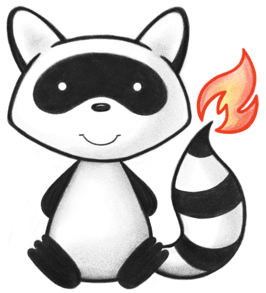
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * A measured amount (or an amount that can potentially be measured). Note that 047 * measured amounts include amounts that are not precisely quantified, including 048 * amounts involving arbitrary units and floating currencies. 049 */ 050@DatatypeDef(name = "Quantity") 051public class Quantity extends Type implements ICompositeType, ICoding { 052 053 public enum QuantityComparator { 054 /** 055 * The actual value is less than the given value. 056 */ 057 LESS_THAN, 058 /** 059 * The actual value is less than or equal to the given value. 060 */ 061 LESS_OR_EQUAL, 062 /** 063 * The actual value is greater than or equal to the given value. 064 */ 065 GREATER_OR_EQUAL, 066 /** 067 * The actual value is greater than the given value. 068 */ 069 GREATER_THAN, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 075 public static QuantityComparator fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("<".equals(codeString)) 079 return LESS_THAN; 080 if ("<=".equals(codeString)) 081 return LESS_OR_EQUAL; 082 if (">=".equals(codeString)) 083 return GREATER_OR_EQUAL; 084 if (">".equals(codeString)) 085 return GREATER_THAN; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown QuantityComparator code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case LESS_THAN: 095 return "<"; 096 case LESS_OR_EQUAL: 097 return "<="; 098 case GREATER_OR_EQUAL: 099 return ">="; 100 case GREATER_THAN: 101 return ">"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case LESS_THAN: 112 return "http://hl7.org/fhir/quantity-comparator"; 113 case LESS_OR_EQUAL: 114 return "http://hl7.org/fhir/quantity-comparator"; 115 case GREATER_OR_EQUAL: 116 return "http://hl7.org/fhir/quantity-comparator"; 117 case GREATER_THAN: 118 return "http://hl7.org/fhir/quantity-comparator"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case LESS_THAN: 129 return "The actual value is less than the given value."; 130 case LESS_OR_EQUAL: 131 return "The actual value is less than or equal to the given value."; 132 case GREATER_OR_EQUAL: 133 return "The actual value is greater than or equal to the given value."; 134 case GREATER_THAN: 135 return "The actual value is greater than the given value."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case LESS_THAN: 146 return "Less than"; 147 case LESS_OR_EQUAL: 148 return "Less or Equal to"; 149 case GREATER_OR_EQUAL: 150 return "Greater or Equal to"; 151 case GREATER_THAN: 152 return "Greater than"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class QuantityComparatorEnumFactory implements EnumFactory<QuantityComparator> { 162 public QuantityComparator fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("<".equals(codeString)) 167 return QuantityComparator.LESS_THAN; 168 if ("<=".equals(codeString)) 169 return QuantityComparator.LESS_OR_EQUAL; 170 if (">=".equals(codeString)) 171 return QuantityComparator.GREATER_OR_EQUAL; 172 if (">".equals(codeString)) 173 return QuantityComparator.GREATER_THAN; 174 throw new IllegalArgumentException("Unknown QuantityComparator code '" + codeString + "'"); 175 } 176 177 public Enumeration<QuantityComparator> fromType(PrimitiveType<?> code) throws FHIRException { 178 if (code == null) 179 return null; 180 if (code.isEmpty()) 181 return new Enumeration<QuantityComparator>(this, QuantityComparator.NULL, code); 182 String codeString = code.asStringValue(); 183 if (codeString == null || "".equals(codeString)) 184 return new Enumeration<QuantityComparator>(this, QuantityComparator.NULL, code); 185 if ("<".equals(codeString)) 186 return new Enumeration<QuantityComparator>(this, QuantityComparator.LESS_THAN, code); 187 if ("<=".equals(codeString)) 188 return new Enumeration<QuantityComparator>(this, QuantityComparator.LESS_OR_EQUAL, code); 189 if (">=".equals(codeString)) 190 return new Enumeration<QuantityComparator>(this, QuantityComparator.GREATER_OR_EQUAL, code); 191 if (">".equals(codeString)) 192 return new Enumeration<QuantityComparator>(this, QuantityComparator.GREATER_THAN, code); 193 throw new FHIRException("Unknown QuantityComparator code '" + codeString + "'"); 194 } 195 196 public String toCode(QuantityComparator code) { 197 if (code == QuantityComparator.NULL) 198 return null; 199 if (code == QuantityComparator.LESS_THAN) 200 return "<"; 201 if (code == QuantityComparator.LESS_OR_EQUAL) 202 return "<="; 203 if (code == QuantityComparator.GREATER_OR_EQUAL) 204 return ">="; 205 if (code == QuantityComparator.GREATER_THAN) 206 return ">"; 207 return "?"; 208 } 209 210 public String toSystem(QuantityComparator code) { 211 return code.getSystem(); 212 } 213 } 214 215 /** 216 * The value of the measured amount. The value includes an implicit precision in 217 * the presentation of the value. 218 */ 219 @Child(name = "value", type = { DecimalType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 220 @Description(shortDefinition = "Numerical value (with implicit precision)", formalDefinition = "The value of the measured amount. The value includes an implicit precision in the presentation of the value.") 221 protected DecimalType value; 222 223 /** 224 * How the value should be understood and represented - whether the actual value 225 * is greater or less than the stated value due to measurement issues; e.g. if 226 * the comparator is "<" , then the real value is < stated value. 227 */ 228 @Child(name = "comparator", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 229 @Description(shortDefinition = "< | <= | >= | > - how to understand the value", formalDefinition = "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.") 230 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/quantity-comparator") 231 protected Enumeration<QuantityComparator> comparator; 232 233 /** 234 * A human-readable form of the unit. 235 */ 236 @Child(name = "unit", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 237 @Description(shortDefinition = "Unit representation", formalDefinition = "A human-readable form of the unit.") 238 protected StringType unit; 239 240 /** 241 * The identification of the system that provides the coded form of the unit. 242 */ 243 @Child(name = "system", type = { UriType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 244 @Description(shortDefinition = "System that defines coded unit form", formalDefinition = "The identification of the system that provides the coded form of the unit.") 245 protected UriType system; 246 247 /** 248 * A computer processable form of the unit in some unit representation system. 249 */ 250 @Child(name = "code", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 251 @Description(shortDefinition = "Coded form of the unit", formalDefinition = "A computer processable form of the unit in some unit representation system.") 252 protected CodeType code; 253 254 private static final long serialVersionUID = 1069574054L; 255 256 /** 257 * Constructor 258 */ 259 public Quantity() { 260 super(); 261 } 262 263 /** 264 * Convenience constructor 265 * 266 * @param theValue The {@link #setValue(double) value} 267 */ 268 public Quantity(double theValue) { 269 setValue(theValue); 270 } 271 272 /** 273 * Convenience constructor 274 * 275 * @param theValue The {@link #setValue(long) value} 276 */ 277 public Quantity(long theValue) { 278 setValue(theValue); 279 } 280 281 /** 282 * Convenience constructor 283 * 284 * @param theComparator The {@link #setComparator(QuantityComparator) 285 * comparator} 286 * @param theValue The {@link #setValue(BigDecimal) value} 287 * @param theSystem The {@link #setSystem(String)} (the code system for the 288 * units} 289 * @param theCode The {@link #setCode(String)} (the code for the units} 290 * @param theUnit The {@link #setUnit(String)} (the human readable display 291 * name for the units} 292 */ 293 public Quantity(QuantityComparator theComparator, double theValue, String theSystem, String theCode, String theUnit) { 294 setValue(theValue); 295 setComparator(theComparator); 296 setSystem(theSystem); 297 setCode(theCode); 298 setUnit(theUnit); 299 } 300 301 /** 302 * Convenience constructor 303 * 304 * @param theComparator The {@link #setComparator(QuantityComparator) 305 * comparator} 306 * @param theValue The {@link #setValue(BigDecimal) value} 307 * @param theSystem The {@link #setSystem(String)} (the code system for the 308 * units} 309 * @param theCode The {@link #setCode(String)} (the code for the units} 310 * @param theUnit The {@link #setUnit(String)} (the human readable display 311 * name for the units} 312 */ 313 public Quantity(QuantityComparator theComparator, long theValue, String theSystem, String theCode, String theUnit) { 314 setValue(theValue); 315 setComparator(theComparator); 316 setSystem(theSystem); 317 setCode(theCode); 318 setUnit(theUnit); 319 } 320 321 /** 322 * @return {@link #value} (The value of the measured amount. The value includes 323 * an implicit precision in the presentation of the value.). This is the 324 * underlying object with id, value and extensions. The accessor 325 * "getValue" gives direct access to the value 326 */ 327 public DecimalType getValueElement() { 328 if (this.value == null) 329 if (Configuration.errorOnAutoCreate()) 330 throw new Error("Attempt to auto-create Quantity.value"); 331 else if (Configuration.doAutoCreate()) 332 this.value = new DecimalType(); // bb 333 return this.value; 334 } 335 336 public boolean hasValueElement() { 337 return this.value != null && !this.value.isEmpty(); 338 } 339 340 public boolean hasValue() { 341 return this.value != null && !this.value.isEmpty(); 342 } 343 344 /** 345 * @param value {@link #value} (The value of the measured amount. The value 346 * includes an implicit precision in the presentation of the 347 * value.). This is the underlying object with id, value and 348 * extensions. The accessor "getValue" gives direct access to the 349 * value 350 */ 351 public Quantity setValueElement(DecimalType value) { 352 this.value = value; 353 return this; 354 } 355 356 /** 357 * @return The value of the measured amount. The value includes an implicit 358 * precision in the presentation of the value. 359 */ 360 public BigDecimal getValue() { 361 return this.value == null ? null : this.value.getValue(); 362 } 363 364 /** 365 * @param value The value of the measured amount. The value includes an implicit 366 * precision in the presentation of the value. 367 */ 368 public Quantity setValue(BigDecimal value) { 369 if (value == null) 370 this.value = null; 371 else { 372 if (this.value == null) 373 this.value = new DecimalType(); 374 this.value.setValue(value); 375 } 376 return this; 377 } 378 379 /** 380 * @param value The value of the measured amount. The value includes an implicit 381 * precision in the presentation of the value. 382 */ 383 public Quantity setValue(long value) { 384 this.value = new DecimalType(); 385 this.value.setValue(value); 386 return this; 387 } 388 389 /** 390 * @param value The value of the measured amount. The value includes an implicit 391 * precision in the presentation of the value. 392 */ 393 public Quantity setValue(double value) { 394 this.value = new DecimalType(); 395 this.value.setValue(value); 396 return this; 397 } 398 399 /** 400 * @return {@link #comparator} (How the value should be understood and 401 * represented - whether the actual value is greater or less than the 402 * stated value due to measurement issues; e.g. if the comparator is "<" 403 * , then the real value is < stated value.). This is the underlying 404 * object with id, value and extensions. The accessor "getComparator" 405 * gives direct access to the value 406 */ 407 public Enumeration<QuantityComparator> getComparatorElement() { 408 if (this.comparator == null) 409 if (Configuration.errorOnAutoCreate()) 410 throw new Error("Attempt to auto-create Quantity.comparator"); 411 else if (Configuration.doAutoCreate()) 412 this.comparator = new Enumeration<QuantityComparator>(new QuantityComparatorEnumFactory()); // bb 413 return this.comparator; 414 } 415 416 public boolean hasComparatorElement() { 417 return this.comparator != null && !this.comparator.isEmpty(); 418 } 419 420 public boolean hasComparator() { 421 return this.comparator != null && !this.comparator.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #comparator} (How the value should be understood and 426 * represented - whether the actual value is greater or less than 427 * the stated value due to measurement issues; e.g. if the 428 * comparator is "<" , then the real value is < stated value.). 429 * This is the underlying object with id, value and extensions. The 430 * accessor "getComparator" gives direct access to the value 431 */ 432 public Quantity setComparatorElement(Enumeration<QuantityComparator> value) { 433 this.comparator = value; 434 return this; 435 } 436 437 /** 438 * @return How the value should be understood and represented - whether the 439 * actual value is greater or less than the stated value due to 440 * measurement issues; e.g. if the comparator is "<" , then the real 441 * value is < stated value. 442 */ 443 public QuantityComparator getComparator() { 444 return this.comparator == null ? null : this.comparator.getValue(); 445 } 446 447 /** 448 * @param value How the value should be understood and represented - whether the 449 * actual value is greater or less than the stated value due to 450 * measurement issues; e.g. if the comparator is "<" , then the 451 * real value is < stated value. 452 */ 453 public Quantity setComparator(QuantityComparator value) { 454 if (value == null) 455 this.comparator = null; 456 else { 457 if (this.comparator == null) 458 this.comparator = new Enumeration<QuantityComparator>(new QuantityComparatorEnumFactory()); 459 this.comparator.setValue(value); 460 } 461 return this; 462 } 463 464 /** 465 * @return {@link #unit} (A human-readable form of the unit.). This is the 466 * underlying object with id, value and extensions. The accessor 467 * "getUnit" gives direct access to the value 468 */ 469 public StringType getUnitElement() { 470 if (this.unit == null) 471 if (Configuration.errorOnAutoCreate()) 472 throw new Error("Attempt to auto-create Quantity.unit"); 473 else if (Configuration.doAutoCreate()) 474 this.unit = new StringType(); // bb 475 return this.unit; 476 } 477 478 public boolean hasUnitElement() { 479 return this.unit != null && !this.unit.isEmpty(); 480 } 481 482 public boolean hasUnit() { 483 return this.unit != null && !this.unit.isEmpty(); 484 } 485 486 /** 487 * @param value {@link #unit} (A human-readable form of the unit.). This is the 488 * underlying object with id, value and extensions. The accessor 489 * "getUnit" gives direct access to the value 490 */ 491 public Quantity setUnitElement(StringType value) { 492 this.unit = value; 493 return this; 494 } 495 496 /** 497 * @return A human-readable form of the unit. 498 */ 499 public String getUnit() { 500 return this.unit == null ? null : this.unit.getValue(); 501 } 502 503 /** 504 * @param value A human-readable form of the unit. 505 */ 506 public Quantity setUnit(String value) { 507 if (Utilities.noString(value)) 508 this.unit = null; 509 else { 510 if (this.unit == null) 511 this.unit = new StringType(); 512 this.unit.setValue(value); 513 } 514 return this; 515 } 516 517 /** 518 * @return {@link #system} (The identification of the system that provides the 519 * coded form of the unit.). This is the underlying object with id, 520 * value and extensions. The accessor "getSystem" gives direct access to 521 * the value 522 */ 523 public UriType getSystemElement() { 524 if (this.system == null) 525 if (Configuration.errorOnAutoCreate()) 526 throw new Error("Attempt to auto-create Quantity.system"); 527 else if (Configuration.doAutoCreate()) 528 this.system = new UriType(); // bb 529 return this.system; 530 } 531 532 public boolean hasSystemElement() { 533 return this.system != null && !this.system.isEmpty(); 534 } 535 536 public boolean hasSystem() { 537 return this.system != null && !this.system.isEmpty(); 538 } 539 540 /** 541 * @param value {@link #system} (The identification of the system that provides 542 * the coded form of the unit.). This is the underlying object with 543 * id, value and extensions. The accessor "getSystem" gives direct 544 * access to the value 545 */ 546 public Quantity setSystemElement(UriType value) { 547 this.system = value; 548 return this; 549 } 550 551 /** 552 * @return The identification of the system that provides the coded form of the 553 * unit. 554 */ 555 public String getSystem() { 556 return this.system == null ? null : this.system.getValue(); 557 } 558 559 /** 560 * @param value The identification of the system that provides the coded form of 561 * the unit. 562 */ 563 public Quantity setSystem(String value) { 564 if (Utilities.noString(value)) 565 this.system = null; 566 else { 567 if (this.system == null) 568 this.system = new UriType(); 569 this.system.setValue(value); 570 } 571 return this; 572 } 573 574 /** 575 * @return {@link #code} (A computer processable form of the unit in some unit 576 * representation system.). This is the underlying object with id, value 577 * and extensions. The accessor "getCode" gives direct access to the 578 * value 579 */ 580 public CodeType getCodeElement() { 581 if (this.code == null) 582 if (Configuration.errorOnAutoCreate()) 583 throw new Error("Attempt to auto-create Quantity.code"); 584 else if (Configuration.doAutoCreate()) 585 this.code = new CodeType(); // bb 586 return this.code; 587 } 588 589 public boolean hasCodeElement() { 590 return this.code != null && !this.code.isEmpty(); 591 } 592 593 public boolean hasCode() { 594 return this.code != null && !this.code.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #code} (A computer processable form of the unit in some 599 * unit representation system.). This is the underlying object with 600 * id, value and extensions. The accessor "getCode" gives direct 601 * access to the value 602 */ 603 public Quantity setCodeElement(CodeType value) { 604 this.code = value; 605 return this; 606 } 607 608 /** 609 * @return A computer processable form of the unit in some unit representation 610 * system. 611 */ 612 public String getCode() { 613 return this.code == null ? null : this.code.getValue(); 614 } 615 616 /** 617 * @param value A computer processable form of the unit in some unit 618 * representation system. 619 */ 620 public Quantity setCode(String value) { 621 if (Utilities.noString(value)) 622 this.code = null; 623 else { 624 if (this.code == null) 625 this.code = new CodeType(); 626 this.code.setValue(value); 627 } 628 return this; 629 } 630 631 protected void listChildren(List<Property> children) { 632 super.listChildren(children); 633 children.add(new Property("value", "decimal", 634 "The value of the measured amount. The value includes an implicit precision in the presentation of the value.", 635 0, 1, value)); 636 children.add(new Property("comparator", "code", 637 "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.", 638 0, 1, comparator)); 639 children.add(new Property("unit", "string", "A human-readable form of the unit.", 0, 1, unit)); 640 children.add(new Property("system", "uri", 641 "The identification of the system that provides the coded form of the unit.", 0, 1, system)); 642 children.add(new Property("code", "code", 643 "A computer processable form of the unit in some unit representation system.", 0, 1, code)); 644 } 645 646 @Override 647 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 648 switch (_hash) { 649 case 111972721: 650 /* value */ return new Property("value", "decimal", 651 "The value of the measured amount. The value includes an implicit precision in the presentation of the value.", 652 0, 1, value); 653 case -844673834: 654 /* comparator */ return new Property("comparator", "code", 655 "How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value.", 656 0, 1, comparator); 657 case 3594628: 658 /* unit */ return new Property("unit", "string", "A human-readable form of the unit.", 0, 1, unit); 659 case -887328209: 660 /* system */ return new Property("system", "uri", 661 "The identification of the system that provides the coded form of the unit.", 0, 1, system); 662 case 3059181: 663 /* code */ return new Property("code", "code", 664 "A computer processable form of the unit in some unit representation system.", 0, 1, code); 665 default: 666 return super.getNamedProperty(_hash, _name, _checkValid); 667 } 668 669 } 670 671 @Override 672 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 673 switch (hash) { 674 case 111972721: 675 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DecimalType 676 case -844673834: 677 /* comparator */ return this.comparator == null ? new Base[0] : new Base[] { this.comparator }; // Enumeration<QuantityComparator> 678 case 3594628: 679 /* unit */ return this.unit == null ? new Base[0] : new Base[] { this.unit }; // StringType 680 case -887328209: 681 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // UriType 682 case 3059181: 683 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 684 default: 685 return super.getProperty(hash, name, checkValid); 686 } 687 688 } 689 690 @Override 691 public Base setProperty(int hash, String name, Base value) throws FHIRException { 692 switch (hash) { 693 case 111972721: // value 694 this.value = castToDecimal(value); // DecimalType 695 return value; 696 case -844673834: // comparator 697 value = new QuantityComparatorEnumFactory().fromType(castToCode(value)); 698 this.comparator = (Enumeration) value; // Enumeration<QuantityComparator> 699 return value; 700 case 3594628: // unit 701 this.unit = castToString(value); // StringType 702 return value; 703 case -887328209: // system 704 this.system = castToUri(value); // UriType 705 return value; 706 case 3059181: // code 707 this.code = castToCode(value); // CodeType 708 return value; 709 default: 710 return super.setProperty(hash, name, value); 711 } 712 713 } 714 715 @Override 716 public Base setProperty(String name, Base value) throws FHIRException { 717 if (name.equals("value")) { 718 this.value = castToDecimal(value); // DecimalType 719 } else if (name.equals("comparator")) { 720 value = new QuantityComparatorEnumFactory().fromType(castToCode(value)); 721 this.comparator = (Enumeration) value; // Enumeration<QuantityComparator> 722 } else if (name.equals("unit")) { 723 this.unit = castToString(value); // StringType 724 } else if (name.equals("system")) { 725 this.system = castToUri(value); // UriType 726 } else if (name.equals("code")) { 727 this.code = castToCode(value); // CodeType 728 } else 729 return super.setProperty(name, value); 730 return value; 731 } 732 733 @Override 734 public void removeChild(String name, Base value) throws FHIRException { 735 if (name.equals("value")) { 736 this.value = null; 737 } else if (name.equals("comparator")) { 738 this.comparator = null; 739 } else if (name.equals("unit")) { 740 this.unit = null; 741 } else if (name.equals("system")) { 742 this.system = null; 743 } else if (name.equals("code")) { 744 this.code = null; 745 } else 746 super.removeChild(name, value); 747 748 } 749 750 @Override 751 public Base makeProperty(int hash, String name) throws FHIRException { 752 switch (hash) { 753 case 111972721: 754 return getValueElement(); 755 case -844673834: 756 return getComparatorElement(); 757 case 3594628: 758 return getUnitElement(); 759 case -887328209: 760 return getSystemElement(); 761 case 3059181: 762 return getCodeElement(); 763 default: 764 return super.makeProperty(hash, name); 765 } 766 767 } 768 769 @Override 770 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 771 switch (hash) { 772 case 111972721: 773 /* value */ return new String[] { "decimal" }; 774 case -844673834: 775 /* comparator */ return new String[] { "code" }; 776 case 3594628: 777 /* unit */ return new String[] { "string" }; 778 case -887328209: 779 /* system */ return new String[] { "uri" }; 780 case 3059181: 781 /* code */ return new String[] { "code" }; 782 default: 783 return super.getTypesForProperty(hash, name); 784 } 785 786 } 787 788 @Override 789 public Base addChild(String name) throws FHIRException { 790 if (name.equals("value")) { 791 throw new FHIRException("Cannot call addChild on a singleton property Quantity.value"); 792 } else if (name.equals("comparator")) { 793 throw new FHIRException("Cannot call addChild on a singleton property Quantity.comparator"); 794 } else if (name.equals("unit")) { 795 throw new FHIRException("Cannot call addChild on a singleton property Quantity.unit"); 796 } else if (name.equals("system")) { 797 throw new FHIRException("Cannot call addChild on a singleton property Quantity.system"); 798 } else if (name.equals("code")) { 799 throw new FHIRException("Cannot call addChild on a singleton property Quantity.code"); 800 } else 801 return super.addChild(name); 802 } 803 804 public String fhirType() { 805 return "Quantity"; 806 807 } 808 809 public Quantity copy() { 810 Quantity dst = new Quantity(); 811 copyValues(dst); 812 return dst; 813 } 814 815 public void copyValues(Quantity dst) { 816 super.copyValues(dst); 817 dst.value = value == null ? null : value.copy(); 818 dst.comparator = comparator == null ? null : comparator.copy(); 819 dst.unit = unit == null ? null : unit.copy(); 820 dst.system = system == null ? null : system.copy(); 821 dst.code = code == null ? null : code.copy(); 822 } 823 824 protected Quantity typedCopy() { 825 return copy(); 826 } 827 828 @Override 829 public boolean equalsDeep(Base other_) { 830 if (!super.equalsDeep(other_)) 831 return false; 832 if (!(other_ instanceof Quantity)) 833 return false; 834 Quantity o = (Quantity) other_; 835 return compareDeep(value, o.value, true) && compareDeep(comparator, o.comparator, true) 836 && compareDeep(unit, o.unit, true) && compareDeep(system, o.system, true) && compareDeep(code, o.code, true); 837 } 838 839 @Override 840 public boolean equalsShallow(Base other_) { 841 if (!super.equalsShallow(other_)) 842 return false; 843 if (!(other_ instanceof Quantity)) 844 return false; 845 Quantity o = (Quantity) other_; 846 return compareValues(value, o.value, true) && compareValues(comparator, o.comparator, true) 847 && compareValues(unit, o.unit, true) && compareValues(system, o.system, true) 848 && compareValues(code, o.code, true); 849 } 850 851 public boolean isEmpty() { 852 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, comparator, unit, system, code); 853 } 854 855// added from java-adornments.txt: 856 857 @Override 858 public String getVersion() { 859 return null; 860 } 861 862 @Override 863 public boolean hasVersion() { 864 return false; 865 } 866 867 @Override 868 public boolean supportsVersion() { 869 return false; 870 } 871 872 @Override 873 public String getDisplay() { 874 return null; 875 } 876 877 @Override 878 public boolean hasDisplay() { 879 return false; 880 } 881 882 @Override 883 public boolean supportsDisplay() { 884 return false; 885 } 886 887 public static Quantity fromUcum(String v, String code) { 888 Quantity res = new Quantity(); 889 res.setValue(new BigDecimal(v)); 890 res.setSystem("http://unitsofmeasure.org"); 891 res.setCode(code); 892 return res; 893 } 894 895// end addition 896 897}