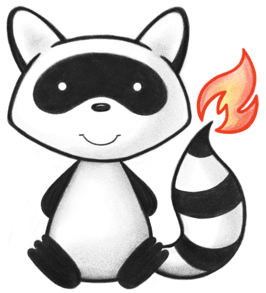
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A group of related requests that can be used to capture intended activities 049 * that have inter-dependencies such as "give this medication after that one". 050 */ 051@ResourceDef(name = "RequestGroup", profile = "http://hl7.org/fhir/StructureDefinition/RequestGroup") 052public class RequestGroup extends DomainResource { 053 054 public enum RequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action. 057 */ 058 DRAFT, 059 /** 060 * The request is in force and ready to be acted upon. 061 */ 062 ACTIVE, 063 /** 064 * The request (and any implicit authorization to act) has been temporarily 065 * withdrawn but is expected to resume in the future. 066 */ 067 ONHOLD, 068 /** 069 * The request (and any implicit authorization to act) has been terminated prior 070 * to the known full completion of the intended actions. No further activity 071 * should occur. 072 */ 073 REVOKED, 074 /** 075 * The activity described by the request has been fully performed. No further 076 * activity will occur. 077 */ 078 COMPLETED, 079 /** 080 * This request should never have existed and should be considered 'void'. (It 081 * is possible that real-world decisions were based on it. If real-world 082 * activity has occurred, the status should be "revoked" rather than 083 * "entered-in-error".). 084 */ 085 ENTEREDINERROR, 086 /** 087 * The authoring/source system does not know which of the status values 088 * currently applies for this request. Note: This concept is not to be used for 089 * "other" - one of the listed statuses is presumed to apply, but the 090 * authoring/source system does not know which. 091 */ 092 UNKNOWN, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static RequestStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("draft".equals(codeString)) 102 return DRAFT; 103 if ("active".equals(codeString)) 104 return ACTIVE; 105 if ("on-hold".equals(codeString)) 106 return ONHOLD; 107 if ("revoked".equals(codeString)) 108 return REVOKED; 109 if ("completed".equals(codeString)) 110 return COMPLETED; 111 if ("entered-in-error".equals(codeString)) 112 return ENTEREDINERROR; 113 if ("unknown".equals(codeString)) 114 return UNKNOWN; 115 if (Configuration.isAcceptInvalidEnums()) 116 return null; 117 else 118 throw new FHIRException("Unknown RequestStatus code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case DRAFT: 124 return "draft"; 125 case ACTIVE: 126 return "active"; 127 case ONHOLD: 128 return "on-hold"; 129 case REVOKED: 130 return "revoked"; 131 case COMPLETED: 132 return "completed"; 133 case ENTEREDINERROR: 134 return "entered-in-error"; 135 case UNKNOWN: 136 return "unknown"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case DRAFT: 147 return "http://hl7.org/fhir/request-status"; 148 case ACTIVE: 149 return "http://hl7.org/fhir/request-status"; 150 case ONHOLD: 151 return "http://hl7.org/fhir/request-status"; 152 case REVOKED: 153 return "http://hl7.org/fhir/request-status"; 154 case COMPLETED: 155 return "http://hl7.org/fhir/request-status"; 156 case ENTEREDINERROR: 157 return "http://hl7.org/fhir/request-status"; 158 case UNKNOWN: 159 return "http://hl7.org/fhir/request-status"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case DRAFT: 170 return "The request has been created but is not yet complete or ready for action."; 171 case ACTIVE: 172 return "The request is in force and ready to be acted upon."; 173 case ONHOLD: 174 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 175 case REVOKED: 176 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 177 case COMPLETED: 178 return "The activity described by the request has been fully performed. No further activity will occur."; 179 case ENTEREDINERROR: 180 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 181 case UNKNOWN: 182 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case DRAFT: 193 return "Draft"; 194 case ACTIVE: 195 return "Active"; 196 case ONHOLD: 197 return "On Hold"; 198 case REVOKED: 199 return "Revoked"; 200 case COMPLETED: 201 return "Completed"; 202 case ENTEREDINERROR: 203 return "Entered in Error"; 204 case UNKNOWN: 205 return "Unknown"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 } 213 214 public static class RequestStatusEnumFactory implements EnumFactory<RequestStatus> { 215 public RequestStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("draft".equals(codeString)) 220 return RequestStatus.DRAFT; 221 if ("active".equals(codeString)) 222 return RequestStatus.ACTIVE; 223 if ("on-hold".equals(codeString)) 224 return RequestStatus.ONHOLD; 225 if ("revoked".equals(codeString)) 226 return RequestStatus.REVOKED; 227 if ("completed".equals(codeString)) 228 return RequestStatus.COMPLETED; 229 if ("entered-in-error".equals(codeString)) 230 return RequestStatus.ENTEREDINERROR; 231 if ("unknown".equals(codeString)) 232 return RequestStatus.UNKNOWN; 233 throw new IllegalArgumentException("Unknown RequestStatus code '" + codeString + "'"); 234 } 235 236 public Enumeration<RequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<RequestStatus>(this, RequestStatus.NULL, code); 241 String codeString = code.asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<RequestStatus>(this, RequestStatus.NULL, code); 244 if ("draft".equals(codeString)) 245 return new Enumeration<RequestStatus>(this, RequestStatus.DRAFT, code); 246 if ("active".equals(codeString)) 247 return new Enumeration<RequestStatus>(this, RequestStatus.ACTIVE, code); 248 if ("on-hold".equals(codeString)) 249 return new Enumeration<RequestStatus>(this, RequestStatus.ONHOLD, code); 250 if ("revoked".equals(codeString)) 251 return new Enumeration<RequestStatus>(this, RequestStatus.REVOKED, code); 252 if ("completed".equals(codeString)) 253 return new Enumeration<RequestStatus>(this, RequestStatus.COMPLETED, code); 254 if ("entered-in-error".equals(codeString)) 255 return new Enumeration<RequestStatus>(this, RequestStatus.ENTEREDINERROR, code); 256 if ("unknown".equals(codeString)) 257 return new Enumeration<RequestStatus>(this, RequestStatus.UNKNOWN, code); 258 throw new FHIRException("Unknown RequestStatus code '" + codeString + "'"); 259 } 260 261 public String toCode(RequestStatus code) { 262 if (code == RequestStatus.NULL) 263 return null; 264 if (code == RequestStatus.DRAFT) 265 return "draft"; 266 if (code == RequestStatus.ACTIVE) 267 return "active"; 268 if (code == RequestStatus.ONHOLD) 269 return "on-hold"; 270 if (code == RequestStatus.REVOKED) 271 return "revoked"; 272 if (code == RequestStatus.COMPLETED) 273 return "completed"; 274 if (code == RequestStatus.ENTEREDINERROR) 275 return "entered-in-error"; 276 if (code == RequestStatus.UNKNOWN) 277 return "unknown"; 278 return "?"; 279 } 280 281 public String toSystem(RequestStatus code) { 282 return code.getSystem(); 283 } 284 } 285 286 public enum RequestIntent { 287 /** 288 * The request is a suggestion made by someone/something that does not have an 289 * intention to ensure it occurs and without providing an authorization to act. 290 */ 291 PROPOSAL, 292 /** 293 * The request represents an intention to ensure something occurs without 294 * providing an authorization for others to act. 295 */ 296 PLAN, 297 /** 298 * The request represents a legally binding instruction authored by a Patient or 299 * RelatedPerson. 300 */ 301 DIRECTIVE, 302 /** 303 * The request represents a request/demand and authorization for action by a 304 * Practitioner. 305 */ 306 ORDER, 307 /** 308 * The request represents an original authorization for action. 309 */ 310 ORIGINALORDER, 311 /** 312 * The request represents an automatically generated supplemental authorization 313 * for action based on a parent authorization together with initial results of 314 * the action taken against that parent authorization. 315 */ 316 REFLEXORDER, 317 /** 318 * The request represents the view of an authorization instantiated by a 319 * fulfilling system representing the details of the fulfiller's intention to 320 * act upon a submitted order. 321 */ 322 FILLERORDER, 323 /** 324 * An order created in fulfillment of a broader order that represents the 325 * authorization for a single activity occurrence. E.g. The administration of a 326 * single dose of a drug. 327 */ 328 INSTANCEORDER, 329 /** 330 * The request represents a component or option for a RequestGroup that 331 * establishes timing, conditionality and/or other constraints among a set of 332 * requests. Refer to [[[RequestGroup]]] for additional information on how this 333 * status is used. 334 */ 335 OPTION, 336 /** 337 * added to help the parsers with the generic types 338 */ 339 NULL; 340 341 public static RequestIntent fromCode(String codeString) throws FHIRException { 342 if (codeString == null || "".equals(codeString)) 343 return null; 344 if ("proposal".equals(codeString)) 345 return PROPOSAL; 346 if ("plan".equals(codeString)) 347 return PLAN; 348 if ("directive".equals(codeString)) 349 return DIRECTIVE; 350 if ("order".equals(codeString)) 351 return ORDER; 352 if ("original-order".equals(codeString)) 353 return ORIGINALORDER; 354 if ("reflex-order".equals(codeString)) 355 return REFLEXORDER; 356 if ("filler-order".equals(codeString)) 357 return FILLERORDER; 358 if ("instance-order".equals(codeString)) 359 return INSTANCEORDER; 360 if ("option".equals(codeString)) 361 return OPTION; 362 if (Configuration.isAcceptInvalidEnums()) 363 return null; 364 else 365 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 366 } 367 368 public String toCode() { 369 switch (this) { 370 case PROPOSAL: 371 return "proposal"; 372 case PLAN: 373 return "plan"; 374 case DIRECTIVE: 375 return "directive"; 376 case ORDER: 377 return "order"; 378 case ORIGINALORDER: 379 return "original-order"; 380 case REFLEXORDER: 381 return "reflex-order"; 382 case FILLERORDER: 383 return "filler-order"; 384 case INSTANCEORDER: 385 return "instance-order"; 386 case OPTION: 387 return "option"; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 395 public String getSystem() { 396 switch (this) { 397 case PROPOSAL: 398 return "http://hl7.org/fhir/request-intent"; 399 case PLAN: 400 return "http://hl7.org/fhir/request-intent"; 401 case DIRECTIVE: 402 return "http://hl7.org/fhir/request-intent"; 403 case ORDER: 404 return "http://hl7.org/fhir/request-intent"; 405 case ORIGINALORDER: 406 return "http://hl7.org/fhir/request-intent"; 407 case REFLEXORDER: 408 return "http://hl7.org/fhir/request-intent"; 409 case FILLERORDER: 410 return "http://hl7.org/fhir/request-intent"; 411 case INSTANCEORDER: 412 return "http://hl7.org/fhir/request-intent"; 413 case OPTION: 414 return "http://hl7.org/fhir/request-intent"; 415 case NULL: 416 return null; 417 default: 418 return "?"; 419 } 420 } 421 422 public String getDefinition() { 423 switch (this) { 424 case PROPOSAL: 425 return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 426 case PLAN: 427 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 428 case DIRECTIVE: 429 return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 430 case ORDER: 431 return "The request represents a request/demand and authorization for action by a Practitioner."; 432 case ORIGINALORDER: 433 return "The request represents an original authorization for action."; 434 case REFLEXORDER: 435 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 436 case FILLERORDER: 437 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 438 case INSTANCEORDER: 439 return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 440 case OPTION: 441 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 442 case NULL: 443 return null; 444 default: 445 return "?"; 446 } 447 } 448 449 public String getDisplay() { 450 switch (this) { 451 case PROPOSAL: 452 return "Proposal"; 453 case PLAN: 454 return "Plan"; 455 case DIRECTIVE: 456 return "Directive"; 457 case ORDER: 458 return "Order"; 459 case ORIGINALORDER: 460 return "Original Order"; 461 case REFLEXORDER: 462 return "Reflex Order"; 463 case FILLERORDER: 464 return "Filler Order"; 465 case INSTANCEORDER: 466 return "Instance Order"; 467 case OPTION: 468 return "Option"; 469 case NULL: 470 return null; 471 default: 472 return "?"; 473 } 474 } 475 } 476 477 public static class RequestIntentEnumFactory implements EnumFactory<RequestIntent> { 478 public RequestIntent fromCode(String codeString) throws IllegalArgumentException { 479 if (codeString == null || "".equals(codeString)) 480 if (codeString == null || "".equals(codeString)) 481 return null; 482 if ("proposal".equals(codeString)) 483 return RequestIntent.PROPOSAL; 484 if ("plan".equals(codeString)) 485 return RequestIntent.PLAN; 486 if ("directive".equals(codeString)) 487 return RequestIntent.DIRECTIVE; 488 if ("order".equals(codeString)) 489 return RequestIntent.ORDER; 490 if ("original-order".equals(codeString)) 491 return RequestIntent.ORIGINALORDER; 492 if ("reflex-order".equals(codeString)) 493 return RequestIntent.REFLEXORDER; 494 if ("filler-order".equals(codeString)) 495 return RequestIntent.FILLERORDER; 496 if ("instance-order".equals(codeString)) 497 return RequestIntent.INSTANCEORDER; 498 if ("option".equals(codeString)) 499 return RequestIntent.OPTION; 500 throw new IllegalArgumentException("Unknown RequestIntent code '" + codeString + "'"); 501 } 502 503 public Enumeration<RequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 504 if (code == null) 505 return null; 506 if (code.isEmpty()) 507 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 508 String codeString = code.asStringValue(); 509 if (codeString == null || "".equals(codeString)) 510 return new Enumeration<RequestIntent>(this, RequestIntent.NULL, code); 511 if ("proposal".equals(codeString)) 512 return new Enumeration<RequestIntent>(this, RequestIntent.PROPOSAL, code); 513 if ("plan".equals(codeString)) 514 return new Enumeration<RequestIntent>(this, RequestIntent.PLAN, code); 515 if ("directive".equals(codeString)) 516 return new Enumeration<RequestIntent>(this, RequestIntent.DIRECTIVE, code); 517 if ("order".equals(codeString)) 518 return new Enumeration<RequestIntent>(this, RequestIntent.ORDER, code); 519 if ("original-order".equals(codeString)) 520 return new Enumeration<RequestIntent>(this, RequestIntent.ORIGINALORDER, code); 521 if ("reflex-order".equals(codeString)) 522 return new Enumeration<RequestIntent>(this, RequestIntent.REFLEXORDER, code); 523 if ("filler-order".equals(codeString)) 524 return new Enumeration<RequestIntent>(this, RequestIntent.FILLERORDER, code); 525 if ("instance-order".equals(codeString)) 526 return new Enumeration<RequestIntent>(this, RequestIntent.INSTANCEORDER, code); 527 if ("option".equals(codeString)) 528 return new Enumeration<RequestIntent>(this, RequestIntent.OPTION, code); 529 throw new FHIRException("Unknown RequestIntent code '" + codeString + "'"); 530 } 531 532 public String toCode(RequestIntent code) { 533 if (code == RequestIntent.NULL) 534 return null; 535 if (code == RequestIntent.PROPOSAL) 536 return "proposal"; 537 if (code == RequestIntent.PLAN) 538 return "plan"; 539 if (code == RequestIntent.DIRECTIVE) 540 return "directive"; 541 if (code == RequestIntent.ORDER) 542 return "order"; 543 if (code == RequestIntent.ORIGINALORDER) 544 return "original-order"; 545 if (code == RequestIntent.REFLEXORDER) 546 return "reflex-order"; 547 if (code == RequestIntent.FILLERORDER) 548 return "filler-order"; 549 if (code == RequestIntent.INSTANCEORDER) 550 return "instance-order"; 551 if (code == RequestIntent.OPTION) 552 return "option"; 553 return "?"; 554 } 555 556 public String toSystem(RequestIntent code) { 557 return code.getSystem(); 558 } 559 } 560 561 public enum RequestPriority { 562 /** 563 * The request has normal priority. 564 */ 565 ROUTINE, 566 /** 567 * The request should be actioned promptly - higher priority than routine. 568 */ 569 URGENT, 570 /** 571 * The request should be actioned as soon as possible - higher priority than 572 * urgent. 573 */ 574 ASAP, 575 /** 576 * The request should be actioned immediately - highest possible priority. E.g. 577 * an emergency. 578 */ 579 STAT, 580 /** 581 * added to help the parsers with the generic types 582 */ 583 NULL; 584 585 public static RequestPriority fromCode(String codeString) throws FHIRException { 586 if (codeString == null || "".equals(codeString)) 587 return null; 588 if ("routine".equals(codeString)) 589 return ROUTINE; 590 if ("urgent".equals(codeString)) 591 return URGENT; 592 if ("asap".equals(codeString)) 593 return ASAP; 594 if ("stat".equals(codeString)) 595 return STAT; 596 if (Configuration.isAcceptInvalidEnums()) 597 return null; 598 else 599 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 600 } 601 602 public String toCode() { 603 switch (this) { 604 case ROUTINE: 605 return "routine"; 606 case URGENT: 607 return "urgent"; 608 case ASAP: 609 return "asap"; 610 case STAT: 611 return "stat"; 612 case NULL: 613 return null; 614 default: 615 return "?"; 616 } 617 } 618 619 public String getSystem() { 620 switch (this) { 621 case ROUTINE: 622 return "http://hl7.org/fhir/request-priority"; 623 case URGENT: 624 return "http://hl7.org/fhir/request-priority"; 625 case ASAP: 626 return "http://hl7.org/fhir/request-priority"; 627 case STAT: 628 return "http://hl7.org/fhir/request-priority"; 629 case NULL: 630 return null; 631 default: 632 return "?"; 633 } 634 } 635 636 public String getDefinition() { 637 switch (this) { 638 case ROUTINE: 639 return "The request has normal priority."; 640 case URGENT: 641 return "The request should be actioned promptly - higher priority than routine."; 642 case ASAP: 643 return "The request should be actioned as soon as possible - higher priority than urgent."; 644 case STAT: 645 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 646 case NULL: 647 return null; 648 default: 649 return "?"; 650 } 651 } 652 653 public String getDisplay() { 654 switch (this) { 655 case ROUTINE: 656 return "Routine"; 657 case URGENT: 658 return "Urgent"; 659 case ASAP: 660 return "ASAP"; 661 case STAT: 662 return "STAT"; 663 case NULL: 664 return null; 665 default: 666 return "?"; 667 } 668 } 669 } 670 671 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 672 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 673 if (codeString == null || "".equals(codeString)) 674 if (codeString == null || "".equals(codeString)) 675 return null; 676 if ("routine".equals(codeString)) 677 return RequestPriority.ROUTINE; 678 if ("urgent".equals(codeString)) 679 return RequestPriority.URGENT; 680 if ("asap".equals(codeString)) 681 return RequestPriority.ASAP; 682 if ("stat".equals(codeString)) 683 return RequestPriority.STAT; 684 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 685 } 686 687 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 688 if (code == null) 689 return null; 690 if (code.isEmpty()) 691 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 692 String codeString = code.asStringValue(); 693 if (codeString == null || "".equals(codeString)) 694 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 695 if ("routine".equals(codeString)) 696 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 697 if ("urgent".equals(codeString)) 698 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 699 if ("asap".equals(codeString)) 700 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 701 if ("stat".equals(codeString)) 702 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 703 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 704 } 705 706 public String toCode(RequestPriority code) { 707 if (code == RequestPriority.NULL) 708 return null; 709 if (code == RequestPriority.ROUTINE) 710 return "routine"; 711 if (code == RequestPriority.URGENT) 712 return "urgent"; 713 if (code == RequestPriority.ASAP) 714 return "asap"; 715 if (code == RequestPriority.STAT) 716 return "stat"; 717 return "?"; 718 } 719 720 public String toSystem(RequestPriority code) { 721 return code.getSystem(); 722 } 723 } 724 725 public enum ActionConditionKind { 726 /** 727 * The condition describes whether or not a given action is applicable. 728 */ 729 APPLICABILITY, 730 /** 731 * The condition is a starting condition for the action. 732 */ 733 START, 734 /** 735 * The condition is a stop, or exit condition for the action. 736 */ 737 STOP, 738 /** 739 * added to help the parsers with the generic types 740 */ 741 NULL; 742 743 public static ActionConditionKind fromCode(String codeString) throws FHIRException { 744 if (codeString == null || "".equals(codeString)) 745 return null; 746 if ("applicability".equals(codeString)) 747 return APPLICABILITY; 748 if ("start".equals(codeString)) 749 return START; 750 if ("stop".equals(codeString)) 751 return STOP; 752 if (Configuration.isAcceptInvalidEnums()) 753 return null; 754 else 755 throw new FHIRException("Unknown ActionConditionKind code '" + codeString + "'"); 756 } 757 758 public String toCode() { 759 switch (this) { 760 case APPLICABILITY: 761 return "applicability"; 762 case START: 763 return "start"; 764 case STOP: 765 return "stop"; 766 case NULL: 767 return null; 768 default: 769 return "?"; 770 } 771 } 772 773 public String getSystem() { 774 switch (this) { 775 case APPLICABILITY: 776 return "http://hl7.org/fhir/action-condition-kind"; 777 case START: 778 return "http://hl7.org/fhir/action-condition-kind"; 779 case STOP: 780 return "http://hl7.org/fhir/action-condition-kind"; 781 case NULL: 782 return null; 783 default: 784 return "?"; 785 } 786 } 787 788 public String getDefinition() { 789 switch (this) { 790 case APPLICABILITY: 791 return "The condition describes whether or not a given action is applicable."; 792 case START: 793 return "The condition is a starting condition for the action."; 794 case STOP: 795 return "The condition is a stop, or exit condition for the action."; 796 case NULL: 797 return null; 798 default: 799 return "?"; 800 } 801 } 802 803 public String getDisplay() { 804 switch (this) { 805 case APPLICABILITY: 806 return "Applicability"; 807 case START: 808 return "Start"; 809 case STOP: 810 return "Stop"; 811 case NULL: 812 return null; 813 default: 814 return "?"; 815 } 816 } 817 } 818 819 public static class ActionConditionKindEnumFactory implements EnumFactory<ActionConditionKind> { 820 public ActionConditionKind fromCode(String codeString) throws IllegalArgumentException { 821 if (codeString == null || "".equals(codeString)) 822 if (codeString == null || "".equals(codeString)) 823 return null; 824 if ("applicability".equals(codeString)) 825 return ActionConditionKind.APPLICABILITY; 826 if ("start".equals(codeString)) 827 return ActionConditionKind.START; 828 if ("stop".equals(codeString)) 829 return ActionConditionKind.STOP; 830 throw new IllegalArgumentException("Unknown ActionConditionKind code '" + codeString + "'"); 831 } 832 833 public Enumeration<ActionConditionKind> fromType(PrimitiveType<?> code) throws FHIRException { 834 if (code == null) 835 return null; 836 if (code.isEmpty()) 837 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.NULL, code); 838 String codeString = code.asStringValue(); 839 if (codeString == null || "".equals(codeString)) 840 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.NULL, code); 841 if ("applicability".equals(codeString)) 842 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.APPLICABILITY, code); 843 if ("start".equals(codeString)) 844 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.START, code); 845 if ("stop".equals(codeString)) 846 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.STOP, code); 847 throw new FHIRException("Unknown ActionConditionKind code '" + codeString + "'"); 848 } 849 850 public String toCode(ActionConditionKind code) { 851 if (code == ActionConditionKind.NULL) 852 return null; 853 if (code == ActionConditionKind.APPLICABILITY) 854 return "applicability"; 855 if (code == ActionConditionKind.START) 856 return "start"; 857 if (code == ActionConditionKind.STOP) 858 return "stop"; 859 return "?"; 860 } 861 862 public String toSystem(ActionConditionKind code) { 863 return code.getSystem(); 864 } 865 } 866 867 public enum ActionRelationshipType { 868 /** 869 * The action must be performed before the start of the related action. 870 */ 871 BEFORESTART, 872 /** 873 * The action must be performed before the related action. 874 */ 875 BEFORE, 876 /** 877 * The action must be performed before the end of the related action. 878 */ 879 BEFOREEND, 880 /** 881 * The action must be performed concurrent with the start of the related action. 882 */ 883 CONCURRENTWITHSTART, 884 /** 885 * The action must be performed concurrent with the related action. 886 */ 887 CONCURRENT, 888 /** 889 * The action must be performed concurrent with the end of the related action. 890 */ 891 CONCURRENTWITHEND, 892 /** 893 * The action must be performed after the start of the related action. 894 */ 895 AFTERSTART, 896 /** 897 * The action must be performed after the related action. 898 */ 899 AFTER, 900 /** 901 * The action must be performed after the end of the related action. 902 */ 903 AFTEREND, 904 /** 905 * added to help the parsers with the generic types 906 */ 907 NULL; 908 909 public static ActionRelationshipType fromCode(String codeString) throws FHIRException { 910 if (codeString == null || "".equals(codeString)) 911 return null; 912 if ("before-start".equals(codeString)) 913 return BEFORESTART; 914 if ("before".equals(codeString)) 915 return BEFORE; 916 if ("before-end".equals(codeString)) 917 return BEFOREEND; 918 if ("concurrent-with-start".equals(codeString)) 919 return CONCURRENTWITHSTART; 920 if ("concurrent".equals(codeString)) 921 return CONCURRENT; 922 if ("concurrent-with-end".equals(codeString)) 923 return CONCURRENTWITHEND; 924 if ("after-start".equals(codeString)) 925 return AFTERSTART; 926 if ("after".equals(codeString)) 927 return AFTER; 928 if ("after-end".equals(codeString)) 929 return AFTEREND; 930 if (Configuration.isAcceptInvalidEnums()) 931 return null; 932 else 933 throw new FHIRException("Unknown ActionRelationshipType code '" + codeString + "'"); 934 } 935 936 public String toCode() { 937 switch (this) { 938 case BEFORESTART: 939 return "before-start"; 940 case BEFORE: 941 return "before"; 942 case BEFOREEND: 943 return "before-end"; 944 case CONCURRENTWITHSTART: 945 return "concurrent-with-start"; 946 case CONCURRENT: 947 return "concurrent"; 948 case CONCURRENTWITHEND: 949 return "concurrent-with-end"; 950 case AFTERSTART: 951 return "after-start"; 952 case AFTER: 953 return "after"; 954 case AFTEREND: 955 return "after-end"; 956 case NULL: 957 return null; 958 default: 959 return "?"; 960 } 961 } 962 963 public String getSystem() { 964 switch (this) { 965 case BEFORESTART: 966 return "http://hl7.org/fhir/action-relationship-type"; 967 case BEFORE: 968 return "http://hl7.org/fhir/action-relationship-type"; 969 case BEFOREEND: 970 return "http://hl7.org/fhir/action-relationship-type"; 971 case CONCURRENTWITHSTART: 972 return "http://hl7.org/fhir/action-relationship-type"; 973 case CONCURRENT: 974 return "http://hl7.org/fhir/action-relationship-type"; 975 case CONCURRENTWITHEND: 976 return "http://hl7.org/fhir/action-relationship-type"; 977 case AFTERSTART: 978 return "http://hl7.org/fhir/action-relationship-type"; 979 case AFTER: 980 return "http://hl7.org/fhir/action-relationship-type"; 981 case AFTEREND: 982 return "http://hl7.org/fhir/action-relationship-type"; 983 case NULL: 984 return null; 985 default: 986 return "?"; 987 } 988 } 989 990 public String getDefinition() { 991 switch (this) { 992 case BEFORESTART: 993 return "The action must be performed before the start of the related action."; 994 case BEFORE: 995 return "The action must be performed before the related action."; 996 case BEFOREEND: 997 return "The action must be performed before the end of the related action."; 998 case CONCURRENTWITHSTART: 999 return "The action must be performed concurrent with the start of the related action."; 1000 case CONCURRENT: 1001 return "The action must be performed concurrent with the related action."; 1002 case CONCURRENTWITHEND: 1003 return "The action must be performed concurrent with the end of the related action."; 1004 case AFTERSTART: 1005 return "The action must be performed after the start of the related action."; 1006 case AFTER: 1007 return "The action must be performed after the related action."; 1008 case AFTEREND: 1009 return "The action must be performed after the end of the related action."; 1010 case NULL: 1011 return null; 1012 default: 1013 return "?"; 1014 } 1015 } 1016 1017 public String getDisplay() { 1018 switch (this) { 1019 case BEFORESTART: 1020 return "Before Start"; 1021 case BEFORE: 1022 return "Before"; 1023 case BEFOREEND: 1024 return "Before End"; 1025 case CONCURRENTWITHSTART: 1026 return "Concurrent With Start"; 1027 case CONCURRENT: 1028 return "Concurrent"; 1029 case CONCURRENTWITHEND: 1030 return "Concurrent With End"; 1031 case AFTERSTART: 1032 return "After Start"; 1033 case AFTER: 1034 return "After"; 1035 case AFTEREND: 1036 return "After End"; 1037 case NULL: 1038 return null; 1039 default: 1040 return "?"; 1041 } 1042 } 1043 } 1044 1045 public static class ActionRelationshipTypeEnumFactory implements EnumFactory<ActionRelationshipType> { 1046 public ActionRelationshipType fromCode(String codeString) throws IllegalArgumentException { 1047 if (codeString == null || "".equals(codeString)) 1048 if (codeString == null || "".equals(codeString)) 1049 return null; 1050 if ("before-start".equals(codeString)) 1051 return ActionRelationshipType.BEFORESTART; 1052 if ("before".equals(codeString)) 1053 return ActionRelationshipType.BEFORE; 1054 if ("before-end".equals(codeString)) 1055 return ActionRelationshipType.BEFOREEND; 1056 if ("concurrent-with-start".equals(codeString)) 1057 return ActionRelationshipType.CONCURRENTWITHSTART; 1058 if ("concurrent".equals(codeString)) 1059 return ActionRelationshipType.CONCURRENT; 1060 if ("concurrent-with-end".equals(codeString)) 1061 return ActionRelationshipType.CONCURRENTWITHEND; 1062 if ("after-start".equals(codeString)) 1063 return ActionRelationshipType.AFTERSTART; 1064 if ("after".equals(codeString)) 1065 return ActionRelationshipType.AFTER; 1066 if ("after-end".equals(codeString)) 1067 return ActionRelationshipType.AFTEREND; 1068 throw new IllegalArgumentException("Unknown ActionRelationshipType code '" + codeString + "'"); 1069 } 1070 1071 public Enumeration<ActionRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 1072 if (code == null) 1073 return null; 1074 if (code.isEmpty()) 1075 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.NULL, code); 1076 String codeString = code.asStringValue(); 1077 if (codeString == null || "".equals(codeString)) 1078 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.NULL, code); 1079 if ("before-start".equals(codeString)) 1080 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORESTART, code); 1081 if ("before".equals(codeString)) 1082 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORE, code); 1083 if ("before-end".equals(codeString)) 1084 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFOREEND, code); 1085 if ("concurrent-with-start".equals(codeString)) 1086 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHSTART, code); 1087 if ("concurrent".equals(codeString)) 1088 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENT, code); 1089 if ("concurrent-with-end".equals(codeString)) 1090 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHEND, code); 1091 if ("after-start".equals(codeString)) 1092 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTERSTART, code); 1093 if ("after".equals(codeString)) 1094 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTER, code); 1095 if ("after-end".equals(codeString)) 1096 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTEREND, code); 1097 throw new FHIRException("Unknown ActionRelationshipType code '" + codeString + "'"); 1098 } 1099 1100 public String toCode(ActionRelationshipType code) { 1101 if (code == ActionRelationshipType.NULL) 1102 return null; 1103 if (code == ActionRelationshipType.BEFORESTART) 1104 return "before-start"; 1105 if (code == ActionRelationshipType.BEFORE) 1106 return "before"; 1107 if (code == ActionRelationshipType.BEFOREEND) 1108 return "before-end"; 1109 if (code == ActionRelationshipType.CONCURRENTWITHSTART) 1110 return "concurrent-with-start"; 1111 if (code == ActionRelationshipType.CONCURRENT) 1112 return "concurrent"; 1113 if (code == ActionRelationshipType.CONCURRENTWITHEND) 1114 return "concurrent-with-end"; 1115 if (code == ActionRelationshipType.AFTERSTART) 1116 return "after-start"; 1117 if (code == ActionRelationshipType.AFTER) 1118 return "after"; 1119 if (code == ActionRelationshipType.AFTEREND) 1120 return "after-end"; 1121 return "?"; 1122 } 1123 1124 public String toSystem(ActionRelationshipType code) { 1125 return code.getSystem(); 1126 } 1127 } 1128 1129 public enum ActionGroupingBehavior { 1130 /** 1131 * Any group marked with this behavior should be displayed as a visual group to 1132 * the end user. 1133 */ 1134 VISUALGROUP, 1135 /** 1136 * A group with this behavior logically groups its sub-elements, and may be 1137 * shown as a visual group to the end user, but it is not required to do so. 1138 */ 1139 LOGICALGROUP, 1140 /** 1141 * A group of related alternative actions is a sentence group if the target 1142 * referenced by the action is the same in all the actions and each action 1143 * simply constitutes a different variation on how to specify the details for 1144 * the target. For example, two actions that could be in a SentenceGroup are 1145 * "aspirin, 500 mg, 2 times per day" and "aspirin, 300 mg, 3 times per day". In 1146 * both cases, aspirin is the target referenced by the action, and the two 1147 * actions represent different options for how aspirin might be ordered for the 1148 * patient. Note that a SentenceGroup would almost always have an associated 1149 * selection behavior of "AtMostOne", unless it's a required action, in which 1150 * case, it would be "ExactlyOne". 1151 */ 1152 SENTENCEGROUP, 1153 /** 1154 * added to help the parsers with the generic types 1155 */ 1156 NULL; 1157 1158 public static ActionGroupingBehavior fromCode(String codeString) throws FHIRException { 1159 if (codeString == null || "".equals(codeString)) 1160 return null; 1161 if ("visual-group".equals(codeString)) 1162 return VISUALGROUP; 1163 if ("logical-group".equals(codeString)) 1164 return LOGICALGROUP; 1165 if ("sentence-group".equals(codeString)) 1166 return SENTENCEGROUP; 1167 if (Configuration.isAcceptInvalidEnums()) 1168 return null; 1169 else 1170 throw new FHIRException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 1171 } 1172 1173 public String toCode() { 1174 switch (this) { 1175 case VISUALGROUP: 1176 return "visual-group"; 1177 case LOGICALGROUP: 1178 return "logical-group"; 1179 case SENTENCEGROUP: 1180 return "sentence-group"; 1181 case NULL: 1182 return null; 1183 default: 1184 return "?"; 1185 } 1186 } 1187 1188 public String getSystem() { 1189 switch (this) { 1190 case VISUALGROUP: 1191 return "http://hl7.org/fhir/action-grouping-behavior"; 1192 case LOGICALGROUP: 1193 return "http://hl7.org/fhir/action-grouping-behavior"; 1194 case SENTENCEGROUP: 1195 return "http://hl7.org/fhir/action-grouping-behavior"; 1196 case NULL: 1197 return null; 1198 default: 1199 return "?"; 1200 } 1201 } 1202 1203 public String getDefinition() { 1204 switch (this) { 1205 case VISUALGROUP: 1206 return "Any group marked with this behavior should be displayed as a visual group to the end user."; 1207 case LOGICALGROUP: 1208 return "A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so."; 1209 case SENTENCEGROUP: 1210 return "A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are \"aspirin, 500 mg, 2 times per day\" and \"aspirin, 300 mg, 3 times per day\". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of \"AtMostOne\", unless it's a required action, in which case, it would be \"ExactlyOne\"."; 1211 case NULL: 1212 return null; 1213 default: 1214 return "?"; 1215 } 1216 } 1217 1218 public String getDisplay() { 1219 switch (this) { 1220 case VISUALGROUP: 1221 return "Visual Group"; 1222 case LOGICALGROUP: 1223 return "Logical Group"; 1224 case SENTENCEGROUP: 1225 return "Sentence Group"; 1226 case NULL: 1227 return null; 1228 default: 1229 return "?"; 1230 } 1231 } 1232 } 1233 1234 public static class ActionGroupingBehaviorEnumFactory implements EnumFactory<ActionGroupingBehavior> { 1235 public ActionGroupingBehavior fromCode(String codeString) throws IllegalArgumentException { 1236 if (codeString == null || "".equals(codeString)) 1237 if (codeString == null || "".equals(codeString)) 1238 return null; 1239 if ("visual-group".equals(codeString)) 1240 return ActionGroupingBehavior.VISUALGROUP; 1241 if ("logical-group".equals(codeString)) 1242 return ActionGroupingBehavior.LOGICALGROUP; 1243 if ("sentence-group".equals(codeString)) 1244 return ActionGroupingBehavior.SENTENCEGROUP; 1245 throw new IllegalArgumentException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 1246 } 1247 1248 public Enumeration<ActionGroupingBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1249 if (code == null) 1250 return null; 1251 if (code.isEmpty()) 1252 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.NULL, code); 1253 String codeString = code.asStringValue(); 1254 if (codeString == null || "".equals(codeString)) 1255 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.NULL, code); 1256 if ("visual-group".equals(codeString)) 1257 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.VISUALGROUP, code); 1258 if ("logical-group".equals(codeString)) 1259 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.LOGICALGROUP, code); 1260 if ("sentence-group".equals(codeString)) 1261 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.SENTENCEGROUP, code); 1262 throw new FHIRException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 1263 } 1264 1265 public String toCode(ActionGroupingBehavior code) { 1266 if (code == ActionGroupingBehavior.NULL) 1267 return null; 1268 if (code == ActionGroupingBehavior.VISUALGROUP) 1269 return "visual-group"; 1270 if (code == ActionGroupingBehavior.LOGICALGROUP) 1271 return "logical-group"; 1272 if (code == ActionGroupingBehavior.SENTENCEGROUP) 1273 return "sentence-group"; 1274 return "?"; 1275 } 1276 1277 public String toSystem(ActionGroupingBehavior code) { 1278 return code.getSystem(); 1279 } 1280 } 1281 1282 public enum ActionSelectionBehavior { 1283 /** 1284 * Any number of the actions in the group may be chosen, from zero to all. 1285 */ 1286 ANY, 1287 /** 1288 * All the actions in the group must be selected as a single unit. 1289 */ 1290 ALL, 1291 /** 1292 * All the actions in the group are meant to be chosen as a single unit: either 1293 * all must be selected by the end user, or none may be selected. 1294 */ 1295 ALLORNONE, 1296 /** 1297 * The end user must choose one and only one of the selectable actions in the 1298 * group. The user SHALL NOT choose none of the actions in the group. 1299 */ 1300 EXACTLYONE, 1301 /** 1302 * The end user may choose zero or at most one of the actions in the group. 1303 */ 1304 ATMOSTONE, 1305 /** 1306 * The end user must choose a minimum of one, and as many additional as desired. 1307 */ 1308 ONEORMORE, 1309 /** 1310 * added to help the parsers with the generic types 1311 */ 1312 NULL; 1313 1314 public static ActionSelectionBehavior fromCode(String codeString) throws FHIRException { 1315 if (codeString == null || "".equals(codeString)) 1316 return null; 1317 if ("any".equals(codeString)) 1318 return ANY; 1319 if ("all".equals(codeString)) 1320 return ALL; 1321 if ("all-or-none".equals(codeString)) 1322 return ALLORNONE; 1323 if ("exactly-one".equals(codeString)) 1324 return EXACTLYONE; 1325 if ("at-most-one".equals(codeString)) 1326 return ATMOSTONE; 1327 if ("one-or-more".equals(codeString)) 1328 return ONEORMORE; 1329 if (Configuration.isAcceptInvalidEnums()) 1330 return null; 1331 else 1332 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1333 } 1334 1335 public String toCode() { 1336 switch (this) { 1337 case ANY: 1338 return "any"; 1339 case ALL: 1340 return "all"; 1341 case ALLORNONE: 1342 return "all-or-none"; 1343 case EXACTLYONE: 1344 return "exactly-one"; 1345 case ATMOSTONE: 1346 return "at-most-one"; 1347 case ONEORMORE: 1348 return "one-or-more"; 1349 case NULL: 1350 return null; 1351 default: 1352 return "?"; 1353 } 1354 } 1355 1356 public String getSystem() { 1357 switch (this) { 1358 case ANY: 1359 return "http://hl7.org/fhir/action-selection-behavior"; 1360 case ALL: 1361 return "http://hl7.org/fhir/action-selection-behavior"; 1362 case ALLORNONE: 1363 return "http://hl7.org/fhir/action-selection-behavior"; 1364 case EXACTLYONE: 1365 return "http://hl7.org/fhir/action-selection-behavior"; 1366 case ATMOSTONE: 1367 return "http://hl7.org/fhir/action-selection-behavior"; 1368 case ONEORMORE: 1369 return "http://hl7.org/fhir/action-selection-behavior"; 1370 case NULL: 1371 return null; 1372 default: 1373 return "?"; 1374 } 1375 } 1376 1377 public String getDefinition() { 1378 switch (this) { 1379 case ANY: 1380 return "Any number of the actions in the group may be chosen, from zero to all."; 1381 case ALL: 1382 return "All the actions in the group must be selected as a single unit."; 1383 case ALLORNONE: 1384 return "All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected."; 1385 case EXACTLYONE: 1386 return "The end user must choose one and only one of the selectable actions in the group. The user SHALL NOT choose none of the actions in the group."; 1387 case ATMOSTONE: 1388 return "The end user may choose zero or at most one of the actions in the group."; 1389 case ONEORMORE: 1390 return "The end user must choose a minimum of one, and as many additional as desired."; 1391 case NULL: 1392 return null; 1393 default: 1394 return "?"; 1395 } 1396 } 1397 1398 public String getDisplay() { 1399 switch (this) { 1400 case ANY: 1401 return "Any"; 1402 case ALL: 1403 return "All"; 1404 case ALLORNONE: 1405 return "All Or None"; 1406 case EXACTLYONE: 1407 return "Exactly One"; 1408 case ATMOSTONE: 1409 return "At Most One"; 1410 case ONEORMORE: 1411 return "One Or More"; 1412 case NULL: 1413 return null; 1414 default: 1415 return "?"; 1416 } 1417 } 1418 } 1419 1420 public static class ActionSelectionBehaviorEnumFactory implements EnumFactory<ActionSelectionBehavior> { 1421 public ActionSelectionBehavior fromCode(String codeString) throws IllegalArgumentException { 1422 if (codeString == null || "".equals(codeString)) 1423 if (codeString == null || "".equals(codeString)) 1424 return null; 1425 if ("any".equals(codeString)) 1426 return ActionSelectionBehavior.ANY; 1427 if ("all".equals(codeString)) 1428 return ActionSelectionBehavior.ALL; 1429 if ("all-or-none".equals(codeString)) 1430 return ActionSelectionBehavior.ALLORNONE; 1431 if ("exactly-one".equals(codeString)) 1432 return ActionSelectionBehavior.EXACTLYONE; 1433 if ("at-most-one".equals(codeString)) 1434 return ActionSelectionBehavior.ATMOSTONE; 1435 if ("one-or-more".equals(codeString)) 1436 return ActionSelectionBehavior.ONEORMORE; 1437 throw new IllegalArgumentException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1438 } 1439 1440 public Enumeration<ActionSelectionBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1441 if (code == null) 1442 return null; 1443 if (code.isEmpty()) 1444 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.NULL, code); 1445 String codeString = code.asStringValue(); 1446 if (codeString == null || "".equals(codeString)) 1447 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.NULL, code); 1448 if ("any".equals(codeString)) 1449 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ANY, code); 1450 if ("all".equals(codeString)) 1451 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALL, code); 1452 if ("all-or-none".equals(codeString)) 1453 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALLORNONE, code); 1454 if ("exactly-one".equals(codeString)) 1455 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.EXACTLYONE, code); 1456 if ("at-most-one".equals(codeString)) 1457 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ATMOSTONE, code); 1458 if ("one-or-more".equals(codeString)) 1459 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ONEORMORE, code); 1460 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1461 } 1462 1463 public String toCode(ActionSelectionBehavior code) { 1464 if (code == ActionSelectionBehavior.NULL) 1465 return null; 1466 if (code == ActionSelectionBehavior.ANY) 1467 return "any"; 1468 if (code == ActionSelectionBehavior.ALL) 1469 return "all"; 1470 if (code == ActionSelectionBehavior.ALLORNONE) 1471 return "all-or-none"; 1472 if (code == ActionSelectionBehavior.EXACTLYONE) 1473 return "exactly-one"; 1474 if (code == ActionSelectionBehavior.ATMOSTONE) 1475 return "at-most-one"; 1476 if (code == ActionSelectionBehavior.ONEORMORE) 1477 return "one-or-more"; 1478 return "?"; 1479 } 1480 1481 public String toSystem(ActionSelectionBehavior code) { 1482 return code.getSystem(); 1483 } 1484 } 1485 1486 public enum ActionRequiredBehavior { 1487 /** 1488 * An action with this behavior must be included in the actions processed by the 1489 * end user; the end user SHALL NOT choose not to include this action. 1490 */ 1491 MUST, 1492 /** 1493 * An action with this behavior may be included in the set of actions processed 1494 * by the end user. 1495 */ 1496 COULD, 1497 /** 1498 * An action with this behavior must be included in the set of actions processed 1499 * by the end user, unless the end user provides documentation as to why the 1500 * action was not included. 1501 */ 1502 MUSTUNLESSDOCUMENTED, 1503 /** 1504 * added to help the parsers with the generic types 1505 */ 1506 NULL; 1507 1508 public static ActionRequiredBehavior fromCode(String codeString) throws FHIRException { 1509 if (codeString == null || "".equals(codeString)) 1510 return null; 1511 if ("must".equals(codeString)) 1512 return MUST; 1513 if ("could".equals(codeString)) 1514 return COULD; 1515 if ("must-unless-documented".equals(codeString)) 1516 return MUSTUNLESSDOCUMENTED; 1517 if (Configuration.isAcceptInvalidEnums()) 1518 return null; 1519 else 1520 throw new FHIRException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1521 } 1522 1523 public String toCode() { 1524 switch (this) { 1525 case MUST: 1526 return "must"; 1527 case COULD: 1528 return "could"; 1529 case MUSTUNLESSDOCUMENTED: 1530 return "must-unless-documented"; 1531 case NULL: 1532 return null; 1533 default: 1534 return "?"; 1535 } 1536 } 1537 1538 public String getSystem() { 1539 switch (this) { 1540 case MUST: 1541 return "http://hl7.org/fhir/action-required-behavior"; 1542 case COULD: 1543 return "http://hl7.org/fhir/action-required-behavior"; 1544 case MUSTUNLESSDOCUMENTED: 1545 return "http://hl7.org/fhir/action-required-behavior"; 1546 case NULL: 1547 return null; 1548 default: 1549 return "?"; 1550 } 1551 } 1552 1553 public String getDefinition() { 1554 switch (this) { 1555 case MUST: 1556 return "An action with this behavior must be included in the actions processed by the end user; the end user SHALL NOT choose not to include this action."; 1557 case COULD: 1558 return "An action with this behavior may be included in the set of actions processed by the end user."; 1559 case MUSTUNLESSDOCUMENTED: 1560 return "An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included."; 1561 case NULL: 1562 return null; 1563 default: 1564 return "?"; 1565 } 1566 } 1567 1568 public String getDisplay() { 1569 switch (this) { 1570 case MUST: 1571 return "Must"; 1572 case COULD: 1573 return "Could"; 1574 case MUSTUNLESSDOCUMENTED: 1575 return "Must Unless Documented"; 1576 case NULL: 1577 return null; 1578 default: 1579 return "?"; 1580 } 1581 } 1582 } 1583 1584 public static class ActionRequiredBehaviorEnumFactory implements EnumFactory<ActionRequiredBehavior> { 1585 public ActionRequiredBehavior fromCode(String codeString) throws IllegalArgumentException { 1586 if (codeString == null || "".equals(codeString)) 1587 if (codeString == null || "".equals(codeString)) 1588 return null; 1589 if ("must".equals(codeString)) 1590 return ActionRequiredBehavior.MUST; 1591 if ("could".equals(codeString)) 1592 return ActionRequiredBehavior.COULD; 1593 if ("must-unless-documented".equals(codeString)) 1594 return ActionRequiredBehavior.MUSTUNLESSDOCUMENTED; 1595 throw new IllegalArgumentException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1596 } 1597 1598 public Enumeration<ActionRequiredBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1599 if (code == null) 1600 return null; 1601 if (code.isEmpty()) 1602 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.NULL, code); 1603 String codeString = code.asStringValue(); 1604 if (codeString == null || "".equals(codeString)) 1605 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.NULL, code); 1606 if ("must".equals(codeString)) 1607 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUST, code); 1608 if ("could".equals(codeString)) 1609 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.COULD, code); 1610 if ("must-unless-documented".equals(codeString)) 1611 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUSTUNLESSDOCUMENTED, code); 1612 throw new FHIRException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1613 } 1614 1615 public String toCode(ActionRequiredBehavior code) { 1616 if (code == ActionRequiredBehavior.NULL) 1617 return null; 1618 if (code == ActionRequiredBehavior.MUST) 1619 return "must"; 1620 if (code == ActionRequiredBehavior.COULD) 1621 return "could"; 1622 if (code == ActionRequiredBehavior.MUSTUNLESSDOCUMENTED) 1623 return "must-unless-documented"; 1624 return "?"; 1625 } 1626 1627 public String toSystem(ActionRequiredBehavior code) { 1628 return code.getSystem(); 1629 } 1630 } 1631 1632 public enum ActionPrecheckBehavior { 1633 /** 1634 * An action with this behavior is one of the most frequent action that is, or 1635 * should be, included by an end user, for the particular context in which the 1636 * action occurs. The system displaying the action to the end user should 1637 * consider "pre-checking" such an action as a convenience for the user. 1638 */ 1639 YES, 1640 /** 1641 * An action with this behavior is one of the less frequent actions included by 1642 * the end user, for the particular context in which the action occurs. The 1643 * system displaying the actions to the end user would typically not "pre-check" 1644 * such an action. 1645 */ 1646 NO, 1647 /** 1648 * added to help the parsers with the generic types 1649 */ 1650 NULL; 1651 1652 public static ActionPrecheckBehavior fromCode(String codeString) throws FHIRException { 1653 if (codeString == null || "".equals(codeString)) 1654 return null; 1655 if ("yes".equals(codeString)) 1656 return YES; 1657 if ("no".equals(codeString)) 1658 return NO; 1659 if (Configuration.isAcceptInvalidEnums()) 1660 return null; 1661 else 1662 throw new FHIRException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1663 } 1664 1665 public String toCode() { 1666 switch (this) { 1667 case YES: 1668 return "yes"; 1669 case NO: 1670 return "no"; 1671 case NULL: 1672 return null; 1673 default: 1674 return "?"; 1675 } 1676 } 1677 1678 public String getSystem() { 1679 switch (this) { 1680 case YES: 1681 return "http://hl7.org/fhir/action-precheck-behavior"; 1682 case NO: 1683 return "http://hl7.org/fhir/action-precheck-behavior"; 1684 case NULL: 1685 return null; 1686 default: 1687 return "?"; 1688 } 1689 } 1690 1691 public String getDefinition() { 1692 switch (this) { 1693 case YES: 1694 return "An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider \"pre-checking\" such an action as a convenience for the user."; 1695 case NO: 1696 return "An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not \"pre-check\" such an action."; 1697 case NULL: 1698 return null; 1699 default: 1700 return "?"; 1701 } 1702 } 1703 1704 public String getDisplay() { 1705 switch (this) { 1706 case YES: 1707 return "Yes"; 1708 case NO: 1709 return "No"; 1710 case NULL: 1711 return null; 1712 default: 1713 return "?"; 1714 } 1715 } 1716 } 1717 1718 public static class ActionPrecheckBehaviorEnumFactory implements EnumFactory<ActionPrecheckBehavior> { 1719 public ActionPrecheckBehavior fromCode(String codeString) throws IllegalArgumentException { 1720 if (codeString == null || "".equals(codeString)) 1721 if (codeString == null || "".equals(codeString)) 1722 return null; 1723 if ("yes".equals(codeString)) 1724 return ActionPrecheckBehavior.YES; 1725 if ("no".equals(codeString)) 1726 return ActionPrecheckBehavior.NO; 1727 throw new IllegalArgumentException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1728 } 1729 1730 public Enumeration<ActionPrecheckBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1731 if (code == null) 1732 return null; 1733 if (code.isEmpty()) 1734 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NULL, code); 1735 String codeString = code.asStringValue(); 1736 if (codeString == null || "".equals(codeString)) 1737 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NULL, code); 1738 if ("yes".equals(codeString)) 1739 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.YES, code); 1740 if ("no".equals(codeString)) 1741 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NO, code); 1742 throw new FHIRException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1743 } 1744 1745 public String toCode(ActionPrecheckBehavior code) { 1746 if (code == ActionPrecheckBehavior.NULL) 1747 return null; 1748 if (code == ActionPrecheckBehavior.YES) 1749 return "yes"; 1750 if (code == ActionPrecheckBehavior.NO) 1751 return "no"; 1752 return "?"; 1753 } 1754 1755 public String toSystem(ActionPrecheckBehavior code) { 1756 return code.getSystem(); 1757 } 1758 } 1759 1760 public enum ActionCardinalityBehavior { 1761 /** 1762 * The action may only be selected one time. 1763 */ 1764 SINGLE, 1765 /** 1766 * The action may be selected multiple times. 1767 */ 1768 MULTIPLE, 1769 /** 1770 * added to help the parsers with the generic types 1771 */ 1772 NULL; 1773 1774 public static ActionCardinalityBehavior fromCode(String codeString) throws FHIRException { 1775 if (codeString == null || "".equals(codeString)) 1776 return null; 1777 if ("single".equals(codeString)) 1778 return SINGLE; 1779 if ("multiple".equals(codeString)) 1780 return MULTIPLE; 1781 if (Configuration.isAcceptInvalidEnums()) 1782 return null; 1783 else 1784 throw new FHIRException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1785 } 1786 1787 public String toCode() { 1788 switch (this) { 1789 case SINGLE: 1790 return "single"; 1791 case MULTIPLE: 1792 return "multiple"; 1793 case NULL: 1794 return null; 1795 default: 1796 return "?"; 1797 } 1798 } 1799 1800 public String getSystem() { 1801 switch (this) { 1802 case SINGLE: 1803 return "http://hl7.org/fhir/action-cardinality-behavior"; 1804 case MULTIPLE: 1805 return "http://hl7.org/fhir/action-cardinality-behavior"; 1806 case NULL: 1807 return null; 1808 default: 1809 return "?"; 1810 } 1811 } 1812 1813 public String getDefinition() { 1814 switch (this) { 1815 case SINGLE: 1816 return "The action may only be selected one time."; 1817 case MULTIPLE: 1818 return "The action may be selected multiple times."; 1819 case NULL: 1820 return null; 1821 default: 1822 return "?"; 1823 } 1824 } 1825 1826 public String getDisplay() { 1827 switch (this) { 1828 case SINGLE: 1829 return "Single"; 1830 case MULTIPLE: 1831 return "Multiple"; 1832 case NULL: 1833 return null; 1834 default: 1835 return "?"; 1836 } 1837 } 1838 } 1839 1840 public static class ActionCardinalityBehaviorEnumFactory implements EnumFactory<ActionCardinalityBehavior> { 1841 public ActionCardinalityBehavior fromCode(String codeString) throws IllegalArgumentException { 1842 if (codeString == null || "".equals(codeString)) 1843 if (codeString == null || "".equals(codeString)) 1844 return null; 1845 if ("single".equals(codeString)) 1846 return ActionCardinalityBehavior.SINGLE; 1847 if ("multiple".equals(codeString)) 1848 return ActionCardinalityBehavior.MULTIPLE; 1849 throw new IllegalArgumentException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1850 } 1851 1852 public Enumeration<ActionCardinalityBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1853 if (code == null) 1854 return null; 1855 if (code.isEmpty()) 1856 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.NULL, code); 1857 String codeString = code.asStringValue(); 1858 if (codeString == null || "".equals(codeString)) 1859 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.NULL, code); 1860 if ("single".equals(codeString)) 1861 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.SINGLE, code); 1862 if ("multiple".equals(codeString)) 1863 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.MULTIPLE, code); 1864 throw new FHIRException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1865 } 1866 1867 public String toCode(ActionCardinalityBehavior code) { 1868 if (code == ActionCardinalityBehavior.NULL) 1869 return null; 1870 if (code == ActionCardinalityBehavior.SINGLE) 1871 return "single"; 1872 if (code == ActionCardinalityBehavior.MULTIPLE) 1873 return "multiple"; 1874 return "?"; 1875 } 1876 1877 public String toSystem(ActionCardinalityBehavior code) { 1878 return code.getSystem(); 1879 } 1880 } 1881 1882 @Block() 1883 public static class RequestGroupActionComponent extends BackboneElement implements IBaseBackboneElement { 1884 /** 1885 * A user-visible prefix for the action. 1886 */ 1887 @Child(name = "prefix", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1888 @Description(shortDefinition = "User-visible prefix for the action (e.g. 1. or A.)", formalDefinition = "A user-visible prefix for the action.") 1889 protected StringType prefix; 1890 1891 /** 1892 * The title of the action displayed to a user. 1893 */ 1894 @Child(name = "title", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1895 @Description(shortDefinition = "User-visible title", formalDefinition = "The title of the action displayed to a user.") 1896 protected StringType title; 1897 1898 /** 1899 * A short description of the action used to provide a summary to display to the 1900 * user. 1901 */ 1902 @Child(name = "description", type = { 1903 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1904 @Description(shortDefinition = "Short description of the action", formalDefinition = "A short description of the action used to provide a summary to display to the user.") 1905 protected StringType description; 1906 1907 /** 1908 * A text equivalent of the action to be performed. This provides a 1909 * human-interpretable description of the action when the definition is consumed 1910 * by a system that might not be capable of interpreting it dynamically. 1911 */ 1912 @Child(name = "textEquivalent", type = { 1913 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1914 @Description(shortDefinition = "Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition = "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.") 1915 protected StringType textEquivalent; 1916 1917 /** 1918 * Indicates how quickly the action should be addressed with respect to other 1919 * actions. 1920 */ 1921 @Child(name = "priority", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1922 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the action should be addressed with respect to other actions.") 1923 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 1924 protected Enumeration<RequestPriority> priority; 1925 1926 /** 1927 * A code that provides meaning for the action or action group. For example, a 1928 * section may have a LOINC code for a section of a documentation template. 1929 */ 1930 @Child(name = "code", type = { 1931 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1932 @Description(shortDefinition = "Code representing the meaning of the action or sub-actions", formalDefinition = "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.") 1933 protected List<CodeableConcept> code; 1934 1935 /** 1936 * Didactic or other informational resources associated with the action that can 1937 * be provided to the CDS recipient. Information resources can include inline 1938 * text commentary and links to web resources. 1939 */ 1940 @Child(name = "documentation", type = { 1941 RelatedArtifact.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1942 @Description(shortDefinition = "Supporting documentation for the intended performer of the action", formalDefinition = "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.") 1943 protected List<RelatedArtifact> documentation; 1944 1945 /** 1946 * An expression that describes applicability criteria, or start/stop conditions 1947 * for the action. 1948 */ 1949 @Child(name = "condition", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1950 @Description(shortDefinition = "Whether or not the action is applicable", formalDefinition = "An expression that describes applicability criteria, or start/stop conditions for the action.") 1951 protected List<RequestGroupActionConditionComponent> condition; 1952 1953 /** 1954 * A relationship to another action such as "before" or "30-60 minutes after 1955 * start of". 1956 */ 1957 @Child(name = "relatedAction", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1958 @Description(shortDefinition = "Relationship to another action", formalDefinition = "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".") 1959 protected List<RequestGroupActionRelatedActionComponent> relatedAction; 1960 1961 /** 1962 * An optional value describing when the action should be performed. 1963 */ 1964 @Child(name = "timing", type = { DateTimeType.class, Age.class, Period.class, Duration.class, Range.class, 1965 Timing.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1966 @Description(shortDefinition = "When the action should take place", formalDefinition = "An optional value describing when the action should be performed.") 1967 protected Type timing; 1968 1969 /** 1970 * The participant that should perform or be responsible for this action. 1971 */ 1972 @Child(name = "participant", type = { Patient.class, Practitioner.class, PractitionerRole.class, 1973 RelatedPerson.class, 1974 Device.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1975 @Description(shortDefinition = "Who should perform the action", formalDefinition = "The participant that should perform or be responsible for this action.") 1976 protected List<Reference> participant; 1977 /** 1978 * The actual objects that are the target of the reference (The participant that 1979 * should perform or be responsible for this action.) 1980 */ 1981 protected List<Resource> participantTarget; 1982 1983 /** 1984 * The type of action to perform (create, update, remove). 1985 */ 1986 @Child(name = "type", type = { 1987 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1988 @Description(shortDefinition = "create | update | remove | fire-event", formalDefinition = "The type of action to perform (create, update, remove).") 1989 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-type") 1990 protected CodeableConcept type; 1991 1992 /** 1993 * Defines the grouping behavior for the action and its children. 1994 */ 1995 @Child(name = "groupingBehavior", type = { 1996 CodeType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1997 @Description(shortDefinition = "visual-group | logical-group | sentence-group", formalDefinition = "Defines the grouping behavior for the action and its children.") 1998 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-grouping-behavior") 1999 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 2000 2001 /** 2002 * Defines the selection behavior for the action and its children. 2003 */ 2004 @Child(name = "selectionBehavior", type = { 2005 CodeType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 2006 @Description(shortDefinition = "any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition = "Defines the selection behavior for the action and its children.") 2007 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-selection-behavior") 2008 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 2009 2010 /** 2011 * Defines expectations around whether an action is required. 2012 */ 2013 @Child(name = "requiredBehavior", type = { 2014 CodeType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 2015 @Description(shortDefinition = "must | could | must-unless-documented", formalDefinition = "Defines expectations around whether an action is required.") 2016 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-required-behavior") 2017 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 2018 2019 /** 2020 * Defines whether the action should usually be preselected. 2021 */ 2022 @Child(name = "precheckBehavior", type = { 2023 CodeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 2024 @Description(shortDefinition = "yes | no", formalDefinition = "Defines whether the action should usually be preselected.") 2025 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-precheck-behavior") 2026 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 2027 2028 /** 2029 * Defines whether the action can be selected multiple times. 2030 */ 2031 @Child(name = "cardinalityBehavior", type = { 2032 CodeType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 2033 @Description(shortDefinition = "single | multiple", formalDefinition = "Defines whether the action can be selected multiple times.") 2034 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 2035 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 2036 2037 /** 2038 * The resource that is the target of the action (e.g. CommunicationRequest). 2039 */ 2040 @Child(name = "resource", type = { 2041 Reference.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 2042 @Description(shortDefinition = "The target of the action", formalDefinition = "The resource that is the target of the action (e.g. CommunicationRequest).") 2043 protected Reference resource; 2044 2045 /** 2046 * The actual object that is the target of the reference (The resource that is 2047 * the target of the action (e.g. CommunicationRequest).) 2048 */ 2049 protected Resource resourceTarget; 2050 2051 /** 2052 * Sub actions. 2053 */ 2054 @Child(name = "action", type = { 2055 RequestGroupActionComponent.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2056 @Description(shortDefinition = "Sub action", formalDefinition = "Sub actions.") 2057 protected List<RequestGroupActionComponent> action; 2058 2059 private static final long serialVersionUID = 296752321L; 2060 2061 /** 2062 * Constructor 2063 */ 2064 public RequestGroupActionComponent() { 2065 super(); 2066 } 2067 2068 /** 2069 * @return {@link #prefix} (A user-visible prefix for the action.). This is the 2070 * underlying object with id, value and extensions. The accessor 2071 * "getPrefix" gives direct access to the value 2072 */ 2073 public StringType getPrefixElement() { 2074 if (this.prefix == null) 2075 if (Configuration.errorOnAutoCreate()) 2076 throw new Error("Attempt to auto-create RequestGroupActionComponent.prefix"); 2077 else if (Configuration.doAutoCreate()) 2078 this.prefix = new StringType(); // bb 2079 return this.prefix; 2080 } 2081 2082 public boolean hasPrefixElement() { 2083 return this.prefix != null && !this.prefix.isEmpty(); 2084 } 2085 2086 public boolean hasPrefix() { 2087 return this.prefix != null && !this.prefix.isEmpty(); 2088 } 2089 2090 /** 2091 * @param value {@link #prefix} (A user-visible prefix for the action.). This is 2092 * the underlying object with id, value and extensions. The 2093 * accessor "getPrefix" gives direct access to the value 2094 */ 2095 public RequestGroupActionComponent setPrefixElement(StringType value) { 2096 this.prefix = value; 2097 return this; 2098 } 2099 2100 /** 2101 * @return A user-visible prefix for the action. 2102 */ 2103 public String getPrefix() { 2104 return this.prefix == null ? null : this.prefix.getValue(); 2105 } 2106 2107 /** 2108 * @param value A user-visible prefix for the action. 2109 */ 2110 public RequestGroupActionComponent setPrefix(String value) { 2111 if (Utilities.noString(value)) 2112 this.prefix = null; 2113 else { 2114 if (this.prefix == null) 2115 this.prefix = new StringType(); 2116 this.prefix.setValue(value); 2117 } 2118 return this; 2119 } 2120 2121 /** 2122 * @return {@link #title} (The title of the action displayed to a user.). This 2123 * is the underlying object with id, value and extensions. The accessor 2124 * "getTitle" gives direct access to the value 2125 */ 2126 public StringType getTitleElement() { 2127 if (this.title == null) 2128 if (Configuration.errorOnAutoCreate()) 2129 throw new Error("Attempt to auto-create RequestGroupActionComponent.title"); 2130 else if (Configuration.doAutoCreate()) 2131 this.title = new StringType(); // bb 2132 return this.title; 2133 } 2134 2135 public boolean hasTitleElement() { 2136 return this.title != null && !this.title.isEmpty(); 2137 } 2138 2139 public boolean hasTitle() { 2140 return this.title != null && !this.title.isEmpty(); 2141 } 2142 2143 /** 2144 * @param value {@link #title} (The title of the action displayed to a user.). 2145 * This is the underlying object with id, value and extensions. The 2146 * accessor "getTitle" gives direct access to the value 2147 */ 2148 public RequestGroupActionComponent setTitleElement(StringType value) { 2149 this.title = value; 2150 return this; 2151 } 2152 2153 /** 2154 * @return The title of the action displayed to a user. 2155 */ 2156 public String getTitle() { 2157 return this.title == null ? null : this.title.getValue(); 2158 } 2159 2160 /** 2161 * @param value The title of the action displayed to a user. 2162 */ 2163 public RequestGroupActionComponent setTitle(String value) { 2164 if (Utilities.noString(value)) 2165 this.title = null; 2166 else { 2167 if (this.title == null) 2168 this.title = new StringType(); 2169 this.title.setValue(value); 2170 } 2171 return this; 2172 } 2173 2174 /** 2175 * @return {@link #description} (A short description of the action used to 2176 * provide a summary to display to the user.). This is the underlying 2177 * object with id, value and extensions. The accessor "getDescription" 2178 * gives direct access to the value 2179 */ 2180 public StringType getDescriptionElement() { 2181 if (this.description == null) 2182 if (Configuration.errorOnAutoCreate()) 2183 throw new Error("Attempt to auto-create RequestGroupActionComponent.description"); 2184 else if (Configuration.doAutoCreate()) 2185 this.description = new StringType(); // bb 2186 return this.description; 2187 } 2188 2189 public boolean hasDescriptionElement() { 2190 return this.description != null && !this.description.isEmpty(); 2191 } 2192 2193 public boolean hasDescription() { 2194 return this.description != null && !this.description.isEmpty(); 2195 } 2196 2197 /** 2198 * @param value {@link #description} (A short description of the action used to 2199 * provide a summary to display to the user.). This is the 2200 * underlying object with id, value and extensions. The accessor 2201 * "getDescription" gives direct access to the value 2202 */ 2203 public RequestGroupActionComponent setDescriptionElement(StringType value) { 2204 this.description = value; 2205 return this; 2206 } 2207 2208 /** 2209 * @return A short description of the action used to provide a summary to 2210 * display to the user. 2211 */ 2212 public String getDescription() { 2213 return this.description == null ? null : this.description.getValue(); 2214 } 2215 2216 /** 2217 * @param value A short description of the action used to provide a summary to 2218 * display to the user. 2219 */ 2220 public RequestGroupActionComponent setDescription(String value) { 2221 if (Utilities.noString(value)) 2222 this.description = null; 2223 else { 2224 if (this.description == null) 2225 this.description = new StringType(); 2226 this.description.setValue(value); 2227 } 2228 return this; 2229 } 2230 2231 /** 2232 * @return {@link #textEquivalent} (A text equivalent of the action to be 2233 * performed. This provides a human-interpretable description of the 2234 * action when the definition is consumed by a system that might not be 2235 * capable of interpreting it dynamically.). This is the underlying 2236 * object with id, value and extensions. The accessor 2237 * "getTextEquivalent" gives direct access to the value 2238 */ 2239 public StringType getTextEquivalentElement() { 2240 if (this.textEquivalent == null) 2241 if (Configuration.errorOnAutoCreate()) 2242 throw new Error("Attempt to auto-create RequestGroupActionComponent.textEquivalent"); 2243 else if (Configuration.doAutoCreate()) 2244 this.textEquivalent = new StringType(); // bb 2245 return this.textEquivalent; 2246 } 2247 2248 public boolean hasTextEquivalentElement() { 2249 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2250 } 2251 2252 public boolean hasTextEquivalent() { 2253 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2254 } 2255 2256 /** 2257 * @param value {@link #textEquivalent} (A text equivalent of the action to be 2258 * performed. This provides a human-interpretable description of 2259 * the action when the definition is consumed by a system that 2260 * might not be capable of interpreting it dynamically.). This is 2261 * the underlying object with id, value and extensions. The 2262 * accessor "getTextEquivalent" gives direct access to the value 2263 */ 2264 public RequestGroupActionComponent setTextEquivalentElement(StringType value) { 2265 this.textEquivalent = value; 2266 return this; 2267 } 2268 2269 /** 2270 * @return A text equivalent of the action to be performed. This provides a 2271 * human-interpretable description of the action when the definition is 2272 * consumed by a system that might not be capable of interpreting it 2273 * dynamically. 2274 */ 2275 public String getTextEquivalent() { 2276 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 2277 } 2278 2279 /** 2280 * @param value A text equivalent of the action to be performed. This provides a 2281 * human-interpretable description of the action when the 2282 * definition is consumed by a system that might not be capable of 2283 * interpreting it dynamically. 2284 */ 2285 public RequestGroupActionComponent setTextEquivalent(String value) { 2286 if (Utilities.noString(value)) 2287 this.textEquivalent = null; 2288 else { 2289 if (this.textEquivalent == null) 2290 this.textEquivalent = new StringType(); 2291 this.textEquivalent.setValue(value); 2292 } 2293 return this; 2294 } 2295 2296 /** 2297 * @return {@link #priority} (Indicates how quickly the action should be 2298 * addressed with respect to other actions.). This is the underlying 2299 * object with id, value and extensions. The accessor "getPriority" 2300 * gives direct access to the value 2301 */ 2302 public Enumeration<RequestPriority> getPriorityElement() { 2303 if (this.priority == null) 2304 if (Configuration.errorOnAutoCreate()) 2305 throw new Error("Attempt to auto-create RequestGroupActionComponent.priority"); 2306 else if (Configuration.doAutoCreate()) 2307 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 2308 return this.priority; 2309 } 2310 2311 public boolean hasPriorityElement() { 2312 return this.priority != null && !this.priority.isEmpty(); 2313 } 2314 2315 public boolean hasPriority() { 2316 return this.priority != null && !this.priority.isEmpty(); 2317 } 2318 2319 /** 2320 * @param value {@link #priority} (Indicates how quickly the action should be 2321 * addressed with respect to other actions.). This is the 2322 * underlying object with id, value and extensions. The accessor 2323 * "getPriority" gives direct access to the value 2324 */ 2325 public RequestGroupActionComponent setPriorityElement(Enumeration<RequestPriority> value) { 2326 this.priority = value; 2327 return this; 2328 } 2329 2330 /** 2331 * @return Indicates how quickly the action should be addressed with respect to 2332 * other actions. 2333 */ 2334 public RequestPriority getPriority() { 2335 return this.priority == null ? null : this.priority.getValue(); 2336 } 2337 2338 /** 2339 * @param value Indicates how quickly the action should be addressed with 2340 * respect to other actions. 2341 */ 2342 public RequestGroupActionComponent setPriority(RequestPriority value) { 2343 if (value == null) 2344 this.priority = null; 2345 else { 2346 if (this.priority == null) 2347 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 2348 this.priority.setValue(value); 2349 } 2350 return this; 2351 } 2352 2353 /** 2354 * @return {@link #code} (A code that provides meaning for the action or action 2355 * group. For example, a section may have a LOINC code for a section of 2356 * a documentation template.) 2357 */ 2358 public List<CodeableConcept> getCode() { 2359 if (this.code == null) 2360 this.code = new ArrayList<CodeableConcept>(); 2361 return this.code; 2362 } 2363 2364 /** 2365 * @return Returns a reference to <code>this</code> for easy method chaining 2366 */ 2367 public RequestGroupActionComponent setCode(List<CodeableConcept> theCode) { 2368 this.code = theCode; 2369 return this; 2370 } 2371 2372 public boolean hasCode() { 2373 if (this.code == null) 2374 return false; 2375 for (CodeableConcept item : this.code) 2376 if (!item.isEmpty()) 2377 return true; 2378 return false; 2379 } 2380 2381 public CodeableConcept addCode() { // 3 2382 CodeableConcept t = new CodeableConcept(); 2383 if (this.code == null) 2384 this.code = new ArrayList<CodeableConcept>(); 2385 this.code.add(t); 2386 return t; 2387 } 2388 2389 public RequestGroupActionComponent addCode(CodeableConcept t) { // 3 2390 if (t == null) 2391 return this; 2392 if (this.code == null) 2393 this.code = new ArrayList<CodeableConcept>(); 2394 this.code.add(t); 2395 return this; 2396 } 2397 2398 /** 2399 * @return The first repetition of repeating field {@link #code}, creating it if 2400 * it does not already exist 2401 */ 2402 public CodeableConcept getCodeFirstRep() { 2403 if (getCode().isEmpty()) { 2404 addCode(); 2405 } 2406 return getCode().get(0); 2407 } 2408 2409 /** 2410 * @return {@link #documentation} (Didactic or other informational resources 2411 * associated with the action that can be provided to the CDS recipient. 2412 * Information resources can include inline text commentary and links to 2413 * web resources.) 2414 */ 2415 public List<RelatedArtifact> getDocumentation() { 2416 if (this.documentation == null) 2417 this.documentation = new ArrayList<RelatedArtifact>(); 2418 return this.documentation; 2419 } 2420 2421 /** 2422 * @return Returns a reference to <code>this</code> for easy method chaining 2423 */ 2424 public RequestGroupActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 2425 this.documentation = theDocumentation; 2426 return this; 2427 } 2428 2429 public boolean hasDocumentation() { 2430 if (this.documentation == null) 2431 return false; 2432 for (RelatedArtifact item : this.documentation) 2433 if (!item.isEmpty()) 2434 return true; 2435 return false; 2436 } 2437 2438 public RelatedArtifact addDocumentation() { // 3 2439 RelatedArtifact t = new RelatedArtifact(); 2440 if (this.documentation == null) 2441 this.documentation = new ArrayList<RelatedArtifact>(); 2442 this.documentation.add(t); 2443 return t; 2444 } 2445 2446 public RequestGroupActionComponent addDocumentation(RelatedArtifact t) { // 3 2447 if (t == null) 2448 return this; 2449 if (this.documentation == null) 2450 this.documentation = new ArrayList<RelatedArtifact>(); 2451 this.documentation.add(t); 2452 return this; 2453 } 2454 2455 /** 2456 * @return The first repetition of repeating field {@link #documentation}, 2457 * creating it if it does not already exist 2458 */ 2459 public RelatedArtifact getDocumentationFirstRep() { 2460 if (getDocumentation().isEmpty()) { 2461 addDocumentation(); 2462 } 2463 return getDocumentation().get(0); 2464 } 2465 2466 /** 2467 * @return {@link #condition} (An expression that describes applicability 2468 * criteria, or start/stop conditions for the action.) 2469 */ 2470 public List<RequestGroupActionConditionComponent> getCondition() { 2471 if (this.condition == null) 2472 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 2473 return this.condition; 2474 } 2475 2476 /** 2477 * @return Returns a reference to <code>this</code> for easy method chaining 2478 */ 2479 public RequestGroupActionComponent setCondition(List<RequestGroupActionConditionComponent> theCondition) { 2480 this.condition = theCondition; 2481 return this; 2482 } 2483 2484 public boolean hasCondition() { 2485 if (this.condition == null) 2486 return false; 2487 for (RequestGroupActionConditionComponent item : this.condition) 2488 if (!item.isEmpty()) 2489 return true; 2490 return false; 2491 } 2492 2493 public RequestGroupActionConditionComponent addCondition() { // 3 2494 RequestGroupActionConditionComponent t = new RequestGroupActionConditionComponent(); 2495 if (this.condition == null) 2496 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 2497 this.condition.add(t); 2498 return t; 2499 } 2500 2501 public RequestGroupActionComponent addCondition(RequestGroupActionConditionComponent t) { // 3 2502 if (t == null) 2503 return this; 2504 if (this.condition == null) 2505 this.condition = new ArrayList<RequestGroupActionConditionComponent>(); 2506 this.condition.add(t); 2507 return this; 2508 } 2509 2510 /** 2511 * @return The first repetition of repeating field {@link #condition}, creating 2512 * it if it does not already exist 2513 */ 2514 public RequestGroupActionConditionComponent getConditionFirstRep() { 2515 if (getCondition().isEmpty()) { 2516 addCondition(); 2517 } 2518 return getCondition().get(0); 2519 } 2520 2521 /** 2522 * @return {@link #relatedAction} (A relationship to another action such as 2523 * "before" or "30-60 minutes after start of".) 2524 */ 2525 public List<RequestGroupActionRelatedActionComponent> getRelatedAction() { 2526 if (this.relatedAction == null) 2527 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2528 return this.relatedAction; 2529 } 2530 2531 /** 2532 * @return Returns a reference to <code>this</code> for easy method chaining 2533 */ 2534 public RequestGroupActionComponent setRelatedAction( 2535 List<RequestGroupActionRelatedActionComponent> theRelatedAction) { 2536 this.relatedAction = theRelatedAction; 2537 return this; 2538 } 2539 2540 public boolean hasRelatedAction() { 2541 if (this.relatedAction == null) 2542 return false; 2543 for (RequestGroupActionRelatedActionComponent item : this.relatedAction) 2544 if (!item.isEmpty()) 2545 return true; 2546 return false; 2547 } 2548 2549 public RequestGroupActionRelatedActionComponent addRelatedAction() { // 3 2550 RequestGroupActionRelatedActionComponent t = new RequestGroupActionRelatedActionComponent(); 2551 if (this.relatedAction == null) 2552 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2553 this.relatedAction.add(t); 2554 return t; 2555 } 2556 2557 public RequestGroupActionComponent addRelatedAction(RequestGroupActionRelatedActionComponent t) { // 3 2558 if (t == null) 2559 return this; 2560 if (this.relatedAction == null) 2561 this.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 2562 this.relatedAction.add(t); 2563 return this; 2564 } 2565 2566 /** 2567 * @return The first repetition of repeating field {@link #relatedAction}, 2568 * creating it if it does not already exist 2569 */ 2570 public RequestGroupActionRelatedActionComponent getRelatedActionFirstRep() { 2571 if (getRelatedAction().isEmpty()) { 2572 addRelatedAction(); 2573 } 2574 return getRelatedAction().get(0); 2575 } 2576 2577 /** 2578 * @return {@link #timing} (An optional value describing when the action should 2579 * be performed.) 2580 */ 2581 public Type getTiming() { 2582 return this.timing; 2583 } 2584 2585 /** 2586 * @return {@link #timing} (An optional value describing when the action should 2587 * be performed.) 2588 */ 2589 public DateTimeType getTimingDateTimeType() throws FHIRException { 2590 if (this.timing == null) 2591 this.timing = new DateTimeType(); 2592 if (!(this.timing instanceof DateTimeType)) 2593 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2594 + this.timing.getClass().getName() + " was encountered"); 2595 return (DateTimeType) this.timing; 2596 } 2597 2598 public boolean hasTimingDateTimeType() { 2599 return this.timing instanceof DateTimeType; 2600 } 2601 2602 /** 2603 * @return {@link #timing} (An optional value describing when the action should 2604 * be performed.) 2605 */ 2606 public Age getTimingAge() throws FHIRException { 2607 if (this.timing == null) 2608 this.timing = new Age(); 2609 if (!(this.timing instanceof Age)) 2610 throw new FHIRException( 2611 "Type mismatch: the type Age was expected, but " + this.timing.getClass().getName() + " was encountered"); 2612 return (Age) this.timing; 2613 } 2614 2615 public boolean hasTimingAge() { 2616 return this.timing instanceof Age; 2617 } 2618 2619 /** 2620 * @return {@link #timing} (An optional value describing when the action should 2621 * be performed.) 2622 */ 2623 public Period getTimingPeriod() throws FHIRException { 2624 if (this.timing == null) 2625 this.timing = new Period(); 2626 if (!(this.timing instanceof Period)) 2627 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() 2628 + " was encountered"); 2629 return (Period) this.timing; 2630 } 2631 2632 public boolean hasTimingPeriod() { 2633 return this.timing instanceof Period; 2634 } 2635 2636 /** 2637 * @return {@link #timing} (An optional value describing when the action should 2638 * be performed.) 2639 */ 2640 public Duration getTimingDuration() throws FHIRException { 2641 if (this.timing == null) 2642 this.timing = new Duration(); 2643 if (!(this.timing instanceof Duration)) 2644 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.timing.getClass().getName() 2645 + " was encountered"); 2646 return (Duration) this.timing; 2647 } 2648 2649 public boolean hasTimingDuration() { 2650 return this.timing instanceof Duration; 2651 } 2652 2653 /** 2654 * @return {@link #timing} (An optional value describing when the action should 2655 * be performed.) 2656 */ 2657 public Range getTimingRange() throws FHIRException { 2658 if (this.timing == null) 2659 this.timing = new Range(); 2660 if (!(this.timing instanceof Range)) 2661 throw new FHIRException( 2662 "Type mismatch: the type Range was expected, but " + this.timing.getClass().getName() + " was encountered"); 2663 return (Range) this.timing; 2664 } 2665 2666 public boolean hasTimingRange() { 2667 return this.timing instanceof Range; 2668 } 2669 2670 /** 2671 * @return {@link #timing} (An optional value describing when the action should 2672 * be performed.) 2673 */ 2674 public Timing getTimingTiming() throws FHIRException { 2675 if (this.timing == null) 2676 this.timing = new Timing(); 2677 if (!(this.timing instanceof Timing)) 2678 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() 2679 + " was encountered"); 2680 return (Timing) this.timing; 2681 } 2682 2683 public boolean hasTimingTiming() { 2684 return this.timing instanceof Timing; 2685 } 2686 2687 public boolean hasTiming() { 2688 return this.timing != null && !this.timing.isEmpty(); 2689 } 2690 2691 /** 2692 * @param value {@link #timing} (An optional value describing when the action 2693 * should be performed.) 2694 */ 2695 public RequestGroupActionComponent setTiming(Type value) { 2696 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period 2697 || value instanceof Duration || value instanceof Range || value instanceof Timing)) 2698 throw new Error("Not the right type for RequestGroup.action.timing[x]: " + value.fhirType()); 2699 this.timing = value; 2700 return this; 2701 } 2702 2703 /** 2704 * @return {@link #participant} (The participant that should perform or be 2705 * responsible for this action.) 2706 */ 2707 public List<Reference> getParticipant() { 2708 if (this.participant == null) 2709 this.participant = new ArrayList<Reference>(); 2710 return this.participant; 2711 } 2712 2713 /** 2714 * @return Returns a reference to <code>this</code> for easy method chaining 2715 */ 2716 public RequestGroupActionComponent setParticipant(List<Reference> theParticipant) { 2717 this.participant = theParticipant; 2718 return this; 2719 } 2720 2721 public boolean hasParticipant() { 2722 if (this.participant == null) 2723 return false; 2724 for (Reference item : this.participant) 2725 if (!item.isEmpty()) 2726 return true; 2727 return false; 2728 } 2729 2730 public Reference addParticipant() { // 3 2731 Reference t = new Reference(); 2732 if (this.participant == null) 2733 this.participant = new ArrayList<Reference>(); 2734 this.participant.add(t); 2735 return t; 2736 } 2737 2738 public RequestGroupActionComponent addParticipant(Reference t) { // 3 2739 if (t == null) 2740 return this; 2741 if (this.participant == null) 2742 this.participant = new ArrayList<Reference>(); 2743 this.participant.add(t); 2744 return this; 2745 } 2746 2747 /** 2748 * @return The first repetition of repeating field {@link #participant}, 2749 * creating it if it does not already exist 2750 */ 2751 public Reference getParticipantFirstRep() { 2752 if (getParticipant().isEmpty()) { 2753 addParticipant(); 2754 } 2755 return getParticipant().get(0); 2756 } 2757 2758 /** 2759 * @return {@link #type} (The type of action to perform (create, update, 2760 * remove).) 2761 */ 2762 public CodeableConcept getType() { 2763 if (this.type == null) 2764 if (Configuration.errorOnAutoCreate()) 2765 throw new Error("Attempt to auto-create RequestGroupActionComponent.type"); 2766 else if (Configuration.doAutoCreate()) 2767 this.type = new CodeableConcept(); // cc 2768 return this.type; 2769 } 2770 2771 public boolean hasType() { 2772 return this.type != null && !this.type.isEmpty(); 2773 } 2774 2775 /** 2776 * @param value {@link #type} (The type of action to perform (create, update, 2777 * remove).) 2778 */ 2779 public RequestGroupActionComponent setType(CodeableConcept value) { 2780 this.type = value; 2781 return this; 2782 } 2783 2784 /** 2785 * @return {@link #groupingBehavior} (Defines the grouping behavior for the 2786 * action and its children.). This is the underlying object with id, 2787 * value and extensions. The accessor "getGroupingBehavior" gives direct 2788 * access to the value 2789 */ 2790 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 2791 if (this.groupingBehavior == null) 2792 if (Configuration.errorOnAutoCreate()) 2793 throw new Error("Attempt to auto-create RequestGroupActionComponent.groupingBehavior"); 2794 else if (Configuration.doAutoCreate()) 2795 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 2796 return this.groupingBehavior; 2797 } 2798 2799 public boolean hasGroupingBehaviorElement() { 2800 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2801 } 2802 2803 public boolean hasGroupingBehavior() { 2804 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 2805 } 2806 2807 /** 2808 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the 2809 * action and its children.). This is the underlying object with 2810 * id, value and extensions. The accessor "getGroupingBehavior" 2811 * gives direct access to the value 2812 */ 2813 public RequestGroupActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 2814 this.groupingBehavior = value; 2815 return this; 2816 } 2817 2818 /** 2819 * @return Defines the grouping behavior for the action and its children. 2820 */ 2821 public ActionGroupingBehavior getGroupingBehavior() { 2822 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 2823 } 2824 2825 /** 2826 * @param value Defines the grouping behavior for the action and its children. 2827 */ 2828 public RequestGroupActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 2829 if (value == null) 2830 this.groupingBehavior = null; 2831 else { 2832 if (this.groupingBehavior == null) 2833 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 2834 this.groupingBehavior.setValue(value); 2835 } 2836 return this; 2837 } 2838 2839 /** 2840 * @return {@link #selectionBehavior} (Defines the selection behavior for the 2841 * action and its children.). This is the underlying object with id, 2842 * value and extensions. The accessor "getSelectionBehavior" gives 2843 * direct access to the value 2844 */ 2845 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 2846 if (this.selectionBehavior == null) 2847 if (Configuration.errorOnAutoCreate()) 2848 throw new Error("Attempt to auto-create RequestGroupActionComponent.selectionBehavior"); 2849 else if (Configuration.doAutoCreate()) 2850 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 2851 return this.selectionBehavior; 2852 } 2853 2854 public boolean hasSelectionBehaviorElement() { 2855 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 2856 } 2857 2858 public boolean hasSelectionBehavior() { 2859 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 2860 } 2861 2862 /** 2863 * @param value {@link #selectionBehavior} (Defines the selection behavior for 2864 * the action and its children.). This is the underlying object 2865 * with id, value and extensions. The accessor 2866 * "getSelectionBehavior" gives direct access to the value 2867 */ 2868 public RequestGroupActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 2869 this.selectionBehavior = value; 2870 return this; 2871 } 2872 2873 /** 2874 * @return Defines the selection behavior for the action and its children. 2875 */ 2876 public ActionSelectionBehavior getSelectionBehavior() { 2877 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 2878 } 2879 2880 /** 2881 * @param value Defines the selection behavior for the action and its children. 2882 */ 2883 public RequestGroupActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 2884 if (value == null) 2885 this.selectionBehavior = null; 2886 else { 2887 if (this.selectionBehavior == null) 2888 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 2889 this.selectionBehavior.setValue(value); 2890 } 2891 return this; 2892 } 2893 2894 /** 2895 * @return {@link #requiredBehavior} (Defines expectations around whether an 2896 * action is required.). This is the underlying object with id, value 2897 * and extensions. The accessor "getRequiredBehavior" gives direct 2898 * access to the value 2899 */ 2900 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 2901 if (this.requiredBehavior == null) 2902 if (Configuration.errorOnAutoCreate()) 2903 throw new Error("Attempt to auto-create RequestGroupActionComponent.requiredBehavior"); 2904 else if (Configuration.doAutoCreate()) 2905 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 2906 return this.requiredBehavior; 2907 } 2908 2909 public boolean hasRequiredBehaviorElement() { 2910 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 2911 } 2912 2913 public boolean hasRequiredBehavior() { 2914 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 2915 } 2916 2917 /** 2918 * @param value {@link #requiredBehavior} (Defines expectations around whether 2919 * an action is required.). This is the underlying object with id, 2920 * value and extensions. The accessor "getRequiredBehavior" gives 2921 * direct access to the value 2922 */ 2923 public RequestGroupActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 2924 this.requiredBehavior = value; 2925 return this; 2926 } 2927 2928 /** 2929 * @return Defines expectations around whether an action is required. 2930 */ 2931 public ActionRequiredBehavior getRequiredBehavior() { 2932 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 2933 } 2934 2935 /** 2936 * @param value Defines expectations around whether an action is required. 2937 */ 2938 public RequestGroupActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 2939 if (value == null) 2940 this.requiredBehavior = null; 2941 else { 2942 if (this.requiredBehavior == null) 2943 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 2944 this.requiredBehavior.setValue(value); 2945 } 2946 return this; 2947 } 2948 2949 /** 2950 * @return {@link #precheckBehavior} (Defines whether the action should usually 2951 * be preselected.). This is the underlying object with id, value and 2952 * extensions. The accessor "getPrecheckBehavior" gives direct access to 2953 * the value 2954 */ 2955 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 2956 if (this.precheckBehavior == null) 2957 if (Configuration.errorOnAutoCreate()) 2958 throw new Error("Attempt to auto-create RequestGroupActionComponent.precheckBehavior"); 2959 else if (Configuration.doAutoCreate()) 2960 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 2961 return this.precheckBehavior; 2962 } 2963 2964 public boolean hasPrecheckBehaviorElement() { 2965 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 2966 } 2967 2968 public boolean hasPrecheckBehavior() { 2969 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 2970 } 2971 2972 /** 2973 * @param value {@link #precheckBehavior} (Defines whether the action should 2974 * usually be preselected.). This is the underlying object with id, 2975 * value and extensions. The accessor "getPrecheckBehavior" gives 2976 * direct access to the value 2977 */ 2978 public RequestGroupActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 2979 this.precheckBehavior = value; 2980 return this; 2981 } 2982 2983 /** 2984 * @return Defines whether the action should usually be preselected. 2985 */ 2986 public ActionPrecheckBehavior getPrecheckBehavior() { 2987 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 2988 } 2989 2990 /** 2991 * @param value Defines whether the action should usually be preselected. 2992 */ 2993 public RequestGroupActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 2994 if (value == null) 2995 this.precheckBehavior = null; 2996 else { 2997 if (this.precheckBehavior == null) 2998 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 2999 this.precheckBehavior.setValue(value); 3000 } 3001 return this; 3002 } 3003 3004 /** 3005 * @return {@link #cardinalityBehavior} (Defines whether the action can be 3006 * selected multiple times.). This is the underlying object with id, 3007 * value and extensions. The accessor "getCardinalityBehavior" gives 3008 * direct access to the value 3009 */ 3010 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 3011 if (this.cardinalityBehavior == null) 3012 if (Configuration.errorOnAutoCreate()) 3013 throw new Error("Attempt to auto-create RequestGroupActionComponent.cardinalityBehavior"); 3014 else if (Configuration.doAutoCreate()) 3015 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>( 3016 new ActionCardinalityBehaviorEnumFactory()); // bb 3017 return this.cardinalityBehavior; 3018 } 3019 3020 public boolean hasCardinalityBehaviorElement() { 3021 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 3022 } 3023 3024 public boolean hasCardinalityBehavior() { 3025 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 3026 } 3027 3028 /** 3029 * @param value {@link #cardinalityBehavior} (Defines whether the action can be 3030 * selected multiple times.). This is the underlying object with 3031 * id, value and extensions. The accessor "getCardinalityBehavior" 3032 * gives direct access to the value 3033 */ 3034 public RequestGroupActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 3035 this.cardinalityBehavior = value; 3036 return this; 3037 } 3038 3039 /** 3040 * @return Defines whether the action can be selected multiple times. 3041 */ 3042 public ActionCardinalityBehavior getCardinalityBehavior() { 3043 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 3044 } 3045 3046 /** 3047 * @param value Defines whether the action can be selected multiple times. 3048 */ 3049 public RequestGroupActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 3050 if (value == null) 3051 this.cardinalityBehavior = null; 3052 else { 3053 if (this.cardinalityBehavior == null) 3054 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>( 3055 new ActionCardinalityBehaviorEnumFactory()); 3056 this.cardinalityBehavior.setValue(value); 3057 } 3058 return this; 3059 } 3060 3061 /** 3062 * @return {@link #resource} (The resource that is the target of the action 3063 * (e.g. CommunicationRequest).) 3064 */ 3065 public Reference getResource() { 3066 if (this.resource == null) 3067 if (Configuration.errorOnAutoCreate()) 3068 throw new Error("Attempt to auto-create RequestGroupActionComponent.resource"); 3069 else if (Configuration.doAutoCreate()) 3070 this.resource = new Reference(); // cc 3071 return this.resource; 3072 } 3073 3074 public boolean hasResource() { 3075 return this.resource != null && !this.resource.isEmpty(); 3076 } 3077 3078 /** 3079 * @param value {@link #resource} (The resource that is the target of the action 3080 * (e.g. CommunicationRequest).) 3081 */ 3082 public RequestGroupActionComponent setResource(Reference value) { 3083 this.resource = value; 3084 return this; 3085 } 3086 3087 /** 3088 * @return {@link #resource} The actual object that is the target of the 3089 * reference. The reference library doesn't populate this, but you can 3090 * use it to hold the resource if you resolve it. (The resource that is 3091 * the target of the action (e.g. CommunicationRequest).) 3092 */ 3093 public Resource getResourceTarget() { 3094 return this.resourceTarget; 3095 } 3096 3097 /** 3098 * @param value {@link #resource} The actual object that is the target of the 3099 * reference. The reference library doesn't use these, but you can 3100 * use it to hold the resource if you resolve it. (The resource 3101 * that is the target of the action (e.g. CommunicationRequest).) 3102 */ 3103 public RequestGroupActionComponent setResourceTarget(Resource value) { 3104 this.resourceTarget = value; 3105 return this; 3106 } 3107 3108 /** 3109 * @return {@link #action} (Sub actions.) 3110 */ 3111 public List<RequestGroupActionComponent> getAction() { 3112 if (this.action == null) 3113 this.action = new ArrayList<RequestGroupActionComponent>(); 3114 return this.action; 3115 } 3116 3117 /** 3118 * @return Returns a reference to <code>this</code> for easy method chaining 3119 */ 3120 public RequestGroupActionComponent setAction(List<RequestGroupActionComponent> theAction) { 3121 this.action = theAction; 3122 return this; 3123 } 3124 3125 public boolean hasAction() { 3126 if (this.action == null) 3127 return false; 3128 for (RequestGroupActionComponent item : this.action) 3129 if (!item.isEmpty()) 3130 return true; 3131 return false; 3132 } 3133 3134 public RequestGroupActionComponent addAction() { // 3 3135 RequestGroupActionComponent t = new RequestGroupActionComponent(); 3136 if (this.action == null) 3137 this.action = new ArrayList<RequestGroupActionComponent>(); 3138 this.action.add(t); 3139 return t; 3140 } 3141 3142 public RequestGroupActionComponent addAction(RequestGroupActionComponent t) { // 3 3143 if (t == null) 3144 return this; 3145 if (this.action == null) 3146 this.action = new ArrayList<RequestGroupActionComponent>(); 3147 this.action.add(t); 3148 return this; 3149 } 3150 3151 /** 3152 * @return The first repetition of repeating field {@link #action}, creating it 3153 * if it does not already exist 3154 */ 3155 public RequestGroupActionComponent getActionFirstRep() { 3156 if (getAction().isEmpty()) { 3157 addAction(); 3158 } 3159 return getAction().get(0); 3160 } 3161 3162 protected void listChildren(List<Property> children) { 3163 super.listChildren(children); 3164 children.add(new Property("prefix", "string", "A user-visible prefix for the action.", 0, 1, prefix)); 3165 children.add(new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title)); 3166 children.add(new Property("description", "string", 3167 "A short description of the action used to provide a summary to display to the user.", 0, 1, description)); 3168 children.add(new Property("textEquivalent", "string", 3169 "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 3170 0, 1, textEquivalent)); 3171 children.add(new Property("priority", "code", 3172 "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority)); 3173 children.add(new Property("code", "CodeableConcept", 3174 "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.", 3175 0, java.lang.Integer.MAX_VALUE, code)); 3176 children.add(new Property("documentation", "RelatedArtifact", 3177 "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 3178 0, java.lang.Integer.MAX_VALUE, documentation)); 3179 children.add(new Property("condition", "", 3180 "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, 3181 java.lang.Integer.MAX_VALUE, condition)); 3182 children.add(new Property("relatedAction", "", 3183 "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, 3184 java.lang.Integer.MAX_VALUE, relatedAction)); 3185 children.add(new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3186 "An optional value describing when the action should be performed.", 0, 1, timing)); 3187 children.add(new Property("participant", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device)", 3188 "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, 3189 participant)); 3190 children.add(new Property("type", "CodeableConcept", "The type of action to perform (create, update, remove).", 0, 3191 1, type)); 3192 children.add(new Property("groupingBehavior", "code", 3193 "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 3194 children.add(new Property("selectionBehavior", "code", 3195 "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 3196 children.add(new Property("requiredBehavior", "code", 3197 "Defines expectations around whether an action is required.", 0, 1, requiredBehavior)); 3198 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 3199 0, 1, precheckBehavior)); 3200 children.add(new Property("cardinalityBehavior", "code", 3201 "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 3202 children.add(new Property("resource", "Reference(Any)", 3203 "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource)); 3204 children 3205 .add(new Property("action", "@RequestGroup.action", "Sub actions.", 0, java.lang.Integer.MAX_VALUE, action)); 3206 } 3207 3208 @Override 3209 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3210 switch (_hash) { 3211 case -980110702: 3212 /* prefix */ return new Property("prefix", "string", "A user-visible prefix for the action.", 0, 1, prefix); 3213 case 110371416: 3214 /* title */ return new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title); 3215 case -1724546052: 3216 /* description */ return new Property("description", "string", 3217 "A short description of the action used to provide a summary to display to the user.", 0, 1, description); 3218 case -900391049: 3219 /* textEquivalent */ return new Property("textEquivalent", "string", 3220 "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 3221 0, 1, textEquivalent); 3222 case -1165461084: 3223 /* priority */ return new Property("priority", "code", 3224 "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority); 3225 case 3059181: 3226 /* code */ return new Property("code", "CodeableConcept", 3227 "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for a section of a documentation template.", 3228 0, java.lang.Integer.MAX_VALUE, code); 3229 case 1587405498: 3230 /* documentation */ return new Property("documentation", "RelatedArtifact", 3231 "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 3232 0, java.lang.Integer.MAX_VALUE, documentation); 3233 case -861311717: 3234 /* condition */ return new Property("condition", "", 3235 "An expression that describes applicability criteria, or start/stop conditions for the action.", 0, 3236 java.lang.Integer.MAX_VALUE, condition); 3237 case -384107967: 3238 /* relatedAction */ return new Property("relatedAction", "", 3239 "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, 3240 java.lang.Integer.MAX_VALUE, relatedAction); 3241 case 164632566: 3242 /* timing[x] */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3243 "An optional value describing when the action should be performed.", 0, 1, timing); 3244 case -873664438: 3245 /* timing */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3246 "An optional value describing when the action should be performed.", 0, 1, timing); 3247 case -1837458939: 3248 /* timingDateTime */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3249 "An optional value describing when the action should be performed.", 0, 1, timing); 3250 case 164607061: 3251 /* timingAge */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3252 "An optional value describing when the action should be performed.", 0, 1, timing); 3253 case -615615829: 3254 /* timingPeriod */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3255 "An optional value describing when the action should be performed.", 0, 1, timing); 3256 case -1327253506: 3257 /* timingDuration */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3258 "An optional value describing when the action should be performed.", 0, 1, timing); 3259 case -710871277: 3260 /* timingRange */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3261 "An optional value describing when the action should be performed.", 0, 1, timing); 3262 case -497554124: 3263 /* timingTiming */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 3264 "An optional value describing when the action should be performed.", 0, 1, timing); 3265 case 767422259: 3266 /* participant */ return new Property("participant", 3267 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device)", 3268 "The participant that should perform or be responsible for this action.", 0, java.lang.Integer.MAX_VALUE, 3269 participant); 3270 case 3575610: 3271 /* type */ return new Property("type", "CodeableConcept", 3272 "The type of action to perform (create, update, remove).", 0, 1, type); 3273 case 586678389: 3274 /* groupingBehavior */ return new Property("groupingBehavior", "code", 3275 "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 3276 case 168639486: 3277 /* selectionBehavior */ return new Property("selectionBehavior", "code", 3278 "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 3279 case -1163906287: 3280 /* requiredBehavior */ return new Property("requiredBehavior", "code", 3281 "Defines expectations around whether an action is required.", 0, 1, requiredBehavior); 3282 case -1174249033: 3283 /* precheckBehavior */ return new Property("precheckBehavior", "code", 3284 "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 3285 case -922577408: 3286 /* cardinalityBehavior */ return new Property("cardinalityBehavior", "code", 3287 "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 3288 case -341064690: 3289 /* resource */ return new Property("resource", "Reference(Any)", 3290 "The resource that is the target of the action (e.g. CommunicationRequest).", 0, 1, resource); 3291 case -1422950858: 3292 /* action */ return new Property("action", "@RequestGroup.action", "Sub actions.", 0, 3293 java.lang.Integer.MAX_VALUE, action); 3294 default: 3295 return super.getNamedProperty(_hash, _name, _checkValid); 3296 } 3297 3298 } 3299 3300 @Override 3301 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3302 switch (hash) { 3303 case -980110702: 3304 /* prefix */ return this.prefix == null ? new Base[0] : new Base[] { this.prefix }; // StringType 3305 case 110371416: 3306 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 3307 case -1724546052: 3308 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 3309 case -900391049: 3310 /* textEquivalent */ return this.textEquivalent == null ? new Base[0] : new Base[] { this.textEquivalent }; // StringType 3311 case -1165461084: 3312 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 3313 case 3059181: 3314 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 3315 case 1587405498: 3316 /* documentation */ return this.documentation == null ? new Base[0] 3317 : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 3318 case -861311717: 3319 /* condition */ return this.condition == null ? new Base[0] 3320 : this.condition.toArray(new Base[this.condition.size()]); // RequestGroupActionConditionComponent 3321 case -384107967: 3322 /* relatedAction */ return this.relatedAction == null ? new Base[0] 3323 : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // RequestGroupActionRelatedActionComponent 3324 case -873664438: 3325 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 3326 case 767422259: 3327 /* participant */ return this.participant == null ? new Base[0] 3328 : this.participant.toArray(new Base[this.participant.size()]); // Reference 3329 case 3575610: 3330 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3331 case 586678389: 3332 /* groupingBehavior */ return this.groupingBehavior == null ? new Base[0] 3333 : new Base[] { this.groupingBehavior }; // Enumeration<ActionGroupingBehavior> 3334 case 168639486: 3335 /* selectionBehavior */ return this.selectionBehavior == null ? new Base[0] 3336 : new Base[] { this.selectionBehavior }; // Enumeration<ActionSelectionBehavior> 3337 case -1163906287: 3338 /* requiredBehavior */ return this.requiredBehavior == null ? new Base[0] 3339 : new Base[] { this.requiredBehavior }; // Enumeration<ActionRequiredBehavior> 3340 case -1174249033: 3341 /* precheckBehavior */ return this.precheckBehavior == null ? new Base[0] 3342 : new Base[] { this.precheckBehavior }; // Enumeration<ActionPrecheckBehavior> 3343 case -922577408: 3344 /* cardinalityBehavior */ return this.cardinalityBehavior == null ? new Base[0] 3345 : new Base[] { this.cardinalityBehavior }; // Enumeration<ActionCardinalityBehavior> 3346 case -341064690: 3347 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // Reference 3348 case -1422950858: 3349 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestGroupActionComponent 3350 default: 3351 return super.getProperty(hash, name, checkValid); 3352 } 3353 3354 } 3355 3356 @Override 3357 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3358 switch (hash) { 3359 case -980110702: // prefix 3360 this.prefix = castToString(value); // StringType 3361 return value; 3362 case 110371416: // title 3363 this.title = castToString(value); // StringType 3364 return value; 3365 case -1724546052: // description 3366 this.description = castToString(value); // StringType 3367 return value; 3368 case -900391049: // textEquivalent 3369 this.textEquivalent = castToString(value); // StringType 3370 return value; 3371 case -1165461084: // priority 3372 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 3373 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3374 return value; 3375 case 3059181: // code 3376 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 3377 return value; 3378 case 1587405498: // documentation 3379 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 3380 return value; 3381 case -861311717: // condition 3382 this.getCondition().add((RequestGroupActionConditionComponent) value); // RequestGroupActionConditionComponent 3383 return value; 3384 case -384107967: // relatedAction 3385 this.getRelatedAction().add((RequestGroupActionRelatedActionComponent) value); // RequestGroupActionRelatedActionComponent 3386 return value; 3387 case -873664438: // timing 3388 this.timing = castToType(value); // Type 3389 return value; 3390 case 767422259: // participant 3391 this.getParticipant().add(castToReference(value)); // Reference 3392 return value; 3393 case 3575610: // type 3394 this.type = castToCodeableConcept(value); // CodeableConcept 3395 return value; 3396 case 586678389: // groupingBehavior 3397 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 3398 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3399 return value; 3400 case 168639486: // selectionBehavior 3401 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 3402 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3403 return value; 3404 case -1163906287: // requiredBehavior 3405 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 3406 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3407 return value; 3408 case -1174249033: // precheckBehavior 3409 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 3410 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3411 return value; 3412 case -922577408: // cardinalityBehavior 3413 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 3414 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3415 return value; 3416 case -341064690: // resource 3417 this.resource = castToReference(value); // Reference 3418 return value; 3419 case -1422950858: // action 3420 this.getAction().add((RequestGroupActionComponent) value); // RequestGroupActionComponent 3421 return value; 3422 default: 3423 return super.setProperty(hash, name, value); 3424 } 3425 3426 } 3427 3428 @Override 3429 public Base setProperty(String name, Base value) throws FHIRException { 3430 if (name.equals("prefix")) { 3431 this.prefix = castToString(value); // StringType 3432 } else if (name.equals("title")) { 3433 this.title = castToString(value); // StringType 3434 } else if (name.equals("description")) { 3435 this.description = castToString(value); // StringType 3436 } else if (name.equals("textEquivalent")) { 3437 this.textEquivalent = castToString(value); // StringType 3438 } else if (name.equals("priority")) { 3439 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 3440 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 3441 } else if (name.equals("code")) { 3442 this.getCode().add(castToCodeableConcept(value)); 3443 } else if (name.equals("documentation")) { 3444 this.getDocumentation().add(castToRelatedArtifact(value)); 3445 } else if (name.equals("condition")) { 3446 this.getCondition().add((RequestGroupActionConditionComponent) value); 3447 } else if (name.equals("relatedAction")) { 3448 this.getRelatedAction().add((RequestGroupActionRelatedActionComponent) value); 3449 } else if (name.equals("timing[x]")) { 3450 this.timing = castToType(value); // Type 3451 } else if (name.equals("participant")) { 3452 this.getParticipant().add(castToReference(value)); 3453 } else if (name.equals("type")) { 3454 this.type = castToCodeableConcept(value); // CodeableConcept 3455 } else if (name.equals("groupingBehavior")) { 3456 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 3457 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 3458 } else if (name.equals("selectionBehavior")) { 3459 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 3460 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 3461 } else if (name.equals("requiredBehavior")) { 3462 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 3463 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 3464 } else if (name.equals("precheckBehavior")) { 3465 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 3466 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 3467 } else if (name.equals("cardinalityBehavior")) { 3468 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 3469 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 3470 } else if (name.equals("resource")) { 3471 this.resource = castToReference(value); // Reference 3472 } else if (name.equals("action")) { 3473 this.getAction().add((RequestGroupActionComponent) value); 3474 } else 3475 return super.setProperty(name, value); 3476 return value; 3477 } 3478 3479 @Override 3480 public void removeChild(String name, Base value) throws FHIRException { 3481 if (name.equals("prefix")) { 3482 this.prefix = null; 3483 } else if (name.equals("title")) { 3484 this.title = null; 3485 } else if (name.equals("description")) { 3486 this.description = null; 3487 } else if (name.equals("textEquivalent")) { 3488 this.textEquivalent = null; 3489 } else if (name.equals("priority")) { 3490 this.priority = null; 3491 } else if (name.equals("code")) { 3492 this.getCode().remove(castToCodeableConcept(value)); 3493 } else if (name.equals("documentation")) { 3494 this.getDocumentation().remove(castToRelatedArtifact(value)); 3495 } else if (name.equals("condition")) { 3496 this.getCondition().remove((RequestGroupActionConditionComponent) value); 3497 } else if (name.equals("relatedAction")) { 3498 this.getRelatedAction().remove((RequestGroupActionRelatedActionComponent) value); 3499 } else if (name.equals("timing[x]")) { 3500 this.timing = null; 3501 } else if (name.equals("participant")) { 3502 this.getParticipant().remove(castToReference(value)); 3503 } else if (name.equals("type")) { 3504 this.type = null; 3505 } else if (name.equals("groupingBehavior")) { 3506 this.groupingBehavior = null; 3507 } else if (name.equals("selectionBehavior")) { 3508 this.selectionBehavior = null; 3509 } else if (name.equals("requiredBehavior")) { 3510 this.requiredBehavior = null; 3511 } else if (name.equals("precheckBehavior")) { 3512 this.precheckBehavior = null; 3513 } else if (name.equals("cardinalityBehavior")) { 3514 this.cardinalityBehavior = null; 3515 } else if (name.equals("resource")) { 3516 this.resource = null; 3517 } else if (name.equals("action")) { 3518 this.getAction().remove((RequestGroupActionComponent) value); 3519 } else 3520 super.removeChild(name, value); 3521 3522 } 3523 3524 @Override 3525 public Base makeProperty(int hash, String name) throws FHIRException { 3526 switch (hash) { 3527 case -980110702: 3528 return getPrefixElement(); 3529 case 110371416: 3530 return getTitleElement(); 3531 case -1724546052: 3532 return getDescriptionElement(); 3533 case -900391049: 3534 return getTextEquivalentElement(); 3535 case -1165461084: 3536 return getPriorityElement(); 3537 case 3059181: 3538 return addCode(); 3539 case 1587405498: 3540 return addDocumentation(); 3541 case -861311717: 3542 return addCondition(); 3543 case -384107967: 3544 return addRelatedAction(); 3545 case 164632566: 3546 return getTiming(); 3547 case -873664438: 3548 return getTiming(); 3549 case 767422259: 3550 return addParticipant(); 3551 case 3575610: 3552 return getType(); 3553 case 586678389: 3554 return getGroupingBehaviorElement(); 3555 case 168639486: 3556 return getSelectionBehaviorElement(); 3557 case -1163906287: 3558 return getRequiredBehaviorElement(); 3559 case -1174249033: 3560 return getPrecheckBehaviorElement(); 3561 case -922577408: 3562 return getCardinalityBehaviorElement(); 3563 case -341064690: 3564 return getResource(); 3565 case -1422950858: 3566 return addAction(); 3567 default: 3568 return super.makeProperty(hash, name); 3569 } 3570 3571 } 3572 3573 @Override 3574 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3575 switch (hash) { 3576 case -980110702: 3577 /* prefix */ return new String[] { "string" }; 3578 case 110371416: 3579 /* title */ return new String[] { "string" }; 3580 case -1724546052: 3581 /* description */ return new String[] { "string" }; 3582 case -900391049: 3583 /* textEquivalent */ return new String[] { "string" }; 3584 case -1165461084: 3585 /* priority */ return new String[] { "code" }; 3586 case 3059181: 3587 /* code */ return new String[] { "CodeableConcept" }; 3588 case 1587405498: 3589 /* documentation */ return new String[] { "RelatedArtifact" }; 3590 case -861311717: 3591 /* condition */ return new String[] {}; 3592 case -384107967: 3593 /* relatedAction */ return new String[] {}; 3594 case -873664438: 3595 /* timing */ return new String[] { "dateTime", "Age", "Period", "Duration", "Range", "Timing" }; 3596 case 767422259: 3597 /* participant */ return new String[] { "Reference" }; 3598 case 3575610: 3599 /* type */ return new String[] { "CodeableConcept" }; 3600 case 586678389: 3601 /* groupingBehavior */ return new String[] { "code" }; 3602 case 168639486: 3603 /* selectionBehavior */ return new String[] { "code" }; 3604 case -1163906287: 3605 /* requiredBehavior */ return new String[] { "code" }; 3606 case -1174249033: 3607 /* precheckBehavior */ return new String[] { "code" }; 3608 case -922577408: 3609 /* cardinalityBehavior */ return new String[] { "code" }; 3610 case -341064690: 3611 /* resource */ return new String[] { "Reference" }; 3612 case -1422950858: 3613 /* action */ return new String[] { "@RequestGroup.action" }; 3614 default: 3615 return super.getTypesForProperty(hash, name); 3616 } 3617 3618 } 3619 3620 @Override 3621 public Base addChild(String name) throws FHIRException { 3622 if (name.equals("prefix")) { 3623 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.prefix"); 3624 } else if (name.equals("title")) { 3625 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.title"); 3626 } else if (name.equals("description")) { 3627 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.description"); 3628 } else if (name.equals("textEquivalent")) { 3629 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.textEquivalent"); 3630 } else if (name.equals("priority")) { 3631 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.priority"); 3632 } else if (name.equals("code")) { 3633 return addCode(); 3634 } else if (name.equals("documentation")) { 3635 return addDocumentation(); 3636 } else if (name.equals("condition")) { 3637 return addCondition(); 3638 } else if (name.equals("relatedAction")) { 3639 return addRelatedAction(); 3640 } else if (name.equals("timingDateTime")) { 3641 this.timing = new DateTimeType(); 3642 return this.timing; 3643 } else if (name.equals("timingAge")) { 3644 this.timing = new Age(); 3645 return this.timing; 3646 } else if (name.equals("timingPeriod")) { 3647 this.timing = new Period(); 3648 return this.timing; 3649 } else if (name.equals("timingDuration")) { 3650 this.timing = new Duration(); 3651 return this.timing; 3652 } else if (name.equals("timingRange")) { 3653 this.timing = new Range(); 3654 return this.timing; 3655 } else if (name.equals("timingTiming")) { 3656 this.timing = new Timing(); 3657 return this.timing; 3658 } else if (name.equals("participant")) { 3659 return addParticipant(); 3660 } else if (name.equals("type")) { 3661 this.type = new CodeableConcept(); 3662 return this.type; 3663 } else if (name.equals("groupingBehavior")) { 3664 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.groupingBehavior"); 3665 } else if (name.equals("selectionBehavior")) { 3666 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.selectionBehavior"); 3667 } else if (name.equals("requiredBehavior")) { 3668 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.requiredBehavior"); 3669 } else if (name.equals("precheckBehavior")) { 3670 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.precheckBehavior"); 3671 } else if (name.equals("cardinalityBehavior")) { 3672 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.cardinalityBehavior"); 3673 } else if (name.equals("resource")) { 3674 this.resource = new Reference(); 3675 return this.resource; 3676 } else if (name.equals("action")) { 3677 return addAction(); 3678 } else 3679 return super.addChild(name); 3680 } 3681 3682 public RequestGroupActionComponent copy() { 3683 RequestGroupActionComponent dst = new RequestGroupActionComponent(); 3684 copyValues(dst); 3685 return dst; 3686 } 3687 3688 public void copyValues(RequestGroupActionComponent dst) { 3689 super.copyValues(dst); 3690 dst.prefix = prefix == null ? null : prefix.copy(); 3691 dst.title = title == null ? null : title.copy(); 3692 dst.description = description == null ? null : description.copy(); 3693 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 3694 dst.priority = priority == null ? null : priority.copy(); 3695 if (code != null) { 3696 dst.code = new ArrayList<CodeableConcept>(); 3697 for (CodeableConcept i : code) 3698 dst.code.add(i.copy()); 3699 } 3700 ; 3701 if (documentation != null) { 3702 dst.documentation = new ArrayList<RelatedArtifact>(); 3703 for (RelatedArtifact i : documentation) 3704 dst.documentation.add(i.copy()); 3705 } 3706 ; 3707 if (condition != null) { 3708 dst.condition = new ArrayList<RequestGroupActionConditionComponent>(); 3709 for (RequestGroupActionConditionComponent i : condition) 3710 dst.condition.add(i.copy()); 3711 } 3712 ; 3713 if (relatedAction != null) { 3714 dst.relatedAction = new ArrayList<RequestGroupActionRelatedActionComponent>(); 3715 for (RequestGroupActionRelatedActionComponent i : relatedAction) 3716 dst.relatedAction.add(i.copy()); 3717 } 3718 ; 3719 dst.timing = timing == null ? null : timing.copy(); 3720 if (participant != null) { 3721 dst.participant = new ArrayList<Reference>(); 3722 for (Reference i : participant) 3723 dst.participant.add(i.copy()); 3724 } 3725 ; 3726 dst.type = type == null ? null : type.copy(); 3727 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 3728 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 3729 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 3730 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 3731 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 3732 dst.resource = resource == null ? null : resource.copy(); 3733 if (action != null) { 3734 dst.action = new ArrayList<RequestGroupActionComponent>(); 3735 for (RequestGroupActionComponent i : action) 3736 dst.action.add(i.copy()); 3737 } 3738 ; 3739 } 3740 3741 @Override 3742 public boolean equalsDeep(Base other_) { 3743 if (!super.equalsDeep(other_)) 3744 return false; 3745 if (!(other_ instanceof RequestGroupActionComponent)) 3746 return false; 3747 RequestGroupActionComponent o = (RequestGroupActionComponent) other_; 3748 return compareDeep(prefix, o.prefix, true) && compareDeep(title, o.title, true) 3749 && compareDeep(description, o.description, true) && compareDeep(textEquivalent, o.textEquivalent, true) 3750 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 3751 && compareDeep(documentation, o.documentation, true) && compareDeep(condition, o.condition, true) 3752 && compareDeep(relatedAction, o.relatedAction, true) && compareDeep(timing, o.timing, true) 3753 && compareDeep(participant, o.participant, true) && compareDeep(type, o.type, true) 3754 && compareDeep(groupingBehavior, o.groupingBehavior, true) 3755 && compareDeep(selectionBehavior, o.selectionBehavior, true) 3756 && compareDeep(requiredBehavior, o.requiredBehavior, true) 3757 && compareDeep(precheckBehavior, o.precheckBehavior, true) 3758 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) && compareDeep(resource, o.resource, true) 3759 && compareDeep(action, o.action, true); 3760 } 3761 3762 @Override 3763 public boolean equalsShallow(Base other_) { 3764 if (!super.equalsShallow(other_)) 3765 return false; 3766 if (!(other_ instanceof RequestGroupActionComponent)) 3767 return false; 3768 RequestGroupActionComponent o = (RequestGroupActionComponent) other_; 3769 return compareValues(prefix, o.prefix, true) && compareValues(title, o.title, true) 3770 && compareValues(description, o.description, true) && compareValues(textEquivalent, o.textEquivalent, true) 3771 && compareValues(priority, o.priority, true) && compareValues(groupingBehavior, o.groupingBehavior, true) 3772 && compareValues(selectionBehavior, o.selectionBehavior, true) 3773 && compareValues(requiredBehavior, o.requiredBehavior, true) 3774 && compareValues(precheckBehavior, o.precheckBehavior, true) 3775 && compareValues(cardinalityBehavior, o.cardinalityBehavior, true); 3776 } 3777 3778 public boolean isEmpty() { 3779 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(prefix, title, description, textEquivalent, 3780 priority, code, documentation, condition, relatedAction, timing, participant, type, groupingBehavior, 3781 selectionBehavior, requiredBehavior, precheckBehavior, cardinalityBehavior, resource, action); 3782 } 3783 3784 public String fhirType() { 3785 return "RequestGroup.action"; 3786 3787 } 3788 3789 } 3790 3791 @Block() 3792 public static class RequestGroupActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 3793 /** 3794 * The kind of condition. 3795 */ 3796 @Child(name = "kind", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3797 @Description(shortDefinition = "applicability | start | stop", formalDefinition = "The kind of condition.") 3798 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-condition-kind") 3799 protected Enumeration<ActionConditionKind> kind; 3800 3801 /** 3802 * An expression that returns true or false, indicating whether or not the 3803 * condition is satisfied. 3804 */ 3805 @Child(name = "expression", type = { 3806 Expression.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3807 @Description(shortDefinition = "Boolean-valued expression", formalDefinition = "An expression that returns true or false, indicating whether or not the condition is satisfied.") 3808 protected Expression expression; 3809 3810 private static final long serialVersionUID = -455150438L; 3811 3812 /** 3813 * Constructor 3814 */ 3815 public RequestGroupActionConditionComponent() { 3816 super(); 3817 } 3818 3819 /** 3820 * Constructor 3821 */ 3822 public RequestGroupActionConditionComponent(Enumeration<ActionConditionKind> kind) { 3823 super(); 3824 this.kind = kind; 3825 } 3826 3827 /** 3828 * @return {@link #kind} (The kind of condition.). This is the underlying object 3829 * with id, value and extensions. The accessor "getKind" gives direct 3830 * access to the value 3831 */ 3832 public Enumeration<ActionConditionKind> getKindElement() { 3833 if (this.kind == null) 3834 if (Configuration.errorOnAutoCreate()) 3835 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.kind"); 3836 else if (Configuration.doAutoCreate()) 3837 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 3838 return this.kind; 3839 } 3840 3841 public boolean hasKindElement() { 3842 return this.kind != null && !this.kind.isEmpty(); 3843 } 3844 3845 public boolean hasKind() { 3846 return this.kind != null && !this.kind.isEmpty(); 3847 } 3848 3849 /** 3850 * @param value {@link #kind} (The kind of condition.). This is the underlying 3851 * object with id, value and extensions. The accessor "getKind" 3852 * gives direct access to the value 3853 */ 3854 public RequestGroupActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 3855 this.kind = value; 3856 return this; 3857 } 3858 3859 /** 3860 * @return The kind of condition. 3861 */ 3862 public ActionConditionKind getKind() { 3863 return this.kind == null ? null : this.kind.getValue(); 3864 } 3865 3866 /** 3867 * @param value The kind of condition. 3868 */ 3869 public RequestGroupActionConditionComponent setKind(ActionConditionKind value) { 3870 if (this.kind == null) 3871 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 3872 this.kind.setValue(value); 3873 return this; 3874 } 3875 3876 /** 3877 * @return {@link #expression} (An expression that returns true or false, 3878 * indicating whether or not the condition is satisfied.) 3879 */ 3880 public Expression getExpression() { 3881 if (this.expression == null) 3882 if (Configuration.errorOnAutoCreate()) 3883 throw new Error("Attempt to auto-create RequestGroupActionConditionComponent.expression"); 3884 else if (Configuration.doAutoCreate()) 3885 this.expression = new Expression(); // cc 3886 return this.expression; 3887 } 3888 3889 public boolean hasExpression() { 3890 return this.expression != null && !this.expression.isEmpty(); 3891 } 3892 3893 /** 3894 * @param value {@link #expression} (An expression that returns true or false, 3895 * indicating whether or not the condition is satisfied.) 3896 */ 3897 public RequestGroupActionConditionComponent setExpression(Expression value) { 3898 this.expression = value; 3899 return this; 3900 } 3901 3902 protected void listChildren(List<Property> children) { 3903 super.listChildren(children); 3904 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 3905 children.add(new Property("expression", "Expression", 3906 "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, 3907 expression)); 3908 } 3909 3910 @Override 3911 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3912 switch (_hash) { 3913 case 3292052: 3914 /* kind */ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 3915 case -1795452264: 3916 /* expression */ return new Property("expression", "Expression", 3917 "An expression that returns true or false, indicating whether or not the condition is satisfied.", 0, 1, 3918 expression); 3919 default: 3920 return super.getNamedProperty(_hash, _name, _checkValid); 3921 } 3922 3923 } 3924 3925 @Override 3926 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3927 switch (hash) { 3928 case 3292052: 3929 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<ActionConditionKind> 3930 case -1795452264: 3931 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // Expression 3932 default: 3933 return super.getProperty(hash, name, checkValid); 3934 } 3935 3936 } 3937 3938 @Override 3939 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3940 switch (hash) { 3941 case 3292052: // kind 3942 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 3943 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 3944 return value; 3945 case -1795452264: // expression 3946 this.expression = castToExpression(value); // Expression 3947 return value; 3948 default: 3949 return super.setProperty(hash, name, value); 3950 } 3951 3952 } 3953 3954 @Override 3955 public Base setProperty(String name, Base value) throws FHIRException { 3956 if (name.equals("kind")) { 3957 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 3958 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 3959 } else if (name.equals("expression")) { 3960 this.expression = castToExpression(value); // Expression 3961 } else 3962 return super.setProperty(name, value); 3963 return value; 3964 } 3965 3966 @Override 3967 public void removeChild(String name, Base value) throws FHIRException { 3968 if (name.equals("kind")) { 3969 this.kind = null; 3970 } else if (name.equals("expression")) { 3971 this.expression = null; 3972 } else 3973 super.removeChild(name, value); 3974 3975 } 3976 3977 @Override 3978 public Base makeProperty(int hash, String name) throws FHIRException { 3979 switch (hash) { 3980 case 3292052: 3981 return getKindElement(); 3982 case -1795452264: 3983 return getExpression(); 3984 default: 3985 return super.makeProperty(hash, name); 3986 } 3987 3988 } 3989 3990 @Override 3991 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3992 switch (hash) { 3993 case 3292052: 3994 /* kind */ return new String[] { "code" }; 3995 case -1795452264: 3996 /* expression */ return new String[] { "Expression" }; 3997 default: 3998 return super.getTypesForProperty(hash, name); 3999 } 4000 4001 } 4002 4003 @Override 4004 public Base addChild(String name) throws FHIRException { 4005 if (name.equals("kind")) { 4006 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.kind"); 4007 } else if (name.equals("expression")) { 4008 this.expression = new Expression(); 4009 return this.expression; 4010 } else 4011 return super.addChild(name); 4012 } 4013 4014 public RequestGroupActionConditionComponent copy() { 4015 RequestGroupActionConditionComponent dst = new RequestGroupActionConditionComponent(); 4016 copyValues(dst); 4017 return dst; 4018 } 4019 4020 public void copyValues(RequestGroupActionConditionComponent dst) { 4021 super.copyValues(dst); 4022 dst.kind = kind == null ? null : kind.copy(); 4023 dst.expression = expression == null ? null : expression.copy(); 4024 } 4025 4026 @Override 4027 public boolean equalsDeep(Base other_) { 4028 if (!super.equalsDeep(other_)) 4029 return false; 4030 if (!(other_ instanceof RequestGroupActionConditionComponent)) 4031 return false; 4032 RequestGroupActionConditionComponent o = (RequestGroupActionConditionComponent) other_; 4033 return compareDeep(kind, o.kind, true) && compareDeep(expression, o.expression, true); 4034 } 4035 4036 @Override 4037 public boolean equalsShallow(Base other_) { 4038 if (!super.equalsShallow(other_)) 4039 return false; 4040 if (!(other_ instanceof RequestGroupActionConditionComponent)) 4041 return false; 4042 RequestGroupActionConditionComponent o = (RequestGroupActionConditionComponent) other_; 4043 return compareValues(kind, o.kind, true); 4044 } 4045 4046 public boolean isEmpty() { 4047 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, expression); 4048 } 4049 4050 public String fhirType() { 4051 return "RequestGroup.action.condition"; 4052 4053 } 4054 4055 } 4056 4057 @Block() 4058 public static class RequestGroupActionRelatedActionComponent extends BackboneElement implements IBaseBackboneElement { 4059 /** 4060 * The element id of the action this is related to. 4061 */ 4062 @Child(name = "actionId", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4063 @Description(shortDefinition = "What action this is related to", formalDefinition = "The element id of the action this is related to.") 4064 protected IdType actionId; 4065 4066 /** 4067 * The relationship of this action to the related action. 4068 */ 4069 @Child(name = "relationship", type = { 4070 CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 4071 @Description(shortDefinition = "before-start | before | before-end | concurrent-with-start | concurrent | concurrent-with-end | after-start | after | after-end", formalDefinition = "The relationship of this action to the related action.") 4072 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-relationship-type") 4073 protected Enumeration<ActionRelationshipType> relationship; 4074 4075 /** 4076 * A duration or range of durations to apply to the relationship. For example, 4077 * 30-60 minutes before. 4078 */ 4079 @Child(name = "offset", type = { Duration.class, 4080 Range.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4081 @Description(shortDefinition = "Time offset for the relationship", formalDefinition = "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.") 4082 protected Type offset; 4083 4084 private static final long serialVersionUID = 1063306770L; 4085 4086 /** 4087 * Constructor 4088 */ 4089 public RequestGroupActionRelatedActionComponent() { 4090 super(); 4091 } 4092 4093 /** 4094 * Constructor 4095 */ 4096 public RequestGroupActionRelatedActionComponent(IdType actionId, Enumeration<ActionRelationshipType> relationship) { 4097 super(); 4098 this.actionId = actionId; 4099 this.relationship = relationship; 4100 } 4101 4102 /** 4103 * @return {@link #actionId} (The element id of the action this is related to.). 4104 * This is the underlying object with id, value and extensions. The 4105 * accessor "getActionId" gives direct access to the value 4106 */ 4107 public IdType getActionIdElement() { 4108 if (this.actionId == null) 4109 if (Configuration.errorOnAutoCreate()) 4110 throw new Error("Attempt to auto-create RequestGroupActionRelatedActionComponent.actionId"); 4111 else if (Configuration.doAutoCreate()) 4112 this.actionId = new IdType(); // bb 4113 return this.actionId; 4114 } 4115 4116 public boolean hasActionIdElement() { 4117 return this.actionId != null && !this.actionId.isEmpty(); 4118 } 4119 4120 public boolean hasActionId() { 4121 return this.actionId != null && !this.actionId.isEmpty(); 4122 } 4123 4124 /** 4125 * @param value {@link #actionId} (The element id of the action this is related 4126 * to.). This is the underlying object with id, value and 4127 * extensions. The accessor "getActionId" gives direct access to 4128 * the value 4129 */ 4130 public RequestGroupActionRelatedActionComponent setActionIdElement(IdType value) { 4131 this.actionId = value; 4132 return this; 4133 } 4134 4135 /** 4136 * @return The element id of the action this is related to. 4137 */ 4138 public String getActionId() { 4139 return this.actionId == null ? null : this.actionId.getValue(); 4140 } 4141 4142 /** 4143 * @param value The element id of the action this is related to. 4144 */ 4145 public RequestGroupActionRelatedActionComponent setActionId(String value) { 4146 if (this.actionId == null) 4147 this.actionId = new IdType(); 4148 this.actionId.setValue(value); 4149 return this; 4150 } 4151 4152 /** 4153 * @return {@link #relationship} (The relationship of this action to the related 4154 * action.). This is the underlying object with id, value and 4155 * extensions. The accessor "getRelationship" gives direct access to the 4156 * value 4157 */ 4158 public Enumeration<ActionRelationshipType> getRelationshipElement() { 4159 if (this.relationship == null) 4160 if (Configuration.errorOnAutoCreate()) 4161 throw new Error("Attempt to auto-create RequestGroupActionRelatedActionComponent.relationship"); 4162 else if (Configuration.doAutoCreate()) 4163 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 4164 return this.relationship; 4165 } 4166 4167 public boolean hasRelationshipElement() { 4168 return this.relationship != null && !this.relationship.isEmpty(); 4169 } 4170 4171 public boolean hasRelationship() { 4172 return this.relationship != null && !this.relationship.isEmpty(); 4173 } 4174 4175 /** 4176 * @param value {@link #relationship} (The relationship of this action to the 4177 * related action.). This is the underlying object with id, value 4178 * and extensions. The accessor "getRelationship" gives direct 4179 * access to the value 4180 */ 4181 public RequestGroupActionRelatedActionComponent setRelationshipElement(Enumeration<ActionRelationshipType> value) { 4182 this.relationship = value; 4183 return this; 4184 } 4185 4186 /** 4187 * @return The relationship of this action to the related action. 4188 */ 4189 public ActionRelationshipType getRelationship() { 4190 return this.relationship == null ? null : this.relationship.getValue(); 4191 } 4192 4193 /** 4194 * @param value The relationship of this action to the related action. 4195 */ 4196 public RequestGroupActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 4197 if (this.relationship == null) 4198 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 4199 this.relationship.setValue(value); 4200 return this; 4201 } 4202 4203 /** 4204 * @return {@link #offset} (A duration or range of durations to apply to the 4205 * relationship. For example, 30-60 minutes before.) 4206 */ 4207 public Type getOffset() { 4208 return this.offset; 4209 } 4210 4211 /** 4212 * @return {@link #offset} (A duration or range of durations to apply to the 4213 * relationship. For example, 30-60 minutes before.) 4214 */ 4215 public Duration getOffsetDuration() throws FHIRException { 4216 if (this.offset == null) 4217 this.offset = new Duration(); 4218 if (!(this.offset instanceof Duration)) 4219 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.offset.getClass().getName() 4220 + " was encountered"); 4221 return (Duration) this.offset; 4222 } 4223 4224 public boolean hasOffsetDuration() { 4225 return this.offset instanceof Duration; 4226 } 4227 4228 /** 4229 * @return {@link #offset} (A duration or range of durations to apply to the 4230 * relationship. For example, 30-60 minutes before.) 4231 */ 4232 public Range getOffsetRange() throws FHIRException { 4233 if (this.offset == null) 4234 this.offset = new Range(); 4235 if (!(this.offset instanceof Range)) 4236 throw new FHIRException( 4237 "Type mismatch: the type Range was expected, but " + this.offset.getClass().getName() + " was encountered"); 4238 return (Range) this.offset; 4239 } 4240 4241 public boolean hasOffsetRange() { 4242 return this.offset instanceof Range; 4243 } 4244 4245 public boolean hasOffset() { 4246 return this.offset != null && !this.offset.isEmpty(); 4247 } 4248 4249 /** 4250 * @param value {@link #offset} (A duration or range of durations to apply to 4251 * the relationship. For example, 30-60 minutes before.) 4252 */ 4253 public RequestGroupActionRelatedActionComponent setOffset(Type value) { 4254 if (value != null && !(value instanceof Duration || value instanceof Range)) 4255 throw new Error("Not the right type for RequestGroup.action.relatedAction.offset[x]: " + value.fhirType()); 4256 this.offset = value; 4257 return this; 4258 } 4259 4260 protected void listChildren(List<Property> children) { 4261 super.listChildren(children); 4262 children.add(new Property("actionId", "id", "The element id of the action this is related to.", 0, 1, actionId)); 4263 children.add(new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, 4264 relationship)); 4265 children.add(new Property("offset[x]", "Duration|Range", 4266 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4267 offset)); 4268 } 4269 4270 @Override 4271 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4272 switch (_hash) { 4273 case -1656172047: 4274 /* actionId */ return new Property("actionId", "id", "The element id of the action this is related to.", 0, 1, 4275 actionId); 4276 case -261851592: 4277 /* relationship */ return new Property("relationship", "code", 4278 "The relationship of this action to the related action.", 0, 1, relationship); 4279 case -1960684787: 4280 /* offset[x] */ return new Property("offset[x]", "Duration|Range", 4281 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4282 offset); 4283 case -1019779949: 4284 /* offset */ return new Property("offset[x]", "Duration|Range", 4285 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4286 offset); 4287 case 134075207: 4288 /* offsetDuration */ return new Property("offset[x]", "Duration|Range", 4289 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4290 offset); 4291 case 1263585386: 4292 /* offsetRange */ return new Property("offset[x]", "Duration|Range", 4293 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 4294 offset); 4295 default: 4296 return super.getNamedProperty(_hash, _name, _checkValid); 4297 } 4298 4299 } 4300 4301 @Override 4302 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4303 switch (hash) { 4304 case -1656172047: 4305 /* actionId */ return this.actionId == null ? new Base[0] : new Base[] { this.actionId }; // IdType 4306 case -261851592: 4307 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // Enumeration<ActionRelationshipType> 4308 case -1019779949: 4309 /* offset */ return this.offset == null ? new Base[0] : new Base[] { this.offset }; // Type 4310 default: 4311 return super.getProperty(hash, name, checkValid); 4312 } 4313 4314 } 4315 4316 @Override 4317 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4318 switch (hash) { 4319 case -1656172047: // actionId 4320 this.actionId = castToId(value); // IdType 4321 return value; 4322 case -261851592: // relationship 4323 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 4324 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 4325 return value; 4326 case -1019779949: // offset 4327 this.offset = castToType(value); // Type 4328 return value; 4329 default: 4330 return super.setProperty(hash, name, value); 4331 } 4332 4333 } 4334 4335 @Override 4336 public Base setProperty(String name, Base value) throws FHIRException { 4337 if (name.equals("actionId")) { 4338 this.actionId = castToId(value); // IdType 4339 } else if (name.equals("relationship")) { 4340 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 4341 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 4342 } else if (name.equals("offset[x]")) { 4343 this.offset = castToType(value); // Type 4344 } else 4345 return super.setProperty(name, value); 4346 return value; 4347 } 4348 4349 @Override 4350 public void removeChild(String name, Base value) throws FHIRException { 4351 if (name.equals("actionId")) { 4352 this.actionId = null; 4353 } else if (name.equals("relationship")) { 4354 this.relationship = null; 4355 } else if (name.equals("offset[x]")) { 4356 this.offset = null; 4357 } else 4358 super.removeChild(name, value); 4359 4360 } 4361 4362 @Override 4363 public Base makeProperty(int hash, String name) throws FHIRException { 4364 switch (hash) { 4365 case -1656172047: 4366 return getActionIdElement(); 4367 case -261851592: 4368 return getRelationshipElement(); 4369 case -1960684787: 4370 return getOffset(); 4371 case -1019779949: 4372 return getOffset(); 4373 default: 4374 return super.makeProperty(hash, name); 4375 } 4376 4377 } 4378 4379 @Override 4380 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4381 switch (hash) { 4382 case -1656172047: 4383 /* actionId */ return new String[] { "id" }; 4384 case -261851592: 4385 /* relationship */ return new String[] { "code" }; 4386 case -1019779949: 4387 /* offset */ return new String[] { "Duration", "Range" }; 4388 default: 4389 return super.getTypesForProperty(hash, name); 4390 } 4391 4392 } 4393 4394 @Override 4395 public Base addChild(String name) throws FHIRException { 4396 if (name.equals("actionId")) { 4397 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.actionId"); 4398 } else if (name.equals("relationship")) { 4399 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.relationship"); 4400 } else if (name.equals("offsetDuration")) { 4401 this.offset = new Duration(); 4402 return this.offset; 4403 } else if (name.equals("offsetRange")) { 4404 this.offset = new Range(); 4405 return this.offset; 4406 } else 4407 return super.addChild(name); 4408 } 4409 4410 public RequestGroupActionRelatedActionComponent copy() { 4411 RequestGroupActionRelatedActionComponent dst = new RequestGroupActionRelatedActionComponent(); 4412 copyValues(dst); 4413 return dst; 4414 } 4415 4416 public void copyValues(RequestGroupActionRelatedActionComponent dst) { 4417 super.copyValues(dst); 4418 dst.actionId = actionId == null ? null : actionId.copy(); 4419 dst.relationship = relationship == null ? null : relationship.copy(); 4420 dst.offset = offset == null ? null : offset.copy(); 4421 } 4422 4423 @Override 4424 public boolean equalsDeep(Base other_) { 4425 if (!super.equalsDeep(other_)) 4426 return false; 4427 if (!(other_ instanceof RequestGroupActionRelatedActionComponent)) 4428 return false; 4429 RequestGroupActionRelatedActionComponent o = (RequestGroupActionRelatedActionComponent) other_; 4430 return compareDeep(actionId, o.actionId, true) && compareDeep(relationship, o.relationship, true) 4431 && compareDeep(offset, o.offset, true); 4432 } 4433 4434 @Override 4435 public boolean equalsShallow(Base other_) { 4436 if (!super.equalsShallow(other_)) 4437 return false; 4438 if (!(other_ instanceof RequestGroupActionRelatedActionComponent)) 4439 return false; 4440 RequestGroupActionRelatedActionComponent o = (RequestGroupActionRelatedActionComponent) other_; 4441 return compareValues(actionId, o.actionId, true) && compareValues(relationship, o.relationship, true); 4442 } 4443 4444 public boolean isEmpty() { 4445 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actionId, relationship, offset); 4446 } 4447 4448 public String fhirType() { 4449 return "RequestGroup.action.relatedAction"; 4450 4451 } 4452 4453 } 4454 4455 /** 4456 * Allows a service to provide a unique, business identifier for the request. 4457 */ 4458 @Child(name = "identifier", type = { 4459 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4460 @Description(shortDefinition = "Business identifier", formalDefinition = "Allows a service to provide a unique, business identifier for the request.") 4461 protected List<Identifier> identifier; 4462 4463 /** 4464 * A canonical URL referencing a FHIR-defined protocol, guideline, orderset or 4465 * other definition that is adhered to in whole or in part by this request. 4466 */ 4467 @Child(name = "instantiatesCanonical", type = { 4468 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4469 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.") 4470 protected List<CanonicalType> instantiatesCanonical; 4471 4472 /** 4473 * A URL referencing an externally defined protocol, guideline, orderset or 4474 * other definition that is adhered to in whole or in part by this request. 4475 */ 4476 @Child(name = "instantiatesUri", type = { 4477 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4478 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.") 4479 protected List<UriType> instantiatesUri; 4480 4481 /** 4482 * A plan, proposal or order that is fulfilled in whole or in part by this 4483 * request. 4484 */ 4485 @Child(name = "basedOn", type = { 4486 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4487 @Description(shortDefinition = "Fulfills plan, proposal, or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this request.") 4488 protected List<Reference> basedOn; 4489 /** 4490 * The actual objects that are the target of the reference (A plan, proposal or 4491 * order that is fulfilled in whole or in part by this request.) 4492 */ 4493 protected List<Resource> basedOnTarget; 4494 4495 /** 4496 * Completed or terminated request(s) whose function is taken by this new 4497 * request. 4498 */ 4499 @Child(name = "replaces", type = { 4500 Reference.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4501 @Description(shortDefinition = "Request(s) replaced by this request", formalDefinition = "Completed or terminated request(s) whose function is taken by this new request.") 4502 protected List<Reference> replaces; 4503 /** 4504 * The actual objects that are the target of the reference (Completed or 4505 * terminated request(s) whose function is taken by this new request.) 4506 */ 4507 protected List<Resource> replacesTarget; 4508 4509 /** 4510 * A shared identifier common to all requests that were authorized more or less 4511 * simultaneously by a single author, representing the identifier of the 4512 * requisition, prescription or similar form. 4513 */ 4514 @Child(name = "groupIdentifier", type = { 4515 Identifier.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 4516 @Description(shortDefinition = "Composite request this is part of", formalDefinition = "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.") 4517 protected Identifier groupIdentifier; 4518 4519 /** 4520 * The current state of the request. For request groups, the status reflects the 4521 * status of all the requests in the group. 4522 */ 4523 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 4524 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "The current state of the request. For request groups, the status reflects the status of all the requests in the group.") 4525 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 4526 protected Enumeration<RequestStatus> status; 4527 4528 /** 4529 * Indicates the level of authority/intentionality associated with the request 4530 * and where the request fits into the workflow chain. 4531 */ 4532 @Child(name = "intent", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = true, summary = true) 4533 @Description(shortDefinition = "proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.") 4534 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-intent") 4535 protected Enumeration<RequestIntent> intent; 4536 4537 /** 4538 * Indicates how quickly the request should be addressed with respect to other 4539 * requests. 4540 */ 4541 @Child(name = "priority", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 4542 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the request should be addressed with respect to other requests.") 4543 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 4544 protected Enumeration<RequestPriority> priority; 4545 4546 /** 4547 * A code that identifies what the overall request group is. 4548 */ 4549 @Child(name = "code", type = { CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 4550 @Description(shortDefinition = "What's being requested/ordered", formalDefinition = "A code that identifies what the overall request group is.") 4551 protected CodeableConcept code; 4552 4553 /** 4554 * The subject for which the request group was created. 4555 */ 4556 @Child(name = "subject", type = { Patient.class, 4557 Group.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 4558 @Description(shortDefinition = "Who the request group is about", formalDefinition = "The subject for which the request group was created.") 4559 protected Reference subject; 4560 4561 /** 4562 * The actual object that is the target of the reference (The subject for which 4563 * the request group was created.) 4564 */ 4565 protected Resource subjectTarget; 4566 4567 /** 4568 * Describes the context of the request group, if any. 4569 */ 4570 @Child(name = "encounter", type = { 4571 Encounter.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4572 @Description(shortDefinition = "Created as part of", formalDefinition = "Describes the context of the request group, if any.") 4573 protected Reference encounter; 4574 4575 /** 4576 * The actual object that is the target of the reference (Describes the context 4577 * of the request group, if any.) 4578 */ 4579 protected Encounter encounterTarget; 4580 4581 /** 4582 * Indicates when the request group was created. 4583 */ 4584 @Child(name = "authoredOn", type = { 4585 DateTimeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 4586 @Description(shortDefinition = "When the request group was authored", formalDefinition = "Indicates when the request group was created.") 4587 protected DateTimeType authoredOn; 4588 4589 /** 4590 * Provides a reference to the author of the request group. 4591 */ 4592 @Child(name = "author", type = { Device.class, Practitioner.class, 4593 PractitionerRole.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 4594 @Description(shortDefinition = "Device or practitioner that authored the request group", formalDefinition = "Provides a reference to the author of the request group.") 4595 protected Reference author; 4596 4597 /** 4598 * The actual object that is the target of the reference (Provides a reference 4599 * to the author of the request group.) 4600 */ 4601 protected Resource authorTarget; 4602 4603 /** 4604 * Describes the reason for the request group in coded or textual form. 4605 */ 4606 @Child(name = "reasonCode", type = { 4607 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4608 @Description(shortDefinition = "Why the request group is needed", formalDefinition = "Describes the reason for the request group in coded or textual form.") 4609 protected List<CodeableConcept> reasonCode; 4610 4611 /** 4612 * Indicates another resource whose existence justifies this request group. 4613 */ 4614 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 4615 DocumentReference.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4616 @Description(shortDefinition = "Why the request group is needed", formalDefinition = "Indicates another resource whose existence justifies this request group.") 4617 protected List<Reference> reasonReference; 4618 /** 4619 * The actual objects that are the target of the reference (Indicates another 4620 * resource whose existence justifies this request group.) 4621 */ 4622 protected List<Resource> reasonReferenceTarget; 4623 4624 /** 4625 * Provides a mechanism to communicate additional information about the 4626 * response. 4627 */ 4628 @Child(name = "note", type = { 4629 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4630 @Description(shortDefinition = "Additional notes about the response", formalDefinition = "Provides a mechanism to communicate additional information about the response.") 4631 protected List<Annotation> note; 4632 4633 /** 4634 * The actions, if any, produced by the evaluation of the artifact. 4635 */ 4636 @Child(name = "action", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4637 @Description(shortDefinition = "Proposed actions, if any", formalDefinition = "The actions, if any, produced by the evaluation of the artifact.") 4638 protected List<RequestGroupActionComponent> action; 4639 4640 private static final long serialVersionUID = -2053492070L; 4641 4642 /** 4643 * Constructor 4644 */ 4645 public RequestGroup() { 4646 super(); 4647 } 4648 4649 /** 4650 * Constructor 4651 */ 4652 public RequestGroup(Enumeration<RequestStatus> status, Enumeration<RequestIntent> intent) { 4653 super(); 4654 this.status = status; 4655 this.intent = intent; 4656 } 4657 4658 /** 4659 * @return {@link #identifier} (Allows a service to provide a unique, business 4660 * identifier for the request.) 4661 */ 4662 public List<Identifier> getIdentifier() { 4663 if (this.identifier == null) 4664 this.identifier = new ArrayList<Identifier>(); 4665 return this.identifier; 4666 } 4667 4668 /** 4669 * @return Returns a reference to <code>this</code> for easy method chaining 4670 */ 4671 public RequestGroup setIdentifier(List<Identifier> theIdentifier) { 4672 this.identifier = theIdentifier; 4673 return this; 4674 } 4675 4676 public boolean hasIdentifier() { 4677 if (this.identifier == null) 4678 return false; 4679 for (Identifier item : this.identifier) 4680 if (!item.isEmpty()) 4681 return true; 4682 return false; 4683 } 4684 4685 public Identifier addIdentifier() { // 3 4686 Identifier t = new Identifier(); 4687 if (this.identifier == null) 4688 this.identifier = new ArrayList<Identifier>(); 4689 this.identifier.add(t); 4690 return t; 4691 } 4692 4693 public RequestGroup addIdentifier(Identifier t) { // 3 4694 if (t == null) 4695 return this; 4696 if (this.identifier == null) 4697 this.identifier = new ArrayList<Identifier>(); 4698 this.identifier.add(t); 4699 return this; 4700 } 4701 4702 /** 4703 * @return The first repetition of repeating field {@link #identifier}, creating 4704 * it if it does not already exist 4705 */ 4706 public Identifier getIdentifierFirstRep() { 4707 if (getIdentifier().isEmpty()) { 4708 addIdentifier(); 4709 } 4710 return getIdentifier().get(0); 4711 } 4712 4713 /** 4714 * @return {@link #instantiatesCanonical} (A canonical URL referencing a 4715 * FHIR-defined protocol, guideline, orderset or other definition that 4716 * is adhered to in whole or in part by this request.) 4717 */ 4718 public List<CanonicalType> getInstantiatesCanonical() { 4719 if (this.instantiatesCanonical == null) 4720 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4721 return this.instantiatesCanonical; 4722 } 4723 4724 /** 4725 * @return Returns a reference to <code>this</code> for easy method chaining 4726 */ 4727 public RequestGroup setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 4728 this.instantiatesCanonical = theInstantiatesCanonical; 4729 return this; 4730 } 4731 4732 public boolean hasInstantiatesCanonical() { 4733 if (this.instantiatesCanonical == null) 4734 return false; 4735 for (CanonicalType item : this.instantiatesCanonical) 4736 if (!item.isEmpty()) 4737 return true; 4738 return false; 4739 } 4740 4741 /** 4742 * @return {@link #instantiatesCanonical} (A canonical URL referencing a 4743 * FHIR-defined protocol, guideline, orderset or other definition that 4744 * is adhered to in whole or in part by this request.) 4745 */ 4746 public CanonicalType addInstantiatesCanonicalElement() {// 2 4747 CanonicalType t = new CanonicalType(); 4748 if (this.instantiatesCanonical == null) 4749 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4750 this.instantiatesCanonical.add(t); 4751 return t; 4752 } 4753 4754 /** 4755 * @param value {@link #instantiatesCanonical} (A canonical URL referencing a 4756 * FHIR-defined protocol, guideline, orderset or other definition 4757 * that is adhered to in whole or in part by this request.) 4758 */ 4759 public RequestGroup addInstantiatesCanonical(String value) { // 1 4760 CanonicalType t = new CanonicalType(); 4761 t.setValue(value); 4762 if (this.instantiatesCanonical == null) 4763 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 4764 this.instantiatesCanonical.add(t); 4765 return this; 4766 } 4767 4768 /** 4769 * @param value {@link #instantiatesCanonical} (A canonical URL referencing a 4770 * FHIR-defined protocol, guideline, orderset or other definition 4771 * that is adhered to in whole or in part by this request.) 4772 */ 4773 public boolean hasInstantiatesCanonical(String value) { 4774 if (this.instantiatesCanonical == null) 4775 return false; 4776 for (CanonicalType v : this.instantiatesCanonical) 4777 if (v.getValue().equals(value)) // canonical 4778 return true; 4779 return false; 4780 } 4781 4782 /** 4783 * @return {@link #instantiatesUri} (A URL referencing an externally defined 4784 * protocol, guideline, orderset or other definition that is adhered to 4785 * in whole or in part by this request.) 4786 */ 4787 public List<UriType> getInstantiatesUri() { 4788 if (this.instantiatesUri == null) 4789 this.instantiatesUri = new ArrayList<UriType>(); 4790 return this.instantiatesUri; 4791 } 4792 4793 /** 4794 * @return Returns a reference to <code>this</code> for easy method chaining 4795 */ 4796 public RequestGroup setInstantiatesUri(List<UriType> theInstantiatesUri) { 4797 this.instantiatesUri = theInstantiatesUri; 4798 return this; 4799 } 4800 4801 public boolean hasInstantiatesUri() { 4802 if (this.instantiatesUri == null) 4803 return false; 4804 for (UriType item : this.instantiatesUri) 4805 if (!item.isEmpty()) 4806 return true; 4807 return false; 4808 } 4809 4810 /** 4811 * @return {@link #instantiatesUri} (A URL referencing an externally defined 4812 * protocol, guideline, orderset or other definition that is adhered to 4813 * in whole or in part by this request.) 4814 */ 4815 public UriType addInstantiatesUriElement() {// 2 4816 UriType t = new UriType(); 4817 if (this.instantiatesUri == null) 4818 this.instantiatesUri = new ArrayList<UriType>(); 4819 this.instantiatesUri.add(t); 4820 return t; 4821 } 4822 4823 /** 4824 * @param value {@link #instantiatesUri} (A URL referencing an externally 4825 * defined protocol, guideline, orderset or other definition that 4826 * is adhered to in whole or in part by this request.) 4827 */ 4828 public RequestGroup addInstantiatesUri(String value) { // 1 4829 UriType t = new UriType(); 4830 t.setValue(value); 4831 if (this.instantiatesUri == null) 4832 this.instantiatesUri = new ArrayList<UriType>(); 4833 this.instantiatesUri.add(t); 4834 return this; 4835 } 4836 4837 /** 4838 * @param value {@link #instantiatesUri} (A URL referencing an externally 4839 * defined protocol, guideline, orderset or other definition that 4840 * is adhered to in whole or in part by this request.) 4841 */ 4842 public boolean hasInstantiatesUri(String value) { 4843 if (this.instantiatesUri == null) 4844 return false; 4845 for (UriType v : this.instantiatesUri) 4846 if (v.getValue().equals(value)) // uri 4847 return true; 4848 return false; 4849 } 4850 4851 /** 4852 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 4853 * whole or in part by this request.) 4854 */ 4855 public List<Reference> getBasedOn() { 4856 if (this.basedOn == null) 4857 this.basedOn = new ArrayList<Reference>(); 4858 return this.basedOn; 4859 } 4860 4861 /** 4862 * @return Returns a reference to <code>this</code> for easy method chaining 4863 */ 4864 public RequestGroup setBasedOn(List<Reference> theBasedOn) { 4865 this.basedOn = theBasedOn; 4866 return this; 4867 } 4868 4869 public boolean hasBasedOn() { 4870 if (this.basedOn == null) 4871 return false; 4872 for (Reference item : this.basedOn) 4873 if (!item.isEmpty()) 4874 return true; 4875 return false; 4876 } 4877 4878 public Reference addBasedOn() { // 3 4879 Reference t = new Reference(); 4880 if (this.basedOn == null) 4881 this.basedOn = new ArrayList<Reference>(); 4882 this.basedOn.add(t); 4883 return t; 4884 } 4885 4886 public RequestGroup addBasedOn(Reference t) { // 3 4887 if (t == null) 4888 return this; 4889 if (this.basedOn == null) 4890 this.basedOn = new ArrayList<Reference>(); 4891 this.basedOn.add(t); 4892 return this; 4893 } 4894 4895 /** 4896 * @return The first repetition of repeating field {@link #basedOn}, creating it 4897 * if it does not already exist 4898 */ 4899 public Reference getBasedOnFirstRep() { 4900 if (getBasedOn().isEmpty()) { 4901 addBasedOn(); 4902 } 4903 return getBasedOn().get(0); 4904 } 4905 4906 /** 4907 * @return {@link #replaces} (Completed or terminated request(s) whose function 4908 * is taken by this new request.) 4909 */ 4910 public List<Reference> getReplaces() { 4911 if (this.replaces == null) 4912 this.replaces = new ArrayList<Reference>(); 4913 return this.replaces; 4914 } 4915 4916 /** 4917 * @return Returns a reference to <code>this</code> for easy method chaining 4918 */ 4919 public RequestGroup setReplaces(List<Reference> theReplaces) { 4920 this.replaces = theReplaces; 4921 return this; 4922 } 4923 4924 public boolean hasReplaces() { 4925 if (this.replaces == null) 4926 return false; 4927 for (Reference item : this.replaces) 4928 if (!item.isEmpty()) 4929 return true; 4930 return false; 4931 } 4932 4933 public Reference addReplaces() { // 3 4934 Reference t = new Reference(); 4935 if (this.replaces == null) 4936 this.replaces = new ArrayList<Reference>(); 4937 this.replaces.add(t); 4938 return t; 4939 } 4940 4941 public RequestGroup addReplaces(Reference t) { // 3 4942 if (t == null) 4943 return this; 4944 if (this.replaces == null) 4945 this.replaces = new ArrayList<Reference>(); 4946 this.replaces.add(t); 4947 return this; 4948 } 4949 4950 /** 4951 * @return The first repetition of repeating field {@link #replaces}, creating 4952 * it if it does not already exist 4953 */ 4954 public Reference getReplacesFirstRep() { 4955 if (getReplaces().isEmpty()) { 4956 addReplaces(); 4957 } 4958 return getReplaces().get(0); 4959 } 4960 4961 /** 4962 * @return {@link #groupIdentifier} (A shared identifier common to all requests 4963 * that were authorized more or less simultaneously by a single author, 4964 * representing the identifier of the requisition, prescription or 4965 * similar form.) 4966 */ 4967 public Identifier getGroupIdentifier() { 4968 if (this.groupIdentifier == null) 4969 if (Configuration.errorOnAutoCreate()) 4970 throw new Error("Attempt to auto-create RequestGroup.groupIdentifier"); 4971 else if (Configuration.doAutoCreate()) 4972 this.groupIdentifier = new Identifier(); // cc 4973 return this.groupIdentifier; 4974 } 4975 4976 public boolean hasGroupIdentifier() { 4977 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 4978 } 4979 4980 /** 4981 * @param value {@link #groupIdentifier} (A shared identifier common to all 4982 * requests that were authorized more or less simultaneously by a 4983 * single author, representing the identifier of the requisition, 4984 * prescription or similar form.) 4985 */ 4986 public RequestGroup setGroupIdentifier(Identifier value) { 4987 this.groupIdentifier = value; 4988 return this; 4989 } 4990 4991 /** 4992 * @return {@link #status} (The current state of the request. For request 4993 * groups, the status reflects the status of all the requests in the 4994 * group.). This is the underlying object with id, value and extensions. 4995 * The accessor "getStatus" gives direct access to the value 4996 */ 4997 public Enumeration<RequestStatus> getStatusElement() { 4998 if (this.status == null) 4999 if (Configuration.errorOnAutoCreate()) 5000 throw new Error("Attempt to auto-create RequestGroup.status"); 5001 else if (Configuration.doAutoCreate()) 5002 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); // bb 5003 return this.status; 5004 } 5005 5006 public boolean hasStatusElement() { 5007 return this.status != null && !this.status.isEmpty(); 5008 } 5009 5010 public boolean hasStatus() { 5011 return this.status != null && !this.status.isEmpty(); 5012 } 5013 5014 /** 5015 * @param value {@link #status} (The current state of the request. For request 5016 * groups, the status reflects the status of all the requests in 5017 * the group.). This is the underlying object with id, value and 5018 * extensions. The accessor "getStatus" gives direct access to the 5019 * value 5020 */ 5021 public RequestGroup setStatusElement(Enumeration<RequestStatus> value) { 5022 this.status = value; 5023 return this; 5024 } 5025 5026 /** 5027 * @return The current state of the request. For request groups, the status 5028 * reflects the status of all the requests in the group. 5029 */ 5030 public RequestStatus getStatus() { 5031 return this.status == null ? null : this.status.getValue(); 5032 } 5033 5034 /** 5035 * @param value The current state of the request. For request groups, the status 5036 * reflects the status of all the requests in the group. 5037 */ 5038 public RequestGroup setStatus(RequestStatus value) { 5039 if (this.status == null) 5040 this.status = new Enumeration<RequestStatus>(new RequestStatusEnumFactory()); 5041 this.status.setValue(value); 5042 return this; 5043 } 5044 5045 /** 5046 * @return {@link #intent} (Indicates the level of authority/intentionality 5047 * associated with the request and where the request fits into the 5048 * workflow chain.). This is the underlying object with id, value and 5049 * extensions. The accessor "getIntent" gives direct access to the value 5050 */ 5051 public Enumeration<RequestIntent> getIntentElement() { 5052 if (this.intent == null) 5053 if (Configuration.errorOnAutoCreate()) 5054 throw new Error("Attempt to auto-create RequestGroup.intent"); 5055 else if (Configuration.doAutoCreate()) 5056 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); // bb 5057 return this.intent; 5058 } 5059 5060 public boolean hasIntentElement() { 5061 return this.intent != null && !this.intent.isEmpty(); 5062 } 5063 5064 public boolean hasIntent() { 5065 return this.intent != null && !this.intent.isEmpty(); 5066 } 5067 5068 /** 5069 * @param value {@link #intent} (Indicates the level of authority/intentionality 5070 * associated with the request and where the request fits into the 5071 * workflow chain.). This is the underlying object with id, value 5072 * and extensions. The accessor "getIntent" gives direct access to 5073 * the value 5074 */ 5075 public RequestGroup setIntentElement(Enumeration<RequestIntent> value) { 5076 this.intent = value; 5077 return this; 5078 } 5079 5080 /** 5081 * @return Indicates the level of authority/intentionality associated with the 5082 * request and where the request fits into the workflow chain. 5083 */ 5084 public RequestIntent getIntent() { 5085 return this.intent == null ? null : this.intent.getValue(); 5086 } 5087 5088 /** 5089 * @param value Indicates the level of authority/intentionality associated with 5090 * the request and where the request fits into the workflow chain. 5091 */ 5092 public RequestGroup setIntent(RequestIntent value) { 5093 if (this.intent == null) 5094 this.intent = new Enumeration<RequestIntent>(new RequestIntentEnumFactory()); 5095 this.intent.setValue(value); 5096 return this; 5097 } 5098 5099 /** 5100 * @return {@link #priority} (Indicates how quickly the request should be 5101 * addressed with respect to other requests.). This is the underlying 5102 * object with id, value and extensions. The accessor "getPriority" 5103 * gives direct access to the value 5104 */ 5105 public Enumeration<RequestPriority> getPriorityElement() { 5106 if (this.priority == null) 5107 if (Configuration.errorOnAutoCreate()) 5108 throw new Error("Attempt to auto-create RequestGroup.priority"); 5109 else if (Configuration.doAutoCreate()) 5110 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 5111 return this.priority; 5112 } 5113 5114 public boolean hasPriorityElement() { 5115 return this.priority != null && !this.priority.isEmpty(); 5116 } 5117 5118 public boolean hasPriority() { 5119 return this.priority != null && !this.priority.isEmpty(); 5120 } 5121 5122 /** 5123 * @param value {@link #priority} (Indicates how quickly the request should be 5124 * addressed with respect to other requests.). This is the 5125 * underlying object with id, value and extensions. The accessor 5126 * "getPriority" gives direct access to the value 5127 */ 5128 public RequestGroup setPriorityElement(Enumeration<RequestPriority> value) { 5129 this.priority = value; 5130 return this; 5131 } 5132 5133 /** 5134 * @return Indicates how quickly the request should be addressed with respect to 5135 * other requests. 5136 */ 5137 public RequestPriority getPriority() { 5138 return this.priority == null ? null : this.priority.getValue(); 5139 } 5140 5141 /** 5142 * @param value Indicates how quickly the request should be addressed with 5143 * respect to other requests. 5144 */ 5145 public RequestGroup setPriority(RequestPriority value) { 5146 if (value == null) 5147 this.priority = null; 5148 else { 5149 if (this.priority == null) 5150 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 5151 this.priority.setValue(value); 5152 } 5153 return this; 5154 } 5155 5156 /** 5157 * @return {@link #code} (A code that identifies what the overall request group 5158 * is.) 5159 */ 5160 public CodeableConcept getCode() { 5161 if (this.code == null) 5162 if (Configuration.errorOnAutoCreate()) 5163 throw new Error("Attempt to auto-create RequestGroup.code"); 5164 else if (Configuration.doAutoCreate()) 5165 this.code = new CodeableConcept(); // cc 5166 return this.code; 5167 } 5168 5169 public boolean hasCode() { 5170 return this.code != null && !this.code.isEmpty(); 5171 } 5172 5173 /** 5174 * @param value {@link #code} (A code that identifies what the overall request 5175 * group is.) 5176 */ 5177 public RequestGroup setCode(CodeableConcept value) { 5178 this.code = value; 5179 return this; 5180 } 5181 5182 /** 5183 * @return {@link #subject} (The subject for which the request group was 5184 * created.) 5185 */ 5186 public Reference getSubject() { 5187 if (this.subject == null) 5188 if (Configuration.errorOnAutoCreate()) 5189 throw new Error("Attempt to auto-create RequestGroup.subject"); 5190 else if (Configuration.doAutoCreate()) 5191 this.subject = new Reference(); // cc 5192 return this.subject; 5193 } 5194 5195 public boolean hasSubject() { 5196 return this.subject != null && !this.subject.isEmpty(); 5197 } 5198 5199 /** 5200 * @param value {@link #subject} (The subject for which the request group was 5201 * created.) 5202 */ 5203 public RequestGroup setSubject(Reference value) { 5204 this.subject = value; 5205 return this; 5206 } 5207 5208 /** 5209 * @return {@link #subject} The actual object that is the target of the 5210 * reference. The reference library doesn't populate this, but you can 5211 * use it to hold the resource if you resolve it. (The subject for which 5212 * the request group was created.) 5213 */ 5214 public Resource getSubjectTarget() { 5215 return this.subjectTarget; 5216 } 5217 5218 /** 5219 * @param value {@link #subject} The actual object that is the target of the 5220 * reference. The reference library doesn't use these, but you can 5221 * use it to hold the resource if you resolve it. (The subject for 5222 * which the request group was created.) 5223 */ 5224 public RequestGroup setSubjectTarget(Resource value) { 5225 this.subjectTarget = value; 5226 return this; 5227 } 5228 5229 /** 5230 * @return {@link #encounter} (Describes the context of the request group, if 5231 * any.) 5232 */ 5233 public Reference getEncounter() { 5234 if (this.encounter == null) 5235 if (Configuration.errorOnAutoCreate()) 5236 throw new Error("Attempt to auto-create RequestGroup.encounter"); 5237 else if (Configuration.doAutoCreate()) 5238 this.encounter = new Reference(); // cc 5239 return this.encounter; 5240 } 5241 5242 public boolean hasEncounter() { 5243 return this.encounter != null && !this.encounter.isEmpty(); 5244 } 5245 5246 /** 5247 * @param value {@link #encounter} (Describes the context of the request group, 5248 * if any.) 5249 */ 5250 public RequestGroup setEncounter(Reference value) { 5251 this.encounter = value; 5252 return this; 5253 } 5254 5255 /** 5256 * @return {@link #encounter} The actual object that is the target of the 5257 * reference. The reference library doesn't populate this, but you can 5258 * use it to hold the resource if you resolve it. (Describes the context 5259 * of the request group, if any.) 5260 */ 5261 public Encounter getEncounterTarget() { 5262 if (this.encounterTarget == null) 5263 if (Configuration.errorOnAutoCreate()) 5264 throw new Error("Attempt to auto-create RequestGroup.encounter"); 5265 else if (Configuration.doAutoCreate()) 5266 this.encounterTarget = new Encounter(); // aa 5267 return this.encounterTarget; 5268 } 5269 5270 /** 5271 * @param value {@link #encounter} The actual object that is the target of the 5272 * reference. The reference library doesn't use these, but you can 5273 * use it to hold the resource if you resolve it. (Describes the 5274 * context of the request group, if any.) 5275 */ 5276 public RequestGroup setEncounterTarget(Encounter value) { 5277 this.encounterTarget = value; 5278 return this; 5279 } 5280 5281 /** 5282 * @return {@link #authoredOn} (Indicates when the request group was created.). 5283 * This is the underlying object with id, value and extensions. The 5284 * accessor "getAuthoredOn" gives direct access to the value 5285 */ 5286 public DateTimeType getAuthoredOnElement() { 5287 if (this.authoredOn == null) 5288 if (Configuration.errorOnAutoCreate()) 5289 throw new Error("Attempt to auto-create RequestGroup.authoredOn"); 5290 else if (Configuration.doAutoCreate()) 5291 this.authoredOn = new DateTimeType(); // bb 5292 return this.authoredOn; 5293 } 5294 5295 public boolean hasAuthoredOnElement() { 5296 return this.authoredOn != null && !this.authoredOn.isEmpty(); 5297 } 5298 5299 public boolean hasAuthoredOn() { 5300 return this.authoredOn != null && !this.authoredOn.isEmpty(); 5301 } 5302 5303 /** 5304 * @param value {@link #authoredOn} (Indicates when the request group was 5305 * created.). This is the underlying object with id, value and 5306 * extensions. The accessor "getAuthoredOn" gives direct access to 5307 * the value 5308 */ 5309 public RequestGroup setAuthoredOnElement(DateTimeType value) { 5310 this.authoredOn = value; 5311 return this; 5312 } 5313 5314 /** 5315 * @return Indicates when the request group was created. 5316 */ 5317 public Date getAuthoredOn() { 5318 return this.authoredOn == null ? null : this.authoredOn.getValue(); 5319 } 5320 5321 /** 5322 * @param value Indicates when the request group was created. 5323 */ 5324 public RequestGroup setAuthoredOn(Date value) { 5325 if (value == null) 5326 this.authoredOn = null; 5327 else { 5328 if (this.authoredOn == null) 5329 this.authoredOn = new DateTimeType(); 5330 this.authoredOn.setValue(value); 5331 } 5332 return this; 5333 } 5334 5335 /** 5336 * @return {@link #author} (Provides a reference to the author of the request 5337 * group.) 5338 */ 5339 public Reference getAuthor() { 5340 if (this.author == null) 5341 if (Configuration.errorOnAutoCreate()) 5342 throw new Error("Attempt to auto-create RequestGroup.author"); 5343 else if (Configuration.doAutoCreate()) 5344 this.author = new Reference(); // cc 5345 return this.author; 5346 } 5347 5348 public boolean hasAuthor() { 5349 return this.author != null && !this.author.isEmpty(); 5350 } 5351 5352 /** 5353 * @param value {@link #author} (Provides a reference to the author of the 5354 * request group.) 5355 */ 5356 public RequestGroup setAuthor(Reference value) { 5357 this.author = value; 5358 return this; 5359 } 5360 5361 /** 5362 * @return {@link #author} The actual object that is the target of the 5363 * reference. The reference library doesn't populate this, but you can 5364 * use it to hold the resource if you resolve it. (Provides a reference 5365 * to the author of the request group.) 5366 */ 5367 public Resource getAuthorTarget() { 5368 return this.authorTarget; 5369 } 5370 5371 /** 5372 * @param value {@link #author} The actual object that is the target of the 5373 * reference. The reference library doesn't use these, but you can 5374 * use it to hold the resource if you resolve it. (Provides a 5375 * reference to the author of the request group.) 5376 */ 5377 public RequestGroup setAuthorTarget(Resource value) { 5378 this.authorTarget = value; 5379 return this; 5380 } 5381 5382 /** 5383 * @return {@link #reasonCode} (Describes the reason for the request group in 5384 * coded or textual form.) 5385 */ 5386 public List<CodeableConcept> getReasonCode() { 5387 if (this.reasonCode == null) 5388 this.reasonCode = new ArrayList<CodeableConcept>(); 5389 return this.reasonCode; 5390 } 5391 5392 /** 5393 * @return Returns a reference to <code>this</code> for easy method chaining 5394 */ 5395 public RequestGroup setReasonCode(List<CodeableConcept> theReasonCode) { 5396 this.reasonCode = theReasonCode; 5397 return this; 5398 } 5399 5400 public boolean hasReasonCode() { 5401 if (this.reasonCode == null) 5402 return false; 5403 for (CodeableConcept item : this.reasonCode) 5404 if (!item.isEmpty()) 5405 return true; 5406 return false; 5407 } 5408 5409 public CodeableConcept addReasonCode() { // 3 5410 CodeableConcept t = new CodeableConcept(); 5411 if (this.reasonCode == null) 5412 this.reasonCode = new ArrayList<CodeableConcept>(); 5413 this.reasonCode.add(t); 5414 return t; 5415 } 5416 5417 public RequestGroup addReasonCode(CodeableConcept t) { // 3 5418 if (t == null) 5419 return this; 5420 if (this.reasonCode == null) 5421 this.reasonCode = new ArrayList<CodeableConcept>(); 5422 this.reasonCode.add(t); 5423 return this; 5424 } 5425 5426 /** 5427 * @return The first repetition of repeating field {@link #reasonCode}, creating 5428 * it if it does not already exist 5429 */ 5430 public CodeableConcept getReasonCodeFirstRep() { 5431 if (getReasonCode().isEmpty()) { 5432 addReasonCode(); 5433 } 5434 return getReasonCode().get(0); 5435 } 5436 5437 /** 5438 * @return {@link #reasonReference} (Indicates another resource whose existence 5439 * justifies this request group.) 5440 */ 5441 public List<Reference> getReasonReference() { 5442 if (this.reasonReference == null) 5443 this.reasonReference = new ArrayList<Reference>(); 5444 return this.reasonReference; 5445 } 5446 5447 /** 5448 * @return Returns a reference to <code>this</code> for easy method chaining 5449 */ 5450 public RequestGroup setReasonReference(List<Reference> theReasonReference) { 5451 this.reasonReference = theReasonReference; 5452 return this; 5453 } 5454 5455 public boolean hasReasonReference() { 5456 if (this.reasonReference == null) 5457 return false; 5458 for (Reference item : this.reasonReference) 5459 if (!item.isEmpty()) 5460 return true; 5461 return false; 5462 } 5463 5464 public Reference addReasonReference() { // 3 5465 Reference t = new Reference(); 5466 if (this.reasonReference == null) 5467 this.reasonReference = new ArrayList<Reference>(); 5468 this.reasonReference.add(t); 5469 return t; 5470 } 5471 5472 public RequestGroup addReasonReference(Reference t) { // 3 5473 if (t == null) 5474 return this; 5475 if (this.reasonReference == null) 5476 this.reasonReference = new ArrayList<Reference>(); 5477 this.reasonReference.add(t); 5478 return this; 5479 } 5480 5481 /** 5482 * @return The first repetition of repeating field {@link #reasonReference}, 5483 * creating it if it does not already exist 5484 */ 5485 public Reference getReasonReferenceFirstRep() { 5486 if (getReasonReference().isEmpty()) { 5487 addReasonReference(); 5488 } 5489 return getReasonReference().get(0); 5490 } 5491 5492 /** 5493 * @return {@link #note} (Provides a mechanism to communicate additional 5494 * information about the response.) 5495 */ 5496 public List<Annotation> getNote() { 5497 if (this.note == null) 5498 this.note = new ArrayList<Annotation>(); 5499 return this.note; 5500 } 5501 5502 /** 5503 * @return Returns a reference to <code>this</code> for easy method chaining 5504 */ 5505 public RequestGroup setNote(List<Annotation> theNote) { 5506 this.note = theNote; 5507 return this; 5508 } 5509 5510 public boolean hasNote() { 5511 if (this.note == null) 5512 return false; 5513 for (Annotation item : this.note) 5514 if (!item.isEmpty()) 5515 return true; 5516 return false; 5517 } 5518 5519 public Annotation addNote() { // 3 5520 Annotation t = new Annotation(); 5521 if (this.note == null) 5522 this.note = new ArrayList<Annotation>(); 5523 this.note.add(t); 5524 return t; 5525 } 5526 5527 public RequestGroup addNote(Annotation t) { // 3 5528 if (t == null) 5529 return this; 5530 if (this.note == null) 5531 this.note = new ArrayList<Annotation>(); 5532 this.note.add(t); 5533 return this; 5534 } 5535 5536 /** 5537 * @return The first repetition of repeating field {@link #note}, creating it if 5538 * it does not already exist 5539 */ 5540 public Annotation getNoteFirstRep() { 5541 if (getNote().isEmpty()) { 5542 addNote(); 5543 } 5544 return getNote().get(0); 5545 } 5546 5547 /** 5548 * @return {@link #action} (The actions, if any, produced by the evaluation of 5549 * the artifact.) 5550 */ 5551 public List<RequestGroupActionComponent> getAction() { 5552 if (this.action == null) 5553 this.action = new ArrayList<RequestGroupActionComponent>(); 5554 return this.action; 5555 } 5556 5557 /** 5558 * @return Returns a reference to <code>this</code> for easy method chaining 5559 */ 5560 public RequestGroup setAction(List<RequestGroupActionComponent> theAction) { 5561 this.action = theAction; 5562 return this; 5563 } 5564 5565 public boolean hasAction() { 5566 if (this.action == null) 5567 return false; 5568 for (RequestGroupActionComponent item : this.action) 5569 if (!item.isEmpty()) 5570 return true; 5571 return false; 5572 } 5573 5574 public RequestGroupActionComponent addAction() { // 3 5575 RequestGroupActionComponent t = new RequestGroupActionComponent(); 5576 if (this.action == null) 5577 this.action = new ArrayList<RequestGroupActionComponent>(); 5578 this.action.add(t); 5579 return t; 5580 } 5581 5582 public RequestGroup addAction(RequestGroupActionComponent t) { // 3 5583 if (t == null) 5584 return this; 5585 if (this.action == null) 5586 this.action = new ArrayList<RequestGroupActionComponent>(); 5587 this.action.add(t); 5588 return this; 5589 } 5590 5591 /** 5592 * @return The first repetition of repeating field {@link #action}, creating it 5593 * if it does not already exist 5594 */ 5595 public RequestGroupActionComponent getActionFirstRep() { 5596 if (getAction().isEmpty()) { 5597 addAction(); 5598 } 5599 return getAction().get(0); 5600 } 5601 5602 protected void listChildren(List<Property> children) { 5603 super.listChildren(children); 5604 children.add(new Property("identifier", "Identifier", 5605 "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, 5606 identifier)); 5607 children.add(new Property("instantiatesCanonical", "canonical", 5608 "A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 5609 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 5610 children.add(new Property("instantiatesUri", "uri", 5611 "A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 5612 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 5613 children.add(new Property("basedOn", "Reference(Any)", 5614 "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, 5615 java.lang.Integer.MAX_VALUE, basedOn)); 5616 children.add(new Property("replaces", "Reference(Any)", 5617 "Completed or terminated request(s) whose function is taken by this new request.", 0, 5618 java.lang.Integer.MAX_VALUE, replaces)); 5619 children.add(new Property("groupIdentifier", "Identifier", 5620 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 5621 0, 1, groupIdentifier)); 5622 children.add(new Property("status", "code", 5623 "The current state of the request. For request groups, the status reflects the status of all the requests in the group.", 5624 0, 1, status)); 5625 children.add(new Property("intent", "code", 5626 "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 5627 0, 1, intent)); 5628 children.add(new Property("priority", "code", 5629 "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority)); 5630 children.add(new Property("code", "CodeableConcept", "A code that identifies what the overall request group is.", 0, 5631 1, code)); 5632 children.add(new Property("subject", "Reference(Patient|Group)", 5633 "The subject for which the request group was created.", 0, 1, subject)); 5634 children.add(new Property("encounter", "Reference(Encounter)", 5635 "Describes the context of the request group, if any.", 0, 1, encounter)); 5636 children 5637 .add(new Property("authoredOn", "dateTime", "Indicates when the request group was created.", 0, 1, authoredOn)); 5638 children.add(new Property("author", "Reference(Device|Practitioner|PractitionerRole)", 5639 "Provides a reference to the author of the request group.", 0, 1, author)); 5640 children.add(new Property("reasonCode", "CodeableConcept", 5641 "Describes the reason for the request group in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 5642 reasonCode)); 5643 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 5644 "Indicates another resource whose existence justifies this request group.", 0, java.lang.Integer.MAX_VALUE, 5645 reasonReference)); 5646 children.add(new Property("note", "Annotation", 5647 "Provides a mechanism to communicate additional information about the response.", 0, 5648 java.lang.Integer.MAX_VALUE, note)); 5649 children.add(new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 0, 5650 java.lang.Integer.MAX_VALUE, action)); 5651 } 5652 5653 @Override 5654 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5655 switch (_hash) { 5656 case -1618432855: 5657 /* identifier */ return new Property("identifier", "Identifier", 5658 "Allows a service to provide a unique, business identifier for the request.", 0, java.lang.Integer.MAX_VALUE, 5659 identifier); 5660 case 8911915: 5661 /* instantiatesCanonical */ return new Property("instantiatesCanonical", "canonical", 5662 "A canonical URL referencing a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 5663 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 5664 case -1926393373: 5665 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 5666 "A URL referencing an externally defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this request.", 5667 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 5668 case -332612366: 5669 /* basedOn */ return new Property("basedOn", "Reference(Any)", 5670 "A plan, proposal or order that is fulfilled in whole or in part by this request.", 0, 5671 java.lang.Integer.MAX_VALUE, basedOn); 5672 case -430332865: 5673 /* replaces */ return new Property("replaces", "Reference(Any)", 5674 "Completed or terminated request(s) whose function is taken by this new request.", 0, 5675 java.lang.Integer.MAX_VALUE, replaces); 5676 case -445338488: 5677 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", 5678 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 5679 0, 1, groupIdentifier); 5680 case -892481550: 5681 /* status */ return new Property("status", "code", 5682 "The current state of the request. For request groups, the status reflects the status of all the requests in the group.", 5683 0, 1, status); 5684 case -1183762788: 5685 /* intent */ return new Property("intent", "code", 5686 "Indicates the level of authority/intentionality associated with the request and where the request fits into the workflow chain.", 5687 0, 1, intent); 5688 case -1165461084: 5689 /* priority */ return new Property("priority", "code", 5690 "Indicates how quickly the request should be addressed with respect to other requests.", 0, 1, priority); 5691 case 3059181: 5692 /* code */ return new Property("code", "CodeableConcept", 5693 "A code that identifies what the overall request group is.", 0, 1, code); 5694 case -1867885268: 5695 /* subject */ return new Property("subject", "Reference(Patient|Group)", 5696 "The subject for which the request group was created.", 0, 1, subject); 5697 case 1524132147: 5698 /* encounter */ return new Property("encounter", "Reference(Encounter)", 5699 "Describes the context of the request group, if any.", 0, 1, encounter); 5700 case -1500852503: 5701 /* authoredOn */ return new Property("authoredOn", "dateTime", "Indicates when the request group was created.", 0, 5702 1, authoredOn); 5703 case -1406328437: 5704 /* author */ return new Property("author", "Reference(Device|Practitioner|PractitionerRole)", 5705 "Provides a reference to the author of the request group.", 0, 1, author); 5706 case 722137681: 5707 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 5708 "Describes the reason for the request group in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 5709 reasonCode); 5710 case -1146218137: 5711 /* reasonReference */ return new Property("reasonReference", 5712 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 5713 "Indicates another resource whose existence justifies this request group.", 0, java.lang.Integer.MAX_VALUE, 5714 reasonReference); 5715 case 3387378: 5716 /* note */ return new Property("note", "Annotation", 5717 "Provides a mechanism to communicate additional information about the response.", 0, 5718 java.lang.Integer.MAX_VALUE, note); 5719 case -1422950858: 5720 /* action */ return new Property("action", "", "The actions, if any, produced by the evaluation of the artifact.", 5721 0, java.lang.Integer.MAX_VALUE, action); 5722 default: 5723 return super.getNamedProperty(_hash, _name, _checkValid); 5724 } 5725 5726 } 5727 5728 @Override 5729 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5730 switch (hash) { 5731 case -1618432855: 5732 /* identifier */ return this.identifier == null ? new Base[0] 5733 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5734 case 8911915: 5735 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 5736 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 5737 case -1926393373: 5738 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 5739 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 5740 case -332612366: 5741 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 5742 case -430332865: 5743 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 5744 case -445338488: 5745 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 5746 case -892481550: 5747 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<RequestStatus> 5748 case -1183762788: 5749 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<RequestIntent> 5750 case -1165461084: 5751 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 5752 case 3059181: 5753 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 5754 case -1867885268: 5755 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 5756 case 1524132147: 5757 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 5758 case -1500852503: 5759 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 5760 case -1406328437: 5761 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 5762 case 722137681: 5763 /* reasonCode */ return this.reasonCode == null ? new Base[0] 5764 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 5765 case -1146218137: 5766 /* reasonReference */ return this.reasonReference == null ? new Base[0] 5767 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 5768 case 3387378: 5769 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 5770 case -1422950858: 5771 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // RequestGroupActionComponent 5772 default: 5773 return super.getProperty(hash, name, checkValid); 5774 } 5775 5776 } 5777 5778 @Override 5779 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5780 switch (hash) { 5781 case -1618432855: // identifier 5782 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5783 return value; 5784 case 8911915: // instantiatesCanonical 5785 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 5786 return value; 5787 case -1926393373: // instantiatesUri 5788 this.getInstantiatesUri().add(castToUri(value)); // UriType 5789 return value; 5790 case -332612366: // basedOn 5791 this.getBasedOn().add(castToReference(value)); // Reference 5792 return value; 5793 case -430332865: // replaces 5794 this.getReplaces().add(castToReference(value)); // Reference 5795 return value; 5796 case -445338488: // groupIdentifier 5797 this.groupIdentifier = castToIdentifier(value); // Identifier 5798 return value; 5799 case -892481550: // status 5800 value = new RequestStatusEnumFactory().fromType(castToCode(value)); 5801 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5802 return value; 5803 case -1183762788: // intent 5804 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 5805 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5806 return value; 5807 case -1165461084: // priority 5808 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 5809 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5810 return value; 5811 case 3059181: // code 5812 this.code = castToCodeableConcept(value); // CodeableConcept 5813 return value; 5814 case -1867885268: // subject 5815 this.subject = castToReference(value); // Reference 5816 return value; 5817 case 1524132147: // encounter 5818 this.encounter = castToReference(value); // Reference 5819 return value; 5820 case -1500852503: // authoredOn 5821 this.authoredOn = castToDateTime(value); // DateTimeType 5822 return value; 5823 case -1406328437: // author 5824 this.author = castToReference(value); // Reference 5825 return value; 5826 case 722137681: // reasonCode 5827 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 5828 return value; 5829 case -1146218137: // reasonReference 5830 this.getReasonReference().add(castToReference(value)); // Reference 5831 return value; 5832 case 3387378: // note 5833 this.getNote().add(castToAnnotation(value)); // Annotation 5834 return value; 5835 case -1422950858: // action 5836 this.getAction().add((RequestGroupActionComponent) value); // RequestGroupActionComponent 5837 return value; 5838 default: 5839 return super.setProperty(hash, name, value); 5840 } 5841 5842 } 5843 5844 @Override 5845 public Base setProperty(String name, Base value) throws FHIRException { 5846 if (name.equals("identifier")) { 5847 this.getIdentifier().add(castToIdentifier(value)); 5848 } else if (name.equals("instantiatesCanonical")) { 5849 this.getInstantiatesCanonical().add(castToCanonical(value)); 5850 } else if (name.equals("instantiatesUri")) { 5851 this.getInstantiatesUri().add(castToUri(value)); 5852 } else if (name.equals("basedOn")) { 5853 this.getBasedOn().add(castToReference(value)); 5854 } else if (name.equals("replaces")) { 5855 this.getReplaces().add(castToReference(value)); 5856 } else if (name.equals("groupIdentifier")) { 5857 this.groupIdentifier = castToIdentifier(value); // Identifier 5858 } else if (name.equals("status")) { 5859 value = new RequestStatusEnumFactory().fromType(castToCode(value)); 5860 this.status = (Enumeration) value; // Enumeration<RequestStatus> 5861 } else if (name.equals("intent")) { 5862 value = new RequestIntentEnumFactory().fromType(castToCode(value)); 5863 this.intent = (Enumeration) value; // Enumeration<RequestIntent> 5864 } else if (name.equals("priority")) { 5865 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 5866 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 5867 } else if (name.equals("code")) { 5868 this.code = castToCodeableConcept(value); // CodeableConcept 5869 } else if (name.equals("subject")) { 5870 this.subject = castToReference(value); // Reference 5871 } else if (name.equals("encounter")) { 5872 this.encounter = castToReference(value); // Reference 5873 } else if (name.equals("authoredOn")) { 5874 this.authoredOn = castToDateTime(value); // DateTimeType 5875 } else if (name.equals("author")) { 5876 this.author = castToReference(value); // Reference 5877 } else if (name.equals("reasonCode")) { 5878 this.getReasonCode().add(castToCodeableConcept(value)); 5879 } else if (name.equals("reasonReference")) { 5880 this.getReasonReference().add(castToReference(value)); 5881 } else if (name.equals("note")) { 5882 this.getNote().add(castToAnnotation(value)); 5883 } else if (name.equals("action")) { 5884 this.getAction().add((RequestGroupActionComponent) value); 5885 } else 5886 return super.setProperty(name, value); 5887 return value; 5888 } 5889 5890 @Override 5891 public void removeChild(String name, Base value) throws FHIRException { 5892 if (name.equals("identifier")) { 5893 this.getIdentifier().remove(castToIdentifier(value)); 5894 } else if (name.equals("instantiatesCanonical")) { 5895 this.getInstantiatesCanonical().remove(castToCanonical(value)); 5896 } else if (name.equals("instantiatesUri")) { 5897 this.getInstantiatesUri().remove(castToUri(value)); 5898 } else if (name.equals("basedOn")) { 5899 this.getBasedOn().remove(castToReference(value)); 5900 } else if (name.equals("replaces")) { 5901 this.getReplaces().remove(castToReference(value)); 5902 } else if (name.equals("groupIdentifier")) { 5903 this.groupIdentifier = null; 5904 } else if (name.equals("status")) { 5905 this.status = null; 5906 } else if (name.equals("intent")) { 5907 this.intent = null; 5908 } else if (name.equals("priority")) { 5909 this.priority = null; 5910 } else if (name.equals("code")) { 5911 this.code = null; 5912 } else if (name.equals("subject")) { 5913 this.subject = null; 5914 } else if (name.equals("encounter")) { 5915 this.encounter = null; 5916 } else if (name.equals("authoredOn")) { 5917 this.authoredOn = null; 5918 } else if (name.equals("author")) { 5919 this.author = null; 5920 } else if (name.equals("reasonCode")) { 5921 this.getReasonCode().remove(castToCodeableConcept(value)); 5922 } else if (name.equals("reasonReference")) { 5923 this.getReasonReference().remove(castToReference(value)); 5924 } else if (name.equals("note")) { 5925 this.getNote().remove(castToAnnotation(value)); 5926 } else if (name.equals("action")) { 5927 this.getAction().remove((RequestGroupActionComponent) value); 5928 } else 5929 super.removeChild(name, value); 5930 5931 } 5932 5933 @Override 5934 public Base makeProperty(int hash, String name) throws FHIRException { 5935 switch (hash) { 5936 case -1618432855: 5937 return addIdentifier(); 5938 case 8911915: 5939 return addInstantiatesCanonicalElement(); 5940 case -1926393373: 5941 return addInstantiatesUriElement(); 5942 case -332612366: 5943 return addBasedOn(); 5944 case -430332865: 5945 return addReplaces(); 5946 case -445338488: 5947 return getGroupIdentifier(); 5948 case -892481550: 5949 return getStatusElement(); 5950 case -1183762788: 5951 return getIntentElement(); 5952 case -1165461084: 5953 return getPriorityElement(); 5954 case 3059181: 5955 return getCode(); 5956 case -1867885268: 5957 return getSubject(); 5958 case 1524132147: 5959 return getEncounter(); 5960 case -1500852503: 5961 return getAuthoredOnElement(); 5962 case -1406328437: 5963 return getAuthor(); 5964 case 722137681: 5965 return addReasonCode(); 5966 case -1146218137: 5967 return addReasonReference(); 5968 case 3387378: 5969 return addNote(); 5970 case -1422950858: 5971 return addAction(); 5972 default: 5973 return super.makeProperty(hash, name); 5974 } 5975 5976 } 5977 5978 @Override 5979 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5980 switch (hash) { 5981 case -1618432855: 5982 /* identifier */ return new String[] { "Identifier" }; 5983 case 8911915: 5984 /* instantiatesCanonical */ return new String[] { "canonical" }; 5985 case -1926393373: 5986 /* instantiatesUri */ return new String[] { "uri" }; 5987 case -332612366: 5988 /* basedOn */ return new String[] { "Reference" }; 5989 case -430332865: 5990 /* replaces */ return new String[] { "Reference" }; 5991 case -445338488: 5992 /* groupIdentifier */ return new String[] { "Identifier" }; 5993 case -892481550: 5994 /* status */ return new String[] { "code" }; 5995 case -1183762788: 5996 /* intent */ return new String[] { "code" }; 5997 case -1165461084: 5998 /* priority */ return new String[] { "code" }; 5999 case 3059181: 6000 /* code */ return new String[] { "CodeableConcept" }; 6001 case -1867885268: 6002 /* subject */ return new String[] { "Reference" }; 6003 case 1524132147: 6004 /* encounter */ return new String[] { "Reference" }; 6005 case -1500852503: 6006 /* authoredOn */ return new String[] { "dateTime" }; 6007 case -1406328437: 6008 /* author */ return new String[] { "Reference" }; 6009 case 722137681: 6010 /* reasonCode */ return new String[] { "CodeableConcept" }; 6011 case -1146218137: 6012 /* reasonReference */ return new String[] { "Reference" }; 6013 case 3387378: 6014 /* note */ return new String[] { "Annotation" }; 6015 case -1422950858: 6016 /* action */ return new String[] {}; 6017 default: 6018 return super.getTypesForProperty(hash, name); 6019 } 6020 6021 } 6022 6023 @Override 6024 public Base addChild(String name) throws FHIRException { 6025 if (name.equals("identifier")) { 6026 return addIdentifier(); 6027 } else if (name.equals("instantiatesCanonical")) { 6028 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.instantiatesCanonical"); 6029 } else if (name.equals("instantiatesUri")) { 6030 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.instantiatesUri"); 6031 } else if (name.equals("basedOn")) { 6032 return addBasedOn(); 6033 } else if (name.equals("replaces")) { 6034 return addReplaces(); 6035 } else if (name.equals("groupIdentifier")) { 6036 this.groupIdentifier = new Identifier(); 6037 return this.groupIdentifier; 6038 } else if (name.equals("status")) { 6039 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.status"); 6040 } else if (name.equals("intent")) { 6041 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.intent"); 6042 } else if (name.equals("priority")) { 6043 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.priority"); 6044 } else if (name.equals("code")) { 6045 this.code = new CodeableConcept(); 6046 return this.code; 6047 } else if (name.equals("subject")) { 6048 this.subject = new Reference(); 6049 return this.subject; 6050 } else if (name.equals("encounter")) { 6051 this.encounter = new Reference(); 6052 return this.encounter; 6053 } else if (name.equals("authoredOn")) { 6054 throw new FHIRException("Cannot call addChild on a singleton property RequestGroup.authoredOn"); 6055 } else if (name.equals("author")) { 6056 this.author = new Reference(); 6057 return this.author; 6058 } else if (name.equals("reasonCode")) { 6059 return addReasonCode(); 6060 } else if (name.equals("reasonReference")) { 6061 return addReasonReference(); 6062 } else if (name.equals("note")) { 6063 return addNote(); 6064 } else if (name.equals("action")) { 6065 return addAction(); 6066 } else 6067 return super.addChild(name); 6068 } 6069 6070 public String fhirType() { 6071 return "RequestGroup"; 6072 6073 } 6074 6075 public RequestGroup copy() { 6076 RequestGroup dst = new RequestGroup(); 6077 copyValues(dst); 6078 return dst; 6079 } 6080 6081 public void copyValues(RequestGroup dst) { 6082 super.copyValues(dst); 6083 if (identifier != null) { 6084 dst.identifier = new ArrayList<Identifier>(); 6085 for (Identifier i : identifier) 6086 dst.identifier.add(i.copy()); 6087 } 6088 ; 6089 if (instantiatesCanonical != null) { 6090 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 6091 for (CanonicalType i : instantiatesCanonical) 6092 dst.instantiatesCanonical.add(i.copy()); 6093 } 6094 ; 6095 if (instantiatesUri != null) { 6096 dst.instantiatesUri = new ArrayList<UriType>(); 6097 for (UriType i : instantiatesUri) 6098 dst.instantiatesUri.add(i.copy()); 6099 } 6100 ; 6101 if (basedOn != null) { 6102 dst.basedOn = new ArrayList<Reference>(); 6103 for (Reference i : basedOn) 6104 dst.basedOn.add(i.copy()); 6105 } 6106 ; 6107 if (replaces != null) { 6108 dst.replaces = new ArrayList<Reference>(); 6109 for (Reference i : replaces) 6110 dst.replaces.add(i.copy()); 6111 } 6112 ; 6113 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 6114 dst.status = status == null ? null : status.copy(); 6115 dst.intent = intent == null ? null : intent.copy(); 6116 dst.priority = priority == null ? null : priority.copy(); 6117 dst.code = code == null ? null : code.copy(); 6118 dst.subject = subject == null ? null : subject.copy(); 6119 dst.encounter = encounter == null ? null : encounter.copy(); 6120 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 6121 dst.author = author == null ? null : author.copy(); 6122 if (reasonCode != null) { 6123 dst.reasonCode = new ArrayList<CodeableConcept>(); 6124 for (CodeableConcept i : reasonCode) 6125 dst.reasonCode.add(i.copy()); 6126 } 6127 ; 6128 if (reasonReference != null) { 6129 dst.reasonReference = new ArrayList<Reference>(); 6130 for (Reference i : reasonReference) 6131 dst.reasonReference.add(i.copy()); 6132 } 6133 ; 6134 if (note != null) { 6135 dst.note = new ArrayList<Annotation>(); 6136 for (Annotation i : note) 6137 dst.note.add(i.copy()); 6138 } 6139 ; 6140 if (action != null) { 6141 dst.action = new ArrayList<RequestGroupActionComponent>(); 6142 for (RequestGroupActionComponent i : action) 6143 dst.action.add(i.copy()); 6144 } 6145 ; 6146 } 6147 6148 protected RequestGroup typedCopy() { 6149 return copy(); 6150 } 6151 6152 @Override 6153 public boolean equalsDeep(Base other_) { 6154 if (!super.equalsDeep(other_)) 6155 return false; 6156 if (!(other_ instanceof RequestGroup)) 6157 return false; 6158 RequestGroup o = (RequestGroup) other_; 6159 return compareDeep(identifier, o.identifier, true) 6160 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 6161 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 6162 && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 6163 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) 6164 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 6165 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 6166 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(author, o.author, true) 6167 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 6168 && compareDeep(note, o.note, true) && compareDeep(action, o.action, true); 6169 } 6170 6171 @Override 6172 public boolean equalsShallow(Base other_) { 6173 if (!super.equalsShallow(other_)) 6174 return false; 6175 if (!(other_ instanceof RequestGroup)) 6176 return false; 6177 RequestGroup o = (RequestGroup) other_; 6178 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) 6179 && compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 6180 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 6181 && compareValues(authoredOn, o.authoredOn, true); 6182 } 6183 6184 public boolean isEmpty() { 6185 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 6186 basedOn, replaces, groupIdentifier, status, intent, priority, code, subject, encounter, authoredOn, author, 6187 reasonCode, reasonReference, note, action); 6188 } 6189 6190 @Override 6191 public ResourceType getResourceType() { 6192 return ResourceType.RequestGroup; 6193 } 6194 6195 /** 6196 * Search parameter: <b>authored</b> 6197 * <p> 6198 * Description: <b>The date the request group was authored</b><br> 6199 * Type: <b>date</b><br> 6200 * Path: <b>RequestGroup.authoredOn</b><br> 6201 * </p> 6202 */ 6203 @SearchParamDefinition(name = "authored", path = "RequestGroup.authoredOn", description = "The date the request group was authored", type = "date") 6204 public static final String SP_AUTHORED = "authored"; 6205 /** 6206 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 6207 * <p> 6208 * Description: <b>The date the request group was authored</b><br> 6209 * Type: <b>date</b><br> 6210 * Path: <b>RequestGroup.authoredOn</b><br> 6211 * </p> 6212 */ 6213 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam( 6214 SP_AUTHORED); 6215 6216 /** 6217 * Search parameter: <b>identifier</b> 6218 * <p> 6219 * Description: <b>External identifiers for the request group</b><br> 6220 * Type: <b>token</b><br> 6221 * Path: <b>RequestGroup.identifier</b><br> 6222 * </p> 6223 */ 6224 @SearchParamDefinition(name = "identifier", path = "RequestGroup.identifier", description = "External identifiers for the request group", type = "token") 6225 public static final String SP_IDENTIFIER = "identifier"; 6226 /** 6227 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6228 * <p> 6229 * Description: <b>External identifiers for the request group</b><br> 6230 * Type: <b>token</b><br> 6231 * Path: <b>RequestGroup.identifier</b><br> 6232 * </p> 6233 */ 6234 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6235 SP_IDENTIFIER); 6236 6237 /** 6238 * Search parameter: <b>code</b> 6239 * <p> 6240 * Description: <b>The code of the request group</b><br> 6241 * Type: <b>token</b><br> 6242 * Path: <b>RequestGroup.code</b><br> 6243 * </p> 6244 */ 6245 @SearchParamDefinition(name = "code", path = "RequestGroup.code", description = "The code of the request group", type = "token") 6246 public static final String SP_CODE = "code"; 6247 /** 6248 * <b>Fluent Client</b> search parameter constant for <b>code</b> 6249 * <p> 6250 * Description: <b>The code of the request group</b><br> 6251 * Type: <b>token</b><br> 6252 * Path: <b>RequestGroup.code</b><br> 6253 * </p> 6254 */ 6255 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6256 SP_CODE); 6257 6258 /** 6259 * Search parameter: <b>subject</b> 6260 * <p> 6261 * Description: <b>The subject that the request group is about</b><br> 6262 * Type: <b>reference</b><br> 6263 * Path: <b>RequestGroup.subject</b><br> 6264 * </p> 6265 */ 6266 @SearchParamDefinition(name = "subject", path = "RequestGroup.subject", description = "The subject that the request group is about", type = "reference", providesMembershipIn = { 6267 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 6268 public static final String SP_SUBJECT = "subject"; 6269 /** 6270 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 6271 * <p> 6272 * Description: <b>The subject that the request group is about</b><br> 6273 * Type: <b>reference</b><br> 6274 * Path: <b>RequestGroup.subject</b><br> 6275 * </p> 6276 */ 6277 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6278 SP_SUBJECT); 6279 6280 /** 6281 * Constant for fluent queries to be used to add include statements. Specifies 6282 * the path value of "<b>RequestGroup:subject</b>". 6283 */ 6284 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 6285 "RequestGroup:subject").toLocked(); 6286 6287 /** 6288 * Search parameter: <b>author</b> 6289 * <p> 6290 * Description: <b>The author of the request group</b><br> 6291 * Type: <b>reference</b><br> 6292 * Path: <b>RequestGroup.author</b><br> 6293 * </p> 6294 */ 6295 @SearchParamDefinition(name = "author", path = "RequestGroup.author", description = "The author of the request group", type = "reference", providesMembershipIn = { 6296 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 6297 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 6298 Practitioner.class, PractitionerRole.class }) 6299 public static final String SP_AUTHOR = "author"; 6300 /** 6301 * <b>Fluent Client</b> search parameter constant for <b>author</b> 6302 * <p> 6303 * Description: <b>The author of the request group</b><br> 6304 * Type: <b>reference</b><br> 6305 * Path: <b>RequestGroup.author</b><br> 6306 * </p> 6307 */ 6308 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6309 SP_AUTHOR); 6310 6311 /** 6312 * Constant for fluent queries to be used to add include statements. Specifies 6313 * the path value of "<b>RequestGroup:author</b>". 6314 */ 6315 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 6316 "RequestGroup:author").toLocked(); 6317 6318 /** 6319 * Search parameter: <b>instantiates-canonical</b> 6320 * <p> 6321 * Description: <b>The FHIR-based definition from which the request group is 6322 * realized</b><br> 6323 * Type: <b>reference</b><br> 6324 * Path: <b>RequestGroup.instantiatesCanonical</b><br> 6325 * </p> 6326 */ 6327 @SearchParamDefinition(name = "instantiates-canonical", path = "RequestGroup.instantiatesCanonical", description = "The FHIR-based definition from which the request group is realized", type = "reference") 6328 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 6329 /** 6330 * <b>Fluent Client</b> search parameter constant for 6331 * <b>instantiates-canonical</b> 6332 * <p> 6333 * Description: <b>The FHIR-based definition from which the request group is 6334 * realized</b><br> 6335 * Type: <b>reference</b><br> 6336 * Path: <b>RequestGroup.instantiatesCanonical</b><br> 6337 * </p> 6338 */ 6339 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6340 SP_INSTANTIATES_CANONICAL); 6341 6342 /** 6343 * Constant for fluent queries to be used to add include statements. Specifies 6344 * the path value of "<b>RequestGroup:instantiates-canonical</b>". 6345 */ 6346 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 6347 "RequestGroup:instantiates-canonical").toLocked(); 6348 6349 /** 6350 * Search parameter: <b>encounter</b> 6351 * <p> 6352 * Description: <b>The encounter the request group applies to</b><br> 6353 * Type: <b>reference</b><br> 6354 * Path: <b>RequestGroup.encounter</b><br> 6355 * </p> 6356 */ 6357 @SearchParamDefinition(name = "encounter", path = "RequestGroup.encounter", description = "The encounter the request group applies to", type = "reference", providesMembershipIn = { 6358 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 6359 public static final String SP_ENCOUNTER = "encounter"; 6360 /** 6361 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 6362 * <p> 6363 * Description: <b>The encounter the request group applies to</b><br> 6364 * Type: <b>reference</b><br> 6365 * Path: <b>RequestGroup.encounter</b><br> 6366 * </p> 6367 */ 6368 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6369 SP_ENCOUNTER); 6370 6371 /** 6372 * Constant for fluent queries to be used to add include statements. Specifies 6373 * the path value of "<b>RequestGroup:encounter</b>". 6374 */ 6375 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 6376 "RequestGroup:encounter").toLocked(); 6377 6378 /** 6379 * Search parameter: <b>priority</b> 6380 * <p> 6381 * Description: <b>The priority of the request group</b><br> 6382 * Type: <b>token</b><br> 6383 * Path: <b>RequestGroup.priority</b><br> 6384 * </p> 6385 */ 6386 @SearchParamDefinition(name = "priority", path = "RequestGroup.priority", description = "The priority of the request group", type = "token") 6387 public static final String SP_PRIORITY = "priority"; 6388 /** 6389 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 6390 * <p> 6391 * Description: <b>The priority of the request group</b><br> 6392 * Type: <b>token</b><br> 6393 * Path: <b>RequestGroup.priority</b><br> 6394 * </p> 6395 */ 6396 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6397 SP_PRIORITY); 6398 6399 /** 6400 * Search parameter: <b>intent</b> 6401 * <p> 6402 * Description: <b>The intent of the request group</b><br> 6403 * Type: <b>token</b><br> 6404 * Path: <b>RequestGroup.intent</b><br> 6405 * </p> 6406 */ 6407 @SearchParamDefinition(name = "intent", path = "RequestGroup.intent", description = "The intent of the request group", type = "token") 6408 public static final String SP_INTENT = "intent"; 6409 /** 6410 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 6411 * <p> 6412 * Description: <b>The intent of the request group</b><br> 6413 * Type: <b>token</b><br> 6414 * Path: <b>RequestGroup.intent</b><br> 6415 * </p> 6416 */ 6417 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6418 SP_INTENT); 6419 6420 /** 6421 * Search parameter: <b>participant</b> 6422 * <p> 6423 * Description: <b>The participant in the requests in the group</b><br> 6424 * Type: <b>reference</b><br> 6425 * Path: <b>RequestGroup.action.participant</b><br> 6426 * </p> 6427 */ 6428 @SearchParamDefinition(name = "participant", path = "RequestGroup.action.participant", description = "The participant in the requests in the group", type = "reference", providesMembershipIn = { 6429 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 6430 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 6431 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, Patient.class, 6432 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 6433 public static final String SP_PARTICIPANT = "participant"; 6434 /** 6435 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 6436 * <p> 6437 * Description: <b>The participant in the requests in the group</b><br> 6438 * Type: <b>reference</b><br> 6439 * Path: <b>RequestGroup.action.participant</b><br> 6440 * </p> 6441 */ 6442 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6443 SP_PARTICIPANT); 6444 6445 /** 6446 * Constant for fluent queries to be used to add include statements. Specifies 6447 * the path value of "<b>RequestGroup:participant</b>". 6448 */ 6449 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include( 6450 "RequestGroup:participant").toLocked(); 6451 6452 /** 6453 * Search parameter: <b>group-identifier</b> 6454 * <p> 6455 * Description: <b>The group identifier for the request group</b><br> 6456 * Type: <b>token</b><br> 6457 * Path: <b>RequestGroup.groupIdentifier</b><br> 6458 * </p> 6459 */ 6460 @SearchParamDefinition(name = "group-identifier", path = "RequestGroup.groupIdentifier", description = "The group identifier for the request group", type = "token") 6461 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 6462 /** 6463 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 6464 * <p> 6465 * Description: <b>The group identifier for the request group</b><br> 6466 * Type: <b>token</b><br> 6467 * Path: <b>RequestGroup.groupIdentifier</b><br> 6468 * </p> 6469 */ 6470 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6471 SP_GROUP_IDENTIFIER); 6472 6473 /** 6474 * Search parameter: <b>patient</b> 6475 * <p> 6476 * Description: <b>The identity of a patient to search for request 6477 * groups</b><br> 6478 * Type: <b>reference</b><br> 6479 * Path: <b>RequestGroup.subject</b><br> 6480 * </p> 6481 */ 6482 @SearchParamDefinition(name = "patient", path = "RequestGroup.subject.where(resolve() is Patient)", description = "The identity of a patient to search for request groups", type = "reference", target = { 6483 Patient.class }) 6484 public static final String SP_PATIENT = "patient"; 6485 /** 6486 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 6487 * <p> 6488 * Description: <b>The identity of a patient to search for request 6489 * groups</b><br> 6490 * Type: <b>reference</b><br> 6491 * Path: <b>RequestGroup.subject</b><br> 6492 * </p> 6493 */ 6494 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6495 SP_PATIENT); 6496 6497 /** 6498 * Constant for fluent queries to be used to add include statements. Specifies 6499 * the path value of "<b>RequestGroup:patient</b>". 6500 */ 6501 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 6502 "RequestGroup:patient").toLocked(); 6503 6504 /** 6505 * Search parameter: <b>instantiates-uri</b> 6506 * <p> 6507 * Description: <b>The external definition from which the request group is 6508 * realized</b><br> 6509 * Type: <b>uri</b><br> 6510 * Path: <b>RequestGroup.instantiatesUri</b><br> 6511 * </p> 6512 */ 6513 @SearchParamDefinition(name = "instantiates-uri", path = "RequestGroup.instantiatesUri", description = "The external definition from which the request group is realized", type = "uri") 6514 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 6515 /** 6516 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 6517 * <p> 6518 * Description: <b>The external definition from which the request group is 6519 * realized</b><br> 6520 * Type: <b>uri</b><br> 6521 * Path: <b>RequestGroup.instantiatesUri</b><br> 6522 * </p> 6523 */ 6524 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 6525 SP_INSTANTIATES_URI); 6526 6527 /** 6528 * Search parameter: <b>status</b> 6529 * <p> 6530 * Description: <b>The status of the request group</b><br> 6531 * Type: <b>token</b><br> 6532 * Path: <b>RequestGroup.status</b><br> 6533 * </p> 6534 */ 6535 @SearchParamDefinition(name = "status", path = "RequestGroup.status", description = "The status of the request group", type = "token") 6536 public static final String SP_STATUS = "status"; 6537 /** 6538 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6539 * <p> 6540 * Description: <b>The status of the request group</b><br> 6541 * Type: <b>token</b><br> 6542 * Path: <b>RequestGroup.status</b><br> 6543 * </p> 6544 */ 6545 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6546 SP_STATUS); 6547 6548}