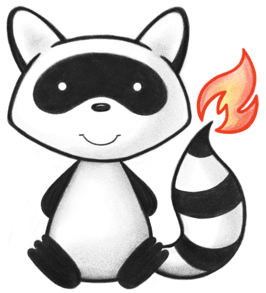
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.SearchParamType; 042import org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * A search parameter that defines a named search item that can be used to 054 * search/filter on a resource. 055 */ 056@ResourceDef(name = "SearchParameter", profile = "http://hl7.org/fhir/StructureDefinition/SearchParameter") 057@ChildOrder(names = { "url", "version", "name", "derivedFrom", "status", "experimental", "date", "publisher", "contact", 058 "description", "useContext", "jurisdiction", "purpose", "code", "base", "type", "expression", "xpath", "xpathUsage", 059 "target", "multipleOr", "multipleAnd", "comparator", "modifier", "chain", "component" }) 060public class SearchParameter extends MetadataResource { 061 062 public enum XPathUsageType { 063 /** 064 * The search parameter is derived directly from the selected nodes based on the 065 * type definitions. 066 */ 067 NORMAL, 068 /** 069 * The search parameter is derived by a phonetic transform from the selected 070 * nodes. 071 */ 072 PHONETIC, 073 /** 074 * The search parameter is based on a spatial transform of the selected nodes. 075 */ 076 NEARBY, 077 /** 078 * The search parameter is based on a spatial transform of the selected nodes, 079 * using physical distance from the middle. 080 */ 081 DISTANCE, 082 /** 083 * The interpretation of the xpath statement is unknown (and can't be 084 * automated). 085 */ 086 OTHER, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 092 public static XPathUsageType fromCode(String codeString) throws FHIRException { 093 if (codeString == null || "".equals(codeString)) 094 return null; 095 if ("normal".equals(codeString)) 096 return NORMAL; 097 if ("phonetic".equals(codeString)) 098 return PHONETIC; 099 if ("nearby".equals(codeString)) 100 return NEARBY; 101 if ("distance".equals(codeString)) 102 return DISTANCE; 103 if ("other".equals(codeString)) 104 return OTHER; 105 if (Configuration.isAcceptInvalidEnums()) 106 return null; 107 else 108 throw new FHIRException("Unknown XPathUsageType code '" + codeString + "'"); 109 } 110 111 public String toCode() { 112 switch (this) { 113 case NORMAL: 114 return "normal"; 115 case PHONETIC: 116 return "phonetic"; 117 case NEARBY: 118 return "nearby"; 119 case DISTANCE: 120 return "distance"; 121 case OTHER: 122 return "other"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getSystem() { 131 switch (this) { 132 case NORMAL: 133 return "http://hl7.org/fhir/search-xpath-usage"; 134 case PHONETIC: 135 return "http://hl7.org/fhir/search-xpath-usage"; 136 case NEARBY: 137 return "http://hl7.org/fhir/search-xpath-usage"; 138 case DISTANCE: 139 return "http://hl7.org/fhir/search-xpath-usage"; 140 case OTHER: 141 return "http://hl7.org/fhir/search-xpath-usage"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDefinition() { 150 switch (this) { 151 case NORMAL: 152 return "The search parameter is derived directly from the selected nodes based on the type definitions."; 153 case PHONETIC: 154 return "The search parameter is derived by a phonetic transform from the selected nodes."; 155 case NEARBY: 156 return "The search parameter is based on a spatial transform of the selected nodes."; 157 case DISTANCE: 158 return "The search parameter is based on a spatial transform of the selected nodes, using physical distance from the middle."; 159 case OTHER: 160 return "The interpretation of the xpath statement is unknown (and can't be automated)."; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDisplay() { 169 switch (this) { 170 case NORMAL: 171 return "Normal"; 172 case PHONETIC: 173 return "Phonetic"; 174 case NEARBY: 175 return "Nearby"; 176 case DISTANCE: 177 return "Distance"; 178 case OTHER: 179 return "Other"; 180 case NULL: 181 return null; 182 default: 183 return "?"; 184 } 185 } 186 } 187 188 public static class XPathUsageTypeEnumFactory implements EnumFactory<XPathUsageType> { 189 public XPathUsageType fromCode(String codeString) throws IllegalArgumentException { 190 if (codeString == null || "".equals(codeString)) 191 if (codeString == null || "".equals(codeString)) 192 return null; 193 if ("normal".equals(codeString)) 194 return XPathUsageType.NORMAL; 195 if ("phonetic".equals(codeString)) 196 return XPathUsageType.PHONETIC; 197 if ("nearby".equals(codeString)) 198 return XPathUsageType.NEARBY; 199 if ("distance".equals(codeString)) 200 return XPathUsageType.DISTANCE; 201 if ("other".equals(codeString)) 202 return XPathUsageType.OTHER; 203 throw new IllegalArgumentException("Unknown XPathUsageType code '" + codeString + "'"); 204 } 205 206 public Enumeration<XPathUsageType> fromType(PrimitiveType<?> code) throws FHIRException { 207 if (code == null) 208 return null; 209 if (code.isEmpty()) 210 return new Enumeration<XPathUsageType>(this, XPathUsageType.NULL, code); 211 String codeString = code.asStringValue(); 212 if (codeString == null || "".equals(codeString)) 213 return new Enumeration<XPathUsageType>(this, XPathUsageType.NULL, code); 214 if ("normal".equals(codeString)) 215 return new Enumeration<XPathUsageType>(this, XPathUsageType.NORMAL, code); 216 if ("phonetic".equals(codeString)) 217 return new Enumeration<XPathUsageType>(this, XPathUsageType.PHONETIC, code); 218 if ("nearby".equals(codeString)) 219 return new Enumeration<XPathUsageType>(this, XPathUsageType.NEARBY, code); 220 if ("distance".equals(codeString)) 221 return new Enumeration<XPathUsageType>(this, XPathUsageType.DISTANCE, code); 222 if ("other".equals(codeString)) 223 return new Enumeration<XPathUsageType>(this, XPathUsageType.OTHER, code); 224 throw new FHIRException("Unknown XPathUsageType code '" + codeString + "'"); 225 } 226 227 public String toCode(XPathUsageType code) { 228 if (code == XPathUsageType.NULL) 229 return null; 230 if (code == XPathUsageType.NORMAL) 231 return "normal"; 232 if (code == XPathUsageType.PHONETIC) 233 return "phonetic"; 234 if (code == XPathUsageType.NEARBY) 235 return "nearby"; 236 if (code == XPathUsageType.DISTANCE) 237 return "distance"; 238 if (code == XPathUsageType.OTHER) 239 return "other"; 240 return "?"; 241 } 242 243 public String toSystem(XPathUsageType code) { 244 return code.getSystem(); 245 } 246 } 247 248 public enum SearchComparator { 249 /** 250 * the value for the parameter in the resource is equal to the provided value. 251 */ 252 EQ, 253 /** 254 * the value for the parameter in the resource is not equal to the provided 255 * value. 256 */ 257 NE, 258 /** 259 * the value for the parameter in the resource is greater than the provided 260 * value. 261 */ 262 GT, 263 /** 264 * the value for the parameter in the resource is less than the provided value. 265 */ 266 LT, 267 /** 268 * the value for the parameter in the resource is greater or equal to the 269 * provided value. 270 */ 271 GE, 272 /** 273 * the value for the parameter in the resource is less or equal to the provided 274 * value. 275 */ 276 LE, 277 /** 278 * the value for the parameter in the resource starts after the provided value. 279 */ 280 SA, 281 /** 282 * the value for the parameter in the resource ends before the provided value. 283 */ 284 EB, 285 /** 286 * the value for the parameter in the resource is approximately the same to the 287 * provided value. 288 */ 289 AP, 290 /** 291 * added to help the parsers with the generic types 292 */ 293 NULL; 294 295 public static SearchComparator fromCode(String codeString) throws FHIRException { 296 if (codeString == null || "".equals(codeString)) 297 return null; 298 if ("eq".equals(codeString)) 299 return EQ; 300 if ("ne".equals(codeString)) 301 return NE; 302 if ("gt".equals(codeString)) 303 return GT; 304 if ("lt".equals(codeString)) 305 return LT; 306 if ("ge".equals(codeString)) 307 return GE; 308 if ("le".equals(codeString)) 309 return LE; 310 if ("sa".equals(codeString)) 311 return SA; 312 if ("eb".equals(codeString)) 313 return EB; 314 if ("ap".equals(codeString)) 315 return AP; 316 if (Configuration.isAcceptInvalidEnums()) 317 return null; 318 else 319 throw new FHIRException("Unknown SearchComparator code '" + codeString + "'"); 320 } 321 322 public String toCode() { 323 switch (this) { 324 case EQ: 325 return "eq"; 326 case NE: 327 return "ne"; 328 case GT: 329 return "gt"; 330 case LT: 331 return "lt"; 332 case GE: 333 return "ge"; 334 case LE: 335 return "le"; 336 case SA: 337 return "sa"; 338 case EB: 339 return "eb"; 340 case AP: 341 return "ap"; 342 case NULL: 343 return null; 344 default: 345 return "?"; 346 } 347 } 348 349 public String getSystem() { 350 switch (this) { 351 case EQ: 352 return "http://hl7.org/fhir/search-comparator"; 353 case NE: 354 return "http://hl7.org/fhir/search-comparator"; 355 case GT: 356 return "http://hl7.org/fhir/search-comparator"; 357 case LT: 358 return "http://hl7.org/fhir/search-comparator"; 359 case GE: 360 return "http://hl7.org/fhir/search-comparator"; 361 case LE: 362 return "http://hl7.org/fhir/search-comparator"; 363 case SA: 364 return "http://hl7.org/fhir/search-comparator"; 365 case EB: 366 return "http://hl7.org/fhir/search-comparator"; 367 case AP: 368 return "http://hl7.org/fhir/search-comparator"; 369 case NULL: 370 return null; 371 default: 372 return "?"; 373 } 374 } 375 376 public String getDefinition() { 377 switch (this) { 378 case EQ: 379 return "the value for the parameter in the resource is equal to the provided value."; 380 case NE: 381 return "the value for the parameter in the resource is not equal to the provided value."; 382 case GT: 383 return "the value for the parameter in the resource is greater than the provided value."; 384 case LT: 385 return "the value for the parameter in the resource is less than the provided value."; 386 case GE: 387 return "the value for the parameter in the resource is greater or equal to the provided value."; 388 case LE: 389 return "the value for the parameter in the resource is less or equal to the provided value."; 390 case SA: 391 return "the value for the parameter in the resource starts after the provided value."; 392 case EB: 393 return "the value for the parameter in the resource ends before the provided value."; 394 case AP: 395 return "the value for the parameter in the resource is approximately the same to the provided value."; 396 case NULL: 397 return null; 398 default: 399 return "?"; 400 } 401 } 402 403 public String getDisplay() { 404 switch (this) { 405 case EQ: 406 return "Equals"; 407 case NE: 408 return "Not Equals"; 409 case GT: 410 return "Greater Than"; 411 case LT: 412 return "Less Than"; 413 case GE: 414 return "Greater or Equals"; 415 case LE: 416 return "Less of Equal"; 417 case SA: 418 return "Starts After"; 419 case EB: 420 return "Ends Before"; 421 case AP: 422 return "Approximately"; 423 case NULL: 424 return null; 425 default: 426 return "?"; 427 } 428 } 429 } 430 431 public static class SearchComparatorEnumFactory implements EnumFactory<SearchComparator> { 432 public SearchComparator fromCode(String codeString) throws IllegalArgumentException { 433 if (codeString == null || "".equals(codeString)) 434 if (codeString == null || "".equals(codeString)) 435 return null; 436 if ("eq".equals(codeString)) 437 return SearchComparator.EQ; 438 if ("ne".equals(codeString)) 439 return SearchComparator.NE; 440 if ("gt".equals(codeString)) 441 return SearchComparator.GT; 442 if ("lt".equals(codeString)) 443 return SearchComparator.LT; 444 if ("ge".equals(codeString)) 445 return SearchComparator.GE; 446 if ("le".equals(codeString)) 447 return SearchComparator.LE; 448 if ("sa".equals(codeString)) 449 return SearchComparator.SA; 450 if ("eb".equals(codeString)) 451 return SearchComparator.EB; 452 if ("ap".equals(codeString)) 453 return SearchComparator.AP; 454 throw new IllegalArgumentException("Unknown SearchComparator code '" + codeString + "'"); 455 } 456 457 public Enumeration<SearchComparator> fromType(PrimitiveType<?> code) throws FHIRException { 458 if (code == null) 459 return null; 460 if (code.isEmpty()) 461 return new Enumeration<SearchComparator>(this, SearchComparator.NULL, code); 462 String codeString = code.asStringValue(); 463 if (codeString == null || "".equals(codeString)) 464 return new Enumeration<SearchComparator>(this, SearchComparator.NULL, code); 465 if ("eq".equals(codeString)) 466 return new Enumeration<SearchComparator>(this, SearchComparator.EQ, code); 467 if ("ne".equals(codeString)) 468 return new Enumeration<SearchComparator>(this, SearchComparator.NE, code); 469 if ("gt".equals(codeString)) 470 return new Enumeration<SearchComparator>(this, SearchComparator.GT, code); 471 if ("lt".equals(codeString)) 472 return new Enumeration<SearchComparator>(this, SearchComparator.LT, code); 473 if ("ge".equals(codeString)) 474 return new Enumeration<SearchComparator>(this, SearchComparator.GE, code); 475 if ("le".equals(codeString)) 476 return new Enumeration<SearchComparator>(this, SearchComparator.LE, code); 477 if ("sa".equals(codeString)) 478 return new Enumeration<SearchComparator>(this, SearchComparator.SA, code); 479 if ("eb".equals(codeString)) 480 return new Enumeration<SearchComparator>(this, SearchComparator.EB, code); 481 if ("ap".equals(codeString)) 482 return new Enumeration<SearchComparator>(this, SearchComparator.AP, code); 483 throw new FHIRException("Unknown SearchComparator code '" + codeString + "'"); 484 } 485 486 public String toCode(SearchComparator code) { 487 if (code == SearchComparator.NULL) 488 return null; 489 if (code == SearchComparator.EQ) 490 return "eq"; 491 if (code == SearchComparator.NE) 492 return "ne"; 493 if (code == SearchComparator.GT) 494 return "gt"; 495 if (code == SearchComparator.LT) 496 return "lt"; 497 if (code == SearchComparator.GE) 498 return "ge"; 499 if (code == SearchComparator.LE) 500 return "le"; 501 if (code == SearchComparator.SA) 502 return "sa"; 503 if (code == SearchComparator.EB) 504 return "eb"; 505 if (code == SearchComparator.AP) 506 return "ap"; 507 return "?"; 508 } 509 510 public String toSystem(SearchComparator code) { 511 return code.getSystem(); 512 } 513 } 514 515 public enum SearchModifierCode { 516 /** 517 * The search parameter returns resources that have a value or not. 518 */ 519 MISSING, 520 /** 521 * The search parameter returns resources that have a value that exactly matches 522 * the supplied parameter (the whole string, including casing and accents). 523 */ 524 EXACT, 525 /** 526 * The search parameter returns resources that include the supplied parameter 527 * value anywhere within the field being searched. 528 */ 529 CONTAINS, 530 /** 531 * The search parameter returns resources that do not contain a match. 532 */ 533 NOT, 534 /** 535 * The search parameter is processed as a string that searches text associated 536 * with the code/value - either CodeableConcept.text, Coding.display, or 537 * Identifier.type.text. 538 */ 539 TEXT, 540 /** 541 * The search parameter is a URI (relative or absolute) that identifies a value 542 * set, and the search parameter tests whether the coding is in the specified 543 * value set. 544 */ 545 IN, 546 /** 547 * The search parameter is a URI (relative or absolute) that identifies a value 548 * set, and the search parameter tests whether the coding is not in the 549 * specified value set. 550 */ 551 NOTIN, 552 /** 553 * The search parameter tests whether the value in a resource is subsumed by the 554 * specified value (is-a, or hierarchical relationships). 555 */ 556 BELOW, 557 /** 558 * The search parameter tests whether the value in a resource subsumes the 559 * specified value (is-a, or hierarchical relationships). 560 */ 561 ABOVE, 562 /** 563 * The search parameter only applies to the Resource Type specified as a 564 * modifier (e.g. the modifier is not actually :type, but :Patient etc.). 565 */ 566 TYPE, 567 /** 568 * The search parameter applies to the identifier on the resource, not the 569 * reference. 570 */ 571 IDENTIFIER, 572 /** 573 * The search parameter has the format system|code|value, where the system and 574 * code refer to an Identifier.type.coding.system and .code, and match if any of 575 * the type codes match. All 3 parts must be present. 576 */ 577 OFTYPE, 578 /** 579 * added to help the parsers with the generic types 580 */ 581 NULL; 582 583 public static SearchModifierCode fromCode(String codeString) throws FHIRException { 584 if (codeString == null || "".equals(codeString)) 585 return null; 586 if ("missing".equals(codeString)) 587 return MISSING; 588 if ("exact".equals(codeString)) 589 return EXACT; 590 if ("contains".equals(codeString)) 591 return CONTAINS; 592 if ("not".equals(codeString)) 593 return NOT; 594 if ("text".equals(codeString)) 595 return TEXT; 596 if ("in".equals(codeString)) 597 return IN; 598 if ("not-in".equals(codeString)) 599 return NOTIN; 600 if ("below".equals(codeString)) 601 return BELOW; 602 if ("above".equals(codeString)) 603 return ABOVE; 604 if ("type".equals(codeString)) 605 return TYPE; 606 if ("identifier".equals(codeString)) 607 return IDENTIFIER; 608 if ("ofType".equals(codeString)) 609 return OFTYPE; 610 if (Configuration.isAcceptInvalidEnums()) 611 return null; 612 else 613 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 614 } 615 616 public String toCode() { 617 switch (this) { 618 case MISSING: 619 return "missing"; 620 case EXACT: 621 return "exact"; 622 case CONTAINS: 623 return "contains"; 624 case NOT: 625 return "not"; 626 case TEXT: 627 return "text"; 628 case IN: 629 return "in"; 630 case NOTIN: 631 return "not-in"; 632 case BELOW: 633 return "below"; 634 case ABOVE: 635 return "above"; 636 case TYPE: 637 return "type"; 638 case IDENTIFIER: 639 return "identifier"; 640 case OFTYPE: 641 return "ofType"; 642 case NULL: 643 return null; 644 default: 645 return "?"; 646 } 647 } 648 649 public String getSystem() { 650 switch (this) { 651 case MISSING: 652 return "http://hl7.org/fhir/search-modifier-code"; 653 case EXACT: 654 return "http://hl7.org/fhir/search-modifier-code"; 655 case CONTAINS: 656 return "http://hl7.org/fhir/search-modifier-code"; 657 case NOT: 658 return "http://hl7.org/fhir/search-modifier-code"; 659 case TEXT: 660 return "http://hl7.org/fhir/search-modifier-code"; 661 case IN: 662 return "http://hl7.org/fhir/search-modifier-code"; 663 case NOTIN: 664 return "http://hl7.org/fhir/search-modifier-code"; 665 case BELOW: 666 return "http://hl7.org/fhir/search-modifier-code"; 667 case ABOVE: 668 return "http://hl7.org/fhir/search-modifier-code"; 669 case TYPE: 670 return "http://hl7.org/fhir/search-modifier-code"; 671 case IDENTIFIER: 672 return "http://hl7.org/fhir/search-modifier-code"; 673 case OFTYPE: 674 return "http://hl7.org/fhir/search-modifier-code"; 675 case NULL: 676 return null; 677 default: 678 return "?"; 679 } 680 } 681 682 public String getDefinition() { 683 switch (this) { 684 case MISSING: 685 return "The search parameter returns resources that have a value or not."; 686 case EXACT: 687 return "The search parameter returns resources that have a value that exactly matches the supplied parameter (the whole string, including casing and accents)."; 688 case CONTAINS: 689 return "The search parameter returns resources that include the supplied parameter value anywhere within the field being searched."; 690 case NOT: 691 return "The search parameter returns resources that do not contain a match."; 692 case TEXT: 693 return "The search parameter is processed as a string that searches text associated with the code/value - either CodeableConcept.text, Coding.display, or Identifier.type.text."; 694 case IN: 695 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is in the specified value set."; 696 case NOTIN: 697 return "The search parameter is a URI (relative or absolute) that identifies a value set, and the search parameter tests whether the coding is not in the specified value set."; 698 case BELOW: 699 return "The search parameter tests whether the value in a resource is subsumed by the specified value (is-a, or hierarchical relationships)."; 700 case ABOVE: 701 return "The search parameter tests whether the value in a resource subsumes the specified value (is-a, or hierarchical relationships)."; 702 case TYPE: 703 return "The search parameter only applies to the Resource Type specified as a modifier (e.g. the modifier is not actually :type, but :Patient etc.)."; 704 case IDENTIFIER: 705 return "The search parameter applies to the identifier on the resource, not the reference."; 706 case OFTYPE: 707 return "The search parameter has the format system|code|value, where the system and code refer to an Identifier.type.coding.system and .code, and match if any of the type codes match. All 3 parts must be present."; 708 case NULL: 709 return null; 710 default: 711 return "?"; 712 } 713 } 714 715 public String getDisplay() { 716 switch (this) { 717 case MISSING: 718 return "Missing"; 719 case EXACT: 720 return "Exact"; 721 case CONTAINS: 722 return "Contains"; 723 case NOT: 724 return "Not"; 725 case TEXT: 726 return "Text"; 727 case IN: 728 return "In"; 729 case NOTIN: 730 return "Not In"; 731 case BELOW: 732 return "Below"; 733 case ABOVE: 734 return "Above"; 735 case TYPE: 736 return "Type"; 737 case IDENTIFIER: 738 return "Identifier"; 739 case OFTYPE: 740 return "Of Type"; 741 case NULL: 742 return null; 743 default: 744 return "?"; 745 } 746 } 747 } 748 749 public static class SearchModifierCodeEnumFactory implements EnumFactory<SearchModifierCode> { 750 public SearchModifierCode fromCode(String codeString) throws IllegalArgumentException { 751 if (codeString == null || "".equals(codeString)) 752 if (codeString == null || "".equals(codeString)) 753 return null; 754 if ("missing".equals(codeString)) 755 return SearchModifierCode.MISSING; 756 if ("exact".equals(codeString)) 757 return SearchModifierCode.EXACT; 758 if ("contains".equals(codeString)) 759 return SearchModifierCode.CONTAINS; 760 if ("not".equals(codeString)) 761 return SearchModifierCode.NOT; 762 if ("text".equals(codeString)) 763 return SearchModifierCode.TEXT; 764 if ("in".equals(codeString)) 765 return SearchModifierCode.IN; 766 if ("not-in".equals(codeString)) 767 return SearchModifierCode.NOTIN; 768 if ("below".equals(codeString)) 769 return SearchModifierCode.BELOW; 770 if ("above".equals(codeString)) 771 return SearchModifierCode.ABOVE; 772 if ("type".equals(codeString)) 773 return SearchModifierCode.TYPE; 774 if ("identifier".equals(codeString)) 775 return SearchModifierCode.IDENTIFIER; 776 if ("ofType".equals(codeString)) 777 return SearchModifierCode.OFTYPE; 778 throw new IllegalArgumentException("Unknown SearchModifierCode code '" + codeString + "'"); 779 } 780 781 public Enumeration<SearchModifierCode> fromType(PrimitiveType<?> code) throws FHIRException { 782 if (code == null) 783 return null; 784 if (code.isEmpty()) 785 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NULL, code); 786 String codeString = code.asStringValue(); 787 if (codeString == null || "".equals(codeString)) 788 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NULL, code); 789 if ("missing".equals(codeString)) 790 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.MISSING, code); 791 if ("exact".equals(codeString)) 792 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.EXACT, code); 793 if ("contains".equals(codeString)) 794 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.CONTAINS, code); 795 if ("not".equals(codeString)) 796 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOT, code); 797 if ("text".equals(codeString)) 798 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TEXT, code); 799 if ("in".equals(codeString)) 800 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.IN, code); 801 if ("not-in".equals(codeString)) 802 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.NOTIN, code); 803 if ("below".equals(codeString)) 804 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.BELOW, code); 805 if ("above".equals(codeString)) 806 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.ABOVE, code); 807 if ("type".equals(codeString)) 808 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.TYPE, code); 809 if ("identifier".equals(codeString)) 810 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.IDENTIFIER, code); 811 if ("ofType".equals(codeString)) 812 return new Enumeration<SearchModifierCode>(this, SearchModifierCode.OFTYPE, code); 813 throw new FHIRException("Unknown SearchModifierCode code '" + codeString + "'"); 814 } 815 816 public String toCode(SearchModifierCode code) { 817 if (code == SearchModifierCode.NULL) 818 return null; 819 if (code == SearchModifierCode.MISSING) 820 return "missing"; 821 if (code == SearchModifierCode.EXACT) 822 return "exact"; 823 if (code == SearchModifierCode.CONTAINS) 824 return "contains"; 825 if (code == SearchModifierCode.NOT) 826 return "not"; 827 if (code == SearchModifierCode.TEXT) 828 return "text"; 829 if (code == SearchModifierCode.IN) 830 return "in"; 831 if (code == SearchModifierCode.NOTIN) 832 return "not-in"; 833 if (code == SearchModifierCode.BELOW) 834 return "below"; 835 if (code == SearchModifierCode.ABOVE) 836 return "above"; 837 if (code == SearchModifierCode.TYPE) 838 return "type"; 839 if (code == SearchModifierCode.IDENTIFIER) 840 return "identifier"; 841 if (code == SearchModifierCode.OFTYPE) 842 return "ofType"; 843 return "?"; 844 } 845 846 public String toSystem(SearchModifierCode code) { 847 return code.getSystem(); 848 } 849 } 850 851 @Block() 852 public static class SearchParameterComponentComponent extends BackboneElement implements IBaseBackboneElement { 853 /** 854 * The definition of the search parameter that describes this part. 855 */ 856 @Child(name = "definition", type = { 857 CanonicalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 858 @Description(shortDefinition = "Defines how the part works", formalDefinition = "The definition of the search parameter that describes this part.") 859 protected CanonicalType definition; 860 861 /** 862 * A sub-expression that defines how to extract values for this component from 863 * the output of the main SearchParameter.expression. 864 */ 865 @Child(name = "expression", type = { 866 StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 867 @Description(shortDefinition = "Subexpression relative to main expression", formalDefinition = "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.") 868 protected StringType expression; 869 870 private static final long serialVersionUID = -1469435618L; 871 872 /** 873 * Constructor 874 */ 875 public SearchParameterComponentComponent() { 876 super(); 877 } 878 879 /** 880 * Constructor 881 */ 882 public SearchParameterComponentComponent(CanonicalType definition, StringType expression) { 883 super(); 884 this.definition = definition; 885 this.expression = expression; 886 } 887 888 /** 889 * @return {@link #definition} (The definition of the search parameter that 890 * describes this part.). This is the underlying object with id, value 891 * and extensions. The accessor "getDefinition" gives direct access to 892 * the value 893 */ 894 public CanonicalType getDefinitionElement() { 895 if (this.definition == null) 896 if (Configuration.errorOnAutoCreate()) 897 throw new Error("Attempt to auto-create SearchParameterComponentComponent.definition"); 898 else if (Configuration.doAutoCreate()) 899 this.definition = new CanonicalType(); // bb 900 return this.definition; 901 } 902 903 public boolean hasDefinitionElement() { 904 return this.definition != null && !this.definition.isEmpty(); 905 } 906 907 public boolean hasDefinition() { 908 return this.definition != null && !this.definition.isEmpty(); 909 } 910 911 /** 912 * @param value {@link #definition} (The definition of the search parameter that 913 * describes this part.). This is the underlying object with id, 914 * value and extensions. The accessor "getDefinition" gives direct 915 * access to the value 916 */ 917 public SearchParameterComponentComponent setDefinitionElement(CanonicalType value) { 918 this.definition = value; 919 return this; 920 } 921 922 /** 923 * @return The definition of the search parameter that describes this part. 924 */ 925 public String getDefinition() { 926 return this.definition == null ? null : this.definition.getValue(); 927 } 928 929 /** 930 * @param value The definition of the search parameter that describes this part. 931 */ 932 public SearchParameterComponentComponent setDefinition(String value) { 933 if (this.definition == null) 934 this.definition = new CanonicalType(); 935 this.definition.setValue(value); 936 return this; 937 } 938 939 /** 940 * @return {@link #expression} (A sub-expression that defines how to extract 941 * values for this component from the output of the main 942 * SearchParameter.expression.). This is the underlying object with id, 943 * value and extensions. The accessor "getExpression" gives direct 944 * access to the value 945 */ 946 public StringType getExpressionElement() { 947 if (this.expression == null) 948 if (Configuration.errorOnAutoCreate()) 949 throw new Error("Attempt to auto-create SearchParameterComponentComponent.expression"); 950 else if (Configuration.doAutoCreate()) 951 this.expression = new StringType(); // bb 952 return this.expression; 953 } 954 955 public boolean hasExpressionElement() { 956 return this.expression != null && !this.expression.isEmpty(); 957 } 958 959 public boolean hasExpression() { 960 return this.expression != null && !this.expression.isEmpty(); 961 } 962 963 /** 964 * @param value {@link #expression} (A sub-expression that defines how to 965 * extract values for this component from the output of the main 966 * SearchParameter.expression.). This is the underlying object with 967 * id, value and extensions. The accessor "getExpression" gives 968 * direct access to the value 969 */ 970 public SearchParameterComponentComponent setExpressionElement(StringType value) { 971 this.expression = value; 972 return this; 973 } 974 975 /** 976 * @return A sub-expression that defines how to extract values for this 977 * component from the output of the main SearchParameter.expression. 978 */ 979 public String getExpression() { 980 return this.expression == null ? null : this.expression.getValue(); 981 } 982 983 /** 984 * @param value A sub-expression that defines how to extract values for this 985 * component from the output of the main 986 * SearchParameter.expression. 987 */ 988 public SearchParameterComponentComponent setExpression(String value) { 989 if (this.expression == null) 990 this.expression = new StringType(); 991 this.expression.setValue(value); 992 return this; 993 } 994 995 protected void listChildren(List<Property> children) { 996 super.listChildren(children); 997 children.add(new Property("definition", "canonical(SearchParameter)", 998 "The definition of the search parameter that describes this part.", 0, 1, definition)); 999 children.add(new Property("expression", "string", 1000 "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 1001 0, 1, expression)); 1002 } 1003 1004 @Override 1005 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1006 switch (_hash) { 1007 case -1014418093: 1008 /* definition */ return new Property("definition", "canonical(SearchParameter)", 1009 "The definition of the search parameter that describes this part.", 0, 1, definition); 1010 case -1795452264: 1011 /* expression */ return new Property("expression", "string", 1012 "A sub-expression that defines how to extract values for this component from the output of the main SearchParameter.expression.", 1013 0, 1, expression); 1014 default: 1015 return super.getNamedProperty(_hash, _name, _checkValid); 1016 } 1017 1018 } 1019 1020 @Override 1021 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1022 switch (hash) { 1023 case -1014418093: 1024 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 1025 case -1795452264: 1026 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 1027 default: 1028 return super.getProperty(hash, name, checkValid); 1029 } 1030 1031 } 1032 1033 @Override 1034 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1035 switch (hash) { 1036 case -1014418093: // definition 1037 this.definition = castToCanonical(value); // CanonicalType 1038 return value; 1039 case -1795452264: // expression 1040 this.expression = castToString(value); // StringType 1041 return value; 1042 default: 1043 return super.setProperty(hash, name, value); 1044 } 1045 1046 } 1047 1048 @Override 1049 public Base setProperty(String name, Base value) throws FHIRException { 1050 if (name.equals("definition")) { 1051 this.definition = castToCanonical(value); // CanonicalType 1052 } else if (name.equals("expression")) { 1053 this.expression = castToString(value); // StringType 1054 } else 1055 return super.setProperty(name, value); 1056 return value; 1057 } 1058 1059 @Override 1060 public void removeChild(String name, Base value) throws FHIRException { 1061 if (name.equals("definition")) { 1062 this.definition = null; 1063 } else if (name.equals("expression")) { 1064 this.expression = null; 1065 } else 1066 super.removeChild(name, value); 1067 1068 } 1069 1070 @Override 1071 public Base makeProperty(int hash, String name) throws FHIRException { 1072 switch (hash) { 1073 case -1014418093: 1074 return getDefinitionElement(); 1075 case -1795452264: 1076 return getExpressionElement(); 1077 default: 1078 return super.makeProperty(hash, name); 1079 } 1080 1081 } 1082 1083 @Override 1084 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1085 switch (hash) { 1086 case -1014418093: 1087 /* definition */ return new String[] { "canonical" }; 1088 case -1795452264: 1089 /* expression */ return new String[] { "string" }; 1090 default: 1091 return super.getTypesForProperty(hash, name); 1092 } 1093 1094 } 1095 1096 @Override 1097 public Base addChild(String name) throws FHIRException { 1098 if (name.equals("definition")) { 1099 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.definition"); 1100 } else if (name.equals("expression")) { 1101 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 1102 } else 1103 return super.addChild(name); 1104 } 1105 1106 public SearchParameterComponentComponent copy() { 1107 SearchParameterComponentComponent dst = new SearchParameterComponentComponent(); 1108 copyValues(dst); 1109 return dst; 1110 } 1111 1112 public void copyValues(SearchParameterComponentComponent dst) { 1113 super.copyValues(dst); 1114 dst.definition = definition == null ? null : definition.copy(); 1115 dst.expression = expression == null ? null : expression.copy(); 1116 } 1117 1118 @Override 1119 public boolean equalsDeep(Base other_) { 1120 if (!super.equalsDeep(other_)) 1121 return false; 1122 if (!(other_ instanceof SearchParameterComponentComponent)) 1123 return false; 1124 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 1125 return compareDeep(definition, o.definition, true) && compareDeep(expression, o.expression, true); 1126 } 1127 1128 @Override 1129 public boolean equalsShallow(Base other_) { 1130 if (!super.equalsShallow(other_)) 1131 return false; 1132 if (!(other_ instanceof SearchParameterComponentComponent)) 1133 return false; 1134 SearchParameterComponentComponent o = (SearchParameterComponentComponent) other_; 1135 return compareValues(expression, o.expression, true); 1136 } 1137 1138 public boolean isEmpty() { 1139 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, expression); 1140 } 1141 1142 public String fhirType() { 1143 return "SearchParameter.component"; 1144 1145 } 1146 1147 } 1148 1149 /** 1150 * Where this search parameter is originally defined. If a derivedFrom is 1151 * provided, then the details in the search parameter must be consistent with 1152 * the definition from which it is defined. i.e. the parameter should have the 1153 * same meaning, and (usually) the functionality should be a proper subset of 1154 * the underlying search parameter. 1155 */ 1156 @Child(name = "derivedFrom", type = { 1157 CanonicalType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 1158 @Description(shortDefinition = "Original definition for the search parameter", formalDefinition = "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.") 1159 protected CanonicalType derivedFrom; 1160 1161 /** 1162 * Explanation of why this search parameter is needed and why it has been 1163 * designed as it has. 1164 */ 1165 @Child(name = "purpose", type = { 1166 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1167 @Description(shortDefinition = "Why this search parameter is defined", formalDefinition = "Explanation of why this search parameter is needed and why it has been designed as it has.") 1168 protected MarkdownType purpose; 1169 1170 /** 1171 * The code used in the URL or the parameter name in a parameters resource for 1172 * this search parameter. 1173 */ 1174 @Child(name = "code", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1175 @Description(shortDefinition = "Code used in URL", formalDefinition = "The code used in the URL or the parameter name in a parameters resource for this search parameter.") 1176 protected CodeType code; 1177 1178 /** 1179 * The base resource type(s) that this search parameter can be used against. 1180 */ 1181 @Child(name = "base", type = { 1182 CodeType.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1183 @Description(shortDefinition = "The resource type(s) this search parameter applies to", formalDefinition = "The base resource type(s) that this search parameter can be used against.") 1184 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 1185 protected List<CodeType> base; 1186 1187 /** 1188 * The type of value that a search parameter may contain, and how the content is 1189 * interpreted. 1190 */ 1191 @Child(name = "type", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1192 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri | special", formalDefinition = "The type of value that a search parameter may contain, and how the content is interpreted.") 1193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-param-type") 1194 protected Enumeration<SearchParamType> type; 1195 1196 /** 1197 * A FHIRPath expression that returns a set of elements for the search 1198 * parameter. 1199 */ 1200 @Child(name = "expression", type = { 1201 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1202 @Description(shortDefinition = "FHIRPath expression that extracts the values", formalDefinition = "A FHIRPath expression that returns a set of elements for the search parameter.") 1203 protected StringType expression; 1204 1205 /** 1206 * An XPath expression that returns a set of elements for the search parameter. 1207 */ 1208 @Child(name = "xpath", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1209 @Description(shortDefinition = "XPath that extracts the values", formalDefinition = "An XPath expression that returns a set of elements for the search parameter.") 1210 protected StringType xpath; 1211 1212 /** 1213 * How the search parameter relates to the set of elements returned by 1214 * evaluating the xpath query. 1215 */ 1216 @Child(name = "xpathUsage", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1217 @Description(shortDefinition = "normal | phonetic | nearby | distance | other", formalDefinition = "How the search parameter relates to the set of elements returned by evaluating the xpath query.") 1218 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-xpath-usage") 1219 protected Enumeration<XPathUsageType> xpathUsage; 1220 1221 /** 1222 * Types of resource (if a resource is referenced). 1223 */ 1224 @Child(name = "target", type = { 1225 CodeType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1226 @Description(shortDefinition = "Types of resource (if a resource reference)", formalDefinition = "Types of resource (if a resource is referenced).") 1227 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 1228 protected List<CodeType> target; 1229 1230 /** 1231 * Whether multiple values are allowed for each time the parameter exists. 1232 * Values are separated by commas, and the parameter matches if any of the 1233 * values match. 1234 */ 1235 @Child(name = "multipleOr", type = { 1236 BooleanType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1237 @Description(shortDefinition = "Allow multiple values per parameter (or)", formalDefinition = "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.") 1238 protected BooleanType multipleOr; 1239 1240 /** 1241 * Whether multiple parameters are allowed - e.g. more than one parameter with 1242 * the same name. The search matches if all the parameters match. 1243 */ 1244 @Child(name = "multipleAnd", type = { 1245 BooleanType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1246 @Description(shortDefinition = "Allow multiple parameters (and)", formalDefinition = "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.") 1247 protected BooleanType multipleAnd; 1248 1249 /** 1250 * Comparators supported for the search parameter. 1251 */ 1252 @Child(name = "comparator", type = { 1253 CodeType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1254 @Description(shortDefinition = "eq | ne | gt | lt | ge | le | sa | eb | ap", formalDefinition = "Comparators supported for the search parameter.") 1255 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-comparator") 1256 protected List<Enumeration<SearchComparator>> comparator; 1257 1258 /** 1259 * A modifier supported for the search parameter. 1260 */ 1261 @Child(name = "modifier", type = { 1262 CodeType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1263 @Description(shortDefinition = "missing | exact | contains | not | text | in | not-in | below | above | type | identifier | ofType", formalDefinition = "A modifier supported for the search parameter.") 1264 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-modifier-code") 1265 protected List<Enumeration<SearchModifierCode>> modifier; 1266 1267 /** 1268 * Contains the names of any search parameters which may be chained to the 1269 * containing search parameter. Chained parameters may be added to search 1270 * parameters of type reference and specify that resources will only be returned 1271 * if they contain a reference to a resource which matches the chained parameter 1272 * value. Values for this field should be drawn from SearchParameter.code for a 1273 * parameter on the target resource type. 1274 */ 1275 @Child(name = "chain", type = { 1276 StringType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1277 @Description(shortDefinition = "Chained names supported", formalDefinition = "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.") 1278 protected List<StringType> chain; 1279 1280 /** 1281 * Used to define the parts of a composite search parameter. 1282 */ 1283 @Child(name = "component", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1284 @Description(shortDefinition = "For Composite resources to define the parts", formalDefinition = "Used to define the parts of a composite search parameter.") 1285 protected List<SearchParameterComponentComponent> component; 1286 1287 private static final long serialVersionUID = -533803519L; 1288 1289 /** 1290 * Constructor 1291 */ 1292 public SearchParameter() { 1293 super(); 1294 } 1295 1296 /** 1297 * Constructor 1298 */ 1299 public SearchParameter(UriType url, StringType name, Enumeration<PublicationStatus> status, MarkdownType description, 1300 CodeType code, Enumeration<SearchParamType> type) { 1301 super(); 1302 this.url = url; 1303 this.name = name; 1304 this.status = status; 1305 this.description = description; 1306 this.code = code; 1307 this.type = type; 1308 } 1309 1310 /** 1311 * @return {@link #url} (An absolute URI that is used to identify this search 1312 * parameter when it is referenced in a specification, model, design or 1313 * an instance; also called its canonical identifier. This SHOULD be 1314 * globally unique and SHOULD be a literal address at which at which an 1315 * authoritative instance of this search parameter is (or will be) 1316 * published. This URL can be the target of a canonical reference. It 1317 * SHALL remain the same when the search parameter is stored on 1318 * different servers.). This is the underlying object with id, value and 1319 * extensions. The accessor "getUrl" gives direct access to the value 1320 */ 1321 public UriType getUrlElement() { 1322 if (this.url == null) 1323 if (Configuration.errorOnAutoCreate()) 1324 throw new Error("Attempt to auto-create SearchParameter.url"); 1325 else if (Configuration.doAutoCreate()) 1326 this.url = new UriType(); // bb 1327 return this.url; 1328 } 1329 1330 public boolean hasUrlElement() { 1331 return this.url != null && !this.url.isEmpty(); 1332 } 1333 1334 public boolean hasUrl() { 1335 return this.url != null && !this.url.isEmpty(); 1336 } 1337 1338 /** 1339 * @param value {@link #url} (An absolute URI that is used to identify this 1340 * search parameter when it is referenced in a specification, 1341 * model, design or an instance; also called its canonical 1342 * identifier. This SHOULD be globally unique and SHOULD be a 1343 * literal address at which at which an authoritative instance of 1344 * this search parameter is (or will be) published. This URL can be 1345 * the target of a canonical reference. It SHALL remain the same 1346 * when the search parameter is stored on different servers.). This 1347 * is the underlying object with id, value and extensions. The 1348 * accessor "getUrl" gives direct access to the value 1349 */ 1350 public SearchParameter setUrlElement(UriType value) { 1351 this.url = value; 1352 return this; 1353 } 1354 1355 /** 1356 * @return An absolute URI that is used to identify this search parameter when 1357 * it is referenced in a specification, model, design or an instance; 1358 * also called its canonical identifier. This SHOULD be globally unique 1359 * and SHOULD be a literal address at which at which an authoritative 1360 * instance of this search parameter is (or will be) published. This URL 1361 * can be the target of a canonical reference. It SHALL remain the same 1362 * when the search parameter is stored on different servers. 1363 */ 1364 public String getUrl() { 1365 return this.url == null ? null : this.url.getValue(); 1366 } 1367 1368 /** 1369 * @param value An absolute URI that is used to identify this search parameter 1370 * when it is referenced in a specification, model, design or an 1371 * instance; also called its canonical identifier. This SHOULD be 1372 * globally unique and SHOULD be a literal address at which at 1373 * which an authoritative instance of this search parameter is (or 1374 * will be) published. This URL can be the target of a canonical 1375 * reference. It SHALL remain the same when the search parameter is 1376 * stored on different servers. 1377 */ 1378 public SearchParameter setUrl(String value) { 1379 if (this.url == null) 1380 this.url = new UriType(); 1381 this.url.setValue(value); 1382 return this; 1383 } 1384 1385 /** 1386 * @return {@link #version} (The identifier that is used to identify this 1387 * version of the search parameter when it is referenced in a 1388 * specification, model, design or instance. This is an arbitrary value 1389 * managed by the search parameter author and is not expected to be 1390 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 1391 * if a managed version is not available. There is also no expectation 1392 * that versions can be placed in a lexicographical sequence.). This is 1393 * the underlying object with id, value and extensions. The accessor 1394 * "getVersion" gives direct access to the value 1395 */ 1396 public StringType getVersionElement() { 1397 if (this.version == null) 1398 if (Configuration.errorOnAutoCreate()) 1399 throw new Error("Attempt to auto-create SearchParameter.version"); 1400 else if (Configuration.doAutoCreate()) 1401 this.version = new StringType(); // bb 1402 return this.version; 1403 } 1404 1405 public boolean hasVersionElement() { 1406 return this.version != null && !this.version.isEmpty(); 1407 } 1408 1409 public boolean hasVersion() { 1410 return this.version != null && !this.version.isEmpty(); 1411 } 1412 1413 /** 1414 * @param value {@link #version} (The identifier that is used to identify this 1415 * version of the search parameter when it is referenced in a 1416 * specification, model, design or instance. This is an arbitrary 1417 * value managed by the search parameter author and is not expected 1418 * to be globally unique. For example, it might be a timestamp 1419 * (e.g. yyyymmdd) if a managed version is not available. There is 1420 * also no expectation that versions can be placed in a 1421 * lexicographical sequence.). This is the underlying object with 1422 * id, value and extensions. The accessor "getVersion" gives direct 1423 * access to the value 1424 */ 1425 public SearchParameter setVersionElement(StringType value) { 1426 this.version = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return The identifier that is used to identify this version of the search 1432 * parameter when it is referenced in a specification, model, design or 1433 * instance. This is an arbitrary value managed by the search parameter 1434 * author and is not expected to be globally unique. For example, it 1435 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 1436 * available. There is also no expectation that versions can be placed 1437 * in a lexicographical sequence. 1438 */ 1439 public String getVersion() { 1440 return this.version == null ? null : this.version.getValue(); 1441 } 1442 1443 /** 1444 * @param value The identifier that is used to identify this version of the 1445 * search parameter when it is referenced in a specification, 1446 * model, design or instance. This is an arbitrary value managed by 1447 * the search parameter author and is not expected to be globally 1448 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 1449 * a managed version is not available. There is also no expectation 1450 * that versions can be placed in a lexicographical sequence. 1451 */ 1452 public SearchParameter setVersion(String value) { 1453 if (Utilities.noString(value)) 1454 this.version = null; 1455 else { 1456 if (this.version == null) 1457 this.version = new StringType(); 1458 this.version.setValue(value); 1459 } 1460 return this; 1461 } 1462 1463 /** 1464 * @return {@link #name} (A natural language name identifying the search 1465 * parameter. This name should be usable as an identifier for the module 1466 * by machine processing applications such as code generation.). This is 1467 * the underlying object with id, value and extensions. The accessor 1468 * "getName" gives direct access to the value 1469 */ 1470 public StringType getNameElement() { 1471 if (this.name == null) 1472 if (Configuration.errorOnAutoCreate()) 1473 throw new Error("Attempt to auto-create SearchParameter.name"); 1474 else if (Configuration.doAutoCreate()) 1475 this.name = new StringType(); // bb 1476 return this.name; 1477 } 1478 1479 public boolean hasNameElement() { 1480 return this.name != null && !this.name.isEmpty(); 1481 } 1482 1483 public boolean hasName() { 1484 return this.name != null && !this.name.isEmpty(); 1485 } 1486 1487 /** 1488 * @param value {@link #name} (A natural language name identifying the search 1489 * parameter. This name should be usable as an identifier for the 1490 * module by machine processing applications such as code 1491 * generation.). This is the underlying object with id, value and 1492 * extensions. The accessor "getName" gives direct access to the 1493 * value 1494 */ 1495 public SearchParameter setNameElement(StringType value) { 1496 this.name = value; 1497 return this; 1498 } 1499 1500 /** 1501 * @return A natural language name identifying the search parameter. This name 1502 * should be usable as an identifier for the module by machine 1503 * processing applications such as code generation. 1504 */ 1505 public String getName() { 1506 return this.name == null ? null : this.name.getValue(); 1507 } 1508 1509 /** 1510 * @param value A natural language name identifying the search parameter. This 1511 * name should be usable as an identifier for the module by machine 1512 * processing applications such as code generation. 1513 */ 1514 public SearchParameter setName(String value) { 1515 if (this.name == null) 1516 this.name = new StringType(); 1517 this.name.setValue(value); 1518 return this; 1519 } 1520 1521 /** 1522 * @return {@link #derivedFrom} (Where this search parameter is originally 1523 * defined. If a derivedFrom is provided, then the details in the search 1524 * parameter must be consistent with the definition from which it is 1525 * defined. i.e. the parameter should have the same meaning, and 1526 * (usually) the functionality should be a proper subset of the 1527 * underlying search parameter.). This is the underlying object with id, 1528 * value and extensions. The accessor "getDerivedFrom" gives direct 1529 * access to the value 1530 */ 1531 public CanonicalType getDerivedFromElement() { 1532 if (this.derivedFrom == null) 1533 if (Configuration.errorOnAutoCreate()) 1534 throw new Error("Attempt to auto-create SearchParameter.derivedFrom"); 1535 else if (Configuration.doAutoCreate()) 1536 this.derivedFrom = new CanonicalType(); // bb 1537 return this.derivedFrom; 1538 } 1539 1540 public boolean hasDerivedFromElement() { 1541 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 1542 } 1543 1544 public boolean hasDerivedFrom() { 1545 return this.derivedFrom != null && !this.derivedFrom.isEmpty(); 1546 } 1547 1548 /** 1549 * @param value {@link #derivedFrom} (Where this search parameter is originally 1550 * defined. If a derivedFrom is provided, then the details in the 1551 * search parameter must be consistent with the definition from 1552 * which it is defined. i.e. the parameter should have the same 1553 * meaning, and (usually) the functionality should be a proper 1554 * subset of the underlying search parameter.). This is the 1555 * underlying object with id, value and extensions. The accessor 1556 * "getDerivedFrom" gives direct access to the value 1557 */ 1558 public SearchParameter setDerivedFromElement(CanonicalType value) { 1559 this.derivedFrom = value; 1560 return this; 1561 } 1562 1563 /** 1564 * @return Where this search parameter is originally defined. If a derivedFrom 1565 * is provided, then the details in the search parameter must be 1566 * consistent with the definition from which it is defined. i.e. the 1567 * parameter should have the same meaning, and (usually) the 1568 * functionality should be a proper subset of the underlying search 1569 * parameter. 1570 */ 1571 public String getDerivedFrom() { 1572 return this.derivedFrom == null ? null : this.derivedFrom.getValue(); 1573 } 1574 1575 /** 1576 * @param value Where this search parameter is originally defined. If a 1577 * derivedFrom is provided, then the details in the search 1578 * parameter must be consistent with the definition from which it 1579 * is defined. i.e. the parameter should have the same meaning, and 1580 * (usually) the functionality should be a proper subset of the 1581 * underlying search parameter. 1582 */ 1583 public SearchParameter setDerivedFrom(String value) { 1584 if (Utilities.noString(value)) 1585 this.derivedFrom = null; 1586 else { 1587 if (this.derivedFrom == null) 1588 this.derivedFrom = new CanonicalType(); 1589 this.derivedFrom.setValue(value); 1590 } 1591 return this; 1592 } 1593 1594 /** 1595 * @return {@link #status} (The status of this search parameter. Enables 1596 * tracking the life-cycle of the content.). This is the underlying 1597 * object with id, value and extensions. The accessor "getStatus" gives 1598 * direct access to the value 1599 */ 1600 public Enumeration<PublicationStatus> getStatusElement() { 1601 if (this.status == null) 1602 if (Configuration.errorOnAutoCreate()) 1603 throw new Error("Attempt to auto-create SearchParameter.status"); 1604 else if (Configuration.doAutoCreate()) 1605 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1606 return this.status; 1607 } 1608 1609 public boolean hasStatusElement() { 1610 return this.status != null && !this.status.isEmpty(); 1611 } 1612 1613 public boolean hasStatus() { 1614 return this.status != null && !this.status.isEmpty(); 1615 } 1616 1617 /** 1618 * @param value {@link #status} (The status of this search parameter. Enables 1619 * tracking the life-cycle of the content.). This is the underlying 1620 * object with id, value and extensions. The accessor "getStatus" 1621 * gives direct access to the value 1622 */ 1623 public SearchParameter setStatusElement(Enumeration<PublicationStatus> value) { 1624 this.status = value; 1625 return this; 1626 } 1627 1628 /** 1629 * @return The status of this search parameter. Enables tracking the life-cycle 1630 * of the content. 1631 */ 1632 public PublicationStatus getStatus() { 1633 return this.status == null ? null : this.status.getValue(); 1634 } 1635 1636 /** 1637 * @param value The status of this search parameter. Enables tracking the 1638 * life-cycle of the content. 1639 */ 1640 public SearchParameter setStatus(PublicationStatus value) { 1641 if (this.status == null) 1642 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1643 this.status.setValue(value); 1644 return this; 1645 } 1646 1647 /** 1648 * @return {@link #experimental} (A Boolean value to indicate that this search 1649 * parameter is authored for testing purposes (or 1650 * education/evaluation/marketing) and is not intended to be used for 1651 * genuine usage.). This is the underlying object with id, value and 1652 * extensions. The accessor "getExperimental" gives direct access to the 1653 * value 1654 */ 1655 public BooleanType getExperimentalElement() { 1656 if (this.experimental == null) 1657 if (Configuration.errorOnAutoCreate()) 1658 throw new Error("Attempt to auto-create SearchParameter.experimental"); 1659 else if (Configuration.doAutoCreate()) 1660 this.experimental = new BooleanType(); // bb 1661 return this.experimental; 1662 } 1663 1664 public boolean hasExperimentalElement() { 1665 return this.experimental != null && !this.experimental.isEmpty(); 1666 } 1667 1668 public boolean hasExperimental() { 1669 return this.experimental != null && !this.experimental.isEmpty(); 1670 } 1671 1672 /** 1673 * @param value {@link #experimental} (A Boolean value to indicate that this 1674 * search parameter is authored for testing purposes (or 1675 * education/evaluation/marketing) and is not intended to be used 1676 * for genuine usage.). This is the underlying object with id, 1677 * value and extensions. The accessor "getExperimental" gives 1678 * direct access to the value 1679 */ 1680 public SearchParameter setExperimentalElement(BooleanType value) { 1681 this.experimental = value; 1682 return this; 1683 } 1684 1685 /** 1686 * @return A Boolean value to indicate that this search parameter is authored 1687 * for testing purposes (or education/evaluation/marketing) and is not 1688 * intended to be used for genuine usage. 1689 */ 1690 public boolean getExperimental() { 1691 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1692 } 1693 1694 /** 1695 * @param value A Boolean value to indicate that this search parameter is 1696 * authored for testing purposes (or 1697 * education/evaluation/marketing) and is not intended to be used 1698 * for genuine usage. 1699 */ 1700 public SearchParameter setExperimental(boolean value) { 1701 if (this.experimental == null) 1702 this.experimental = new BooleanType(); 1703 this.experimental.setValue(value); 1704 return this; 1705 } 1706 1707 /** 1708 * @return {@link #date} (The date (and optionally time) when the search 1709 * parameter was published. The date must change when the business 1710 * version changes and it must change if the status code changes. In 1711 * addition, it should change when the substantive content of the search 1712 * parameter changes.). This is the underlying object with id, value and 1713 * extensions. The accessor "getDate" gives direct access to the value 1714 */ 1715 public DateTimeType getDateElement() { 1716 if (this.date == null) 1717 if (Configuration.errorOnAutoCreate()) 1718 throw new Error("Attempt to auto-create SearchParameter.date"); 1719 else if (Configuration.doAutoCreate()) 1720 this.date = new DateTimeType(); // bb 1721 return this.date; 1722 } 1723 1724 public boolean hasDateElement() { 1725 return this.date != null && !this.date.isEmpty(); 1726 } 1727 1728 public boolean hasDate() { 1729 return this.date != null && !this.date.isEmpty(); 1730 } 1731 1732 /** 1733 * @param value {@link #date} (The date (and optionally time) when the search 1734 * parameter was published. The date must change when the business 1735 * version changes and it must change if the status code changes. 1736 * In addition, it should change when the substantive content of 1737 * the search parameter changes.). This is the underlying object 1738 * with id, value and extensions. The accessor "getDate" gives 1739 * direct access to the value 1740 */ 1741 public SearchParameter setDateElement(DateTimeType value) { 1742 this.date = value; 1743 return this; 1744 } 1745 1746 /** 1747 * @return The date (and optionally time) when the search parameter was 1748 * published. The date must change when the business version changes and 1749 * it must change if the status code changes. In addition, it should 1750 * change when the substantive content of the search parameter changes. 1751 */ 1752 public Date getDate() { 1753 return this.date == null ? null : this.date.getValue(); 1754 } 1755 1756 /** 1757 * @param value The date (and optionally time) when the search parameter was 1758 * published. The date must change when the business version 1759 * changes and it must change if the status code changes. In 1760 * addition, it should change when the substantive content of the 1761 * search parameter changes. 1762 */ 1763 public SearchParameter setDate(Date value) { 1764 if (value == null) 1765 this.date = null; 1766 else { 1767 if (this.date == null) 1768 this.date = new DateTimeType(); 1769 this.date.setValue(value); 1770 } 1771 return this; 1772 } 1773 1774 /** 1775 * @return {@link #publisher} (The name of the organization or individual that 1776 * published the search parameter.). This is the underlying object with 1777 * id, value and extensions. The accessor "getPublisher" gives direct 1778 * access to the value 1779 */ 1780 public StringType getPublisherElement() { 1781 if (this.publisher == null) 1782 if (Configuration.errorOnAutoCreate()) 1783 throw new Error("Attempt to auto-create SearchParameter.publisher"); 1784 else if (Configuration.doAutoCreate()) 1785 this.publisher = new StringType(); // bb 1786 return this.publisher; 1787 } 1788 1789 public boolean hasPublisherElement() { 1790 return this.publisher != null && !this.publisher.isEmpty(); 1791 } 1792 1793 public boolean hasPublisher() { 1794 return this.publisher != null && !this.publisher.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #publisher} (The name of the organization or individual 1799 * that published the search parameter.). This is the underlying 1800 * object with id, value and extensions. The accessor 1801 * "getPublisher" gives direct access to the value 1802 */ 1803 public SearchParameter setPublisherElement(StringType value) { 1804 this.publisher = value; 1805 return this; 1806 } 1807 1808 /** 1809 * @return The name of the organization or individual that published the search 1810 * parameter. 1811 */ 1812 public String getPublisher() { 1813 return this.publisher == null ? null : this.publisher.getValue(); 1814 } 1815 1816 /** 1817 * @param value The name of the organization or individual that published the 1818 * search parameter. 1819 */ 1820 public SearchParameter setPublisher(String value) { 1821 if (Utilities.noString(value)) 1822 this.publisher = null; 1823 else { 1824 if (this.publisher == null) 1825 this.publisher = new StringType(); 1826 this.publisher.setValue(value); 1827 } 1828 return this; 1829 } 1830 1831 /** 1832 * @return {@link #contact} (Contact details to assist a user in finding and 1833 * communicating with the publisher.) 1834 */ 1835 public List<ContactDetail> getContact() { 1836 if (this.contact == null) 1837 this.contact = new ArrayList<ContactDetail>(); 1838 return this.contact; 1839 } 1840 1841 /** 1842 * @return Returns a reference to <code>this</code> for easy method chaining 1843 */ 1844 public SearchParameter setContact(List<ContactDetail> theContact) { 1845 this.contact = theContact; 1846 return this; 1847 } 1848 1849 public boolean hasContact() { 1850 if (this.contact == null) 1851 return false; 1852 for (ContactDetail item : this.contact) 1853 if (!item.isEmpty()) 1854 return true; 1855 return false; 1856 } 1857 1858 public ContactDetail addContact() { // 3 1859 ContactDetail t = new ContactDetail(); 1860 if (this.contact == null) 1861 this.contact = new ArrayList<ContactDetail>(); 1862 this.contact.add(t); 1863 return t; 1864 } 1865 1866 public SearchParameter addContact(ContactDetail t) { // 3 1867 if (t == null) 1868 return this; 1869 if (this.contact == null) 1870 this.contact = new ArrayList<ContactDetail>(); 1871 this.contact.add(t); 1872 return this; 1873 } 1874 1875 /** 1876 * @return The first repetition of repeating field {@link #contact}, creating it 1877 * if it does not already exist 1878 */ 1879 public ContactDetail getContactFirstRep() { 1880 if (getContact().isEmpty()) { 1881 addContact(); 1882 } 1883 return getContact().get(0); 1884 } 1885 1886 /** 1887 * @return {@link #description} (And how it used.). This is the underlying 1888 * object with id, value and extensions. The accessor "getDescription" 1889 * gives direct access to the value 1890 */ 1891 public MarkdownType getDescriptionElement() { 1892 if (this.description == null) 1893 if (Configuration.errorOnAutoCreate()) 1894 throw new Error("Attempt to auto-create SearchParameter.description"); 1895 else if (Configuration.doAutoCreate()) 1896 this.description = new MarkdownType(); // bb 1897 return this.description; 1898 } 1899 1900 public boolean hasDescriptionElement() { 1901 return this.description != null && !this.description.isEmpty(); 1902 } 1903 1904 public boolean hasDescription() { 1905 return this.description != null && !this.description.isEmpty(); 1906 } 1907 1908 /** 1909 * @param value {@link #description} (And how it used.). This is the underlying 1910 * object with id, value and extensions. The accessor 1911 * "getDescription" gives direct access to the value 1912 */ 1913 public SearchParameter setDescriptionElement(MarkdownType value) { 1914 this.description = value; 1915 return this; 1916 } 1917 1918 /** 1919 * @return And how it used. 1920 */ 1921 public String getDescription() { 1922 return this.description == null ? null : this.description.getValue(); 1923 } 1924 1925 /** 1926 * @param value And how it used. 1927 */ 1928 public SearchParameter setDescription(String value) { 1929 if (this.description == null) 1930 this.description = new MarkdownType(); 1931 this.description.setValue(value); 1932 return this; 1933 } 1934 1935 /** 1936 * @return {@link #useContext} (The content was developed with a focus and 1937 * intent of supporting the contexts that are listed. These contexts may 1938 * be general categories (gender, age, ...) or may be references to 1939 * specific programs (insurance plans, studies, ...) and may be used to 1940 * assist with indexing and searching for appropriate search parameter 1941 * instances.) 1942 */ 1943 public List<UsageContext> getUseContext() { 1944 if (this.useContext == null) 1945 this.useContext = new ArrayList<UsageContext>(); 1946 return this.useContext; 1947 } 1948 1949 /** 1950 * @return Returns a reference to <code>this</code> for easy method chaining 1951 */ 1952 public SearchParameter setUseContext(List<UsageContext> theUseContext) { 1953 this.useContext = theUseContext; 1954 return this; 1955 } 1956 1957 public boolean hasUseContext() { 1958 if (this.useContext == null) 1959 return false; 1960 for (UsageContext item : this.useContext) 1961 if (!item.isEmpty()) 1962 return true; 1963 return false; 1964 } 1965 1966 public UsageContext addUseContext() { // 3 1967 UsageContext t = new UsageContext(); 1968 if (this.useContext == null) 1969 this.useContext = new ArrayList<UsageContext>(); 1970 this.useContext.add(t); 1971 return t; 1972 } 1973 1974 public SearchParameter addUseContext(UsageContext t) { // 3 1975 if (t == null) 1976 return this; 1977 if (this.useContext == null) 1978 this.useContext = new ArrayList<UsageContext>(); 1979 this.useContext.add(t); 1980 return this; 1981 } 1982 1983 /** 1984 * @return The first repetition of repeating field {@link #useContext}, creating 1985 * it if it does not already exist 1986 */ 1987 public UsageContext getUseContextFirstRep() { 1988 if (getUseContext().isEmpty()) { 1989 addUseContext(); 1990 } 1991 return getUseContext().get(0); 1992 } 1993 1994 /** 1995 * @return {@link #jurisdiction} (A legal or geographic region in which the 1996 * search parameter is intended to be used.) 1997 */ 1998 public List<CodeableConcept> getJurisdiction() { 1999 if (this.jurisdiction == null) 2000 this.jurisdiction = new ArrayList<CodeableConcept>(); 2001 return this.jurisdiction; 2002 } 2003 2004 /** 2005 * @return Returns a reference to <code>this</code> for easy method chaining 2006 */ 2007 public SearchParameter setJurisdiction(List<CodeableConcept> theJurisdiction) { 2008 this.jurisdiction = theJurisdiction; 2009 return this; 2010 } 2011 2012 public boolean hasJurisdiction() { 2013 if (this.jurisdiction == null) 2014 return false; 2015 for (CodeableConcept item : this.jurisdiction) 2016 if (!item.isEmpty()) 2017 return true; 2018 return false; 2019 } 2020 2021 public CodeableConcept addJurisdiction() { // 3 2022 CodeableConcept t = new CodeableConcept(); 2023 if (this.jurisdiction == null) 2024 this.jurisdiction = new ArrayList<CodeableConcept>(); 2025 this.jurisdiction.add(t); 2026 return t; 2027 } 2028 2029 public SearchParameter addJurisdiction(CodeableConcept t) { // 3 2030 if (t == null) 2031 return this; 2032 if (this.jurisdiction == null) 2033 this.jurisdiction = new ArrayList<CodeableConcept>(); 2034 this.jurisdiction.add(t); 2035 return this; 2036 } 2037 2038 /** 2039 * @return The first repetition of repeating field {@link #jurisdiction}, 2040 * creating it if it does not already exist 2041 */ 2042 public CodeableConcept getJurisdictionFirstRep() { 2043 if (getJurisdiction().isEmpty()) { 2044 addJurisdiction(); 2045 } 2046 return getJurisdiction().get(0); 2047 } 2048 2049 /** 2050 * @return {@link #purpose} (Explanation of why this search parameter is needed 2051 * and why it has been designed as it has.). This is the underlying 2052 * object with id, value and extensions. The accessor "getPurpose" gives 2053 * direct access to the value 2054 */ 2055 public MarkdownType getPurposeElement() { 2056 if (this.purpose == null) 2057 if (Configuration.errorOnAutoCreate()) 2058 throw new Error("Attempt to auto-create SearchParameter.purpose"); 2059 else if (Configuration.doAutoCreate()) 2060 this.purpose = new MarkdownType(); // bb 2061 return this.purpose; 2062 } 2063 2064 public boolean hasPurposeElement() { 2065 return this.purpose != null && !this.purpose.isEmpty(); 2066 } 2067 2068 public boolean hasPurpose() { 2069 return this.purpose != null && !this.purpose.isEmpty(); 2070 } 2071 2072 /** 2073 * @param value {@link #purpose} (Explanation of why this search parameter is 2074 * needed and why it has been designed as it has.). This is the 2075 * underlying object with id, value and extensions. The accessor 2076 * "getPurpose" gives direct access to the value 2077 */ 2078 public SearchParameter setPurposeElement(MarkdownType value) { 2079 this.purpose = value; 2080 return this; 2081 } 2082 2083 /** 2084 * @return Explanation of why this search parameter is needed and why it has 2085 * been designed as it has. 2086 */ 2087 public String getPurpose() { 2088 return this.purpose == null ? null : this.purpose.getValue(); 2089 } 2090 2091 /** 2092 * @param value Explanation of why this search parameter is needed and why it 2093 * has been designed as it has. 2094 */ 2095 public SearchParameter setPurpose(String value) { 2096 if (value == null) 2097 this.purpose = null; 2098 else { 2099 if (this.purpose == null) 2100 this.purpose = new MarkdownType(); 2101 this.purpose.setValue(value); 2102 } 2103 return this; 2104 } 2105 2106 /** 2107 * @return {@link #code} (The code used in the URL or the parameter name in a 2108 * parameters resource for this search parameter.). This is the 2109 * underlying object with id, value and extensions. The accessor 2110 * "getCode" gives direct access to the value 2111 */ 2112 public CodeType getCodeElement() { 2113 if (this.code == null) 2114 if (Configuration.errorOnAutoCreate()) 2115 throw new Error("Attempt to auto-create SearchParameter.code"); 2116 else if (Configuration.doAutoCreate()) 2117 this.code = new CodeType(); // bb 2118 return this.code; 2119 } 2120 2121 public boolean hasCodeElement() { 2122 return this.code != null && !this.code.isEmpty(); 2123 } 2124 2125 public boolean hasCode() { 2126 return this.code != null && !this.code.isEmpty(); 2127 } 2128 2129 /** 2130 * @param value {@link #code} (The code used in the URL or the parameter name in 2131 * a parameters resource for this search parameter.). This is the 2132 * underlying object with id, value and extensions. The accessor 2133 * "getCode" gives direct access to the value 2134 */ 2135 public SearchParameter setCodeElement(CodeType value) { 2136 this.code = value; 2137 return this; 2138 } 2139 2140 /** 2141 * @return The code used in the URL or the parameter name in a parameters 2142 * resource for this search parameter. 2143 */ 2144 public String getCode() { 2145 return this.code == null ? null : this.code.getValue(); 2146 } 2147 2148 /** 2149 * @param value The code used in the URL or the parameter name in a parameters 2150 * resource for this search parameter. 2151 */ 2152 public SearchParameter setCode(String value) { 2153 if (this.code == null) 2154 this.code = new CodeType(); 2155 this.code.setValue(value); 2156 return this; 2157 } 2158 2159 /** 2160 * @return {@link #base} (The base resource type(s) that this search parameter 2161 * can be used against.) 2162 */ 2163 public List<CodeType> getBase() { 2164 if (this.base == null) 2165 this.base = new ArrayList<CodeType>(); 2166 return this.base; 2167 } 2168 2169 /** 2170 * @return Returns a reference to <code>this</code> for easy method chaining 2171 */ 2172 public SearchParameter setBase(List<CodeType> theBase) { 2173 this.base = theBase; 2174 return this; 2175 } 2176 2177 public boolean hasBase() { 2178 if (this.base == null) 2179 return false; 2180 for (CodeType item : this.base) 2181 if (!item.isEmpty()) 2182 return true; 2183 return false; 2184 } 2185 2186 /** 2187 * @return {@link #base} (The base resource type(s) that this search parameter 2188 * can be used against.) 2189 */ 2190 public CodeType addBaseElement() {// 2 2191 CodeType t = new CodeType(); 2192 if (this.base == null) 2193 this.base = new ArrayList<CodeType>(); 2194 this.base.add(t); 2195 return t; 2196 } 2197 2198 /** 2199 * @param value {@link #base} (The base resource type(s) that this search 2200 * parameter can be used against.) 2201 */ 2202 public SearchParameter addBase(String value) { // 1 2203 CodeType t = new CodeType(); 2204 t.setValue(value); 2205 if (this.base == null) 2206 this.base = new ArrayList<CodeType>(); 2207 this.base.add(t); 2208 return this; 2209 } 2210 2211 /** 2212 * @param value {@link #base} (The base resource type(s) that this search 2213 * parameter can be used against.) 2214 */ 2215 public boolean hasBase(String value) { 2216 if (this.base == null) 2217 return false; 2218 for (CodeType v : this.base) 2219 if (v.getValue().equals(value)) // code 2220 return true; 2221 return false; 2222 } 2223 2224 /** 2225 * @return {@link #type} (The type of value that a search parameter may contain, 2226 * and how the content is interpreted.). This is the underlying object 2227 * with id, value and extensions. The accessor "getType" gives direct 2228 * access to the value 2229 */ 2230 public Enumeration<SearchParamType> getTypeElement() { 2231 if (this.type == null) 2232 if (Configuration.errorOnAutoCreate()) 2233 throw new Error("Attempt to auto-create SearchParameter.type"); 2234 else if (Configuration.doAutoCreate()) 2235 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 2236 return this.type; 2237 } 2238 2239 public boolean hasTypeElement() { 2240 return this.type != null && !this.type.isEmpty(); 2241 } 2242 2243 public boolean hasType() { 2244 return this.type != null && !this.type.isEmpty(); 2245 } 2246 2247 /** 2248 * @param value {@link #type} (The type of value that a search parameter may 2249 * contain, and how the content is interpreted.). This is the 2250 * underlying object with id, value and extensions. The accessor 2251 * "getType" gives direct access to the value 2252 */ 2253 public SearchParameter setTypeElement(Enumeration<SearchParamType> value) { 2254 this.type = value; 2255 return this; 2256 } 2257 2258 /** 2259 * @return The type of value that a search parameter may contain, and how the 2260 * content is interpreted. 2261 */ 2262 public SearchParamType getType() { 2263 return this.type == null ? null : this.type.getValue(); 2264 } 2265 2266 /** 2267 * @param value The type of value that a search parameter may contain, and how 2268 * the content is interpreted. 2269 */ 2270 public SearchParameter setType(SearchParamType value) { 2271 if (this.type == null) 2272 this.type = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 2273 this.type.setValue(value); 2274 return this; 2275 } 2276 2277 /** 2278 * @return {@link #expression} (A FHIRPath expression that returns a set of 2279 * elements for the search parameter.). This is the underlying object 2280 * with id, value and extensions. The accessor "getExpression" gives 2281 * direct access to the value 2282 */ 2283 public StringType getExpressionElement() { 2284 if (this.expression == null) 2285 if (Configuration.errorOnAutoCreate()) 2286 throw new Error("Attempt to auto-create SearchParameter.expression"); 2287 else if (Configuration.doAutoCreate()) 2288 this.expression = new StringType(); // bb 2289 return this.expression; 2290 } 2291 2292 public boolean hasExpressionElement() { 2293 return this.expression != null && !this.expression.isEmpty(); 2294 } 2295 2296 public boolean hasExpression() { 2297 return this.expression != null && !this.expression.isEmpty(); 2298 } 2299 2300 /** 2301 * @param value {@link #expression} (A FHIRPath expression that returns a set of 2302 * elements for the search parameter.). This is the underlying 2303 * object with id, value and extensions. The accessor 2304 * "getExpression" gives direct access to the value 2305 */ 2306 public SearchParameter setExpressionElement(StringType value) { 2307 this.expression = value; 2308 return this; 2309 } 2310 2311 /** 2312 * @return A FHIRPath expression that returns a set of elements for the search 2313 * parameter. 2314 */ 2315 public String getExpression() { 2316 return this.expression == null ? null : this.expression.getValue(); 2317 } 2318 2319 /** 2320 * @param value A FHIRPath expression that returns a set of elements for the 2321 * search parameter. 2322 */ 2323 public SearchParameter setExpression(String value) { 2324 if (Utilities.noString(value)) 2325 this.expression = null; 2326 else { 2327 if (this.expression == null) 2328 this.expression = new StringType(); 2329 this.expression.setValue(value); 2330 } 2331 return this; 2332 } 2333 2334 /** 2335 * @return {@link #xpath} (An XPath expression that returns a set of elements 2336 * for the search parameter.). This is the underlying object with id, 2337 * value and extensions. The accessor "getXpath" gives direct access to 2338 * the value 2339 */ 2340 public StringType getXpathElement() { 2341 if (this.xpath == null) 2342 if (Configuration.errorOnAutoCreate()) 2343 throw new Error("Attempt to auto-create SearchParameter.xpath"); 2344 else if (Configuration.doAutoCreate()) 2345 this.xpath = new StringType(); // bb 2346 return this.xpath; 2347 } 2348 2349 public boolean hasXpathElement() { 2350 return this.xpath != null && !this.xpath.isEmpty(); 2351 } 2352 2353 public boolean hasXpath() { 2354 return this.xpath != null && !this.xpath.isEmpty(); 2355 } 2356 2357 /** 2358 * @param value {@link #xpath} (An XPath expression that returns a set of 2359 * elements for the search parameter.). This is the underlying 2360 * object with id, value and extensions. The accessor "getXpath" 2361 * gives direct access to the value 2362 */ 2363 public SearchParameter setXpathElement(StringType value) { 2364 this.xpath = value; 2365 return this; 2366 } 2367 2368 /** 2369 * @return An XPath expression that returns a set of elements for the search 2370 * parameter. 2371 */ 2372 public String getXpath() { 2373 return this.xpath == null ? null : this.xpath.getValue(); 2374 } 2375 2376 /** 2377 * @param value An XPath expression that returns a set of elements for the 2378 * search parameter. 2379 */ 2380 public SearchParameter setXpath(String value) { 2381 if (Utilities.noString(value)) 2382 this.xpath = null; 2383 else { 2384 if (this.xpath == null) 2385 this.xpath = new StringType(); 2386 this.xpath.setValue(value); 2387 } 2388 return this; 2389 } 2390 2391 /** 2392 * @return {@link #xpathUsage} (How the search parameter relates to the set of 2393 * elements returned by evaluating the xpath query.). This is the 2394 * underlying object with id, value and extensions. The accessor 2395 * "getXpathUsage" gives direct access to the value 2396 */ 2397 public Enumeration<XPathUsageType> getXpathUsageElement() { 2398 if (this.xpathUsage == null) 2399 if (Configuration.errorOnAutoCreate()) 2400 throw new Error("Attempt to auto-create SearchParameter.xpathUsage"); 2401 else if (Configuration.doAutoCreate()) 2402 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); // bb 2403 return this.xpathUsage; 2404 } 2405 2406 public boolean hasXpathUsageElement() { 2407 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 2408 } 2409 2410 public boolean hasXpathUsage() { 2411 return this.xpathUsage != null && !this.xpathUsage.isEmpty(); 2412 } 2413 2414 /** 2415 * @param value {@link #xpathUsage} (How the search parameter relates to the set 2416 * of elements returned by evaluating the xpath query.). This is 2417 * the underlying object with id, value and extensions. The 2418 * accessor "getXpathUsage" gives direct access to the value 2419 */ 2420 public SearchParameter setXpathUsageElement(Enumeration<XPathUsageType> value) { 2421 this.xpathUsage = value; 2422 return this; 2423 } 2424 2425 /** 2426 * @return How the search parameter relates to the set of elements returned by 2427 * evaluating the xpath query. 2428 */ 2429 public XPathUsageType getXpathUsage() { 2430 return this.xpathUsage == null ? null : this.xpathUsage.getValue(); 2431 } 2432 2433 /** 2434 * @param value How the search parameter relates to the set of elements returned 2435 * by evaluating the xpath query. 2436 */ 2437 public SearchParameter setXpathUsage(XPathUsageType value) { 2438 if (value == null) 2439 this.xpathUsage = null; 2440 else { 2441 if (this.xpathUsage == null) 2442 this.xpathUsage = new Enumeration<XPathUsageType>(new XPathUsageTypeEnumFactory()); 2443 this.xpathUsage.setValue(value); 2444 } 2445 return this; 2446 } 2447 2448 /** 2449 * @return {@link #target} (Types of resource (if a resource is referenced).) 2450 */ 2451 public List<CodeType> getTarget() { 2452 if (this.target == null) 2453 this.target = new ArrayList<CodeType>(); 2454 return this.target; 2455 } 2456 2457 /** 2458 * @return Returns a reference to <code>this</code> for easy method chaining 2459 */ 2460 public SearchParameter setTarget(List<CodeType> theTarget) { 2461 this.target = theTarget; 2462 return this; 2463 } 2464 2465 public boolean hasTarget() { 2466 if (this.target == null) 2467 return false; 2468 for (CodeType item : this.target) 2469 if (!item.isEmpty()) 2470 return true; 2471 return false; 2472 } 2473 2474 /** 2475 * @return {@link #target} (Types of resource (if a resource is referenced).) 2476 */ 2477 public CodeType addTargetElement() {// 2 2478 CodeType t = new CodeType(); 2479 if (this.target == null) 2480 this.target = new ArrayList<CodeType>(); 2481 this.target.add(t); 2482 return t; 2483 } 2484 2485 /** 2486 * @param value {@link #target} (Types of resource (if a resource is 2487 * referenced).) 2488 */ 2489 public SearchParameter addTarget(String value) { // 1 2490 CodeType t = new CodeType(); 2491 t.setValue(value); 2492 if (this.target == null) 2493 this.target = new ArrayList<CodeType>(); 2494 this.target.add(t); 2495 return this; 2496 } 2497 2498 /** 2499 * @param value {@link #target} (Types of resource (if a resource is 2500 * referenced).) 2501 */ 2502 public boolean hasTarget(String value) { 2503 if (this.target == null) 2504 return false; 2505 for (CodeType v : this.target) 2506 if (v.getValue().equals(value)) // code 2507 return true; 2508 return false; 2509 } 2510 2511 /** 2512 * @return {@link #multipleOr} (Whether multiple values are allowed for each 2513 * time the parameter exists. Values are separated by commas, and the 2514 * parameter matches if any of the values match.). This is the 2515 * underlying object with id, value and extensions. The accessor 2516 * "getMultipleOr" gives direct access to the value 2517 */ 2518 public BooleanType getMultipleOrElement() { 2519 if (this.multipleOr == null) 2520 if (Configuration.errorOnAutoCreate()) 2521 throw new Error("Attempt to auto-create SearchParameter.multipleOr"); 2522 else if (Configuration.doAutoCreate()) 2523 this.multipleOr = new BooleanType(); // bb 2524 return this.multipleOr; 2525 } 2526 2527 public boolean hasMultipleOrElement() { 2528 return this.multipleOr != null && !this.multipleOr.isEmpty(); 2529 } 2530 2531 public boolean hasMultipleOr() { 2532 return this.multipleOr != null && !this.multipleOr.isEmpty(); 2533 } 2534 2535 /** 2536 * @param value {@link #multipleOr} (Whether multiple values are allowed for 2537 * each time the parameter exists. Values are separated by commas, 2538 * and the parameter matches if any of the values match.). This is 2539 * the underlying object with id, value and extensions. The 2540 * accessor "getMultipleOr" gives direct access to the value 2541 */ 2542 public SearchParameter setMultipleOrElement(BooleanType value) { 2543 this.multipleOr = value; 2544 return this; 2545 } 2546 2547 /** 2548 * @return Whether multiple values are allowed for each time the parameter 2549 * exists. Values are separated by commas, and the parameter matches if 2550 * any of the values match. 2551 */ 2552 public boolean getMultipleOr() { 2553 return this.multipleOr == null || this.multipleOr.isEmpty() ? false : this.multipleOr.getValue(); 2554 } 2555 2556 /** 2557 * @param value Whether multiple values are allowed for each time the parameter 2558 * exists. Values are separated by commas, and the parameter 2559 * matches if any of the values match. 2560 */ 2561 public SearchParameter setMultipleOr(boolean value) { 2562 if (this.multipleOr == null) 2563 this.multipleOr = new BooleanType(); 2564 this.multipleOr.setValue(value); 2565 return this; 2566 } 2567 2568 /** 2569 * @return {@link #multipleAnd} (Whether multiple parameters are allowed - e.g. 2570 * more than one parameter with the same name. The search matches if all 2571 * the parameters match.). This is the underlying object with id, value 2572 * and extensions. The accessor "getMultipleAnd" gives direct access to 2573 * the value 2574 */ 2575 public BooleanType getMultipleAndElement() { 2576 if (this.multipleAnd == null) 2577 if (Configuration.errorOnAutoCreate()) 2578 throw new Error("Attempt to auto-create SearchParameter.multipleAnd"); 2579 else if (Configuration.doAutoCreate()) 2580 this.multipleAnd = new BooleanType(); // bb 2581 return this.multipleAnd; 2582 } 2583 2584 public boolean hasMultipleAndElement() { 2585 return this.multipleAnd != null && !this.multipleAnd.isEmpty(); 2586 } 2587 2588 public boolean hasMultipleAnd() { 2589 return this.multipleAnd != null && !this.multipleAnd.isEmpty(); 2590 } 2591 2592 /** 2593 * @param value {@link #multipleAnd} (Whether multiple parameters are allowed - 2594 * e.g. more than one parameter with the same name. The search 2595 * matches if all the parameters match.). This is the underlying 2596 * object with id, value and extensions. The accessor 2597 * "getMultipleAnd" gives direct access to the value 2598 */ 2599 public SearchParameter setMultipleAndElement(BooleanType value) { 2600 this.multipleAnd = value; 2601 return this; 2602 } 2603 2604 /** 2605 * @return Whether multiple parameters are allowed - e.g. more than one 2606 * parameter with the same name. The search matches if all the 2607 * parameters match. 2608 */ 2609 public boolean getMultipleAnd() { 2610 return this.multipleAnd == null || this.multipleAnd.isEmpty() ? false : this.multipleAnd.getValue(); 2611 } 2612 2613 /** 2614 * @param value Whether multiple parameters are allowed - e.g. more than one 2615 * parameter with the same name. The search matches if all the 2616 * parameters match. 2617 */ 2618 public SearchParameter setMultipleAnd(boolean value) { 2619 if (this.multipleAnd == null) 2620 this.multipleAnd = new BooleanType(); 2621 this.multipleAnd.setValue(value); 2622 return this; 2623 } 2624 2625 /** 2626 * @return {@link #comparator} (Comparators supported for the search parameter.) 2627 */ 2628 public List<Enumeration<SearchComparator>> getComparator() { 2629 if (this.comparator == null) 2630 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2631 return this.comparator; 2632 } 2633 2634 /** 2635 * @return Returns a reference to <code>this</code> for easy method chaining 2636 */ 2637 public SearchParameter setComparator(List<Enumeration<SearchComparator>> theComparator) { 2638 this.comparator = theComparator; 2639 return this; 2640 } 2641 2642 public boolean hasComparator() { 2643 if (this.comparator == null) 2644 return false; 2645 for (Enumeration<SearchComparator> item : this.comparator) 2646 if (!item.isEmpty()) 2647 return true; 2648 return false; 2649 } 2650 2651 /** 2652 * @return {@link #comparator} (Comparators supported for the search parameter.) 2653 */ 2654 public Enumeration<SearchComparator> addComparatorElement() {// 2 2655 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2656 if (this.comparator == null) 2657 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2658 this.comparator.add(t); 2659 return t; 2660 } 2661 2662 /** 2663 * @param value {@link #comparator} (Comparators supported for the search 2664 * parameter.) 2665 */ 2666 public SearchParameter addComparator(SearchComparator value) { // 1 2667 Enumeration<SearchComparator> t = new Enumeration<SearchComparator>(new SearchComparatorEnumFactory()); 2668 t.setValue(value); 2669 if (this.comparator == null) 2670 this.comparator = new ArrayList<Enumeration<SearchComparator>>(); 2671 this.comparator.add(t); 2672 return this; 2673 } 2674 2675 /** 2676 * @param value {@link #comparator} (Comparators supported for the search 2677 * parameter.) 2678 */ 2679 public boolean hasComparator(SearchComparator value) { 2680 if (this.comparator == null) 2681 return false; 2682 for (Enumeration<SearchComparator> v : this.comparator) 2683 if (v.getValue().equals(value)) // code 2684 return true; 2685 return false; 2686 } 2687 2688 /** 2689 * @return {@link #modifier} (A modifier supported for the search parameter.) 2690 */ 2691 public List<Enumeration<SearchModifierCode>> getModifier() { 2692 if (this.modifier == null) 2693 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2694 return this.modifier; 2695 } 2696 2697 /** 2698 * @return Returns a reference to <code>this</code> for easy method chaining 2699 */ 2700 public SearchParameter setModifier(List<Enumeration<SearchModifierCode>> theModifier) { 2701 this.modifier = theModifier; 2702 return this; 2703 } 2704 2705 public boolean hasModifier() { 2706 if (this.modifier == null) 2707 return false; 2708 for (Enumeration<SearchModifierCode> item : this.modifier) 2709 if (!item.isEmpty()) 2710 return true; 2711 return false; 2712 } 2713 2714 /** 2715 * @return {@link #modifier} (A modifier supported for the search parameter.) 2716 */ 2717 public Enumeration<SearchModifierCode> addModifierElement() {// 2 2718 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2719 if (this.modifier == null) 2720 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2721 this.modifier.add(t); 2722 return t; 2723 } 2724 2725 /** 2726 * @param value {@link #modifier} (A modifier supported for the search 2727 * parameter.) 2728 */ 2729 public SearchParameter addModifier(SearchModifierCode value) { // 1 2730 Enumeration<SearchModifierCode> t = new Enumeration<SearchModifierCode>(new SearchModifierCodeEnumFactory()); 2731 t.setValue(value); 2732 if (this.modifier == null) 2733 this.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 2734 this.modifier.add(t); 2735 return this; 2736 } 2737 2738 /** 2739 * @param value {@link #modifier} (A modifier supported for the search 2740 * parameter.) 2741 */ 2742 public boolean hasModifier(SearchModifierCode value) { 2743 if (this.modifier == null) 2744 return false; 2745 for (Enumeration<SearchModifierCode> v : this.modifier) 2746 if (v.getValue().equals(value)) // code 2747 return true; 2748 return false; 2749 } 2750 2751 /** 2752 * @return {@link #chain} (Contains the names of any search parameters which may 2753 * be chained to the containing search parameter. Chained parameters may 2754 * be added to search parameters of type reference and specify that 2755 * resources will only be returned if they contain a reference to a 2756 * resource which matches the chained parameter value. Values for this 2757 * field should be drawn from SearchParameter.code for a parameter on 2758 * the target resource type.) 2759 */ 2760 public List<StringType> getChain() { 2761 if (this.chain == null) 2762 this.chain = new ArrayList<StringType>(); 2763 return this.chain; 2764 } 2765 2766 /** 2767 * @return Returns a reference to <code>this</code> for easy method chaining 2768 */ 2769 public SearchParameter setChain(List<StringType> theChain) { 2770 this.chain = theChain; 2771 return this; 2772 } 2773 2774 public boolean hasChain() { 2775 if (this.chain == null) 2776 return false; 2777 for (StringType item : this.chain) 2778 if (!item.isEmpty()) 2779 return true; 2780 return false; 2781 } 2782 2783 /** 2784 * @return {@link #chain} (Contains the names of any search parameters which may 2785 * be chained to the containing search parameter. Chained parameters may 2786 * be added to search parameters of type reference and specify that 2787 * resources will only be returned if they contain a reference to a 2788 * resource which matches the chained parameter value. Values for this 2789 * field should be drawn from SearchParameter.code for a parameter on 2790 * the target resource type.) 2791 */ 2792 public StringType addChainElement() {// 2 2793 StringType t = new StringType(); 2794 if (this.chain == null) 2795 this.chain = new ArrayList<StringType>(); 2796 this.chain.add(t); 2797 return t; 2798 } 2799 2800 /** 2801 * @param value {@link #chain} (Contains the names of any search parameters 2802 * which may be chained to the containing search parameter. Chained 2803 * parameters may be added to search parameters of type reference 2804 * and specify that resources will only be returned if they contain 2805 * a reference to a resource which matches the chained parameter 2806 * value. Values for this field should be drawn from 2807 * SearchParameter.code for a parameter on the target resource 2808 * type.) 2809 */ 2810 public SearchParameter addChain(String value) { // 1 2811 StringType t = new StringType(); 2812 t.setValue(value); 2813 if (this.chain == null) 2814 this.chain = new ArrayList<StringType>(); 2815 this.chain.add(t); 2816 return this; 2817 } 2818 2819 /** 2820 * @param value {@link #chain} (Contains the names of any search parameters 2821 * which may be chained to the containing search parameter. Chained 2822 * parameters may be added to search parameters of type reference 2823 * and specify that resources will only be returned if they contain 2824 * a reference to a resource which matches the chained parameter 2825 * value. Values for this field should be drawn from 2826 * SearchParameter.code for a parameter on the target resource 2827 * type.) 2828 */ 2829 public boolean hasChain(String value) { 2830 if (this.chain == null) 2831 return false; 2832 for (StringType v : this.chain) 2833 if (v.getValue().equals(value)) // string 2834 return true; 2835 return false; 2836 } 2837 2838 /** 2839 * @return {@link #component} (Used to define the parts of a composite search 2840 * parameter.) 2841 */ 2842 public List<SearchParameterComponentComponent> getComponent() { 2843 if (this.component == null) 2844 this.component = new ArrayList<SearchParameterComponentComponent>(); 2845 return this.component; 2846 } 2847 2848 /** 2849 * @return Returns a reference to <code>this</code> for easy method chaining 2850 */ 2851 public SearchParameter setComponent(List<SearchParameterComponentComponent> theComponent) { 2852 this.component = theComponent; 2853 return this; 2854 } 2855 2856 public boolean hasComponent() { 2857 if (this.component == null) 2858 return false; 2859 for (SearchParameterComponentComponent item : this.component) 2860 if (!item.isEmpty()) 2861 return true; 2862 return false; 2863 } 2864 2865 public SearchParameterComponentComponent addComponent() { // 3 2866 SearchParameterComponentComponent t = new SearchParameterComponentComponent(); 2867 if (this.component == null) 2868 this.component = new ArrayList<SearchParameterComponentComponent>(); 2869 this.component.add(t); 2870 return t; 2871 } 2872 2873 public SearchParameter addComponent(SearchParameterComponentComponent t) { // 3 2874 if (t == null) 2875 return this; 2876 if (this.component == null) 2877 this.component = new ArrayList<SearchParameterComponentComponent>(); 2878 this.component.add(t); 2879 return this; 2880 } 2881 2882 /** 2883 * @return The first repetition of repeating field {@link #component}, creating 2884 * it if it does not already exist 2885 */ 2886 public SearchParameterComponentComponent getComponentFirstRep() { 2887 if (getComponent().isEmpty()) { 2888 addComponent(); 2889 } 2890 return getComponent().get(0); 2891 } 2892 2893 protected void listChildren(List<Property> children) { 2894 super.listChildren(children); 2895 children.add(new Property("url", "uri", 2896 "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.", 2897 0, 1, url)); 2898 children.add(new Property("version", "string", 2899 "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 2900 0, 1, version)); 2901 children.add(new Property("name", "string", 2902 "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2903 0, 1, name)); 2904 children.add(new Property("derivedFrom", "canonical(SearchParameter)", 2905 "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 2906 0, 1, derivedFrom)); 2907 children.add(new Property("status", "code", 2908 "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status)); 2909 children.add(new Property("experimental", "boolean", 2910 "A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2911 0, 1, experimental)); 2912 children.add(new Property("date", "dateTime", 2913 "The date (and optionally time) when the search parameter was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 2914 0, 1, date)); 2915 children.add(new Property("publisher", "string", 2916 "The name of the organization or individual that published the search parameter.", 0, 1, publisher)); 2917 children.add(new Property("contact", "ContactDetail", 2918 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2919 java.lang.Integer.MAX_VALUE, contact)); 2920 children.add(new Property("description", "markdown", "And how it used.", 0, 1, description)); 2921 children.add(new Property("useContext", "UsageContext", 2922 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.", 2923 0, java.lang.Integer.MAX_VALUE, useContext)); 2924 children.add(new Property("jurisdiction", "CodeableConcept", 2925 "A legal or geographic region in which the search parameter is intended to be used.", 0, 2926 java.lang.Integer.MAX_VALUE, jurisdiction)); 2927 children.add(new Property("purpose", "markdown", 2928 "Explanation of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose)); 2929 children.add(new Property("code", "code", 2930 "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1, 2931 code)); 2932 children 2933 .add(new Property("base", "code", "The base resource type(s) that this search parameter can be used against.", 2934 0, java.lang.Integer.MAX_VALUE, base)); 2935 children.add(new Property("type", "code", 2936 "The type of value that a search parameter may contain, and how the content is interpreted.", 0, 1, type)); 2937 children.add(new Property("expression", "string", 2938 "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression)); 2939 children.add(new Property("xpath", "string", 2940 "An XPath expression that returns a set of elements for the search parameter.", 0, 1, xpath)); 2941 children.add(new Property("xpathUsage", "code", 2942 "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1, 2943 xpathUsage)); 2944 children.add(new Property("target", "code", "Types of resource (if a resource is referenced).", 0, 2945 java.lang.Integer.MAX_VALUE, target)); 2946 children.add(new Property("multipleOr", "boolean", 2947 "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.", 2948 0, 1, multipleOr)); 2949 children.add(new Property("multipleAnd", "boolean", 2950 "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.", 2951 0, 1, multipleAnd)); 2952 children.add(new Property("comparator", "code", "Comparators supported for the search parameter.", 0, 2953 java.lang.Integer.MAX_VALUE, comparator)); 2954 children.add(new Property("modifier", "code", "A modifier supported for the search parameter.", 0, 2955 java.lang.Integer.MAX_VALUE, modifier)); 2956 children.add(new Property("chain", "string", 2957 "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 2958 0, java.lang.Integer.MAX_VALUE, chain)); 2959 children.add(new Property("component", "", "Used to define the parts of a composite search parameter.", 0, 2960 java.lang.Integer.MAX_VALUE, component)); 2961 } 2962 2963 @Override 2964 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2965 switch (_hash) { 2966 case 116079: 2967 /* url */ return new Property("url", "uri", 2968 "An absolute URI that is used to identify this search parameter when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this search parameter is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the search parameter is stored on different servers.", 2969 0, 1, url); 2970 case 351608024: 2971 /* version */ return new Property("version", "string", 2972 "The identifier that is used to identify this version of the search parameter when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the search parameter author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 2973 0, 1, version); 2974 case 3373707: 2975 /* name */ return new Property("name", "string", 2976 "A natural language name identifying the search parameter. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2977 0, 1, name); 2978 case 1077922663: 2979 /* derivedFrom */ return new Property("derivedFrom", "canonical(SearchParameter)", 2980 "Where this search parameter is originally defined. If a derivedFrom is provided, then the details in the search parameter must be consistent with the definition from which it is defined. i.e. the parameter should have the same meaning, and (usually) the functionality should be a proper subset of the underlying search parameter.", 2981 0, 1, derivedFrom); 2982 case -892481550: 2983 /* status */ return new Property("status", "code", 2984 "The status of this search parameter. Enables tracking the life-cycle of the content.", 0, 1, status); 2985 case -404562712: 2986 /* experimental */ return new Property("experimental", "boolean", 2987 "A Boolean value to indicate that this search parameter is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2988 0, 1, experimental); 2989 case 3076014: 2990 /* date */ return new Property("date", "dateTime", 2991 "The date (and optionally time) when the search parameter was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the search parameter changes.", 2992 0, 1, date); 2993 case 1447404028: 2994 /* publisher */ return new Property("publisher", "string", 2995 "The name of the organization or individual that published the search parameter.", 0, 1, publisher); 2996 case 951526432: 2997 /* contact */ return new Property("contact", "ContactDetail", 2998 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2999 java.lang.Integer.MAX_VALUE, contact); 3000 case -1724546052: 3001 /* description */ return new Property("description", "markdown", "And how it used.", 0, 1, description); 3002 case -669707736: 3003 /* useContext */ return new Property("useContext", "UsageContext", 3004 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate search parameter instances.", 3005 0, java.lang.Integer.MAX_VALUE, useContext); 3006 case -507075711: 3007 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 3008 "A legal or geographic region in which the search parameter is intended to be used.", 0, 3009 java.lang.Integer.MAX_VALUE, jurisdiction); 3010 case -220463842: 3011 /* purpose */ return new Property("purpose", "markdown", 3012 "Explanation of why this search parameter is needed and why it has been designed as it has.", 0, 1, purpose); 3013 case 3059181: 3014 /* code */ return new Property("code", "code", 3015 "The code used in the URL or the parameter name in a parameters resource for this search parameter.", 0, 1, 3016 code); 3017 case 3016401: 3018 /* base */ return new Property("base", "code", 3019 "The base resource type(s) that this search parameter can be used against.", 0, java.lang.Integer.MAX_VALUE, 3020 base); 3021 case 3575610: 3022 /* type */ return new Property("type", "code", 3023 "The type of value that a search parameter may contain, and how the content is interpreted.", 0, 1, type); 3024 case -1795452264: 3025 /* expression */ return new Property("expression", "string", 3026 "A FHIRPath expression that returns a set of elements for the search parameter.", 0, 1, expression); 3027 case 114256029: 3028 /* xpath */ return new Property("xpath", "string", 3029 "An XPath expression that returns a set of elements for the search parameter.", 0, 1, xpath); 3030 case 1801322244: 3031 /* xpathUsage */ return new Property("xpathUsage", "code", 3032 "How the search parameter relates to the set of elements returned by evaluating the xpath query.", 0, 1, 3033 xpathUsage); 3034 case -880905839: 3035 /* target */ return new Property("target", "code", "Types of resource (if a resource is referenced).", 0, 3036 java.lang.Integer.MAX_VALUE, target); 3037 case 1265069075: 3038 /* multipleOr */ return new Property("multipleOr", "boolean", 3039 "Whether multiple values are allowed for each time the parameter exists. Values are separated by commas, and the parameter matches if any of the values match.", 3040 0, 1, multipleOr); 3041 case 562422183: 3042 /* multipleAnd */ return new Property("multipleAnd", "boolean", 3043 "Whether multiple parameters are allowed - e.g. more than one parameter with the same name. The search matches if all the parameters match.", 3044 0, 1, multipleAnd); 3045 case -844673834: 3046 /* comparator */ return new Property("comparator", "code", "Comparators supported for the search parameter.", 0, 3047 java.lang.Integer.MAX_VALUE, comparator); 3048 case -615513385: 3049 /* modifier */ return new Property("modifier", "code", "A modifier supported for the search parameter.", 0, 3050 java.lang.Integer.MAX_VALUE, modifier); 3051 case 94623425: 3052 /* chain */ return new Property("chain", "string", 3053 "Contains the names of any search parameters which may be chained to the containing search parameter. Chained parameters may be added to search parameters of type reference and specify that resources will only be returned if they contain a reference to a resource which matches the chained parameter value. Values for this field should be drawn from SearchParameter.code for a parameter on the target resource type.", 3054 0, java.lang.Integer.MAX_VALUE, chain); 3055 case -1399907075: 3056 /* component */ return new Property("component", "", "Used to define the parts of a composite search parameter.", 3057 0, java.lang.Integer.MAX_VALUE, component); 3058 default: 3059 return super.getNamedProperty(_hash, _name, _checkValid); 3060 } 3061 3062 } 3063 3064 @Override 3065 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3066 switch (hash) { 3067 case 116079: 3068 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3069 case 351608024: 3070 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 3071 case 3373707: 3072 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3073 case 1077922663: 3074 /* derivedFrom */ return this.derivedFrom == null ? new Base[0] : new Base[] { this.derivedFrom }; // CanonicalType 3075 case -892481550: 3076 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 3077 case -404562712: 3078 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 3079 case 3076014: 3080 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3081 case 1447404028: 3082 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 3083 case 951526432: 3084 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3085 case -1724546052: 3086 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3087 case -669707736: 3088 /* useContext */ return this.useContext == null ? new Base[0] 3089 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3090 case -507075711: 3091 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 3092 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3093 case -220463842: 3094 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 3095 case 3059181: 3096 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 3097 case 3016401: 3098 /* base */ return this.base == null ? new Base[0] : this.base.toArray(new Base[this.base.size()]); // CodeType 3099 case 3575610: 3100 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<SearchParamType> 3101 case -1795452264: 3102 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 3103 case 114256029: 3104 /* xpath */ return this.xpath == null ? new Base[0] : new Base[] { this.xpath }; // StringType 3105 case 1801322244: 3106 /* xpathUsage */ return this.xpathUsage == null ? new Base[0] : new Base[] { this.xpathUsage }; // Enumeration<XPathUsageType> 3107 case -880905839: 3108 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // CodeType 3109 case 1265069075: 3110 /* multipleOr */ return this.multipleOr == null ? new Base[0] : new Base[] { this.multipleOr }; // BooleanType 3111 case 562422183: 3112 /* multipleAnd */ return this.multipleAnd == null ? new Base[0] : new Base[] { this.multipleAnd }; // BooleanType 3113 case -844673834: 3114 /* comparator */ return this.comparator == null ? new Base[0] 3115 : this.comparator.toArray(new Base[this.comparator.size()]); // Enumeration<SearchComparator> 3116 case -615513385: 3117 /* modifier */ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // Enumeration<SearchModifierCode> 3118 case 94623425: 3119 /* chain */ return this.chain == null ? new Base[0] : this.chain.toArray(new Base[this.chain.size()]); // StringType 3120 case -1399907075: 3121 /* component */ return this.component == null ? new Base[0] 3122 : this.component.toArray(new Base[this.component.size()]); // SearchParameterComponentComponent 3123 default: 3124 return super.getProperty(hash, name, checkValid); 3125 } 3126 3127 } 3128 3129 @Override 3130 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3131 switch (hash) { 3132 case 116079: // url 3133 this.url = castToUri(value); // UriType 3134 return value; 3135 case 351608024: // version 3136 this.version = castToString(value); // StringType 3137 return value; 3138 case 3373707: // name 3139 this.name = castToString(value); // StringType 3140 return value; 3141 case 1077922663: // derivedFrom 3142 this.derivedFrom = castToCanonical(value); // CanonicalType 3143 return value; 3144 case -892481550: // status 3145 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3146 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3147 return value; 3148 case -404562712: // experimental 3149 this.experimental = castToBoolean(value); // BooleanType 3150 return value; 3151 case 3076014: // date 3152 this.date = castToDateTime(value); // DateTimeType 3153 return value; 3154 case 1447404028: // publisher 3155 this.publisher = castToString(value); // StringType 3156 return value; 3157 case 951526432: // contact 3158 this.getContact().add(castToContactDetail(value)); // ContactDetail 3159 return value; 3160 case -1724546052: // description 3161 this.description = castToMarkdown(value); // MarkdownType 3162 return value; 3163 case -669707736: // useContext 3164 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3165 return value; 3166 case -507075711: // jurisdiction 3167 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3168 return value; 3169 case -220463842: // purpose 3170 this.purpose = castToMarkdown(value); // MarkdownType 3171 return value; 3172 case 3059181: // code 3173 this.code = castToCode(value); // CodeType 3174 return value; 3175 case 3016401: // base 3176 this.getBase().add(castToCode(value)); // CodeType 3177 return value; 3178 case 3575610: // type 3179 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 3180 this.type = (Enumeration) value; // Enumeration<SearchParamType> 3181 return value; 3182 case -1795452264: // expression 3183 this.expression = castToString(value); // StringType 3184 return value; 3185 case 114256029: // xpath 3186 this.xpath = castToString(value); // StringType 3187 return value; 3188 case 1801322244: // xpathUsage 3189 value = new XPathUsageTypeEnumFactory().fromType(castToCode(value)); 3190 this.xpathUsage = (Enumeration) value; // Enumeration<XPathUsageType> 3191 return value; 3192 case -880905839: // target 3193 this.getTarget().add(castToCode(value)); // CodeType 3194 return value; 3195 case 1265069075: // multipleOr 3196 this.multipleOr = castToBoolean(value); // BooleanType 3197 return value; 3198 case 562422183: // multipleAnd 3199 this.multipleAnd = castToBoolean(value); // BooleanType 3200 return value; 3201 case -844673834: // comparator 3202 value = new SearchComparatorEnumFactory().fromType(castToCode(value)); 3203 this.getComparator().add((Enumeration) value); // Enumeration<SearchComparator> 3204 return value; 3205 case -615513385: // modifier 3206 value = new SearchModifierCodeEnumFactory().fromType(castToCode(value)); 3207 this.getModifier().add((Enumeration) value); // Enumeration<SearchModifierCode> 3208 return value; 3209 case 94623425: // chain 3210 this.getChain().add(castToString(value)); // StringType 3211 return value; 3212 case -1399907075: // component 3213 this.getComponent().add((SearchParameterComponentComponent) value); // SearchParameterComponentComponent 3214 return value; 3215 default: 3216 return super.setProperty(hash, name, value); 3217 } 3218 3219 } 3220 3221 @Override 3222 public Base setProperty(String name, Base value) throws FHIRException { 3223 if (name.equals("url")) { 3224 this.url = castToUri(value); // UriType 3225 } else if (name.equals("version")) { 3226 this.version = castToString(value); // StringType 3227 } else if (name.equals("name")) { 3228 this.name = castToString(value); // StringType 3229 } else if (name.equals("derivedFrom")) { 3230 this.derivedFrom = castToCanonical(value); // CanonicalType 3231 } else if (name.equals("status")) { 3232 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3233 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3234 } else if (name.equals("experimental")) { 3235 this.experimental = castToBoolean(value); // BooleanType 3236 } else if (name.equals("date")) { 3237 this.date = castToDateTime(value); // DateTimeType 3238 } else if (name.equals("publisher")) { 3239 this.publisher = castToString(value); // StringType 3240 } else if (name.equals("contact")) { 3241 this.getContact().add(castToContactDetail(value)); 3242 } else if (name.equals("description")) { 3243 this.description = castToMarkdown(value); // MarkdownType 3244 } else if (name.equals("useContext")) { 3245 this.getUseContext().add(castToUsageContext(value)); 3246 } else if (name.equals("jurisdiction")) { 3247 this.getJurisdiction().add(castToCodeableConcept(value)); 3248 } else if (name.equals("purpose")) { 3249 this.purpose = castToMarkdown(value); // MarkdownType 3250 } else if (name.equals("code")) { 3251 this.code = castToCode(value); // CodeType 3252 } else if (name.equals("base")) { 3253 this.getBase().add(castToCode(value)); 3254 } else if (name.equals("type")) { 3255 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 3256 this.type = (Enumeration) value; // Enumeration<SearchParamType> 3257 } else if (name.equals("expression")) { 3258 this.expression = castToString(value); // StringType 3259 } else if (name.equals("xpath")) { 3260 this.xpath = castToString(value); // StringType 3261 } else if (name.equals("xpathUsage")) { 3262 value = new XPathUsageTypeEnumFactory().fromType(castToCode(value)); 3263 this.xpathUsage = (Enumeration) value; // Enumeration<XPathUsageType> 3264 } else if (name.equals("target")) { 3265 this.getTarget().add(castToCode(value)); 3266 } else if (name.equals("multipleOr")) { 3267 this.multipleOr = castToBoolean(value); // BooleanType 3268 } else if (name.equals("multipleAnd")) { 3269 this.multipleAnd = castToBoolean(value); // BooleanType 3270 } else if (name.equals("comparator")) { 3271 value = new SearchComparatorEnumFactory().fromType(castToCode(value)); 3272 this.getComparator().add((Enumeration) value); 3273 } else if (name.equals("modifier")) { 3274 value = new SearchModifierCodeEnumFactory().fromType(castToCode(value)); 3275 this.getModifier().add((Enumeration) value); 3276 } else if (name.equals("chain")) { 3277 this.getChain().add(castToString(value)); 3278 } else if (name.equals("component")) { 3279 this.getComponent().add((SearchParameterComponentComponent) value); 3280 } else 3281 return super.setProperty(name, value); 3282 return value; 3283 } 3284 3285 @Override 3286 public void removeChild(String name, Base value) throws FHIRException { 3287 if (name.equals("url")) { 3288 this.url = null; 3289 } else if (name.equals("version")) { 3290 this.version = null; 3291 } else if (name.equals("name")) { 3292 this.name = null; 3293 } else if (name.equals("derivedFrom")) { 3294 this.derivedFrom = null; 3295 } else if (name.equals("status")) { 3296 this.status = null; 3297 } else if (name.equals("experimental")) { 3298 this.experimental = null; 3299 } else if (name.equals("date")) { 3300 this.date = null; 3301 } else if (name.equals("publisher")) { 3302 this.publisher = null; 3303 } else if (name.equals("contact")) { 3304 this.getContact().remove(castToContactDetail(value)); 3305 } else if (name.equals("description")) { 3306 this.description = null; 3307 } else if (name.equals("useContext")) { 3308 this.getUseContext().remove(castToUsageContext(value)); 3309 } else if (name.equals("jurisdiction")) { 3310 this.getJurisdiction().remove(castToCodeableConcept(value)); 3311 } else if (name.equals("purpose")) { 3312 this.purpose = null; 3313 } else if (name.equals("code")) { 3314 this.code = null; 3315 } else if (name.equals("base")) { 3316 this.getBase().remove(castToCode(value)); 3317 } else if (name.equals("type")) { 3318 this.type = null; 3319 } else if (name.equals("expression")) { 3320 this.expression = null; 3321 } else if (name.equals("xpath")) { 3322 this.xpath = null; 3323 } else if (name.equals("xpathUsage")) { 3324 this.xpathUsage = null; 3325 } else if (name.equals("target")) { 3326 this.getTarget().remove(castToCode(value)); 3327 } else if (name.equals("multipleOr")) { 3328 this.multipleOr = null; 3329 } else if (name.equals("multipleAnd")) { 3330 this.multipleAnd = null; 3331 } else if (name.equals("comparator")) { 3332 this.getComparator().remove((Enumeration) value); 3333 } else if (name.equals("modifier")) { 3334 value = null; 3335 this.getModifier().remove((Enumeration) value); 3336 } else if (name.equals("chain")) { 3337 this.getChain().remove(castToString(value)); 3338 } else if (name.equals("component")) { 3339 this.getComponent().remove((SearchParameterComponentComponent) value); 3340 } else 3341 super.removeChild(name, value); 3342 3343 } 3344 3345 @Override 3346 public Base makeProperty(int hash, String name) throws FHIRException { 3347 switch (hash) { 3348 case 116079: 3349 return getUrlElement(); 3350 case 351608024: 3351 return getVersionElement(); 3352 case 3373707: 3353 return getNameElement(); 3354 case 1077922663: 3355 return getDerivedFromElement(); 3356 case -892481550: 3357 return getStatusElement(); 3358 case -404562712: 3359 return getExperimentalElement(); 3360 case 3076014: 3361 return getDateElement(); 3362 case 1447404028: 3363 return getPublisherElement(); 3364 case 951526432: 3365 return addContact(); 3366 case -1724546052: 3367 return getDescriptionElement(); 3368 case -669707736: 3369 return addUseContext(); 3370 case -507075711: 3371 return addJurisdiction(); 3372 case -220463842: 3373 return getPurposeElement(); 3374 case 3059181: 3375 return getCodeElement(); 3376 case 3016401: 3377 return addBaseElement(); 3378 case 3575610: 3379 return getTypeElement(); 3380 case -1795452264: 3381 return getExpressionElement(); 3382 case 114256029: 3383 return getXpathElement(); 3384 case 1801322244: 3385 return getXpathUsageElement(); 3386 case -880905839: 3387 return addTargetElement(); 3388 case 1265069075: 3389 return getMultipleOrElement(); 3390 case 562422183: 3391 return getMultipleAndElement(); 3392 case -844673834: 3393 return addComparatorElement(); 3394 case -615513385: 3395 return addModifierElement(); 3396 case 94623425: 3397 return addChainElement(); 3398 case -1399907075: 3399 return addComponent(); 3400 default: 3401 return super.makeProperty(hash, name); 3402 } 3403 3404 } 3405 3406 @Override 3407 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3408 switch (hash) { 3409 case 116079: 3410 /* url */ return new String[] { "uri" }; 3411 case 351608024: 3412 /* version */ return new String[] { "string" }; 3413 case 3373707: 3414 /* name */ return new String[] { "string" }; 3415 case 1077922663: 3416 /* derivedFrom */ return new String[] { "canonical" }; 3417 case -892481550: 3418 /* status */ return new String[] { "code" }; 3419 case -404562712: 3420 /* experimental */ return new String[] { "boolean" }; 3421 case 3076014: 3422 /* date */ return new String[] { "dateTime" }; 3423 case 1447404028: 3424 /* publisher */ return new String[] { "string" }; 3425 case 951526432: 3426 /* contact */ return new String[] { "ContactDetail" }; 3427 case -1724546052: 3428 /* description */ return new String[] { "markdown" }; 3429 case -669707736: 3430 /* useContext */ return new String[] { "UsageContext" }; 3431 case -507075711: 3432 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3433 case -220463842: 3434 /* purpose */ return new String[] { "markdown" }; 3435 case 3059181: 3436 /* code */ return new String[] { "code" }; 3437 case 3016401: 3438 /* base */ return new String[] { "code" }; 3439 case 3575610: 3440 /* type */ return new String[] { "code" }; 3441 case -1795452264: 3442 /* expression */ return new String[] { "string" }; 3443 case 114256029: 3444 /* xpath */ return new String[] { "string" }; 3445 case 1801322244: 3446 /* xpathUsage */ return new String[] { "code" }; 3447 case -880905839: 3448 /* target */ return new String[] { "code" }; 3449 case 1265069075: 3450 /* multipleOr */ return new String[] { "boolean" }; 3451 case 562422183: 3452 /* multipleAnd */ return new String[] { "boolean" }; 3453 case -844673834: 3454 /* comparator */ return new String[] { "code" }; 3455 case -615513385: 3456 /* modifier */ return new String[] { "code" }; 3457 case 94623425: 3458 /* chain */ return new String[] { "string" }; 3459 case -1399907075: 3460 /* component */ return new String[] {}; 3461 default: 3462 return super.getTypesForProperty(hash, name); 3463 } 3464 3465 } 3466 3467 @Override 3468 public Base addChild(String name) throws FHIRException { 3469 if (name.equals("url")) { 3470 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.url"); 3471 } else if (name.equals("version")) { 3472 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.version"); 3473 } else if (name.equals("name")) { 3474 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.name"); 3475 } else if (name.equals("derivedFrom")) { 3476 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.derivedFrom"); 3477 } else if (name.equals("status")) { 3478 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.status"); 3479 } else if (name.equals("experimental")) { 3480 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.experimental"); 3481 } else if (name.equals("date")) { 3482 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.date"); 3483 } else if (name.equals("publisher")) { 3484 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.publisher"); 3485 } else if (name.equals("contact")) { 3486 return addContact(); 3487 } else if (name.equals("description")) { 3488 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.description"); 3489 } else if (name.equals("useContext")) { 3490 return addUseContext(); 3491 } else if (name.equals("jurisdiction")) { 3492 return addJurisdiction(); 3493 } else if (name.equals("purpose")) { 3494 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.purpose"); 3495 } else if (name.equals("code")) { 3496 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.code"); 3497 } else if (name.equals("base")) { 3498 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.base"); 3499 } else if (name.equals("type")) { 3500 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.type"); 3501 } else if (name.equals("expression")) { 3502 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.expression"); 3503 } else if (name.equals("xpath")) { 3504 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpath"); 3505 } else if (name.equals("xpathUsage")) { 3506 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.xpathUsage"); 3507 } else if (name.equals("target")) { 3508 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.target"); 3509 } else if (name.equals("multipleOr")) { 3510 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.multipleOr"); 3511 } else if (name.equals("multipleAnd")) { 3512 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.multipleAnd"); 3513 } else if (name.equals("comparator")) { 3514 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.comparator"); 3515 } else if (name.equals("modifier")) { 3516 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.modifier"); 3517 } else if (name.equals("chain")) { 3518 throw new FHIRException("Cannot call addChild on a singleton property SearchParameter.chain"); 3519 } else if (name.equals("component")) { 3520 return addComponent(); 3521 } else 3522 return super.addChild(name); 3523 } 3524 3525 public String fhirType() { 3526 return "SearchParameter"; 3527 3528 } 3529 3530 public SearchParameter copy() { 3531 SearchParameter dst = new SearchParameter(); 3532 copyValues(dst); 3533 return dst; 3534 } 3535 3536 public void copyValues(SearchParameter dst) { 3537 super.copyValues(dst); 3538 dst.url = url == null ? null : url.copy(); 3539 dst.version = version == null ? null : version.copy(); 3540 dst.name = name == null ? null : name.copy(); 3541 dst.derivedFrom = derivedFrom == null ? null : derivedFrom.copy(); 3542 dst.status = status == null ? null : status.copy(); 3543 dst.experimental = experimental == null ? null : experimental.copy(); 3544 dst.date = date == null ? null : date.copy(); 3545 dst.publisher = publisher == null ? null : publisher.copy(); 3546 if (contact != null) { 3547 dst.contact = new ArrayList<ContactDetail>(); 3548 for (ContactDetail i : contact) 3549 dst.contact.add(i.copy()); 3550 } 3551 ; 3552 dst.description = description == null ? null : description.copy(); 3553 if (useContext != null) { 3554 dst.useContext = new ArrayList<UsageContext>(); 3555 for (UsageContext i : useContext) 3556 dst.useContext.add(i.copy()); 3557 } 3558 ; 3559 if (jurisdiction != null) { 3560 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3561 for (CodeableConcept i : jurisdiction) 3562 dst.jurisdiction.add(i.copy()); 3563 } 3564 ; 3565 dst.purpose = purpose == null ? null : purpose.copy(); 3566 dst.code = code == null ? null : code.copy(); 3567 if (base != null) { 3568 dst.base = new ArrayList<CodeType>(); 3569 for (CodeType i : base) 3570 dst.base.add(i.copy()); 3571 } 3572 ; 3573 dst.type = type == null ? null : type.copy(); 3574 dst.expression = expression == null ? null : expression.copy(); 3575 dst.xpath = xpath == null ? null : xpath.copy(); 3576 dst.xpathUsage = xpathUsage == null ? null : xpathUsage.copy(); 3577 if (target != null) { 3578 dst.target = new ArrayList<CodeType>(); 3579 for (CodeType i : target) 3580 dst.target.add(i.copy()); 3581 } 3582 ; 3583 dst.multipleOr = multipleOr == null ? null : multipleOr.copy(); 3584 dst.multipleAnd = multipleAnd == null ? null : multipleAnd.copy(); 3585 if (comparator != null) { 3586 dst.comparator = new ArrayList<Enumeration<SearchComparator>>(); 3587 for (Enumeration<SearchComparator> i : comparator) 3588 dst.comparator.add(i.copy()); 3589 } 3590 ; 3591 if (modifier != null) { 3592 dst.modifier = new ArrayList<Enumeration<SearchModifierCode>>(); 3593 for (Enumeration<SearchModifierCode> i : modifier) 3594 dst.modifier.add(i.copy()); 3595 } 3596 ; 3597 if (chain != null) { 3598 dst.chain = new ArrayList<StringType>(); 3599 for (StringType i : chain) 3600 dst.chain.add(i.copy()); 3601 } 3602 ; 3603 if (component != null) { 3604 dst.component = new ArrayList<SearchParameterComponentComponent>(); 3605 for (SearchParameterComponentComponent i : component) 3606 dst.component.add(i.copy()); 3607 } 3608 ; 3609 } 3610 3611 protected SearchParameter typedCopy() { 3612 return copy(); 3613 } 3614 3615 @Override 3616 public boolean equalsDeep(Base other_) { 3617 if (!super.equalsDeep(other_)) 3618 return false; 3619 if (!(other_ instanceof SearchParameter)) 3620 return false; 3621 SearchParameter o = (SearchParameter) other_; 3622 return compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(purpose, o.purpose, true) 3623 && compareDeep(code, o.code, true) && compareDeep(base, o.base, true) && compareDeep(type, o.type, true) 3624 && compareDeep(expression, o.expression, true) && compareDeep(xpath, o.xpath, true) 3625 && compareDeep(xpathUsage, o.xpathUsage, true) && compareDeep(target, o.target, true) 3626 && compareDeep(multipleOr, o.multipleOr, true) && compareDeep(multipleAnd, o.multipleAnd, true) 3627 && compareDeep(comparator, o.comparator, true) && compareDeep(modifier, o.modifier, true) 3628 && compareDeep(chain, o.chain, true) && compareDeep(component, o.component, true); 3629 } 3630 3631 @Override 3632 public boolean equalsShallow(Base other_) { 3633 if (!super.equalsShallow(other_)) 3634 return false; 3635 if (!(other_ instanceof SearchParameter)) 3636 return false; 3637 SearchParameter o = (SearchParameter) other_; 3638 return compareValues(purpose, o.purpose, true) && compareValues(code, o.code, true) 3639 && compareValues(base, o.base, true) && compareValues(type, o.type, true) 3640 && compareValues(expression, o.expression, true) && compareValues(xpath, o.xpath, true) 3641 && compareValues(xpathUsage, o.xpathUsage, true) && compareValues(target, o.target, true) 3642 && compareValues(multipleOr, o.multipleOr, true) && compareValues(multipleAnd, o.multipleAnd, true) 3643 && compareValues(comparator, o.comparator, true) && compareValues(modifier, o.modifier, true) 3644 && compareValues(chain, o.chain, true); 3645 } 3646 3647 public boolean isEmpty() { 3648 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(derivedFrom, purpose, code, base, type, expression, 3649 xpath, xpathUsage, target, multipleOr, multipleAnd, comparator, modifier, chain, component); 3650 } 3651 3652 @Override 3653 public ResourceType getResourceType() { 3654 return ResourceType.SearchParameter; 3655 } 3656 3657 /** 3658 * Search parameter: <b>date</b> 3659 * <p> 3660 * Description: <b>The search parameter publication date</b><br> 3661 * Type: <b>date</b><br> 3662 * Path: <b>SearchParameter.date</b><br> 3663 * </p> 3664 */ 3665 @SearchParamDefinition(name = "date", path = "SearchParameter.date", description = "The search parameter publication date", type = "date") 3666 public static final String SP_DATE = "date"; 3667 /** 3668 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3669 * <p> 3670 * Description: <b>The search parameter publication date</b><br> 3671 * Type: <b>date</b><br> 3672 * Path: <b>SearchParameter.date</b><br> 3673 * </p> 3674 */ 3675 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3676 SP_DATE); 3677 3678 /** 3679 * Search parameter: <b>code</b> 3680 * <p> 3681 * Description: <b>Code used in URL</b><br> 3682 * Type: <b>token</b><br> 3683 * Path: <b>SearchParameter.code</b><br> 3684 * </p> 3685 */ 3686 @SearchParamDefinition(name = "code", path = "SearchParameter.code", description = "Code used in URL", type = "token") 3687 public static final String SP_CODE = "code"; 3688 /** 3689 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3690 * <p> 3691 * Description: <b>Code used in URL</b><br> 3692 * Type: <b>token</b><br> 3693 * Path: <b>SearchParameter.code</b><br> 3694 * </p> 3695 */ 3696 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3697 SP_CODE); 3698 3699 /** 3700 * Search parameter: <b>context-type-value</b> 3701 * <p> 3702 * Description: <b>A use context type and value assigned to the search 3703 * parameter</b><br> 3704 * Type: <b>composite</b><br> 3705 * Path: <b></b><br> 3706 * </p> 3707 */ 3708 @SearchParamDefinition(name = "context-type-value", path = "SearchParameter.useContext", description = "A use context type and value assigned to the search parameter", type = "composite", compositeOf = { 3709 "context-type", "context" }) 3710 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3711 /** 3712 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3713 * <p> 3714 * Description: <b>A use context type and value assigned to the search 3715 * parameter</b><br> 3716 * Type: <b>composite</b><br> 3717 * Path: <b></b><br> 3718 * </p> 3719 */ 3720 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3721 SP_CONTEXT_TYPE_VALUE); 3722 3723 /** 3724 * Search parameter: <b>jurisdiction</b> 3725 * <p> 3726 * Description: <b>Intended jurisdiction for the search parameter</b><br> 3727 * Type: <b>token</b><br> 3728 * Path: <b>SearchParameter.jurisdiction</b><br> 3729 * </p> 3730 */ 3731 @SearchParamDefinition(name = "jurisdiction", path = "SearchParameter.jurisdiction", description = "Intended jurisdiction for the search parameter", type = "token") 3732 public static final String SP_JURISDICTION = "jurisdiction"; 3733 /** 3734 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3735 * <p> 3736 * Description: <b>Intended jurisdiction for the search parameter</b><br> 3737 * Type: <b>token</b><br> 3738 * Path: <b>SearchParameter.jurisdiction</b><br> 3739 * </p> 3740 */ 3741 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3742 SP_JURISDICTION); 3743 3744 /** 3745 * Search parameter: <b>description</b> 3746 * <p> 3747 * Description: <b>The description of the search parameter</b><br> 3748 * Type: <b>string</b><br> 3749 * Path: <b>SearchParameter.description</b><br> 3750 * </p> 3751 */ 3752 @SearchParamDefinition(name = "description", path = "SearchParameter.description", description = "The description of the search parameter", type = "string") 3753 public static final String SP_DESCRIPTION = "description"; 3754 /** 3755 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3756 * <p> 3757 * Description: <b>The description of the search parameter</b><br> 3758 * Type: <b>string</b><br> 3759 * Path: <b>SearchParameter.description</b><br> 3760 * </p> 3761 */ 3762 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3763 SP_DESCRIPTION); 3764 3765 /** 3766 * Search parameter: <b>derived-from</b> 3767 * <p> 3768 * Description: <b>Original definition for the search parameter</b><br> 3769 * Type: <b>reference</b><br> 3770 * Path: <b>SearchParameter.derivedFrom</b><br> 3771 * </p> 3772 */ 3773 @SearchParamDefinition(name = "derived-from", path = "SearchParameter.derivedFrom", description = "Original definition for the search parameter", type = "reference", target = { 3774 SearchParameter.class }) 3775 public static final String SP_DERIVED_FROM = "derived-from"; 3776 /** 3777 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 3778 * <p> 3779 * Description: <b>Original definition for the search parameter</b><br> 3780 * Type: <b>reference</b><br> 3781 * Path: <b>SearchParameter.derivedFrom</b><br> 3782 * </p> 3783 */ 3784 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3785 SP_DERIVED_FROM); 3786 3787 /** 3788 * Constant for fluent queries to be used to add include statements. Specifies 3789 * the path value of "<b>SearchParameter:derived-from</b>". 3790 */ 3791 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 3792 "SearchParameter:derived-from").toLocked(); 3793 3794 /** 3795 * Search parameter: <b>context-type</b> 3796 * <p> 3797 * Description: <b>A type of use context assigned to the search 3798 * parameter</b><br> 3799 * Type: <b>token</b><br> 3800 * Path: <b>SearchParameter.useContext.code</b><br> 3801 * </p> 3802 */ 3803 @SearchParamDefinition(name = "context-type", path = "SearchParameter.useContext.code", description = "A type of use context assigned to the search parameter", type = "token") 3804 public static final String SP_CONTEXT_TYPE = "context-type"; 3805 /** 3806 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3807 * <p> 3808 * Description: <b>A type of use context assigned to the search 3809 * parameter</b><br> 3810 * Type: <b>token</b><br> 3811 * Path: <b>SearchParameter.useContext.code</b><br> 3812 * </p> 3813 */ 3814 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3815 SP_CONTEXT_TYPE); 3816 3817 /** 3818 * Search parameter: <b>type</b> 3819 * <p> 3820 * Description: <b>number | date | string | token | reference | composite | 3821 * quantity | uri | special</b><br> 3822 * Type: <b>token</b><br> 3823 * Path: <b>SearchParameter.type</b><br> 3824 * </p> 3825 */ 3826 @SearchParamDefinition(name = "type", path = "SearchParameter.type", description = "number | date | string | token | reference | composite | quantity | uri | special", type = "token") 3827 public static final String SP_TYPE = "type"; 3828 /** 3829 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3830 * <p> 3831 * Description: <b>number | date | string | token | reference | composite | 3832 * quantity | uri | special</b><br> 3833 * Type: <b>token</b><br> 3834 * Path: <b>SearchParameter.type</b><br> 3835 * </p> 3836 */ 3837 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3838 SP_TYPE); 3839 3840 /** 3841 * Search parameter: <b>version</b> 3842 * <p> 3843 * Description: <b>The business version of the search parameter</b><br> 3844 * Type: <b>token</b><br> 3845 * Path: <b>SearchParameter.version</b><br> 3846 * </p> 3847 */ 3848 @SearchParamDefinition(name = "version", path = "SearchParameter.version", description = "The business version of the search parameter", type = "token") 3849 public static final String SP_VERSION = "version"; 3850 /** 3851 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3852 * <p> 3853 * Description: <b>The business version of the search parameter</b><br> 3854 * Type: <b>token</b><br> 3855 * Path: <b>SearchParameter.version</b><br> 3856 * </p> 3857 */ 3858 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3859 SP_VERSION); 3860 3861 /** 3862 * Search parameter: <b>url</b> 3863 * <p> 3864 * Description: <b>The uri that identifies the search parameter</b><br> 3865 * Type: <b>uri</b><br> 3866 * Path: <b>SearchParameter.url</b><br> 3867 * </p> 3868 */ 3869 @SearchParamDefinition(name = "url", path = "SearchParameter.url", description = "The uri that identifies the search parameter", type = "uri") 3870 public static final String SP_URL = "url"; 3871 /** 3872 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3873 * <p> 3874 * Description: <b>The uri that identifies the search parameter</b><br> 3875 * Type: <b>uri</b><br> 3876 * Path: <b>SearchParameter.url</b><br> 3877 * </p> 3878 */ 3879 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3880 3881 /** 3882 * Search parameter: <b>target</b> 3883 * <p> 3884 * Description: <b>Types of resource (if a resource reference)</b><br> 3885 * Type: <b>token</b><br> 3886 * Path: <b>SearchParameter.target</b><br> 3887 * </p> 3888 */ 3889 @SearchParamDefinition(name = "target", path = "SearchParameter.target", description = "Types of resource (if a resource reference)", type = "token") 3890 public static final String SP_TARGET = "target"; 3891 /** 3892 * <b>Fluent Client</b> search parameter constant for <b>target</b> 3893 * <p> 3894 * Description: <b>Types of resource (if a resource reference)</b><br> 3895 * Type: <b>token</b><br> 3896 * Path: <b>SearchParameter.target</b><br> 3897 * </p> 3898 */ 3899 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3900 SP_TARGET); 3901 3902 /** 3903 * Search parameter: <b>context-quantity</b> 3904 * <p> 3905 * Description: <b>A quantity- or range-valued use context assigned to the 3906 * search parameter</b><br> 3907 * Type: <b>quantity</b><br> 3908 * Path: <b>SearchParameter.useContext.valueQuantity, 3909 * SearchParameter.useContext.valueRange</b><br> 3910 * </p> 3911 */ 3912 @SearchParamDefinition(name = "context-quantity", path = "(SearchParameter.useContext.value as Quantity) | (SearchParameter.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the search parameter", type = "quantity") 3913 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3914 /** 3915 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3916 * <p> 3917 * Description: <b>A quantity- or range-valued use context assigned to the 3918 * search parameter</b><br> 3919 * Type: <b>quantity</b><br> 3920 * Path: <b>SearchParameter.useContext.valueQuantity, 3921 * SearchParameter.useContext.valueRange</b><br> 3922 * </p> 3923 */ 3924 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3925 SP_CONTEXT_QUANTITY); 3926 3927 /** 3928 * Search parameter: <b>component</b> 3929 * <p> 3930 * Description: <b>Defines how the part works</b><br> 3931 * Type: <b>reference</b><br> 3932 * Path: <b>SearchParameter.component.definition</b><br> 3933 * </p> 3934 */ 3935 @SearchParamDefinition(name = "component", path = "SearchParameter.component.definition", description = "Defines how the part works", type = "reference", target = { 3936 SearchParameter.class }) 3937 public static final String SP_COMPONENT = "component"; 3938 /** 3939 * <b>Fluent Client</b> search parameter constant for <b>component</b> 3940 * <p> 3941 * Description: <b>Defines how the part works</b><br> 3942 * Type: <b>reference</b><br> 3943 * Path: <b>SearchParameter.component.definition</b><br> 3944 * </p> 3945 */ 3946 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPONENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3947 SP_COMPONENT); 3948 3949 /** 3950 * Constant for fluent queries to be used to add include statements. Specifies 3951 * the path value of "<b>SearchParameter:component</b>". 3952 */ 3953 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPONENT = new ca.uhn.fhir.model.api.Include( 3954 "SearchParameter:component").toLocked(); 3955 3956 /** 3957 * Search parameter: <b>name</b> 3958 * <p> 3959 * Description: <b>Computationally friendly name of the search parameter</b><br> 3960 * Type: <b>string</b><br> 3961 * Path: <b>SearchParameter.name</b><br> 3962 * </p> 3963 */ 3964 @SearchParamDefinition(name = "name", path = "SearchParameter.name", description = "Computationally friendly name of the search parameter", type = "string") 3965 public static final String SP_NAME = "name"; 3966 /** 3967 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3968 * <p> 3969 * Description: <b>Computationally friendly name of the search parameter</b><br> 3970 * Type: <b>string</b><br> 3971 * Path: <b>SearchParameter.name</b><br> 3972 * </p> 3973 */ 3974 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3975 SP_NAME); 3976 3977 /** 3978 * Search parameter: <b>context</b> 3979 * <p> 3980 * Description: <b>A use context assigned to the search parameter</b><br> 3981 * Type: <b>token</b><br> 3982 * Path: <b>SearchParameter.useContext.valueCodeableConcept</b><br> 3983 * </p> 3984 */ 3985 @SearchParamDefinition(name = "context", path = "(SearchParameter.useContext.value as CodeableConcept)", description = "A use context assigned to the search parameter", type = "token") 3986 public static final String SP_CONTEXT = "context"; 3987 /** 3988 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3989 * <p> 3990 * Description: <b>A use context assigned to the search parameter</b><br> 3991 * Type: <b>token</b><br> 3992 * Path: <b>SearchParameter.useContext.valueCodeableConcept</b><br> 3993 * </p> 3994 */ 3995 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3996 SP_CONTEXT); 3997 3998 /** 3999 * Search parameter: <b>publisher</b> 4000 * <p> 4001 * Description: <b>Name of the publisher of the search parameter</b><br> 4002 * Type: <b>string</b><br> 4003 * Path: <b>SearchParameter.publisher</b><br> 4004 * </p> 4005 */ 4006 @SearchParamDefinition(name = "publisher", path = "SearchParameter.publisher", description = "Name of the publisher of the search parameter", type = "string") 4007 public static final String SP_PUBLISHER = "publisher"; 4008 /** 4009 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4010 * <p> 4011 * Description: <b>Name of the publisher of the search parameter</b><br> 4012 * Type: <b>string</b><br> 4013 * Path: <b>SearchParameter.publisher</b><br> 4014 * </p> 4015 */ 4016 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 4017 SP_PUBLISHER); 4018 4019 /** 4020 * Search parameter: <b>context-type-quantity</b> 4021 * <p> 4022 * Description: <b>A use context type and quantity- or range-based value 4023 * assigned to the search parameter</b><br> 4024 * Type: <b>composite</b><br> 4025 * Path: <b></b><br> 4026 * </p> 4027 */ 4028 @SearchParamDefinition(name = "context-type-quantity", path = "SearchParameter.useContext", description = "A use context type and quantity- or range-based value assigned to the search parameter", type = "composite", compositeOf = { 4029 "context-type", "context-quantity" }) 4030 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4031 /** 4032 * <b>Fluent Client</b> search parameter constant for 4033 * <b>context-type-quantity</b> 4034 * <p> 4035 * Description: <b>A use context type and quantity- or range-based value 4036 * assigned to the search parameter</b><br> 4037 * Type: <b>composite</b><br> 4038 * Path: <b></b><br> 4039 * </p> 4040 */ 4041 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4042 SP_CONTEXT_TYPE_QUANTITY); 4043 4044 /** 4045 * Search parameter: <b>status</b> 4046 * <p> 4047 * Description: <b>The current status of the search parameter</b><br> 4048 * Type: <b>token</b><br> 4049 * Path: <b>SearchParameter.status</b><br> 4050 * </p> 4051 */ 4052 @SearchParamDefinition(name = "status", path = "SearchParameter.status", description = "The current status of the search parameter", type = "token") 4053 public static final String SP_STATUS = "status"; 4054 /** 4055 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4056 * <p> 4057 * Description: <b>The current status of the search parameter</b><br> 4058 * Type: <b>token</b><br> 4059 * Path: <b>SearchParameter.status</b><br> 4060 * </p> 4061 */ 4062 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4063 SP_STATUS); 4064 4065 /** 4066 * Search parameter: <b>base</b> 4067 * <p> 4068 * Description: <b>The resource type(s) this search parameter applies to</b><br> 4069 * Type: <b>token</b><br> 4070 * Path: <b>SearchParameter.base</b><br> 4071 * </p> 4072 */ 4073 @SearchParamDefinition(name = "base", path = "SearchParameter.base", description = "The resource type(s) this search parameter applies to", type = "token") 4074 public static final String SP_BASE = "base"; 4075 /** 4076 * <b>Fluent Client</b> search parameter constant for <b>base</b> 4077 * <p> 4078 * Description: <b>The resource type(s) this search parameter applies to</b><br> 4079 * Type: <b>token</b><br> 4080 * Path: <b>SearchParameter.base</b><br> 4081 * </p> 4082 */ 4083 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BASE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4084 SP_BASE); 4085 4086}