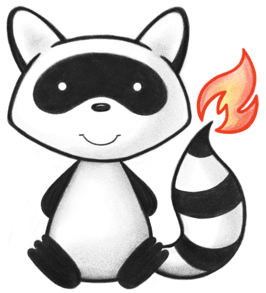
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * An assessment of the likely outcome(s) for a patient or other subject as well 050 * as the likelihood of each outcome. 051 */ 052@ResourceDef(name = "RiskAssessment", profile = "http://hl7.org/fhir/StructureDefinition/RiskAssessment") 053public class RiskAssessment extends DomainResource { 054 055 public enum RiskAssessmentStatus { 056 /** 057 * The existence of the observation is registered, but there is no result yet 058 * available. 059 */ 060 REGISTERED, 061 /** 062 * This is an initial or interim observation: data may be incomplete or 063 * unverified. 064 */ 065 PRELIMINARY, 066 /** 067 * The observation is complete and there are no further actions needed. 068 * Additional information such "released", "signed", etc would be represented 069 * using [Provenance](provenance.html) which provides not only the act but also 070 * the actors and dates and other related data. These act states would be 071 * associated with an observation status of `preliminary` until they are all 072 * completed and then a status of `final` would be applied. 073 */ 074 FINAL, 075 /** 076 * Subsequent to being Final, the observation has been modified subsequent. This 077 * includes updates/new information and corrections. 078 */ 079 AMENDED, 080 /** 081 * Subsequent to being Final, the observation has been modified to correct an 082 * error in the test result. 083 */ 084 CORRECTED, 085 /** 086 * The observation is unavailable because the measurement was not started or not 087 * completed (also sometimes called "aborted"). 088 */ 089 CANCELLED, 090 /** 091 * The observation has been withdrawn following previous final release. This 092 * electronic record should never have existed, though it is possible that 093 * real-world decisions were based on it. (If real-world activity has occurred, 094 * the status should be "cancelled" rather than "entered-in-error".). 095 */ 096 ENTEREDINERROR, 097 /** 098 * The authoring/source system does not know which of the status values 099 * currently applies for this observation. Note: This concept is not to be used 100 * for "other" - one of the listed statuses is presumed to apply, but the 101 * authoring/source system does not know which. 102 */ 103 UNKNOWN, 104 /** 105 * added to help the parsers with the generic types 106 */ 107 NULL; 108 109 public static RiskAssessmentStatus fromCode(String codeString) throws FHIRException { 110 if (codeString == null || "".equals(codeString)) 111 return null; 112 if ("registered".equals(codeString)) 113 return REGISTERED; 114 if ("preliminary".equals(codeString)) 115 return PRELIMINARY; 116 if ("final".equals(codeString)) 117 return FINAL; 118 if ("amended".equals(codeString)) 119 return AMENDED; 120 if ("corrected".equals(codeString)) 121 return CORRECTED; 122 if ("cancelled".equals(codeString)) 123 return CANCELLED; 124 if ("entered-in-error".equals(codeString)) 125 return ENTEREDINERROR; 126 if ("unknown".equals(codeString)) 127 return UNKNOWN; 128 if (Configuration.isAcceptInvalidEnums()) 129 return null; 130 else 131 throw new FHIRException("Unknown RiskAssessmentStatus code '" + codeString + "'"); 132 } 133 134 public String toCode() { 135 switch (this) { 136 case REGISTERED: 137 return "registered"; 138 case PRELIMINARY: 139 return "preliminary"; 140 case FINAL: 141 return "final"; 142 case AMENDED: 143 return "amended"; 144 case CORRECTED: 145 return "corrected"; 146 case CANCELLED: 147 return "cancelled"; 148 case ENTEREDINERROR: 149 return "entered-in-error"; 150 case UNKNOWN: 151 return "unknown"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getSystem() { 160 switch (this) { 161 case REGISTERED: 162 return "http://hl7.org/fhir/observation-status"; 163 case PRELIMINARY: 164 return "http://hl7.org/fhir/observation-status"; 165 case FINAL: 166 return "http://hl7.org/fhir/observation-status"; 167 case AMENDED: 168 return "http://hl7.org/fhir/observation-status"; 169 case CORRECTED: 170 return "http://hl7.org/fhir/observation-status"; 171 case CANCELLED: 172 return "http://hl7.org/fhir/observation-status"; 173 case ENTEREDINERROR: 174 return "http://hl7.org/fhir/observation-status"; 175 case UNKNOWN: 176 return "http://hl7.org/fhir/observation-status"; 177 case NULL: 178 return null; 179 default: 180 return "?"; 181 } 182 } 183 184 public String getDefinition() { 185 switch (this) { 186 case REGISTERED: 187 return "The existence of the observation is registered, but there is no result yet available."; 188 case PRELIMINARY: 189 return "This is an initial or interim observation: data may be incomplete or unverified."; 190 case FINAL: 191 return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 192 case AMENDED: 193 return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 194 case CORRECTED: 195 return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 196 case CANCELLED: 197 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 198 case ENTEREDINERROR: 199 return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 200 case UNKNOWN: 201 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 202 case NULL: 203 return null; 204 default: 205 return "?"; 206 } 207 } 208 209 public String getDisplay() { 210 switch (this) { 211 case REGISTERED: 212 return "Registered"; 213 case PRELIMINARY: 214 return "Preliminary"; 215 case FINAL: 216 return "Final"; 217 case AMENDED: 218 return "Amended"; 219 case CORRECTED: 220 return "Corrected"; 221 case CANCELLED: 222 return "Cancelled"; 223 case ENTEREDINERROR: 224 return "Entered in Error"; 225 case UNKNOWN: 226 return "Unknown"; 227 case NULL: 228 return null; 229 default: 230 return "?"; 231 } 232 } 233 } 234 235 public static class RiskAssessmentStatusEnumFactory implements EnumFactory<RiskAssessmentStatus> { 236 public RiskAssessmentStatus fromCode(String codeString) throws IllegalArgumentException { 237 if (codeString == null || "".equals(codeString)) 238 if (codeString == null || "".equals(codeString)) 239 return null; 240 if ("registered".equals(codeString)) 241 return RiskAssessmentStatus.REGISTERED; 242 if ("preliminary".equals(codeString)) 243 return RiskAssessmentStatus.PRELIMINARY; 244 if ("final".equals(codeString)) 245 return RiskAssessmentStatus.FINAL; 246 if ("amended".equals(codeString)) 247 return RiskAssessmentStatus.AMENDED; 248 if ("corrected".equals(codeString)) 249 return RiskAssessmentStatus.CORRECTED; 250 if ("cancelled".equals(codeString)) 251 return RiskAssessmentStatus.CANCELLED; 252 if ("entered-in-error".equals(codeString)) 253 return RiskAssessmentStatus.ENTEREDINERROR; 254 if ("unknown".equals(codeString)) 255 return RiskAssessmentStatus.UNKNOWN; 256 throw new IllegalArgumentException("Unknown RiskAssessmentStatus code '" + codeString + "'"); 257 } 258 259 public Enumeration<RiskAssessmentStatus> fromType(PrimitiveType<?> code) throws FHIRException { 260 if (code == null) 261 return null; 262 if (code.isEmpty()) 263 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.NULL, code); 264 String codeString = code.asStringValue(); 265 if (codeString == null || "".equals(codeString)) 266 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.NULL, code); 267 if ("registered".equals(codeString)) 268 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.REGISTERED, code); 269 if ("preliminary".equals(codeString)) 270 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.PRELIMINARY, code); 271 if ("final".equals(codeString)) 272 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.FINAL, code); 273 if ("amended".equals(codeString)) 274 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.AMENDED, code); 275 if ("corrected".equals(codeString)) 276 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.CORRECTED, code); 277 if ("cancelled".equals(codeString)) 278 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.CANCELLED, code); 279 if ("entered-in-error".equals(codeString)) 280 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.ENTEREDINERROR, code); 281 if ("unknown".equals(codeString)) 282 return new Enumeration<RiskAssessmentStatus>(this, RiskAssessmentStatus.UNKNOWN, code); 283 throw new FHIRException("Unknown RiskAssessmentStatus code '" + codeString + "'"); 284 } 285 286 public String toCode(RiskAssessmentStatus code) { 287 if (code == RiskAssessmentStatus.REGISTERED) 288 return "registered"; 289 if (code == RiskAssessmentStatus.PRELIMINARY) 290 return "preliminary"; 291 if (code == RiskAssessmentStatus.FINAL) 292 return "final"; 293 if (code == RiskAssessmentStatus.AMENDED) 294 return "amended"; 295 if (code == RiskAssessmentStatus.CORRECTED) 296 return "corrected"; 297 if (code == RiskAssessmentStatus.CANCELLED) 298 return "cancelled"; 299 if (code == RiskAssessmentStatus.ENTEREDINERROR) 300 return "entered-in-error"; 301 if (code == RiskAssessmentStatus.UNKNOWN) 302 return "unknown"; 303 return "?"; 304 } 305 306 public String toSystem(RiskAssessmentStatus code) { 307 return code.getSystem(); 308 } 309 } 310 311 @Block() 312 public static class RiskAssessmentPredictionComponent extends BackboneElement implements IBaseBackboneElement { 313 /** 314 * One of the potential outcomes for the patient (e.g. remission, death, a 315 * particular condition). 316 */ 317 @Child(name = "outcome", type = { 318 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 319 @Description(shortDefinition = "Possible outcome for the subject", formalDefinition = "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).") 320 protected CodeableConcept outcome; 321 322 /** 323 * Indicates how likely the outcome is (in the specified timeframe). 324 */ 325 @Child(name = "probability", type = { DecimalType.class, 326 Range.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 327 @Description(shortDefinition = "Likelihood of specified outcome", formalDefinition = "Indicates how likely the outcome is (in the specified timeframe).") 328 protected Type probability; 329 330 /** 331 * Indicates how likely the outcome is (in the specified timeframe), expressed 332 * as a qualitative value (e.g. low, medium, or high). 333 */ 334 @Child(name = "qualitativeRisk", type = { 335 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 336 @Description(shortDefinition = "Likelihood of specified outcome as a qualitative value", formalDefinition = "Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).") 337 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/risk-probability") 338 protected CodeableConcept qualitativeRisk; 339 340 /** 341 * Indicates the risk for this particular subject (with their specific 342 * characteristics) divided by the risk of the population in general. (Numbers 343 * greater than 1 = higher risk than the population, numbers less than 1 = lower 344 * risk.). 345 */ 346 @Child(name = "relativeRisk", type = { 347 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 348 @Description(shortDefinition = "Relative likelihood", formalDefinition = "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).") 349 protected DecimalType relativeRisk; 350 351 /** 352 * Indicates the period of time or age range of the subject to which the 353 * specified probability applies. 354 */ 355 @Child(name = "when", type = { Period.class, 356 Range.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 357 @Description(shortDefinition = "Timeframe or age range", formalDefinition = "Indicates the period of time or age range of the subject to which the specified probability applies.") 358 protected Type when; 359 360 /** 361 * Additional information explaining the basis for the prediction. 362 */ 363 @Child(name = "rationale", type = { 364 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 365 @Description(shortDefinition = "Explanation of prediction", formalDefinition = "Additional information explaining the basis for the prediction.") 366 protected StringType rationale; 367 368 private static final long serialVersionUID = 1283401747L; 369 370 /** 371 * Constructor 372 */ 373 public RiskAssessmentPredictionComponent() { 374 super(); 375 } 376 377 /** 378 * @return {@link #outcome} (One of the potential outcomes for the patient (e.g. 379 * remission, death, a particular condition).) 380 */ 381 public CodeableConcept getOutcome() { 382 if (this.outcome == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.outcome"); 385 else if (Configuration.doAutoCreate()) 386 this.outcome = new CodeableConcept(); // cc 387 return this.outcome; 388 } 389 390 public boolean hasOutcome() { 391 return this.outcome != null && !this.outcome.isEmpty(); 392 } 393 394 /** 395 * @param value {@link #outcome} (One of the potential outcomes for the patient 396 * (e.g. remission, death, a particular condition).) 397 */ 398 public RiskAssessmentPredictionComponent setOutcome(CodeableConcept value) { 399 this.outcome = value; 400 return this; 401 } 402 403 /** 404 * @return {@link #probability} (Indicates how likely the outcome is (in the 405 * specified timeframe).) 406 */ 407 public Type getProbability() { 408 return this.probability; 409 } 410 411 /** 412 * @return {@link #probability} (Indicates how likely the outcome is (in the 413 * specified timeframe).) 414 */ 415 public DecimalType getProbabilityDecimalType() throws FHIRException { 416 if (this.probability == null) 417 this.probability = new DecimalType(); 418 if (!(this.probability instanceof DecimalType)) 419 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 420 + this.probability.getClass().getName() + " was encountered"); 421 return (DecimalType) this.probability; 422 } 423 424 public boolean hasProbabilityDecimalType() { 425 return this != null && this.probability instanceof DecimalType; 426 } 427 428 /** 429 * @return {@link #probability} (Indicates how likely the outcome is (in the 430 * specified timeframe).) 431 */ 432 public Range getProbabilityRange() throws FHIRException { 433 if (this.probability == null) 434 this.probability = new Range(); 435 if (!(this.probability instanceof Range)) 436 throw new FHIRException("Type mismatch: the type Range was expected, but " 437 + this.probability.getClass().getName() + " was encountered"); 438 return (Range) this.probability; 439 } 440 441 public boolean hasProbabilityRange() { 442 return this != null && this.probability instanceof Range; 443 } 444 445 public boolean hasProbability() { 446 return this.probability != null && !this.probability.isEmpty(); 447 } 448 449 /** 450 * @param value {@link #probability} (Indicates how likely the outcome is (in 451 * the specified timeframe).) 452 */ 453 public RiskAssessmentPredictionComponent setProbability(Type value) { 454 if (value != null && !(value instanceof DecimalType || value instanceof Range)) 455 throw new Error("Not the right type for RiskAssessment.prediction.probability[x]: " + value.fhirType()); 456 this.probability = value; 457 return this; 458 } 459 460 /** 461 * @return {@link #qualitativeRisk} (Indicates how likely the outcome is (in the 462 * specified timeframe), expressed as a qualitative value (e.g. low, 463 * medium, or high).) 464 */ 465 public CodeableConcept getQualitativeRisk() { 466 if (this.qualitativeRisk == null) 467 if (Configuration.errorOnAutoCreate()) 468 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.qualitativeRisk"); 469 else if (Configuration.doAutoCreate()) 470 this.qualitativeRisk = new CodeableConcept(); // cc 471 return this.qualitativeRisk; 472 } 473 474 public boolean hasQualitativeRisk() { 475 return this.qualitativeRisk != null && !this.qualitativeRisk.isEmpty(); 476 } 477 478 /** 479 * @param value {@link #qualitativeRisk} (Indicates how likely the outcome is 480 * (in the specified timeframe), expressed as a qualitative value 481 * (e.g. low, medium, or high).) 482 */ 483 public RiskAssessmentPredictionComponent setQualitativeRisk(CodeableConcept value) { 484 this.qualitativeRisk = value; 485 return this; 486 } 487 488 /** 489 * @return {@link #relativeRisk} (Indicates the risk for this particular subject 490 * (with their specific characteristics) divided by the risk of the 491 * population in general. (Numbers greater than 1 = higher risk than the 492 * population, numbers less than 1 = lower risk.).). This is the 493 * underlying object with id, value and extensions. The accessor 494 * "getRelativeRisk" gives direct access to the value 495 */ 496 public DecimalType getRelativeRiskElement() { 497 if (this.relativeRisk == null) 498 if (Configuration.errorOnAutoCreate()) 499 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.relativeRisk"); 500 else if (Configuration.doAutoCreate()) 501 this.relativeRisk = new DecimalType(); // bb 502 return this.relativeRisk; 503 } 504 505 public boolean hasRelativeRiskElement() { 506 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 507 } 508 509 public boolean hasRelativeRisk() { 510 return this.relativeRisk != null && !this.relativeRisk.isEmpty(); 511 } 512 513 /** 514 * @param value {@link #relativeRisk} (Indicates the risk for this particular 515 * subject (with their specific characteristics) divided by the 516 * risk of the population in general. (Numbers greater than 1 = 517 * higher risk than the population, numbers less than 1 = lower 518 * risk.).). This is the underlying object with id, value and 519 * extensions. The accessor "getRelativeRisk" gives direct access 520 * to the value 521 */ 522 public RiskAssessmentPredictionComponent setRelativeRiskElement(DecimalType value) { 523 this.relativeRisk = value; 524 return this; 525 } 526 527 /** 528 * @return Indicates the risk for this particular subject (with their specific 529 * characteristics) divided by the risk of the population in general. 530 * (Numbers greater than 1 = higher risk than the population, numbers 531 * less than 1 = lower risk.). 532 */ 533 public BigDecimal getRelativeRisk() { 534 return this.relativeRisk == null ? null : this.relativeRisk.getValue(); 535 } 536 537 /** 538 * @param value Indicates the risk for this particular subject (with their 539 * specific characteristics) divided by the risk of the population 540 * in general. (Numbers greater than 1 = higher risk than the 541 * population, numbers less than 1 = lower risk.). 542 */ 543 public RiskAssessmentPredictionComponent setRelativeRisk(BigDecimal value) { 544 if (value == null) 545 this.relativeRisk = null; 546 else { 547 if (this.relativeRisk == null) 548 this.relativeRisk = new DecimalType(); 549 this.relativeRisk.setValue(value); 550 } 551 return this; 552 } 553 554 /** 555 * @param value Indicates the risk for this particular subject (with their 556 * specific characteristics) divided by the risk of the population 557 * in general. (Numbers greater than 1 = higher risk than the 558 * population, numbers less than 1 = lower risk.). 559 */ 560 public RiskAssessmentPredictionComponent setRelativeRisk(long value) { 561 this.relativeRisk = new DecimalType(); 562 this.relativeRisk.setValue(value); 563 return this; 564 } 565 566 /** 567 * @param value Indicates the risk for this particular subject (with their 568 * specific characteristics) divided by the risk of the population 569 * in general. (Numbers greater than 1 = higher risk than the 570 * population, numbers less than 1 = lower risk.). 571 */ 572 public RiskAssessmentPredictionComponent setRelativeRisk(double value) { 573 this.relativeRisk = new DecimalType(); 574 this.relativeRisk.setValue(value); 575 return this; 576 } 577 578 /** 579 * @return {@link #when} (Indicates the period of time or age range of the 580 * subject to which the specified probability applies.) 581 */ 582 public Type getWhen() { 583 return this.when; 584 } 585 586 /** 587 * @return {@link #when} (Indicates the period of time or age range of the 588 * subject to which the specified probability applies.) 589 */ 590 public Period getWhenPeriod() throws FHIRException { 591 if (this.when == null) 592 this.when = new Period(); 593 if (!(this.when instanceof Period)) 594 throw new FHIRException( 595 "Type mismatch: the type Period was expected, but " + this.when.getClass().getName() + " was encountered"); 596 return (Period) this.when; 597 } 598 599 public boolean hasWhenPeriod() { 600 return this != null && this.when instanceof Period; 601 } 602 603 /** 604 * @return {@link #when} (Indicates the period of time or age range of the 605 * subject to which the specified probability applies.) 606 */ 607 public Range getWhenRange() throws FHIRException { 608 if (this.when == null) 609 this.when = new Range(); 610 if (!(this.when instanceof Range)) 611 throw new FHIRException( 612 "Type mismatch: the type Range was expected, but " + this.when.getClass().getName() + " was encountered"); 613 return (Range) this.when; 614 } 615 616 public boolean hasWhenRange() { 617 return this != null && this.when instanceof Range; 618 } 619 620 public boolean hasWhen() { 621 return this.when != null && !this.when.isEmpty(); 622 } 623 624 /** 625 * @param value {@link #when} (Indicates the period of time or age range of the 626 * subject to which the specified probability applies.) 627 */ 628 public RiskAssessmentPredictionComponent setWhen(Type value) { 629 if (value != null && !(value instanceof Period || value instanceof Range)) 630 throw new Error("Not the right type for RiskAssessment.prediction.when[x]: " + value.fhirType()); 631 this.when = value; 632 return this; 633 } 634 635 /** 636 * @return {@link #rationale} (Additional information explaining the basis for 637 * the prediction.). This is the underlying object with id, value and 638 * extensions. The accessor "getRationale" gives direct access to the 639 * value 640 */ 641 public StringType getRationaleElement() { 642 if (this.rationale == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create RiskAssessmentPredictionComponent.rationale"); 645 else if (Configuration.doAutoCreate()) 646 this.rationale = new StringType(); // bb 647 return this.rationale; 648 } 649 650 public boolean hasRationaleElement() { 651 return this.rationale != null && !this.rationale.isEmpty(); 652 } 653 654 public boolean hasRationale() { 655 return this.rationale != null && !this.rationale.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #rationale} (Additional information explaining the basis 660 * for the prediction.). This is the underlying object with id, 661 * value and extensions. The accessor "getRationale" gives direct 662 * access to the value 663 */ 664 public RiskAssessmentPredictionComponent setRationaleElement(StringType value) { 665 this.rationale = value; 666 return this; 667 } 668 669 /** 670 * @return Additional information explaining the basis for the prediction. 671 */ 672 public String getRationale() { 673 return this.rationale == null ? null : this.rationale.getValue(); 674 } 675 676 /** 677 * @param value Additional information explaining the basis for the prediction. 678 */ 679 public RiskAssessmentPredictionComponent setRationale(String value) { 680 if (Utilities.noString(value)) 681 this.rationale = null; 682 else { 683 if (this.rationale == null) 684 this.rationale = new StringType(); 685 this.rationale.setValue(value); 686 } 687 return this; 688 } 689 690 protected void listChildren(List<Property> children) { 691 super.listChildren(children); 692 children.add(new Property("outcome", "CodeableConcept", 693 "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 1, 694 outcome)); 695 children.add(new Property("probability[x]", "decimal|Range", 696 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability)); 697 children.add(new Property("qualitativeRisk", "CodeableConcept", 698 "Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).", 699 0, 1, qualitativeRisk)); 700 children.add(new Property("relativeRisk", "decimal", 701 "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 702 0, 1, relativeRisk)); 703 children.add(new Property("when[x]", "Period|Range", 704 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 1, 705 when)); 706 children.add(new Property("rationale", "string", 707 "Additional information explaining the basis for the prediction.", 0, 1, rationale)); 708 } 709 710 @Override 711 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 712 switch (_hash) { 713 case -1106507950: 714 /* outcome */ return new Property("outcome", "CodeableConcept", 715 "One of the potential outcomes for the patient (e.g. remission, death, a particular condition).", 0, 1, 716 outcome); 717 case 1430185003: 718 /* probability[x] */ return new Property("probability[x]", "decimal|Range", 719 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 720 case -1290561483: 721 /* probability */ return new Property("probability[x]", "decimal|Range", 722 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 723 case 888495452: 724 /* probabilityDecimal */ return new Property("probability[x]", "decimal|Range", 725 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 726 case 9275912: 727 /* probabilityRange */ return new Property("probability[x]", "decimal|Range", 728 "Indicates how likely the outcome is (in the specified timeframe).", 0, 1, probability); 729 case 123308730: 730 /* qualitativeRisk */ return new Property("qualitativeRisk", "CodeableConcept", 731 "Indicates how likely the outcome is (in the specified timeframe), expressed as a qualitative value (e.g. low, medium, or high).", 732 0, 1, qualitativeRisk); 733 case -70741061: 734 /* relativeRisk */ return new Property("relativeRisk", "decimal", 735 "Indicates the risk for this particular subject (with their specific characteristics) divided by the risk of the population in general. (Numbers greater than 1 = higher risk than the population, numbers less than 1 = lower risk.).", 736 0, 1, relativeRisk); 737 case 1312831238: 738 /* when[x] */ return new Property("when[x]", "Period|Range", 739 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 740 1, when); 741 case 3648314: 742 /* when */ return new Property("when[x]", "Period|Range", 743 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 744 1, when); 745 case 251476379: 746 /* whenPeriod */ return new Property("when[x]", "Period|Range", 747 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 748 1, when); 749 case -1098542557: 750 /* whenRange */ return new Property("when[x]", "Period|Range", 751 "Indicates the period of time or age range of the subject to which the specified probability applies.", 0, 752 1, when); 753 case 345689335: 754 /* rationale */ return new Property("rationale", "string", 755 "Additional information explaining the basis for the prediction.", 0, 1, rationale); 756 default: 757 return super.getNamedProperty(_hash, _name, _checkValid); 758 } 759 760 } 761 762 @Override 763 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 764 switch (hash) { 765 case -1106507950: 766 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // CodeableConcept 767 case -1290561483: 768 /* probability */ return this.probability == null ? new Base[0] : new Base[] { this.probability }; // Type 769 case 123308730: 770 /* qualitativeRisk */ return this.qualitativeRisk == null ? new Base[0] : new Base[] { this.qualitativeRisk }; // CodeableConcept 771 case -70741061: 772 /* relativeRisk */ return this.relativeRisk == null ? new Base[0] : new Base[] { this.relativeRisk }; // DecimalType 773 case 3648314: 774 /* when */ return this.when == null ? new Base[0] : new Base[] { this.when }; // Type 775 case 345689335: 776 /* rationale */ return this.rationale == null ? new Base[0] : new Base[] { this.rationale }; // StringType 777 default: 778 return super.getProperty(hash, name, checkValid); 779 } 780 781 } 782 783 @Override 784 public Base setProperty(int hash, String name, Base value) throws FHIRException { 785 switch (hash) { 786 case -1106507950: // outcome 787 this.outcome = castToCodeableConcept(value); // CodeableConcept 788 return value; 789 case -1290561483: // probability 790 this.probability = castToType(value); // Type 791 return value; 792 case 123308730: // qualitativeRisk 793 this.qualitativeRisk = castToCodeableConcept(value); // CodeableConcept 794 return value; 795 case -70741061: // relativeRisk 796 this.relativeRisk = castToDecimal(value); // DecimalType 797 return value; 798 case 3648314: // when 799 this.when = castToType(value); // Type 800 return value; 801 case 345689335: // rationale 802 this.rationale = castToString(value); // StringType 803 return value; 804 default: 805 return super.setProperty(hash, name, value); 806 } 807 808 } 809 810 @Override 811 public Base setProperty(String name, Base value) throws FHIRException { 812 if (name.equals("outcome")) { 813 this.outcome = castToCodeableConcept(value); // CodeableConcept 814 } else if (name.equals("probability[x]")) { 815 this.probability = castToType(value); // Type 816 } else if (name.equals("qualitativeRisk")) { 817 this.qualitativeRisk = castToCodeableConcept(value); // CodeableConcept 818 } else if (name.equals("relativeRisk")) { 819 this.relativeRisk = castToDecimal(value); // DecimalType 820 } else if (name.equals("when[x]")) { 821 this.when = castToType(value); // Type 822 } else if (name.equals("rationale")) { 823 this.rationale = castToString(value); // StringType 824 } else 825 return super.setProperty(name, value); 826 return value; 827 } 828 829 @Override 830 public void removeChild(String name, Base value) throws FHIRException { 831 if (name.equals("outcome")) { 832 this.outcome = null; 833 } else if (name.equals("probability[x]")) { 834 this.probability = null; 835 } else if (name.equals("qualitativeRisk")) { 836 this.qualitativeRisk = null; 837 } else if (name.equals("relativeRisk")) { 838 this.relativeRisk = null; 839 } else if (name.equals("when[x]")) { 840 this.when = null; 841 } else if (name.equals("rationale")) { 842 this.rationale = null; 843 } else 844 super.removeChild(name, value); 845 846 } 847 848 @Override 849 public Base makeProperty(int hash, String name) throws FHIRException { 850 switch (hash) { 851 case -1106507950: 852 return getOutcome(); 853 case 1430185003: 854 return getProbability(); 855 case -1290561483: 856 return getProbability(); 857 case 123308730: 858 return getQualitativeRisk(); 859 case -70741061: 860 return getRelativeRiskElement(); 861 case 1312831238: 862 return getWhen(); 863 case 3648314: 864 return getWhen(); 865 case 345689335: 866 return getRationaleElement(); 867 default: 868 return super.makeProperty(hash, name); 869 } 870 871 } 872 873 @Override 874 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 875 switch (hash) { 876 case -1106507950: 877 /* outcome */ return new String[] { "CodeableConcept" }; 878 case -1290561483: 879 /* probability */ return new String[] { "decimal", "Range" }; 880 case 123308730: 881 /* qualitativeRisk */ return new String[] { "CodeableConcept" }; 882 case -70741061: 883 /* relativeRisk */ return new String[] { "decimal" }; 884 case 3648314: 885 /* when */ return new String[] { "Period", "Range" }; 886 case 345689335: 887 /* rationale */ return new String[] { "string" }; 888 default: 889 return super.getTypesForProperty(hash, name); 890 } 891 892 } 893 894 @Override 895 public Base addChild(String name) throws FHIRException { 896 if (name.equals("outcome")) { 897 this.outcome = new CodeableConcept(); 898 return this.outcome; 899 } else if (name.equals("probabilityDecimal")) { 900 this.probability = new DecimalType(); 901 return this.probability; 902 } else if (name.equals("probabilityRange")) { 903 this.probability = new Range(); 904 return this.probability; 905 } else if (name.equals("qualitativeRisk")) { 906 this.qualitativeRisk = new CodeableConcept(); 907 return this.qualitativeRisk; 908 } else if (name.equals("relativeRisk")) { 909 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.relativeRisk"); 910 } else if (name.equals("whenPeriod")) { 911 this.when = new Period(); 912 return this.when; 913 } else if (name.equals("whenRange")) { 914 this.when = new Range(); 915 return this.when; 916 } else if (name.equals("rationale")) { 917 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.rationale"); 918 } else 919 return super.addChild(name); 920 } 921 922 public RiskAssessmentPredictionComponent copy() { 923 RiskAssessmentPredictionComponent dst = new RiskAssessmentPredictionComponent(); 924 copyValues(dst); 925 return dst; 926 } 927 928 public void copyValues(RiskAssessmentPredictionComponent dst) { 929 super.copyValues(dst); 930 dst.outcome = outcome == null ? null : outcome.copy(); 931 dst.probability = probability == null ? null : probability.copy(); 932 dst.qualitativeRisk = qualitativeRisk == null ? null : qualitativeRisk.copy(); 933 dst.relativeRisk = relativeRisk == null ? null : relativeRisk.copy(); 934 dst.when = when == null ? null : when.copy(); 935 dst.rationale = rationale == null ? null : rationale.copy(); 936 } 937 938 @Override 939 public boolean equalsDeep(Base other_) { 940 if (!super.equalsDeep(other_)) 941 return false; 942 if (!(other_ instanceof RiskAssessmentPredictionComponent)) 943 return false; 944 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other_; 945 return compareDeep(outcome, o.outcome, true) && compareDeep(probability, o.probability, true) 946 && compareDeep(qualitativeRisk, o.qualitativeRisk, true) && compareDeep(relativeRisk, o.relativeRisk, true) 947 && compareDeep(when, o.when, true) && compareDeep(rationale, o.rationale, true); 948 } 949 950 @Override 951 public boolean equalsShallow(Base other_) { 952 if (!super.equalsShallow(other_)) 953 return false; 954 if (!(other_ instanceof RiskAssessmentPredictionComponent)) 955 return false; 956 RiskAssessmentPredictionComponent o = (RiskAssessmentPredictionComponent) other_; 957 return compareValues(relativeRisk, o.relativeRisk, true) && compareValues(rationale, o.rationale, true); 958 } 959 960 public boolean isEmpty() { 961 return super.isEmpty() 962 && ca.uhn.fhir.util.ElementUtil.isEmpty(outcome, probability, qualitativeRisk, relativeRisk, when, rationale); 963 } 964 965 public String fhirType() { 966 return "RiskAssessment.prediction"; 967 968 } 969 970 } 971 972 /** 973 * Business identifier assigned to the risk assessment. 974 */ 975 @Child(name = "identifier", type = { 976 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 977 @Description(shortDefinition = "Unique identifier for the assessment", formalDefinition = "Business identifier assigned to the risk assessment.") 978 protected List<Identifier> identifier; 979 980 /** 981 * A reference to the request that is fulfilled by this risk assessment. 982 */ 983 @Child(name = "basedOn", type = { Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 984 @Description(shortDefinition = "Request fulfilled by this assessment", formalDefinition = "A reference to the request that is fulfilled by this risk assessment.") 985 protected Reference basedOn; 986 987 /** 988 * The actual object that is the target of the reference (A reference to the 989 * request that is fulfilled by this risk assessment.) 990 */ 991 protected Resource basedOnTarget; 992 993 /** 994 * A reference to a resource that this risk assessment is part of, such as a 995 * Procedure. 996 */ 997 @Child(name = "parent", type = { Reference.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 998 @Description(shortDefinition = "Part of this occurrence", formalDefinition = "A reference to a resource that this risk assessment is part of, such as a Procedure.") 999 protected Reference parent; 1000 1001 /** 1002 * The actual object that is the target of the reference (A reference to a 1003 * resource that this risk assessment is part of, such as a Procedure.) 1004 */ 1005 protected Resource parentTarget; 1006 1007 /** 1008 * The status of the RiskAssessment, using the same statuses as an Observation. 1009 */ 1010 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1011 @Description(shortDefinition = "registered | preliminary | final | amended +", formalDefinition = "The status of the RiskAssessment, using the same statuses as an Observation.") 1012 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-status") 1013 protected Enumeration<RiskAssessmentStatus> status; 1014 1015 /** 1016 * The algorithm, process or mechanism used to evaluate the risk. 1017 */ 1018 @Child(name = "method", type = { 1019 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1020 @Description(shortDefinition = "Evaluation mechanism", formalDefinition = "The algorithm, process or mechanism used to evaluate the risk.") 1021 protected CodeableConcept method; 1022 1023 /** 1024 * The type of the risk assessment performed. 1025 */ 1026 @Child(name = "code", type = { CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1027 @Description(shortDefinition = "Type of assessment", formalDefinition = "The type of the risk assessment performed.") 1028 protected CodeableConcept code; 1029 1030 /** 1031 * The patient or group the risk assessment applies to. 1032 */ 1033 @Child(name = "subject", type = { Patient.class, 1034 Group.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1035 @Description(shortDefinition = "Who/what does assessment apply to?", formalDefinition = "The patient or group the risk assessment applies to.") 1036 protected Reference subject; 1037 1038 /** 1039 * The actual object that is the target of the reference (The patient or group 1040 * the risk assessment applies to.) 1041 */ 1042 protected Resource subjectTarget; 1043 1044 /** 1045 * The encounter where the assessment was performed. 1046 */ 1047 @Child(name = "encounter", type = { Encounter.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1048 @Description(shortDefinition = "Where was assessment performed?", formalDefinition = "The encounter where the assessment was performed.") 1049 protected Reference encounter; 1050 1051 /** 1052 * The actual object that is the target of the reference (The encounter where 1053 * the assessment was performed.) 1054 */ 1055 protected Encounter encounterTarget; 1056 1057 /** 1058 * The date (and possibly time) the risk assessment was performed. 1059 */ 1060 @Child(name = "occurrence", type = { DateTimeType.class, 1061 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1062 @Description(shortDefinition = "When was assessment made?", formalDefinition = "The date (and possibly time) the risk assessment was performed.") 1063 protected Type occurrence; 1064 1065 /** 1066 * For assessments or prognosis specific to a particular condition, indicates 1067 * the condition being assessed. 1068 */ 1069 @Child(name = "condition", type = { Condition.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1070 @Description(shortDefinition = "Condition assessed", formalDefinition = "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.") 1071 protected Reference condition; 1072 1073 /** 1074 * The actual object that is the target of the reference (For assessments or 1075 * prognosis specific to a particular condition, indicates the condition being 1076 * assessed.) 1077 */ 1078 protected Condition conditionTarget; 1079 1080 /** 1081 * The provider or software application that performed the assessment. 1082 */ 1083 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, 1084 Device.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1085 @Description(shortDefinition = "Who did assessment?", formalDefinition = "The provider or software application that performed the assessment.") 1086 protected Reference performer; 1087 1088 /** 1089 * The actual object that is the target of the reference (The provider or 1090 * software application that performed the assessment.) 1091 */ 1092 protected Resource performerTarget; 1093 1094 /** 1095 * The reason the risk assessment was performed. 1096 */ 1097 @Child(name = "reasonCode", type = { 1098 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1099 @Description(shortDefinition = "Why the assessment was necessary?", formalDefinition = "The reason the risk assessment was performed.") 1100 protected List<CodeableConcept> reasonCode; 1101 1102 /** 1103 * Resources supporting the reason the risk assessment was performed. 1104 */ 1105 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 1106 DocumentReference.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1107 @Description(shortDefinition = "Why the assessment was necessary?", formalDefinition = "Resources supporting the reason the risk assessment was performed.") 1108 protected List<Reference> reasonReference; 1109 /** 1110 * The actual objects that are the target of the reference (Resources supporting 1111 * the reason the risk assessment was performed.) 1112 */ 1113 protected List<Resource> reasonReferenceTarget; 1114 1115 /** 1116 * Indicates the source data considered as part of the assessment (for example, 1117 * FamilyHistory, Observations, Procedures, Conditions, etc.). 1118 */ 1119 @Child(name = "basis", type = { 1120 Reference.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1121 @Description(shortDefinition = "Information used in assessment", formalDefinition = "Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).") 1122 protected List<Reference> basis; 1123 /** 1124 * The actual objects that are the target of the reference (Indicates the source 1125 * data considered as part of the assessment (for example, FamilyHistory, 1126 * Observations, Procedures, Conditions, etc.).) 1127 */ 1128 protected List<Resource> basisTarget; 1129 1130 /** 1131 * Describes the expected outcome for the subject. 1132 */ 1133 @Child(name = "prediction", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1134 @Description(shortDefinition = "Outcome predicted", formalDefinition = "Describes the expected outcome for the subject.") 1135 protected List<RiskAssessmentPredictionComponent> prediction; 1136 1137 /** 1138 * A description of the steps that might be taken to reduce the identified 1139 * risk(s). 1140 */ 1141 @Child(name = "mitigation", type = { 1142 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1143 @Description(shortDefinition = "How to reduce risk", formalDefinition = "A description of the steps that might be taken to reduce the identified risk(s).") 1144 protected StringType mitigation; 1145 1146 /** 1147 * Additional comments about the risk assessment. 1148 */ 1149 @Child(name = "note", type = { 1150 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1151 @Description(shortDefinition = "Comments on the risk assessment", formalDefinition = "Additional comments about the risk assessment.") 1152 protected List<Annotation> note; 1153 1154 private static final long serialVersionUID = -2137260218L; 1155 1156 /** 1157 * Constructor 1158 */ 1159 public RiskAssessment() { 1160 super(); 1161 } 1162 1163 /** 1164 * Constructor 1165 */ 1166 public RiskAssessment(Enumeration<RiskAssessmentStatus> status, Reference subject) { 1167 super(); 1168 this.status = status; 1169 this.subject = subject; 1170 } 1171 1172 /** 1173 * @return {@link #identifier} (Business identifier assigned to the risk 1174 * assessment.) 1175 */ 1176 public List<Identifier> getIdentifier() { 1177 if (this.identifier == null) 1178 this.identifier = new ArrayList<Identifier>(); 1179 return this.identifier; 1180 } 1181 1182 /** 1183 * @return Returns a reference to <code>this</code> for easy method chaining 1184 */ 1185 public RiskAssessment setIdentifier(List<Identifier> theIdentifier) { 1186 this.identifier = theIdentifier; 1187 return this; 1188 } 1189 1190 public boolean hasIdentifier() { 1191 if (this.identifier == null) 1192 return false; 1193 for (Identifier item : this.identifier) 1194 if (!item.isEmpty()) 1195 return true; 1196 return false; 1197 } 1198 1199 public Identifier addIdentifier() { // 3 1200 Identifier t = new Identifier(); 1201 if (this.identifier == null) 1202 this.identifier = new ArrayList<Identifier>(); 1203 this.identifier.add(t); 1204 return t; 1205 } 1206 1207 public RiskAssessment addIdentifier(Identifier t) { // 3 1208 if (t == null) 1209 return this; 1210 if (this.identifier == null) 1211 this.identifier = new ArrayList<Identifier>(); 1212 this.identifier.add(t); 1213 return this; 1214 } 1215 1216 /** 1217 * @return The first repetition of repeating field {@link #identifier}, creating 1218 * it if it does not already exist 1219 */ 1220 public Identifier getIdentifierFirstRep() { 1221 if (getIdentifier().isEmpty()) { 1222 addIdentifier(); 1223 } 1224 return getIdentifier().get(0); 1225 } 1226 1227 /** 1228 * @return {@link #basedOn} (A reference to the request that is fulfilled by 1229 * this risk assessment.) 1230 */ 1231 public Reference getBasedOn() { 1232 if (this.basedOn == null) 1233 if (Configuration.errorOnAutoCreate()) 1234 throw new Error("Attempt to auto-create RiskAssessment.basedOn"); 1235 else if (Configuration.doAutoCreate()) 1236 this.basedOn = new Reference(); // cc 1237 return this.basedOn; 1238 } 1239 1240 public boolean hasBasedOn() { 1241 return this.basedOn != null && !this.basedOn.isEmpty(); 1242 } 1243 1244 /** 1245 * @param value {@link #basedOn} (A reference to the request that is fulfilled 1246 * by this risk assessment.) 1247 */ 1248 public RiskAssessment setBasedOn(Reference value) { 1249 this.basedOn = value; 1250 return this; 1251 } 1252 1253 /** 1254 * @return {@link #basedOn} The actual object that is the target of the 1255 * reference. The reference library doesn't populate this, but you can 1256 * use it to hold the resource if you resolve it. (A reference to the 1257 * request that is fulfilled by this risk assessment.) 1258 */ 1259 public Resource getBasedOnTarget() { 1260 return this.basedOnTarget; 1261 } 1262 1263 /** 1264 * @param value {@link #basedOn} The actual object that is the target of the 1265 * reference. The reference library doesn't use these, but you can 1266 * use it to hold the resource if you resolve it. (A reference to 1267 * the request that is fulfilled by this risk assessment.) 1268 */ 1269 public RiskAssessment setBasedOnTarget(Resource value) { 1270 this.basedOnTarget = value; 1271 return this; 1272 } 1273 1274 /** 1275 * @return {@link #parent} (A reference to a resource that this risk assessment 1276 * is part of, such as a Procedure.) 1277 */ 1278 public Reference getParent() { 1279 if (this.parent == null) 1280 if (Configuration.errorOnAutoCreate()) 1281 throw new Error("Attempt to auto-create RiskAssessment.parent"); 1282 else if (Configuration.doAutoCreate()) 1283 this.parent = new Reference(); // cc 1284 return this.parent; 1285 } 1286 1287 public boolean hasParent() { 1288 return this.parent != null && !this.parent.isEmpty(); 1289 } 1290 1291 /** 1292 * @param value {@link #parent} (A reference to a resource that this risk 1293 * assessment is part of, such as a Procedure.) 1294 */ 1295 public RiskAssessment setParent(Reference value) { 1296 this.parent = value; 1297 return this; 1298 } 1299 1300 /** 1301 * @return {@link #parent} The actual object that is the target of the 1302 * reference. The reference library doesn't populate this, but you can 1303 * use it to hold the resource if you resolve it. (A reference to a 1304 * resource that this risk assessment is part of, such as a Procedure.) 1305 */ 1306 public Resource getParentTarget() { 1307 return this.parentTarget; 1308 } 1309 1310 /** 1311 * @param value {@link #parent} The actual object that is the target of the 1312 * reference. The reference library doesn't use these, but you can 1313 * use it to hold the resource if you resolve it. (A reference to a 1314 * resource that this risk assessment is part of, such as a 1315 * Procedure.) 1316 */ 1317 public RiskAssessment setParentTarget(Resource value) { 1318 this.parentTarget = value; 1319 return this; 1320 } 1321 1322 /** 1323 * @return {@link #status} (The status of the RiskAssessment, using the same 1324 * statuses as an Observation.). This is the underlying object with id, 1325 * value and extensions. The accessor "getStatus" gives direct access to 1326 * the value 1327 */ 1328 public Enumeration<RiskAssessmentStatus> getStatusElement() { 1329 if (this.status == null) 1330 if (Configuration.errorOnAutoCreate()) 1331 throw new Error("Attempt to auto-create RiskAssessment.status"); 1332 else if (Configuration.doAutoCreate()) 1333 this.status = new Enumeration<RiskAssessmentStatus>(new RiskAssessmentStatusEnumFactory()); // bb 1334 return this.status; 1335 } 1336 1337 public boolean hasStatusElement() { 1338 return this.status != null && !this.status.isEmpty(); 1339 } 1340 1341 public boolean hasStatus() { 1342 return this.status != null && !this.status.isEmpty(); 1343 } 1344 1345 /** 1346 * @param value {@link #status} (The status of the RiskAssessment, using the 1347 * same statuses as an Observation.). This is the underlying object 1348 * with id, value and extensions. The accessor "getStatus" gives 1349 * direct access to the value 1350 */ 1351 public RiskAssessment setStatusElement(Enumeration<RiskAssessmentStatus> value) { 1352 this.status = value; 1353 return this; 1354 } 1355 1356 /** 1357 * @return The status of the RiskAssessment, using the same statuses as an 1358 * Observation. 1359 */ 1360 public RiskAssessmentStatus getStatus() { 1361 return this.status == null ? null : this.status.getValue(); 1362 } 1363 1364 /** 1365 * @param value The status of the RiskAssessment, using the same statuses as an 1366 * Observation. 1367 */ 1368 public RiskAssessment setStatus(RiskAssessmentStatus value) { 1369 if (this.status == null) 1370 this.status = new Enumeration<RiskAssessmentStatus>(new RiskAssessmentStatusEnumFactory()); 1371 this.status.setValue(value); 1372 return this; 1373 } 1374 1375 /** 1376 * @return {@link #method} (The algorithm, process or mechanism used to evaluate 1377 * the risk.) 1378 */ 1379 public CodeableConcept getMethod() { 1380 if (this.method == null) 1381 if (Configuration.errorOnAutoCreate()) 1382 throw new Error("Attempt to auto-create RiskAssessment.method"); 1383 else if (Configuration.doAutoCreate()) 1384 this.method = new CodeableConcept(); // cc 1385 return this.method; 1386 } 1387 1388 public boolean hasMethod() { 1389 return this.method != null && !this.method.isEmpty(); 1390 } 1391 1392 /** 1393 * @param value {@link #method} (The algorithm, process or mechanism used to 1394 * evaluate the risk.) 1395 */ 1396 public RiskAssessment setMethod(CodeableConcept value) { 1397 this.method = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return {@link #code} (The type of the risk assessment performed.) 1403 */ 1404 public CodeableConcept getCode() { 1405 if (this.code == null) 1406 if (Configuration.errorOnAutoCreate()) 1407 throw new Error("Attempt to auto-create RiskAssessment.code"); 1408 else if (Configuration.doAutoCreate()) 1409 this.code = new CodeableConcept(); // cc 1410 return this.code; 1411 } 1412 1413 public boolean hasCode() { 1414 return this.code != null && !this.code.isEmpty(); 1415 } 1416 1417 /** 1418 * @param value {@link #code} (The type of the risk assessment performed.) 1419 */ 1420 public RiskAssessment setCode(CodeableConcept value) { 1421 this.code = value; 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #subject} (The patient or group the risk assessment applies 1427 * to.) 1428 */ 1429 public Reference getSubject() { 1430 if (this.subject == null) 1431 if (Configuration.errorOnAutoCreate()) 1432 throw new Error("Attempt to auto-create RiskAssessment.subject"); 1433 else if (Configuration.doAutoCreate()) 1434 this.subject = new Reference(); // cc 1435 return this.subject; 1436 } 1437 1438 public boolean hasSubject() { 1439 return this.subject != null && !this.subject.isEmpty(); 1440 } 1441 1442 /** 1443 * @param value {@link #subject} (The patient or group the risk assessment 1444 * applies to.) 1445 */ 1446 public RiskAssessment setSubject(Reference value) { 1447 this.subject = value; 1448 return this; 1449 } 1450 1451 /** 1452 * @return {@link #subject} The actual object that is the target of the 1453 * reference. The reference library doesn't populate this, but you can 1454 * use it to hold the resource if you resolve it. (The patient or group 1455 * the risk assessment applies to.) 1456 */ 1457 public Resource getSubjectTarget() { 1458 return this.subjectTarget; 1459 } 1460 1461 /** 1462 * @param value {@link #subject} The actual object that is the target of the 1463 * reference. The reference library doesn't use these, but you can 1464 * use it to hold the resource if you resolve it. (The patient or 1465 * group the risk assessment applies to.) 1466 */ 1467 public RiskAssessment setSubjectTarget(Resource value) { 1468 this.subjectTarget = value; 1469 return this; 1470 } 1471 1472 /** 1473 * @return {@link #encounter} (The encounter where the assessment was 1474 * performed.) 1475 */ 1476 public Reference getEncounter() { 1477 if (this.encounter == null) 1478 if (Configuration.errorOnAutoCreate()) 1479 throw new Error("Attempt to auto-create RiskAssessment.encounter"); 1480 else if (Configuration.doAutoCreate()) 1481 this.encounter = new Reference(); // cc 1482 return this.encounter; 1483 } 1484 1485 public boolean hasEncounter() { 1486 return this.encounter != null && !this.encounter.isEmpty(); 1487 } 1488 1489 /** 1490 * @param value {@link #encounter} (The encounter where the assessment was 1491 * performed.) 1492 */ 1493 public RiskAssessment setEncounter(Reference value) { 1494 this.encounter = value; 1495 return this; 1496 } 1497 1498 /** 1499 * @return {@link #encounter} The actual object that is the target of the 1500 * reference. The reference library doesn't populate this, but you can 1501 * use it to hold the resource if you resolve it. (The encounter where 1502 * the assessment was performed.) 1503 */ 1504 public Encounter getEncounterTarget() { 1505 if (this.encounterTarget == null) 1506 if (Configuration.errorOnAutoCreate()) 1507 throw new Error("Attempt to auto-create RiskAssessment.encounter"); 1508 else if (Configuration.doAutoCreate()) 1509 this.encounterTarget = new Encounter(); // aa 1510 return this.encounterTarget; 1511 } 1512 1513 /** 1514 * @param value {@link #encounter} The actual object that is the target of the 1515 * reference. The reference library doesn't use these, but you can 1516 * use it to hold the resource if you resolve it. (The encounter 1517 * where the assessment was performed.) 1518 */ 1519 public RiskAssessment setEncounterTarget(Encounter value) { 1520 this.encounterTarget = value; 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #occurrence} (The date (and possibly time) the risk assessment 1526 * was performed.) 1527 */ 1528 public Type getOccurrence() { 1529 return this.occurrence; 1530 } 1531 1532 /** 1533 * @return {@link #occurrence} (The date (and possibly time) the risk assessment 1534 * was performed.) 1535 */ 1536 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1537 if (this.occurrence == null) 1538 this.occurrence = new DateTimeType(); 1539 if (!(this.occurrence instanceof DateTimeType)) 1540 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1541 + this.occurrence.getClass().getName() + " was encountered"); 1542 return (DateTimeType) this.occurrence; 1543 } 1544 1545 public boolean hasOccurrenceDateTimeType() { 1546 return this != null && this.occurrence instanceof DateTimeType; 1547 } 1548 1549 /** 1550 * @return {@link #occurrence} (The date (and possibly time) the risk assessment 1551 * was performed.) 1552 */ 1553 public Period getOccurrencePeriod() throws FHIRException { 1554 if (this.occurrence == null) 1555 this.occurrence = new Period(); 1556 if (!(this.occurrence instanceof Period)) 1557 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1558 + " was encountered"); 1559 return (Period) this.occurrence; 1560 } 1561 1562 public boolean hasOccurrencePeriod() { 1563 return this != null && this.occurrence instanceof Period; 1564 } 1565 1566 public boolean hasOccurrence() { 1567 return this.occurrence != null && !this.occurrence.isEmpty(); 1568 } 1569 1570 /** 1571 * @param value {@link #occurrence} (The date (and possibly time) the risk 1572 * assessment was performed.) 1573 */ 1574 public RiskAssessment setOccurrence(Type value) { 1575 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1576 throw new Error("Not the right type for RiskAssessment.occurrence[x]: " + value.fhirType()); 1577 this.occurrence = value; 1578 return this; 1579 } 1580 1581 /** 1582 * @return {@link #condition} (For assessments or prognosis specific to a 1583 * particular condition, indicates the condition being assessed.) 1584 */ 1585 public Reference getCondition() { 1586 if (this.condition == null) 1587 if (Configuration.errorOnAutoCreate()) 1588 throw new Error("Attempt to auto-create RiskAssessment.condition"); 1589 else if (Configuration.doAutoCreate()) 1590 this.condition = new Reference(); // cc 1591 return this.condition; 1592 } 1593 1594 public boolean hasCondition() { 1595 return this.condition != null && !this.condition.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #condition} (For assessments or prognosis specific to a 1600 * particular condition, indicates the condition being assessed.) 1601 */ 1602 public RiskAssessment setCondition(Reference value) { 1603 this.condition = value; 1604 return this; 1605 } 1606 1607 /** 1608 * @return {@link #condition} The actual object that is the target of the 1609 * reference. The reference library doesn't populate this, but you can 1610 * use it to hold the resource if you resolve it. (For assessments or 1611 * prognosis specific to a particular condition, indicates the condition 1612 * being assessed.) 1613 */ 1614 public Condition getConditionTarget() { 1615 if (this.conditionTarget == null) 1616 if (Configuration.errorOnAutoCreate()) 1617 throw new Error("Attempt to auto-create RiskAssessment.condition"); 1618 else if (Configuration.doAutoCreate()) 1619 this.conditionTarget = new Condition(); // aa 1620 return this.conditionTarget; 1621 } 1622 1623 /** 1624 * @param value {@link #condition} The actual object that is the target of the 1625 * reference. The reference library doesn't use these, but you can 1626 * use it to hold the resource if you resolve it. (For assessments 1627 * or prognosis specific to a particular condition, indicates the 1628 * condition being assessed.) 1629 */ 1630 public RiskAssessment setConditionTarget(Condition value) { 1631 this.conditionTarget = value; 1632 return this; 1633 } 1634 1635 /** 1636 * @return {@link #performer} (The provider or software application that 1637 * performed the assessment.) 1638 */ 1639 public Reference getPerformer() { 1640 if (this.performer == null) 1641 if (Configuration.errorOnAutoCreate()) 1642 throw new Error("Attempt to auto-create RiskAssessment.performer"); 1643 else if (Configuration.doAutoCreate()) 1644 this.performer = new Reference(); // cc 1645 return this.performer; 1646 } 1647 1648 public boolean hasPerformer() { 1649 return this.performer != null && !this.performer.isEmpty(); 1650 } 1651 1652 /** 1653 * @param value {@link #performer} (The provider or software application that 1654 * performed the assessment.) 1655 */ 1656 public RiskAssessment setPerformer(Reference value) { 1657 this.performer = value; 1658 return this; 1659 } 1660 1661 /** 1662 * @return {@link #performer} The actual object that is the target of the 1663 * reference. The reference library doesn't populate this, but you can 1664 * use it to hold the resource if you resolve it. (The provider or 1665 * software application that performed the assessment.) 1666 */ 1667 public Resource getPerformerTarget() { 1668 return this.performerTarget; 1669 } 1670 1671 /** 1672 * @param value {@link #performer} The actual object that is the target of the 1673 * reference. The reference library doesn't use these, but you can 1674 * use it to hold the resource if you resolve it. (The provider or 1675 * software application that performed the assessment.) 1676 */ 1677 public RiskAssessment setPerformerTarget(Resource value) { 1678 this.performerTarget = value; 1679 return this; 1680 } 1681 1682 /** 1683 * @return {@link #reasonCode} (The reason the risk assessment was performed.) 1684 */ 1685 public List<CodeableConcept> getReasonCode() { 1686 if (this.reasonCode == null) 1687 this.reasonCode = new ArrayList<CodeableConcept>(); 1688 return this.reasonCode; 1689 } 1690 1691 /** 1692 * @return Returns a reference to <code>this</code> for easy method chaining 1693 */ 1694 public RiskAssessment setReasonCode(List<CodeableConcept> theReasonCode) { 1695 this.reasonCode = theReasonCode; 1696 return this; 1697 } 1698 1699 public boolean hasReasonCode() { 1700 if (this.reasonCode == null) 1701 return false; 1702 for (CodeableConcept item : this.reasonCode) 1703 if (!item.isEmpty()) 1704 return true; 1705 return false; 1706 } 1707 1708 public CodeableConcept addReasonCode() { // 3 1709 CodeableConcept t = new CodeableConcept(); 1710 if (this.reasonCode == null) 1711 this.reasonCode = new ArrayList<CodeableConcept>(); 1712 this.reasonCode.add(t); 1713 return t; 1714 } 1715 1716 public RiskAssessment addReasonCode(CodeableConcept t) { // 3 1717 if (t == null) 1718 return this; 1719 if (this.reasonCode == null) 1720 this.reasonCode = new ArrayList<CodeableConcept>(); 1721 this.reasonCode.add(t); 1722 return this; 1723 } 1724 1725 /** 1726 * @return The first repetition of repeating field {@link #reasonCode}, creating 1727 * it if it does not already exist 1728 */ 1729 public CodeableConcept getReasonCodeFirstRep() { 1730 if (getReasonCode().isEmpty()) { 1731 addReasonCode(); 1732 } 1733 return getReasonCode().get(0); 1734 } 1735 1736 /** 1737 * @return {@link #reasonReference} (Resources supporting the reason the risk 1738 * assessment was performed.) 1739 */ 1740 public List<Reference> getReasonReference() { 1741 if (this.reasonReference == null) 1742 this.reasonReference = new ArrayList<Reference>(); 1743 return this.reasonReference; 1744 } 1745 1746 /** 1747 * @return Returns a reference to <code>this</code> for easy method chaining 1748 */ 1749 public RiskAssessment setReasonReference(List<Reference> theReasonReference) { 1750 this.reasonReference = theReasonReference; 1751 return this; 1752 } 1753 1754 public boolean hasReasonReference() { 1755 if (this.reasonReference == null) 1756 return false; 1757 for (Reference item : this.reasonReference) 1758 if (!item.isEmpty()) 1759 return true; 1760 return false; 1761 } 1762 1763 public Reference addReasonReference() { // 3 1764 Reference t = new Reference(); 1765 if (this.reasonReference == null) 1766 this.reasonReference = new ArrayList<Reference>(); 1767 this.reasonReference.add(t); 1768 return t; 1769 } 1770 1771 public RiskAssessment addReasonReference(Reference t) { // 3 1772 if (t == null) 1773 return this; 1774 if (this.reasonReference == null) 1775 this.reasonReference = new ArrayList<Reference>(); 1776 this.reasonReference.add(t); 1777 return this; 1778 } 1779 1780 /** 1781 * @return The first repetition of repeating field {@link #reasonReference}, 1782 * creating it if it does not already exist 1783 */ 1784 public Reference getReasonReferenceFirstRep() { 1785 if (getReasonReference().isEmpty()) { 1786 addReasonReference(); 1787 } 1788 return getReasonReference().get(0); 1789 } 1790 1791 /** 1792 * @deprecated Use Reference#setResource(IBaseResource) instead 1793 */ 1794 @Deprecated 1795 public List<Resource> getReasonReferenceTarget() { 1796 if (this.reasonReferenceTarget == null) 1797 this.reasonReferenceTarget = new ArrayList<Resource>(); 1798 return this.reasonReferenceTarget; 1799 } 1800 1801 /** 1802 * @return {@link #basis} (Indicates the source data considered as part of the 1803 * assessment (for example, FamilyHistory, Observations, Procedures, 1804 * Conditions, etc.).) 1805 */ 1806 public List<Reference> getBasis() { 1807 if (this.basis == null) 1808 this.basis = new ArrayList<Reference>(); 1809 return this.basis; 1810 } 1811 1812 /** 1813 * @return Returns a reference to <code>this</code> for easy method chaining 1814 */ 1815 public RiskAssessment setBasis(List<Reference> theBasis) { 1816 this.basis = theBasis; 1817 return this; 1818 } 1819 1820 public boolean hasBasis() { 1821 if (this.basis == null) 1822 return false; 1823 for (Reference item : this.basis) 1824 if (!item.isEmpty()) 1825 return true; 1826 return false; 1827 } 1828 1829 public Reference addBasis() { // 3 1830 Reference t = new Reference(); 1831 if (this.basis == null) 1832 this.basis = new ArrayList<Reference>(); 1833 this.basis.add(t); 1834 return t; 1835 } 1836 1837 public RiskAssessment addBasis(Reference t) { // 3 1838 if (t == null) 1839 return this; 1840 if (this.basis == null) 1841 this.basis = new ArrayList<Reference>(); 1842 this.basis.add(t); 1843 return this; 1844 } 1845 1846 /** 1847 * @return The first repetition of repeating field {@link #basis}, creating it 1848 * if it does not already exist 1849 */ 1850 public Reference getBasisFirstRep() { 1851 if (getBasis().isEmpty()) { 1852 addBasis(); 1853 } 1854 return getBasis().get(0); 1855 } 1856 1857 /** 1858 * @deprecated Use Reference#setResource(IBaseResource) instead 1859 */ 1860 @Deprecated 1861 public List<Resource> getBasisTarget() { 1862 if (this.basisTarget == null) 1863 this.basisTarget = new ArrayList<Resource>(); 1864 return this.basisTarget; 1865 } 1866 1867 /** 1868 * @return {@link #prediction} (Describes the expected outcome for the subject.) 1869 */ 1870 public List<RiskAssessmentPredictionComponent> getPrediction() { 1871 if (this.prediction == null) 1872 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1873 return this.prediction; 1874 } 1875 1876 /** 1877 * @return Returns a reference to <code>this</code> for easy method chaining 1878 */ 1879 public RiskAssessment setPrediction(List<RiskAssessmentPredictionComponent> thePrediction) { 1880 this.prediction = thePrediction; 1881 return this; 1882 } 1883 1884 public boolean hasPrediction() { 1885 if (this.prediction == null) 1886 return false; 1887 for (RiskAssessmentPredictionComponent item : this.prediction) 1888 if (!item.isEmpty()) 1889 return true; 1890 return false; 1891 } 1892 1893 public RiskAssessmentPredictionComponent addPrediction() { // 3 1894 RiskAssessmentPredictionComponent t = new RiskAssessmentPredictionComponent(); 1895 if (this.prediction == null) 1896 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1897 this.prediction.add(t); 1898 return t; 1899 } 1900 1901 public RiskAssessment addPrediction(RiskAssessmentPredictionComponent t) { // 3 1902 if (t == null) 1903 return this; 1904 if (this.prediction == null) 1905 this.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 1906 this.prediction.add(t); 1907 return this; 1908 } 1909 1910 /** 1911 * @return The first repetition of repeating field {@link #prediction}, creating 1912 * it if it does not already exist 1913 */ 1914 public RiskAssessmentPredictionComponent getPredictionFirstRep() { 1915 if (getPrediction().isEmpty()) { 1916 addPrediction(); 1917 } 1918 return getPrediction().get(0); 1919 } 1920 1921 /** 1922 * @return {@link #mitigation} (A description of the steps that might be taken 1923 * to reduce the identified risk(s).). This is the underlying object 1924 * with id, value and extensions. The accessor "getMitigation" gives 1925 * direct access to the value 1926 */ 1927 public StringType getMitigationElement() { 1928 if (this.mitigation == null) 1929 if (Configuration.errorOnAutoCreate()) 1930 throw new Error("Attempt to auto-create RiskAssessment.mitigation"); 1931 else if (Configuration.doAutoCreate()) 1932 this.mitigation = new StringType(); // bb 1933 return this.mitigation; 1934 } 1935 1936 public boolean hasMitigationElement() { 1937 return this.mitigation != null && !this.mitigation.isEmpty(); 1938 } 1939 1940 public boolean hasMitigation() { 1941 return this.mitigation != null && !this.mitigation.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #mitigation} (A description of the steps that might be 1946 * taken to reduce the identified risk(s).). This is the underlying 1947 * object with id, value and extensions. The accessor 1948 * "getMitigation" gives direct access to the value 1949 */ 1950 public RiskAssessment setMitigationElement(StringType value) { 1951 this.mitigation = value; 1952 return this; 1953 } 1954 1955 /** 1956 * @return A description of the steps that might be taken to reduce the 1957 * identified risk(s). 1958 */ 1959 public String getMitigation() { 1960 return this.mitigation == null ? null : this.mitigation.getValue(); 1961 } 1962 1963 /** 1964 * @param value A description of the steps that might be taken to reduce the 1965 * identified risk(s). 1966 */ 1967 public RiskAssessment setMitigation(String value) { 1968 if (Utilities.noString(value)) 1969 this.mitigation = null; 1970 else { 1971 if (this.mitigation == null) 1972 this.mitigation = new StringType(); 1973 this.mitigation.setValue(value); 1974 } 1975 return this; 1976 } 1977 1978 /** 1979 * @return {@link #note} (Additional comments about the risk assessment.) 1980 */ 1981 public List<Annotation> getNote() { 1982 if (this.note == null) 1983 this.note = new ArrayList<Annotation>(); 1984 return this.note; 1985 } 1986 1987 /** 1988 * @return Returns a reference to <code>this</code> for easy method chaining 1989 */ 1990 public RiskAssessment setNote(List<Annotation> theNote) { 1991 this.note = theNote; 1992 return this; 1993 } 1994 1995 public boolean hasNote() { 1996 if (this.note == null) 1997 return false; 1998 for (Annotation item : this.note) 1999 if (!item.isEmpty()) 2000 return true; 2001 return false; 2002 } 2003 2004 public Annotation addNote() { // 3 2005 Annotation t = new Annotation(); 2006 if (this.note == null) 2007 this.note = new ArrayList<Annotation>(); 2008 this.note.add(t); 2009 return t; 2010 } 2011 2012 public RiskAssessment addNote(Annotation t) { // 3 2013 if (t == null) 2014 return this; 2015 if (this.note == null) 2016 this.note = new ArrayList<Annotation>(); 2017 this.note.add(t); 2018 return this; 2019 } 2020 2021 /** 2022 * @return The first repetition of repeating field {@link #note}, creating it if 2023 * it does not already exist 2024 */ 2025 public Annotation getNoteFirstRep() { 2026 if (getNote().isEmpty()) { 2027 addNote(); 2028 } 2029 return getNote().get(0); 2030 } 2031 2032 protected void listChildren(List<Property> children) { 2033 super.listChildren(children); 2034 children.add(new Property("identifier", "Identifier", "Business identifier assigned to the risk assessment.", 0, 2035 java.lang.Integer.MAX_VALUE, identifier)); 2036 children.add(new Property("basedOn", "Reference(Any)", 2037 "A reference to the request that is fulfilled by this risk assessment.", 0, 1, basedOn)); 2038 children.add(new Property("parent", "Reference(Any)", 2039 "A reference to a resource that this risk assessment is part of, such as a Procedure.", 0, 1, parent)); 2040 children.add(new Property("status", "code", 2041 "The status of the RiskAssessment, using the same statuses as an Observation.", 0, 1, status)); 2042 children.add(new Property("method", "CodeableConcept", 2043 "The algorithm, process or mechanism used to evaluate the risk.", 0, 1, method)); 2044 children.add(new Property("code", "CodeableConcept", "The type of the risk assessment performed.", 0, 1, code)); 2045 children.add(new Property("subject", "Reference(Patient|Group)", 2046 "The patient or group the risk assessment applies to.", 0, 1, subject)); 2047 children.add(new Property("encounter", "Reference(Encounter)", "The encounter where the assessment was performed.", 2048 0, 1, encounter)); 2049 children.add(new Property("occurrence[x]", "dateTime|Period", 2050 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence)); 2051 children.add(new Property("condition", "Reference(Condition)", 2052 "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 2053 1, condition)); 2054 children.add(new Property("performer", "Reference(Practitioner|PractitionerRole|Device)", 2055 "The provider or software application that performed the assessment.", 0, 1, performer)); 2056 children.add(new Property("reasonCode", "CodeableConcept", "The reason the risk assessment was performed.", 0, 2057 java.lang.Integer.MAX_VALUE, reasonCode)); 2058 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2059 "Resources supporting the reason the risk assessment was performed.", 0, java.lang.Integer.MAX_VALUE, 2060 reasonReference)); 2061 children.add(new Property("basis", "Reference(Any)", 2062 "Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).", 2063 0, java.lang.Integer.MAX_VALUE, basis)); 2064 children.add(new Property("prediction", "", "Describes the expected outcome for the subject.", 0, 2065 java.lang.Integer.MAX_VALUE, prediction)); 2066 children.add(new Property("mitigation", "string", 2067 "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1, mitigation)); 2068 children.add(new Property("note", "Annotation", "Additional comments about the risk assessment.", 0, 2069 java.lang.Integer.MAX_VALUE, note)); 2070 } 2071 2072 @Override 2073 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2074 switch (_hash) { 2075 case -1618432855: 2076 /* identifier */ return new Property("identifier", "Identifier", 2077 "Business identifier assigned to the risk assessment.", 0, java.lang.Integer.MAX_VALUE, identifier); 2078 case -332612366: 2079 /* basedOn */ return new Property("basedOn", "Reference(Any)", 2080 "A reference to the request that is fulfilled by this risk assessment.", 0, 1, basedOn); 2081 case -995424086: 2082 /* parent */ return new Property("parent", "Reference(Any)", 2083 "A reference to a resource that this risk assessment is part of, such as a Procedure.", 0, 1, parent); 2084 case -892481550: 2085 /* status */ return new Property("status", "code", 2086 "The status of the RiskAssessment, using the same statuses as an Observation.", 0, 1, status); 2087 case -1077554975: 2088 /* method */ return new Property("method", "CodeableConcept", 2089 "The algorithm, process or mechanism used to evaluate the risk.", 0, 1, method); 2090 case 3059181: 2091 /* code */ return new Property("code", "CodeableConcept", "The type of the risk assessment performed.", 0, 1, 2092 code); 2093 case -1867885268: 2094 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2095 "The patient or group the risk assessment applies to.", 0, 1, subject); 2096 case 1524132147: 2097 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2098 "The encounter where the assessment was performed.", 0, 1, encounter); 2099 case -2022646513: 2100 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period", 2101 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 2102 case 1687874001: 2103 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period", 2104 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 2105 case -298443636: 2106 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period", 2107 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 2108 case 1397156594: 2109 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period", 2110 "The date (and possibly time) the risk assessment was performed.", 0, 1, occurrence); 2111 case -861311717: 2112 /* condition */ return new Property("condition", "Reference(Condition)", 2113 "For assessments or prognosis specific to a particular condition, indicates the condition being assessed.", 0, 2114 1, condition); 2115 case 481140686: 2116 /* performer */ return new Property("performer", "Reference(Practitioner|PractitionerRole|Device)", 2117 "The provider or software application that performed the assessment.", 0, 1, performer); 2118 case 722137681: 2119 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2120 "The reason the risk assessment was performed.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2121 case -1146218137: 2122 /* reasonReference */ return new Property("reasonReference", 2123 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2124 "Resources supporting the reason the risk assessment was performed.", 0, java.lang.Integer.MAX_VALUE, 2125 reasonReference); 2126 case 93508670: 2127 /* basis */ return new Property("basis", "Reference(Any)", 2128 "Indicates the source data considered as part of the assessment (for example, FamilyHistory, Observations, Procedures, Conditions, etc.).", 2129 0, java.lang.Integer.MAX_VALUE, basis); 2130 case 1161234575: 2131 /* prediction */ return new Property("prediction", "", "Describes the expected outcome for the subject.", 0, 2132 java.lang.Integer.MAX_VALUE, prediction); 2133 case 1293793087: 2134 /* mitigation */ return new Property("mitigation", "string", 2135 "A description of the steps that might be taken to reduce the identified risk(s).", 0, 1, mitigation); 2136 case 3387378: 2137 /* note */ return new Property("note", "Annotation", "Additional comments about the risk assessment.", 0, 2138 java.lang.Integer.MAX_VALUE, note); 2139 default: 2140 return super.getNamedProperty(_hash, _name, _checkValid); 2141 } 2142 2143 } 2144 2145 @Override 2146 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2147 switch (hash) { 2148 case -1618432855: 2149 /* identifier */ return this.identifier == null ? new Base[0] 2150 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2151 case -332612366: 2152 /* basedOn */ return this.basedOn == null ? new Base[0] : new Base[] { this.basedOn }; // Reference 2153 case -995424086: 2154 /* parent */ return this.parent == null ? new Base[0] : new Base[] { this.parent }; // Reference 2155 case -892481550: 2156 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<RiskAssessmentStatus> 2157 case -1077554975: 2158 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 2159 case 3059181: 2160 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2161 case -1867885268: 2162 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2163 case 1524132147: 2164 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2165 case 1687874001: 2166 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 2167 case -861311717: 2168 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // Reference 2169 case 481140686: 2170 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 2171 case 722137681: 2172 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2173 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2174 case -1146218137: 2175 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2176 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2177 case 93508670: 2178 /* basis */ return this.basis == null ? new Base[0] : this.basis.toArray(new Base[this.basis.size()]); // Reference 2179 case 1161234575: 2180 /* prediction */ return this.prediction == null ? new Base[0] 2181 : this.prediction.toArray(new Base[this.prediction.size()]); // RiskAssessmentPredictionComponent 2182 case 1293793087: 2183 /* mitigation */ return this.mitigation == null ? new Base[0] : new Base[] { this.mitigation }; // StringType 2184 case 3387378: 2185 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2186 default: 2187 return super.getProperty(hash, name, checkValid); 2188 } 2189 2190 } 2191 2192 @Override 2193 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2194 switch (hash) { 2195 case -1618432855: // identifier 2196 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2197 return value; 2198 case -332612366: // basedOn 2199 this.basedOn = castToReference(value); // Reference 2200 return value; 2201 case -995424086: // parent 2202 this.parent = castToReference(value); // Reference 2203 return value; 2204 case -892481550: // status 2205 value = new RiskAssessmentStatusEnumFactory().fromType(castToCode(value)); 2206 this.status = (Enumeration) value; // Enumeration<RiskAssessmentStatus> 2207 return value; 2208 case -1077554975: // method 2209 this.method = castToCodeableConcept(value); // CodeableConcept 2210 return value; 2211 case 3059181: // code 2212 this.code = castToCodeableConcept(value); // CodeableConcept 2213 return value; 2214 case -1867885268: // subject 2215 this.subject = castToReference(value); // Reference 2216 return value; 2217 case 1524132147: // encounter 2218 this.encounter = castToReference(value); // Reference 2219 return value; 2220 case 1687874001: // occurrence 2221 this.occurrence = castToType(value); // Type 2222 return value; 2223 case -861311717: // condition 2224 this.condition = castToReference(value); // Reference 2225 return value; 2226 case 481140686: // performer 2227 this.performer = castToReference(value); // Reference 2228 return value; 2229 case 722137681: // reasonCode 2230 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2231 return value; 2232 case -1146218137: // reasonReference 2233 this.getReasonReference().add(castToReference(value)); // Reference 2234 return value; 2235 case 93508670: // basis 2236 this.getBasis().add(castToReference(value)); // Reference 2237 return value; 2238 case 1161234575: // prediction 2239 this.getPrediction().add((RiskAssessmentPredictionComponent) value); // RiskAssessmentPredictionComponent 2240 return value; 2241 case 1293793087: // mitigation 2242 this.mitigation = castToString(value); // StringType 2243 return value; 2244 case 3387378: // note 2245 this.getNote().add(castToAnnotation(value)); // Annotation 2246 return value; 2247 default: 2248 return super.setProperty(hash, name, value); 2249 } 2250 2251 } 2252 2253 @Override 2254 public Base setProperty(String name, Base value) throws FHIRException { 2255 if (name.equals("identifier")) { 2256 this.getIdentifier().add(castToIdentifier(value)); 2257 } else if (name.equals("basedOn")) { 2258 this.basedOn = castToReference(value); // Reference 2259 } else if (name.equals("parent")) { 2260 this.parent = castToReference(value); // Reference 2261 } else if (name.equals("status")) { 2262 value = new RiskAssessmentStatusEnumFactory().fromType(castToCode(value)); 2263 this.status = (Enumeration) value; // Enumeration<RiskAssessmentStatus> 2264 } else if (name.equals("method")) { 2265 this.method = castToCodeableConcept(value); // CodeableConcept 2266 } else if (name.equals("code")) { 2267 this.code = castToCodeableConcept(value); // CodeableConcept 2268 } else if (name.equals("subject")) { 2269 this.subject = castToReference(value); // Reference 2270 } else if (name.equals("encounter")) { 2271 this.encounter = castToReference(value); // Reference 2272 } else if (name.equals("occurrence[x]")) { 2273 this.occurrence = castToType(value); // Type 2274 } else if (name.equals("condition")) { 2275 this.condition = castToReference(value); // Reference 2276 } else if (name.equals("performer")) { 2277 this.performer = castToReference(value); // Reference 2278 } else if (name.equals("reasonCode")) { 2279 this.getReasonCode().add(castToCodeableConcept(value)); 2280 } else if (name.equals("reasonReference")) { 2281 this.getReasonReference().add(castToReference(value)); 2282 } else if (name.equals("basis")) { 2283 this.getBasis().add(castToReference(value)); 2284 } else if (name.equals("prediction")) { 2285 this.getPrediction().add((RiskAssessmentPredictionComponent) value); 2286 } else if (name.equals("mitigation")) { 2287 this.mitigation = castToString(value); // StringType 2288 } else if (name.equals("note")) { 2289 this.getNote().add(castToAnnotation(value)); 2290 } else 2291 return super.setProperty(name, value); 2292 return value; 2293 } 2294 2295 @Override 2296 public void removeChild(String name, Base value) throws FHIRException { 2297 if (name.equals("identifier")) { 2298 this.getIdentifier().remove(castToIdentifier(value)); 2299 } else if (name.equals("basedOn")) { 2300 this.basedOn = null; 2301 } else if (name.equals("parent")) { 2302 this.parent = null; 2303 } else if (name.equals("status")) { 2304 this.status = null; 2305 } else if (name.equals("method")) { 2306 this.method = null; 2307 } else if (name.equals("code")) { 2308 this.code = null; 2309 } else if (name.equals("subject")) { 2310 this.subject = null; 2311 } else if (name.equals("encounter")) { 2312 this.encounter = null; 2313 } else if (name.equals("occurrence[x]")) { 2314 this.occurrence = null; 2315 } else if (name.equals("condition")) { 2316 this.condition = null; 2317 } else if (name.equals("performer")) { 2318 this.performer = null; 2319 } else if (name.equals("reasonCode")) { 2320 this.getReasonCode().remove(castToCodeableConcept(value)); 2321 } else if (name.equals("reasonReference")) { 2322 this.getReasonReference().remove(castToReference(value)); 2323 } else if (name.equals("basis")) { 2324 this.getBasis().remove(castToReference(value)); 2325 } else if (name.equals("prediction")) { 2326 this.getPrediction().remove((RiskAssessmentPredictionComponent) value); 2327 } else if (name.equals("mitigation")) { 2328 this.mitigation = null; 2329 } else if (name.equals("note")) { 2330 this.getNote().remove(castToAnnotation(value)); 2331 } else 2332 super.removeChild(name, value); 2333 2334 } 2335 2336 @Override 2337 public Base makeProperty(int hash, String name) throws FHIRException { 2338 switch (hash) { 2339 case -1618432855: 2340 return addIdentifier(); 2341 case -332612366: 2342 return getBasedOn(); 2343 case -995424086: 2344 return getParent(); 2345 case -892481550: 2346 return getStatusElement(); 2347 case -1077554975: 2348 return getMethod(); 2349 case 3059181: 2350 return getCode(); 2351 case -1867885268: 2352 return getSubject(); 2353 case 1524132147: 2354 return getEncounter(); 2355 case -2022646513: 2356 return getOccurrence(); 2357 case 1687874001: 2358 return getOccurrence(); 2359 case -861311717: 2360 return getCondition(); 2361 case 481140686: 2362 return getPerformer(); 2363 case 722137681: 2364 return addReasonCode(); 2365 case -1146218137: 2366 return addReasonReference(); 2367 case 93508670: 2368 return addBasis(); 2369 case 1161234575: 2370 return addPrediction(); 2371 case 1293793087: 2372 return getMitigationElement(); 2373 case 3387378: 2374 return addNote(); 2375 default: 2376 return super.makeProperty(hash, name); 2377 } 2378 2379 } 2380 2381 @Override 2382 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2383 switch (hash) { 2384 case -1618432855: 2385 /* identifier */ return new String[] { "Identifier" }; 2386 case -332612366: 2387 /* basedOn */ return new String[] { "Reference" }; 2388 case -995424086: 2389 /* parent */ return new String[] { "Reference" }; 2390 case -892481550: 2391 /* status */ return new String[] { "code" }; 2392 case -1077554975: 2393 /* method */ return new String[] { "CodeableConcept" }; 2394 case 3059181: 2395 /* code */ return new String[] { "CodeableConcept" }; 2396 case -1867885268: 2397 /* subject */ return new String[] { "Reference" }; 2398 case 1524132147: 2399 /* encounter */ return new String[] { "Reference" }; 2400 case 1687874001: 2401 /* occurrence */ return new String[] { "dateTime", "Period" }; 2402 case -861311717: 2403 /* condition */ return new String[] { "Reference" }; 2404 case 481140686: 2405 /* performer */ return new String[] { "Reference" }; 2406 case 722137681: 2407 /* reasonCode */ return new String[] { "CodeableConcept" }; 2408 case -1146218137: 2409 /* reasonReference */ return new String[] { "Reference" }; 2410 case 93508670: 2411 /* basis */ return new String[] { "Reference" }; 2412 case 1161234575: 2413 /* prediction */ return new String[] {}; 2414 case 1293793087: 2415 /* mitigation */ return new String[] { "string" }; 2416 case 3387378: 2417 /* note */ return new String[] { "Annotation" }; 2418 default: 2419 return super.getTypesForProperty(hash, name); 2420 } 2421 2422 } 2423 2424 @Override 2425 public Base addChild(String name) throws FHIRException { 2426 if (name.equals("identifier")) { 2427 return addIdentifier(); 2428 } else if (name.equals("basedOn")) { 2429 this.basedOn = new Reference(); 2430 return this.basedOn; 2431 } else if (name.equals("parent")) { 2432 this.parent = new Reference(); 2433 return this.parent; 2434 } else if (name.equals("status")) { 2435 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.status"); 2436 } else if (name.equals("method")) { 2437 this.method = new CodeableConcept(); 2438 return this.method; 2439 } else if (name.equals("code")) { 2440 this.code = new CodeableConcept(); 2441 return this.code; 2442 } else if (name.equals("subject")) { 2443 this.subject = new Reference(); 2444 return this.subject; 2445 } else if (name.equals("encounter")) { 2446 this.encounter = new Reference(); 2447 return this.encounter; 2448 } else if (name.equals("occurrenceDateTime")) { 2449 this.occurrence = new DateTimeType(); 2450 return this.occurrence; 2451 } else if (name.equals("occurrencePeriod")) { 2452 this.occurrence = new Period(); 2453 return this.occurrence; 2454 } else if (name.equals("condition")) { 2455 this.condition = new Reference(); 2456 return this.condition; 2457 } else if (name.equals("performer")) { 2458 this.performer = new Reference(); 2459 return this.performer; 2460 } else if (name.equals("reasonCode")) { 2461 return addReasonCode(); 2462 } else if (name.equals("reasonReference")) { 2463 return addReasonReference(); 2464 } else if (name.equals("basis")) { 2465 return addBasis(); 2466 } else if (name.equals("prediction")) { 2467 return addPrediction(); 2468 } else if (name.equals("mitigation")) { 2469 throw new FHIRException("Cannot call addChild on a singleton property RiskAssessment.mitigation"); 2470 } else if (name.equals("note")) { 2471 return addNote(); 2472 } else 2473 return super.addChild(name); 2474 } 2475 2476 public String fhirType() { 2477 return "RiskAssessment"; 2478 2479 } 2480 2481 public RiskAssessment copy() { 2482 RiskAssessment dst = new RiskAssessment(); 2483 copyValues(dst); 2484 return dst; 2485 } 2486 2487 public void copyValues(RiskAssessment dst) { 2488 super.copyValues(dst); 2489 if (identifier != null) { 2490 dst.identifier = new ArrayList<Identifier>(); 2491 for (Identifier i : identifier) 2492 dst.identifier.add(i.copy()); 2493 } 2494 ; 2495 dst.basedOn = basedOn == null ? null : basedOn.copy(); 2496 dst.parent = parent == null ? null : parent.copy(); 2497 dst.status = status == null ? null : status.copy(); 2498 dst.method = method == null ? null : method.copy(); 2499 dst.code = code == null ? null : code.copy(); 2500 dst.subject = subject == null ? null : subject.copy(); 2501 dst.encounter = encounter == null ? null : encounter.copy(); 2502 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2503 dst.condition = condition == null ? null : condition.copy(); 2504 dst.performer = performer == null ? null : performer.copy(); 2505 if (reasonCode != null) { 2506 dst.reasonCode = new ArrayList<CodeableConcept>(); 2507 for (CodeableConcept i : reasonCode) 2508 dst.reasonCode.add(i.copy()); 2509 } 2510 ; 2511 if (reasonReference != null) { 2512 dst.reasonReference = new ArrayList<Reference>(); 2513 for (Reference i : reasonReference) 2514 dst.reasonReference.add(i.copy()); 2515 } 2516 ; 2517 if (basis != null) { 2518 dst.basis = new ArrayList<Reference>(); 2519 for (Reference i : basis) 2520 dst.basis.add(i.copy()); 2521 } 2522 ; 2523 if (prediction != null) { 2524 dst.prediction = new ArrayList<RiskAssessmentPredictionComponent>(); 2525 for (RiskAssessmentPredictionComponent i : prediction) 2526 dst.prediction.add(i.copy()); 2527 } 2528 ; 2529 dst.mitigation = mitigation == null ? null : mitigation.copy(); 2530 if (note != null) { 2531 dst.note = new ArrayList<Annotation>(); 2532 for (Annotation i : note) 2533 dst.note.add(i.copy()); 2534 } 2535 ; 2536 } 2537 2538 protected RiskAssessment typedCopy() { 2539 return copy(); 2540 } 2541 2542 @Override 2543 public boolean equalsDeep(Base other_) { 2544 if (!super.equalsDeep(other_)) 2545 return false; 2546 if (!(other_ instanceof RiskAssessment)) 2547 return false; 2548 RiskAssessment o = (RiskAssessment) other_; 2549 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2550 && compareDeep(parent, o.parent, true) && compareDeep(status, o.status, true) 2551 && compareDeep(method, o.method, true) && compareDeep(code, o.code, true) 2552 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2553 && compareDeep(occurrence, o.occurrence, true) && compareDeep(condition, o.condition, true) 2554 && compareDeep(performer, o.performer, true) && compareDeep(reasonCode, o.reasonCode, true) 2555 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(basis, o.basis, true) 2556 && compareDeep(prediction, o.prediction, true) && compareDeep(mitigation, o.mitigation, true) 2557 && compareDeep(note, o.note, true); 2558 } 2559 2560 @Override 2561 public boolean equalsShallow(Base other_) { 2562 if (!super.equalsShallow(other_)) 2563 return false; 2564 if (!(other_ instanceof RiskAssessment)) 2565 return false; 2566 RiskAssessment o = (RiskAssessment) other_; 2567 return compareValues(status, o.status, true) && compareValues(mitigation, o.mitigation, true); 2568 } 2569 2570 public boolean isEmpty() { 2571 return super.isEmpty() 2572 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, parent, status, method, code, subject, encounter, 2573 occurrence, condition, performer, reasonCode, reasonReference, basis, prediction, mitigation, note); 2574 } 2575 2576 @Override 2577 public ResourceType getResourceType() { 2578 return ResourceType.RiskAssessment; 2579 } 2580 2581 /** 2582 * Search parameter: <b>date</b> 2583 * <p> 2584 * Description: <b>When was assessment made?</b><br> 2585 * Type: <b>date</b><br> 2586 * Path: <b>RiskAssessment.occurrenceDateTime</b><br> 2587 * </p> 2588 */ 2589 @SearchParamDefinition(name = "date", path = "(RiskAssessment.occurrence as dateTime)", description = "When was assessment made?", type = "date") 2590 public static final String SP_DATE = "date"; 2591 /** 2592 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2593 * <p> 2594 * Description: <b>When was assessment made?</b><br> 2595 * Type: <b>date</b><br> 2596 * Path: <b>RiskAssessment.occurrenceDateTime</b><br> 2597 * </p> 2598 */ 2599 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2600 SP_DATE); 2601 2602 /** 2603 * Search parameter: <b>identifier</b> 2604 * <p> 2605 * Description: <b>Unique identifier for the assessment</b><br> 2606 * Type: <b>token</b><br> 2607 * Path: <b>RiskAssessment.identifier</b><br> 2608 * </p> 2609 */ 2610 @SearchParamDefinition(name = "identifier", path = "RiskAssessment.identifier", description = "Unique identifier for the assessment", type = "token") 2611 public static final String SP_IDENTIFIER = "identifier"; 2612 /** 2613 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2614 * <p> 2615 * Description: <b>Unique identifier for the assessment</b><br> 2616 * Type: <b>token</b><br> 2617 * Path: <b>RiskAssessment.identifier</b><br> 2618 * </p> 2619 */ 2620 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2621 SP_IDENTIFIER); 2622 2623 /** 2624 * Search parameter: <b>condition</b> 2625 * <p> 2626 * Description: <b>Condition assessed</b><br> 2627 * Type: <b>reference</b><br> 2628 * Path: <b>RiskAssessment.condition</b><br> 2629 * </p> 2630 */ 2631 @SearchParamDefinition(name = "condition", path = "RiskAssessment.condition", description = "Condition assessed", type = "reference", target = { 2632 Condition.class }) 2633 public static final String SP_CONDITION = "condition"; 2634 /** 2635 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 2636 * <p> 2637 * Description: <b>Condition assessed</b><br> 2638 * Type: <b>reference</b><br> 2639 * Path: <b>RiskAssessment.condition</b><br> 2640 * </p> 2641 */ 2642 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2643 SP_CONDITION); 2644 2645 /** 2646 * Constant for fluent queries to be used to add include statements. Specifies 2647 * the path value of "<b>RiskAssessment:condition</b>". 2648 */ 2649 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include( 2650 "RiskAssessment:condition").toLocked(); 2651 2652 /** 2653 * Search parameter: <b>performer</b> 2654 * <p> 2655 * Description: <b>Who did assessment?</b><br> 2656 * Type: <b>reference</b><br> 2657 * Path: <b>RiskAssessment.performer</b><br> 2658 * </p> 2659 */ 2660 @SearchParamDefinition(name = "performer", path = "RiskAssessment.performer", description = "Who did assessment?", type = "reference", providesMembershipIn = { 2661 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2662 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 2663 Practitioner.class, PractitionerRole.class }) 2664 public static final String SP_PERFORMER = "performer"; 2665 /** 2666 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2667 * <p> 2668 * Description: <b>Who did assessment?</b><br> 2669 * Type: <b>reference</b><br> 2670 * Path: <b>RiskAssessment.performer</b><br> 2671 * </p> 2672 */ 2673 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2674 SP_PERFORMER); 2675 2676 /** 2677 * Constant for fluent queries to be used to add include statements. Specifies 2678 * the path value of "<b>RiskAssessment:performer</b>". 2679 */ 2680 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 2681 "RiskAssessment:performer").toLocked(); 2682 2683 /** 2684 * Search parameter: <b>method</b> 2685 * <p> 2686 * Description: <b>Evaluation mechanism</b><br> 2687 * Type: <b>token</b><br> 2688 * Path: <b>RiskAssessment.method</b><br> 2689 * </p> 2690 */ 2691 @SearchParamDefinition(name = "method", path = "RiskAssessment.method", description = "Evaluation mechanism", type = "token") 2692 public static final String SP_METHOD = "method"; 2693 /** 2694 * <b>Fluent Client</b> search parameter constant for <b>method</b> 2695 * <p> 2696 * Description: <b>Evaluation mechanism</b><br> 2697 * Type: <b>token</b><br> 2698 * Path: <b>RiskAssessment.method</b><br> 2699 * </p> 2700 */ 2701 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2702 SP_METHOD); 2703 2704 /** 2705 * Search parameter: <b>subject</b> 2706 * <p> 2707 * Description: <b>Who/what does assessment apply to?</b><br> 2708 * Type: <b>reference</b><br> 2709 * Path: <b>RiskAssessment.subject</b><br> 2710 * </p> 2711 */ 2712 @SearchParamDefinition(name = "subject", path = "RiskAssessment.subject", description = "Who/what does assessment apply to?", type = "reference", providesMembershipIn = { 2713 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 2714 public static final String SP_SUBJECT = "subject"; 2715 /** 2716 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2717 * <p> 2718 * Description: <b>Who/what does assessment apply to?</b><br> 2719 * Type: <b>reference</b><br> 2720 * Path: <b>RiskAssessment.subject</b><br> 2721 * </p> 2722 */ 2723 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2724 SP_SUBJECT); 2725 2726 /** 2727 * Constant for fluent queries to be used to add include statements. Specifies 2728 * the path value of "<b>RiskAssessment:subject</b>". 2729 */ 2730 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2731 "RiskAssessment:subject").toLocked(); 2732 2733 /** 2734 * Search parameter: <b>patient</b> 2735 * <p> 2736 * Description: <b>Who/what does assessment apply to?</b><br> 2737 * Type: <b>reference</b><br> 2738 * Path: <b>RiskAssessment.subject</b><br> 2739 * </p> 2740 */ 2741 @SearchParamDefinition(name = "patient", path = "RiskAssessment.subject.where(resolve() is Patient)", description = "Who/what does assessment apply to?", type = "reference", target = { 2742 Patient.class }) 2743 public static final String SP_PATIENT = "patient"; 2744 /** 2745 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2746 * <p> 2747 * Description: <b>Who/what does assessment apply to?</b><br> 2748 * Type: <b>reference</b><br> 2749 * Path: <b>RiskAssessment.subject</b><br> 2750 * </p> 2751 */ 2752 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2753 SP_PATIENT); 2754 2755 /** 2756 * Constant for fluent queries to be used to add include statements. Specifies 2757 * the path value of "<b>RiskAssessment:patient</b>". 2758 */ 2759 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2760 "RiskAssessment:patient").toLocked(); 2761 2762 /** 2763 * Search parameter: <b>probability</b> 2764 * <p> 2765 * Description: <b>Likelihood of specified outcome</b><br> 2766 * Type: <b>number</b><br> 2767 * Path: <b>RiskAssessment.prediction.probability[x]</b><br> 2768 * </p> 2769 */ 2770 @SearchParamDefinition(name = "probability", path = "RiskAssessment.prediction.probability", description = "Likelihood of specified outcome", type = "number") 2771 public static final String SP_PROBABILITY = "probability"; 2772 /** 2773 * <b>Fluent Client</b> search parameter constant for <b>probability</b> 2774 * <p> 2775 * Description: <b>Likelihood of specified outcome</b><br> 2776 * Type: <b>number</b><br> 2777 * Path: <b>RiskAssessment.prediction.probability[x]</b><br> 2778 * </p> 2779 */ 2780 public static final ca.uhn.fhir.rest.gclient.NumberClientParam PROBABILITY = new ca.uhn.fhir.rest.gclient.NumberClientParam( 2781 SP_PROBABILITY); 2782 2783 /** 2784 * Search parameter: <b>risk</b> 2785 * <p> 2786 * Description: <b>Likelihood of specified outcome as a qualitative 2787 * value</b><br> 2788 * Type: <b>token</b><br> 2789 * Path: <b>RiskAssessment.prediction.qualitativeRisk</b><br> 2790 * </p> 2791 */ 2792 @SearchParamDefinition(name = "risk", path = "RiskAssessment.prediction.qualitativeRisk", description = "Likelihood of specified outcome as a qualitative value", type = "token") 2793 public static final String SP_RISK = "risk"; 2794 /** 2795 * <b>Fluent Client</b> search parameter constant for <b>risk</b> 2796 * <p> 2797 * Description: <b>Likelihood of specified outcome as a qualitative 2798 * value</b><br> 2799 * Type: <b>token</b><br> 2800 * Path: <b>RiskAssessment.prediction.qualitativeRisk</b><br> 2801 * </p> 2802 */ 2803 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RISK = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2804 SP_RISK); 2805 2806 /** 2807 * Search parameter: <b>encounter</b> 2808 * <p> 2809 * Description: <b>Where was assessment performed?</b><br> 2810 * Type: <b>reference</b><br> 2811 * Path: <b>RiskAssessment.encounter</b><br> 2812 * </p> 2813 */ 2814 @SearchParamDefinition(name = "encounter", path = "RiskAssessment.encounter", description = "Where was assessment performed?", type = "reference", target = { 2815 Encounter.class }) 2816 public static final String SP_ENCOUNTER = "encounter"; 2817 /** 2818 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2819 * <p> 2820 * Description: <b>Where was assessment performed?</b><br> 2821 * Type: <b>reference</b><br> 2822 * Path: <b>RiskAssessment.encounter</b><br> 2823 * </p> 2824 */ 2825 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2826 SP_ENCOUNTER); 2827 2828 /** 2829 * Constant for fluent queries to be used to add include statements. Specifies 2830 * the path value of "<b>RiskAssessment:encounter</b>". 2831 */ 2832 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2833 "RiskAssessment:encounter").toLocked(); 2834 2835}