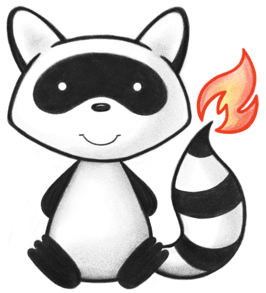
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * An assessment of the likely outcome(s) for a patient or other subject as well 050 * as the likelihood of each outcome. 051 */ 052@ResourceDef(name = "RiskAssessment", profile = "http://hl7.org/fhir/StructureDefinition/RiskAssessment") 053public class RiskAssessment extends DomainResource { 054 055 public enum RiskAssessmentStatus { 056 /** 057 * The existence of the observation is registered, but there is no result yet 058 * available. 059 */ 060 REGISTERED, 061 /** 062 * This is an initial or interim observation: data may be incomplete or 063 * unverified. 064 */ 065 PRELIMINARY, 066 /** 067 * The observation is complete and there are no further actions needed. 068 * Additional information such "released", "signed", etc would be represented 069 * using [Provenance](provenance.html) which provides not only the act but also 070 * the actors and dates and other related data. These act states would be 071 * associated with an observation status of `preliminary` until they are all 072 * completed and then a status of `final` would be applied. 073 */ 074 FINAL, 075 /** 076 * Subsequent to being Final, the observation has been modified subsequent. This 077 * includes updates/new information and corrections. 078 */ 079 AMENDED, 080 /** 081 * Subsequent to being Final, the observation has been modified to correct an 082 * error in the test result. 083 */ 084 CORRECTED, 085 /** 086 * The observation is unavailable because the measurement was not started or not 087 * completed (also sometimes called "aborted"). 088 */ 089 CANCELLED, 090 /** 091 * The observation has been withdrawn following previous final release. This 092 * electronic record should never have existed, though it is possible that 093 * real-world decisions were based on it. (If real-world activity has occurred, 094 * the status should be "cancelled" rather than "entered-in-error".). 095 */ 096 ENTEREDINERROR, 097 /** 098 * The authoring/source system does not know which of the status values 099 * currently applies for this observation. Note: This concept is not to be used 100 * for "other" - one of the listed statuses is presumed to apply, but the 101 * authoring/source system does not know which. 102 */ 103 UNKNOWN, 104 /** 105 * added to help the parsers with the generic types 106 */ 107 NULL; 108 109 public static RiskAssessmentStatus fromCode(String codeString) throws FHIRException { 110 if (codeString == null || "".equals(codeString)) 111 return null; 112 if ("registered".equals(codeString)) 113 return REGISTERED; 114 if ("preliminary".equals(codeString)) 115 return PRELIMINARY; 116 if ("final".equals(codeString)) 117 return FINAL; 118 if ("amended".equals(codeString)) 119 return AMENDED; 120 if ("corrected".equals(codeString)) 121 return CORRECTED; 122 if ("cancelled".equals(codeString)) 123 return CANCELLED; 124 if ("entered-in-error".equals(codeString)) 125 return ENTEREDINERROR; 126 if ("unknown".equals(codeString)) 127 return UNKNOWN; 128 if (Configuration.isAcceptInvalidEnums()) 129 return null; 130 else 131 throw new FHIRException("Unknown RiskAssessmentStatus code '" + codeString + "'"); 132 } 133 134 public String toCode() { 135 switch (this) { 136 case REGISTERED: 137 return "registered"; 138 case PRELIMINARY: 139 return "preliminary"; 140 case FINAL: 141 return "final"; 142 case AMENDED: 143 return "amended"; 144 case CORRECTED: 145 return "corrected"; 146 case CANCELLED: 147 return "cancelled"; 148 case ENTEREDINERROR: 149 return "entered-in-error"; 150 case UNKNOWN: 151 return "unknown"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getSystem() { 160 switch (this) { 161 case REGISTERED: 162 return "http://hl7.org/fhir/observation-status"; 163 case PRELIMINARY: 164 return "http://hl7.org/fhir/observation-status"; 165 case FINAL: 166 return "http://hl7.org/fhir/observation-status"; 167 case AMENDED: 168 return "http://hl7.org/fhir/observation-status"; 169 case CORRECTED: 170 return "http://hl7.org/fhir/observation-status"; 171 case CANCELLED: 172 return "http://hl7.org/fhir/observation-status"; 173 case ENTEREDINERROR: 174 return "http://hl7.org/fhir/observation-status"; 175 case UNKNOWN: 176 return "http://hl7.org/fhir/observation-status"; 177 case NULL: 178 return null; 179 default: 180 return "?"; 181 } 182 } 183 184 public String getDefinition() { 185 switch (this) { 186 case REGISTERED: 187 return "The existence of the observation is registered, but there is no result yet available."; 188 case PRELIMINARY: 189 return "This is an initial or interim observation: data may be incomplete or unverified."; 190 case FINAL: 191 return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 192 case AMENDED: 193 return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 194 case CORRECTED: 195 return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 196 case CANCELLED: 197 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 198 case ENTEREDINERROR: 199 return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 200 case UNKNOWN: 201 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 202 case NULL: 203 return null; 204 default: 205 return "?"; 206 } 207 } 208 209 public String getDisplay() { 210 switch (this) { 211 case REGISTERED: 212 return "Registered"; 213 case PRELIMINARY: 214 return "Preliminary"; 215 case FINAL: 216 return "Final"; 217 case AMENDED: 218 return "Amended"; 219 case CORRECTED: 220 return "Corrected"; 221 case CANCELLED: 222 return "Cancelled"; 223 case ENTEREDINERROR: 224 return "Entered in Error"; 225 case UNKNOWN: 226 return "Unknown"; 227 case NULL: 228 return null; 229 default: 230 return "?"; 231 } 232 } 233 } 234 235 public static class RiskAssessmentStatusEnumFactory implements EnumFactory<RiskAssessmentStatus> { 236 public RiskAssessmentStatus fromCode(String codeString) throws IllegalArgumentException { 237 if (codeString == null || "".equals(codeString)) 238 if (codeString == null || "".equals(codeString)) 239 return null; 240 if ("registered".equals(codeString)) 241 return RiskAssessmentStatus.REGISTERED; 242 if ("preliminary".equals(codeString)) 243 return RiskAssessmentStatus.PRELIMINARY; 244 if ("final".equals(codeString)) 245 return RiskAssessmentStatus.FINAL; 246 if ("amended".equals(codeString)) 247 return RiskAssessmentStatus.AMENDED; 248 if ("corrected".equals(codeString)) 249 return RiskAssessmentStatus.CORRECTED; 250 if ("cancelled".equals(codeString)) 251 return RiskAssessmentStatus.CANCELLED; 252 if ("entered-in-error".equals(codeString)) 253 return RiskAssessmentStatus.ENTEREDINERROR; 254 if ("unknown".equals(codeString)) 255 return RiskAssessmentStatus.UNKNOWN; 256 throw new IllegalArgumentException("Unknown RiskAssessmentStatus code '" + codeString + "'"); 257 } 258 259 public Enumeration<RiskAssessmentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 260 if (code == null) 261 return null; 262 if (code.isEmpty()) 263 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.NULL, code); 264 String codeString = code.asStringValue(); 265 if (codeString == null || "".equals(codeString)) 266 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.NULL, code); 267 if ("registered".equals(codeString)) 268 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.REGISTERED, code); 269 if ("preliminary".equals(codeString)) 270 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.PRELIMINARY, code); 271 if ("final".equals(codeString)) 272 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.FINAL, code); 273 if ("amended".equals(codeString)) 274 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.AMENDED, code); 275 if ("corrected".equals(codeString)) 276 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.CORRECTED, code); 277 if ("cancelled".equals(codeString)) 278 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.CANCELLED, code); 279 if ("entered-in-error".equals(codeString)) 280 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.ENTEREDINERROR, code); 281 if ("unknown".equals(codeString)) 282 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.UNKNOWN, code); 283 throw new FHIRException("Unknown RiskAssessmentStatus code '" + codeString + "'"); 284 } 285 286 public String toCode(RiskAssessmentStatus code) { 287 if (code == RiskAssessmentStatus.NULL) 288 return null; 289 if (code == RiskAssessmentStatus.REGISTERED) 290 return "registered"; 291 if (code == RiskAssessmentStatus.PRELIMINARY) 292 return "preliminary"; 293 if (code == RiskAssessmentStatus.FINAL) 294 return "final"; 295 if (code == RiskAssessmentStatus.AMENDED) 296 return "amended"; 297 if (code == RiskAssessmentStatus.CORRECTED) 298 return "corrected"; 299 if (code == RiskAssessmentStatus.CANCELLED) 300 return "cancelled"; 301 if (code == RiskAssessmentStatus.ENTEREDINERROR) 302 return "entered-in-error"; 303 if (code == RiskAssessmentStatus.UNKNOWN) 304 return "unknown"; 305 return "?"; 306 } 307 308 public String toSystem(RiskAssessmentStatus code) { 309 return code.getSystem(); 310 } 311 } 312 313 @Block() 314 public static class RiskAssessmentPredictionComponent extends BackboneElement implements IBaseBackboneElement { 315 /** 316 * One of the potential outcomes for the patient (e.g. remission, death, a 317 * particular condition). 318 */ 319 @Child(name = "outcome", type = { 320 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 321 @Description(shortDefinition = "Possible outcome for the subject", formalDefinition = "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).") 322 protected CodeableConcept outcome; 323 324 /** 325 * Indicates how likely the outcome is (in the specified timeframe). 326 */ 327 @Child(name = "probability", type = { DecimalType.class, 328 Range.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 329 @Description(shortDefinition = "Likelihood of specified outcome", formalDefinition = "Indicates how likely the outcome is (in the specified timeframe).") 330 protected Type probability; 331 332 /** 333 * Indicates how likely the outcome is (in the specified timeframe), expressed 334 * as a qualitative value (e.g. low, medium, or high). 335 */ 336 @Child(name = "qualitativeRisk", type = { 337 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 338 @Description(shortDefinition = "Likelihood of specified outcome as a qualitative value", formalDefinition = "Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).") 339 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/risk-probability") 340 protected CodeableConcept qualitativeRisk; 341 342 /** 343 * Indicates the risk for this particular subject (with their specific 344 * characteristics) divided by the risk of the population in general. (Numbers 345 * greater than 1 = higher risk than the population, numbers less than 1 = lower 346 * risk.). 347 */ 348 @Child(name = "relativeRisk", type = { 349 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 350 @Description(shortDefinition = "Relative likelihood", formalDefinition = "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).") 351 protected DecimalType relativeRisk; 352 353 /** 354 * Indicates the period of time or age range of the subject to which the 355 * specified probability applies. 356 */ 357 @Child(name = "when", type = { Period.class, 358 Range.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 359 @Description(shortDefinition = "Timeframe or age range", formalDefinition = "Indicates the period of time or age range of the subject to which the specified probability applies.") 360 protected Type when; 361 362 /** 363 * Additional information explaining the basis for the prediction. 364 */ 365 @Child(name = "rationale", type = { 366 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 367 @Description(shortDefinition = "Explanation of prediction", formalDefinition = "Additional information explaining the basis for the prediction.") 368 protected StringType rationale; 369 370 private static final long serialVersionUID = 1283401747L; 371 372 /** 373 * Constructor 374 */ 375 public RiskAssessmentPredictionComponent() { 376 super(); 377 } 378 379 /** 380 * @return {@link #outcome} (One of the potential outcomes for the patient (e.g. 381 * remission, death, a particular condition).) 382 */ 383 public CodeableConcept getOutcome() { 384 if (this.outcome == null) 385 if (Configuration.errorOnAutoCreate()) 386 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.outcome"); 387 else if (Configuration.doAutoCreate()) 388 this.outcome = new CodeableConcept(); // cc 389 return this.outcome; 390 } 391 392 public boolean hasOutcome() { 393 return this.outcome != null && !this.outcome.isEmpty(); 394 } 395 396 /** 397 * @param value {@link #outcome} (One of the potential outcomes for the patient 398 * (e.g. remission, death, a particular condition).) 399 */ 400 public RiskAssessmentPredictionComponent setOutcome(CodeableConcept value) { 401 this.outcome = value; 402 return this; 403 } 404 405 /** 406 * @return {@link #probability} (Indicates how likely the outcome is (in the 407 * specified timeframe).) 408 */ 409 public Type getProbability() { 410 return this.probability; 411 } 412 413 /** 414 * @return {@link #probability} (Indicates how likely the outcome is (in the 415 * specified timeframe).) 416 */ 417 public DecimalType getProbabilityDecimalType() throws FHIRException { 418 if (this.probability == null) 419 this.probability = new DecimalType(); 420 if (!(this.probability instanceof DecimalType)) 421 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 422 + this.probability.getClass().getName() + " was encountered"); 423 return (DecimalType) this.probability; 424 } 425 426 public boolean hasProbabilityDecimalType() { 427 return this != null && this.probability instanceof DecimalType; 428 } 429 430 /** 431 * @return {@link #probability} (Indicates how likely the outcome is (in the 432 * specified timeframe).) 433 */ 434 public Range getProbabilityRange() throws FHIRException { 435 if (this.probability == null) 436 this.probability = new Range(); 437 if (!(this.probability instanceof Range)) 438 throw new FHIRException("Type mismatch: the type Range was expected, but " 439 + this.probability.getClass().getName() + " was encountered"); 440 return (Range) this.probability; 441 } 442 443 public boolean hasProbabilityRange() { 444 return this != null && this.probability instanceof Range; 445 } 446 447 public boolean hasProbability() { 448 return this.probability != null && !this.probability.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #probability} (Indicates how likely the outcome is (in 453 * the specified timeframe).) 454 */ 455 public RiskAssessmentPredictionComponent setProbability(Type value) { 456 if (value != null && !(value instanceof DecimalType || value instanceof Range)) 457 throw new Error("Not the right type for RiskAssessment.prediction.probability[x]: " + value.fhirType()); 458 this.probability = value; 459 return this; 460 } 461 462 /** 463 * @return {@link #qualitativeRisk} (Indicates how likely the outcome is (in the 464 * specified timeframe), expressed as a qualitative value (e.g. low, 465 * medium, or high).) 466 */ 467 public CodeableConcept getQualitativeRisk() { 468 if (this.qualitativeRisk == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.qualitativeRisk"); 471 else if (Configuration.doAutoCreate()) 472 this.qualitativeRisk = new CodeableConcept(); // cc 473 return this.qualitativeRisk; 474 } 475 476 public boolean hasQualitativeRisk() { 477 return this.qualitativeRisk != null && !this.qualitativeRisk.isEmpty(); 478 } 479 480 /** 481 * @param value {@link #qualitativeRisk} (Indicates how likely the outcome is 482 * (in the specified timeframe), expressed as a qualitative value 483 * (e.g. low, medium, or high).) 484 */ 485 public RiskAssessmentPredictionComponent setQualitativeRisk(CodeableConcept value) { 486 this.qualitativeRisk = value; 487 return this; 488 } 489 490 /** 491 * @return {@link #relativeRisk} (Indicates the risk for this particular subject 492 * (with their specific characteristics) divided by the risk of the 493 * population in general. (Numbers greater than 1 = higher risk than the 494 * population, numbers less than 1 = lower risk.).). This is the 495 * underlying object with id, value and extensions. The accessor 496 * "getRelativeRisk" gives direct access to the value 497 */ 498 public DecimalType getRelativeRiskElement() { 499 if (this.relativeRisk == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.relativeRisk"); 502 else if (Configuration.doAutoCreate()) 503 this.relativeRisk = new DecimalType(); // bb 504 return this.relativeRisk; 505 } 506 507 public boolean hasRelativeRiskElement() { 508 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 509 } 510 511 public boolean hasRelativeRisk() { 512 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 513 } 514 515 /** 516 * @param value {@link #relativeRisk} (Indicates the risk for this particular 517 * subject (with their specific characteristics) divided by the 518 * risk of the population in general. (Numbers greater than 1 = 519 * higher risk than the population, numbers less than 1 = lower 520 * risk.).). This is the underlying object with id, value and 521 * extensions. The accessor "getRelativeRisk" gives direct access 522 * to the value 523 */ 524 public RiskAssessmentPredictionComponent setRelativeRiskElement(DecimalType value) { 525 this.relativeRisk = value; 526 return this; 527 } 528 529 /** 530 * @return Indicates the risk for this particular subject (with their specific 531 * characteristics) divided by the risk of the population in general. 532 * (Numbers greater than 1 = higher risk than the population, numbers 533 * less than 1 = lower risk.). 534 */ 535 public BigDecimal getRelativeRisk() { 536 return this.relativeRisk == null ? null : this.relativeRisk.getValue(); 537 } 538 539 /** 540 * @param value Indicates the risk for this particular subject (with their 541 * specific characteristics) divided by the risk of the population 542 * in general. (Numbers greater than 1 = higher risk than the 543 * population, numbers less than 1 = lower risk.). 544 */ 545 public RiskAssessmentPredictionComponent setRelativeRisk(BigDecimal value) { 546 if (value == null) 547 this.relativeRisk = null; 548 else { 549 if (this.relativeRisk == null) 550 this.relativeRisk = new DecimalType(); 551 this.relativeRisk.setValue(value); 552 } 553 return this; 554 } 555 556 /** 557 * @param value Indicates the risk for this particular subject (with their 558 * specific characteristics) divided by the risk of the population 559 * in general. (Numbers greater than 1 = higher risk than the 560 * population, numbers less than 1 = lower risk.). 561 */ 562 public RiskAssessmentPredictionComponent setRelativeRisk(long value) { 563 this.relativeRisk = new DecimalType(); 564 this.relativeRisk.setValue(value); 565 return this; 566 } 567 568 /** 569 * @param value Indicates the risk for this particular subject (with their 570 * specific characteristics) divided by the risk of the population 571 * in general. (Numbers greater than 1 = higher risk than the 572 * population, numbers less than 1 = lower risk.). 573 */ 574 public RiskAssessmentPredictionComponent setRelativeRisk(double value) { 575 this.relativeRisk = new DecimalType(); 576 this.relativeRisk.setValue(value); 577 return this; 578 } 579 580 /** 581 * @return {@link #when} (Indicates the period of time or age range of the 582 * subject to which the specified probability applies.) 583 */ 584 public Type getWhen() { 585 return this.when; 586 } 587 588 /** 589 * @return {@link #when} (Indicates the period of time or age range of the 590 * subject to which the specified probability applies.) 591 */ 592 public Period getWhenPeriod() throws FHIRException { 593 if (this.when == null) 594 this.when = new Period(); 595 if (!(this.when instanceof Period)) 596 throw new FHIRException( 597 "Type mismatch: the type Period was expected, but " + this.when.getClass().getName() + " was encountered"); 598 return (Period) this.when; 599 } 600 601 public boolean hasWhenPeriod() { 602 return this != null && this.when instanceof Period; 603 } 604 605 /** 606 * @return {@link #when} (Indicates the period of time or age range of the 607 * subject to which the specified probability applies.) 608 */ 609 public Range getWhenRange() throws FHIRException { 610 if (this.when == null) 611 this.when = new Range(); 612 if (!(this.when instanceof Range)) 613 throw new FHIRException( 614 "Type mismatch: the type Range was expected, but " + this.when.getClass().getName() + " was encountered"); 615 return (Range) this.when; 616 } 617 618 public boolean hasWhenRange() { 619 return this != null && this.when instanceof Range; 620 } 621 622 public boolean hasWhen() { 623 return this.when != null && !this.when.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #when} (Indicates the period of time or age range of the 628 * subject to which the specified probability applies.) 629 */ 630 public RiskAssessmentPredictionComponent setWhen(Type value) { 631 if (value != null && !(value instanceof Period || value instanceof Range)) 632 throw new Error("Not the right type for RiskAssessment.prediction.when[x]: " + value.fhirType()); 633 this.when = value; 634 return this; 635 } 636 637 /** 638 * @return {@link #rationale} (Additional information explaining the basis for 639 * the prediction.). This is the underlying object with id, value and 640 * extensions. The accessor "getRationale" gives direct access to the 641 * value 642 */ 643 public StringType getRationaleElement() { 644 if (this.rationale == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.rationale"); 647 else if (Configuration.doAutoCreate()) 648 this.rationale = new StringType(); // bb 649 return this.rationale; 650 } 651 652 public boolean hasRationaleElement() { 653 return this.rationale != null && !this.rationale.isEmpty(); 654 } 655 656 public boolean hasRationale() { 657 return this.rationale != null && !this.rationale.isEmpty(); 658 } 659 660 /** 661 * @param value {@link #rationale} (Additional information explaining the basis 662 * for the prediction.). This is the underlying object with id, 663 * value and extensions. The accessor "getRationale" gives direct 664 * access to the value 665 */ 666 public RiskAssessmentPredictionComponent setRationaleElement(StringType value) { 667 this.rationale = value; 668 return this; 669 } 670 671 /** 672 * @return Additional information explaining the basis for the prediction. 673 */ 674 public String getRationale() { 675 return this.rationale == null ? null : this.rationale.getValue(); 676 } 677 678 /** 679 * @param value Additional information explaining the basis for the prediction. 680 */ 681 public RiskAssessmentPredictionComponent setRationale(String value) { 682 if (Utilities.noString(value)) 683 this.rationale = null; 684 else { 685 if (this.rationale == null) 686 this.rationale = new StringType(); 687 this.rationale.setValue(value); 688 } 689 return this; 690 } 691 692 protected void listChildren(List<Property> children) { 693 super.listChildren(children); 694 children.add(new Property("outcome", "CodeableConcept", 695 "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 1, 696 outcome)); 697 children.add(new Property("probability[x]", "decimal|Range", 698 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability)); 699 children.add(new Property("qualitativeRisk", "CodeableConcept", 700 "Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).", 701 0, 1, qualitativeRisk)); 702 children.add(new Property("relativeRisk", "decimal", 703 "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 704 0, 1, relativeRisk)); 705 children.add(new Property("when[x]", "Period|Range", 706 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, 707 when)); 708 children.add(new Property("rationale", "string", 709 "Additional information explaining the basis for the prediction.", 0, 1, rationale)); 710 } 711 712 @Override 713 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 714 switch (_hash) { 715 case -1106507950: 716 /* outcome */ return new Property("outcome", "CodeableConcept", 717 "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 1, 718 outcome); 719 case 1430185003: 720 /* probability[x] */ return new Property("probability[x]", "decimal|Range", 721 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 722 case -1290561483: 723 /* probability */ return new Property("probability[x]", "decimal|Range", 724 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 725 case 888495452: 726 /* probabilityDecimal */ return new Property("probability[x]", "decimal|Range", 727 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 728 case 9275912: 729 /* probabilityRange */ return new Property("probability[x]", "decimal|Range", 730 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 731 case 123308730: 732 /* qualitativeRisk */ return new Property("qualitativeRisk", "CodeableConcept", 733 "Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).", 734 0, 1, qualitativeRisk); 735 case -70741061: 736 /* relativeRisk */ return new Property("relativeRisk", "decimal", 737 "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 738 0, 1, relativeRisk); 739 case 1312831238: 740 /* when[x] */ return new Property("when[x]", "Period|Range", 741 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 742 1, when); 743 case 3648314: 744 /* when */ return new Property("when[x]", "Period|Range", 745 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 746 1, when); 747 case 251476379: 748 /* whenPeriod */ return new Property("when[x]", "Period|Range", 749 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 750 1, when); 751 case -1098542557: 752 /* whenRange */ return new Property("when[x]", "Period|Range", 753 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 754 1, when); 755 case 345689335: 756 /* rationale */ return new Property("rationale", "string", 757 "Additional information explaining the basis for the prediction.", 0, 1, rationale); 758 default: 759 return super.getNamedProperty(_hash, _name, _checkValid); 760 } 761 762 } 763 764 @Override 765 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 766 switch (hash) { 767 case -1106507950: 768 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // CodeableConcept 769 case -1290561483: 770 /* probability */ return this.probability == null ? new Base[0] : new Base[] { this.probability }; // Type 771 case 123308730: 772 /* qualitativeRisk */ return this.qualitativeRisk == null ? new Base[0] : new Base[] { this.qualitativeRisk }; // CodeableConcept 773 case -70741061: 774 /* relativeRisk */ return this.relativeRisk == null ? new Base[0] : new Base[] { this.relativeRisk }; // DecimalType 775 case 3648314: 776 /* when */ return this.when == null ? new Base[0] : new Base[] { this.when }; // Type 777 case 345689335: 778 /* rationale */ return this.rationale == null ? new Base[0] : new Base[] { this.rationale }; // StringType 779 default: 780 return super.getProperty(hash, name, checkValid); 781 } 782 783 } 784 785 @Override 786 public Base setProperty(int hash, String name, Base value) throws FHIRException { 787 switch (hash) { 788 case -1106507950: // outcome 789 this.outcome = castToCodeableConcept(value); // CodeableConcept 790 return value; 791 case -1290561483: // probability 792 this.probability = castToType(value); // Type 793 return value; 794 case 123308730: // qualitativeRisk 795 this.qualitativeRisk = castToCodeableConcept(value); // CodeableConcept 796 return value; 797 case -70741061: // relativeRisk 798 this.relativeRisk = castToDecimal(value); // DecimalType 799 return value; 800 case 3648314: // when 801 this.when = castToType(value); // Type 802 return value; 803 case 345689335: // rationale 804 this.rationale = castToString(value); // StringType 805 return value; 806 default: 807 return super.setProperty(hash, name, value); 808 } 809 810 } 811 812 @Override 813 public Base setProperty(String name, Base value) throws FHIRException { 814 if (name.equals("outcome")) { 815 this.outcome = castToCodeableConcept(value); // CodeableConcept 816 } else if (name.equals("probability[x]")) { 817 this.probability = castToType(value); // Type 818 } else if (name.equals("qualitativeRisk")) { 819 this.qualitativeRisk = castToCodeableConcept(value); // CodeableConcept 820 } else if (name.equals("relativeRisk")) { 821 this.relativeRisk = castToDecimal(value); // DecimalType 822 } else if (name.equals("when[x]")) { 823 this.when = castToType(value); // Type 824 } else if (name.equals("rationale")) { 825 this.rationale = castToString(value); // StringType 826 } else 827 return super.setProperty(name, value); 828 return value; 829 } 830 831 @Override 832 public void removeChild(String name, Base value) throws FHIRException { 833 if (name.equals("outcome")) { 834 this.outcome = null; 835 } else if (name.equals("probability[x]")) { 836 this.probability = null; 837 } else if (name.equals("qualitativeRisk")) { 838 this.qualitativeRisk = null; 839 } else if (name.equals("relativeRisk")) { 840 this.relativeRisk = null; 841 } else if (name.equals("when[x]")) { 842 this.when = null; 843 } else if (name.equals("rationale")) { 844 this.rationale = null; 845 } else 846 super.removeChild(name, value); 847 848 } 849 850 @Override 851 public Base makeProperty(int hash, String name) throws FHIRException { 852 switch (hash) { 853 case -1106507950: 854 return getOutcome(); 855 case 1430185003: 856 return getProbability(); 857 case -1290561483: 858 return getProbability(); 859 case 123308730: 860 return getQualitativeRisk(); 861 case -70741061: 862 return getRelativeRiskElement(); 863 case 1312831238: 864 return getWhen(); 865 case 3648314: 866 return getWhen(); 867 case 345689335: 868 return getRationaleElement(); 869 default: 870 return super.makeProperty(hash, name); 871 } 872 873 } 874 875 @Override 876 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 877 switch (hash) { 878 case -1106507950: 879 /* outcome */ return new String[] { "CodeableConcept" }; 880 case -1290561483: 881 /* probability */ return new String[] { "decimal", "Range" }; 882 case 123308730: 883 /* qualitativeRisk */ return new String[] { "CodeableConcept" }; 884 case -70741061: 885 /* relativeRisk */ return new String[] { "decimal" }; 886 case 3648314: 887 /* when */ return new String[] { "Period", "Range" }; 888 case 345689335: 889 /* rationale */ return new String[] { "string" }; 890 default: 891 return super.getTypesForProperty(hash, name); 892 } 893 894 } 895 896 @Override 897 public Base addChild(String name) throws FHIRException { 898 if (name.equals("outcome")) { 899 this.outcome = new CodeableConcept(); 900 return this.outcome; 901 } else if (name.equals("probabilityDecimal")) { 902 this.probability = new DecimalType(); 903 return this.probability; 904 } else if (name.equals("probabilityRange")) { 905 this.probability = new Range(); 906 return this.probability; 907 } else if (name.equals("qualitativeRisk")) { 908 this.qualitativeRisk = new CodeableConcept(); 909 return this.qualitativeRisk; 910 } else if (name.equals("relativeRisk")) { 911 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.relativeRisk"); 912 } else if (name.equals("whenPeriod")) { 913 this.when = new Period(); 914 return this.when; 915 } else if (name.equals("whenRange")) { 916 this.when = new Range(); 917 return this.when; 918 } else if (name.equals("rationale")) { 919 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.rationale"); 920 } else 921 return super.addChild(name); 922 } 923 924 public RiskAssessmentPredictionComponent copy() { 925 RiskAssessmentPredictionComponent dst = new RiskAssessmentPredictionComponent(); 926 copyValues(dst); 927 return dst; 928 } 929 930 public void copyValues(RiskAssessmentPredictionComponent dst) { 931 super.copyValues(dst); 932 dst.outcome = outcome == null ? null : outcome.copy(); 933 dst.probability = probability == null ? null : probability.copy(); 934 dst.qualitativeRisk = qualitativeRisk == null ? null : qualitativeRisk.copy(); 935 dst.relativeRisk = relativeRisk == null ? null : relativeRisk.copy(); 936 dst.when = when == null ? null : when.copy(); 937 dst.rationale = rationale == null ? null : rationale.copy(); 938 } 939 940 @Override 941 public boolean equalsDeep(Base other_) { 942 if (!super.equalsDeep(other_)) 943 return false; 944 if (!(other_ instanceof RiskAssessmentPredictionComponent)) 945 return false; 946 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other_; 947 return compareDeep(outcome, o.outcome, true) && compareDeep(probability, o.probability, true) 948 && compareDeep(qualitativeRisk, o.qualitativeRisk, true) && compareDeep(relativeRisk, o.relativeRisk, true) 949 && compareDeep(when, o.when, true) && compareDeep(rationale, o.rationale, true); 950 } 951 952 @Override 953 public boolean equalsShallow(Base other_) { 954 if (!super.equalsShallow(other_)) 955 return false; 956 if (!(other_ instanceof RiskAssessmentPredictionComponent)) 957 return false; 958 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other_; 959 return compareValues(relativeRisk, o.relativeRisk, true) && compareValues(rationale, o.rationale, true); 960 } 961 962 public boolean isEmpty() { 963 return super.isEmpty() 964 && ca.uhn.fhir.util.ElementUtil.isEmpty(outcome, probability, qualitativeRisk, relativeRisk, when, rationale); 965 } 966 967 public String fhirType() { 968 return "RiskAssessment.prediction"; 969 970 } 971 972 } 973 974 /** 975 * Business identifier assigned to the risk assessment. 976 */ 977 @Child(name = "identifier", type = { 978 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 979 @Description(shortDefinition = "Unique identifier for the assessment", formalDefinition = "Business identifier assigned to the risk assessment.") 980 protected List<Identifier> identifier; 981 982 /** 983 * A reference to the request that is fulfilled by this risk assessment. 984 */ 985 @Child(name = "basedOn", type = { Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 986 @Description(shortDefinition = "Request fulfilled by this assessment", formalDefinition = "A reference to the request that is fulfilled by this risk assessment.") 987 protected Reference basedOn; 988 989 /** 990 * The actual object that is the target of the reference (A reference to the 991 * request that is fulfilled by this risk assessment.) 992 */ 993 protected Resource basedOnTarget; 994 995 /** 996 * A reference to a resource that this risk assessment is part of, such as a 997 * Procedure. 998 */ 999 @Child(name = "parent", type = { Reference.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1000 @Description(shortDefinition = "Part of this occurrence", formalDefinition = "A reference to a resource that this risk assessment is part of, such as a Procedure.") 1001 protected Reference parent; 1002 1003 /** 1004 * The actual object that is the target of the reference (A reference to a 1005 * resource that this risk assessment is part of, such as a Procedure.) 1006 */ 1007 protected Resource parentTarget; 1008 1009 /** 1010 * The status of the RiskAssessment, using the same statuses as an Observation. 1011 */ 1012 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1013 @Description(shortDefinition = "registered | preliminary | final | amended +", formalDefinition = "The status of the RiskAssessment, using the same statuses as an Observation.") 1014 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-status") 1015 protected Enumeration<RiskAssessmentStatus> status; 1016 1017 /** 1018 * The algorithm, process or mechanism used to evaluate the risk. 1019 */ 1020 @Child(name = "method", type = { 1021 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1022 @Description(shortDefinition = "Evaluation mechanism", formalDefinition = "The algorithm, process or mechanism used to evaluate the risk.") 1023 protected CodeableConcept method; 1024 1025 /** 1026 * The type of the risk assessment performed. 1027 */ 1028 @Child(name = "code", type = { CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1029 @Description(shortDefinition = "Type of assessment", formalDefinition = "The type of the risk assessment performed.") 1030 protected CodeableConcept code; 1031 1032 /** 1033 * The patient or group the risk assessment applies to. 1034 */ 1035 @Child(name = "subject", type = { Patient.class, 1036 Group.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1037 @Description(shortDefinition = "Who/what does assessment apply to?", formalDefinition = "The patient or group the risk assessment applies to.") 1038 protected Reference subject; 1039 1040 /** 1041 * The actual object that is the target of the reference (The patient or group 1042 * the risk assessment applies to.) 1043 */ 1044 protected Resource subjectTarget; 1045 1046 /** 1047 * The encounter where the assessment was performed. 1048 */ 1049 @Child(name = "encounter", type = { Encounter.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1050 @Description(shortDefinition = "Where was assessment performed?", formalDefinition = "The encounter where the assessment was performed.") 1051 protected Reference encounter; 1052 1053 /** 1054 * The actual object that is the target of the reference (The encounter where 1055 * the assessment was performed.) 1056 */ 1057 protected Encounter encounterTarget; 1058 1059 /** 1060 * The date (and possibly time) the risk assessment was performed. 1061 */ 1062 @Child(name = "occurrence", type = { DateTimeType.class, 1063 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1064 @Description(shortDefinition = "When was assessment made?", formalDefinition = "The date (and possibly time) the risk assessment was performed.") 1065 protected Type occurrence; 1066 1067 /** 1068 * For assessments or prognosis specific to a particular condition, indicates 1069 * the condition being assessed. 1070 */ 1071 @Child(name = "condition", type = { Condition.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1072 @Description(shortDefinition = "Condition assessed", formalDefinition = "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.") 1073 protected Reference condition; 1074 1075 /** 1076 * The actual object that is the target of the reference (For assessments or 1077 * prognosis specific to a particular condition, indicates the condition being 1078 * assessed.) 1079 */ 1080 protected Condition conditionTarget; 1081 1082 /** 1083 * The provider or software application that performed the assessment. 1084 */ 1085 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, 1086 Device.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1087 @Description(shortDefinition = "Who did assessment?", formalDefinition = "The provider or software application that performed the assessment.") 1088 protected Reference performer; 1089 1090 /** 1091 * The actual object that is the target of the reference (The provider or 1092 * software application that performed the assessment.) 1093 */ 1094 protected Resource performerTarget; 1095 1096 /** 1097 * The reason the risk assessment was performed. 1098 */ 1099 @Child(name = "reasonCode", type = { 1100 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1101 @Description(shortDefinition = "Why the assessment was necessary?", formalDefinition = "The reason the risk assessment was performed.") 1102 protected List<CodeableConcept> reasonCode; 1103 1104 /** 1105 * Resources supporting the reason the risk assessment was performed. 1106 */ 1107 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 1108 DocumentReference.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1109 @Description(shortDefinition = "Why the assessment was necessary?", formalDefinition = "Resources supporting the reason the risk assessment was performed.") 1110 protected List<Reference> reasonReference; 1111 /** 1112 * The actual objects that are the target of the reference (Resources supporting 1113 * the reason the risk assessment was performed.) 1114 */ 1115 protected List<Resource> reasonReferenceTarget; 1116 1117 /** 1118 * Indicates the source data considered as part of the assessment (for example, 1119 * FamilyHistory, Observations, Procedures, Conditions, etc.). 1120 */ 1121 @Child(name = "basis", type = { 1122 Reference.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1123 @Description(shortDefinition = "Information used in assessment", formalDefinition = "Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).") 1124 protected List<Reference> basis; 1125 /** 1126 * The actual objects that are the target of the reference (Indicates the source 1127 * data considered as part of the assessment (for example, FamilyHistory, 1128 * Observations, Procedures, Conditions, etc.).) 1129 */ 1130 protected List<Resource> basisTarget; 1131 1132 /** 1133 * Describes the expected outcome for the subject. 1134 */ 1135 @Child(name = "prediction", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1136 @Description(shortDefinition = "Outcome predicted", formalDefinition = "Describes the expected outcome for the subject.") 1137 protected List<RiskAssessmentPredictionComponent> prediction; 1138 1139 /** 1140 * A description of the steps that might be taken to reduce the identified 1141 * risk(s). 1142 */ 1143 @Child(name = "mitigation", type = { 1144 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1145 @Description(shortDefinition = "How to reduce risk", formalDefinition = "A description of the steps that might be taken to reduce the identified risk(s).") 1146 protected StringType mitigation; 1147 1148 /** 1149 * Additional comments about the risk assessment. 1150 */ 1151 @Child(name = "note", type = { 1152 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1153 @Description(shortDefinition = "Comments on the risk assessment", formalDefinition = "Additional comments about the risk assessment.") 1154 protected List<Annotation> note; 1155 1156 private static final long serialVersionUID = -2137260218L; 1157 1158 /** 1159 * Constructor 1160 */ 1161 public RiskAssessment() { 1162 super(); 1163 } 1164 1165 /** 1166 * Constructor 1167 */ 1168 public RiskAssessment(Enumeration<RiskAssessmentStatus> status, Reference subject) { 1169 super(); 1170 this.status = status; 1171 this.subject = subject; 1172 } 1173 1174 /** 1175 * @return {@link #identifier} (Business identifier assigned to the risk 1176 * assessment.) 1177 */ 1178 public List<Identifier> getIdentifier() { 1179 if (this.identifier == null) 1180 this.identifier = new ArrayList<Identifier>(); 1181 return this.identifier; 1182 } 1183 1184 /** 1185 * @return Returns a reference to <code>this</code> for easy method chaining 1186 */ 1187 public RiskAssessment setIdentifier(List<Identifier> theIdentifier) { 1188 this.identifier = theIdentifier; 1189 return this; 1190 } 1191 1192 public boolean hasIdentifier() { 1193 if (this.identifier == null) 1194 return false; 1195 for (Identifier item : this.identifier) 1196 if (!item.isEmpty()) 1197 return true; 1198 return false; 1199 } 1200 1201 public Identifier addIdentifier() { // 3 1202 Identifier t = new Identifier(); 1203 if (this.identifier == null) 1204 this.identifier = new ArrayList<Identifier>(); 1205 this.identifier.add(t); 1206 return t; 1207 } 1208 1209 public RiskAssessment addIdentifier(Identifier t) { // 3 1210 if (t == null) 1211 return this; 1212 if (this.identifier == null) 1213 this.identifier = new ArrayList<Identifier>(); 1214 this.identifier.add(t); 1215 return this; 1216 } 1217 1218 /** 1219 * @return The first repetition of repeating field {@link #identifier}, creating 1220 * it if it does not already exist 1221 */ 1222 public Identifier getIdentifierFirstRep() { 1223 if (getIdentifier().isEmpty()) { 1224 addIdentifier(); 1225 } 1226 return getIdentifier().get(0); 1227 } 1228 1229 /** 1230 * @return {@link #basedOn} (A reference to the request that is fulfilled by 1231 * this risk assessment.) 1232 */ 1233 public Reference getBasedOn() { 1234 if (this.basedOn == null) 1235 if (Configuration.errorOnAutoCreate()) 1236 throw new Error("Attempt to auto-create RiskAssessment.basedOn"); 1237 else if (Configuration.doAutoCreate()) 1238 this.basedOn = new Reference(); // cc 1239 return this.basedOn; 1240 } 1241 1242 public boolean hasBasedOn() { 1243 return this.basedOn != null && !this.basedOn.isEmpty(); 1244 } 1245 1246 /** 1247 * @param value {@link #basedOn} (A reference to the request that is fulfilled 1248 * by this risk assessment.) 1249 */ 1250 public RiskAssessment setBasedOn(Reference value) { 1251 this.basedOn = value; 1252 return this; 1253 } 1254 1255 /** 1256 * @return {@link #basedOn} The actual object that is the target of the 1257 * reference. The reference library doesn't populate this, but you can 1258 * use it to hold the resource if you resolve it. (A reference to the 1259 * request that is fulfilled by this risk assessment.) 1260 */ 1261 public Resource getBasedOnTarget() { 1262 return this.basedOnTarget; 1263 } 1264 1265 /** 1266 * @param value {@link #basedOn} The actual object that is the target of the 1267 * reference. The reference library doesn't use these, but you can 1268 * use it to hold the resource if you resolve it. (A reference to 1269 * the request that is fulfilled by this risk assessment.) 1270 */ 1271 public RiskAssessment setBasedOnTarget(Resource value) { 1272 this.basedOnTarget = value; 1273 return this; 1274 } 1275 1276 /** 1277 * @return {@link #parent} (A reference to a resource that this risk assessment 1278 * is part of, such as a Procedure.) 1279 */ 1280 public Reference getParent() { 1281 if (this.parent == null) 1282 if (Configuration.errorOnAutoCreate()) 1283 throw new Error("Attempt to auto-create RiskAssessment.parent"); 1284 else if (Configuration.doAutoCreate()) 1285 this.parent = new Reference(); // cc 1286 return this.parent; 1287 } 1288 1289 public boolean hasParent() { 1290 return this.parent != null && !this.parent.isEmpty(); 1291 } 1292 1293 /** 1294 * @param value {@link #parent} (A reference to a resource that this risk 1295 * assessment is part of, such as a Procedure.) 1296 */ 1297 public RiskAssessment setParent(Reference value) { 1298 this.parent = value; 1299 return this; 1300 } 1301 1302 /** 1303 * @return {@link #parent} The actual object that is the target of the 1304 * reference. The reference library doesn't populate this, but you can 1305 * use it to hold the resource if you resolve it. (A reference to a 1306 * resource that this risk assessment is part of, such as a Procedure.) 1307 */ 1308 public Resource getParentTarget() { 1309 return this.parentTarget; 1310 } 1311 1312 /** 1313 * @param value {@link #parent} The actual object that is the target of the 1314 * reference. The reference library doesn't use these, but you can 1315 * use it to hold the resource if you resolve it. (A reference to a 1316 * resource that this risk assessment is part of, such as a 1317 * Procedure.) 1318 */ 1319 public RiskAssessment setParentTarget(Resource value) { 1320 this.parentTarget = value; 1321 return this; 1322 } 1323 1324 /** 1325 * @return {@link #status} (The status of the RiskAssessment, using the same 1326 * statuses as an Observation.). This is the underlying object with id, 1327 * value and extensions. The accessor "getStatus" gives direct access to 1328 * the value 1329 */ 1330 public Enumeration<RiskAssessmentStatus> getStatusElement() { 1331 if (this.status == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create RiskAssessment.status"); 1334 else if (Configuration.doAutoCreate()) 1335 this.status = new Enumeration<RiskAssessmentStatus>(new RiskAssessmentStatusEnumFactory()); // bb 1336 return this.status; 1337 } 1338 1339 public boolean hasStatusElement() { 1340 return this.status != null && !this.status.isEmpty(); 1341 } 1342 1343 public boolean hasStatus() { 1344 return this.status != null && !this.status.isEmpty(); 1345 } 1346 1347 /** 1348 * @param value {@link #status} (The status of the RiskAssessment, using the 1349 * same statuses as an Observation.). This is the underlying object 1350 * with id, value and extensions. The accessor "getStatus" gives 1351 * direct access to the value 1352 */ 1353 public RiskAssessment setStatusElement(Enumeration<RiskAssessmentStatus> value) { 1354 this.status = value; 1355 return this; 1356 } 1357 1358 /** 1359 * @return The status of the RiskAssessment, using the same statuses as an 1360 * Observation. 1361 */ 1362 public RiskAssessmentStatus getStatus() { 1363 return this.status == null ? null : this.status.getValue(); 1364 } 1365 1366 /** 1367 * @param value The status of the RiskAssessment, using the same statuses as an 1368 * Observation. 1369 */ 1370 public RiskAssessment setStatus(RiskAssessmentStatus value) { 1371 if (this.status == null) 1372 this.status = new Enumeration<RiskAssessmentStatus>(new RiskAssessmentStatusEnumFactory()); 1373 this.status.setValue(value); 1374 return this; 1375 } 1376 1377 /** 1378 * @return {@link #method} (The algorithm, process or mechanism used to evaluate 1379 * the risk.) 1380 */ 1381 public CodeableConcept getMethod() { 1382 if (this.method == null) 1383 if (Configuration.errorOnAutoCreate()) 1384 throw new Error("Attempt to auto-create RiskAssessment.method"); 1385 else if (Configuration.doAutoCreate()) 1386 this.method = new CodeableConcept(); // cc 1387 return this.method; 1388 } 1389 1390 public boolean hasMethod() { 1391 return this.method != null && !this.method.isEmpty(); 1392 } 1393 1394 /** 1395 * @param value {@link #method} (The algorithm, process or mechanism used to 1396 * evaluate the risk.) 1397 */ 1398 public RiskAssessment setMethod(CodeableConcept value) { 1399 this.method = value; 1400 return this; 1401 } 1402 1403 /** 1404 * @return {@link #code} (The type of the risk assessment performed.) 1405 */ 1406 public CodeableConcept getCode() { 1407 if (this.code == null) 1408 if (Configuration.errorOnAutoCreate()) 1409 throw new Error("Attempt to auto-create RiskAssessment.code"); 1410 else if (Configuration.doAutoCreate()) 1411 this.code = new CodeableConcept(); // cc 1412 return this.code; 1413 } 1414 1415 public boolean hasCode() { 1416 return this.code != null && !this.code.isEmpty(); 1417 } 1418 1419 /** 1420 * @param value {@link #code} (The type of the risk assessment performed.) 1421 */ 1422 public RiskAssessment setCode(CodeableConcept value) { 1423 this.code = value; 1424 return this; 1425 } 1426 1427 /** 1428 * @return {@link #subject} (The patient or group the risk assessment applies 1429 * to.) 1430 */ 1431 public Reference getSubject() { 1432 if (this.subject == null) 1433 if (Configuration.errorOnAutoCreate()) 1434 throw new Error("Attempt to auto-create RiskAssessment.subject"); 1435 else if (Configuration.doAutoCreate()) 1436 this.subject = new Reference(); // cc 1437 return this.subject; 1438 } 1439 1440 public boolean hasSubject() { 1441 return this.subject != null && !this.subject.isEmpty(); 1442 } 1443 1444 /** 1445 * @param value {@link #subject} (The patient or group the risk assessment 1446 * applies to.) 1447 */ 1448 public RiskAssessment setSubject(Reference value) { 1449 this.subject = value; 1450 return this; 1451 } 1452 1453 /** 1454 * @return {@link #subject} The actual object that is the target of the 1455 * reference. The reference library doesn't populate this, but you can 1456 * use it to hold the resource if you resolve it. (The patient or group 1457 * the risk assessment applies to.) 1458 */ 1459 public Resource getSubjectTarget() { 1460 return this.subjectTarget; 1461 } 1462 1463 /** 1464 * @param value {@link #subject} The actual object that is the target of the 1465 * reference. The reference library doesn't use these, but you can 1466 * use it to hold the resource if you resolve it. (The patient or 1467 * group the risk assessment applies to.) 1468 */ 1469 public RiskAssessment setSubjectTarget(Resource value) { 1470 this.subjectTarget = value; 1471 return this; 1472 } 1473 1474 /** 1475 * @return {@link #encounter} (The encounter where the assessment was 1476 * performed.) 1477 */ 1478 public Reference getEncounter() { 1479 if (this.encounter == null) 1480 if (Configuration.errorOnAutoCreate()) 1481 throw new Error("Attempt to auto-create RiskAssessment.encounter"); 1482 else if (Configuration.doAutoCreate()) 1483 this.encounter = new Reference(); // cc 1484 return this.encounter; 1485 } 1486 1487 public boolean hasEncounter() { 1488 return this.encounter != null && !this.encounter.isEmpty(); 1489 } 1490 1491 /** 1492 * @param value {@link #encounter} (The encounter where the assessment was 1493 * performed.) 1494 */ 1495 public RiskAssessment setEncounter(Reference value) { 1496 this.encounter = value; 1497 return this; 1498 } 1499 1500 /** 1501 * @return {@link #encounter} The actual object that is the target of the 1502 * reference. The reference library doesn't populate this, but you can 1503 * use it to hold the resource if you resolve it. (The encounter where 1504 * the assessment was performed.) 1505 */ 1506 public Encounter getEncounterTarget() { 1507 if (this.encounterTarget == null) 1508 if (Configuration.errorOnAutoCreate()) 1509 throw new Error("Attempt to auto-create RiskAssessment.encounter"); 1510 else if (Configuration.doAutoCreate()) 1511 this.encounterTarget = new Encounter(); // aa 1512 return this.encounterTarget; 1513 } 1514 1515 /** 1516 * @param value {@link #encounter} The actual object that is the target of the 1517 * reference. The reference library doesn't use these, but you can 1518 * use it to hold the resource if you resolve it. (The encounter 1519 * where the assessment was performed.) 1520 */ 1521 public RiskAssessment setEncounterTarget(Encounter value) { 1522 this.encounterTarget = value; 1523 return this; 1524 } 1525 1526 /** 1527 * @return {@link #occurrence} (The date (and possibly time) the risk assessment 1528 * was performed.) 1529 */ 1530 public Type getOccurrence() { 1531 return this.occurrence; 1532 } 1533 1534 /** 1535 * @return {@link #occurrence} (The date (and possibly time) the risk assessment 1536 * was performed.) 1537 */ 1538 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1539 if (this.occurrence == null) 1540 this.occurrence = new DateTimeType(); 1541 if (!(this.occurrence instanceof DateTimeType)) 1542 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1543 + this.occurrence.getClass().getName() + " was encountered"); 1544 return (DateTimeType) this.occurrence; 1545 } 1546 1547 public boolean hasOccurrenceDateTimeType() { 1548 return this != null && this.occurrence instanceof DateTimeType; 1549 } 1550 1551 /** 1552 * @return {@link #occurrence} (The date (and possibly time) the risk assessment 1553 * was performed.) 1554 */ 1555 public Period getOccurrencePeriod() throws FHIRException { 1556 if (this.occurrence == null) 1557 this.occurrence = new Period(); 1558 if (!(this.occurrence instanceof Period)) 1559 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1560 + " was encountered"); 1561 return (Period) this.occurrence; 1562 } 1563 1564 public boolean hasOccurrencePeriod() { 1565 return this != null && this.occurrence instanceof Period; 1566 } 1567 1568 public boolean hasOccurrence() { 1569 return this.occurrence != null && !this.occurrence.isEmpty(); 1570 } 1571 1572 /** 1573 * @param value {@link #occurrence} (The date (and possibly time) the risk 1574 * assessment was performed.) 1575 */ 1576 public RiskAssessment setOccurrence(Type value) { 1577 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1578 throw new Error("Not the right type for RiskAssessment.occurrence[x]: " + value.fhirType()); 1579 this.occurrence = value; 1580 return this; 1581 } 1582 1583 /** 1584 * @return {@link #condition} (For assessments or prognosis specific to a 1585 * particular condition, indicates the condition being assessed.) 1586 */ 1587 public Reference getCondition() { 1588 if (this.condition == null) 1589 if (Configuration.errorOnAutoCreate()) 1590 throw new Error("Attempt to auto-create RiskAssessment.condition"); 1591 else if (Configuration.doAutoCreate()) 1592 this.condition = new Reference(); // cc 1593 return this.condition; 1594 } 1595 1596 public boolean hasCondition() { 1597 return this.condition != null && !this.condition.isEmpty(); 1598 } 1599 1600 /** 1601 * @param value {@link #condition} (For assessments or prognosis specific to a 1602 * particular condition, indicates the condition being assessed.) 1603 */ 1604 public RiskAssessment setCondition(Reference value) { 1605 this.condition = value; 1606 return this; 1607 } 1608 1609 /** 1610 * @return {@link #condition} The actual object that is the target of the 1611 * reference. The reference library doesn't populate this, but you can 1612 * use it to hold the resource if you resolve it. (For assessments or 1613 * prognosis specific to a particular condition, indicates the condition 1614 * being assessed.) 1615 */ 1616 public Condition getConditionTarget() { 1617 if (this.conditionTarget == null) 1618 if (Configuration.errorOnAutoCreate()) 1619 throw new Error("Attempt to auto-create RiskAssessment.condition"); 1620 else if (Configuration.doAutoCreate()) 1621 this.conditionTarget = new Condition(); // aa 1622 return this.conditionTarget; 1623 } 1624 1625 /** 1626 * @param value {@link #condition} The actual object that is the target of the 1627 * reference. The reference library doesn't use these, but you can 1628 * use it to hold the resource if you resolve it. (For assessments 1629 * or prognosis specific to a particular condition, indicates the 1630 * condition being assessed.) 1631 */ 1632 public RiskAssessment setConditionTarget(Condition value) { 1633 this.conditionTarget = value; 1634 return this; 1635 } 1636 1637 /** 1638 * @return {@link #performer} (The provider or software application that 1639 * performed the assessment.) 1640 */ 1641 public Reference getPerformer() { 1642 if (this.performer == null) 1643 if (Configuration.errorOnAutoCreate()) 1644 throw new Error("Attempt to auto-create RiskAssessment.performer"); 1645 else if (Configuration.doAutoCreate()) 1646 this.performer = new Reference(); // cc 1647 return this.performer; 1648 } 1649 1650 public boolean hasPerformer() { 1651 return this.performer != null && !this.performer.isEmpty(); 1652 } 1653 1654 /** 1655 * @param value {@link #performer} (The provider or software application that 1656 * performed the assessment.) 1657 */ 1658 public RiskAssessment setPerformer(Reference value) { 1659 this.performer = value; 1660 return this; 1661 } 1662 1663 /** 1664 * @return {@link #performer} The actual object that is the target of the 1665 * reference. The reference library doesn't populate this, but you can 1666 * use it to hold the resource if you resolve it. (The provider or 1667 * software application that performed the assessment.) 1668 */ 1669 public Resource getPerformerTarget() { 1670 return this.performerTarget; 1671 } 1672 1673 /** 1674 * @param value {@link #performer} The actual object that is the target of the 1675 * reference. The reference library doesn't use these, but you can 1676 * use it to hold the resource if you resolve it. (The provider or 1677 * software application that performed the assessment.) 1678 */ 1679 public RiskAssessment setPerformerTarget(Resource value) { 1680 this.performerTarget = value; 1681 return this; 1682 } 1683 1684 /** 1685 * @return {@link #reasonCode} (The reason the risk assessment was performed.) 1686 */ 1687 public List<CodeableConcept> getReasonCode() { 1688 if (this.reasonCode == null) 1689 this.reasonCode = new ArrayList<CodeableConcept>(); 1690 return this.reasonCode; 1691 } 1692 1693 /** 1694 * @return Returns a reference to <code>this</code> for easy method chaining 1695 */ 1696 public RiskAssessment setReasonCode(List<CodeableConcept> theReasonCode) { 1697 this.reasonCode = theReasonCode; 1698 return this; 1699 } 1700 1701 public boolean hasReasonCode() { 1702 if (this.reasonCode == null) 1703 return false; 1704 for (CodeableConcept item : this.reasonCode) 1705 if (!item.isEmpty()) 1706 return true; 1707 return false; 1708 } 1709 1710 public CodeableConcept addReasonCode() { // 3 1711 CodeableConcept t = new CodeableConcept(); 1712 if (this.reasonCode == null) 1713 this.reasonCode = new ArrayList<CodeableConcept>(); 1714 this.reasonCode.add(t); 1715 return t; 1716 } 1717 1718 public RiskAssessment addReasonCode(CodeableConcept t) { // 3 1719 if (t == null) 1720 return this; 1721 if (this.reasonCode == null) 1722 this.reasonCode = new ArrayList<CodeableConcept>(); 1723 this.reasonCode.add(t); 1724 return this; 1725 } 1726 1727 /** 1728 * @return The first repetition of repeating field {@link #reasonCode}, creating 1729 * it if it does not already exist 1730 */ 1731 public CodeableConcept getReasonCodeFirstRep() { 1732 if (getReasonCode().isEmpty()) { 1733 addReasonCode(); 1734 } 1735 return getReasonCode().get(0); 1736 } 1737 1738 /** 1739 * @return {@link #reasonReference} (Resources supporting the reason the risk 1740 * assessment was performed.) 1741 */ 1742 public List<Reference> getReasonReference() { 1743 if (this.reasonReference == null) 1744 this.reasonReference = new ArrayList<Reference>(); 1745 return this.reasonReference; 1746 } 1747 1748 /** 1749 * @return Returns a reference to <code>this</code> for easy method chaining 1750 */ 1751 public RiskAssessment setReasonReference(List<Reference> theReasonReference) { 1752 this.reasonReference = theReasonReference; 1753 return this; 1754 } 1755 1756 public boolean hasReasonReference() { 1757 if (this.reasonReference == null) 1758 return false; 1759 for (Reference item : this.reasonReference) 1760 if (!item.isEmpty()) 1761 return true; 1762 return false; 1763 } 1764 1765 public Reference addReasonReference() { // 3 1766 Reference t = new Reference(); 1767 if (this.reasonReference == null) 1768 this.reasonReference = new ArrayList<Reference>(); 1769 this.reasonReference.add(t); 1770 return t; 1771 } 1772 1773 public RiskAssessment addReasonReference(Reference t) { // 3 1774 if (t == null) 1775 return this; 1776 if (this.reasonReference == null) 1777 this.reasonReference = new ArrayList<Reference>(); 1778 this.reasonReference.add(t); 1779 return this; 1780 } 1781 1782 /** 1783 * @return The first repetition of repeating field {@link #reasonReference}, 1784 * creating it if it does not already exist 1785 */ 1786 public Reference getReasonReferenceFirstRep() { 1787 if (getReasonReference().isEmpty()) { 1788 addReasonReference(); 1789 } 1790 return getReasonReference().get(0); 1791 } 1792 1793 /** 1794 * @deprecated Use Reference#setResource(IBaseResource) instead 1795 */ 1796 @Deprecated 1797 public List<Resource> getReasonReferenceTarget() { 1798 if (this.reasonReferenceTarget == null) 1799 this.reasonReferenceTarget = new ArrayList<Resource>(); 1800 return this.reasonReferenceTarget; 1801 } 1802 1803 /** 1804 * @return {@link #basis} (Indicates the source data considered as part of the 1805 * assessment (for example, FamilyHistory, Observations, Procedures, 1806 * Conditions, etc.).) 1807 */ 1808 public List<Reference> getBasis() { 1809 if (this.basis == null) 1810 this.basis = new ArrayList<Reference>(); 1811 return this.basis; 1812 } 1813 1814 /** 1815 * @return Returns a reference to <code>this</code> for easy method chaining 1816 */ 1817 public RiskAssessment setBasis(List<Reference> theBasis) { 1818 this.basis = theBasis; 1819 return this; 1820 } 1821 1822 public boolean hasBasis() { 1823 if (this.basis == null) 1824 return false; 1825 for (Reference item : this.basis) 1826 if (!item.isEmpty()) 1827 return true; 1828 return false; 1829 } 1830 1831 public Reference addBasis() { // 3 1832 Reference t = new Reference(); 1833 if (this.basis == null) 1834 this.basis = new ArrayList<Reference>(); 1835 this.basis.add(t); 1836 return t; 1837 } 1838 1839 public RiskAssessment addBasis(Reference t) { // 3 1840 if (t == null) 1841 return this; 1842 if (this.basis == null) 1843 this.basis = new ArrayList<Reference>(); 1844 this.basis.add(t); 1845 return this; 1846 } 1847 1848 /** 1849 * @return The first repetition of repeating field {@link #basis}, creating it 1850 * if it does not already exist 1851 */ 1852 public Reference getBasisFirstRep() { 1853 if (getBasis().isEmpty()) { 1854 addBasis(); 1855 } 1856 return getBasis().get(0); 1857 } 1858 1859 /** 1860 * @deprecated Use Reference#setResource(IBaseResource) instead 1861 */ 1862 @Deprecated 1863 public List<Resource> getBasisTarget() { 1864 if (this.basisTarget == null) 1865 this.basisTarget = new ArrayList<Resource>(); 1866 return this.basisTarget; 1867 } 1868 1869 /** 1870 * @return {@link #prediction} (Describes the expected outcome for the subject.) 1871 */ 1872 public List<RiskAssessmentPredictionComponent> getPrediction() { 1873 if (this.prediction == null) 1874 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1875 return this.prediction; 1876 } 1877 1878 /** 1879 * @return Returns a reference to <code>this</code> for easy method chaining 1880 */ 1881 public RiskAssessment setPrediction(List<RiskAssessmentPredictionComponent> thePrediction) { 1882 this.prediction = thePrediction; 1883 return this; 1884 } 1885 1886 public boolean hasPrediction() { 1887 if (this.prediction == null) 1888 return false; 1889 for (RiskAssessmentPredictionComponent item : this.prediction) 1890 if (!item.isEmpty()) 1891 return true; 1892 return false; 1893 } 1894 1895 public RiskAssessmentPredictionComponent addPrediction() { // 3 1896 RiskAssessmentPredictionComponent t = new RiskAssessmentPredictionComponent(); 1897 if (this.prediction == null) 1898 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1899 this.prediction.add(t); 1900 return t; 1901 } 1902 1903 public RiskAssessment addPrediction(RiskAssessmentPredictionComponent t) { // 3 1904 if (t == null) 1905 return this; 1906 if (this.prediction == null) 1907 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1908 this.prediction.add(t); 1909 return this; 1910 } 1911 1912 /** 1913 * @return The first repetition of repeating field {@link #prediction}, creating 1914 * it if it does not already exist 1915 */ 1916 public RiskAssessmentPredictionComponent getPredictionFirstRep() { 1917 if (getPrediction().isEmpty()) { 1918 addPrediction(); 1919 } 1920 return getPrediction().get(0); 1921 } 1922 1923 /** 1924 * @return {@link #mitigation} (A description of the steps that might be taken 1925 * to reduce the identified risk(s).). This is the underlying object 1926 * with id, value and extensions. The accessor "getMitigation" gives 1927 * direct access to the value 1928 */ 1929 public StringType getMitigationElement() { 1930 if (this.mitigation == null) 1931 if (Configuration.errorOnAutoCreate()) 1932 throw new Error("Attempt to auto-create RiskAssessment.mitigation"); 1933 else if (Configuration.doAutoCreate()) 1934 this.mitigation = new StringType(); // bb 1935 return this.mitigation; 1936 } 1937 1938 public boolean hasMitigationElement() { 1939 return this.mitigation != null && !this.mitigation.isEmpty(); 1940 } 1941 1942 public boolean hasMitigation() { 1943 return this.mitigation != null && !this.mitigation.isEmpty(); 1944 } 1945 1946 /** 1947 * @param value {@link #mitigation} (A description of the steps that might be 1948 * taken to reduce the identified risk(s).). This is the underlying 1949 * object with id, value and extensions. The accessor 1950 * "getMitigation" gives direct access to the value 1951 */ 1952 public RiskAssessment setMitigationElement(StringType value) { 1953 this.mitigation = value; 1954 return this; 1955 } 1956 1957 /** 1958 * @return A description of the steps that might be taken to reduce the 1959 * identified risk(s). 1960 */ 1961 public String getMitigation() { 1962 return this.mitigation == null ? null : this.mitigation.getValue(); 1963 } 1964 1965 /** 1966 * @param value A description of the steps that might be taken to reduce the 1967 * identified risk(s). 1968 */ 1969 public RiskAssessment setMitigation(String value) { 1970 if (Utilities.noString(value)) 1971 this.mitigation = null; 1972 else { 1973 if (this.mitigation == null) 1974 this.mitigation = new StringType(); 1975 this.mitigation.setValue(value); 1976 } 1977 return this; 1978 } 1979 1980 /** 1981 * @return {@link #note} (Additional comments about the risk assessment.) 1982 */ 1983 public List<Annotation> getNote() { 1984 if (this.note == null) 1985 this.note = new ArrayList<Annotation>(); 1986 return this.note; 1987 } 1988 1989 /** 1990 * @return Returns a reference to <code>this</code> for easy method chaining 1991 */ 1992 public RiskAssessment setNote(List<Annotation> theNote) { 1993 this.note = theNote; 1994 return this; 1995 } 1996 1997 public boolean hasNote() { 1998 if (this.note == null) 1999 return false; 2000 for (Annotation item : this.note) 2001 if (!item.isEmpty()) 2002 return true; 2003 return false; 2004 } 2005 2006 public Annotation addNote() { // 3 2007 Annotation t = new Annotation(); 2008 if (this.note == null) 2009 this.note = new ArrayList<Annotation>(); 2010 this.note.add(t); 2011 return t; 2012 } 2013 2014 public RiskAssessment addNote(Annotation t) { // 3 2015 if (t == null) 2016 return this; 2017 if (this.note == null) 2018 this.note = new ArrayList<Annotation>(); 2019 this.note.add(t); 2020 return this; 2021 } 2022 2023 /** 2024 * @return The first repetition of repeating field {@link #note}, creating it if 2025 * it does not already exist 2026 */ 2027 public Annotation getNoteFirstRep() { 2028 if (getNote().isEmpty()) { 2029 addNote(); 2030 } 2031 return getNote().get(0); 2032 } 2033 2034 protected void listChildren(List<Property> children) { 2035 super.listChildren(children); 2036 children.add(new Property("identifier", "Identifier", "Business identifier assigned to the risk assessment.", 0, 2037 java.lang.Integer.MAX_VALUE, identifier)); 2038 children.add(new Property("basedOn", "Reference(Any)", 2039 "A reference to the request that is fulfilled by this risk assessment.", 0, 1, basedOn)); 2040 children.add(new Property("parent", "Reference(Any)", 2041 "A reference to a resource that this risk assessment is part of, such as a Procedure.", 0, 1, parent)); 2042 children.add(new Property("status", "code", 2043 "The status of the RiskAssessment, using the same statuses as an Observation.", 0, 1, status)); 2044 children.add(new Property("method", "CodeableConcept", 2045 "The algorithm, process or mechanism used to evaluate the risk.", 0, 1, method)); 2046 children.add(new Property("code", "CodeableConcept", "The type of the risk assessment performed.", 0, 1, code)); 2047 children.add(new Property("subject", "Reference(Patient|Group)", 2048 "The patient or group the risk assessment applies to.", 0, 1, subject)); 2049 children.add(new Property("encounter", "Reference(Encounter)", "The encounter where the assessment was performed.", 2050 0, 1, encounter)); 2051 children.add(new Property("occurrence[x]", "dateTime|Period", 2052 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence)); 2053 children.add(new Property("condition", "Reference(Condition)", 2054 "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 2055 1, condition)); 2056 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Device)", 2057 "The provider or software application that performed the assessment.", 0, 1, performer)); 2058 children.add(new Property("reasonCode", "CodeableConcept", "The reason the risk assessment was performed.", 0, 2059 java.lang.Integer.MAX_VALUE, reasonCode)); 2060 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2061 "Resources supporting the reason the risk assessment was performed.", 0, java.lang.Integer.MAX_VALUE, 2062 reasonReference)); 2063 children.add(new Property("basis", "Reference(Any)", 2064 "Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).", 2065 0, java.lang.Integer.MAX_VALUE, basis)); 2066 children.add(new Property("prediction", "", "Describes the expected outcome for the subject.", 0, 2067 java.lang.Integer.MAX_VALUE, prediction)); 2068 children.add(new Property("mitigation", "string", 2069 "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1, mitigation)); 2070 children.add(new Property("note", "Annotation", "Additional comments about the risk assessment.", 0, 2071 java.lang.Integer.MAX_VALUE, note)); 2072 } 2073 2074 @Override 2075 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2076 switch (_hash) { 2077 case -1618432855: 2078 /* identifier */ return new Property("identifier", "Identifier", 2079 "Business identifier assigned to the risk assessment.", 0, java.lang.Integer.MAX_VALUE, identifier); 2080 case -332612366: 2081 /* basedOn */ return new Property("basedOn", "Reference(Any)", 2082 "A reference to the request that is fulfilled by this risk assessment.", 0, 1, basedOn); 2083 case -995424086: 2084 /* parent */ return new Property("parent", "Reference(Any)", 2085 "A reference to a resource that this risk assessment is part of, such as a Procedure.", 0, 1, parent); 2086 case -892481550: 2087 /* status */ return new Property("status", "code", 2088 "The status of the RiskAssessment, using the same statuses as an Observation.", 0, 1, status); 2089 case -1077554975: 2090 /* method */ return new Property("method", "CodeableConcept", 2091 "The algorithm, process or mechanism used to evaluate the risk.", 0, 1, method); 2092 case 3059181: 2093 /* code */ return new Property("code", "CodeableConcept", "The type of the risk assessment performed.", 0, 1, 2094 code); 2095 case -1867885268: 2096 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2097 "The patient or group the risk assessment applies to.", 0, 1, subject); 2098 case 1524132147: 2099 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2100 "The encounter where the assessment was performed.", 0, 1, encounter); 2101 case -2022646513: 2102 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period", 2103 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 2104 case 1687874001: 2105 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period", 2106 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 2107 case -298443636: 2108 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period", 2109 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 2110 case 1397156594: 2111 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period", 2112 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 2113 case -861311717: 2114 /* condition */ return new Property("condition", "Reference(Condition)", 2115 "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 2116 1, condition); 2117 case 481140686: 2118 /* performer */ return new Property("performer", "Reference(Practitioner|PractitionerRole|Device)", 2119 "The provider or software application that performed the assessment.", 0, 1, performer); 2120 case 722137681: 2121 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2122 "The reason the risk assessment was performed.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2123 case -1146218137: 2124 /* reasonReference */ return new Property("reasonReference", 2125 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2126 "Resources supporting the reason the risk assessment was performed.", 0, java.lang.Integer.MAX_VALUE, 2127 reasonReference); 2128 case 93508670: 2129 /* basis */ return new Property("basis", "Reference(Any)", 2130 "Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).", 2131 0, java.lang.Integer.MAX_VALUE, basis); 2132 case 1161234575: 2133 /* prediction */ return new Property("prediction", "", "Describes the expected outcome for the subject.", 0, 2134 java.lang.Integer.MAX_VALUE, prediction); 2135 case 1293793087: 2136 /* mitigation */ return new Property("mitigation", "string", 2137 "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1, mitigation); 2138 case 3387378: 2139 /* note */ return new Property("note", "Annotation", "Additional comments about the risk assessment.", 0, 2140 java.lang.Integer.MAX_VALUE, note); 2141 default: 2142 return super.getNamedProperty(_hash, _name, _checkValid); 2143 } 2144 2145 } 2146 2147 @Override 2148 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2149 switch (hash) { 2150 case -1618432855: 2151 /* identifier */ return this.identifier == null ? new Base[0] 2152 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2153 case -332612366: 2154 /* basedOn */ return this.basedOn == null ? new Base[0] : new Base[] { this.basedOn }; // Reference 2155 case -995424086: 2156 /* parent */ return this.parent == null ? new Base[0] : new Base[] { this.parent }; // Reference 2157 case -892481550: 2158 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<RiskAssessmentStatus> 2159 case -1077554975: 2160 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 2161 case 3059181: 2162 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2163 case -1867885268: 2164 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2165 case 1524132147: 2166 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2167 case 1687874001: 2168 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 2169 case -861311717: 2170 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // Reference 2171 case 481140686: 2172 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 2173 case 722137681: 2174 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2175 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2176 case -1146218137: 2177 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2178 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2179 case 93508670: 2180 /* basis */ return this.basis == null ? new Base[0] : this.basis.toArray(new Base[this.basis.size()]); // Reference 2181 case 1161234575: 2182 /* prediction */ return this.prediction == null ? new Base[0] 2183 : this.prediction.toArray(new Base[this.prediction.size()]); // RiskAssessmentPredictionComponent 2184 case 1293793087: 2185 /* mitigation */ return this.mitigation == null ? new Base[0] : new Base[] { this.mitigation }; // StringType 2186 case 3387378: 2187 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2188 default: 2189 return super.getProperty(hash, name, checkValid); 2190 } 2191 2192 } 2193 2194 @Override 2195 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2196 switch (hash) { 2197 case -1618432855: // identifier 2198 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2199 return value; 2200 case -332612366: // basedOn 2201 this.basedOn = castToReference(value); // Reference 2202 return value; 2203 case -995424086: // parent 2204 this.parent = castToReference(value); // Reference 2205 return value; 2206 case -892481550: // status 2207 value = new RiskAssessmentStatusEnumFactory().fromType(castToCode(value)); 2208 this.status = (Enumeration) value; // Enumeration<RiskAssessmentStatus> 2209 return value; 2210 case -1077554975: // method 2211 this.method = castToCodeableConcept(value); // CodeableConcept 2212 return value; 2213 case 3059181: // code 2214 this.code = castToCodeableConcept(value); // CodeableConcept 2215 return value; 2216 case -1867885268: // subject 2217 this.subject = castToReference(value); // Reference 2218 return value; 2219 case 1524132147: // encounter 2220 this.encounter = castToReference(value); // Reference 2221 return value; 2222 case 1687874001: // occurrence 2223 this.occurrence = castToType(value); // Type 2224 return value; 2225 case -861311717: // condition 2226 this.condition = castToReference(value); // Reference 2227 return value; 2228 case 481140686: // performer 2229 this.performer = castToReference(value); // Reference 2230 return value; 2231 case 722137681: // reasonCode 2232 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2233 return value; 2234 case -1146218137: // reasonReference 2235 this.getReasonReference().add(castToReference(value)); // Reference 2236 return value; 2237 case 93508670: // basis 2238 this.getBasis().add(castToReference(value)); // Reference 2239 return value; 2240 case 1161234575: // prediction 2241 this.getPrediction().add((RiskAssessmentPredictionComponent) value); // RiskAssessmentPredictionComponent 2242 return value; 2243 case 1293793087: // mitigation 2244 this.mitigation = castToString(value); // StringType 2245 return value; 2246 case 3387378: // note 2247 this.getNote().add(castToAnnotation(value)); // Annotation 2248 return value; 2249 default: 2250 return super.setProperty(hash, name, value); 2251 } 2252 2253 } 2254 2255 @Override 2256 public Base setProperty(String name, Base value) throws FHIRException { 2257 if (name.equals("identifier")) { 2258 this.getIdentifier().add(castToIdentifier(value)); 2259 } else if (name.equals("basedOn")) { 2260 this.basedOn = castToReference(value); // Reference 2261 } else if (name.equals("parent")) { 2262 this.parent = castToReference(value); // Reference 2263 } else if (name.equals("status")) { 2264 value = new RiskAssessmentStatusEnumFactory().fromType(castToCode(value)); 2265 this.status = (Enumeration) value; // Enumeration<RiskAssessmentStatus> 2266 } else if (name.equals("method")) { 2267 this.method = castToCodeableConcept(value); // CodeableConcept 2268 } else if (name.equals("code")) { 2269 this.code = castToCodeableConcept(value); // CodeableConcept 2270 } else if (name.equals("subject")) { 2271 this.subject = castToReference(value); // Reference 2272 } else if (name.equals("encounter")) { 2273 this.encounter = castToReference(value); // Reference 2274 } else if (name.equals("occurrence[x]")) { 2275 this.occurrence = castToType(value); // Type 2276 } else if (name.equals("condition")) { 2277 this.condition = castToReference(value); // Reference 2278 } else if (name.equals("performer")) { 2279 this.performer = castToReference(value); // Reference 2280 } else if (name.equals("reasonCode")) { 2281 this.getReasonCode().add(castToCodeableConcept(value)); 2282 } else if (name.equals("reasonReference")) { 2283 this.getReasonReference().add(castToReference(value)); 2284 } else if (name.equals("basis")) { 2285 this.getBasis().add(castToReference(value)); 2286 } else if (name.equals("prediction")) { 2287 this.getPrediction().add((RiskAssessmentPredictionComponent) value); 2288 } else if (name.equals("mitigation")) { 2289 this.mitigation = castToString(value); // StringType 2290 } else if (name.equals("note")) { 2291 this.getNote().add(castToAnnotation(value)); 2292 } else 2293 return super.setProperty(name, value); 2294 return value; 2295 } 2296 2297 @Override 2298 public void removeChild(String name, Base value) throws FHIRException { 2299 if (name.equals("identifier")) { 2300 this.getIdentifier().remove(castToIdentifier(value)); 2301 } else if (name.equals("basedOn")) { 2302 this.basedOn = null; 2303 } else if (name.equals("parent")) { 2304 this.parent = null; 2305 } else if (name.equals("status")) { 2306 this.status = null; 2307 } else if (name.equals("method")) { 2308 this.method = null; 2309 } else if (name.equals("code")) { 2310 this.code = null; 2311 } else if (name.equals("subject")) { 2312 this.subject = null; 2313 } else if (name.equals("encounter")) { 2314 this.encounter = null; 2315 } else if (name.equals("occurrence[x]")) { 2316 this.occurrence = null; 2317 } else if (name.equals("condition")) { 2318 this.condition = null; 2319 } else if (name.equals("performer")) { 2320 this.performer = null; 2321 } else if (name.equals("reasonCode")) { 2322 this.getReasonCode().remove(castToCodeableConcept(value)); 2323 } else if (name.equals("reasonReference")) { 2324 this.getReasonReference().remove(castToReference(value)); 2325 } else if (name.equals("basis")) { 2326 this.getBasis().remove(castToReference(value)); 2327 } else if (name.equals("prediction")) { 2328 this.getPrediction().remove((RiskAssessmentPredictionComponent) value); 2329 } else if (name.equals("mitigation")) { 2330 this.mitigation = null; 2331 } else if (name.equals("note")) { 2332 this.getNote().remove(castToAnnotation(value)); 2333 } else 2334 super.removeChild(name, value); 2335 2336 } 2337 2338 @Override 2339 public Base makeProperty(int hash, String name) throws FHIRException { 2340 switch (hash) { 2341 case -1618432855: 2342 return addIdentifier(); 2343 case -332612366: 2344 return getBasedOn(); 2345 case -995424086: 2346 return getParent(); 2347 case -892481550: 2348 return getStatusElement(); 2349 case -1077554975: 2350 return getMethod(); 2351 case 3059181: 2352 return getCode(); 2353 case -1867885268: 2354 return getSubject(); 2355 case 1524132147: 2356 return getEncounter(); 2357 case -2022646513: 2358 return getOccurrence(); 2359 case 1687874001: 2360 return getOccurrence(); 2361 case -861311717: 2362 return getCondition(); 2363 case 481140686: 2364 return getPerformer(); 2365 case 722137681: 2366 return addReasonCode(); 2367 case -1146218137: 2368 return addReasonReference(); 2369 case 93508670: 2370 return addBasis(); 2371 case 1161234575: 2372 return addPrediction(); 2373 case 1293793087: 2374 return getMitigationElement(); 2375 case 3387378: 2376 return addNote(); 2377 default: 2378 return super.makeProperty(hash, name); 2379 } 2380 2381 } 2382 2383 @Override 2384 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2385 switch (hash) { 2386 case -1618432855: 2387 /* identifier */ return new String[] { "Identifier" }; 2388 case -332612366: 2389 /* basedOn */ return new String[] { "Reference" }; 2390 case -995424086: 2391 /* parent */ return new String[] { "Reference" }; 2392 case -892481550: 2393 /* status */ return new String[] { "code" }; 2394 case -1077554975: 2395 /* method */ return new String[] { "CodeableConcept" }; 2396 case 3059181: 2397 /* code */ return new String[] { "CodeableConcept" }; 2398 case -1867885268: 2399 /* subject */ return new String[] { "Reference" }; 2400 case 1524132147: 2401 /* encounter */ return new String[] { "Reference" }; 2402 case 1687874001: 2403 /* occurrence */ return new String[] { "dateTime", "Period" }; 2404 case -861311717: 2405 /* condition */ return new String[] { "Reference" }; 2406 case 481140686: 2407 /* performer */ return new String[] { "Reference" }; 2408 case 722137681: 2409 /* reasonCode */ return new String[] { "CodeableConcept" }; 2410 case -1146218137: 2411 /* reasonReference */ return new String[] { "Reference" }; 2412 case 93508670: 2413 /* basis */ return new String[] { "Reference" }; 2414 case 1161234575: 2415 /* prediction */ return new String[] {}; 2416 case 1293793087: 2417 /* mitigation */ return new String[] { "string" }; 2418 case 3387378: 2419 /* note */ return new String[] { "Annotation" }; 2420 default: 2421 return super.getTypesForProperty(hash, name); 2422 } 2423 2424 } 2425 2426 @Override 2427 public Base addChild(String name) throws FHIRException { 2428 if (name.equals("identifier")) { 2429 return addIdentifier(); 2430 } else if (name.equals("basedOn")) { 2431 this.basedOn = new Reference(); 2432 return this.basedOn; 2433 } else if (name.equals("parent")) { 2434 this.parent = new Reference(); 2435 return this.parent; 2436 } else if (name.equals("status")) { 2437 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.status"); 2438 } else if (name.equals("method")) { 2439 this.method = new CodeableConcept(); 2440 return this.method; 2441 } else if (name.equals("code")) { 2442 this.code = new CodeableConcept(); 2443 return this.code; 2444 } else if (name.equals("subject")) { 2445 this.subject = new Reference(); 2446 return this.subject; 2447 } else if (name.equals("encounter")) { 2448 this.encounter = new Reference(); 2449 return this.encounter; 2450 } else if (name.equals("occurrenceDateTime")) { 2451 this.occurrence = new DateTimeType(); 2452 return this.occurrence; 2453 } else if (name.equals("occurrencePeriod")) { 2454 this.occurrence = new Period(); 2455 return this.occurrence; 2456 } else if (name.equals("condition")) { 2457 this.condition = new Reference(); 2458 return this.condition; 2459 } else if (name.equals("performer")) { 2460 this.performer = new Reference(); 2461 return this.performer; 2462 } else if (name.equals("reasonCode")) { 2463 return addReasonCode(); 2464 } else if (name.equals("reasonReference")) { 2465 return addReasonReference(); 2466 } else if (name.equals("basis")) { 2467 return addBasis(); 2468 } else if (name.equals("prediction")) { 2469 return addPrediction(); 2470 } else if (name.equals("mitigation")) { 2471 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.mitigation"); 2472 } else if (name.equals("note")) { 2473 return addNote(); 2474 } else 2475 return super.addChild(name); 2476 } 2477 2478 public String fhirType() { 2479 return "RiskAssessment"; 2480 2481 } 2482 2483 public RiskAssessment copy() { 2484 RiskAssessment dst = new RiskAssessment(); 2485 copyValues(dst); 2486 return dst; 2487 } 2488 2489 public void copyValues(RiskAssessment dst) { 2490 super.copyValues(dst); 2491 if (identifier != null) { 2492 dst.identifier = new ArrayList<Identifier>(); 2493 for (Identifier i : identifier) 2494 dst.identifier.add(i.copy()); 2495 } 2496 ; 2497 dst.basedOn = basedOn == null ? null : basedOn.copy(); 2498 dst.parent = parent == null ? null : parent.copy(); 2499 dst.status = status == null ? null : status.copy(); 2500 dst.method = method == null ? null : method.copy(); 2501 dst.code = code == null ? null : code.copy(); 2502 dst.subject = subject == null ? null : subject.copy(); 2503 dst.encounter = encounter == null ? null : encounter.copy(); 2504 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2505 dst.condition = condition == null ? null : condition.copy(); 2506 dst.performer = performer == null ? null : performer.copy(); 2507 if (reasonCode != null) { 2508 dst.reasonCode = new ArrayList<CodeableConcept>(); 2509 for (CodeableConcept i : reasonCode) 2510 dst.reasonCode.add(i.copy()); 2511 } 2512 ; 2513 if (reasonReference != null) { 2514 dst.reasonReference = new ArrayList<Reference>(); 2515 for (Reference i : reasonReference) 2516 dst.reasonReference.add(i.copy()); 2517 } 2518 ; 2519 if (basis != null) { 2520 dst.basis = new ArrayList<Reference>(); 2521 for (Reference i : basis) 2522 dst.basis.add(i.copy()); 2523 } 2524 ; 2525 if (prediction != null) { 2526 dst.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 2527 for (RiskAssessmentPredictionComponent i : prediction) 2528 dst.prediction.add(i.copy()); 2529 } 2530 ; 2531 dst.mitigation = mitigation == null ? null : mitigation.copy(); 2532 if (note != null) { 2533 dst.note = new ArrayList<Annotation>(); 2534 for (Annotation i : note) 2535 dst.note.add(i.copy()); 2536 } 2537 ; 2538 } 2539 2540 protected RiskAssessment typedCopy() { 2541 return copy(); 2542 } 2543 2544 @Override 2545 public boolean equalsDeep(Base other_) { 2546 if (!super.equalsDeep(other_)) 2547 return false; 2548 if (!(other_ instanceof RiskAssessment)) 2549 return false; 2550 RiskAssessment o = (RiskAssessment) other_; 2551 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2552 && compareDeep(parent, o.parent, true) && compareDeep(status, o.status, true) 2553 && compareDeep(method, o.method, true) && compareDeep(code, o.code, true) 2554 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2555 && compareDeep(occurrence, o.occurrence, true) && compareDeep(condition, o.condition, true) 2556 && compareDeep(performer, o.performer, true) && compareDeep(reasonCode, o.reasonCode, true) 2557 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(basis, o.basis, true) 2558 && compareDeep(prediction, o.prediction, true) && compareDeep(mitigation, o.mitigation, true) 2559 && compareDeep(note, o.note, true); 2560 } 2561 2562 @Override 2563 public boolean equalsShallow(Base other_) { 2564 if (!super.equalsShallow(other_)) 2565 return false; 2566 if (!(other_ instanceof RiskAssessment)) 2567 return false; 2568 RiskAssessment o = (RiskAssessment) other_; 2569 return compareValues(status, o.status, true) && compareValues(mitigation, o.mitigation, true); 2570 } 2571 2572 public boolean isEmpty() { 2573 return super.isEmpty() 2574 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, parent, status, method, code, subject, encounter, 2575 occurrence, condition, performer, reasonCode, reasonReference, basis, prediction, mitigation, note); 2576 } 2577 2578 @Override 2579 public ResourceType getResourceType() { 2580 return ResourceType.RiskAssessment; 2581 } 2582 2583 /** 2584 * Search parameter: <b>date</b> 2585 * <p> 2586 * Description: <b>When was assessment made?</b><br> 2587 * Type: <b>date</b><br> 2588 * Path: <b>RiskAssessment.occurrenceDateTime</b><br> 2589 * </p> 2590 */ 2591 @SearchParamDefinition(name = "date", path = "(RiskAssessment.occurrence as dateTime)", description = "When was assessment made?", type = "date") 2592 public static final String SP_DATE = "date"; 2593 /** 2594 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2595 * <p> 2596 * Description: <b>When was assessment made?</b><br> 2597 * Type: <b>date</b><br> 2598 * Path: <b>RiskAssessment.occurrenceDateTime</b><br> 2599 * </p> 2600 */ 2601 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2602 SP_DATE); 2603 2604 /** 2605 * Search parameter: <b>identifier</b> 2606 * <p> 2607 * Description: <b>Unique identifier for the assessment</b><br> 2608 * Type: <b>token</b><br> 2609 * Path: <b>RiskAssessment.identifier</b><br> 2610 * </p> 2611 */ 2612 @SearchParamDefinition(name = "identifier", path = "RiskAssessment.identifier", description = "Unique identifier for the assessment", type = "token") 2613 public static final String SP_IDENTIFIER = "identifier"; 2614 /** 2615 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2616 * <p> 2617 * Description: <b>Unique identifier for the assessment</b><br> 2618 * Type: <b>token</b><br> 2619 * Path: <b>RiskAssessment.identifier</b><br> 2620 * </p> 2621 */ 2622 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2623 SP_IDENTIFIER); 2624 2625 /** 2626 * Search parameter: <b>condition</b> 2627 * <p> 2628 * Description: <b>Condition assessed</b><br> 2629 * Type: <b>reference</b><br> 2630 * Path: <b>RiskAssessment.condition</b><br> 2631 * </p> 2632 */ 2633 @SearchParamDefinition(name = "condition", path = "RiskAssessment.condition", description = "Condition assessed", type = "reference", target = { 2634 Condition.class }) 2635 public static final String SP_CONDITION = "condition"; 2636 /** 2637 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 2638 * <p> 2639 * Description: <b>Condition assessed</b><br> 2640 * Type: <b>reference</b><br> 2641 * Path: <b>RiskAssessment.condition</b><br> 2642 * </p> 2643 */ 2644 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2645 SP_CONDITION); 2646 2647 /** 2648 * Constant for fluent queries to be used to add include statements. Specifies 2649 * the path value of "<b>RiskAssessment:condition</b>". 2650 */ 2651 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include( 2652 "RiskAssessment:condition").toLocked(); 2653 2654 /** 2655 * Search parameter: <b>performer</b> 2656 * <p> 2657 * Description: <b>Who did assessment?</b><br> 2658 * Type: <b>reference</b><br> 2659 * Path: <b>RiskAssessment.performer</b><br> 2660 * </p> 2661 */ 2662 @SearchParamDefinition(name = "performer", path = "RiskAssessment.performer", description = "Who did assessment?", type = "reference", providesMembershipIn = { 2663 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2664 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 2665 Practitioner.class, PractitionerRole.class }) 2666 public static final String SP_PERFORMER = "performer"; 2667 /** 2668 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2669 * <p> 2670 * Description: <b>Who did assessment?</b><br> 2671 * Type: <b>reference</b><br> 2672 * Path: <b>RiskAssessment.performer</b><br> 2673 * </p> 2674 */ 2675 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2676 SP_PERFORMER); 2677 2678 /** 2679 * Constant for fluent queries to be used to add include statements. Specifies 2680 * the path value of "<b>RiskAssessment:performer</b>". 2681 */ 2682 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 2683 "RiskAssessment:performer").toLocked(); 2684 2685 /** 2686 * Search parameter: <b>method</b> 2687 * <p> 2688 * Description: <b>Evaluation mechanism</b><br> 2689 * Type: <b>token</b><br> 2690 * Path: <b>RiskAssessment.method</b><br> 2691 * </p> 2692 */ 2693 @SearchParamDefinition(name = "method", path = "RiskAssessment.method", description = "Evaluation mechanism", type = "token") 2694 public static final String SP_METHOD = "method"; 2695 /** 2696 * <b>Fluent Client</b> search parameter constant for <b>method</b> 2697 * <p> 2698 * Description: <b>Evaluation mechanism</b><br> 2699 * Type: <b>token</b><br> 2700 * Path: <b>RiskAssessment.method</b><br> 2701 * </p> 2702 */ 2703 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2704 SP_METHOD); 2705 2706 /** 2707 * Search parameter: <b>subject</b> 2708 * <p> 2709 * Description: <b>Who/what does assessment apply to?</b><br> 2710 * Type: <b>reference</b><br> 2711 * Path: <b>RiskAssessment.subject</b><br> 2712 * </p> 2713 */ 2714 @SearchParamDefinition(name = "subject", path = "RiskAssessment.subject", description = "Who/what does assessment apply to?", type = "reference", providesMembershipIn = { 2715 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 2716 public static final String SP_SUBJECT = "subject"; 2717 /** 2718 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2719 * <p> 2720 * Description: <b>Who/what does assessment apply to?</b><br> 2721 * Type: <b>reference</b><br> 2722 * Path: <b>RiskAssessment.subject</b><br> 2723 * </p> 2724 */ 2725 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2726 SP_SUBJECT); 2727 2728 /** 2729 * Constant for fluent queries to be used to add include statements. Specifies 2730 * the path value of "<b>RiskAssessment:subject</b>". 2731 */ 2732 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2733 "RiskAssessment:subject").toLocked(); 2734 2735 /** 2736 * Search parameter: <b>patient</b> 2737 * <p> 2738 * Description: <b>Who/what does assessment apply to?</b><br> 2739 * Type: <b>reference</b><br> 2740 * Path: <b>RiskAssessment.subject</b><br> 2741 * </p> 2742 */ 2743 @SearchParamDefinition(name = "patient", path = "RiskAssessment.subject.where(resolve() is Patient)", description = "Who/what does assessment apply to?", type = "reference", target = { 2744 Patient.class }) 2745 public static final String SP_PATIENT = "patient"; 2746 /** 2747 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2748 * <p> 2749 * Description: <b>Who/what does assessment apply to?</b><br> 2750 * Type: <b>reference</b><br> 2751 * Path: <b>RiskAssessment.subject</b><br> 2752 * </p> 2753 */ 2754 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2755 SP_PATIENT); 2756 2757 /** 2758 * Constant for fluent queries to be used to add include statements. Specifies 2759 * the path value of "<b>RiskAssessment:patient</b>". 2760 */ 2761 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2762 "RiskAssessment:patient").toLocked(); 2763 2764 /** 2765 * Search parameter: <b>probability</b> 2766 * <p> 2767 * Description: <b>Likelihood of specified outcome</b><br> 2768 * Type: <b>number</b><br> 2769 * Path: <b>RiskAssessment.prediction.probability[x]</b><br> 2770 * </p> 2771 */ 2772 @SearchParamDefinition(name = "probability", path = "RiskAssessment.prediction.probability", description = "Likelihood of specified outcome", type = "number") 2773 public static final String SP_PROBABILITY = "probability"; 2774 /** 2775 * <b>Fluent Client</b> search parameter constant for <b>probability</b> 2776 * <p> 2777 * Description: <b>Likelihood of specified outcome</b><br> 2778 * Type: <b>number</b><br> 2779 * Path: <b>RiskAssessment.prediction.probability[x]</b><br> 2780 * </p> 2781 */ 2782 public static final ca.uhn.fhir.rest.gclient.NumberClientParam PROBABILITY = new ca.uhn.fhir.rest.gclient.NumberClientParam( 2783 SP_PROBABILITY); 2784 2785 /** 2786 * Search parameter: <b>risk</b> 2787 * <p> 2788 * Description: <b>Likelihood of specified outcome as a qualitative 2789 * value</b><br> 2790 * Type: <b>token</b><br> 2791 * Path: <b>RiskAssessment.prediction.qualitativeRisk</b><br> 2792 * </p> 2793 */ 2794 @SearchParamDefinition(name = "risk", path = "RiskAssessment.prediction.qualitativeRisk", description = "Likelihood of specified outcome as a qualitative value", type = "token") 2795 public static final String SP_RISK = "risk"; 2796 /** 2797 * <b>Fluent Client</b> search parameter constant for <b>risk</b> 2798 * <p> 2799 * Description: <b>Likelihood of specified outcome as a qualitative 2800 * value</b><br> 2801 * Type: <b>token</b><br> 2802 * Path: <b>RiskAssessment.prediction.qualitativeRisk</b><br> 2803 * </p> 2804 */ 2805 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RISK = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2806 SP_RISK); 2807 2808 /** 2809 * Search parameter: <b>encounter</b> 2810 * <p> 2811 * Description: <b>Where was assessment performed?</b><br> 2812 * Type: <b>reference</b><br> 2813 * Path: <b>RiskAssessment.encounter</b><br> 2814 * </p> 2815 */ 2816 @SearchParamDefinition(name = "encounter", path = "RiskAssessment.encounter", description = "Where was assessment performed?", type = "reference", target = { 2817 Encounter.class }) 2818 public static final String SP_ENCOUNTER = "encounter"; 2819 /** 2820 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2821 * <p> 2822 * Description: <b>Where was assessment performed?</b><br> 2823 * Type: <b>reference</b><br> 2824 * Path: <b>RiskAssessment.encounter</b><br> 2825 * </p> 2826 */ 2827 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2828 SP_ENCOUNTER); 2829 2830 /** 2831 * Constant for fluent queries to be used to add include statements. Specifies 2832 * the path value of "<b>RiskAssessment:encounter</b>". 2833 */ 2834 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2835 "RiskAssessment:encounter").toLocked(); 2836 2837}