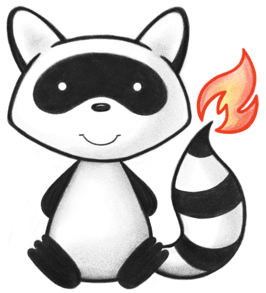
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * Related artifacts such as additional documentation, justification, or 045 * bibliographic references. 046 */ 047@DatatypeDef(name = "RelatedArtifact") 048public class RelatedArtifact extends Type implements ICompositeType { 049 050 public enum RelatedArtifactType { 051 /** 052 * Additional documentation for the knowledge resource. This would include 053 * additional instructions on usage as well as additional information on 054 * clinical context or appropriateness. 055 */ 056 DOCUMENTATION, 057 /** 058 * A summary of the justification for the knowledge resource including 059 * supporting evidence, relevant guidelines, or other clinically important 060 * information. This information is intended to provide a way to make the 061 * justification for the knowledge resource available to the consumer of 062 * interventions or results produced by the knowledge resource. 063 */ 064 JUSTIFICATION, 065 /** 066 * Bibliographic citation for papers, references, or other relevant material for 067 * the knowledge resource. This is intended to allow for citation of related 068 * material, but that was not necessarily specifically prepared in connection 069 * with this knowledge resource. 070 */ 071 CITATION, 072 /** 073 * The previous version of the knowledge resource. 074 */ 075 PREDECESSOR, 076 /** 077 * The next version of the knowledge resource. 078 */ 079 SUCCESSOR, 080 /** 081 * The knowledge resource is derived from the related artifact. This is intended 082 * to capture the relationship in which a particular knowledge resource is based 083 * on the content of another artifact, but is modified to capture either a 084 * different set of overall requirements, or a more specific set of requirements 085 * such as those involved in a particular institution or clinical setting. 086 */ 087 DERIVEDFROM, 088 /** 089 * The knowledge resource depends on the given related artifact. 090 */ 091 DEPENDSON, 092 /** 093 * The knowledge resource is composed of the given related artifact. 094 */ 095 COMPOSEDOF, 096 /** 097 * added to help the parsers with the generic types 098 */ 099 NULL; 100 101 public static RelatedArtifactType fromCode(String codeString) throws FHIRException { 102 if (codeString == null || "".equals(codeString)) 103 return null; 104 if ("documentation".equals(codeString)) 105 return DOCUMENTATION; 106 if ("justification".equals(codeString)) 107 return JUSTIFICATION; 108 if ("citation".equals(codeString)) 109 return CITATION; 110 if ("predecessor".equals(codeString)) 111 return PREDECESSOR; 112 if ("successor".equals(codeString)) 113 return SUCCESSOR; 114 if ("derived-from".equals(codeString)) 115 return DERIVEDFROM; 116 if ("depends-on".equals(codeString)) 117 return DEPENDSON; 118 if ("composed-of".equals(codeString)) 119 return COMPOSEDOF; 120 if (Configuration.isAcceptInvalidEnums()) 121 return null; 122 else 123 throw new FHIRException("Unknown RelatedArtifactType code '" + codeString + "'"); 124 } 125 126 public String toCode() { 127 switch (this) { 128 case DOCUMENTATION: 129 return "documentation"; 130 case JUSTIFICATION: 131 return "justification"; 132 case CITATION: 133 return "citation"; 134 case PREDECESSOR: 135 return "predecessor"; 136 case SUCCESSOR: 137 return "successor"; 138 case DERIVEDFROM: 139 return "derived-from"; 140 case DEPENDSON: 141 return "depends-on"; 142 case COMPOSEDOF: 143 return "composed-of"; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getSystem() { 152 switch (this) { 153 case DOCUMENTATION: 154 return "http://hl7.org/fhir/related-artifact-type"; 155 case JUSTIFICATION: 156 return "http://hl7.org/fhir/related-artifact-type"; 157 case CITATION: 158 return "http://hl7.org/fhir/related-artifact-type"; 159 case PREDECESSOR: 160 return "http://hl7.org/fhir/related-artifact-type"; 161 case SUCCESSOR: 162 return "http://hl7.org/fhir/related-artifact-type"; 163 case DERIVEDFROM: 164 return "http://hl7.org/fhir/related-artifact-type"; 165 case DEPENDSON: 166 return "http://hl7.org/fhir/related-artifact-type"; 167 case COMPOSEDOF: 168 return "http://hl7.org/fhir/related-artifact-type"; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 176 public String getDefinition() { 177 switch (this) { 178 case DOCUMENTATION: 179 return "Additional documentation for the knowledge resource. This would include additional instructions on usage as well as additional information on clinical context or appropriateness."; 180 case JUSTIFICATION: 181 return "A summary of the justification for the knowledge resource including supporting evidence, relevant guidelines, or other clinically important information. This information is intended to provide a way to make the justification for the knowledge resource available to the consumer of interventions or results produced by the knowledge resource."; 182 case CITATION: 183 return "Bibliographic citation for papers, references, or other relevant material for the knowledge resource. This is intended to allow for citation of related material, but that was not necessarily specifically prepared in connection with this knowledge resource."; 184 case PREDECESSOR: 185 return "The previous version of the knowledge resource."; 186 case SUCCESSOR: 187 return "The next version of the knowledge resource."; 188 case DERIVEDFROM: 189 return "The knowledge resource is derived from the related artifact. This is intended to capture the relationship in which a particular knowledge resource is based on the content of another artifact, but is modified to capture either a different set of overall requirements, or a more specific set of requirements such as those involved in a particular institution or clinical setting."; 190 case DEPENDSON: 191 return "The knowledge resource depends on the given related artifact."; 192 case COMPOSEDOF: 193 return "The knowledge resource is composed of the given related artifact."; 194 case NULL: 195 return null; 196 default: 197 return "?"; 198 } 199 } 200 201 public String getDisplay() { 202 switch (this) { 203 case DOCUMENTATION: 204 return "Documentation"; 205 case JUSTIFICATION: 206 return "Justification"; 207 case CITATION: 208 return "Citation"; 209 case PREDECESSOR: 210 return "Predecessor"; 211 case SUCCESSOR: 212 return "Successor"; 213 case DERIVEDFROM: 214 return "Derived From"; 215 case DEPENDSON: 216 return "Depends On"; 217 case COMPOSEDOF: 218 return "Composed Of"; 219 case NULL: 220 return null; 221 default: 222 return "?"; 223 } 224 } 225 } 226 227 public static class RelatedArtifactTypeEnumFactory implements EnumFactory<RelatedArtifactType> { 228 public RelatedArtifactType fromCode(String codeString) throws IllegalArgumentException { 229 if (codeString == null || "".equals(codeString)) 230 if (codeString == null || "".equals(codeString)) 231 return null; 232 if ("documentation".equals(codeString)) 233 return RelatedArtifactType.DOCUMENTATION; 234 if ("justification".equals(codeString)) 235 return RelatedArtifactType.JUSTIFICATION; 236 if ("citation".equals(codeString)) 237 return RelatedArtifactType.CITATION; 238 if ("predecessor".equals(codeString)) 239 return RelatedArtifactType.PREDECESSOR; 240 if ("successor".equals(codeString)) 241 return RelatedArtifactType.SUCCESSOR; 242 if ("derived-from".equals(codeString)) 243 return RelatedArtifactType.DERIVEDFROM; 244 if ("depends-on".equals(codeString)) 245 return RelatedArtifactType.DEPENDSON; 246 if ("composed-of".equals(codeString)) 247 return RelatedArtifactType.COMPOSEDOF; 248 throw new IllegalArgumentException("Unknown RelatedArtifactType code '" + codeString + "'"); 249 } 250 251 public Enumeration<RelatedArtifactType> fromType(PrimitiveType<?> code) throws FHIRException { 252 if (code == null) 253 return null; 254 if (code.isEmpty()) 255 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.NULL, code); 256 String codeString = code.asStringValue(); 257 if (codeString == null || "".equals(codeString)) 258 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.NULL, code); 259 if ("documentation".equals(codeString)) 260 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DOCUMENTATION, code); 261 if ("justification".equals(codeString)) 262 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.JUSTIFICATION, code); 263 if ("citation".equals(codeString)) 264 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.CITATION, code); 265 if ("predecessor".equals(codeString)) 266 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.PREDECESSOR, code); 267 if ("successor".equals(codeString)) 268 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.SUCCESSOR, code); 269 if ("derived-from".equals(codeString)) 270 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DERIVEDFROM, code); 271 if ("depends-on".equals(codeString)) 272 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.DEPENDSON, code); 273 if ("composed-of".equals(codeString)) 274 return new Enumeration<RelatedArtifactType>(this, RelatedArtifactType.COMPOSEDOF, code); 275 throw new FHIRException("Unknown RelatedArtifactType code '" + codeString + "'"); 276 } 277 278 public String toCode(RelatedArtifactType code) { 279 if (code == RelatedArtifactType.DOCUMENTATION) 280 return "documentation"; 281 if (code == RelatedArtifactType.JUSTIFICATION) 282 return "justification"; 283 if (code == RelatedArtifactType.CITATION) 284 return "citation"; 285 if (code == RelatedArtifactType.PREDECESSOR) 286 return "predecessor"; 287 if (code == RelatedArtifactType.SUCCESSOR) 288 return "successor"; 289 if (code == RelatedArtifactType.DERIVEDFROM) 290 return "derived-from"; 291 if (code == RelatedArtifactType.DEPENDSON) 292 return "depends-on"; 293 if (code == RelatedArtifactType.COMPOSEDOF) 294 return "composed-of"; 295 return "?"; 296 } 297 298 public String toSystem(RelatedArtifactType code) { 299 return code.getSystem(); 300 } 301 } 302 303 /** 304 * The type of relationship to the related artifact. 305 */ 306 @Child(name = "type", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 307 @Description(shortDefinition = "documentation | justification | citation | predecessor | successor | derived-from | depends-on | composed-of", formalDefinition = "The type of relationship to the related artifact.") 308 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/related-artifact-type") 309 protected Enumeration<RelatedArtifactType> type; 310 311 /** 312 * A short label that can be used to reference the citation from elsewhere in 313 * the containing artifact, such as a footnote index. 314 */ 315 @Child(name = "label", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 316 @Description(shortDefinition = "Short label", formalDefinition = "A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.") 317 protected StringType label; 318 319 /** 320 * A brief description of the document or knowledge resource being referenced, 321 * suitable for display to a consumer. 322 */ 323 @Child(name = "display", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 324 @Description(shortDefinition = "Brief description of the related artifact", formalDefinition = "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.") 325 protected StringType display; 326 327 /** 328 * A bibliographic citation for the related artifact. This text SHOULD be 329 * formatted according to an accepted citation format. 330 */ 331 @Child(name = "citation", type = { 332 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 333 @Description(shortDefinition = "Bibliographic citation for the artifact", formalDefinition = "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.") 334 protected MarkdownType citation; 335 336 /** 337 * A url for the artifact that can be followed to access the actual content. 338 */ 339 @Child(name = "url", type = { UrlType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 340 @Description(shortDefinition = "Where the artifact can be accessed", formalDefinition = "A url for the artifact that can be followed to access the actual content.") 341 protected UrlType url; 342 343 /** 344 * The document being referenced, represented as an attachment. This is 345 * exclusive with the resource element. 346 */ 347 @Child(name = "document", type = { Attachment.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 348 @Description(shortDefinition = "What document is being referenced", formalDefinition = "The document being referenced, represented as an attachment. This is exclusive with the resource element.") 349 protected Attachment document; 350 351 /** 352 * The related resource, such as a library, value set, profile, or other 353 * knowledge resource. 354 */ 355 @Child(name = "resource", type = { 356 CanonicalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 357 @Description(shortDefinition = "What resource is being referenced", formalDefinition = "The related resource, such as a library, value set, profile, or other knowledge resource.") 358 protected CanonicalType resource; 359 360 private static final long serialVersionUID = -695743528L; 361 362 /** 363 * Constructor 364 */ 365 public RelatedArtifact() { 366 super(); 367 } 368 369 /** 370 * Constructor 371 */ 372 public RelatedArtifact(Enumeration<RelatedArtifactType> type) { 373 super(); 374 this.type = type; 375 } 376 377 /** 378 * @return {@link #type} (The type of relationship to the related artifact.). 379 * This is the underlying object with id, value and extensions. The 380 * accessor "getType" gives direct access to the value 381 */ 382 public Enumeration<RelatedArtifactType> getTypeElement() { 383 if (this.type == null) 384 if (Configuration.errorOnAutoCreate()) 385 throw new Error("Attempt to auto-create RelatedArtifact.type"); 386 else if (Configuration.doAutoCreate()) 387 this.type = new Enumeration<RelatedArtifactType>(new RelatedArtifactTypeEnumFactory()); // bb 388 return this.type; 389 } 390 391 public boolean hasTypeElement() { 392 return this.type != null && !this.type.isEmpty(); 393 } 394 395 public boolean hasType() { 396 return this.type != null && !this.type.isEmpty(); 397 } 398 399 /** 400 * @param value {@link #type} (The type of relationship to the related 401 * artifact.). This is the underlying object with id, value and 402 * extensions. The accessor "getType" gives direct access to the 403 * value 404 */ 405 public RelatedArtifact setTypeElement(Enumeration<RelatedArtifactType> value) { 406 this.type = value; 407 return this; 408 } 409 410 /** 411 * @return The type of relationship to the related artifact. 412 */ 413 public RelatedArtifactType getType() { 414 return this.type == null ? null : this.type.getValue(); 415 } 416 417 /** 418 * @param value The type of relationship to the related artifact. 419 */ 420 public RelatedArtifact setType(RelatedArtifactType value) { 421 if (this.type == null) 422 this.type = new Enumeration<RelatedArtifactType>(new RelatedArtifactTypeEnumFactory()); 423 this.type.setValue(value); 424 return this; 425 } 426 427 /** 428 * @return {@link #label} (A short label that can be used to reference the 429 * citation from elsewhere in the containing artifact, such as a 430 * footnote index.). This is the underlying object with id, value and 431 * extensions. The accessor "getLabel" gives direct access to the value 432 */ 433 public StringType getLabelElement() { 434 if (this.label == null) 435 if (Configuration.errorOnAutoCreate()) 436 throw new Error("Attempt to auto-create RelatedArtifact.label"); 437 else if (Configuration.doAutoCreate()) 438 this.label = new StringType(); // bb 439 return this.label; 440 } 441 442 public boolean hasLabelElement() { 443 return this.label != null && !this.label.isEmpty(); 444 } 445 446 public boolean hasLabel() { 447 return this.label != null && !this.label.isEmpty(); 448 } 449 450 /** 451 * @param value {@link #label} (A short label that can be used to reference the 452 * citation from elsewhere in the containing artifact, such as a 453 * footnote index.). This is the underlying object with id, value 454 * and extensions. The accessor "getLabel" gives direct access to 455 * the value 456 */ 457 public RelatedArtifact setLabelElement(StringType value) { 458 this.label = value; 459 return this; 460 } 461 462 /** 463 * @return A short label that can be used to reference the citation from 464 * elsewhere in the containing artifact, such as a footnote index. 465 */ 466 public String getLabel() { 467 return this.label == null ? null : this.label.getValue(); 468 } 469 470 /** 471 * @param value A short label that can be used to reference the citation from 472 * elsewhere in the containing artifact, such as a footnote index. 473 */ 474 public RelatedArtifact setLabel(String value) { 475 if (Utilities.noString(value)) 476 this.label = null; 477 else { 478 if (this.label == null) 479 this.label = new StringType(); 480 this.label.setValue(value); 481 } 482 return this; 483 } 484 485 /** 486 * @return {@link #display} (A brief description of the document or knowledge 487 * resource being referenced, suitable for display to a consumer.). This 488 * is the underlying object with id, value and extensions. The accessor 489 * "getDisplay" gives direct access to the value 490 */ 491 public StringType getDisplayElement() { 492 if (this.display == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error("Attempt to auto-create RelatedArtifact.display"); 495 else if (Configuration.doAutoCreate()) 496 this.display = new StringType(); // bb 497 return this.display; 498 } 499 500 public boolean hasDisplayElement() { 501 return this.display != null && !this.display.isEmpty(); 502 } 503 504 public boolean hasDisplay() { 505 return this.display != null && !this.display.isEmpty(); 506 } 507 508 /** 509 * @param value {@link #display} (A brief description of the document or 510 * knowledge resource being referenced, suitable for display to a 511 * consumer.). This is the underlying object with id, value and 512 * extensions. The accessor "getDisplay" gives direct access to the 513 * value 514 */ 515 public RelatedArtifact setDisplayElement(StringType value) { 516 this.display = value; 517 return this; 518 } 519 520 /** 521 * @return A brief description of the document or knowledge resource being 522 * referenced, suitable for display to a consumer. 523 */ 524 public String getDisplay() { 525 return this.display == null ? null : this.display.getValue(); 526 } 527 528 /** 529 * @param value A brief description of the document or knowledge resource being 530 * referenced, suitable for display to a consumer. 531 */ 532 public RelatedArtifact setDisplay(String value) { 533 if (Utilities.noString(value)) 534 this.display = null; 535 else { 536 if (this.display == null) 537 this.display = new StringType(); 538 this.display.setValue(value); 539 } 540 return this; 541 } 542 543 /** 544 * @return {@link #citation} (A bibliographic citation for the related artifact. 545 * This text SHOULD be formatted according to an accepted citation 546 * format.). This is the underlying object with id, value and 547 * extensions. The accessor "getCitation" gives direct access to the 548 * value 549 */ 550 public MarkdownType getCitationElement() { 551 if (this.citation == null) 552 if (Configuration.errorOnAutoCreate()) 553 throw new Error("Attempt to auto-create RelatedArtifact.citation"); 554 else if (Configuration.doAutoCreate()) 555 this.citation = new MarkdownType(); // bb 556 return this.citation; 557 } 558 559 public boolean hasCitationElement() { 560 return this.citation != null && !this.citation.isEmpty(); 561 } 562 563 public boolean hasCitation() { 564 return this.citation != null && !this.citation.isEmpty(); 565 } 566 567 /** 568 * @param value {@link #citation} (A bibliographic citation for the related 569 * artifact. This text SHOULD be formatted according to an accepted 570 * citation format.). This is the underlying object with id, value 571 * and extensions. The accessor "getCitation" gives direct access 572 * to the value 573 */ 574 public RelatedArtifact setCitationElement(MarkdownType value) { 575 this.citation = value; 576 return this; 577 } 578 579 /** 580 * @return A bibliographic citation for the related artifact. This text SHOULD 581 * be formatted according to an accepted citation format. 582 */ 583 public String getCitation() { 584 return this.citation == null ? null : this.citation.getValue(); 585 } 586 587 /** 588 * @param value A bibliographic citation for the related artifact. This text 589 * SHOULD be formatted according to an accepted citation format. 590 */ 591 public RelatedArtifact setCitation(String value) { 592 if (value == null) 593 this.citation = null; 594 else { 595 if (this.citation == null) 596 this.citation = new MarkdownType(); 597 this.citation.setValue(value); 598 } 599 return this; 600 } 601 602 /** 603 * @return {@link #url} (A url for the artifact that can be followed to access 604 * the actual content.). This is the underlying object with id, value 605 * and extensions. The accessor "getUrl" gives direct access to the 606 * value 607 */ 608 public UrlType getUrlElement() { 609 if (this.url == null) 610 if (Configuration.errorOnAutoCreate()) 611 throw new Error("Attempt to auto-create RelatedArtifact.url"); 612 else if (Configuration.doAutoCreate()) 613 this.url = new UrlType(); // bb 614 return this.url; 615 } 616 617 public boolean hasUrlElement() { 618 return this.url != null && !this.url.isEmpty(); 619 } 620 621 public boolean hasUrl() { 622 return this.url != null && !this.url.isEmpty(); 623 } 624 625 /** 626 * @param value {@link #url} (A url for the artifact that can be followed to 627 * access the actual content.). This is the underlying object with 628 * id, value and extensions. The accessor "getUrl" gives direct 629 * access to the value 630 */ 631 public RelatedArtifact setUrlElement(UrlType value) { 632 this.url = value; 633 return this; 634 } 635 636 /** 637 * @return A url for the artifact that can be followed to access the actual 638 * content. 639 */ 640 public String getUrl() { 641 return this.url == null ? null : this.url.getValue(); 642 } 643 644 /** 645 * @param value A url for the artifact that can be followed to access the actual 646 * content. 647 */ 648 public RelatedArtifact setUrl(String value) { 649 if (Utilities.noString(value)) 650 this.url = null; 651 else { 652 if (this.url == null) 653 this.url = new UrlType(); 654 this.url.setValue(value); 655 } 656 return this; 657 } 658 659 /** 660 * @return {@link #document} (The document being referenced, represented as an 661 * attachment. This is exclusive with the resource element.) 662 */ 663 public Attachment getDocument() { 664 if (this.document == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create RelatedArtifact.document"); 667 else if (Configuration.doAutoCreate()) 668 this.document = new Attachment(); // cc 669 return this.document; 670 } 671 672 public boolean hasDocument() { 673 return this.document != null && !this.document.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #document} (The document being referenced, represented as 678 * an attachment. This is exclusive with the resource element.) 679 */ 680 public RelatedArtifact setDocument(Attachment value) { 681 this.document = value; 682 return this; 683 } 684 685 /** 686 * @return {@link #resource} (The related resource, such as a library, value 687 * set, profile, or other knowledge resource.). This is the underlying 688 * object with id, value and extensions. The accessor "getResource" 689 * gives direct access to the value 690 */ 691 public CanonicalType getResourceElement() { 692 if (this.resource == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create RelatedArtifact.resource"); 695 else if (Configuration.doAutoCreate()) 696 this.resource = new CanonicalType(); // bb 697 return this.resource; 698 } 699 700 public boolean hasResourceElement() { 701 return this.resource != null && !this.resource.isEmpty(); 702 } 703 704 public boolean hasResource() { 705 return this.resource != null && !this.resource.isEmpty(); 706 } 707 708 /** 709 * @param value {@link #resource} (The related resource, such as a library, 710 * value set, profile, or other knowledge resource.). This is the 711 * underlying object with id, value and extensions. The accessor 712 * "getResource" gives direct access to the value 713 */ 714 public RelatedArtifact setResourceElement(CanonicalType value) { 715 this.resource = value; 716 return this; 717 } 718 719 /** 720 * @return The related resource, such as a library, value set, profile, or other 721 * knowledge resource. 722 */ 723 public String getResource() { 724 return this.resource == null ? null : this.resource.getValue(); 725 } 726 727 /** 728 * @param value The related resource, such as a library, value set, profile, or 729 * other knowledge resource. 730 */ 731 public RelatedArtifact setResource(String value) { 732 if (Utilities.noString(value)) 733 this.resource = null; 734 else { 735 if (this.resource == null) 736 this.resource = new CanonicalType(); 737 this.resource.setValue(value); 738 } 739 return this; 740 } 741 742 protected void listChildren(List<Property> children) { 743 super.listChildren(children); 744 children.add(new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type)); 745 children.add(new Property("label", "string", 746 "A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.", 747 0, 1, label)); 748 children.add(new Property("display", "string", 749 "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 750 0, 1, display)); 751 children.add(new Property("citation", "markdown", 752 "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 753 0, 1, citation)); 754 children.add(new Property("url", "url", "A url for the artifact that can be followed to access the actual content.", 755 0, 1, url)); 756 children.add(new Property("document", "Attachment", 757 "The document being referenced, represented as an attachment. This is exclusive with the resource element.", 0, 758 1, document)); 759 children.add(new Property("resource", "canonical(Any)", 760 "The related resource, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource)); 761 } 762 763 @Override 764 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 765 switch (_hash) { 766 case 3575610: 767 /* type */ return new Property("type", "code", "The type of relationship to the related artifact.", 0, 1, type); 768 case 102727412: 769 /* label */ return new Property("label", "string", 770 "A short label that can be used to reference the citation from elsewhere in the containing artifact, such as a footnote index.", 771 0, 1, label); 772 case 1671764162: 773 /* display */ return new Property("display", "string", 774 "A brief description of the document or knowledge resource being referenced, suitable for display to a consumer.", 775 0, 1, display); 776 case -1442706713: 777 /* citation */ return new Property("citation", "markdown", 778 "A bibliographic citation for the related artifact. This text SHOULD be formatted according to an accepted citation format.", 779 0, 1, citation); 780 case 116079: 781 /* url */ return new Property("url", "url", 782 "A url for the artifact that can be followed to access the actual content.", 0, 1, url); 783 case 861720859: 784 /* document */ return new Property("document", "Attachment", 785 "The document being referenced, represented as an attachment. This is exclusive with the resource element.", 786 0, 1, document); 787 case -341064690: 788 /* resource */ return new Property("resource", "canonical(Any)", 789 "The related resource, such as a library, value set, profile, or other knowledge resource.", 0, 1, resource); 790 default: 791 return super.getNamedProperty(_hash, _name, _checkValid); 792 } 793 794 } 795 796 @Override 797 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 798 switch (hash) { 799 case 3575610: 800 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<RelatedArtifactType> 801 case 102727412: 802 /* label */ return this.label == null ? new Base[0] : new Base[] { this.label }; // StringType 803 case 1671764162: 804 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 805 case -1442706713: 806 /* citation */ return this.citation == null ? new Base[0] : new Base[] { this.citation }; // MarkdownType 807 case 116079: 808 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UrlType 809 case 861720859: 810 /* document */ return this.document == null ? new Base[0] : new Base[] { this.document }; // Attachment 811 case -341064690: 812 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // CanonicalType 813 default: 814 return super.getProperty(hash, name, checkValid); 815 } 816 817 } 818 819 @Override 820 public Base setProperty(int hash, String name, Base value) throws FHIRException { 821 switch (hash) { 822 case 3575610: // type 823 value = new RelatedArtifactTypeEnumFactory().fromType(castToCode(value)); 824 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 825 return value; 826 case 102727412: // label 827 this.label = castToString(value); // StringType 828 return value; 829 case 1671764162: // display 830 this.display = castToString(value); // StringType 831 return value; 832 case -1442706713: // citation 833 this.citation = castToMarkdown(value); // MarkdownType 834 return value; 835 case 116079: // url 836 this.url = castToUrl(value); // UrlType 837 return value; 838 case 861720859: // document 839 this.document = castToAttachment(value); // Attachment 840 return value; 841 case -341064690: // resource 842 this.resource = castToCanonical(value); // CanonicalType 843 return value; 844 default: 845 return super.setProperty(hash, name, value); 846 } 847 848 } 849 850 @Override 851 public Base setProperty(String name, Base value) throws FHIRException { 852 if (name.equals("type")) { 853 value = new RelatedArtifactTypeEnumFactory().fromType(castToCode(value)); 854 this.type = (Enumeration) value; // Enumeration<RelatedArtifactType> 855 } else if (name.equals("label")) { 856 this.label = castToString(value); // StringType 857 } else if (name.equals("display")) { 858 this.display = castToString(value); // StringType 859 } else if (name.equals("citation")) { 860 this.citation = castToMarkdown(value); // MarkdownType 861 } else if (name.equals("url")) { 862 this.url = castToUrl(value); // UrlType 863 } else if (name.equals("document")) { 864 this.document = castToAttachment(value); // Attachment 865 } else if (name.equals("resource")) { 866 this.resource = castToCanonical(value); // CanonicalType 867 } else 868 return super.setProperty(name, value); 869 return value; 870 } 871 872 @Override 873 public Base makeProperty(int hash, String name) throws FHIRException { 874 switch (hash) { 875 case 3575610: 876 return getTypeElement(); 877 case 102727412: 878 return getLabelElement(); 879 case 1671764162: 880 return getDisplayElement(); 881 case -1442706713: 882 return getCitationElement(); 883 case 116079: 884 return getUrlElement(); 885 case 861720859: 886 return getDocument(); 887 case -341064690: 888 return getResourceElement(); 889 default: 890 return super.makeProperty(hash, name); 891 } 892 893 } 894 895 @Override 896 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 897 switch (hash) { 898 case 3575610: 899 /* type */ return new String[] { "code" }; 900 case 102727412: 901 /* label */ return new String[] { "string" }; 902 case 1671764162: 903 /* display */ return new String[] { "string" }; 904 case -1442706713: 905 /* citation */ return new String[] { "markdown" }; 906 case 116079: 907 /* url */ return new String[] { "url" }; 908 case 861720859: 909 /* document */ return new String[] { "Attachment" }; 910 case -341064690: 911 /* resource */ return new String[] { "canonical" }; 912 default: 913 return super.getTypesForProperty(hash, name); 914 } 915 916 } 917 918 @Override 919 public Base addChild(String name) throws FHIRException { 920 if (name.equals("type")) { 921 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.type"); 922 } else if (name.equals("label")) { 923 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.label"); 924 } else if (name.equals("display")) { 925 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.display"); 926 } else if (name.equals("citation")) { 927 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.citation"); 928 } else if (name.equals("url")) { 929 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.url"); 930 } else if (name.equals("document")) { 931 this.document = new Attachment(); 932 return this.document; 933 } else if (name.equals("resource")) { 934 throw new FHIRException("Cannot call addChild on a singleton property RelatedArtifact.resource"); 935 } else 936 return super.addChild(name); 937 } 938 939 public String fhirType() { 940 return "RelatedArtifact"; 941 942 } 943 944 public RelatedArtifact copy() { 945 RelatedArtifact dst = new RelatedArtifact(); 946 copyValues(dst); 947 return dst; 948 } 949 950 public void copyValues(RelatedArtifact dst) { 951 super.copyValues(dst); 952 dst.type = type == null ? null : type.copy(); 953 dst.label = label == null ? null : label.copy(); 954 dst.display = display == null ? null : display.copy(); 955 dst.citation = citation == null ? null : citation.copy(); 956 dst.url = url == null ? null : url.copy(); 957 dst.document = document == null ? null : document.copy(); 958 dst.resource = resource == null ? null : resource.copy(); 959 } 960 961 protected RelatedArtifact typedCopy() { 962 return copy(); 963 } 964 965 @Override 966 public boolean equalsDeep(Base other_) { 967 if (!super.equalsDeep(other_)) 968 return false; 969 if (!(other_ instanceof RelatedArtifact)) 970 return false; 971 RelatedArtifact o = (RelatedArtifact) other_; 972 return compareDeep(type, o.type, true) && compareDeep(label, o.label, true) && compareDeep(display, o.display, true) 973 && compareDeep(citation, o.citation, true) && compareDeep(url, o.url, true) 974 && compareDeep(document, o.document, true) && compareDeep(resource, o.resource, true); 975 } 976 977 @Override 978 public boolean equalsShallow(Base other_) { 979 if (!super.equalsShallow(other_)) 980 return false; 981 if (!(other_ instanceof RelatedArtifact)) 982 return false; 983 RelatedArtifact o = (RelatedArtifact) other_; 984 return compareValues(type, o.type, true) && compareValues(label, o.label, true) 985 && compareValues(display, o.display, true) && compareValues(citation, o.citation, true) 986 && compareValues(url, o.url, true); 987 } 988 989 public boolean isEmpty() { 990 return super.isEmpty() 991 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, label, display, citation, url, document, resource); 992 } 993 994}