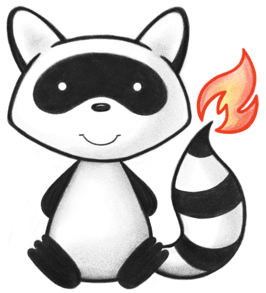
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A structured set of questions intended to guide the collection of answers 052 * from end-users. Questionnaires provide detailed control over order, 053 * presentation, phraseology and grouping to allow coherent, consistent data 054 * collection. 055 */ 056@ResourceDef(name = "Questionnaire", profile = "http://hl7.org/fhir/StructureDefinition/Questionnaire") 057@ChildOrder(names = { "url", "identifier", "version", "name", "title", "derivedFrom", "status", "experimental", 058 "subjectType", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", 059 "approvalDate", "lastReviewDate", "effectivePeriod", "code", "item" }) 060public class Questionnaire extends MetadataResource { 061 062 public enum QuestionnaireItemType { 063 /** 064 * An item with no direct answer but should have at least one child item. 065 */ 066 GROUP, 067 /** 068 * Text for display that will not capture an answer or have child items. 069 */ 070 DISPLAY, 071 /** 072 * An item that defines a specific answer to be captured, and which may have 073 * child items. (the answer provided in the QuestionnaireResponse should be of 074 * the defined datatype). 075 */ 076 QUESTION, 077 /** 078 * Question with a yes/no answer (valueBoolean). 079 */ 080 BOOLEAN, 081 /** 082 * Question with is a real number answer (valueDecimal). 083 */ 084 DECIMAL, 085 /** 086 * Question with an integer answer (valueInteger). 087 */ 088 INTEGER, 089 /** 090 * Question with a date answer (valueDate). 091 */ 092 DATE, 093 /** 094 * Question with a date and time answer (valueDateTime). 095 */ 096 DATETIME, 097 /** 098 * Question with a time (hour:minute:second) answer independent of date. 099 * (valueTime). 100 */ 101 TIME, 102 /** 103 * Question with a short (few words to short sentence) free-text entry answer 104 * (valueString). 105 */ 106 STRING, 107 /** 108 * Question with a long (potentially multi-paragraph) free-text entry answer 109 * (valueString). 110 */ 111 TEXT, 112 /** 113 * Question with a URL (website, FTP site, etc.) answer (valueUri). 114 */ 115 URL, 116 /** 117 * Question with a Coding drawn from a list of possible answers (specified in 118 * either the answerOption property, or via the valueset referenced in the 119 * answerValueSet property) as an answer (valueCoding). 120 */ 121 CHOICE, 122 /** 123 * Answer is a Coding drawn from a list of possible answers (as with the choice 124 * type) or a free-text entry in a string (valueCoding or valueString). 125 */ 126 OPENCHOICE, 127 /** 128 * Question with binary content such as an image, PDF, etc. as an answer 129 * (valueAttachment). 130 */ 131 ATTACHMENT, 132 /** 133 * Question with a reference to another resource (practitioner, organization, 134 * etc.) as an answer (valueReference). 135 */ 136 REFERENCE, 137 /** 138 * Question with a combination of a numeric value and unit, potentially with a 139 * comparator (<, >, etc.) as an answer. (valueQuantity) There is an extension 140 * 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used 141 * to define what unit should be captured (or the unit that has a ucum 142 * conversion from the provided unit). 143 */ 144 QUANTITY, 145 /** 146 * added to help the parsers with the generic types 147 */ 148 NULL; 149 150 public static QuestionnaireItemType fromCode(String codeString) throws FHIRException { 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("group".equals(codeString)) 154 return GROUP; 155 if ("display".equals(codeString)) 156 return DISPLAY; 157 if ("question".equals(codeString)) 158 return QUESTION; 159 if ("boolean".equals(codeString)) 160 return BOOLEAN; 161 if ("decimal".equals(codeString)) 162 return DECIMAL; 163 if ("integer".equals(codeString)) 164 return INTEGER; 165 if ("date".equals(codeString)) 166 return DATE; 167 if ("dateTime".equals(codeString)) 168 return DATETIME; 169 if ("time".equals(codeString)) 170 return TIME; 171 if ("string".equals(codeString)) 172 return STRING; 173 if ("text".equals(codeString)) 174 return TEXT; 175 if ("url".equals(codeString)) 176 return URL; 177 if ("choice".equals(codeString)) 178 return CHOICE; 179 if ("open-choice".equals(codeString)) 180 return OPENCHOICE; 181 if ("attachment".equals(codeString)) 182 return ATTACHMENT; 183 if ("reference".equals(codeString)) 184 return REFERENCE; 185 if ("quantity".equals(codeString)) 186 return QUANTITY; 187 if (Configuration.isAcceptInvalidEnums()) 188 return null; 189 else 190 throw new FHIRException("Unknown QuestionnaireItemType code '" + codeString + "'"); 191 } 192 193 public String toCode() { 194 switch (this) { 195 case GROUP: 196 return "group"; 197 case DISPLAY: 198 return "display"; 199 case QUESTION: 200 return "question"; 201 case BOOLEAN: 202 return "boolean"; 203 case DECIMAL: 204 return "decimal"; 205 case INTEGER: 206 return "integer"; 207 case DATE: 208 return "date"; 209 case DATETIME: 210 return "dateTime"; 211 case TIME: 212 return "time"; 213 case STRING: 214 return "string"; 215 case TEXT: 216 return "text"; 217 case URL: 218 return "url"; 219 case CHOICE: 220 return "choice"; 221 case OPENCHOICE: 222 return "open-choice"; 223 case ATTACHMENT: 224 return "attachment"; 225 case REFERENCE: 226 return "reference"; 227 case QUANTITY: 228 return "quantity"; 229 case NULL: 230 return null; 231 default: 232 return "?"; 233 } 234 } 235 236 public String getSystem() { 237 switch (this) { 238 case GROUP: 239 return "http://hl7.org/fhir/item-type"; 240 case DISPLAY: 241 return "http://hl7.org/fhir/item-type"; 242 case QUESTION: 243 return "http://hl7.org/fhir/item-type"; 244 case BOOLEAN: 245 return "http://hl7.org/fhir/item-type"; 246 case DECIMAL: 247 return "http://hl7.org/fhir/item-type"; 248 case INTEGER: 249 return "http://hl7.org/fhir/item-type"; 250 case DATE: 251 return "http://hl7.org/fhir/item-type"; 252 case DATETIME: 253 return "http://hl7.org/fhir/item-type"; 254 case TIME: 255 return "http://hl7.org/fhir/item-type"; 256 case STRING: 257 return "http://hl7.org/fhir/item-type"; 258 case TEXT: 259 return "http://hl7.org/fhir/item-type"; 260 case URL: 261 return "http://hl7.org/fhir/item-type"; 262 case CHOICE: 263 return "http://hl7.org/fhir/item-type"; 264 case OPENCHOICE: 265 return "http://hl7.org/fhir/item-type"; 266 case ATTACHMENT: 267 return "http://hl7.org/fhir/item-type"; 268 case REFERENCE: 269 return "http://hl7.org/fhir/item-type"; 270 case QUANTITY: 271 return "http://hl7.org/fhir/item-type"; 272 case NULL: 273 return null; 274 default: 275 return "?"; 276 } 277 } 278 279 public String getDefinition() { 280 switch (this) { 281 case GROUP: 282 return "An item with no direct answer but should have at least one child item."; 283 case DISPLAY: 284 return "Text for display that will not capture an answer or have child items."; 285 case QUESTION: 286 return "An item that defines a specific answer to be captured, and which may have child items. (the answer provided in the QuestionnaireResponse should be of the defined datatype)."; 287 case BOOLEAN: 288 return "Question with a yes/no answer (valueBoolean)."; 289 case DECIMAL: 290 return "Question with is a real number answer (valueDecimal)."; 291 case INTEGER: 292 return "Question with an integer answer (valueInteger)."; 293 case DATE: 294 return "Question with a date answer (valueDate)."; 295 case DATETIME: 296 return "Question with a date and time answer (valueDateTime)."; 297 case TIME: 298 return "Question with a time (hour:minute:second) answer independent of date. (valueTime)."; 299 case STRING: 300 return "Question with a short (few words to short sentence) free-text entry answer (valueString)."; 301 case TEXT: 302 return "Question with a long (potentially multi-paragraph) free-text entry answer (valueString)."; 303 case URL: 304 return "Question with a URL (website, FTP site, etc.) answer (valueUri)."; 305 case CHOICE: 306 return "Question with a Coding drawn from a list of possible answers (specified in either the answerOption property, or via the valueset referenced in the answerValueSet property) as an answer (valueCoding)."; 307 case OPENCHOICE: 308 return "Answer is a Coding drawn from a list of possible answers (as with the choice type) or a free-text entry in a string (valueCoding or valueString)."; 309 case ATTACHMENT: 310 return "Question with binary content such as an image, PDF, etc. as an answer (valueAttachment)."; 311 case REFERENCE: 312 return "Question with a reference to another resource (practitioner, organization, etc.) as an answer (valueReference)."; 313 case QUANTITY: 314 return "Question with a combination of a numeric value and unit, potentially with a comparator (<, >, etc.) as an answer. (valueQuantity) There is an extension 'http://hl7.org/fhir/StructureDefinition/questionnaire-unit' that can be used to define what unit should be captured (or the unit that has a ucum conversion from the provided unit)."; 315 case NULL: 316 return null; 317 default: 318 return "?"; 319 } 320 } 321 322 public String getDisplay() { 323 switch (this) { 324 case GROUP: 325 return "Group"; 326 case DISPLAY: 327 return "Display"; 328 case QUESTION: 329 return "Question"; 330 case BOOLEAN: 331 return "Boolean"; 332 case DECIMAL: 333 return "Decimal"; 334 case INTEGER: 335 return "Integer"; 336 case DATE: 337 return "Date"; 338 case DATETIME: 339 return "Date Time"; 340 case TIME: 341 return "Time"; 342 case STRING: 343 return "String"; 344 case TEXT: 345 return "Text"; 346 case URL: 347 return "Url"; 348 case CHOICE: 349 return "Choice"; 350 case OPENCHOICE: 351 return "Open Choice"; 352 case ATTACHMENT: 353 return "Attachment"; 354 case REFERENCE: 355 return "Reference"; 356 case QUANTITY: 357 return "Quantity"; 358 case NULL: 359 return null; 360 default: 361 return "?"; 362 } 363 } 364 } 365 366 public static class QuestionnaireItemTypeEnumFactory implements EnumFactory<QuestionnaireItemType> { 367 public QuestionnaireItemType fromCode(String codeString) throws IllegalArgumentException { 368 if (codeString == null || "".equals(codeString)) 369 if (codeString == null || "".equals(codeString)) 370 return null; 371 if ("group".equals(codeString)) 372 return QuestionnaireItemType.GROUP; 373 if ("display".equals(codeString)) 374 return QuestionnaireItemType.DISPLAY; 375 if ("question".equals(codeString)) 376 return QuestionnaireItemType.QUESTION; 377 if ("boolean".equals(codeString)) 378 return QuestionnaireItemType.BOOLEAN; 379 if ("decimal".equals(codeString)) 380 return QuestionnaireItemType.DECIMAL; 381 if ("integer".equals(codeString)) 382 return QuestionnaireItemType.INTEGER; 383 if ("date".equals(codeString)) 384 return QuestionnaireItemType.DATE; 385 if ("dateTime".equals(codeString)) 386 return QuestionnaireItemType.DATETIME; 387 if ("time".equals(codeString)) 388 return QuestionnaireItemType.TIME; 389 if ("string".equals(codeString)) 390 return QuestionnaireItemType.STRING; 391 if ("text".equals(codeString)) 392 return QuestionnaireItemType.TEXT; 393 if ("url".equals(codeString)) 394 return QuestionnaireItemType.URL; 395 if ("choice".equals(codeString)) 396 return QuestionnaireItemType.CHOICE; 397 if ("open-choice".equals(codeString)) 398 return QuestionnaireItemType.OPENCHOICE; 399 if ("attachment".equals(codeString)) 400 return QuestionnaireItemType.ATTACHMENT; 401 if ("reference".equals(codeString)) 402 return QuestionnaireItemType.REFERENCE; 403 if ("quantity".equals(codeString)) 404 return QuestionnaireItemType.QUANTITY; 405 throw new IllegalArgumentException("Unknown QuestionnaireItemType code '" + codeString + "'"); 406 } 407 408 public Enumeration<QuestionnaireItemType> fromType(PrimitiveType<?> code) throws FHIRException { 409 if (code == null) 410 return null; 411 if (code.isEmpty()) 412 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.NULL, code); 413 String codeString = code.asStringValue(); 414 if (codeString == null || "".equals(codeString)) 415 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.NULL, code); 416 if ("group".equals(codeString)) 417 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.GROUP, code); 418 if ("display".equals(codeString)) 419 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DISPLAY, code); 420 if ("question".equals(codeString)) 421 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.QUESTION, code); 422 if ("boolean".equals(codeString)) 423 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.BOOLEAN, code); 424 if ("decimal".equals(codeString)) 425 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DECIMAL, code); 426 if ("integer".equals(codeString)) 427 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.INTEGER, code); 428 if ("date".equals(codeString)) 429 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DATE, code); 430 if ("dateTime".equals(codeString)) 431 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.DATETIME, code); 432 if ("time".equals(codeString)) 433 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.TIME, code); 434 if ("string".equals(codeString)) 435 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.STRING, code); 436 if ("text".equals(codeString)) 437 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.TEXT, code); 438 if ("url".equals(codeString)) 439 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.URL, code); 440 if ("choice".equals(codeString)) 441 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.CHOICE, code); 442 if ("open-choice".equals(codeString)) 443 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.OPENCHOICE, code); 444 if ("attachment".equals(codeString)) 445 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.ATTACHMENT, code); 446 if ("reference".equals(codeString)) 447 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.REFERENCE, code); 448 if ("quantity".equals(codeString)) 449 return new Enumeration<QuestionnaireItemType>(this, QuestionnaireItemType.QUANTITY, code); 450 throw new FHIRException("Unknown QuestionnaireItemType code '" + codeString + "'"); 451 } 452 453 public String toCode(QuestionnaireItemType code) { 454 if (code == QuestionnaireItemType.NULL) 455 return null; 456 if (code == QuestionnaireItemType.GROUP) 457 return "group"; 458 if (code == QuestionnaireItemType.DISPLAY) 459 return "display"; 460 if (code == QuestionnaireItemType.QUESTION) 461 return "question"; 462 if (code == QuestionnaireItemType.BOOLEAN) 463 return "boolean"; 464 if (code == QuestionnaireItemType.DECIMAL) 465 return "decimal"; 466 if (code == QuestionnaireItemType.INTEGER) 467 return "integer"; 468 if (code == QuestionnaireItemType.DATE) 469 return "date"; 470 if (code == QuestionnaireItemType.DATETIME) 471 return "dateTime"; 472 if (code == QuestionnaireItemType.TIME) 473 return "time"; 474 if (code == QuestionnaireItemType.STRING) 475 return "string"; 476 if (code == QuestionnaireItemType.TEXT) 477 return "text"; 478 if (code == QuestionnaireItemType.URL) 479 return "url"; 480 if (code == QuestionnaireItemType.CHOICE) 481 return "choice"; 482 if (code == QuestionnaireItemType.OPENCHOICE) 483 return "open-choice"; 484 if (code == QuestionnaireItemType.ATTACHMENT) 485 return "attachment"; 486 if (code == QuestionnaireItemType.REFERENCE) 487 return "reference"; 488 if (code == QuestionnaireItemType.QUANTITY) 489 return "quantity"; 490 return "?"; 491 } 492 493 public String toSystem(QuestionnaireItemType code) { 494 return code.getSystem(); 495 } 496 } 497 498 public enum QuestionnaireItemOperator { 499 /** 500 * True if whether an answer exists is equal to the enableWhen answer (which 501 * must be a boolean). 502 */ 503 EXISTS, 504 /** 505 * True if whether at least one answer has a value that is equal to the 506 * enableWhen answer. 507 */ 508 EQUAL, 509 /** 510 * True if whether at least no answer has a value that is equal to the 511 * enableWhen answer. 512 */ 513 NOT_EQUAL, 514 /** 515 * True if whether at least no answer has a value that is greater than the 516 * enableWhen answer. 517 */ 518 GREATER_THAN, 519 /** 520 * True if whether at least no answer has a value that is less than the 521 * enableWhen answer. 522 */ 523 LESS_THAN, 524 /** 525 * True if whether at least no answer has a value that is greater or equal to 526 * the enableWhen answer. 527 */ 528 GREATER_OR_EQUAL, 529 /** 530 * True if whether at least no answer has a value that is less or equal to the 531 * enableWhen answer. 532 */ 533 LESS_OR_EQUAL, 534 /** 535 * added to help the parsers with the generic types 536 */ 537 NULL; 538 539 public static QuestionnaireItemOperator fromCode(String codeString) throws FHIRException { 540 if (codeString == null || "".equals(codeString)) 541 return null; 542 if ("exists".equals(codeString)) 543 return EXISTS; 544 if ("=".equals(codeString)) 545 return EQUAL; 546 if ("!=".equals(codeString)) 547 return NOT_EQUAL; 548 if (">".equals(codeString)) 549 return GREATER_THAN; 550 if ("<".equals(codeString)) 551 return LESS_THAN; 552 if (">=".equals(codeString)) 553 return GREATER_OR_EQUAL; 554 if ("<=".equals(codeString)) 555 return LESS_OR_EQUAL; 556 if (Configuration.isAcceptInvalidEnums()) 557 return null; 558 else 559 throw new FHIRException("Unknown QuestionnaireItemOperator code '" + codeString + "'"); 560 } 561 562 public String toCode() { 563 switch (this) { 564 case EXISTS: 565 return "exists"; 566 case EQUAL: 567 return "="; 568 case NOT_EQUAL: 569 return "!="; 570 case GREATER_THAN: 571 return ">"; 572 case LESS_THAN: 573 return "<"; 574 case GREATER_OR_EQUAL: 575 return ">="; 576 case LESS_OR_EQUAL: 577 return "<="; 578 case NULL: 579 return null; 580 default: 581 return "?"; 582 } 583 } 584 585 public String getSystem() { 586 switch (this) { 587 case EXISTS: 588 return "http://hl7.org/fhir/questionnaire-enable-operator"; 589 case EQUAL: 590 return "http://hl7.org/fhir/questionnaire-enable-operator"; 591 case NOT_EQUAL: 592 return "http://hl7.org/fhir/questionnaire-enable-operator"; 593 case GREATER_THAN: 594 return "http://hl7.org/fhir/questionnaire-enable-operator"; 595 case LESS_THAN: 596 return "http://hl7.org/fhir/questionnaire-enable-operator"; 597 case GREATER_OR_EQUAL: 598 return "http://hl7.org/fhir/questionnaire-enable-operator"; 599 case LESS_OR_EQUAL: 600 return "http://hl7.org/fhir/questionnaire-enable-operator"; 601 case NULL: 602 return null; 603 default: 604 return "?"; 605 } 606 } 607 608 public String getDefinition() { 609 switch (this) { 610 case EXISTS: 611 return "True if whether an answer exists is equal to the enableWhen answer (which must be a boolean)."; 612 case EQUAL: 613 return "True if whether at least one answer has a value that is equal to the enableWhen answer."; 614 case NOT_EQUAL: 615 return "True if whether at least no answer has a value that is equal to the enableWhen answer."; 616 case GREATER_THAN: 617 return "True if whether at least no answer has a value that is greater than the enableWhen answer."; 618 case LESS_THAN: 619 return "True if whether at least no answer has a value that is less than the enableWhen answer."; 620 case GREATER_OR_EQUAL: 621 return "True if whether at least no answer has a value that is greater or equal to the enableWhen answer."; 622 case LESS_OR_EQUAL: 623 return "True if whether at least no answer has a value that is less or equal to the enableWhen answer."; 624 case NULL: 625 return null; 626 default: 627 return "?"; 628 } 629 } 630 631 public String getDisplay() { 632 switch (this) { 633 case EXISTS: 634 return "Exists"; 635 case EQUAL: 636 return "Equals"; 637 case NOT_EQUAL: 638 return "Not Equals"; 639 case GREATER_THAN: 640 return "Greater Than"; 641 case LESS_THAN: 642 return "Less Than"; 643 case GREATER_OR_EQUAL: 644 return "Greater or Equals"; 645 case LESS_OR_EQUAL: 646 return "Less or Equals"; 647 case NULL: 648 return null; 649 default: 650 return "?"; 651 } 652 } 653 } 654 655 public static class QuestionnaireItemOperatorEnumFactory implements EnumFactory<QuestionnaireItemOperator> { 656 public QuestionnaireItemOperator fromCode(String codeString) throws IllegalArgumentException { 657 if (codeString == null || "".equals(codeString)) 658 if (codeString == null || "".equals(codeString)) 659 return null; 660 if ("exists".equals(codeString)) 661 return QuestionnaireItemOperator.EXISTS; 662 if ("=".equals(codeString)) 663 return QuestionnaireItemOperator.EQUAL; 664 if ("!=".equals(codeString)) 665 return QuestionnaireItemOperator.NOT_EQUAL; 666 if (">".equals(codeString)) 667 return QuestionnaireItemOperator.GREATER_THAN; 668 if ("<".equals(codeString)) 669 return QuestionnaireItemOperator.LESS_THAN; 670 if (">=".equals(codeString)) 671 return QuestionnaireItemOperator.GREATER_OR_EQUAL; 672 if ("<=".equals(codeString)) 673 return QuestionnaireItemOperator.LESS_OR_EQUAL; 674 throw new IllegalArgumentException("Unknown QuestionnaireItemOperator code '" + codeString + "'"); 675 } 676 677 public Enumeration<QuestionnaireItemOperator> fromType(PrimitiveType<?> code) throws FHIRException { 678 if (code == null) 679 return null; 680 if (code.isEmpty()) 681 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.NULL, code); 682 String codeString = code.asStringValue(); 683 if (codeString == null || "".equals(codeString)) 684 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.NULL, code); 685 if ("exists".equals(codeString)) 686 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.EXISTS, code); 687 if ("=".equals(codeString)) 688 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.EQUAL, code); 689 if ("!=".equals(codeString)) 690 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.NOT_EQUAL, code); 691 if (">".equals(codeString)) 692 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.GREATER_THAN, code); 693 if ("<".equals(codeString)) 694 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.LESS_THAN, code); 695 if (">=".equals(codeString)) 696 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.GREATER_OR_EQUAL, code); 697 if ("<=".equals(codeString)) 698 return new Enumeration<QuestionnaireItemOperator>(this, QuestionnaireItemOperator.LESS_OR_EQUAL, code); 699 throw new FHIRException("Unknown QuestionnaireItemOperator code '" + codeString + "'"); 700 } 701 702 public String toCode(QuestionnaireItemOperator code) { 703 if (code == QuestionnaireItemOperator.NULL) 704 return null; 705 if (code == QuestionnaireItemOperator.EXISTS) 706 return "exists"; 707 if (code == QuestionnaireItemOperator.EQUAL) 708 return "="; 709 if (code == QuestionnaireItemOperator.NOT_EQUAL) 710 return "!="; 711 if (code == QuestionnaireItemOperator.GREATER_THAN) 712 return ">"; 713 if (code == QuestionnaireItemOperator.LESS_THAN) 714 return "<"; 715 if (code == QuestionnaireItemOperator.GREATER_OR_EQUAL) 716 return ">="; 717 if (code == QuestionnaireItemOperator.LESS_OR_EQUAL) 718 return "<="; 719 return "?"; 720 } 721 722 public String toSystem(QuestionnaireItemOperator code) { 723 return code.getSystem(); 724 } 725 } 726 727 public enum EnableWhenBehavior { 728 /** 729 * Enable the question when all the enableWhen criteria are satisfied. 730 */ 731 ALL, 732 /** 733 * Enable the question when any of the enableWhen criteria are satisfied. 734 */ 735 ANY, 736 /** 737 * added to help the parsers with the generic types 738 */ 739 NULL; 740 741 public static EnableWhenBehavior fromCode(String codeString) throws FHIRException { 742 if (codeString == null || "".equals(codeString)) 743 return null; 744 if ("all".equals(codeString)) 745 return ALL; 746 if ("any".equals(codeString)) 747 return ANY; 748 if (Configuration.isAcceptInvalidEnums()) 749 return null; 750 else 751 throw new FHIRException("Unknown EnableWhenBehavior code '" + codeString + "'"); 752 } 753 754 public String toCode() { 755 switch (this) { 756 case ALL: 757 return "all"; 758 case ANY: 759 return "any"; 760 case NULL: 761 return null; 762 default: 763 return "?"; 764 } 765 } 766 767 public String getSystem() { 768 switch (this) { 769 case ALL: 770 return "http://hl7.org/fhir/questionnaire-enable-behavior"; 771 case ANY: 772 return "http://hl7.org/fhir/questionnaire-enable-behavior"; 773 case NULL: 774 return null; 775 default: 776 return "?"; 777 } 778 } 779 780 public String getDefinition() { 781 switch (this) { 782 case ALL: 783 return "Enable the question when all the enableWhen criteria are satisfied."; 784 case ANY: 785 return "Enable the question when any of the enableWhen criteria are satisfied."; 786 case NULL: 787 return null; 788 default: 789 return "?"; 790 } 791 } 792 793 public String getDisplay() { 794 switch (this) { 795 case ALL: 796 return "All"; 797 case ANY: 798 return "Any"; 799 case NULL: 800 return null; 801 default: 802 return "?"; 803 } 804 } 805 } 806 807 public static class EnableWhenBehaviorEnumFactory implements EnumFactory<EnableWhenBehavior> { 808 public EnableWhenBehavior fromCode(String codeString) throws IllegalArgumentException { 809 if (codeString == null || "".equals(codeString)) 810 if (codeString == null || "".equals(codeString)) 811 return null; 812 if ("all".equals(codeString)) 813 return EnableWhenBehavior.ALL; 814 if ("any".equals(codeString)) 815 return EnableWhenBehavior.ANY; 816 throw new IllegalArgumentException("Unknown EnableWhenBehavior code '" + codeString + "'"); 817 } 818 819 public Enumeration<EnableWhenBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 820 if (code == null) 821 return null; 822 if (code.isEmpty()) 823 return new Enumeration<EnableWhenBehavior>(this, EnableWhenBehavior.NULL, code); 824 String codeString = code.asStringValue(); 825 if (codeString == null || "".equals(codeString)) 826 return new Enumeration<EnableWhenBehavior>(this, EnableWhenBehavior.NULL, code); 827 if ("all".equals(codeString)) 828 return new Enumeration<EnableWhenBehavior>(this, EnableWhenBehavior.ALL, code); 829 if ("any".equals(codeString)) 830 return new Enumeration<EnableWhenBehavior>(this, EnableWhenBehavior.ANY, code); 831 throw new FHIRException("Unknown EnableWhenBehavior code '" + codeString + "'"); 832 } 833 834 public String toCode(EnableWhenBehavior code) { 835 if (code == EnableWhenBehavior.NULL) 836 return null; 837 if (code == EnableWhenBehavior.ALL) 838 return "all"; 839 if (code == EnableWhenBehavior.ANY) 840 return "any"; 841 return "?"; 842 } 843 844 public String toSystem(EnableWhenBehavior code) { 845 return code.getSystem(); 846 } 847 } 848 849 @Block() 850 public static class QuestionnaireItemComponent extends BackboneElement implements IBaseBackboneElement { 851 /** 852 * An identifier that is unique within the Questionnaire allowing linkage to the 853 * equivalent item in a QuestionnaireResponse resource. 854 */ 855 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 856 @Description(shortDefinition = "Unique id for item in questionnaire", formalDefinition = "An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.") 857 protected StringType linkId; 858 859 /** 860 * This element is a URI that refers to an [[[ElementDefinition]]] that provides 861 * information about this item, including information that might otherwise be 862 * included in the instance of the Questionnaire resource. A detailed 863 * description of the construction of the URI is shown in Comments, below. If 864 * this element is present then the following element values MAY be derived from 865 * the Element Definition if the corresponding elements of this Questionnaire 866 * resource instance have no value: 867 * 868 * code (ElementDefinition.code) type (ElementDefinition.type) required 869 * (ElementDefinition.min) repeats (ElementDefinition.max) maxLength 870 * (ElementDefinition.maxLength) answerValueSet (ElementDefinition.binding) 871 * options (ElementDefinition.binding). 872 */ 873 @Child(name = "definition", type = { 874 UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 875 @Description(shortDefinition = "ElementDefinition - details for the item", formalDefinition = "This element is a URI that refers to an [[[ElementDefinition]]] that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in Comments, below. If this element is present then the following element values MAY be derived from the Element Definition if the corresponding elements of this Questionnaire resource instance have no value:\n\n* code (ElementDefinition.code) \n* type (ElementDefinition.type) \n* required (ElementDefinition.min) \n* repeats (ElementDefinition.max) \n* maxLength (ElementDefinition.maxLength) \n* answerValueSet (ElementDefinition.binding)\n* options (ElementDefinition.binding).") 876 protected UriType definition; 877 878 /** 879 * A terminology code that corresponds to this group or question (e.g. a code 880 * from LOINC, which defines many questions and answers). 881 */ 882 @Child(name = "code", type = { 883 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 884 @Description(shortDefinition = "Corresponding concept for this item in a terminology", formalDefinition = "A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).") 885 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-questions") 886 protected List<Coding> code; 887 888 /** 889 * A short label for a particular group, question or set of display text within 890 * the questionnaire used for reference by the individual completing the 891 * questionnaire. 892 */ 893 @Child(name = "prefix", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 894 @Description(shortDefinition = "E.g. \"1(a)\", \"2.5.3\"", formalDefinition = "A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.") 895 protected StringType prefix; 896 897 /** 898 * The name of a section, the text of a question or text content for a display 899 * item. 900 */ 901 @Child(name = "text", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 902 @Description(shortDefinition = "Primary text for the item", formalDefinition = "The name of a section, the text of a question or text content for a display item.") 903 protected StringType text; 904 905 /** 906 * The type of questionnaire item this is - whether text for display, a grouping 907 * of other items or a particular type of data to be captured (string, integer, 908 * coded choice, etc.). 909 */ 910 @Child(name = "type", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = false) 911 @Description(shortDefinition = "group | display | boolean | decimal | integer | date | dateTime +", formalDefinition = "The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.).") 912 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/item-type") 913 protected Enumeration<QuestionnaireItemType> type; 914 915 /** 916 * A constraint indicating that this item should only be enabled 917 * (displayed/allow answers to be captured) when the specified condition is 918 * true. 919 */ 920 @Child(name = "enableWhen", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = false) 921 @Description(shortDefinition = "Only allow data when", formalDefinition = "A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.") 922 protected List<QuestionnaireItemEnableWhenComponent> enableWhen; 923 924 /** 925 * Controls how multiple enableWhen values are interpreted - whether all or any 926 * must be true. 927 */ 928 @Child(name = "enableBehavior", type = { 929 CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 930 @Description(shortDefinition = "all | any", formalDefinition = "Controls how multiple enableWhen values are interpreted - whether all or any must be true.") 931 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-enable-behavior") 932 protected Enumeration<EnableWhenBehavior> enableBehavior; 933 934 /** 935 * An indication, if true, that the item must be present in a "completed" 936 * QuestionnaireResponse. If false, the item may be skipped when answering the 937 * questionnaire. 938 */ 939 @Child(name = "required", type = { 940 BooleanType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 941 @Description(shortDefinition = "Whether the item must be included in data results", formalDefinition = "An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.") 942 protected BooleanType required; 943 944 /** 945 * An indication, if true, that the item may occur multiple times in the 946 * response, collecting multiple answers for questions or multiple sets of 947 * answers for groups. 948 */ 949 @Child(name = "repeats", type = { 950 BooleanType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 951 @Description(shortDefinition = "Whether the item may repeat", formalDefinition = "An indication, if true, that the item may occur multiple times in the response, collecting multiple answers for questions or multiple sets of answers for groups.") 952 protected BooleanType repeats; 953 954 /** 955 * An indication, when true, that the value cannot be changed by a human 956 * respondent to the Questionnaire. 957 */ 958 @Child(name = "readOnly", type = { 959 BooleanType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 960 @Description(shortDefinition = "Don't allow human editing", formalDefinition = "An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.") 961 protected BooleanType readOnly; 962 963 /** 964 * The maximum number of characters that are permitted in the answer to be 965 * considered a "valid" QuestionnaireResponse. 966 */ 967 @Child(name = "maxLength", type = { 968 IntegerType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 969 @Description(shortDefinition = "No more than this many characters", formalDefinition = "The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse.") 970 protected IntegerType maxLength; 971 972 /** 973 * A reference to a value set containing a list of codes representing permitted 974 * answers for a "choice" or "open-choice" question. 975 */ 976 @Child(name = "answerValueSet", type = { 977 CanonicalType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 978 @Description(shortDefinition = "Valueset containing permitted answers", formalDefinition = "A reference to a value set containing a list of codes representing permitted answers for a \"choice\" or \"open-choice\" question.") 979 protected CanonicalType answerValueSet; 980 981 /** 982 * One of the permitted answers for a "choice" or "open-choice" question. 983 */ 984 @Child(name = "answerOption", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 985 @Description(shortDefinition = "Permitted answer", formalDefinition = "One of the permitted answers for a \"choice\" or \"open-choice\" question.") 986 protected List<QuestionnaireItemAnswerOptionComponent> answerOption; 987 988 /** 989 * One or more values that should be pre-populated in the answer when initially 990 * rendering the questionnaire for user input. 991 */ 992 @Child(name = "initial", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 993 @Description(shortDefinition = "Initial value(s) when item is first rendered", formalDefinition = "One or more values that should be pre-populated in the answer when initially rendering the questionnaire for user input.") 994 protected List<QuestionnaireItemInitialComponent> initial; 995 996 /** 997 * Text, questions and other groups to be nested beneath a question or group. 998 */ 999 @Child(name = "item", type = { 1000 QuestionnaireItemComponent.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1001 @Description(shortDefinition = "Nested questionnaire items", formalDefinition = "Text, questions and other groups to be nested beneath a question or group.") 1002 protected List<QuestionnaireItemComponent> item; 1003 1004 private static final long serialVersionUID = -1503380450L; 1005 1006 /** 1007 * Constructor 1008 */ 1009 public QuestionnaireItemComponent() { 1010 super(); 1011 } 1012 1013 /** 1014 * Constructor 1015 */ 1016 public QuestionnaireItemComponent(StringType linkId, Enumeration<QuestionnaireItemType> type) { 1017 super(); 1018 this.linkId = linkId; 1019 this.type = type; 1020 } 1021 1022 /** 1023 * @return {@link #linkId} (An identifier that is unique within the 1024 * Questionnaire allowing linkage to the equivalent item in a 1025 * QuestionnaireResponse resource.). This is the underlying object with 1026 * id, value and extensions. The accessor "getLinkId" gives direct 1027 * access to the value 1028 */ 1029 public StringType getLinkIdElement() { 1030 if (this.linkId == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create QuestionnaireItemComponent.linkId"); 1033 else if (Configuration.doAutoCreate()) 1034 this.linkId = new StringType(); // bb 1035 return this.linkId; 1036 } 1037 1038 public boolean hasLinkIdElement() { 1039 return this.linkId != null && !this.linkId.isEmpty(); 1040 } 1041 1042 public boolean hasLinkId() { 1043 return this.linkId != null && !this.linkId.isEmpty(); 1044 } 1045 1046 /** 1047 * @param value {@link #linkId} (An identifier that is unique within the 1048 * Questionnaire allowing linkage to the equivalent item in a 1049 * QuestionnaireResponse resource.). This is the underlying object 1050 * with id, value and extensions. The accessor "getLinkId" gives 1051 * direct access to the value 1052 */ 1053 public QuestionnaireItemComponent setLinkIdElement(StringType value) { 1054 this.linkId = value; 1055 return this; 1056 } 1057 1058 /** 1059 * @return An identifier that is unique within the Questionnaire allowing 1060 * linkage to the equivalent item in a QuestionnaireResponse resource. 1061 */ 1062 public String getLinkId() { 1063 return this.linkId == null ? null : this.linkId.getValue(); 1064 } 1065 1066 /** 1067 * @param value An identifier that is unique within the Questionnaire allowing 1068 * linkage to the equivalent item in a QuestionnaireResponse 1069 * resource. 1070 */ 1071 public QuestionnaireItemComponent setLinkId(String value) { 1072 if (this.linkId == null) 1073 this.linkId = new StringType(); 1074 this.linkId.setValue(value); 1075 return this; 1076 } 1077 1078 /** 1079 * @return {@link #definition} (This element is a URI that refers to an 1080 * [[[ElementDefinition]]] that provides information about this item, 1081 * including information that might otherwise be included in the 1082 * instance of the Questionnaire resource. A detailed description of the 1083 * construction of the URI is shown in Comments, below. If this element 1084 * is present then the following element values MAY be derived from the 1085 * Element Definition if the corresponding elements of this 1086 * Questionnaire resource instance have no value: 1087 * 1088 * code (ElementDefinition.code) type (ElementDefinition.type) required 1089 * (ElementDefinition.min) repeats (ElementDefinition.max) maxLength 1090 * (ElementDefinition.maxLength) answerValueSet 1091 * (ElementDefinition.binding) options (ElementDefinition.binding).). 1092 * This is the underlying object with id, value and extensions. The 1093 * accessor "getDefinition" gives direct access to the value 1094 */ 1095 public UriType getDefinitionElement() { 1096 if (this.definition == null) 1097 if (Configuration.errorOnAutoCreate()) 1098 throw new Error("Attempt to auto-create QuestionnaireItemComponent.definition"); 1099 else if (Configuration.doAutoCreate()) 1100 this.definition = new UriType(); // bb 1101 return this.definition; 1102 } 1103 1104 public boolean hasDefinitionElement() { 1105 return this.definition != null && !this.definition.isEmpty(); 1106 } 1107 1108 public boolean hasDefinition() { 1109 return this.definition != null && !this.definition.isEmpty(); 1110 } 1111 1112 /** 1113 * @param value {@link #definition} (This element is a URI that refers to an 1114 * [[[ElementDefinition]]] that provides information about this 1115 * item, including information that might otherwise be included in 1116 * the instance of the Questionnaire resource. A detailed 1117 * description of the construction of the URI is shown in Comments, 1118 * below. If this element is present then the following element 1119 * values MAY be derived from the Element Definition if the 1120 * corresponding elements of this Questionnaire resource instance 1121 * have no value: 1122 * 1123 * code (ElementDefinition.code) type (ElementDefinition.type) 1124 * required (ElementDefinition.min) repeats (ElementDefinition.max) 1125 * maxLength (ElementDefinition.maxLength) answerValueSet 1126 * (ElementDefinition.binding) options 1127 * (ElementDefinition.binding).). This is the underlying object 1128 * with id, value and extensions. The accessor "getDefinition" 1129 * gives direct access to the value 1130 */ 1131 public QuestionnaireItemComponent setDefinitionElement(UriType value) { 1132 this.definition = value; 1133 return this; 1134 } 1135 1136 /** 1137 * @return This element is a URI that refers to an [[[ElementDefinition]]] that 1138 * provides information about this item, including information that 1139 * might otherwise be included in the instance of the Questionnaire 1140 * resource. A detailed description of the construction of the URI is 1141 * shown in Comments, below. If this element is present then the 1142 * following element values MAY be derived from the Element Definition 1143 * if the corresponding elements of this Questionnaire resource instance 1144 * have no value: 1145 * 1146 * code (ElementDefinition.code) type (ElementDefinition.type) required 1147 * (ElementDefinition.min) repeats (ElementDefinition.max) maxLength 1148 * (ElementDefinition.maxLength) answerValueSet 1149 * (ElementDefinition.binding) options (ElementDefinition.binding). 1150 */ 1151 public String getDefinition() { 1152 return this.definition == null ? null : this.definition.getValue(); 1153 } 1154 1155 /** 1156 * @param value This element is a URI that refers to an [[[ElementDefinition]]] 1157 * that provides information about this item, including information 1158 * that might otherwise be included in the instance of the 1159 * Questionnaire resource. A detailed description of the 1160 * construction of the URI is shown in Comments, below. If this 1161 * element is present then the following element values MAY be 1162 * derived from the Element Definition if the corresponding 1163 * elements of this Questionnaire resource instance have no value: 1164 * 1165 * code (ElementDefinition.code) type (ElementDefinition.type) 1166 * required (ElementDefinition.min) repeats (ElementDefinition.max) 1167 * maxLength (ElementDefinition.maxLength) answerValueSet 1168 * (ElementDefinition.binding) options (ElementDefinition.binding). 1169 */ 1170 public QuestionnaireItemComponent setDefinition(String value) { 1171 if (Utilities.noString(value)) 1172 this.definition = null; 1173 else { 1174 if (this.definition == null) 1175 this.definition = new UriType(); 1176 this.definition.setValue(value); 1177 } 1178 return this; 1179 } 1180 1181 /** 1182 * @return {@link #code} (A terminology code that corresponds to this group or 1183 * question (e.g. a code from LOINC, which defines many questions and 1184 * answers).) 1185 */ 1186 public List<Coding> getCode() { 1187 if (this.code == null) 1188 this.code = new ArrayList<Coding>(); 1189 return this.code; 1190 } 1191 1192 /** 1193 * @return Returns a reference to <code>this</code> for easy method chaining 1194 */ 1195 public QuestionnaireItemComponent setCode(List<Coding> theCode) { 1196 this.code = theCode; 1197 return this; 1198 } 1199 1200 public boolean hasCode() { 1201 if (this.code == null) 1202 return false; 1203 for (Coding item : this.code) 1204 if (!item.isEmpty()) 1205 return true; 1206 return false; 1207 } 1208 1209 public Coding addCode() { // 3 1210 Coding t = new Coding(); 1211 if (this.code == null) 1212 this.code = new ArrayList<Coding>(); 1213 this.code.add(t); 1214 return t; 1215 } 1216 1217 public QuestionnaireItemComponent addCode(Coding t) { // 3 1218 if (t == null) 1219 return this; 1220 if (this.code == null) 1221 this.code = new ArrayList<Coding>(); 1222 this.code.add(t); 1223 return this; 1224 } 1225 1226 /** 1227 * @return The first repetition of repeating field {@link #code}, creating it if 1228 * it does not already exist 1229 */ 1230 public Coding getCodeFirstRep() { 1231 if (getCode().isEmpty()) { 1232 addCode(); 1233 } 1234 return getCode().get(0); 1235 } 1236 1237 /** 1238 * @return {@link #prefix} (A short label for a particular group, question or 1239 * set of display text within the questionnaire used for reference by 1240 * the individual completing the questionnaire.). This is the underlying 1241 * object with id, value and extensions. The accessor "getPrefix" gives 1242 * direct access to the value 1243 */ 1244 public StringType getPrefixElement() { 1245 if (this.prefix == null) 1246 if (Configuration.errorOnAutoCreate()) 1247 throw new Error("Attempt to auto-create QuestionnaireItemComponent.prefix"); 1248 else if (Configuration.doAutoCreate()) 1249 this.prefix = new StringType(); // bb 1250 return this.prefix; 1251 } 1252 1253 public boolean hasPrefixElement() { 1254 return this.prefix != null && !this.prefix.isEmpty(); 1255 } 1256 1257 public boolean hasPrefix() { 1258 return this.prefix != null && !this.prefix.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #prefix} (A short label for a particular group, question 1263 * or set of display text within the questionnaire used for 1264 * reference by the individual completing the questionnaire.). This 1265 * is the underlying object with id, value and extensions. The 1266 * accessor "getPrefix" gives direct access to the value 1267 */ 1268 public QuestionnaireItemComponent setPrefixElement(StringType value) { 1269 this.prefix = value; 1270 return this; 1271 } 1272 1273 /** 1274 * @return A short label for a particular group, question or set of display text 1275 * within the questionnaire used for reference by the individual 1276 * completing the questionnaire. 1277 */ 1278 public String getPrefix() { 1279 return this.prefix == null ? null : this.prefix.getValue(); 1280 } 1281 1282 /** 1283 * @param value A short label for a particular group, question or set of display 1284 * text within the questionnaire used for reference by the 1285 * individual completing the questionnaire. 1286 */ 1287 public QuestionnaireItemComponent setPrefix(String value) { 1288 if (Utilities.noString(value)) 1289 this.prefix = null; 1290 else { 1291 if (this.prefix == null) 1292 this.prefix = new StringType(); 1293 this.prefix.setValue(value); 1294 } 1295 return this; 1296 } 1297 1298 /** 1299 * @return {@link #text} (The name of a section, the text of a question or text 1300 * content for a display item.). This is the underlying object with id, 1301 * value and extensions. The accessor "getText" gives direct access to 1302 * the value 1303 */ 1304 public StringType getTextElement() { 1305 if (this.text == null) 1306 if (Configuration.errorOnAutoCreate()) 1307 throw new Error("Attempt to auto-create QuestionnaireItemComponent.text"); 1308 else if (Configuration.doAutoCreate()) 1309 this.text = new StringType(); // bb 1310 return this.text; 1311 } 1312 1313 public boolean hasTextElement() { 1314 return this.text != null && !this.text.isEmpty(); 1315 } 1316 1317 public boolean hasText() { 1318 return this.text != null && !this.text.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #text} (The name of a section, the text of a question or 1323 * text content for a display item.). This is the underlying object 1324 * with id, value and extensions. The accessor "getText" gives 1325 * direct access to the value 1326 */ 1327 public QuestionnaireItemComponent setTextElement(StringType value) { 1328 this.text = value; 1329 return this; 1330 } 1331 1332 /** 1333 * @return The name of a section, the text of a question or text content for a 1334 * display item. 1335 */ 1336 public String getText() { 1337 return this.text == null ? null : this.text.getValue(); 1338 } 1339 1340 /** 1341 * @param value The name of a section, the text of a question or text content 1342 * for a display item. 1343 */ 1344 public QuestionnaireItemComponent setText(String value) { 1345 if (Utilities.noString(value)) 1346 this.text = null; 1347 else { 1348 if (this.text == null) 1349 this.text = new StringType(); 1350 this.text.setValue(value); 1351 } 1352 return this; 1353 } 1354 1355 /** 1356 * @return {@link #type} (The type of questionnaire item this is - whether text 1357 * for display, a grouping of other items or a particular type of data 1358 * to be captured (string, integer, coded choice, etc.).). This is the 1359 * underlying object with id, value and extensions. The accessor 1360 * "getType" gives direct access to the value 1361 */ 1362 public Enumeration<QuestionnaireItemType> getTypeElement() { 1363 if (this.type == null) 1364 if (Configuration.errorOnAutoCreate()) 1365 throw new Error("Attempt to auto-create QuestionnaireItemComponent.type"); 1366 else if (Configuration.doAutoCreate()) 1367 this.type = new Enumeration<QuestionnaireItemType>(new QuestionnaireItemTypeEnumFactory()); // bb 1368 return this.type; 1369 } 1370 1371 public boolean hasTypeElement() { 1372 return this.type != null && !this.type.isEmpty(); 1373 } 1374 1375 public boolean hasType() { 1376 return this.type != null && !this.type.isEmpty(); 1377 } 1378 1379 /** 1380 * @param value {@link #type} (The type of questionnaire item this is - whether 1381 * text for display, a grouping of other items or a particular type 1382 * of data to be captured (string, integer, coded choice, etc.).). 1383 * This is the underlying object with id, value and extensions. The 1384 * accessor "getType" gives direct access to the value 1385 */ 1386 public QuestionnaireItemComponent setTypeElement(Enumeration<QuestionnaireItemType> value) { 1387 this.type = value; 1388 return this; 1389 } 1390 1391 /** 1392 * @return The type of questionnaire item this is - whether text for display, a 1393 * grouping of other items or a particular type of data to be captured 1394 * (string, integer, coded choice, etc.). 1395 */ 1396 public QuestionnaireItemType getType() { 1397 return this.type == null ? null : this.type.getValue(); 1398 } 1399 1400 /** 1401 * @param value The type of questionnaire item this is - whether text for 1402 * display, a grouping of other items or a particular type of data 1403 * to be captured (string, integer, coded choice, etc.). 1404 */ 1405 public QuestionnaireItemComponent setType(QuestionnaireItemType value) { 1406 if (this.type == null) 1407 this.type = new Enumeration<QuestionnaireItemType>(new QuestionnaireItemTypeEnumFactory()); 1408 this.type.setValue(value); 1409 return this; 1410 } 1411 1412 /** 1413 * @return {@link #enableWhen} (A constraint indicating that this item should 1414 * only be enabled (displayed/allow answers to be captured) when the 1415 * specified condition is true.) 1416 */ 1417 public List<QuestionnaireItemEnableWhenComponent> getEnableWhen() { 1418 if (this.enableWhen == null) 1419 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 1420 return this.enableWhen; 1421 } 1422 1423 /** 1424 * @return Returns a reference to <code>this</code> for easy method chaining 1425 */ 1426 public QuestionnaireItemComponent setEnableWhen(List<QuestionnaireItemEnableWhenComponent> theEnableWhen) { 1427 this.enableWhen = theEnableWhen; 1428 return this; 1429 } 1430 1431 public boolean hasEnableWhen() { 1432 if (this.enableWhen == null) 1433 return false; 1434 for (QuestionnaireItemEnableWhenComponent item : this.enableWhen) 1435 if (!item.isEmpty()) 1436 return true; 1437 return false; 1438 } 1439 1440 public QuestionnaireItemEnableWhenComponent addEnableWhen() { // 3 1441 QuestionnaireItemEnableWhenComponent t = new QuestionnaireItemEnableWhenComponent(); 1442 if (this.enableWhen == null) 1443 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 1444 this.enableWhen.add(t); 1445 return t; 1446 } 1447 1448 public QuestionnaireItemComponent addEnableWhen(QuestionnaireItemEnableWhenComponent t) { // 3 1449 if (t == null) 1450 return this; 1451 if (this.enableWhen == null) 1452 this.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 1453 this.enableWhen.add(t); 1454 return this; 1455 } 1456 1457 /** 1458 * @return The first repetition of repeating field {@link #enableWhen}, creating 1459 * it if it does not already exist 1460 */ 1461 public QuestionnaireItemEnableWhenComponent getEnableWhenFirstRep() { 1462 if (getEnableWhen().isEmpty()) { 1463 addEnableWhen(); 1464 } 1465 return getEnableWhen().get(0); 1466 } 1467 1468 /** 1469 * @return {@link #enableBehavior} (Controls how multiple enableWhen values are 1470 * interpreted - whether all or any must be true.). This is the 1471 * underlying object with id, value and extensions. The accessor 1472 * "getEnableBehavior" gives direct access to the value 1473 */ 1474 public Enumeration<EnableWhenBehavior> getEnableBehaviorElement() { 1475 if (this.enableBehavior == null) 1476 if (Configuration.errorOnAutoCreate()) 1477 throw new Error("Attempt to auto-create QuestionnaireItemComponent.enableBehavior"); 1478 else if (Configuration.doAutoCreate()) 1479 this.enableBehavior = new Enumeration<EnableWhenBehavior>(new EnableWhenBehaviorEnumFactory()); // bb 1480 return this.enableBehavior; 1481 } 1482 1483 public boolean hasEnableBehaviorElement() { 1484 return this.enableBehavior != null && !this.enableBehavior.isEmpty(); 1485 } 1486 1487 public boolean hasEnableBehavior() { 1488 return this.enableBehavior != null && !this.enableBehavior.isEmpty(); 1489 } 1490 1491 /** 1492 * @param value {@link #enableBehavior} (Controls how multiple enableWhen values 1493 * are interpreted - whether all or any must be true.). This is the 1494 * underlying object with id, value and extensions. The accessor 1495 * "getEnableBehavior" gives direct access to the value 1496 */ 1497 public QuestionnaireItemComponent setEnableBehaviorElement(Enumeration<EnableWhenBehavior> value) { 1498 this.enableBehavior = value; 1499 return this; 1500 } 1501 1502 /** 1503 * @return Controls how multiple enableWhen values are interpreted - whether all 1504 * or any must be true. 1505 */ 1506 public EnableWhenBehavior getEnableBehavior() { 1507 return this.enableBehavior == null ? null : this.enableBehavior.getValue(); 1508 } 1509 1510 /** 1511 * @param value Controls how multiple enableWhen values are interpreted - 1512 * whether all or any must be true. 1513 */ 1514 public QuestionnaireItemComponent setEnableBehavior(EnableWhenBehavior value) { 1515 if (value == null) 1516 this.enableBehavior = null; 1517 else { 1518 if (this.enableBehavior == null) 1519 this.enableBehavior = new Enumeration<EnableWhenBehavior>(new EnableWhenBehaviorEnumFactory()); 1520 this.enableBehavior.setValue(value); 1521 } 1522 return this; 1523 } 1524 1525 /** 1526 * @return {@link #required} (An indication, if true, that the item must be 1527 * present in a "completed" QuestionnaireResponse. If false, the item 1528 * may be skipped when answering the questionnaire.). This is the 1529 * underlying object with id, value and extensions. The accessor 1530 * "getRequired" gives direct access to the value 1531 */ 1532 public BooleanType getRequiredElement() { 1533 if (this.required == null) 1534 if (Configuration.errorOnAutoCreate()) 1535 throw new Error("Attempt to auto-create QuestionnaireItemComponent.required"); 1536 else if (Configuration.doAutoCreate()) 1537 this.required = new BooleanType(); // bb 1538 return this.required; 1539 } 1540 1541 public boolean hasRequiredElement() { 1542 return this.required != null && !this.required.isEmpty(); 1543 } 1544 1545 public boolean hasRequired() { 1546 return this.required != null && !this.required.isEmpty(); 1547 } 1548 1549 /** 1550 * @param value {@link #required} (An indication, if true, that the item must be 1551 * present in a "completed" QuestionnaireResponse. If false, the 1552 * item may be skipped when answering the questionnaire.). This is 1553 * the underlying object with id, value and extensions. The 1554 * accessor "getRequired" gives direct access to the value 1555 */ 1556 public QuestionnaireItemComponent setRequiredElement(BooleanType value) { 1557 this.required = value; 1558 return this; 1559 } 1560 1561 /** 1562 * @return An indication, if true, that the item must be present in a 1563 * "completed" QuestionnaireResponse. If false, the item may be skipped 1564 * when answering the questionnaire. 1565 */ 1566 public boolean getRequired() { 1567 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 1568 } 1569 1570 /** 1571 * @param value An indication, if true, that the item must be present in a 1572 * "completed" QuestionnaireResponse. If false, the item may be 1573 * skipped when answering the questionnaire. 1574 */ 1575 public QuestionnaireItemComponent setRequired(boolean value) { 1576 if (this.required == null) 1577 this.required = new BooleanType(); 1578 this.required.setValue(value); 1579 return this; 1580 } 1581 1582 /** 1583 * @return {@link #repeats} (An indication, if true, that the item may occur 1584 * multiple times in the response, collecting multiple answers for 1585 * questions or multiple sets of answers for groups.). This is the 1586 * underlying object with id, value and extensions. The accessor 1587 * "getRepeats" gives direct access to the value 1588 */ 1589 public BooleanType getRepeatsElement() { 1590 if (this.repeats == null) 1591 if (Configuration.errorOnAutoCreate()) 1592 throw new Error("Attempt to auto-create QuestionnaireItemComponent.repeats"); 1593 else if (Configuration.doAutoCreate()) 1594 this.repeats = new BooleanType(); // bb 1595 return this.repeats; 1596 } 1597 1598 public boolean hasRepeatsElement() { 1599 return this.repeats != null && !this.repeats.isEmpty(); 1600 } 1601 1602 public boolean hasRepeats() { 1603 return this.repeats != null && !this.repeats.isEmpty(); 1604 } 1605 1606 /** 1607 * @param value {@link #repeats} (An indication, if true, that the item may 1608 * occur multiple times in the response, collecting multiple 1609 * answers for questions or multiple sets of answers for groups.). 1610 * This is the underlying object with id, value and extensions. The 1611 * accessor "getRepeats" gives direct access to the value 1612 */ 1613 public QuestionnaireItemComponent setRepeatsElement(BooleanType value) { 1614 this.repeats = value; 1615 return this; 1616 } 1617 1618 /** 1619 * @return An indication, if true, that the item may occur multiple times in the 1620 * response, collecting multiple answers for questions or multiple sets 1621 * of answers for groups. 1622 */ 1623 public boolean getRepeats() { 1624 return this.repeats == null || this.repeats.isEmpty() ? false : this.repeats.getValue(); 1625 } 1626 1627 /** 1628 * @param value An indication, if true, that the item may occur multiple times 1629 * in the response, collecting multiple answers for questions or 1630 * multiple sets of answers for groups. 1631 */ 1632 public QuestionnaireItemComponent setRepeats(boolean value) { 1633 if (this.repeats == null) 1634 this.repeats = new BooleanType(); 1635 this.repeats.setValue(value); 1636 return this; 1637 } 1638 1639 /** 1640 * @return {@link #readOnly} (An indication, when true, that the value cannot be 1641 * changed by a human respondent to the Questionnaire.). This is the 1642 * underlying object with id, value and extensions. The accessor 1643 * "getReadOnly" gives direct access to the value 1644 */ 1645 public BooleanType getReadOnlyElement() { 1646 if (this.readOnly == null) 1647 if (Configuration.errorOnAutoCreate()) 1648 throw new Error("Attempt to auto-create QuestionnaireItemComponent.readOnly"); 1649 else if (Configuration.doAutoCreate()) 1650 this.readOnly = new BooleanType(); // bb 1651 return this.readOnly; 1652 } 1653 1654 public boolean hasReadOnlyElement() { 1655 return this.readOnly != null && !this.readOnly.isEmpty(); 1656 } 1657 1658 public boolean hasReadOnly() { 1659 return this.readOnly != null && !this.readOnly.isEmpty(); 1660 } 1661 1662 /** 1663 * @param value {@link #readOnly} (An indication, when true, that the value 1664 * cannot be changed by a human respondent to the Questionnaire.). 1665 * This is the underlying object with id, value and extensions. The 1666 * accessor "getReadOnly" gives direct access to the value 1667 */ 1668 public QuestionnaireItemComponent setReadOnlyElement(BooleanType value) { 1669 this.readOnly = value; 1670 return this; 1671 } 1672 1673 /** 1674 * @return An indication, when true, that the value cannot be changed by a human 1675 * respondent to the Questionnaire. 1676 */ 1677 public boolean getReadOnly() { 1678 return this.readOnly == null || this.readOnly.isEmpty() ? false : this.readOnly.getValue(); 1679 } 1680 1681 /** 1682 * @param value An indication, when true, that the value cannot be changed by a 1683 * human respondent to the Questionnaire. 1684 */ 1685 public QuestionnaireItemComponent setReadOnly(boolean value) { 1686 if (this.readOnly == null) 1687 this.readOnly = new BooleanType(); 1688 this.readOnly.setValue(value); 1689 return this; 1690 } 1691 1692 /** 1693 * @return {@link #maxLength} (The maximum number of characters that are 1694 * permitted in the answer to be considered a "valid" 1695 * QuestionnaireResponse.). This is the underlying object with id, value 1696 * and extensions. The accessor "getMaxLength" gives direct access to 1697 * the value 1698 */ 1699 public IntegerType getMaxLengthElement() { 1700 if (this.maxLength == null) 1701 if (Configuration.errorOnAutoCreate()) 1702 throw new Error("Attempt to auto-create QuestionnaireItemComponent.maxLength"); 1703 else if (Configuration.doAutoCreate()) 1704 this.maxLength = new IntegerType(); // bb 1705 return this.maxLength; 1706 } 1707 1708 public boolean hasMaxLengthElement() { 1709 return this.maxLength != null && !this.maxLength.isEmpty(); 1710 } 1711 1712 public boolean hasMaxLength() { 1713 return this.maxLength != null && !this.maxLength.isEmpty(); 1714 } 1715 1716 /** 1717 * @param value {@link #maxLength} (The maximum number of characters that are 1718 * permitted in the answer to be considered a "valid" 1719 * QuestionnaireResponse.). This is the underlying object with id, 1720 * value and extensions. The accessor "getMaxLength" gives direct 1721 * access to the value 1722 */ 1723 public QuestionnaireItemComponent setMaxLengthElement(IntegerType value) { 1724 this.maxLength = value; 1725 return this; 1726 } 1727 1728 /** 1729 * @return The maximum number of characters that are permitted in the answer to 1730 * be considered a "valid" QuestionnaireResponse. 1731 */ 1732 public int getMaxLength() { 1733 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 1734 } 1735 1736 /** 1737 * @param value The maximum number of characters that are permitted in the 1738 * answer to be considered a "valid" QuestionnaireResponse. 1739 */ 1740 public QuestionnaireItemComponent setMaxLength(int value) { 1741 if (this.maxLength == null) 1742 this.maxLength = new IntegerType(); 1743 this.maxLength.setValue(value); 1744 return this; 1745 } 1746 1747 /** 1748 * @return {@link #answerValueSet} (A reference to a value set containing a list 1749 * of codes representing permitted answers for a "choice" or 1750 * "open-choice" question.). This is the underlying object with id, 1751 * value and extensions. The accessor "getAnswerValueSet" gives direct 1752 * access to the value 1753 */ 1754 public CanonicalType getAnswerValueSetElement() { 1755 if (this.answerValueSet == null) 1756 if (Configuration.errorOnAutoCreate()) 1757 throw new Error("Attempt to auto-create QuestionnaireItemComponent.answerValueSet"); 1758 else if (Configuration.doAutoCreate()) 1759 this.answerValueSet = new CanonicalType(); // bb 1760 return this.answerValueSet; 1761 } 1762 1763 public boolean hasAnswerValueSetElement() { 1764 return this.answerValueSet != null && !this.answerValueSet.isEmpty(); 1765 } 1766 1767 public boolean hasAnswerValueSet() { 1768 return this.answerValueSet != null && !this.answerValueSet.isEmpty(); 1769 } 1770 1771 /** 1772 * @param value {@link #answerValueSet} (A reference to a value set containing a 1773 * list of codes representing permitted answers for a "choice" or 1774 * "open-choice" question.). This is the underlying object with id, 1775 * value and extensions. The accessor "getAnswerValueSet" gives 1776 * direct access to the value 1777 */ 1778 public QuestionnaireItemComponent setAnswerValueSetElement(CanonicalType value) { 1779 this.answerValueSet = value; 1780 return this; 1781 } 1782 1783 /** 1784 * @return A reference to a value set containing a list of codes representing 1785 * permitted answers for a "choice" or "open-choice" question. 1786 */ 1787 public String getAnswerValueSet() { 1788 return this.answerValueSet == null ? null : this.answerValueSet.getValue(); 1789 } 1790 1791 /** 1792 * @param value A reference to a value set containing a list of codes 1793 * representing permitted answers for a "choice" or "open-choice" 1794 * question. 1795 */ 1796 public QuestionnaireItemComponent setAnswerValueSet(String value) { 1797 if (Utilities.noString(value)) 1798 this.answerValueSet = null; 1799 else { 1800 if (this.answerValueSet == null) 1801 this.answerValueSet = new CanonicalType(); 1802 this.answerValueSet.setValue(value); 1803 } 1804 return this; 1805 } 1806 1807 /** 1808 * @return {@link #answerOption} (One of the permitted answers for a "choice" or 1809 * "open-choice" question.) 1810 */ 1811 public List<QuestionnaireItemAnswerOptionComponent> getAnswerOption() { 1812 if (this.answerOption == null) 1813 this.answerOption = new ArrayList<QuestionnaireItemAnswerOptionComponent>(); 1814 return this.answerOption; 1815 } 1816 1817 /** 1818 * @return Returns a reference to <code>this</code> for easy method chaining 1819 */ 1820 public QuestionnaireItemComponent setAnswerOption(List<QuestionnaireItemAnswerOptionComponent> theAnswerOption) { 1821 this.answerOption = theAnswerOption; 1822 return this; 1823 } 1824 1825 public boolean hasAnswerOption() { 1826 if (this.answerOption == null) 1827 return false; 1828 for (QuestionnaireItemAnswerOptionComponent item : this.answerOption) 1829 if (!item.isEmpty()) 1830 return true; 1831 return false; 1832 } 1833 1834 public QuestionnaireItemAnswerOptionComponent addAnswerOption() { // 3 1835 QuestionnaireItemAnswerOptionComponent t = new QuestionnaireItemAnswerOptionComponent(); 1836 if (this.answerOption == null) 1837 this.answerOption = new ArrayList<QuestionnaireItemAnswerOptionComponent>(); 1838 this.answerOption.add(t); 1839 return t; 1840 } 1841 1842 public QuestionnaireItemComponent addAnswerOption(QuestionnaireItemAnswerOptionComponent t) { // 3 1843 if (t == null) 1844 return this; 1845 if (this.answerOption == null) 1846 this.answerOption = new ArrayList<QuestionnaireItemAnswerOptionComponent>(); 1847 this.answerOption.add(t); 1848 return this; 1849 } 1850 1851 /** 1852 * @return The first repetition of repeating field {@link #answerOption}, 1853 * creating it if it does not already exist 1854 */ 1855 public QuestionnaireItemAnswerOptionComponent getAnswerOptionFirstRep() { 1856 if (getAnswerOption().isEmpty()) { 1857 addAnswerOption(); 1858 } 1859 return getAnswerOption().get(0); 1860 } 1861 1862 /** 1863 * @return {@link #initial} (One or more values that should be pre-populated in 1864 * the answer when initially rendering the questionnaire for user 1865 * input.) 1866 */ 1867 public List<QuestionnaireItemInitialComponent> getInitial() { 1868 if (this.initial == null) 1869 this.initial = new ArrayList<QuestionnaireItemInitialComponent>(); 1870 return this.initial; 1871 } 1872 1873 /** 1874 * @return Returns a reference to <code>this</code> for easy method chaining 1875 */ 1876 public QuestionnaireItemComponent setInitial(List<QuestionnaireItemInitialComponent> theInitial) { 1877 this.initial = theInitial; 1878 return this; 1879 } 1880 1881 public boolean hasInitial() { 1882 if (this.initial == null) 1883 return false; 1884 for (QuestionnaireItemInitialComponent item : this.initial) 1885 if (!item.isEmpty()) 1886 return true; 1887 return false; 1888 } 1889 1890 public QuestionnaireItemInitialComponent addInitial() { // 3 1891 QuestionnaireItemInitialComponent t = new QuestionnaireItemInitialComponent(); 1892 if (this.initial == null) 1893 this.initial = new ArrayList<QuestionnaireItemInitialComponent>(); 1894 this.initial.add(t); 1895 return t; 1896 } 1897 1898 public QuestionnaireItemComponent addInitial(QuestionnaireItemInitialComponent t) { // 3 1899 if (t == null) 1900 return this; 1901 if (this.initial == null) 1902 this.initial = new ArrayList<QuestionnaireItemInitialComponent>(); 1903 this.initial.add(t); 1904 return this; 1905 } 1906 1907 /** 1908 * @return The first repetition of repeating field {@link #initial}, creating it 1909 * if it does not already exist 1910 */ 1911 public QuestionnaireItemInitialComponent getInitialFirstRep() { 1912 if (getInitial().isEmpty()) { 1913 addInitial(); 1914 } 1915 return getInitial().get(0); 1916 } 1917 1918 /** 1919 * @return {@link #item} (Text, questions and other groups to be nested beneath 1920 * a question or group.) 1921 */ 1922 public List<QuestionnaireItemComponent> getItem() { 1923 if (this.item == null) 1924 this.item = new ArrayList<QuestionnaireItemComponent>(); 1925 return this.item; 1926 } 1927 1928 /** 1929 * @return Returns a reference to <code>this</code> for easy method chaining 1930 */ 1931 public QuestionnaireItemComponent setItem(List<QuestionnaireItemComponent> theItem) { 1932 this.item = theItem; 1933 return this; 1934 } 1935 1936 public boolean hasItem() { 1937 if (this.item == null) 1938 return false; 1939 for (QuestionnaireItemComponent item : this.item) 1940 if (!item.isEmpty()) 1941 return true; 1942 return false; 1943 } 1944 1945 public QuestionnaireItemComponent addItem() { // 3 1946 QuestionnaireItemComponent t = new QuestionnaireItemComponent(); 1947 if (this.item == null) 1948 this.item = new ArrayList<QuestionnaireItemComponent>(); 1949 this.item.add(t); 1950 return t; 1951 } 1952 1953 public QuestionnaireItemComponent addItem(QuestionnaireItemComponent t) { // 3 1954 if (t == null) 1955 return this; 1956 if (this.item == null) 1957 this.item = new ArrayList<QuestionnaireItemComponent>(); 1958 this.item.add(t); 1959 return this; 1960 } 1961 1962 /** 1963 * @return The first repetition of repeating field {@link #item}, creating it if 1964 * it does not already exist 1965 */ 1966 public QuestionnaireItemComponent getItemFirstRep() { 1967 if (getItem().isEmpty()) { 1968 addItem(); 1969 } 1970 return getItem().get(0); 1971 } 1972 1973 protected void listChildren(List<Property> children) { 1974 super.listChildren(children); 1975 children.add(new Property("linkId", "string", 1976 "An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.", 1977 0, 1, linkId)); 1978 children.add(new Property("definition", "uri", 1979 "This element is a URI that refers to an [[[ElementDefinition]]] that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in Comments, below. If this element is present then the following element values MAY be derived from the Element Definition if the corresponding elements of this Questionnaire resource instance have no value:\n\n* code (ElementDefinition.code) \n* type (ElementDefinition.type) \n* required (ElementDefinition.min) \n* repeats (ElementDefinition.max) \n* maxLength (ElementDefinition.maxLength) \n* answerValueSet (ElementDefinition.binding)\n* options (ElementDefinition.binding).", 1980 0, 1, definition)); 1981 children.add(new Property("code", "Coding", 1982 "A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).", 1983 0, java.lang.Integer.MAX_VALUE, code)); 1984 children.add(new Property("prefix", "string", 1985 "A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.", 1986 0, 1, prefix)); 1987 children.add(new Property("text", "string", 1988 "The name of a section, the text of a question or text content for a display item.", 0, 1, text)); 1989 children.add(new Property("type", "code", 1990 "The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.).", 1991 0, 1, type)); 1992 children.add(new Property("enableWhen", "", 1993 "A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.", 1994 0, java.lang.Integer.MAX_VALUE, enableWhen)); 1995 children.add(new Property("enableBehavior", "code", 1996 "Controls how multiple enableWhen values are interpreted - whether all or any must be true.", 0, 1, 1997 enableBehavior)); 1998 children.add(new Property("required", "boolean", 1999 "An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.", 2000 0, 1, required)); 2001 children.add(new Property("repeats", "boolean", 2002 "An indication, if true, that the item may occur multiple times in the response, collecting multiple answers for questions or multiple sets of answers for groups.", 2003 0, 1, repeats)); 2004 children.add(new Property("readOnly", "boolean", 2005 "An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.", 0, 2006 1, readOnly)); 2007 children.add(new Property("maxLength", "integer", 2008 "The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse.", 2009 0, 1, maxLength)); 2010 children.add(new Property("answerValueSet", "canonical(ValueSet)", 2011 "A reference to a value set containing a list of codes representing permitted answers for a \"choice\" or \"open-choice\" question.", 2012 0, 1, answerValueSet)); 2013 children.add( 2014 new Property("answerOption", "", "One of the permitted answers for a \"choice\" or \"open-choice\" question.", 2015 0, java.lang.Integer.MAX_VALUE, answerOption)); 2016 children.add(new Property("initial", "", 2017 "One or more values that should be pre-populated in the answer when initially rendering the questionnaire for user input.", 2018 0, java.lang.Integer.MAX_VALUE, initial)); 2019 children.add(new Property("item", "@Questionnaire.item", 2020 "Text, questions and other groups to be nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, 2021 item)); 2022 } 2023 2024 @Override 2025 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2026 switch (_hash) { 2027 case -1102667083: 2028 /* linkId */ return new Property("linkId", "string", 2029 "An identifier that is unique within the Questionnaire allowing linkage to the equivalent item in a QuestionnaireResponse resource.", 2030 0, 1, linkId); 2031 case -1014418093: 2032 /* definition */ return new Property("definition", "uri", 2033 "This element is a URI that refers to an [[[ElementDefinition]]] that provides information about this item, including information that might otherwise be included in the instance of the Questionnaire resource. A detailed description of the construction of the URI is shown in Comments, below. If this element is present then the following element values MAY be derived from the Element Definition if the corresponding elements of this Questionnaire resource instance have no value:\n\n* code (ElementDefinition.code) \n* type (ElementDefinition.type) \n* required (ElementDefinition.min) \n* repeats (ElementDefinition.max) \n* maxLength (ElementDefinition.maxLength) \n* answerValueSet (ElementDefinition.binding)\n* options (ElementDefinition.binding).", 2034 0, 1, definition); 2035 case 3059181: 2036 /* code */ return new Property("code", "Coding", 2037 "A terminology code that corresponds to this group or question (e.g. a code from LOINC, which defines many questions and answers).", 2038 0, java.lang.Integer.MAX_VALUE, code); 2039 case -980110702: 2040 /* prefix */ return new Property("prefix", "string", 2041 "A short label for a particular group, question or set of display text within the questionnaire used for reference by the individual completing the questionnaire.", 2042 0, 1, prefix); 2043 case 3556653: 2044 /* text */ return new Property("text", "string", 2045 "The name of a section, the text of a question or text content for a display item.", 0, 1, text); 2046 case 3575610: 2047 /* type */ return new Property("type", "code", 2048 "The type of questionnaire item this is - whether text for display, a grouping of other items or a particular type of data to be captured (string, integer, coded choice, etc.).", 2049 0, 1, type); 2050 case 1893321565: 2051 /* enableWhen */ return new Property("enableWhen", "", 2052 "A constraint indicating that this item should only be enabled (displayed/allow answers to be captured) when the specified condition is true.", 2053 0, java.lang.Integer.MAX_VALUE, enableWhen); 2054 case 1854802165: 2055 /* enableBehavior */ return new Property("enableBehavior", "code", 2056 "Controls how multiple enableWhen values are interpreted - whether all or any must be true.", 0, 1, 2057 enableBehavior); 2058 case -393139297: 2059 /* required */ return new Property("required", "boolean", 2060 "An indication, if true, that the item must be present in a \"completed\" QuestionnaireResponse. If false, the item may be skipped when answering the questionnaire.", 2061 0, 1, required); 2062 case 1094288952: 2063 /* repeats */ return new Property("repeats", "boolean", 2064 "An indication, if true, that the item may occur multiple times in the response, collecting multiple answers for questions or multiple sets of answers for groups.", 2065 0, 1, repeats); 2066 case -867683742: 2067 /* readOnly */ return new Property("readOnly", "boolean", 2068 "An indication, when true, that the value cannot be changed by a human respondent to the Questionnaire.", 0, 2069 1, readOnly); 2070 case -791400086: 2071 /* maxLength */ return new Property("maxLength", "integer", 2072 "The maximum number of characters that are permitted in the answer to be considered a \"valid\" QuestionnaireResponse.", 2073 0, 1, maxLength); 2074 case -743278833: 2075 /* answerValueSet */ return new Property("answerValueSet", "canonical(ValueSet)", 2076 "A reference to a value set containing a list of codes representing permitted answers for a \"choice\" or \"open-choice\" question.", 2077 0, 1, answerValueSet); 2078 case -1527878189: 2079 /* answerOption */ return new Property("answerOption", "", 2080 "One of the permitted answers for a \"choice\" or \"open-choice\" question.", 0, 2081 java.lang.Integer.MAX_VALUE, answerOption); 2082 case 1948342084: 2083 /* initial */ return new Property("initial", "", 2084 "One or more values that should be pre-populated in the answer when initially rendering the questionnaire for user input.", 2085 0, java.lang.Integer.MAX_VALUE, initial); 2086 case 3242771: 2087 /* item */ return new Property("item", "@Questionnaire.item", 2088 "Text, questions and other groups to be nested beneath a question or group.", 0, 2089 java.lang.Integer.MAX_VALUE, item); 2090 default: 2091 return super.getNamedProperty(_hash, _name, _checkValid); 2092 } 2093 2094 } 2095 2096 @Override 2097 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2098 switch (hash) { 2099 case -1102667083: 2100 /* linkId */ return this.linkId == null ? new Base[0] : new Base[] { this.linkId }; // StringType 2101 case -1014418093: 2102 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // UriType 2103 case 3059181: 2104 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 2105 case -980110702: 2106 /* prefix */ return this.prefix == null ? new Base[0] : new Base[] { this.prefix }; // StringType 2107 case 3556653: 2108 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 2109 case 3575610: 2110 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<QuestionnaireItemType> 2111 case 1893321565: 2112 /* enableWhen */ return this.enableWhen == null ? new Base[0] 2113 : this.enableWhen.toArray(new Base[this.enableWhen.size()]); // QuestionnaireItemEnableWhenComponent 2114 case 1854802165: 2115 /* enableBehavior */ return this.enableBehavior == null ? new Base[0] : new Base[] { this.enableBehavior }; // Enumeration<EnableWhenBehavior> 2116 case -393139297: 2117 /* required */ return this.required == null ? new Base[0] : new Base[] { this.required }; // BooleanType 2118 case 1094288952: 2119 /* repeats */ return this.repeats == null ? new Base[0] : new Base[] { this.repeats }; // BooleanType 2120 case -867683742: 2121 /* readOnly */ return this.readOnly == null ? new Base[0] : new Base[] { this.readOnly }; // BooleanType 2122 case -791400086: 2123 /* maxLength */ return this.maxLength == null ? new Base[0] : new Base[] { this.maxLength }; // IntegerType 2124 case -743278833: 2125 /* answerValueSet */ return this.answerValueSet == null ? new Base[0] : new Base[] { this.answerValueSet }; // CanonicalType 2126 case -1527878189: 2127 /* answerOption */ return this.answerOption == null ? new Base[0] 2128 : this.answerOption.toArray(new Base[this.answerOption.size()]); // QuestionnaireItemAnswerOptionComponent 2129 case 1948342084: 2130 /* initial */ return this.initial == null ? new Base[0] : this.initial.toArray(new Base[this.initial.size()]); // QuestionnaireItemInitialComponent 2131 case 3242771: 2132 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireItemComponent 2133 default: 2134 return super.getProperty(hash, name, checkValid); 2135 } 2136 2137 } 2138 2139 @Override 2140 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2141 switch (hash) { 2142 case -1102667083: // linkId 2143 this.linkId = castToString(value); // StringType 2144 return value; 2145 case -1014418093: // definition 2146 this.definition = castToUri(value); // UriType 2147 return value; 2148 case 3059181: // code 2149 this.getCode().add(castToCoding(value)); // Coding 2150 return value; 2151 case -980110702: // prefix 2152 this.prefix = castToString(value); // StringType 2153 return value; 2154 case 3556653: // text 2155 this.text = castToString(value); // StringType 2156 return value; 2157 case 3575610: // type 2158 value = new QuestionnaireItemTypeEnumFactory().fromType(castToCode(value)); 2159 this.type = (Enumeration) value; // Enumeration<QuestionnaireItemType> 2160 return value; 2161 case 1893321565: // enableWhen 2162 this.getEnableWhen().add((QuestionnaireItemEnableWhenComponent) value); // QuestionnaireItemEnableWhenComponent 2163 return value; 2164 case 1854802165: // enableBehavior 2165 value = new EnableWhenBehaviorEnumFactory().fromType(castToCode(value)); 2166 this.enableBehavior = (Enumeration) value; // Enumeration<EnableWhenBehavior> 2167 return value; 2168 case -393139297: // required 2169 this.required = castToBoolean(value); // BooleanType 2170 return value; 2171 case 1094288952: // repeats 2172 this.repeats = castToBoolean(value); // BooleanType 2173 return value; 2174 case -867683742: // readOnly 2175 this.readOnly = castToBoolean(value); // BooleanType 2176 return value; 2177 case -791400086: // maxLength 2178 this.maxLength = castToInteger(value); // IntegerType 2179 return value; 2180 case -743278833: // answerValueSet 2181 this.answerValueSet = castToCanonical(value); // CanonicalType 2182 return value; 2183 case -1527878189: // answerOption 2184 this.getAnswerOption().add((QuestionnaireItemAnswerOptionComponent) value); // QuestionnaireItemAnswerOptionComponent 2185 return value; 2186 case 1948342084: // initial 2187 this.getInitial().add((QuestionnaireItemInitialComponent) value); // QuestionnaireItemInitialComponent 2188 return value; 2189 case 3242771: // item 2190 this.getItem().add((QuestionnaireItemComponent) value); // QuestionnaireItemComponent 2191 return value; 2192 default: 2193 return super.setProperty(hash, name, value); 2194 } 2195 2196 } 2197 2198 @Override 2199 public Base setProperty(String name, Base value) throws FHIRException { 2200 if (name.equals("linkId")) { 2201 this.linkId = castToString(value); // StringType 2202 } else if (name.equals("definition")) { 2203 this.definition = castToUri(value); // UriType 2204 } else if (name.equals("code")) { 2205 this.getCode().add(castToCoding(value)); 2206 } else if (name.equals("prefix")) { 2207 this.prefix = castToString(value); // StringType 2208 } else if (name.equals("text")) { 2209 this.text = castToString(value); // StringType 2210 } else if (name.equals("type")) { 2211 value = new QuestionnaireItemTypeEnumFactory().fromType(castToCode(value)); 2212 this.type = (Enumeration) value; // Enumeration<QuestionnaireItemType> 2213 } else if (name.equals("enableWhen")) { 2214 this.getEnableWhen().add((QuestionnaireItemEnableWhenComponent) value); 2215 } else if (name.equals("enableBehavior")) { 2216 value = new EnableWhenBehaviorEnumFactory().fromType(castToCode(value)); 2217 this.enableBehavior = (Enumeration) value; // Enumeration<EnableWhenBehavior> 2218 } else if (name.equals("required")) { 2219 this.required = castToBoolean(value); // BooleanType 2220 } else if (name.equals("repeats")) { 2221 this.repeats = castToBoolean(value); // BooleanType 2222 } else if (name.equals("readOnly")) { 2223 this.readOnly = castToBoolean(value); // BooleanType 2224 } else if (name.equals("maxLength")) { 2225 this.maxLength = castToInteger(value); // IntegerType 2226 } else if (name.equals("answerValueSet")) { 2227 this.answerValueSet = castToCanonical(value); // CanonicalType 2228 } else if (name.equals("answerOption")) { 2229 this.getAnswerOption().add((QuestionnaireItemAnswerOptionComponent) value); 2230 } else if (name.equals("initial")) { 2231 this.getInitial().add((QuestionnaireItemInitialComponent) value); 2232 } else if (name.equals("item")) { 2233 this.getItem().add((QuestionnaireItemComponent) value); 2234 } else 2235 return super.setProperty(name, value); 2236 return value; 2237 } 2238 2239 @Override 2240 public void removeChild(String name, Base value) throws FHIRException { 2241 if (name.equals("linkId")) { 2242 this.linkId = null; 2243 } else if (name.equals("definition")) { 2244 this.definition = null; 2245 } else if (name.equals("code")) { 2246 this.getCode().remove(castToCoding(value)); 2247 } else if (name.equals("prefix")) { 2248 this.prefix = null; 2249 } else if (name.equals("text")) { 2250 this.text = null; 2251 } else if (name.equals("type")) { 2252 this.type = null; 2253 } else if (name.equals("enableWhen")) { 2254 this.getEnableWhen().remove((QuestionnaireItemEnableWhenComponent) value); 2255 } else if (name.equals("enableBehavior")) { 2256 this.enableBehavior = null; 2257 } else if (name.equals("required")) { 2258 this.required = null; 2259 } else if (name.equals("repeats")) { 2260 this.repeats = null; 2261 } else if (name.equals("readOnly")) { 2262 this.readOnly = null; 2263 } else if (name.equals("maxLength")) { 2264 this.maxLength = null; 2265 } else if (name.equals("answerValueSet")) { 2266 this.answerValueSet = null; 2267 } else if (name.equals("answerOption")) { 2268 this.getAnswerOption().remove((QuestionnaireItemAnswerOptionComponent) value); 2269 } else if (name.equals("initial")) { 2270 this.getInitial().remove((QuestionnaireItemInitialComponent) value); 2271 } else if (name.equals("item")) { 2272 this.getItem().remove((QuestionnaireItemComponent) value); 2273 } else 2274 super.removeChild(name, value); 2275 2276 } 2277 2278 @Override 2279 public Base makeProperty(int hash, String name) throws FHIRException { 2280 switch (hash) { 2281 case -1102667083: 2282 return getLinkIdElement(); 2283 case -1014418093: 2284 return getDefinitionElement(); 2285 case 3059181: 2286 return addCode(); 2287 case -980110702: 2288 return getPrefixElement(); 2289 case 3556653: 2290 return getTextElement(); 2291 case 3575610: 2292 return getTypeElement(); 2293 case 1893321565: 2294 return addEnableWhen(); 2295 case 1854802165: 2296 return getEnableBehaviorElement(); 2297 case -393139297: 2298 return getRequiredElement(); 2299 case 1094288952: 2300 return getRepeatsElement(); 2301 case -867683742: 2302 return getReadOnlyElement(); 2303 case -791400086: 2304 return getMaxLengthElement(); 2305 case -743278833: 2306 return getAnswerValueSetElement(); 2307 case -1527878189: 2308 return addAnswerOption(); 2309 case 1948342084: 2310 return addInitial(); 2311 case 3242771: 2312 return addItem(); 2313 default: 2314 return super.makeProperty(hash, name); 2315 } 2316 2317 } 2318 2319 @Override 2320 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2321 switch (hash) { 2322 case -1102667083: 2323 /* linkId */ return new String[] { "string" }; 2324 case -1014418093: 2325 /* definition */ return new String[] { "uri" }; 2326 case 3059181: 2327 /* code */ return new String[] { "Coding" }; 2328 case -980110702: 2329 /* prefix */ return new String[] { "string" }; 2330 case 3556653: 2331 /* text */ return new String[] { "string" }; 2332 case 3575610: 2333 /* type */ return new String[] { "code" }; 2334 case 1893321565: 2335 /* enableWhen */ return new String[] {}; 2336 case 1854802165: 2337 /* enableBehavior */ return new String[] { "code" }; 2338 case -393139297: 2339 /* required */ return new String[] { "boolean" }; 2340 case 1094288952: 2341 /* repeats */ return new String[] { "boolean" }; 2342 case -867683742: 2343 /* readOnly */ return new String[] { "boolean" }; 2344 case -791400086: 2345 /* maxLength */ return new String[] { "integer" }; 2346 case -743278833: 2347 /* answerValueSet */ return new String[] { "canonical" }; 2348 case -1527878189: 2349 /* answerOption */ return new String[] {}; 2350 case 1948342084: 2351 /* initial */ return new String[] {}; 2352 case 3242771: 2353 /* item */ return new String[] { "@Questionnaire.item" }; 2354 default: 2355 return super.getTypesForProperty(hash, name); 2356 } 2357 2358 } 2359 2360 @Override 2361 public Base addChild(String name) throws FHIRException { 2362 if (name.equals("linkId")) { 2363 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.linkId"); 2364 } else if (name.equals("definition")) { 2365 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.definition"); 2366 } else if (name.equals("code")) { 2367 return addCode(); 2368 } else if (name.equals("prefix")) { 2369 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.prefix"); 2370 } else if (name.equals("text")) { 2371 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.text"); 2372 } else if (name.equals("type")) { 2373 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.type"); 2374 } else if (name.equals("enableWhen")) { 2375 return addEnableWhen(); 2376 } else if (name.equals("enableBehavior")) { 2377 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.enableBehavior"); 2378 } else if (name.equals("required")) { 2379 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.required"); 2380 } else if (name.equals("repeats")) { 2381 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.repeats"); 2382 } else if (name.equals("readOnly")) { 2383 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.readOnly"); 2384 } else if (name.equals("maxLength")) { 2385 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.maxLength"); 2386 } else if (name.equals("answerValueSet")) { 2387 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.answerValueSet"); 2388 } else if (name.equals("answerOption")) { 2389 return addAnswerOption(); 2390 } else if (name.equals("initial")) { 2391 return addInitial(); 2392 } else if (name.equals("item")) { 2393 return addItem(); 2394 } else 2395 return super.addChild(name); 2396 } 2397 2398 public QuestionnaireItemComponent copy() { 2399 QuestionnaireItemComponent dst = new QuestionnaireItemComponent(); 2400 copyValues(dst); 2401 return dst; 2402 } 2403 2404 public void copyValues(QuestionnaireItemComponent dst) { 2405 super.copyValues(dst); 2406 dst.linkId = linkId == null ? null : linkId.copy(); 2407 dst.definition = definition == null ? null : definition.copy(); 2408 if (code != null) { 2409 dst.code = new ArrayList<Coding>(); 2410 for (Coding i : code) 2411 dst.code.add(i.copy()); 2412 } 2413 ; 2414 dst.prefix = prefix == null ? null : prefix.copy(); 2415 dst.text = text == null ? null : text.copy(); 2416 dst.type = type == null ? null : type.copy(); 2417 if (enableWhen != null) { 2418 dst.enableWhen = new ArrayList<QuestionnaireItemEnableWhenComponent>(); 2419 for (QuestionnaireItemEnableWhenComponent i : enableWhen) 2420 dst.enableWhen.add(i.copy()); 2421 } 2422 ; 2423 dst.enableBehavior = enableBehavior == null ? null : enableBehavior.copy(); 2424 dst.required = required == null ? null : required.copy(); 2425 dst.repeats = repeats == null ? null : repeats.copy(); 2426 dst.readOnly = readOnly == null ? null : readOnly.copy(); 2427 dst.maxLength = maxLength == null ? null : maxLength.copy(); 2428 dst.answerValueSet = answerValueSet == null ? null : answerValueSet.copy(); 2429 if (answerOption != null) { 2430 dst.answerOption = new ArrayList<QuestionnaireItemAnswerOptionComponent>(); 2431 for (QuestionnaireItemAnswerOptionComponent i : answerOption) 2432 dst.answerOption.add(i.copy()); 2433 } 2434 ; 2435 if (initial != null) { 2436 dst.initial = new ArrayList<QuestionnaireItemInitialComponent>(); 2437 for (QuestionnaireItemInitialComponent i : initial) 2438 dst.initial.add(i.copy()); 2439 } 2440 ; 2441 if (item != null) { 2442 dst.item = new ArrayList<QuestionnaireItemComponent>(); 2443 for (QuestionnaireItemComponent i : item) 2444 dst.item.add(i.copy()); 2445 } 2446 ; 2447 } 2448 2449 @Override 2450 public boolean equalsDeep(Base other_) { 2451 if (!super.equalsDeep(other_)) 2452 return false; 2453 if (!(other_ instanceof QuestionnaireItemComponent)) 2454 return false; 2455 QuestionnaireItemComponent o = (QuestionnaireItemComponent) other_; 2456 return compareDeep(linkId, o.linkId, true) && compareDeep(definition, o.definition, true) 2457 && compareDeep(code, o.code, true) && compareDeep(prefix, o.prefix, true) && compareDeep(text, o.text, true) 2458 && compareDeep(type, o.type, true) && compareDeep(enableWhen, o.enableWhen, true) 2459 && compareDeep(enableBehavior, o.enableBehavior, true) && compareDeep(required, o.required, true) 2460 && compareDeep(repeats, o.repeats, true) && compareDeep(readOnly, o.readOnly, true) 2461 && compareDeep(maxLength, o.maxLength, true) && compareDeep(answerValueSet, o.answerValueSet, true) 2462 && compareDeep(answerOption, o.answerOption, true) && compareDeep(initial, o.initial, true) 2463 && compareDeep(item, o.item, true); 2464 } 2465 2466 @Override 2467 public boolean equalsShallow(Base other_) { 2468 if (!super.equalsShallow(other_)) 2469 return false; 2470 if (!(other_ instanceof QuestionnaireItemComponent)) 2471 return false; 2472 QuestionnaireItemComponent o = (QuestionnaireItemComponent) other_; 2473 return compareValues(linkId, o.linkId, true) && compareValues(definition, o.definition, true) 2474 && compareValues(prefix, o.prefix, true) && compareValues(text, o.text, true) 2475 && compareValues(type, o.type, true) && compareValues(enableBehavior, o.enableBehavior, true) 2476 && compareValues(required, o.required, true) && compareValues(repeats, o.repeats, true) 2477 && compareValues(readOnly, o.readOnly, true) && compareValues(maxLength, o.maxLength, true); 2478 } 2479 2480 public boolean isEmpty() { 2481 return super.isEmpty() 2482 && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, definition, code, prefix, text, type, enableWhen, 2483 enableBehavior, required, repeats, readOnly, maxLength, answerValueSet, answerOption, initial, item); 2484 } 2485 2486 public String fhirType() { 2487 return "Questionnaire.item"; 2488 2489 } 2490 2491 } 2492 2493 @Block() 2494 public static class QuestionnaireItemEnableWhenComponent extends BackboneElement implements IBaseBackboneElement { 2495 /** 2496 * The linkId for the question whose answer (or lack of answer) governs whether 2497 * this item is enabled. 2498 */ 2499 @Child(name = "question", type = { 2500 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2501 @Description(shortDefinition = "Question that determines whether item is enabled", formalDefinition = "The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.") 2502 protected StringType question; 2503 2504 /** 2505 * Specifies the criteria by which the question is enabled. 2506 */ 2507 @Child(name = "operator", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2508 @Description(shortDefinition = "exists | = | != | > | < | >= | <=", formalDefinition = "Specifies the criteria by which the question is enabled.") 2509 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-enable-operator") 2510 protected Enumeration<QuestionnaireItemOperator> operator; 2511 2512 /** 2513 * A value that the referenced question is tested using the specified operator 2514 * in order for the item to be enabled. 2515 */ 2516 @Child(name = "answer", type = { BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, 2517 DateTimeType.class, TimeType.class, StringType.class, Coding.class, Quantity.class, 2518 Reference.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 2519 @Description(shortDefinition = "Value for question comparison based on operator", formalDefinition = "A value that the referenced question is tested using the specified operator in order for the item to be enabled.") 2520 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-answers") 2521 protected Type answer; 2522 2523 private static final long serialVersionUID = -1815133868L; 2524 2525 /** 2526 * Constructor 2527 */ 2528 public QuestionnaireItemEnableWhenComponent() { 2529 super(); 2530 } 2531 2532 /** 2533 * Constructor 2534 */ 2535 public QuestionnaireItemEnableWhenComponent(StringType question, Enumeration<QuestionnaireItemOperator> operator, 2536 Type answer) { 2537 super(); 2538 this.question = question; 2539 this.operator = operator; 2540 this.answer = answer; 2541 } 2542 2543 /** 2544 * @return {@link #question} (The linkId for the question whose answer (or lack 2545 * of answer) governs whether this item is enabled.). This is the 2546 * underlying object with id, value and extensions. The accessor 2547 * "getQuestion" gives direct access to the value 2548 */ 2549 public StringType getQuestionElement() { 2550 if (this.question == null) 2551 if (Configuration.errorOnAutoCreate()) 2552 throw new Error("Attempt to auto-create QuestionnaireItemEnableWhenComponent.question"); 2553 else if (Configuration.doAutoCreate()) 2554 this.question = new StringType(); // bb 2555 return this.question; 2556 } 2557 2558 public boolean hasQuestionElement() { 2559 return this.question != null && !this.question.isEmpty(); 2560 } 2561 2562 public boolean hasQuestion() { 2563 return this.question != null && !this.question.isEmpty(); 2564 } 2565 2566 /** 2567 * @param value {@link #question} (The linkId for the question whose answer (or 2568 * lack of answer) governs whether this item is enabled.). This is 2569 * the underlying object with id, value and extensions. The 2570 * accessor "getQuestion" gives direct access to the value 2571 */ 2572 public QuestionnaireItemEnableWhenComponent setQuestionElement(StringType value) { 2573 this.question = value; 2574 return this; 2575 } 2576 2577 /** 2578 * @return The linkId for the question whose answer (or lack of answer) governs 2579 * whether this item is enabled. 2580 */ 2581 public String getQuestion() { 2582 return this.question == null ? null : this.question.getValue(); 2583 } 2584 2585 /** 2586 * @param value The linkId for the question whose answer (or lack of answer) 2587 * governs whether this item is enabled. 2588 */ 2589 public QuestionnaireItemEnableWhenComponent setQuestion(String value) { 2590 if (this.question == null) 2591 this.question = new StringType(); 2592 this.question.setValue(value); 2593 return this; 2594 } 2595 2596 /** 2597 * @return {@link #operator} (Specifies the criteria by which the question is 2598 * enabled.). This is the underlying object with id, value and 2599 * extensions. The accessor "getOperator" gives direct access to the 2600 * value 2601 */ 2602 public Enumeration<QuestionnaireItemOperator> getOperatorElement() { 2603 if (this.operator == null) 2604 if (Configuration.errorOnAutoCreate()) 2605 throw new Error("Attempt to auto-create QuestionnaireItemEnableWhenComponent.operator"); 2606 else if (Configuration.doAutoCreate()) 2607 this.operator = new Enumeration<QuestionnaireItemOperator>(new QuestionnaireItemOperatorEnumFactory()); // bb 2608 return this.operator; 2609 } 2610 2611 public boolean hasOperatorElement() { 2612 return this.operator != null && !this.operator.isEmpty(); 2613 } 2614 2615 public boolean hasOperator() { 2616 return this.operator != null && !this.operator.isEmpty(); 2617 } 2618 2619 /** 2620 * @param value {@link #operator} (Specifies the criteria by which the question 2621 * is enabled.). This is the underlying object with id, value and 2622 * extensions. The accessor "getOperator" gives direct access to 2623 * the value 2624 */ 2625 public QuestionnaireItemEnableWhenComponent setOperatorElement(Enumeration<QuestionnaireItemOperator> value) { 2626 this.operator = value; 2627 return this; 2628 } 2629 2630 /** 2631 * @return Specifies the criteria by which the question is enabled. 2632 */ 2633 public QuestionnaireItemOperator getOperator() { 2634 return this.operator == null ? null : this.operator.getValue(); 2635 } 2636 2637 /** 2638 * @param value Specifies the criteria by which the question is enabled. 2639 */ 2640 public QuestionnaireItemEnableWhenComponent setOperator(QuestionnaireItemOperator value) { 2641 if (this.operator == null) 2642 this.operator = new Enumeration<QuestionnaireItemOperator>(new QuestionnaireItemOperatorEnumFactory()); 2643 this.operator.setValue(value); 2644 return this; 2645 } 2646 2647 /** 2648 * @return {@link #answer} (A value that the referenced question is tested using 2649 * the specified operator in order for the item to be enabled.) 2650 */ 2651 public Type getAnswer() { 2652 return this.answer; 2653 } 2654 2655 /** 2656 * @return {@link #answer} (A value that the referenced question is tested using 2657 * the specified operator in order for the item to be enabled.) 2658 */ 2659 public BooleanType getAnswerBooleanType() throws FHIRException { 2660 if (this.answer == null) 2661 this.answer = new BooleanType(); 2662 if (!(this.answer instanceof BooleanType)) 2663 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 2664 + this.answer.getClass().getName() + " was encountered"); 2665 return (BooleanType) this.answer; 2666 } 2667 2668 public boolean hasAnswerBooleanType() { 2669 return this != null && this.answer instanceof BooleanType; 2670 } 2671 2672 /** 2673 * @return {@link #answer} (A value that the referenced question is tested using 2674 * the specified operator in order for the item to be enabled.) 2675 */ 2676 public DecimalType getAnswerDecimalType() throws FHIRException { 2677 if (this.answer == null) 2678 this.answer = new DecimalType(); 2679 if (!(this.answer instanceof DecimalType)) 2680 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 2681 + this.answer.getClass().getName() + " was encountered"); 2682 return (DecimalType) this.answer; 2683 } 2684 2685 public boolean hasAnswerDecimalType() { 2686 return this != null && this.answer instanceof DecimalType; 2687 } 2688 2689 /** 2690 * @return {@link #answer} (A value that the referenced question is tested using 2691 * the specified operator in order for the item to be enabled.) 2692 */ 2693 public IntegerType getAnswerIntegerType() throws FHIRException { 2694 if (this.answer == null) 2695 this.answer = new IntegerType(); 2696 if (!(this.answer instanceof IntegerType)) 2697 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 2698 + this.answer.getClass().getName() + " was encountered"); 2699 return (IntegerType) this.answer; 2700 } 2701 2702 public boolean hasAnswerIntegerType() { 2703 return this != null && this.answer instanceof IntegerType; 2704 } 2705 2706 /** 2707 * @return {@link #answer} (A value that the referenced question is tested using 2708 * the specified operator in order for the item to be enabled.) 2709 */ 2710 public DateType getAnswerDateType() throws FHIRException { 2711 if (this.answer == null) 2712 this.answer = new DateType(); 2713 if (!(this.answer instanceof DateType)) 2714 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.answer.getClass().getName() 2715 + " was encountered"); 2716 return (DateType) this.answer; 2717 } 2718 2719 public boolean hasAnswerDateType() { 2720 return this != null && this.answer instanceof DateType; 2721 } 2722 2723 /** 2724 * @return {@link #answer} (A value that the referenced question is tested using 2725 * the specified operator in order for the item to be enabled.) 2726 */ 2727 public DateTimeType getAnswerDateTimeType() throws FHIRException { 2728 if (this.answer == null) 2729 this.answer = new DateTimeType(); 2730 if (!(this.answer instanceof DateTimeType)) 2731 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2732 + this.answer.getClass().getName() + " was encountered"); 2733 return (DateTimeType) this.answer; 2734 } 2735 2736 public boolean hasAnswerDateTimeType() { 2737 return this != null && this.answer instanceof DateTimeType; 2738 } 2739 2740 /** 2741 * @return {@link #answer} (A value that the referenced question is tested using 2742 * the specified operator in order for the item to be enabled.) 2743 */ 2744 public TimeType getAnswerTimeType() throws FHIRException { 2745 if (this.answer == null) 2746 this.answer = new TimeType(); 2747 if (!(this.answer instanceof TimeType)) 2748 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.answer.getClass().getName() 2749 + " was encountered"); 2750 return (TimeType) this.answer; 2751 } 2752 2753 public boolean hasAnswerTimeType() { 2754 return this != null && this.answer instanceof TimeType; 2755 } 2756 2757 /** 2758 * @return {@link #answer} (A value that the referenced question is tested using 2759 * the specified operator in order for the item to be enabled.) 2760 */ 2761 public StringType getAnswerStringType() throws FHIRException { 2762 if (this.answer == null) 2763 this.answer = new StringType(); 2764 if (!(this.answer instanceof StringType)) 2765 throw new FHIRException("Type mismatch: the type StringType was expected, but " 2766 + this.answer.getClass().getName() + " was encountered"); 2767 return (StringType) this.answer; 2768 } 2769 2770 public boolean hasAnswerStringType() { 2771 return this != null && this.answer instanceof StringType; 2772 } 2773 2774 /** 2775 * @return {@link #answer} (A value that the referenced question is tested using 2776 * the specified operator in order for the item to be enabled.) 2777 */ 2778 public Coding getAnswerCoding() throws FHIRException { 2779 if (this.answer == null) 2780 this.answer = new Coding(); 2781 if (!(this.answer instanceof Coding)) 2782 throw new FHIRException("Type mismatch: the type Coding was expected, but " + this.answer.getClass().getName() 2783 + " was encountered"); 2784 return (Coding) this.answer; 2785 } 2786 2787 public boolean hasAnswerCoding() { 2788 return this != null && this.answer instanceof Coding; 2789 } 2790 2791 /** 2792 * @return {@link #answer} (A value that the referenced question is tested using 2793 * the specified operator in order for the item to be enabled.) 2794 */ 2795 public Quantity getAnswerQuantity() throws FHIRException { 2796 if (this.answer == null) 2797 this.answer = new Quantity(); 2798 if (!(this.answer instanceof Quantity)) 2799 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.answer.getClass().getName() 2800 + " was encountered"); 2801 return (Quantity) this.answer; 2802 } 2803 2804 public boolean hasAnswerQuantity() { 2805 return this != null && this.answer instanceof Quantity; 2806 } 2807 2808 /** 2809 * @return {@link #answer} (A value that the referenced question is tested using 2810 * the specified operator in order for the item to be enabled.) 2811 */ 2812 public Reference getAnswerReference() throws FHIRException { 2813 if (this.answer == null) 2814 this.answer = new Reference(); 2815 if (!(this.answer instanceof Reference)) 2816 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2817 + this.answer.getClass().getName() + " was encountered"); 2818 return (Reference) this.answer; 2819 } 2820 2821 public boolean hasAnswerReference() { 2822 return this != null && this.answer instanceof Reference; 2823 } 2824 2825 public boolean hasAnswer() { 2826 return this.answer != null && !this.answer.isEmpty(); 2827 } 2828 2829 /** 2830 * @param value {@link #answer} (A value that the referenced question is tested 2831 * using the specified operator in order for the item to be 2832 * enabled.) 2833 */ 2834 public QuestionnaireItemEnableWhenComponent setAnswer(Type value) { 2835 if (value != null && !(value instanceof BooleanType || value instanceof DecimalType 2836 || value instanceof IntegerType || value instanceof DateType || value instanceof DateTimeType 2837 || value instanceof TimeType || value instanceof StringType || value instanceof Coding 2838 || value instanceof Quantity || value instanceof Reference)) 2839 throw new Error("Not the right type for Questionnaire.item.enableWhen.answer[x]: " + value.fhirType()); 2840 this.answer = value; 2841 return this; 2842 } 2843 2844 protected void listChildren(List<Property> children) { 2845 super.listChildren(children); 2846 children.add(new Property("question", "string", 2847 "The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.", 0, 1, 2848 question)); 2849 children.add( 2850 new Property("operator", "code", "Specifies the criteria by which the question is enabled.", 0, 1, operator)); 2851 children.add(new Property("answer[x]", 2852 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2853 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2854 0, 1, answer)); 2855 } 2856 2857 @Override 2858 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2859 switch (_hash) { 2860 case -1165870106: 2861 /* question */ return new Property("question", "string", 2862 "The linkId for the question whose answer (or lack of answer) governs whether this item is enabled.", 0, 1, 2863 question); 2864 case -500553564: 2865 /* operator */ return new Property("operator", "code", 2866 "Specifies the criteria by which the question is enabled.", 0, 1, operator); 2867 case 1693524994: 2868 /* answer[x] */ return new Property("answer[x]", 2869 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2870 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2871 0, 1, answer); 2872 case -1412808770: 2873 /* answer */ return new Property("answer[x]", 2874 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2875 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2876 0, 1, answer); 2877 case 1194603146: 2878 /* answerBoolean */ return new Property("answer[x]", 2879 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2880 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2881 0, 1, answer); 2882 case -1622812237: 2883 /* answerDecimal */ return new Property("answer[x]", 2884 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2885 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2886 0, 1, answer); 2887 case -1207023712: 2888 /* answerInteger */ return new Property("answer[x]", 2889 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2890 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2891 0, 1, answer); 2892 case 958960780: 2893 /* answerDate */ return new Property("answer[x]", 2894 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2895 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2896 0, 1, answer); 2897 case -1835321991: 2898 /* answerDateTime */ return new Property("answer[x]", 2899 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2900 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2901 0, 1, answer); 2902 case 959444907: 2903 /* answerTime */ return new Property("answer[x]", 2904 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2905 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2906 0, 1, answer); 2907 case -1409727121: 2908 /* answerString */ return new Property("answer[x]", 2909 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2910 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2911 0, 1, answer); 2912 case -1872828216: 2913 /* answerCoding */ return new Property("answer[x]", 2914 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2915 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2916 0, 1, answer); 2917 case -618108311: 2918 /* answerQuantity */ return new Property("answer[x]", 2919 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2920 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2921 0, 1, answer); 2922 case -1726221011: 2923 /* answerReference */ return new Property("answer[x]", 2924 "boolean|decimal|integer|date|dateTime|time|string|Coding|Quantity|Reference(Any)", 2925 "A value that the referenced question is tested using the specified operator in order for the item to be enabled.", 2926 0, 1, answer); 2927 default: 2928 return super.getNamedProperty(_hash, _name, _checkValid); 2929 } 2930 2931 } 2932 2933 @Override 2934 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2935 switch (hash) { 2936 case -1165870106: 2937 /* question */ return this.question == null ? new Base[0] : new Base[] { this.question }; // StringType 2938 case -500553564: 2939 /* operator */ return this.operator == null ? new Base[0] : new Base[] { this.operator }; // Enumeration<QuestionnaireItemOperator> 2940 case -1412808770: 2941 /* answer */ return this.answer == null ? new Base[0] : new Base[] { this.answer }; // Type 2942 default: 2943 return super.getProperty(hash, name, checkValid); 2944 } 2945 2946 } 2947 2948 @Override 2949 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2950 switch (hash) { 2951 case -1165870106: // question 2952 this.question = castToString(value); // StringType 2953 return value; 2954 case -500553564: // operator 2955 value = new QuestionnaireItemOperatorEnumFactory().fromType(castToCode(value)); 2956 this.operator = (Enumeration) value; // Enumeration<QuestionnaireItemOperator> 2957 return value; 2958 case -1412808770: // answer 2959 this.answer = castToType(value); // Type 2960 return value; 2961 default: 2962 return super.setProperty(hash, name, value); 2963 } 2964 2965 } 2966 2967 @Override 2968 public Base setProperty(String name, Base value) throws FHIRException { 2969 if (name.equals("question")) { 2970 this.question = castToString(value); // StringType 2971 } else if (name.equals("operator")) { 2972 value = new QuestionnaireItemOperatorEnumFactory().fromType(castToCode(value)); 2973 this.operator = (Enumeration) value; // Enumeration<QuestionnaireItemOperator> 2974 } else if (name.equals("answer[x]")) { 2975 this.answer = castToType(value); // Type 2976 } else 2977 return super.setProperty(name, value); 2978 return value; 2979 } 2980 2981 @Override 2982 public void removeChild(String name, Base value) throws FHIRException { 2983 if (name.equals("question")) { 2984 this.question = null; 2985 } else if (name.equals("operator")) { 2986 this.operator = null; 2987 } else if (name.equals("answer[x]")) { 2988 this.answer = null; 2989 } else 2990 super.removeChild(name, value); 2991 2992 } 2993 2994 @Override 2995 public Base makeProperty(int hash, String name) throws FHIRException { 2996 switch (hash) { 2997 case -1165870106: 2998 return getQuestionElement(); 2999 case -500553564: 3000 return getOperatorElement(); 3001 case 1693524994: 3002 return getAnswer(); 3003 case -1412808770: 3004 return getAnswer(); 3005 default: 3006 return super.makeProperty(hash, name); 3007 } 3008 3009 } 3010 3011 @Override 3012 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3013 switch (hash) { 3014 case -1165870106: 3015 /* question */ return new String[] { "string" }; 3016 case -500553564: 3017 /* operator */ return new String[] { "code" }; 3018 case -1412808770: 3019 /* answer */ return new String[] { "boolean", "decimal", "integer", "date", "dateTime", "time", "string", 3020 "Coding", "Quantity", "Reference" }; 3021 default: 3022 return super.getTypesForProperty(hash, name); 3023 } 3024 3025 } 3026 3027 @Override 3028 public Base addChild(String name) throws FHIRException { 3029 if (name.equals("question")) { 3030 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.question"); 3031 } else if (name.equals("operator")) { 3032 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.operator"); 3033 } else if (name.equals("answerBoolean")) { 3034 this.answer = new BooleanType(); 3035 return this.answer; 3036 } else if (name.equals("answerDecimal")) { 3037 this.answer = new DecimalType(); 3038 return this.answer; 3039 } else if (name.equals("answerInteger")) { 3040 this.answer = new IntegerType(); 3041 return this.answer; 3042 } else if (name.equals("answerDate")) { 3043 this.answer = new DateType(); 3044 return this.answer; 3045 } else if (name.equals("answerDateTime")) { 3046 this.answer = new DateTimeType(); 3047 return this.answer; 3048 } else if (name.equals("answerTime")) { 3049 this.answer = new TimeType(); 3050 return this.answer; 3051 } else if (name.equals("answerString")) { 3052 this.answer = new StringType(); 3053 return this.answer; 3054 } else if (name.equals("answerCoding")) { 3055 this.answer = new Coding(); 3056 return this.answer; 3057 } else if (name.equals("answerQuantity")) { 3058 this.answer = new Quantity(); 3059 return this.answer; 3060 } else if (name.equals("answerReference")) { 3061 this.answer = new Reference(); 3062 return this.answer; 3063 } else 3064 return super.addChild(name); 3065 } 3066 3067 public QuestionnaireItemEnableWhenComponent copy() { 3068 QuestionnaireItemEnableWhenComponent dst = new QuestionnaireItemEnableWhenComponent(); 3069 copyValues(dst); 3070 return dst; 3071 } 3072 3073 public void copyValues(QuestionnaireItemEnableWhenComponent dst) { 3074 super.copyValues(dst); 3075 dst.question = question == null ? null : question.copy(); 3076 dst.operator = operator == null ? null : operator.copy(); 3077 dst.answer = answer == null ? null : answer.copy(); 3078 } 3079 3080 @Override 3081 public boolean equalsDeep(Base other_) { 3082 if (!super.equalsDeep(other_)) 3083 return false; 3084 if (!(other_ instanceof QuestionnaireItemEnableWhenComponent)) 3085 return false; 3086 QuestionnaireItemEnableWhenComponent o = (QuestionnaireItemEnableWhenComponent) other_; 3087 return compareDeep(question, o.question, true) && compareDeep(operator, o.operator, true) 3088 && compareDeep(answer, o.answer, true); 3089 } 3090 3091 @Override 3092 public boolean equalsShallow(Base other_) { 3093 if (!super.equalsShallow(other_)) 3094 return false; 3095 if (!(other_ instanceof QuestionnaireItemEnableWhenComponent)) 3096 return false; 3097 QuestionnaireItemEnableWhenComponent o = (QuestionnaireItemEnableWhenComponent) other_; 3098 return compareValues(question, o.question, true) && compareValues(operator, o.operator, true); 3099 } 3100 3101 public boolean isEmpty() { 3102 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(question, operator, answer); 3103 } 3104 3105 public String fhirType() { 3106 return "Questionnaire.item.enableWhen"; 3107 3108 } 3109 3110 } 3111 3112 @Block() 3113 public static class QuestionnaireItemAnswerOptionComponent extends BackboneElement implements IBaseBackboneElement { 3114 /** 3115 * A potential answer that's allowed as the answer to this question. 3116 */ 3117 @Child(name = "value", type = { IntegerType.class, DateType.class, TimeType.class, StringType.class, Coding.class, 3118 Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3119 @Description(shortDefinition = "Answer value", formalDefinition = "A potential answer that's allowed as the answer to this question.") 3120 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-answers") 3121 protected Type value; 3122 3123 /** 3124 * Indicates whether the answer value is selected when the list of possible 3125 * answers is initially shown. 3126 */ 3127 @Child(name = "initialSelected", type = { 3128 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3129 @Description(shortDefinition = "Whether option is selected by default", formalDefinition = "Indicates whether the answer value is selected when the list of possible answers is initially shown.") 3130 protected BooleanType initialSelected; 3131 3132 private static final long serialVersionUID = 1703686148L; 3133 3134 /** 3135 * Constructor 3136 */ 3137 public QuestionnaireItemAnswerOptionComponent() { 3138 super(); 3139 } 3140 3141 /** 3142 * Constructor 3143 */ 3144 public QuestionnaireItemAnswerOptionComponent(Type value) { 3145 super(); 3146 this.value = value; 3147 } 3148 3149 /** 3150 * @return {@link #value} (A potential answer that's allowed as the answer to 3151 * this question.) 3152 */ 3153 public Type getValue() { 3154 return this.value; 3155 } 3156 3157 /** 3158 * @return {@link #value} (A potential answer that's allowed as the answer to 3159 * this question.) 3160 */ 3161 public IntegerType getValueIntegerType() throws FHIRException { 3162 if (this.value == null) 3163 this.value = new IntegerType(); 3164 if (!(this.value instanceof IntegerType)) 3165 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 3166 + this.value.getClass().getName() + " was encountered"); 3167 return (IntegerType) this.value; 3168 } 3169 3170 public boolean hasValueIntegerType() { 3171 return this != null && this.value instanceof IntegerType; 3172 } 3173 3174 /** 3175 * @return {@link #value} (A potential answer that's allowed as the answer to 3176 * this question.) 3177 */ 3178 public DateType getValueDateType() throws FHIRException { 3179 if (this.value == null) 3180 this.value = new DateType(); 3181 if (!(this.value instanceof DateType)) 3182 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.value.getClass().getName() 3183 + " was encountered"); 3184 return (DateType) this.value; 3185 } 3186 3187 public boolean hasValueDateType() { 3188 return this != null && this.value instanceof DateType; 3189 } 3190 3191 /** 3192 * @return {@link #value} (A potential answer that's allowed as the answer to 3193 * this question.) 3194 */ 3195 public TimeType getValueTimeType() throws FHIRException { 3196 if (this.value == null) 3197 this.value = new TimeType(); 3198 if (!(this.value instanceof TimeType)) 3199 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 3200 + " was encountered"); 3201 return (TimeType) this.value; 3202 } 3203 3204 public boolean hasValueTimeType() { 3205 return this != null && this.value instanceof TimeType; 3206 } 3207 3208 /** 3209 * @return {@link #value} (A potential answer that's allowed as the answer to 3210 * this question.) 3211 */ 3212 public StringType getValueStringType() throws FHIRException { 3213 if (this.value == null) 3214 this.value = new StringType(); 3215 if (!(this.value instanceof StringType)) 3216 throw new FHIRException("Type mismatch: the type StringType was expected, but " 3217 + this.value.getClass().getName() + " was encountered"); 3218 return (StringType) this.value; 3219 } 3220 3221 public boolean hasValueStringType() { 3222 return this != null && this.value instanceof StringType; 3223 } 3224 3225 /** 3226 * @return {@link #value} (A potential answer that's allowed as the answer to 3227 * this question.) 3228 */ 3229 public Coding getValueCoding() throws FHIRException { 3230 if (this.value == null) 3231 this.value = new Coding(); 3232 if (!(this.value instanceof Coding)) 3233 throw new FHIRException( 3234 "Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered"); 3235 return (Coding) this.value; 3236 } 3237 3238 public boolean hasValueCoding() { 3239 return this != null && this.value instanceof Coding; 3240 } 3241 3242 /** 3243 * @return {@link #value} (A potential answer that's allowed as the answer to 3244 * this question.) 3245 */ 3246 public Reference getValueReference() throws FHIRException { 3247 if (this.value == null) 3248 this.value = new Reference(); 3249 if (!(this.value instanceof Reference)) 3250 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 3251 + " was encountered"); 3252 return (Reference) this.value; 3253 } 3254 3255 public boolean hasValueReference() { 3256 return this != null && this.value instanceof Reference; 3257 } 3258 3259 public boolean hasValue() { 3260 return this.value != null && !this.value.isEmpty(); 3261 } 3262 3263 /** 3264 * @param value {@link #value} (A potential answer that's allowed as the answer 3265 * to this question.) 3266 */ 3267 public QuestionnaireItemAnswerOptionComponent setValue(Type value) { 3268 if (value != null && !(value instanceof IntegerType || value instanceof DateType || value instanceof TimeType 3269 || value instanceof StringType || value instanceof Coding || value instanceof Reference)) 3270 throw new Error("Not the right type for Questionnaire.item.answerOption.value[x]: " + value.fhirType()); 3271 this.value = value; 3272 return this; 3273 } 3274 3275 /** 3276 * @return {@link #initialSelected} (Indicates whether the answer value is 3277 * selected when the list of possible answers is initially shown.). This 3278 * is the underlying object with id, value and extensions. The accessor 3279 * "getInitialSelected" gives direct access to the value 3280 */ 3281 public BooleanType getInitialSelectedElement() { 3282 if (this.initialSelected == null) 3283 if (Configuration.errorOnAutoCreate()) 3284 throw new Error("Attempt to auto-create QuestionnaireItemAnswerOptionComponent.initialSelected"); 3285 else if (Configuration.doAutoCreate()) 3286 this.initialSelected = new BooleanType(); // bb 3287 return this.initialSelected; 3288 } 3289 3290 public boolean hasInitialSelectedElement() { 3291 return this.initialSelected != null && !this.initialSelected.isEmpty(); 3292 } 3293 3294 public boolean hasInitialSelected() { 3295 return this.initialSelected != null && !this.initialSelected.isEmpty(); 3296 } 3297 3298 /** 3299 * @param value {@link #initialSelected} (Indicates whether the answer value is 3300 * selected when the list of possible answers is initially shown.). 3301 * This is the underlying object with id, value and extensions. The 3302 * accessor "getInitialSelected" gives direct access to the value 3303 */ 3304 public QuestionnaireItemAnswerOptionComponent setInitialSelectedElement(BooleanType value) { 3305 this.initialSelected = value; 3306 return this; 3307 } 3308 3309 /** 3310 * @return Indicates whether the answer value is selected when the list of 3311 * possible answers is initially shown. 3312 */ 3313 public boolean getInitialSelected() { 3314 return this.initialSelected == null || this.initialSelected.isEmpty() ? false : this.initialSelected.getValue(); 3315 } 3316 3317 /** 3318 * @param value Indicates whether the answer value is selected when the list of 3319 * possible answers is initially shown. 3320 */ 3321 public QuestionnaireItemAnswerOptionComponent setInitialSelected(boolean value) { 3322 if (this.initialSelected == null) 3323 this.initialSelected = new BooleanType(); 3324 this.initialSelected.setValue(value); 3325 return this; 3326 } 3327 3328 protected void listChildren(List<Property> children) { 3329 super.listChildren(children); 3330 children.add(new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3331 "A potential answer that's allowed as the answer to this question.", 0, 1, value)); 3332 children.add(new Property("initialSelected", "boolean", 3333 "Indicates whether the answer value is selected when the list of possible answers is initially shown.", 0, 1, 3334 initialSelected)); 3335 } 3336 3337 @Override 3338 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3339 switch (_hash) { 3340 case -1410166417: 3341 /* value[x] */ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3342 "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3343 case 111972721: 3344 /* value */ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3345 "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3346 case -1668204915: 3347 /* valueInteger */ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3348 "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3349 case -766192449: 3350 /* valueDate */ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3351 "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3352 case -765708322: 3353 /* valueTime */ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3354 "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3355 case -1424603934: 3356 /* valueString */ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3357 "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3358 case -1887705029: 3359 /* valueCoding */ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3360 "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3361 case 1755241690: 3362 /* valueReference */ return new Property("value[x]", "integer|date|time|string|Coding|Reference(Any)", 3363 "A potential answer that's allowed as the answer to this question.", 0, 1, value); 3364 case -1310184961: 3365 /* initialSelected */ return new Property("initialSelected", "boolean", 3366 "Indicates whether the answer value is selected when the list of possible answers is initially shown.", 0, 3367 1, initialSelected); 3368 default: 3369 return super.getNamedProperty(_hash, _name, _checkValid); 3370 } 3371 3372 } 3373 3374 @Override 3375 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3376 switch (hash) { 3377 case 111972721: 3378 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3379 case -1310184961: 3380 /* initialSelected */ return this.initialSelected == null ? new Base[0] : new Base[] { this.initialSelected }; // BooleanType 3381 default: 3382 return super.getProperty(hash, name, checkValid); 3383 } 3384 3385 } 3386 3387 @Override 3388 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3389 switch (hash) { 3390 case 111972721: // value 3391 this.value = castToType(value); // Type 3392 return value; 3393 case -1310184961: // initialSelected 3394 this.initialSelected = castToBoolean(value); // BooleanType 3395 return value; 3396 default: 3397 return super.setProperty(hash, name, value); 3398 } 3399 3400 } 3401 3402 @Override 3403 public Base setProperty(String name, Base value) throws FHIRException { 3404 if (name.equals("value[x]")) { 3405 this.value = castToType(value); // Type 3406 } else if (name.equals("initialSelected")) { 3407 this.initialSelected = castToBoolean(value); // BooleanType 3408 } else 3409 return super.setProperty(name, value); 3410 return value; 3411 } 3412 3413 @Override 3414 public void removeChild(String name, Base value) throws FHIRException { 3415 if (name.equals("value[x]")) { 3416 this.value = null; 3417 } else if (name.equals("initialSelected")) { 3418 this.initialSelected = null; 3419 } else 3420 super.removeChild(name, value); 3421 3422 } 3423 3424 @Override 3425 public Base makeProperty(int hash, String name) throws FHIRException { 3426 switch (hash) { 3427 case -1410166417: 3428 return getValue(); 3429 case 111972721: 3430 return getValue(); 3431 case -1310184961: 3432 return getInitialSelectedElement(); 3433 default: 3434 return super.makeProperty(hash, name); 3435 } 3436 3437 } 3438 3439 @Override 3440 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3441 switch (hash) { 3442 case 111972721: 3443 /* value */ return new String[] { "integer", "date", "time", "string", "Coding", "Reference" }; 3444 case -1310184961: 3445 /* initialSelected */ return new String[] { "boolean" }; 3446 default: 3447 return super.getTypesForProperty(hash, name); 3448 } 3449 3450 } 3451 3452 @Override 3453 public Base addChild(String name) throws FHIRException { 3454 if (name.equals("valueInteger")) { 3455 this.value = new IntegerType(); 3456 return this.value; 3457 } else if (name.equals("valueDate")) { 3458 this.value = new DateType(); 3459 return this.value; 3460 } else if (name.equals("valueTime")) { 3461 this.value = new TimeType(); 3462 return this.value; 3463 } else if (name.equals("valueString")) { 3464 this.value = new StringType(); 3465 return this.value; 3466 } else if (name.equals("valueCoding")) { 3467 this.value = new Coding(); 3468 return this.value; 3469 } else if (name.equals("valueReference")) { 3470 this.value = new Reference(); 3471 return this.value; 3472 } else if (name.equals("initialSelected")) { 3473 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.initialSelected"); 3474 } else 3475 return super.addChild(name); 3476 } 3477 3478 public QuestionnaireItemAnswerOptionComponent copy() { 3479 QuestionnaireItemAnswerOptionComponent dst = new QuestionnaireItemAnswerOptionComponent(); 3480 copyValues(dst); 3481 return dst; 3482 } 3483 3484 public void copyValues(QuestionnaireItemAnswerOptionComponent dst) { 3485 super.copyValues(dst); 3486 dst.value = value == null ? null : value.copy(); 3487 dst.initialSelected = initialSelected == null ? null : initialSelected.copy(); 3488 } 3489 3490 @Override 3491 public boolean equalsDeep(Base other_) { 3492 if (!super.equalsDeep(other_)) 3493 return false; 3494 if (!(other_ instanceof QuestionnaireItemAnswerOptionComponent)) 3495 return false; 3496 QuestionnaireItemAnswerOptionComponent o = (QuestionnaireItemAnswerOptionComponent) other_; 3497 return compareDeep(value, o.value, true) && compareDeep(initialSelected, o.initialSelected, true); 3498 } 3499 3500 @Override 3501 public boolean equalsShallow(Base other_) { 3502 if (!super.equalsShallow(other_)) 3503 return false; 3504 if (!(other_ instanceof QuestionnaireItemAnswerOptionComponent)) 3505 return false; 3506 QuestionnaireItemAnswerOptionComponent o = (QuestionnaireItemAnswerOptionComponent) other_; 3507 return compareValues(initialSelected, o.initialSelected, true); 3508 } 3509 3510 public boolean isEmpty() { 3511 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, initialSelected); 3512 } 3513 3514 public String fhirType() { 3515 return "Questionnaire.item.answerOption"; 3516 3517 } 3518 3519 } 3520 3521 @Block() 3522 public static class QuestionnaireItemInitialComponent extends BackboneElement implements IBaseBackboneElement { 3523 /** 3524 * The actual value to for an initial answer. 3525 */ 3526 @Child(name = "value", type = { BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, 3527 DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, 3528 Quantity.class, Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3529 @Description(shortDefinition = "Actual value for initializing the question", formalDefinition = "The actual value to for an initial answer.") 3530 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-answers") 3531 protected Type value; 3532 3533 private static final long serialVersionUID = -732981989L; 3534 3535 /** 3536 * Constructor 3537 */ 3538 public QuestionnaireItemInitialComponent() { 3539 super(); 3540 } 3541 3542 /** 3543 * Constructor 3544 */ 3545 public QuestionnaireItemInitialComponent(Type value) { 3546 super(); 3547 this.value = value; 3548 } 3549 3550 /** 3551 * @return {@link #value} (The actual value to for an initial answer.) 3552 */ 3553 public Type getValue() { 3554 return this.value; 3555 } 3556 3557 /** 3558 * @return {@link #value} (The actual value to for an initial answer.) 3559 */ 3560 public BooleanType getValueBooleanType() throws FHIRException { 3561 if (this.value == null) 3562 this.value = new BooleanType(); 3563 if (!(this.value instanceof BooleanType)) 3564 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 3565 + this.value.getClass().getName() + " was encountered"); 3566 return (BooleanType) this.value; 3567 } 3568 3569 public boolean hasValueBooleanType() { 3570 return this != null && this.value instanceof BooleanType; 3571 } 3572 3573 /** 3574 * @return {@link #value} (The actual value to for an initial answer.) 3575 */ 3576 public DecimalType getValueDecimalType() throws FHIRException { 3577 if (this.value == null) 3578 this.value = new DecimalType(); 3579 if (!(this.value instanceof DecimalType)) 3580 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 3581 + this.value.getClass().getName() + " was encountered"); 3582 return (DecimalType) this.value; 3583 } 3584 3585 public boolean hasValueDecimalType() { 3586 return this != null && this.value instanceof DecimalType; 3587 } 3588 3589 /** 3590 * @return {@link #value} (The actual value to for an initial answer.) 3591 */ 3592 public IntegerType getValueIntegerType() throws FHIRException { 3593 if (this.value == null) 3594 this.value = new IntegerType(); 3595 if (!(this.value instanceof IntegerType)) 3596 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 3597 + this.value.getClass().getName() + " was encountered"); 3598 return (IntegerType) this.value; 3599 } 3600 3601 public boolean hasValueIntegerType() { 3602 return this != null && this.value instanceof IntegerType; 3603 } 3604 3605 /** 3606 * @return {@link #value} (The actual value to for an initial answer.) 3607 */ 3608 public DateType getValueDateType() throws FHIRException { 3609 if (this.value == null) 3610 this.value = new DateType(); 3611 if (!(this.value instanceof DateType)) 3612 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.value.getClass().getName() 3613 + " was encountered"); 3614 return (DateType) this.value; 3615 } 3616 3617 public boolean hasValueDateType() { 3618 return this != null && this.value instanceof DateType; 3619 } 3620 3621 /** 3622 * @return {@link #value} (The actual value to for an initial answer.) 3623 */ 3624 public DateTimeType getValueDateTimeType() throws FHIRException { 3625 if (this.value == null) 3626 this.value = new DateTimeType(); 3627 if (!(this.value instanceof DateTimeType)) 3628 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 3629 + this.value.getClass().getName() + " was encountered"); 3630 return (DateTimeType) this.value; 3631 } 3632 3633 public boolean hasValueDateTimeType() { 3634 return this != null && this.value instanceof DateTimeType; 3635 } 3636 3637 /** 3638 * @return {@link #value} (The actual value to for an initial answer.) 3639 */ 3640 public TimeType getValueTimeType() throws FHIRException { 3641 if (this.value == null) 3642 this.value = new TimeType(); 3643 if (!(this.value instanceof TimeType)) 3644 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 3645 + " was encountered"); 3646 return (TimeType) this.value; 3647 } 3648 3649 public boolean hasValueTimeType() { 3650 return this != null && this.value instanceof TimeType; 3651 } 3652 3653 /** 3654 * @return {@link #value} (The actual value to for an initial answer.) 3655 */ 3656 public StringType getValueStringType() throws FHIRException { 3657 if (this.value == null) 3658 this.value = new StringType(); 3659 if (!(this.value instanceof StringType)) 3660 throw new FHIRException("Type mismatch: the type StringType was expected, but " 3661 + this.value.getClass().getName() + " was encountered"); 3662 return (StringType) this.value; 3663 } 3664 3665 public boolean hasValueStringType() { 3666 return this != null && this.value instanceof StringType; 3667 } 3668 3669 /** 3670 * @return {@link #value} (The actual value to for an initial answer.) 3671 */ 3672 public UriType getValueUriType() throws FHIRException { 3673 if (this.value == null) 3674 this.value = new UriType(); 3675 if (!(this.value instanceof UriType)) 3676 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName() 3677 + " was encountered"); 3678 return (UriType) this.value; 3679 } 3680 3681 public boolean hasValueUriType() { 3682 return this != null && this.value instanceof UriType; 3683 } 3684 3685 /** 3686 * @return {@link #value} (The actual value to for an initial answer.) 3687 */ 3688 public Attachment getValueAttachment() throws FHIRException { 3689 if (this.value == null) 3690 this.value = new Attachment(); 3691 if (!(this.value instanceof Attachment)) 3692 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 3693 + this.value.getClass().getName() + " was encountered"); 3694 return (Attachment) this.value; 3695 } 3696 3697 public boolean hasValueAttachment() { 3698 return this != null && this.value instanceof Attachment; 3699 } 3700 3701 /** 3702 * @return {@link #value} (The actual value to for an initial answer.) 3703 */ 3704 public Coding getValueCoding() throws FHIRException { 3705 if (this.value == null) 3706 this.value = new Coding(); 3707 if (!(this.value instanceof Coding)) 3708 throw new FHIRException( 3709 "Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered"); 3710 return (Coding) this.value; 3711 } 3712 3713 public boolean hasValueCoding() { 3714 return this != null && this.value instanceof Coding; 3715 } 3716 3717 /** 3718 * @return {@link #value} (The actual value to for an initial answer.) 3719 */ 3720 public Quantity getValueQuantity() throws FHIRException { 3721 if (this.value == null) 3722 this.value = new Quantity(); 3723 if (!(this.value instanceof Quantity)) 3724 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 3725 + " was encountered"); 3726 return (Quantity) this.value; 3727 } 3728 3729 public boolean hasValueQuantity() { 3730 return this != null && this.value instanceof Quantity; 3731 } 3732 3733 /** 3734 * @return {@link #value} (The actual value to for an initial answer.) 3735 */ 3736 public Reference getValueReference() throws FHIRException { 3737 if (this.value == null) 3738 this.value = new Reference(); 3739 if (!(this.value instanceof Reference)) 3740 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 3741 + " was encountered"); 3742 return (Reference) this.value; 3743 } 3744 3745 public boolean hasValueReference() { 3746 return this != null && this.value instanceof Reference; 3747 } 3748 3749 public boolean hasValue() { 3750 return this.value != null && !this.value.isEmpty(); 3751 } 3752 3753 /** 3754 * @param value {@link #value} (The actual value to for an initial answer.) 3755 */ 3756 public QuestionnaireItemInitialComponent setValue(Type value) { 3757 if (value != null 3758 && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType 3759 || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType 3760 || value instanceof StringType || value instanceof UriType || value instanceof Attachment 3761 || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 3762 throw new Error("Not the right type for Questionnaire.item.initial.value[x]: " + value.fhirType()); 3763 this.value = value; 3764 return this; 3765 } 3766 3767 protected void listChildren(List<Property> children) { 3768 super.listChildren(children); 3769 children.add(new Property("value[x]", 3770 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3771 "The actual value to for an initial answer.", 0, 1, value)); 3772 } 3773 3774 @Override 3775 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3776 switch (_hash) { 3777 case -1410166417: 3778 /* value[x] */ return new Property("value[x]", 3779 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3780 "The actual value to for an initial answer.", 0, 1, value); 3781 case 111972721: 3782 /* value */ return new Property("value[x]", 3783 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3784 "The actual value to for an initial answer.", 0, 1, value); 3785 case 733421943: 3786 /* valueBoolean */ return new Property("value[x]", 3787 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3788 "The actual value to for an initial answer.", 0, 1, value); 3789 case -2083993440: 3790 /* valueDecimal */ return new Property("value[x]", 3791 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3792 "The actual value to for an initial answer.", 0, 1, value); 3793 case -1668204915: 3794 /* valueInteger */ return new Property("value[x]", 3795 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3796 "The actual value to for an initial answer.", 0, 1, value); 3797 case -766192449: 3798 /* valueDate */ return new Property("value[x]", 3799 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3800 "The actual value to for an initial answer.", 0, 1, value); 3801 case 1047929900: 3802 /* valueDateTime */ return new Property("value[x]", 3803 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3804 "The actual value to for an initial answer.", 0, 1, value); 3805 case -765708322: 3806 /* valueTime */ return new Property("value[x]", 3807 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3808 "The actual value to for an initial answer.", 0, 1, value); 3809 case -1424603934: 3810 /* valueString */ return new Property("value[x]", 3811 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3812 "The actual value to for an initial answer.", 0, 1, value); 3813 case -1410172357: 3814 /* valueUri */ return new Property("value[x]", 3815 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3816 "The actual value to for an initial answer.", 0, 1, value); 3817 case -475566732: 3818 /* valueAttachment */ return new Property("value[x]", 3819 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3820 "The actual value to for an initial answer.", 0, 1, value); 3821 case -1887705029: 3822 /* valueCoding */ return new Property("value[x]", 3823 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3824 "The actual value to for an initial answer.", 0, 1, value); 3825 case -2029823716: 3826 /* valueQuantity */ return new Property("value[x]", 3827 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3828 "The actual value to for an initial answer.", 0, 1, value); 3829 case 1755241690: 3830 /* valueReference */ return new Property("value[x]", 3831 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 3832 "The actual value to for an initial answer.", 0, 1, value); 3833 default: 3834 return super.getNamedProperty(_hash, _name, _checkValid); 3835 } 3836 3837 } 3838 3839 @Override 3840 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3841 switch (hash) { 3842 case 111972721: 3843 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3844 default: 3845 return super.getProperty(hash, name, checkValid); 3846 } 3847 3848 } 3849 3850 @Override 3851 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3852 switch (hash) { 3853 case 111972721: // value 3854 this.value = castToType(value); // Type 3855 return value; 3856 default: 3857 return super.setProperty(hash, name, value); 3858 } 3859 3860 } 3861 3862 @Override 3863 public Base setProperty(String name, Base value) throws FHIRException { 3864 if (name.equals("value[x]")) { 3865 this.value = castToType(value); // Type 3866 } else 3867 return super.setProperty(name, value); 3868 return value; 3869 } 3870 3871 @Override 3872 public void removeChild(String name, Base value) throws FHIRException { 3873 if (name.equals("value[x]")) { 3874 this.value = null; 3875 } else 3876 super.removeChild(name, value); 3877 3878 } 3879 3880 @Override 3881 public Base makeProperty(int hash, String name) throws FHIRException { 3882 switch (hash) { 3883 case -1410166417: 3884 return getValue(); 3885 case 111972721: 3886 return getValue(); 3887 default: 3888 return super.makeProperty(hash, name); 3889 } 3890 3891 } 3892 3893 @Override 3894 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3895 switch (hash) { 3896 case 111972721: 3897 /* value */ return new String[] { "boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", 3898 "Attachment", "Coding", "Quantity", "Reference" }; 3899 default: 3900 return super.getTypesForProperty(hash, name); 3901 } 3902 3903 } 3904 3905 @Override 3906 public Base addChild(String name) throws FHIRException { 3907 if (name.equals("valueBoolean")) { 3908 this.value = new BooleanType(); 3909 return this.value; 3910 } else if (name.equals("valueDecimal")) { 3911 this.value = new DecimalType(); 3912 return this.value; 3913 } else if (name.equals("valueInteger")) { 3914 this.value = new IntegerType(); 3915 return this.value; 3916 } else if (name.equals("valueDate")) { 3917 this.value = new DateType(); 3918 return this.value; 3919 } else if (name.equals("valueDateTime")) { 3920 this.value = new DateTimeType(); 3921 return this.value; 3922 } else if (name.equals("valueTime")) { 3923 this.value = new TimeType(); 3924 return this.value; 3925 } else if (name.equals("valueString")) { 3926 this.value = new StringType(); 3927 return this.value; 3928 } else if (name.equals("valueUri")) { 3929 this.value = new UriType(); 3930 return this.value; 3931 } else if (name.equals("valueAttachment")) { 3932 this.value = new Attachment(); 3933 return this.value; 3934 } else if (name.equals("valueCoding")) { 3935 this.value = new Coding(); 3936 return this.value; 3937 } else if (name.equals("valueQuantity")) { 3938 this.value = new Quantity(); 3939 return this.value; 3940 } else if (name.equals("valueReference")) { 3941 this.value = new Reference(); 3942 return this.value; 3943 } else 3944 return super.addChild(name); 3945 } 3946 3947 public QuestionnaireItemInitialComponent copy() { 3948 QuestionnaireItemInitialComponent dst = new QuestionnaireItemInitialComponent(); 3949 copyValues(dst); 3950 return dst; 3951 } 3952 3953 public void copyValues(QuestionnaireItemInitialComponent dst) { 3954 super.copyValues(dst); 3955 dst.value = value == null ? null : value.copy(); 3956 } 3957 3958 @Override 3959 public boolean equalsDeep(Base other_) { 3960 if (!super.equalsDeep(other_)) 3961 return false; 3962 if (!(other_ instanceof QuestionnaireItemInitialComponent)) 3963 return false; 3964 QuestionnaireItemInitialComponent o = (QuestionnaireItemInitialComponent) other_; 3965 return compareDeep(value, o.value, true); 3966 } 3967 3968 @Override 3969 public boolean equalsShallow(Base other_) { 3970 if (!super.equalsShallow(other_)) 3971 return false; 3972 if (!(other_ instanceof QuestionnaireItemInitialComponent)) 3973 return false; 3974 QuestionnaireItemInitialComponent o = (QuestionnaireItemInitialComponent) other_; 3975 return true; 3976 } 3977 3978 public boolean isEmpty() { 3979 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 3980 } 3981 3982 public String fhirType() { 3983 return "Questionnaire.item.initial"; 3984 3985 } 3986 3987 } 3988 3989 /** 3990 * A formal identifier that is used to identify this questionnaire when it is 3991 * represented in other formats, or referenced in a specification, model, design 3992 * or an instance. 3993 */ 3994 @Child(name = "identifier", type = { 3995 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3996 @Description(shortDefinition = "Additional identifier for the questionnaire", formalDefinition = "A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.") 3997 protected List<Identifier> identifier; 3998 3999 /** 4000 * The URL of a Questionnaire that this Questionnaire is based on. 4001 */ 4002 @Child(name = "derivedFrom", type = { 4003 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4004 @Description(shortDefinition = "Instantiates protocol or definition", formalDefinition = "The URL of a Questionnaire that this Questionnaire is based on.") 4005 protected List<CanonicalType> derivedFrom; 4006 4007 /** 4008 * The types of subjects that can be the subject of responses created for the 4009 * questionnaire. 4010 */ 4011 @Child(name = "subjectType", type = { 4012 CodeType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4013 @Description(shortDefinition = "Resource that can be subject of QuestionnaireResponse", formalDefinition = "The types of subjects that can be the subject of responses created for the questionnaire.") 4014 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 4015 protected List<CodeType> subjectType; 4016 4017 /** 4018 * Explanation of why this questionnaire is needed and why it has been designed 4019 * as it has. 4020 */ 4021 @Child(name = "purpose", type = { 4022 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4023 @Description(shortDefinition = "Why this questionnaire is defined", formalDefinition = "Explanation of why this questionnaire is needed and why it has been designed as it has.") 4024 protected MarkdownType purpose; 4025 4026 /** 4027 * A copyright statement relating to the questionnaire and/or its contents. 4028 * Copyright statements are generally legal restrictions on the use and 4029 * publishing of the questionnaire. 4030 */ 4031 @Child(name = "copyright", type = { 4032 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 4033 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.") 4034 protected MarkdownType copyright; 4035 4036 /** 4037 * The date on which the resource content was approved by the publisher. 4038 * Approval happens once when the content is officially approved for usage. 4039 */ 4040 @Child(name = "approvalDate", type = { 4041 DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 4042 @Description(shortDefinition = "When the questionnaire was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 4043 protected DateType approvalDate; 4044 4045 /** 4046 * The date on which the resource content was last reviewed. Review happens 4047 * periodically after approval but does not change the original approval date. 4048 */ 4049 @Child(name = "lastReviewDate", type = { 4050 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 4051 @Description(shortDefinition = "When the questionnaire was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 4052 protected DateType lastReviewDate; 4053 4054 /** 4055 * The period during which the questionnaire content was or is planned to be in 4056 * active use. 4057 */ 4058 @Child(name = "effectivePeriod", type = { 4059 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 4060 @Description(shortDefinition = "When the questionnaire is expected to be used", formalDefinition = "The period during which the questionnaire content was or is planned to be in active use.") 4061 protected Period effectivePeriod; 4062 4063 /** 4064 * An identifier for this question or group of questions in a particular 4065 * terminology such as LOINC. 4066 */ 4067 @Child(name = "code", type = { 4068 Coding.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4069 @Description(shortDefinition = "Concept that represents the overall questionnaire", formalDefinition = "An identifier for this question or group of questions in a particular terminology such as LOINC.") 4070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-questions") 4071 protected List<Coding> code; 4072 4073 /** 4074 * A particular question, question grouping or display text that is part of the 4075 * questionnaire. 4076 */ 4077 @Child(name = "item", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4078 @Description(shortDefinition = "Questions and sections within the Questionnaire", formalDefinition = "A particular question, question grouping or display text that is part of the questionnaire.") 4079 protected List<QuestionnaireItemComponent> item; 4080 4081 private static final long serialVersionUID = 1036031192L; 4082 4083 /** 4084 * Constructor 4085 */ 4086 public Questionnaire() { 4087 super(); 4088 } 4089 4090 /** 4091 * Constructor 4092 */ 4093 public Questionnaire(Enumeration<PublicationStatus> status) { 4094 super(); 4095 this.status = status; 4096 } 4097 4098 /** 4099 * @return {@link #url} (An absolute URI that is used to identify this 4100 * questionnaire when it is referenced in a specification, model, design 4101 * or an instance; also called its canonical identifier. This SHOULD be 4102 * globally unique and SHOULD be a literal address at which at which an 4103 * authoritative instance of this questionnaire is (or will be) 4104 * published. This URL can be the target of a canonical reference. It 4105 * SHALL remain the same when the questionnaire is stored on different 4106 * servers.). This is the underlying object with id, value and 4107 * extensions. The accessor "getUrl" gives direct access to the value 4108 */ 4109 public UriType getUrlElement() { 4110 if (this.url == null) 4111 if (Configuration.errorOnAutoCreate()) 4112 throw new Error("Attempt to auto-create Questionnaire.url"); 4113 else if (Configuration.doAutoCreate()) 4114 this.url = new UriType(); // bb 4115 return this.url; 4116 } 4117 4118 public boolean hasUrlElement() { 4119 return this.url != null && !this.url.isEmpty(); 4120 } 4121 4122 public boolean hasUrl() { 4123 return this.url != null && !this.url.isEmpty(); 4124 } 4125 4126 /** 4127 * @param value {@link #url} (An absolute URI that is used to identify this 4128 * questionnaire when it is referenced in a specification, model, 4129 * design or an instance; also called its canonical identifier. 4130 * This SHOULD be globally unique and SHOULD be a literal address 4131 * at which at which an authoritative instance of this 4132 * questionnaire is (or will be) published. This URL can be the 4133 * target of a canonical reference. It SHALL remain the same when 4134 * the questionnaire is stored on different servers.). This is the 4135 * underlying object with id, value and extensions. The accessor 4136 * "getUrl" gives direct access to the value 4137 */ 4138 public Questionnaire setUrlElement(UriType value) { 4139 this.url = value; 4140 return this; 4141 } 4142 4143 /** 4144 * @return An absolute URI that is used to identify this questionnaire when it 4145 * is referenced in a specification, model, design or an instance; also 4146 * called its canonical identifier. This SHOULD be globally unique and 4147 * SHOULD be a literal address at which at which an authoritative 4148 * instance of this questionnaire is (or will be) published. This URL 4149 * can be the target of a canonical reference. It SHALL remain the same 4150 * when the questionnaire is stored on different servers. 4151 */ 4152 public String getUrl() { 4153 return this.url == null ? null : this.url.getValue(); 4154 } 4155 4156 /** 4157 * @param value An absolute URI that is used to identify this questionnaire when 4158 * it is referenced in a specification, model, design or an 4159 * instance; also called its canonical identifier. This SHOULD be 4160 * globally unique and SHOULD be a literal address at which at 4161 * which an authoritative instance of this questionnaire is (or 4162 * will be) published. This URL can be the target of a canonical 4163 * reference. It SHALL remain the same when the questionnaire is 4164 * stored on different servers. 4165 */ 4166 public Questionnaire setUrl(String value) { 4167 if (Utilities.noString(value)) 4168 this.url = null; 4169 else { 4170 if (this.url == null) 4171 this.url = new UriType(); 4172 this.url.setValue(value); 4173 } 4174 return this; 4175 } 4176 4177 /** 4178 * @return {@link #identifier} (A formal identifier that is used to identify 4179 * this questionnaire when it is represented in other formats, or 4180 * referenced in a specification, model, design or an instance.) 4181 */ 4182 public List<Identifier> getIdentifier() { 4183 if (this.identifier == null) 4184 this.identifier = new ArrayList<Identifier>(); 4185 return this.identifier; 4186 } 4187 4188 /** 4189 * @return Returns a reference to <code>this</code> for easy method chaining 4190 */ 4191 public Questionnaire setIdentifier(List<Identifier> theIdentifier) { 4192 this.identifier = theIdentifier; 4193 return this; 4194 } 4195 4196 public boolean hasIdentifier() { 4197 if (this.identifier == null) 4198 return false; 4199 for (Identifier item : this.identifier) 4200 if (!item.isEmpty()) 4201 return true; 4202 return false; 4203 } 4204 4205 public Identifier addIdentifier() { // 3 4206 Identifier t = new Identifier(); 4207 if (this.identifier == null) 4208 this.identifier = new ArrayList<Identifier>(); 4209 this.identifier.add(t); 4210 return t; 4211 } 4212 4213 public Questionnaire addIdentifier(Identifier t) { // 3 4214 if (t == null) 4215 return this; 4216 if (this.identifier == null) 4217 this.identifier = new ArrayList<Identifier>(); 4218 this.identifier.add(t); 4219 return this; 4220 } 4221 4222 /** 4223 * @return The first repetition of repeating field {@link #identifier}, creating 4224 * it if it does not already exist 4225 */ 4226 public Identifier getIdentifierFirstRep() { 4227 if (getIdentifier().isEmpty()) { 4228 addIdentifier(); 4229 } 4230 return getIdentifier().get(0); 4231 } 4232 4233 /** 4234 * @return {@link #version} (The identifier that is used to identify this 4235 * version of the questionnaire when it is referenced in a 4236 * specification, model, design or instance. This is an arbitrary value 4237 * managed by the questionnaire author and is not expected to be 4238 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 4239 * if a managed version is not available. There is also no expectation 4240 * that versions can be placed in a lexicographical sequence.). This is 4241 * the underlying object with id, value and extensions. The accessor 4242 * "getVersion" gives direct access to the value 4243 */ 4244 public StringType getVersionElement() { 4245 if (this.version == null) 4246 if (Configuration.errorOnAutoCreate()) 4247 throw new Error("Attempt to auto-create Questionnaire.version"); 4248 else if (Configuration.doAutoCreate()) 4249 this.version = new StringType(); // bb 4250 return this.version; 4251 } 4252 4253 public boolean hasVersionElement() { 4254 return this.version != null && !this.version.isEmpty(); 4255 } 4256 4257 public boolean hasVersion() { 4258 return this.version != null && !this.version.isEmpty(); 4259 } 4260 4261 /** 4262 * @param value {@link #version} (The identifier that is used to identify this 4263 * version of the questionnaire when it is referenced in a 4264 * specification, model, design or instance. This is an arbitrary 4265 * value managed by the questionnaire author and is not expected to 4266 * be globally unique. For example, it might be a timestamp (e.g. 4267 * yyyymmdd) if a managed version is not available. There is also 4268 * no expectation that versions can be placed in a lexicographical 4269 * sequence.). This is the underlying object with id, value and 4270 * extensions. The accessor "getVersion" gives direct access to the 4271 * value 4272 */ 4273 public Questionnaire setVersionElement(StringType value) { 4274 this.version = value; 4275 return this; 4276 } 4277 4278 /** 4279 * @return The identifier that is used to identify this version of the 4280 * questionnaire when it is referenced in a specification, model, design 4281 * or instance. This is an arbitrary value managed by the questionnaire 4282 * author and is not expected to be globally unique. For example, it 4283 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 4284 * available. There is also no expectation that versions can be placed 4285 * in a lexicographical sequence. 4286 */ 4287 public String getVersion() { 4288 return this.version == null ? null : this.version.getValue(); 4289 } 4290 4291 /** 4292 * @param value The identifier that is used to identify this version of the 4293 * questionnaire when it is referenced in a specification, model, 4294 * design or instance. This is an arbitrary value managed by the 4295 * questionnaire author and is not expected to be globally unique. 4296 * For example, it might be a timestamp (e.g. yyyymmdd) if a 4297 * managed version is not available. There is also no expectation 4298 * that versions can be placed in a lexicographical sequence. 4299 */ 4300 public Questionnaire setVersion(String value) { 4301 if (Utilities.noString(value)) 4302 this.version = null; 4303 else { 4304 if (this.version == null) 4305 this.version = new StringType(); 4306 this.version.setValue(value); 4307 } 4308 return this; 4309 } 4310 4311 /** 4312 * @return {@link #name} (A natural language name identifying the questionnaire. 4313 * This name should be usable as an identifier for the module by machine 4314 * processing applications such as code generation.). This is the 4315 * underlying object with id, value and extensions. The accessor 4316 * "getName" gives direct access to the value 4317 */ 4318 public StringType getNameElement() { 4319 if (this.name == null) 4320 if (Configuration.errorOnAutoCreate()) 4321 throw new Error("Attempt to auto-create Questionnaire.name"); 4322 else if (Configuration.doAutoCreate()) 4323 this.name = new StringType(); // bb 4324 return this.name; 4325 } 4326 4327 public boolean hasNameElement() { 4328 return this.name != null && !this.name.isEmpty(); 4329 } 4330 4331 public boolean hasName() { 4332 return this.name != null && !this.name.isEmpty(); 4333 } 4334 4335 /** 4336 * @param value {@link #name} (A natural language name identifying the 4337 * questionnaire. This name should be usable as an identifier for 4338 * the module by machine processing applications such as code 4339 * generation.). This is the underlying object with id, value and 4340 * extensions. The accessor "getName" gives direct access to the 4341 * value 4342 */ 4343 public Questionnaire setNameElement(StringType value) { 4344 this.name = value; 4345 return this; 4346 } 4347 4348 /** 4349 * @return A natural language name identifying the questionnaire. This name 4350 * should be usable as an identifier for the module by machine 4351 * processing applications such as code generation. 4352 */ 4353 public String getName() { 4354 return this.name == null ? null : this.name.getValue(); 4355 } 4356 4357 /** 4358 * @param value A natural language name identifying the questionnaire. This name 4359 * should be usable as an identifier for the module by machine 4360 * processing applications such as code generation. 4361 */ 4362 public Questionnaire setName(String value) { 4363 if (Utilities.noString(value)) 4364 this.name = null; 4365 else { 4366 if (this.name == null) 4367 this.name = new StringType(); 4368 this.name.setValue(value); 4369 } 4370 return this; 4371 } 4372 4373 /** 4374 * @return {@link #title} (A short, descriptive, user-friendly title for the 4375 * questionnaire.). This is the underlying object with id, value and 4376 * extensions. The accessor "getTitle" gives direct access to the value 4377 */ 4378 public StringType getTitleElement() { 4379 if (this.title == null) 4380 if (Configuration.errorOnAutoCreate()) 4381 throw new Error("Attempt to auto-create Questionnaire.title"); 4382 else if (Configuration.doAutoCreate()) 4383 this.title = new StringType(); // bb 4384 return this.title; 4385 } 4386 4387 public boolean hasTitleElement() { 4388 return this.title != null && !this.title.isEmpty(); 4389 } 4390 4391 public boolean hasTitle() { 4392 return this.title != null && !this.title.isEmpty(); 4393 } 4394 4395 /** 4396 * @param value {@link #title} (A short, descriptive, user-friendly title for 4397 * the questionnaire.). This is the underlying object with id, 4398 * value and extensions. The accessor "getTitle" gives direct 4399 * access to the value 4400 */ 4401 public Questionnaire setTitleElement(StringType value) { 4402 this.title = value; 4403 return this; 4404 } 4405 4406 /** 4407 * @return A short, descriptive, user-friendly title for the questionnaire. 4408 */ 4409 public String getTitle() { 4410 return this.title == null ? null : this.title.getValue(); 4411 } 4412 4413 /** 4414 * @param value A short, descriptive, user-friendly title for the questionnaire. 4415 */ 4416 public Questionnaire setTitle(String value) { 4417 if (Utilities.noString(value)) 4418 this.title = null; 4419 else { 4420 if (this.title == null) 4421 this.title = new StringType(); 4422 this.title.setValue(value); 4423 } 4424 return this; 4425 } 4426 4427 /** 4428 * @return {@link #derivedFrom} (The URL of a Questionnaire that this 4429 * Questionnaire is based on.) 4430 */ 4431 public List<CanonicalType> getDerivedFrom() { 4432 if (this.derivedFrom == null) 4433 this.derivedFrom = new ArrayList<CanonicalType>(); 4434 return this.derivedFrom; 4435 } 4436 4437 /** 4438 * @return Returns a reference to <code>this</code> for easy method chaining 4439 */ 4440 public Questionnaire setDerivedFrom(List<CanonicalType> theDerivedFrom) { 4441 this.derivedFrom = theDerivedFrom; 4442 return this; 4443 } 4444 4445 public boolean hasDerivedFrom() { 4446 if (this.derivedFrom == null) 4447 return false; 4448 for (CanonicalType item : this.derivedFrom) 4449 if (!item.isEmpty()) 4450 return true; 4451 return false; 4452 } 4453 4454 /** 4455 * @return {@link #derivedFrom} (The URL of a Questionnaire that this 4456 * Questionnaire is based on.) 4457 */ 4458 public CanonicalType addDerivedFromElement() {// 2 4459 CanonicalType t = new CanonicalType(); 4460 if (this.derivedFrom == null) 4461 this.derivedFrom = new ArrayList<CanonicalType>(); 4462 this.derivedFrom.add(t); 4463 return t; 4464 } 4465 4466 /** 4467 * @param value {@link #derivedFrom} (The URL of a Questionnaire that this 4468 * Questionnaire is based on.) 4469 */ 4470 public Questionnaire addDerivedFrom(String value) { // 1 4471 CanonicalType t = new CanonicalType(); 4472 t.setValue(value); 4473 if (this.derivedFrom == null) 4474 this.derivedFrom = new ArrayList<CanonicalType>(); 4475 this.derivedFrom.add(t); 4476 return this; 4477 } 4478 4479 /** 4480 * @param value {@link #derivedFrom} (The URL of a Questionnaire that this 4481 * Questionnaire is based on.) 4482 */ 4483 public boolean hasDerivedFrom(String value) { 4484 if (this.derivedFrom == null) 4485 return false; 4486 for (CanonicalType v : this.derivedFrom) 4487 if (v.getValue().equals(value)) // canonical(Questionnaire) 4488 return true; 4489 return false; 4490 } 4491 4492 /** 4493 * @return {@link #status} (The status of this questionnaire. Enables tracking 4494 * the life-cycle of the content.). This is the underlying object with 4495 * id, value and extensions. The accessor "getStatus" gives direct 4496 * access to the value 4497 */ 4498 public Enumeration<PublicationStatus> getStatusElement() { 4499 if (this.status == null) 4500 if (Configuration.errorOnAutoCreate()) 4501 throw new Error("Attempt to auto-create Questionnaire.status"); 4502 else if (Configuration.doAutoCreate()) 4503 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 4504 return this.status; 4505 } 4506 4507 public boolean hasStatusElement() { 4508 return this.status != null && !this.status.isEmpty(); 4509 } 4510 4511 public boolean hasStatus() { 4512 return this.status != null && !this.status.isEmpty(); 4513 } 4514 4515 /** 4516 * @param value {@link #status} (The status of this questionnaire. Enables 4517 * tracking the life-cycle of the content.). This is the underlying 4518 * object with id, value and extensions. The accessor "getStatus" 4519 * gives direct access to the value 4520 */ 4521 public Questionnaire setStatusElement(Enumeration<PublicationStatus> value) { 4522 this.status = value; 4523 return this; 4524 } 4525 4526 /** 4527 * @return The status of this questionnaire. Enables tracking the life-cycle of 4528 * the content. 4529 */ 4530 public PublicationStatus getStatus() { 4531 return this.status == null ? null : this.status.getValue(); 4532 } 4533 4534 /** 4535 * @param value The status of this questionnaire. Enables tracking the 4536 * life-cycle of the content. 4537 */ 4538 public Questionnaire setStatus(PublicationStatus value) { 4539 if (this.status == null) 4540 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 4541 this.status.setValue(value); 4542 return this; 4543 } 4544 4545 /** 4546 * @return {@link #experimental} (A Boolean value to indicate that this 4547 * questionnaire is authored for testing purposes (or 4548 * education/evaluation/marketing) and is not intended to be used for 4549 * genuine usage.). This is the underlying object with id, value and 4550 * extensions. The accessor "getExperimental" gives direct access to the 4551 * value 4552 */ 4553 public BooleanType getExperimentalElement() { 4554 if (this.experimental == null) 4555 if (Configuration.errorOnAutoCreate()) 4556 throw new Error("Attempt to auto-create Questionnaire.experimental"); 4557 else if (Configuration.doAutoCreate()) 4558 this.experimental = new BooleanType(); // bb 4559 return this.experimental; 4560 } 4561 4562 public boolean hasExperimentalElement() { 4563 return this.experimental != null && !this.experimental.isEmpty(); 4564 } 4565 4566 public boolean hasExperimental() { 4567 return this.experimental != null && !this.experimental.isEmpty(); 4568 } 4569 4570 /** 4571 * @param value {@link #experimental} (A Boolean value to indicate that this 4572 * questionnaire is authored for testing purposes (or 4573 * education/evaluation/marketing) and is not intended to be used 4574 * for genuine usage.). This is the underlying object with id, 4575 * value and extensions. The accessor "getExperimental" gives 4576 * direct access to the value 4577 */ 4578 public Questionnaire setExperimentalElement(BooleanType value) { 4579 this.experimental = value; 4580 return this; 4581 } 4582 4583 /** 4584 * @return A Boolean value to indicate that this questionnaire is authored for 4585 * testing purposes (or education/evaluation/marketing) and is not 4586 * intended to be used for genuine usage. 4587 */ 4588 public boolean getExperimental() { 4589 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 4590 } 4591 4592 /** 4593 * @param value A Boolean value to indicate that this questionnaire is authored 4594 * for testing purposes (or education/evaluation/marketing) and is 4595 * not intended to be used for genuine usage. 4596 */ 4597 public Questionnaire setExperimental(boolean value) { 4598 if (this.experimental == null) 4599 this.experimental = new BooleanType(); 4600 this.experimental.setValue(value); 4601 return this; 4602 } 4603 4604 /** 4605 * @return {@link #subjectType} (The types of subjects that can be the subject 4606 * of responses created for the questionnaire.) 4607 */ 4608 public List<CodeType> getSubjectType() { 4609 if (this.subjectType == null) 4610 this.subjectType = new ArrayList<CodeType>(); 4611 return this.subjectType; 4612 } 4613 4614 /** 4615 * @return Returns a reference to <code>this</code> for easy method chaining 4616 */ 4617 public Questionnaire setSubjectType(List<CodeType> theSubjectType) { 4618 this.subjectType = theSubjectType; 4619 return this; 4620 } 4621 4622 public boolean hasSubjectType() { 4623 if (this.subjectType == null) 4624 return false; 4625 for (CodeType item : this.subjectType) 4626 if (!item.isEmpty()) 4627 return true; 4628 return false; 4629 } 4630 4631 /** 4632 * @return {@link #subjectType} (The types of subjects that can be the subject 4633 * of responses created for the questionnaire.) 4634 */ 4635 public CodeType addSubjectTypeElement() {// 2 4636 CodeType t = new CodeType(); 4637 if (this.subjectType == null) 4638 this.subjectType = new ArrayList<CodeType>(); 4639 this.subjectType.add(t); 4640 return t; 4641 } 4642 4643 /** 4644 * @param value {@link #subjectType} (The types of subjects that can be the 4645 * subject of responses created for the questionnaire.) 4646 */ 4647 public Questionnaire addSubjectType(String value) { // 1 4648 CodeType t = new CodeType(); 4649 t.setValue(value); 4650 if (this.subjectType == null) 4651 this.subjectType = new ArrayList<CodeType>(); 4652 this.subjectType.add(t); 4653 return this; 4654 } 4655 4656 /** 4657 * @param value {@link #subjectType} (The types of subjects that can be the 4658 * subject of responses created for the questionnaire.) 4659 */ 4660 public boolean hasSubjectType(String value) { 4661 if (this.subjectType == null) 4662 return false; 4663 for (CodeType v : this.subjectType) 4664 if (v.getValue().equals(value)) // code 4665 return true; 4666 return false; 4667 } 4668 4669 /** 4670 * @return {@link #date} (The date (and optionally time) when the questionnaire 4671 * was published. The date must change when the business version changes 4672 * and it must change if the status code changes. In addition, it should 4673 * change when the substantive content of the questionnaire changes.). 4674 * This is the underlying object with id, value and extensions. The 4675 * accessor "getDate" gives direct access to the value 4676 */ 4677 public DateTimeType getDateElement() { 4678 if (this.date == null) 4679 if (Configuration.errorOnAutoCreate()) 4680 throw new Error("Attempt to auto-create Questionnaire.date"); 4681 else if (Configuration.doAutoCreate()) 4682 this.date = new DateTimeType(); // bb 4683 return this.date; 4684 } 4685 4686 public boolean hasDateElement() { 4687 return this.date != null && !this.date.isEmpty(); 4688 } 4689 4690 public boolean hasDate() { 4691 return this.date != null && !this.date.isEmpty(); 4692 } 4693 4694 /** 4695 * @param value {@link #date} (The date (and optionally time) when the 4696 * questionnaire was published. The date must change when the 4697 * business version changes and it must change if the status code 4698 * changes. In addition, it should change when the substantive 4699 * content of the questionnaire changes.). This is the underlying 4700 * object with id, value and extensions. The accessor "getDate" 4701 * gives direct access to the value 4702 */ 4703 public Questionnaire setDateElement(DateTimeType value) { 4704 this.date = value; 4705 return this; 4706 } 4707 4708 /** 4709 * @return The date (and optionally time) when the questionnaire was published. 4710 * The date must change when the business version changes and it must 4711 * change if the status code changes. In addition, it should change when 4712 * the substantive content of the questionnaire changes. 4713 */ 4714 public Date getDate() { 4715 return this.date == null ? null : this.date.getValue(); 4716 } 4717 4718 /** 4719 * @param value The date (and optionally time) when the questionnaire was 4720 * published. The date must change when the business version 4721 * changes and it must change if the status code changes. In 4722 * addition, it should change when the substantive content of the 4723 * questionnaire changes. 4724 */ 4725 public Questionnaire setDate(Date value) { 4726 if (value == null) 4727 this.date = null; 4728 else { 4729 if (this.date == null) 4730 this.date = new DateTimeType(); 4731 this.date.setValue(value); 4732 } 4733 return this; 4734 } 4735 4736 /** 4737 * @return {@link #publisher} (The name of the organization or individual that 4738 * published the questionnaire.). This is the underlying object with id, 4739 * value and extensions. The accessor "getPublisher" gives direct access 4740 * to the value 4741 */ 4742 public StringType getPublisherElement() { 4743 if (this.publisher == null) 4744 if (Configuration.errorOnAutoCreate()) 4745 throw new Error("Attempt to auto-create Questionnaire.publisher"); 4746 else if (Configuration.doAutoCreate()) 4747 this.publisher = new StringType(); // bb 4748 return this.publisher; 4749 } 4750 4751 public boolean hasPublisherElement() { 4752 return this.publisher != null && !this.publisher.isEmpty(); 4753 } 4754 4755 public boolean hasPublisher() { 4756 return this.publisher != null && !this.publisher.isEmpty(); 4757 } 4758 4759 /** 4760 * @param value {@link #publisher} (The name of the organization or individual 4761 * that published the questionnaire.). This is the underlying 4762 * object with id, value and extensions. The accessor 4763 * "getPublisher" gives direct access to the value 4764 */ 4765 public Questionnaire setPublisherElement(StringType value) { 4766 this.publisher = value; 4767 return this; 4768 } 4769 4770 /** 4771 * @return The name of the organization or individual that published the 4772 * questionnaire. 4773 */ 4774 public String getPublisher() { 4775 return this.publisher == null ? null : this.publisher.getValue(); 4776 } 4777 4778 /** 4779 * @param value The name of the organization or individual that published the 4780 * questionnaire. 4781 */ 4782 public Questionnaire setPublisher(String value) { 4783 if (Utilities.noString(value)) 4784 this.publisher = null; 4785 else { 4786 if (this.publisher == null) 4787 this.publisher = new StringType(); 4788 this.publisher.setValue(value); 4789 } 4790 return this; 4791 } 4792 4793 /** 4794 * @return {@link #contact} (Contact details to assist a user in finding and 4795 * communicating with the publisher.) 4796 */ 4797 public List<ContactDetail> getContact() { 4798 if (this.contact == null) 4799 this.contact = new ArrayList<ContactDetail>(); 4800 return this.contact; 4801 } 4802 4803 /** 4804 * @return Returns a reference to <code>this</code> for easy method chaining 4805 */ 4806 public Questionnaire setContact(List<ContactDetail> theContact) { 4807 this.contact = theContact; 4808 return this; 4809 } 4810 4811 public boolean hasContact() { 4812 if (this.contact == null) 4813 return false; 4814 for (ContactDetail item : this.contact) 4815 if (!item.isEmpty()) 4816 return true; 4817 return false; 4818 } 4819 4820 public ContactDetail addContact() { // 3 4821 ContactDetail t = new ContactDetail(); 4822 if (this.contact == null) 4823 this.contact = new ArrayList<ContactDetail>(); 4824 this.contact.add(t); 4825 return t; 4826 } 4827 4828 public Questionnaire addContact(ContactDetail t) { // 3 4829 if (t == null) 4830 return this; 4831 if (this.contact == null) 4832 this.contact = new ArrayList<ContactDetail>(); 4833 this.contact.add(t); 4834 return this; 4835 } 4836 4837 /** 4838 * @return The first repetition of repeating field {@link #contact}, creating it 4839 * if it does not already exist 4840 */ 4841 public ContactDetail getContactFirstRep() { 4842 if (getContact().isEmpty()) { 4843 addContact(); 4844 } 4845 return getContact().get(0); 4846 } 4847 4848 /** 4849 * @return {@link #description} (A free text natural language description of the 4850 * questionnaire from a consumer's perspective.). This is the underlying 4851 * object with id, value and extensions. The accessor "getDescription" 4852 * gives direct access to the value 4853 */ 4854 public MarkdownType getDescriptionElement() { 4855 if (this.description == null) 4856 if (Configuration.errorOnAutoCreate()) 4857 throw new Error("Attempt to auto-create Questionnaire.description"); 4858 else if (Configuration.doAutoCreate()) 4859 this.description = new MarkdownType(); // bb 4860 return this.description; 4861 } 4862 4863 public boolean hasDescriptionElement() { 4864 return this.description != null && !this.description.isEmpty(); 4865 } 4866 4867 public boolean hasDescription() { 4868 return this.description != null && !this.description.isEmpty(); 4869 } 4870 4871 /** 4872 * @param value {@link #description} (A free text natural language description 4873 * of the questionnaire from a consumer's perspective.). This is 4874 * the underlying object with id, value and extensions. The 4875 * accessor "getDescription" gives direct access to the value 4876 */ 4877 public Questionnaire setDescriptionElement(MarkdownType value) { 4878 this.description = value; 4879 return this; 4880 } 4881 4882 /** 4883 * @return A free text natural language description of the questionnaire from a 4884 * consumer's perspective. 4885 */ 4886 public String getDescription() { 4887 return this.description == null ? null : this.description.getValue(); 4888 } 4889 4890 /** 4891 * @param value A free text natural language description of the questionnaire 4892 * from a consumer's perspective. 4893 */ 4894 public Questionnaire setDescription(String value) { 4895 if (value == null) 4896 this.description = null; 4897 else { 4898 if (this.description == null) 4899 this.description = new MarkdownType(); 4900 this.description.setValue(value); 4901 } 4902 return this; 4903 } 4904 4905 /** 4906 * @return {@link #useContext} (The content was developed with a focus and 4907 * intent of supporting the contexts that are listed. These contexts may 4908 * be general categories (gender, age, ...) or may be references to 4909 * specific programs (insurance plans, studies, ...) and may be used to 4910 * assist with indexing and searching for appropriate questionnaire 4911 * instances.) 4912 */ 4913 public List<UsageContext> getUseContext() { 4914 if (this.useContext == null) 4915 this.useContext = new ArrayList<UsageContext>(); 4916 return this.useContext; 4917 } 4918 4919 /** 4920 * @return Returns a reference to <code>this</code> for easy method chaining 4921 */ 4922 public Questionnaire setUseContext(List<UsageContext> theUseContext) { 4923 this.useContext = theUseContext; 4924 return this; 4925 } 4926 4927 public boolean hasUseContext() { 4928 if (this.useContext == null) 4929 return false; 4930 for (UsageContext item : this.useContext) 4931 if (!item.isEmpty()) 4932 return true; 4933 return false; 4934 } 4935 4936 public UsageContext addUseContext() { // 3 4937 UsageContext t = new UsageContext(); 4938 if (this.useContext == null) 4939 this.useContext = new ArrayList<UsageContext>(); 4940 this.useContext.add(t); 4941 return t; 4942 } 4943 4944 public Questionnaire addUseContext(UsageContext t) { // 3 4945 if (t == null) 4946 return this; 4947 if (this.useContext == null) 4948 this.useContext = new ArrayList<UsageContext>(); 4949 this.useContext.add(t); 4950 return this; 4951 } 4952 4953 /** 4954 * @return The first repetition of repeating field {@link #useContext}, creating 4955 * it if it does not already exist 4956 */ 4957 public UsageContext getUseContextFirstRep() { 4958 if (getUseContext().isEmpty()) { 4959 addUseContext(); 4960 } 4961 return getUseContext().get(0); 4962 } 4963 4964 /** 4965 * @return {@link #jurisdiction} (A legal or geographic region in which the 4966 * questionnaire is intended to be used.) 4967 */ 4968 public List<CodeableConcept> getJurisdiction() { 4969 if (this.jurisdiction == null) 4970 this.jurisdiction = new ArrayList<CodeableConcept>(); 4971 return this.jurisdiction; 4972 } 4973 4974 /** 4975 * @return Returns a reference to <code>this</code> for easy method chaining 4976 */ 4977 public Questionnaire setJurisdiction(List<CodeableConcept> theJurisdiction) { 4978 this.jurisdiction = theJurisdiction; 4979 return this; 4980 } 4981 4982 public boolean hasJurisdiction() { 4983 if (this.jurisdiction == null) 4984 return false; 4985 for (CodeableConcept item : this.jurisdiction) 4986 if (!item.isEmpty()) 4987 return true; 4988 return false; 4989 } 4990 4991 public CodeableConcept addJurisdiction() { // 3 4992 CodeableConcept t = new CodeableConcept(); 4993 if (this.jurisdiction == null) 4994 this.jurisdiction = new ArrayList<CodeableConcept>(); 4995 this.jurisdiction.add(t); 4996 return t; 4997 } 4998 4999 public Questionnaire addJurisdiction(CodeableConcept t) { // 3 5000 if (t == null) 5001 return this; 5002 if (this.jurisdiction == null) 5003 this.jurisdiction = new ArrayList<CodeableConcept>(); 5004 this.jurisdiction.add(t); 5005 return this; 5006 } 5007 5008 /** 5009 * @return The first repetition of repeating field {@link #jurisdiction}, 5010 * creating it if it does not already exist 5011 */ 5012 public CodeableConcept getJurisdictionFirstRep() { 5013 if (getJurisdiction().isEmpty()) { 5014 addJurisdiction(); 5015 } 5016 return getJurisdiction().get(0); 5017 } 5018 5019 /** 5020 * @return {@link #purpose} (Explanation of why this questionnaire is needed and 5021 * why it has been designed as it has.). This is the underlying object 5022 * with id, value and extensions. The accessor "getPurpose" gives direct 5023 * access to the value 5024 */ 5025 public MarkdownType getPurposeElement() { 5026 if (this.purpose == null) 5027 if (Configuration.errorOnAutoCreate()) 5028 throw new Error("Attempt to auto-create Questionnaire.purpose"); 5029 else if (Configuration.doAutoCreate()) 5030 this.purpose = new MarkdownType(); // bb 5031 return this.purpose; 5032 } 5033 5034 public boolean hasPurposeElement() { 5035 return this.purpose != null && !this.purpose.isEmpty(); 5036 } 5037 5038 public boolean hasPurpose() { 5039 return this.purpose != null && !this.purpose.isEmpty(); 5040 } 5041 5042 /** 5043 * @param value {@link #purpose} (Explanation of why this questionnaire is 5044 * needed and why it has been designed as it has.). This is the 5045 * underlying object with id, value and extensions. The accessor 5046 * "getPurpose" gives direct access to the value 5047 */ 5048 public Questionnaire setPurposeElement(MarkdownType value) { 5049 this.purpose = value; 5050 return this; 5051 } 5052 5053 /** 5054 * @return Explanation of why this questionnaire is needed and why it has been 5055 * designed as it has. 5056 */ 5057 public String getPurpose() { 5058 return this.purpose == null ? null : this.purpose.getValue(); 5059 } 5060 5061 /** 5062 * @param value Explanation of why this questionnaire is needed and why it has 5063 * been designed as it has. 5064 */ 5065 public Questionnaire setPurpose(String value) { 5066 if (value == null) 5067 this.purpose = null; 5068 else { 5069 if (this.purpose == null) 5070 this.purpose = new MarkdownType(); 5071 this.purpose.setValue(value); 5072 } 5073 return this; 5074 } 5075 5076 /** 5077 * @return {@link #copyright} (A copyright statement relating to the 5078 * questionnaire and/or its contents. Copyright statements are generally 5079 * legal restrictions on the use and publishing of the questionnaire.). 5080 * This is the underlying object with id, value and extensions. The 5081 * accessor "getCopyright" gives direct access to the value 5082 */ 5083 public MarkdownType getCopyrightElement() { 5084 if (this.copyright == null) 5085 if (Configuration.errorOnAutoCreate()) 5086 throw new Error("Attempt to auto-create Questionnaire.copyright"); 5087 else if (Configuration.doAutoCreate()) 5088 this.copyright = new MarkdownType(); // bb 5089 return this.copyright; 5090 } 5091 5092 public boolean hasCopyrightElement() { 5093 return this.copyright != null && !this.copyright.isEmpty(); 5094 } 5095 5096 public boolean hasCopyright() { 5097 return this.copyright != null && !this.copyright.isEmpty(); 5098 } 5099 5100 /** 5101 * @param value {@link #copyright} (A copyright statement relating to the 5102 * questionnaire and/or its contents. Copyright statements are 5103 * generally legal restrictions on the use and publishing of the 5104 * questionnaire.). This is the underlying object with id, value 5105 * and extensions. The accessor "getCopyright" gives direct access 5106 * to the value 5107 */ 5108 public Questionnaire setCopyrightElement(MarkdownType value) { 5109 this.copyright = value; 5110 return this; 5111 } 5112 5113 /** 5114 * @return A copyright statement relating to the questionnaire and/or its 5115 * contents. Copyright statements are generally legal restrictions on 5116 * the use and publishing of the questionnaire. 5117 */ 5118 public String getCopyright() { 5119 return this.copyright == null ? null : this.copyright.getValue(); 5120 } 5121 5122 /** 5123 * @param value A copyright statement relating to the questionnaire and/or its 5124 * contents. Copyright statements are generally legal restrictions 5125 * on the use and publishing of the questionnaire. 5126 */ 5127 public Questionnaire setCopyright(String value) { 5128 if (value == null) 5129 this.copyright = null; 5130 else { 5131 if (this.copyright == null) 5132 this.copyright = new MarkdownType(); 5133 this.copyright.setValue(value); 5134 } 5135 return this; 5136 } 5137 5138 /** 5139 * @return {@link #approvalDate} (The date on which the resource content was 5140 * approved by the publisher. Approval happens once when the content is 5141 * officially approved for usage.). This is the underlying object with 5142 * id, value and extensions. The accessor "getApprovalDate" gives direct 5143 * access to the value 5144 */ 5145 public DateType getApprovalDateElement() { 5146 if (this.approvalDate == null) 5147 if (Configuration.errorOnAutoCreate()) 5148 throw new Error("Attempt to auto-create Questionnaire.approvalDate"); 5149 else if (Configuration.doAutoCreate()) 5150 this.approvalDate = new DateType(); // bb 5151 return this.approvalDate; 5152 } 5153 5154 public boolean hasApprovalDateElement() { 5155 return this.approvalDate != null && !this.approvalDate.isEmpty(); 5156 } 5157 5158 public boolean hasApprovalDate() { 5159 return this.approvalDate != null && !this.approvalDate.isEmpty(); 5160 } 5161 5162 /** 5163 * @param value {@link #approvalDate} (The date on which the resource content 5164 * was approved by the publisher. Approval happens once when the 5165 * content is officially approved for usage.). This is the 5166 * underlying object with id, value and extensions. The accessor 5167 * "getApprovalDate" gives direct access to the value 5168 */ 5169 public Questionnaire setApprovalDateElement(DateType value) { 5170 this.approvalDate = value; 5171 return this; 5172 } 5173 5174 /** 5175 * @return The date on which the resource content was approved by the publisher. 5176 * Approval happens once when the content is officially approved for 5177 * usage. 5178 */ 5179 public Date getApprovalDate() { 5180 return this.approvalDate == null ? null : this.approvalDate.getValue(); 5181 } 5182 5183 /** 5184 * @param value The date on which the resource content was approved by the 5185 * publisher. Approval happens once when the content is officially 5186 * approved for usage. 5187 */ 5188 public Questionnaire setApprovalDate(Date value) { 5189 if (value == null) 5190 this.approvalDate = null; 5191 else { 5192 if (this.approvalDate == null) 5193 this.approvalDate = new DateType(); 5194 this.approvalDate.setValue(value); 5195 } 5196 return this; 5197 } 5198 5199 /** 5200 * @return {@link #lastReviewDate} (The date on which the resource content was 5201 * last reviewed. Review happens periodically after approval but does 5202 * not change the original approval date.). This is the underlying 5203 * object with id, value and extensions. The accessor 5204 * "getLastReviewDate" gives direct access to the value 5205 */ 5206 public DateType getLastReviewDateElement() { 5207 if (this.lastReviewDate == null) 5208 if (Configuration.errorOnAutoCreate()) 5209 throw new Error("Attempt to auto-create Questionnaire.lastReviewDate"); 5210 else if (Configuration.doAutoCreate()) 5211 this.lastReviewDate = new DateType(); // bb 5212 return this.lastReviewDate; 5213 } 5214 5215 public boolean hasLastReviewDateElement() { 5216 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 5217 } 5218 5219 public boolean hasLastReviewDate() { 5220 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 5221 } 5222 5223 /** 5224 * @param value {@link #lastReviewDate} (The date on which the resource content 5225 * was last reviewed. Review happens periodically after approval 5226 * but does not change the original approval date.). This is the 5227 * underlying object with id, value and extensions. The accessor 5228 * "getLastReviewDate" gives direct access to the value 5229 */ 5230 public Questionnaire setLastReviewDateElement(DateType value) { 5231 this.lastReviewDate = value; 5232 return this; 5233 } 5234 5235 /** 5236 * @return The date on which the resource content was last reviewed. Review 5237 * happens periodically after approval but does not change the original 5238 * approval date. 5239 */ 5240 public Date getLastReviewDate() { 5241 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 5242 } 5243 5244 /** 5245 * @param value The date on which the resource content was last reviewed. Review 5246 * happens periodically after approval but does not change the 5247 * original approval date. 5248 */ 5249 public Questionnaire setLastReviewDate(Date value) { 5250 if (value == null) 5251 this.lastReviewDate = null; 5252 else { 5253 if (this.lastReviewDate == null) 5254 this.lastReviewDate = new DateType(); 5255 this.lastReviewDate.setValue(value); 5256 } 5257 return this; 5258 } 5259 5260 /** 5261 * @return {@link #effectivePeriod} (The period during which the questionnaire 5262 * content was or is planned to be in active use.) 5263 */ 5264 public Period getEffectivePeriod() { 5265 if (this.effectivePeriod == null) 5266 if (Configuration.errorOnAutoCreate()) 5267 throw new Error("Attempt to auto-create Questionnaire.effectivePeriod"); 5268 else if (Configuration.doAutoCreate()) 5269 this.effectivePeriod = new Period(); // cc 5270 return this.effectivePeriod; 5271 } 5272 5273 public boolean hasEffectivePeriod() { 5274 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 5275 } 5276 5277 /** 5278 * @param value {@link #effectivePeriod} (The period during which the 5279 * questionnaire content was or is planned to be in active use.) 5280 */ 5281 public Questionnaire setEffectivePeriod(Period value) { 5282 this.effectivePeriod = value; 5283 return this; 5284 } 5285 5286 /** 5287 * @return {@link #code} (An identifier for this question or group of questions 5288 * in a particular terminology such as LOINC.) 5289 */ 5290 public List<Coding> getCode() { 5291 if (this.code == null) 5292 this.code = new ArrayList<Coding>(); 5293 return this.code; 5294 } 5295 5296 /** 5297 * @return Returns a reference to <code>this</code> for easy method chaining 5298 */ 5299 public Questionnaire setCode(List<Coding> theCode) { 5300 this.code = theCode; 5301 return this; 5302 } 5303 5304 public boolean hasCode() { 5305 if (this.code == null) 5306 return false; 5307 for (Coding item : this.code) 5308 if (!item.isEmpty()) 5309 return true; 5310 return false; 5311 } 5312 5313 public Coding addCode() { // 3 5314 Coding t = new Coding(); 5315 if (this.code == null) 5316 this.code = new ArrayList<Coding>(); 5317 this.code.add(t); 5318 return t; 5319 } 5320 5321 public Questionnaire addCode(Coding t) { // 3 5322 if (t == null) 5323 return this; 5324 if (this.code == null) 5325 this.code = new ArrayList<Coding>(); 5326 this.code.add(t); 5327 return this; 5328 } 5329 5330 /** 5331 * @return The first repetition of repeating field {@link #code}, creating it if 5332 * it does not already exist 5333 */ 5334 public Coding getCodeFirstRep() { 5335 if (getCode().isEmpty()) { 5336 addCode(); 5337 } 5338 return getCode().get(0); 5339 } 5340 5341 /** 5342 * @return {@link #item} (A particular question, question grouping or display 5343 * text that is part of the questionnaire.) 5344 */ 5345 public List<QuestionnaireItemComponent> getItem() { 5346 if (this.item == null) 5347 this.item = new ArrayList<QuestionnaireItemComponent>(); 5348 return this.item; 5349 } 5350 5351 /** 5352 * @return Returns a reference to <code>this</code> for easy method chaining 5353 */ 5354 public Questionnaire setItem(List<QuestionnaireItemComponent> theItem) { 5355 this.item = theItem; 5356 return this; 5357 } 5358 5359 public boolean hasItem() { 5360 if (this.item == null) 5361 return false; 5362 for (QuestionnaireItemComponent item : this.item) 5363 if (!item.isEmpty()) 5364 return true; 5365 return false; 5366 } 5367 5368 public QuestionnaireItemComponent addItem() { // 3 5369 QuestionnaireItemComponent t = new QuestionnaireItemComponent(); 5370 if (this.item == null) 5371 this.item = new ArrayList<QuestionnaireItemComponent>(); 5372 this.item.add(t); 5373 return t; 5374 } 5375 5376 public Questionnaire addItem(QuestionnaireItemComponent t) { // 3 5377 if (t == null) 5378 return this; 5379 if (this.item == null) 5380 this.item = new ArrayList<QuestionnaireItemComponent>(); 5381 this.item.add(t); 5382 return this; 5383 } 5384 5385 /** 5386 * @return The first repetition of repeating field {@link #item}, creating it if 5387 * it does not already exist 5388 */ 5389 public QuestionnaireItemComponent getItemFirstRep() { 5390 if (getItem().isEmpty()) { 5391 addItem(); 5392 } 5393 return getItem().get(0); 5394 } 5395 5396 protected void listChildren(List<Property> children) { 5397 super.listChildren(children); 5398 children.add(new Property("url", "uri", 5399 "An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers.", 5400 0, 1, url)); 5401 children.add(new Property("identifier", "Identifier", 5402 "A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.", 5403 0, java.lang.Integer.MAX_VALUE, identifier)); 5404 children.add(new Property("version", "string", 5405 "The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 5406 0, 1, version)); 5407 children.add(new Property("name", "string", 5408 "A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 5409 0, 1, name)); 5410 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the questionnaire.", 0, 5411 1, title)); 5412 children.add(new Property("derivedFrom", "canonical(Questionnaire)", 5413 "The URL of a Questionnaire that this Questionnaire is based on.", 0, java.lang.Integer.MAX_VALUE, 5414 derivedFrom)); 5415 children.add(new Property("status", "code", 5416 "The status of this questionnaire. Enables tracking the life-cycle of the content.", 0, 1, status)); 5417 children.add(new Property("experimental", "boolean", 5418 "A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 5419 0, 1, experimental)); 5420 children.add(new Property("subjectType", "code", 5421 "The types of subjects that can be the subject of responses created for the questionnaire.", 0, 5422 java.lang.Integer.MAX_VALUE, subjectType)); 5423 children.add(new Property("date", "dateTime", 5424 "The date (and optionally time) when the questionnaire was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.", 5425 0, 1, date)); 5426 children.add(new Property("publisher", "string", 5427 "The name of the organization or individual that published the questionnaire.", 0, 1, publisher)); 5428 children.add(new Property("contact", "ContactDetail", 5429 "Contact details to assist a user in finding and communicating with the publisher.", 0, 5430 java.lang.Integer.MAX_VALUE, contact)); 5431 children.add(new Property("description", "markdown", 5432 "A free text natural language description of the questionnaire from a consumer's perspective.", 0, 1, 5433 description)); 5434 children.add(new Property("useContext", "UsageContext", 5435 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate questionnaire instances.", 5436 0, java.lang.Integer.MAX_VALUE, useContext)); 5437 children.add(new Property("jurisdiction", "CodeableConcept", 5438 "A legal or geographic region in which the questionnaire is intended to be used.", 0, 5439 java.lang.Integer.MAX_VALUE, jurisdiction)); 5440 children.add(new Property("purpose", "markdown", 5441 "Explanation of why this questionnaire is needed and why it has been designed as it has.", 0, 1, purpose)); 5442 children.add(new Property("copyright", "markdown", 5443 "A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.", 5444 0, 1, copyright)); 5445 children.add(new Property("approvalDate", "date", 5446 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 5447 0, 1, approvalDate)); 5448 children.add(new Property("lastReviewDate", "date", 5449 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 5450 0, 1, lastReviewDate)); 5451 children.add(new Property("effectivePeriod", "Period", 5452 "The period during which the questionnaire content was or is planned to be in active use.", 0, 1, 5453 effectivePeriod)); 5454 children.add(new Property("code", "Coding", 5455 "An identifier for this question or group of questions in a particular terminology such as LOINC.", 0, 5456 java.lang.Integer.MAX_VALUE, code)); 5457 children.add(new Property("item", "", 5458 "A particular question, question grouping or display text that is part of the questionnaire.", 0, 5459 java.lang.Integer.MAX_VALUE, item)); 5460 } 5461 5462 @Override 5463 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5464 switch (_hash) { 5465 case 116079: 5466 /* url */ return new Property("url", "uri", 5467 "An absolute URI that is used to identify this questionnaire when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this questionnaire is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the questionnaire is stored on different servers.", 5468 0, 1, url); 5469 case -1618432855: 5470 /* identifier */ return new Property("identifier", "Identifier", 5471 "A formal identifier that is used to identify this questionnaire when it is represented in other formats, or referenced in a specification, model, design or an instance.", 5472 0, java.lang.Integer.MAX_VALUE, identifier); 5473 case 351608024: 5474 /* version */ return new Property("version", "string", 5475 "The identifier that is used to identify this version of the questionnaire when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the questionnaire author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 5476 0, 1, version); 5477 case 3373707: 5478 /* name */ return new Property("name", "string", 5479 "A natural language name identifying the questionnaire. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 5480 0, 1, name); 5481 case 110371416: 5482 /* title */ return new Property("title", "string", 5483 "A short, descriptive, user-friendly title for the questionnaire.", 0, 1, title); 5484 case 1077922663: 5485 /* derivedFrom */ return new Property("derivedFrom", "canonical(Questionnaire)", 5486 "The URL of a Questionnaire that this Questionnaire is based on.", 0, java.lang.Integer.MAX_VALUE, 5487 derivedFrom); 5488 case -892481550: 5489 /* status */ return new Property("status", "code", 5490 "The status of this questionnaire. Enables tracking the life-cycle of the content.", 0, 1, status); 5491 case -404562712: 5492 /* experimental */ return new Property("experimental", "boolean", 5493 "A Boolean value to indicate that this questionnaire is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 5494 0, 1, experimental); 5495 case -603200890: 5496 /* subjectType */ return new Property("subjectType", "code", 5497 "The types of subjects that can be the subject of responses created for the questionnaire.", 0, 5498 java.lang.Integer.MAX_VALUE, subjectType); 5499 case 3076014: 5500 /* date */ return new Property("date", "dateTime", 5501 "The date (and optionally time) when the questionnaire was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the questionnaire changes.", 5502 0, 1, date); 5503 case 1447404028: 5504 /* publisher */ return new Property("publisher", "string", 5505 "The name of the organization or individual that published the questionnaire.", 0, 1, publisher); 5506 case 951526432: 5507 /* contact */ return new Property("contact", "ContactDetail", 5508 "Contact details to assist a user in finding and communicating with the publisher.", 0, 5509 java.lang.Integer.MAX_VALUE, contact); 5510 case -1724546052: 5511 /* description */ return new Property("description", "markdown", 5512 "A free text natural language description of the questionnaire from a consumer's perspective.", 0, 1, 5513 description); 5514 case -669707736: 5515 /* useContext */ return new Property("useContext", "UsageContext", 5516 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate questionnaire instances.", 5517 0, java.lang.Integer.MAX_VALUE, useContext); 5518 case -507075711: 5519 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 5520 "A legal or geographic region in which the questionnaire is intended to be used.", 0, 5521 java.lang.Integer.MAX_VALUE, jurisdiction); 5522 case -220463842: 5523 /* purpose */ return new Property("purpose", "markdown", 5524 "Explanation of why this questionnaire is needed and why it has been designed as it has.", 0, 1, purpose); 5525 case 1522889671: 5526 /* copyright */ return new Property("copyright", "markdown", 5527 "A copyright statement relating to the questionnaire and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the questionnaire.", 5528 0, 1, copyright); 5529 case 223539345: 5530 /* approvalDate */ return new Property("approvalDate", "date", 5531 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 5532 0, 1, approvalDate); 5533 case -1687512484: 5534 /* lastReviewDate */ return new Property("lastReviewDate", "date", 5535 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 5536 0, 1, lastReviewDate); 5537 case -403934648: 5538 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 5539 "The period during which the questionnaire content was or is planned to be in active use.", 0, 1, 5540 effectivePeriod); 5541 case 3059181: 5542 /* code */ return new Property("code", "Coding", 5543 "An identifier for this question or group of questions in a particular terminology such as LOINC.", 0, 5544 java.lang.Integer.MAX_VALUE, code); 5545 case 3242771: 5546 /* item */ return new Property("item", "", 5547 "A particular question, question grouping or display text that is part of the questionnaire.", 0, 5548 java.lang.Integer.MAX_VALUE, item); 5549 default: 5550 return super.getNamedProperty(_hash, _name, _checkValid); 5551 } 5552 5553 } 5554 5555 @Override 5556 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5557 switch (hash) { 5558 case 116079: 5559 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5560 case -1618432855: 5561 /* identifier */ return this.identifier == null ? new Base[0] 5562 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5563 case 351608024: 5564 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 5565 case 3373707: 5566 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5567 case 110371416: 5568 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5569 case 1077922663: 5570 /* derivedFrom */ return this.derivedFrom == null ? new Base[0] 5571 : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // CanonicalType 5572 case -892481550: 5573 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 5574 case -404562712: 5575 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 5576 case -603200890: 5577 /* subjectType */ return this.subjectType == null ? new Base[0] 5578 : this.subjectType.toArray(new Base[this.subjectType.size()]); // CodeType 5579 case 3076014: 5580 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 5581 case 1447404028: 5582 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 5583 case 951526432: 5584 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5585 case -1724546052: 5586 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5587 case -669707736: 5588 /* useContext */ return this.useContext == null ? new Base[0] 5589 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5590 case -507075711: 5591 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5592 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5593 case -220463842: 5594 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 5595 case 1522889671: 5596 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5597 case 223539345: 5598 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 5599 case -1687512484: 5600 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 5601 case -403934648: 5602 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 5603 case 3059181: 5604 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 5605 case 3242771: 5606 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireItemComponent 5607 default: 5608 return super.getProperty(hash, name, checkValid); 5609 } 5610 5611 } 5612 5613 @Override 5614 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5615 switch (hash) { 5616 case 116079: // url 5617 this.url = castToUri(value); // UriType 5618 return value; 5619 case -1618432855: // identifier 5620 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5621 return value; 5622 case 351608024: // version 5623 this.version = castToString(value); // StringType 5624 return value; 5625 case 3373707: // name 5626 this.name = castToString(value); // StringType 5627 return value; 5628 case 110371416: // title 5629 this.title = castToString(value); // StringType 5630 return value; 5631 case 1077922663: // derivedFrom 5632 this.getDerivedFrom().add(castToCanonical(value)); // CanonicalType 5633 return value; 5634 case -892481550: // status 5635 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5636 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5637 return value; 5638 case -404562712: // experimental 5639 this.experimental = castToBoolean(value); // BooleanType 5640 return value; 5641 case -603200890: // subjectType 5642 this.getSubjectType().add(castToCode(value)); // CodeType 5643 return value; 5644 case 3076014: // date 5645 this.date = castToDateTime(value); // DateTimeType 5646 return value; 5647 case 1447404028: // publisher 5648 this.publisher = castToString(value); // StringType 5649 return value; 5650 case 951526432: // contact 5651 this.getContact().add(castToContactDetail(value)); // ContactDetail 5652 return value; 5653 case -1724546052: // description 5654 this.description = castToMarkdown(value); // MarkdownType 5655 return value; 5656 case -669707736: // useContext 5657 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5658 return value; 5659 case -507075711: // jurisdiction 5660 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5661 return value; 5662 case -220463842: // purpose 5663 this.purpose = castToMarkdown(value); // MarkdownType 5664 return value; 5665 case 1522889671: // copyright 5666 this.copyright = castToMarkdown(value); // MarkdownType 5667 return value; 5668 case 223539345: // approvalDate 5669 this.approvalDate = castToDate(value); // DateType 5670 return value; 5671 case -1687512484: // lastReviewDate 5672 this.lastReviewDate = castToDate(value); // DateType 5673 return value; 5674 case -403934648: // effectivePeriod 5675 this.effectivePeriod = castToPeriod(value); // Period 5676 return value; 5677 case 3059181: // code 5678 this.getCode().add(castToCoding(value)); // Coding 5679 return value; 5680 case 3242771: // item 5681 this.getItem().add((QuestionnaireItemComponent) value); // QuestionnaireItemComponent 5682 return value; 5683 default: 5684 return super.setProperty(hash, name, value); 5685 } 5686 5687 } 5688 5689 @Override 5690 public Base setProperty(String name, Base value) throws FHIRException { 5691 if (name.equals("url")) { 5692 this.url = castToUri(value); // UriType 5693 } else if (name.equals("identifier")) { 5694 this.getIdentifier().add(castToIdentifier(value)); 5695 } else if (name.equals("version")) { 5696 this.version = castToString(value); // StringType 5697 } else if (name.equals("name")) { 5698 this.name = castToString(value); // StringType 5699 } else if (name.equals("title")) { 5700 this.title = castToString(value); // StringType 5701 } else if (name.equals("derivedFrom")) { 5702 this.getDerivedFrom().add(castToCanonical(value)); 5703 } else if (name.equals("status")) { 5704 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5705 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5706 } else if (name.equals("experimental")) { 5707 this.experimental = castToBoolean(value); // BooleanType 5708 } else if (name.equals("subjectType")) { 5709 this.getSubjectType().add(castToCode(value)); 5710 } else if (name.equals("date")) { 5711 this.date = castToDateTime(value); // DateTimeType 5712 } else if (name.equals("publisher")) { 5713 this.publisher = castToString(value); // StringType 5714 } else if (name.equals("contact")) { 5715 this.getContact().add(castToContactDetail(value)); 5716 } else if (name.equals("description")) { 5717 this.description = castToMarkdown(value); // MarkdownType 5718 } else if (name.equals("useContext")) { 5719 this.getUseContext().add(castToUsageContext(value)); 5720 } else if (name.equals("jurisdiction")) { 5721 this.getJurisdiction().add(castToCodeableConcept(value)); 5722 } else if (name.equals("purpose")) { 5723 this.purpose = castToMarkdown(value); // MarkdownType 5724 } else if (name.equals("copyright")) { 5725 this.copyright = castToMarkdown(value); // MarkdownType 5726 } else if (name.equals("approvalDate")) { 5727 this.approvalDate = castToDate(value); // DateType 5728 } else if (name.equals("lastReviewDate")) { 5729 this.lastReviewDate = castToDate(value); // DateType 5730 } else if (name.equals("effectivePeriod")) { 5731 this.effectivePeriod = castToPeriod(value); // Period 5732 } else if (name.equals("code")) { 5733 this.getCode().add(castToCoding(value)); 5734 } else if (name.equals("item")) { 5735 this.getItem().add((QuestionnaireItemComponent) value); 5736 } else 5737 return super.setProperty(name, value); 5738 return value; 5739 } 5740 5741 @Override 5742 public void removeChild(String name, Base value) throws FHIRException { 5743 if (name.equals("url")) { 5744 this.url = null; 5745 } else if (name.equals("identifier")) { 5746 this.getIdentifier().remove(castToIdentifier(value)); 5747 } else if (name.equals("version")) { 5748 this.version = null; 5749 } else if (name.equals("name")) { 5750 this.name = null; 5751 } else if (name.equals("title")) { 5752 this.title = null; 5753 } else if (name.equals("derivedFrom")) { 5754 this.getDerivedFrom().remove(castToCanonical(value)); 5755 } else if (name.equals("status")) { 5756 this.status = null; 5757 } else if (name.equals("experimental")) { 5758 this.experimental = null; 5759 } else if (name.equals("subjectType")) { 5760 this.getSubjectType().remove(castToCode(value)); 5761 } else if (name.equals("date")) { 5762 this.date = null; 5763 } else if (name.equals("publisher")) { 5764 this.publisher = null; 5765 } else if (name.equals("contact")) { 5766 this.getContact().remove(castToContactDetail(value)); 5767 } else if (name.equals("description")) { 5768 this.description = null; 5769 } else if (name.equals("useContext")) { 5770 this.getUseContext().remove(castToUsageContext(value)); 5771 } else if (name.equals("jurisdiction")) { 5772 this.getJurisdiction().remove(castToCodeableConcept(value)); 5773 } else if (name.equals("purpose")) { 5774 this.purpose = null; 5775 } else if (name.equals("copyright")) { 5776 this.copyright = null; 5777 } else if (name.equals("approvalDate")) { 5778 this.approvalDate = null; 5779 } else if (name.equals("lastReviewDate")) { 5780 this.lastReviewDate = null; 5781 } else if (name.equals("effectivePeriod")) { 5782 this.effectivePeriod = null; 5783 } else if (name.equals("code")) { 5784 this.getCode().remove(castToCoding(value)); 5785 } else if (name.equals("item")) { 5786 this.getItem().remove((QuestionnaireItemComponent) value); 5787 } else 5788 super.removeChild(name, value); 5789 5790 } 5791 5792 @Override 5793 public Base makeProperty(int hash, String name) throws FHIRException { 5794 switch (hash) { 5795 case 116079: 5796 return getUrlElement(); 5797 case -1618432855: 5798 return addIdentifier(); 5799 case 351608024: 5800 return getVersionElement(); 5801 case 3373707: 5802 return getNameElement(); 5803 case 110371416: 5804 return getTitleElement(); 5805 case 1077922663: 5806 return addDerivedFromElement(); 5807 case -892481550: 5808 return getStatusElement(); 5809 case -404562712: 5810 return getExperimentalElement(); 5811 case -603200890: 5812 return addSubjectTypeElement(); 5813 case 3076014: 5814 return getDateElement(); 5815 case 1447404028: 5816 return getPublisherElement(); 5817 case 951526432: 5818 return addContact(); 5819 case -1724546052: 5820 return getDescriptionElement(); 5821 case -669707736: 5822 return addUseContext(); 5823 case -507075711: 5824 return addJurisdiction(); 5825 case -220463842: 5826 return getPurposeElement(); 5827 case 1522889671: 5828 return getCopyrightElement(); 5829 case 223539345: 5830 return getApprovalDateElement(); 5831 case -1687512484: 5832 return getLastReviewDateElement(); 5833 case -403934648: 5834 return getEffectivePeriod(); 5835 case 3059181: 5836 return addCode(); 5837 case 3242771: 5838 return addItem(); 5839 default: 5840 return super.makeProperty(hash, name); 5841 } 5842 5843 } 5844 5845 @Override 5846 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5847 switch (hash) { 5848 case 116079: 5849 /* url */ return new String[] { "uri" }; 5850 case -1618432855: 5851 /* identifier */ return new String[] { "Identifier" }; 5852 case 351608024: 5853 /* version */ return new String[] { "string" }; 5854 case 3373707: 5855 /* name */ return new String[] { "string" }; 5856 case 110371416: 5857 /* title */ return new String[] { "string" }; 5858 case 1077922663: 5859 /* derivedFrom */ return new String[] { "canonical" }; 5860 case -892481550: 5861 /* status */ return new String[] { "code" }; 5862 case -404562712: 5863 /* experimental */ return new String[] { "boolean" }; 5864 case -603200890: 5865 /* subjectType */ return new String[] { "code" }; 5866 case 3076014: 5867 /* date */ return new String[] { "dateTime" }; 5868 case 1447404028: 5869 /* publisher */ return new String[] { "string" }; 5870 case 951526432: 5871 /* contact */ return new String[] { "ContactDetail" }; 5872 case -1724546052: 5873 /* description */ return new String[] { "markdown" }; 5874 case -669707736: 5875 /* useContext */ return new String[] { "UsageContext" }; 5876 case -507075711: 5877 /* jurisdiction */ return new String[] { "CodeableConcept" }; 5878 case -220463842: 5879 /* purpose */ return new String[] { "markdown" }; 5880 case 1522889671: 5881 /* copyright */ return new String[] { "markdown" }; 5882 case 223539345: 5883 /* approvalDate */ return new String[] { "date" }; 5884 case -1687512484: 5885 /* lastReviewDate */ return new String[] { "date" }; 5886 case -403934648: 5887 /* effectivePeriod */ return new String[] { "Period" }; 5888 case 3059181: 5889 /* code */ return new String[] { "Coding" }; 5890 case 3242771: 5891 /* item */ return new String[] {}; 5892 default: 5893 return super.getTypesForProperty(hash, name); 5894 } 5895 5896 } 5897 5898 @Override 5899 public Base addChild(String name) throws FHIRException { 5900 if (name.equals("url")) { 5901 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.url"); 5902 } else if (name.equals("identifier")) { 5903 return addIdentifier(); 5904 } else if (name.equals("version")) { 5905 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.version"); 5906 } else if (name.equals("name")) { 5907 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.name"); 5908 } else if (name.equals("title")) { 5909 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.title"); 5910 } else if (name.equals("derivedFrom")) { 5911 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.derivedFrom"); 5912 } else if (name.equals("status")) { 5913 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.status"); 5914 } else if (name.equals("experimental")) { 5915 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.experimental"); 5916 } else if (name.equals("subjectType")) { 5917 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.subjectType"); 5918 } else if (name.equals("date")) { 5919 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.date"); 5920 } else if (name.equals("publisher")) { 5921 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.publisher"); 5922 } else if (name.equals("contact")) { 5923 return addContact(); 5924 } else if (name.equals("description")) { 5925 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.description"); 5926 } else if (name.equals("useContext")) { 5927 return addUseContext(); 5928 } else if (name.equals("jurisdiction")) { 5929 return addJurisdiction(); 5930 } else if (name.equals("purpose")) { 5931 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.purpose"); 5932 } else if (name.equals("copyright")) { 5933 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.copyright"); 5934 } else if (name.equals("approvalDate")) { 5935 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.approvalDate"); 5936 } else if (name.equals("lastReviewDate")) { 5937 throw new FHIRException("Cannot call addChild on a singleton property Questionnaire.lastReviewDate"); 5938 } else if (name.equals("effectivePeriod")) { 5939 this.effectivePeriod = new Period(); 5940 return this.effectivePeriod; 5941 } else if (name.equals("code")) { 5942 return addCode(); 5943 } else if (name.equals("item")) { 5944 return addItem(); 5945 } else 5946 return super.addChild(name); 5947 } 5948 5949 public String fhirType() { 5950 return "Questionnaire"; 5951 5952 } 5953 5954 public Questionnaire copy() { 5955 Questionnaire dst = new Questionnaire(); 5956 copyValues(dst); 5957 return dst; 5958 } 5959 5960 public void copyValues(Questionnaire dst) { 5961 super.copyValues(dst); 5962 dst.url = url == null ? null : url.copy(); 5963 if (identifier != null) { 5964 dst.identifier = new ArrayList<Identifier>(); 5965 for (Identifier i : identifier) 5966 dst.identifier.add(i.copy()); 5967 } 5968 ; 5969 dst.version = version == null ? null : version.copy(); 5970 dst.name = name == null ? null : name.copy(); 5971 dst.title = title == null ? null : title.copy(); 5972 if (derivedFrom != null) { 5973 dst.derivedFrom = new ArrayList<CanonicalType>(); 5974 for (CanonicalType i : derivedFrom) 5975 dst.derivedFrom.add(i.copy()); 5976 } 5977 ; 5978 dst.status = status == null ? null : status.copy(); 5979 dst.experimental = experimental == null ? null : experimental.copy(); 5980 if (subjectType != null) { 5981 dst.subjectType = new ArrayList<CodeType>(); 5982 for (CodeType i : subjectType) 5983 dst.subjectType.add(i.copy()); 5984 } 5985 ; 5986 dst.date = date == null ? null : date.copy(); 5987 dst.publisher = publisher == null ? null : publisher.copy(); 5988 if (contact != null) { 5989 dst.contact = new ArrayList<ContactDetail>(); 5990 for (ContactDetail i : contact) 5991 dst.contact.add(i.copy()); 5992 } 5993 ; 5994 dst.description = description == null ? null : description.copy(); 5995 if (useContext != null) { 5996 dst.useContext = new ArrayList<UsageContext>(); 5997 for (UsageContext i : useContext) 5998 dst.useContext.add(i.copy()); 5999 } 6000 ; 6001 if (jurisdiction != null) { 6002 dst.jurisdiction = new ArrayList<CodeableConcept>(); 6003 for (CodeableConcept i : jurisdiction) 6004 dst.jurisdiction.add(i.copy()); 6005 } 6006 ; 6007 dst.purpose = purpose == null ? null : purpose.copy(); 6008 dst.copyright = copyright == null ? null : copyright.copy(); 6009 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 6010 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 6011 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 6012 if (code != null) { 6013 dst.code = new ArrayList<Coding>(); 6014 for (Coding i : code) 6015 dst.code.add(i.copy()); 6016 } 6017 ; 6018 if (item != null) { 6019 dst.item = new ArrayList<QuestionnaireItemComponent>(); 6020 for (QuestionnaireItemComponent i : item) 6021 dst.item.add(i.copy()); 6022 } 6023 ; 6024 } 6025 6026 protected Questionnaire typedCopy() { 6027 return copy(); 6028 } 6029 6030 @Override 6031 public boolean equalsDeep(Base other_) { 6032 if (!super.equalsDeep(other_)) 6033 return false; 6034 if (!(other_ instanceof Questionnaire)) 6035 return false; 6036 Questionnaire o = (Questionnaire) other_; 6037 return compareDeep(identifier, o.identifier, true) && compareDeep(derivedFrom, o.derivedFrom, true) 6038 && compareDeep(subjectType, o.subjectType, true) && compareDeep(purpose, o.purpose, true) 6039 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 6040 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 6041 && compareDeep(code, o.code, true) && compareDeep(item, o.item, true); 6042 } 6043 6044 @Override 6045 public boolean equalsShallow(Base other_) { 6046 if (!super.equalsShallow(other_)) 6047 return false; 6048 if (!(other_ instanceof Questionnaire)) 6049 return false; 6050 Questionnaire o = (Questionnaire) other_; 6051 return compareValues(subjectType, o.subjectType, true) && compareValues(purpose, o.purpose, true) 6052 && compareValues(copyright, o.copyright, true) && compareValues(approvalDate, o.approvalDate, true) 6053 && compareValues(lastReviewDate, o.lastReviewDate, true); 6054 } 6055 6056 public boolean isEmpty() { 6057 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, derivedFrom, subjectType, purpose, 6058 copyright, approvalDate, lastReviewDate, effectivePeriod, code, item); 6059 } 6060 6061 @Override 6062 public ResourceType getResourceType() { 6063 return ResourceType.Questionnaire; 6064 } 6065 6066 /** 6067 * Search parameter: <b>date</b> 6068 * <p> 6069 * Description: <b>The questionnaire publication date</b><br> 6070 * Type: <b>date</b><br> 6071 * Path: <b>Questionnaire.date</b><br> 6072 * </p> 6073 */ 6074 @SearchParamDefinition(name = "date", path = "Questionnaire.date", description = "The questionnaire publication date", type = "date") 6075 public static final String SP_DATE = "date"; 6076 /** 6077 * <b>Fluent Client</b> search parameter constant for <b>date</b> 6078 * <p> 6079 * Description: <b>The questionnaire publication date</b><br> 6080 * Type: <b>date</b><br> 6081 * Path: <b>Questionnaire.date</b><br> 6082 * </p> 6083 */ 6084 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6085 SP_DATE); 6086 6087 /** 6088 * Search parameter: <b>identifier</b> 6089 * <p> 6090 * Description: <b>External identifier for the questionnaire</b><br> 6091 * Type: <b>token</b><br> 6092 * Path: <b>Questionnaire.identifier</b><br> 6093 * </p> 6094 */ 6095 @SearchParamDefinition(name = "identifier", path = "Questionnaire.identifier", description = "External identifier for the questionnaire", type = "token") 6096 public static final String SP_IDENTIFIER = "identifier"; 6097 /** 6098 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6099 * <p> 6100 * Description: <b>External identifier for the questionnaire</b><br> 6101 * Type: <b>token</b><br> 6102 * Path: <b>Questionnaire.identifier</b><br> 6103 * </p> 6104 */ 6105 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6106 SP_IDENTIFIER); 6107 6108 /** 6109 * Search parameter: <b>code</b> 6110 * <p> 6111 * Description: <b>A code that corresponds to one of its items in the 6112 * questionnaire</b><br> 6113 * Type: <b>token</b><br> 6114 * Path: <b>Questionnaire.item.code</b><br> 6115 * </p> 6116 */ 6117 @SearchParamDefinition(name = "code", path = "Questionnaire.item.code", description = "A code that corresponds to one of its items in the questionnaire", type = "token") 6118 public static final String SP_CODE = "code"; 6119 /** 6120 * <b>Fluent Client</b> search parameter constant for <b>code</b> 6121 * <p> 6122 * Description: <b>A code that corresponds to one of its items in the 6123 * questionnaire</b><br> 6124 * Type: <b>token</b><br> 6125 * Path: <b>Questionnaire.item.code</b><br> 6126 * </p> 6127 */ 6128 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6129 SP_CODE); 6130 6131 /** 6132 * Search parameter: <b>context-type-value</b> 6133 * <p> 6134 * Description: <b>A use context type and value assigned to the 6135 * questionnaire</b><br> 6136 * Type: <b>composite</b><br> 6137 * Path: <b></b><br> 6138 * </p> 6139 */ 6140 @SearchParamDefinition(name = "context-type-value", path = "Questionnaire.useContext", description = "A use context type and value assigned to the questionnaire", type = "composite", compositeOf = { 6141 "context-type", "context" }) 6142 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 6143 /** 6144 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 6145 * <p> 6146 * Description: <b>A use context type and value assigned to the 6147 * questionnaire</b><br> 6148 * Type: <b>composite</b><br> 6149 * Path: <b></b><br> 6150 * </p> 6151 */ 6152 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 6153 SP_CONTEXT_TYPE_VALUE); 6154 6155 /** 6156 * Search parameter: <b>jurisdiction</b> 6157 * <p> 6158 * Description: <b>Intended jurisdiction for the questionnaire</b><br> 6159 * Type: <b>token</b><br> 6160 * Path: <b>Questionnaire.jurisdiction</b><br> 6161 * </p> 6162 */ 6163 @SearchParamDefinition(name = "jurisdiction", path = "Questionnaire.jurisdiction", description = "Intended jurisdiction for the questionnaire", type = "token") 6164 public static final String SP_JURISDICTION = "jurisdiction"; 6165 /** 6166 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6167 * <p> 6168 * Description: <b>Intended jurisdiction for the questionnaire</b><br> 6169 * Type: <b>token</b><br> 6170 * Path: <b>Questionnaire.jurisdiction</b><br> 6171 * </p> 6172 */ 6173 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6174 SP_JURISDICTION); 6175 6176 /** 6177 * Search parameter: <b>description</b> 6178 * <p> 6179 * Description: <b>The description of the questionnaire</b><br> 6180 * Type: <b>string</b><br> 6181 * Path: <b>Questionnaire.description</b><br> 6182 * </p> 6183 */ 6184 @SearchParamDefinition(name = "description", path = "Questionnaire.description", description = "The description of the questionnaire", type = "string") 6185 public static final String SP_DESCRIPTION = "description"; 6186 /** 6187 * <b>Fluent Client</b> search parameter constant for <b>description</b> 6188 * <p> 6189 * Description: <b>The description of the questionnaire</b><br> 6190 * Type: <b>string</b><br> 6191 * Path: <b>Questionnaire.description</b><br> 6192 * </p> 6193 */ 6194 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 6195 SP_DESCRIPTION); 6196 6197 /** 6198 * Search parameter: <b>context-type</b> 6199 * <p> 6200 * Description: <b>A type of use context assigned to the questionnaire</b><br> 6201 * Type: <b>token</b><br> 6202 * Path: <b>Questionnaire.useContext.code</b><br> 6203 * </p> 6204 */ 6205 @SearchParamDefinition(name = "context-type", path = "Questionnaire.useContext.code", description = "A type of use context assigned to the questionnaire", type = "token") 6206 public static final String SP_CONTEXT_TYPE = "context-type"; 6207 /** 6208 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 6209 * <p> 6210 * Description: <b>A type of use context assigned to the questionnaire</b><br> 6211 * Type: <b>token</b><br> 6212 * Path: <b>Questionnaire.useContext.code</b><br> 6213 * </p> 6214 */ 6215 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6216 SP_CONTEXT_TYPE); 6217 6218 /** 6219 * Search parameter: <b>title</b> 6220 * <p> 6221 * Description: <b>The human-friendly name of the questionnaire</b><br> 6222 * Type: <b>string</b><br> 6223 * Path: <b>Questionnaire.title</b><br> 6224 * </p> 6225 */ 6226 @SearchParamDefinition(name = "title", path = "Questionnaire.title", description = "The human-friendly name of the questionnaire", type = "string") 6227 public static final String SP_TITLE = "title"; 6228 /** 6229 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6230 * <p> 6231 * Description: <b>The human-friendly name of the questionnaire</b><br> 6232 * Type: <b>string</b><br> 6233 * Path: <b>Questionnaire.title</b><br> 6234 * </p> 6235 */ 6236 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 6237 SP_TITLE); 6238 6239 /** 6240 * Search parameter: <b>version</b> 6241 * <p> 6242 * Description: <b>The business version of the questionnaire</b><br> 6243 * Type: <b>token</b><br> 6244 * Path: <b>Questionnaire.version</b><br> 6245 * </p> 6246 */ 6247 @SearchParamDefinition(name = "version", path = "Questionnaire.version", description = "The business version of the questionnaire", type = "token") 6248 public static final String SP_VERSION = "version"; 6249 /** 6250 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6251 * <p> 6252 * Description: <b>The business version of the questionnaire</b><br> 6253 * Type: <b>token</b><br> 6254 * Path: <b>Questionnaire.version</b><br> 6255 * </p> 6256 */ 6257 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6258 SP_VERSION); 6259 6260 /** 6261 * Search parameter: <b>url</b> 6262 * <p> 6263 * Description: <b>The uri that identifies the questionnaire</b><br> 6264 * Type: <b>uri</b><br> 6265 * Path: <b>Questionnaire.url</b><br> 6266 * </p> 6267 */ 6268 @SearchParamDefinition(name = "url", path = "Questionnaire.url", description = "The uri that identifies the questionnaire", type = "uri") 6269 public static final String SP_URL = "url"; 6270 /** 6271 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6272 * <p> 6273 * Description: <b>The uri that identifies the questionnaire</b><br> 6274 * Type: <b>uri</b><br> 6275 * Path: <b>Questionnaire.url</b><br> 6276 * </p> 6277 */ 6278 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6279 6280 /** 6281 * Search parameter: <b>context-quantity</b> 6282 * <p> 6283 * Description: <b>A quantity- or range-valued use context assigned to the 6284 * questionnaire</b><br> 6285 * Type: <b>quantity</b><br> 6286 * Path: <b>Questionnaire.useContext.valueQuantity, 6287 * Questionnaire.useContext.valueRange</b><br> 6288 * </p> 6289 */ 6290 @SearchParamDefinition(name = "context-quantity", path = "(Questionnaire.useContext.value as Quantity) | (Questionnaire.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the questionnaire", type = "quantity") 6291 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6292 /** 6293 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6294 * <p> 6295 * Description: <b>A quantity- or range-valued use context assigned to the 6296 * questionnaire</b><br> 6297 * Type: <b>quantity</b><br> 6298 * Path: <b>Questionnaire.useContext.valueQuantity, 6299 * Questionnaire.useContext.valueRange</b><br> 6300 * </p> 6301 */ 6302 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 6303 SP_CONTEXT_QUANTITY); 6304 6305 /** 6306 * Search parameter: <b>effective</b> 6307 * <p> 6308 * Description: <b>The time during which the questionnaire is intended to be in 6309 * use</b><br> 6310 * Type: <b>date</b><br> 6311 * Path: <b>Questionnaire.effectivePeriod</b><br> 6312 * </p> 6313 */ 6314 @SearchParamDefinition(name = "effective", path = "Questionnaire.effectivePeriod", description = "The time during which the questionnaire is intended to be in use", type = "date") 6315 public static final String SP_EFFECTIVE = "effective"; 6316 /** 6317 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 6318 * <p> 6319 * Description: <b>The time during which the questionnaire is intended to be in 6320 * use</b><br> 6321 * Type: <b>date</b><br> 6322 * Path: <b>Questionnaire.effectivePeriod</b><br> 6323 * </p> 6324 */ 6325 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6326 SP_EFFECTIVE); 6327 6328 /** 6329 * Search parameter: <b>subject-type</b> 6330 * <p> 6331 * Description: <b>Resource that can be subject of QuestionnaireResponse</b><br> 6332 * Type: <b>token</b><br> 6333 * Path: <b>Questionnaire.subjectType</b><br> 6334 * </p> 6335 */ 6336 @SearchParamDefinition(name = "subject-type", path = "Questionnaire.subjectType", description = "Resource that can be subject of QuestionnaireResponse", type = "token") 6337 public static final String SP_SUBJECT_TYPE = "subject-type"; 6338 /** 6339 * <b>Fluent Client</b> search parameter constant for <b>subject-type</b> 6340 * <p> 6341 * Description: <b>Resource that can be subject of QuestionnaireResponse</b><br> 6342 * Type: <b>token</b><br> 6343 * Path: <b>Questionnaire.subjectType</b><br> 6344 * </p> 6345 */ 6346 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBJECT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6347 SP_SUBJECT_TYPE); 6348 6349 /** 6350 * Search parameter: <b>name</b> 6351 * <p> 6352 * Description: <b>Computationally friendly name of the questionnaire</b><br> 6353 * Type: <b>string</b><br> 6354 * Path: <b>Questionnaire.name</b><br> 6355 * </p> 6356 */ 6357 @SearchParamDefinition(name = "name", path = "Questionnaire.name", description = "Computationally friendly name of the questionnaire", type = "string") 6358 public static final String SP_NAME = "name"; 6359 /** 6360 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6361 * <p> 6362 * Description: <b>Computationally friendly name of the questionnaire</b><br> 6363 * Type: <b>string</b><br> 6364 * Path: <b>Questionnaire.name</b><br> 6365 * </p> 6366 */ 6367 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 6368 SP_NAME); 6369 6370 /** 6371 * Search parameter: <b>context</b> 6372 * <p> 6373 * Description: <b>A use context assigned to the questionnaire</b><br> 6374 * Type: <b>token</b><br> 6375 * Path: <b>Questionnaire.useContext.valueCodeableConcept</b><br> 6376 * </p> 6377 */ 6378 @SearchParamDefinition(name = "context", path = "(Questionnaire.useContext.value as CodeableConcept)", description = "A use context assigned to the questionnaire", type = "token") 6379 public static final String SP_CONTEXT = "context"; 6380 /** 6381 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6382 * <p> 6383 * Description: <b>A use context assigned to the questionnaire</b><br> 6384 * Type: <b>token</b><br> 6385 * Path: <b>Questionnaire.useContext.valueCodeableConcept</b><br> 6386 * </p> 6387 */ 6388 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6389 SP_CONTEXT); 6390 6391 /** 6392 * Search parameter: <b>publisher</b> 6393 * <p> 6394 * Description: <b>Name of the publisher of the questionnaire</b><br> 6395 * Type: <b>string</b><br> 6396 * Path: <b>Questionnaire.publisher</b><br> 6397 * </p> 6398 */ 6399 @SearchParamDefinition(name = "publisher", path = "Questionnaire.publisher", description = "Name of the publisher of the questionnaire", type = "string") 6400 public static final String SP_PUBLISHER = "publisher"; 6401 /** 6402 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6403 * <p> 6404 * Description: <b>Name of the publisher of the questionnaire</b><br> 6405 * Type: <b>string</b><br> 6406 * Path: <b>Questionnaire.publisher</b><br> 6407 * </p> 6408 */ 6409 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 6410 SP_PUBLISHER); 6411 6412 /** 6413 * Search parameter: <b>definition</b> 6414 * <p> 6415 * Description: <b>ElementDefinition - details for the item</b><br> 6416 * Type: <b>uri</b><br> 6417 * Path: <b>Questionnaire.item.definition</b><br> 6418 * </p> 6419 */ 6420 @SearchParamDefinition(name = "definition", path = "Questionnaire.item.definition", description = "ElementDefinition - details for the item", type = "uri") 6421 public static final String SP_DEFINITION = "definition"; 6422 /** 6423 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 6424 * <p> 6425 * Description: <b>ElementDefinition - details for the item</b><br> 6426 * Type: <b>uri</b><br> 6427 * Path: <b>Questionnaire.item.definition</b><br> 6428 * </p> 6429 */ 6430 public static final ca.uhn.fhir.rest.gclient.UriClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.UriClientParam( 6431 SP_DEFINITION); 6432 6433 /** 6434 * Search parameter: <b>context-type-quantity</b> 6435 * <p> 6436 * Description: <b>A use context type and quantity- or range-based value 6437 * assigned to the questionnaire</b><br> 6438 * Type: <b>composite</b><br> 6439 * Path: <b></b><br> 6440 * </p> 6441 */ 6442 @SearchParamDefinition(name = "context-type-quantity", path = "Questionnaire.useContext", description = "A use context type and quantity- or range-based value assigned to the questionnaire", type = "composite", compositeOf = { 6443 "context-type", "context-quantity" }) 6444 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6445 /** 6446 * <b>Fluent Client</b> search parameter constant for 6447 * <b>context-type-quantity</b> 6448 * <p> 6449 * Description: <b>A use context type and quantity- or range-based value 6450 * assigned to the questionnaire</b><br> 6451 * Type: <b>composite</b><br> 6452 * Path: <b></b><br> 6453 * </p> 6454 */ 6455 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 6456 SP_CONTEXT_TYPE_QUANTITY); 6457 6458 /** 6459 * Search parameter: <b>status</b> 6460 * <p> 6461 * Description: <b>The current status of the questionnaire</b><br> 6462 * Type: <b>token</b><br> 6463 * Path: <b>Questionnaire.status</b><br> 6464 * </p> 6465 */ 6466 @SearchParamDefinition(name = "status", path = "Questionnaire.status", description = "The current status of the questionnaire", type = "token") 6467 public static final String SP_STATUS = "status"; 6468 /** 6469 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6470 * <p> 6471 * Description: <b>The current status of the questionnaire</b><br> 6472 * Type: <b>token</b><br> 6473 * Path: <b>Questionnaire.status</b><br> 6474 * </p> 6475 */ 6476 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6477 SP_STATUS); 6478 6479}