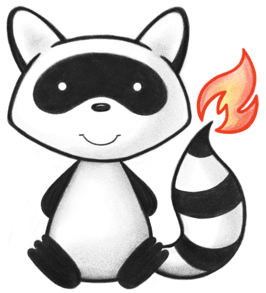
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * The ResearchElementDefinition resource describes a "PICO" element that 052 * knowledge (evidence, assertion, recommendation) is about. 053 */ 054@ResourceDef(name = "ResearchElementDefinition", profile = "http://hl7.org/fhir/StructureDefinition/ResearchElementDefinition") 055@ChildOrder(names = { "url", "identifier", "version", "name", "title", "shortTitle", "subtitle", "status", 056 "experimental", "subject[x]", "date", "publisher", "contact", "description", "comment", "useContext", 057 "jurisdiction", "purpose", "usage", "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", 058 "author", "editor", "reviewer", "endorser", "relatedArtifact", "library", "type", "variableType", 059 "characteristic" }) 060public class ResearchElementDefinition extends MetadataResource { 061 062 public enum ResearchElementType { 063 /** 064 * The element defines the population that forms the basis for research. 065 */ 066 POPULATION, 067 /** 068 * The element defines an exposure within the population that is being 069 * researched. 070 */ 071 EXPOSURE, 072 /** 073 * The element defines an outcome within the population that is being 074 * researched. 075 */ 076 OUTCOME, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static ResearchElementType fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("population".equals(codeString)) 086 return POPULATION; 087 if ("exposure".equals(codeString)) 088 return EXPOSURE; 089 if ("outcome".equals(codeString)) 090 return OUTCOME; 091 if (Configuration.isAcceptInvalidEnums()) 092 return null; 093 else 094 throw new FHIRException("Unknown ResearchElementType code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case POPULATION: 100 return "population"; 101 case EXPOSURE: 102 return "exposure"; 103 case OUTCOME: 104 return "outcome"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getSystem() { 113 switch (this) { 114 case POPULATION: 115 return "http://hl7.org/fhir/research-element-type"; 116 case EXPOSURE: 117 return "http://hl7.org/fhir/research-element-type"; 118 case OUTCOME: 119 return "http://hl7.org/fhir/research-element-type"; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 127 public String getDefinition() { 128 switch (this) { 129 case POPULATION: 130 return "The element defines the population that forms the basis for research."; 131 case EXPOSURE: 132 return "The element defines an exposure within the population that is being researched."; 133 case OUTCOME: 134 return "The element defines an outcome within the population that is being researched."; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getDisplay() { 143 switch (this) { 144 case POPULATION: 145 return "Population"; 146 case EXPOSURE: 147 return "Exposure"; 148 case OUTCOME: 149 return "Outcome"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 } 157 158 public static class ResearchElementTypeEnumFactory implements EnumFactory<ResearchElementType> { 159 public ResearchElementType fromCode(String codeString) throws IllegalArgumentException { 160 if (codeString == null || "".equals(codeString)) 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("population".equals(codeString)) 164 return ResearchElementType.POPULATION; 165 if ("exposure".equals(codeString)) 166 return ResearchElementType.EXPOSURE; 167 if ("outcome".equals(codeString)) 168 return ResearchElementType.OUTCOME; 169 throw new IllegalArgumentException("Unknown ResearchElementType code '" + codeString + "'"); 170 } 171 172 public Enumeration<ResearchElementType> fromType(PrimitiveType<?> code) throws FHIRException { 173 if (code == null) 174 return null; 175 if (code.isEmpty()) 176 return new Enumeration<ResearchElementType>(this, ResearchElementType.NULL, code); 177 String codeString = code.asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return new Enumeration<ResearchElementType>(this, ResearchElementType.NULL, code); 180 if ("population".equals(codeString)) 181 return new Enumeration<ResearchElementType>(this, ResearchElementType.POPULATION, code); 182 if ("exposure".equals(codeString)) 183 return new Enumeration<ResearchElementType>(this, ResearchElementType.EXPOSURE, code); 184 if ("outcome".equals(codeString)) 185 return new Enumeration<ResearchElementType>(this, ResearchElementType.OUTCOME, code); 186 throw new FHIRException("Unknown ResearchElementType code '" + codeString + "'"); 187 } 188 189 public String toCode(ResearchElementType code) { 190 if (code == ResearchElementType.POPULATION) 191 return "population"; 192 if (code == ResearchElementType.EXPOSURE) 193 return "exposure"; 194 if (code == ResearchElementType.OUTCOME) 195 return "outcome"; 196 return "?"; 197 } 198 199 public String toSystem(ResearchElementType code) { 200 return code.getSystem(); 201 } 202 } 203 204 public enum VariableType { 205 /** 206 * The variable is dichotomous, such as present or absent. 207 */ 208 DICHOTOMOUS, 209 /** 210 * The variable is a continuous result such as a quantity. 211 */ 212 CONTINUOUS, 213 /** 214 * The variable is described narratively rather than quantitatively. 215 */ 216 DESCRIPTIVE, 217 /** 218 * added to help the parsers with the generic types 219 */ 220 NULL; 221 222 public static VariableType fromCode(String codeString) throws FHIRException { 223 if (codeString == null || "".equals(codeString)) 224 return null; 225 if ("dichotomous".equals(codeString)) 226 return DICHOTOMOUS; 227 if ("continuous".equals(codeString)) 228 return CONTINUOUS; 229 if ("descriptive".equals(codeString)) 230 return DESCRIPTIVE; 231 if (Configuration.isAcceptInvalidEnums()) 232 return null; 233 else 234 throw new FHIRException("Unknown VariableType code '" + codeString + "'"); 235 } 236 237 public String toCode() { 238 switch (this) { 239 case DICHOTOMOUS: 240 return "dichotomous"; 241 case CONTINUOUS: 242 return "continuous"; 243 case DESCRIPTIVE: 244 return "descriptive"; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 252 public String getSystem() { 253 switch (this) { 254 case DICHOTOMOUS: 255 return "http://hl7.org/fhir/variable-type"; 256 case CONTINUOUS: 257 return "http://hl7.org/fhir/variable-type"; 258 case DESCRIPTIVE: 259 return "http://hl7.org/fhir/variable-type"; 260 case NULL: 261 return null; 262 default: 263 return "?"; 264 } 265 } 266 267 public String getDefinition() { 268 switch (this) { 269 case DICHOTOMOUS: 270 return "The variable is dichotomous, such as present or absent."; 271 case CONTINUOUS: 272 return "The variable is a continuous result such as a quantity."; 273 case DESCRIPTIVE: 274 return "The variable is described narratively rather than quantitatively."; 275 case NULL: 276 return null; 277 default: 278 return "?"; 279 } 280 } 281 282 public String getDisplay() { 283 switch (this) { 284 case DICHOTOMOUS: 285 return "Dichotomous"; 286 case CONTINUOUS: 287 return "Continuous"; 288 case DESCRIPTIVE: 289 return "Descriptive"; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 } 297 298 public static class VariableTypeEnumFactory implements EnumFactory<VariableType> { 299 public VariableType fromCode(String codeString) throws IllegalArgumentException { 300 if (codeString == null || "".equals(codeString)) 301 if (codeString == null || "".equals(codeString)) 302 return null; 303 if ("dichotomous".equals(codeString)) 304 return VariableType.DICHOTOMOUS; 305 if ("continuous".equals(codeString)) 306 return VariableType.CONTINUOUS; 307 if ("descriptive".equals(codeString)) 308 return VariableType.DESCRIPTIVE; 309 throw new IllegalArgumentException("Unknown VariableType code '" + codeString + "'"); 310 } 311 312 public Enumeration<VariableType> fromType(PrimitiveType<?> code) throws FHIRException { 313 if (code == null) 314 return null; 315 if (code.isEmpty()) 316 return new Enumeration<VariableType>(this, VariableType.NULL, code); 317 String codeString = code.asStringValue(); 318 if (codeString == null || "".equals(codeString)) 319 return new Enumeration<VariableType>(this, VariableType.NULL, code); 320 if ("dichotomous".equals(codeString)) 321 return new Enumeration<VariableType>(this, VariableType.DICHOTOMOUS, code); 322 if ("continuous".equals(codeString)) 323 return new Enumeration<VariableType>(this, VariableType.CONTINUOUS, code); 324 if ("descriptive".equals(codeString)) 325 return new Enumeration<VariableType>(this, VariableType.DESCRIPTIVE, code); 326 throw new FHIRException("Unknown VariableType code '" + codeString + "'"); 327 } 328 329 public String toCode(VariableType code) { 330 if (code == VariableType.DICHOTOMOUS) 331 return "dichotomous"; 332 if (code == VariableType.CONTINUOUS) 333 return "continuous"; 334 if (code == VariableType.DESCRIPTIVE) 335 return "descriptive"; 336 return "?"; 337 } 338 339 public String toSystem(VariableType code) { 340 return code.getSystem(); 341 } 342 } 343 344 public enum GroupMeasure { 345 /** 346 * Aggregated using Mean of participant values. 347 */ 348 MEAN, 349 /** 350 * Aggregated using Median of participant values. 351 */ 352 MEDIAN, 353 /** 354 * Aggregated using Mean of study mean values. 355 */ 356 MEANOFMEAN, 357 /** 358 * Aggregated using Mean of study median values. 359 */ 360 MEANOFMEDIAN, 361 /** 362 * Aggregated using Median of study mean values. 363 */ 364 MEDIANOFMEAN, 365 /** 366 * Aggregated using Median of study median values. 367 */ 368 MEDIANOFMEDIAN, 369 /** 370 * added to help the parsers with the generic types 371 */ 372 NULL; 373 374 public static GroupMeasure fromCode(String codeString) throws FHIRException { 375 if (codeString == null || "".equals(codeString)) 376 return null; 377 if ("mean".equals(codeString)) 378 return MEAN; 379 if ("median".equals(codeString)) 380 return MEDIAN; 381 if ("mean-of-mean".equals(codeString)) 382 return MEANOFMEAN; 383 if ("mean-of-median".equals(codeString)) 384 return MEANOFMEDIAN; 385 if ("median-of-mean".equals(codeString)) 386 return MEDIANOFMEAN; 387 if ("median-of-median".equals(codeString)) 388 return MEDIANOFMEDIAN; 389 if (Configuration.isAcceptInvalidEnums()) 390 return null; 391 else 392 throw new FHIRException("Unknown GroupMeasure code '" + codeString + "'"); 393 } 394 395 public String toCode() { 396 switch (this) { 397 case MEAN: 398 return "mean"; 399 case MEDIAN: 400 return "median"; 401 case MEANOFMEAN: 402 return "mean-of-mean"; 403 case MEANOFMEDIAN: 404 return "mean-of-median"; 405 case MEDIANOFMEAN: 406 return "median-of-mean"; 407 case MEDIANOFMEDIAN: 408 return "median-of-median"; 409 case NULL: 410 return null; 411 default: 412 return "?"; 413 } 414 } 415 416 public String getSystem() { 417 switch (this) { 418 case MEAN: 419 return "http://hl7.org/fhir/group-measure"; 420 case MEDIAN: 421 return "http://hl7.org/fhir/group-measure"; 422 case MEANOFMEAN: 423 return "http://hl7.org/fhir/group-measure"; 424 case MEANOFMEDIAN: 425 return "http://hl7.org/fhir/group-measure"; 426 case MEDIANOFMEAN: 427 return "http://hl7.org/fhir/group-measure"; 428 case MEDIANOFMEDIAN: 429 return "http://hl7.org/fhir/group-measure"; 430 case NULL: 431 return null; 432 default: 433 return "?"; 434 } 435 } 436 437 public String getDefinition() { 438 switch (this) { 439 case MEAN: 440 return "Aggregated using Mean of participant values."; 441 case MEDIAN: 442 return "Aggregated using Median of participant values."; 443 case MEANOFMEAN: 444 return "Aggregated using Mean of study mean values."; 445 case MEANOFMEDIAN: 446 return "Aggregated using Mean of study median values."; 447 case MEDIANOFMEAN: 448 return "Aggregated using Median of study mean values."; 449 case MEDIANOFMEDIAN: 450 return "Aggregated using Median of study median values."; 451 case NULL: 452 return null; 453 default: 454 return "?"; 455 } 456 } 457 458 public String getDisplay() { 459 switch (this) { 460 case MEAN: 461 return "Mean"; 462 case MEDIAN: 463 return "Median"; 464 case MEANOFMEAN: 465 return "Mean of Study Means"; 466 case MEANOFMEDIAN: 467 return "Mean of Study Medins"; 468 case MEDIANOFMEAN: 469 return "Median of Study Means"; 470 case MEDIANOFMEDIAN: 471 return "Median of Study Medians"; 472 case NULL: 473 return null; 474 default: 475 return "?"; 476 } 477 } 478 } 479 480 public static class GroupMeasureEnumFactory implements EnumFactory<GroupMeasure> { 481 public GroupMeasure fromCode(String codeString) throws IllegalArgumentException { 482 if (codeString == null || "".equals(codeString)) 483 if (codeString == null || "".equals(codeString)) 484 return null; 485 if ("mean".equals(codeString)) 486 return GroupMeasure.MEAN; 487 if ("median".equals(codeString)) 488 return GroupMeasure.MEDIAN; 489 if ("mean-of-mean".equals(codeString)) 490 return GroupMeasure.MEANOFMEAN; 491 if ("mean-of-median".equals(codeString)) 492 return GroupMeasure.MEANOFMEDIAN; 493 if ("median-of-mean".equals(codeString)) 494 return GroupMeasure.MEDIANOFMEAN; 495 if ("median-of-median".equals(codeString)) 496 return GroupMeasure.MEDIANOFMEDIAN; 497 throw new IllegalArgumentException("Unknown GroupMeasure code '" + codeString + "'"); 498 } 499 500 public Enumeration<GroupMeasure> fromType(PrimitiveType<?> code) throws FHIRException { 501 if (code == null) 502 return null; 503 if (code.isEmpty()) 504 return new Enumeration<GroupMeasure>(this, GroupMeasure.NULL, code); 505 String codeString = code.asStringValue(); 506 if (codeString == null || "".equals(codeString)) 507 return new Enumeration<GroupMeasure>(this, GroupMeasure.NULL, code); 508 if ("mean".equals(codeString)) 509 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEAN, code); 510 if ("median".equals(codeString)) 511 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIAN, code); 512 if ("mean-of-mean".equals(codeString)) 513 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEANOFMEAN, code); 514 if ("mean-of-median".equals(codeString)) 515 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEANOFMEDIAN, code); 516 if ("median-of-mean".equals(codeString)) 517 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIANOFMEAN, code); 518 if ("median-of-median".equals(codeString)) 519 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIANOFMEDIAN, code); 520 throw new FHIRException("Unknown GroupMeasure code '" + codeString + "'"); 521 } 522 523 public String toCode(GroupMeasure code) { 524 if (code == GroupMeasure.MEAN) 525 return "mean"; 526 if (code == GroupMeasure.MEDIAN) 527 return "median"; 528 if (code == GroupMeasure.MEANOFMEAN) 529 return "mean-of-mean"; 530 if (code == GroupMeasure.MEANOFMEDIAN) 531 return "mean-of-median"; 532 if (code == GroupMeasure.MEDIANOFMEAN) 533 return "median-of-mean"; 534 if (code == GroupMeasure.MEDIANOFMEDIAN) 535 return "median-of-median"; 536 return "?"; 537 } 538 539 public String toSystem(GroupMeasure code) { 540 return code.getSystem(); 541 } 542 } 543 544 @Block() 545 public static class ResearchElementDefinitionCharacteristicComponent extends BackboneElement 546 implements IBaseBackboneElement { 547 /** 548 * Define members of the research element using Codes (such as condition, 549 * medication, or observation), Expressions ( using an expression language such 550 * as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in 551 * the last year). 552 */ 553 @Child(name = "definition", type = { CodeableConcept.class, CanonicalType.class, Expression.class, 554 DataRequirement.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 555 @Description(shortDefinition = "What code or expression defines members?", formalDefinition = "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).") 556 protected Type definition; 557 558 /** 559 * Use UsageContext to define the members of the population, such as Age Ranges, 560 * Genders, Settings. 561 */ 562 @Child(name = "usageContext", type = { 563 UsageContext.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 564 @Description(shortDefinition = "What code/value pairs define members?", formalDefinition = "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.") 565 protected List<UsageContext> usageContext; 566 567 /** 568 * When true, members with this characteristic are excluded from the element. 569 */ 570 @Child(name = "exclude", type = { 571 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 572 @Description(shortDefinition = "Whether the characteristic includes or excludes members", formalDefinition = "When true, members with this characteristic are excluded from the element.") 573 protected BooleanType exclude; 574 575 /** 576 * Specifies the UCUM unit for the outcome. 577 */ 578 @Child(name = "unitOfMeasure", type = { 579 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 580 @Description(shortDefinition = "What unit is the outcome described in?", formalDefinition = "Specifies the UCUM unit for the outcome.") 581 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ucum-units") 582 protected CodeableConcept unitOfMeasure; 583 584 /** 585 * A narrative description of the time period the study covers. 586 */ 587 @Child(name = "studyEffectiveDescription", type = { 588 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 589 @Description(shortDefinition = "What time period does the study cover", formalDefinition = "A narrative description of the time period the study covers.") 590 protected StringType studyEffectiveDescription; 591 592 /** 593 * Indicates what effective period the study covers. 594 */ 595 @Child(name = "studyEffective", type = { DateTimeType.class, Period.class, Duration.class, 596 Timing.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 597 @Description(shortDefinition = "What time period does the study cover", formalDefinition = "Indicates what effective period the study covers.") 598 protected Type studyEffective; 599 600 /** 601 * Indicates duration from the study initiation. 602 */ 603 @Child(name = "studyEffectiveTimeFromStart", type = { 604 Duration.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 605 @Description(shortDefinition = "Observation time from study start", formalDefinition = "Indicates duration from the study initiation.") 606 protected Duration studyEffectiveTimeFromStart; 607 608 /** 609 * Indicates how elements are aggregated within the study effective period. 610 */ 611 @Child(name = "studyEffectiveGroupMeasure", type = { 612 CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 613 @Description(shortDefinition = "mean | median | mean-of-mean | mean-of-median | median-of-mean | median-of-median", formalDefinition = "Indicates how elements are aggregated within the study effective period.") 614 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-measure") 615 protected Enumeration<GroupMeasure> studyEffectiveGroupMeasure; 616 617 /** 618 * A narrative description of the time period the study covers. 619 */ 620 @Child(name = "participantEffectiveDescription", type = { 621 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 622 @Description(shortDefinition = "What time period do participants cover", formalDefinition = "A narrative description of the time period the study covers.") 623 protected StringType participantEffectiveDescription; 624 625 /** 626 * Indicates what effective period the study covers. 627 */ 628 @Child(name = "participantEffective", type = { DateTimeType.class, Period.class, Duration.class, 629 Timing.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 630 @Description(shortDefinition = "What time period do participants cover", formalDefinition = "Indicates what effective period the study covers.") 631 protected Type participantEffective; 632 633 /** 634 * Indicates duration from the participant's study entry. 635 */ 636 @Child(name = "participantEffectiveTimeFromStart", type = { 637 Duration.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 638 @Description(shortDefinition = "Observation time from study start", formalDefinition = "Indicates duration from the participant's study entry.") 639 protected Duration participantEffectiveTimeFromStart; 640 641 /** 642 * Indicates how elements are aggregated within the study effective period. 643 */ 644 @Child(name = "participantEffectiveGroupMeasure", type = { 645 CodeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 646 @Description(shortDefinition = "mean | median | mean-of-mean | mean-of-median | median-of-mean | median-of-median", formalDefinition = "Indicates how elements are aggregated within the study effective period.") 647 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-measure") 648 protected Enumeration<GroupMeasure> participantEffectiveGroupMeasure; 649 650 private static final long serialVersionUID = -1102952665L; 651 652 /** 653 * Constructor 654 */ 655 public ResearchElementDefinitionCharacteristicComponent() { 656 super(); 657 } 658 659 /** 660 * Constructor 661 */ 662 public ResearchElementDefinitionCharacteristicComponent(Type definition) { 663 super(); 664 this.definition = definition; 665 } 666 667 /** 668 * @return {@link #definition} (Define members of the research element using 669 * Codes (such as condition, medication, or observation), Expressions ( 670 * using an expression language such as FHIRPath or CQL) or 671 * DataRequirements (such as Diabetes diagnosis onset in the last 672 * year).) 673 */ 674 public Type getDefinition() { 675 return this.definition; 676 } 677 678 /** 679 * @return {@link #definition} (Define members of the research element using 680 * Codes (such as condition, medication, or observation), Expressions ( 681 * using an expression language such as FHIRPath or CQL) or 682 * DataRequirements (such as Diabetes diagnosis onset in the last 683 * year).) 684 */ 685 public CodeableConcept getDefinitionCodeableConcept() throws FHIRException { 686 if (this.definition == null) 687 this.definition = new CodeableConcept(); 688 if (!(this.definition instanceof CodeableConcept)) 689 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 690 + this.definition.getClass().getName() + " was encountered"); 691 return (CodeableConcept) this.definition; 692 } 693 694 public boolean hasDefinitionCodeableConcept() { 695 return this != null && this.definition instanceof CodeableConcept; 696 } 697 698 /** 699 * @return {@link #definition} (Define members of the research element using 700 * Codes (such as condition, medication, or observation), Expressions ( 701 * using an expression language such as FHIRPath or CQL) or 702 * DataRequirements (such as Diabetes diagnosis onset in the last 703 * year).) 704 */ 705 public CanonicalType getDefinitionCanonicalType() throws FHIRException { 706 if (this.definition == null) 707 this.definition = new CanonicalType(); 708 if (!(this.definition instanceof CanonicalType)) 709 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 710 + this.definition.getClass().getName() + " was encountered"); 711 return (CanonicalType) this.definition; 712 } 713 714 public boolean hasDefinitionCanonicalType() { 715 return this != null && this.definition instanceof CanonicalType; 716 } 717 718 /** 719 * @return {@link #definition} (Define members of the research element using 720 * Codes (such as condition, medication, or observation), Expressions ( 721 * using an expression language such as FHIRPath or CQL) or 722 * DataRequirements (such as Diabetes diagnosis onset in the last 723 * year).) 724 */ 725 public Expression getDefinitionExpression() throws FHIRException { 726 if (this.definition == null) 727 this.definition = new Expression(); 728 if (!(this.definition instanceof Expression)) 729 throw new FHIRException("Type mismatch: the type Expression was expected, but " 730 + this.definition.getClass().getName() + " was encountered"); 731 return (Expression) this.definition; 732 } 733 734 public boolean hasDefinitionExpression() { 735 return this != null && this.definition instanceof Expression; 736 } 737 738 /** 739 * @return {@link #definition} (Define members of the research element using 740 * Codes (such as condition, medication, or observation), Expressions ( 741 * using an expression language such as FHIRPath or CQL) or 742 * DataRequirements (such as Diabetes diagnosis onset in the last 743 * year).) 744 */ 745 public DataRequirement getDefinitionDataRequirement() throws FHIRException { 746 if (this.definition == null) 747 this.definition = new DataRequirement(); 748 if (!(this.definition instanceof DataRequirement)) 749 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but " 750 + this.definition.getClass().getName() + " was encountered"); 751 return (DataRequirement) this.definition; 752 } 753 754 public boolean hasDefinitionDataRequirement() { 755 return this != null && this.definition instanceof DataRequirement; 756 } 757 758 public boolean hasDefinition() { 759 return this.definition != null && !this.definition.isEmpty(); 760 } 761 762 /** 763 * @param value {@link #definition} (Define members of the research element 764 * using Codes (such as condition, medication, or observation), 765 * Expressions ( using an expression language such as FHIRPath or 766 * CQL) or DataRequirements (such as Diabetes diagnosis onset in 767 * the last year).) 768 */ 769 public ResearchElementDefinitionCharacteristicComponent setDefinition(Type value) { 770 if (value != null && !(value instanceof CodeableConcept || value instanceof CanonicalType 771 || value instanceof Expression || value instanceof DataRequirement)) 772 throw new Error( 773 "Not the right type for ResearchElementDefinition.characteristic.definition[x]: " + value.fhirType()); 774 this.definition = value; 775 return this; 776 } 777 778 /** 779 * @return {@link #usageContext} (Use UsageContext to define the members of the 780 * population, such as Age Ranges, Genders, Settings.) 781 */ 782 public List<UsageContext> getUsageContext() { 783 if (this.usageContext == null) 784 this.usageContext = new ArrayList<UsageContext>(); 785 return this.usageContext; 786 } 787 788 /** 789 * @return Returns a reference to <code>this</code> for easy method chaining 790 */ 791 public ResearchElementDefinitionCharacteristicComponent setUsageContext(List<UsageContext> theUsageContext) { 792 this.usageContext = theUsageContext; 793 return this; 794 } 795 796 public boolean hasUsageContext() { 797 if (this.usageContext == null) 798 return false; 799 for (UsageContext item : this.usageContext) 800 if (!item.isEmpty()) 801 return true; 802 return false; 803 } 804 805 public UsageContext addUsageContext() { // 3 806 UsageContext t = new UsageContext(); 807 if (this.usageContext == null) 808 this.usageContext = new ArrayList<UsageContext>(); 809 this.usageContext.add(t); 810 return t; 811 } 812 813 public ResearchElementDefinitionCharacteristicComponent addUsageContext(UsageContext t) { // 3 814 if (t == null) 815 return this; 816 if (this.usageContext == null) 817 this.usageContext = new ArrayList<UsageContext>(); 818 this.usageContext.add(t); 819 return this; 820 } 821 822 /** 823 * @return The first repetition of repeating field {@link #usageContext}, 824 * creating it if it does not already exist 825 */ 826 public UsageContext getUsageContextFirstRep() { 827 if (getUsageContext().isEmpty()) { 828 addUsageContext(); 829 } 830 return getUsageContext().get(0); 831 } 832 833 /** 834 * @return {@link #exclude} (When true, members with this characteristic are 835 * excluded from the element.). This is the underlying object with id, 836 * value and extensions. The accessor "getExclude" gives direct access 837 * to the value 838 */ 839 public BooleanType getExcludeElement() { 840 if (this.exclude == null) 841 if (Configuration.errorOnAutoCreate()) 842 throw new Error("Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.exclude"); 843 else if (Configuration.doAutoCreate()) 844 this.exclude = new BooleanType(); // bb 845 return this.exclude; 846 } 847 848 public boolean hasExcludeElement() { 849 return this.exclude != null && !this.exclude.isEmpty(); 850 } 851 852 public boolean hasExclude() { 853 return this.exclude != null && !this.exclude.isEmpty(); 854 } 855 856 /** 857 * @param value {@link #exclude} (When true, members with this characteristic 858 * are excluded from the element.). This is the underlying object 859 * with id, value and extensions. The accessor "getExclude" gives 860 * direct access to the value 861 */ 862 public ResearchElementDefinitionCharacteristicComponent setExcludeElement(BooleanType value) { 863 this.exclude = value; 864 return this; 865 } 866 867 /** 868 * @return When true, members with this characteristic are excluded from the 869 * element. 870 */ 871 public boolean getExclude() { 872 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 873 } 874 875 /** 876 * @param value When true, members with this characteristic are excluded from 877 * the element. 878 */ 879 public ResearchElementDefinitionCharacteristicComponent setExclude(boolean value) { 880 if (this.exclude == null) 881 this.exclude = new BooleanType(); 882 this.exclude.setValue(value); 883 return this; 884 } 885 886 /** 887 * @return {@link #unitOfMeasure} (Specifies the UCUM unit for the outcome.) 888 */ 889 public CodeableConcept getUnitOfMeasure() { 890 if (this.unitOfMeasure == null) 891 if (Configuration.errorOnAutoCreate()) 892 throw new Error("Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.unitOfMeasure"); 893 else if (Configuration.doAutoCreate()) 894 this.unitOfMeasure = new CodeableConcept(); // cc 895 return this.unitOfMeasure; 896 } 897 898 public boolean hasUnitOfMeasure() { 899 return this.unitOfMeasure != null && !this.unitOfMeasure.isEmpty(); 900 } 901 902 /** 903 * @param value {@link #unitOfMeasure} (Specifies the UCUM unit for the 904 * outcome.) 905 */ 906 public ResearchElementDefinitionCharacteristicComponent setUnitOfMeasure(CodeableConcept value) { 907 this.unitOfMeasure = value; 908 return this; 909 } 910 911 /** 912 * @return {@link #studyEffectiveDescription} (A narrative description of the 913 * time period the study covers.). This is the underlying object with 914 * id, value and extensions. The accessor "getStudyEffectiveDescription" 915 * gives direct access to the value 916 */ 917 public StringType getStudyEffectiveDescriptionElement() { 918 if (this.studyEffectiveDescription == null) 919 if (Configuration.errorOnAutoCreate()) 920 throw new Error( 921 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.studyEffectiveDescription"); 922 else if (Configuration.doAutoCreate()) 923 this.studyEffectiveDescription = new StringType(); // bb 924 return this.studyEffectiveDescription; 925 } 926 927 public boolean hasStudyEffectiveDescriptionElement() { 928 return this.studyEffectiveDescription != null && !this.studyEffectiveDescription.isEmpty(); 929 } 930 931 public boolean hasStudyEffectiveDescription() { 932 return this.studyEffectiveDescription != null && !this.studyEffectiveDescription.isEmpty(); 933 } 934 935 /** 936 * @param value {@link #studyEffectiveDescription} (A narrative description of 937 * the time period the study covers.). This is the underlying 938 * object with id, value and extensions. The accessor 939 * "getStudyEffectiveDescription" gives direct access to the value 940 */ 941 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveDescriptionElement(StringType value) { 942 this.studyEffectiveDescription = value; 943 return this; 944 } 945 946 /** 947 * @return A narrative description of the time period the study covers. 948 */ 949 public String getStudyEffectiveDescription() { 950 return this.studyEffectiveDescription == null ? null : this.studyEffectiveDescription.getValue(); 951 } 952 953 /** 954 * @param value A narrative description of the time period the study covers. 955 */ 956 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveDescription(String value) { 957 if (Utilities.noString(value)) 958 this.studyEffectiveDescription = null; 959 else { 960 if (this.studyEffectiveDescription == null) 961 this.studyEffectiveDescription = new StringType(); 962 this.studyEffectiveDescription.setValue(value); 963 } 964 return this; 965 } 966 967 /** 968 * @return {@link #studyEffective} (Indicates what effective period the study 969 * covers.) 970 */ 971 public Type getStudyEffective() { 972 return this.studyEffective; 973 } 974 975 /** 976 * @return {@link #studyEffective} (Indicates what effective period the study 977 * covers.) 978 */ 979 public DateTimeType getStudyEffectiveDateTimeType() throws FHIRException { 980 if (this.studyEffective == null) 981 this.studyEffective = new DateTimeType(); 982 if (!(this.studyEffective instanceof DateTimeType)) 983 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 984 + this.studyEffective.getClass().getName() + " was encountered"); 985 return (DateTimeType) this.studyEffective; 986 } 987 988 public boolean hasStudyEffectiveDateTimeType() { 989 return this != null && this.studyEffective instanceof DateTimeType; 990 } 991 992 /** 993 * @return {@link #studyEffective} (Indicates what effective period the study 994 * covers.) 995 */ 996 public Period getStudyEffectivePeriod() throws FHIRException { 997 if (this.studyEffective == null) 998 this.studyEffective = new Period(); 999 if (!(this.studyEffective instanceof Period)) 1000 throw new FHIRException("Type mismatch: the type Period was expected, but " 1001 + this.studyEffective.getClass().getName() + " was encountered"); 1002 return (Period) this.studyEffective; 1003 } 1004 1005 public boolean hasStudyEffectivePeriod() { 1006 return this != null && this.studyEffective instanceof Period; 1007 } 1008 1009 /** 1010 * @return {@link #studyEffective} (Indicates what effective period the study 1011 * covers.) 1012 */ 1013 public Duration getStudyEffectiveDuration() throws FHIRException { 1014 if (this.studyEffective == null) 1015 this.studyEffective = new Duration(); 1016 if (!(this.studyEffective instanceof Duration)) 1017 throw new FHIRException("Type mismatch: the type Duration was expected, but " 1018 + this.studyEffective.getClass().getName() + " was encountered"); 1019 return (Duration) this.studyEffective; 1020 } 1021 1022 public boolean hasStudyEffectiveDuration() { 1023 return this != null && this.studyEffective instanceof Duration; 1024 } 1025 1026 /** 1027 * @return {@link #studyEffective} (Indicates what effective period the study 1028 * covers.) 1029 */ 1030 public Timing getStudyEffectiveTiming() throws FHIRException { 1031 if (this.studyEffective == null) 1032 this.studyEffective = new Timing(); 1033 if (!(this.studyEffective instanceof Timing)) 1034 throw new FHIRException("Type mismatch: the type Timing was expected, but " 1035 + this.studyEffective.getClass().getName() + " was encountered"); 1036 return (Timing) this.studyEffective; 1037 } 1038 1039 public boolean hasStudyEffectiveTiming() { 1040 return this != null && this.studyEffective instanceof Timing; 1041 } 1042 1043 public boolean hasStudyEffective() { 1044 return this.studyEffective != null && !this.studyEffective.isEmpty(); 1045 } 1046 1047 /** 1048 * @param value {@link #studyEffective} (Indicates what effective period the 1049 * study covers.) 1050 */ 1051 public ResearchElementDefinitionCharacteristicComponent setStudyEffective(Type value) { 1052 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration 1053 || value instanceof Timing)) 1054 throw new Error( 1055 "Not the right type for ResearchElementDefinition.characteristic.studyEffective[x]: " + value.fhirType()); 1056 this.studyEffective = value; 1057 return this; 1058 } 1059 1060 /** 1061 * @return {@link #studyEffectiveTimeFromStart} (Indicates duration from the 1062 * study initiation.) 1063 */ 1064 public Duration getStudyEffectiveTimeFromStart() { 1065 if (this.studyEffectiveTimeFromStart == null) 1066 if (Configuration.errorOnAutoCreate()) 1067 throw new Error( 1068 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.studyEffectiveTimeFromStart"); 1069 else if (Configuration.doAutoCreate()) 1070 this.studyEffectiveTimeFromStart = new Duration(); // cc 1071 return this.studyEffectiveTimeFromStart; 1072 } 1073 1074 public boolean hasStudyEffectiveTimeFromStart() { 1075 return this.studyEffectiveTimeFromStart != null && !this.studyEffectiveTimeFromStart.isEmpty(); 1076 } 1077 1078 /** 1079 * @param value {@link #studyEffectiveTimeFromStart} (Indicates duration from 1080 * the study initiation.) 1081 */ 1082 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveTimeFromStart(Duration value) { 1083 this.studyEffectiveTimeFromStart = value; 1084 return this; 1085 } 1086 1087 /** 1088 * @return {@link #studyEffectiveGroupMeasure} (Indicates how elements are 1089 * aggregated within the study effective period.). This is the 1090 * underlying object with id, value and extensions. The accessor 1091 * "getStudyEffectiveGroupMeasure" gives direct access to the value 1092 */ 1093 public Enumeration<GroupMeasure> getStudyEffectiveGroupMeasureElement() { 1094 if (this.studyEffectiveGroupMeasure == null) 1095 if (Configuration.errorOnAutoCreate()) 1096 throw new Error( 1097 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.studyEffectiveGroupMeasure"); 1098 else if (Configuration.doAutoCreate()) 1099 this.studyEffectiveGroupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); // bb 1100 return this.studyEffectiveGroupMeasure; 1101 } 1102 1103 public boolean hasStudyEffectiveGroupMeasureElement() { 1104 return this.studyEffectiveGroupMeasure != null && !this.studyEffectiveGroupMeasure.isEmpty(); 1105 } 1106 1107 public boolean hasStudyEffectiveGroupMeasure() { 1108 return this.studyEffectiveGroupMeasure != null && !this.studyEffectiveGroupMeasure.isEmpty(); 1109 } 1110 1111 /** 1112 * @param value {@link #studyEffectiveGroupMeasure} (Indicates how elements are 1113 * aggregated within the study effective period.). This is the 1114 * underlying object with id, value and extensions. The accessor 1115 * "getStudyEffectiveGroupMeasure" gives direct access to the value 1116 */ 1117 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveGroupMeasureElement( 1118 Enumeration<GroupMeasure> value) { 1119 this.studyEffectiveGroupMeasure = value; 1120 return this; 1121 } 1122 1123 /** 1124 * @return Indicates how elements are aggregated within the study effective 1125 * period. 1126 */ 1127 public GroupMeasure getStudyEffectiveGroupMeasure() { 1128 return this.studyEffectiveGroupMeasure == null ? null : this.studyEffectiveGroupMeasure.getValue(); 1129 } 1130 1131 /** 1132 * @param value Indicates how elements are aggregated within the study effective 1133 * period. 1134 */ 1135 public ResearchElementDefinitionCharacteristicComponent setStudyEffectiveGroupMeasure(GroupMeasure value) { 1136 if (value == null) 1137 this.studyEffectiveGroupMeasure = null; 1138 else { 1139 if (this.studyEffectiveGroupMeasure == null) 1140 this.studyEffectiveGroupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); 1141 this.studyEffectiveGroupMeasure.setValue(value); 1142 } 1143 return this; 1144 } 1145 1146 /** 1147 * @return {@link #participantEffectiveDescription} (A narrative description of 1148 * the time period the study covers.). This is the underlying object 1149 * with id, value and extensions. The accessor 1150 * "getParticipantEffectiveDescription" gives direct access to the value 1151 */ 1152 public StringType getParticipantEffectiveDescriptionElement() { 1153 if (this.participantEffectiveDescription == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error( 1156 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.participantEffectiveDescription"); 1157 else if (Configuration.doAutoCreate()) 1158 this.participantEffectiveDescription = new StringType(); // bb 1159 return this.participantEffectiveDescription; 1160 } 1161 1162 public boolean hasParticipantEffectiveDescriptionElement() { 1163 return this.participantEffectiveDescription != null && !this.participantEffectiveDescription.isEmpty(); 1164 } 1165 1166 public boolean hasParticipantEffectiveDescription() { 1167 return this.participantEffectiveDescription != null && !this.participantEffectiveDescription.isEmpty(); 1168 } 1169 1170 /** 1171 * @param value {@link #participantEffectiveDescription} (A narrative 1172 * description of the time period the study covers.). This is the 1173 * underlying object with id, value and extensions. The accessor 1174 * "getParticipantEffectiveDescription" gives direct access to the 1175 * value 1176 */ 1177 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveDescriptionElement( 1178 StringType value) { 1179 this.participantEffectiveDescription = value; 1180 return this; 1181 } 1182 1183 /** 1184 * @return A narrative description of the time period the study covers. 1185 */ 1186 public String getParticipantEffectiveDescription() { 1187 return this.participantEffectiveDescription == null ? null : this.participantEffectiveDescription.getValue(); 1188 } 1189 1190 /** 1191 * @param value A narrative description of the time period the study covers. 1192 */ 1193 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveDescription(String value) { 1194 if (Utilities.noString(value)) 1195 this.participantEffectiveDescription = null; 1196 else { 1197 if (this.participantEffectiveDescription == null) 1198 this.participantEffectiveDescription = new StringType(); 1199 this.participantEffectiveDescription.setValue(value); 1200 } 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #participantEffective} (Indicates what effective period the 1206 * study covers.) 1207 */ 1208 public Type getParticipantEffective() { 1209 return this.participantEffective; 1210 } 1211 1212 /** 1213 * @return {@link #participantEffective} (Indicates what effective period the 1214 * study covers.) 1215 */ 1216 public DateTimeType getParticipantEffectiveDateTimeType() throws FHIRException { 1217 if (this.participantEffective == null) 1218 this.participantEffective = new DateTimeType(); 1219 if (!(this.participantEffective instanceof DateTimeType)) 1220 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1221 + this.participantEffective.getClass().getName() + " was encountered"); 1222 return (DateTimeType) this.participantEffective; 1223 } 1224 1225 public boolean hasParticipantEffectiveDateTimeType() { 1226 return this != null && this.participantEffective instanceof DateTimeType; 1227 } 1228 1229 /** 1230 * @return {@link #participantEffective} (Indicates what effective period the 1231 * study covers.) 1232 */ 1233 public Period getParticipantEffectivePeriod() throws FHIRException { 1234 if (this.participantEffective == null) 1235 this.participantEffective = new Period(); 1236 if (!(this.participantEffective instanceof Period)) 1237 throw new FHIRException("Type mismatch: the type Period was expected, but " 1238 + this.participantEffective.getClass().getName() + " was encountered"); 1239 return (Period) this.participantEffective; 1240 } 1241 1242 public boolean hasParticipantEffectivePeriod() { 1243 return this != null && this.participantEffective instanceof Period; 1244 } 1245 1246 /** 1247 * @return {@link #participantEffective} (Indicates what effective period the 1248 * study covers.) 1249 */ 1250 public Duration getParticipantEffectiveDuration() throws FHIRException { 1251 if (this.participantEffective == null) 1252 this.participantEffective = new Duration(); 1253 if (!(this.participantEffective instanceof Duration)) 1254 throw new FHIRException("Type mismatch: the type Duration was expected, but " 1255 + this.participantEffective.getClass().getName() + " was encountered"); 1256 return (Duration) this.participantEffective; 1257 } 1258 1259 public boolean hasParticipantEffectiveDuration() { 1260 return this != null && this.participantEffective instanceof Duration; 1261 } 1262 1263 /** 1264 * @return {@link #participantEffective} (Indicates what effective period the 1265 * study covers.) 1266 */ 1267 public Timing getParticipantEffectiveTiming() throws FHIRException { 1268 if (this.participantEffective == null) 1269 this.participantEffective = new Timing(); 1270 if (!(this.participantEffective instanceof Timing)) 1271 throw new FHIRException("Type mismatch: the type Timing was expected, but " 1272 + this.participantEffective.getClass().getName() + " was encountered"); 1273 return (Timing) this.participantEffective; 1274 } 1275 1276 public boolean hasParticipantEffectiveTiming() { 1277 return this != null && this.participantEffective instanceof Timing; 1278 } 1279 1280 public boolean hasParticipantEffective() { 1281 return this.participantEffective != null && !this.participantEffective.isEmpty(); 1282 } 1283 1284 /** 1285 * @param value {@link #participantEffective} (Indicates what effective period 1286 * the study covers.) 1287 */ 1288 public ResearchElementDefinitionCharacteristicComponent setParticipantEffective(Type value) { 1289 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration 1290 || value instanceof Timing)) 1291 throw new Error("Not the right type for ResearchElementDefinition.characteristic.participantEffective[x]: " 1292 + value.fhirType()); 1293 this.participantEffective = value; 1294 return this; 1295 } 1296 1297 /** 1298 * @return {@link #participantEffectiveTimeFromStart} (Indicates duration from 1299 * the participant's study entry.) 1300 */ 1301 public Duration getParticipantEffectiveTimeFromStart() { 1302 if (this.participantEffectiveTimeFromStart == null) 1303 if (Configuration.errorOnAutoCreate()) 1304 throw new Error( 1305 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.participantEffectiveTimeFromStart"); 1306 else if (Configuration.doAutoCreate()) 1307 this.participantEffectiveTimeFromStart = new Duration(); // cc 1308 return this.participantEffectiveTimeFromStart; 1309 } 1310 1311 public boolean hasParticipantEffectiveTimeFromStart() { 1312 return this.participantEffectiveTimeFromStart != null && !this.participantEffectiveTimeFromStart.isEmpty(); 1313 } 1314 1315 /** 1316 * @param value {@link #participantEffectiveTimeFromStart} (Indicates duration 1317 * from the participant's study entry.) 1318 */ 1319 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveTimeFromStart(Duration value) { 1320 this.participantEffectiveTimeFromStart = value; 1321 return this; 1322 } 1323 1324 /** 1325 * @return {@link #participantEffectiveGroupMeasure} (Indicates how elements are 1326 * aggregated within the study effective period.). This is the 1327 * underlying object with id, value and extensions. The accessor 1328 * "getParticipantEffectiveGroupMeasure" gives direct access to the 1329 * value 1330 */ 1331 public Enumeration<GroupMeasure> getParticipantEffectiveGroupMeasureElement() { 1332 if (this.participantEffectiveGroupMeasure == null) 1333 if (Configuration.errorOnAutoCreate()) 1334 throw new Error( 1335 "Attempt to auto-create ResearchElementDefinitionCharacteristicComponent.participantEffectiveGroupMeasure"); 1336 else if (Configuration.doAutoCreate()) 1337 this.participantEffectiveGroupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); // bb 1338 return this.participantEffectiveGroupMeasure; 1339 } 1340 1341 public boolean hasParticipantEffectiveGroupMeasureElement() { 1342 return this.participantEffectiveGroupMeasure != null && !this.participantEffectiveGroupMeasure.isEmpty(); 1343 } 1344 1345 public boolean hasParticipantEffectiveGroupMeasure() { 1346 return this.participantEffectiveGroupMeasure != null && !this.participantEffectiveGroupMeasure.isEmpty(); 1347 } 1348 1349 /** 1350 * @param value {@link #participantEffectiveGroupMeasure} (Indicates how 1351 * elements are aggregated within the study effective period.). 1352 * This is the underlying object with id, value and extensions. The 1353 * accessor "getParticipantEffectiveGroupMeasure" gives direct 1354 * access to the value 1355 */ 1356 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveGroupMeasureElement( 1357 Enumeration<GroupMeasure> value) { 1358 this.participantEffectiveGroupMeasure = value; 1359 return this; 1360 } 1361 1362 /** 1363 * @return Indicates how elements are aggregated within the study effective 1364 * period. 1365 */ 1366 public GroupMeasure getParticipantEffectiveGroupMeasure() { 1367 return this.participantEffectiveGroupMeasure == null ? null : this.participantEffectiveGroupMeasure.getValue(); 1368 } 1369 1370 /** 1371 * @param value Indicates how elements are aggregated within the study effective 1372 * period. 1373 */ 1374 public ResearchElementDefinitionCharacteristicComponent setParticipantEffectiveGroupMeasure(GroupMeasure value) { 1375 if (value == null) 1376 this.participantEffectiveGroupMeasure = null; 1377 else { 1378 if (this.participantEffectiveGroupMeasure == null) 1379 this.participantEffectiveGroupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); 1380 this.participantEffectiveGroupMeasure.setValue(value); 1381 } 1382 return this; 1383 } 1384 1385 protected void listChildren(List<Property> children) { 1386 super.listChildren(children); 1387 children.add(new Property("definition[x]", "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1388 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1389 0, 1, definition)); 1390 children.add(new Property("usageContext", "UsageContext", 1391 "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.", 0, 1392 java.lang.Integer.MAX_VALUE, usageContext)); 1393 children.add(new Property("exclude", "boolean", 1394 "When true, members with this characteristic are excluded from the element.", 0, 1, exclude)); 1395 children.add(new Property("unitOfMeasure", "CodeableConcept", "Specifies the UCUM unit for the outcome.", 0, 1, 1396 unitOfMeasure)); 1397 children.add(new Property("studyEffectiveDescription", "string", 1398 "A narrative description of the time period the study covers.", 0, 1, studyEffectiveDescription)); 1399 children.add(new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1400 "Indicates what effective period the study covers.", 0, 1, studyEffective)); 1401 children.add(new Property("studyEffectiveTimeFromStart", "Duration", 1402 "Indicates duration from the study initiation.", 0, 1, studyEffectiveTimeFromStart)); 1403 children.add(new Property("studyEffectiveGroupMeasure", "code", 1404 "Indicates how elements are aggregated within the study effective period.", 0, 1, 1405 studyEffectiveGroupMeasure)); 1406 children.add(new Property("participantEffectiveDescription", "string", 1407 "A narrative description of the time period the study covers.", 0, 1, participantEffectiveDescription)); 1408 children.add(new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1409 "Indicates what effective period the study covers.", 0, 1, participantEffective)); 1410 children.add(new Property("participantEffectiveTimeFromStart", "Duration", 1411 "Indicates duration from the participant's study entry.", 0, 1, participantEffectiveTimeFromStart)); 1412 children.add(new Property("participantEffectiveGroupMeasure", "code", 1413 "Indicates how elements are aggregated within the study effective period.", 0, 1, 1414 participantEffectiveGroupMeasure)); 1415 } 1416 1417 @Override 1418 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1419 switch (_hash) { 1420 case -1139422643: 1421 /* definition[x] */ return new Property("definition[x]", 1422 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1423 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1424 0, 1, definition); 1425 case -1014418093: 1426 /* definition */ return new Property("definition[x]", 1427 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1428 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1429 0, 1, definition); 1430 case -1446002226: 1431 /* definitionCodeableConcept */ return new Property("definition[x]", 1432 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1433 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1434 0, 1, definition); 1435 case 933485793: 1436 /* definitionCanonical */ return new Property("definition[x]", 1437 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1438 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1439 0, 1, definition); 1440 case 1463703627: 1441 /* definitionExpression */ return new Property("definition[x]", 1442 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1443 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1444 0, 1, definition); 1445 case -660350874: 1446 /* definitionDataRequirement */ return new Property("definition[x]", 1447 "CodeableConcept|canonical(ValueSet)|Expression|DataRequirement", 1448 "Define members of the research element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1449 0, 1, definition); 1450 case 907012302: 1451 /* usageContext */ return new Property("usageContext", "UsageContext", 1452 "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.", 0, 1453 java.lang.Integer.MAX_VALUE, usageContext); 1454 case -1321148966: 1455 /* exclude */ return new Property("exclude", "boolean", 1456 "When true, members with this characteristic are excluded from the element.", 0, 1, exclude); 1457 case -750257565: 1458 /* unitOfMeasure */ return new Property("unitOfMeasure", "CodeableConcept", 1459 "Specifies the UCUM unit for the outcome.", 0, 1, unitOfMeasure); 1460 case 237553470: 1461 /* studyEffectiveDescription */ return new Property("studyEffectiveDescription", "string", 1462 "A narrative description of the time period the study covers.", 0, 1, studyEffectiveDescription); 1463 case -1832549918: 1464 /* studyEffective[x] */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1465 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1466 case -836391458: 1467 /* studyEffective */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1468 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1469 case 439780249: 1470 /* studyEffectiveDateTime */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1471 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1472 case -497045185: 1473 /* studyEffectivePeriod */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1474 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1475 case 949985682: 1476 /* studyEffectiveDuration */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1477 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1478 case -378983480: 1479 /* studyEffectiveTiming */ return new Property("studyEffective[x]", "dateTime|Period|Duration|Timing", 1480 "Indicates what effective period the study covers.", 0, 1, studyEffective); 1481 case -2107828915: 1482 /* studyEffectiveTimeFromStart */ return new Property("studyEffectiveTimeFromStart", "Duration", 1483 "Indicates duration from the study initiation.", 0, 1, studyEffectiveTimeFromStart); 1484 case 1284435677: 1485 /* studyEffectiveGroupMeasure */ return new Property("studyEffectiveGroupMeasure", "code", 1486 "Indicates how elements are aggregated within the study effective period.", 0, 1, 1487 studyEffectiveGroupMeasure); 1488 case 1333186472: 1489 /* participantEffectiveDescription */ return new Property("participantEffectiveDescription", "string", 1490 "A narrative description of the time period the study covers.", 0, 1, participantEffectiveDescription); 1491 case 1777308748: 1492 /* participantEffective[x] */ return new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1493 "Indicates what effective period the study covers.", 0, 1, participantEffective); 1494 case 1376306100: 1495 /* participantEffective */ return new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1496 "Indicates what effective period the study covers.", 0, 1, participantEffective); 1497 case -1721146513: 1498 /* participantEffectiveDateTime */ return new Property("participantEffective[x]", 1499 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1500 participantEffective); 1501 case -883650923: 1502 /* participantEffectivePeriod */ return new Property("participantEffective[x]", 1503 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1504 participantEffective); 1505 case -1210941080: 1506 /* participantEffectiveDuration */ return new Property("participantEffective[x]", 1507 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1508 participantEffective); 1509 case -765589218: 1510 /* participantEffectiveTiming */ return new Property("participantEffective[x]", 1511 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1512 participantEffective); 1513 case -1471501513: 1514 /* participantEffectiveTimeFromStart */ return new Property("participantEffectiveTimeFromStart", "Duration", 1515 "Indicates duration from the participant's study entry.", 0, 1, participantEffectiveTimeFromStart); 1516 case 889320371: 1517 /* participantEffectiveGroupMeasure */ return new Property("participantEffectiveGroupMeasure", "code", 1518 "Indicates how elements are aggregated within the study effective period.", 0, 1, 1519 participantEffectiveGroupMeasure); 1520 default: 1521 return super.getNamedProperty(_hash, _name, _checkValid); 1522 } 1523 1524 } 1525 1526 @Override 1527 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1528 switch (hash) { 1529 case -1014418093: 1530 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // Type 1531 case 907012302: 1532 /* usageContext */ return this.usageContext == null ? new Base[0] 1533 : this.usageContext.toArray(new Base[this.usageContext.size()]); // UsageContext 1534 case -1321148966: 1535 /* exclude */ return this.exclude == null ? new Base[0] : new Base[] { this.exclude }; // BooleanType 1536 case -750257565: 1537 /* unitOfMeasure */ return this.unitOfMeasure == null ? new Base[0] : new Base[] { this.unitOfMeasure }; // CodeableConcept 1538 case 237553470: 1539 /* studyEffectiveDescription */ return this.studyEffectiveDescription == null ? new Base[0] 1540 : new Base[] { this.studyEffectiveDescription }; // StringType 1541 case -836391458: 1542 /* studyEffective */ return this.studyEffective == null ? new Base[0] : new Base[] { this.studyEffective }; // Type 1543 case -2107828915: 1544 /* studyEffectiveTimeFromStart */ return this.studyEffectiveTimeFromStart == null ? new Base[0] 1545 : new Base[] { this.studyEffectiveTimeFromStart }; // Duration 1546 case 1284435677: 1547 /* studyEffectiveGroupMeasure */ return this.studyEffectiveGroupMeasure == null ? new Base[0] 1548 : new Base[] { this.studyEffectiveGroupMeasure }; // Enumeration<GroupMeasure> 1549 case 1333186472: 1550 /* participantEffectiveDescription */ return this.participantEffectiveDescription == null ? new Base[0] 1551 : new Base[] { this.participantEffectiveDescription }; // StringType 1552 case 1376306100: 1553 /* participantEffective */ return this.participantEffective == null ? new Base[0] 1554 : new Base[] { this.participantEffective }; // Type 1555 case -1471501513: 1556 /* participantEffectiveTimeFromStart */ return this.participantEffectiveTimeFromStart == null ? new Base[0] 1557 : new Base[] { this.participantEffectiveTimeFromStart }; // Duration 1558 case 889320371: 1559 /* participantEffectiveGroupMeasure */ return this.participantEffectiveGroupMeasure == null ? new Base[0] 1560 : new Base[] { this.participantEffectiveGroupMeasure }; // Enumeration<GroupMeasure> 1561 default: 1562 return super.getProperty(hash, name, checkValid); 1563 } 1564 1565 } 1566 1567 @Override 1568 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1569 switch (hash) { 1570 case -1014418093: // definition 1571 this.definition = castToType(value); // Type 1572 return value; 1573 case 907012302: // usageContext 1574 this.getUsageContext().add(castToUsageContext(value)); // UsageContext 1575 return value; 1576 case -1321148966: // exclude 1577 this.exclude = castToBoolean(value); // BooleanType 1578 return value; 1579 case -750257565: // unitOfMeasure 1580 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 1581 return value; 1582 case 237553470: // studyEffectiveDescription 1583 this.studyEffectiveDescription = castToString(value); // StringType 1584 return value; 1585 case -836391458: // studyEffective 1586 this.studyEffective = castToType(value); // Type 1587 return value; 1588 case -2107828915: // studyEffectiveTimeFromStart 1589 this.studyEffectiveTimeFromStart = castToDuration(value); // Duration 1590 return value; 1591 case 1284435677: // studyEffectiveGroupMeasure 1592 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1593 this.studyEffectiveGroupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1594 return value; 1595 case 1333186472: // participantEffectiveDescription 1596 this.participantEffectiveDescription = castToString(value); // StringType 1597 return value; 1598 case 1376306100: // participantEffective 1599 this.participantEffective = castToType(value); // Type 1600 return value; 1601 case -1471501513: // participantEffectiveTimeFromStart 1602 this.participantEffectiveTimeFromStart = castToDuration(value); // Duration 1603 return value; 1604 case 889320371: // participantEffectiveGroupMeasure 1605 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1606 this.participantEffectiveGroupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1607 return value; 1608 default: 1609 return super.setProperty(hash, name, value); 1610 } 1611 1612 } 1613 1614 @Override 1615 public Base setProperty(String name, Base value) throws FHIRException { 1616 if (name.equals("definition[x]")) { 1617 this.definition = castToType(value); // Type 1618 } else if (name.equals("usageContext")) { 1619 this.getUsageContext().add(castToUsageContext(value)); 1620 } else if (name.equals("exclude")) { 1621 this.exclude = castToBoolean(value); // BooleanType 1622 } else if (name.equals("unitOfMeasure")) { 1623 this.unitOfMeasure = castToCodeableConcept(value); // CodeableConcept 1624 } else if (name.equals("studyEffectiveDescription")) { 1625 this.studyEffectiveDescription = castToString(value); // StringType 1626 } else if (name.equals("studyEffective[x]")) { 1627 this.studyEffective = castToType(value); // Type 1628 } else if (name.equals("studyEffectiveTimeFromStart")) { 1629 this.studyEffectiveTimeFromStart = castToDuration(value); // Duration 1630 } else if (name.equals("studyEffectiveGroupMeasure")) { 1631 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1632 this.studyEffectiveGroupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1633 } else if (name.equals("participantEffectiveDescription")) { 1634 this.participantEffectiveDescription = castToString(value); // StringType 1635 } else if (name.equals("participantEffective[x]")) { 1636 this.participantEffective = castToType(value); // Type 1637 } else if (name.equals("participantEffectiveTimeFromStart")) { 1638 this.participantEffectiveTimeFromStart = castToDuration(value); // Duration 1639 } else if (name.equals("participantEffectiveGroupMeasure")) { 1640 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1641 this.participantEffectiveGroupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1642 } else 1643 return super.setProperty(name, value); 1644 return value; 1645 } 1646 1647 @Override 1648 public void removeChild(String name, Base value) throws FHIRException { 1649 if (name.equals("definition[x]")) { 1650 this.definition = null; 1651 } else if (name.equals("usageContext")) { 1652 this.getUsageContext().remove(castToUsageContext(value)); 1653 } else if (name.equals("exclude")) { 1654 this.exclude = null; 1655 } else if (name.equals("unitOfMeasure")) { 1656 this.unitOfMeasure = null; 1657 } else if (name.equals("studyEffectiveDescription")) { 1658 this.studyEffectiveDescription = null; 1659 } else if (name.equals("studyEffective[x]")) { 1660 this.studyEffective = null; 1661 } else if (name.equals("studyEffectiveTimeFromStart")) { 1662 this.studyEffectiveTimeFromStart = null; 1663 } else if (name.equals("studyEffectiveGroupMeasure")) { 1664 this.studyEffectiveGroupMeasure = null; 1665 } else if (name.equals("participantEffectiveDescription")) { 1666 this.participantEffectiveDescription = null; 1667 } else if (name.equals("participantEffective[x]")) { 1668 this.participantEffective = null; 1669 } else if (name.equals("participantEffectiveTimeFromStart")) { 1670 this.participantEffectiveTimeFromStart = null; 1671 } else if (name.equals("participantEffectiveGroupMeasure")) { 1672 this.participantEffectiveGroupMeasure = null; 1673 } else 1674 super.removeChild(name, value); 1675 1676 } 1677 1678 @Override 1679 public Base makeProperty(int hash, String name) throws FHIRException { 1680 switch (hash) { 1681 case -1139422643: 1682 return getDefinition(); 1683 case -1014418093: 1684 return getDefinition(); 1685 case 907012302: 1686 return addUsageContext(); 1687 case -1321148966: 1688 return getExcludeElement(); 1689 case -750257565: 1690 return getUnitOfMeasure(); 1691 case 237553470: 1692 return getStudyEffectiveDescriptionElement(); 1693 case -1832549918: 1694 return getStudyEffective(); 1695 case -836391458: 1696 return getStudyEffective(); 1697 case -2107828915: 1698 return getStudyEffectiveTimeFromStart(); 1699 case 1284435677: 1700 return getStudyEffectiveGroupMeasureElement(); 1701 case 1333186472: 1702 return getParticipantEffectiveDescriptionElement(); 1703 case 1777308748: 1704 return getParticipantEffective(); 1705 case 1376306100: 1706 return getParticipantEffective(); 1707 case -1471501513: 1708 return getParticipantEffectiveTimeFromStart(); 1709 case 889320371: 1710 return getParticipantEffectiveGroupMeasureElement(); 1711 default: 1712 return super.makeProperty(hash, name); 1713 } 1714 1715 } 1716 1717 @Override 1718 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1719 switch (hash) { 1720 case -1014418093: 1721 /* definition */ return new String[] { "CodeableConcept", "canonical", "Expression", "DataRequirement" }; 1722 case 907012302: 1723 /* usageContext */ return new String[] { "UsageContext" }; 1724 case -1321148966: 1725 /* exclude */ return new String[] { "boolean" }; 1726 case -750257565: 1727 /* unitOfMeasure */ return new String[] { "CodeableConcept" }; 1728 case 237553470: 1729 /* studyEffectiveDescription */ return new String[] { "string" }; 1730 case -836391458: 1731 /* studyEffective */ return new String[] { "dateTime", "Period", "Duration", "Timing" }; 1732 case -2107828915: 1733 /* studyEffectiveTimeFromStart */ return new String[] { "Duration" }; 1734 case 1284435677: 1735 /* studyEffectiveGroupMeasure */ return new String[] { "code" }; 1736 case 1333186472: 1737 /* participantEffectiveDescription */ return new String[] { "string" }; 1738 case 1376306100: 1739 /* participantEffective */ return new String[] { "dateTime", "Period", "Duration", "Timing" }; 1740 case -1471501513: 1741 /* participantEffectiveTimeFromStart */ return new String[] { "Duration" }; 1742 case 889320371: 1743 /* participantEffectiveGroupMeasure */ return new String[] { "code" }; 1744 default: 1745 return super.getTypesForProperty(hash, name); 1746 } 1747 1748 } 1749 1750 @Override 1751 public Base addChild(String name) throws FHIRException { 1752 if (name.equals("definitionCodeableConcept")) { 1753 this.definition = new CodeableConcept(); 1754 return this.definition; 1755 } else if (name.equals("definitionCanonical")) { 1756 this.definition = new CanonicalType(); 1757 return this.definition; 1758 } else if (name.equals("definitionExpression")) { 1759 this.definition = new Expression(); 1760 return this.definition; 1761 } else if (name.equals("definitionDataRequirement")) { 1762 this.definition = new DataRequirement(); 1763 return this.definition; 1764 } else if (name.equals("usageContext")) { 1765 return addUsageContext(); 1766 } else if (name.equals("exclude")) { 1767 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.exclude"); 1768 } else if (name.equals("unitOfMeasure")) { 1769 this.unitOfMeasure = new CodeableConcept(); 1770 return this.unitOfMeasure; 1771 } else if (name.equals("studyEffectiveDescription")) { 1772 throw new FHIRException( 1773 "Cannot call addChild on a singleton property ResearchElementDefinition.studyEffectiveDescription"); 1774 } else if (name.equals("studyEffectiveDateTime")) { 1775 this.studyEffective = new DateTimeType(); 1776 return this.studyEffective; 1777 } else if (name.equals("studyEffectivePeriod")) { 1778 this.studyEffective = new Period(); 1779 return this.studyEffective; 1780 } else if (name.equals("studyEffectiveDuration")) { 1781 this.studyEffective = new Duration(); 1782 return this.studyEffective; 1783 } else if (name.equals("studyEffectiveTiming")) { 1784 this.studyEffective = new Timing(); 1785 return this.studyEffective; 1786 } else if (name.equals("studyEffectiveTimeFromStart")) { 1787 this.studyEffectiveTimeFromStart = new Duration(); 1788 return this.studyEffectiveTimeFromStart; 1789 } else if (name.equals("studyEffectiveGroupMeasure")) { 1790 throw new FHIRException( 1791 "Cannot call addChild on a singleton property ResearchElementDefinition.studyEffectiveGroupMeasure"); 1792 } else if (name.equals("participantEffectiveDescription")) { 1793 throw new FHIRException( 1794 "Cannot call addChild on a singleton property ResearchElementDefinition.participantEffectiveDescription"); 1795 } else if (name.equals("participantEffectiveDateTime")) { 1796 this.participantEffective = new DateTimeType(); 1797 return this.participantEffective; 1798 } else if (name.equals("participantEffectivePeriod")) { 1799 this.participantEffective = new Period(); 1800 return this.participantEffective; 1801 } else if (name.equals("participantEffectiveDuration")) { 1802 this.participantEffective = new Duration(); 1803 return this.participantEffective; 1804 } else if (name.equals("participantEffectiveTiming")) { 1805 this.participantEffective = new Timing(); 1806 return this.participantEffective; 1807 } else if (name.equals("participantEffectiveTimeFromStart")) { 1808 this.participantEffectiveTimeFromStart = new Duration(); 1809 return this.participantEffectiveTimeFromStart; 1810 } else if (name.equals("participantEffectiveGroupMeasure")) { 1811 throw new FHIRException( 1812 "Cannot call addChild on a singleton property ResearchElementDefinition.participantEffectiveGroupMeasure"); 1813 } else 1814 return super.addChild(name); 1815 } 1816 1817 public ResearchElementDefinitionCharacteristicComponent copy() { 1818 ResearchElementDefinitionCharacteristicComponent dst = new ResearchElementDefinitionCharacteristicComponent(); 1819 copyValues(dst); 1820 return dst; 1821 } 1822 1823 public void copyValues(ResearchElementDefinitionCharacteristicComponent dst) { 1824 super.copyValues(dst); 1825 dst.definition = definition == null ? null : definition.copy(); 1826 if (usageContext != null) { 1827 dst.usageContext = new ArrayList<UsageContext>(); 1828 for (UsageContext i : usageContext) 1829 dst.usageContext.add(i.copy()); 1830 } 1831 ; 1832 dst.exclude = exclude == null ? null : exclude.copy(); 1833 dst.unitOfMeasure = unitOfMeasure == null ? null : unitOfMeasure.copy(); 1834 dst.studyEffectiveDescription = studyEffectiveDescription == null ? null : studyEffectiveDescription.copy(); 1835 dst.studyEffective = studyEffective == null ? null : studyEffective.copy(); 1836 dst.studyEffectiveTimeFromStart = studyEffectiveTimeFromStart == null ? null : studyEffectiveTimeFromStart.copy(); 1837 dst.studyEffectiveGroupMeasure = studyEffectiveGroupMeasure == null ? null : studyEffectiveGroupMeasure.copy(); 1838 dst.participantEffectiveDescription = participantEffectiveDescription == null ? null 1839 : participantEffectiveDescription.copy(); 1840 dst.participantEffective = participantEffective == null ? null : participantEffective.copy(); 1841 dst.participantEffectiveTimeFromStart = participantEffectiveTimeFromStart == null ? null 1842 : participantEffectiveTimeFromStart.copy(); 1843 dst.participantEffectiveGroupMeasure = participantEffectiveGroupMeasure == null ? null 1844 : participantEffectiveGroupMeasure.copy(); 1845 } 1846 1847 @Override 1848 public boolean equalsDeep(Base other_) { 1849 if (!super.equalsDeep(other_)) 1850 return false; 1851 if (!(other_ instanceof ResearchElementDefinitionCharacteristicComponent)) 1852 return false; 1853 ResearchElementDefinitionCharacteristicComponent o = (ResearchElementDefinitionCharacteristicComponent) other_; 1854 return compareDeep(definition, o.definition, true) && compareDeep(usageContext, o.usageContext, true) 1855 && compareDeep(exclude, o.exclude, true) && compareDeep(unitOfMeasure, o.unitOfMeasure, true) 1856 && compareDeep(studyEffectiveDescription, o.studyEffectiveDescription, true) 1857 && compareDeep(studyEffective, o.studyEffective, true) 1858 && compareDeep(studyEffectiveTimeFromStart, o.studyEffectiveTimeFromStart, true) 1859 && compareDeep(studyEffectiveGroupMeasure, o.studyEffectiveGroupMeasure, true) 1860 && compareDeep(participantEffectiveDescription, o.participantEffectiveDescription, true) 1861 && compareDeep(participantEffective, o.participantEffective, true) 1862 && compareDeep(participantEffectiveTimeFromStart, o.participantEffectiveTimeFromStart, true) 1863 && compareDeep(participantEffectiveGroupMeasure, o.participantEffectiveGroupMeasure, true); 1864 } 1865 1866 @Override 1867 public boolean equalsShallow(Base other_) { 1868 if (!super.equalsShallow(other_)) 1869 return false; 1870 if (!(other_ instanceof ResearchElementDefinitionCharacteristicComponent)) 1871 return false; 1872 ResearchElementDefinitionCharacteristicComponent o = (ResearchElementDefinitionCharacteristicComponent) other_; 1873 return compareValues(exclude, o.exclude, true) 1874 && compareValues(studyEffectiveDescription, o.studyEffectiveDescription, true) 1875 && compareValues(studyEffectiveGroupMeasure, o.studyEffectiveGroupMeasure, true) 1876 && compareValues(participantEffectiveDescription, o.participantEffectiveDescription, true) 1877 && compareValues(participantEffectiveGroupMeasure, o.participantEffectiveGroupMeasure, true); 1878 } 1879 1880 public boolean isEmpty() { 1881 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, usageContext, exclude, unitOfMeasure, 1882 studyEffectiveDescription, studyEffective, studyEffectiveTimeFromStart, studyEffectiveGroupMeasure, 1883 participantEffectiveDescription, participantEffective, participantEffectiveTimeFromStart, 1884 participantEffectiveGroupMeasure); 1885 } 1886 1887 public String fhirType() { 1888 return "ResearchElementDefinition.characteristic"; 1889 1890 } 1891 1892 } 1893 1894 /** 1895 * A formal identifier that is used to identify this research element definition 1896 * when it is represented in other formats, or referenced in a specification, 1897 * model, design or an instance. 1898 */ 1899 @Child(name = "identifier", type = { 1900 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1901 @Description(shortDefinition = "Additional identifier for the research element definition", formalDefinition = "A formal identifier that is used to identify this research element definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1902 protected List<Identifier> identifier; 1903 1904 /** 1905 * The short title provides an alternate title for use in informal descriptive 1906 * contexts where the full, formal title is not necessary. 1907 */ 1908 @Child(name = "shortTitle", type = { 1909 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1910 @Description(shortDefinition = "Title for use in informal contexts", formalDefinition = "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.") 1911 protected StringType shortTitle; 1912 1913 /** 1914 * An explanatory or alternate title for the ResearchElementDefinition giving 1915 * additional information about its content. 1916 */ 1917 @Child(name = "subtitle", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1918 @Description(shortDefinition = "Subordinate title of the ResearchElementDefinition", formalDefinition = "An explanatory or alternate title for the ResearchElementDefinition giving additional information about its content.") 1919 protected StringType subtitle; 1920 1921 /** 1922 * The intended subjects for the ResearchElementDefinition. If this element is 1923 * not provided, a Patient subject is assumed, but the subject of the 1924 * ResearchElementDefinition can be anything. 1925 */ 1926 @Child(name = "subject", type = { CodeableConcept.class, 1927 Group.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1928 @Description(shortDefinition = "E.g. Patient, Practitioner, RelatedPerson, Organization, Location, Device", formalDefinition = "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.") 1929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 1930 protected Type subject; 1931 1932 /** 1933 * A human-readable string to clarify or explain concepts about the resource. 1934 */ 1935 @Child(name = "comment", type = { 1936 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1937 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 1938 protected List<StringType> comment; 1939 1940 /** 1941 * Explanation of why this research element definition is needed and why it has 1942 * been designed as it has. 1943 */ 1944 @Child(name = "purpose", type = { 1945 MarkdownType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1946 @Description(shortDefinition = "Why this research element definition is defined", formalDefinition = "Explanation of why this research element definition is needed and why it has been designed as it has.") 1947 protected MarkdownType purpose; 1948 1949 /** 1950 * A detailed description, from a clinical perspective, of how the 1951 * ResearchElementDefinition is used. 1952 */ 1953 @Child(name = "usage", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1954 @Description(shortDefinition = "Describes the clinical usage of the ResearchElementDefinition", formalDefinition = "A detailed description, from a clinical perspective, of how the ResearchElementDefinition is used.") 1955 protected StringType usage; 1956 1957 /** 1958 * A copyright statement relating to the research element definition and/or its 1959 * contents. Copyright statements are generally legal restrictions on the use 1960 * and publishing of the research element definition. 1961 */ 1962 @Child(name = "copyright", type = { 1963 MarkdownType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1964 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the research element definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research element definition.") 1965 protected MarkdownType copyright; 1966 1967 /** 1968 * The date on which the resource content was approved by the publisher. 1969 * Approval happens once when the content is officially approved for usage. 1970 */ 1971 @Child(name = "approvalDate", type = { 1972 DateType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1973 @Description(shortDefinition = "When the research element definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 1974 protected DateType approvalDate; 1975 1976 /** 1977 * The date on which the resource content was last reviewed. Review happens 1978 * periodically after approval but does not change the original approval date. 1979 */ 1980 @Child(name = "lastReviewDate", type = { 1981 DateType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1982 @Description(shortDefinition = "When the research element definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 1983 protected DateType lastReviewDate; 1984 1985 /** 1986 * The period during which the research element definition content was or is 1987 * planned to be in active use. 1988 */ 1989 @Child(name = "effectivePeriod", type = { 1990 Period.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1991 @Description(shortDefinition = "When the research element definition is expected to be used", formalDefinition = "The period during which the research element definition content was or is planned to be in active use.") 1992 protected Period effectivePeriod; 1993 1994 /** 1995 * Descriptive topics related to the content of the ResearchElementDefinition. 1996 * Topics provide a high-level categorization grouping types of 1997 * ResearchElementDefinitions that can be useful for filtering and searching. 1998 */ 1999 @Child(name = "topic", type = { 2000 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2001 @Description(shortDefinition = "The category of the ResearchElementDefinition, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the ResearchElementDefinition. Topics provide a high-level categorization grouping types of ResearchElementDefinitions that can be useful for filtering and searching.") 2002 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 2003 protected List<CodeableConcept> topic; 2004 2005 /** 2006 * An individiual or organization primarily involved in the creation and 2007 * maintenance of the content. 2008 */ 2009 @Child(name = "author", type = { 2010 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2011 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 2012 protected List<ContactDetail> author; 2013 2014 /** 2015 * An individual or organization primarily responsible for internal coherence of 2016 * the content. 2017 */ 2018 @Child(name = "editor", type = { 2019 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2020 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 2021 protected List<ContactDetail> editor; 2022 2023 /** 2024 * An individual or organization primarily responsible for review of some aspect 2025 * of the content. 2026 */ 2027 @Child(name = "reviewer", type = { 2028 ContactDetail.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2029 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 2030 protected List<ContactDetail> reviewer; 2031 2032 /** 2033 * An individual or organization responsible for officially endorsing the 2034 * content for use in some setting. 2035 */ 2036 @Child(name = "endorser", type = { 2037 ContactDetail.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2038 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 2039 protected List<ContactDetail> endorser; 2040 2041 /** 2042 * Related artifacts such as additional documentation, justification, or 2043 * bibliographic references. 2044 */ 2045 @Child(name = "relatedArtifact", type = { 2046 RelatedArtifact.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2047 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 2048 protected List<RelatedArtifact> relatedArtifact; 2049 2050 /** 2051 * A reference to a Library resource containing the formal logic used by the 2052 * ResearchElementDefinition. 2053 */ 2054 @Child(name = "library", type = { 2055 CanonicalType.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2056 @Description(shortDefinition = "Logic used by the ResearchElementDefinition", formalDefinition = "A reference to a Library resource containing the formal logic used by the ResearchElementDefinition.") 2057 protected List<CanonicalType> library; 2058 2059 /** 2060 * The type of research element, a population, an exposure, or an outcome. 2061 */ 2062 @Child(name = "type", type = { CodeType.class }, order = 18, min = 1, max = 1, modifier = false, summary = true) 2063 @Description(shortDefinition = "population | exposure | outcome", formalDefinition = "The type of research element, a population, an exposure, or an outcome.") 2064 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/research-element-type") 2065 protected Enumeration<ResearchElementType> type; 2066 2067 /** 2068 * The type of the outcome (e.g. Dichotomous, Continuous, or Descriptive). 2069 */ 2070 @Child(name = "variableType", type = { 2071 CodeType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 2072 @Description(shortDefinition = "dichotomous | continuous | descriptive", formalDefinition = "The type of the outcome (e.g. Dichotomous, Continuous, or Descriptive).") 2073 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/variable-type") 2074 protected Enumeration<VariableType> variableType; 2075 2076 /** 2077 * A characteristic that defines the members of the research element. Multiple 2078 * characteristics are applied with "and" semantics. 2079 */ 2080 @Child(name = "characteristic", type = {}, order = 20, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2081 @Description(shortDefinition = "What defines the members of the research element", formalDefinition = "A characteristic that defines the members of the research element. Multiple characteristics are applied with \"and\" semantics.") 2082 protected List<ResearchElementDefinitionCharacteristicComponent> characteristic; 2083 2084 private static final long serialVersionUID = 1483216033L; 2085 2086 /** 2087 * Constructor 2088 */ 2089 public ResearchElementDefinition() { 2090 super(); 2091 } 2092 2093 /** 2094 * Constructor 2095 */ 2096 public ResearchElementDefinition(Enumeration<PublicationStatus> status, Enumeration<ResearchElementType> type) { 2097 super(); 2098 this.status = status; 2099 this.type = type; 2100 } 2101 2102 /** 2103 * @return {@link #url} (An absolute URI that is used to identify this research 2104 * element definition when it is referenced in a specification, model, 2105 * design or an instance; also called its canonical identifier. This 2106 * SHOULD be globally unique and SHOULD be a literal address at which at 2107 * which an authoritative instance of this research element definition 2108 * is (or will be) published. This URL can be the target of a canonical 2109 * reference. It SHALL remain the same when the research element 2110 * definition is stored on different servers.). This is the underlying 2111 * object with id, value and extensions. The accessor "getUrl" gives 2112 * direct access to the value 2113 */ 2114 public UriType getUrlElement() { 2115 if (this.url == null) 2116 if (Configuration.errorOnAutoCreate()) 2117 throw new Error("Attempt to auto-create ResearchElementDefinition.url"); 2118 else if (Configuration.doAutoCreate()) 2119 this.url = new UriType(); // bb 2120 return this.url; 2121 } 2122 2123 public boolean hasUrlElement() { 2124 return this.url != null && !this.url.isEmpty(); 2125 } 2126 2127 public boolean hasUrl() { 2128 return this.url != null && !this.url.isEmpty(); 2129 } 2130 2131 /** 2132 * @param value {@link #url} (An absolute URI that is used to identify this 2133 * research element definition when it is referenced in a 2134 * specification, model, design or an instance; also called its 2135 * canonical identifier. This SHOULD be globally unique and SHOULD 2136 * be a literal address at which at which an authoritative instance 2137 * of this research element definition is (or will be) published. 2138 * This URL can be the target of a canonical reference. It SHALL 2139 * remain the same when the research element definition is stored 2140 * on different servers.). This is the underlying object with id, 2141 * value and extensions. The accessor "getUrl" gives direct access 2142 * to the value 2143 */ 2144 public ResearchElementDefinition setUrlElement(UriType value) { 2145 this.url = value; 2146 return this; 2147 } 2148 2149 /** 2150 * @return An absolute URI that is used to identify this research element 2151 * definition when it is referenced in a specification, model, design or 2152 * an instance; also called its canonical identifier. This SHOULD be 2153 * globally unique and SHOULD be a literal address at which at which an 2154 * authoritative instance of this research element definition is (or 2155 * will be) published. This URL can be the target of a canonical 2156 * reference. It SHALL remain the same when the research element 2157 * definition is stored on different servers. 2158 */ 2159 public String getUrl() { 2160 return this.url == null ? null : this.url.getValue(); 2161 } 2162 2163 /** 2164 * @param value An absolute URI that is used to identify this research element 2165 * definition when it is referenced in a specification, model, 2166 * design or an instance; also called its canonical identifier. 2167 * This SHOULD be globally unique and SHOULD be a literal address 2168 * at which at which an authoritative instance of this research 2169 * element definition is (or will be) published. This URL can be 2170 * the target of a canonical reference. It SHALL remain the same 2171 * when the research element definition is stored on different 2172 * servers. 2173 */ 2174 public ResearchElementDefinition setUrl(String value) { 2175 if (Utilities.noString(value)) 2176 this.url = null; 2177 else { 2178 if (this.url == null) 2179 this.url = new UriType(); 2180 this.url.setValue(value); 2181 } 2182 return this; 2183 } 2184 2185 /** 2186 * @return {@link #identifier} (A formal identifier that is used to identify 2187 * this research element definition when it is represented in other 2188 * formats, or referenced in a specification, model, design or an 2189 * instance.) 2190 */ 2191 public List<Identifier> getIdentifier() { 2192 if (this.identifier == null) 2193 this.identifier = new ArrayList<Identifier>(); 2194 return this.identifier; 2195 } 2196 2197 /** 2198 * @return Returns a reference to <code>this</code> for easy method chaining 2199 */ 2200 public ResearchElementDefinition setIdentifier(List<Identifier> theIdentifier) { 2201 this.identifier = theIdentifier; 2202 return this; 2203 } 2204 2205 public boolean hasIdentifier() { 2206 if (this.identifier == null) 2207 return false; 2208 for (Identifier item : this.identifier) 2209 if (!item.isEmpty()) 2210 return true; 2211 return false; 2212 } 2213 2214 public Identifier addIdentifier() { // 3 2215 Identifier t = new Identifier(); 2216 if (this.identifier == null) 2217 this.identifier = new ArrayList<Identifier>(); 2218 this.identifier.add(t); 2219 return t; 2220 } 2221 2222 public ResearchElementDefinition addIdentifier(Identifier t) { // 3 2223 if (t == null) 2224 return this; 2225 if (this.identifier == null) 2226 this.identifier = new ArrayList<Identifier>(); 2227 this.identifier.add(t); 2228 return this; 2229 } 2230 2231 /** 2232 * @return The first repetition of repeating field {@link #identifier}, creating 2233 * it if it does not already exist 2234 */ 2235 public Identifier getIdentifierFirstRep() { 2236 if (getIdentifier().isEmpty()) { 2237 addIdentifier(); 2238 } 2239 return getIdentifier().get(0); 2240 } 2241 2242 /** 2243 * @return {@link #version} (The identifier that is used to identify this 2244 * version of the research element definition when it is referenced in a 2245 * specification, model, design or instance. This is an arbitrary value 2246 * managed by the research element definition author and is not expected 2247 * to be globally unique. For example, it might be a timestamp (e.g. 2248 * yyyymmdd) if a managed version is not available. There is also no 2249 * expectation that versions can be placed in a lexicographical 2250 * sequence. To provide a version consistent with the Decision Support 2251 * Service specification, use the format Major.Minor.Revision (e.g. 2252 * 1.0.0). For more information on versioning knowledge assets, refer to 2253 * the Decision Support Service specification. Note that a version is 2254 * required for non-experimental active artifacts.). This is the 2255 * underlying object with id, value and extensions. The accessor 2256 * "getVersion" gives direct access to the value 2257 */ 2258 public StringType getVersionElement() { 2259 if (this.version == null) 2260 if (Configuration.errorOnAutoCreate()) 2261 throw new Error("Attempt to auto-create ResearchElementDefinition.version"); 2262 else if (Configuration.doAutoCreate()) 2263 this.version = new StringType(); // bb 2264 return this.version; 2265 } 2266 2267 public boolean hasVersionElement() { 2268 return this.version != null && !this.version.isEmpty(); 2269 } 2270 2271 public boolean hasVersion() { 2272 return this.version != null && !this.version.isEmpty(); 2273 } 2274 2275 /** 2276 * @param value {@link #version} (The identifier that is used to identify this 2277 * version of the research element definition when it is referenced 2278 * in a specification, model, design or instance. This is an 2279 * arbitrary value managed by the research element definition 2280 * author and is not expected to be globally unique. For example, 2281 * it might be a timestamp (e.g. yyyymmdd) if a managed version is 2282 * not available. There is also no expectation that versions can be 2283 * placed in a lexicographical sequence. To provide a version 2284 * consistent with the Decision Support Service specification, use 2285 * the format Major.Minor.Revision (e.g. 1.0.0). For more 2286 * information on versioning knowledge assets, refer to the 2287 * Decision Support Service specification. Note that a version is 2288 * required for non-experimental active artifacts.). This is the 2289 * underlying object with id, value and extensions. The accessor 2290 * "getVersion" gives direct access to the value 2291 */ 2292 public ResearchElementDefinition setVersionElement(StringType value) { 2293 this.version = value; 2294 return this; 2295 } 2296 2297 /** 2298 * @return The identifier that is used to identify this version of the research 2299 * element definition when it is referenced in a specification, model, 2300 * design or instance. This is an arbitrary value managed by the 2301 * research element definition author and is not expected to be globally 2302 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if a 2303 * managed version is not available. There is also no expectation that 2304 * versions can be placed in a lexicographical sequence. To provide a 2305 * version consistent with the Decision Support Service specification, 2306 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 2307 * information on versioning knowledge assets, refer to the Decision 2308 * Support Service specification. Note that a version is required for 2309 * non-experimental active artifacts. 2310 */ 2311 public String getVersion() { 2312 return this.version == null ? null : this.version.getValue(); 2313 } 2314 2315 /** 2316 * @param value The identifier that is used to identify this version of the 2317 * research element definition when it is referenced in a 2318 * specification, model, design or instance. This is an arbitrary 2319 * value managed by the research element definition author and is 2320 * not expected to be globally unique. For example, it might be a 2321 * timestamp (e.g. yyyymmdd) if a managed version is not available. 2322 * There is also no expectation that versions can be placed in a 2323 * lexicographical sequence. To provide a version consistent with 2324 * the Decision Support Service specification, use the format 2325 * Major.Minor.Revision (e.g. 1.0.0). For more information on 2326 * versioning knowledge assets, refer to the Decision Support 2327 * Service specification. Note that a version is required for 2328 * non-experimental active artifacts. 2329 */ 2330 public ResearchElementDefinition setVersion(String value) { 2331 if (Utilities.noString(value)) 2332 this.version = null; 2333 else { 2334 if (this.version == null) 2335 this.version = new StringType(); 2336 this.version.setValue(value); 2337 } 2338 return this; 2339 } 2340 2341 /** 2342 * @return {@link #name} (A natural language name identifying the research 2343 * element definition. This name should be usable as an identifier for 2344 * the module by machine processing applications such as code 2345 * generation.). This is the underlying object with id, value and 2346 * extensions. The accessor "getName" gives direct access to the value 2347 */ 2348 public StringType getNameElement() { 2349 if (this.name == null) 2350 if (Configuration.errorOnAutoCreate()) 2351 throw new Error("Attempt to auto-create ResearchElementDefinition.name"); 2352 else if (Configuration.doAutoCreate()) 2353 this.name = new StringType(); // bb 2354 return this.name; 2355 } 2356 2357 public boolean hasNameElement() { 2358 return this.name != null && !this.name.isEmpty(); 2359 } 2360 2361 public boolean hasName() { 2362 return this.name != null && !this.name.isEmpty(); 2363 } 2364 2365 /** 2366 * @param value {@link #name} (A natural language name identifying the research 2367 * element definition. This name should be usable as an identifier 2368 * for the module by machine processing applications such as code 2369 * generation.). This is the underlying object with id, value and 2370 * extensions. The accessor "getName" gives direct access to the 2371 * value 2372 */ 2373 public ResearchElementDefinition setNameElement(StringType value) { 2374 this.name = value; 2375 return this; 2376 } 2377 2378 /** 2379 * @return A natural language name identifying the research element definition. 2380 * This name should be usable as an identifier for the module by machine 2381 * processing applications such as code generation. 2382 */ 2383 public String getName() { 2384 return this.name == null ? null : this.name.getValue(); 2385 } 2386 2387 /** 2388 * @param value A natural language name identifying the research element 2389 * definition. This name should be usable as an identifier for the 2390 * module by machine processing applications such as code 2391 * generation. 2392 */ 2393 public ResearchElementDefinition setName(String value) { 2394 if (Utilities.noString(value)) 2395 this.name = null; 2396 else { 2397 if (this.name == null) 2398 this.name = new StringType(); 2399 this.name.setValue(value); 2400 } 2401 return this; 2402 } 2403 2404 /** 2405 * @return {@link #title} (A short, descriptive, user-friendly title for the 2406 * research element definition.). This is the underlying object with id, 2407 * value and extensions. The accessor "getTitle" gives direct access to 2408 * the value 2409 */ 2410 public StringType getTitleElement() { 2411 if (this.title == null) 2412 if (Configuration.errorOnAutoCreate()) 2413 throw new Error("Attempt to auto-create ResearchElementDefinition.title"); 2414 else if (Configuration.doAutoCreate()) 2415 this.title = new StringType(); // bb 2416 return this.title; 2417 } 2418 2419 public boolean hasTitleElement() { 2420 return this.title != null && !this.title.isEmpty(); 2421 } 2422 2423 public boolean hasTitle() { 2424 return this.title != null && !this.title.isEmpty(); 2425 } 2426 2427 /** 2428 * @param value {@link #title} (A short, descriptive, user-friendly title for 2429 * the research element definition.). This is the underlying object 2430 * with id, value and extensions. The accessor "getTitle" gives 2431 * direct access to the value 2432 */ 2433 public ResearchElementDefinition setTitleElement(StringType value) { 2434 this.title = value; 2435 return this; 2436 } 2437 2438 /** 2439 * @return A short, descriptive, user-friendly title for the research element 2440 * definition. 2441 */ 2442 public String getTitle() { 2443 return this.title == null ? null : this.title.getValue(); 2444 } 2445 2446 /** 2447 * @param value A short, descriptive, user-friendly title for the research 2448 * element definition. 2449 */ 2450 public ResearchElementDefinition setTitle(String value) { 2451 if (Utilities.noString(value)) 2452 this.title = null; 2453 else { 2454 if (this.title == null) 2455 this.title = new StringType(); 2456 this.title.setValue(value); 2457 } 2458 return this; 2459 } 2460 2461 /** 2462 * @return {@link #shortTitle} (The short title provides an alternate title for 2463 * use in informal descriptive contexts where the full, formal title is 2464 * not necessary.). This is the underlying object with id, value and 2465 * extensions. The accessor "getShortTitle" gives direct access to the 2466 * value 2467 */ 2468 public StringType getShortTitleElement() { 2469 if (this.shortTitle == null) 2470 if (Configuration.errorOnAutoCreate()) 2471 throw new Error("Attempt to auto-create ResearchElementDefinition.shortTitle"); 2472 else if (Configuration.doAutoCreate()) 2473 this.shortTitle = new StringType(); // bb 2474 return this.shortTitle; 2475 } 2476 2477 public boolean hasShortTitleElement() { 2478 return this.shortTitle != null && !this.shortTitle.isEmpty(); 2479 } 2480 2481 public boolean hasShortTitle() { 2482 return this.shortTitle != null && !this.shortTitle.isEmpty(); 2483 } 2484 2485 /** 2486 * @param value {@link #shortTitle} (The short title provides an alternate title 2487 * for use in informal descriptive contexts where the full, formal 2488 * title is not necessary.). This is the underlying object with id, 2489 * value and extensions. The accessor "getShortTitle" gives direct 2490 * access to the value 2491 */ 2492 public ResearchElementDefinition setShortTitleElement(StringType value) { 2493 this.shortTitle = value; 2494 return this; 2495 } 2496 2497 /** 2498 * @return The short title provides an alternate title for use in informal 2499 * descriptive contexts where the full, formal title is not necessary. 2500 */ 2501 public String getShortTitle() { 2502 return this.shortTitle == null ? null : this.shortTitle.getValue(); 2503 } 2504 2505 /** 2506 * @param value The short title provides an alternate title for use in informal 2507 * descriptive contexts where the full, formal title is not 2508 * necessary. 2509 */ 2510 public ResearchElementDefinition setShortTitle(String value) { 2511 if (Utilities.noString(value)) 2512 this.shortTitle = null; 2513 else { 2514 if (this.shortTitle == null) 2515 this.shortTitle = new StringType(); 2516 this.shortTitle.setValue(value); 2517 } 2518 return this; 2519 } 2520 2521 /** 2522 * @return {@link #subtitle} (An explanatory or alternate title for the 2523 * ResearchElementDefinition giving additional information about its 2524 * content.). This is the underlying object with id, value and 2525 * extensions. The accessor "getSubtitle" gives direct access to the 2526 * value 2527 */ 2528 public StringType getSubtitleElement() { 2529 if (this.subtitle == null) 2530 if (Configuration.errorOnAutoCreate()) 2531 throw new Error("Attempt to auto-create ResearchElementDefinition.subtitle"); 2532 else if (Configuration.doAutoCreate()) 2533 this.subtitle = new StringType(); // bb 2534 return this.subtitle; 2535 } 2536 2537 public boolean hasSubtitleElement() { 2538 return this.subtitle != null && !this.subtitle.isEmpty(); 2539 } 2540 2541 public boolean hasSubtitle() { 2542 return this.subtitle != null && !this.subtitle.isEmpty(); 2543 } 2544 2545 /** 2546 * @param value {@link #subtitle} (An explanatory or alternate title for the 2547 * ResearchElementDefinition giving additional information about 2548 * its content.). This is the underlying object with id, value and 2549 * extensions. The accessor "getSubtitle" gives direct access to 2550 * the value 2551 */ 2552 public ResearchElementDefinition setSubtitleElement(StringType value) { 2553 this.subtitle = value; 2554 return this; 2555 } 2556 2557 /** 2558 * @return An explanatory or alternate title for the ResearchElementDefinition 2559 * giving additional information about its content. 2560 */ 2561 public String getSubtitle() { 2562 return this.subtitle == null ? null : this.subtitle.getValue(); 2563 } 2564 2565 /** 2566 * @param value An explanatory or alternate title for the 2567 * ResearchElementDefinition giving additional information about 2568 * its content. 2569 */ 2570 public ResearchElementDefinition setSubtitle(String value) { 2571 if (Utilities.noString(value)) 2572 this.subtitle = null; 2573 else { 2574 if (this.subtitle == null) 2575 this.subtitle = new StringType(); 2576 this.subtitle.setValue(value); 2577 } 2578 return this; 2579 } 2580 2581 /** 2582 * @return {@link #status} (The status of this research element definition. 2583 * Enables tracking the life-cycle of the content.). This is the 2584 * underlying object with id, value and extensions. The accessor 2585 * "getStatus" gives direct access to the value 2586 */ 2587 public Enumeration<PublicationStatus> getStatusElement() { 2588 if (this.status == null) 2589 if (Configuration.errorOnAutoCreate()) 2590 throw new Error("Attempt to auto-create ResearchElementDefinition.status"); 2591 else if (Configuration.doAutoCreate()) 2592 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2593 return this.status; 2594 } 2595 2596 public boolean hasStatusElement() { 2597 return this.status != null && !this.status.isEmpty(); 2598 } 2599 2600 public boolean hasStatus() { 2601 return this.status != null && !this.status.isEmpty(); 2602 } 2603 2604 /** 2605 * @param value {@link #status} (The status of this research element definition. 2606 * Enables tracking the life-cycle of the content.). This is the 2607 * underlying object with id, value and extensions. The accessor 2608 * "getStatus" gives direct access to the value 2609 */ 2610 public ResearchElementDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2611 this.status = value; 2612 return this; 2613 } 2614 2615 /** 2616 * @return The status of this research element definition. Enables tracking the 2617 * life-cycle of the content. 2618 */ 2619 public PublicationStatus getStatus() { 2620 return this.status == null ? null : this.status.getValue(); 2621 } 2622 2623 /** 2624 * @param value The status of this research element definition. Enables tracking 2625 * the life-cycle of the content. 2626 */ 2627 public ResearchElementDefinition setStatus(PublicationStatus value) { 2628 if (this.status == null) 2629 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2630 this.status.setValue(value); 2631 return this; 2632 } 2633 2634 /** 2635 * @return {@link #experimental} (A Boolean value to indicate that this research 2636 * element definition is authored for testing purposes (or 2637 * education/evaluation/marketing) and is not intended to be used for 2638 * genuine usage.). This is the underlying object with id, value and 2639 * extensions. The accessor "getExperimental" gives direct access to the 2640 * value 2641 */ 2642 public BooleanType getExperimentalElement() { 2643 if (this.experimental == null) 2644 if (Configuration.errorOnAutoCreate()) 2645 throw new Error("Attempt to auto-create ResearchElementDefinition.experimental"); 2646 else if (Configuration.doAutoCreate()) 2647 this.experimental = new BooleanType(); // bb 2648 return this.experimental; 2649 } 2650 2651 public boolean hasExperimentalElement() { 2652 return this.experimental != null && !this.experimental.isEmpty(); 2653 } 2654 2655 public boolean hasExperimental() { 2656 return this.experimental != null && !this.experimental.isEmpty(); 2657 } 2658 2659 /** 2660 * @param value {@link #experimental} (A Boolean value to indicate that this 2661 * research element definition is authored for testing purposes (or 2662 * education/evaluation/marketing) and is not intended to be used 2663 * for genuine usage.). This is the underlying object with id, 2664 * value and extensions. The accessor "getExperimental" gives 2665 * direct access to the value 2666 */ 2667 public ResearchElementDefinition setExperimentalElement(BooleanType value) { 2668 this.experimental = value; 2669 return this; 2670 } 2671 2672 /** 2673 * @return A Boolean value to indicate that this research element definition is 2674 * authored for testing purposes (or education/evaluation/marketing) and 2675 * is not intended to be used for genuine usage. 2676 */ 2677 public boolean getExperimental() { 2678 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2679 } 2680 2681 /** 2682 * @param value A Boolean value to indicate that this research element 2683 * definition is authored for testing purposes (or 2684 * education/evaluation/marketing) and is not intended to be used 2685 * for genuine usage. 2686 */ 2687 public ResearchElementDefinition setExperimental(boolean value) { 2688 if (this.experimental == null) 2689 this.experimental = new BooleanType(); 2690 this.experimental.setValue(value); 2691 return this; 2692 } 2693 2694 /** 2695 * @return {@link #subject} (The intended subjects for the 2696 * ResearchElementDefinition. If this element is not provided, a Patient 2697 * subject is assumed, but the subject of the ResearchElementDefinition 2698 * can be anything.) 2699 */ 2700 public Type getSubject() { 2701 return this.subject; 2702 } 2703 2704 /** 2705 * @return {@link #subject} (The intended subjects for the 2706 * ResearchElementDefinition. If this element is not provided, a Patient 2707 * subject is assumed, but the subject of the ResearchElementDefinition 2708 * can be anything.) 2709 */ 2710 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 2711 if (this.subject == null) 2712 this.subject = new CodeableConcept(); 2713 if (!(this.subject instanceof CodeableConcept)) 2714 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2715 + this.subject.getClass().getName() + " was encountered"); 2716 return (CodeableConcept) this.subject; 2717 } 2718 2719 public boolean hasSubjectCodeableConcept() { 2720 return this != null && this.subject instanceof CodeableConcept; 2721 } 2722 2723 /** 2724 * @return {@link #subject} (The intended subjects for the 2725 * ResearchElementDefinition. If this element is not provided, a Patient 2726 * subject is assumed, but the subject of the ResearchElementDefinition 2727 * can be anything.) 2728 */ 2729 public Reference getSubjectReference() throws FHIRException { 2730 if (this.subject == null) 2731 this.subject = new Reference(); 2732 if (!(this.subject instanceof Reference)) 2733 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 2734 + " was encountered"); 2735 return (Reference) this.subject; 2736 } 2737 2738 public boolean hasSubjectReference() { 2739 return this != null && this.subject instanceof Reference; 2740 } 2741 2742 public boolean hasSubject() { 2743 return this.subject != null && !this.subject.isEmpty(); 2744 } 2745 2746 /** 2747 * @param value {@link #subject} (The intended subjects for the 2748 * ResearchElementDefinition. If this element is not provided, a 2749 * Patient subject is assumed, but the subject of the 2750 * ResearchElementDefinition can be anything.) 2751 */ 2752 public ResearchElementDefinition setSubject(Type value) { 2753 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2754 throw new Error("Not the right type for ResearchElementDefinition.subject[x]: " + value.fhirType()); 2755 this.subject = value; 2756 return this; 2757 } 2758 2759 /** 2760 * @return {@link #date} (The date (and optionally time) when the research 2761 * element definition was published. The date must change when the 2762 * business version changes and it must change if the status code 2763 * changes. In addition, it should change when the substantive content 2764 * of the research element definition changes.). This is the underlying 2765 * object with id, value and extensions. The accessor "getDate" gives 2766 * direct access to the value 2767 */ 2768 public DateTimeType getDateElement() { 2769 if (this.date == null) 2770 if (Configuration.errorOnAutoCreate()) 2771 throw new Error("Attempt to auto-create ResearchElementDefinition.date"); 2772 else if (Configuration.doAutoCreate()) 2773 this.date = new DateTimeType(); // bb 2774 return this.date; 2775 } 2776 2777 public boolean hasDateElement() { 2778 return this.date != null && !this.date.isEmpty(); 2779 } 2780 2781 public boolean hasDate() { 2782 return this.date != null && !this.date.isEmpty(); 2783 } 2784 2785 /** 2786 * @param value {@link #date} (The date (and optionally time) when the research 2787 * element definition was published. The date must change when the 2788 * business version changes and it must change if the status code 2789 * changes. In addition, it should change when the substantive 2790 * content of the research element definition changes.). This is 2791 * the underlying object with id, value and extensions. The 2792 * accessor "getDate" gives direct access to the value 2793 */ 2794 public ResearchElementDefinition setDateElement(DateTimeType value) { 2795 this.date = value; 2796 return this; 2797 } 2798 2799 /** 2800 * @return The date (and optionally time) when the research element definition 2801 * was published. The date must change when the business version changes 2802 * and it must change if the status code changes. In addition, it should 2803 * change when the substantive content of the research element 2804 * definition changes. 2805 */ 2806 public Date getDate() { 2807 return this.date == null ? null : this.date.getValue(); 2808 } 2809 2810 /** 2811 * @param value The date (and optionally time) when the research element 2812 * definition was published. The date must change when the business 2813 * version changes and it must change if the status code changes. 2814 * In addition, it should change when the substantive content of 2815 * the research element definition changes. 2816 */ 2817 public ResearchElementDefinition setDate(Date value) { 2818 if (value == null) 2819 this.date = null; 2820 else { 2821 if (this.date == null) 2822 this.date = new DateTimeType(); 2823 this.date.setValue(value); 2824 } 2825 return this; 2826 } 2827 2828 /** 2829 * @return {@link #publisher} (The name of the organization or individual that 2830 * published the research element definition.). This is the underlying 2831 * object with id, value and extensions. The accessor "getPublisher" 2832 * gives direct access to the value 2833 */ 2834 public StringType getPublisherElement() { 2835 if (this.publisher == null) 2836 if (Configuration.errorOnAutoCreate()) 2837 throw new Error("Attempt to auto-create ResearchElementDefinition.publisher"); 2838 else if (Configuration.doAutoCreate()) 2839 this.publisher = new StringType(); // bb 2840 return this.publisher; 2841 } 2842 2843 public boolean hasPublisherElement() { 2844 return this.publisher != null && !this.publisher.isEmpty(); 2845 } 2846 2847 public boolean hasPublisher() { 2848 return this.publisher != null && !this.publisher.isEmpty(); 2849 } 2850 2851 /** 2852 * @param value {@link #publisher} (The name of the organization or individual 2853 * that published the research element definition.). This is the 2854 * underlying object with id, value and extensions. The accessor 2855 * "getPublisher" gives direct access to the value 2856 */ 2857 public ResearchElementDefinition setPublisherElement(StringType value) { 2858 this.publisher = value; 2859 return this; 2860 } 2861 2862 /** 2863 * @return The name of the organization or individual that published the 2864 * research element definition. 2865 */ 2866 public String getPublisher() { 2867 return this.publisher == null ? null : this.publisher.getValue(); 2868 } 2869 2870 /** 2871 * @param value The name of the organization or individual that published the 2872 * research element definition. 2873 */ 2874 public ResearchElementDefinition setPublisher(String value) { 2875 if (Utilities.noString(value)) 2876 this.publisher = null; 2877 else { 2878 if (this.publisher == null) 2879 this.publisher = new StringType(); 2880 this.publisher.setValue(value); 2881 } 2882 return this; 2883 } 2884 2885 /** 2886 * @return {@link #contact} (Contact details to assist a user in finding and 2887 * communicating with the publisher.) 2888 */ 2889 public List<ContactDetail> getContact() { 2890 if (this.contact == null) 2891 this.contact = new ArrayList<ContactDetail>(); 2892 return this.contact; 2893 } 2894 2895 /** 2896 * @return Returns a reference to <code>this</code> for easy method chaining 2897 */ 2898 public ResearchElementDefinition setContact(List<ContactDetail> theContact) { 2899 this.contact = theContact; 2900 return this; 2901 } 2902 2903 public boolean hasContact() { 2904 if (this.contact == null) 2905 return false; 2906 for (ContactDetail item : this.contact) 2907 if (!item.isEmpty()) 2908 return true; 2909 return false; 2910 } 2911 2912 public ContactDetail addContact() { // 3 2913 ContactDetail t = new ContactDetail(); 2914 if (this.contact == null) 2915 this.contact = new ArrayList<ContactDetail>(); 2916 this.contact.add(t); 2917 return t; 2918 } 2919 2920 public ResearchElementDefinition addContact(ContactDetail t) { // 3 2921 if (t == null) 2922 return this; 2923 if (this.contact == null) 2924 this.contact = new ArrayList<ContactDetail>(); 2925 this.contact.add(t); 2926 return this; 2927 } 2928 2929 /** 2930 * @return The first repetition of repeating field {@link #contact}, creating it 2931 * if it does not already exist 2932 */ 2933 public ContactDetail getContactFirstRep() { 2934 if (getContact().isEmpty()) { 2935 addContact(); 2936 } 2937 return getContact().get(0); 2938 } 2939 2940 /** 2941 * @return {@link #description} (A free text natural language description of the 2942 * research element definition from a consumer's perspective.). This is 2943 * the underlying object with id, value and extensions. The accessor 2944 * "getDescription" gives direct access to the value 2945 */ 2946 public MarkdownType getDescriptionElement() { 2947 if (this.description == null) 2948 if (Configuration.errorOnAutoCreate()) 2949 throw new Error("Attempt to auto-create ResearchElementDefinition.description"); 2950 else if (Configuration.doAutoCreate()) 2951 this.description = new MarkdownType(); // bb 2952 return this.description; 2953 } 2954 2955 public boolean hasDescriptionElement() { 2956 return this.description != null && !this.description.isEmpty(); 2957 } 2958 2959 public boolean hasDescription() { 2960 return this.description != null && !this.description.isEmpty(); 2961 } 2962 2963 /** 2964 * @param value {@link #description} (A free text natural language description 2965 * of the research element definition from a consumer's 2966 * perspective.). This is the underlying object with id, value and 2967 * extensions. The accessor "getDescription" gives direct access to 2968 * the value 2969 */ 2970 public ResearchElementDefinition setDescriptionElement(MarkdownType value) { 2971 this.description = value; 2972 return this; 2973 } 2974 2975 /** 2976 * @return A free text natural language description of the research element 2977 * definition from a consumer's perspective. 2978 */ 2979 public String getDescription() { 2980 return this.description == null ? null : this.description.getValue(); 2981 } 2982 2983 /** 2984 * @param value A free text natural language description of the research element 2985 * definition from a consumer's perspective. 2986 */ 2987 public ResearchElementDefinition setDescription(String value) { 2988 if (value == null) 2989 this.description = null; 2990 else { 2991 if (this.description == null) 2992 this.description = new MarkdownType(); 2993 this.description.setValue(value); 2994 } 2995 return this; 2996 } 2997 2998 /** 2999 * @return {@link #comment} (A human-readable string to clarify or explain 3000 * concepts about the resource.) 3001 */ 3002 public List<StringType> getComment() { 3003 if (this.comment == null) 3004 this.comment = new ArrayList<StringType>(); 3005 return this.comment; 3006 } 3007 3008 /** 3009 * @return Returns a reference to <code>this</code> for easy method chaining 3010 */ 3011 public ResearchElementDefinition setComment(List<StringType> theComment) { 3012 this.comment = theComment; 3013 return this; 3014 } 3015 3016 public boolean hasComment() { 3017 if (this.comment == null) 3018 return false; 3019 for (StringType item : this.comment) 3020 if (!item.isEmpty()) 3021 return true; 3022 return false; 3023 } 3024 3025 /** 3026 * @return {@link #comment} (A human-readable string to clarify or explain 3027 * concepts about the resource.) 3028 */ 3029 public StringType addCommentElement() {// 2 3030 StringType t = new StringType(); 3031 if (this.comment == null) 3032 this.comment = new ArrayList<StringType>(); 3033 this.comment.add(t); 3034 return t; 3035 } 3036 3037 /** 3038 * @param value {@link #comment} (A human-readable string to clarify or explain 3039 * concepts about the resource.) 3040 */ 3041 public ResearchElementDefinition addComment(String value) { // 1 3042 StringType t = new StringType(); 3043 t.setValue(value); 3044 if (this.comment == null) 3045 this.comment = new ArrayList<StringType>(); 3046 this.comment.add(t); 3047 return this; 3048 } 3049 3050 /** 3051 * @param value {@link #comment} (A human-readable string to clarify or explain 3052 * concepts about the resource.) 3053 */ 3054 public boolean hasComment(String value) { 3055 if (this.comment == null) 3056 return false; 3057 for (StringType v : this.comment) 3058 if (v.getValue().equals(value)) // string 3059 return true; 3060 return false; 3061 } 3062 3063 /** 3064 * @return {@link #useContext} (The content was developed with a focus and 3065 * intent of supporting the contexts that are listed. These contexts may 3066 * be general categories (gender, age, ...) or may be references to 3067 * specific programs (insurance plans, studies, ...) and may be used to 3068 * assist with indexing and searching for appropriate research element 3069 * definition instances.) 3070 */ 3071 public List<UsageContext> getUseContext() { 3072 if (this.useContext == null) 3073 this.useContext = new ArrayList<UsageContext>(); 3074 return this.useContext; 3075 } 3076 3077 /** 3078 * @return Returns a reference to <code>this</code> for easy method chaining 3079 */ 3080 public ResearchElementDefinition setUseContext(List<UsageContext> theUseContext) { 3081 this.useContext = theUseContext; 3082 return this; 3083 } 3084 3085 public boolean hasUseContext() { 3086 if (this.useContext == null) 3087 return false; 3088 for (UsageContext item : this.useContext) 3089 if (!item.isEmpty()) 3090 return true; 3091 return false; 3092 } 3093 3094 public UsageContext addUseContext() { // 3 3095 UsageContext t = new UsageContext(); 3096 if (this.useContext == null) 3097 this.useContext = new ArrayList<UsageContext>(); 3098 this.useContext.add(t); 3099 return t; 3100 } 3101 3102 public ResearchElementDefinition addUseContext(UsageContext t) { // 3 3103 if (t == null) 3104 return this; 3105 if (this.useContext == null) 3106 this.useContext = new ArrayList<UsageContext>(); 3107 this.useContext.add(t); 3108 return this; 3109 } 3110 3111 /** 3112 * @return The first repetition of repeating field {@link #useContext}, creating 3113 * it if it does not already exist 3114 */ 3115 public UsageContext getUseContextFirstRep() { 3116 if (getUseContext().isEmpty()) { 3117 addUseContext(); 3118 } 3119 return getUseContext().get(0); 3120 } 3121 3122 /** 3123 * @return {@link #jurisdiction} (A legal or geographic region in which the 3124 * research element definition is intended to be used.) 3125 */ 3126 public List<CodeableConcept> getJurisdiction() { 3127 if (this.jurisdiction == null) 3128 this.jurisdiction = new ArrayList<CodeableConcept>(); 3129 return this.jurisdiction; 3130 } 3131 3132 /** 3133 * @return Returns a reference to <code>this</code> for easy method chaining 3134 */ 3135 public ResearchElementDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 3136 this.jurisdiction = theJurisdiction; 3137 return this; 3138 } 3139 3140 public boolean hasJurisdiction() { 3141 if (this.jurisdiction == null) 3142 return false; 3143 for (CodeableConcept item : this.jurisdiction) 3144 if (!item.isEmpty()) 3145 return true; 3146 return false; 3147 } 3148 3149 public CodeableConcept addJurisdiction() { // 3 3150 CodeableConcept t = new CodeableConcept(); 3151 if (this.jurisdiction == null) 3152 this.jurisdiction = new ArrayList<CodeableConcept>(); 3153 this.jurisdiction.add(t); 3154 return t; 3155 } 3156 3157 public ResearchElementDefinition addJurisdiction(CodeableConcept t) { // 3 3158 if (t == null) 3159 return this; 3160 if (this.jurisdiction == null) 3161 this.jurisdiction = new ArrayList<CodeableConcept>(); 3162 this.jurisdiction.add(t); 3163 return this; 3164 } 3165 3166 /** 3167 * @return The first repetition of repeating field {@link #jurisdiction}, 3168 * creating it if it does not already exist 3169 */ 3170 public CodeableConcept getJurisdictionFirstRep() { 3171 if (getJurisdiction().isEmpty()) { 3172 addJurisdiction(); 3173 } 3174 return getJurisdiction().get(0); 3175 } 3176 3177 /** 3178 * @return {@link #purpose} (Explanation of why this research element definition 3179 * is needed and why it has been designed as it has.). This is the 3180 * underlying object with id, value and extensions. The accessor 3181 * "getPurpose" gives direct access to the value 3182 */ 3183 public MarkdownType getPurposeElement() { 3184 if (this.purpose == null) 3185 if (Configuration.errorOnAutoCreate()) 3186 throw new Error("Attempt to auto-create ResearchElementDefinition.purpose"); 3187 else if (Configuration.doAutoCreate()) 3188 this.purpose = new MarkdownType(); // bb 3189 return this.purpose; 3190 } 3191 3192 public boolean hasPurposeElement() { 3193 return this.purpose != null && !this.purpose.isEmpty(); 3194 } 3195 3196 public boolean hasPurpose() { 3197 return this.purpose != null && !this.purpose.isEmpty(); 3198 } 3199 3200 /** 3201 * @param value {@link #purpose} (Explanation of why this research element 3202 * definition is needed and why it has been designed as it has.). 3203 * This is the underlying object with id, value and extensions. The 3204 * accessor "getPurpose" gives direct access to the value 3205 */ 3206 public ResearchElementDefinition setPurposeElement(MarkdownType value) { 3207 this.purpose = value; 3208 return this; 3209 } 3210 3211 /** 3212 * @return Explanation of why this research element definition is needed and why 3213 * it has been designed as it has. 3214 */ 3215 public String getPurpose() { 3216 return this.purpose == null ? null : this.purpose.getValue(); 3217 } 3218 3219 /** 3220 * @param value Explanation of why this research element definition is needed 3221 * and why it has been designed as it has. 3222 */ 3223 public ResearchElementDefinition setPurpose(String value) { 3224 if (value == null) 3225 this.purpose = null; 3226 else { 3227 if (this.purpose == null) 3228 this.purpose = new MarkdownType(); 3229 this.purpose.setValue(value); 3230 } 3231 return this; 3232 } 3233 3234 /** 3235 * @return {@link #usage} (A detailed description, from a clinical perspective, 3236 * of how the ResearchElementDefinition is used.). This is the 3237 * underlying object with id, value and extensions. The accessor 3238 * "getUsage" gives direct access to the value 3239 */ 3240 public StringType getUsageElement() { 3241 if (this.usage == null) 3242 if (Configuration.errorOnAutoCreate()) 3243 throw new Error("Attempt to auto-create ResearchElementDefinition.usage"); 3244 else if (Configuration.doAutoCreate()) 3245 this.usage = new StringType(); // bb 3246 return this.usage; 3247 } 3248 3249 public boolean hasUsageElement() { 3250 return this.usage != null && !this.usage.isEmpty(); 3251 } 3252 3253 public boolean hasUsage() { 3254 return this.usage != null && !this.usage.isEmpty(); 3255 } 3256 3257 /** 3258 * @param value {@link #usage} (A detailed description, from a clinical 3259 * perspective, of how the ResearchElementDefinition is used.). 3260 * This is the underlying object with id, value and extensions. The 3261 * accessor "getUsage" gives direct access to the value 3262 */ 3263 public ResearchElementDefinition setUsageElement(StringType value) { 3264 this.usage = value; 3265 return this; 3266 } 3267 3268 /** 3269 * @return A detailed description, from a clinical perspective, of how the 3270 * ResearchElementDefinition is used. 3271 */ 3272 public String getUsage() { 3273 return this.usage == null ? null : this.usage.getValue(); 3274 } 3275 3276 /** 3277 * @param value A detailed description, from a clinical perspective, of how the 3278 * ResearchElementDefinition is used. 3279 */ 3280 public ResearchElementDefinition setUsage(String value) { 3281 if (Utilities.noString(value)) 3282 this.usage = null; 3283 else { 3284 if (this.usage == null) 3285 this.usage = new StringType(); 3286 this.usage.setValue(value); 3287 } 3288 return this; 3289 } 3290 3291 /** 3292 * @return {@link #copyright} (A copyright statement relating to the research 3293 * element definition and/or its contents. Copyright statements are 3294 * generally legal restrictions on the use and publishing of the 3295 * research element definition.). This is the underlying object with id, 3296 * value and extensions. The accessor "getCopyright" gives direct access 3297 * to the value 3298 */ 3299 public MarkdownType getCopyrightElement() { 3300 if (this.copyright == null) 3301 if (Configuration.errorOnAutoCreate()) 3302 throw new Error("Attempt to auto-create ResearchElementDefinition.copyright"); 3303 else if (Configuration.doAutoCreate()) 3304 this.copyright = new MarkdownType(); // bb 3305 return this.copyright; 3306 } 3307 3308 public boolean hasCopyrightElement() { 3309 return this.copyright != null && !this.copyright.isEmpty(); 3310 } 3311 3312 public boolean hasCopyright() { 3313 return this.copyright != null && !this.copyright.isEmpty(); 3314 } 3315 3316 /** 3317 * @param value {@link #copyright} (A copyright statement relating to the 3318 * research element definition and/or its contents. Copyright 3319 * statements are generally legal restrictions on the use and 3320 * publishing of the research element definition.). This is the 3321 * underlying object with id, value and extensions. The accessor 3322 * "getCopyright" gives direct access to the value 3323 */ 3324 public ResearchElementDefinition setCopyrightElement(MarkdownType value) { 3325 this.copyright = value; 3326 return this; 3327 } 3328 3329 /** 3330 * @return A copyright statement relating to the research element definition 3331 * and/or its contents. Copyright statements are generally legal 3332 * restrictions on the use and publishing of the research element 3333 * definition. 3334 */ 3335 public String getCopyright() { 3336 return this.copyright == null ? null : this.copyright.getValue(); 3337 } 3338 3339 /** 3340 * @param value A copyright statement relating to the research element 3341 * definition and/or its contents. Copyright statements are 3342 * generally legal restrictions on the use and publishing of the 3343 * research element definition. 3344 */ 3345 public ResearchElementDefinition setCopyright(String value) { 3346 if (value == null) 3347 this.copyright = null; 3348 else { 3349 if (this.copyright == null) 3350 this.copyright = new MarkdownType(); 3351 this.copyright.setValue(value); 3352 } 3353 return this; 3354 } 3355 3356 /** 3357 * @return {@link #approvalDate} (The date on which the resource content was 3358 * approved by the publisher. Approval happens once when the content is 3359 * officially approved for usage.). This is the underlying object with 3360 * id, value and extensions. The accessor "getApprovalDate" gives direct 3361 * access to the value 3362 */ 3363 public DateType getApprovalDateElement() { 3364 if (this.approvalDate == null) 3365 if (Configuration.errorOnAutoCreate()) 3366 throw new Error("Attempt to auto-create ResearchElementDefinition.approvalDate"); 3367 else if (Configuration.doAutoCreate()) 3368 this.approvalDate = new DateType(); // bb 3369 return this.approvalDate; 3370 } 3371 3372 public boolean hasApprovalDateElement() { 3373 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3374 } 3375 3376 public boolean hasApprovalDate() { 3377 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3378 } 3379 3380 /** 3381 * @param value {@link #approvalDate} (The date on which the resource content 3382 * was approved by the publisher. Approval happens once when the 3383 * content is officially approved for usage.). This is the 3384 * underlying object with id, value and extensions. The accessor 3385 * "getApprovalDate" gives direct access to the value 3386 */ 3387 public ResearchElementDefinition setApprovalDateElement(DateType value) { 3388 this.approvalDate = value; 3389 return this; 3390 } 3391 3392 /** 3393 * @return The date on which the resource content was approved by the publisher. 3394 * Approval happens once when the content is officially approved for 3395 * usage. 3396 */ 3397 public Date getApprovalDate() { 3398 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3399 } 3400 3401 /** 3402 * @param value The date on which the resource content was approved by the 3403 * publisher. Approval happens once when the content is officially 3404 * approved for usage. 3405 */ 3406 public ResearchElementDefinition setApprovalDate(Date value) { 3407 if (value == null) 3408 this.approvalDate = null; 3409 else { 3410 if (this.approvalDate == null) 3411 this.approvalDate = new DateType(); 3412 this.approvalDate.setValue(value); 3413 } 3414 return this; 3415 } 3416 3417 /** 3418 * @return {@link #lastReviewDate} (The date on which the resource content was 3419 * last reviewed. Review happens periodically after approval but does 3420 * not change the original approval date.). This is the underlying 3421 * object with id, value and extensions. The accessor 3422 * "getLastReviewDate" gives direct access to the value 3423 */ 3424 public DateType getLastReviewDateElement() { 3425 if (this.lastReviewDate == null) 3426 if (Configuration.errorOnAutoCreate()) 3427 throw new Error("Attempt to auto-create ResearchElementDefinition.lastReviewDate"); 3428 else if (Configuration.doAutoCreate()) 3429 this.lastReviewDate = new DateType(); // bb 3430 return this.lastReviewDate; 3431 } 3432 3433 public boolean hasLastReviewDateElement() { 3434 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3435 } 3436 3437 public boolean hasLastReviewDate() { 3438 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3439 } 3440 3441 /** 3442 * @param value {@link #lastReviewDate} (The date on which the resource content 3443 * was last reviewed. Review happens periodically after approval 3444 * but does not change the original approval date.). This is the 3445 * underlying object with id, value and extensions. The accessor 3446 * "getLastReviewDate" gives direct access to the value 3447 */ 3448 public ResearchElementDefinition setLastReviewDateElement(DateType value) { 3449 this.lastReviewDate = value; 3450 return this; 3451 } 3452 3453 /** 3454 * @return The date on which the resource content was last reviewed. Review 3455 * happens periodically after approval but does not change the original 3456 * approval date. 3457 */ 3458 public Date getLastReviewDate() { 3459 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3460 } 3461 3462 /** 3463 * @param value The date on which the resource content was last reviewed. Review 3464 * happens periodically after approval but does not change the 3465 * original approval date. 3466 */ 3467 public ResearchElementDefinition setLastReviewDate(Date value) { 3468 if (value == null) 3469 this.lastReviewDate = null; 3470 else { 3471 if (this.lastReviewDate == null) 3472 this.lastReviewDate = new DateType(); 3473 this.lastReviewDate.setValue(value); 3474 } 3475 return this; 3476 } 3477 3478 /** 3479 * @return {@link #effectivePeriod} (The period during which the research 3480 * element definition content was or is planned to be in active use.) 3481 */ 3482 public Period getEffectivePeriod() { 3483 if (this.effectivePeriod == null) 3484 if (Configuration.errorOnAutoCreate()) 3485 throw new Error("Attempt to auto-create ResearchElementDefinition.effectivePeriod"); 3486 else if (Configuration.doAutoCreate()) 3487 this.effectivePeriod = new Period(); // cc 3488 return this.effectivePeriod; 3489 } 3490 3491 public boolean hasEffectivePeriod() { 3492 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3493 } 3494 3495 /** 3496 * @param value {@link #effectivePeriod} (The period during which the research 3497 * element definition content was or is planned to be in active 3498 * use.) 3499 */ 3500 public ResearchElementDefinition setEffectivePeriod(Period value) { 3501 this.effectivePeriod = value; 3502 return this; 3503 } 3504 3505 /** 3506 * @return {@link #topic} (Descriptive topics related to the content of the 3507 * ResearchElementDefinition. Topics provide a high-level categorization 3508 * grouping types of ResearchElementDefinitions that can be useful for 3509 * filtering and searching.) 3510 */ 3511 public List<CodeableConcept> getTopic() { 3512 if (this.topic == null) 3513 this.topic = new ArrayList<CodeableConcept>(); 3514 return this.topic; 3515 } 3516 3517 /** 3518 * @return Returns a reference to <code>this</code> for easy method chaining 3519 */ 3520 public ResearchElementDefinition setTopic(List<CodeableConcept> theTopic) { 3521 this.topic = theTopic; 3522 return this; 3523 } 3524 3525 public boolean hasTopic() { 3526 if (this.topic == null) 3527 return false; 3528 for (CodeableConcept item : this.topic) 3529 if (!item.isEmpty()) 3530 return true; 3531 return false; 3532 } 3533 3534 public CodeableConcept addTopic() { // 3 3535 CodeableConcept t = new CodeableConcept(); 3536 if (this.topic == null) 3537 this.topic = new ArrayList<CodeableConcept>(); 3538 this.topic.add(t); 3539 return t; 3540 } 3541 3542 public ResearchElementDefinition addTopic(CodeableConcept t) { // 3 3543 if (t == null) 3544 return this; 3545 if (this.topic == null) 3546 this.topic = new ArrayList<CodeableConcept>(); 3547 this.topic.add(t); 3548 return this; 3549 } 3550 3551 /** 3552 * @return The first repetition of repeating field {@link #topic}, creating it 3553 * if it does not already exist 3554 */ 3555 public CodeableConcept getTopicFirstRep() { 3556 if (getTopic().isEmpty()) { 3557 addTopic(); 3558 } 3559 return getTopic().get(0); 3560 } 3561 3562 /** 3563 * @return {@link #author} (An individiual or organization primarily involved in 3564 * the creation and maintenance of the content.) 3565 */ 3566 public List<ContactDetail> getAuthor() { 3567 if (this.author == null) 3568 this.author = new ArrayList<ContactDetail>(); 3569 return this.author; 3570 } 3571 3572 /** 3573 * @return Returns a reference to <code>this</code> for easy method chaining 3574 */ 3575 public ResearchElementDefinition setAuthor(List<ContactDetail> theAuthor) { 3576 this.author = theAuthor; 3577 return this; 3578 } 3579 3580 public boolean hasAuthor() { 3581 if (this.author == null) 3582 return false; 3583 for (ContactDetail item : this.author) 3584 if (!item.isEmpty()) 3585 return true; 3586 return false; 3587 } 3588 3589 public ContactDetail addAuthor() { // 3 3590 ContactDetail t = new ContactDetail(); 3591 if (this.author == null) 3592 this.author = new ArrayList<ContactDetail>(); 3593 this.author.add(t); 3594 return t; 3595 } 3596 3597 public ResearchElementDefinition addAuthor(ContactDetail t) { // 3 3598 if (t == null) 3599 return this; 3600 if (this.author == null) 3601 this.author = new ArrayList<ContactDetail>(); 3602 this.author.add(t); 3603 return this; 3604 } 3605 3606 /** 3607 * @return The first repetition of repeating field {@link #author}, creating it 3608 * if it does not already exist 3609 */ 3610 public ContactDetail getAuthorFirstRep() { 3611 if (getAuthor().isEmpty()) { 3612 addAuthor(); 3613 } 3614 return getAuthor().get(0); 3615 } 3616 3617 /** 3618 * @return {@link #editor} (An individual or organization primarily responsible 3619 * for internal coherence of the content.) 3620 */ 3621 public List<ContactDetail> getEditor() { 3622 if (this.editor == null) 3623 this.editor = new ArrayList<ContactDetail>(); 3624 return this.editor; 3625 } 3626 3627 /** 3628 * @return Returns a reference to <code>this</code> for easy method chaining 3629 */ 3630 public ResearchElementDefinition setEditor(List<ContactDetail> theEditor) { 3631 this.editor = theEditor; 3632 return this; 3633 } 3634 3635 public boolean hasEditor() { 3636 if (this.editor == null) 3637 return false; 3638 for (ContactDetail item : this.editor) 3639 if (!item.isEmpty()) 3640 return true; 3641 return false; 3642 } 3643 3644 public ContactDetail addEditor() { // 3 3645 ContactDetail t = new ContactDetail(); 3646 if (this.editor == null) 3647 this.editor = new ArrayList<ContactDetail>(); 3648 this.editor.add(t); 3649 return t; 3650 } 3651 3652 public ResearchElementDefinition addEditor(ContactDetail t) { // 3 3653 if (t == null) 3654 return this; 3655 if (this.editor == null) 3656 this.editor = new ArrayList<ContactDetail>(); 3657 this.editor.add(t); 3658 return this; 3659 } 3660 3661 /** 3662 * @return The first repetition of repeating field {@link #editor}, creating it 3663 * if it does not already exist 3664 */ 3665 public ContactDetail getEditorFirstRep() { 3666 if (getEditor().isEmpty()) { 3667 addEditor(); 3668 } 3669 return getEditor().get(0); 3670 } 3671 3672 /** 3673 * @return {@link #reviewer} (An individual or organization primarily 3674 * responsible for review of some aspect of the content.) 3675 */ 3676 public List<ContactDetail> getReviewer() { 3677 if (this.reviewer == null) 3678 this.reviewer = new ArrayList<ContactDetail>(); 3679 return this.reviewer; 3680 } 3681 3682 /** 3683 * @return Returns a reference to <code>this</code> for easy method chaining 3684 */ 3685 public ResearchElementDefinition setReviewer(List<ContactDetail> theReviewer) { 3686 this.reviewer = theReviewer; 3687 return this; 3688 } 3689 3690 public boolean hasReviewer() { 3691 if (this.reviewer == null) 3692 return false; 3693 for (ContactDetail item : this.reviewer) 3694 if (!item.isEmpty()) 3695 return true; 3696 return false; 3697 } 3698 3699 public ContactDetail addReviewer() { // 3 3700 ContactDetail t = new ContactDetail(); 3701 if (this.reviewer == null) 3702 this.reviewer = new ArrayList<ContactDetail>(); 3703 this.reviewer.add(t); 3704 return t; 3705 } 3706 3707 public ResearchElementDefinition addReviewer(ContactDetail t) { // 3 3708 if (t == null) 3709 return this; 3710 if (this.reviewer == null) 3711 this.reviewer = new ArrayList<ContactDetail>(); 3712 this.reviewer.add(t); 3713 return this; 3714 } 3715 3716 /** 3717 * @return The first repetition of repeating field {@link #reviewer}, creating 3718 * it if it does not already exist 3719 */ 3720 public ContactDetail getReviewerFirstRep() { 3721 if (getReviewer().isEmpty()) { 3722 addReviewer(); 3723 } 3724 return getReviewer().get(0); 3725 } 3726 3727 /** 3728 * @return {@link #endorser} (An individual or organization responsible for 3729 * officially endorsing the content for use in some setting.) 3730 */ 3731 public List<ContactDetail> getEndorser() { 3732 if (this.endorser == null) 3733 this.endorser = new ArrayList<ContactDetail>(); 3734 return this.endorser; 3735 } 3736 3737 /** 3738 * @return Returns a reference to <code>this</code> for easy method chaining 3739 */ 3740 public ResearchElementDefinition setEndorser(List<ContactDetail> theEndorser) { 3741 this.endorser = theEndorser; 3742 return this; 3743 } 3744 3745 public boolean hasEndorser() { 3746 if (this.endorser == null) 3747 return false; 3748 for (ContactDetail item : this.endorser) 3749 if (!item.isEmpty()) 3750 return true; 3751 return false; 3752 } 3753 3754 public ContactDetail addEndorser() { // 3 3755 ContactDetail t = new ContactDetail(); 3756 if (this.endorser == null) 3757 this.endorser = new ArrayList<ContactDetail>(); 3758 this.endorser.add(t); 3759 return t; 3760 } 3761 3762 public ResearchElementDefinition addEndorser(ContactDetail t) { // 3 3763 if (t == null) 3764 return this; 3765 if (this.endorser == null) 3766 this.endorser = new ArrayList<ContactDetail>(); 3767 this.endorser.add(t); 3768 return this; 3769 } 3770 3771 /** 3772 * @return The first repetition of repeating field {@link #endorser}, creating 3773 * it if it does not already exist 3774 */ 3775 public ContactDetail getEndorserFirstRep() { 3776 if (getEndorser().isEmpty()) { 3777 addEndorser(); 3778 } 3779 return getEndorser().get(0); 3780 } 3781 3782 /** 3783 * @return {@link #relatedArtifact} (Related artifacts such as additional 3784 * documentation, justification, or bibliographic references.) 3785 */ 3786 public List<RelatedArtifact> getRelatedArtifact() { 3787 if (this.relatedArtifact == null) 3788 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3789 return this.relatedArtifact; 3790 } 3791 3792 /** 3793 * @return Returns a reference to <code>this</code> for easy method chaining 3794 */ 3795 public ResearchElementDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3796 this.relatedArtifact = theRelatedArtifact; 3797 return this; 3798 } 3799 3800 public boolean hasRelatedArtifact() { 3801 if (this.relatedArtifact == null) 3802 return false; 3803 for (RelatedArtifact item : this.relatedArtifact) 3804 if (!item.isEmpty()) 3805 return true; 3806 return false; 3807 } 3808 3809 public RelatedArtifact addRelatedArtifact() { // 3 3810 RelatedArtifact t = new RelatedArtifact(); 3811 if (this.relatedArtifact == null) 3812 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3813 this.relatedArtifact.add(t); 3814 return t; 3815 } 3816 3817 public ResearchElementDefinition addRelatedArtifact(RelatedArtifact t) { // 3 3818 if (t == null) 3819 return this; 3820 if (this.relatedArtifact == null) 3821 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3822 this.relatedArtifact.add(t); 3823 return this; 3824 } 3825 3826 /** 3827 * @return The first repetition of repeating field {@link #relatedArtifact}, 3828 * creating it if it does not already exist 3829 */ 3830 public RelatedArtifact getRelatedArtifactFirstRep() { 3831 if (getRelatedArtifact().isEmpty()) { 3832 addRelatedArtifact(); 3833 } 3834 return getRelatedArtifact().get(0); 3835 } 3836 3837 /** 3838 * @return {@link #library} (A reference to a Library resource containing the 3839 * formal logic used by the ResearchElementDefinition.) 3840 */ 3841 public List<CanonicalType> getLibrary() { 3842 if (this.library == null) 3843 this.library = new ArrayList<CanonicalType>(); 3844 return this.library; 3845 } 3846 3847 /** 3848 * @return Returns a reference to <code>this</code> for easy method chaining 3849 */ 3850 public ResearchElementDefinition setLibrary(List<CanonicalType> theLibrary) { 3851 this.library = theLibrary; 3852 return this; 3853 } 3854 3855 public boolean hasLibrary() { 3856 if (this.library == null) 3857 return false; 3858 for (CanonicalType item : this.library) 3859 if (!item.isEmpty()) 3860 return true; 3861 return false; 3862 } 3863 3864 /** 3865 * @return {@link #library} (A reference to a Library resource containing the 3866 * formal logic used by the ResearchElementDefinition.) 3867 */ 3868 public CanonicalType addLibraryElement() {// 2 3869 CanonicalType t = new CanonicalType(); 3870 if (this.library == null) 3871 this.library = new ArrayList<CanonicalType>(); 3872 this.library.add(t); 3873 return t; 3874 } 3875 3876 /** 3877 * @param value {@link #library} (A reference to a Library resource containing 3878 * the formal logic used by the ResearchElementDefinition.) 3879 */ 3880 public ResearchElementDefinition addLibrary(String value) { // 1 3881 CanonicalType t = new CanonicalType(); 3882 t.setValue(value); 3883 if (this.library == null) 3884 this.library = new ArrayList<CanonicalType>(); 3885 this.library.add(t); 3886 return this; 3887 } 3888 3889 /** 3890 * @param value {@link #library} (A reference to a Library resource containing 3891 * the formal logic used by the ResearchElementDefinition.) 3892 */ 3893 public boolean hasLibrary(String value) { 3894 if (this.library == null) 3895 return false; 3896 for (CanonicalType v : this.library) 3897 if (v.getValue().equals(value)) // canonical(Library) 3898 return true; 3899 return false; 3900 } 3901 3902 /** 3903 * @return {@link #type} (The type of research element, a population, an 3904 * exposure, or an outcome.). This is the underlying object with id, 3905 * value and extensions. The accessor "getType" gives direct access to 3906 * the value 3907 */ 3908 public Enumeration<ResearchElementType> getTypeElement() { 3909 if (this.type == null) 3910 if (Configuration.errorOnAutoCreate()) 3911 throw new Error("Attempt to auto-create ResearchElementDefinition.type"); 3912 else if (Configuration.doAutoCreate()) 3913 this.type = new Enumeration<ResearchElementType>(new ResearchElementTypeEnumFactory()); // bb 3914 return this.type; 3915 } 3916 3917 public boolean hasTypeElement() { 3918 return this.type != null && !this.type.isEmpty(); 3919 } 3920 3921 public boolean hasType() { 3922 return this.type != null && !this.type.isEmpty(); 3923 } 3924 3925 /** 3926 * @param value {@link #type} (The type of research element, a population, an 3927 * exposure, or an outcome.). This is the underlying object with 3928 * id, value and extensions. The accessor "getType" gives direct 3929 * access to the value 3930 */ 3931 public ResearchElementDefinition setTypeElement(Enumeration<ResearchElementType> value) { 3932 this.type = value; 3933 return this; 3934 } 3935 3936 /** 3937 * @return The type of research element, a population, an exposure, or an 3938 * outcome. 3939 */ 3940 public ResearchElementType getType() { 3941 return this.type == null ? null : this.type.getValue(); 3942 } 3943 3944 /** 3945 * @param value The type of research element, a population, an exposure, or an 3946 * outcome. 3947 */ 3948 public ResearchElementDefinition setType(ResearchElementType value) { 3949 if (this.type == null) 3950 this.type = new Enumeration<ResearchElementType>(new ResearchElementTypeEnumFactory()); 3951 this.type.setValue(value); 3952 return this; 3953 } 3954 3955 /** 3956 * @return {@link #variableType} (The type of the outcome (e.g. Dichotomous, 3957 * Continuous, or Descriptive).). This is the underlying object with id, 3958 * value and extensions. The accessor "getVariableType" gives direct 3959 * access to the value 3960 */ 3961 public Enumeration<VariableType> getVariableTypeElement() { 3962 if (this.variableType == null) 3963 if (Configuration.errorOnAutoCreate()) 3964 throw new Error("Attempt to auto-create ResearchElementDefinition.variableType"); 3965 else if (Configuration.doAutoCreate()) 3966 this.variableType = new Enumeration<VariableType>(new VariableTypeEnumFactory()); // bb 3967 return this.variableType; 3968 } 3969 3970 public boolean hasVariableTypeElement() { 3971 return this.variableType != null && !this.variableType.isEmpty(); 3972 } 3973 3974 public boolean hasVariableType() { 3975 return this.variableType != null && !this.variableType.isEmpty(); 3976 } 3977 3978 /** 3979 * @param value {@link #variableType} (The type of the outcome (e.g. 3980 * Dichotomous, Continuous, or Descriptive).). This is the 3981 * underlying object with id, value and extensions. The accessor 3982 * "getVariableType" gives direct access to the value 3983 */ 3984 public ResearchElementDefinition setVariableTypeElement(Enumeration<VariableType> value) { 3985 this.variableType = value; 3986 return this; 3987 } 3988 3989 /** 3990 * @return The type of the outcome (e.g. Dichotomous, Continuous, or 3991 * Descriptive). 3992 */ 3993 public VariableType getVariableType() { 3994 return this.variableType == null ? null : this.variableType.getValue(); 3995 } 3996 3997 /** 3998 * @param value The type of the outcome (e.g. Dichotomous, Continuous, or 3999 * Descriptive). 4000 */ 4001 public ResearchElementDefinition setVariableType(VariableType value) { 4002 if (value == null) 4003 this.variableType = null; 4004 else { 4005 if (this.variableType == null) 4006 this.variableType = new Enumeration<VariableType>(new VariableTypeEnumFactory()); 4007 this.variableType.setValue(value); 4008 } 4009 return this; 4010 } 4011 4012 /** 4013 * @return {@link #characteristic} (A characteristic that defines the members of 4014 * the research element. Multiple characteristics are applied with "and" 4015 * semantics.) 4016 */ 4017 public List<ResearchElementDefinitionCharacteristicComponent> getCharacteristic() { 4018 if (this.characteristic == null) 4019 this.characteristic = new ArrayList<ResearchElementDefinitionCharacteristicComponent>(); 4020 return this.characteristic; 4021 } 4022 4023 /** 4024 * @return Returns a reference to <code>this</code> for easy method chaining 4025 */ 4026 public ResearchElementDefinition setCharacteristic( 4027 List<ResearchElementDefinitionCharacteristicComponent> theCharacteristic) { 4028 this.characteristic = theCharacteristic; 4029 return this; 4030 } 4031 4032 public boolean hasCharacteristic() { 4033 if (this.characteristic == null) 4034 return false; 4035 for (ResearchElementDefinitionCharacteristicComponent item : this.characteristic) 4036 if (!item.isEmpty()) 4037 return true; 4038 return false; 4039 } 4040 4041 public ResearchElementDefinitionCharacteristicComponent addCharacteristic() { // 3 4042 ResearchElementDefinitionCharacteristicComponent t = new ResearchElementDefinitionCharacteristicComponent(); 4043 if (this.characteristic == null) 4044 this.characteristic = new ArrayList<ResearchElementDefinitionCharacteristicComponent>(); 4045 this.characteristic.add(t); 4046 return t; 4047 } 4048 4049 public ResearchElementDefinition addCharacteristic(ResearchElementDefinitionCharacteristicComponent t) { // 3 4050 if (t == null) 4051 return this; 4052 if (this.characteristic == null) 4053 this.characteristic = new ArrayList<ResearchElementDefinitionCharacteristicComponent>(); 4054 this.characteristic.add(t); 4055 return this; 4056 } 4057 4058 /** 4059 * @return The first repetition of repeating field {@link #characteristic}, 4060 * creating it if it does not already exist 4061 */ 4062 public ResearchElementDefinitionCharacteristicComponent getCharacteristicFirstRep() { 4063 if (getCharacteristic().isEmpty()) { 4064 addCharacteristic(); 4065 } 4066 return getCharacteristic().get(0); 4067 } 4068 4069 protected void listChildren(List<Property> children) { 4070 super.listChildren(children); 4071 children.add(new Property("url", "uri", 4072 "An absolute URI that is used to identify this research element definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this research element definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the research element definition is stored on different servers.", 4073 0, 1, url)); 4074 children.add(new Property("identifier", "Identifier", 4075 "A formal identifier that is used to identify this research element definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4076 0, java.lang.Integer.MAX_VALUE, identifier)); 4077 children.add(new Property("version", "string", 4078 "The identifier that is used to identify this version of the research element definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the research element definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 4079 0, 1, version)); 4080 children.add(new Property("name", "string", 4081 "A natural language name identifying the research element definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4082 0, 1, name)); 4083 children.add(new Property("title", "string", 4084 "A short, descriptive, user-friendly title for the research element definition.", 0, 1, title)); 4085 children.add(new Property("shortTitle", "string", 4086 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 4087 0, 1, shortTitle)); 4088 children.add(new Property("subtitle", "string", 4089 "An explanatory or alternate title for the ResearchElementDefinition giving additional information about its content.", 4090 0, 1, subtitle)); 4091 children.add(new Property("status", "code", 4092 "The status of this research element definition. Enables tracking the life-cycle of the content.", 0, 1, 4093 status)); 4094 children.add(new Property("experimental", "boolean", 4095 "A Boolean value to indicate that this research element definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4096 0, 1, experimental)); 4097 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 4098 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4099 0, 1, subject)); 4100 children.add(new Property("date", "dateTime", 4101 "The date (and optionally time) when the research element definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the research element definition changes.", 4102 0, 1, date)); 4103 children.add(new Property("publisher", "string", 4104 "The name of the organization or individual that published the research element definition.", 0, 1, publisher)); 4105 children.add(new Property("contact", "ContactDetail", 4106 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4107 java.lang.Integer.MAX_VALUE, contact)); 4108 children.add(new Property("description", "markdown", 4109 "A free text natural language description of the research element definition from a consumer's perspective.", 0, 4110 1, description)); 4111 children.add( 4112 new Property("comment", "string", "A human-readable string to clarify or explain concepts about the resource.", 4113 0, java.lang.Integer.MAX_VALUE, comment)); 4114 children.add(new Property("useContext", "UsageContext", 4115 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate research element definition instances.", 4116 0, java.lang.Integer.MAX_VALUE, useContext)); 4117 children.add(new Property("jurisdiction", "CodeableConcept", 4118 "A legal or geographic region in which the research element definition is intended to be used.", 0, 4119 java.lang.Integer.MAX_VALUE, jurisdiction)); 4120 children.add(new Property("purpose", "markdown", 4121 "Explanation of why this research element definition is needed and why it has been designed as it has.", 0, 1, 4122 purpose)); 4123 children.add(new Property("usage", "string", 4124 "A detailed description, from a clinical perspective, of how the ResearchElementDefinition is used.", 0, 1, 4125 usage)); 4126 children.add(new Property("copyright", "markdown", 4127 "A copyright statement relating to the research element definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research element definition.", 4128 0, 1, copyright)); 4129 children.add(new Property("approvalDate", "date", 4130 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4131 0, 1, approvalDate)); 4132 children.add(new Property("lastReviewDate", "date", 4133 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4134 0, 1, lastReviewDate)); 4135 children.add(new Property("effectivePeriod", "Period", 4136 "The period during which the research element definition content was or is planned to be in active use.", 0, 1, 4137 effectivePeriod)); 4138 children.add(new Property("topic", "CodeableConcept", 4139 "Descriptive topics related to the content of the ResearchElementDefinition. Topics provide a high-level categorization grouping types of ResearchElementDefinitions that can be useful for filtering and searching.", 4140 0, java.lang.Integer.MAX_VALUE, topic)); 4141 children.add(new Property("author", "ContactDetail", 4142 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4143 java.lang.Integer.MAX_VALUE, author)); 4144 children.add(new Property("editor", "ContactDetail", 4145 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4146 java.lang.Integer.MAX_VALUE, editor)); 4147 children.add(new Property("reviewer", "ContactDetail", 4148 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4149 java.lang.Integer.MAX_VALUE, reviewer)); 4150 children.add(new Property("endorser", "ContactDetail", 4151 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4152 java.lang.Integer.MAX_VALUE, endorser)); 4153 children.add(new Property("relatedArtifact", "RelatedArtifact", 4154 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4155 java.lang.Integer.MAX_VALUE, relatedArtifact)); 4156 children.add(new Property("library", "canonical(Library)", 4157 "A reference to a Library resource containing the formal logic used by the ResearchElementDefinition.", 0, 4158 java.lang.Integer.MAX_VALUE, library)); 4159 children.add(new Property("type", "code", "The type of research element, a population, an exposure, or an outcome.", 4160 0, 1, type)); 4161 children.add(new Property("variableType", "code", 4162 "The type of the outcome (e.g. Dichotomous, Continuous, or Descriptive).", 0, 1, variableType)); 4163 children.add(new Property("characteristic", "", 4164 "A characteristic that defines the members of the research element. Multiple characteristics are applied with \"and\" semantics.", 4165 0, java.lang.Integer.MAX_VALUE, characteristic)); 4166 } 4167 4168 @Override 4169 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4170 switch (_hash) { 4171 case 116079: 4172 /* url */ return new Property("url", "uri", 4173 "An absolute URI that is used to identify this research element definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this research element definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the research element definition is stored on different servers.", 4174 0, 1, url); 4175 case -1618432855: 4176 /* identifier */ return new Property("identifier", "Identifier", 4177 "A formal identifier that is used to identify this research element definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4178 0, java.lang.Integer.MAX_VALUE, identifier); 4179 case 351608024: 4180 /* version */ return new Property("version", "string", 4181 "The identifier that is used to identify this version of the research element definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the research element definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 4182 0, 1, version); 4183 case 3373707: 4184 /* name */ return new Property("name", "string", 4185 "A natural language name identifying the research element definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4186 0, 1, name); 4187 case 110371416: 4188 /* title */ return new Property("title", "string", 4189 "A short, descriptive, user-friendly title for the research element definition.", 0, 1, title); 4190 case 1555503932: 4191 /* shortTitle */ return new Property("shortTitle", "string", 4192 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 4193 0, 1, shortTitle); 4194 case -2060497896: 4195 /* subtitle */ return new Property("subtitle", "string", 4196 "An explanatory or alternate title for the ResearchElementDefinition giving additional information about its content.", 4197 0, 1, subtitle); 4198 case -892481550: 4199 /* status */ return new Property("status", "code", 4200 "The status of this research element definition. Enables tracking the life-cycle of the content.", 0, 1, 4201 status); 4202 case -404562712: 4203 /* experimental */ return new Property("experimental", "boolean", 4204 "A Boolean value to indicate that this research element definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4205 0, 1, experimental); 4206 case -573640748: 4207 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4208 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4209 0, 1, subject); 4210 case -1867885268: 4211 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4212 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4213 0, 1, subject); 4214 case -1257122603: 4215 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4216 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4217 0, 1, subject); 4218 case 772938623: 4219 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4220 "The intended subjects for the ResearchElementDefinition. If this element is not provided, a Patient subject is assumed, but the subject of the ResearchElementDefinition can be anything.", 4221 0, 1, subject); 4222 case 3076014: 4223 /* date */ return new Property("date", "dateTime", 4224 "The date (and optionally time) when the research element definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the research element definition changes.", 4225 0, 1, date); 4226 case 1447404028: 4227 /* publisher */ return new Property("publisher", "string", 4228 "The name of the organization or individual that published the research element definition.", 0, 1, 4229 publisher); 4230 case 951526432: 4231 /* contact */ return new Property("contact", "ContactDetail", 4232 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4233 java.lang.Integer.MAX_VALUE, contact); 4234 case -1724546052: 4235 /* description */ return new Property("description", "markdown", 4236 "A free text natural language description of the research element definition from a consumer's perspective.", 4237 0, 1, description); 4238 case 950398559: 4239 /* comment */ return new Property("comment", "string", 4240 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 4241 comment); 4242 case -669707736: 4243 /* useContext */ return new Property("useContext", "UsageContext", 4244 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate research element definition instances.", 4245 0, java.lang.Integer.MAX_VALUE, useContext); 4246 case -507075711: 4247 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4248 "A legal or geographic region in which the research element definition is intended to be used.", 0, 4249 java.lang.Integer.MAX_VALUE, jurisdiction); 4250 case -220463842: 4251 /* purpose */ return new Property("purpose", "markdown", 4252 "Explanation of why this research element definition is needed and why it has been designed as it has.", 0, 1, 4253 purpose); 4254 case 111574433: 4255 /* usage */ return new Property("usage", "string", 4256 "A detailed description, from a clinical perspective, of how the ResearchElementDefinition is used.", 0, 1, 4257 usage); 4258 case 1522889671: 4259 /* copyright */ return new Property("copyright", "markdown", 4260 "A copyright statement relating to the research element definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the research element definition.", 4261 0, 1, copyright); 4262 case 223539345: 4263 /* approvalDate */ return new Property("approvalDate", "date", 4264 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4265 0, 1, approvalDate); 4266 case -1687512484: 4267 /* lastReviewDate */ return new Property("lastReviewDate", "date", 4268 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4269 0, 1, lastReviewDate); 4270 case -403934648: 4271 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 4272 "The period during which the research element definition content was or is planned to be in active use.", 0, 4273 1, effectivePeriod); 4274 case 110546223: 4275 /* topic */ return new Property("topic", "CodeableConcept", 4276 "Descriptive topics related to the content of the ResearchElementDefinition. Topics provide a high-level categorization grouping types of ResearchElementDefinitions that can be useful for filtering and searching.", 4277 0, java.lang.Integer.MAX_VALUE, topic); 4278 case -1406328437: 4279 /* author */ return new Property("author", "ContactDetail", 4280 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4281 java.lang.Integer.MAX_VALUE, author); 4282 case -1307827859: 4283 /* editor */ return new Property("editor", "ContactDetail", 4284 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4285 java.lang.Integer.MAX_VALUE, editor); 4286 case -261190139: 4287 /* reviewer */ return new Property("reviewer", "ContactDetail", 4288 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4289 java.lang.Integer.MAX_VALUE, reviewer); 4290 case 1740277666: 4291 /* endorser */ return new Property("endorser", "ContactDetail", 4292 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4293 java.lang.Integer.MAX_VALUE, endorser); 4294 case 666807069: 4295 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 4296 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4297 java.lang.Integer.MAX_VALUE, relatedArtifact); 4298 case 166208699: 4299 /* library */ return new Property("library", "canonical(Library)", 4300 "A reference to a Library resource containing the formal logic used by the ResearchElementDefinition.", 0, 4301 java.lang.Integer.MAX_VALUE, library); 4302 case 3575610: 4303 /* type */ return new Property("type", "code", 4304 "The type of research element, a population, an exposure, or an outcome.", 0, 1, type); 4305 case -372820010: 4306 /* variableType */ return new Property("variableType", "code", 4307 "The type of the outcome (e.g. Dichotomous, Continuous, or Descriptive).", 0, 1, variableType); 4308 case 366313883: 4309 /* characteristic */ return new Property("characteristic", "", 4310 "A characteristic that defines the members of the research element. Multiple characteristics are applied with \"and\" semantics.", 4311 0, java.lang.Integer.MAX_VALUE, characteristic); 4312 default: 4313 return super.getNamedProperty(_hash, _name, _checkValid); 4314 } 4315 4316 } 4317 4318 @Override 4319 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4320 switch (hash) { 4321 case 116079: 4322 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4323 case -1618432855: 4324 /* identifier */ return this.identifier == null ? new Base[0] 4325 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4326 case 351608024: 4327 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4328 case 3373707: 4329 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4330 case 110371416: 4331 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4332 case 1555503932: 4333 /* shortTitle */ return this.shortTitle == null ? new Base[0] : new Base[] { this.shortTitle }; // StringType 4334 case -2060497896: 4335 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 4336 case -892481550: 4337 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4338 case -404562712: 4339 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 4340 case -1867885268: 4341 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 4342 case 3076014: 4343 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4344 case 1447404028: 4345 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 4346 case 951526432: 4347 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4348 case -1724546052: 4349 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4350 case 950398559: 4351 /* comment */ return this.comment == null ? new Base[0] : this.comment.toArray(new Base[this.comment.size()]); // StringType 4352 case -669707736: 4353 /* useContext */ return this.useContext == null ? new Base[0] 4354 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4355 case -507075711: 4356 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 4357 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4358 case -220463842: 4359 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 4360 case 111574433: 4361 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 4362 case 1522889671: 4363 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 4364 case 223539345: 4365 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 4366 case -1687512484: 4367 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 4368 case -403934648: 4369 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 4370 case 110546223: 4371 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 4372 case -1406328437: 4373 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 4374 case -1307827859: 4375 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 4376 case -261190139: 4377 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 4378 case 1740277666: 4379 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 4380 case 666807069: 4381 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 4382 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 4383 case 166208699: 4384 /* library */ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 4385 case 3575610: 4386 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ResearchElementType> 4387 case -372820010: 4388 /* variableType */ return this.variableType == null ? new Base[0] : new Base[] { this.variableType }; // Enumeration<VariableType> 4389 case 366313883: 4390 /* characteristic */ return this.characteristic == null ? new Base[0] 4391 : this.characteristic.toArray(new Base[this.characteristic.size()]); // ResearchElementDefinitionCharacteristicComponent 4392 default: 4393 return super.getProperty(hash, name, checkValid); 4394 } 4395 4396 } 4397 4398 @Override 4399 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4400 switch (hash) { 4401 case 116079: // url 4402 this.url = castToUri(value); // UriType 4403 return value; 4404 case -1618432855: // identifier 4405 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4406 return value; 4407 case 351608024: // version 4408 this.version = castToString(value); // StringType 4409 return value; 4410 case 3373707: // name 4411 this.name = castToString(value); // StringType 4412 return value; 4413 case 110371416: // title 4414 this.title = castToString(value); // StringType 4415 return value; 4416 case 1555503932: // shortTitle 4417 this.shortTitle = castToString(value); // StringType 4418 return value; 4419 case -2060497896: // subtitle 4420 this.subtitle = castToString(value); // StringType 4421 return value; 4422 case -892481550: // status 4423 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4424 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4425 return value; 4426 case -404562712: // experimental 4427 this.experimental = castToBoolean(value); // BooleanType 4428 return value; 4429 case -1867885268: // subject 4430 this.subject = castToType(value); // Type 4431 return value; 4432 case 3076014: // date 4433 this.date = castToDateTime(value); // DateTimeType 4434 return value; 4435 case 1447404028: // publisher 4436 this.publisher = castToString(value); // StringType 4437 return value; 4438 case 951526432: // contact 4439 this.getContact().add(castToContactDetail(value)); // ContactDetail 4440 return value; 4441 case -1724546052: // description 4442 this.description = castToMarkdown(value); // MarkdownType 4443 return value; 4444 case 950398559: // comment 4445 this.getComment().add(castToString(value)); // StringType 4446 return value; 4447 case -669707736: // useContext 4448 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4449 return value; 4450 case -507075711: // jurisdiction 4451 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4452 return value; 4453 case -220463842: // purpose 4454 this.purpose = castToMarkdown(value); // MarkdownType 4455 return value; 4456 case 111574433: // usage 4457 this.usage = castToString(value); // StringType 4458 return value; 4459 case 1522889671: // copyright 4460 this.copyright = castToMarkdown(value); // MarkdownType 4461 return value; 4462 case 223539345: // approvalDate 4463 this.approvalDate = castToDate(value); // DateType 4464 return value; 4465 case -1687512484: // lastReviewDate 4466 this.lastReviewDate = castToDate(value); // DateType 4467 return value; 4468 case -403934648: // effectivePeriod 4469 this.effectivePeriod = castToPeriod(value); // Period 4470 return value; 4471 case 110546223: // topic 4472 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 4473 return value; 4474 case -1406328437: // author 4475 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 4476 return value; 4477 case -1307827859: // editor 4478 this.getEditor().add(castToContactDetail(value)); // ContactDetail 4479 return value; 4480 case -261190139: // reviewer 4481 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 4482 return value; 4483 case 1740277666: // endorser 4484 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 4485 return value; 4486 case 666807069: // relatedArtifact 4487 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 4488 return value; 4489 case 166208699: // library 4490 this.getLibrary().add(castToCanonical(value)); // CanonicalType 4491 return value; 4492 case 3575610: // type 4493 value = new ResearchElementTypeEnumFactory().fromType(castToCode(value)); 4494 this.type = (Enumeration) value; // Enumeration<ResearchElementType> 4495 return value; 4496 case -372820010: // variableType 4497 value = new VariableTypeEnumFactory().fromType(castToCode(value)); 4498 this.variableType = (Enumeration) value; // Enumeration<VariableType> 4499 return value; 4500 case 366313883: // characteristic 4501 this.getCharacteristic().add((ResearchElementDefinitionCharacteristicComponent) value); // ResearchElementDefinitionCharacteristicComponent 4502 return value; 4503 default: 4504 return super.setProperty(hash, name, value); 4505 } 4506 4507 } 4508 4509 @Override 4510 public Base setProperty(String name, Base value) throws FHIRException { 4511 if (name.equals("url")) { 4512 this.url = castToUri(value); // UriType 4513 } else if (name.equals("identifier")) { 4514 this.getIdentifier().add(castToIdentifier(value)); 4515 } else if (name.equals("version")) { 4516 this.version = castToString(value); // StringType 4517 } else if (name.equals("name")) { 4518 this.name = castToString(value); // StringType 4519 } else if (name.equals("title")) { 4520 this.title = castToString(value); // StringType 4521 } else if (name.equals("shortTitle")) { 4522 this.shortTitle = castToString(value); // StringType 4523 } else if (name.equals("subtitle")) { 4524 this.subtitle = castToString(value); // StringType 4525 } else if (name.equals("status")) { 4526 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4527 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4528 } else if (name.equals("experimental")) { 4529 this.experimental = castToBoolean(value); // BooleanType 4530 } else if (name.equals("subject[x]")) { 4531 this.subject = castToType(value); // Type 4532 } else if (name.equals("date")) { 4533 this.date = castToDateTime(value); // DateTimeType 4534 } else if (name.equals("publisher")) { 4535 this.publisher = castToString(value); // StringType 4536 } else if (name.equals("contact")) { 4537 this.getContact().add(castToContactDetail(value)); 4538 } else if (name.equals("description")) { 4539 this.description = castToMarkdown(value); // MarkdownType 4540 } else if (name.equals("comment")) { 4541 this.getComment().add(castToString(value)); 4542 } else if (name.equals("useContext")) { 4543 this.getUseContext().add(castToUsageContext(value)); 4544 } else if (name.equals("jurisdiction")) { 4545 this.getJurisdiction().add(castToCodeableConcept(value)); 4546 } else if (name.equals("purpose")) { 4547 this.purpose = castToMarkdown(value); // MarkdownType 4548 } else if (name.equals("usage")) { 4549 this.usage = castToString(value); // StringType 4550 } else if (name.equals("copyright")) { 4551 this.copyright = castToMarkdown(value); // MarkdownType 4552 } else if (name.equals("approvalDate")) { 4553 this.approvalDate = castToDate(value); // DateType 4554 } else if (name.equals("lastReviewDate")) { 4555 this.lastReviewDate = castToDate(value); // DateType 4556 } else if (name.equals("effectivePeriod")) { 4557 this.effectivePeriod = castToPeriod(value); // Period 4558 } else if (name.equals("topic")) { 4559 this.getTopic().add(castToCodeableConcept(value)); 4560 } else if (name.equals("author")) { 4561 this.getAuthor().add(castToContactDetail(value)); 4562 } else if (name.equals("editor")) { 4563 this.getEditor().add(castToContactDetail(value)); 4564 } else if (name.equals("reviewer")) { 4565 this.getReviewer().add(castToContactDetail(value)); 4566 } else if (name.equals("endorser")) { 4567 this.getEndorser().add(castToContactDetail(value)); 4568 } else if (name.equals("relatedArtifact")) { 4569 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 4570 } else if (name.equals("library")) { 4571 this.getLibrary().add(castToCanonical(value)); 4572 } else if (name.equals("type")) { 4573 value = new ResearchElementTypeEnumFactory().fromType(castToCode(value)); 4574 this.type = (Enumeration) value; // Enumeration<ResearchElementType> 4575 } else if (name.equals("variableType")) { 4576 value = new VariableTypeEnumFactory().fromType(castToCode(value)); 4577 this.variableType = (Enumeration) value; // Enumeration<VariableType> 4578 } else if (name.equals("characteristic")) { 4579 this.getCharacteristic().add((ResearchElementDefinitionCharacteristicComponent) value); 4580 } else 4581 return super.setProperty(name, value); 4582 return value; 4583 } 4584 4585 @Override 4586 public void removeChild(String name, Base value) throws FHIRException { 4587 if (name.equals("url")) { 4588 this.url = null; 4589 } else if (name.equals("identifier")) { 4590 this.getIdentifier().remove(castToIdentifier(value)); 4591 } else if (name.equals("version")) { 4592 this.version = null; 4593 } else if (name.equals("name")) { 4594 this.name = null; 4595 } else if (name.equals("title")) { 4596 this.title = null; 4597 } else if (name.equals("shortTitle")) { 4598 this.shortTitle = null; 4599 } else if (name.equals("subtitle")) { 4600 this.subtitle = null; 4601 } else if (name.equals("status")) { 4602 this.status = null; 4603 } else if (name.equals("experimental")) { 4604 this.experimental = null; 4605 } else if (name.equals("subject[x]")) { 4606 this.subject = null; 4607 } else if (name.equals("date")) { 4608 this.date = null; 4609 } else if (name.equals("publisher")) { 4610 this.publisher = null; 4611 } else if (name.equals("contact")) { 4612 this.getContact().remove(castToContactDetail(value)); 4613 } else if (name.equals("description")) { 4614 this.description = null; 4615 } else if (name.equals("comment")) { 4616 this.getComment().remove(castToString(value)); 4617 } else if (name.equals("useContext")) { 4618 this.getUseContext().remove(castToUsageContext(value)); 4619 } else if (name.equals("jurisdiction")) { 4620 this.getJurisdiction().remove(castToCodeableConcept(value)); 4621 } else if (name.equals("purpose")) { 4622 this.purpose = null; 4623 } else if (name.equals("usage")) { 4624 this.usage = null; 4625 } else if (name.equals("copyright")) { 4626 this.copyright = null; 4627 } else if (name.equals("approvalDate")) { 4628 this.approvalDate = null; 4629 } else if (name.equals("lastReviewDate")) { 4630 this.lastReviewDate = null; 4631 } else if (name.equals("effectivePeriod")) { 4632 this.effectivePeriod = null; 4633 } else if (name.equals("topic")) { 4634 this.getTopic().remove(castToCodeableConcept(value)); 4635 } else if (name.equals("author")) { 4636 this.getAuthor().remove(castToContactDetail(value)); 4637 } else if (name.equals("editor")) { 4638 this.getEditor().remove(castToContactDetail(value)); 4639 } else if (name.equals("reviewer")) { 4640 this.getReviewer().remove(castToContactDetail(value)); 4641 } else if (name.equals("endorser")) { 4642 this.getEndorser().remove(castToContactDetail(value)); 4643 } else if (name.equals("relatedArtifact")) { 4644 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 4645 } else if (name.equals("library")) { 4646 this.getLibrary().remove(castToCanonical(value)); 4647 } else if (name.equals("type")) { 4648 this.type = null; 4649 } else if (name.equals("variableType")) { 4650 this.variableType = null; 4651 } else if (name.equals("characteristic")) { 4652 this.getCharacteristic().remove((ResearchElementDefinitionCharacteristicComponent) value); 4653 } else 4654 super.removeChild(name, value); 4655 4656 } 4657 4658 @Override 4659 public Base makeProperty(int hash, String name) throws FHIRException { 4660 switch (hash) { 4661 case 116079: 4662 return getUrlElement(); 4663 case -1618432855: 4664 return addIdentifier(); 4665 case 351608024: 4666 return getVersionElement(); 4667 case 3373707: 4668 return getNameElement(); 4669 case 110371416: 4670 return getTitleElement(); 4671 case 1555503932: 4672 return getShortTitleElement(); 4673 case -2060497896: 4674 return getSubtitleElement(); 4675 case -892481550: 4676 return getStatusElement(); 4677 case -404562712: 4678 return getExperimentalElement(); 4679 case -573640748: 4680 return getSubject(); 4681 case -1867885268: 4682 return getSubject(); 4683 case 3076014: 4684 return getDateElement(); 4685 case 1447404028: 4686 return getPublisherElement(); 4687 case 951526432: 4688 return addContact(); 4689 case -1724546052: 4690 return getDescriptionElement(); 4691 case 950398559: 4692 return addCommentElement(); 4693 case -669707736: 4694 return addUseContext(); 4695 case -507075711: 4696 return addJurisdiction(); 4697 case -220463842: 4698 return getPurposeElement(); 4699 case 111574433: 4700 return getUsageElement(); 4701 case 1522889671: 4702 return getCopyrightElement(); 4703 case 223539345: 4704 return getApprovalDateElement(); 4705 case -1687512484: 4706 return getLastReviewDateElement(); 4707 case -403934648: 4708 return getEffectivePeriod(); 4709 case 110546223: 4710 return addTopic(); 4711 case -1406328437: 4712 return addAuthor(); 4713 case -1307827859: 4714 return addEditor(); 4715 case -261190139: 4716 return addReviewer(); 4717 case 1740277666: 4718 return addEndorser(); 4719 case 666807069: 4720 return addRelatedArtifact(); 4721 case 166208699: 4722 return addLibraryElement(); 4723 case 3575610: 4724 return getTypeElement(); 4725 case -372820010: 4726 return getVariableTypeElement(); 4727 case 366313883: 4728 return addCharacteristic(); 4729 default: 4730 return super.makeProperty(hash, name); 4731 } 4732 4733 } 4734 4735 @Override 4736 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4737 switch (hash) { 4738 case 116079: 4739 /* url */ return new String[] { "uri" }; 4740 case -1618432855: 4741 /* identifier */ return new String[] { "Identifier" }; 4742 case 351608024: 4743 /* version */ return new String[] { "string" }; 4744 case 3373707: 4745 /* name */ return new String[] { "string" }; 4746 case 110371416: 4747 /* title */ return new String[] { "string" }; 4748 case 1555503932: 4749 /* shortTitle */ return new String[] { "string" }; 4750 case -2060497896: 4751 /* subtitle */ return new String[] { "string" }; 4752 case -892481550: 4753 /* status */ return new String[] { "code" }; 4754 case -404562712: 4755 /* experimental */ return new String[] { "boolean" }; 4756 case -1867885268: 4757 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 4758 case 3076014: 4759 /* date */ return new String[] { "dateTime" }; 4760 case 1447404028: 4761 /* publisher */ return new String[] { "string" }; 4762 case 951526432: 4763 /* contact */ return new String[] { "ContactDetail" }; 4764 case -1724546052: 4765 /* description */ return new String[] { "markdown" }; 4766 case 950398559: 4767 /* comment */ return new String[] { "string" }; 4768 case -669707736: 4769 /* useContext */ return new String[] { "UsageContext" }; 4770 case -507075711: 4771 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4772 case -220463842: 4773 /* purpose */ return new String[] { "markdown" }; 4774 case 111574433: 4775 /* usage */ return new String[] { "string" }; 4776 case 1522889671: 4777 /* copyright */ return new String[] { "markdown" }; 4778 case 223539345: 4779 /* approvalDate */ return new String[] { "date" }; 4780 case -1687512484: 4781 /* lastReviewDate */ return new String[] { "date" }; 4782 case -403934648: 4783 /* effectivePeriod */ return new String[] { "Period" }; 4784 case 110546223: 4785 /* topic */ return new String[] { "CodeableConcept" }; 4786 case -1406328437: 4787 /* author */ return new String[] { "ContactDetail" }; 4788 case -1307827859: 4789 /* editor */ return new String[] { "ContactDetail" }; 4790 case -261190139: 4791 /* reviewer */ return new String[] { "ContactDetail" }; 4792 case 1740277666: 4793 /* endorser */ return new String[] { "ContactDetail" }; 4794 case 666807069: 4795 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 4796 case 166208699: 4797 /* library */ return new String[] { "canonical" }; 4798 case 3575610: 4799 /* type */ return new String[] { "code" }; 4800 case -372820010: 4801 /* variableType */ return new String[] { "code" }; 4802 case 366313883: 4803 /* characteristic */ return new String[] {}; 4804 default: 4805 return super.getTypesForProperty(hash, name); 4806 } 4807 4808 } 4809 4810 @Override 4811 public Base addChild(String name) throws FHIRException { 4812 if (name.equals("url")) { 4813 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.url"); 4814 } else if (name.equals("identifier")) { 4815 return addIdentifier(); 4816 } else if (name.equals("version")) { 4817 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.version"); 4818 } else if (name.equals("name")) { 4819 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.name"); 4820 } else if (name.equals("title")) { 4821 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.title"); 4822 } else if (name.equals("shortTitle")) { 4823 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.shortTitle"); 4824 } else if (name.equals("subtitle")) { 4825 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.subtitle"); 4826 } else if (name.equals("status")) { 4827 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.status"); 4828 } else if (name.equals("experimental")) { 4829 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.experimental"); 4830 } else if (name.equals("subjectCodeableConcept")) { 4831 this.subject = new CodeableConcept(); 4832 return this.subject; 4833 } else if (name.equals("subjectReference")) { 4834 this.subject = new Reference(); 4835 return this.subject; 4836 } else if (name.equals("date")) { 4837 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.date"); 4838 } else if (name.equals("publisher")) { 4839 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.publisher"); 4840 } else if (name.equals("contact")) { 4841 return addContact(); 4842 } else if (name.equals("description")) { 4843 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.description"); 4844 } else if (name.equals("comment")) { 4845 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.comment"); 4846 } else if (name.equals("useContext")) { 4847 return addUseContext(); 4848 } else if (name.equals("jurisdiction")) { 4849 return addJurisdiction(); 4850 } else if (name.equals("purpose")) { 4851 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.purpose"); 4852 } else if (name.equals("usage")) { 4853 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.usage"); 4854 } else if (name.equals("copyright")) { 4855 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.copyright"); 4856 } else if (name.equals("approvalDate")) { 4857 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.approvalDate"); 4858 } else if (name.equals("lastReviewDate")) { 4859 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.lastReviewDate"); 4860 } else if (name.equals("effectivePeriod")) { 4861 this.effectivePeriod = new Period(); 4862 return this.effectivePeriod; 4863 } else if (name.equals("topic")) { 4864 return addTopic(); 4865 } else if (name.equals("author")) { 4866 return addAuthor(); 4867 } else if (name.equals("editor")) { 4868 return addEditor(); 4869 } else if (name.equals("reviewer")) { 4870 return addReviewer(); 4871 } else if (name.equals("endorser")) { 4872 return addEndorser(); 4873 } else if (name.equals("relatedArtifact")) { 4874 return addRelatedArtifact(); 4875 } else if (name.equals("library")) { 4876 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.library"); 4877 } else if (name.equals("type")) { 4878 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.type"); 4879 } else if (name.equals("variableType")) { 4880 throw new FHIRException("Cannot call addChild on a singleton property ResearchElementDefinition.variableType"); 4881 } else if (name.equals("characteristic")) { 4882 return addCharacteristic(); 4883 } else 4884 return super.addChild(name); 4885 } 4886 4887 public String fhirType() { 4888 return "ResearchElementDefinition"; 4889 4890 } 4891 4892 public ResearchElementDefinition copy() { 4893 ResearchElementDefinition dst = new ResearchElementDefinition(); 4894 copyValues(dst); 4895 return dst; 4896 } 4897 4898 public void copyValues(ResearchElementDefinition dst) { 4899 super.copyValues(dst); 4900 dst.url = url == null ? null : url.copy(); 4901 if (identifier != null) { 4902 dst.identifier = new ArrayList<Identifier>(); 4903 for (Identifier i : identifier) 4904 dst.identifier.add(i.copy()); 4905 } 4906 ; 4907 dst.version = version == null ? null : version.copy(); 4908 dst.name = name == null ? null : name.copy(); 4909 dst.title = title == null ? null : title.copy(); 4910 dst.shortTitle = shortTitle == null ? null : shortTitle.copy(); 4911 dst.subtitle = subtitle == null ? null : subtitle.copy(); 4912 dst.status = status == null ? null : status.copy(); 4913 dst.experimental = experimental == null ? null : experimental.copy(); 4914 dst.subject = subject == null ? null : subject.copy(); 4915 dst.date = date == null ? null : date.copy(); 4916 dst.publisher = publisher == null ? null : publisher.copy(); 4917 if (contact != null) { 4918 dst.contact = new ArrayList<ContactDetail>(); 4919 for (ContactDetail i : contact) 4920 dst.contact.add(i.copy()); 4921 } 4922 ; 4923 dst.description = description == null ? null : description.copy(); 4924 if (comment != null) { 4925 dst.comment = new ArrayList<StringType>(); 4926 for (StringType i : comment) 4927 dst.comment.add(i.copy()); 4928 } 4929 ; 4930 if (useContext != null) { 4931 dst.useContext = new ArrayList<UsageContext>(); 4932 for (UsageContext i : useContext) 4933 dst.useContext.add(i.copy()); 4934 } 4935 ; 4936 if (jurisdiction != null) { 4937 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4938 for (CodeableConcept i : jurisdiction) 4939 dst.jurisdiction.add(i.copy()); 4940 } 4941 ; 4942 dst.purpose = purpose == null ? null : purpose.copy(); 4943 dst.usage = usage == null ? null : usage.copy(); 4944 dst.copyright = copyright == null ? null : copyright.copy(); 4945 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4946 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4947 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4948 if (topic != null) { 4949 dst.topic = new ArrayList<CodeableConcept>(); 4950 for (CodeableConcept i : topic) 4951 dst.topic.add(i.copy()); 4952 } 4953 ; 4954 if (author != null) { 4955 dst.author = new ArrayList<ContactDetail>(); 4956 for (ContactDetail i : author) 4957 dst.author.add(i.copy()); 4958 } 4959 ; 4960 if (editor != null) { 4961 dst.editor = new ArrayList<ContactDetail>(); 4962 for (ContactDetail i : editor) 4963 dst.editor.add(i.copy()); 4964 } 4965 ; 4966 if (reviewer != null) { 4967 dst.reviewer = new ArrayList<ContactDetail>(); 4968 for (ContactDetail i : reviewer) 4969 dst.reviewer.add(i.copy()); 4970 } 4971 ; 4972 if (endorser != null) { 4973 dst.endorser = new ArrayList<ContactDetail>(); 4974 for (ContactDetail i : endorser) 4975 dst.endorser.add(i.copy()); 4976 } 4977 ; 4978 if (relatedArtifact != null) { 4979 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 4980 for (RelatedArtifact i : relatedArtifact) 4981 dst.relatedArtifact.add(i.copy()); 4982 } 4983 ; 4984 if (library != null) { 4985 dst.library = new ArrayList<CanonicalType>(); 4986 for (CanonicalType i : library) 4987 dst.library.add(i.copy()); 4988 } 4989 ; 4990 dst.type = type == null ? null : type.copy(); 4991 dst.variableType = variableType == null ? null : variableType.copy(); 4992 if (characteristic != null) { 4993 dst.characteristic = new ArrayList<ResearchElementDefinitionCharacteristicComponent>(); 4994 for (ResearchElementDefinitionCharacteristicComponent i : characteristic) 4995 dst.characteristic.add(i.copy()); 4996 } 4997 ; 4998 } 4999 5000 protected ResearchElementDefinition typedCopy() { 5001 return copy(); 5002 } 5003 5004 @Override 5005 public boolean equalsDeep(Base other_) { 5006 if (!super.equalsDeep(other_)) 5007 return false; 5008 if (!(other_ instanceof ResearchElementDefinition)) 5009 return false; 5010 ResearchElementDefinition o = (ResearchElementDefinition) other_; 5011 return compareDeep(identifier, o.identifier, true) && compareDeep(shortTitle, o.shortTitle, true) 5012 && compareDeep(subtitle, o.subtitle, true) && compareDeep(subject, o.subject, true) 5013 && compareDeep(comment, o.comment, true) && compareDeep(purpose, o.purpose, true) 5014 && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 5015 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 5016 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) 5017 && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 5018 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 5019 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(library, o.library, true) 5020 && compareDeep(type, o.type, true) && compareDeep(variableType, o.variableType, true) 5021 && compareDeep(characteristic, o.characteristic, true); 5022 } 5023 5024 @Override 5025 public boolean equalsShallow(Base other_) { 5026 if (!super.equalsShallow(other_)) 5027 return false; 5028 if (!(other_ instanceof ResearchElementDefinition)) 5029 return false; 5030 ResearchElementDefinition o = (ResearchElementDefinition) other_; 5031 return compareValues(shortTitle, o.shortTitle, true) && compareValues(subtitle, o.subtitle, true) 5032 && compareValues(comment, o.comment, true) && compareValues(purpose, o.purpose, true) 5033 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 5034 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 5035 && compareValues(type, o.type, true) && compareValues(variableType, o.variableType, true); 5036 } 5037 5038 public boolean isEmpty() { 5039 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, shortTitle, subtitle, subject, comment, 5040 purpose, usage, copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, 5041 endorser, relatedArtifact, library, type, variableType, characteristic); 5042 } 5043 5044 @Override 5045 public ResourceType getResourceType() { 5046 return ResourceType.ResearchElementDefinition; 5047 } 5048 5049 /** 5050 * Search parameter: <b>date</b> 5051 * <p> 5052 * Description: <b>The research element definition publication date</b><br> 5053 * Type: <b>date</b><br> 5054 * Path: <b>ResearchElementDefinition.date</b><br> 5055 * </p> 5056 */ 5057 @SearchParamDefinition(name = "date", path = "ResearchElementDefinition.date", description = "The research element definition publication date", type = "date") 5058 public static final String SP_DATE = "date"; 5059 /** 5060 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5061 * <p> 5062 * Description: <b>The research element definition publication date</b><br> 5063 * Type: <b>date</b><br> 5064 * Path: <b>ResearchElementDefinition.date</b><br> 5065 * </p> 5066 */ 5067 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5068 SP_DATE); 5069 5070 /** 5071 * Search parameter: <b>identifier</b> 5072 * <p> 5073 * Description: <b>External identifier for the research element 5074 * definition</b><br> 5075 * Type: <b>token</b><br> 5076 * Path: <b>ResearchElementDefinition.identifier</b><br> 5077 * </p> 5078 */ 5079 @SearchParamDefinition(name = "identifier", path = "ResearchElementDefinition.identifier", description = "External identifier for the research element definition", type = "token") 5080 public static final String SP_IDENTIFIER = "identifier"; 5081 /** 5082 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5083 * <p> 5084 * Description: <b>External identifier for the research element 5085 * definition</b><br> 5086 * Type: <b>token</b><br> 5087 * Path: <b>ResearchElementDefinition.identifier</b><br> 5088 * </p> 5089 */ 5090 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5091 SP_IDENTIFIER); 5092 5093 /** 5094 * Search parameter: <b>successor</b> 5095 * <p> 5096 * Description: <b>What resource is being referenced</b><br> 5097 * Type: <b>reference</b><br> 5098 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5099 * </p> 5100 */ 5101 @SearchParamDefinition(name = "successor", path = "ResearchElementDefinition.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 5102 public static final String SP_SUCCESSOR = "successor"; 5103 /** 5104 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 5105 * <p> 5106 * Description: <b>What resource is being referenced</b><br> 5107 * Type: <b>reference</b><br> 5108 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5109 * </p> 5110 */ 5111 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5112 SP_SUCCESSOR); 5113 5114 /** 5115 * Constant for fluent queries to be used to add include statements. Specifies 5116 * the path value of "<b>ResearchElementDefinition:successor</b>". 5117 */ 5118 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 5119 "ResearchElementDefinition:successor").toLocked(); 5120 5121 /** 5122 * Search parameter: <b>context-type-value</b> 5123 * <p> 5124 * Description: <b>A use context type and value assigned to the research element 5125 * definition</b><br> 5126 * Type: <b>composite</b><br> 5127 * Path: <b></b><br> 5128 * </p> 5129 */ 5130 @SearchParamDefinition(name = "context-type-value", path = "ResearchElementDefinition.useContext", description = "A use context type and value assigned to the research element definition", type = "composite", compositeOf = { 5131 "context-type", "context" }) 5132 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5133 /** 5134 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5135 * <p> 5136 * Description: <b>A use context type and value assigned to the research element 5137 * definition</b><br> 5138 * Type: <b>composite</b><br> 5139 * Path: <b></b><br> 5140 * </p> 5141 */ 5142 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5143 SP_CONTEXT_TYPE_VALUE); 5144 5145 /** 5146 * Search parameter: <b>jurisdiction</b> 5147 * <p> 5148 * Description: <b>Intended jurisdiction for the research element 5149 * definition</b><br> 5150 * Type: <b>token</b><br> 5151 * Path: <b>ResearchElementDefinition.jurisdiction</b><br> 5152 * </p> 5153 */ 5154 @SearchParamDefinition(name = "jurisdiction", path = "ResearchElementDefinition.jurisdiction", description = "Intended jurisdiction for the research element definition", type = "token") 5155 public static final String SP_JURISDICTION = "jurisdiction"; 5156 /** 5157 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5158 * <p> 5159 * Description: <b>Intended jurisdiction for the research element 5160 * definition</b><br> 5161 * Type: <b>token</b><br> 5162 * Path: <b>ResearchElementDefinition.jurisdiction</b><br> 5163 * </p> 5164 */ 5165 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5166 SP_JURISDICTION); 5167 5168 /** 5169 * Search parameter: <b>description</b> 5170 * <p> 5171 * Description: <b>The description of the research element definition</b><br> 5172 * Type: <b>string</b><br> 5173 * Path: <b>ResearchElementDefinition.description</b><br> 5174 * </p> 5175 */ 5176 @SearchParamDefinition(name = "description", path = "ResearchElementDefinition.description", description = "The description of the research element definition", type = "string") 5177 public static final String SP_DESCRIPTION = "description"; 5178 /** 5179 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5180 * <p> 5181 * Description: <b>The description of the research element definition</b><br> 5182 * Type: <b>string</b><br> 5183 * Path: <b>ResearchElementDefinition.description</b><br> 5184 * </p> 5185 */ 5186 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 5187 SP_DESCRIPTION); 5188 5189 /** 5190 * Search parameter: <b>derived-from</b> 5191 * <p> 5192 * Description: <b>What resource is being referenced</b><br> 5193 * Type: <b>reference</b><br> 5194 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5195 * </p> 5196 */ 5197 @SearchParamDefinition(name = "derived-from", path = "ResearchElementDefinition.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 5198 public static final String SP_DERIVED_FROM = "derived-from"; 5199 /** 5200 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 5201 * <p> 5202 * Description: <b>What resource is being referenced</b><br> 5203 * Type: <b>reference</b><br> 5204 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5205 * </p> 5206 */ 5207 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5208 SP_DERIVED_FROM); 5209 5210 /** 5211 * Constant for fluent queries to be used to add include statements. Specifies 5212 * the path value of "<b>ResearchElementDefinition:derived-from</b>". 5213 */ 5214 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 5215 "ResearchElementDefinition:derived-from").toLocked(); 5216 5217 /** 5218 * Search parameter: <b>context-type</b> 5219 * <p> 5220 * Description: <b>A type of use context assigned to the research element 5221 * definition</b><br> 5222 * Type: <b>token</b><br> 5223 * Path: <b>ResearchElementDefinition.useContext.code</b><br> 5224 * </p> 5225 */ 5226 @SearchParamDefinition(name = "context-type", path = "ResearchElementDefinition.useContext.code", description = "A type of use context assigned to the research element definition", type = "token") 5227 public static final String SP_CONTEXT_TYPE = "context-type"; 5228 /** 5229 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5230 * <p> 5231 * Description: <b>A type of use context assigned to the research element 5232 * definition</b><br> 5233 * Type: <b>token</b><br> 5234 * Path: <b>ResearchElementDefinition.useContext.code</b><br> 5235 * </p> 5236 */ 5237 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5238 SP_CONTEXT_TYPE); 5239 5240 /** 5241 * Search parameter: <b>predecessor</b> 5242 * <p> 5243 * Description: <b>What resource is being referenced</b><br> 5244 * Type: <b>reference</b><br> 5245 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5246 * </p> 5247 */ 5248 @SearchParamDefinition(name = "predecessor", path = "ResearchElementDefinition.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 5249 public static final String SP_PREDECESSOR = "predecessor"; 5250 /** 5251 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 5252 * <p> 5253 * Description: <b>What resource is being referenced</b><br> 5254 * Type: <b>reference</b><br> 5255 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5256 * </p> 5257 */ 5258 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5259 SP_PREDECESSOR); 5260 5261 /** 5262 * Constant for fluent queries to be used to add include statements. Specifies 5263 * the path value of "<b>ResearchElementDefinition:predecessor</b>". 5264 */ 5265 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 5266 "ResearchElementDefinition:predecessor").toLocked(); 5267 5268 /** 5269 * Search parameter: <b>title</b> 5270 * <p> 5271 * Description: <b>The human-friendly name of the research element 5272 * definition</b><br> 5273 * Type: <b>string</b><br> 5274 * Path: <b>ResearchElementDefinition.title</b><br> 5275 * </p> 5276 */ 5277 @SearchParamDefinition(name = "title", path = "ResearchElementDefinition.title", description = "The human-friendly name of the research element definition", type = "string") 5278 public static final String SP_TITLE = "title"; 5279 /** 5280 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5281 * <p> 5282 * Description: <b>The human-friendly name of the research element 5283 * definition</b><br> 5284 * Type: <b>string</b><br> 5285 * Path: <b>ResearchElementDefinition.title</b><br> 5286 * </p> 5287 */ 5288 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 5289 SP_TITLE); 5290 5291 /** 5292 * Search parameter: <b>composed-of</b> 5293 * <p> 5294 * Description: <b>What resource is being referenced</b><br> 5295 * Type: <b>reference</b><br> 5296 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5297 * </p> 5298 */ 5299 @SearchParamDefinition(name = "composed-of", path = "ResearchElementDefinition.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 5300 public static final String SP_COMPOSED_OF = "composed-of"; 5301 /** 5302 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 5303 * <p> 5304 * Description: <b>What resource is being referenced</b><br> 5305 * Type: <b>reference</b><br> 5306 * Path: <b>ResearchElementDefinition.relatedArtifact.resource</b><br> 5307 * </p> 5308 */ 5309 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5310 SP_COMPOSED_OF); 5311 5312 /** 5313 * Constant for fluent queries to be used to add include statements. Specifies 5314 * the path value of "<b>ResearchElementDefinition:composed-of</b>". 5315 */ 5316 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 5317 "ResearchElementDefinition:composed-of").toLocked(); 5318 5319 /** 5320 * Search parameter: <b>version</b> 5321 * <p> 5322 * Description: <b>The business version of the research element 5323 * definition</b><br> 5324 * Type: <b>token</b><br> 5325 * Path: <b>ResearchElementDefinition.version</b><br> 5326 * </p> 5327 */ 5328 @SearchParamDefinition(name = "version", path = "ResearchElementDefinition.version", description = "The business version of the research element definition", type = "token") 5329 public static final String SP_VERSION = "version"; 5330 /** 5331 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5332 * <p> 5333 * Description: <b>The business version of the research element 5334 * definition</b><br> 5335 * Type: <b>token</b><br> 5336 * Path: <b>ResearchElementDefinition.version</b><br> 5337 * </p> 5338 */ 5339 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5340 SP_VERSION); 5341 5342 /** 5343 * Search parameter: <b>url</b> 5344 * <p> 5345 * Description: <b>The uri that identifies the research element 5346 * definition</b><br> 5347 * Type: <b>uri</b><br> 5348 * Path: <b>ResearchElementDefinition.url</b><br> 5349 * </p> 5350 */ 5351 @SearchParamDefinition(name = "url", path = "ResearchElementDefinition.url", description = "The uri that identifies the research element definition", type = "uri") 5352 public static final String SP_URL = "url"; 5353 /** 5354 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5355 * <p> 5356 * Description: <b>The uri that identifies the research element 5357 * definition</b><br> 5358 * Type: <b>uri</b><br> 5359 * Path: <b>ResearchElementDefinition.url</b><br> 5360 * </p> 5361 */ 5362 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5363 5364 /** 5365 * Search parameter: <b>context-quantity</b> 5366 * <p> 5367 * Description: <b>A quantity- or range-valued use context assigned to the 5368 * research element definition</b><br> 5369 * Type: <b>quantity</b><br> 5370 * Path: <b>ResearchElementDefinition.useContext.valueQuantity, 5371 * ResearchElementDefinition.useContext.valueRange</b><br> 5372 * </p> 5373 */ 5374 @SearchParamDefinition(name = "context-quantity", path = "(ResearchElementDefinition.useContext.value as Quantity) | (ResearchElementDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the research element definition", type = "quantity") 5375 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5376 /** 5377 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5378 * <p> 5379 * Description: <b>A quantity- or range-valued use context assigned to the 5380 * research element definition</b><br> 5381 * Type: <b>quantity</b><br> 5382 * Path: <b>ResearchElementDefinition.useContext.valueQuantity, 5383 * ResearchElementDefinition.useContext.valueRange</b><br> 5384 * </p> 5385 */ 5386 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5387 SP_CONTEXT_QUANTITY); 5388 5389 /** 5390 * Search parameter: <b>effective</b> 5391 * <p> 5392 * Description: <b>The time during which the research element definition is 5393 * intended to be in use</b><br> 5394 * Type: <b>date</b><br> 5395 * Path: <b>ResearchElementDefinition.effectivePeriod</b><br> 5396 * </p> 5397 */ 5398 @SearchParamDefinition(name = "effective", path = "ResearchElementDefinition.effectivePeriod", description = "The time during which the research element definition is intended to be in use", type = "date") 5399 public static final String SP_EFFECTIVE = "effective"; 5400 /** 5401 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 5402 * <p> 5403 * Description: <b>The time during which the research element definition is 5404 * intended to be in use</b><br> 5405 * Type: <b>date</b><br> 5406 * Path: <b>ResearchElementDefinition.effectivePeriod</b><br> 5407 * </p> 5408 */ 5409 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5410 SP_EFFECTIVE); 5411 5412 /** 5413 * Search parameter: <b>depends-on</b> 5414 * <p> 5415 * Description: <b>What resource is being referenced</b><br> 5416 * Type: <b>reference</b><br> 5417 * Path: <b>ResearchElementDefinition.relatedArtifact.resource, 5418 * ResearchElementDefinition.library</b><br> 5419 * </p> 5420 */ 5421 @SearchParamDefinition(name = "depends-on", path = "ResearchElementDefinition.relatedArtifact.where(type='depends-on').resource | ResearchElementDefinition.library", description = "What resource is being referenced", type = "reference") 5422 public static final String SP_DEPENDS_ON = "depends-on"; 5423 /** 5424 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 5425 * <p> 5426 * Description: <b>What resource is being referenced</b><br> 5427 * Type: <b>reference</b><br> 5428 * Path: <b>ResearchElementDefinition.relatedArtifact.resource, 5429 * ResearchElementDefinition.library</b><br> 5430 * </p> 5431 */ 5432 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5433 SP_DEPENDS_ON); 5434 5435 /** 5436 * Constant for fluent queries to be used to add include statements. Specifies 5437 * the path value of "<b>ResearchElementDefinition:depends-on</b>". 5438 */ 5439 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 5440 "ResearchElementDefinition:depends-on").toLocked(); 5441 5442 /** 5443 * Search parameter: <b>name</b> 5444 * <p> 5445 * Description: <b>Computationally friendly name of the research element 5446 * definition</b><br> 5447 * Type: <b>string</b><br> 5448 * Path: <b>ResearchElementDefinition.name</b><br> 5449 * </p> 5450 */ 5451 @SearchParamDefinition(name = "name", path = "ResearchElementDefinition.name", description = "Computationally friendly name of the research element definition", type = "string") 5452 public static final String SP_NAME = "name"; 5453 /** 5454 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5455 * <p> 5456 * Description: <b>Computationally friendly name of the research element 5457 * definition</b><br> 5458 * Type: <b>string</b><br> 5459 * Path: <b>ResearchElementDefinition.name</b><br> 5460 * </p> 5461 */ 5462 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5463 SP_NAME); 5464 5465 /** 5466 * Search parameter: <b>context</b> 5467 * <p> 5468 * Description: <b>A use context assigned to the research element 5469 * definition</b><br> 5470 * Type: <b>token</b><br> 5471 * Path: <b>ResearchElementDefinition.useContext.valueCodeableConcept</b><br> 5472 * </p> 5473 */ 5474 @SearchParamDefinition(name = "context", path = "(ResearchElementDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the research element definition", type = "token") 5475 public static final String SP_CONTEXT = "context"; 5476 /** 5477 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5478 * <p> 5479 * Description: <b>A use context assigned to the research element 5480 * definition</b><br> 5481 * Type: <b>token</b><br> 5482 * Path: <b>ResearchElementDefinition.useContext.valueCodeableConcept</b><br> 5483 * </p> 5484 */ 5485 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5486 SP_CONTEXT); 5487 5488 /** 5489 * Search parameter: <b>publisher</b> 5490 * <p> 5491 * Description: <b>Name of the publisher of the research element 5492 * definition</b><br> 5493 * Type: <b>string</b><br> 5494 * Path: <b>ResearchElementDefinition.publisher</b><br> 5495 * </p> 5496 */ 5497 @SearchParamDefinition(name = "publisher", path = "ResearchElementDefinition.publisher", description = "Name of the publisher of the research element definition", type = "string") 5498 public static final String SP_PUBLISHER = "publisher"; 5499 /** 5500 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5501 * <p> 5502 * Description: <b>Name of the publisher of the research element 5503 * definition</b><br> 5504 * Type: <b>string</b><br> 5505 * Path: <b>ResearchElementDefinition.publisher</b><br> 5506 * </p> 5507 */ 5508 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5509 SP_PUBLISHER); 5510 5511 /** 5512 * Search parameter: <b>topic</b> 5513 * <p> 5514 * Description: <b>Topics associated with the ResearchElementDefinition</b><br> 5515 * Type: <b>token</b><br> 5516 * Path: <b>ResearchElementDefinition.topic</b><br> 5517 * </p> 5518 */ 5519 @SearchParamDefinition(name = "topic", path = "ResearchElementDefinition.topic", description = "Topics associated with the ResearchElementDefinition", type = "token") 5520 public static final String SP_TOPIC = "topic"; 5521 /** 5522 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 5523 * <p> 5524 * Description: <b>Topics associated with the ResearchElementDefinition</b><br> 5525 * Type: <b>token</b><br> 5526 * Path: <b>ResearchElementDefinition.topic</b><br> 5527 * </p> 5528 */ 5529 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5530 SP_TOPIC); 5531 5532 /** 5533 * Search parameter: <b>context-type-quantity</b> 5534 * <p> 5535 * Description: <b>A use context type and quantity- or range-based value 5536 * assigned to the research element definition</b><br> 5537 * Type: <b>composite</b><br> 5538 * Path: <b></b><br> 5539 * </p> 5540 */ 5541 @SearchParamDefinition(name = "context-type-quantity", path = "ResearchElementDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the research element definition", type = "composite", compositeOf = { 5542 "context-type", "context-quantity" }) 5543 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5544 /** 5545 * <b>Fluent Client</b> search parameter constant for 5546 * <b>context-type-quantity</b> 5547 * <p> 5548 * Description: <b>A use context type and quantity- or range-based value 5549 * assigned to the research element definition</b><br> 5550 * Type: <b>composite</b><br> 5551 * Path: <b></b><br> 5552 * </p> 5553 */ 5554 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 5555 SP_CONTEXT_TYPE_QUANTITY); 5556 5557 /** 5558 * Search parameter: <b>status</b> 5559 * <p> 5560 * Description: <b>The current status of the research element definition</b><br> 5561 * Type: <b>token</b><br> 5562 * Path: <b>ResearchElementDefinition.status</b><br> 5563 * </p> 5564 */ 5565 @SearchParamDefinition(name = "status", path = "ResearchElementDefinition.status", description = "The current status of the research element definition", type = "token") 5566 public static final String SP_STATUS = "status"; 5567 /** 5568 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5569 * <p> 5570 * Description: <b>The current status of the research element definition</b><br> 5571 * Type: <b>token</b><br> 5572 * Path: <b>ResearchElementDefinition.status</b><br> 5573 * </p> 5574 */ 5575 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5576 SP_STATUS); 5577 5578}