableConcept type, Quantity period) { 103 super(); 104 this.type = type; 105 this.period = period; 106 } 107 108 /** 109 * @return {@link #identifier} (Unique identifier for the packaged Medicinal 110 * Product.) 111 */ 112 public Identifier getIdentifier() { 113 if (this.identifier == null) 114 if (Configuration.errorOnAutoCreate()) 115 throw new Error("Attempt to auto-create ProductShelfLife.identifier"); 116 else if (Configuration.doAutoCreate()) 117 this.identifier = new Identifier(); // cc 118 return this.identifier; 119 } 120 121 public boolean hasIdentifier() { 122 return this.identifier != null && !this.identifier.isEmpty(); 123 } 124 125 /** 126 * @param value {@link #identifier} (Unique identifier for the packaged 127 * Medicinal Product.) 128 */ 129 public ProductShelfLife setIdentifier(Identifier value) { 130 this.identifier = value; 131 return this; 132 } 133 134 /** 135 * @return {@link #type} (This describes the shelf life, taking into account 136 * various scenarios such as shelf life of the packaged Medicinal 137 * Product itself, shelf life after transformation where necessary and 138 * shelf life after the first opening of a bottle, etc. The shelf life 139 * type shall be specified using an appropriate controlled vocabulary 140 * The controlled term and the controlled term identifier shall be 141 * specified.) 142 */ 143 public CodeableConcept getType() { 144 if (this.type == null) 145 if (Configuration.errorOnAutoCreate()) 146 throw new Error("Attempt to auto-create ProductShelfLife.type"); 147 else if (Configuration.doAutoCreate()) 148 this.type = new CodeableConcept(); // cc 149 return this.type; 150 } 151 152 public boolean hasType() { 153 return this.type != null && !this.type.isEmpty(); 154 } 155 156 /** 157 * @param value {@link #type} (This describes the shelf life, taking into 158 * account various scenarios such as shelf life of the packaged 159 * Medicinal Product itself, shelf life after transformation where 160 * necessary and shelf life after the first opening of a bottle, 161 * etc. The shelf life type shall be specified using an appropriate 162 * controlled vocabulary The controlled term and the controlled 163 * term identifier shall be specified.) 164 */ 165 public ProductShelfLife setType(CodeableConcept value) { 166 this.type = value; 167 return this; 168 } 169 170 /** 171 * @return {@link #period} (The shelf life time period can be specified using a 172 * numerical value for the period of time and its unit of time 173 * measurement The unit of measurement shall be specified in accordance 174 * with ISO 11240 and the resulting terminology The symbol and the 175 * symbol identifier shall be used.) 176 */ 177 public Quantity getPeriod() { 178 if (this.period == null) 179 if (Configuration.errorOnAutoCreate()) 180 throw new Error("Attempt to auto-create ProductShelfLife.period"); 181 else if (Configuration.doAutoCreate()) 182 this.period = new Quantity(); // cc 183 return this.period; 184 } 185 186 public boolean hasPeriod() { 187 return this.period != null && !this.period.isEmpty(); 188 } 189 190 /** 191 * @param value {@link #period} (The shelf life time period can be specified 192 * using a numerical value for the period of time and its unit of 193 * time measurement The unit of measurement shall be specified in 194 * accordance with ISO 11240 and the resulting terminology The 195 * symbol and the symbol identifier shall be used.) 196 */ 197 public ProductShelfLife setPeriod(Quantity value) { 198 this.period = value; 199 return this; 200 } 201 202 /** 203 * @return {@link #specialPrecautionsForStorage} (Special precautions for 204 * storage, if any, can be specified using an appropriate controlled 205 * vocabulary The controlled term and the controlled term identifier 206 * shall be specified.) 207 */ 208 public List<CodeableConcept> getSpecialPrecautionsForStorage() { 209 if (this.specialPrecautionsForStorage == null) 210 this.specialPrecautionsForStorage = new ArrayList<CodeableConcept>(); 211 return this.specialPrecautionsForStorage; 212 } 213 214 /** 215 * @return Returns a reference to <code>this</code> for easy method chaining 216 */ 217 public ProductShelfLife setSpecialPrecautionsForStorage(List<CodeableConcept> theSpecialPrecautionsForStorage) { 218 this.specialPrecautionsForStorage = theSpecialPrecautionsForStorage; 219 return this; 220 } 221 222 public boolean hasSpecialPrecautionsForStorage() { 223 if (this.specialPrecautionsForStorage == null) 224 return false; 225 for (CodeableConcept item : this.specialPrecautionsForStorage) 226 if (!item.isEmpty()) 227 return true; 228 return false; 229 } 230 231 public CodeableConcept addSpecialPrecautionsForStorage() { // 3 232 CodeableConcept t = new CodeableConcept(); 233 if (this.specialPrecautionsForStorage == null) 234 this.specialPrecautionsForStorage = new ArrayList<CodeableConcept>(); 235 this.specialPrecautionsForStorage.add(t); 236 return t; 237 } 238 239 public ProductShelfLife addSpecialPrecautionsForStorage(CodeableConcept t) { // 3 240 if (t == null) 241 return this; 242 if (this.specialPrecautionsForStorage == null) 243 this.specialPrecautionsForStorage = new ArrayList<CodeableConcept>(); 244 this.specialPrecautionsForStorage.add(t); 245 return this; 246 } 247 248 /** 249 * @return The first repetition of repeating field 250 * {@link #specialPrecautionsForStorage}, creating it if it does not 251 * already exist 252 */ 253 public CodeableConcept getSpecialPrecautionsForStorageFirstRep() { 254 if (getSpecialPrecautionsForStorage().isEmpty()) { 255 addSpecialPrecautionsForStorage(); 256 } 257 return getSpecialPrecautionsForStorage().get(0); 258 } 259 260 protected void listChildren(List<Property> children) { 261 super.listChildren(children); 262 children.add(new Property("identifier", "Identifier", "Unique identifier for the packaged Medicinal Product.", 0, 1, 263 identifier)); 264 children.add(new Property("type", "CodeableConcept", 265 "This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.", 266 0, 1, type)); 267 children.add(new Property("period", "Quantity", 268 "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 269 0, 1, period)); 270 children.add(new Property("specialPrecautionsForStorage", "CodeableConcept", 271 "Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.", 272 0, java.lang.Integer.MAX_VALUE, specialPrecautionsForStorage)); 273 } 274 275 @Override 276 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 277 switch (_hash) { 278 case -1618432855: 279 /* identifier */ return new Property("identifier", "Identifier", 280 "Unique identifier for the packaged Medicinal Product.", 0, 1, identifier); 281 case 3575610: 282 /* type */ return new Property("type", "CodeableConcept", 283 "This describes the shelf life, taking into account various scenarios such as shelf life of the packaged Medicinal Product itself, shelf life after transformation where necessary and shelf life after the first opening of a bottle, etc. The shelf life type shall be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.", 284 0, 1, type); 285 case -991726143: 286 /* period */ return new Property("period", "Quantity", 287 "The shelf life time period can be specified using a numerical value for the period of time and its unit of time measurement The unit of measurement shall be specified in accordance with ISO 11240 and the resulting terminology The symbol and the symbol identifier shall be used.", 288 0, 1, period); 289 case 2103459492: 290 /* specialPrecautionsForStorage */ return new Property("specialPrecautionsForStorage", "CodeableConcept", 291 "Special precautions for storage, if any, can be specified using an appropriate controlled vocabulary The controlled term and the controlled term identifier shall be specified.", 292 0, java.lang.Integer.MAX_VALUE, specialPrecautionsForStorage); 293 default: 294 return super.getNamedProperty(_hash, _name, _checkValid); 295 } 296 297 } 298 299 @Override 300 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 301 switch (hash) { 302 case -1618432855: 303 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 304 case 3575610: 305 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 306 case -991726143: 307 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Quantity 308 case 2103459492: 309 /* specialPrecautionsForStorage */ return this.specialPrecautionsForStorage == null ? new Base[0] 310 : this.specialPrecautionsForStorage.toArray(new Base[this.specialPrecautionsForStorage.size()]); // CodeableConcept 311 default: 312 return super.getProperty(hash, name, checkValid); 313 } 314 315 } 316 317 @Override 318 public Base setProperty(int hash, String name, Base value) throws FHIRException { 319 switch (hash) { 320 case -1618432855: // identifier 321 this.identifier = castToIdentifier(value); // Identifier 322 return value; 323 case 3575610: // type 324 this.type = castToCodeableConcept(value); // CodeableConcept 325 return value; 326 case -991726143: // period 327 this.period = castToQuantity(value); // Quantity 328 return value; 329 case 2103459492: // specialPrecautionsForStorage 330 this.getSpecialPrecautionsForStorage().add(castToCodeableConcept(value)); // CodeableConcept 331 return value; 332 default: 333 return super.setProperty(hash, name, value); 334 } 335 336 } 337 338 @Override 339 public Base setProperty(String name, Base value) throws FHIRException { 340 if (name.equals("identifier")) { 341 this.identifier = castToIdentifier(value); // Identifier 342 } else if (name.equals("type")) { 343 this.type = castToCodeableConcept(value); // CodeableConcept 344 } else if (name.equals("period")) { 345 this.period = castToQuantity(value); // Quantity 346 } else if (name.equals("specialPrecautionsForStorage")) { 347 this.getSpecialPrecautionsForStorage().add(castToCodeableConcept(value)); 348 } else 349 return super.setProperty(name, value); 350 return value; 351 } 352 353 @Override 354 public Base makeProperty(int hash, String name) throws FHIRException { 355 switch (hash) { 356 case -1618432855: 357 return getIdentifier(); 358 case 3575610: 359 return getType(); 360 case -991726143: 361 return getPeriod(); 362 case 2103459492: 363 return addSpecialPrecautionsForStorage(); 364 default: 365 return super.makeProperty(hash, name); 366 } 367 368 } 369 370 @Override 371 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 372 switch (hash) { 373 case -1618432855: 374 /* identifier */ return new String[] { "Identifier" }; 375 case 3575610: 376 /* type */ return new String[] { "CodeableConcept" }; 377 case -991726143: 378 /* period */ return new String[] { "Quantity" }; 379 case 2103459492: 380 /* specialPrecautionsForStorage */ return new String[] { "CodeableConcept" }; 381 default: 382 return super.getTypesForProperty(hash, name); 383 } 384 385 } 386 387 @Override 388 public Base addChild(String name) throws FHIRException { 389 if (name.equals("identifier")) { 390 this.identifier = new Identifier(); 391 return this.identifier; 392 } else if (name.equals("type")) { 393 this.type = new CodeableConcept(); 394 return this.type; 395 } else if (name.equals("period")) { 396 this.period = new Quantity(); 397 return this.period; 398 } else if (name.equals("specialPrecautionsForStorage")) { 399 return addSpecialPrecautionsForStorage(); 400 } else 401 return super.addChild(name); 402 } 403 404 public String fhirType() { 405 return "ProductShelfLife"; 406 407 } 408 409 public ProductShelfLife copy() { 410 ProductShelfLife dst = new ProductShelfLife(); 411 copyValues(dst); 412 return dst; 413 } 414 415 public void copyValues(ProductShelfLife dst) { 416 super.copyValues(dst); 417 dst.identifier = identifier == null ? null : identifier.copy(); 418 dst.type = type == null ? null : type.copy(); 419 dst.period = period == null ? null : period.copy(); 420 if (specialPrecautionsForStorage != null) { 421 dst.specialPrecautionsForStorage = new ArrayList<CodeableConcept>(); 422 for (CodeableConcept i : specialPrecautionsForStorage) 423 dst.specialPrecautionsForStorage.add(i.copy()); 424 } 425 ; 426 } 427 428 protected ProductShelfLife typedCopy() { 429 return copy(); 430 } 431 432 @Override 433 public boolean equalsDeep(Base other_) { 434 if (!super.equalsDeep(other_)) 435 return false; 436 if (!(other_ instanceof ProductShelfLife)) 437 return false; 438 ProductShelfLife o = (ProductShelfLife) other_; 439 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 440 && compareDeep(period, o.period, true) 441 && compareDeep(specialPrecautionsForStorage, o.specialPrecautionsForStorage, true); 442 } 443 444 @Override 445 public boolean equalsShallow(Base other_) { 446 if (!super.equalsShallow(other_)) 447 return false; 448 if (!(other_ instanceof ProductShelfLife)) 449 return false; 450 ProductShelfLife o = (ProductShelfLife) other_; 451 return true; 452 } 453 454 public boolean isEmpty() { 455 return super.isEmpty() 456 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, period, specialPrecautionsForStorage); 457 } 458 459}