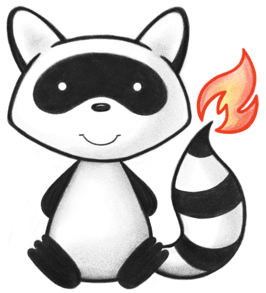
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * A series of measurements taken by a device, with upper and lower limits. 047 * There may be more than one dimension in the data. 048 */ 049@DatatypeDef(name = "SampledData") 050public class SampledData extends Type implements ICompositeType { 051 052 /** 053 * The base quantity that a measured value of zero represents. In addition, this 054 * provides the units of the entire measurement series. 055 */ 056 @Child(name = "origin", type = { Quantity.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 057 @Description(shortDefinition = "Zero value and units", formalDefinition = "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.") 058 protected Quantity origin; 059 060 /** 061 * The length of time between sampling times, measured in milliseconds. 062 */ 063 @Child(name = "period", type = { DecimalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 064 @Description(shortDefinition = "Number of milliseconds between samples", formalDefinition = "The length of time between sampling times, measured in milliseconds.") 065 protected DecimalType period; 066 067 /** 068 * A correction factor that is applied to the sampled data points before they 069 * are added to the origin. 070 */ 071 @Child(name = "factor", type = { DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 072 @Description(shortDefinition = "Multiply data by this before adding to origin", formalDefinition = "A correction factor that is applied to the sampled data points before they are added to the origin.") 073 protected DecimalType factor; 074 075 /** 076 * The lower limit of detection of the measured points. This is needed if any of 077 * the data points have the value "L" (lower than detection limit). 078 */ 079 @Child(name = "lowerLimit", type = { 080 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 081 @Description(shortDefinition = "Lower limit of detection", formalDefinition = "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).") 082 protected DecimalType lowerLimit; 083 084 /** 085 * The upper limit of detection of the measured points. This is needed if any of 086 * the data points have the value "U" (higher than detection limit). 087 */ 088 @Child(name = "upperLimit", type = { 089 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 090 @Description(shortDefinition = "Upper limit of detection", formalDefinition = "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).") 091 protected DecimalType upperLimit; 092 093 /** 094 * The number of sample points at each time point. If this value is greater than 095 * one, then the dimensions will be interlaced - all the sample points for a 096 * point in time will be recorded at once. 097 */ 098 @Child(name = "dimensions", type = { 099 PositiveIntType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 100 @Description(shortDefinition = "Number of sample points at each time point", formalDefinition = "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.") 101 protected PositiveIntType dimensions; 102 103 /** 104 * A series of data points which are decimal values separated by a single space 105 * (character u20). The special values "E" (error), "L" (below detection limit) 106 * and "U" (above detection limit) can also be used in place of a decimal value. 107 */ 108 @Child(name = "data", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 109 @Description(shortDefinition = "Decimal values with spaces, or \"E\" | \"U\" | \"L\"", formalDefinition = "A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value.") 110 protected StringType data; 111 112 private static final long serialVersionUID = -1984181262L; 113 114 /** 115 * Constructor 116 */ 117 public SampledData() { 118 super(); 119 } 120 121 /** 122 * Constructor 123 */ 124 public SampledData(Quantity origin, DecimalType period, PositiveIntType dimensions) { 125 super(); 126 this.origin = origin; 127 this.period = period; 128 this.dimensions = dimensions; 129 } 130 131 /** 132 * @return {@link #origin} (The base quantity that a measured value of zero 133 * represents. In addition, this provides the units of the entire 134 * measurement series.) 135 */ 136 public Quantity getOrigin() { 137 if (this.origin == null) 138 if (Configuration.errorOnAutoCreate()) 139 throw new Error("Attempt to auto-create SampledData.origin"); 140 else if (Configuration.doAutoCreate()) 141 this.origin = new Quantity(); // cc 142 return this.origin; 143 } 144 145 public boolean hasOrigin() { 146 return this.origin != null && !this.origin.isEmpty(); 147 } 148 149 /** 150 * @param value {@link #origin} (The base quantity that a measured value of zero 151 * represents. In addition, this provides the units of the entire 152 * measurement series.) 153 */ 154 public SampledData setOrigin(Quantity value) { 155 this.origin = value; 156 return this; 157 } 158 159 /** 160 * @return {@link #period} (The length of time between sampling times, measured 161 * in milliseconds.). This is the underlying object with id, value and 162 * extensions. The accessor "getPeriod" gives direct access to the value 163 */ 164 public DecimalType getPeriodElement() { 165 if (this.period == null) 166 if (Configuration.errorOnAutoCreate()) 167 throw new Error("Attempt to auto-create SampledData.period"); 168 else if (Configuration.doAutoCreate()) 169 this.period = new DecimalType(); // bb 170 return this.period; 171 } 172 173 public boolean hasPeriodElement() { 174 return this.period != null && !this.period.isEmpty(); 175 } 176 177 public boolean hasPeriod() { 178 return this.period != null && !this.period.isEmpty(); 179 } 180 181 /** 182 * @param value {@link #period} (The length of time between sampling times, 183 * measured in milliseconds.). This is the underlying object with 184 * id, value and extensions. The accessor "getPeriod" gives direct 185 * access to the value 186 */ 187 public SampledData setPeriodElement(DecimalType value) { 188 this.period = value; 189 return this; 190 } 191 192 /** 193 * @return The length of time between sampling times, measured in milliseconds. 194 */ 195 public BigDecimal getPeriod() { 196 return this.period == null ? null : this.period.getValue(); 197 } 198 199 /** 200 * @param value The length of time between sampling times, measured in 201 * milliseconds. 202 */ 203 public SampledData setPeriod(BigDecimal value) { 204 if (this.period == null) 205 this.period = new DecimalType(); 206 this.period.setValue(value); 207 return this; 208 } 209 210 /** 211 * @param value The length of time between sampling times, measured in 212 * milliseconds. 213 */ 214 public SampledData setPeriod(long value) { 215 this.period = new DecimalType(); 216 this.period.setValue(value); 217 return this; 218 } 219 220 /** 221 * @param value The length of time between sampling times, measured in 222 * milliseconds. 223 */ 224 public SampledData setPeriod(double value) { 225 this.period = new DecimalType(); 226 this.period.setValue(value); 227 return this; 228 } 229 230 /** 231 * @return {@link #factor} (A correction factor that is applied to the sampled 232 * data points before they are added to the origin.). This is the 233 * underlying object with id, value and extensions. The accessor 234 * "getFactor" gives direct access to the value 235 */ 236 public DecimalType getFactorElement() { 237 if (this.factor == null) 238 if (Configuration.errorOnAutoCreate()) 239 throw new Error("Attempt to auto-create SampledData.factor"); 240 else if (Configuration.doAutoCreate()) 241 this.factor = new DecimalType(); // bb 242 return this.factor; 243 } 244 245 public boolean hasFactorElement() { 246 return this.factor != null && !this.factor.isEmpty(); 247 } 248 249 public boolean hasFactor() { 250 return this.factor != null && !this.factor.isEmpty(); 251 } 252 253 /** 254 * @param value {@link #factor} (A correction factor that is applied to the 255 * sampled data points before they are added to the origin.). This 256 * is the underlying object with id, value and extensions. The 257 * accessor "getFactor" gives direct access to the value 258 */ 259 public SampledData setFactorElement(DecimalType value) { 260 this.factor = value; 261 return this; 262 } 263 264 /** 265 * @return A correction factor that is applied to the sampled data points before 266 * they are added to the origin. 267 */ 268 public BigDecimal getFactor() { 269 return this.factor == null ? null : this.factor.getValue(); 270 } 271 272 /** 273 * @param value A correction factor that is applied to the sampled data points 274 * before they are added to the origin. 275 */ 276 public SampledData setFactor(BigDecimal value) { 277 if (value == null) 278 this.factor = null; 279 else { 280 if (this.factor == null) 281 this.factor = new DecimalType(); 282 this.factor.setValue(value); 283 } 284 return this; 285 } 286 287 /** 288 * @param value A correction factor that is applied to the sampled data points 289 * before they are added to the origin. 290 */ 291 public SampledData setFactor(long value) { 292 this.factor = new DecimalType(); 293 this.factor.setValue(value); 294 return this; 295 } 296 297 /** 298 * @param value A correction factor that is applied to the sampled data points 299 * before they are added to the origin. 300 */ 301 public SampledData setFactor(double value) { 302 this.factor = new DecimalType(); 303 this.factor.setValue(value); 304 return this; 305 } 306 307 /** 308 * @return {@link #lowerLimit} (The lower limit of detection of the measured 309 * points. This is needed if any of the data points have the value "L" 310 * (lower than detection limit).). This is the underlying object with 311 * id, value and extensions. The accessor "getLowerLimit" gives direct 312 * access to the value 313 */ 314 public DecimalType getLowerLimitElement() { 315 if (this.lowerLimit == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create SampledData.lowerLimit"); 318 else if (Configuration.doAutoCreate()) 319 this.lowerLimit = new DecimalType(); // bb 320 return this.lowerLimit; 321 } 322 323 public boolean hasLowerLimitElement() { 324 return this.lowerLimit != null && !this.lowerLimit.isEmpty(); 325 } 326 327 public boolean hasLowerLimit() { 328 return this.lowerLimit != null && !this.lowerLimit.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #lowerLimit} (The lower limit of detection of the 333 * measured points. This is needed if any of the data points have 334 * the value "L" (lower than detection limit).). This is the 335 * underlying object with id, value and extensions. The accessor 336 * "getLowerLimit" gives direct access to the value 337 */ 338 public SampledData setLowerLimitElement(DecimalType value) { 339 this.lowerLimit = value; 340 return this; 341 } 342 343 /** 344 * @return The lower limit of detection of the measured points. This is needed 345 * if any of the data points have the value "L" (lower than detection 346 * limit). 347 */ 348 public BigDecimal getLowerLimit() { 349 return this.lowerLimit == null ? null : this.lowerLimit.getValue(); 350 } 351 352 /** 353 * @param value The lower limit of detection of the measured points. This is 354 * needed if any of the data points have the value "L" (lower than 355 * detection limit). 356 */ 357 public SampledData setLowerLimit(BigDecimal value) { 358 if (value == null) 359 this.lowerLimit = null; 360 else { 361 if (this.lowerLimit == null) 362 this.lowerLimit = new DecimalType(); 363 this.lowerLimit.setValue(value); 364 } 365 return this; 366 } 367 368 /** 369 * @param value The lower limit of detection of the measured points. This is 370 * needed if any of the data points have the value "L" (lower than 371 * detection limit). 372 */ 373 public SampledData setLowerLimit(long value) { 374 this.lowerLimit = new DecimalType(); 375 this.lowerLimit.setValue(value); 376 return this; 377 } 378 379 /** 380 * @param value The lower limit of detection of the measured points. This is 381 * needed if any of the data points have the value "L" (lower than 382 * detection limit). 383 */ 384 public SampledData setLowerLimit(double value) { 385 this.lowerLimit = new DecimalType(); 386 this.lowerLimit.setValue(value); 387 return this; 388 } 389 390 /** 391 * @return {@link #upperLimit} (The upper limit of detection of the measured 392 * points. This is needed if any of the data points have the value "U" 393 * (higher than detection limit).). This is the underlying object with 394 * id, value and extensions. The accessor "getUpperLimit" gives direct 395 * access to the value 396 */ 397 public DecimalType getUpperLimitElement() { 398 if (this.upperLimit == null) 399 if (Configuration.errorOnAutoCreate()) 400 throw new Error("Attempt to auto-create SampledData.upperLimit"); 401 else if (Configuration.doAutoCreate()) 402 this.upperLimit = new DecimalType(); // bb 403 return this.upperLimit; 404 } 405 406 public boolean hasUpperLimitElement() { 407 return this.upperLimit != null && !this.upperLimit.isEmpty(); 408 } 409 410 public boolean hasUpperLimit() { 411 return this.upperLimit != null && !this.upperLimit.isEmpty(); 412 } 413 414 /** 415 * @param value {@link #upperLimit} (The upper limit of detection of the 416 * measured points. This is needed if any of the data points have 417 * the value "U" (higher than detection limit).). This is the 418 * underlying object with id, value and extensions. The accessor 419 * "getUpperLimit" gives direct access to the value 420 */ 421 public SampledData setUpperLimitElement(DecimalType value) { 422 this.upperLimit = value; 423 return this; 424 } 425 426 /** 427 * @return The upper limit of detection of the measured points. This is needed 428 * if any of the data points have the value "U" (higher than detection 429 * limit). 430 */ 431 public BigDecimal getUpperLimit() { 432 return this.upperLimit == null ? null : this.upperLimit.getValue(); 433 } 434 435 /** 436 * @param value The upper limit of detection of the measured points. This is 437 * needed if any of the data points have the value "U" (higher than 438 * detection limit). 439 */ 440 public SampledData setUpperLimit(BigDecimal value) { 441 if (value == null) 442 this.upperLimit = null; 443 else { 444 if (this.upperLimit == null) 445 this.upperLimit = new DecimalType(); 446 this.upperLimit.setValue(value); 447 } 448 return this; 449 } 450 451 /** 452 * @param value The upper limit of detection of the measured points. This is 453 * needed if any of the data points have the value "U" (higher than 454 * detection limit). 455 */ 456 public SampledData setUpperLimit(long value) { 457 this.upperLimit = new DecimalType(); 458 this.upperLimit.setValue(value); 459 return this; 460 } 461 462 /** 463 * @param value The upper limit of detection of the measured points. This is 464 * needed if any of the data points have the value "U" (higher than 465 * detection limit). 466 */ 467 public SampledData setUpperLimit(double value) { 468 this.upperLimit = new DecimalType(); 469 this.upperLimit.setValue(value); 470 return this; 471 } 472 473 /** 474 * @return {@link #dimensions} (The number of sample points at each time point. 475 * If this value is greater than one, then the dimensions will be 476 * interlaced - all the sample points for a point in time will be 477 * recorded at once.). This is the underlying object with id, value and 478 * extensions. The accessor "getDimensions" gives direct access to the 479 * value 480 */ 481 public PositiveIntType getDimensionsElement() { 482 if (this.dimensions == null) 483 if (Configuration.errorOnAutoCreate()) 484 throw new Error("Attempt to auto-create SampledData.dimensions"); 485 else if (Configuration.doAutoCreate()) 486 this.dimensions = new PositiveIntType(); // bb 487 return this.dimensions; 488 } 489 490 public boolean hasDimensionsElement() { 491 return this.dimensions != null && !this.dimensions.isEmpty(); 492 } 493 494 public boolean hasDimensions() { 495 return this.dimensions != null && !this.dimensions.isEmpty(); 496 } 497 498 /** 499 * @param value {@link #dimensions} (The number of sample points at each time 500 * point. If this value is greater than one, then the dimensions 501 * will be interlaced - all the sample points for a point in time 502 * will be recorded at once.). This is the underlying object with 503 * id, value and extensions. The accessor "getDimensions" gives 504 * direct access to the value 505 */ 506 public SampledData setDimensionsElement(PositiveIntType value) { 507 this.dimensions = value; 508 return this; 509 } 510 511 /** 512 * @return The number of sample points at each time point. If this value is 513 * greater than one, then the dimensions will be interlaced - all the 514 * sample points for a point in time will be recorded at once. 515 */ 516 public int getDimensions() { 517 return this.dimensions == null || this.dimensions.isEmpty() ? 0 : this.dimensions.getValue(); 518 } 519 520 /** 521 * @param value The number of sample points at each time point. If this value is 522 * greater than one, then the dimensions will be interlaced - all 523 * the sample points for a point in time will be recorded at once. 524 */ 525 public SampledData setDimensions(int value) { 526 if (this.dimensions == null) 527 this.dimensions = new PositiveIntType(); 528 this.dimensions.setValue(value); 529 return this; 530 } 531 532 /** 533 * @return {@link #data} (A series of data points which are decimal values 534 * separated by a single space (character u20). The special values "E" 535 * (error), "L" (below detection limit) and "U" (above detection limit) 536 * can also be used in place of a decimal value.). This is the 537 * underlying object with id, value and extensions. The accessor 538 * "getData" gives direct access to the value 539 */ 540 public StringType getDataElement() { 541 if (this.data == null) 542 if (Configuration.errorOnAutoCreate()) 543 throw new Error("Attempt to auto-create SampledData.data"); 544 else if (Configuration.doAutoCreate()) 545 this.data = new StringType(); // bb 546 return this.data; 547 } 548 549 public boolean hasDataElement() { 550 return this.data != null && !this.data.isEmpty(); 551 } 552 553 public boolean hasData() { 554 return this.data != null && !this.data.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #data} (A series of data points which are decimal values 559 * separated by a single space (character u20). The special values 560 * "E" (error), "L" (below detection limit) and "U" (above 561 * detection limit) can also be used in place of a decimal value.). 562 * This is the underlying object with id, value and extensions. The 563 * accessor "getData" gives direct access to the value 564 */ 565 public SampledData setDataElement(StringType value) { 566 this.data = value; 567 return this; 568 } 569 570 /** 571 * @return A series of data points which are decimal values separated by a 572 * single space (character u20). The special values "E" (error), "L" 573 * (below detection limit) and "U" (above detection limit) can also be 574 * used in place of a decimal value. 575 */ 576 public String getData() { 577 return this.data == null ? null : this.data.getValue(); 578 } 579 580 /** 581 * @param value A series of data points which are decimal values separated by a 582 * single space (character u20). The special values "E" (error), 583 * "L" (below detection limit) and "U" (above detection limit) can 584 * also be used in place of a decimal value. 585 */ 586 public SampledData setData(String value) { 587 if (Utilities.noString(value)) 588 this.data = null; 589 else { 590 if (this.data == null) 591 this.data = new StringType(); 592 this.data.setValue(value); 593 } 594 return this; 595 } 596 597 protected void listChildren(List<Property> children) { 598 super.listChildren(children); 599 children.add(new Property("origin", "SimpleQuantity", 600 "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.", 601 0, 1, origin)); 602 children.add(new Property("period", "decimal", 603 "The length of time between sampling times, measured in milliseconds.", 0, 1, period)); 604 children.add(new Property("factor", "decimal", 605 "A correction factor that is applied to the sampled data points before they are added to the origin.", 0, 1, 606 factor)); 607 children.add(new Property("lowerLimit", "decimal", 608 "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).", 609 0, 1, lowerLimit)); 610 children.add(new Property("upperLimit", "decimal", 611 "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).", 612 0, 1, upperLimit)); 613 children.add(new Property("dimensions", "positiveInt", 614 "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.", 615 0, 1, dimensions)); 616 children.add(new Property("data", "string", 617 "A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value.", 618 0, 1, data)); 619 } 620 621 @Override 622 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 623 switch (_hash) { 624 case -1008619738: 625 /* origin */ return new Property("origin", "SimpleQuantity", 626 "The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series.", 627 0, 1, origin); 628 case -991726143: 629 /* period */ return new Property("period", "decimal", 630 "The length of time between sampling times, measured in milliseconds.", 0, 1, period); 631 case -1282148017: 632 /* factor */ return new Property("factor", "decimal", 633 "A correction factor that is applied to the sampled data points before they are added to the origin.", 0, 1, 634 factor); 635 case 1209133370: 636 /* lowerLimit */ return new Property("lowerLimit", "decimal", 637 "The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit).", 638 0, 1, lowerLimit); 639 case -1681713095: 640 /* upperLimit */ return new Property("upperLimit", "decimal", 641 "The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit).", 642 0, 1, upperLimit); 643 case 414334925: 644 /* dimensions */ return new Property("dimensions", "positiveInt", 645 "The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once.", 646 0, 1, dimensions); 647 case 3076010: 648 /* data */ return new Property("data", "string", 649 "A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value.", 650 0, 1, data); 651 default: 652 return super.getNamedProperty(_hash, _name, _checkValid); 653 } 654 655 } 656 657 @Override 658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 659 switch (hash) { 660 case -1008619738: 661 /* origin */ return this.origin == null ? new Base[0] : new Base[] { this.origin }; // Quantity 662 case -991726143: 663 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // DecimalType 664 case -1282148017: 665 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 666 case 1209133370: 667 /* lowerLimit */ return this.lowerLimit == null ? new Base[0] : new Base[] { this.lowerLimit }; // DecimalType 668 case -1681713095: 669 /* upperLimit */ return this.upperLimit == null ? new Base[0] : new Base[] { this.upperLimit }; // DecimalType 670 case 414334925: 671 /* dimensions */ return this.dimensions == null ? new Base[0] : new Base[] { this.dimensions }; // PositiveIntType 672 case 3076010: 673 /* data */ return this.data == null ? new Base[0] : new Base[] { this.data }; // StringType 674 default: 675 return super.getProperty(hash, name, checkValid); 676 } 677 678 } 679 680 @Override 681 public Base setProperty(int hash, String name, Base value) throws FHIRException { 682 switch (hash) { 683 case -1008619738: // origin 684 this.origin = castToQuantity(value); // Quantity 685 return value; 686 case -991726143: // period 687 this.period = castToDecimal(value); // DecimalType 688 return value; 689 case -1282148017: // factor 690 this.factor = castToDecimal(value); // DecimalType 691 return value; 692 case 1209133370: // lowerLimit 693 this.lowerLimit = castToDecimal(value); // DecimalType 694 return value; 695 case -1681713095: // upperLimit 696 this.upperLimit = castToDecimal(value); // DecimalType 697 return value; 698 case 414334925: // dimensions 699 this.dimensions = castToPositiveInt(value); // PositiveIntType 700 return value; 701 case 3076010: // data 702 this.data = castToString(value); // StringType 703 return value; 704 default: 705 return super.setProperty(hash, name, value); 706 } 707 708 } 709 710 @Override 711 public Base setProperty(String name, Base value) throws FHIRException { 712 if (name.equals("origin")) { 713 this.origin = castToQuantity(value); // Quantity 714 } else if (name.equals("period")) { 715 this.period = castToDecimal(value); // DecimalType 716 } else if (name.equals("factor")) { 717 this.factor = castToDecimal(value); // DecimalType 718 } else if (name.equals("lowerLimit")) { 719 this.lowerLimit = castToDecimal(value); // DecimalType 720 } else if (name.equals("upperLimit")) { 721 this.upperLimit = castToDecimal(value); // DecimalType 722 } else if (name.equals("dimensions")) { 723 this.dimensions = castToPositiveInt(value); // PositiveIntType 724 } else if (name.equals("data")) { 725 this.data = castToString(value); // StringType 726 } else 727 return super.setProperty(name, value); 728 return value; 729 } 730 731 @Override 732 public void removeChild(String name, Base value) throws FHIRException { 733 if (name.equals("origin")) { 734 this.origin = null; 735 } else if (name.equals("period")) { 736 this.period = null; 737 } else if (name.equals("factor")) { 738 this.factor = null; 739 } else if (name.equals("lowerLimit")) { 740 this.lowerLimit = null; 741 } else if (name.equals("upperLimit")) { 742 this.upperLimit = null; 743 } else if (name.equals("dimensions")) { 744 this.dimensions = null; 745 } else if (name.equals("data")) { 746 this.data = null; 747 } else 748 super.removeChild(name, value); 749 750 } 751 752 @Override 753 public Base makeProperty(int hash, String name) throws FHIRException { 754 switch (hash) { 755 case -1008619738: 756 return getOrigin(); 757 case -991726143: 758 return getPeriodElement(); 759 case -1282148017: 760 return getFactorElement(); 761 case 1209133370: 762 return getLowerLimitElement(); 763 case -1681713095: 764 return getUpperLimitElement(); 765 case 414334925: 766 return getDimensionsElement(); 767 case 3076010: 768 return getDataElement(); 769 default: 770 return super.makeProperty(hash, name); 771 } 772 773 } 774 775 @Override 776 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 777 switch (hash) { 778 case -1008619738: 779 /* origin */ return new String[] { "SimpleQuantity" }; 780 case -991726143: 781 /* period */ return new String[] { "decimal" }; 782 case -1282148017: 783 /* factor */ return new String[] { "decimal" }; 784 case 1209133370: 785 /* lowerLimit */ return new String[] { "decimal" }; 786 case -1681713095: 787 /* upperLimit */ return new String[] { "decimal" }; 788 case 414334925: 789 /* dimensions */ return new String[] { "positiveInt" }; 790 case 3076010: 791 /* data */ return new String[] { "string" }; 792 default: 793 return super.getTypesForProperty(hash, name); 794 } 795 796 } 797 798 @Override 799 public Base addChild(String name) throws FHIRException { 800 if (name.equals("origin")) { 801 this.origin = new Quantity(); 802 return this.origin; 803 } else if (name.equals("period")) { 804 throw new FHIRException("Cannot call addChild on a singleton property SampledData.period"); 805 } else if (name.equals("factor")) { 806 throw new FHIRException("Cannot call addChild on a singleton property SampledData.factor"); 807 } else if (name.equals("lowerLimit")) { 808 throw new FHIRException("Cannot call addChild on a singleton property SampledData.lowerLimit"); 809 } else if (name.equals("upperLimit")) { 810 throw new FHIRException("Cannot call addChild on a singleton property SampledData.upperLimit"); 811 } else if (name.equals("dimensions")) { 812 throw new FHIRException("Cannot call addChild on a singleton property SampledData.dimensions"); 813 } else if (name.equals("data")) { 814 throw new FHIRException("Cannot call addChild on a singleton property SampledData.data"); 815 } else 816 return super.addChild(name); 817 } 818 819 public String fhirType() { 820 return "SampledData"; 821 822 } 823 824 public SampledData copy() { 825 SampledData dst = new SampledData(); 826 copyValues(dst); 827 return dst; 828 } 829 830 public void copyValues(SampledData dst) { 831 super.copyValues(dst); 832 dst.origin = origin == null ? null : origin.copy(); 833 dst.period = period == null ? null : period.copy(); 834 dst.factor = factor == null ? null : factor.copy(); 835 dst.lowerLimit = lowerLimit == null ? null : lowerLimit.copy(); 836 dst.upperLimit = upperLimit == null ? null : upperLimit.copy(); 837 dst.dimensions = dimensions == null ? null : dimensions.copy(); 838 dst.data = data == null ? null : data.copy(); 839 } 840 841 protected SampledData typedCopy() { 842 return copy(); 843 } 844 845 @Override 846 public boolean equalsDeep(Base other_) { 847 if (!super.equalsDeep(other_)) 848 return false; 849 if (!(other_ instanceof SampledData)) 850 return false; 851 SampledData o = (SampledData) other_; 852 return compareDeep(origin, o.origin, true) && compareDeep(period, o.period, true) 853 && compareDeep(factor, o.factor, true) && compareDeep(lowerLimit, o.lowerLimit, true) 854 && compareDeep(upperLimit, o.upperLimit, true) && compareDeep(dimensions, o.dimensions, true) 855 && compareDeep(data, o.data, true); 856 } 857 858 @Override 859 public boolean equalsShallow(Base other_) { 860 if (!super.equalsShallow(other_)) 861 return false; 862 if (!(other_ instanceof SampledData)) 863 return false; 864 SampledData o = (SampledData) other_; 865 return compareValues(period, o.period, true) && compareValues(factor, o.factor, true) 866 && compareValues(lowerLimit, o.lowerLimit, true) && compareValues(upperLimit, o.upperLimit, true) 867 && compareValues(dimensions, o.dimensions, true) && compareValues(data, o.data, true); 868 } 869 870 public boolean isEmpty() { 871 return super.isEmpty() 872 && ca.uhn.fhir.util.ElementUtil.isEmpty(origin, period, factor, lowerLimit, upperLimit, dimensions, data); 873 } 874 875}