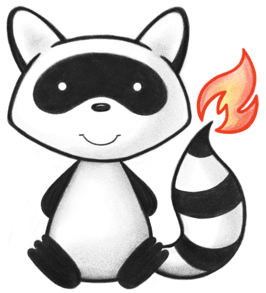
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A structured set of questions and their answers. The questions are ordered 049 * and grouped into coherent subsets, corresponding to the structure of the 050 * grouping of the questionnaire being responded to. 051 */ 052@ResourceDef(name = "QuestionnaireResponse", profile = "http://hl7.org/fhir/StructureDefinition/QuestionnaireResponse") 053public class QuestionnaireResponse extends DomainResource { 054 055 public enum QuestionnaireResponseStatus { 056 /** 057 * This QuestionnaireResponse has been partially filled out with answers but 058 * changes or additions are still expected to be made to it. 059 */ 060 INPROGRESS, 061 /** 062 * This QuestionnaireResponse has been filled out with answers and the current 063 * content is regarded as definitive. 064 */ 065 COMPLETED, 066 /** 067 * This QuestionnaireResponse has been filled out with answers, then marked as 068 * complete, yet changes or additions have been made to it afterwards. 069 */ 070 AMENDED, 071 /** 072 * This QuestionnaireResponse was entered in error and voided. 073 */ 074 ENTEREDINERROR, 075 /** 076 * This QuestionnaireResponse has been partially filled out with answers but has 077 * been abandoned. It is unknown whether changes or additions are expected to be 078 * made to it. 079 */ 080 STOPPED, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 086 public static QuestionnaireResponseStatus fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("in-progress".equals(codeString)) 090 return INPROGRESS; 091 if ("completed".equals(codeString)) 092 return COMPLETED; 093 if ("amended".equals(codeString)) 094 return AMENDED; 095 if ("entered-in-error".equals(codeString)) 096 return ENTEREDINERROR; 097 if ("stopped".equals(codeString)) 098 return STOPPED; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case INPROGRESS: 108 return "in-progress"; 109 case COMPLETED: 110 return "completed"; 111 case AMENDED: 112 return "amended"; 113 case ENTEREDINERROR: 114 return "entered-in-error"; 115 case STOPPED: 116 return "stopped"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getSystem() { 125 switch (this) { 126 case INPROGRESS: 127 return "http://hl7.org/fhir/questionnaire-answers-status"; 128 case COMPLETED: 129 return "http://hl7.org/fhir/questionnaire-answers-status"; 130 case AMENDED: 131 return "http://hl7.org/fhir/questionnaire-answers-status"; 132 case ENTEREDINERROR: 133 return "http://hl7.org/fhir/questionnaire-answers-status"; 134 case STOPPED: 135 return "http://hl7.org/fhir/questionnaire-answers-status"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDefinition() { 144 switch (this) { 145 case INPROGRESS: 146 return "This QuestionnaireResponse has been partially filled out with answers but changes or additions are still expected to be made to it."; 147 case COMPLETED: 148 return "This QuestionnaireResponse has been filled out with answers and the current content is regarded as definitive."; 149 case AMENDED: 150 return "This QuestionnaireResponse has been filled out with answers, then marked as complete, yet changes or additions have been made to it afterwards."; 151 case ENTEREDINERROR: 152 return "This QuestionnaireResponse was entered in error and voided."; 153 case STOPPED: 154 return "This QuestionnaireResponse has been partially filled out with answers but has been abandoned. It is unknown whether changes or additions are expected to be made to it."; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 162 public String getDisplay() { 163 switch (this) { 164 case INPROGRESS: 165 return "In Progress"; 166 case COMPLETED: 167 return "Completed"; 168 case AMENDED: 169 return "Amended"; 170 case ENTEREDINERROR: 171 return "Entered in Error"; 172 case STOPPED: 173 return "Stopped"; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 } 181 182 public static class QuestionnaireResponseStatusEnumFactory implements EnumFactory<QuestionnaireResponseStatus> { 183 public QuestionnaireResponseStatus fromCode(String codeString) throws IllegalArgumentException { 184 if (codeString == null || "".equals(codeString)) 185 if (codeString == null || "".equals(codeString)) 186 return null; 187 if ("in-progress".equals(codeString)) 188 return QuestionnaireResponseStatus.INPROGRESS; 189 if ("completed".equals(codeString)) 190 return QuestionnaireResponseStatus.COMPLETED; 191 if ("amended".equals(codeString)) 192 return QuestionnaireResponseStatus.AMENDED; 193 if ("entered-in-error".equals(codeString)) 194 return QuestionnaireResponseStatus.ENTEREDINERROR; 195 if ("stopped".equals(codeString)) 196 return QuestionnaireResponseStatus.STOPPED; 197 throw new IllegalArgumentException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 198 } 199 200 public Enumeration<QuestionnaireResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 201 if (code == null) 202 return null; 203 if (code.isEmpty()) 204 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.NULL, code); 205 String codeString = code.asStringValue(); 206 if (codeString == null || "".equals(codeString)) 207 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.NULL, code); 208 if ("in-progress".equals(codeString)) 209 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.INPROGRESS, code); 210 if ("completed".equals(codeString)) 211 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.COMPLETED, code); 212 if ("amended".equals(codeString)) 213 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.AMENDED, code); 214 if ("entered-in-error".equals(codeString)) 215 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.ENTEREDINERROR, code); 216 if ("stopped".equals(codeString)) 217 return new Enumeration<QuestionnaireResponseStatus>(this, QuestionnaireResponseStatus.STOPPED, code); 218 throw new FHIRException("Unknown QuestionnaireResponseStatus code '" + codeString + "'"); 219 } 220 221 public String toCode(QuestionnaireResponseStatus code) { 222 if (code == QuestionnaireResponseStatus.NULL) 223 return null; 224 if (code == QuestionnaireResponseStatus.INPROGRESS) 225 return "in-progress"; 226 if (code == QuestionnaireResponseStatus.COMPLETED) 227 return "completed"; 228 if (code == QuestionnaireResponseStatus.AMENDED) 229 return "amended"; 230 if (code == QuestionnaireResponseStatus.ENTEREDINERROR) 231 return "entered-in-error"; 232 if (code == QuestionnaireResponseStatus.STOPPED) 233 return "stopped"; 234 return "?"; 235 } 236 237 public String toSystem(QuestionnaireResponseStatus code) { 238 return code.getSystem(); 239 } 240 } 241 242 @Block() 243 public static class QuestionnaireResponseItemComponent extends BackboneElement implements IBaseBackboneElement { 244 /** 245 * The item from the Questionnaire that corresponds to this item in the 246 * QuestionnaireResponse resource. 247 */ 248 @Child(name = "linkId", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 249 @Description(shortDefinition = "Pointer to specific item from Questionnaire", formalDefinition = "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.") 250 protected StringType linkId; 251 252 /** 253 * A reference to an [[[ElementDefinition]]] that provides the details for the 254 * item. 255 */ 256 @Child(name = "definition", type = { 257 UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 258 @Description(shortDefinition = "ElementDefinition - details for the item", formalDefinition = "A reference to an [[[ElementDefinition]]] that provides the details for the item.") 259 protected UriType definition; 260 261 /** 262 * Text that is displayed above the contents of the group or as the text of the 263 * question being answered. 264 */ 265 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 266 @Description(shortDefinition = "Name for group or question text", formalDefinition = "Text that is displayed above the contents of the group or as the text of the question being answered.") 267 protected StringType text; 268 269 /** 270 * The respondent's answer(s) to the question. 271 */ 272 @Child(name = "answer", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 273 @Description(shortDefinition = "The response(s) to the question", formalDefinition = "The respondent's answer(s) to the question.") 274 protected List<QuestionnaireResponseItemAnswerComponent> answer; 275 276 /** 277 * Questions or sub-groups nested beneath a question or group. 278 */ 279 @Child(name = "item", type = { 280 QuestionnaireResponseItemComponent.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 281 @Description(shortDefinition = "Nested questionnaire response items", formalDefinition = "Questions or sub-groups nested beneath a question or group.") 282 protected List<QuestionnaireResponseItemComponent> item; 283 284 private static final long serialVersionUID = -1395483402L; 285 286 /** 287 * Constructor 288 */ 289 public QuestionnaireResponseItemComponent() { 290 super(); 291 } 292 293 /** 294 * Constructor 295 */ 296 public QuestionnaireResponseItemComponent(StringType linkId) { 297 super(); 298 this.linkId = linkId; 299 } 300 301 /** 302 * @return {@link #linkId} (The item from the Questionnaire that corresponds to 303 * this item in the QuestionnaireResponse resource.). This is the 304 * underlying object with id, value and extensions. The accessor 305 * "getLinkId" gives direct access to the value 306 */ 307 public StringType getLinkIdElement() { 308 if (this.linkId == null) 309 if (Configuration.errorOnAutoCreate()) 310 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.linkId"); 311 else if (Configuration.doAutoCreate()) 312 this.linkId = new StringType(); // bb 313 return this.linkId; 314 } 315 316 public boolean hasLinkIdElement() { 317 return this.linkId != null && !this.linkId.isEmpty(); 318 } 319 320 public boolean hasLinkId() { 321 return this.linkId != null && !this.linkId.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #linkId} (The item from the Questionnaire that 326 * corresponds to this item in the QuestionnaireResponse 327 * resource.). This is the underlying object with id, value and 328 * extensions. The accessor "getLinkId" gives direct access to the 329 * value 330 */ 331 public QuestionnaireResponseItemComponent setLinkIdElement(StringType value) { 332 this.linkId = value; 333 return this; 334 } 335 336 /** 337 * @return The item from the Questionnaire that corresponds to this item in the 338 * QuestionnaireResponse resource. 339 */ 340 public String getLinkId() { 341 return this.linkId == null ? null : this.linkId.getValue(); 342 } 343 344 /** 345 * @param value The item from the Questionnaire that corresponds to this item in 346 * the QuestionnaireResponse resource. 347 */ 348 public QuestionnaireResponseItemComponent setLinkId(String value) { 349 if (this.linkId == null) 350 this.linkId = new StringType(); 351 this.linkId.setValue(value); 352 return this; 353 } 354 355 /** 356 * @return {@link #definition} (A reference to an [[[ElementDefinition]]] that 357 * provides the details for the item.). This is the underlying object 358 * with id, value and extensions. The accessor "getDefinition" gives 359 * direct access to the value 360 */ 361 public UriType getDefinitionElement() { 362 if (this.definition == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.definition"); 365 else if (Configuration.doAutoCreate()) 366 this.definition = new UriType(); // bb 367 return this.definition; 368 } 369 370 public boolean hasDefinitionElement() { 371 return this.definition != null && !this.definition.isEmpty(); 372 } 373 374 public boolean hasDefinition() { 375 return this.definition != null && !this.definition.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #definition} (A reference to an [[[ElementDefinition]]] 380 * that provides the details for the item.). This is the underlying 381 * object with id, value and extensions. The accessor 382 * "getDefinition" gives direct access to the value 383 */ 384 public QuestionnaireResponseItemComponent setDefinitionElement(UriType value) { 385 this.definition = value; 386 return this; 387 } 388 389 /** 390 * @return A reference to an [[[ElementDefinition]]] that provides the details 391 * for the item. 392 */ 393 public String getDefinition() { 394 return this.definition == null ? null : this.definition.getValue(); 395 } 396 397 /** 398 * @param value A reference to an [[[ElementDefinition]]] that provides the 399 * details for the item. 400 */ 401 public QuestionnaireResponseItemComponent setDefinition(String value) { 402 if (Utilities.noString(value)) 403 this.definition = null; 404 else { 405 if (this.definition == null) 406 this.definition = new UriType(); 407 this.definition.setValue(value); 408 } 409 return this; 410 } 411 412 /** 413 * @return {@link #text} (Text that is displayed above the contents of the group 414 * or as the text of the question being answered.). This is the 415 * underlying object with id, value and extensions. The accessor 416 * "getText" gives direct access to the value 417 */ 418 public StringType getTextElement() { 419 if (this.text == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create QuestionnaireResponseItemComponent.text"); 422 else if (Configuration.doAutoCreate()) 423 this.text = new StringType(); // bb 424 return this.text; 425 } 426 427 public boolean hasTextElement() { 428 return this.text != null && !this.text.isEmpty(); 429 } 430 431 public boolean hasText() { 432 return this.text != null && !this.text.isEmpty(); 433 } 434 435 /** 436 * @param value {@link #text} (Text that is displayed above the contents of the 437 * group or as the text of the question being answered.). This is 438 * the underlying object with id, value and extensions. The 439 * accessor "getText" gives direct access to the value 440 */ 441 public QuestionnaireResponseItemComponent setTextElement(StringType value) { 442 this.text = value; 443 return this; 444 } 445 446 /** 447 * @return Text that is displayed above the contents of the group or as the text 448 * of the question being answered. 449 */ 450 public String getText() { 451 return this.text == null ? null : this.text.getValue(); 452 } 453 454 /** 455 * @param value Text that is displayed above the contents of the group or as the 456 * text of the question being answered. 457 */ 458 public QuestionnaireResponseItemComponent setText(String value) { 459 if (Utilities.noString(value)) 460 this.text = null; 461 else { 462 if (this.text == null) 463 this.text = new StringType(); 464 this.text.setValue(value); 465 } 466 return this; 467 } 468 469 /** 470 * @return {@link #answer} (The respondent's answer(s) to the question.) 471 */ 472 public List<QuestionnaireResponseItemAnswerComponent> getAnswer() { 473 if (this.answer == null) 474 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 475 return this.answer; 476 } 477 478 /** 479 * @return Returns a reference to <code>this</code> for easy method chaining 480 */ 481 public QuestionnaireResponseItemComponent setAnswer(List<QuestionnaireResponseItemAnswerComponent> theAnswer) { 482 this.answer = theAnswer; 483 return this; 484 } 485 486 public boolean hasAnswer() { 487 if (this.answer == null) 488 return false; 489 for (QuestionnaireResponseItemAnswerComponent item : this.answer) 490 if (!item.isEmpty()) 491 return true; 492 return false; 493 } 494 495 public QuestionnaireResponseItemAnswerComponent addAnswer() { // 3 496 QuestionnaireResponseItemAnswerComponent t = new QuestionnaireResponseItemAnswerComponent(); 497 if (this.answer == null) 498 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 499 this.answer.add(t); 500 return t; 501 } 502 503 public QuestionnaireResponseItemComponent addAnswer(QuestionnaireResponseItemAnswerComponent t) { // 3 504 if (t == null) 505 return this; 506 if (this.answer == null) 507 this.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 508 this.answer.add(t); 509 return this; 510 } 511 512 /** 513 * @return The first repetition of repeating field {@link #answer}, creating it 514 * if it does not already exist 515 */ 516 public QuestionnaireResponseItemAnswerComponent getAnswerFirstRep() { 517 if (getAnswer().isEmpty()) { 518 addAnswer(); 519 } 520 return getAnswer().get(0); 521 } 522 523 /** 524 * @return {@link #item} (Questions or sub-groups nested beneath a question or 525 * group.) 526 */ 527 public List<QuestionnaireResponseItemComponent> getItem() { 528 if (this.item == null) 529 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 530 return this.item; 531 } 532 533 /** 534 * @return Returns a reference to <code>this</code> for easy method chaining 535 */ 536 public QuestionnaireResponseItemComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 537 this.item = theItem; 538 return this; 539 } 540 541 public boolean hasItem() { 542 if (this.item == null) 543 return false; 544 for (QuestionnaireResponseItemComponent item : this.item) 545 if (!item.isEmpty()) 546 return true; 547 return false; 548 } 549 550 public QuestionnaireResponseItemComponent addItem() { // 3 551 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 552 if (this.item == null) 553 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 554 this.item.add(t); 555 return t; 556 } 557 558 public QuestionnaireResponseItemComponent addItem(QuestionnaireResponseItemComponent t) { // 3 559 if (t == null) 560 return this; 561 if (this.item == null) 562 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 563 this.item.add(t); 564 return this; 565 } 566 567 /** 568 * @return The first repetition of repeating field {@link #item}, creating it if 569 * it does not already exist 570 */ 571 public QuestionnaireResponseItemComponent getItemFirstRep() { 572 if (getItem().isEmpty()) { 573 addItem(); 574 } 575 return getItem().get(0); 576 } 577 578 protected void listChildren(List<Property> children) { 579 super.listChildren(children); 580 children.add(new Property("linkId", "string", 581 "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 1, 582 linkId)); 583 children.add(new Property("definition", "uri", 584 "A reference to an [[[ElementDefinition]]] that provides the details for the item.", 0, 1, definition)); 585 children.add(new Property("text", "string", 586 "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 1, 587 text)); 588 children.add(new Property("answer", "", "The respondent's answer(s) to the question.", 0, 589 java.lang.Integer.MAX_VALUE, answer)); 590 children.add(new Property("item", "@QuestionnaireResponse.item", 591 "Questions or sub-groups nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item)); 592 } 593 594 @Override 595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 596 switch (_hash) { 597 case -1102667083: 598 /* linkId */ return new Property("linkId", "string", 599 "The item from the Questionnaire that corresponds to this item in the QuestionnaireResponse resource.", 0, 600 1, linkId); 601 case -1014418093: 602 /* definition */ return new Property("definition", "uri", 603 "A reference to an [[[ElementDefinition]]] that provides the details for the item.", 0, 1, definition); 604 case 3556653: 605 /* text */ return new Property("text", "string", 606 "Text that is displayed above the contents of the group or as the text of the question being answered.", 0, 607 1, text); 608 case -1412808770: 609 /* answer */ return new Property("answer", "", "The respondent's answer(s) to the question.", 0, 610 java.lang.Integer.MAX_VALUE, answer); 611 case 3242771: 612 /* item */ return new Property("item", "@QuestionnaireResponse.item", 613 "Questions or sub-groups nested beneath a question or group.", 0, java.lang.Integer.MAX_VALUE, item); 614 default: 615 return super.getNamedProperty(_hash, _name, _checkValid); 616 } 617 618 } 619 620 @Override 621 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 622 switch (hash) { 623 case -1102667083: 624 /* linkId */ return this.linkId == null ? new Base[0] : new Base[] { this.linkId }; // StringType 625 case -1014418093: 626 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // UriType 627 case 3556653: 628 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 629 case -1412808770: 630 /* answer */ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // QuestionnaireResponseItemAnswerComponent 631 case 3242771: 632 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 633 default: 634 return super.getProperty(hash, name, checkValid); 635 } 636 637 } 638 639 @Override 640 public Base setProperty(int hash, String name, Base value) throws FHIRException { 641 switch (hash) { 642 case -1102667083: // linkId 643 this.linkId = castToString(value); // StringType 644 return value; 645 case -1014418093: // definition 646 this.definition = castToUri(value); // UriType 647 return value; 648 case 3556653: // text 649 this.text = castToString(value); // StringType 650 return value; 651 case -1412808770: // answer 652 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); // QuestionnaireResponseItemAnswerComponent 653 return value; 654 case 3242771: // item 655 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 656 return value; 657 default: 658 return super.setProperty(hash, name, value); 659 } 660 661 } 662 663 @Override 664 public Base setProperty(String name, Base value) throws FHIRException { 665 if (name.equals("linkId")) { 666 this.linkId = castToString(value); // StringType 667 } else if (name.equals("definition")) { 668 this.definition = castToUri(value); // UriType 669 } else if (name.equals("text")) { 670 this.text = castToString(value); // StringType 671 } else if (name.equals("answer")) { 672 this.getAnswer().add((QuestionnaireResponseItemAnswerComponent) value); 673 } else if (name.equals("item")) { 674 this.getItem().add((QuestionnaireResponseItemComponent) value); 675 } else 676 return super.setProperty(name, value); 677 return value; 678 } 679 680 @Override 681 public void removeChild(String name, Base value) throws FHIRException { 682 if (name.equals("linkId")) { 683 this.linkId = null; 684 } else if (name.equals("definition")) { 685 this.definition = null; 686 } else if (name.equals("text")) { 687 this.text = null; 688 } else if (name.equals("answer")) { 689 this.getAnswer().remove((QuestionnaireResponseItemAnswerComponent) value); 690 } else if (name.equals("item")) { 691 this.getItem().remove((QuestionnaireResponseItemComponent) value); 692 } else 693 super.removeChild(name, value); 694 695 } 696 697 @Override 698 public Base makeProperty(int hash, String name) throws FHIRException { 699 switch (hash) { 700 case -1102667083: 701 return getLinkIdElement(); 702 case -1014418093: 703 return getDefinitionElement(); 704 case 3556653: 705 return getTextElement(); 706 case -1412808770: 707 return addAnswer(); 708 case 3242771: 709 return addItem(); 710 default: 711 return super.makeProperty(hash, name); 712 } 713 714 } 715 716 @Override 717 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 718 switch (hash) { 719 case -1102667083: 720 /* linkId */ return new String[] { "string" }; 721 case -1014418093: 722 /* definition */ return new String[] { "uri" }; 723 case 3556653: 724 /* text */ return new String[] { "string" }; 725 case -1412808770: 726 /* answer */ return new String[] {}; 727 case 3242771: 728 /* item */ return new String[] { "@QuestionnaireResponse.item" }; 729 default: 730 return super.getTypesForProperty(hash, name); 731 } 732 733 } 734 735 @Override 736 public Base addChild(String name) throws FHIRException { 737 if (name.equals("linkId")) { 738 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.linkId"); 739 } else if (name.equals("definition")) { 740 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.definition"); 741 } else if (name.equals("text")) { 742 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.text"); 743 } else if (name.equals("answer")) { 744 return addAnswer(); 745 } else if (name.equals("item")) { 746 return addItem(); 747 } else 748 return super.addChild(name); 749 } 750 751 public QuestionnaireResponseItemComponent copy() { 752 QuestionnaireResponseItemComponent dst = new QuestionnaireResponseItemComponent(); 753 copyValues(dst); 754 return dst; 755 } 756 757 public void copyValues(QuestionnaireResponseItemComponent dst) { 758 super.copyValues(dst); 759 dst.linkId = linkId == null ? null : linkId.copy(); 760 dst.definition = definition == null ? null : definition.copy(); 761 dst.text = text == null ? null : text.copy(); 762 if (answer != null) { 763 dst.answer = new ArrayList<QuestionnaireResponseItemAnswerComponent>(); 764 for (QuestionnaireResponseItemAnswerComponent i : answer) 765 dst.answer.add(i.copy()); 766 } 767 ; 768 if (item != null) { 769 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 770 for (QuestionnaireResponseItemComponent i : item) 771 dst.item.add(i.copy()); 772 } 773 ; 774 } 775 776 @Override 777 public boolean equalsDeep(Base other_) { 778 if (!super.equalsDeep(other_)) 779 return false; 780 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 781 return false; 782 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 783 return compareDeep(linkId, o.linkId, true) && compareDeep(definition, o.definition, true) 784 && compareDeep(text, o.text, true) && compareDeep(answer, o.answer, true) && compareDeep(item, o.item, true); 785 } 786 787 @Override 788 public boolean equalsShallow(Base other_) { 789 if (!super.equalsShallow(other_)) 790 return false; 791 if (!(other_ instanceof QuestionnaireResponseItemComponent)) 792 return false; 793 QuestionnaireResponseItemComponent o = (QuestionnaireResponseItemComponent) other_; 794 return compareValues(linkId, o.linkId, true) && compareValues(definition, o.definition, true) 795 && compareValues(text, o.text, true); 796 } 797 798 public boolean isEmpty() { 799 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, definition, text, answer, item); 800 } 801 802 public String fhirType() { 803 return "QuestionnaireResponse.item"; 804 805 } 806 807 } 808 809 @Block() 810 public static class QuestionnaireResponseItemAnswerComponent extends BackboneElement implements IBaseBackboneElement { 811 /** 812 * The answer (or one of the answers) provided by the respondent to the 813 * question. 814 */ 815 @Child(name = "value", type = { BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, 816 DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, 817 Quantity.class, Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 818 @Description(shortDefinition = "Single-valued answer to the question", formalDefinition = "The answer (or one of the answers) provided by the respondent to the question.") 819 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-answers") 820 protected Type value; 821 822 /** 823 * Nested groups and/or questions found within this particular answer. 824 */ 825 @Child(name = "item", type = { 826 QuestionnaireResponseItemComponent.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 827 @Description(shortDefinition = "Nested groups and questions", formalDefinition = "Nested groups and/or questions found within this particular answer.") 828 protected List<QuestionnaireResponseItemComponent> item; 829 830 private static final long serialVersionUID = 2052422636L; 831 832 /** 833 * Constructor 834 */ 835 public QuestionnaireResponseItemAnswerComponent() { 836 super(); 837 } 838 839 /** 840 * @return {@link #value} (The answer (or one of the answers) provided by the 841 * respondent to the question.) 842 */ 843 public Type getValue() { 844 return this.value; 845 } 846 847 /** 848 * @return {@link #value} (The answer (or one of the answers) provided by the 849 * respondent to the question.) 850 */ 851 public BooleanType getValueBooleanType() throws FHIRException { 852 if (this.value == null) 853 this.value = new BooleanType(); 854 if (!(this.value instanceof BooleanType)) 855 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 856 + this.value.getClass().getName() + " was encountered"); 857 return (BooleanType) this.value; 858 } 859 860 public boolean hasValueBooleanType() { 861 return this != null && this.value instanceof BooleanType; 862 } 863 864 /** 865 * @return {@link #value} (The answer (or one of the answers) provided by the 866 * respondent to the question.) 867 */ 868 public DecimalType getValueDecimalType() throws FHIRException { 869 if (this.value == null) 870 this.value = new DecimalType(); 871 if (!(this.value instanceof DecimalType)) 872 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 873 + this.value.getClass().getName() + " was encountered"); 874 return (DecimalType) this.value; 875 } 876 877 public boolean hasValueDecimalType() { 878 return this != null && this.value instanceof DecimalType; 879 } 880 881 /** 882 * @return {@link #value} (The answer (or one of the answers) provided by the 883 * respondent to the question.) 884 */ 885 public IntegerType getValueIntegerType() throws FHIRException { 886 if (this.value == null) 887 this.value = new IntegerType(); 888 if (!(this.value instanceof IntegerType)) 889 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 890 + this.value.getClass().getName() + " was encountered"); 891 return (IntegerType) this.value; 892 } 893 894 public boolean hasValueIntegerType() { 895 return this != null && this.value instanceof IntegerType; 896 } 897 898 /** 899 * @return {@link #value} (The answer (or one of the answers) provided by the 900 * respondent to the question.) 901 */ 902 public DateType getValueDateType() throws FHIRException { 903 if (this.value == null) 904 this.value = new DateType(); 905 if (!(this.value instanceof DateType)) 906 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.value.getClass().getName() 907 + " was encountered"); 908 return (DateType) this.value; 909 } 910 911 public boolean hasValueDateType() { 912 return this != null && this.value instanceof DateType; 913 } 914 915 /** 916 * @return {@link #value} (The answer (or one of the answers) provided by the 917 * respondent to the question.) 918 */ 919 public DateTimeType getValueDateTimeType() throws FHIRException { 920 if (this.value == null) 921 this.value = new DateTimeType(); 922 if (!(this.value instanceof DateTimeType)) 923 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 924 + this.value.getClass().getName() + " was encountered"); 925 return (DateTimeType) this.value; 926 } 927 928 public boolean hasValueDateTimeType() { 929 return this != null && this.value instanceof DateTimeType; 930 } 931 932 /** 933 * @return {@link #value} (The answer (or one of the answers) provided by the 934 * respondent to the question.) 935 */ 936 public TimeType getValueTimeType() throws FHIRException { 937 if (this.value == null) 938 this.value = new TimeType(); 939 if (!(this.value instanceof TimeType)) 940 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 941 + " was encountered"); 942 return (TimeType) this.value; 943 } 944 945 public boolean hasValueTimeType() { 946 return this != null && this.value instanceof TimeType; 947 } 948 949 /** 950 * @return {@link #value} (The answer (or one of the answers) provided by the 951 * respondent to the question.) 952 */ 953 public StringType getValueStringType() throws FHIRException { 954 if (this.value == null) 955 this.value = new StringType(); 956 if (!(this.value instanceof StringType)) 957 throw new FHIRException("Type mismatch: the type StringType was expected, but " 958 + this.value.getClass().getName() + " was encountered"); 959 return (StringType) this.value; 960 } 961 962 public boolean hasValueStringType() { 963 return this != null && this.value instanceof StringType; 964 } 965 966 /** 967 * @return {@link #value} (The answer (or one of the answers) provided by the 968 * respondent to the question.) 969 */ 970 public UriType getValueUriType() throws FHIRException { 971 if (this.value == null) 972 this.value = new UriType(); 973 if (!(this.value instanceof UriType)) 974 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName() 975 + " was encountered"); 976 return (UriType) this.value; 977 } 978 979 public boolean hasValueUriType() { 980 return this != null && this.value instanceof UriType; 981 } 982 983 /** 984 * @return {@link #value} (The answer (or one of the answers) provided by the 985 * respondent to the question.) 986 */ 987 public Attachment getValueAttachment() throws FHIRException { 988 if (this.value == null) 989 this.value = new Attachment(); 990 if (!(this.value instanceof Attachment)) 991 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 992 + this.value.getClass().getName() + " was encountered"); 993 return (Attachment) this.value; 994 } 995 996 public boolean hasValueAttachment() { 997 return this != null && this.value instanceof Attachment; 998 } 999 1000 /** 1001 * @return {@link #value} (The answer (or one of the answers) provided by the 1002 * respondent to the question.) 1003 */ 1004 public Coding getValueCoding() throws FHIRException { 1005 if (this.value == null) 1006 this.value = new Coding(); 1007 if (!(this.value instanceof Coding)) 1008 throw new FHIRException( 1009 "Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered"); 1010 return (Coding) this.value; 1011 } 1012 1013 public boolean hasValueCoding() { 1014 return this != null && this.value instanceof Coding; 1015 } 1016 1017 /** 1018 * @return {@link #value} (The answer (or one of the answers) provided by the 1019 * respondent to the question.) 1020 */ 1021 public Quantity getValueQuantity() throws FHIRException { 1022 if (this.value == null) 1023 this.value = new Quantity(); 1024 if (!(this.value instanceof Quantity)) 1025 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 1026 + " was encountered"); 1027 return (Quantity) this.value; 1028 } 1029 1030 public boolean hasValueQuantity() { 1031 return this != null && this.value instanceof Quantity; 1032 } 1033 1034 /** 1035 * @return {@link #value} (The answer (or one of the answers) provided by the 1036 * respondent to the question.) 1037 */ 1038 public Reference getValueReference() throws FHIRException { 1039 if (this.value == null) 1040 this.value = new Reference(); 1041 if (!(this.value instanceof Reference)) 1042 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 1043 + " was encountered"); 1044 return (Reference) this.value; 1045 } 1046 1047 public boolean hasValueReference() { 1048 return this != null && this.value instanceof Reference; 1049 } 1050 1051 public boolean hasValue() { 1052 return this.value != null && !this.value.isEmpty(); 1053 } 1054 1055 /** 1056 * @param value {@link #value} (The answer (or one of the answers) provided by 1057 * the respondent to the question.) 1058 */ 1059 public QuestionnaireResponseItemAnswerComponent setValue(Type value) { 1060 if (value != null 1061 && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType 1062 || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType 1063 || value instanceof StringType || value instanceof UriType || value instanceof Attachment 1064 || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 1065 throw new Error("Not the right type for QuestionnaireResponse.item.answer.value[x]: " + value.fhirType()); 1066 this.value = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #item} (Nested groups and/or questions found within this 1072 * particular answer.) 1073 */ 1074 public List<QuestionnaireResponseItemComponent> getItem() { 1075 if (this.item == null) 1076 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1077 return this.item; 1078 } 1079 1080 /** 1081 * @return Returns a reference to <code>this</code> for easy method chaining 1082 */ 1083 public QuestionnaireResponseItemAnswerComponent setItem(List<QuestionnaireResponseItemComponent> theItem) { 1084 this.item = theItem; 1085 return this; 1086 } 1087 1088 public boolean hasItem() { 1089 if (this.item == null) 1090 return false; 1091 for (QuestionnaireResponseItemComponent item : this.item) 1092 if (!item.isEmpty()) 1093 return true; 1094 return false; 1095 } 1096 1097 public QuestionnaireResponseItemComponent addItem() { // 3 1098 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 1099 if (this.item == null) 1100 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1101 this.item.add(t); 1102 return t; 1103 } 1104 1105 public QuestionnaireResponseItemAnswerComponent addItem(QuestionnaireResponseItemComponent t) { // 3 1106 if (t == null) 1107 return this; 1108 if (this.item == null) 1109 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1110 this.item.add(t); 1111 return this; 1112 } 1113 1114 /** 1115 * @return The first repetition of repeating field {@link #item}, creating it if 1116 * it does not already exist 1117 */ 1118 public QuestionnaireResponseItemComponent getItemFirstRep() { 1119 if (getItem().isEmpty()) { 1120 addItem(); 1121 } 1122 return getItem().get(0); 1123 } 1124 1125 protected void listChildren(List<Property> children) { 1126 super.listChildren(children); 1127 children.add(new Property("value[x]", 1128 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1129 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value)); 1130 children.add(new Property("item", "@QuestionnaireResponse.item", 1131 "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, item)); 1132 } 1133 1134 @Override 1135 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1136 switch (_hash) { 1137 case -1410166417: 1138 /* value[x] */ return new Property("value[x]", 1139 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1140 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1141 case 111972721: 1142 /* value */ return new Property("value[x]", 1143 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1144 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1145 case 733421943: 1146 /* valueBoolean */ return new Property("value[x]", 1147 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1148 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1149 case -2083993440: 1150 /* valueDecimal */ return new Property("value[x]", 1151 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1152 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1153 case -1668204915: 1154 /* valueInteger */ return new Property("value[x]", 1155 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1156 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1157 case -766192449: 1158 /* valueDate */ return new Property("value[x]", 1159 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1160 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1161 case 1047929900: 1162 /* valueDateTime */ return new Property("value[x]", 1163 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1164 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1165 case -765708322: 1166 /* valueTime */ return new Property("value[x]", 1167 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1168 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1169 case -1424603934: 1170 /* valueString */ return new Property("value[x]", 1171 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1172 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1173 case -1410172357: 1174 /* valueUri */ return new Property("value[x]", 1175 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1176 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1177 case -475566732: 1178 /* valueAttachment */ return new Property("value[x]", 1179 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1180 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1181 case -1887705029: 1182 /* valueCoding */ return new Property("value[x]", 1183 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1184 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1185 case -2029823716: 1186 /* valueQuantity */ return new Property("value[x]", 1187 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1188 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1189 case 1755241690: 1190 /* valueReference */ return new Property("value[x]", 1191 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 1192 "The answer (or one of the answers) provided by the respondent to the question.", 0, 1, value); 1193 case 3242771: 1194 /* item */ return new Property("item", "@QuestionnaireResponse.item", 1195 "Nested groups and/or questions found within this particular answer.", 0, java.lang.Integer.MAX_VALUE, 1196 item); 1197 default: 1198 return super.getNamedProperty(_hash, _name, _checkValid); 1199 } 1200 1201 } 1202 1203 @Override 1204 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1205 switch (hash) { 1206 case 111972721: 1207 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 1208 case 3242771: 1209 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 1210 default: 1211 return super.getProperty(hash, name, checkValid); 1212 } 1213 1214 } 1215 1216 @Override 1217 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1218 switch (hash) { 1219 case 111972721: // value 1220 this.value = castToType(value); // Type 1221 return value; 1222 case 3242771: // item 1223 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 1224 return value; 1225 default: 1226 return super.setProperty(hash, name, value); 1227 } 1228 1229 } 1230 1231 @Override 1232 public Base setProperty(String name, Base value) throws FHIRException { 1233 if (name.equals("value[x]")) { 1234 this.value = castToType(value); // Type 1235 } else if (name.equals("item")) { 1236 this.getItem().add((QuestionnaireResponseItemComponent) value); 1237 } else 1238 return super.setProperty(name, value); 1239 return value; 1240 } 1241 1242 @Override 1243 public void removeChild(String name, Base value) throws FHIRException { 1244 if (name.equals("value[x]")) { 1245 this.value = null; 1246 } else if (name.equals("item")) { 1247 this.getItem().remove((QuestionnaireResponseItemComponent) value); 1248 } else 1249 super.removeChild(name, value); 1250 1251 } 1252 1253 @Override 1254 public Base makeProperty(int hash, String name) throws FHIRException { 1255 switch (hash) { 1256 case -1410166417: 1257 return getValue(); 1258 case 111972721: 1259 return getValue(); 1260 case 3242771: 1261 return addItem(); 1262 default: 1263 return super.makeProperty(hash, name); 1264 } 1265 1266 } 1267 1268 @Override 1269 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1270 switch (hash) { 1271 case 111972721: 1272 /* value */ return new String[] { "boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", 1273 "Attachment", "Coding", "Quantity", "Reference" }; 1274 case 3242771: 1275 /* item */ return new String[] { "@QuestionnaireResponse.item" }; 1276 default: 1277 return super.getTypesForProperty(hash, name); 1278 } 1279 1280 } 1281 1282 @Override 1283 public Base addChild(String name) throws FHIRException { 1284 if (name.equals("valueBoolean")) { 1285 this.value = new BooleanType(); 1286 return this.value; 1287 } else if (name.equals("valueDecimal")) { 1288 this.value = new DecimalType(); 1289 return this.value; 1290 } else if (name.equals("valueInteger")) { 1291 this.value = new IntegerType(); 1292 return this.value; 1293 } else if (name.equals("valueDate")) { 1294 this.value = new DateType(); 1295 return this.value; 1296 } else if (name.equals("valueDateTime")) { 1297 this.value = new DateTimeType(); 1298 return this.value; 1299 } else if (name.equals("valueTime")) { 1300 this.value = new TimeType(); 1301 return this.value; 1302 } else if (name.equals("valueString")) { 1303 this.value = new StringType(); 1304 return this.value; 1305 } else if (name.equals("valueUri")) { 1306 this.value = new UriType(); 1307 return this.value; 1308 } else if (name.equals("valueAttachment")) { 1309 this.value = new Attachment(); 1310 return this.value; 1311 } else if (name.equals("valueCoding")) { 1312 this.value = new Coding(); 1313 return this.value; 1314 } else if (name.equals("valueQuantity")) { 1315 this.value = new Quantity(); 1316 return this.value; 1317 } else if (name.equals("valueReference")) { 1318 this.value = new Reference(); 1319 return this.value; 1320 } else if (name.equals("item")) { 1321 return addItem(); 1322 } else 1323 return super.addChild(name); 1324 } 1325 1326 public QuestionnaireResponseItemAnswerComponent copy() { 1327 QuestionnaireResponseItemAnswerComponent dst = new QuestionnaireResponseItemAnswerComponent(); 1328 copyValues(dst); 1329 return dst; 1330 } 1331 1332 public void copyValues(QuestionnaireResponseItemAnswerComponent dst) { 1333 super.copyValues(dst); 1334 dst.value = value == null ? null : value.copy(); 1335 if (item != null) { 1336 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 1337 for (QuestionnaireResponseItemComponent i : item) 1338 dst.item.add(i.copy()); 1339 } 1340 ; 1341 } 1342 1343 @Override 1344 public boolean equalsDeep(Base other_) { 1345 if (!super.equalsDeep(other_)) 1346 return false; 1347 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1348 return false; 1349 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1350 return compareDeep(value, o.value, true) && compareDeep(item, o.item, true); 1351 } 1352 1353 @Override 1354 public boolean equalsShallow(Base other_) { 1355 if (!super.equalsShallow(other_)) 1356 return false; 1357 if (!(other_ instanceof QuestionnaireResponseItemAnswerComponent)) 1358 return false; 1359 QuestionnaireResponseItemAnswerComponent o = (QuestionnaireResponseItemAnswerComponent) other_; 1360 return true; 1361 } 1362 1363 public boolean isEmpty() { 1364 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, item); 1365 } 1366 1367 public String fhirType() { 1368 return "QuestionnaireResponse.item.answer"; 1369 1370 } 1371 1372 } 1373 1374 /** 1375 * A business identifier assigned to a particular completed (or partially 1376 * completed) questionnaire. 1377 */ 1378 @Child(name = "identifier", type = { 1379 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1380 @Description(shortDefinition = "Unique id for this set of answers", formalDefinition = "A business identifier assigned to a particular completed (or partially completed) questionnaire.") 1381 protected Identifier identifier; 1382 1383 /** 1384 * The order, proposal or plan that is fulfilled in whole or in part by this 1385 * QuestionnaireResponse. For example, a ServiceRequest seeking an intake 1386 * assessment or a decision support recommendation to assess for post-partum 1387 * depression. 1388 */ 1389 @Child(name = "basedOn", type = { CarePlan.class, 1390 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1391 @Description(shortDefinition = "Request fulfilled by this QuestionnaireResponse", formalDefinition = "The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.") 1392 protected List<Reference> basedOn; 1393 /** 1394 * The actual objects that are the target of the reference (The order, proposal 1395 * or plan that is fulfilled in whole or in part by this QuestionnaireResponse. 1396 * For example, a ServiceRequest seeking an intake assessment or a decision 1397 * support recommendation to assess for post-partum depression.) 1398 */ 1399 protected List<Resource> basedOnTarget; 1400 1401 /** 1402 * A procedure or observation that this questionnaire was performed as part of 1403 * the execution of. For example, the surgery a checklist was executed as part 1404 * of. 1405 */ 1406 @Child(name = "partOf", type = { Observation.class, 1407 Procedure.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1408 @Description(shortDefinition = "Part of this action", formalDefinition = "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.") 1409 protected List<Reference> partOf; 1410 /** 1411 * The actual objects that are the target of the reference (A procedure or 1412 * observation that this questionnaire was performed as part of the execution 1413 * of. For example, the surgery a checklist was executed as part of.) 1414 */ 1415 protected List<Resource> partOfTarget; 1416 1417 /** 1418 * The Questionnaire that defines and organizes the questions for which answers 1419 * are being provided. 1420 */ 1421 @Child(name = "questionnaire", type = { 1422 CanonicalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1423 @Description(shortDefinition = "Form being answered", formalDefinition = "The Questionnaire that defines and organizes the questions for which answers are being provided.") 1424 protected CanonicalType questionnaire; 1425 1426 /** 1427 * The position of the questionnaire response within its overall lifecycle. 1428 */ 1429 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 1430 @Description(shortDefinition = "in-progress | completed | amended | entered-in-error | stopped", formalDefinition = "The position of the questionnaire response within its overall lifecycle.") 1431 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/questionnaire-answers-status") 1432 protected Enumeration<QuestionnaireResponseStatus> status; 1433 1434 /** 1435 * The subject of the questionnaire response. This could be a patient, 1436 * organization, practitioner, device, etc. This is who/what the answers apply 1437 * to, but is not necessarily the source of information. 1438 */ 1439 @Child(name = "subject", type = { Reference.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1440 @Description(shortDefinition = "The subject of the questions", formalDefinition = "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.") 1441 protected Reference subject; 1442 1443 /** 1444 * The actual object that is the target of the reference (The subject of the 1445 * questionnaire response. This could be a patient, organization, practitioner, 1446 * device, etc. This is who/what the answers apply to, but is not necessarily 1447 * the source of information.) 1448 */ 1449 protected Resource subjectTarget; 1450 1451 /** 1452 * The Encounter during which this questionnaire response was created or to 1453 * which the creation of this record is tightly associated. 1454 */ 1455 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1456 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.") 1457 protected Reference encounter; 1458 1459 /** 1460 * The actual object that is the target of the reference (The Encounter during 1461 * which this questionnaire response was created or to which the creation of 1462 * this record is tightly associated.) 1463 */ 1464 protected Encounter encounterTarget; 1465 1466 /** 1467 * The date and/or time that this set of answers were last changed. 1468 */ 1469 @Child(name = "authored", type = { 1470 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1471 @Description(shortDefinition = "Date the answers were gathered", formalDefinition = "The date and/or time that this set of answers were last changed.") 1472 protected DateTimeType authored; 1473 1474 /** 1475 * Person who received the answers to the questions in the QuestionnaireResponse 1476 * and recorded them in the system. 1477 */ 1478 @Child(name = "author", type = { Device.class, Practitioner.class, PractitionerRole.class, Patient.class, 1479 RelatedPerson.class, Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1480 @Description(shortDefinition = "Person who received and recorded the answers", formalDefinition = "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.") 1481 protected Reference author; 1482 1483 /** 1484 * The actual object that is the target of the reference (Person who received 1485 * the answers to the questions in the QuestionnaireResponse and recorded them 1486 * in the system.) 1487 */ 1488 protected Resource authorTarget; 1489 1490 /** 1491 * The person who answered the questions about the subject. 1492 */ 1493 @Child(name = "source", type = { Patient.class, Practitioner.class, PractitionerRole.class, 1494 RelatedPerson.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1495 @Description(shortDefinition = "The person who answered the questions", formalDefinition = "The person who answered the questions about the subject.") 1496 protected Reference source; 1497 1498 /** 1499 * The actual object that is the target of the reference (The person who 1500 * answered the questions about the subject.) 1501 */ 1502 protected Resource sourceTarget; 1503 1504 /** 1505 * A group or question item from the original questionnaire for which answers 1506 * are provided. 1507 */ 1508 @Child(name = "item", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1509 @Description(shortDefinition = "Groups and questions", formalDefinition = "A group or question item from the original questionnaire for which answers are provided.") 1510 protected List<QuestionnaireResponseItemComponent> item; 1511 1512 private static final long serialVersionUID = -259908687L; 1513 1514 /** 1515 * Constructor 1516 */ 1517 public QuestionnaireResponse() { 1518 super(); 1519 } 1520 1521 /** 1522 * Constructor 1523 */ 1524 public QuestionnaireResponse(Enumeration<QuestionnaireResponseStatus> status) { 1525 super(); 1526 this.status = status; 1527 } 1528 1529 /** 1530 * @return {@link #identifier} (A business identifier assigned to a particular 1531 * completed (or partially completed) questionnaire.) 1532 */ 1533 public Identifier getIdentifier() { 1534 if (this.identifier == null) 1535 if (Configuration.errorOnAutoCreate()) 1536 throw new Error("Attempt to auto-create QuestionnaireResponse.identifier"); 1537 else if (Configuration.doAutoCreate()) 1538 this.identifier = new Identifier(); // cc 1539 return this.identifier; 1540 } 1541 1542 public boolean hasIdentifier() { 1543 return this.identifier != null && !this.identifier.isEmpty(); 1544 } 1545 1546 /** 1547 * @param value {@link #identifier} (A business identifier assigned to a 1548 * particular completed (or partially completed) questionnaire.) 1549 */ 1550 public QuestionnaireResponse setIdentifier(Identifier value) { 1551 this.identifier = value; 1552 return this; 1553 } 1554 1555 /** 1556 * @return {@link #basedOn} (The order, proposal or plan that is fulfilled in 1557 * whole or in part by this QuestionnaireResponse. For example, a 1558 * ServiceRequest seeking an intake assessment or a decision support 1559 * recommendation to assess for post-partum depression.) 1560 */ 1561 public List<Reference> getBasedOn() { 1562 if (this.basedOn == null) 1563 this.basedOn = new ArrayList<Reference>(); 1564 return this.basedOn; 1565 } 1566 1567 /** 1568 * @return Returns a reference to <code>this</code> for easy method chaining 1569 */ 1570 public QuestionnaireResponse setBasedOn(List<Reference> theBasedOn) { 1571 this.basedOn = theBasedOn; 1572 return this; 1573 } 1574 1575 public boolean hasBasedOn() { 1576 if (this.basedOn == null) 1577 return false; 1578 for (Reference item : this.basedOn) 1579 if (!item.isEmpty()) 1580 return true; 1581 return false; 1582 } 1583 1584 public Reference addBasedOn() { // 3 1585 Reference t = new Reference(); 1586 if (this.basedOn == null) 1587 this.basedOn = new ArrayList<Reference>(); 1588 this.basedOn.add(t); 1589 return t; 1590 } 1591 1592 public QuestionnaireResponse addBasedOn(Reference t) { // 3 1593 if (t == null) 1594 return this; 1595 if (this.basedOn == null) 1596 this.basedOn = new ArrayList<Reference>(); 1597 this.basedOn.add(t); 1598 return this; 1599 } 1600 1601 /** 1602 * @return The first repetition of repeating field {@link #basedOn}, creating it 1603 * if it does not already exist 1604 */ 1605 public Reference getBasedOnFirstRep() { 1606 if (getBasedOn().isEmpty()) { 1607 addBasedOn(); 1608 } 1609 return getBasedOn().get(0); 1610 } 1611 1612 /** 1613 * @deprecated Use Reference#setResource(IBaseResource) instead 1614 */ 1615 @Deprecated 1616 public List<Resource> getBasedOnTarget() { 1617 if (this.basedOnTarget == null) 1618 this.basedOnTarget = new ArrayList<Resource>(); 1619 return this.basedOnTarget; 1620 } 1621 1622 /** 1623 * @return {@link #partOf} (A procedure or observation that this questionnaire 1624 * was performed as part of the execution of. For example, the surgery a 1625 * checklist was executed as part of.) 1626 */ 1627 public List<Reference> getPartOf() { 1628 if (this.partOf == null) 1629 this.partOf = new ArrayList<Reference>(); 1630 return this.partOf; 1631 } 1632 1633 /** 1634 * @return Returns a reference to <code>this</code> for easy method chaining 1635 */ 1636 public QuestionnaireResponse setPartOf(List<Reference> thePartOf) { 1637 this.partOf = thePartOf; 1638 return this; 1639 } 1640 1641 public boolean hasPartOf() { 1642 if (this.partOf == null) 1643 return false; 1644 for (Reference item : this.partOf) 1645 if (!item.isEmpty()) 1646 return true; 1647 return false; 1648 } 1649 1650 public Reference addPartOf() { // 3 1651 Reference t = new Reference(); 1652 if (this.partOf == null) 1653 this.partOf = new ArrayList<Reference>(); 1654 this.partOf.add(t); 1655 return t; 1656 } 1657 1658 public QuestionnaireResponse addPartOf(Reference t) { // 3 1659 if (t == null) 1660 return this; 1661 if (this.partOf == null) 1662 this.partOf = new ArrayList<Reference>(); 1663 this.partOf.add(t); 1664 return this; 1665 } 1666 1667 /** 1668 * @return The first repetition of repeating field {@link #partOf}, creating it 1669 * if it does not already exist 1670 */ 1671 public Reference getPartOfFirstRep() { 1672 if (getPartOf().isEmpty()) { 1673 addPartOf(); 1674 } 1675 return getPartOf().get(0); 1676 } 1677 1678 /** 1679 * @deprecated Use Reference#setResource(IBaseResource) instead 1680 */ 1681 @Deprecated 1682 public List<Resource> getPartOfTarget() { 1683 if (this.partOfTarget == null) 1684 this.partOfTarget = new ArrayList<Resource>(); 1685 return this.partOfTarget; 1686 } 1687 1688 /** 1689 * @return {@link #questionnaire} (The Questionnaire that defines and organizes 1690 * the questions for which answers are being provided.). This is the 1691 * underlying object with id, value and extensions. The accessor 1692 * "getQuestionnaire" gives direct access to the value 1693 */ 1694 public CanonicalType getQuestionnaireElement() { 1695 if (this.questionnaire == null) 1696 if (Configuration.errorOnAutoCreate()) 1697 throw new Error("Attempt to auto-create QuestionnaireResponse.questionnaire"); 1698 else if (Configuration.doAutoCreate()) 1699 this.questionnaire = new CanonicalType(); // bb 1700 return this.questionnaire; 1701 } 1702 1703 public boolean hasQuestionnaireElement() { 1704 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1705 } 1706 1707 public boolean hasQuestionnaire() { 1708 return this.questionnaire != null && !this.questionnaire.isEmpty(); 1709 } 1710 1711 /** 1712 * @param value {@link #questionnaire} (The Questionnaire that defines and 1713 * organizes the questions for which answers are being provided.). 1714 * This is the underlying object with id, value and extensions. The 1715 * accessor "getQuestionnaire" gives direct access to the value 1716 */ 1717 public QuestionnaireResponse setQuestionnaireElement(CanonicalType value) { 1718 this.questionnaire = value; 1719 return this; 1720 } 1721 1722 /** 1723 * @return The Questionnaire that defines and organizes the questions for which 1724 * answers are being provided. 1725 */ 1726 public String getQuestionnaire() { 1727 return this.questionnaire == null ? null : this.questionnaire.getValue(); 1728 } 1729 1730 /** 1731 * @param value The Questionnaire that defines and organizes the questions for 1732 * which answers are being provided. 1733 */ 1734 public QuestionnaireResponse setQuestionnaire(String value) { 1735 if (Utilities.noString(value)) 1736 this.questionnaire = null; 1737 else { 1738 if (this.questionnaire == null) 1739 this.questionnaire = new CanonicalType(); 1740 this.questionnaire.setValue(value); 1741 } 1742 return this; 1743 } 1744 1745 /** 1746 * @return {@link #status} (The position of the questionnaire response within 1747 * its overall lifecycle.). This is the underlying object with id, value 1748 * and extensions. The accessor "getStatus" gives direct access to the 1749 * value 1750 */ 1751 public Enumeration<QuestionnaireResponseStatus> getStatusElement() { 1752 if (this.status == null) 1753 if (Configuration.errorOnAutoCreate()) 1754 throw new Error("Attempt to auto-create QuestionnaireResponse.status"); 1755 else if (Configuration.doAutoCreate()) 1756 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); // bb 1757 return this.status; 1758 } 1759 1760 public boolean hasStatusElement() { 1761 return this.status != null && !this.status.isEmpty(); 1762 } 1763 1764 public boolean hasStatus() { 1765 return this.status != null && !this.status.isEmpty(); 1766 } 1767 1768 /** 1769 * @param value {@link #status} (The position of the questionnaire response 1770 * within its overall lifecycle.). This is the underlying object 1771 * with id, value and extensions. The accessor "getStatus" gives 1772 * direct access to the value 1773 */ 1774 public QuestionnaireResponse setStatusElement(Enumeration<QuestionnaireResponseStatus> value) { 1775 this.status = value; 1776 return this; 1777 } 1778 1779 /** 1780 * @return The position of the questionnaire response within its overall 1781 * lifecycle. 1782 */ 1783 public QuestionnaireResponseStatus getStatus() { 1784 return this.status == null ? null : this.status.getValue(); 1785 } 1786 1787 /** 1788 * @param value The position of the questionnaire response within its overall 1789 * lifecycle. 1790 */ 1791 public QuestionnaireResponse setStatus(QuestionnaireResponseStatus value) { 1792 if (this.status == null) 1793 this.status = new Enumeration<QuestionnaireResponseStatus>(new QuestionnaireResponseStatusEnumFactory()); 1794 this.status.setValue(value); 1795 return this; 1796 } 1797 1798 /** 1799 * @return {@link #subject} (The subject of the questionnaire response. This 1800 * could be a patient, organization, practitioner, device, etc. This is 1801 * who/what the answers apply to, but is not necessarily the source of 1802 * information.) 1803 */ 1804 public Reference getSubject() { 1805 if (this.subject == null) 1806 if (Configuration.errorOnAutoCreate()) 1807 throw new Error("Attempt to auto-create QuestionnaireResponse.subject"); 1808 else if (Configuration.doAutoCreate()) 1809 this.subject = new Reference(); // cc 1810 return this.subject; 1811 } 1812 1813 public boolean hasSubject() { 1814 return this.subject != null && !this.subject.isEmpty(); 1815 } 1816 1817 /** 1818 * @param value {@link #subject} (The subject of the questionnaire response. 1819 * This could be a patient, organization, practitioner, device, 1820 * etc. This is who/what the answers apply to, but is not 1821 * necessarily the source of information.) 1822 */ 1823 public QuestionnaireResponse setSubject(Reference value) { 1824 this.subject = value; 1825 return this; 1826 } 1827 1828 /** 1829 * @return {@link #subject} The actual object that is the target of the 1830 * reference. The reference library doesn't populate this, but you can 1831 * use it to hold the resource if you resolve it. (The subject of the 1832 * questionnaire response. This could be a patient, organization, 1833 * practitioner, device, etc. This is who/what the answers apply to, but 1834 * is not necessarily the source of information.) 1835 */ 1836 public Resource getSubjectTarget() { 1837 return this.subjectTarget; 1838 } 1839 1840 /** 1841 * @param value {@link #subject} The actual object that is the target of the 1842 * reference. The reference library doesn't use these, but you can 1843 * use it to hold the resource if you resolve it. (The subject of 1844 * the questionnaire response. This could be a patient, 1845 * organization, practitioner, device, etc. This is who/what the 1846 * answers apply to, but is not necessarily the source of 1847 * information.) 1848 */ 1849 public QuestionnaireResponse setSubjectTarget(Resource value) { 1850 this.subjectTarget = value; 1851 return this; 1852 } 1853 1854 /** 1855 * @return {@link #encounter} (The Encounter during which this questionnaire 1856 * response was created or to which the creation of this record is 1857 * tightly associated.) 1858 */ 1859 public Reference getEncounter() { 1860 if (this.encounter == null) 1861 if (Configuration.errorOnAutoCreate()) 1862 throw new Error("Attempt to auto-create QuestionnaireResponse.encounter"); 1863 else if (Configuration.doAutoCreate()) 1864 this.encounter = new Reference(); // cc 1865 return this.encounter; 1866 } 1867 1868 public boolean hasEncounter() { 1869 return this.encounter != null && !this.encounter.isEmpty(); 1870 } 1871 1872 /** 1873 * @param value {@link #encounter} (The Encounter during which this 1874 * questionnaire response was created or to which the creation of 1875 * this record is tightly associated.) 1876 */ 1877 public QuestionnaireResponse setEncounter(Reference value) { 1878 this.encounter = value; 1879 return this; 1880 } 1881 1882 /** 1883 * @return {@link #encounter} The actual object that is the target of the 1884 * reference. The reference library doesn't populate this, but you can 1885 * use it to hold the resource if you resolve it. (The Encounter during 1886 * which this questionnaire response was created or to which the 1887 * creation of this record is tightly associated.) 1888 */ 1889 public Encounter getEncounterTarget() { 1890 if (this.encounterTarget == null) 1891 if (Configuration.errorOnAutoCreate()) 1892 throw new Error("Attempt to auto-create QuestionnaireResponse.encounter"); 1893 else if (Configuration.doAutoCreate()) 1894 this.encounterTarget = new Encounter(); // aa 1895 return this.encounterTarget; 1896 } 1897 1898 /** 1899 * @param value {@link #encounter} The actual object that is the target of the 1900 * reference. The reference library doesn't use these, but you can 1901 * use it to hold the resource if you resolve it. (The Encounter 1902 * during which this questionnaire response was created or to which 1903 * the creation of this record is tightly associated.) 1904 */ 1905 public QuestionnaireResponse setEncounterTarget(Encounter value) { 1906 this.encounterTarget = value; 1907 return this; 1908 } 1909 1910 /** 1911 * @return {@link #authored} (The date and/or time that this set of answers were 1912 * last changed.). This is the underlying object with id, value and 1913 * extensions. The accessor "getAuthored" gives direct access to the 1914 * value 1915 */ 1916 public DateTimeType getAuthoredElement() { 1917 if (this.authored == null) 1918 if (Configuration.errorOnAutoCreate()) 1919 throw new Error("Attempt to auto-create QuestionnaireResponse.authored"); 1920 else if (Configuration.doAutoCreate()) 1921 this.authored = new DateTimeType(); // bb 1922 return this.authored; 1923 } 1924 1925 public boolean hasAuthoredElement() { 1926 return this.authored != null && !this.authored.isEmpty(); 1927 } 1928 1929 public boolean hasAuthored() { 1930 return this.authored != null && !this.authored.isEmpty(); 1931 } 1932 1933 /** 1934 * @param value {@link #authored} (The date and/or time that this set of answers 1935 * were last changed.). This is the underlying object with id, 1936 * value and extensions. The accessor "getAuthored" gives direct 1937 * access to the value 1938 */ 1939 public QuestionnaireResponse setAuthoredElement(DateTimeType value) { 1940 this.authored = value; 1941 return this; 1942 } 1943 1944 /** 1945 * @return The date and/or time that this set of answers were last changed. 1946 */ 1947 public Date getAuthored() { 1948 return this.authored == null ? null : this.authored.getValue(); 1949 } 1950 1951 /** 1952 * @param value The date and/or time that this set of answers were last changed. 1953 */ 1954 public QuestionnaireResponse setAuthored(Date value) { 1955 if (value == null) 1956 this.authored = null; 1957 else { 1958 if (this.authored == null) 1959 this.authored = new DateTimeType(); 1960 this.authored.setValue(value); 1961 } 1962 return this; 1963 } 1964 1965 /** 1966 * @return {@link #author} (Person who received the answers to the questions in 1967 * the QuestionnaireResponse and recorded them in the system.) 1968 */ 1969 public Reference getAuthor() { 1970 if (this.author == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create QuestionnaireResponse.author"); 1973 else if (Configuration.doAutoCreate()) 1974 this.author = new Reference(); // cc 1975 return this.author; 1976 } 1977 1978 public boolean hasAuthor() { 1979 return this.author != null && !this.author.isEmpty(); 1980 } 1981 1982 /** 1983 * @param value {@link #author} (Person who received the answers to the 1984 * questions in the QuestionnaireResponse and recorded them in the 1985 * system.) 1986 */ 1987 public QuestionnaireResponse setAuthor(Reference value) { 1988 this.author = value; 1989 return this; 1990 } 1991 1992 /** 1993 * @return {@link #author} The actual object that is the target of the 1994 * reference. The reference library doesn't populate this, but you can 1995 * use it to hold the resource if you resolve it. (Person who received 1996 * the answers to the questions in the QuestionnaireResponse and 1997 * recorded them in the system.) 1998 */ 1999 public Resource getAuthorTarget() { 2000 return this.authorTarget; 2001 } 2002 2003 /** 2004 * @param value {@link #author} The actual object that is the target of the 2005 * reference. The reference library doesn't use these, but you can 2006 * use it to hold the resource if you resolve it. (Person who 2007 * received the answers to the questions in the 2008 * QuestionnaireResponse and recorded them in the system.) 2009 */ 2010 public QuestionnaireResponse setAuthorTarget(Resource value) { 2011 this.authorTarget = value; 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #source} (The person who answered the questions about the 2017 * subject.) 2018 */ 2019 public Reference getSource() { 2020 if (this.source == null) 2021 if (Configuration.errorOnAutoCreate()) 2022 throw new Error("Attempt to auto-create QuestionnaireResponse.source"); 2023 else if (Configuration.doAutoCreate()) 2024 this.source = new Reference(); // cc 2025 return this.source; 2026 } 2027 2028 public boolean hasSource() { 2029 return this.source != null && !this.source.isEmpty(); 2030 } 2031 2032 /** 2033 * @param value {@link #source} (The person who answered the questions about the 2034 * subject.) 2035 */ 2036 public QuestionnaireResponse setSource(Reference value) { 2037 this.source = value; 2038 return this; 2039 } 2040 2041 /** 2042 * @return {@link #source} The actual object that is the target of the 2043 * reference. The reference library doesn't populate this, but you can 2044 * use it to hold the resource if you resolve it. (The person who 2045 * answered the questions about the subject.) 2046 */ 2047 public Resource getSourceTarget() { 2048 return this.sourceTarget; 2049 } 2050 2051 /** 2052 * @param value {@link #source} The actual object that is the target of the 2053 * reference. The reference library doesn't use these, but you can 2054 * use it to hold the resource if you resolve it. (The person who 2055 * answered the questions about the subject.) 2056 */ 2057 public QuestionnaireResponse setSourceTarget(Resource value) { 2058 this.sourceTarget = value; 2059 return this; 2060 } 2061 2062 /** 2063 * @return {@link #item} (A group or question item from the original 2064 * questionnaire for which answers are provided.) 2065 */ 2066 public List<QuestionnaireResponseItemComponent> getItem() { 2067 if (this.item == null) 2068 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2069 return this.item; 2070 } 2071 2072 /** 2073 * @return Returns a reference to <code>this</code> for easy method chaining 2074 */ 2075 public QuestionnaireResponse setItem(List<QuestionnaireResponseItemComponent> theItem) { 2076 this.item = theItem; 2077 return this; 2078 } 2079 2080 public boolean hasItem() { 2081 if (this.item == null) 2082 return false; 2083 for (QuestionnaireResponseItemComponent item : this.item) 2084 if (!item.isEmpty()) 2085 return true; 2086 return false; 2087 } 2088 2089 public QuestionnaireResponseItemComponent addItem() { // 3 2090 QuestionnaireResponseItemComponent t = new QuestionnaireResponseItemComponent(); 2091 if (this.item == null) 2092 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2093 this.item.add(t); 2094 return t; 2095 } 2096 2097 public QuestionnaireResponse addItem(QuestionnaireResponseItemComponent t) { // 3 2098 if (t == null) 2099 return this; 2100 if (this.item == null) 2101 this.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2102 this.item.add(t); 2103 return this; 2104 } 2105 2106 /** 2107 * @return The first repetition of repeating field {@link #item}, creating it if 2108 * it does not already exist 2109 */ 2110 public QuestionnaireResponseItemComponent getItemFirstRep() { 2111 if (getItem().isEmpty()) { 2112 addItem(); 2113 } 2114 return getItem().get(0); 2115 } 2116 2117 protected void listChildren(List<Property> children) { 2118 super.listChildren(children); 2119 children.add(new Property("identifier", "Identifier", 2120 "A business identifier assigned to a particular completed (or partially completed) questionnaire.", 0, 1, 2121 identifier)); 2122 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest)", 2123 "The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 2124 0, java.lang.Integer.MAX_VALUE, basedOn)); 2125 children.add(new Property("partOf", "Reference(Observation|Procedure)", 2126 "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 2127 0, java.lang.Integer.MAX_VALUE, partOf)); 2128 children.add(new Property("questionnaire", "canonical(Questionnaire)", 2129 "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, 2130 questionnaire)); 2131 children.add(new Property("status", "code", 2132 "The position of the questionnaire response within its overall lifecycle.", 0, 1, status)); 2133 children.add(new Property("subject", "Reference(Any)", 2134 "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 2135 0, 1, subject)); 2136 children.add(new Property("encounter", "Reference(Encounter)", 2137 "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.", 2138 0, 1, encounter)); 2139 children.add(new Property("authored", "dateTime", 2140 "The date and/or time that this set of answers were last changed.", 0, 1, authored)); 2141 children.add(new Property("author", 2142 "Reference(Device|Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", 2143 "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 2144 0, 1, author)); 2145 children.add(new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 2146 "The person who answered the questions about the subject.", 0, 1, source)); 2147 children.add(new Property("item", "", 2148 "A group or question item from the original questionnaire for which answers are provided.", 0, 2149 java.lang.Integer.MAX_VALUE, item)); 2150 } 2151 2152 @Override 2153 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2154 switch (_hash) { 2155 case -1618432855: 2156 /* identifier */ return new Property("identifier", "Identifier", 2157 "A business identifier assigned to a particular completed (or partially completed) questionnaire.", 0, 1, 2158 identifier); 2159 case -332612366: 2160 /* basedOn */ return new Property("basedOn", "Reference(CarePlan|ServiceRequest)", 2161 "The order, proposal or plan that is fulfilled in whole or in part by this QuestionnaireResponse. For example, a ServiceRequest seeking an intake assessment or a decision support recommendation to assess for post-partum depression.", 2162 0, java.lang.Integer.MAX_VALUE, basedOn); 2163 case -995410646: 2164 /* partOf */ return new Property("partOf", "Reference(Observation|Procedure)", 2165 "A procedure or observation that this questionnaire was performed as part of the execution of. For example, the surgery a checklist was executed as part of.", 2166 0, java.lang.Integer.MAX_VALUE, partOf); 2167 case -1017049693: 2168 /* questionnaire */ return new Property("questionnaire", "canonical(Questionnaire)", 2169 "The Questionnaire that defines and organizes the questions for which answers are being provided.", 0, 1, 2170 questionnaire); 2171 case -892481550: 2172 /* status */ return new Property("status", "code", 2173 "The position of the questionnaire response within its overall lifecycle.", 0, 1, status); 2174 case -1867885268: 2175 /* subject */ return new Property("subject", "Reference(Any)", 2176 "The subject of the questionnaire response. This could be a patient, organization, practitioner, device, etc. This is who/what the answers apply to, but is not necessarily the source of information.", 2177 0, 1, subject); 2178 case 1524132147: 2179 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2180 "The Encounter during which this questionnaire response was created or to which the creation of this record is tightly associated.", 2181 0, 1, encounter); 2182 case 1433073514: 2183 /* authored */ return new Property("authored", "dateTime", 2184 "The date and/or time that this set of answers were last changed.", 0, 1, authored); 2185 case -1406328437: 2186 /* author */ return new Property("author", 2187 "Reference(Device|Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", 2188 "Person who received the answers to the questions in the QuestionnaireResponse and recorded them in the system.", 2189 0, 1, author); 2190 case -896505829: 2191 /* source */ return new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 2192 "The person who answered the questions about the subject.", 0, 1, source); 2193 case 3242771: 2194 /* item */ return new Property("item", "", 2195 "A group or question item from the original questionnaire for which answers are provided.", 0, 2196 java.lang.Integer.MAX_VALUE, item); 2197 default: 2198 return super.getNamedProperty(_hash, _name, _checkValid); 2199 } 2200 2201 } 2202 2203 @Override 2204 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2205 switch (hash) { 2206 case -1618432855: 2207 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 2208 case -332612366: 2209 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2210 case -995410646: 2211 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2212 case -1017049693: 2213 /* questionnaire */ return this.questionnaire == null ? new Base[0] : new Base[] { this.questionnaire }; // CanonicalType 2214 case -892481550: 2215 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<QuestionnaireResponseStatus> 2216 case -1867885268: 2217 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2218 case 1524132147: 2219 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2220 case 1433073514: 2221 /* authored */ return this.authored == null ? new Base[0] : new Base[] { this.authored }; // DateTimeType 2222 case -1406328437: 2223 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 2224 case -896505829: 2225 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 2226 case 3242771: 2227 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // QuestionnaireResponseItemComponent 2228 default: 2229 return super.getProperty(hash, name, checkValid); 2230 } 2231 2232 } 2233 2234 @Override 2235 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2236 switch (hash) { 2237 case -1618432855: // identifier 2238 this.identifier = castToIdentifier(value); // Identifier 2239 return value; 2240 case -332612366: // basedOn 2241 this.getBasedOn().add(castToReference(value)); // Reference 2242 return value; 2243 case -995410646: // partOf 2244 this.getPartOf().add(castToReference(value)); // Reference 2245 return value; 2246 case -1017049693: // questionnaire 2247 this.questionnaire = castToCanonical(value); // CanonicalType 2248 return value; 2249 case -892481550: // status 2250 value = new QuestionnaireResponseStatusEnumFactory().fromType(castToCode(value)); 2251 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 2252 return value; 2253 case -1867885268: // subject 2254 this.subject = castToReference(value); // Reference 2255 return value; 2256 case 1524132147: // encounter 2257 this.encounter = castToReference(value); // Reference 2258 return value; 2259 case 1433073514: // authored 2260 this.authored = castToDateTime(value); // DateTimeType 2261 return value; 2262 case -1406328437: // author 2263 this.author = castToReference(value); // Reference 2264 return value; 2265 case -896505829: // source 2266 this.source = castToReference(value); // Reference 2267 return value; 2268 case 3242771: // item 2269 this.getItem().add((QuestionnaireResponseItemComponent) value); // QuestionnaireResponseItemComponent 2270 return value; 2271 default: 2272 return super.setProperty(hash, name, value); 2273 } 2274 2275 } 2276 2277 @Override 2278 public Base setProperty(String name, Base value) throws FHIRException { 2279 if (name.equals("identifier")) { 2280 this.identifier = castToIdentifier(value); // Identifier 2281 } else if (name.equals("basedOn")) { 2282 this.getBasedOn().add(castToReference(value)); 2283 } else if (name.equals("partOf")) { 2284 this.getPartOf().add(castToReference(value)); 2285 } else if (name.equals("questionnaire")) { 2286 this.questionnaire = castToCanonical(value); // CanonicalType 2287 } else if (name.equals("status")) { 2288 value = new QuestionnaireResponseStatusEnumFactory().fromType(castToCode(value)); 2289 this.status = (Enumeration) value; // Enumeration<QuestionnaireResponseStatus> 2290 } else if (name.equals("subject")) { 2291 this.subject = castToReference(value); // Reference 2292 } else if (name.equals("encounter")) { 2293 this.encounter = castToReference(value); // Reference 2294 } else if (name.equals("authored")) { 2295 this.authored = castToDateTime(value); // DateTimeType 2296 } else if (name.equals("author")) { 2297 this.author = castToReference(value); // Reference 2298 } else if (name.equals("source")) { 2299 this.source = castToReference(value); // Reference 2300 } else if (name.equals("item")) { 2301 this.getItem().add((QuestionnaireResponseItemComponent) value); 2302 } else 2303 return super.setProperty(name, value); 2304 return value; 2305 } 2306 2307 @Override 2308 public void removeChild(String name, Base value) throws FHIRException { 2309 if (name.equals("identifier")) { 2310 this.identifier = null; 2311 } else if (name.equals("basedOn")) { 2312 this.getBasedOn().remove(castToReference(value)); 2313 } else if (name.equals("partOf")) { 2314 this.getPartOf().remove(castToReference(value)); 2315 } else if (name.equals("questionnaire")) { 2316 this.questionnaire = null; 2317 } else if (name.equals("status")) { 2318 this.status = null; 2319 } else if (name.equals("subject")) { 2320 this.subject = null; 2321 } else if (name.equals("encounter")) { 2322 this.encounter = null; 2323 } else if (name.equals("authored")) { 2324 this.authored = null; 2325 } else if (name.equals("author")) { 2326 this.author = null; 2327 } else if (name.equals("source")) { 2328 this.source = null; 2329 } else if (name.equals("item")) { 2330 this.getItem().remove((QuestionnaireResponseItemComponent) value); 2331 } else 2332 super.removeChild(name, value); 2333 2334 } 2335 2336 @Override 2337 public Base makeProperty(int hash, String name) throws FHIRException { 2338 switch (hash) { 2339 case -1618432855: 2340 return getIdentifier(); 2341 case -332612366: 2342 return addBasedOn(); 2343 case -995410646: 2344 return addPartOf(); 2345 case -1017049693: 2346 return getQuestionnaireElement(); 2347 case -892481550: 2348 return getStatusElement(); 2349 case -1867885268: 2350 return getSubject(); 2351 case 1524132147: 2352 return getEncounter(); 2353 case 1433073514: 2354 return getAuthoredElement(); 2355 case -1406328437: 2356 return getAuthor(); 2357 case -896505829: 2358 return getSource(); 2359 case 3242771: 2360 return addItem(); 2361 default: 2362 return super.makeProperty(hash, name); 2363 } 2364 2365 } 2366 2367 @Override 2368 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2369 switch (hash) { 2370 case -1618432855: 2371 /* identifier */ return new String[] { "Identifier" }; 2372 case -332612366: 2373 /* basedOn */ return new String[] { "Reference" }; 2374 case -995410646: 2375 /* partOf */ return new String[] { "Reference" }; 2376 case -1017049693: 2377 /* questionnaire */ return new String[] { "canonical" }; 2378 case -892481550: 2379 /* status */ return new String[] { "code" }; 2380 case -1867885268: 2381 /* subject */ return new String[] { "Reference" }; 2382 case 1524132147: 2383 /* encounter */ return new String[] { "Reference" }; 2384 case 1433073514: 2385 /* authored */ return new String[] { "dateTime" }; 2386 case -1406328437: 2387 /* author */ return new String[] { "Reference" }; 2388 case -896505829: 2389 /* source */ return new String[] { "Reference" }; 2390 case 3242771: 2391 /* item */ return new String[] {}; 2392 default: 2393 return super.getTypesForProperty(hash, name); 2394 } 2395 2396 } 2397 2398 @Override 2399 public Base addChild(String name) throws FHIRException { 2400 if (name.equals("identifier")) { 2401 this.identifier = new Identifier(); 2402 return this.identifier; 2403 } else if (name.equals("basedOn")) { 2404 return addBasedOn(); 2405 } else if (name.equals("partOf")) { 2406 return addPartOf(); 2407 } else if (name.equals("questionnaire")) { 2408 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.questionnaire"); 2409 } else if (name.equals("status")) { 2410 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.status"); 2411 } else if (name.equals("subject")) { 2412 this.subject = new Reference(); 2413 return this.subject; 2414 } else if (name.equals("encounter")) { 2415 this.encounter = new Reference(); 2416 return this.encounter; 2417 } else if (name.equals("authored")) { 2418 throw new FHIRException("Cannot call addChild on a singleton property QuestionnaireResponse.authored"); 2419 } else if (name.equals("author")) { 2420 this.author = new Reference(); 2421 return this.author; 2422 } else if (name.equals("source")) { 2423 this.source = new Reference(); 2424 return this.source; 2425 } else if (name.equals("item")) { 2426 return addItem(); 2427 } else 2428 return super.addChild(name); 2429 } 2430 2431 public String fhirType() { 2432 return "QuestionnaireResponse"; 2433 2434 } 2435 2436 public QuestionnaireResponse copy() { 2437 QuestionnaireResponse dst = new QuestionnaireResponse(); 2438 copyValues(dst); 2439 return dst; 2440 } 2441 2442 public void copyValues(QuestionnaireResponse dst) { 2443 super.copyValues(dst); 2444 dst.identifier = identifier == null ? null : identifier.copy(); 2445 if (basedOn != null) { 2446 dst.basedOn = new ArrayList<Reference>(); 2447 for (Reference i : basedOn) 2448 dst.basedOn.add(i.copy()); 2449 } 2450 ; 2451 if (partOf != null) { 2452 dst.partOf = new ArrayList<Reference>(); 2453 for (Reference i : partOf) 2454 dst.partOf.add(i.copy()); 2455 } 2456 ; 2457 dst.questionnaire = questionnaire == null ? null : questionnaire.copy(); 2458 dst.status = status == null ? null : status.copy(); 2459 dst.subject = subject == null ? null : subject.copy(); 2460 dst.encounter = encounter == null ? null : encounter.copy(); 2461 dst.authored = authored == null ? null : authored.copy(); 2462 dst.author = author == null ? null : author.copy(); 2463 dst.source = source == null ? null : source.copy(); 2464 if (item != null) { 2465 dst.item = new ArrayList<QuestionnaireResponseItemComponent>(); 2466 for (QuestionnaireResponseItemComponent i : item) 2467 dst.item.add(i.copy()); 2468 } 2469 ; 2470 } 2471 2472 protected QuestionnaireResponse typedCopy() { 2473 return copy(); 2474 } 2475 2476 @Override 2477 public boolean equalsDeep(Base other_) { 2478 if (!super.equalsDeep(other_)) 2479 return false; 2480 if (!(other_ instanceof QuestionnaireResponse)) 2481 return false; 2482 QuestionnaireResponse o = (QuestionnaireResponse) other_; 2483 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2484 && compareDeep(partOf, o.partOf, true) && compareDeep(questionnaire, o.questionnaire, true) 2485 && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 2486 && compareDeep(encounter, o.encounter, true) && compareDeep(authored, o.authored, true) 2487 && compareDeep(author, o.author, true) && compareDeep(source, o.source, true) 2488 && compareDeep(item, o.item, true); 2489 } 2490 2491 @Override 2492 public boolean equalsShallow(Base other_) { 2493 if (!super.equalsShallow(other_)) 2494 return false; 2495 if (!(other_ instanceof QuestionnaireResponse)) 2496 return false; 2497 QuestionnaireResponse o = (QuestionnaireResponse) other_; 2498 return compareValues(status, o.status, true) && compareValues(authored, o.authored, true); 2499 } 2500 2501 public boolean isEmpty() { 2502 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, questionnaire, status, 2503 subject, encounter, authored, author, source, item); 2504 } 2505 2506 @Override 2507 public ResourceType getResourceType() { 2508 return ResourceType.QuestionnaireResponse; 2509 } 2510 2511 /** 2512 * Search parameter: <b>authored</b> 2513 * <p> 2514 * Description: <b>When the questionnaire response was last changed</b><br> 2515 * Type: <b>date</b><br> 2516 * Path: <b>QuestionnaireResponse.authored</b><br> 2517 * </p> 2518 */ 2519 @SearchParamDefinition(name = "authored", path = "QuestionnaireResponse.authored", description = "When the questionnaire response was last changed", type = "date") 2520 public static final String SP_AUTHORED = "authored"; 2521 /** 2522 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2523 * <p> 2524 * Description: <b>When the questionnaire response was last changed</b><br> 2525 * Type: <b>date</b><br> 2526 * Path: <b>QuestionnaireResponse.authored</b><br> 2527 * </p> 2528 */ 2529 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2530 SP_AUTHORED); 2531 2532 /** 2533 * Search parameter: <b>identifier</b> 2534 * <p> 2535 * Description: <b>The unique identifier for the questionnaire response</b><br> 2536 * Type: <b>token</b><br> 2537 * Path: <b>QuestionnaireResponse.identifier</b><br> 2538 * </p> 2539 */ 2540 @SearchParamDefinition(name = "identifier", path = "QuestionnaireResponse.identifier", description = "The unique identifier for the questionnaire response", type = "token") 2541 public static final String SP_IDENTIFIER = "identifier"; 2542 /** 2543 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2544 * <p> 2545 * Description: <b>The unique identifier for the questionnaire response</b><br> 2546 * Type: <b>token</b><br> 2547 * Path: <b>QuestionnaireResponse.identifier</b><br> 2548 * </p> 2549 */ 2550 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2551 SP_IDENTIFIER); 2552 2553 /** 2554 * Search parameter: <b>questionnaire</b> 2555 * <p> 2556 * Description: <b>The questionnaire the answers are provided for</b><br> 2557 * Type: <b>reference</b><br> 2558 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2559 * </p> 2560 */ 2561 @SearchParamDefinition(name = "questionnaire", path = "QuestionnaireResponse.questionnaire", description = "The questionnaire the answers are provided for", type = "reference", target = { 2562 Questionnaire.class }) 2563 public static final String SP_QUESTIONNAIRE = "questionnaire"; 2564 /** 2565 * <b>Fluent Client</b> search parameter constant for <b>questionnaire</b> 2566 * <p> 2567 * Description: <b>The questionnaire the answers are provided for</b><br> 2568 * Type: <b>reference</b><br> 2569 * Path: <b>QuestionnaireResponse.questionnaire</b><br> 2570 * </p> 2571 */ 2572 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam QUESTIONNAIRE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2573 SP_QUESTIONNAIRE); 2574 2575 /** 2576 * Constant for fluent queries to be used to add include statements. Specifies 2577 * the path value of "<b>QuestionnaireResponse:questionnaire</b>". 2578 */ 2579 public static final ca.uhn.fhir.model.api.Include INCLUDE_QUESTIONNAIRE = new ca.uhn.fhir.model.api.Include( 2580 "QuestionnaireResponse:questionnaire").toLocked(); 2581 2582 /** 2583 * Search parameter: <b>based-on</b> 2584 * <p> 2585 * Description: <b>Plan/proposal/order fulfilled by this questionnaire 2586 * response</b><br> 2587 * Type: <b>reference</b><br> 2588 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2589 * </p> 2590 */ 2591 @SearchParamDefinition(name = "based-on", path = "QuestionnaireResponse.basedOn", description = "Plan/proposal/order fulfilled by this questionnaire response", type = "reference", target = { 2592 CarePlan.class, ServiceRequest.class }) 2593 public static final String SP_BASED_ON = "based-on"; 2594 /** 2595 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2596 * <p> 2597 * Description: <b>Plan/proposal/order fulfilled by this questionnaire 2598 * response</b><br> 2599 * Type: <b>reference</b><br> 2600 * Path: <b>QuestionnaireResponse.basedOn</b><br> 2601 * </p> 2602 */ 2603 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2604 SP_BASED_ON); 2605 2606 /** 2607 * Constant for fluent queries to be used to add include statements. Specifies 2608 * the path value of "<b>QuestionnaireResponse:based-on</b>". 2609 */ 2610 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 2611 "QuestionnaireResponse:based-on").toLocked(); 2612 2613 /** 2614 * Search parameter: <b>subject</b> 2615 * <p> 2616 * Description: <b>The subject of the questionnaire response</b><br> 2617 * Type: <b>reference</b><br> 2618 * Path: <b>QuestionnaireResponse.subject</b><br> 2619 * </p> 2620 */ 2621 @SearchParamDefinition(name = "subject", path = "QuestionnaireResponse.subject", description = "The subject of the questionnaire response", type = "reference", providesMembershipIn = { 2622 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }) 2623 public static final String SP_SUBJECT = "subject"; 2624 /** 2625 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2626 * <p> 2627 * Description: <b>The subject of the questionnaire response</b><br> 2628 * Type: <b>reference</b><br> 2629 * Path: <b>QuestionnaireResponse.subject</b><br> 2630 * </p> 2631 */ 2632 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2633 SP_SUBJECT); 2634 2635 /** 2636 * Constant for fluent queries to be used to add include statements. Specifies 2637 * the path value of "<b>QuestionnaireResponse:subject</b>". 2638 */ 2639 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2640 "QuestionnaireResponse:subject").toLocked(); 2641 2642 /** 2643 * Search parameter: <b>author</b> 2644 * <p> 2645 * Description: <b>The author of the questionnaire response</b><br> 2646 * Type: <b>reference</b><br> 2647 * Path: <b>QuestionnaireResponse.author</b><br> 2648 * </p> 2649 */ 2650 @SearchParamDefinition(name = "author", path = "QuestionnaireResponse.author", description = "The author of the questionnaire response", type = "reference", providesMembershipIn = { 2651 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2652 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2653 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2654 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 2655 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2656 public static final String SP_AUTHOR = "author"; 2657 /** 2658 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2659 * <p> 2660 * Description: <b>The author of the questionnaire response</b><br> 2661 * Type: <b>reference</b><br> 2662 * Path: <b>QuestionnaireResponse.author</b><br> 2663 * </p> 2664 */ 2665 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2666 SP_AUTHOR); 2667 2668 /** 2669 * Constant for fluent queries to be used to add include statements. Specifies 2670 * the path value of "<b>QuestionnaireResponse:author</b>". 2671 */ 2672 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 2673 "QuestionnaireResponse:author").toLocked(); 2674 2675 /** 2676 * Search parameter: <b>patient</b> 2677 * <p> 2678 * Description: <b>The patient that is the subject of the questionnaire 2679 * response</b><br> 2680 * Type: <b>reference</b><br> 2681 * Path: <b>QuestionnaireResponse.subject</b><br> 2682 * </p> 2683 */ 2684 @SearchParamDefinition(name = "patient", path = "QuestionnaireResponse.subject.where(resolve() is Patient)", description = "The patient that is the subject of the questionnaire response", type = "reference", target = { 2685 Patient.class }) 2686 public static final String SP_PATIENT = "patient"; 2687 /** 2688 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2689 * <p> 2690 * Description: <b>The patient that is the subject of the questionnaire 2691 * response</b><br> 2692 * Type: <b>reference</b><br> 2693 * Path: <b>QuestionnaireResponse.subject</b><br> 2694 * </p> 2695 */ 2696 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2697 SP_PATIENT); 2698 2699 /** 2700 * Constant for fluent queries to be used to add include statements. Specifies 2701 * the path value of "<b>QuestionnaireResponse:patient</b>". 2702 */ 2703 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2704 "QuestionnaireResponse:patient").toLocked(); 2705 2706 /** 2707 * Search parameter: <b>part-of</b> 2708 * <p> 2709 * Description: <b>Procedure or observation this questionnaire response was 2710 * performed as a part of</b><br> 2711 * Type: <b>reference</b><br> 2712 * Path: <b>QuestionnaireResponse.partOf</b><br> 2713 * </p> 2714 */ 2715 @SearchParamDefinition(name = "part-of", path = "QuestionnaireResponse.partOf", description = "Procedure or observation this questionnaire response was performed as a part of", type = "reference", target = { 2716 Observation.class, Procedure.class }) 2717 public static final String SP_PART_OF = "part-of"; 2718 /** 2719 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2720 * <p> 2721 * Description: <b>Procedure or observation this questionnaire response was 2722 * performed as a part of</b><br> 2723 * Type: <b>reference</b><br> 2724 * Path: <b>QuestionnaireResponse.partOf</b><br> 2725 * </p> 2726 */ 2727 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2728 SP_PART_OF); 2729 2730 /** 2731 * Constant for fluent queries to be used to add include statements. Specifies 2732 * the path value of "<b>QuestionnaireResponse:part-of</b>". 2733 */ 2734 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 2735 "QuestionnaireResponse:part-of").toLocked(); 2736 2737 /** 2738 * Search parameter: <b>encounter</b> 2739 * <p> 2740 * Description: <b>Encounter associated with the questionnaire response</b><br> 2741 * Type: <b>reference</b><br> 2742 * Path: <b>QuestionnaireResponse.encounter</b><br> 2743 * </p> 2744 */ 2745 @SearchParamDefinition(name = "encounter", path = "QuestionnaireResponse.encounter", description = "Encounter associated with the questionnaire response", type = "reference", providesMembershipIn = { 2746 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2747 public static final String SP_ENCOUNTER = "encounter"; 2748 /** 2749 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2750 * <p> 2751 * Description: <b>Encounter associated with the questionnaire response</b><br> 2752 * Type: <b>reference</b><br> 2753 * Path: <b>QuestionnaireResponse.encounter</b><br> 2754 * </p> 2755 */ 2756 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2757 SP_ENCOUNTER); 2758 2759 /** 2760 * Constant for fluent queries to be used to add include statements. Specifies 2761 * the path value of "<b>QuestionnaireResponse:encounter</b>". 2762 */ 2763 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2764 "QuestionnaireResponse:encounter").toLocked(); 2765 2766 /** 2767 * Search parameter: <b>source</b> 2768 * <p> 2769 * Description: <b>The individual providing the information reflected in the 2770 * questionnaire respose</b><br> 2771 * Type: <b>reference</b><br> 2772 * Path: <b>QuestionnaireResponse.source</b><br> 2773 * </p> 2774 */ 2775 @SearchParamDefinition(name = "source", path = "QuestionnaireResponse.source", description = "The individual providing the information reflected in the questionnaire respose", type = "reference", providesMembershipIn = { 2776 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2777 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 2778 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2779 public static final String SP_SOURCE = "source"; 2780 /** 2781 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2782 * <p> 2783 * Description: <b>The individual providing the information reflected in the 2784 * questionnaire respose</b><br> 2785 * Type: <b>reference</b><br> 2786 * Path: <b>QuestionnaireResponse.source</b><br> 2787 * </p> 2788 */ 2789 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2790 SP_SOURCE); 2791 2792 /** 2793 * Constant for fluent queries to be used to add include statements. Specifies 2794 * the path value of "<b>QuestionnaireResponse:source</b>". 2795 */ 2796 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 2797 "QuestionnaireResponse:source").toLocked(); 2798 2799 /** 2800 * Search parameter: <b>status</b> 2801 * <p> 2802 * Description: <b>The status of the questionnaire response</b><br> 2803 * Type: <b>token</b><br> 2804 * Path: <b>QuestionnaireResponse.status</b><br> 2805 * </p> 2806 */ 2807 @SearchParamDefinition(name = "status", path = "QuestionnaireResponse.status", description = "The status of the questionnaire response", type = "token") 2808 public static final String SP_STATUS = "status"; 2809 /** 2810 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2811 * <p> 2812 * Description: <b>The status of the questionnaire response</b><br> 2813 * Type: <b>token</b><br> 2814 * Path: <b>QuestionnaireResponse.status</b><br> 2815 * </p> 2816 */ 2817 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2818 SP_STATUS); 2819 2820}