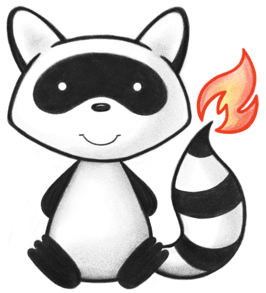
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * A physical entity which is the primary unit of operational and/or 046 * administrative interest in a study. 047 */ 048@ResourceDef(name = "ResearchSubject", profile = "http://hl7.org/fhir/StructureDefinition/ResearchSubject") 049public class ResearchSubject extends DomainResource { 050 051 public enum ResearchSubjectStatus { 052 /** 053 * An identified person that can be considered for inclusion in a study. 054 */ 055 CANDIDATE, 056 /** 057 * A person that has met the eligibility criteria for inclusion in a study. 058 */ 059 ELIGIBLE, 060 /** 061 * A person is no longer receiving study intervention and/or being evaluated 062 * with tests and procedures according to the protocol, but they are being 063 * monitored on a protocol-prescribed schedule. 064 */ 065 FOLLOWUP, 066 /** 067 * A person who did not meet one or more criteria required for participation in 068 * a study is considered to have failed screening or is ineligible for the 069 * study. 070 */ 071 INELIGIBLE, 072 /** 073 * A person for whom registration was not completed. 074 */ 075 NOTREGISTERED, 076 /** 077 * A person that has ended their participation on a study either because their 078 * treatment/observation is complete or through not responding, withdrawal, 079 * non-compliance and/or adverse event. 080 */ 081 OFFSTUDY, 082 /** 083 * A person that is enrolled or registered on a study. 084 */ 085 ONSTUDY, 086 /** 087 * The person is receiving the treatment or participating in an activity (e.g. 088 * yoga, diet, etc.) that the study is evaluating. 089 */ 090 ONSTUDYINTERVENTION, 091 /** 092 * The subject is being evaluated via tests and assessments according to the 093 * study calendar, but is not receiving any intervention. Note that this state 094 * is study-dependent and might not exist in all studies. A synonym for this is 095 * "short-term follow-up". 096 */ 097 ONSTUDYOBSERVATION, 098 /** 099 * A person is pre-registered for a study. 100 */ 101 PENDINGONSTUDY, 102 /** 103 * A person that is potentially eligible for participation in the study. 104 */ 105 POTENTIALCANDIDATE, 106 /** 107 * A person who is being evaluated for eligibility for a study. 108 */ 109 SCREENING, 110 /** 111 * The person has withdrawn their participation in the study before 112 * registration. 113 */ 114 WITHDRAWN, 115 /** 116 * added to help the parsers with the generic types 117 */ 118 NULL; 119 120 public static ResearchSubjectStatus fromCode(String codeString) throws FHIRException { 121 if (codeString == null || "".equals(codeString)) 122 return null; 123 if ("candidate".equals(codeString)) 124 return CANDIDATE; 125 if ("eligible".equals(codeString)) 126 return ELIGIBLE; 127 if ("follow-up".equals(codeString)) 128 return FOLLOWUP; 129 if ("ineligible".equals(codeString)) 130 return INELIGIBLE; 131 if ("not-registered".equals(codeString)) 132 return NOTREGISTERED; 133 if ("off-study".equals(codeString)) 134 return OFFSTUDY; 135 if ("on-study".equals(codeString)) 136 return ONSTUDY; 137 if ("on-study-intervention".equals(codeString)) 138 return ONSTUDYINTERVENTION; 139 if ("on-study-observation".equals(codeString)) 140 return ONSTUDYOBSERVATION; 141 if ("pending-on-study".equals(codeString)) 142 return PENDINGONSTUDY; 143 if ("potential-candidate".equals(codeString)) 144 return POTENTIALCANDIDATE; 145 if ("screening".equals(codeString)) 146 return SCREENING; 147 if ("withdrawn".equals(codeString)) 148 return WITHDRAWN; 149 if (Configuration.isAcceptInvalidEnums()) 150 return null; 151 else 152 throw new FHIRException("Unknown ResearchSubjectStatus code '" + codeString + "'"); 153 } 154 155 public String toCode() { 156 switch (this) { 157 case CANDIDATE: 158 return "candidate"; 159 case ELIGIBLE: 160 return "eligible"; 161 case FOLLOWUP: 162 return "follow-up"; 163 case INELIGIBLE: 164 return "ineligible"; 165 case NOTREGISTERED: 166 return "not-registered"; 167 case OFFSTUDY: 168 return "off-study"; 169 case ONSTUDY: 170 return "on-study"; 171 case ONSTUDYINTERVENTION: 172 return "on-study-intervention"; 173 case ONSTUDYOBSERVATION: 174 return "on-study-observation"; 175 case PENDINGONSTUDY: 176 return "pending-on-study"; 177 case POTENTIALCANDIDATE: 178 return "potential-candidate"; 179 case SCREENING: 180 return "screening"; 181 case WITHDRAWN: 182 return "withdrawn"; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getSystem() { 191 switch (this) { 192 case CANDIDATE: 193 return "http://hl7.org/fhir/research-subject-status"; 194 case ELIGIBLE: 195 return "http://hl7.org/fhir/research-subject-status"; 196 case FOLLOWUP: 197 return "http://hl7.org/fhir/research-subject-status"; 198 case INELIGIBLE: 199 return "http://hl7.org/fhir/research-subject-status"; 200 case NOTREGISTERED: 201 return "http://hl7.org/fhir/research-subject-status"; 202 case OFFSTUDY: 203 return "http://hl7.org/fhir/research-subject-status"; 204 case ONSTUDY: 205 return "http://hl7.org/fhir/research-subject-status"; 206 case ONSTUDYINTERVENTION: 207 return "http://hl7.org/fhir/research-subject-status"; 208 case ONSTUDYOBSERVATION: 209 return "http://hl7.org/fhir/research-subject-status"; 210 case PENDINGONSTUDY: 211 return "http://hl7.org/fhir/research-subject-status"; 212 case POTENTIALCANDIDATE: 213 return "http://hl7.org/fhir/research-subject-status"; 214 case SCREENING: 215 return "http://hl7.org/fhir/research-subject-status"; 216 case WITHDRAWN: 217 return "http://hl7.org/fhir/research-subject-status"; 218 case NULL: 219 return null; 220 default: 221 return "?"; 222 } 223 } 224 225 public String getDefinition() { 226 switch (this) { 227 case CANDIDATE: 228 return "An identified person that can be considered for inclusion in a study."; 229 case ELIGIBLE: 230 return "A person that has met the eligibility criteria for inclusion in a study."; 231 case FOLLOWUP: 232 return "A person is no longer receiving study intervention and/or being evaluated with tests and procedures according to the protocol, but they are being monitored on a protocol-prescribed schedule."; 233 case INELIGIBLE: 234 return "A person who did not meet one or more criteria required for participation in a study is considered to have failed screening or\nis ineligible for the study."; 235 case NOTREGISTERED: 236 return "A person for whom registration was not completed."; 237 case OFFSTUDY: 238 return "A person that has ended their participation on a study either because their treatment/observation is complete or through not\nresponding, withdrawal, non-compliance and/or adverse event."; 239 case ONSTUDY: 240 return "A person that is enrolled or registered on a study."; 241 case ONSTUDYINTERVENTION: 242 return "The person is receiving the treatment or participating in an activity (e.g. yoga, diet, etc.) that the study is evaluating."; 243 case ONSTUDYOBSERVATION: 244 return "The subject is being evaluated via tests and assessments according to the study calendar, but is not receiving any intervention. Note that this state is study-dependent and might not exist in all studies. A synonym for this is \"short-term follow-up\"."; 245 case PENDINGONSTUDY: 246 return "A person is pre-registered for a study."; 247 case POTENTIALCANDIDATE: 248 return "A person that is potentially eligible for participation in the study."; 249 case SCREENING: 250 return "A person who is being evaluated for eligibility for a study."; 251 case WITHDRAWN: 252 return "The person has withdrawn their participation in the study before registration."; 253 case NULL: 254 return null; 255 default: 256 return "?"; 257 } 258 } 259 260 public String getDisplay() { 261 switch (this) { 262 case CANDIDATE: 263 return "Candidate"; 264 case ELIGIBLE: 265 return "Eligible"; 266 case FOLLOWUP: 267 return "Follow-up"; 268 case INELIGIBLE: 269 return "Ineligible"; 270 case NOTREGISTERED: 271 return "Not Registered"; 272 case OFFSTUDY: 273 return "Off-study"; 274 case ONSTUDY: 275 return "On-study"; 276 case ONSTUDYINTERVENTION: 277 return "On-study-intervention"; 278 case ONSTUDYOBSERVATION: 279 return "On-study-observation"; 280 case PENDINGONSTUDY: 281 return "Pending on-study"; 282 case POTENTIALCANDIDATE: 283 return "Potential Candidate"; 284 case SCREENING: 285 return "Screening"; 286 case WITHDRAWN: 287 return "Withdrawn"; 288 case NULL: 289 return null; 290 default: 291 return "?"; 292 } 293 } 294 } 295 296 public static class ResearchSubjectStatusEnumFactory implements EnumFactory<ResearchSubjectStatus> { 297 public ResearchSubjectStatus fromCode(String codeString) throws IllegalArgumentException { 298 if (codeString == null || "".equals(codeString)) 299 if (codeString == null || "".equals(codeString)) 300 return null; 301 if ("candidate".equals(codeString)) 302 return ResearchSubjectStatus.CANDIDATE; 303 if ("eligible".equals(codeString)) 304 return ResearchSubjectStatus.ELIGIBLE; 305 if ("follow-up".equals(codeString)) 306 return ResearchSubjectStatus.FOLLOWUP; 307 if ("ineligible".equals(codeString)) 308 return ResearchSubjectStatus.INELIGIBLE; 309 if ("not-registered".equals(codeString)) 310 return ResearchSubjectStatus.NOTREGISTERED; 311 if ("off-study".equals(codeString)) 312 return ResearchSubjectStatus.OFFSTUDY; 313 if ("on-study".equals(codeString)) 314 return ResearchSubjectStatus.ONSTUDY; 315 if ("on-study-intervention".equals(codeString)) 316 return ResearchSubjectStatus.ONSTUDYINTERVENTION; 317 if ("on-study-observation".equals(codeString)) 318 return ResearchSubjectStatus.ONSTUDYOBSERVATION; 319 if ("pending-on-study".equals(codeString)) 320 return ResearchSubjectStatus.PENDINGONSTUDY; 321 if ("potential-candidate".equals(codeString)) 322 return ResearchSubjectStatus.POTENTIALCANDIDATE; 323 if ("screening".equals(codeString)) 324 return ResearchSubjectStatus.SCREENING; 325 if ("withdrawn".equals(codeString)) 326 return ResearchSubjectStatus.WITHDRAWN; 327 throw new IllegalArgumentException("Unknown ResearchSubjectStatus code '" + codeString + "'"); 328 } 329 330 public Enumeration<ResearchSubjectStatus> fromType(PrimitiveType<?> code) throws FHIRException { 331 if (code == null) 332 return null; 333 if (code.isEmpty()) 334 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.NULL, code); 335 String codeString = code.asStringValue(); 336 if (codeString == null || "".equals(codeString)) 337 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.NULL, code); 338 if ("candidate".equals(codeString)) 339 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.CANDIDATE, code); 340 if ("eligible".equals(codeString)) 341 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.ELIGIBLE, code); 342 if ("follow-up".equals(codeString)) 343 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.FOLLOWUP, code); 344 if ("ineligible".equals(codeString)) 345 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.INELIGIBLE, code); 346 if ("not-registered".equals(codeString)) 347 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.NOTREGISTERED, code); 348 if ("off-study".equals(codeString)) 349 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.OFFSTUDY, code); 350 if ("on-study".equals(codeString)) 351 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.ONSTUDY, code); 352 if ("on-study-intervention".equals(codeString)) 353 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.ONSTUDYINTERVENTION, code); 354 if ("on-study-observation".equals(codeString)) 355 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.ONSTUDYOBSERVATION, code); 356 if ("pending-on-study".equals(codeString)) 357 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.PENDINGONSTUDY, code); 358 if ("potential-candidate".equals(codeString)) 359 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.POTENTIALCANDIDATE, code); 360 if ("screening".equals(codeString)) 361 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.SCREENING, code); 362 if ("withdrawn".equals(codeString)) 363 return new Enumeration<ResearchSubjectStatus>(this, ResearchSubjectStatus.WITHDRAWN, code); 364 throw new FHIRException("Unknown ResearchSubjectStatus code '" + codeString + "'"); 365 } 366 367 public String toCode(ResearchSubjectStatus code) { 368 if (code == ResearchSubjectStatus.NULL) 369 return null; 370 if (code == ResearchSubjectStatus.CANDIDATE) 371 return "candidate"; 372 if (code == ResearchSubjectStatus.ELIGIBLE) 373 return "eligible"; 374 if (code == ResearchSubjectStatus.FOLLOWUP) 375 return "follow-up"; 376 if (code == ResearchSubjectStatus.INELIGIBLE) 377 return "ineligible"; 378 if (code == ResearchSubjectStatus.NOTREGISTERED) 379 return "not-registered"; 380 if (code == ResearchSubjectStatus.OFFSTUDY) 381 return "off-study"; 382 if (code == ResearchSubjectStatus.ONSTUDY) 383 return "on-study"; 384 if (code == ResearchSubjectStatus.ONSTUDYINTERVENTION) 385 return "on-study-intervention"; 386 if (code == ResearchSubjectStatus.ONSTUDYOBSERVATION) 387 return "on-study-observation"; 388 if (code == ResearchSubjectStatus.PENDINGONSTUDY) 389 return "pending-on-study"; 390 if (code == ResearchSubjectStatus.POTENTIALCANDIDATE) 391 return "potential-candidate"; 392 if (code == ResearchSubjectStatus.SCREENING) 393 return "screening"; 394 if (code == ResearchSubjectStatus.WITHDRAWN) 395 return "withdrawn"; 396 return "?"; 397 } 398 399 public String toSystem(ResearchSubjectStatus code) { 400 return code.getSystem(); 401 } 402 } 403 404 /** 405 * Identifiers assigned to this research subject for a study. 406 */ 407 @Child(name = "identifier", type = { 408 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 409 @Description(shortDefinition = "Business Identifier for research subject in a study", formalDefinition = "Identifiers assigned to this research subject for a study.") 410 protected List<Identifier> identifier; 411 412 /** 413 * The current state of the subject. 414 */ 415 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 416 @Description(shortDefinition = "candidate | eligible | follow-up | ineligible | not-registered | off-study | on-study | on-study-intervention | on-study-observation | pending-on-study | potential-candidate | screening | withdrawn", formalDefinition = "The current state of the subject.") 417 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/research-subject-status") 418 protected Enumeration<ResearchSubjectStatus> status; 419 420 /** 421 * The dates the subject began and ended their participation in the study. 422 */ 423 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 424 @Description(shortDefinition = "Start and end of participation", formalDefinition = "The dates the subject began and ended their participation in the study.") 425 protected Period period; 426 427 /** 428 * Reference to the study the subject is participating in. 429 */ 430 @Child(name = "study", type = { ResearchStudy.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 431 @Description(shortDefinition = "Study subject is part of", formalDefinition = "Reference to the study the subject is participating in.") 432 protected Reference study; 433 434 /** 435 * The actual object that is the target of the reference (Reference to the study 436 * the subject is participating in.) 437 */ 438 protected ResearchStudy studyTarget; 439 440 /** 441 * The record of the person or animal who is involved in the study. 442 */ 443 @Child(name = "individual", type = { Patient.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 444 @Description(shortDefinition = "Who is part of study", formalDefinition = "The record of the person or animal who is involved in the study.") 445 protected Reference individual; 446 447 /** 448 * The actual object that is the target of the reference (The record of the 449 * person or animal who is involved in the study.) 450 */ 451 protected Patient individualTarget; 452 453 /** 454 * The name of the arm in the study the subject is expected to follow as part of 455 * this study. 456 */ 457 @Child(name = "assignedArm", type = { 458 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 459 @Description(shortDefinition = "What path should be followed", formalDefinition = "The name of the arm in the study the subject is expected to follow as part of this study.") 460 protected StringType assignedArm; 461 462 /** 463 * The name of the arm in the study the subject actually followed as part of 464 * this study. 465 */ 466 @Child(name = "actualArm", type = { 467 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 468 @Description(shortDefinition = "What path was followed", formalDefinition = "The name of the arm in the study the subject actually followed as part of this study.") 469 protected StringType actualArm; 470 471 /** 472 * A record of the patient's informed agreement to participate in the study. 473 */ 474 @Child(name = "consent", type = { Consent.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 475 @Description(shortDefinition = "Agreement to participate in study", formalDefinition = "A record of the patient's informed agreement to participate in the study.") 476 protected Reference consent; 477 478 /** 479 * The actual object that is the target of the reference (A record of the 480 * patient's informed agreement to participate in the study.) 481 */ 482 protected Consent consentTarget; 483 484 private static final long serialVersionUID = -884133739L; 485 486 /** 487 * Constructor 488 */ 489 public ResearchSubject() { 490 super(); 491 } 492 493 /** 494 * Constructor 495 */ 496 public ResearchSubject(Enumeration<ResearchSubjectStatus> status, Reference study, Reference individual) { 497 super(); 498 this.status = status; 499 this.study = study; 500 this.individual = individual; 501 } 502 503 /** 504 * @return {@link #identifier} (Identifiers assigned to this research subject 505 * for a study.) 506 */ 507 public List<Identifier> getIdentifier() { 508 if (this.identifier == null) 509 this.identifier = new ArrayList<Identifier>(); 510 return this.identifier; 511 } 512 513 /** 514 * @return Returns a reference to <code>this</code> for easy method chaining 515 */ 516 public ResearchSubject setIdentifier(List<Identifier> theIdentifier) { 517 this.identifier = theIdentifier; 518 return this; 519 } 520 521 public boolean hasIdentifier() { 522 if (this.identifier == null) 523 return false; 524 for (Identifier item : this.identifier) 525 if (!item.isEmpty()) 526 return true; 527 return false; 528 } 529 530 public Identifier addIdentifier() { // 3 531 Identifier t = new Identifier(); 532 if (this.identifier == null) 533 this.identifier = new ArrayList<Identifier>(); 534 this.identifier.add(t); 535 return t; 536 } 537 538 public ResearchSubject addIdentifier(Identifier t) { // 3 539 if (t == null) 540 return this; 541 if (this.identifier == null) 542 this.identifier = new ArrayList<Identifier>(); 543 this.identifier.add(t); 544 return this; 545 } 546 547 /** 548 * @return The first repetition of repeating field {@link #identifier}, creating 549 * it if it does not already exist 550 */ 551 public Identifier getIdentifierFirstRep() { 552 if (getIdentifier().isEmpty()) { 553 addIdentifier(); 554 } 555 return getIdentifier().get(0); 556 } 557 558 /** 559 * @return {@link #status} (The current state of the subject.). This is the 560 * underlying object with id, value and extensions. The accessor 561 * "getStatus" gives direct access to the value 562 */ 563 public Enumeration<ResearchSubjectStatus> getStatusElement() { 564 if (this.status == null) 565 if (Configuration.errorOnAutoCreate()) 566 throw new Error("Attempt to auto-create ResearchSubject.status"); 567 else if (Configuration.doAutoCreate()) 568 this.status = new Enumeration<ResearchSubjectStatus>(new ResearchSubjectStatusEnumFactory()); // bb 569 return this.status; 570 } 571 572 public boolean hasStatusElement() { 573 return this.status != null && !this.status.isEmpty(); 574 } 575 576 public boolean hasStatus() { 577 return this.status != null && !this.status.isEmpty(); 578 } 579 580 /** 581 * @param value {@link #status} (The current state of the subject.). This is the 582 * underlying object with id, value and extensions. The accessor 583 * "getStatus" gives direct access to the value 584 */ 585 public ResearchSubject setStatusElement(Enumeration<ResearchSubjectStatus> value) { 586 this.status = value; 587 return this; 588 } 589 590 /** 591 * @return The current state of the subject. 592 */ 593 public ResearchSubjectStatus getStatus() { 594 return this.status == null ? null : this.status.getValue(); 595 } 596 597 /** 598 * @param value The current state of the subject. 599 */ 600 public ResearchSubject setStatus(ResearchSubjectStatus value) { 601 if (this.status == null) 602 this.status = new Enumeration<ResearchSubjectStatus>(new ResearchSubjectStatusEnumFactory()); 603 this.status.setValue(value); 604 return this; 605 } 606 607 /** 608 * @return {@link #period} (The dates the subject began and ended their 609 * participation in the study.) 610 */ 611 public Period getPeriod() { 612 if (this.period == null) 613 if (Configuration.errorOnAutoCreate()) 614 throw new Error("Attempt to auto-create ResearchSubject.period"); 615 else if (Configuration.doAutoCreate()) 616 this.period = new Period(); // cc 617 return this.period; 618 } 619 620 public boolean hasPeriod() { 621 return this.period != null && !this.period.isEmpty(); 622 } 623 624 /** 625 * @param value {@link #period} (The dates the subject began and ended their 626 * participation in the study.) 627 */ 628 public ResearchSubject setPeriod(Period value) { 629 this.period = value; 630 return this; 631 } 632 633 /** 634 * @return {@link #study} (Reference to the study the subject is participating 635 * in.) 636 */ 637 public Reference getStudy() { 638 if (this.study == null) 639 if (Configuration.errorOnAutoCreate()) 640 throw new Error("Attempt to auto-create ResearchSubject.study"); 641 else if (Configuration.doAutoCreate()) 642 this.study = new Reference(); // cc 643 return this.study; 644 } 645 646 public boolean hasStudy() { 647 return this.study != null && !this.study.isEmpty(); 648 } 649 650 /** 651 * @param value {@link #study} (Reference to the study the subject is 652 * participating in.) 653 */ 654 public ResearchSubject setStudy(Reference value) { 655 this.study = value; 656 return this; 657 } 658 659 /** 660 * @return {@link #study} The actual object that is the target of the reference. 661 * The reference library doesn't populate this, but you can use it to 662 * hold the resource if you resolve it. (Reference to the study the 663 * subject is participating in.) 664 */ 665 public ResearchStudy getStudyTarget() { 666 if (this.studyTarget == null) 667 if (Configuration.errorOnAutoCreate()) 668 throw new Error("Attempt to auto-create ResearchSubject.study"); 669 else if (Configuration.doAutoCreate()) 670 this.studyTarget = new ResearchStudy(); // aa 671 return this.studyTarget; 672 } 673 674 /** 675 * @param value {@link #study} The actual object that is the target of the 676 * reference. The reference library doesn't use these, but you can 677 * use it to hold the resource if you resolve it. (Reference to the 678 * study the subject is participating in.) 679 */ 680 public ResearchSubject setStudyTarget(ResearchStudy value) { 681 this.studyTarget = value; 682 return this; 683 } 684 685 /** 686 * @return {@link #individual} (The record of the person or animal who is 687 * involved in the study.) 688 */ 689 public Reference getIndividual() { 690 if (this.individual == null) 691 if (Configuration.errorOnAutoCreate()) 692 throw new Error("Attempt to auto-create ResearchSubject.individual"); 693 else if (Configuration.doAutoCreate()) 694 this.individual = new Reference(); // cc 695 return this.individual; 696 } 697 698 public boolean hasIndividual() { 699 return this.individual != null && !this.individual.isEmpty(); 700 } 701 702 /** 703 * @param value {@link #individual} (The record of the person or animal who is 704 * involved in the study.) 705 */ 706 public ResearchSubject setIndividual(Reference value) { 707 this.individual = value; 708 return this; 709 } 710 711 /** 712 * @return {@link #individual} The actual object that is the target of the 713 * reference. The reference library doesn't populate this, but you can 714 * use it to hold the resource if you resolve it. (The record of the 715 * person or animal who is involved in the study.) 716 */ 717 public Patient getIndividualTarget() { 718 if (this.individualTarget == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create ResearchSubject.individual"); 721 else if (Configuration.doAutoCreate()) 722 this.individualTarget = new Patient(); // aa 723 return this.individualTarget; 724 } 725 726 /** 727 * @param value {@link #individual} The actual object that is the target of the 728 * reference. The reference library doesn't use these, but you can 729 * use it to hold the resource if you resolve it. (The record of 730 * the person or animal who is involved in the study.) 731 */ 732 public ResearchSubject setIndividualTarget(Patient value) { 733 this.individualTarget = value; 734 return this; 735 } 736 737 /** 738 * @return {@link #assignedArm} (The name of the arm in the study the subject is 739 * expected to follow as part of this study.). This is the underlying 740 * object with id, value and extensions. The accessor "getAssignedArm" 741 * gives direct access to the value 742 */ 743 public StringType getAssignedArmElement() { 744 if (this.assignedArm == null) 745 if (Configuration.errorOnAutoCreate()) 746 throw new Error("Attempt to auto-create ResearchSubject.assignedArm"); 747 else if (Configuration.doAutoCreate()) 748 this.assignedArm = new StringType(); // bb 749 return this.assignedArm; 750 } 751 752 public boolean hasAssignedArmElement() { 753 return this.assignedArm != null && !this.assignedArm.isEmpty(); 754 } 755 756 public boolean hasAssignedArm() { 757 return this.assignedArm != null && !this.assignedArm.isEmpty(); 758 } 759 760 /** 761 * @param value {@link #assignedArm} (The name of the arm in the study the 762 * subject is expected to follow as part of this study.). This is 763 * the underlying object with id, value and extensions. The 764 * accessor "getAssignedArm" gives direct access to the value 765 */ 766 public ResearchSubject setAssignedArmElement(StringType value) { 767 this.assignedArm = value; 768 return this; 769 } 770 771 /** 772 * @return The name of the arm in the study the subject is expected to follow as 773 * part of this study. 774 */ 775 public String getAssignedArm() { 776 return this.assignedArm == null ? null : this.assignedArm.getValue(); 777 } 778 779 /** 780 * @param value The name of the arm in the study the subject is expected to 781 * follow as part of this study. 782 */ 783 public ResearchSubject setAssignedArm(String value) { 784 if (Utilities.noString(value)) 785 this.assignedArm = null; 786 else { 787 if (this.assignedArm == null) 788 this.assignedArm = new StringType(); 789 this.assignedArm.setValue(value); 790 } 791 return this; 792 } 793 794 /** 795 * @return {@link #actualArm} (The name of the arm in the study the subject 796 * actually followed as part of this study.). This is the underlying 797 * object with id, value and extensions. The accessor "getActualArm" 798 * gives direct access to the value 799 */ 800 public StringType getActualArmElement() { 801 if (this.actualArm == null) 802 if (Configuration.errorOnAutoCreate()) 803 throw new Error("Attempt to auto-create ResearchSubject.actualArm"); 804 else if (Configuration.doAutoCreate()) 805 this.actualArm = new StringType(); // bb 806 return this.actualArm; 807 } 808 809 public boolean hasActualArmElement() { 810 return this.actualArm != null && !this.actualArm.isEmpty(); 811 } 812 813 public boolean hasActualArm() { 814 return this.actualArm != null && !this.actualArm.isEmpty(); 815 } 816 817 /** 818 * @param value {@link #actualArm} (The name of the arm in the study the subject 819 * actually followed as part of this study.). This is the 820 * underlying object with id, value and extensions. The accessor 821 * "getActualArm" gives direct access to the value 822 */ 823 public ResearchSubject setActualArmElement(StringType value) { 824 this.actualArm = value; 825 return this; 826 } 827 828 /** 829 * @return The name of the arm in the study the subject actually followed as 830 * part of this study. 831 */ 832 public String getActualArm() { 833 return this.actualArm == null ? null : this.actualArm.getValue(); 834 } 835 836 /** 837 * @param value The name of the arm in the study the subject actually followed 838 * as part of this study. 839 */ 840 public ResearchSubject setActualArm(String value) { 841 if (Utilities.noString(value)) 842 this.actualArm = null; 843 else { 844 if (this.actualArm == null) 845 this.actualArm = new StringType(); 846 this.actualArm.setValue(value); 847 } 848 return this; 849 } 850 851 /** 852 * @return {@link #consent} (A record of the patient's informed agreement to 853 * participate in the study.) 854 */ 855 public Reference getConsent() { 856 if (this.consent == null) 857 if (Configuration.errorOnAutoCreate()) 858 throw new Error("Attempt to auto-create ResearchSubject.consent"); 859 else if (Configuration.doAutoCreate()) 860 this.consent = new Reference(); // cc 861 return this.consent; 862 } 863 864 public boolean hasConsent() { 865 return this.consent != null && !this.consent.isEmpty(); 866 } 867 868 /** 869 * @param value {@link #consent} (A record of the patient's informed agreement 870 * to participate in the study.) 871 */ 872 public ResearchSubject setConsent(Reference value) { 873 this.consent = value; 874 return this; 875 } 876 877 /** 878 * @return {@link #consent} The actual object that is the target of the 879 * reference. The reference library doesn't populate this, but you can 880 * use it to hold the resource if you resolve it. (A record of the 881 * patient's informed agreement to participate in the study.) 882 */ 883 public Consent getConsentTarget() { 884 if (this.consentTarget == null) 885 if (Configuration.errorOnAutoCreate()) 886 throw new Error("Attempt to auto-create ResearchSubject.consent"); 887 else if (Configuration.doAutoCreate()) 888 this.consentTarget = new Consent(); // aa 889 return this.consentTarget; 890 } 891 892 /** 893 * @param value {@link #consent} The actual object that is the target of the 894 * reference. The reference library doesn't use these, but you can 895 * use it to hold the resource if you resolve it. (A record of the 896 * patient's informed agreement to participate in the study.) 897 */ 898 public ResearchSubject setConsentTarget(Consent value) { 899 this.consentTarget = value; 900 return this; 901 } 902 903 protected void listChildren(List<Property> children) { 904 super.listChildren(children); 905 children.add(new Property("identifier", "Identifier", "Identifiers assigned to this research subject for a study.", 906 0, java.lang.Integer.MAX_VALUE, identifier)); 907 children.add(new Property("status", "code", "The current state of the subject.", 0, 1, status)); 908 children.add(new Property("period", "Period", 909 "The dates the subject began and ended their participation in the study.", 0, 1, period)); 910 children.add(new Property("study", "Reference(ResearchStudy)", 911 "Reference to the study the subject is participating in.", 0, 1, study)); 912 children.add(new Property("individual", "Reference(Patient)", 913 "The record of the person or animal who is involved in the study.", 0, 1, individual)); 914 children.add(new Property("assignedArm", "string", 915 "The name of the arm in the study the subject is expected to follow as part of this study.", 0, 1, 916 assignedArm)); 917 children.add(new Property("actualArm", "string", 918 "The name of the arm in the study the subject actually followed as part of this study.", 0, 1, actualArm)); 919 children.add(new Property("consent", "Reference(Consent)", 920 "A record of the patient's informed agreement to participate in the study.", 0, 1, consent)); 921 } 922 923 @Override 924 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 925 switch (_hash) { 926 case -1618432855: 927 /* identifier */ return new Property("identifier", "Identifier", 928 "Identifiers assigned to this research subject for a study.", 0, java.lang.Integer.MAX_VALUE, identifier); 929 case -892481550: 930 /* status */ return new Property("status", "code", "The current state of the subject.", 0, 1, status); 931 case -991726143: 932 /* period */ return new Property("period", "Period", 933 "The dates the subject began and ended their participation in the study.", 0, 1, period); 934 case 109776329: 935 /* study */ return new Property("study", "Reference(ResearchStudy)", 936 "Reference to the study the subject is participating in.", 0, 1, study); 937 case -46292327: 938 /* individual */ return new Property("individual", "Reference(Patient)", 939 "The record of the person or animal who is involved in the study.", 0, 1, individual); 940 case 1741912494: 941 /* assignedArm */ return new Property("assignedArm", "string", 942 "The name of the arm in the study the subject is expected to follow as part of this study.", 0, 1, 943 assignedArm); 944 case 528827886: 945 /* actualArm */ return new Property("actualArm", "string", 946 "The name of the arm in the study the subject actually followed as part of this study.", 0, 1, actualArm); 947 case 951500826: 948 /* consent */ return new Property("consent", "Reference(Consent)", 949 "A record of the patient's informed agreement to participate in the study.", 0, 1, consent); 950 default: 951 return super.getNamedProperty(_hash, _name, _checkValid); 952 } 953 954 } 955 956 @Override 957 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 958 switch (hash) { 959 case -1618432855: 960 /* identifier */ return this.identifier == null ? new Base[0] 961 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 962 case -892481550: 963 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ResearchSubjectStatus> 964 case -991726143: 965 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 966 case 109776329: 967 /* study */ return this.study == null ? new Base[0] : new Base[] { this.study }; // Reference 968 case -46292327: 969 /* individual */ return this.individual == null ? new Base[0] : new Base[] { this.individual }; // Reference 970 case 1741912494: 971 /* assignedArm */ return this.assignedArm == null ? new Base[0] : new Base[] { this.assignedArm }; // StringType 972 case 528827886: 973 /* actualArm */ return this.actualArm == null ? new Base[0] : new Base[] { this.actualArm }; // StringType 974 case 951500826: 975 /* consent */ return this.consent == null ? new Base[0] : new Base[] { this.consent }; // Reference 976 default: 977 return super.getProperty(hash, name, checkValid); 978 } 979 980 } 981 982 @Override 983 public Base setProperty(int hash, String name, Base value) throws FHIRException { 984 switch (hash) { 985 case -1618432855: // identifier 986 this.getIdentifier().add(castToIdentifier(value)); // Identifier 987 return value; 988 case -892481550: // status 989 value = new ResearchSubjectStatusEnumFactory().fromType(castToCode(value)); 990 this.status = (Enumeration) value; // Enumeration<ResearchSubjectStatus> 991 return value; 992 case -991726143: // period 993 this.period = castToPeriod(value); // Period 994 return value; 995 case 109776329: // study 996 this.study = castToReference(value); // Reference 997 return value; 998 case -46292327: // individual 999 this.individual = castToReference(value); // Reference 1000 return value; 1001 case 1741912494: // assignedArm 1002 this.assignedArm = castToString(value); // StringType 1003 return value; 1004 case 528827886: // actualArm 1005 this.actualArm = castToString(value); // StringType 1006 return value; 1007 case 951500826: // consent 1008 this.consent = castToReference(value); // Reference 1009 return value; 1010 default: 1011 return super.setProperty(hash, name, value); 1012 } 1013 1014 } 1015 1016 @Override 1017 public Base setProperty(String name, Base value) throws FHIRException { 1018 if (name.equals("identifier")) { 1019 this.getIdentifier().add(castToIdentifier(value)); 1020 } else if (name.equals("status")) { 1021 value = new ResearchSubjectStatusEnumFactory().fromType(castToCode(value)); 1022 this.status = (Enumeration) value; // Enumeration<ResearchSubjectStatus> 1023 } else if (name.equals("period")) { 1024 this.period = castToPeriod(value); // Period 1025 } else if (name.equals("study")) { 1026 this.study = castToReference(value); // Reference 1027 } else if (name.equals("individual")) { 1028 this.individual = castToReference(value); // Reference 1029 } else if (name.equals("assignedArm")) { 1030 this.assignedArm = castToString(value); // StringType 1031 } else if (name.equals("actualArm")) { 1032 this.actualArm = castToString(value); // StringType 1033 } else if (name.equals("consent")) { 1034 this.consent = castToReference(value); // Reference 1035 } else 1036 return super.setProperty(name, value); 1037 return value; 1038 } 1039 1040 @Override 1041 public void removeChild(String name, Base value) throws FHIRException { 1042 if (name.equals("identifier")) { 1043 this.getIdentifier().remove(castToIdentifier(value)); 1044 } else if (name.equals("status")) { 1045 this.status = null; 1046 } else if (name.equals("period")) { 1047 this.period = null; 1048 } else if (name.equals("study")) { 1049 this.study = null; 1050 } else if (name.equals("individual")) { 1051 this.individual = null; 1052 } else if (name.equals("assignedArm")) { 1053 this.assignedArm = null; 1054 } else if (name.equals("actualArm")) { 1055 this.actualArm = null; 1056 } else if (name.equals("consent")) { 1057 this.consent = null; 1058 } else 1059 super.removeChild(name, value); 1060 1061 } 1062 1063 @Override 1064 public Base makeProperty(int hash, String name) throws FHIRException { 1065 switch (hash) { 1066 case -1618432855: 1067 return addIdentifier(); 1068 case -892481550: 1069 return getStatusElement(); 1070 case -991726143: 1071 return getPeriod(); 1072 case 109776329: 1073 return getStudy(); 1074 case -46292327: 1075 return getIndividual(); 1076 case 1741912494: 1077 return getAssignedArmElement(); 1078 case 528827886: 1079 return getActualArmElement(); 1080 case 951500826: 1081 return getConsent(); 1082 default: 1083 return super.makeProperty(hash, name); 1084 } 1085 1086 } 1087 1088 @Override 1089 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1090 switch (hash) { 1091 case -1618432855: 1092 /* identifier */ return new String[] { "Identifier" }; 1093 case -892481550: 1094 /* status */ return new String[] { "code" }; 1095 case -991726143: 1096 /* period */ return new String[] { "Period" }; 1097 case 109776329: 1098 /* study */ return new String[] { "Reference" }; 1099 case -46292327: 1100 /* individual */ return new String[] { "Reference" }; 1101 case 1741912494: 1102 /* assignedArm */ return new String[] { "string" }; 1103 case 528827886: 1104 /* actualArm */ return new String[] { "string" }; 1105 case 951500826: 1106 /* consent */ return new String[] { "Reference" }; 1107 default: 1108 return super.getTypesForProperty(hash, name); 1109 } 1110 1111 } 1112 1113 @Override 1114 public Base addChild(String name) throws FHIRException { 1115 if (name.equals("identifier")) { 1116 return addIdentifier(); 1117 } else if (name.equals("status")) { 1118 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.status"); 1119 } else if (name.equals("period")) { 1120 this.period = new Period(); 1121 return this.period; 1122 } else if (name.equals("study")) { 1123 this.study = new Reference(); 1124 return this.study; 1125 } else if (name.equals("individual")) { 1126 this.individual = new Reference(); 1127 return this.individual; 1128 } else if (name.equals("assignedArm")) { 1129 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.assignedArm"); 1130 } else if (name.equals("actualArm")) { 1131 throw new FHIRException("Cannot call addChild on a singleton property ResearchSubject.actualArm"); 1132 } else if (name.equals("consent")) { 1133 this.consent = new Reference(); 1134 return this.consent; 1135 } else 1136 return super.addChild(name); 1137 } 1138 1139 public String fhirType() { 1140 return "ResearchSubject"; 1141 1142 } 1143 1144 public ResearchSubject copy() { 1145 ResearchSubject dst = new ResearchSubject(); 1146 copyValues(dst); 1147 return dst; 1148 } 1149 1150 public void copyValues(ResearchSubject dst) { 1151 super.copyValues(dst); 1152 if (identifier != null) { 1153 dst.identifier = new ArrayList<Identifier>(); 1154 for (Identifier i : identifier) 1155 dst.identifier.add(i.copy()); 1156 } 1157 ; 1158 dst.status = status == null ? null : status.copy(); 1159 dst.period = period == null ? null : period.copy(); 1160 dst.study = study == null ? null : study.copy(); 1161 dst.individual = individual == null ? null : individual.copy(); 1162 dst.assignedArm = assignedArm == null ? null : assignedArm.copy(); 1163 dst.actualArm = actualArm == null ? null : actualArm.copy(); 1164 dst.consent = consent == null ? null : consent.copy(); 1165 } 1166 1167 protected ResearchSubject typedCopy() { 1168 return copy(); 1169 } 1170 1171 @Override 1172 public boolean equalsDeep(Base other_) { 1173 if (!super.equalsDeep(other_)) 1174 return false; 1175 if (!(other_ instanceof ResearchSubject)) 1176 return false; 1177 ResearchSubject o = (ResearchSubject) other_; 1178 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1179 && compareDeep(period, o.period, true) && compareDeep(study, o.study, true) 1180 && compareDeep(individual, o.individual, true) && compareDeep(assignedArm, o.assignedArm, true) 1181 && compareDeep(actualArm, o.actualArm, true) && compareDeep(consent, o.consent, true); 1182 } 1183 1184 @Override 1185 public boolean equalsShallow(Base other_) { 1186 if (!super.equalsShallow(other_)) 1187 return false; 1188 if (!(other_ instanceof ResearchSubject)) 1189 return false; 1190 ResearchSubject o = (ResearchSubject) other_; 1191 return compareValues(status, o.status, true) && compareValues(assignedArm, o.assignedArm, true) 1192 && compareValues(actualArm, o.actualArm, true); 1193 } 1194 1195 public boolean isEmpty() { 1196 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, period, study, individual, 1197 assignedArm, actualArm, consent); 1198 } 1199 1200 @Override 1201 public ResourceType getResourceType() { 1202 return ResourceType.ResearchSubject; 1203 } 1204 1205 /** 1206 * Search parameter: <b>date</b> 1207 * <p> 1208 * Description: <b>Start and end of participation</b><br> 1209 * Type: <b>date</b><br> 1210 * Path: <b>ResearchSubject.period</b><br> 1211 * </p> 1212 */ 1213 @SearchParamDefinition(name = "date", path = "ResearchSubject.period", description = "Start and end of participation", type = "date") 1214 public static final String SP_DATE = "date"; 1215 /** 1216 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1217 * <p> 1218 * Description: <b>Start and end of participation</b><br> 1219 * Type: <b>date</b><br> 1220 * Path: <b>ResearchSubject.period</b><br> 1221 * </p> 1222 */ 1223 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1224 SP_DATE); 1225 1226 /** 1227 * Search parameter: <b>identifier</b> 1228 * <p> 1229 * Description: <b>Business Identifier for research subject in a study</b><br> 1230 * Type: <b>token</b><br> 1231 * Path: <b>ResearchSubject.identifier</b><br> 1232 * </p> 1233 */ 1234 @SearchParamDefinition(name = "identifier", path = "ResearchSubject.identifier", description = "Business Identifier for research subject in a study", type = "token") 1235 public static final String SP_IDENTIFIER = "identifier"; 1236 /** 1237 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1238 * <p> 1239 * Description: <b>Business Identifier for research subject in a study</b><br> 1240 * Type: <b>token</b><br> 1241 * Path: <b>ResearchSubject.identifier</b><br> 1242 * </p> 1243 */ 1244 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1245 SP_IDENTIFIER); 1246 1247 /** 1248 * Search parameter: <b>study</b> 1249 * <p> 1250 * Description: <b>Study subject is part of</b><br> 1251 * Type: <b>reference</b><br> 1252 * Path: <b>ResearchSubject.study</b><br> 1253 * </p> 1254 */ 1255 @SearchParamDefinition(name = "study", path = "ResearchSubject.study", description = "Study subject is part of", type = "reference", target = { 1256 ResearchStudy.class }) 1257 public static final String SP_STUDY = "study"; 1258 /** 1259 * <b>Fluent Client</b> search parameter constant for <b>study</b> 1260 * <p> 1261 * Description: <b>Study subject is part of</b><br> 1262 * Type: <b>reference</b><br> 1263 * Path: <b>ResearchSubject.study</b><br> 1264 * </p> 1265 */ 1266 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam STUDY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1267 SP_STUDY); 1268 1269 /** 1270 * Constant for fluent queries to be used to add include statements. Specifies 1271 * the path value of "<b>ResearchSubject:study</b>". 1272 */ 1273 public static final ca.uhn.fhir.model.api.Include INCLUDE_STUDY = new ca.uhn.fhir.model.api.Include( 1274 "ResearchSubject:study").toLocked(); 1275 1276 /** 1277 * Search parameter: <b>individual</b> 1278 * <p> 1279 * Description: <b>Who is part of study</b><br> 1280 * Type: <b>reference</b><br> 1281 * Path: <b>ResearchSubject.individual</b><br> 1282 * </p> 1283 */ 1284 @SearchParamDefinition(name = "individual", path = "ResearchSubject.individual", description = "Who is part of study", type = "reference", providesMembershipIn = { 1285 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 1286 public static final String SP_INDIVIDUAL = "individual"; 1287 /** 1288 * <b>Fluent Client</b> search parameter constant for <b>individual</b> 1289 * <p> 1290 * Description: <b>Who is part of study</b><br> 1291 * Type: <b>reference</b><br> 1292 * Path: <b>ResearchSubject.individual</b><br> 1293 * </p> 1294 */ 1295 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INDIVIDUAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1296 SP_INDIVIDUAL); 1297 1298 /** 1299 * Constant for fluent queries to be used to add include statements. Specifies 1300 * the path value of "<b>ResearchSubject:individual</b>". 1301 */ 1302 public static final ca.uhn.fhir.model.api.Include INCLUDE_INDIVIDUAL = new ca.uhn.fhir.model.api.Include( 1303 "ResearchSubject:individual").toLocked(); 1304 1305 /** 1306 * Search parameter: <b>patient</b> 1307 * <p> 1308 * Description: <b>Who is part of study</b><br> 1309 * Type: <b>reference</b><br> 1310 * Path: <b>ResearchSubject.individual</b><br> 1311 * </p> 1312 */ 1313 @SearchParamDefinition(name = "patient", path = "ResearchSubject.individual", description = "Who is part of study", type = "reference", target = { 1314 Patient.class }) 1315 public static final String SP_PATIENT = "patient"; 1316 /** 1317 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1318 * <p> 1319 * Description: <b>Who is part of study</b><br> 1320 * Type: <b>reference</b><br> 1321 * Path: <b>ResearchSubject.individual</b><br> 1322 * </p> 1323 */ 1324 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1325 SP_PATIENT); 1326 1327 /** 1328 * Constant for fluent queries to be used to add include statements. Specifies 1329 * the path value of "<b>ResearchSubject:patient</b>". 1330 */ 1331 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1332 "ResearchSubject:patient").toLocked(); 1333 1334 /** 1335 * Search parameter: <b>status</b> 1336 * <p> 1337 * Description: <b>candidate | eligible | follow-up | ineligible | 1338 * not-registered | off-study | on-study | on-study-intervention | 1339 * on-study-observation | pending-on-study | potential-candidate | screening | 1340 * withdrawn</b><br> 1341 * Type: <b>token</b><br> 1342 * Path: <b>ResearchSubject.status</b><br> 1343 * </p> 1344 */ 1345 @SearchParamDefinition(name = "status", path = "ResearchSubject.status", description = "candidate | eligible | follow-up | ineligible | not-registered | off-study | on-study | on-study-intervention | on-study-observation | pending-on-study | potential-candidate | screening | withdrawn", type = "token") 1346 public static final String SP_STATUS = "status"; 1347 /** 1348 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1349 * <p> 1350 * Description: <b>candidate | eligible | follow-up | ineligible | 1351 * not-registered | off-study | on-study | on-study-intervention | 1352 * on-study-observation | pending-on-study | potential-candidate | screening | 1353 * withdrawn</b><br> 1354 * Type: <b>token</b><br> 1355 * Path: <b>ResearchSubject.status</b><br> 1356 * </p> 1357 */ 1358 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1359 SP_STATUS); 1360 1361}