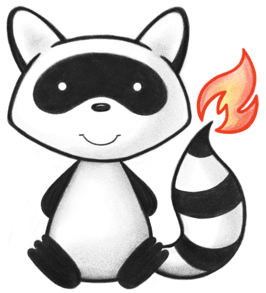
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A process where a researcher or organization plans and then executes a series 048 * of steps intended to increase the field of healthcare-related knowledge. This 049 * includes studies of safety, efficacy, comparative effectiveness and other 050 * information about medications, devices, therapies and other interventional 051 * and investigative techniques. A ResearchStudy involves the gathering of 052 * information about human or animal subjects. 053 */ 054@ResourceDef(name = "ResearchStudy", profile = "http://hl7.org/fhir/StructureDefinition/ResearchStudy") 055public class ResearchStudy extends DomainResource { 056 057 public enum ResearchStudyStatus { 058 /** 059 * Study is opened for accrual. 060 */ 061 ACTIVE, 062 /** 063 * Study is completed prematurely and will not resume; patients are no longer 064 * examined nor treated. 065 */ 066 ADMINISTRATIVELYCOMPLETED, 067 /** 068 * Protocol is approved by the review board. 069 */ 070 APPROVED, 071 /** 072 * Study is closed for accrual; patients can be examined and treated. 073 */ 074 CLOSEDTOACCRUAL, 075 /** 076 * Study is closed to accrual and intervention, i.e. the study is closed to 077 * enrollment, all study subjects have completed treatment or intervention but 078 * are still being followed according to the primary objective of the study. 079 */ 080 CLOSEDTOACCRUALANDINTERVENTION, 081 /** 082 * Study is closed to accrual and intervention, i.e. the study is closed to 083 * enrollment, all study subjects have completed treatment or intervention but 084 * are still being followed according to the primary objective of the study. 085 */ 086 COMPLETED, 087 /** 088 * Protocol was disapproved by the review board. 089 */ 090 DISAPPROVED, 091 /** 092 * Protocol is submitted to the review board for approval. 093 */ 094 INREVIEW, 095 /** 096 * Study is temporarily closed for accrual; can be potentially resumed in the 097 * future; patients can be examined and treated. 098 */ 099 TEMPORARILYCLOSEDTOACCRUAL, 100 /** 101 * Study is temporarily closed for accrual and intervention and potentially can 102 * be resumed in the future. 103 */ 104 TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION, 105 /** 106 * Protocol was withdrawn by the lead organization. 107 */ 108 WITHDRAWN, 109 /** 110 * added to help the parsers with the generic types 111 */ 112 NULL; 113 114 public static ResearchStudyStatus fromCode(String codeString) throws FHIRException { 115 if (codeString == null || "".equals(codeString)) 116 return null; 117 if ("active".equals(codeString)) 118 return ACTIVE; 119 if ("administratively-completed".equals(codeString)) 120 return ADMINISTRATIVELYCOMPLETED; 121 if ("approved".equals(codeString)) 122 return APPROVED; 123 if ("closed-to-accrual".equals(codeString)) 124 return CLOSEDTOACCRUAL; 125 if ("closed-to-accrual-and-intervention".equals(codeString)) 126 return CLOSEDTOACCRUALANDINTERVENTION; 127 if ("completed".equals(codeString)) 128 return COMPLETED; 129 if ("disapproved".equals(codeString)) 130 return DISAPPROVED; 131 if ("in-review".equals(codeString)) 132 return INREVIEW; 133 if ("temporarily-closed-to-accrual".equals(codeString)) 134 return TEMPORARILYCLOSEDTOACCRUAL; 135 if ("temporarily-closed-to-accrual-and-intervention".equals(codeString)) 136 return TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION; 137 if ("withdrawn".equals(codeString)) 138 return WITHDRAWN; 139 if (Configuration.isAcceptInvalidEnums()) 140 return null; 141 else 142 throw new FHIRException("Unknown ResearchStudyStatus code '" + codeString + "'"); 143 } 144 145 public String toCode() { 146 switch (this) { 147 case ACTIVE: 148 return "active"; 149 case ADMINISTRATIVELYCOMPLETED: 150 return "administratively-completed"; 151 case APPROVED: 152 return "approved"; 153 case CLOSEDTOACCRUAL: 154 return "closed-to-accrual"; 155 case CLOSEDTOACCRUALANDINTERVENTION: 156 return "closed-to-accrual-and-intervention"; 157 case COMPLETED: 158 return "completed"; 159 case DISAPPROVED: 160 return "disapproved"; 161 case INREVIEW: 162 return "in-review"; 163 case TEMPORARILYCLOSEDTOACCRUAL: 164 return "temporarily-closed-to-accrual"; 165 case TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION: 166 return "temporarily-closed-to-accrual-and-intervention"; 167 case WITHDRAWN: 168 return "withdrawn"; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 176 public String getSystem() { 177 switch (this) { 178 case ACTIVE: 179 return "http://hl7.org/fhir/research-study-status"; 180 case ADMINISTRATIVELYCOMPLETED: 181 return "http://hl7.org/fhir/research-study-status"; 182 case APPROVED: 183 return "http://hl7.org/fhir/research-study-status"; 184 case CLOSEDTOACCRUAL: 185 return "http://hl7.org/fhir/research-study-status"; 186 case CLOSEDTOACCRUALANDINTERVENTION: 187 return "http://hl7.org/fhir/research-study-status"; 188 case COMPLETED: 189 return "http://hl7.org/fhir/research-study-status"; 190 case DISAPPROVED: 191 return "http://hl7.org/fhir/research-study-status"; 192 case INREVIEW: 193 return "http://hl7.org/fhir/research-study-status"; 194 case TEMPORARILYCLOSEDTOACCRUAL: 195 return "http://hl7.org/fhir/research-study-status"; 196 case TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION: 197 return "http://hl7.org/fhir/research-study-status"; 198 case WITHDRAWN: 199 return "http://hl7.org/fhir/research-study-status"; 200 case NULL: 201 return null; 202 default: 203 return "?"; 204 } 205 } 206 207 public String getDefinition() { 208 switch (this) { 209 case ACTIVE: 210 return "Study is opened for accrual."; 211 case ADMINISTRATIVELYCOMPLETED: 212 return "Study is completed prematurely and will not resume; patients are no longer examined nor treated."; 213 case APPROVED: 214 return "Protocol is approved by the review board."; 215 case CLOSEDTOACCRUAL: 216 return "Study is closed for accrual; patients can be examined and treated."; 217 case CLOSEDTOACCRUALANDINTERVENTION: 218 return "Study is closed to accrual and intervention, i.e. the study is closed to enrollment, all study subjects have completed treatment or intervention but are still being followed according to the primary objective of the study."; 219 case COMPLETED: 220 return "Study is closed to accrual and intervention, i.e. the study is closed to enrollment, all study subjects have completed treatment\nor intervention but are still being followed according to the primary objective of the study."; 221 case DISAPPROVED: 222 return "Protocol was disapproved by the review board."; 223 case INREVIEW: 224 return "Protocol is submitted to the review board for approval."; 225 case TEMPORARILYCLOSEDTOACCRUAL: 226 return "Study is temporarily closed for accrual; can be potentially resumed in the future; patients can be examined and treated."; 227 case TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION: 228 return "Study is temporarily closed for accrual and intervention and potentially can be resumed in the future."; 229 case WITHDRAWN: 230 return "Protocol was withdrawn by the lead organization."; 231 case NULL: 232 return null; 233 default: 234 return "?"; 235 } 236 } 237 238 public String getDisplay() { 239 switch (this) { 240 case ACTIVE: 241 return "Active"; 242 case ADMINISTRATIVELYCOMPLETED: 243 return "Administratively Completed"; 244 case APPROVED: 245 return "Approved"; 246 case CLOSEDTOACCRUAL: 247 return "Closed to Accrual"; 248 case CLOSEDTOACCRUALANDINTERVENTION: 249 return "Closed to Accrual and Intervention"; 250 case COMPLETED: 251 return "Completed"; 252 case DISAPPROVED: 253 return "Disapproved"; 254 case INREVIEW: 255 return "In Review"; 256 case TEMPORARILYCLOSEDTOACCRUAL: 257 return "Temporarily Closed to Accrual"; 258 case TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION: 259 return "Temporarily Closed to Accrual and Intervention"; 260 case WITHDRAWN: 261 return "Withdrawn"; 262 case NULL: 263 return null; 264 default: 265 return "?"; 266 } 267 } 268 } 269 270 public static class ResearchStudyStatusEnumFactory implements EnumFactory<ResearchStudyStatus> { 271 public ResearchStudyStatus fromCode(String codeString) throws IllegalArgumentException { 272 if (codeString == null || "".equals(codeString)) 273 if (codeString == null || "".equals(codeString)) 274 return null; 275 if ("active".equals(codeString)) 276 return ResearchStudyStatus.ACTIVE; 277 if ("administratively-completed".equals(codeString)) 278 return ResearchStudyStatus.ADMINISTRATIVELYCOMPLETED; 279 if ("approved".equals(codeString)) 280 return ResearchStudyStatus.APPROVED; 281 if ("closed-to-accrual".equals(codeString)) 282 return ResearchStudyStatus.CLOSEDTOACCRUAL; 283 if ("closed-to-accrual-and-intervention".equals(codeString)) 284 return ResearchStudyStatus.CLOSEDTOACCRUALANDINTERVENTION; 285 if ("completed".equals(codeString)) 286 return ResearchStudyStatus.COMPLETED; 287 if ("disapproved".equals(codeString)) 288 return ResearchStudyStatus.DISAPPROVED; 289 if ("in-review".equals(codeString)) 290 return ResearchStudyStatus.INREVIEW; 291 if ("temporarily-closed-to-accrual".equals(codeString)) 292 return ResearchStudyStatus.TEMPORARILYCLOSEDTOACCRUAL; 293 if ("temporarily-closed-to-accrual-and-intervention".equals(codeString)) 294 return ResearchStudyStatus.TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION; 295 if ("withdrawn".equals(codeString)) 296 return ResearchStudyStatus.WITHDRAWN; 297 throw new IllegalArgumentException("Unknown ResearchStudyStatus code '" + codeString + "'"); 298 } 299 300 public Enumeration<ResearchStudyStatus> fromType(PrimitiveType<?> code) throws FHIRException { 301 if (code == null) 302 return null; 303 if (code.isEmpty()) 304 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.NULL, code); 305 String codeString = code.asStringValue(); 306 if (codeString == null || "".equals(codeString)) 307 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.NULL, code); 308 if ("active".equals(codeString)) 309 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.ACTIVE, code); 310 if ("administratively-completed".equals(codeString)) 311 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.ADMINISTRATIVELYCOMPLETED, code); 312 if ("approved".equals(codeString)) 313 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.APPROVED, code); 314 if ("closed-to-accrual".equals(codeString)) 315 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.CLOSEDTOACCRUAL, code); 316 if ("closed-to-accrual-and-intervention".equals(codeString)) 317 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.CLOSEDTOACCRUALANDINTERVENTION, code); 318 if ("completed".equals(codeString)) 319 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.COMPLETED, code); 320 if ("disapproved".equals(codeString)) 321 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.DISAPPROVED, code); 322 if ("in-review".equals(codeString)) 323 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.INREVIEW, code); 324 if ("temporarily-closed-to-accrual".equals(codeString)) 325 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.TEMPORARILYCLOSEDTOACCRUAL, code); 326 if ("temporarily-closed-to-accrual-and-intervention".equals(codeString)) 327 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION, 328 code); 329 if ("withdrawn".equals(codeString)) 330 return new Enumeration<ResearchStudyStatus>(this, ResearchStudyStatus.WITHDRAWN, code); 331 throw new FHIRException("Unknown ResearchStudyStatus code '" + codeString + "'"); 332 } 333 334 public String toCode(ResearchStudyStatus code) { 335 if (code == ResearchStudyStatus.NULL) 336 return null; 337 if (code == ResearchStudyStatus.ACTIVE) 338 return "active"; 339 if (code == ResearchStudyStatus.ADMINISTRATIVELYCOMPLETED) 340 return "administratively-completed"; 341 if (code == ResearchStudyStatus.APPROVED) 342 return "approved"; 343 if (code == ResearchStudyStatus.CLOSEDTOACCRUAL) 344 return "closed-to-accrual"; 345 if (code == ResearchStudyStatus.CLOSEDTOACCRUALANDINTERVENTION) 346 return "closed-to-accrual-and-intervention"; 347 if (code == ResearchStudyStatus.COMPLETED) 348 return "completed"; 349 if (code == ResearchStudyStatus.DISAPPROVED) 350 return "disapproved"; 351 if (code == ResearchStudyStatus.INREVIEW) 352 return "in-review"; 353 if (code == ResearchStudyStatus.TEMPORARILYCLOSEDTOACCRUAL) 354 return "temporarily-closed-to-accrual"; 355 if (code == ResearchStudyStatus.TEMPORARILYCLOSEDTOACCRUALANDINTERVENTION) 356 return "temporarily-closed-to-accrual-and-intervention"; 357 if (code == ResearchStudyStatus.WITHDRAWN) 358 return "withdrawn"; 359 return "?"; 360 } 361 362 public String toSystem(ResearchStudyStatus code) { 363 return code.getSystem(); 364 } 365 } 366 367 @Block() 368 public static class ResearchStudyArmComponent extends BackboneElement implements IBaseBackboneElement { 369 /** 370 * Unique, human-readable label for this arm of the study. 371 */ 372 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 373 @Description(shortDefinition = "Label for study arm", formalDefinition = "Unique, human-readable label for this arm of the study.") 374 protected StringType name; 375 376 /** 377 * Categorization of study arm, e.g. experimental, active comparator, placebo 378 * comparater. 379 */ 380 @Child(name = "type", type = { 381 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 382 @Description(shortDefinition = "Categorization of study arm", formalDefinition = "Categorization of study arm, e.g. experimental, active comparator, placebo comparater.") 383 protected CodeableConcept type; 384 385 /** 386 * A succinct description of the path through the study that would be followed 387 * by a subject adhering to this arm. 388 */ 389 @Child(name = "description", type = { 390 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 391 @Description(shortDefinition = "Short explanation of study path", formalDefinition = "A succinct description of the path through the study that would be followed by a subject adhering to this arm.") 392 protected StringType description; 393 394 private static final long serialVersionUID = 311445244L; 395 396 /** 397 * Constructor 398 */ 399 public ResearchStudyArmComponent() { 400 super(); 401 } 402 403 /** 404 * Constructor 405 */ 406 public ResearchStudyArmComponent(StringType name) { 407 super(); 408 this.name = name; 409 } 410 411 /** 412 * @return {@link #name} (Unique, human-readable label for this arm of the 413 * study.). This is the underlying object with id, value and extensions. 414 * The accessor "getName" gives direct access to the value 415 */ 416 public StringType getNameElement() { 417 if (this.name == null) 418 if (Configuration.errorOnAutoCreate()) 419 throw new Error("Attempt to auto-create ResearchStudyArmComponent.name"); 420 else if (Configuration.doAutoCreate()) 421 this.name = new StringType(); // bb 422 return this.name; 423 } 424 425 public boolean hasNameElement() { 426 return this.name != null && !this.name.isEmpty(); 427 } 428 429 public boolean hasName() { 430 return this.name != null && !this.name.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #name} (Unique, human-readable label for this arm of the 435 * study.). This is the underlying object with id, value and 436 * extensions. The accessor "getName" gives direct access to the 437 * value 438 */ 439 public ResearchStudyArmComponent setNameElement(StringType value) { 440 this.name = value; 441 return this; 442 } 443 444 /** 445 * @return Unique, human-readable label for this arm of the study. 446 */ 447 public String getName() { 448 return this.name == null ? null : this.name.getValue(); 449 } 450 451 /** 452 * @param value Unique, human-readable label for this arm of the study. 453 */ 454 public ResearchStudyArmComponent setName(String value) { 455 if (this.name == null) 456 this.name = new StringType(); 457 this.name.setValue(value); 458 return this; 459 } 460 461 /** 462 * @return {@link #type} (Categorization of study arm, e.g. experimental, active 463 * comparator, placebo comparater.) 464 */ 465 public CodeableConcept getType() { 466 if (this.type == null) 467 if (Configuration.errorOnAutoCreate()) 468 throw new Error("Attempt to auto-create ResearchStudyArmComponent.type"); 469 else if (Configuration.doAutoCreate()) 470 this.type = new CodeableConcept(); // cc 471 return this.type; 472 } 473 474 public boolean hasType() { 475 return this.type != null && !this.type.isEmpty(); 476 } 477 478 /** 479 * @param value {@link #type} (Categorization of study arm, e.g. experimental, 480 * active comparator, placebo comparater.) 481 */ 482 public ResearchStudyArmComponent setType(CodeableConcept value) { 483 this.type = value; 484 return this; 485 } 486 487 /** 488 * @return {@link #description} (A succinct description of the path through the 489 * study that would be followed by a subject adhering to this arm.). 490 * This is the underlying object with id, value and extensions. The 491 * accessor "getDescription" gives direct access to the value 492 */ 493 public StringType getDescriptionElement() { 494 if (this.description == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create ResearchStudyArmComponent.description"); 497 else if (Configuration.doAutoCreate()) 498 this.description = new StringType(); // bb 499 return this.description; 500 } 501 502 public boolean hasDescriptionElement() { 503 return this.description != null && !this.description.isEmpty(); 504 } 505 506 public boolean hasDescription() { 507 return this.description != null && !this.description.isEmpty(); 508 } 509 510 /** 511 * @param value {@link #description} (A succinct description of the path through 512 * the study that would be followed by a subject adhering to this 513 * arm.). This is the underlying object with id, value and 514 * extensions. The accessor "getDescription" gives direct access to 515 * the value 516 */ 517 public ResearchStudyArmComponent setDescriptionElement(StringType value) { 518 this.description = value; 519 return this; 520 } 521 522 /** 523 * @return A succinct description of the path through the study that would be 524 * followed by a subject adhering to this arm. 525 */ 526 public String getDescription() { 527 return this.description == null ? null : this.description.getValue(); 528 } 529 530 /** 531 * @param value A succinct description of the path through the study that would 532 * be followed by a subject adhering to this arm. 533 */ 534 public ResearchStudyArmComponent setDescription(String value) { 535 if (Utilities.noString(value)) 536 this.description = null; 537 else { 538 if (this.description == null) 539 this.description = new StringType(); 540 this.description.setValue(value); 541 } 542 return this; 543 } 544 545 protected void listChildren(List<Property> children) { 546 super.listChildren(children); 547 children 548 .add(new Property("name", "string", "Unique, human-readable label for this arm of the study.", 0, 1, name)); 549 children.add(new Property("type", "CodeableConcept", 550 "Categorization of study arm, e.g. experimental, active comparator, placebo comparater.", 0, 1, type)); 551 children.add(new Property("description", "string", 552 "A succinct description of the path through the study that would be followed by a subject adhering to this arm.", 553 0, 1, description)); 554 } 555 556 @Override 557 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 558 switch (_hash) { 559 case 3373707: 560 /* name */ return new Property("name", "string", "Unique, human-readable label for this arm of the study.", 0, 561 1, name); 562 case 3575610: 563 /* type */ return new Property("type", "CodeableConcept", 564 "Categorization of study arm, e.g. experimental, active comparator, placebo comparater.", 0, 1, type); 565 case -1724546052: 566 /* description */ return new Property("description", "string", 567 "A succinct description of the path through the study that would be followed by a subject adhering to this arm.", 568 0, 1, description); 569 default: 570 return super.getNamedProperty(_hash, _name, _checkValid); 571 } 572 573 } 574 575 @Override 576 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 577 switch (hash) { 578 case 3373707: 579 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 580 case 3575610: 581 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 582 case -1724546052: 583 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 584 default: 585 return super.getProperty(hash, name, checkValid); 586 } 587 588 } 589 590 @Override 591 public Base setProperty(int hash, String name, Base value) throws FHIRException { 592 switch (hash) { 593 case 3373707: // name 594 this.name = castToString(value); // StringType 595 return value; 596 case 3575610: // type 597 this.type = castToCodeableConcept(value); // CodeableConcept 598 return value; 599 case -1724546052: // description 600 this.description = castToString(value); // StringType 601 return value; 602 default: 603 return super.setProperty(hash, name, value); 604 } 605 606 } 607 608 @Override 609 public Base setProperty(String name, Base value) throws FHIRException { 610 if (name.equals("name")) { 611 this.name = castToString(value); // StringType 612 } else if (name.equals("type")) { 613 this.type = castToCodeableConcept(value); // CodeableConcept 614 } else if (name.equals("description")) { 615 this.description = castToString(value); // StringType 616 } else 617 return super.setProperty(name, value); 618 return value; 619 } 620 621 @Override 622 public void removeChild(String name, Base value) throws FHIRException { 623 if (name.equals("name")) { 624 this.name = null; 625 } else if (name.equals("type")) { 626 this.type = null; 627 } else if (name.equals("description")) { 628 this.description = null; 629 } else 630 super.removeChild(name, value); 631 632 } 633 634 @Override 635 public Base makeProperty(int hash, String name) throws FHIRException { 636 switch (hash) { 637 case 3373707: 638 return getNameElement(); 639 case 3575610: 640 return getType(); 641 case -1724546052: 642 return getDescriptionElement(); 643 default: 644 return super.makeProperty(hash, name); 645 } 646 647 } 648 649 @Override 650 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 651 switch (hash) { 652 case 3373707: 653 /* name */ return new String[] { "string" }; 654 case 3575610: 655 /* type */ return new String[] { "CodeableConcept" }; 656 case -1724546052: 657 /* description */ return new String[] { "string" }; 658 default: 659 return super.getTypesForProperty(hash, name); 660 } 661 662 } 663 664 @Override 665 public Base addChild(String name) throws FHIRException { 666 if (name.equals("name")) { 667 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.name"); 668 } else if (name.equals("type")) { 669 this.type = new CodeableConcept(); 670 return this.type; 671 } else if (name.equals("description")) { 672 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.description"); 673 } else 674 return super.addChild(name); 675 } 676 677 public ResearchStudyArmComponent copy() { 678 ResearchStudyArmComponent dst = new ResearchStudyArmComponent(); 679 copyValues(dst); 680 return dst; 681 } 682 683 public void copyValues(ResearchStudyArmComponent dst) { 684 super.copyValues(dst); 685 dst.name = name == null ? null : name.copy(); 686 dst.type = type == null ? null : type.copy(); 687 dst.description = description == null ? null : description.copy(); 688 } 689 690 @Override 691 public boolean equalsDeep(Base other_) { 692 if (!super.equalsDeep(other_)) 693 return false; 694 if (!(other_ instanceof ResearchStudyArmComponent)) 695 return false; 696 ResearchStudyArmComponent o = (ResearchStudyArmComponent) other_; 697 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) 698 && compareDeep(description, o.description, true); 699 } 700 701 @Override 702 public boolean equalsShallow(Base other_) { 703 if (!super.equalsShallow(other_)) 704 return false; 705 if (!(other_ instanceof ResearchStudyArmComponent)) 706 return false; 707 ResearchStudyArmComponent o = (ResearchStudyArmComponent) other_; 708 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 709 } 710 711 public boolean isEmpty() { 712 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, description); 713 } 714 715 public String fhirType() { 716 return "ResearchStudy.arm"; 717 718 } 719 720 } 721 722 @Block() 723 public static class ResearchStudyObjectiveComponent extends BackboneElement implements IBaseBackboneElement { 724 /** 725 * Unique, human-readable label for this objective of the study. 726 */ 727 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 728 @Description(shortDefinition = "Label for the objective", formalDefinition = "Unique, human-readable label for this objective of the study.") 729 protected StringType name; 730 731 /** 732 * The kind of study objective. 733 */ 734 @Child(name = "type", type = { 735 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 736 @Description(shortDefinition = "primary | secondary | exploratory", formalDefinition = "The kind of study objective.") 737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/research-study-objective-type") 738 protected CodeableConcept type; 739 740 private static final long serialVersionUID = -1935215997L; 741 742 /** 743 * Constructor 744 */ 745 public ResearchStudyObjectiveComponent() { 746 super(); 747 } 748 749 /** 750 * @return {@link #name} (Unique, human-readable label for this objective of the 751 * study.). This is the underlying object with id, value and extensions. 752 * The accessor "getName" gives direct access to the value 753 */ 754 public StringType getNameElement() { 755 if (this.name == null) 756 if (Configuration.errorOnAutoCreate()) 757 throw new Error("Attempt to auto-create ResearchStudyObjectiveComponent.name"); 758 else if (Configuration.doAutoCreate()) 759 this.name = new StringType(); // bb 760 return this.name; 761 } 762 763 public boolean hasNameElement() { 764 return this.name != null && !this.name.isEmpty(); 765 } 766 767 public boolean hasName() { 768 return this.name != null && !this.name.isEmpty(); 769 } 770 771 /** 772 * @param value {@link #name} (Unique, human-readable label for this objective 773 * of the study.). This is the underlying object with id, value and 774 * extensions. The accessor "getName" gives direct access to the 775 * value 776 */ 777 public ResearchStudyObjectiveComponent setNameElement(StringType value) { 778 this.name = value; 779 return this; 780 } 781 782 /** 783 * @return Unique, human-readable label for this objective of the study. 784 */ 785 public String getName() { 786 return this.name == null ? null : this.name.getValue(); 787 } 788 789 /** 790 * @param value Unique, human-readable label for this objective of the study. 791 */ 792 public ResearchStudyObjectiveComponent setName(String value) { 793 if (Utilities.noString(value)) 794 this.name = null; 795 else { 796 if (this.name == null) 797 this.name = new StringType(); 798 this.name.setValue(value); 799 } 800 return this; 801 } 802 803 /** 804 * @return {@link #type} (The kind of study objective.) 805 */ 806 public CodeableConcept getType() { 807 if (this.type == null) 808 if (Configuration.errorOnAutoCreate()) 809 throw new Error("Attempt to auto-create ResearchStudyObjectiveComponent.type"); 810 else if (Configuration.doAutoCreate()) 811 this.type = new CodeableConcept(); // cc 812 return this.type; 813 } 814 815 public boolean hasType() { 816 return this.type != null && !this.type.isEmpty(); 817 } 818 819 /** 820 * @param value {@link #type} (The kind of study objective.) 821 */ 822 public ResearchStudyObjectiveComponent setType(CodeableConcept value) { 823 this.type = value; 824 return this; 825 } 826 827 protected void listChildren(List<Property> children) { 828 super.listChildren(children); 829 children.add( 830 new Property("name", "string", "Unique, human-readable label for this objective of the study.", 0, 1, name)); 831 children.add(new Property("type", "CodeableConcept", "The kind of study objective.", 0, 1, type)); 832 } 833 834 @Override 835 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 836 switch (_hash) { 837 case 3373707: 838 /* name */ return new Property("name", "string", 839 "Unique, human-readable label for this objective of the study.", 0, 1, name); 840 case 3575610: 841 /* type */ return new Property("type", "CodeableConcept", "The kind of study objective.", 0, 1, type); 842 default: 843 return super.getNamedProperty(_hash, _name, _checkValid); 844 } 845 846 } 847 848 @Override 849 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 850 switch (hash) { 851 case 3373707: 852 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 853 case 3575610: 854 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 855 default: 856 return super.getProperty(hash, name, checkValid); 857 } 858 859 } 860 861 @Override 862 public Base setProperty(int hash, String name, Base value) throws FHIRException { 863 switch (hash) { 864 case 3373707: // name 865 this.name = castToString(value); // StringType 866 return value; 867 case 3575610: // type 868 this.type = castToCodeableConcept(value); // CodeableConcept 869 return value; 870 default: 871 return super.setProperty(hash, name, value); 872 } 873 874 } 875 876 @Override 877 public Base setProperty(String name, Base value) throws FHIRException { 878 if (name.equals("name")) { 879 this.name = castToString(value); // StringType 880 } else if (name.equals("type")) { 881 this.type = castToCodeableConcept(value); // CodeableConcept 882 } else 883 return super.setProperty(name, value); 884 return value; 885 } 886 887 @Override 888 public void removeChild(String name, Base value) throws FHIRException { 889 if (name.equals("name")) { 890 this.name = null; 891 } else if (name.equals("type")) { 892 this.type = null; 893 } else 894 super.removeChild(name, value); 895 896 } 897 898 @Override 899 public Base makeProperty(int hash, String name) throws FHIRException { 900 switch (hash) { 901 case 3373707: 902 return getNameElement(); 903 case 3575610: 904 return getType(); 905 default: 906 return super.makeProperty(hash, name); 907 } 908 909 } 910 911 @Override 912 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 913 switch (hash) { 914 case 3373707: 915 /* name */ return new String[] { "string" }; 916 case 3575610: 917 /* type */ return new String[] { "CodeableConcept" }; 918 default: 919 return super.getTypesForProperty(hash, name); 920 } 921 922 } 923 924 @Override 925 public Base addChild(String name) throws FHIRException { 926 if (name.equals("name")) { 927 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.name"); 928 } else if (name.equals("type")) { 929 this.type = new CodeableConcept(); 930 return this.type; 931 } else 932 return super.addChild(name); 933 } 934 935 public ResearchStudyObjectiveComponent copy() { 936 ResearchStudyObjectiveComponent dst = new ResearchStudyObjectiveComponent(); 937 copyValues(dst); 938 return dst; 939 } 940 941 public void copyValues(ResearchStudyObjectiveComponent dst) { 942 super.copyValues(dst); 943 dst.name = name == null ? null : name.copy(); 944 dst.type = type == null ? null : type.copy(); 945 } 946 947 @Override 948 public boolean equalsDeep(Base other_) { 949 if (!super.equalsDeep(other_)) 950 return false; 951 if (!(other_ instanceof ResearchStudyObjectiveComponent)) 952 return false; 953 ResearchStudyObjectiveComponent o = (ResearchStudyObjectiveComponent) other_; 954 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true); 955 } 956 957 @Override 958 public boolean equalsShallow(Base other_) { 959 if (!super.equalsShallow(other_)) 960 return false; 961 if (!(other_ instanceof ResearchStudyObjectiveComponent)) 962 return false; 963 ResearchStudyObjectiveComponent o = (ResearchStudyObjectiveComponent) other_; 964 return compareValues(name, o.name, true); 965 } 966 967 public boolean isEmpty() { 968 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type); 969 } 970 971 public String fhirType() { 972 return "ResearchStudy.objective"; 973 974 } 975 976 } 977 978 /** 979 * Identifiers assigned to this research study by the sponsor or other systems. 980 */ 981 @Child(name = "identifier", type = { 982 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 983 @Description(shortDefinition = "Business Identifier for study", formalDefinition = "Identifiers assigned to this research study by the sponsor or other systems.") 984 protected List<Identifier> identifier; 985 986 /** 987 * A short, descriptive user-friendly label for the study. 988 */ 989 @Child(name = "title", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 990 @Description(shortDefinition = "Name for this study", formalDefinition = "A short, descriptive user-friendly label for the study.") 991 protected StringType title; 992 993 /** 994 * The set of steps expected to be performed as part of the execution of the 995 * study. 996 */ 997 @Child(name = "protocol", type = { 998 PlanDefinition.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 999 @Description(shortDefinition = "Steps followed in executing study", formalDefinition = "The set of steps expected to be performed as part of the execution of the study.") 1000 protected List<Reference> protocol; 1001 /** 1002 * The actual objects that are the target of the reference (The set of steps 1003 * expected to be performed as part of the execution of the study.) 1004 */ 1005 protected List<PlanDefinition> protocolTarget; 1006 1007 /** 1008 * A larger research study of which this particular study is a component or 1009 * step. 1010 */ 1011 @Child(name = "partOf", type = { 1012 ResearchStudy.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1013 @Description(shortDefinition = "Part of larger study", formalDefinition = "A larger research study of which this particular study is a component or step.") 1014 protected List<Reference> partOf; 1015 /** 1016 * The actual objects that are the target of the reference (A larger research 1017 * study of which this particular study is a component or step.) 1018 */ 1019 protected List<ResearchStudy> partOfTarget; 1020 1021 /** 1022 * The current state of the study. 1023 */ 1024 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 1025 @Description(shortDefinition = "active | administratively-completed | approved | closed-to-accrual | closed-to-accrual-and-intervention | completed | disapproved | in-review | temporarily-closed-to-accrual | temporarily-closed-to-accrual-and-intervention | withdrawn", formalDefinition = "The current state of the study.") 1026 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/research-study-status") 1027 protected Enumeration<ResearchStudyStatus> status; 1028 1029 /** 1030 * The type of study based upon the intent of the study's activities. A 1031 * classification of the intent of the study. 1032 */ 1033 @Child(name = "primaryPurposeType", type = { 1034 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1035 @Description(shortDefinition = "treatment | prevention | diagnostic | supportive-care | screening | health-services-research | basic-science | device-feasibility", formalDefinition = "The type of study based upon the intent of the study's activities. A classification of the intent of the study.") 1036 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/research-study-prim-purp-type") 1037 protected CodeableConcept primaryPurposeType; 1038 1039 /** 1040 * The stage in the progression of a therapy from initial experimental use in 1041 * humans in clinical trials to post-market evaluation. 1042 */ 1043 @Child(name = "phase", type = { 1044 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1045 @Description(shortDefinition = "n-a | early-phase-1 | phase-1 | phase-1-phase-2 | phase-2 | phase-2-phase-3 | phase-3 | phase-4", formalDefinition = "The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.") 1046 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/research-study-phase") 1047 protected CodeableConcept phase; 1048 1049 /** 1050 * Codes categorizing the type of study such as investigational vs. 1051 * observational, type of blinding, type of randomization, safety vs. efficacy, 1052 * etc. 1053 */ 1054 @Child(name = "category", type = { 1055 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1056 @Description(shortDefinition = "Classifications for the study", formalDefinition = "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.") 1057 protected List<CodeableConcept> category; 1058 1059 /** 1060 * The medication(s), food(s), therapy(ies), device(s) or other concerns or 1061 * interventions that the study is seeking to gain more information about. 1062 */ 1063 @Child(name = "focus", type = { 1064 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1065 @Description(shortDefinition = "Drugs, devices, etc. under study", formalDefinition = "The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.") 1066 protected List<CodeableConcept> focus; 1067 1068 /** 1069 * The condition that is the focus of the study. For example, In a study to 1070 * examine risk factors for Lupus, might have as an inclusion criterion "healthy 1071 * volunteer", but the target condition code would be a Lupus SNOMED code. 1072 */ 1073 @Child(name = "condition", type = { 1074 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1075 @Description(shortDefinition = "Condition being studied", formalDefinition = "The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code.") 1076 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 1077 protected List<CodeableConcept> condition; 1078 1079 /** 1080 * Contact details to assist a user in learning more about or engaging with the 1081 * study. 1082 */ 1083 @Child(name = "contact", type = { 1084 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1085 @Description(shortDefinition = "Contact details for the study", formalDefinition = "Contact details to assist a user in learning more about or engaging with the study.") 1086 protected List<ContactDetail> contact; 1087 1088 /** 1089 * Citations, references and other related documents. 1090 */ 1091 @Child(name = "relatedArtifact", type = { 1092 RelatedArtifact.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1093 @Description(shortDefinition = "References and dependencies", formalDefinition = "Citations, references and other related documents.") 1094 protected List<RelatedArtifact> relatedArtifact; 1095 1096 /** 1097 * Key terms to aid in searching for or filtering the study. 1098 */ 1099 @Child(name = "keyword", type = { 1100 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1101 @Description(shortDefinition = "Used to search for the study", formalDefinition = "Key terms to aid in searching for or filtering the study.") 1102 protected List<CodeableConcept> keyword; 1103 1104 /** 1105 * Indicates a country, state or other region where the study is taking place. 1106 */ 1107 @Child(name = "location", type = { 1108 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1109 @Description(shortDefinition = "Geographic region(s) for study", formalDefinition = "Indicates a country, state or other region where the study is taking place.") 1110 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/jurisdiction") 1111 protected List<CodeableConcept> location; 1112 1113 /** 1114 * A full description of how the study is being conducted. 1115 */ 1116 @Child(name = "description", type = { 1117 MarkdownType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1118 @Description(shortDefinition = "What this is study doing", formalDefinition = "A full description of how the study is being conducted.") 1119 protected MarkdownType description; 1120 1121 /** 1122 * Reference to a Group that defines the criteria for and quantity of subjects 1123 * participating in the study. E.g. " 200 female Europeans between the ages of 1124 * 20 and 45 with early onset diabetes". 1125 */ 1126 @Child(name = "enrollment", type = { 1127 Group.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1128 @Description(shortDefinition = "Inclusion & exclusion criteria", formalDefinition = "Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. \" 200 female Europeans between the ages of 20 and 45 with early onset diabetes\".") 1129 protected List<Reference> enrollment; 1130 /** 1131 * The actual objects that are the target of the reference (Reference to a Group 1132 * that defines the criteria for and quantity of subjects participating in the 1133 * study. E.g. " 200 female Europeans between the ages of 20 and 45 with early 1134 * onset diabetes".) 1135 */ 1136 protected List<Group> enrollmentTarget; 1137 1138 /** 1139 * Identifies the start date and the expected (or actual, depending on status) 1140 * end date for the study. 1141 */ 1142 @Child(name = "period", type = { Period.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 1143 @Description(shortDefinition = "When the study began and ended", formalDefinition = "Identifies the start date and the expected (or actual, depending on status) end date for the study.") 1144 protected Period period; 1145 1146 /** 1147 * An organization that initiates the investigation and is legally responsible 1148 * for the study. 1149 */ 1150 @Child(name = "sponsor", type = { 1151 Organization.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 1152 @Description(shortDefinition = "Organization that initiates and is legally responsible for the study", formalDefinition = "An organization that initiates the investigation and is legally responsible for the study.") 1153 protected Reference sponsor; 1154 1155 /** 1156 * The actual object that is the target of the reference (An organization that 1157 * initiates the investigation and is legally responsible for the study.) 1158 */ 1159 protected Organization sponsorTarget; 1160 1161 /** 1162 * A researcher in a study who oversees multiple aspects of the study, such as 1163 * concept development, protocol writing, protocol submission for IRB approval, 1164 * participant recruitment, informed consent, data collection, analysis, 1165 * interpretation and presentation. 1166 */ 1167 @Child(name = "principalInvestigator", type = { Practitioner.class, 1168 PractitionerRole.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 1169 @Description(shortDefinition = "Researcher who oversees multiple aspects of the study", formalDefinition = "A researcher in a study who oversees multiple aspects of the study, such as concept development, protocol writing, protocol submission for IRB approval, participant recruitment, informed consent, data collection, analysis, interpretation and presentation.") 1170 protected Reference principalInvestigator; 1171 1172 /** 1173 * The actual object that is the target of the reference (A researcher in a 1174 * study who oversees multiple aspects of the study, such as concept 1175 * development, protocol writing, protocol submission for IRB approval, 1176 * participant recruitment, informed consent, data collection, analysis, 1177 * interpretation and presentation.) 1178 */ 1179 protected Resource principalInvestigatorTarget; 1180 1181 /** 1182 * A facility in which study activities are conducted. 1183 */ 1184 @Child(name = "site", type = { 1185 Location.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1186 @Description(shortDefinition = "Facility where study activities are conducted", formalDefinition = "A facility in which study activities are conducted.") 1187 protected List<Reference> site; 1188 /** 1189 * The actual objects that are the target of the reference (A facility in which 1190 * study activities are conducted.) 1191 */ 1192 protected List<Location> siteTarget; 1193 1194 /** 1195 * A description and/or code explaining the premature termination of the study. 1196 */ 1197 @Child(name = "reasonStopped", type = { 1198 CodeableConcept.class }, order = 20, min = 0, max = 1, modifier = false, summary = true) 1199 @Description(shortDefinition = "accrual-goal-met | closed-due-to-toxicity | closed-due-to-lack-of-study-progress | temporarily-closed-per-study-design", formalDefinition = "A description and/or code explaining the premature termination of the study.") 1200 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/research-study-reason-stopped") 1201 protected CodeableConcept reasonStopped; 1202 1203 /** 1204 * Comments made about the study by the performer, subject or other 1205 * participants. 1206 */ 1207 @Child(name = "note", type = { 1208 Annotation.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1209 @Description(shortDefinition = "Comments made about the study", formalDefinition = "Comments made about the study by the performer, subject or other participants.") 1210 protected List<Annotation> note; 1211 1212 /** 1213 * Describes an expected sequence of events for one of the participants of a 1214 * study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, 1215 * follow-up. 1216 */ 1217 @Child(name = "arm", type = {}, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1218 @Description(shortDefinition = "Defined path through the study for a subject", formalDefinition = "Describes an expected sequence of events for one of the participants of a study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up.") 1219 protected List<ResearchStudyArmComponent> arm; 1220 1221 /** 1222 * A goal that the study is aiming to achieve in terms of a scientific question 1223 * to be answered by the analysis of data collected during the study. 1224 */ 1225 @Child(name = "objective", type = {}, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1226 @Description(shortDefinition = "A goal for the study", formalDefinition = "A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.") 1227 protected List<ResearchStudyObjectiveComponent> objective; 1228 1229 private static final long serialVersionUID = -911538323L; 1230 1231 /** 1232 * Constructor 1233 */ 1234 public ResearchStudy() { 1235 super(); 1236 } 1237 1238 /** 1239 * Constructor 1240 */ 1241 public ResearchStudy(Enumeration<ResearchStudyStatus> status) { 1242 super(); 1243 this.status = status; 1244 } 1245 1246 /** 1247 * @return {@link #identifier} (Identifiers assigned to this research study by 1248 * the sponsor or other systems.) 1249 */ 1250 public List<Identifier> getIdentifier() { 1251 if (this.identifier == null) 1252 this.identifier = new ArrayList<Identifier>(); 1253 return this.identifier; 1254 } 1255 1256 /** 1257 * @return Returns a reference to <code>this</code> for easy method chaining 1258 */ 1259 public ResearchStudy setIdentifier(List<Identifier> theIdentifier) { 1260 this.identifier = theIdentifier; 1261 return this; 1262 } 1263 1264 public boolean hasIdentifier() { 1265 if (this.identifier == null) 1266 return false; 1267 for (Identifier item : this.identifier) 1268 if (!item.isEmpty()) 1269 return true; 1270 return false; 1271 } 1272 1273 public Identifier addIdentifier() { // 3 1274 Identifier t = new Identifier(); 1275 if (this.identifier == null) 1276 this.identifier = new ArrayList<Identifier>(); 1277 this.identifier.add(t); 1278 return t; 1279 } 1280 1281 public ResearchStudy addIdentifier(Identifier t) { // 3 1282 if (t == null) 1283 return this; 1284 if (this.identifier == null) 1285 this.identifier = new ArrayList<Identifier>(); 1286 this.identifier.add(t); 1287 return this; 1288 } 1289 1290 /** 1291 * @return The first repetition of repeating field {@link #identifier}, creating 1292 * it if it does not already exist 1293 */ 1294 public Identifier getIdentifierFirstRep() { 1295 if (getIdentifier().isEmpty()) { 1296 addIdentifier(); 1297 } 1298 return getIdentifier().get(0); 1299 } 1300 1301 /** 1302 * @return {@link #title} (A short, descriptive user-friendly label for the 1303 * study.). This is the underlying object with id, value and extensions. 1304 * The accessor "getTitle" gives direct access to the value 1305 */ 1306 public StringType getTitleElement() { 1307 if (this.title == null) 1308 if (Configuration.errorOnAutoCreate()) 1309 throw new Error("Attempt to auto-create ResearchStudy.title"); 1310 else if (Configuration.doAutoCreate()) 1311 this.title = new StringType(); // bb 1312 return this.title; 1313 } 1314 1315 public boolean hasTitleElement() { 1316 return this.title != null && !this.title.isEmpty(); 1317 } 1318 1319 public boolean hasTitle() { 1320 return this.title != null && !this.title.isEmpty(); 1321 } 1322 1323 /** 1324 * @param value {@link #title} (A short, descriptive user-friendly label for the 1325 * study.). This is the underlying object with id, value and 1326 * extensions. The accessor "getTitle" gives direct access to the 1327 * value 1328 */ 1329 public ResearchStudy setTitleElement(StringType value) { 1330 this.title = value; 1331 return this; 1332 } 1333 1334 /** 1335 * @return A short, descriptive user-friendly label for the study. 1336 */ 1337 public String getTitle() { 1338 return this.title == null ? null : this.title.getValue(); 1339 } 1340 1341 /** 1342 * @param value A short, descriptive user-friendly label for the study. 1343 */ 1344 public ResearchStudy setTitle(String value) { 1345 if (Utilities.noString(value)) 1346 this.title = null; 1347 else { 1348 if (this.title == null) 1349 this.title = new StringType(); 1350 this.title.setValue(value); 1351 } 1352 return this; 1353 } 1354 1355 /** 1356 * @return {@link #protocol} (The set of steps expected to be performed as part 1357 * of the execution of the study.) 1358 */ 1359 public List<Reference> getProtocol() { 1360 if (this.protocol == null) 1361 this.protocol = new ArrayList<Reference>(); 1362 return this.protocol; 1363 } 1364 1365 /** 1366 * @return Returns a reference to <code>this</code> for easy method chaining 1367 */ 1368 public ResearchStudy setProtocol(List<Reference> theProtocol) { 1369 this.protocol = theProtocol; 1370 return this; 1371 } 1372 1373 public boolean hasProtocol() { 1374 if (this.protocol == null) 1375 return false; 1376 for (Reference item : this.protocol) 1377 if (!item.isEmpty()) 1378 return true; 1379 return false; 1380 } 1381 1382 public Reference addProtocol() { // 3 1383 Reference t = new Reference(); 1384 if (this.protocol == null) 1385 this.protocol = new ArrayList<Reference>(); 1386 this.protocol.add(t); 1387 return t; 1388 } 1389 1390 public ResearchStudy addProtocol(Reference t) { // 3 1391 if (t == null) 1392 return this; 1393 if (this.protocol == null) 1394 this.protocol = new ArrayList<Reference>(); 1395 this.protocol.add(t); 1396 return this; 1397 } 1398 1399 /** 1400 * @return The first repetition of repeating field {@link #protocol}, creating 1401 * it if it does not already exist 1402 */ 1403 public Reference getProtocolFirstRep() { 1404 if (getProtocol().isEmpty()) { 1405 addProtocol(); 1406 } 1407 return getProtocol().get(0); 1408 } 1409 1410 /** 1411 * @deprecated Use Reference#setResource(IBaseResource) instead 1412 */ 1413 @Deprecated 1414 public List<PlanDefinition> getProtocolTarget() { 1415 if (this.protocolTarget == null) 1416 this.protocolTarget = new ArrayList<PlanDefinition>(); 1417 return this.protocolTarget; 1418 } 1419 1420 /** 1421 * @deprecated Use Reference#setResource(IBaseResource) instead 1422 */ 1423 @Deprecated 1424 public PlanDefinition addProtocolTarget() { 1425 PlanDefinition r = new PlanDefinition(); 1426 if (this.protocolTarget == null) 1427 this.protocolTarget = new ArrayList<PlanDefinition>(); 1428 this.protocolTarget.add(r); 1429 return r; 1430 } 1431 1432 /** 1433 * @return {@link #partOf} (A larger research study of which this particular 1434 * study is a component or step.) 1435 */ 1436 public List<Reference> getPartOf() { 1437 if (this.partOf == null) 1438 this.partOf = new ArrayList<Reference>(); 1439 return this.partOf; 1440 } 1441 1442 /** 1443 * @return Returns a reference to <code>this</code> for easy method chaining 1444 */ 1445 public ResearchStudy setPartOf(List<Reference> thePartOf) { 1446 this.partOf = thePartOf; 1447 return this; 1448 } 1449 1450 public boolean hasPartOf() { 1451 if (this.partOf == null) 1452 return false; 1453 for (Reference item : this.partOf) 1454 if (!item.isEmpty()) 1455 return true; 1456 return false; 1457 } 1458 1459 public Reference addPartOf() { // 3 1460 Reference t = new Reference(); 1461 if (this.partOf == null) 1462 this.partOf = new ArrayList<Reference>(); 1463 this.partOf.add(t); 1464 return t; 1465 } 1466 1467 public ResearchStudy addPartOf(Reference t) { // 3 1468 if (t == null) 1469 return this; 1470 if (this.partOf == null) 1471 this.partOf = new ArrayList<Reference>(); 1472 this.partOf.add(t); 1473 return this; 1474 } 1475 1476 /** 1477 * @return The first repetition of repeating field {@link #partOf}, creating it 1478 * if it does not already exist 1479 */ 1480 public Reference getPartOfFirstRep() { 1481 if (getPartOf().isEmpty()) { 1482 addPartOf(); 1483 } 1484 return getPartOf().get(0); 1485 } 1486 1487 /** 1488 * @deprecated Use Reference#setResource(IBaseResource) instead 1489 */ 1490 @Deprecated 1491 public List<ResearchStudy> getPartOfTarget() { 1492 if (this.partOfTarget == null) 1493 this.partOfTarget = new ArrayList<ResearchStudy>(); 1494 return this.partOfTarget; 1495 } 1496 1497 /** 1498 * @deprecated Use Reference#setResource(IBaseResource) instead 1499 */ 1500 @Deprecated 1501 public ResearchStudy addPartOfTarget() { 1502 ResearchStudy r = new ResearchStudy(); 1503 if (this.partOfTarget == null) 1504 this.partOfTarget = new ArrayList<ResearchStudy>(); 1505 this.partOfTarget.add(r); 1506 return r; 1507 } 1508 1509 /** 1510 * @return {@link #status} (The current state of the study.). This is the 1511 * underlying object with id, value and extensions. The accessor 1512 * "getStatus" gives direct access to the value 1513 */ 1514 public Enumeration<ResearchStudyStatus> getStatusElement() { 1515 if (this.status == null) 1516 if (Configuration.errorOnAutoCreate()) 1517 throw new Error("Attempt to auto-create ResearchStudy.status"); 1518 else if (Configuration.doAutoCreate()) 1519 this.status = new Enumeration<ResearchStudyStatus>(new ResearchStudyStatusEnumFactory()); // bb 1520 return this.status; 1521 } 1522 1523 public boolean hasStatusElement() { 1524 return this.status != null && !this.status.isEmpty(); 1525 } 1526 1527 public boolean hasStatus() { 1528 return this.status != null && !this.status.isEmpty(); 1529 } 1530 1531 /** 1532 * @param value {@link #status} (The current state of the study.). This is the 1533 * underlying object with id, value and extensions. The accessor 1534 * "getStatus" gives direct access to the value 1535 */ 1536 public ResearchStudy setStatusElement(Enumeration<ResearchStudyStatus> value) { 1537 this.status = value; 1538 return this; 1539 } 1540 1541 /** 1542 * @return The current state of the study. 1543 */ 1544 public ResearchStudyStatus getStatus() { 1545 return this.status == null ? null : this.status.getValue(); 1546 } 1547 1548 /** 1549 * @param value The current state of the study. 1550 */ 1551 public ResearchStudy setStatus(ResearchStudyStatus value) { 1552 if (this.status == null) 1553 this.status = new Enumeration<ResearchStudyStatus>(new ResearchStudyStatusEnumFactory()); 1554 this.status.setValue(value); 1555 return this; 1556 } 1557 1558 /** 1559 * @return {@link #primaryPurposeType} (The type of study based upon the intent 1560 * of the study's activities. A classification of the intent of the 1561 * study.) 1562 */ 1563 public CodeableConcept getPrimaryPurposeType() { 1564 if (this.primaryPurposeType == null) 1565 if (Configuration.errorOnAutoCreate()) 1566 throw new Error("Attempt to auto-create ResearchStudy.primaryPurposeType"); 1567 else if (Configuration.doAutoCreate()) 1568 this.primaryPurposeType = new CodeableConcept(); // cc 1569 return this.primaryPurposeType; 1570 } 1571 1572 public boolean hasPrimaryPurposeType() { 1573 return this.primaryPurposeType != null && !this.primaryPurposeType.isEmpty(); 1574 } 1575 1576 /** 1577 * @param value {@link #primaryPurposeType} (The type of study based upon the 1578 * intent of the study's activities. A classification of the intent 1579 * of the study.) 1580 */ 1581 public ResearchStudy setPrimaryPurposeType(CodeableConcept value) { 1582 this.primaryPurposeType = value; 1583 return this; 1584 } 1585 1586 /** 1587 * @return {@link #phase} (The stage in the progression of a therapy from 1588 * initial experimental use in humans in clinical trials to post-market 1589 * evaluation.) 1590 */ 1591 public CodeableConcept getPhase() { 1592 if (this.phase == null) 1593 if (Configuration.errorOnAutoCreate()) 1594 throw new Error("Attempt to auto-create ResearchStudy.phase"); 1595 else if (Configuration.doAutoCreate()) 1596 this.phase = new CodeableConcept(); // cc 1597 return this.phase; 1598 } 1599 1600 public boolean hasPhase() { 1601 return this.phase != null && !this.phase.isEmpty(); 1602 } 1603 1604 /** 1605 * @param value {@link #phase} (The stage in the progression of a therapy from 1606 * initial experimental use in humans in clinical trials to 1607 * post-market evaluation.) 1608 */ 1609 public ResearchStudy setPhase(CodeableConcept value) { 1610 this.phase = value; 1611 return this; 1612 } 1613 1614 /** 1615 * @return {@link #category} (Codes categorizing the type of study such as 1616 * investigational vs. observational, type of blinding, type of 1617 * randomization, safety vs. efficacy, etc.) 1618 */ 1619 public List<CodeableConcept> getCategory() { 1620 if (this.category == null) 1621 this.category = new ArrayList<CodeableConcept>(); 1622 return this.category; 1623 } 1624 1625 /** 1626 * @return Returns a reference to <code>this</code> for easy method chaining 1627 */ 1628 public ResearchStudy setCategory(List<CodeableConcept> theCategory) { 1629 this.category = theCategory; 1630 return this; 1631 } 1632 1633 public boolean hasCategory() { 1634 if (this.category == null) 1635 return false; 1636 for (CodeableConcept item : this.category) 1637 if (!item.isEmpty()) 1638 return true; 1639 return false; 1640 } 1641 1642 public CodeableConcept addCategory() { // 3 1643 CodeableConcept t = new CodeableConcept(); 1644 if (this.category == null) 1645 this.category = new ArrayList<CodeableConcept>(); 1646 this.category.add(t); 1647 return t; 1648 } 1649 1650 public ResearchStudy addCategory(CodeableConcept t) { // 3 1651 if (t == null) 1652 return this; 1653 if (this.category == null) 1654 this.category = new ArrayList<CodeableConcept>(); 1655 this.category.add(t); 1656 return this; 1657 } 1658 1659 /** 1660 * @return The first repetition of repeating field {@link #category}, creating 1661 * it if it does not already exist 1662 */ 1663 public CodeableConcept getCategoryFirstRep() { 1664 if (getCategory().isEmpty()) { 1665 addCategory(); 1666 } 1667 return getCategory().get(0); 1668 } 1669 1670 /** 1671 * @return {@link #focus} (The medication(s), food(s), therapy(ies), device(s) 1672 * or other concerns or interventions that the study is seeking to gain 1673 * more information about.) 1674 */ 1675 public List<CodeableConcept> getFocus() { 1676 if (this.focus == null) 1677 this.focus = new ArrayList<CodeableConcept>(); 1678 return this.focus; 1679 } 1680 1681 /** 1682 * @return Returns a reference to <code>this</code> for easy method chaining 1683 */ 1684 public ResearchStudy setFocus(List<CodeableConcept> theFocus) { 1685 this.focus = theFocus; 1686 return this; 1687 } 1688 1689 public boolean hasFocus() { 1690 if (this.focus == null) 1691 return false; 1692 for (CodeableConcept item : this.focus) 1693 if (!item.isEmpty()) 1694 return true; 1695 return false; 1696 } 1697 1698 public CodeableConcept addFocus() { // 3 1699 CodeableConcept t = new CodeableConcept(); 1700 if (this.focus == null) 1701 this.focus = new ArrayList<CodeableConcept>(); 1702 this.focus.add(t); 1703 return t; 1704 } 1705 1706 public ResearchStudy addFocus(CodeableConcept t) { // 3 1707 if (t == null) 1708 return this; 1709 if (this.focus == null) 1710 this.focus = new ArrayList<CodeableConcept>(); 1711 this.focus.add(t); 1712 return this; 1713 } 1714 1715 /** 1716 * @return The first repetition of repeating field {@link #focus}, creating it 1717 * if it does not already exist 1718 */ 1719 public CodeableConcept getFocusFirstRep() { 1720 if (getFocus().isEmpty()) { 1721 addFocus(); 1722 } 1723 return getFocus().get(0); 1724 } 1725 1726 /** 1727 * @return {@link #condition} (The condition that is the focus of the study. For 1728 * example, In a study to examine risk factors for Lupus, might have as 1729 * an inclusion criterion "healthy volunteer", but the target condition 1730 * code would be a Lupus SNOMED code.) 1731 */ 1732 public List<CodeableConcept> getCondition() { 1733 if (this.condition == null) 1734 this.condition = new ArrayList<CodeableConcept>(); 1735 return this.condition; 1736 } 1737 1738 /** 1739 * @return Returns a reference to <code>this</code> for easy method chaining 1740 */ 1741 public ResearchStudy setCondition(List<CodeableConcept> theCondition) { 1742 this.condition = theCondition; 1743 return this; 1744 } 1745 1746 public boolean hasCondition() { 1747 if (this.condition == null) 1748 return false; 1749 for (CodeableConcept item : this.condition) 1750 if (!item.isEmpty()) 1751 return true; 1752 return false; 1753 } 1754 1755 public CodeableConcept addCondition() { // 3 1756 CodeableConcept t = new CodeableConcept(); 1757 if (this.condition == null) 1758 this.condition = new ArrayList<CodeableConcept>(); 1759 this.condition.add(t); 1760 return t; 1761 } 1762 1763 public ResearchStudy addCondition(CodeableConcept t) { // 3 1764 if (t == null) 1765 return this; 1766 if (this.condition == null) 1767 this.condition = new ArrayList<CodeableConcept>(); 1768 this.condition.add(t); 1769 return this; 1770 } 1771 1772 /** 1773 * @return The first repetition of repeating field {@link #condition}, creating 1774 * it if it does not already exist 1775 */ 1776 public CodeableConcept getConditionFirstRep() { 1777 if (getCondition().isEmpty()) { 1778 addCondition(); 1779 } 1780 return getCondition().get(0); 1781 } 1782 1783 /** 1784 * @return {@link #contact} (Contact details to assist a user in learning more 1785 * about or engaging with the study.) 1786 */ 1787 public List<ContactDetail> getContact() { 1788 if (this.contact == null) 1789 this.contact = new ArrayList<ContactDetail>(); 1790 return this.contact; 1791 } 1792 1793 /** 1794 * @return Returns a reference to <code>this</code> for easy method chaining 1795 */ 1796 public ResearchStudy setContact(List<ContactDetail> theContact) { 1797 this.contact = theContact; 1798 return this; 1799 } 1800 1801 public boolean hasContact() { 1802 if (this.contact == null) 1803 return false; 1804 for (ContactDetail item : this.contact) 1805 if (!item.isEmpty()) 1806 return true; 1807 return false; 1808 } 1809 1810 public ContactDetail addContact() { // 3 1811 ContactDetail t = new ContactDetail(); 1812 if (this.contact == null) 1813 this.contact = new ArrayList<ContactDetail>(); 1814 this.contact.add(t); 1815 return t; 1816 } 1817 1818 public ResearchStudy addContact(ContactDetail t) { // 3 1819 if (t == null) 1820 return this; 1821 if (this.contact == null) 1822 this.contact = new ArrayList<ContactDetail>(); 1823 this.contact.add(t); 1824 return this; 1825 } 1826 1827 /** 1828 * @return The first repetition of repeating field {@link #contact}, creating it 1829 * if it does not already exist 1830 */ 1831 public ContactDetail getContactFirstRep() { 1832 if (getContact().isEmpty()) { 1833 addContact(); 1834 } 1835 return getContact().get(0); 1836 } 1837 1838 /** 1839 * @return {@link #relatedArtifact} (Citations, references and other related 1840 * documents.) 1841 */ 1842 public List<RelatedArtifact> getRelatedArtifact() { 1843 if (this.relatedArtifact == null) 1844 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1845 return this.relatedArtifact; 1846 } 1847 1848 /** 1849 * @return Returns a reference to <code>this</code> for easy method chaining 1850 */ 1851 public ResearchStudy setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1852 this.relatedArtifact = theRelatedArtifact; 1853 return this; 1854 } 1855 1856 public boolean hasRelatedArtifact() { 1857 if (this.relatedArtifact == null) 1858 return false; 1859 for (RelatedArtifact item : this.relatedArtifact) 1860 if (!item.isEmpty()) 1861 return true; 1862 return false; 1863 } 1864 1865 public RelatedArtifact addRelatedArtifact() { // 3 1866 RelatedArtifact t = new RelatedArtifact(); 1867 if (this.relatedArtifact == null) 1868 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1869 this.relatedArtifact.add(t); 1870 return t; 1871 } 1872 1873 public ResearchStudy addRelatedArtifact(RelatedArtifact t) { // 3 1874 if (t == null) 1875 return this; 1876 if (this.relatedArtifact == null) 1877 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1878 this.relatedArtifact.add(t); 1879 return this; 1880 } 1881 1882 /** 1883 * @return The first repetition of repeating field {@link #relatedArtifact}, 1884 * creating it if it does not already exist 1885 */ 1886 public RelatedArtifact getRelatedArtifactFirstRep() { 1887 if (getRelatedArtifact().isEmpty()) { 1888 addRelatedArtifact(); 1889 } 1890 return getRelatedArtifact().get(0); 1891 } 1892 1893 /** 1894 * @return {@link #keyword} (Key terms to aid in searching for or filtering the 1895 * study.) 1896 */ 1897 public List<CodeableConcept> getKeyword() { 1898 if (this.keyword == null) 1899 this.keyword = new ArrayList<CodeableConcept>(); 1900 return this.keyword; 1901 } 1902 1903 /** 1904 * @return Returns a reference to <code>this</code> for easy method chaining 1905 */ 1906 public ResearchStudy setKeyword(List<CodeableConcept> theKeyword) { 1907 this.keyword = theKeyword; 1908 return this; 1909 } 1910 1911 public boolean hasKeyword() { 1912 if (this.keyword == null) 1913 return false; 1914 for (CodeableConcept item : this.keyword) 1915 if (!item.isEmpty()) 1916 return true; 1917 return false; 1918 } 1919 1920 public CodeableConcept addKeyword() { // 3 1921 CodeableConcept t = new CodeableConcept(); 1922 if (this.keyword == null) 1923 this.keyword = new ArrayList<CodeableConcept>(); 1924 this.keyword.add(t); 1925 return t; 1926 } 1927 1928 public ResearchStudy addKeyword(CodeableConcept t) { // 3 1929 if (t == null) 1930 return this; 1931 if (this.keyword == null) 1932 this.keyword = new ArrayList<CodeableConcept>(); 1933 this.keyword.add(t); 1934 return this; 1935 } 1936 1937 /** 1938 * @return The first repetition of repeating field {@link #keyword}, creating it 1939 * if it does not already exist 1940 */ 1941 public CodeableConcept getKeywordFirstRep() { 1942 if (getKeyword().isEmpty()) { 1943 addKeyword(); 1944 } 1945 return getKeyword().get(0); 1946 } 1947 1948 /** 1949 * @return {@link #location} (Indicates a country, state or other region where 1950 * the study is taking place.) 1951 */ 1952 public List<CodeableConcept> getLocation() { 1953 if (this.location == null) 1954 this.location = new ArrayList<CodeableConcept>(); 1955 return this.location; 1956 } 1957 1958 /** 1959 * @return Returns a reference to <code>this</code> for easy method chaining 1960 */ 1961 public ResearchStudy setLocation(List<CodeableConcept> theLocation) { 1962 this.location = theLocation; 1963 return this; 1964 } 1965 1966 public boolean hasLocation() { 1967 if (this.location == null) 1968 return false; 1969 for (CodeableConcept item : this.location) 1970 if (!item.isEmpty()) 1971 return true; 1972 return false; 1973 } 1974 1975 public CodeableConcept addLocation() { // 3 1976 CodeableConcept t = new CodeableConcept(); 1977 if (this.location == null) 1978 this.location = new ArrayList<CodeableConcept>(); 1979 this.location.add(t); 1980 return t; 1981 } 1982 1983 public ResearchStudy addLocation(CodeableConcept t) { // 3 1984 if (t == null) 1985 return this; 1986 if (this.location == null) 1987 this.location = new ArrayList<CodeableConcept>(); 1988 this.location.add(t); 1989 return this; 1990 } 1991 1992 /** 1993 * @return The first repetition of repeating field {@link #location}, creating 1994 * it if it does not already exist 1995 */ 1996 public CodeableConcept getLocationFirstRep() { 1997 if (getLocation().isEmpty()) { 1998 addLocation(); 1999 } 2000 return getLocation().get(0); 2001 } 2002 2003 /** 2004 * @return {@link #description} (A full description of how the study is being 2005 * conducted.). This is the underlying object with id, value and 2006 * extensions. The accessor "getDescription" gives direct access to the 2007 * value 2008 */ 2009 public MarkdownType getDescriptionElement() { 2010 if (this.description == null) 2011 if (Configuration.errorOnAutoCreate()) 2012 throw new Error("Attempt to auto-create ResearchStudy.description"); 2013 else if (Configuration.doAutoCreate()) 2014 this.description = new MarkdownType(); // bb 2015 return this.description; 2016 } 2017 2018 public boolean hasDescriptionElement() { 2019 return this.description != null && !this.description.isEmpty(); 2020 } 2021 2022 public boolean hasDescription() { 2023 return this.description != null && !this.description.isEmpty(); 2024 } 2025 2026 /** 2027 * @param value {@link #description} (A full description of how the study is 2028 * being conducted.). This is the underlying object with id, value 2029 * and extensions. The accessor "getDescription" gives direct 2030 * access to the value 2031 */ 2032 public ResearchStudy setDescriptionElement(MarkdownType value) { 2033 this.description = value; 2034 return this; 2035 } 2036 2037 /** 2038 * @return A full description of how the study is being conducted. 2039 */ 2040 public String getDescription() { 2041 return this.description == null ? null : this.description.getValue(); 2042 } 2043 2044 /** 2045 * @param value A full description of how the study is being conducted. 2046 */ 2047 public ResearchStudy setDescription(String value) { 2048 if (value == null) 2049 this.description = null; 2050 else { 2051 if (this.description == null) 2052 this.description = new MarkdownType(); 2053 this.description.setValue(value); 2054 } 2055 return this; 2056 } 2057 2058 /** 2059 * @return {@link #enrollment} (Reference to a Group that defines the criteria 2060 * for and quantity of subjects participating in the study. E.g. " 200 2061 * female Europeans between the ages of 20 and 45 with early onset 2062 * diabetes".) 2063 */ 2064 public List<Reference> getEnrollment() { 2065 if (this.enrollment == null) 2066 this.enrollment = new ArrayList<Reference>(); 2067 return this.enrollment; 2068 } 2069 2070 /** 2071 * @return Returns a reference to <code>this</code> for easy method chaining 2072 */ 2073 public ResearchStudy setEnrollment(List<Reference> theEnrollment) { 2074 this.enrollment = theEnrollment; 2075 return this; 2076 } 2077 2078 public boolean hasEnrollment() { 2079 if (this.enrollment == null) 2080 return false; 2081 for (Reference item : this.enrollment) 2082 if (!item.isEmpty()) 2083 return true; 2084 return false; 2085 } 2086 2087 public Reference addEnrollment() { // 3 2088 Reference t = new Reference(); 2089 if (this.enrollment == null) 2090 this.enrollment = new ArrayList<Reference>(); 2091 this.enrollment.add(t); 2092 return t; 2093 } 2094 2095 public ResearchStudy addEnrollment(Reference t) { // 3 2096 if (t == null) 2097 return this; 2098 if (this.enrollment == null) 2099 this.enrollment = new ArrayList<Reference>(); 2100 this.enrollment.add(t); 2101 return this; 2102 } 2103 2104 /** 2105 * @return The first repetition of repeating field {@link #enrollment}, creating 2106 * it if it does not already exist 2107 */ 2108 public Reference getEnrollmentFirstRep() { 2109 if (getEnrollment().isEmpty()) { 2110 addEnrollment(); 2111 } 2112 return getEnrollment().get(0); 2113 } 2114 2115 /** 2116 * @deprecated Use Reference#setResource(IBaseResource) instead 2117 */ 2118 @Deprecated 2119 public List<Group> getEnrollmentTarget() { 2120 if (this.enrollmentTarget == null) 2121 this.enrollmentTarget = new ArrayList<Group>(); 2122 return this.enrollmentTarget; 2123 } 2124 2125 /** 2126 * @deprecated Use Reference#setResource(IBaseResource) instead 2127 */ 2128 @Deprecated 2129 public Group addEnrollmentTarget() { 2130 Group r = new Group(); 2131 if (this.enrollmentTarget == null) 2132 this.enrollmentTarget = new ArrayList<Group>(); 2133 this.enrollmentTarget.add(r); 2134 return r; 2135 } 2136 2137 /** 2138 * @return {@link #period} (Identifies the start date and the expected (or 2139 * actual, depending on status) end date for the study.) 2140 */ 2141 public Period getPeriod() { 2142 if (this.period == null) 2143 if (Configuration.errorOnAutoCreate()) 2144 throw new Error("Attempt to auto-create ResearchStudy.period"); 2145 else if (Configuration.doAutoCreate()) 2146 this.period = new Period(); // cc 2147 return this.period; 2148 } 2149 2150 public boolean hasPeriod() { 2151 return this.period != null && !this.period.isEmpty(); 2152 } 2153 2154 /** 2155 * @param value {@link #period} (Identifies the start date and the expected (or 2156 * actual, depending on status) end date for the study.) 2157 */ 2158 public ResearchStudy setPeriod(Period value) { 2159 this.period = value; 2160 return this; 2161 } 2162 2163 /** 2164 * @return {@link #sponsor} (An organization that initiates the investigation 2165 * and is legally responsible for the study.) 2166 */ 2167 public Reference getSponsor() { 2168 if (this.sponsor == null) 2169 if (Configuration.errorOnAutoCreate()) 2170 throw new Error("Attempt to auto-create ResearchStudy.sponsor"); 2171 else if (Configuration.doAutoCreate()) 2172 this.sponsor = new Reference(); // cc 2173 return this.sponsor; 2174 } 2175 2176 public boolean hasSponsor() { 2177 return this.sponsor != null && !this.sponsor.isEmpty(); 2178 } 2179 2180 /** 2181 * @param value {@link #sponsor} (An organization that initiates the 2182 * investigation and is legally responsible for the study.) 2183 */ 2184 public ResearchStudy setSponsor(Reference value) { 2185 this.sponsor = value; 2186 return this; 2187 } 2188 2189 /** 2190 * @return {@link #sponsor} The actual object that is the target of the 2191 * reference. The reference library doesn't populate this, but you can 2192 * use it to hold the resource if you resolve it. (An organization that 2193 * initiates the investigation and is legally responsible for the 2194 * study.) 2195 */ 2196 public Organization getSponsorTarget() { 2197 if (this.sponsorTarget == null) 2198 if (Configuration.errorOnAutoCreate()) 2199 throw new Error("Attempt to auto-create ResearchStudy.sponsor"); 2200 else if (Configuration.doAutoCreate()) 2201 this.sponsorTarget = new Organization(); // aa 2202 return this.sponsorTarget; 2203 } 2204 2205 /** 2206 * @param value {@link #sponsor} The actual object that is the target of the 2207 * reference. The reference library doesn't use these, but you can 2208 * use it to hold the resource if you resolve it. (An organization 2209 * that initiates the investigation and is legally responsible for 2210 * the study.) 2211 */ 2212 public ResearchStudy setSponsorTarget(Organization value) { 2213 this.sponsorTarget = value; 2214 return this; 2215 } 2216 2217 /** 2218 * @return {@link #principalInvestigator} (A researcher in a study who oversees 2219 * multiple aspects of the study, such as concept development, protocol 2220 * writing, protocol submission for IRB approval, participant 2221 * recruitment, informed consent, data collection, analysis, 2222 * interpretation and presentation.) 2223 */ 2224 public Reference getPrincipalInvestigator() { 2225 if (this.principalInvestigator == null) 2226 if (Configuration.errorOnAutoCreate()) 2227 throw new Error("Attempt to auto-create ResearchStudy.principalInvestigator"); 2228 else if (Configuration.doAutoCreate()) 2229 this.principalInvestigator = new Reference(); // cc 2230 return this.principalInvestigator; 2231 } 2232 2233 public boolean hasPrincipalInvestigator() { 2234 return this.principalInvestigator != null && !this.principalInvestigator.isEmpty(); 2235 } 2236 2237 /** 2238 * @param value {@link #principalInvestigator} (A researcher in a study who 2239 * oversees multiple aspects of the study, such as concept 2240 * development, protocol writing, protocol submission for IRB 2241 * approval, participant recruitment, informed consent, data 2242 * collection, analysis, interpretation and presentation.) 2243 */ 2244 public ResearchStudy setPrincipalInvestigator(Reference value) { 2245 this.principalInvestigator = value; 2246 return this; 2247 } 2248 2249 /** 2250 * @return {@link #principalInvestigator} The actual object that is the target 2251 * of the reference. The reference library doesn't populate this, but 2252 * you can use it to hold the resource if you resolve it. (A researcher 2253 * in a study who oversees multiple aspects of the study, such as 2254 * concept development, protocol writing, protocol submission for IRB 2255 * approval, participant recruitment, informed consent, data collection, 2256 * analysis, interpretation and presentation.) 2257 */ 2258 public Resource getPrincipalInvestigatorTarget() { 2259 return this.principalInvestigatorTarget; 2260 } 2261 2262 /** 2263 * @param value {@link #principalInvestigator} The actual object that is the 2264 * target of the reference. The reference library doesn't use 2265 * these, but you can use it to hold the resource if you resolve 2266 * it. (A researcher in a study who oversees multiple aspects of 2267 * the study, such as concept development, protocol writing, 2268 * protocol submission for IRB approval, participant recruitment, 2269 * informed consent, data collection, analysis, interpretation and 2270 * presentation.) 2271 */ 2272 public ResearchStudy setPrincipalInvestigatorTarget(Resource value) { 2273 this.principalInvestigatorTarget = value; 2274 return this; 2275 } 2276 2277 /** 2278 * @return {@link #site} (A facility in which study activities are conducted.) 2279 */ 2280 public List<Reference> getSite() { 2281 if (this.site == null) 2282 this.site = new ArrayList<Reference>(); 2283 return this.site; 2284 } 2285 2286 /** 2287 * @return Returns a reference to <code>this</code> for easy method chaining 2288 */ 2289 public ResearchStudy setSite(List<Reference> theSite) { 2290 this.site = theSite; 2291 return this; 2292 } 2293 2294 public boolean hasSite() { 2295 if (this.site == null) 2296 return false; 2297 for (Reference item : this.site) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 public Reference addSite() { // 3 2304 Reference t = new Reference(); 2305 if (this.site == null) 2306 this.site = new ArrayList<Reference>(); 2307 this.site.add(t); 2308 return t; 2309 } 2310 2311 public ResearchStudy addSite(Reference t) { // 3 2312 if (t == null) 2313 return this; 2314 if (this.site == null) 2315 this.site = new ArrayList<Reference>(); 2316 this.site.add(t); 2317 return this; 2318 } 2319 2320 /** 2321 * @return The first repetition of repeating field {@link #site}, creating it if 2322 * it does not already exist 2323 */ 2324 public Reference getSiteFirstRep() { 2325 if (getSite().isEmpty()) { 2326 addSite(); 2327 } 2328 return getSite().get(0); 2329 } 2330 2331 /** 2332 * @deprecated Use Reference#setResource(IBaseResource) instead 2333 */ 2334 @Deprecated 2335 public List<Location> getSiteTarget() { 2336 if (this.siteTarget == null) 2337 this.siteTarget = new ArrayList<Location>(); 2338 return this.siteTarget; 2339 } 2340 2341 /** 2342 * @deprecated Use Reference#setResource(IBaseResource) instead 2343 */ 2344 @Deprecated 2345 public Location addSiteTarget() { 2346 Location r = new Location(); 2347 if (this.siteTarget == null) 2348 this.siteTarget = new ArrayList<Location>(); 2349 this.siteTarget.add(r); 2350 return r; 2351 } 2352 2353 /** 2354 * @return {@link #reasonStopped} (A description and/or code explaining the 2355 * premature termination of the study.) 2356 */ 2357 public CodeableConcept getReasonStopped() { 2358 if (this.reasonStopped == null) 2359 if (Configuration.errorOnAutoCreate()) 2360 throw new Error("Attempt to auto-create ResearchStudy.reasonStopped"); 2361 else if (Configuration.doAutoCreate()) 2362 this.reasonStopped = new CodeableConcept(); // cc 2363 return this.reasonStopped; 2364 } 2365 2366 public boolean hasReasonStopped() { 2367 return this.reasonStopped != null && !this.reasonStopped.isEmpty(); 2368 } 2369 2370 /** 2371 * @param value {@link #reasonStopped} (A description and/or code explaining the 2372 * premature termination of the study.) 2373 */ 2374 public ResearchStudy setReasonStopped(CodeableConcept value) { 2375 this.reasonStopped = value; 2376 return this; 2377 } 2378 2379 /** 2380 * @return {@link #note} (Comments made about the study by the performer, 2381 * subject or other participants.) 2382 */ 2383 public List<Annotation> getNote() { 2384 if (this.note == null) 2385 this.note = new ArrayList<Annotation>(); 2386 return this.note; 2387 } 2388 2389 /** 2390 * @return Returns a reference to <code>this</code> for easy method chaining 2391 */ 2392 public ResearchStudy setNote(List<Annotation> theNote) { 2393 this.note = theNote; 2394 return this; 2395 } 2396 2397 public boolean hasNote() { 2398 if (this.note == null) 2399 return false; 2400 for (Annotation item : this.note) 2401 if (!item.isEmpty()) 2402 return true; 2403 return false; 2404 } 2405 2406 public Annotation addNote() { // 3 2407 Annotation t = new Annotation(); 2408 if (this.note == null) 2409 this.note = new ArrayList<Annotation>(); 2410 this.note.add(t); 2411 return t; 2412 } 2413 2414 public ResearchStudy addNote(Annotation t) { // 3 2415 if (t == null) 2416 return this; 2417 if (this.note == null) 2418 this.note = new ArrayList<Annotation>(); 2419 this.note.add(t); 2420 return this; 2421 } 2422 2423 /** 2424 * @return The first repetition of repeating field {@link #note}, creating it if 2425 * it does not already exist 2426 */ 2427 public Annotation getNoteFirstRep() { 2428 if (getNote().isEmpty()) { 2429 addNote(); 2430 } 2431 return getNote().get(0); 2432 } 2433 2434 /** 2435 * @return {@link #arm} (Describes an expected sequence of events for one of the 2436 * participants of a study. E.g. Exposure to drug A, wash-out, exposure 2437 * to drug B, wash-out, follow-up.) 2438 */ 2439 public List<ResearchStudyArmComponent> getArm() { 2440 if (this.arm == null) 2441 this.arm = new ArrayList<ResearchStudyArmComponent>(); 2442 return this.arm; 2443 } 2444 2445 /** 2446 * @return Returns a reference to <code>this</code> for easy method chaining 2447 */ 2448 public ResearchStudy setArm(List<ResearchStudyArmComponent> theArm) { 2449 this.arm = theArm; 2450 return this; 2451 } 2452 2453 public boolean hasArm() { 2454 if (this.arm == null) 2455 return false; 2456 for (ResearchStudyArmComponent item : this.arm) 2457 if (!item.isEmpty()) 2458 return true; 2459 return false; 2460 } 2461 2462 public ResearchStudyArmComponent addArm() { // 3 2463 ResearchStudyArmComponent t = new ResearchStudyArmComponent(); 2464 if (this.arm == null) 2465 this.arm = new ArrayList<ResearchStudyArmComponent>(); 2466 this.arm.add(t); 2467 return t; 2468 } 2469 2470 public ResearchStudy addArm(ResearchStudyArmComponent t) { // 3 2471 if (t == null) 2472 return this; 2473 if (this.arm == null) 2474 this.arm = new ArrayList<ResearchStudyArmComponent>(); 2475 this.arm.add(t); 2476 return this; 2477 } 2478 2479 /** 2480 * @return The first repetition of repeating field {@link #arm}, creating it if 2481 * it does not already exist 2482 */ 2483 public ResearchStudyArmComponent getArmFirstRep() { 2484 if (getArm().isEmpty()) { 2485 addArm(); 2486 } 2487 return getArm().get(0); 2488 } 2489 2490 /** 2491 * @return {@link #objective} (A goal that the study is aiming to achieve in 2492 * terms of a scientific question to be answered by the analysis of data 2493 * collected during the study.) 2494 */ 2495 public List<ResearchStudyObjectiveComponent> getObjective() { 2496 if (this.objective == null) 2497 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 2498 return this.objective; 2499 } 2500 2501 /** 2502 * @return Returns a reference to <code>this</code> for easy method chaining 2503 */ 2504 public ResearchStudy setObjective(List<ResearchStudyObjectiveComponent> theObjective) { 2505 this.objective = theObjective; 2506 return this; 2507 } 2508 2509 public boolean hasObjective() { 2510 if (this.objective == null) 2511 return false; 2512 for (ResearchStudyObjectiveComponent item : this.objective) 2513 if (!item.isEmpty()) 2514 return true; 2515 return false; 2516 } 2517 2518 public ResearchStudyObjectiveComponent addObjective() { // 3 2519 ResearchStudyObjectiveComponent t = new ResearchStudyObjectiveComponent(); 2520 if (this.objective == null) 2521 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 2522 this.objective.add(t); 2523 return t; 2524 } 2525 2526 public ResearchStudy addObjective(ResearchStudyObjectiveComponent t) { // 3 2527 if (t == null) 2528 return this; 2529 if (this.objective == null) 2530 this.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 2531 this.objective.add(t); 2532 return this; 2533 } 2534 2535 /** 2536 * @return The first repetition of repeating field {@link #objective}, creating 2537 * it if it does not already exist 2538 */ 2539 public ResearchStudyObjectiveComponent getObjectiveFirstRep() { 2540 if (getObjective().isEmpty()) { 2541 addObjective(); 2542 } 2543 return getObjective().get(0); 2544 } 2545 2546 protected void listChildren(List<Property> children) { 2547 super.listChildren(children); 2548 children.add(new Property("identifier", "Identifier", 2549 "Identifiers assigned to this research study by the sponsor or other systems.", 0, java.lang.Integer.MAX_VALUE, 2550 identifier)); 2551 children 2552 .add(new Property("title", "string", "A short, descriptive user-friendly label for the study.", 0, 1, title)); 2553 children.add(new Property("protocol", "Reference(PlanDefinition)", 2554 "The set of steps expected to be performed as part of the execution of the study.", 0, 2555 java.lang.Integer.MAX_VALUE, protocol)); 2556 children.add(new Property("partOf", "Reference(ResearchStudy)", 2557 "A larger research study of which this particular study is a component or step.", 0, 2558 java.lang.Integer.MAX_VALUE, partOf)); 2559 children.add(new Property("status", "code", "The current state of the study.", 0, 1, status)); 2560 children.add(new Property("primaryPurposeType", "CodeableConcept", 2561 "The type of study based upon the intent of the study's activities. A classification of the intent of the study.", 2562 0, 1, primaryPurposeType)); 2563 children.add(new Property("phase", "CodeableConcept", 2564 "The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.", 2565 0, 1, phase)); 2566 children.add(new Property("category", "CodeableConcept", 2567 "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.", 2568 0, java.lang.Integer.MAX_VALUE, category)); 2569 children.add(new Property("focus", "CodeableConcept", 2570 "The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.", 2571 0, java.lang.Integer.MAX_VALUE, focus)); 2572 children.add(new Property("condition", "CodeableConcept", 2573 "The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code.", 2574 0, java.lang.Integer.MAX_VALUE, condition)); 2575 children.add(new Property("contact", "ContactDetail", 2576 "Contact details to assist a user in learning more about or engaging with the study.", 0, 2577 java.lang.Integer.MAX_VALUE, contact)); 2578 children.add(new Property("relatedArtifact", "RelatedArtifact", 2579 "Citations, references and other related documents.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 2580 children.add(new Property("keyword", "CodeableConcept", "Key terms to aid in searching for or filtering the study.", 2581 0, java.lang.Integer.MAX_VALUE, keyword)); 2582 children.add(new Property("location", "CodeableConcept", 2583 "Indicates a country, state or other region where the study is taking place.", 0, java.lang.Integer.MAX_VALUE, 2584 location)); 2585 children.add(new Property("description", "markdown", "A full description of how the study is being conducted.", 0, 2586 1, description)); 2587 children.add(new Property("enrollment", "Reference(Group)", 2588 "Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. \" 200 female Europeans between the ages of 20 and 45 with early onset diabetes\".", 2589 0, java.lang.Integer.MAX_VALUE, enrollment)); 2590 children.add(new Property("period", "Period", 2591 "Identifies the start date and the expected (or actual, depending on status) end date for the study.", 0, 1, 2592 period)); 2593 children.add(new Property("sponsor", "Reference(Organization)", 2594 "An organization that initiates the investigation and is legally responsible for the study.", 0, 1, sponsor)); 2595 children.add(new Property("principalInvestigator", "Reference(Practitioner|PractitionerRole)", 2596 "A researcher in a study who oversees multiple aspects of the study, such as concept development, protocol writing, protocol submission for IRB approval, participant recruitment, informed consent, data collection, analysis, interpretation and presentation.", 2597 0, 1, principalInvestigator)); 2598 children.add(new Property("site", "Reference(Location)", "A facility in which study activities are conducted.", 0, 2599 java.lang.Integer.MAX_VALUE, site)); 2600 children.add(new Property("reasonStopped", "CodeableConcept", 2601 "A description and/or code explaining the premature termination of the study.", 0, 1, reasonStopped)); 2602 children.add(new Property("note", "Annotation", 2603 "Comments made about the study by the performer, subject or other participants.", 0, 2604 java.lang.Integer.MAX_VALUE, note)); 2605 children.add(new Property("arm", "", 2606 "Describes an expected sequence of events for one of the participants of a study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up.", 2607 0, java.lang.Integer.MAX_VALUE, arm)); 2608 children.add(new Property("objective", "", 2609 "A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.", 2610 0, java.lang.Integer.MAX_VALUE, objective)); 2611 } 2612 2613 @Override 2614 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2615 switch (_hash) { 2616 case -1618432855: 2617 /* identifier */ return new Property("identifier", "Identifier", 2618 "Identifiers assigned to this research study by the sponsor or other systems.", 0, 2619 java.lang.Integer.MAX_VALUE, identifier); 2620 case 110371416: 2621 /* title */ return new Property("title", "string", "A short, descriptive user-friendly label for the study.", 0, 2622 1, title); 2623 case -989163880: 2624 /* protocol */ return new Property("protocol", "Reference(PlanDefinition)", 2625 "The set of steps expected to be performed as part of the execution of the study.", 0, 2626 java.lang.Integer.MAX_VALUE, protocol); 2627 case -995410646: 2628 /* partOf */ return new Property("partOf", "Reference(ResearchStudy)", 2629 "A larger research study of which this particular study is a component or step.", 0, 2630 java.lang.Integer.MAX_VALUE, partOf); 2631 case -892481550: 2632 /* status */ return new Property("status", "code", "The current state of the study.", 0, 1, status); 2633 case -2132842986: 2634 /* primaryPurposeType */ return new Property("primaryPurposeType", "CodeableConcept", 2635 "The type of study based upon the intent of the study's activities. A classification of the intent of the study.", 2636 0, 1, primaryPurposeType); 2637 case 106629499: 2638 /* phase */ return new Property("phase", "CodeableConcept", 2639 "The stage in the progression of a therapy from initial experimental use in humans in clinical trials to post-market evaluation.", 2640 0, 1, phase); 2641 case 50511102: 2642 /* category */ return new Property("category", "CodeableConcept", 2643 "Codes categorizing the type of study such as investigational vs. observational, type of blinding, type of randomization, safety vs. efficacy, etc.", 2644 0, java.lang.Integer.MAX_VALUE, category); 2645 case 97604824: 2646 /* focus */ return new Property("focus", "CodeableConcept", 2647 "The medication(s), food(s), therapy(ies), device(s) or other concerns or interventions that the study is seeking to gain more information about.", 2648 0, java.lang.Integer.MAX_VALUE, focus); 2649 case -861311717: 2650 /* condition */ return new Property("condition", "CodeableConcept", 2651 "The condition that is the focus of the study. For example, In a study to examine risk factors for Lupus, might have as an inclusion criterion \"healthy volunteer\", but the target condition code would be a Lupus SNOMED code.", 2652 0, java.lang.Integer.MAX_VALUE, condition); 2653 case 951526432: 2654 /* contact */ return new Property("contact", "ContactDetail", 2655 "Contact details to assist a user in learning more about or engaging with the study.", 0, 2656 java.lang.Integer.MAX_VALUE, contact); 2657 case 666807069: 2658 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 2659 "Citations, references and other related documents.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 2660 case -814408215: 2661 /* keyword */ return new Property("keyword", "CodeableConcept", 2662 "Key terms to aid in searching for or filtering the study.", 0, java.lang.Integer.MAX_VALUE, keyword); 2663 case 1901043637: 2664 /* location */ return new Property("location", "CodeableConcept", 2665 "Indicates a country, state or other region where the study is taking place.", 0, java.lang.Integer.MAX_VALUE, 2666 location); 2667 case -1724546052: 2668 /* description */ return new Property("description", "markdown", 2669 "A full description of how the study is being conducted.", 0, 1, description); 2670 case 116089604: 2671 /* enrollment */ return new Property("enrollment", "Reference(Group)", 2672 "Reference to a Group that defines the criteria for and quantity of subjects participating in the study. E.g. \" 200 female Europeans between the ages of 20 and 45 with early onset diabetes\".", 2673 0, java.lang.Integer.MAX_VALUE, enrollment); 2674 case -991726143: 2675 /* period */ return new Property("period", "Period", 2676 "Identifies the start date and the expected (or actual, depending on status) end date for the study.", 0, 1, 2677 period); 2678 case -1998892262: 2679 /* sponsor */ return new Property("sponsor", "Reference(Organization)", 2680 "An organization that initiates the investigation and is legally responsible for the study.", 0, 1, sponsor); 2681 case 1437117175: 2682 /* principalInvestigator */ return new Property("principalInvestigator", 2683 "Reference(Practitioner|PractitionerRole)", 2684 "A researcher in a study who oversees multiple aspects of the study, such as concept development, protocol writing, protocol submission for IRB approval, participant recruitment, informed consent, data collection, analysis, interpretation and presentation.", 2685 0, 1, principalInvestigator); 2686 case 3530567: 2687 /* site */ return new Property("site", "Reference(Location)", 2688 "A facility in which study activities are conducted.", 0, java.lang.Integer.MAX_VALUE, site); 2689 case 1181369065: 2690 /* reasonStopped */ return new Property("reasonStopped", "CodeableConcept", 2691 "A description and/or code explaining the premature termination of the study.", 0, 1, reasonStopped); 2692 case 3387378: 2693 /* note */ return new Property("note", "Annotation", 2694 "Comments made about the study by the performer, subject or other participants.", 0, 2695 java.lang.Integer.MAX_VALUE, note); 2696 case 96860: 2697 /* arm */ return new Property("arm", "", 2698 "Describes an expected sequence of events for one of the participants of a study. E.g. Exposure to drug A, wash-out, exposure to drug B, wash-out, follow-up.", 2699 0, java.lang.Integer.MAX_VALUE, arm); 2700 case -1489585863: 2701 /* objective */ return new Property("objective", "", 2702 "A goal that the study is aiming to achieve in terms of a scientific question to be answered by the analysis of data collected during the study.", 2703 0, java.lang.Integer.MAX_VALUE, objective); 2704 default: 2705 return super.getNamedProperty(_hash, _name, _checkValid); 2706 } 2707 2708 } 2709 2710 @Override 2711 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2712 switch (hash) { 2713 case -1618432855: 2714 /* identifier */ return this.identifier == null ? new Base[0] 2715 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2716 case 110371416: 2717 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2718 case -989163880: 2719 /* protocol */ return this.protocol == null ? new Base[0] : this.protocol.toArray(new Base[this.protocol.size()]); // Reference 2720 case -995410646: 2721 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2722 case -892481550: 2723 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ResearchStudyStatus> 2724 case -2132842986: 2725 /* primaryPurposeType */ return this.primaryPurposeType == null ? new Base[0] 2726 : new Base[] { this.primaryPurposeType }; // CodeableConcept 2727 case 106629499: 2728 /* phase */ return this.phase == null ? new Base[0] : new Base[] { this.phase }; // CodeableConcept 2729 case 50511102: 2730 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2731 case 97604824: 2732 /* focus */ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // CodeableConcept 2733 case -861311717: 2734 /* condition */ return this.condition == null ? new Base[0] 2735 : this.condition.toArray(new Base[this.condition.size()]); // CodeableConcept 2736 case 951526432: 2737 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2738 case 666807069: 2739 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 2740 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 2741 case -814408215: 2742 /* keyword */ return this.keyword == null ? new Base[0] : this.keyword.toArray(new Base[this.keyword.size()]); // CodeableConcept 2743 case 1901043637: 2744 /* location */ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // CodeableConcept 2745 case -1724546052: 2746 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 2747 case 116089604: 2748 /* enrollment */ return this.enrollment == null ? new Base[0] 2749 : this.enrollment.toArray(new Base[this.enrollment.size()]); // Reference 2750 case -991726143: 2751 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 2752 case -1998892262: 2753 /* sponsor */ return this.sponsor == null ? new Base[0] : new Base[] { this.sponsor }; // Reference 2754 case 1437117175: 2755 /* principalInvestigator */ return this.principalInvestigator == null ? new Base[0] 2756 : new Base[] { this.principalInvestigator }; // Reference 2757 case 3530567: 2758 /* site */ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // Reference 2759 case 1181369065: 2760 /* reasonStopped */ return this.reasonStopped == null ? new Base[0] : new Base[] { this.reasonStopped }; // CodeableConcept 2761 case 3387378: 2762 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2763 case 96860: 2764 /* arm */ return this.arm == null ? new Base[0] : this.arm.toArray(new Base[this.arm.size()]); // ResearchStudyArmComponent 2765 case -1489585863: 2766 /* objective */ return this.objective == null ? new Base[0] 2767 : this.objective.toArray(new Base[this.objective.size()]); // ResearchStudyObjectiveComponent 2768 default: 2769 return super.getProperty(hash, name, checkValid); 2770 } 2771 2772 } 2773 2774 @Override 2775 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2776 switch (hash) { 2777 case -1618432855: // identifier 2778 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2779 return value; 2780 case 110371416: // title 2781 this.title = castToString(value); // StringType 2782 return value; 2783 case -989163880: // protocol 2784 this.getProtocol().add(castToReference(value)); // Reference 2785 return value; 2786 case -995410646: // partOf 2787 this.getPartOf().add(castToReference(value)); // Reference 2788 return value; 2789 case -892481550: // status 2790 value = new ResearchStudyStatusEnumFactory().fromType(castToCode(value)); 2791 this.status = (Enumeration) value; // Enumeration<ResearchStudyStatus> 2792 return value; 2793 case -2132842986: // primaryPurposeType 2794 this.primaryPurposeType = castToCodeableConcept(value); // CodeableConcept 2795 return value; 2796 case 106629499: // phase 2797 this.phase = castToCodeableConcept(value); // CodeableConcept 2798 return value; 2799 case 50511102: // category 2800 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2801 return value; 2802 case 97604824: // focus 2803 this.getFocus().add(castToCodeableConcept(value)); // CodeableConcept 2804 return value; 2805 case -861311717: // condition 2806 this.getCondition().add(castToCodeableConcept(value)); // CodeableConcept 2807 return value; 2808 case 951526432: // contact 2809 this.getContact().add(castToContactDetail(value)); // ContactDetail 2810 return value; 2811 case 666807069: // relatedArtifact 2812 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 2813 return value; 2814 case -814408215: // keyword 2815 this.getKeyword().add(castToCodeableConcept(value)); // CodeableConcept 2816 return value; 2817 case 1901043637: // location 2818 this.getLocation().add(castToCodeableConcept(value)); // CodeableConcept 2819 return value; 2820 case -1724546052: // description 2821 this.description = castToMarkdown(value); // MarkdownType 2822 return value; 2823 case 116089604: // enrollment 2824 this.getEnrollment().add(castToReference(value)); // Reference 2825 return value; 2826 case -991726143: // period 2827 this.period = castToPeriod(value); // Period 2828 return value; 2829 case -1998892262: // sponsor 2830 this.sponsor = castToReference(value); // Reference 2831 return value; 2832 case 1437117175: // principalInvestigator 2833 this.principalInvestigator = castToReference(value); // Reference 2834 return value; 2835 case 3530567: // site 2836 this.getSite().add(castToReference(value)); // Reference 2837 return value; 2838 case 1181369065: // reasonStopped 2839 this.reasonStopped = castToCodeableConcept(value); // CodeableConcept 2840 return value; 2841 case 3387378: // note 2842 this.getNote().add(castToAnnotation(value)); // Annotation 2843 return value; 2844 case 96860: // arm 2845 this.getArm().add((ResearchStudyArmComponent) value); // ResearchStudyArmComponent 2846 return value; 2847 case -1489585863: // objective 2848 this.getObjective().add((ResearchStudyObjectiveComponent) value); // ResearchStudyObjectiveComponent 2849 return value; 2850 default: 2851 return super.setProperty(hash, name, value); 2852 } 2853 2854 } 2855 2856 @Override 2857 public Base setProperty(String name, Base value) throws FHIRException { 2858 if (name.equals("identifier")) { 2859 this.getIdentifier().add(castToIdentifier(value)); 2860 } else if (name.equals("title")) { 2861 this.title = castToString(value); // StringType 2862 } else if (name.equals("protocol")) { 2863 this.getProtocol().add(castToReference(value)); 2864 } else if (name.equals("partOf")) { 2865 this.getPartOf().add(castToReference(value)); 2866 } else if (name.equals("status")) { 2867 value = new ResearchStudyStatusEnumFactory().fromType(castToCode(value)); 2868 this.status = (Enumeration) value; // Enumeration<ResearchStudyStatus> 2869 } else if (name.equals("primaryPurposeType")) { 2870 this.primaryPurposeType = castToCodeableConcept(value); // CodeableConcept 2871 } else if (name.equals("phase")) { 2872 this.phase = castToCodeableConcept(value); // CodeableConcept 2873 } else if (name.equals("category")) { 2874 this.getCategory().add(castToCodeableConcept(value)); 2875 } else if (name.equals("focus")) { 2876 this.getFocus().add(castToCodeableConcept(value)); 2877 } else if (name.equals("condition")) { 2878 this.getCondition().add(castToCodeableConcept(value)); 2879 } else if (name.equals("contact")) { 2880 this.getContact().add(castToContactDetail(value)); 2881 } else if (name.equals("relatedArtifact")) { 2882 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 2883 } else if (name.equals("keyword")) { 2884 this.getKeyword().add(castToCodeableConcept(value)); 2885 } else if (name.equals("location")) { 2886 this.getLocation().add(castToCodeableConcept(value)); 2887 } else if (name.equals("description")) { 2888 this.description = castToMarkdown(value); // MarkdownType 2889 } else if (name.equals("enrollment")) { 2890 this.getEnrollment().add(castToReference(value)); 2891 } else if (name.equals("period")) { 2892 this.period = castToPeriod(value); // Period 2893 } else if (name.equals("sponsor")) { 2894 this.sponsor = castToReference(value); // Reference 2895 } else if (name.equals("principalInvestigator")) { 2896 this.principalInvestigator = castToReference(value); // Reference 2897 } else if (name.equals("site")) { 2898 this.getSite().add(castToReference(value)); 2899 } else if (name.equals("reasonStopped")) { 2900 this.reasonStopped = castToCodeableConcept(value); // CodeableConcept 2901 } else if (name.equals("note")) { 2902 this.getNote().add(castToAnnotation(value)); 2903 } else if (name.equals("arm")) { 2904 this.getArm().add((ResearchStudyArmComponent) value); 2905 } else if (name.equals("objective")) { 2906 this.getObjective().add((ResearchStudyObjectiveComponent) value); 2907 } else 2908 return super.setProperty(name, value); 2909 return value; 2910 } 2911 2912 @Override 2913 public void removeChild(String name, Base value) throws FHIRException { 2914 if (name.equals("identifier")) { 2915 this.getIdentifier().remove(castToIdentifier(value)); 2916 } else if (name.equals("title")) { 2917 this.title = null; 2918 } else if (name.equals("protocol")) { 2919 this.getProtocol().remove(castToReference(value)); 2920 } else if (name.equals("partOf")) { 2921 this.getPartOf().remove(castToReference(value)); 2922 } else if (name.equals("status")) { 2923 this.status = null; 2924 } else if (name.equals("primaryPurposeType")) { 2925 this.primaryPurposeType = null; 2926 } else if (name.equals("phase")) { 2927 this.phase = null; 2928 } else if (name.equals("category")) { 2929 this.getCategory().remove(castToCodeableConcept(value)); 2930 } else if (name.equals("focus")) { 2931 this.getFocus().remove(castToCodeableConcept(value)); 2932 } else if (name.equals("condition")) { 2933 this.getCondition().remove(castToCodeableConcept(value)); 2934 } else if (name.equals("contact")) { 2935 this.getContact().remove(castToContactDetail(value)); 2936 } else if (name.equals("relatedArtifact")) { 2937 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 2938 } else if (name.equals("keyword")) { 2939 this.getKeyword().remove(castToCodeableConcept(value)); 2940 } else if (name.equals("location")) { 2941 this.getLocation().remove(castToCodeableConcept(value)); 2942 } else if (name.equals("description")) { 2943 this.description = null; 2944 } else if (name.equals("enrollment")) { 2945 this.getEnrollment().remove(castToReference(value)); 2946 } else if (name.equals("period")) { 2947 this.period = null; 2948 } else if (name.equals("sponsor")) { 2949 this.sponsor = null; 2950 } else if (name.equals("principalInvestigator")) { 2951 this.principalInvestigator = null; 2952 } else if (name.equals("site")) { 2953 this.getSite().remove(castToReference(value)); 2954 } else if (name.equals("reasonStopped")) { 2955 this.reasonStopped = null; 2956 } else if (name.equals("note")) { 2957 this.getNote().remove(castToAnnotation(value)); 2958 } else if (name.equals("arm")) { 2959 this.getArm().remove((ResearchStudyArmComponent) value); 2960 } else if (name.equals("objective")) { 2961 this.getObjective().remove((ResearchStudyObjectiveComponent) value); 2962 } else 2963 super.removeChild(name, value); 2964 2965 } 2966 2967 @Override 2968 public Base makeProperty(int hash, String name) throws FHIRException { 2969 switch (hash) { 2970 case -1618432855: 2971 return addIdentifier(); 2972 case 110371416: 2973 return getTitleElement(); 2974 case -989163880: 2975 return addProtocol(); 2976 case -995410646: 2977 return addPartOf(); 2978 case -892481550: 2979 return getStatusElement(); 2980 case -2132842986: 2981 return getPrimaryPurposeType(); 2982 case 106629499: 2983 return getPhase(); 2984 case 50511102: 2985 return addCategory(); 2986 case 97604824: 2987 return addFocus(); 2988 case -861311717: 2989 return addCondition(); 2990 case 951526432: 2991 return addContact(); 2992 case 666807069: 2993 return addRelatedArtifact(); 2994 case -814408215: 2995 return addKeyword(); 2996 case 1901043637: 2997 return addLocation(); 2998 case -1724546052: 2999 return getDescriptionElement(); 3000 case 116089604: 3001 return addEnrollment(); 3002 case -991726143: 3003 return getPeriod(); 3004 case -1998892262: 3005 return getSponsor(); 3006 case 1437117175: 3007 return getPrincipalInvestigator(); 3008 case 3530567: 3009 return addSite(); 3010 case 1181369065: 3011 return getReasonStopped(); 3012 case 3387378: 3013 return addNote(); 3014 case 96860: 3015 return addArm(); 3016 case -1489585863: 3017 return addObjective(); 3018 default: 3019 return super.makeProperty(hash, name); 3020 } 3021 3022 } 3023 3024 @Override 3025 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3026 switch (hash) { 3027 case -1618432855: 3028 /* identifier */ return new String[] { "Identifier" }; 3029 case 110371416: 3030 /* title */ return new String[] { "string" }; 3031 case -989163880: 3032 /* protocol */ return new String[] { "Reference" }; 3033 case -995410646: 3034 /* partOf */ return new String[] { "Reference" }; 3035 case -892481550: 3036 /* status */ return new String[] { "code" }; 3037 case -2132842986: 3038 /* primaryPurposeType */ return new String[] { "CodeableConcept" }; 3039 case 106629499: 3040 /* phase */ return new String[] { "CodeableConcept" }; 3041 case 50511102: 3042 /* category */ return new String[] { "CodeableConcept" }; 3043 case 97604824: 3044 /* focus */ return new String[] { "CodeableConcept" }; 3045 case -861311717: 3046 /* condition */ return new String[] { "CodeableConcept" }; 3047 case 951526432: 3048 /* contact */ return new String[] { "ContactDetail" }; 3049 case 666807069: 3050 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 3051 case -814408215: 3052 /* keyword */ return new String[] { "CodeableConcept" }; 3053 case 1901043637: 3054 /* location */ return new String[] { "CodeableConcept" }; 3055 case -1724546052: 3056 /* description */ return new String[] { "markdown" }; 3057 case 116089604: 3058 /* enrollment */ return new String[] { "Reference" }; 3059 case -991726143: 3060 /* period */ return new String[] { "Period" }; 3061 case -1998892262: 3062 /* sponsor */ return new String[] { "Reference" }; 3063 case 1437117175: 3064 /* principalInvestigator */ return new String[] { "Reference" }; 3065 case 3530567: 3066 /* site */ return new String[] { "Reference" }; 3067 case 1181369065: 3068 /* reasonStopped */ return new String[] { "CodeableConcept" }; 3069 case 3387378: 3070 /* note */ return new String[] { "Annotation" }; 3071 case 96860: 3072 /* arm */ return new String[] {}; 3073 case -1489585863: 3074 /* objective */ return new String[] {}; 3075 default: 3076 return super.getTypesForProperty(hash, name); 3077 } 3078 3079 } 3080 3081 @Override 3082 public Base addChild(String name) throws FHIRException { 3083 if (name.equals("identifier")) { 3084 return addIdentifier(); 3085 } else if (name.equals("title")) { 3086 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.title"); 3087 } else if (name.equals("protocol")) { 3088 return addProtocol(); 3089 } else if (name.equals("partOf")) { 3090 return addPartOf(); 3091 } else if (name.equals("status")) { 3092 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.status"); 3093 } else if (name.equals("primaryPurposeType")) { 3094 this.primaryPurposeType = new CodeableConcept(); 3095 return this.primaryPurposeType; 3096 } else if (name.equals("phase")) { 3097 this.phase = new CodeableConcept(); 3098 return this.phase; 3099 } else if (name.equals("category")) { 3100 return addCategory(); 3101 } else if (name.equals("focus")) { 3102 return addFocus(); 3103 } else if (name.equals("condition")) { 3104 return addCondition(); 3105 } else if (name.equals("contact")) { 3106 return addContact(); 3107 } else if (name.equals("relatedArtifact")) { 3108 return addRelatedArtifact(); 3109 } else if (name.equals("keyword")) { 3110 return addKeyword(); 3111 } else if (name.equals("location")) { 3112 return addLocation(); 3113 } else if (name.equals("description")) { 3114 throw new FHIRException("Cannot call addChild on a singleton property ResearchStudy.description"); 3115 } else if (name.equals("enrollment")) { 3116 return addEnrollment(); 3117 } else if (name.equals("period")) { 3118 this.period = new Period(); 3119 return this.period; 3120 } else if (name.equals("sponsor")) { 3121 this.sponsor = new Reference(); 3122 return this.sponsor; 3123 } else if (name.equals("principalInvestigator")) { 3124 this.principalInvestigator = new Reference(); 3125 return this.principalInvestigator; 3126 } else if (name.equals("site")) { 3127 return addSite(); 3128 } else if (name.equals("reasonStopped")) { 3129 this.reasonStopped = new CodeableConcept(); 3130 return this.reasonStopped; 3131 } else if (name.equals("note")) { 3132 return addNote(); 3133 } else if (name.equals("arm")) { 3134 return addArm(); 3135 } else if (name.equals("objective")) { 3136 return addObjective(); 3137 } else 3138 return super.addChild(name); 3139 } 3140 3141 public String fhirType() { 3142 return "ResearchStudy"; 3143 3144 } 3145 3146 public ResearchStudy copy() { 3147 ResearchStudy dst = new ResearchStudy(); 3148 copyValues(dst); 3149 return dst; 3150 } 3151 3152 public void copyValues(ResearchStudy dst) { 3153 super.copyValues(dst); 3154 if (identifier != null) { 3155 dst.identifier = new ArrayList<Identifier>(); 3156 for (Identifier i : identifier) 3157 dst.identifier.add(i.copy()); 3158 } 3159 ; 3160 dst.title = title == null ? null : title.copy(); 3161 if (protocol != null) { 3162 dst.protocol = new ArrayList<Reference>(); 3163 for (Reference i : protocol) 3164 dst.protocol.add(i.copy()); 3165 } 3166 ; 3167 if (partOf != null) { 3168 dst.partOf = new ArrayList<Reference>(); 3169 for (Reference i : partOf) 3170 dst.partOf.add(i.copy()); 3171 } 3172 ; 3173 dst.status = status == null ? null : status.copy(); 3174 dst.primaryPurposeType = primaryPurposeType == null ? null : primaryPurposeType.copy(); 3175 dst.phase = phase == null ? null : phase.copy(); 3176 if (category != null) { 3177 dst.category = new ArrayList<CodeableConcept>(); 3178 for (CodeableConcept i : category) 3179 dst.category.add(i.copy()); 3180 } 3181 ; 3182 if (focus != null) { 3183 dst.focus = new ArrayList<CodeableConcept>(); 3184 for (CodeableConcept i : focus) 3185 dst.focus.add(i.copy()); 3186 } 3187 ; 3188 if (condition != null) { 3189 dst.condition = new ArrayList<CodeableConcept>(); 3190 for (CodeableConcept i : condition) 3191 dst.condition.add(i.copy()); 3192 } 3193 ; 3194 if (contact != null) { 3195 dst.contact = new ArrayList<ContactDetail>(); 3196 for (ContactDetail i : contact) 3197 dst.contact.add(i.copy()); 3198 } 3199 ; 3200 if (relatedArtifact != null) { 3201 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 3202 for (RelatedArtifact i : relatedArtifact) 3203 dst.relatedArtifact.add(i.copy()); 3204 } 3205 ; 3206 if (keyword != null) { 3207 dst.keyword = new ArrayList<CodeableConcept>(); 3208 for (CodeableConcept i : keyword) 3209 dst.keyword.add(i.copy()); 3210 } 3211 ; 3212 if (location != null) { 3213 dst.location = new ArrayList<CodeableConcept>(); 3214 for (CodeableConcept i : location) 3215 dst.location.add(i.copy()); 3216 } 3217 ; 3218 dst.description = description == null ? null : description.copy(); 3219 if (enrollment != null) { 3220 dst.enrollment = new ArrayList<Reference>(); 3221 for (Reference i : enrollment) 3222 dst.enrollment.add(i.copy()); 3223 } 3224 ; 3225 dst.period = period == null ? null : period.copy(); 3226 dst.sponsor = sponsor == null ? null : sponsor.copy(); 3227 dst.principalInvestigator = principalInvestigator == null ? null : principalInvestigator.copy(); 3228 if (site != null) { 3229 dst.site = new ArrayList<Reference>(); 3230 for (Reference i : site) 3231 dst.site.add(i.copy()); 3232 } 3233 ; 3234 dst.reasonStopped = reasonStopped == null ? null : reasonStopped.copy(); 3235 if (note != null) { 3236 dst.note = new ArrayList<Annotation>(); 3237 for (Annotation i : note) 3238 dst.note.add(i.copy()); 3239 } 3240 ; 3241 if (arm != null) { 3242 dst.arm = new ArrayList<ResearchStudyArmComponent>(); 3243 for (ResearchStudyArmComponent i : arm) 3244 dst.arm.add(i.copy()); 3245 } 3246 ; 3247 if (objective != null) { 3248 dst.objective = new ArrayList<ResearchStudyObjectiveComponent>(); 3249 for (ResearchStudyObjectiveComponent i : objective) 3250 dst.objective.add(i.copy()); 3251 } 3252 ; 3253 } 3254 3255 protected ResearchStudy typedCopy() { 3256 return copy(); 3257 } 3258 3259 @Override 3260 public boolean equalsDeep(Base other_) { 3261 if (!super.equalsDeep(other_)) 3262 return false; 3263 if (!(other_ instanceof ResearchStudy)) 3264 return false; 3265 ResearchStudy o = (ResearchStudy) other_; 3266 return compareDeep(identifier, o.identifier, true) && compareDeep(title, o.title, true) 3267 && compareDeep(protocol, o.protocol, true) && compareDeep(partOf, o.partOf, true) 3268 && compareDeep(status, o.status, true) && compareDeep(primaryPurposeType, o.primaryPurposeType, true) 3269 && compareDeep(phase, o.phase, true) && compareDeep(category, o.category, true) 3270 && compareDeep(focus, o.focus, true) && compareDeep(condition, o.condition, true) 3271 && compareDeep(contact, o.contact, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 3272 && compareDeep(keyword, o.keyword, true) && compareDeep(location, o.location, true) 3273 && compareDeep(description, o.description, true) && compareDeep(enrollment, o.enrollment, true) 3274 && compareDeep(period, o.period, true) && compareDeep(sponsor, o.sponsor, true) 3275 && compareDeep(principalInvestigator, o.principalInvestigator, true) && compareDeep(site, o.site, true) 3276 && compareDeep(reasonStopped, o.reasonStopped, true) && compareDeep(note, o.note, true) 3277 && compareDeep(arm, o.arm, true) && compareDeep(objective, o.objective, true); 3278 } 3279 3280 @Override 3281 public boolean equalsShallow(Base other_) { 3282 if (!super.equalsShallow(other_)) 3283 return false; 3284 if (!(other_ instanceof ResearchStudy)) 3285 return false; 3286 ResearchStudy o = (ResearchStudy) other_; 3287 return compareValues(title, o.title, true) && compareValues(status, o.status, true) 3288 && compareValues(description, o.description, true); 3289 } 3290 3291 public boolean isEmpty() { 3292 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, title, protocol, partOf, status, 3293 primaryPurposeType, phase, category, focus, condition, contact, relatedArtifact, keyword, location, description, 3294 enrollment, period, sponsor, principalInvestigator, site, reasonStopped, note, arm, objective); 3295 } 3296 3297 @Override 3298 public ResourceType getResourceType() { 3299 return ResourceType.ResearchStudy; 3300 } 3301 3302 /** 3303 * Search parameter: <b>date</b> 3304 * <p> 3305 * Description: <b>When the study began and ended</b><br> 3306 * Type: <b>date</b><br> 3307 * Path: <b>ResearchStudy.period</b><br> 3308 * </p> 3309 */ 3310 @SearchParamDefinition(name = "date", path = "ResearchStudy.period", description = "When the study began and ended", type = "date") 3311 public static final String SP_DATE = "date"; 3312 /** 3313 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3314 * <p> 3315 * Description: <b>When the study began and ended</b><br> 3316 * Type: <b>date</b><br> 3317 * Path: <b>ResearchStudy.period</b><br> 3318 * </p> 3319 */ 3320 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3321 SP_DATE); 3322 3323 /** 3324 * Search parameter: <b>identifier</b> 3325 * <p> 3326 * Description: <b>Business Identifier for study</b><br> 3327 * Type: <b>token</b><br> 3328 * Path: <b>ResearchStudy.identifier</b><br> 3329 * </p> 3330 */ 3331 @SearchParamDefinition(name = "identifier", path = "ResearchStudy.identifier", description = "Business Identifier for study", type = "token") 3332 public static final String SP_IDENTIFIER = "identifier"; 3333 /** 3334 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3335 * <p> 3336 * Description: <b>Business Identifier for study</b><br> 3337 * Type: <b>token</b><br> 3338 * Path: <b>ResearchStudy.identifier</b><br> 3339 * </p> 3340 */ 3341 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3342 SP_IDENTIFIER); 3343 3344 /** 3345 * Search parameter: <b>partof</b> 3346 * <p> 3347 * Description: <b>Part of larger study</b><br> 3348 * Type: <b>reference</b><br> 3349 * Path: <b>ResearchStudy.partOf</b><br> 3350 * </p> 3351 */ 3352 @SearchParamDefinition(name = "partof", path = "ResearchStudy.partOf", description = "Part of larger study", type = "reference", target = { 3353 ResearchStudy.class }) 3354 public static final String SP_PARTOF = "partof"; 3355 /** 3356 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 3357 * <p> 3358 * Description: <b>Part of larger study</b><br> 3359 * Type: <b>reference</b><br> 3360 * Path: <b>ResearchStudy.partOf</b><br> 3361 * </p> 3362 */ 3363 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3364 SP_PARTOF); 3365 3366 /** 3367 * Constant for fluent queries to be used to add include statements. Specifies 3368 * the path value of "<b>ResearchStudy:partof</b>". 3369 */ 3370 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include( 3371 "ResearchStudy:partof").toLocked(); 3372 3373 /** 3374 * Search parameter: <b>sponsor</b> 3375 * <p> 3376 * Description: <b>Organization that initiates and is legally responsible for 3377 * the study</b><br> 3378 * Type: <b>reference</b><br> 3379 * Path: <b>ResearchStudy.sponsor</b><br> 3380 * </p> 3381 */ 3382 @SearchParamDefinition(name = "sponsor", path = "ResearchStudy.sponsor", description = "Organization that initiates and is legally responsible for the study", type = "reference", target = { 3383 Organization.class }) 3384 public static final String SP_SPONSOR = "sponsor"; 3385 /** 3386 * <b>Fluent Client</b> search parameter constant for <b>sponsor</b> 3387 * <p> 3388 * Description: <b>Organization that initiates and is legally responsible for 3389 * the study</b><br> 3390 * Type: <b>reference</b><br> 3391 * Path: <b>ResearchStudy.sponsor</b><br> 3392 * </p> 3393 */ 3394 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPONSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3395 SP_SPONSOR); 3396 3397 /** 3398 * Constant for fluent queries to be used to add include statements. Specifies 3399 * the path value of "<b>ResearchStudy:sponsor</b>". 3400 */ 3401 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPONSOR = new ca.uhn.fhir.model.api.Include( 3402 "ResearchStudy:sponsor").toLocked(); 3403 3404 /** 3405 * Search parameter: <b>focus</b> 3406 * <p> 3407 * Description: <b>Drugs, devices, etc. under study</b><br> 3408 * Type: <b>token</b><br> 3409 * Path: <b>ResearchStudy.focus</b><br> 3410 * </p> 3411 */ 3412 @SearchParamDefinition(name = "focus", path = "ResearchStudy.focus", description = "Drugs, devices, etc. under study", type = "token") 3413 public static final String SP_FOCUS = "focus"; 3414 /** 3415 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 3416 * <p> 3417 * Description: <b>Drugs, devices, etc. under study</b><br> 3418 * Type: <b>token</b><br> 3419 * Path: <b>ResearchStudy.focus</b><br> 3420 * </p> 3421 */ 3422 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3423 SP_FOCUS); 3424 3425 /** 3426 * Search parameter: <b>principalinvestigator</b> 3427 * <p> 3428 * Description: <b>Researcher who oversees multiple aspects of the study</b><br> 3429 * Type: <b>reference</b><br> 3430 * Path: <b>ResearchStudy.principalInvestigator</b><br> 3431 * </p> 3432 */ 3433 @SearchParamDefinition(name = "principalinvestigator", path = "ResearchStudy.principalInvestigator", description = "Researcher who oversees multiple aspects of the study", type = "reference", providesMembershipIn = { 3434 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 3435 PractitionerRole.class }) 3436 public static final String SP_PRINCIPALINVESTIGATOR = "principalinvestigator"; 3437 /** 3438 * <b>Fluent Client</b> search parameter constant for 3439 * <b>principalinvestigator</b> 3440 * <p> 3441 * Description: <b>Researcher who oversees multiple aspects of the study</b><br> 3442 * Type: <b>reference</b><br> 3443 * Path: <b>ResearchStudy.principalInvestigator</b><br> 3444 * </p> 3445 */ 3446 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRINCIPALINVESTIGATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3447 SP_PRINCIPALINVESTIGATOR); 3448 3449 /** 3450 * Constant for fluent queries to be used to add include statements. Specifies 3451 * the path value of "<b>ResearchStudy:principalinvestigator</b>". 3452 */ 3453 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRINCIPALINVESTIGATOR = new ca.uhn.fhir.model.api.Include( 3454 "ResearchStudy:principalinvestigator").toLocked(); 3455 3456 /** 3457 * Search parameter: <b>title</b> 3458 * <p> 3459 * Description: <b>Name for this study</b><br> 3460 * Type: <b>string</b><br> 3461 * Path: <b>ResearchStudy.title</b><br> 3462 * </p> 3463 */ 3464 @SearchParamDefinition(name = "title", path = "ResearchStudy.title", description = "Name for this study", type = "string") 3465 public static final String SP_TITLE = "title"; 3466 /** 3467 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3468 * <p> 3469 * Description: <b>Name for this study</b><br> 3470 * Type: <b>string</b><br> 3471 * Path: <b>ResearchStudy.title</b><br> 3472 * </p> 3473 */ 3474 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3475 SP_TITLE); 3476 3477 /** 3478 * Search parameter: <b>protocol</b> 3479 * <p> 3480 * Description: <b>Steps followed in executing study</b><br> 3481 * Type: <b>reference</b><br> 3482 * Path: <b>ResearchStudy.protocol</b><br> 3483 * </p> 3484 */ 3485 @SearchParamDefinition(name = "protocol", path = "ResearchStudy.protocol", description = "Steps followed in executing study", type = "reference", target = { 3486 PlanDefinition.class }) 3487 public static final String SP_PROTOCOL = "protocol"; 3488 /** 3489 * <b>Fluent Client</b> search parameter constant for <b>protocol</b> 3490 * <p> 3491 * Description: <b>Steps followed in executing study</b><br> 3492 * Type: <b>reference</b><br> 3493 * Path: <b>ResearchStudy.protocol</b><br> 3494 * </p> 3495 */ 3496 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROTOCOL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3497 SP_PROTOCOL); 3498 3499 /** 3500 * Constant for fluent queries to be used to add include statements. Specifies 3501 * the path value of "<b>ResearchStudy:protocol</b>". 3502 */ 3503 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROTOCOL = new ca.uhn.fhir.model.api.Include( 3504 "ResearchStudy:protocol").toLocked(); 3505 3506 /** 3507 * Search parameter: <b>site</b> 3508 * <p> 3509 * Description: <b>Facility where study activities are conducted</b><br> 3510 * Type: <b>reference</b><br> 3511 * Path: <b>ResearchStudy.site</b><br> 3512 * </p> 3513 */ 3514 @SearchParamDefinition(name = "site", path = "ResearchStudy.site", description = "Facility where study activities are conducted", type = "reference", target = { 3515 Location.class }) 3516 public static final String SP_SITE = "site"; 3517 /** 3518 * <b>Fluent Client</b> search parameter constant for <b>site</b> 3519 * <p> 3520 * Description: <b>Facility where study activities are conducted</b><br> 3521 * Type: <b>reference</b><br> 3522 * Path: <b>ResearchStudy.site</b><br> 3523 * </p> 3524 */ 3525 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SITE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3526 SP_SITE); 3527 3528 /** 3529 * Constant for fluent queries to be used to add include statements. Specifies 3530 * the path value of "<b>ResearchStudy:site</b>". 3531 */ 3532 public static final ca.uhn.fhir.model.api.Include INCLUDE_SITE = new ca.uhn.fhir.model.api.Include( 3533 "ResearchStudy:site").toLocked(); 3534 3535 /** 3536 * Search parameter: <b>location</b> 3537 * <p> 3538 * Description: <b>Geographic region(s) for study</b><br> 3539 * Type: <b>token</b><br> 3540 * Path: <b>ResearchStudy.location</b><br> 3541 * </p> 3542 */ 3543 @SearchParamDefinition(name = "location", path = "ResearchStudy.location", description = "Geographic region(s) for study", type = "token") 3544 public static final String SP_LOCATION = "location"; 3545 /** 3546 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3547 * <p> 3548 * Description: <b>Geographic region(s) for study</b><br> 3549 * Type: <b>token</b><br> 3550 * Path: <b>ResearchStudy.location</b><br> 3551 * </p> 3552 */ 3553 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LOCATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3554 SP_LOCATION); 3555 3556 /** 3557 * Search parameter: <b>category</b> 3558 * <p> 3559 * Description: <b>Classifications for the study</b><br> 3560 * Type: <b>token</b><br> 3561 * Path: <b>ResearchStudy.category</b><br> 3562 * </p> 3563 */ 3564 @SearchParamDefinition(name = "category", path = "ResearchStudy.category", description = "Classifications for the study", type = "token") 3565 public static final String SP_CATEGORY = "category"; 3566 /** 3567 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3568 * <p> 3569 * Description: <b>Classifications for the study</b><br> 3570 * Type: <b>token</b><br> 3571 * Path: <b>ResearchStudy.category</b><br> 3572 * </p> 3573 */ 3574 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3575 SP_CATEGORY); 3576 3577 /** 3578 * Search parameter: <b>keyword</b> 3579 * <p> 3580 * Description: <b>Used to search for the study</b><br> 3581 * Type: <b>token</b><br> 3582 * Path: <b>ResearchStudy.keyword</b><br> 3583 * </p> 3584 */ 3585 @SearchParamDefinition(name = "keyword", path = "ResearchStudy.keyword", description = "Used to search for the study", type = "token") 3586 public static final String SP_KEYWORD = "keyword"; 3587 /** 3588 * <b>Fluent Client</b> search parameter constant for <b>keyword</b> 3589 * <p> 3590 * Description: <b>Used to search for the study</b><br> 3591 * Type: <b>token</b><br> 3592 * Path: <b>ResearchStudy.keyword</b><br> 3593 * </p> 3594 */ 3595 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KEYWORD = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3596 SP_KEYWORD); 3597 3598 /** 3599 * Search parameter: <b>status</b> 3600 * <p> 3601 * Description: <b>active | administratively-completed | approved | 3602 * closed-to-accrual | closed-to-accrual-and-intervention | completed | 3603 * disapproved | in-review | temporarily-closed-to-accrual | 3604 * temporarily-closed-to-accrual-and-intervention | withdrawn</b><br> 3605 * Type: <b>token</b><br> 3606 * Path: <b>ResearchStudy.status</b><br> 3607 * </p> 3608 */ 3609 @SearchParamDefinition(name = "status", path = "ResearchStudy.status", description = "active | administratively-completed | approved | closed-to-accrual | closed-to-accrual-and-intervention | completed | disapproved | in-review | temporarily-closed-to-accrual | temporarily-closed-to-accrual-and-intervention | withdrawn", type = "token") 3610 public static final String SP_STATUS = "status"; 3611 /** 3612 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3613 * <p> 3614 * Description: <b>active | administratively-completed | approved | 3615 * closed-to-accrual | closed-to-accrual-and-intervention | completed | 3616 * disapproved | in-review | temporarily-closed-to-accrual | 3617 * temporarily-closed-to-accrual-and-intervention | withdrawn</b><br> 3618 * Type: <b>token</b><br> 3619 * Path: <b>ResearchStudy.status</b><br> 3620 * </p> 3621 */ 3622 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3623 SP_STATUS); 3624 3625}