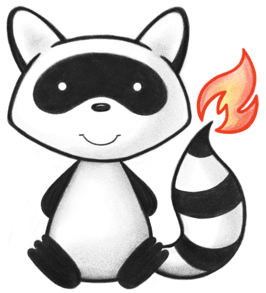
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * A record of a request for service such as diagnostic investigations, 047 * treatments, or operations to be performed. 048 */ 049@ResourceDef(name = "ServiceRequest", profile = "http://hl7.org/fhir/StructureDefinition/ServiceRequest") 050public class ServiceRequest extends DomainResource { 051 052 public enum ServiceRequestStatus { 053 /** 054 * The request has been created but is not yet complete or ready for action. 055 */ 056 DRAFT, 057 /** 058 * The request is in force and ready to be acted upon. 059 */ 060 ACTIVE, 061 /** 062 * The request (and any implicit authorization to act) has been temporarily 063 * withdrawn but is expected to resume in the future. 064 */ 065 ONHOLD, 066 /** 067 * The request (and any implicit authorization to act) has been terminated prior 068 * to the known full completion of the intended actions. No further activity 069 * should occur. 070 */ 071 REVOKED, 072 /** 073 * The activity described by the request has been fully performed. No further 074 * activity will occur. 075 */ 076 COMPLETED, 077 /** 078 * This request should never have existed and should be considered 'void'. (It 079 * is possible that real-world decisions were based on it. If real-world 080 * activity has occurred, the status should be "revoked" rather than 081 * "entered-in-error".). 082 */ 083 ENTEREDINERROR, 084 /** 085 * The authoring/source system does not know which of the status values 086 * currently applies for this request. Note: This concept is not to be used for 087 * "other" - one of the listed statuses is presumed to apply, but the 088 * authoring/source system does not know which. 089 */ 090 UNKNOWN, 091 /** 092 * added to help the parsers with the generic types 093 */ 094 NULL; 095 096 public static ServiceRequestStatus fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("draft".equals(codeString)) 100 return DRAFT; 101 if ("active".equals(codeString)) 102 return ACTIVE; 103 if ("on-hold".equals(codeString)) 104 return ONHOLD; 105 if ("revoked".equals(codeString)) 106 return REVOKED; 107 if ("completed".equals(codeString)) 108 return COMPLETED; 109 if ("entered-in-error".equals(codeString)) 110 return ENTEREDINERROR; 111 if ("unknown".equals(codeString)) 112 return UNKNOWN; 113 if (Configuration.isAcceptInvalidEnums()) 114 return null; 115 else 116 throw new FHIRException("Unknown ServiceRequestStatus code '" + codeString + "'"); 117 } 118 119 public String toCode() { 120 switch (this) { 121 case DRAFT: 122 return "draft"; 123 case ACTIVE: 124 return "active"; 125 case ONHOLD: 126 return "on-hold"; 127 case REVOKED: 128 return "revoked"; 129 case COMPLETED: 130 return "completed"; 131 case ENTEREDINERROR: 132 return "entered-in-error"; 133 case UNKNOWN: 134 return "unknown"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getSystem() { 143 switch (this) { 144 case DRAFT: 145 return "http://hl7.org/fhir/request-status"; 146 case ACTIVE: 147 return "http://hl7.org/fhir/request-status"; 148 case ONHOLD: 149 return "http://hl7.org/fhir/request-status"; 150 case REVOKED: 151 return "http://hl7.org/fhir/request-status"; 152 case COMPLETED: 153 return "http://hl7.org/fhir/request-status"; 154 case ENTEREDINERROR: 155 return "http://hl7.org/fhir/request-status"; 156 case UNKNOWN: 157 return "http://hl7.org/fhir/request-status"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165 public String getDefinition() { 166 switch (this) { 167 case DRAFT: 168 return "The request has been created but is not yet complete or ready for action."; 169 case ACTIVE: 170 return "The request is in force and ready to be acted upon."; 171 case ONHOLD: 172 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 173 case REVOKED: 174 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 175 case COMPLETED: 176 return "The activity described by the request has been fully performed. No further activity will occur."; 177 case ENTEREDINERROR: 178 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 179 case UNKNOWN: 180 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 188 public String getDisplay() { 189 switch (this) { 190 case DRAFT: 191 return "Draft"; 192 case ACTIVE: 193 return "Active"; 194 case ONHOLD: 195 return "On Hold"; 196 case REVOKED: 197 return "Revoked"; 198 case COMPLETED: 199 return "Completed"; 200 case ENTEREDINERROR: 201 return "Entered in Error"; 202 case UNKNOWN: 203 return "Unknown"; 204 case NULL: 205 return null; 206 default: 207 return "?"; 208 } 209 } 210 } 211 212 public static class ServiceRequestStatusEnumFactory implements EnumFactory<ServiceRequestStatus> { 213 public ServiceRequestStatus fromCode(String codeString) throws IllegalArgumentException { 214 if (codeString == null || "".equals(codeString)) 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("draft".equals(codeString)) 218 return ServiceRequestStatus.DRAFT; 219 if ("active".equals(codeString)) 220 return ServiceRequestStatus.ACTIVE; 221 if ("on-hold".equals(codeString)) 222 return ServiceRequestStatus.ONHOLD; 223 if ("revoked".equals(codeString)) 224 return ServiceRequestStatus.REVOKED; 225 if ("completed".equals(codeString)) 226 return ServiceRequestStatus.COMPLETED; 227 if ("entered-in-error".equals(codeString)) 228 return ServiceRequestStatus.ENTEREDINERROR; 229 if ("unknown".equals(codeString)) 230 return ServiceRequestStatus.UNKNOWN; 231 throw new IllegalArgumentException("Unknown ServiceRequestStatus code '" + codeString + "'"); 232 } 233 234 public Enumeration<ServiceRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.NULL, code); 239 String codeString = code.asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.NULL, code); 242 if ("draft".equals(codeString)) 243 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.DRAFT, code); 244 if ("active".equals(codeString)) 245 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.ACTIVE, code); 246 if ("on-hold".equals(codeString)) 247 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.ONHOLD, code); 248 if ("revoked".equals(codeString)) 249 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.REVOKED, code); 250 if ("completed".equals(codeString)) 251 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.COMPLETED, code); 252 if ("entered-in-error".equals(codeString)) 253 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.ENTEREDINERROR, code); 254 if ("unknown".equals(codeString)) 255 return new Enumeration<ServiceRequestStatus>(this, ServiceRequestStatus.UNKNOWN, code); 256 throw new FHIRException("Unknown ServiceRequestStatus code '" + codeString + "'"); 257 } 258 259 public String toCode(ServiceRequestStatus code) { 260 if (code == ServiceRequestStatus.NULL) 261 return null; 262 if (code == ServiceRequestStatus.DRAFT) 263 return "draft"; 264 if (code == ServiceRequestStatus.ACTIVE) 265 return "active"; 266 if (code == ServiceRequestStatus.ONHOLD) 267 return "on-hold"; 268 if (code == ServiceRequestStatus.REVOKED) 269 return "revoked"; 270 if (code == ServiceRequestStatus.COMPLETED) 271 return "completed"; 272 if (code == ServiceRequestStatus.ENTEREDINERROR) 273 return "entered-in-error"; 274 if (code == ServiceRequestStatus.UNKNOWN) 275 return "unknown"; 276 return "?"; 277 } 278 279 public String toSystem(ServiceRequestStatus code) { 280 return code.getSystem(); 281 } 282 } 283 284 public enum ServiceRequestIntent { 285 /** 286 * The request is a suggestion made by someone/something that does not have an 287 * intention to ensure it occurs and without providing an authorization to act. 288 */ 289 PROPOSAL, 290 /** 291 * The request represents an intention to ensure something occurs without 292 * providing an authorization for others to act. 293 */ 294 PLAN, 295 /** 296 * The request represents a legally binding instruction authored by a Patient or 297 * RelatedPerson. 298 */ 299 DIRECTIVE, 300 /** 301 * The request represents a request/demand and authorization for action by a 302 * Practitioner. 303 */ 304 ORDER, 305 /** 306 * The request represents an original authorization for action. 307 */ 308 ORIGINALORDER, 309 /** 310 * The request represents an automatically generated supplemental authorization 311 * for action based on a parent authorization together with initial results of 312 * the action taken against that parent authorization. 313 */ 314 REFLEXORDER, 315 /** 316 * The request represents the view of an authorization instantiated by a 317 * fulfilling system representing the details of the fulfiller's intention to 318 * act upon a submitted order. 319 */ 320 FILLERORDER, 321 /** 322 * An order created in fulfillment of a broader order that represents the 323 * authorization for a single activity occurrence. E.g. The administration of a 324 * single dose of a drug. 325 */ 326 INSTANCEORDER, 327 /** 328 * The request represents a component or option for a RequestGroup that 329 * establishes timing, conditionality and/or other constraints among a set of 330 * requests. Refer to [[[RequestGroup]]] for additional information on how this 331 * status is used. 332 */ 333 OPTION, 334 /** 335 * added to help the parsers with the generic types 336 */ 337 NULL; 338 339 public static ServiceRequestIntent fromCode(String codeString) throws FHIRException { 340 if (codeString == null || "".equals(codeString)) 341 return null; 342 if ("proposal".equals(codeString)) 343 return PROPOSAL; 344 if ("plan".equals(codeString)) 345 return PLAN; 346 if ("directive".equals(codeString)) 347 return DIRECTIVE; 348 if ("order".equals(codeString)) 349 return ORDER; 350 if ("original-order".equals(codeString)) 351 return ORIGINALORDER; 352 if ("reflex-order".equals(codeString)) 353 return REFLEXORDER; 354 if ("filler-order".equals(codeString)) 355 return FILLERORDER; 356 if ("instance-order".equals(codeString)) 357 return INSTANCEORDER; 358 if ("option".equals(codeString)) 359 return OPTION; 360 if (Configuration.isAcceptInvalidEnums()) 361 return null; 362 else 363 throw new FHIRException("Unknown ServiceRequestIntent code '" + codeString + "'"); 364 } 365 366 public String toCode() { 367 switch (this) { 368 case PROPOSAL: 369 return "proposal"; 370 case PLAN: 371 return "plan"; 372 case DIRECTIVE: 373 return "directive"; 374 case ORDER: 375 return "order"; 376 case ORIGINALORDER: 377 return "original-order"; 378 case REFLEXORDER: 379 return "reflex-order"; 380 case FILLERORDER: 381 return "filler-order"; 382 case INSTANCEORDER: 383 return "instance-order"; 384 case OPTION: 385 return "option"; 386 case NULL: 387 return null; 388 default: 389 return "?"; 390 } 391 } 392 393 public String getSystem() { 394 switch (this) { 395 case PROPOSAL: 396 return "http://hl7.org/fhir/request-intent"; 397 case PLAN: 398 return "http://hl7.org/fhir/request-intent"; 399 case DIRECTIVE: 400 return "http://hl7.org/fhir/request-intent"; 401 case ORDER: 402 return "http://hl7.org/fhir/request-intent"; 403 case ORIGINALORDER: 404 return "http://hl7.org/fhir/request-intent"; 405 case REFLEXORDER: 406 return "http://hl7.org/fhir/request-intent"; 407 case FILLERORDER: 408 return "http://hl7.org/fhir/request-intent"; 409 case INSTANCEORDER: 410 return "http://hl7.org/fhir/request-intent"; 411 case OPTION: 412 return "http://hl7.org/fhir/request-intent"; 413 case NULL: 414 return null; 415 default: 416 return "?"; 417 } 418 } 419 420 public String getDefinition() { 421 switch (this) { 422 case PROPOSAL: 423 return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 424 case PLAN: 425 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 426 case DIRECTIVE: 427 return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 428 case ORDER: 429 return "The request represents a request/demand and authorization for action by a Practitioner."; 430 case ORIGINALORDER: 431 return "The request represents an original authorization for action."; 432 case REFLEXORDER: 433 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 434 case FILLERORDER: 435 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 436 case INSTANCEORDER: 437 return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 438 case OPTION: 439 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 440 case NULL: 441 return null; 442 default: 443 return "?"; 444 } 445 } 446 447 public String getDisplay() { 448 switch (this) { 449 case PROPOSAL: 450 return "Proposal"; 451 case PLAN: 452 return "Plan"; 453 case DIRECTIVE: 454 return "Directive"; 455 case ORDER: 456 return "Order"; 457 case ORIGINALORDER: 458 return "Original Order"; 459 case REFLEXORDER: 460 return "Reflex Order"; 461 case FILLERORDER: 462 return "Filler Order"; 463 case INSTANCEORDER: 464 return "Instance Order"; 465 case OPTION: 466 return "Option"; 467 case NULL: 468 return null; 469 default: 470 return "?"; 471 } 472 } 473 } 474 475 public static class ServiceRequestIntentEnumFactory implements EnumFactory<ServiceRequestIntent> { 476 public ServiceRequestIntent fromCode(String codeString) throws IllegalArgumentException { 477 if (codeString == null || "".equals(codeString)) 478 if (codeString == null || "".equals(codeString)) 479 return null; 480 if ("proposal".equals(codeString)) 481 return ServiceRequestIntent.PROPOSAL; 482 if ("plan".equals(codeString)) 483 return ServiceRequestIntent.PLAN; 484 if ("directive".equals(codeString)) 485 return ServiceRequestIntent.DIRECTIVE; 486 if ("order".equals(codeString)) 487 return ServiceRequestIntent.ORDER; 488 if ("original-order".equals(codeString)) 489 return ServiceRequestIntent.ORIGINALORDER; 490 if ("reflex-order".equals(codeString)) 491 return ServiceRequestIntent.REFLEXORDER; 492 if ("filler-order".equals(codeString)) 493 return ServiceRequestIntent.FILLERORDER; 494 if ("instance-order".equals(codeString)) 495 return ServiceRequestIntent.INSTANCEORDER; 496 if ("option".equals(codeString)) 497 return ServiceRequestIntent.OPTION; 498 throw new IllegalArgumentException("Unknown ServiceRequestIntent code '" + codeString + "'"); 499 } 500 501 public Enumeration<ServiceRequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 502 if (code == null) 503 return null; 504 if (code.isEmpty()) 505 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.NULL, code); 506 String codeString = code.asStringValue(); 507 if (codeString == null || "".equals(codeString)) 508 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.NULL, code); 509 if ("proposal".equals(codeString)) 510 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.PROPOSAL, code); 511 if ("plan".equals(codeString)) 512 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.PLAN, code); 513 if ("directive".equals(codeString)) 514 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.DIRECTIVE, code); 515 if ("order".equals(codeString)) 516 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.ORDER, code); 517 if ("original-order".equals(codeString)) 518 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.ORIGINALORDER, code); 519 if ("reflex-order".equals(codeString)) 520 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.REFLEXORDER, code); 521 if ("filler-order".equals(codeString)) 522 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.FILLERORDER, code); 523 if ("instance-order".equals(codeString)) 524 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.INSTANCEORDER, code); 525 if ("option".equals(codeString)) 526 return new Enumeration<ServiceRequestIntent>(this, ServiceRequestIntent.OPTION, code); 527 throw new FHIRException("Unknown ServiceRequestIntent code '" + codeString + "'"); 528 } 529 530 public String toCode(ServiceRequestIntent code) { 531 if (code == ServiceRequestIntent.NULL) 532 return null; 533 if (code == ServiceRequestIntent.PROPOSAL) 534 return "proposal"; 535 if (code == ServiceRequestIntent.PLAN) 536 return "plan"; 537 if (code == ServiceRequestIntent.DIRECTIVE) 538 return "directive"; 539 if (code == ServiceRequestIntent.ORDER) 540 return "order"; 541 if (code == ServiceRequestIntent.ORIGINALORDER) 542 return "original-order"; 543 if (code == ServiceRequestIntent.REFLEXORDER) 544 return "reflex-order"; 545 if (code == ServiceRequestIntent.FILLERORDER) 546 return "filler-order"; 547 if (code == ServiceRequestIntent.INSTANCEORDER) 548 return "instance-order"; 549 if (code == ServiceRequestIntent.OPTION) 550 return "option"; 551 return "?"; 552 } 553 554 public String toSystem(ServiceRequestIntent code) { 555 return code.getSystem(); 556 } 557 } 558 559 public enum ServiceRequestPriority { 560 /** 561 * The request has normal priority. 562 */ 563 ROUTINE, 564 /** 565 * The request should be actioned promptly - higher priority than routine. 566 */ 567 URGENT, 568 /** 569 * The request should be actioned as soon as possible - higher priority than 570 * urgent. 571 */ 572 ASAP, 573 /** 574 * The request should be actioned immediately - highest possible priority. E.g. 575 * an emergency. 576 */ 577 STAT, 578 /** 579 * added to help the parsers with the generic types 580 */ 581 NULL; 582 583 public static ServiceRequestPriority fromCode(String codeString) throws FHIRException { 584 if (codeString == null || "".equals(codeString)) 585 return null; 586 if ("routine".equals(codeString)) 587 return ROUTINE; 588 if ("urgent".equals(codeString)) 589 return URGENT; 590 if ("asap".equals(codeString)) 591 return ASAP; 592 if ("stat".equals(codeString)) 593 return STAT; 594 if (Configuration.isAcceptInvalidEnums()) 595 return null; 596 else 597 throw new FHIRException("Unknown ServiceRequestPriority code '" + codeString + "'"); 598 } 599 600 public String toCode() { 601 switch (this) { 602 case ROUTINE: 603 return "routine"; 604 case URGENT: 605 return "urgent"; 606 case ASAP: 607 return "asap"; 608 case STAT: 609 return "stat"; 610 case NULL: 611 return null; 612 default: 613 return "?"; 614 } 615 } 616 617 public String getSystem() { 618 switch (this) { 619 case ROUTINE: 620 return "http://hl7.org/fhir/request-priority"; 621 case URGENT: 622 return "http://hl7.org/fhir/request-priority"; 623 case ASAP: 624 return "http://hl7.org/fhir/request-priority"; 625 case STAT: 626 return "http://hl7.org/fhir/request-priority"; 627 case NULL: 628 return null; 629 default: 630 return "?"; 631 } 632 } 633 634 public String getDefinition() { 635 switch (this) { 636 case ROUTINE: 637 return "The request has normal priority."; 638 case URGENT: 639 return "The request should be actioned promptly - higher priority than routine."; 640 case ASAP: 641 return "The request should be actioned as soon as possible - higher priority than urgent."; 642 case STAT: 643 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 644 case NULL: 645 return null; 646 default: 647 return "?"; 648 } 649 } 650 651 public String getDisplay() { 652 switch (this) { 653 case ROUTINE: 654 return "Routine"; 655 case URGENT: 656 return "Urgent"; 657 case ASAP: 658 return "ASAP"; 659 case STAT: 660 return "STAT"; 661 case NULL: 662 return null; 663 default: 664 return "?"; 665 } 666 } 667 } 668 669 public static class ServiceRequestPriorityEnumFactory implements EnumFactory<ServiceRequestPriority> { 670 public ServiceRequestPriority fromCode(String codeString) throws IllegalArgumentException { 671 if (codeString == null || "".equals(codeString)) 672 if (codeString == null || "".equals(codeString)) 673 return null; 674 if ("routine".equals(codeString)) 675 return ServiceRequestPriority.ROUTINE; 676 if ("urgent".equals(codeString)) 677 return ServiceRequestPriority.URGENT; 678 if ("asap".equals(codeString)) 679 return ServiceRequestPriority.ASAP; 680 if ("stat".equals(codeString)) 681 return ServiceRequestPriority.STAT; 682 throw new IllegalArgumentException("Unknown ServiceRequestPriority code '" + codeString + "'"); 683 } 684 685 public Enumeration<ServiceRequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 686 if (code == null) 687 return null; 688 if (code.isEmpty()) 689 return new Enumeration<ServiceRequestPriority>(this, ServiceRequestPriority.NULL, code); 690 String codeString = code.asStringValue(); 691 if (codeString == null || "".equals(codeString)) 692 return new Enumeration<ServiceRequestPriority>(this, ServiceRequestPriority.NULL, code); 693 if ("routine".equals(codeString)) 694 return new Enumeration<ServiceRequestPriority>(this, ServiceRequestPriority.ROUTINE, code); 695 if ("urgent".equals(codeString)) 696 return new Enumeration<ServiceRequestPriority>(this, ServiceRequestPriority.URGENT, code); 697 if ("asap".equals(codeString)) 698 return new Enumeration<ServiceRequestPriority>(this, ServiceRequestPriority.ASAP, code); 699 if ("stat".equals(codeString)) 700 return new Enumeration<ServiceRequestPriority>(this, ServiceRequestPriority.STAT, code); 701 throw new FHIRException("Unknown ServiceRequestPriority code '" + codeString + "'"); 702 } 703 704 public String toCode(ServiceRequestPriority code) { 705 if (code == ServiceRequestPriority.NULL) 706 return null; 707 if (code == ServiceRequestPriority.ROUTINE) 708 return "routine"; 709 if (code == ServiceRequestPriority.URGENT) 710 return "urgent"; 711 if (code == ServiceRequestPriority.ASAP) 712 return "asap"; 713 if (code == ServiceRequestPriority.STAT) 714 return "stat"; 715 return "?"; 716 } 717 718 public String toSystem(ServiceRequestPriority code) { 719 return code.getSystem(); 720 } 721 } 722 723 /** 724 * Identifiers assigned to this order instance by the orderer and/or the 725 * receiver and/or order fulfiller. 726 */ 727 @Child(name = "identifier", type = { 728 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 729 @Description(shortDefinition = "Identifiers assigned to this order", formalDefinition = "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.") 730 protected List<Identifier> identifier; 731 732 /** 733 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other 734 * definition that is adhered to in whole or in part by this ServiceRequest. 735 */ 736 @Child(name = "instantiatesCanonical", type = { 737 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 738 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.") 739 protected List<CanonicalType> instantiatesCanonical; 740 741 /** 742 * The URL pointing to an externally maintained protocol, guideline, orderset or 743 * other definition that is adhered to in whole or in part by this 744 * ServiceRequest. 745 */ 746 @Child(name = "instantiatesUri", type = { 747 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 748 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.") 749 protected List<UriType> instantiatesUri; 750 751 /** 752 * Plan/proposal/order fulfilled by this request. 753 */ 754 @Child(name = "basedOn", type = { CarePlan.class, ServiceRequest.class, 755 MedicationRequest.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 756 @Description(shortDefinition = "What request fulfills", formalDefinition = "Plan/proposal/order fulfilled by this request.") 757 protected List<Reference> basedOn; 758 /** 759 * The actual objects that are the target of the reference (Plan/proposal/order 760 * fulfilled by this request.) 761 */ 762 protected List<Resource> basedOnTarget; 763 764 /** 765 * The request takes the place of the referenced completed or terminated 766 * request(s). 767 */ 768 @Child(name = "replaces", type = { 769 ServiceRequest.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 770 @Description(shortDefinition = "What request replaces", formalDefinition = "The request takes the place of the referenced completed or terminated request(s).") 771 protected List<Reference> replaces; 772 /** 773 * The actual objects that are the target of the reference (The request takes 774 * the place of the referenced completed or terminated request(s).) 775 */ 776 protected List<ServiceRequest> replacesTarget; 777 778 /** 779 * A shared identifier common to all service requests that were authorized more 780 * or less simultaneously by a single author, representing the composite or 781 * group identifier. 782 */ 783 @Child(name = "requisition", type = { 784 Identifier.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 785 @Description(shortDefinition = "Composite Request ID", formalDefinition = "A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.") 786 protected Identifier requisition; 787 788 /** 789 * The status of the order. 790 */ 791 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 792 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "The status of the order.") 793 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 794 protected Enumeration<ServiceRequestStatus> status; 795 796 /** 797 * Whether the request is a proposal, plan, an original order or a reflex order. 798 */ 799 @Child(name = "intent", type = { CodeType.class }, order = 7, min = 1, max = 1, modifier = true, summary = true) 800 @Description(shortDefinition = "proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Whether the request is a proposal, plan, an original order or a reflex order.") 801 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-intent") 802 protected Enumeration<ServiceRequestIntent> intent; 803 804 /** 805 * A code that classifies the service for searching, sorting and display 806 * purposes (e.g. "Surgical Procedure"). 807 */ 808 @Child(name = "category", type = { 809 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 810 @Description(shortDefinition = "Classification of service", formalDefinition = "A code that classifies the service for searching, sorting and display purposes (e.g. \"Surgical Procedure\").") 811 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/servicerequest-category") 812 protected List<CodeableConcept> category; 813 814 /** 815 * Indicates how quickly the ServiceRequest should be addressed with respect to 816 * other requests. 817 */ 818 @Child(name = "priority", type = { CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 819 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the ServiceRequest should be addressed with respect to other requests.") 820 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 821 protected Enumeration<ServiceRequestPriority> priority; 822 823 /** 824 * Set this to true if the record is saying that the service/procedure should 825 * NOT be performed. 826 */ 827 @Child(name = "doNotPerform", type = { 828 BooleanType.class }, order = 10, min = 0, max = 1, modifier = true, summary = true) 829 @Description(shortDefinition = "True if service/procedure should not be performed", formalDefinition = "Set this to true if the record is saying that the service/procedure should NOT be performed.") 830 protected BooleanType doNotPerform; 831 832 /** 833 * A code that identifies a particular service (i.e., procedure, diagnostic 834 * investigation, or panel of investigations) that have been requested. 835 */ 836 @Child(name = "code", type = { 837 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 838 @Description(shortDefinition = "What is being requested/ordered", formalDefinition = "A code that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested.") 839 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-code") 840 protected CodeableConcept code; 841 842 /** 843 * Additional details and instructions about the how the services are to be 844 * delivered. For example, and order for a urinary catheter may have an order 845 * detail for an external or indwelling catheter, or an order for a bandage may 846 * require additional instructions specifying how the bandage should be applied. 847 */ 848 @Child(name = "orderDetail", type = { 849 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 850 @Description(shortDefinition = "Additional order information", formalDefinition = "Additional details and instructions about the how the services are to be delivered. For example, and order for a urinary catheter may have an order detail for an external or indwelling catheter, or an order for a bandage may require additional instructions specifying how the bandage should be applied.") 851 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/servicerequest-orderdetail") 852 protected List<CodeableConcept> orderDetail; 853 854 /** 855 * An amount of service being requested which can be a quantity ( for example 856 * $1,500 home modification), a ratio ( for example, 20 half day visits per 857 * month), or a range (2.0 to 1.8 Gy per fraction). 858 */ 859 @Child(name = "quantity", type = { Quantity.class, Ratio.class, 860 Range.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 861 @Description(shortDefinition = "Service amount", formalDefinition = "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).") 862 protected Type quantity; 863 864 /** 865 * On whom or what the service is to be performed. This is usually a human 866 * patient, but can also be requested on animals, groups of humans or animals, 867 * devices such as dialysis machines, or even locations (typically for 868 * environmental scans). 869 */ 870 @Child(name = "subject", type = { Patient.class, Group.class, Location.class, 871 Device.class }, order = 14, min = 1, max = 1, modifier = false, summary = true) 872 @Description(shortDefinition = "Individual or Entity the service is ordered for", formalDefinition = "On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).") 873 protected Reference subject; 874 875 /** 876 * The actual object that is the target of the reference (On whom or what the 877 * service is to be performed. This is usually a human patient, but can also be 878 * requested on animals, groups of humans or animals, devices such as dialysis 879 * machines, or even locations (typically for environmental scans).) 880 */ 881 protected Resource subjectTarget; 882 883 /** 884 * An encounter that provides additional information about the healthcare 885 * context in which this request is made. 886 */ 887 @Child(name = "encounter", type = { Encounter.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 888 @Description(shortDefinition = "Encounter in which the request was created", formalDefinition = "An encounter that provides additional information about the healthcare context in which this request is made.") 889 protected Reference encounter; 890 891 /** 892 * The actual object that is the target of the reference (An encounter that 893 * provides additional information about the healthcare context in which this 894 * request is made.) 895 */ 896 protected Encounter encounterTarget; 897 898 /** 899 * The date/time at which the requested service should occur. 900 */ 901 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 902 Timing.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 903 @Description(shortDefinition = "When service should occur", formalDefinition = "The date/time at which the requested service should occur.") 904 protected Type occurrence; 905 906 /** 907 * If a CodeableConcept is present, it indicates the pre-condition for 908 * performing the service. For example "pain", "on flare-up", etc. 909 */ 910 @Child(name = "asNeeded", type = { BooleanType.class, 911 CodeableConcept.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 912 @Description(shortDefinition = "Preconditions for service", formalDefinition = "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.") 913 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 914 protected Type asNeeded; 915 916 /** 917 * When the request transitioned to being actionable. 918 */ 919 @Child(name = "authoredOn", type = { 920 DateTimeType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 921 @Description(shortDefinition = "Date request signed", formalDefinition = "When the request transitioned to being actionable.") 922 protected DateTimeType authoredOn; 923 924 /** 925 * The individual who initiated the request and has responsibility for its 926 * activation. 927 */ 928 @Child(name = "requester", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 929 RelatedPerson.class, Device.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 930 @Description(shortDefinition = "Who/what is requesting service", formalDefinition = "The individual who initiated the request and has responsibility for its activation.") 931 protected Reference requester; 932 933 /** 934 * The actual object that is the target of the reference (The individual who 935 * initiated the request and has responsibility for its activation.) 936 */ 937 protected Resource requesterTarget; 938 939 /** 940 * Desired type of performer for doing the requested service. 941 */ 942 @Child(name = "performerType", type = { 943 CodeableConcept.class }, order = 20, min = 0, max = 1, modifier = false, summary = true) 944 @Description(shortDefinition = "Performer role", formalDefinition = "Desired type of performer for doing the requested service.") 945 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/participant-role") 946 protected CodeableConcept performerType; 947 948 /** 949 * The desired performer for doing the requested service. For example, the 950 * surgeon, dermatopathologist, endoscopist, etc. 951 */ 952 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 953 HealthcareService.class, Patient.class, Device.class, 954 RelatedPerson.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 955 @Description(shortDefinition = "Requested performer", formalDefinition = "The desired performer for doing the requested service. For example, the surgeon, dermatopathologist, endoscopist, etc.") 956 protected List<Reference> performer; 957 /** 958 * The actual objects that are the target of the reference (The desired 959 * performer for doing the requested service. For example, the surgeon, 960 * dermatopathologist, endoscopist, etc.) 961 */ 962 protected List<Resource> performerTarget; 963 964 /** 965 * The preferred location(s) where the procedure should actually happen in coded 966 * or free text form. E.g. at home or nursing day care center. 967 */ 968 @Child(name = "locationCode", type = { 969 CodeableConcept.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 970 @Description(shortDefinition = "Requested location", formalDefinition = "The preferred location(s) where the procedure should actually happen in coded or free text form. E.g. at home or nursing day care center.") 971 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ServiceDeliveryLocationRoleType") 972 protected List<CodeableConcept> locationCode; 973 974 /** 975 * A reference to the the preferred location(s) where the procedure should 976 * actually happen. E.g. at home or nursing day care center. 977 */ 978 @Child(name = "locationReference", type = { 979 Location.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 980 @Description(shortDefinition = "Requested location", formalDefinition = "A reference to the the preferred location(s) where the procedure should actually happen. E.g. at home or nursing day care center.") 981 protected List<Reference> locationReference; 982 /** 983 * The actual objects that are the target of the reference (A reference to the 984 * the preferred location(s) where the procedure should actually happen. E.g. at 985 * home or nursing day care center.) 986 */ 987 protected List<Location> locationReferenceTarget; 988 989 /** 990 * An explanation or justification for why this service is being requested in 991 * coded or textual form. This is often for billing purposes. May relate to the 992 * resources referred to in `supportingInfo`. 993 */ 994 @Child(name = "reasonCode", type = { 995 CodeableConcept.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 996 @Description(shortDefinition = "Explanation/Justification for procedure or service", formalDefinition = "An explanation or justification for why this service is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in `supportingInfo`.") 997 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-reason") 998 protected List<CodeableConcept> reasonCode; 999 1000 /** 1001 * Indicates another resource that provides a justification for why this service 1002 * is being requested. May relate to the resources referred to in 1003 * `supportingInfo`. 1004 */ 1005 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 1006 DocumentReference.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1007 @Description(shortDefinition = "Explanation/Justification for service or service", formalDefinition = "Indicates another resource that provides a justification for why this service is being requested. May relate to the resources referred to in `supportingInfo`.") 1008 protected List<Reference> reasonReference; 1009 /** 1010 * The actual objects that are the target of the reference (Indicates another 1011 * resource that provides a justification for why this service is being 1012 * requested. May relate to the resources referred to in `supportingInfo`.) 1013 */ 1014 protected List<Resource> reasonReferenceTarget; 1015 1016 /** 1017 * Insurance plans, coverage extensions, pre-authorizations and/or 1018 * pre-determinations that may be needed for delivering the requested service. 1019 */ 1020 @Child(name = "insurance", type = { Coverage.class, 1021 ClaimResponse.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1022 @Description(shortDefinition = "Associated insurance coverage", formalDefinition = "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be needed for delivering the requested service.") 1023 protected List<Reference> insurance; 1024 /** 1025 * The actual objects that are the target of the reference (Insurance plans, 1026 * coverage extensions, pre-authorizations and/or pre-determinations that may be 1027 * needed for delivering the requested service.) 1028 */ 1029 protected List<Resource> insuranceTarget; 1030 1031 /** 1032 * Additional clinical information about the patient or specimen that may 1033 * influence the services or their interpretations. This information includes 1034 * diagnosis, clinical findings and other observations. In laboratory ordering 1035 * these are typically referred to as "ask at order entry questions (AOEs)". 1036 * This includes observations explicitly requested by the producer (filler) to 1037 * provide context or supporting information needed to complete the order. For 1038 * example, reporting the amount of inspired oxygen for blood gas measurements. 1039 */ 1040 @Child(name = "supportingInfo", type = { 1041 Reference.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1042 @Description(shortDefinition = "Additional clinical information", formalDefinition = "Additional clinical information about the patient or specimen that may influence the services or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.") 1043 protected List<Reference> supportingInfo; 1044 /** 1045 * The actual objects that are the target of the reference (Additional clinical 1046 * information about the patient or specimen that may influence the services or 1047 * their interpretations. This information includes diagnosis, clinical findings 1048 * and other observations. In laboratory ordering these are typically referred 1049 * to as "ask at order entry questions (AOEs)". This includes observations 1050 * explicitly requested by the producer (filler) to provide context or 1051 * supporting information needed to complete the order. For example, reporting 1052 * the amount of inspired oxygen for blood gas measurements.) 1053 */ 1054 protected List<Resource> supportingInfoTarget; 1055 1056 /** 1057 * One or more specimens that the laboratory procedure will use. 1058 */ 1059 @Child(name = "specimen", type = { 1060 Specimen.class }, order = 28, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1061 @Description(shortDefinition = "Procedure Samples", formalDefinition = "One or more specimens that the laboratory procedure will use.") 1062 protected List<Reference> specimen; 1063 /** 1064 * The actual objects that are the target of the reference (One or more 1065 * specimens that the laboratory procedure will use.) 1066 */ 1067 protected List<Specimen> specimenTarget; 1068 1069 /** 1070 * Anatomic location where the procedure should be performed. This is the target 1071 * site. 1072 */ 1073 @Child(name = "bodySite", type = { 1074 CodeableConcept.class }, order = 29, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1075 @Description(shortDefinition = "Location on Body", formalDefinition = "Anatomic location where the procedure should be performed. This is the target site.") 1076 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 1077 protected List<CodeableConcept> bodySite; 1078 1079 /** 1080 * Any other notes and comments made about the service request. For example, 1081 * internal billing notes. 1082 */ 1083 @Child(name = "note", type = { 1084 Annotation.class }, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1085 @Description(shortDefinition = "Comments", formalDefinition = "Any other notes and comments made about the service request. For example, internal billing notes.") 1086 protected List<Annotation> note; 1087 1088 /** 1089 * Instructions in terms that are understood by the patient or consumer. 1090 */ 1091 @Child(name = "patientInstruction", type = { 1092 StringType.class }, order = 31, min = 0, max = 1, modifier = false, summary = true) 1093 @Description(shortDefinition = "Patient or consumer-oriented instructions", formalDefinition = "Instructions in terms that are understood by the patient or consumer.") 1094 protected StringType patientInstruction; 1095 1096 /** 1097 * Key events in the history of the request. 1098 */ 1099 @Child(name = "relevantHistory", type = { 1100 Provenance.class }, order = 32, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1101 @Description(shortDefinition = "Request provenance", formalDefinition = "Key events in the history of the request.") 1102 protected List<Reference> relevantHistory; 1103 /** 1104 * The actual objects that are the target of the reference (Key events in the 1105 * history of the request.) 1106 */ 1107 protected List<Provenance> relevantHistoryTarget; 1108 1109 private static final long serialVersionUID = -1202335045L; 1110 1111 /** 1112 * Constructor 1113 */ 1114 public ServiceRequest() { 1115 super(); 1116 } 1117 1118 /** 1119 * Constructor 1120 */ 1121 public ServiceRequest(Enumeration<ServiceRequestStatus> status, Enumeration<ServiceRequestIntent> intent, 1122 Reference subject) { 1123 super(); 1124 this.status = status; 1125 this.intent = intent; 1126 this.subject = subject; 1127 } 1128 1129 /** 1130 * @return {@link #identifier} (Identifiers assigned to this order instance by 1131 * the orderer and/or the receiver and/or order fulfiller.) 1132 */ 1133 public List<Identifier> getIdentifier() { 1134 if (this.identifier == null) 1135 this.identifier = new ArrayList<Identifier>(); 1136 return this.identifier; 1137 } 1138 1139 /** 1140 * @return Returns a reference to <code>this</code> for easy method chaining 1141 */ 1142 public ServiceRequest setIdentifier(List<Identifier> theIdentifier) { 1143 this.identifier = theIdentifier; 1144 return this; 1145 } 1146 1147 public boolean hasIdentifier() { 1148 if (this.identifier == null) 1149 return false; 1150 for (Identifier item : this.identifier) 1151 if (!item.isEmpty()) 1152 return true; 1153 return false; 1154 } 1155 1156 public Identifier addIdentifier() { // 3 1157 Identifier t = new Identifier(); 1158 if (this.identifier == null) 1159 this.identifier = new ArrayList<Identifier>(); 1160 this.identifier.add(t); 1161 return t; 1162 } 1163 1164 public ServiceRequest addIdentifier(Identifier t) { // 3 1165 if (t == null) 1166 return this; 1167 if (this.identifier == null) 1168 this.identifier = new ArrayList<Identifier>(); 1169 this.identifier.add(t); 1170 return this; 1171 } 1172 1173 /** 1174 * @return The first repetition of repeating field {@link #identifier}, creating 1175 * it if it does not already exist 1176 */ 1177 public Identifier getIdentifierFirstRep() { 1178 if (getIdentifier().isEmpty()) { 1179 addIdentifier(); 1180 } 1181 return getIdentifier().get(0); 1182 } 1183 1184 /** 1185 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1186 * protocol, guideline, orderset or other definition that is adhered to 1187 * in whole or in part by this ServiceRequest.) 1188 */ 1189 public List<CanonicalType> getInstantiatesCanonical() { 1190 if (this.instantiatesCanonical == null) 1191 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1192 return this.instantiatesCanonical; 1193 } 1194 1195 /** 1196 * @return Returns a reference to <code>this</code> for easy method chaining 1197 */ 1198 public ServiceRequest setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1199 this.instantiatesCanonical = theInstantiatesCanonical; 1200 return this; 1201 } 1202 1203 public boolean hasInstantiatesCanonical() { 1204 if (this.instantiatesCanonical == null) 1205 return false; 1206 for (CanonicalType item : this.instantiatesCanonical) 1207 if (!item.isEmpty()) 1208 return true; 1209 return false; 1210 } 1211 1212 /** 1213 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1214 * protocol, guideline, orderset or other definition that is adhered to 1215 * in whole or in part by this ServiceRequest.) 1216 */ 1217 public CanonicalType addInstantiatesCanonicalElement() {// 2 1218 CanonicalType t = new CanonicalType(); 1219 if (this.instantiatesCanonical == null) 1220 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1221 this.instantiatesCanonical.add(t); 1222 return t; 1223 } 1224 1225 /** 1226 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1227 * FHIR-defined protocol, guideline, orderset or other definition 1228 * that is adhered to in whole or in part by this ServiceRequest.) 1229 */ 1230 public ServiceRequest addInstantiatesCanonical(String value) { // 1 1231 CanonicalType t = new CanonicalType(); 1232 t.setValue(value); 1233 if (this.instantiatesCanonical == null) 1234 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1235 this.instantiatesCanonical.add(t); 1236 return this; 1237 } 1238 1239 /** 1240 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1241 * FHIR-defined protocol, guideline, orderset or other definition 1242 * that is adhered to in whole or in part by this ServiceRequest.) 1243 */ 1244 public boolean hasInstantiatesCanonical(String value) { 1245 if (this.instantiatesCanonical == null) 1246 return false; 1247 for (CanonicalType v : this.instantiatesCanonical) 1248 if (v.getValue().equals(value)) // canonical(ActivityDefinition|PlanDefinition) 1249 return true; 1250 return false; 1251 } 1252 1253 /** 1254 * @return {@link #instantiatesUri} (The URL pointing to an externally 1255 * maintained protocol, guideline, orderset or other definition that is 1256 * adhered to in whole or in part by this ServiceRequest.) 1257 */ 1258 public List<UriType> getInstantiatesUri() { 1259 if (this.instantiatesUri == null) 1260 this.instantiatesUri = new ArrayList<UriType>(); 1261 return this.instantiatesUri; 1262 } 1263 1264 /** 1265 * @return Returns a reference to <code>this</code> for easy method chaining 1266 */ 1267 public ServiceRequest setInstantiatesUri(List<UriType> theInstantiatesUri) { 1268 this.instantiatesUri = theInstantiatesUri; 1269 return this; 1270 } 1271 1272 public boolean hasInstantiatesUri() { 1273 if (this.instantiatesUri == null) 1274 return false; 1275 for (UriType item : this.instantiatesUri) 1276 if (!item.isEmpty()) 1277 return true; 1278 return false; 1279 } 1280 1281 /** 1282 * @return {@link #instantiatesUri} (The URL pointing to an externally 1283 * maintained protocol, guideline, orderset or other definition that is 1284 * adhered to in whole or in part by this ServiceRequest.) 1285 */ 1286 public UriType addInstantiatesUriElement() {// 2 1287 UriType t = new UriType(); 1288 if (this.instantiatesUri == null) 1289 this.instantiatesUri = new ArrayList<UriType>(); 1290 this.instantiatesUri.add(t); 1291 return t; 1292 } 1293 1294 /** 1295 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1296 * maintained protocol, guideline, orderset or other definition 1297 * that is adhered to in whole or in part by this ServiceRequest.) 1298 */ 1299 public ServiceRequest addInstantiatesUri(String value) { // 1 1300 UriType t = new UriType(); 1301 t.setValue(value); 1302 if (this.instantiatesUri == null) 1303 this.instantiatesUri = new ArrayList<UriType>(); 1304 this.instantiatesUri.add(t); 1305 return this; 1306 } 1307 1308 /** 1309 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1310 * maintained protocol, guideline, orderset or other definition 1311 * that is adhered to in whole or in part by this ServiceRequest.) 1312 */ 1313 public boolean hasInstantiatesUri(String value) { 1314 if (this.instantiatesUri == null) 1315 return false; 1316 for (UriType v : this.instantiatesUri) 1317 if (v.getValue().equals(value)) // uri 1318 return true; 1319 return false; 1320 } 1321 1322 /** 1323 * @return {@link #basedOn} (Plan/proposal/order fulfilled by this request.) 1324 */ 1325 public List<Reference> getBasedOn() { 1326 if (this.basedOn == null) 1327 this.basedOn = new ArrayList<Reference>(); 1328 return this.basedOn; 1329 } 1330 1331 /** 1332 * @return Returns a reference to <code>this</code> for easy method chaining 1333 */ 1334 public ServiceRequest setBasedOn(List<Reference> theBasedOn) { 1335 this.basedOn = theBasedOn; 1336 return this; 1337 } 1338 1339 public boolean hasBasedOn() { 1340 if (this.basedOn == null) 1341 return false; 1342 for (Reference item : this.basedOn) 1343 if (!item.isEmpty()) 1344 return true; 1345 return false; 1346 } 1347 1348 public Reference addBasedOn() { // 3 1349 Reference t = new Reference(); 1350 if (this.basedOn == null) 1351 this.basedOn = new ArrayList<Reference>(); 1352 this.basedOn.add(t); 1353 return t; 1354 } 1355 1356 public ServiceRequest addBasedOn(Reference t) { // 3 1357 if (t == null) 1358 return this; 1359 if (this.basedOn == null) 1360 this.basedOn = new ArrayList<Reference>(); 1361 this.basedOn.add(t); 1362 return this; 1363 } 1364 1365 /** 1366 * @return The first repetition of repeating field {@link #basedOn}, creating it 1367 * if it does not already exist 1368 */ 1369 public Reference getBasedOnFirstRep() { 1370 if (getBasedOn().isEmpty()) { 1371 addBasedOn(); 1372 } 1373 return getBasedOn().get(0); 1374 } 1375 1376 /** 1377 * @deprecated Use Reference#setResource(IBaseResource) instead 1378 */ 1379 @Deprecated 1380 public List<Resource> getBasedOnTarget() { 1381 if (this.basedOnTarget == null) 1382 this.basedOnTarget = new ArrayList<Resource>(); 1383 return this.basedOnTarget; 1384 } 1385 1386 /** 1387 * @return {@link #replaces} (The request takes the place of the referenced 1388 * completed or terminated request(s).) 1389 */ 1390 public List<Reference> getReplaces() { 1391 if (this.replaces == null) 1392 this.replaces = new ArrayList<Reference>(); 1393 return this.replaces; 1394 } 1395 1396 /** 1397 * @return Returns a reference to <code>this</code> for easy method chaining 1398 */ 1399 public ServiceRequest setReplaces(List<Reference> theReplaces) { 1400 this.replaces = theReplaces; 1401 return this; 1402 } 1403 1404 public boolean hasReplaces() { 1405 if (this.replaces == null) 1406 return false; 1407 for (Reference item : this.replaces) 1408 if (!item.isEmpty()) 1409 return true; 1410 return false; 1411 } 1412 1413 public Reference addReplaces() { // 3 1414 Reference t = new Reference(); 1415 if (this.replaces == null) 1416 this.replaces = new ArrayList<Reference>(); 1417 this.replaces.add(t); 1418 return t; 1419 } 1420 1421 public ServiceRequest addReplaces(Reference t) { // 3 1422 if (t == null) 1423 return this; 1424 if (this.replaces == null) 1425 this.replaces = new ArrayList<Reference>(); 1426 this.replaces.add(t); 1427 return this; 1428 } 1429 1430 /** 1431 * @return The first repetition of repeating field {@link #replaces}, creating 1432 * it if it does not already exist 1433 */ 1434 public Reference getReplacesFirstRep() { 1435 if (getReplaces().isEmpty()) { 1436 addReplaces(); 1437 } 1438 return getReplaces().get(0); 1439 } 1440 1441 /** 1442 * @deprecated Use Reference#setResource(IBaseResource) instead 1443 */ 1444 @Deprecated 1445 public List<ServiceRequest> getReplacesTarget() { 1446 if (this.replacesTarget == null) 1447 this.replacesTarget = new ArrayList<ServiceRequest>(); 1448 return this.replacesTarget; 1449 } 1450 1451 /** 1452 * @deprecated Use Reference#setResource(IBaseResource) instead 1453 */ 1454 @Deprecated 1455 public ServiceRequest addReplacesTarget() { 1456 ServiceRequest r = new ServiceRequest(); 1457 if (this.replacesTarget == null) 1458 this.replacesTarget = new ArrayList<ServiceRequest>(); 1459 this.replacesTarget.add(r); 1460 return r; 1461 } 1462 1463 /** 1464 * @return {@link #requisition} (A shared identifier common to all service 1465 * requests that were authorized more or less simultaneously by a single 1466 * author, representing the composite or group identifier.) 1467 */ 1468 public Identifier getRequisition() { 1469 if (this.requisition == null) 1470 if (Configuration.errorOnAutoCreate()) 1471 throw new Error("Attempt to auto-create ServiceRequest.requisition"); 1472 else if (Configuration.doAutoCreate()) 1473 this.requisition = new Identifier(); // cc 1474 return this.requisition; 1475 } 1476 1477 public boolean hasRequisition() { 1478 return this.requisition != null && !this.requisition.isEmpty(); 1479 } 1480 1481 /** 1482 * @param value {@link #requisition} (A shared identifier common to all service 1483 * requests that were authorized more or less simultaneously by a 1484 * single author, representing the composite or group identifier.) 1485 */ 1486 public ServiceRequest setRequisition(Identifier value) { 1487 this.requisition = value; 1488 return this; 1489 } 1490 1491 /** 1492 * @return {@link #status} (The status of the order.). This is the underlying 1493 * object with id, value and extensions. The accessor "getStatus" gives 1494 * direct access to the value 1495 */ 1496 public Enumeration<ServiceRequestStatus> getStatusElement() { 1497 if (this.status == null) 1498 if (Configuration.errorOnAutoCreate()) 1499 throw new Error("Attempt to auto-create ServiceRequest.status"); 1500 else if (Configuration.doAutoCreate()) 1501 this.status = new Enumeration<ServiceRequestStatus>(new ServiceRequestStatusEnumFactory()); // bb 1502 return this.status; 1503 } 1504 1505 public boolean hasStatusElement() { 1506 return this.status != null && !this.status.isEmpty(); 1507 } 1508 1509 public boolean hasStatus() { 1510 return this.status != null && !this.status.isEmpty(); 1511 } 1512 1513 /** 1514 * @param value {@link #status} (The status of the order.). This is the 1515 * underlying object with id, value and extensions. The accessor 1516 * "getStatus" gives direct access to the value 1517 */ 1518 public ServiceRequest setStatusElement(Enumeration<ServiceRequestStatus> value) { 1519 this.status = value; 1520 return this; 1521 } 1522 1523 /** 1524 * @return The status of the order. 1525 */ 1526 public ServiceRequestStatus getStatus() { 1527 return this.status == null ? null : this.status.getValue(); 1528 } 1529 1530 /** 1531 * @param value The status of the order. 1532 */ 1533 public ServiceRequest setStatus(ServiceRequestStatus value) { 1534 if (this.status == null) 1535 this.status = new Enumeration<ServiceRequestStatus>(new ServiceRequestStatusEnumFactory()); 1536 this.status.setValue(value); 1537 return this; 1538 } 1539 1540 /** 1541 * @return {@link #intent} (Whether the request is a proposal, plan, an original 1542 * order or a reflex order.). This is the underlying object with id, 1543 * value and extensions. The accessor "getIntent" gives direct access to 1544 * the value 1545 */ 1546 public Enumeration<ServiceRequestIntent> getIntentElement() { 1547 if (this.intent == null) 1548 if (Configuration.errorOnAutoCreate()) 1549 throw new Error("Attempt to auto-create ServiceRequest.intent"); 1550 else if (Configuration.doAutoCreate()) 1551 this.intent = new Enumeration<ServiceRequestIntent>(new ServiceRequestIntentEnumFactory()); // bb 1552 return this.intent; 1553 } 1554 1555 public boolean hasIntentElement() { 1556 return this.intent != null && !this.intent.isEmpty(); 1557 } 1558 1559 public boolean hasIntent() { 1560 return this.intent != null && !this.intent.isEmpty(); 1561 } 1562 1563 /** 1564 * @param value {@link #intent} (Whether the request is a proposal, plan, an 1565 * original order or a reflex order.). This is the underlying 1566 * object with id, value and extensions. The accessor "getIntent" 1567 * gives direct access to the value 1568 */ 1569 public ServiceRequest setIntentElement(Enumeration<ServiceRequestIntent> value) { 1570 this.intent = value; 1571 return this; 1572 } 1573 1574 /** 1575 * @return Whether the request is a proposal, plan, an original order or a 1576 * reflex order. 1577 */ 1578 public ServiceRequestIntent getIntent() { 1579 return this.intent == null ? null : this.intent.getValue(); 1580 } 1581 1582 /** 1583 * @param value Whether the request is a proposal, plan, an original order or a 1584 * reflex order. 1585 */ 1586 public ServiceRequest setIntent(ServiceRequestIntent value) { 1587 if (this.intent == null) 1588 this.intent = new Enumeration<ServiceRequestIntent>(new ServiceRequestIntentEnumFactory()); 1589 this.intent.setValue(value); 1590 return this; 1591 } 1592 1593 /** 1594 * @return {@link #category} (A code that classifies the service for searching, 1595 * sorting and display purposes (e.g. "Surgical Procedure").) 1596 */ 1597 public List<CodeableConcept> getCategory() { 1598 if (this.category == null) 1599 this.category = new ArrayList<CodeableConcept>(); 1600 return this.category; 1601 } 1602 1603 /** 1604 * @return Returns a reference to <code>this</code> for easy method chaining 1605 */ 1606 public ServiceRequest setCategory(List<CodeableConcept> theCategory) { 1607 this.category = theCategory; 1608 return this; 1609 } 1610 1611 public boolean hasCategory() { 1612 if (this.category == null) 1613 return false; 1614 for (CodeableConcept item : this.category) 1615 if (!item.isEmpty()) 1616 return true; 1617 return false; 1618 } 1619 1620 public CodeableConcept addCategory() { // 3 1621 CodeableConcept t = new CodeableConcept(); 1622 if (this.category == null) 1623 this.category = new ArrayList<CodeableConcept>(); 1624 this.category.add(t); 1625 return t; 1626 } 1627 1628 public ServiceRequest addCategory(CodeableConcept t) { // 3 1629 if (t == null) 1630 return this; 1631 if (this.category == null) 1632 this.category = new ArrayList<CodeableConcept>(); 1633 this.category.add(t); 1634 return this; 1635 } 1636 1637 /** 1638 * @return The first repetition of repeating field {@link #category}, creating 1639 * it if it does not already exist 1640 */ 1641 public CodeableConcept getCategoryFirstRep() { 1642 if (getCategory().isEmpty()) { 1643 addCategory(); 1644 } 1645 return getCategory().get(0); 1646 } 1647 1648 /** 1649 * @return {@link #priority} (Indicates how quickly the ServiceRequest should be 1650 * addressed with respect to other requests.). This is the underlying 1651 * object with id, value and extensions. The accessor "getPriority" 1652 * gives direct access to the value 1653 */ 1654 public Enumeration<ServiceRequestPriority> getPriorityElement() { 1655 if (this.priority == null) 1656 if (Configuration.errorOnAutoCreate()) 1657 throw new Error("Attempt to auto-create ServiceRequest.priority"); 1658 else if (Configuration.doAutoCreate()) 1659 this.priority = new Enumeration<ServiceRequestPriority>(new ServiceRequestPriorityEnumFactory()); // bb 1660 return this.priority; 1661 } 1662 1663 public boolean hasPriorityElement() { 1664 return this.priority != null && !this.priority.isEmpty(); 1665 } 1666 1667 public boolean hasPriority() { 1668 return this.priority != null && !this.priority.isEmpty(); 1669 } 1670 1671 /** 1672 * @param value {@link #priority} (Indicates how quickly the ServiceRequest 1673 * should be addressed with respect to other requests.). This is 1674 * the underlying object with id, value and extensions. The 1675 * accessor "getPriority" gives direct access to the value 1676 */ 1677 public ServiceRequest setPriorityElement(Enumeration<ServiceRequestPriority> value) { 1678 this.priority = value; 1679 return this; 1680 } 1681 1682 /** 1683 * @return Indicates how quickly the ServiceRequest should be addressed with 1684 * respect to other requests. 1685 */ 1686 public ServiceRequestPriority getPriority() { 1687 return this.priority == null ? null : this.priority.getValue(); 1688 } 1689 1690 /** 1691 * @param value Indicates how quickly the ServiceRequest should be addressed 1692 * with respect to other requests. 1693 */ 1694 public ServiceRequest setPriority(ServiceRequestPriority value) { 1695 if (value == null) 1696 this.priority = null; 1697 else { 1698 if (this.priority == null) 1699 this.priority = new Enumeration<ServiceRequestPriority>(new ServiceRequestPriorityEnumFactory()); 1700 this.priority.setValue(value); 1701 } 1702 return this; 1703 } 1704 1705 /** 1706 * @return {@link #doNotPerform} (Set this to true if the record is saying that 1707 * the service/procedure should NOT be performed.). This is the 1708 * underlying object with id, value and extensions. The accessor 1709 * "getDoNotPerform" gives direct access to the value 1710 */ 1711 public BooleanType getDoNotPerformElement() { 1712 if (this.doNotPerform == null) 1713 if (Configuration.errorOnAutoCreate()) 1714 throw new Error("Attempt to auto-create ServiceRequest.doNotPerform"); 1715 else if (Configuration.doAutoCreate()) 1716 this.doNotPerform = new BooleanType(); // bb 1717 return this.doNotPerform; 1718 } 1719 1720 public boolean hasDoNotPerformElement() { 1721 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1722 } 1723 1724 public boolean hasDoNotPerform() { 1725 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1726 } 1727 1728 /** 1729 * @param value {@link #doNotPerform} (Set this to true if the record is saying 1730 * that the service/procedure should NOT be performed.). This is 1731 * the underlying object with id, value and extensions. The 1732 * accessor "getDoNotPerform" gives direct access to the value 1733 */ 1734 public ServiceRequest setDoNotPerformElement(BooleanType value) { 1735 this.doNotPerform = value; 1736 return this; 1737 } 1738 1739 /** 1740 * @return Set this to true if the record is saying that the service/procedure 1741 * should NOT be performed. 1742 */ 1743 public boolean getDoNotPerform() { 1744 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 1745 } 1746 1747 /** 1748 * @param value Set this to true if the record is saying that the 1749 * service/procedure should NOT be performed. 1750 */ 1751 public ServiceRequest setDoNotPerform(boolean value) { 1752 if (this.doNotPerform == null) 1753 this.doNotPerform = new BooleanType(); 1754 this.doNotPerform.setValue(value); 1755 return this; 1756 } 1757 1758 /** 1759 * @return {@link #code} (A code that identifies a particular service (i.e., 1760 * procedure, diagnostic investigation, or panel of investigations) that 1761 * have been requested.) 1762 */ 1763 public CodeableConcept getCode() { 1764 if (this.code == null) 1765 if (Configuration.errorOnAutoCreate()) 1766 throw new Error("Attempt to auto-create ServiceRequest.code"); 1767 else if (Configuration.doAutoCreate()) 1768 this.code = new CodeableConcept(); // cc 1769 return this.code; 1770 } 1771 1772 public boolean hasCode() { 1773 return this.code != null && !this.code.isEmpty(); 1774 } 1775 1776 /** 1777 * @param value {@link #code} (A code that identifies a particular service 1778 * (i.e., procedure, diagnostic investigation, or panel of 1779 * investigations) that have been requested.) 1780 */ 1781 public ServiceRequest setCode(CodeableConcept value) { 1782 this.code = value; 1783 return this; 1784 } 1785 1786 /** 1787 * @return {@link #orderDetail} (Additional details and instructions about the 1788 * how the services are to be delivered. For example, and order for a 1789 * urinary catheter may have an order detail for an external or 1790 * indwelling catheter, or an order for a bandage may require additional 1791 * instructions specifying how the bandage should be applied.) 1792 */ 1793 public List<CodeableConcept> getOrderDetail() { 1794 if (this.orderDetail == null) 1795 this.orderDetail = new ArrayList<CodeableConcept>(); 1796 return this.orderDetail; 1797 } 1798 1799 /** 1800 * @return Returns a reference to <code>this</code> for easy method chaining 1801 */ 1802 public ServiceRequest setOrderDetail(List<CodeableConcept> theOrderDetail) { 1803 this.orderDetail = theOrderDetail; 1804 return this; 1805 } 1806 1807 public boolean hasOrderDetail() { 1808 if (this.orderDetail == null) 1809 return false; 1810 for (CodeableConcept item : this.orderDetail) 1811 if (!item.isEmpty()) 1812 return true; 1813 return false; 1814 } 1815 1816 public CodeableConcept addOrderDetail() { // 3 1817 CodeableConcept t = new CodeableConcept(); 1818 if (this.orderDetail == null) 1819 this.orderDetail = new ArrayList<CodeableConcept>(); 1820 this.orderDetail.add(t); 1821 return t; 1822 } 1823 1824 public ServiceRequest addOrderDetail(CodeableConcept t) { // 3 1825 if (t == null) 1826 return this; 1827 if (this.orderDetail == null) 1828 this.orderDetail = new ArrayList<CodeableConcept>(); 1829 this.orderDetail.add(t); 1830 return this; 1831 } 1832 1833 /** 1834 * @return The first repetition of repeating field {@link #orderDetail}, 1835 * creating it if it does not already exist 1836 */ 1837 public CodeableConcept getOrderDetailFirstRep() { 1838 if (getOrderDetail().isEmpty()) { 1839 addOrderDetail(); 1840 } 1841 return getOrderDetail().get(0); 1842 } 1843 1844 /** 1845 * @return {@link #quantity} (An amount of service being requested which can be 1846 * a quantity ( for example $1,500 home modification), a ratio ( for 1847 * example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per 1848 * fraction).) 1849 */ 1850 public Type getQuantity() { 1851 return this.quantity; 1852 } 1853 1854 /** 1855 * @return {@link #quantity} (An amount of service being requested which can be 1856 * a quantity ( for example $1,500 home modification), a ratio ( for 1857 * example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per 1858 * fraction).) 1859 */ 1860 public Quantity getQuantityQuantity() throws FHIRException { 1861 if (this.quantity == null) 1862 this.quantity = new Quantity(); 1863 if (!(this.quantity instanceof Quantity)) 1864 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.quantity.getClass().getName() 1865 + " was encountered"); 1866 return (Quantity) this.quantity; 1867 } 1868 1869 public boolean hasQuantityQuantity() { 1870 return this.quantity instanceof Quantity; 1871 } 1872 1873 /** 1874 * @return {@link #quantity} (An amount of service being requested which can be 1875 * a quantity ( for example $1,500 home modification), a ratio ( for 1876 * example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per 1877 * fraction).) 1878 */ 1879 public Ratio getQuantityRatio() throws FHIRException { 1880 if (this.quantity == null) 1881 this.quantity = new Ratio(); 1882 if (!(this.quantity instanceof Ratio)) 1883 throw new FHIRException( 1884 "Type mismatch: the type Ratio was expected, but " + this.quantity.getClass().getName() + " was encountered"); 1885 return (Ratio) this.quantity; 1886 } 1887 1888 public boolean hasQuantityRatio() { 1889 return this.quantity instanceof Ratio; 1890 } 1891 1892 /** 1893 * @return {@link #quantity} (An amount of service being requested which can be 1894 * a quantity ( for example $1,500 home modification), a ratio ( for 1895 * example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per 1896 * fraction).) 1897 */ 1898 public Range getQuantityRange() throws FHIRException { 1899 if (this.quantity == null) 1900 this.quantity = new Range(); 1901 if (!(this.quantity instanceof Range)) 1902 throw new FHIRException( 1903 "Type mismatch: the type Range was expected, but " + this.quantity.getClass().getName() + " was encountered"); 1904 return (Range) this.quantity; 1905 } 1906 1907 public boolean hasQuantityRange() { 1908 return this.quantity instanceof Range; 1909 } 1910 1911 public boolean hasQuantity() { 1912 return this.quantity != null && !this.quantity.isEmpty(); 1913 } 1914 1915 /** 1916 * @param value {@link #quantity} (An amount of service being requested which 1917 * can be a quantity ( for example $1,500 home modification), a 1918 * ratio ( for example, 20 half day visits per month), or a range 1919 * (2.0 to 1.8 Gy per fraction).) 1920 */ 1921 public ServiceRequest setQuantity(Type value) { 1922 if (value != null && !(value instanceof Quantity || value instanceof Ratio || value instanceof Range)) 1923 throw new Error("Not the right type for ServiceRequest.quantity[x]: " + value.fhirType()); 1924 this.quantity = value; 1925 return this; 1926 } 1927 1928 /** 1929 * @return {@link #subject} (On whom or what the service is to be performed. 1930 * This is usually a human patient, but can also be requested on 1931 * animals, groups of humans or animals, devices such as dialysis 1932 * machines, or even locations (typically for environmental scans).) 1933 */ 1934 public Reference getSubject() { 1935 if (this.subject == null) 1936 if (Configuration.errorOnAutoCreate()) 1937 throw new Error("Attempt to auto-create ServiceRequest.subject"); 1938 else if (Configuration.doAutoCreate()) 1939 this.subject = new Reference(); // cc 1940 return this.subject; 1941 } 1942 1943 public boolean hasSubject() { 1944 return this.subject != null && !this.subject.isEmpty(); 1945 } 1946 1947 /** 1948 * @param value {@link #subject} (On whom or what the service is to be 1949 * performed. This is usually a human patient, but can also be 1950 * requested on animals, groups of humans or animals, devices such 1951 * as dialysis machines, or even locations (typically for 1952 * environmental scans).) 1953 */ 1954 public ServiceRequest setSubject(Reference value) { 1955 this.subject = value; 1956 return this; 1957 } 1958 1959 /** 1960 * @return {@link #subject} The actual object that is the target of the 1961 * reference. The reference library doesn't populate this, but you can 1962 * use it to hold the resource if you resolve it. (On whom or what the 1963 * service is to be performed. This is usually a human patient, but can 1964 * also be requested on animals, groups of humans or animals, devices 1965 * such as dialysis machines, or even locations (typically for 1966 * environmental scans).) 1967 */ 1968 public Resource getSubjectTarget() { 1969 return this.subjectTarget; 1970 } 1971 1972 /** 1973 * @param value {@link #subject} The actual object that is the target of the 1974 * reference. The reference library doesn't use these, but you can 1975 * use it to hold the resource if you resolve it. (On whom or what 1976 * the service is to be performed. This is usually a human patient, 1977 * but can also be requested on animals, groups of humans or 1978 * animals, devices such as dialysis machines, or even locations 1979 * (typically for environmental scans).) 1980 */ 1981 public ServiceRequest setSubjectTarget(Resource value) { 1982 this.subjectTarget = value; 1983 return this; 1984 } 1985 1986 /** 1987 * @return {@link #encounter} (An encounter that provides additional information 1988 * about the healthcare context in which this request is made.) 1989 */ 1990 public Reference getEncounter() { 1991 if (this.encounter == null) 1992 if (Configuration.errorOnAutoCreate()) 1993 throw new Error("Attempt to auto-create ServiceRequest.encounter"); 1994 else if (Configuration.doAutoCreate()) 1995 this.encounter = new Reference(); // cc 1996 return this.encounter; 1997 } 1998 1999 public boolean hasEncounter() { 2000 return this.encounter != null && !this.encounter.isEmpty(); 2001 } 2002 2003 /** 2004 * @param value {@link #encounter} (An encounter that provides additional 2005 * information about the healthcare context in which this request 2006 * is made.) 2007 */ 2008 public ServiceRequest setEncounter(Reference value) { 2009 this.encounter = value; 2010 return this; 2011 } 2012 2013 /** 2014 * @return {@link #encounter} The actual object that is the target of the 2015 * reference. The reference library doesn't populate this, but you can 2016 * use it to hold the resource if you resolve it. (An encounter that 2017 * provides additional information about the healthcare context in which 2018 * this request is made.) 2019 */ 2020 public Encounter getEncounterTarget() { 2021 if (this.encounterTarget == null) 2022 if (Configuration.errorOnAutoCreate()) 2023 throw new Error("Attempt to auto-create ServiceRequest.encounter"); 2024 else if (Configuration.doAutoCreate()) 2025 this.encounterTarget = new Encounter(); // aa 2026 return this.encounterTarget; 2027 } 2028 2029 /** 2030 * @param value {@link #encounter} The actual object that is the target of the 2031 * reference. The reference library doesn't use these, but you can 2032 * use it to hold the resource if you resolve it. (An encounter 2033 * that provides additional information about the healthcare 2034 * context in which this request is made.) 2035 */ 2036 public ServiceRequest setEncounterTarget(Encounter value) { 2037 this.encounterTarget = value; 2038 return this; 2039 } 2040 2041 /** 2042 * @return {@link #occurrence} (The date/time at which the requested service 2043 * should occur.) 2044 */ 2045 public Type getOccurrence() { 2046 return this.occurrence; 2047 } 2048 2049 /** 2050 * @return {@link #occurrence} (The date/time at which the requested service 2051 * should occur.) 2052 */ 2053 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 2054 if (this.occurrence == null) 2055 this.occurrence = new DateTimeType(); 2056 if (!(this.occurrence instanceof DateTimeType)) 2057 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2058 + this.occurrence.getClass().getName() + " was encountered"); 2059 return (DateTimeType) this.occurrence; 2060 } 2061 2062 public boolean hasOccurrenceDateTimeType() { 2063 return this.occurrence instanceof DateTimeType; 2064 } 2065 2066 /** 2067 * @return {@link #occurrence} (The date/time at which the requested service 2068 * should occur.) 2069 */ 2070 public Period getOccurrencePeriod() throws FHIRException { 2071 if (this.occurrence == null) 2072 this.occurrence = new Period(); 2073 if (!(this.occurrence instanceof Period)) 2074 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 2075 + " was encountered"); 2076 return (Period) this.occurrence; 2077 } 2078 2079 public boolean hasOccurrencePeriod() { 2080 return this.occurrence instanceof Period; 2081 } 2082 2083 /** 2084 * @return {@link #occurrence} (The date/time at which the requested service 2085 * should occur.) 2086 */ 2087 public Timing getOccurrenceTiming() throws FHIRException { 2088 if (this.occurrence == null) 2089 this.occurrence = new Timing(); 2090 if (!(this.occurrence instanceof Timing)) 2091 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.occurrence.getClass().getName() 2092 + " was encountered"); 2093 return (Timing) this.occurrence; 2094 } 2095 2096 public boolean hasOccurrenceTiming() { 2097 return this.occurrence instanceof Timing; 2098 } 2099 2100 public boolean hasOccurrence() { 2101 return this.occurrence != null && !this.occurrence.isEmpty(); 2102 } 2103 2104 /** 2105 * @param value {@link #occurrence} (The date/time at which the requested 2106 * service should occur.) 2107 */ 2108 public ServiceRequest setOccurrence(Type value) { 2109 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 2110 throw new Error("Not the right type for ServiceRequest.occurrence[x]: " + value.fhirType()); 2111 this.occurrence = value; 2112 return this; 2113 } 2114 2115 /** 2116 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the 2117 * pre-condition for performing the service. For example "pain", "on 2118 * flare-up", etc.) 2119 */ 2120 public Type getAsNeeded() { 2121 return this.asNeeded; 2122 } 2123 2124 /** 2125 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the 2126 * pre-condition for performing the service. For example "pain", "on 2127 * flare-up", etc.) 2128 */ 2129 public BooleanType getAsNeededBooleanType() throws FHIRException { 2130 if (this.asNeeded == null) 2131 this.asNeeded = new BooleanType(); 2132 if (!(this.asNeeded instanceof BooleanType)) 2133 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 2134 + this.asNeeded.getClass().getName() + " was encountered"); 2135 return (BooleanType) this.asNeeded; 2136 } 2137 2138 public boolean hasAsNeededBooleanType() { 2139 return this.asNeeded instanceof BooleanType; 2140 } 2141 2142 /** 2143 * @return {@link #asNeeded} (If a CodeableConcept is present, it indicates the 2144 * pre-condition for performing the service. For example "pain", "on 2145 * flare-up", etc.) 2146 */ 2147 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 2148 if (this.asNeeded == null) 2149 this.asNeeded = new CodeableConcept(); 2150 if (!(this.asNeeded instanceof CodeableConcept)) 2151 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2152 + this.asNeeded.getClass().getName() + " was encountered"); 2153 return (CodeableConcept) this.asNeeded; 2154 } 2155 2156 public boolean hasAsNeededCodeableConcept() { 2157 return this.asNeeded instanceof CodeableConcept; 2158 } 2159 2160 public boolean hasAsNeeded() { 2161 return this.asNeeded != null && !this.asNeeded.isEmpty(); 2162 } 2163 2164 /** 2165 * @param value {@link #asNeeded} (If a CodeableConcept is present, it indicates 2166 * the pre-condition for performing the service. For example 2167 * "pain", "on flare-up", etc.) 2168 */ 2169 public ServiceRequest setAsNeeded(Type value) { 2170 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 2171 throw new Error("Not the right type for ServiceRequest.asNeeded[x]: " + value.fhirType()); 2172 this.asNeeded = value; 2173 return this; 2174 } 2175 2176 /** 2177 * @return {@link #authoredOn} (When the request transitioned to being 2178 * actionable.). This is the underlying object with id, value and 2179 * extensions. The accessor "getAuthoredOn" gives direct access to the 2180 * value 2181 */ 2182 public DateTimeType getAuthoredOnElement() { 2183 if (this.authoredOn == null) 2184 if (Configuration.errorOnAutoCreate()) 2185 throw new Error("Attempt to auto-create ServiceRequest.authoredOn"); 2186 else if (Configuration.doAutoCreate()) 2187 this.authoredOn = new DateTimeType(); // bb 2188 return this.authoredOn; 2189 } 2190 2191 public boolean hasAuthoredOnElement() { 2192 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2193 } 2194 2195 public boolean hasAuthoredOn() { 2196 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2197 } 2198 2199 /** 2200 * @param value {@link #authoredOn} (When the request transitioned to being 2201 * actionable.). This is the underlying object with id, value and 2202 * extensions. The accessor "getAuthoredOn" gives direct access to 2203 * the value 2204 */ 2205 public ServiceRequest setAuthoredOnElement(DateTimeType value) { 2206 this.authoredOn = value; 2207 return this; 2208 } 2209 2210 /** 2211 * @return When the request transitioned to being actionable. 2212 */ 2213 public Date getAuthoredOn() { 2214 return this.authoredOn == null ? null : this.authoredOn.getValue(); 2215 } 2216 2217 /** 2218 * @param value When the request transitioned to being actionable. 2219 */ 2220 public ServiceRequest setAuthoredOn(Date value) { 2221 if (value == null) 2222 this.authoredOn = null; 2223 else { 2224 if (this.authoredOn == null) 2225 this.authoredOn = new DateTimeType(); 2226 this.authoredOn.setValue(value); 2227 } 2228 return this; 2229 } 2230 2231 /** 2232 * @return {@link #requester} (The individual who initiated the request and has 2233 * responsibility for its activation.) 2234 */ 2235 public Reference getRequester() { 2236 if (this.requester == null) 2237 if (Configuration.errorOnAutoCreate()) 2238 throw new Error("Attempt to auto-create ServiceRequest.requester"); 2239 else if (Configuration.doAutoCreate()) 2240 this.requester = new Reference(); // cc 2241 return this.requester; 2242 } 2243 2244 public boolean hasRequester() { 2245 return this.requester != null && !this.requester.isEmpty(); 2246 } 2247 2248 /** 2249 * @param value {@link #requester} (The individual who initiated the request and 2250 * has responsibility for its activation.) 2251 */ 2252 public ServiceRequest setRequester(Reference value) { 2253 this.requester = value; 2254 return this; 2255 } 2256 2257 /** 2258 * @return {@link #requester} The actual object that is the target of the 2259 * reference. The reference library doesn't populate this, but you can 2260 * use it to hold the resource if you resolve it. (The individual who 2261 * initiated the request and has responsibility for its activation.) 2262 */ 2263 public Resource getRequesterTarget() { 2264 return this.requesterTarget; 2265 } 2266 2267 /** 2268 * @param value {@link #requester} The actual object that is the target of the 2269 * reference. The reference library doesn't use these, but you can 2270 * use it to hold the resource if you resolve it. (The individual 2271 * who initiated the request and has responsibility for its 2272 * activation.) 2273 */ 2274 public ServiceRequest setRequesterTarget(Resource value) { 2275 this.requesterTarget = value; 2276 return this; 2277 } 2278 2279 /** 2280 * @return {@link #performerType} (Desired type of performer for doing the 2281 * requested service.) 2282 */ 2283 public CodeableConcept getPerformerType() { 2284 if (this.performerType == null) 2285 if (Configuration.errorOnAutoCreate()) 2286 throw new Error("Attempt to auto-create ServiceRequest.performerType"); 2287 else if (Configuration.doAutoCreate()) 2288 this.performerType = new CodeableConcept(); // cc 2289 return this.performerType; 2290 } 2291 2292 public boolean hasPerformerType() { 2293 return this.performerType != null && !this.performerType.isEmpty(); 2294 } 2295 2296 /** 2297 * @param value {@link #performerType} (Desired type of performer for doing the 2298 * requested service.) 2299 */ 2300 public ServiceRequest setPerformerType(CodeableConcept value) { 2301 this.performerType = value; 2302 return this; 2303 } 2304 2305 /** 2306 * @return {@link #performer} (The desired performer for doing the requested 2307 * service. For example, the surgeon, dermatopathologist, endoscopist, 2308 * etc.) 2309 */ 2310 public List<Reference> getPerformer() { 2311 if (this.performer == null) 2312 this.performer = new ArrayList<Reference>(); 2313 return this.performer; 2314 } 2315 2316 /** 2317 * @return Returns a reference to <code>this</code> for easy method chaining 2318 */ 2319 public ServiceRequest setPerformer(List<Reference> thePerformer) { 2320 this.performer = thePerformer; 2321 return this; 2322 } 2323 2324 public boolean hasPerformer() { 2325 if (this.performer == null) 2326 return false; 2327 for (Reference item : this.performer) 2328 if (!item.isEmpty()) 2329 return true; 2330 return false; 2331 } 2332 2333 public Reference addPerformer() { // 3 2334 Reference t = new Reference(); 2335 if (this.performer == null) 2336 this.performer = new ArrayList<Reference>(); 2337 this.performer.add(t); 2338 return t; 2339 } 2340 2341 public ServiceRequest addPerformer(Reference t) { // 3 2342 if (t == null) 2343 return this; 2344 if (this.performer == null) 2345 this.performer = new ArrayList<Reference>(); 2346 this.performer.add(t); 2347 return this; 2348 } 2349 2350 /** 2351 * @return The first repetition of repeating field {@link #performer}, creating 2352 * it if it does not already exist 2353 */ 2354 public Reference getPerformerFirstRep() { 2355 if (getPerformer().isEmpty()) { 2356 addPerformer(); 2357 } 2358 return getPerformer().get(0); 2359 } 2360 2361 /** 2362 * @deprecated Use Reference#setResource(IBaseResource) instead 2363 */ 2364 @Deprecated 2365 public List<Resource> getPerformerTarget() { 2366 if (this.performerTarget == null) 2367 this.performerTarget = new ArrayList<Resource>(); 2368 return this.performerTarget; 2369 } 2370 2371 /** 2372 * @return {@link #locationCode} (The preferred location(s) where the procedure 2373 * should actually happen in coded or free text form. E.g. at home or 2374 * nursing day care center.) 2375 */ 2376 public List<CodeableConcept> getLocationCode() { 2377 if (this.locationCode == null) 2378 this.locationCode = new ArrayList<CodeableConcept>(); 2379 return this.locationCode; 2380 } 2381 2382 /** 2383 * @return Returns a reference to <code>this</code> for easy method chaining 2384 */ 2385 public ServiceRequest setLocationCode(List<CodeableConcept> theLocationCode) { 2386 this.locationCode = theLocationCode; 2387 return this; 2388 } 2389 2390 public boolean hasLocationCode() { 2391 if (this.locationCode == null) 2392 return false; 2393 for (CodeableConcept item : this.locationCode) 2394 if (!item.isEmpty()) 2395 return true; 2396 return false; 2397 } 2398 2399 public CodeableConcept addLocationCode() { // 3 2400 CodeableConcept t = new CodeableConcept(); 2401 if (this.locationCode == null) 2402 this.locationCode = new ArrayList<CodeableConcept>(); 2403 this.locationCode.add(t); 2404 return t; 2405 } 2406 2407 public ServiceRequest addLocationCode(CodeableConcept t) { // 3 2408 if (t == null) 2409 return this; 2410 if (this.locationCode == null) 2411 this.locationCode = new ArrayList<CodeableConcept>(); 2412 this.locationCode.add(t); 2413 return this; 2414 } 2415 2416 /** 2417 * @return The first repetition of repeating field {@link #locationCode}, 2418 * creating it if it does not already exist 2419 */ 2420 public CodeableConcept getLocationCodeFirstRep() { 2421 if (getLocationCode().isEmpty()) { 2422 addLocationCode(); 2423 } 2424 return getLocationCode().get(0); 2425 } 2426 2427 /** 2428 * @return {@link #locationReference} (A reference to the the preferred 2429 * location(s) where the procedure should actually happen. E.g. at home 2430 * or nursing day care center.) 2431 */ 2432 public List<Reference> getLocationReference() { 2433 if (this.locationReference == null) 2434 this.locationReference = new ArrayList<Reference>(); 2435 return this.locationReference; 2436 } 2437 2438 /** 2439 * @return Returns a reference to <code>this</code> for easy method chaining 2440 */ 2441 public ServiceRequest setLocationReference(List<Reference> theLocationReference) { 2442 this.locationReference = theLocationReference; 2443 return this; 2444 } 2445 2446 public boolean hasLocationReference() { 2447 if (this.locationReference == null) 2448 return false; 2449 for (Reference item : this.locationReference) 2450 if (!item.isEmpty()) 2451 return true; 2452 return false; 2453 } 2454 2455 public Reference addLocationReference() { // 3 2456 Reference t = new Reference(); 2457 if (this.locationReference == null) 2458 this.locationReference = new ArrayList<Reference>(); 2459 this.locationReference.add(t); 2460 return t; 2461 } 2462 2463 public ServiceRequest addLocationReference(Reference t) { // 3 2464 if (t == null) 2465 return this; 2466 if (this.locationReference == null) 2467 this.locationReference = new ArrayList<Reference>(); 2468 this.locationReference.add(t); 2469 return this; 2470 } 2471 2472 /** 2473 * @return The first repetition of repeating field {@link #locationReference}, 2474 * creating it if it does not already exist 2475 */ 2476 public Reference getLocationReferenceFirstRep() { 2477 if (getLocationReference().isEmpty()) { 2478 addLocationReference(); 2479 } 2480 return getLocationReference().get(0); 2481 } 2482 2483 /** 2484 * @deprecated Use Reference#setResource(IBaseResource) instead 2485 */ 2486 @Deprecated 2487 public List<Location> getLocationReferenceTarget() { 2488 if (this.locationReferenceTarget == null) 2489 this.locationReferenceTarget = new ArrayList<Location>(); 2490 return this.locationReferenceTarget; 2491 } 2492 2493 /** 2494 * @deprecated Use Reference#setResource(IBaseResource) instead 2495 */ 2496 @Deprecated 2497 public Location addLocationReferenceTarget() { 2498 Location r = new Location(); 2499 if (this.locationReferenceTarget == null) 2500 this.locationReferenceTarget = new ArrayList<Location>(); 2501 this.locationReferenceTarget.add(r); 2502 return r; 2503 } 2504 2505 /** 2506 * @return {@link #reasonCode} (An explanation or justification for why this 2507 * service is being requested in coded or textual form. This is often 2508 * for billing purposes. May relate to the resources referred to in 2509 * `supportingInfo`.) 2510 */ 2511 public List<CodeableConcept> getReasonCode() { 2512 if (this.reasonCode == null) 2513 this.reasonCode = new ArrayList<CodeableConcept>(); 2514 return this.reasonCode; 2515 } 2516 2517 /** 2518 * @return Returns a reference to <code>this</code> for easy method chaining 2519 */ 2520 public ServiceRequest setReasonCode(List<CodeableConcept> theReasonCode) { 2521 this.reasonCode = theReasonCode; 2522 return this; 2523 } 2524 2525 public boolean hasReasonCode() { 2526 if (this.reasonCode == null) 2527 return false; 2528 for (CodeableConcept item : this.reasonCode) 2529 if (!item.isEmpty()) 2530 return true; 2531 return false; 2532 } 2533 2534 public CodeableConcept addReasonCode() { // 3 2535 CodeableConcept t = new CodeableConcept(); 2536 if (this.reasonCode == null) 2537 this.reasonCode = new ArrayList<CodeableConcept>(); 2538 this.reasonCode.add(t); 2539 return t; 2540 } 2541 2542 public ServiceRequest addReasonCode(CodeableConcept t) { // 3 2543 if (t == null) 2544 return this; 2545 if (this.reasonCode == null) 2546 this.reasonCode = new ArrayList<CodeableConcept>(); 2547 this.reasonCode.add(t); 2548 return this; 2549 } 2550 2551 /** 2552 * @return The first repetition of repeating field {@link #reasonCode}, creating 2553 * it if it does not already exist 2554 */ 2555 public CodeableConcept getReasonCodeFirstRep() { 2556 if (getReasonCode().isEmpty()) { 2557 addReasonCode(); 2558 } 2559 return getReasonCode().get(0); 2560 } 2561 2562 /** 2563 * @return {@link #reasonReference} (Indicates another resource that provides a 2564 * justification for why this service is being requested. May relate to 2565 * the resources referred to in `supportingInfo`.) 2566 */ 2567 public List<Reference> getReasonReference() { 2568 if (this.reasonReference == null) 2569 this.reasonReference = new ArrayList<Reference>(); 2570 return this.reasonReference; 2571 } 2572 2573 /** 2574 * @return Returns a reference to <code>this</code> for easy method chaining 2575 */ 2576 public ServiceRequest setReasonReference(List<Reference> theReasonReference) { 2577 this.reasonReference = theReasonReference; 2578 return this; 2579 } 2580 2581 public boolean hasReasonReference() { 2582 if (this.reasonReference == null) 2583 return false; 2584 for (Reference item : this.reasonReference) 2585 if (!item.isEmpty()) 2586 return true; 2587 return false; 2588 } 2589 2590 public Reference addReasonReference() { // 3 2591 Reference t = new Reference(); 2592 if (this.reasonReference == null) 2593 this.reasonReference = new ArrayList<Reference>(); 2594 this.reasonReference.add(t); 2595 return t; 2596 } 2597 2598 public ServiceRequest addReasonReference(Reference t) { // 3 2599 if (t == null) 2600 return this; 2601 if (this.reasonReference == null) 2602 this.reasonReference = new ArrayList<Reference>(); 2603 this.reasonReference.add(t); 2604 return this; 2605 } 2606 2607 /** 2608 * @return The first repetition of repeating field {@link #reasonReference}, 2609 * creating it if it does not already exist 2610 */ 2611 public Reference getReasonReferenceFirstRep() { 2612 if (getReasonReference().isEmpty()) { 2613 addReasonReference(); 2614 } 2615 return getReasonReference().get(0); 2616 } 2617 2618 /** 2619 * @deprecated Use Reference#setResource(IBaseResource) instead 2620 */ 2621 @Deprecated 2622 public List<Resource> getReasonReferenceTarget() { 2623 if (this.reasonReferenceTarget == null) 2624 this.reasonReferenceTarget = new ArrayList<Resource>(); 2625 return this.reasonReferenceTarget; 2626 } 2627 2628 /** 2629 * @return {@link #insurance} (Insurance plans, coverage extensions, 2630 * pre-authorizations and/or pre-determinations that may be needed for 2631 * delivering the requested service.) 2632 */ 2633 public List<Reference> getInsurance() { 2634 if (this.insurance == null) 2635 this.insurance = new ArrayList<Reference>(); 2636 return this.insurance; 2637 } 2638 2639 /** 2640 * @return Returns a reference to <code>this</code> for easy method chaining 2641 */ 2642 public ServiceRequest setInsurance(List<Reference> theInsurance) { 2643 this.insurance = theInsurance; 2644 return this; 2645 } 2646 2647 public boolean hasInsurance() { 2648 if (this.insurance == null) 2649 return false; 2650 for (Reference item : this.insurance) 2651 if (!item.isEmpty()) 2652 return true; 2653 return false; 2654 } 2655 2656 public Reference addInsurance() { // 3 2657 Reference t = new Reference(); 2658 if (this.insurance == null) 2659 this.insurance = new ArrayList<Reference>(); 2660 this.insurance.add(t); 2661 return t; 2662 } 2663 2664 public ServiceRequest addInsurance(Reference t) { // 3 2665 if (t == null) 2666 return this; 2667 if (this.insurance == null) 2668 this.insurance = new ArrayList<Reference>(); 2669 this.insurance.add(t); 2670 return this; 2671 } 2672 2673 /** 2674 * @return The first repetition of repeating field {@link #insurance}, creating 2675 * it if it does not already exist 2676 */ 2677 public Reference getInsuranceFirstRep() { 2678 if (getInsurance().isEmpty()) { 2679 addInsurance(); 2680 } 2681 return getInsurance().get(0); 2682 } 2683 2684 /** 2685 * @deprecated Use Reference#setResource(IBaseResource) instead 2686 */ 2687 @Deprecated 2688 public List<Resource> getInsuranceTarget() { 2689 if (this.insuranceTarget == null) 2690 this.insuranceTarget = new ArrayList<Resource>(); 2691 return this.insuranceTarget; 2692 } 2693 2694 /** 2695 * @return {@link #supportingInfo} (Additional clinical information about the 2696 * patient or specimen that may influence the services or their 2697 * interpretations. This information includes diagnosis, clinical 2698 * findings and other observations. In laboratory ordering these are 2699 * typically referred to as "ask at order entry questions (AOEs)". This 2700 * includes observations explicitly requested by the producer (filler) 2701 * to provide context or supporting information needed to complete the 2702 * order. For example, reporting the amount of inspired oxygen for blood 2703 * gas measurements.) 2704 */ 2705 public List<Reference> getSupportingInfo() { 2706 if (this.supportingInfo == null) 2707 this.supportingInfo = new ArrayList<Reference>(); 2708 return this.supportingInfo; 2709 } 2710 2711 /** 2712 * @return Returns a reference to <code>this</code> for easy method chaining 2713 */ 2714 public ServiceRequest setSupportingInfo(List<Reference> theSupportingInfo) { 2715 this.supportingInfo = theSupportingInfo; 2716 return this; 2717 } 2718 2719 public boolean hasSupportingInfo() { 2720 if (this.supportingInfo == null) 2721 return false; 2722 for (Reference item : this.supportingInfo) 2723 if (!item.isEmpty()) 2724 return true; 2725 return false; 2726 } 2727 2728 public Reference addSupportingInfo() { // 3 2729 Reference t = new Reference(); 2730 if (this.supportingInfo == null) 2731 this.supportingInfo = new ArrayList<Reference>(); 2732 this.supportingInfo.add(t); 2733 return t; 2734 } 2735 2736 public ServiceRequest addSupportingInfo(Reference t) { // 3 2737 if (t == null) 2738 return this; 2739 if (this.supportingInfo == null) 2740 this.supportingInfo = new ArrayList<Reference>(); 2741 this.supportingInfo.add(t); 2742 return this; 2743 } 2744 2745 /** 2746 * @return The first repetition of repeating field {@link #supportingInfo}, 2747 * creating it if it does not already exist 2748 */ 2749 public Reference getSupportingInfoFirstRep() { 2750 if (getSupportingInfo().isEmpty()) { 2751 addSupportingInfo(); 2752 } 2753 return getSupportingInfo().get(0); 2754 } 2755 2756 /** 2757 * @deprecated Use Reference#setResource(IBaseResource) instead 2758 */ 2759 @Deprecated 2760 public List<Resource> getSupportingInfoTarget() { 2761 if (this.supportingInfoTarget == null) 2762 this.supportingInfoTarget = new ArrayList<Resource>(); 2763 return this.supportingInfoTarget; 2764 } 2765 2766 /** 2767 * @return {@link #specimen} (One or more specimens that the laboratory 2768 * procedure will use.) 2769 */ 2770 public List<Reference> getSpecimen() { 2771 if (this.specimen == null) 2772 this.specimen = new ArrayList<Reference>(); 2773 return this.specimen; 2774 } 2775 2776 /** 2777 * @return Returns a reference to <code>this</code> for easy method chaining 2778 */ 2779 public ServiceRequest setSpecimen(List<Reference> theSpecimen) { 2780 this.specimen = theSpecimen; 2781 return this; 2782 } 2783 2784 public boolean hasSpecimen() { 2785 if (this.specimen == null) 2786 return false; 2787 for (Reference item : this.specimen) 2788 if (!item.isEmpty()) 2789 return true; 2790 return false; 2791 } 2792 2793 public Reference addSpecimen() { // 3 2794 Reference t = new Reference(); 2795 if (this.specimen == null) 2796 this.specimen = new ArrayList<Reference>(); 2797 this.specimen.add(t); 2798 return t; 2799 } 2800 2801 public ServiceRequest addSpecimen(Reference t) { // 3 2802 if (t == null) 2803 return this; 2804 if (this.specimen == null) 2805 this.specimen = new ArrayList<Reference>(); 2806 this.specimen.add(t); 2807 return this; 2808 } 2809 2810 /** 2811 * @return The first repetition of repeating field {@link #specimen}, creating 2812 * it if it does not already exist 2813 */ 2814 public Reference getSpecimenFirstRep() { 2815 if (getSpecimen().isEmpty()) { 2816 addSpecimen(); 2817 } 2818 return getSpecimen().get(0); 2819 } 2820 2821 /** 2822 * @deprecated Use Reference#setResource(IBaseResource) instead 2823 */ 2824 @Deprecated 2825 public List<Specimen> getSpecimenTarget() { 2826 if (this.specimenTarget == null) 2827 this.specimenTarget = new ArrayList<Specimen>(); 2828 return this.specimenTarget; 2829 } 2830 2831 /** 2832 * @deprecated Use Reference#setResource(IBaseResource) instead 2833 */ 2834 @Deprecated 2835 public Specimen addSpecimenTarget() { 2836 Specimen r = new Specimen(); 2837 if (this.specimenTarget == null) 2838 this.specimenTarget = new ArrayList<Specimen>(); 2839 this.specimenTarget.add(r); 2840 return r; 2841 } 2842 2843 /** 2844 * @return {@link #bodySite} (Anatomic location where the procedure should be 2845 * performed. This is the target site.) 2846 */ 2847 public List<CodeableConcept> getBodySite() { 2848 if (this.bodySite == null) 2849 this.bodySite = new ArrayList<CodeableConcept>(); 2850 return this.bodySite; 2851 } 2852 2853 /** 2854 * @return Returns a reference to <code>this</code> for easy method chaining 2855 */ 2856 public ServiceRequest setBodySite(List<CodeableConcept> theBodySite) { 2857 this.bodySite = theBodySite; 2858 return this; 2859 } 2860 2861 public boolean hasBodySite() { 2862 if (this.bodySite == null) 2863 return false; 2864 for (CodeableConcept item : this.bodySite) 2865 if (!item.isEmpty()) 2866 return true; 2867 return false; 2868 } 2869 2870 public CodeableConcept addBodySite() { // 3 2871 CodeableConcept t = new CodeableConcept(); 2872 if (this.bodySite == null) 2873 this.bodySite = new ArrayList<CodeableConcept>(); 2874 this.bodySite.add(t); 2875 return t; 2876 } 2877 2878 public ServiceRequest addBodySite(CodeableConcept t) { // 3 2879 if (t == null) 2880 return this; 2881 if (this.bodySite == null) 2882 this.bodySite = new ArrayList<CodeableConcept>(); 2883 this.bodySite.add(t); 2884 return this; 2885 } 2886 2887 /** 2888 * @return The first repetition of repeating field {@link #bodySite}, creating 2889 * it if it does not already exist 2890 */ 2891 public CodeableConcept getBodySiteFirstRep() { 2892 if (getBodySite().isEmpty()) { 2893 addBodySite(); 2894 } 2895 return getBodySite().get(0); 2896 } 2897 2898 /** 2899 * @return {@link #note} (Any other notes and comments made about the service 2900 * request. For example, internal billing notes.) 2901 */ 2902 public List<Annotation> getNote() { 2903 if (this.note == null) 2904 this.note = new ArrayList<Annotation>(); 2905 return this.note; 2906 } 2907 2908 /** 2909 * @return Returns a reference to <code>this</code> for easy method chaining 2910 */ 2911 public ServiceRequest setNote(List<Annotation> theNote) { 2912 this.note = theNote; 2913 return this; 2914 } 2915 2916 public boolean hasNote() { 2917 if (this.note == null) 2918 return false; 2919 for (Annotation item : this.note) 2920 if (!item.isEmpty()) 2921 return true; 2922 return false; 2923 } 2924 2925 public Annotation addNote() { // 3 2926 Annotation t = new Annotation(); 2927 if (this.note == null) 2928 this.note = new ArrayList<Annotation>(); 2929 this.note.add(t); 2930 return t; 2931 } 2932 2933 public ServiceRequest addNote(Annotation t) { // 3 2934 if (t == null) 2935 return this; 2936 if (this.note == null) 2937 this.note = new ArrayList<Annotation>(); 2938 this.note.add(t); 2939 return this; 2940 } 2941 2942 /** 2943 * @return The first repetition of repeating field {@link #note}, creating it if 2944 * it does not already exist 2945 */ 2946 public Annotation getNoteFirstRep() { 2947 if (getNote().isEmpty()) { 2948 addNote(); 2949 } 2950 return getNote().get(0); 2951 } 2952 2953 /** 2954 * @return {@link #patientInstruction} (Instructions in terms that are 2955 * understood by the patient or consumer.). This is the underlying 2956 * object with id, value and extensions. The accessor 2957 * "getPatientInstruction" gives direct access to the value 2958 */ 2959 public StringType getPatientInstructionElement() { 2960 if (this.patientInstruction == null) 2961 if (Configuration.errorOnAutoCreate()) 2962 throw new Error("Attempt to auto-create ServiceRequest.patientInstruction"); 2963 else if (Configuration.doAutoCreate()) 2964 this.patientInstruction = new StringType(); // bb 2965 return this.patientInstruction; 2966 } 2967 2968 public boolean hasPatientInstructionElement() { 2969 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 2970 } 2971 2972 public boolean hasPatientInstruction() { 2973 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 2974 } 2975 2976 /** 2977 * @param value {@link #patientInstruction} (Instructions in terms that are 2978 * understood by the patient or consumer.). This is the underlying 2979 * object with id, value and extensions. The accessor 2980 * "getPatientInstruction" gives direct access to the value 2981 */ 2982 public ServiceRequest setPatientInstructionElement(StringType value) { 2983 this.patientInstruction = value; 2984 return this; 2985 } 2986 2987 /** 2988 * @return Instructions in terms that are understood by the patient or consumer. 2989 */ 2990 public String getPatientInstruction() { 2991 return this.patientInstruction == null ? null : this.patientInstruction.getValue(); 2992 } 2993 2994 /** 2995 * @param value Instructions in terms that are understood by the patient or 2996 * consumer. 2997 */ 2998 public ServiceRequest setPatientInstruction(String value) { 2999 if (Utilities.noString(value)) 3000 this.patientInstruction = null; 3001 else { 3002 if (this.patientInstruction == null) 3003 this.patientInstruction = new StringType(); 3004 this.patientInstruction.setValue(value); 3005 } 3006 return this; 3007 } 3008 3009 /** 3010 * @return {@link #relevantHistory} (Key events in the history of the request.) 3011 */ 3012 public List<Reference> getRelevantHistory() { 3013 if (this.relevantHistory == null) 3014 this.relevantHistory = new ArrayList<Reference>(); 3015 return this.relevantHistory; 3016 } 3017 3018 /** 3019 * @return Returns a reference to <code>this</code> for easy method chaining 3020 */ 3021 public ServiceRequest setRelevantHistory(List<Reference> theRelevantHistory) { 3022 this.relevantHistory = theRelevantHistory; 3023 return this; 3024 } 3025 3026 public boolean hasRelevantHistory() { 3027 if (this.relevantHistory == null) 3028 return false; 3029 for (Reference item : this.relevantHistory) 3030 if (!item.isEmpty()) 3031 return true; 3032 return false; 3033 } 3034 3035 public Reference addRelevantHistory() { // 3 3036 Reference t = new Reference(); 3037 if (this.relevantHistory == null) 3038 this.relevantHistory = new ArrayList<Reference>(); 3039 this.relevantHistory.add(t); 3040 return t; 3041 } 3042 3043 public ServiceRequest addRelevantHistory(Reference t) { // 3 3044 if (t == null) 3045 return this; 3046 if (this.relevantHistory == null) 3047 this.relevantHistory = new ArrayList<Reference>(); 3048 this.relevantHistory.add(t); 3049 return this; 3050 } 3051 3052 /** 3053 * @return The first repetition of repeating field {@link #relevantHistory}, 3054 * creating it if it does not already exist 3055 */ 3056 public Reference getRelevantHistoryFirstRep() { 3057 if (getRelevantHistory().isEmpty()) { 3058 addRelevantHistory(); 3059 } 3060 return getRelevantHistory().get(0); 3061 } 3062 3063 /** 3064 * @deprecated Use Reference#setResource(IBaseResource) instead 3065 */ 3066 @Deprecated 3067 public List<Provenance> getRelevantHistoryTarget() { 3068 if (this.relevantHistoryTarget == null) 3069 this.relevantHistoryTarget = new ArrayList<Provenance>(); 3070 return this.relevantHistoryTarget; 3071 } 3072 3073 /** 3074 * @deprecated Use Reference#setResource(IBaseResource) instead 3075 */ 3076 @Deprecated 3077 public Provenance addRelevantHistoryTarget() { 3078 Provenance r = new Provenance(); 3079 if (this.relevantHistoryTarget == null) 3080 this.relevantHistoryTarget = new ArrayList<Provenance>(); 3081 this.relevantHistoryTarget.add(r); 3082 return r; 3083 } 3084 3085 protected void listChildren(List<Property> children) { 3086 super.listChildren(children); 3087 children.add(new Property("identifier", "Identifier", 3088 "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, 3089 java.lang.Integer.MAX_VALUE, identifier)); 3090 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", 3091 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.", 3092 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 3093 children.add(new Property("instantiatesUri", "uri", 3094 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.", 3095 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 3096 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest|MedicationRequest)", 3097 "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3098 children.add(new Property("replaces", "Reference(ServiceRequest)", 3099 "The request takes the place of the referenced completed or terminated request(s).", 0, 3100 java.lang.Integer.MAX_VALUE, replaces)); 3101 children.add(new Property("requisition", "Identifier", 3102 "A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 3103 0, 1, requisition)); 3104 children.add(new Property("status", "code", "The status of the order.", 0, 1, status)); 3105 children.add(new Property("intent", "code", 3106 "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent)); 3107 children.add(new Property("category", "CodeableConcept", 3108 "A code that classifies the service for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 3109 0, java.lang.Integer.MAX_VALUE, category)); 3110 children.add(new Property("priority", "code", 3111 "Indicates how quickly the ServiceRequest should be addressed with respect to other requests.", 0, 1, 3112 priority)); 3113 children.add(new Property("doNotPerform", "boolean", 3114 "Set this to true if the record is saying that the service/procedure should NOT be performed.", 0, 1, 3115 doNotPerform)); 3116 children.add(new Property("code", "CodeableConcept", 3117 "A code that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested.", 3118 0, 1, code)); 3119 children.add(new Property("orderDetail", "CodeableConcept", 3120 "Additional details and instructions about the how the services are to be delivered. For example, and order for a urinary catheter may have an order detail for an external or indwelling catheter, or an order for a bandage may require additional instructions specifying how the bandage should be applied.", 3121 0, java.lang.Integer.MAX_VALUE, orderDetail)); 3122 children.add(new Property("quantity[x]", "Quantity|Ratio|Range", 3123 "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 3124 0, 1, quantity)); 3125 children.add(new Property("subject", "Reference(Patient|Group|Location|Device)", 3126 "On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 3127 0, 1, subject)); 3128 children.add(new Property("encounter", "Reference(Encounter)", 3129 "An encounter that provides additional information about the healthcare context in which this request is made.", 3130 0, 1, encounter)); 3131 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", 3132 "The date/time at which the requested service should occur.", 0, 1, occurrence)); 3133 children.add(new Property("asNeeded[x]", "boolean|CodeableConcept", 3134 "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 3135 0, 1, asNeeded)); 3136 children.add( 3137 new Property("authoredOn", "dateTime", "When the request transitioned to being actionable.", 0, 1, authoredOn)); 3138 children.add( 3139 new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 3140 "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester)); 3141 children.add(new Property("performerType", "CodeableConcept", 3142 "Desired type of performer for doing the requested service.", 0, 1, performerType)); 3143 children.add(new Property("performer", 3144 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", 3145 "The desired performer for doing the requested service. For example, the surgeon, dermatopathologist, endoscopist, etc.", 3146 0, java.lang.Integer.MAX_VALUE, performer)); 3147 children.add(new Property("locationCode", "CodeableConcept", 3148 "The preferred location(s) where the procedure should actually happen in coded or free text form. E.g. at home or nursing day care center.", 3149 0, java.lang.Integer.MAX_VALUE, locationCode)); 3150 children.add(new Property("locationReference", "Reference(Location)", 3151 "A reference to the the preferred location(s) where the procedure should actually happen. E.g. at home or nursing day care center.", 3152 0, java.lang.Integer.MAX_VALUE, locationReference)); 3153 children.add(new Property("reasonCode", "CodeableConcept", 3154 "An explanation or justification for why this service is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in `supportingInfo`.", 3155 0, java.lang.Integer.MAX_VALUE, reasonCode)); 3156 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 3157 "Indicates another resource that provides a justification for why this service is being requested. May relate to the resources referred to in `supportingInfo`.", 3158 0, java.lang.Integer.MAX_VALUE, reasonReference)); 3159 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", 3160 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be needed for delivering the requested service.", 3161 0, java.lang.Integer.MAX_VALUE, insurance)); 3162 children.add(new Property("supportingInfo", "Reference(Any)", 3163 "Additional clinical information about the patient or specimen that may influence the services or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.", 3164 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 3165 children.add(new Property("specimen", "Reference(Specimen)", 3166 "One or more specimens that the laboratory procedure will use.", 0, java.lang.Integer.MAX_VALUE, specimen)); 3167 children.add(new Property("bodySite", "CodeableConcept", 3168 "Anatomic location where the procedure should be performed. This is the target site.", 0, 3169 java.lang.Integer.MAX_VALUE, bodySite)); 3170 children.add(new Property("note", "Annotation", 3171 "Any other notes and comments made about the service request. For example, internal billing notes.", 0, 3172 java.lang.Integer.MAX_VALUE, note)); 3173 children.add(new Property("patientInstruction", "string", 3174 "Instructions in terms that are understood by the patient or consumer.", 0, 1, patientInstruction)); 3175 children.add(new Property("relevantHistory", "Reference(Provenance)", "Key events in the history of the request.", 3176 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 3177 } 3178 3179 @Override 3180 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3181 switch (_hash) { 3182 case -1618432855: 3183 /* identifier */ return new Property("identifier", "Identifier", 3184 "Identifiers assigned to this order instance by the orderer and/or the receiver and/or order fulfiller.", 0, 3185 java.lang.Integer.MAX_VALUE, identifier); 3186 case 8911915: 3187 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 3188 "canonical(ActivityDefinition|PlanDefinition)", 3189 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.", 3190 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 3191 case -1926393373: 3192 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 3193 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this ServiceRequest.", 3194 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 3195 case -332612366: 3196 /* basedOn */ return new Property("basedOn", "Reference(CarePlan|ServiceRequest|MedicationRequest)", 3197 "Plan/proposal/order fulfilled by this request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3198 case -430332865: 3199 /* replaces */ return new Property("replaces", "Reference(ServiceRequest)", 3200 "The request takes the place of the referenced completed or terminated request(s).", 0, 3201 java.lang.Integer.MAX_VALUE, replaces); 3202 case 395923612: 3203 /* requisition */ return new Property("requisition", "Identifier", 3204 "A shared identifier common to all service requests that were authorized more or less simultaneously by a single author, representing the composite or group identifier.", 3205 0, 1, requisition); 3206 case -892481550: 3207 /* status */ return new Property("status", "code", "The status of the order.", 0, 1, status); 3208 case -1183762788: 3209 /* intent */ return new Property("intent", "code", 3210 "Whether the request is a proposal, plan, an original order or a reflex order.", 0, 1, intent); 3211 case 50511102: 3212 /* category */ return new Property("category", "CodeableConcept", 3213 "A code that classifies the service for searching, sorting and display purposes (e.g. \"Surgical Procedure\").", 3214 0, java.lang.Integer.MAX_VALUE, category); 3215 case -1165461084: 3216 /* priority */ return new Property("priority", "code", 3217 "Indicates how quickly the ServiceRequest should be addressed with respect to other requests.", 0, 1, 3218 priority); 3219 case -1788508167: 3220 /* doNotPerform */ return new Property("doNotPerform", "boolean", 3221 "Set this to true if the record is saying that the service/procedure should NOT be performed.", 0, 1, 3222 doNotPerform); 3223 case 3059181: 3224 /* code */ return new Property("code", "CodeableConcept", 3225 "A code that identifies a particular service (i.e., procedure, diagnostic investigation, or panel of investigations) that have been requested.", 3226 0, 1, code); 3227 case 1187338559: 3228 /* orderDetail */ return new Property("orderDetail", "CodeableConcept", 3229 "Additional details and instructions about the how the services are to be delivered. For example, and order for a urinary catheter may have an order detail for an external or indwelling catheter, or an order for a bandage may require additional instructions specifying how the bandage should be applied.", 3230 0, java.lang.Integer.MAX_VALUE, orderDetail); 3231 case -515002347: 3232 /* quantity[x] */ return new Property("quantity[x]", "Quantity|Ratio|Range", 3233 "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 3234 0, 1, quantity); 3235 case -1285004149: 3236 /* quantity */ return new Property("quantity[x]", "Quantity|Ratio|Range", 3237 "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 3238 0, 1, quantity); 3239 case -1087409610: 3240 /* quantityQuantity */ return new Property("quantity[x]", "Quantity|Ratio|Range", 3241 "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 3242 0, 1, quantity); 3243 case -1004987840: 3244 /* quantityRatio */ return new Property("quantity[x]", "Quantity|Ratio|Range", 3245 "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 3246 0, 1, quantity); 3247 case -1004993678: 3248 /* quantityRange */ return new Property("quantity[x]", "Quantity|Ratio|Range", 3249 "An amount of service being requested which can be a quantity ( for example $1,500 home modification), a ratio ( for example, 20 half day visits per month), or a range (2.0 to 1.8 Gy per fraction).", 3250 0, 1, quantity); 3251 case -1867885268: 3252 /* subject */ return new Property("subject", "Reference(Patient|Group|Location|Device)", 3253 "On whom or what the service is to be performed. This is usually a human patient, but can also be requested on animals, groups of humans or animals, devices such as dialysis machines, or even locations (typically for environmental scans).", 3254 0, 1, subject); 3255 case 1524132147: 3256 /* encounter */ return new Property("encounter", "Reference(Encounter)", 3257 "An encounter that provides additional information about the healthcare context in which this request is made.", 3258 0, 1, encounter); 3259 case -2022646513: 3260 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", 3261 "The date/time at which the requested service should occur.", 0, 1, occurrence); 3262 case 1687874001: 3263 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", 3264 "The date/time at which the requested service should occur.", 0, 1, occurrence); 3265 case -298443636: 3266 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", 3267 "The date/time at which the requested service should occur.", 0, 1, occurrence); 3268 case 1397156594: 3269 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", 3270 "The date/time at which the requested service should occur.", 0, 1, occurrence); 3271 case 1515218299: 3272 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", 3273 "The date/time at which the requested service should occur.", 0, 1, occurrence); 3274 case -544329575: 3275 /* asNeeded[x] */ return new Property("asNeeded[x]", "boolean|CodeableConcept", 3276 "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 3277 0, 1, asNeeded); 3278 case -1432923513: 3279 /* asNeeded */ return new Property("asNeeded[x]", "boolean|CodeableConcept", 3280 "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 3281 0, 1, asNeeded); 3282 case -591717471: 3283 /* asNeededBoolean */ return new Property("asNeeded[x]", "boolean|CodeableConcept", 3284 "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 3285 0, 1, asNeeded); 3286 case 1556420122: 3287 /* asNeededCodeableConcept */ return new Property("asNeeded[x]", "boolean|CodeableConcept", 3288 "If a CodeableConcept is present, it indicates the pre-condition for performing the service. For example \"pain\", \"on flare-up\", etc.", 3289 0, 1, asNeeded); 3290 case -1500852503: 3291 /* authoredOn */ return new Property("authoredOn", "dateTime", 3292 "When the request transitioned to being actionable.", 0, 1, authoredOn); 3293 case 693933948: 3294 /* requester */ return new Property("requester", 3295 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 3296 "The individual who initiated the request and has responsibility for its activation.", 0, 1, requester); 3297 case -901444568: 3298 /* performerType */ return new Property("performerType", "CodeableConcept", 3299 "Desired type of performer for doing the requested service.", 0, 1, performerType); 3300 case 481140686: 3301 /* performer */ return new Property("performer", 3302 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", 3303 "The desired performer for doing the requested service. For example, the surgeon, dermatopathologist, endoscopist, etc.", 3304 0, java.lang.Integer.MAX_VALUE, performer); 3305 case -58794174: 3306 /* locationCode */ return new Property("locationCode", "CodeableConcept", 3307 "The preferred location(s) where the procedure should actually happen in coded or free text form. E.g. at home or nursing day care center.", 3308 0, java.lang.Integer.MAX_VALUE, locationCode); 3309 case 755866390: 3310 /* locationReference */ return new Property("locationReference", "Reference(Location)", 3311 "A reference to the the preferred location(s) where the procedure should actually happen. E.g. at home or nursing day care center.", 3312 0, java.lang.Integer.MAX_VALUE, locationReference); 3313 case 722137681: 3314 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 3315 "An explanation or justification for why this service is being requested in coded or textual form. This is often for billing purposes. May relate to the resources referred to in `supportingInfo`.", 3316 0, java.lang.Integer.MAX_VALUE, reasonCode); 3317 case -1146218137: 3318 /* reasonReference */ return new Property("reasonReference", 3319 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 3320 "Indicates another resource that provides a justification for why this service is being requested. May relate to the resources referred to in `supportingInfo`.", 3321 0, java.lang.Integer.MAX_VALUE, reasonReference); 3322 case 73049818: 3323 /* insurance */ return new Property("insurance", "Reference(Coverage|ClaimResponse)", 3324 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be needed for delivering the requested service.", 3325 0, java.lang.Integer.MAX_VALUE, insurance); 3326 case 1922406657: 3327 /* supportingInfo */ return new Property("supportingInfo", "Reference(Any)", 3328 "Additional clinical information about the patient or specimen that may influence the services or their interpretations. This information includes diagnosis, clinical findings and other observations. In laboratory ordering these are typically referred to as \"ask at order entry questions (AOEs)\". This includes observations explicitly requested by the producer (filler) to provide context or supporting information needed to complete the order. For example, reporting the amount of inspired oxygen for blood gas measurements.", 3329 0, java.lang.Integer.MAX_VALUE, supportingInfo); 3330 case -2132868344: 3331 /* specimen */ return new Property("specimen", "Reference(Specimen)", 3332 "One or more specimens that the laboratory procedure will use.", 0, java.lang.Integer.MAX_VALUE, specimen); 3333 case 1702620169: 3334 /* bodySite */ return new Property("bodySite", "CodeableConcept", 3335 "Anatomic location where the procedure should be performed. This is the target site.", 0, 3336 java.lang.Integer.MAX_VALUE, bodySite); 3337 case 3387378: 3338 /* note */ return new Property("note", "Annotation", 3339 "Any other notes and comments made about the service request. For example, internal billing notes.", 0, 3340 java.lang.Integer.MAX_VALUE, note); 3341 case 737543241: 3342 /* patientInstruction */ return new Property("patientInstruction", "string", 3343 "Instructions in terms that are understood by the patient or consumer.", 0, 1, patientInstruction); 3344 case 1538891575: 3345 /* relevantHistory */ return new Property("relevantHistory", "Reference(Provenance)", 3346 "Key events in the history of the request.", 0, java.lang.Integer.MAX_VALUE, relevantHistory); 3347 default: 3348 return super.getNamedProperty(_hash, _name, _checkValid); 3349 } 3350 3351 } 3352 3353 @Override 3354 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3355 switch (hash) { 3356 case -1618432855: 3357 /* identifier */ return this.identifier == null ? new Base[0] 3358 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3359 case 8911915: 3360 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 3361 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 3362 case -1926393373: 3363 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 3364 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 3365 case -332612366: 3366 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3367 case -430332865: 3368 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 3369 case 395923612: 3370 /* requisition */ return this.requisition == null ? new Base[0] : new Base[] { this.requisition }; // Identifier 3371 case -892481550: 3372 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ServiceRequestStatus> 3373 case -1183762788: 3374 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<ServiceRequestIntent> 3375 case 50511102: 3376 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3377 case -1165461084: 3378 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<ServiceRequestPriority> 3379 case -1788508167: 3380 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 3381 case 3059181: 3382 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 3383 case 1187338559: 3384 /* orderDetail */ return this.orderDetail == null ? new Base[0] 3385 : this.orderDetail.toArray(new Base[this.orderDetail.size()]); // CodeableConcept 3386 case -1285004149: 3387 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Type 3388 case -1867885268: 3389 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 3390 case 1524132147: 3391 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 3392 case 1687874001: 3393 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 3394 case -1432923513: 3395 /* asNeeded */ return this.asNeeded == null ? new Base[0] : new Base[] { this.asNeeded }; // Type 3396 case -1500852503: 3397 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 3398 case 693933948: 3399 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 3400 case -901444568: 3401 /* performerType */ return this.performerType == null ? new Base[0] : new Base[] { this.performerType }; // CodeableConcept 3402 case 481140686: 3403 /* performer */ return this.performer == null ? new Base[0] 3404 : this.performer.toArray(new Base[this.performer.size()]); // Reference 3405 case -58794174: 3406 /* locationCode */ return this.locationCode == null ? new Base[0] 3407 : this.locationCode.toArray(new Base[this.locationCode.size()]); // CodeableConcept 3408 case 755866390: 3409 /* locationReference */ return this.locationReference == null ? new Base[0] 3410 : this.locationReference.toArray(new Base[this.locationReference.size()]); // Reference 3411 case 722137681: 3412 /* reasonCode */ return this.reasonCode == null ? new Base[0] 3413 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 3414 case -1146218137: 3415 /* reasonReference */ return this.reasonReference == null ? new Base[0] 3416 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 3417 case 73049818: 3418 /* insurance */ return this.insurance == null ? new Base[0] 3419 : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 3420 case 1922406657: 3421 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 3422 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 3423 case -2132868344: 3424 /* specimen */ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 3425 case 1702620169: 3426 /* bodySite */ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 3427 case 3387378: 3428 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3429 case 737543241: 3430 /* patientInstruction */ return this.patientInstruction == null ? new Base[0] 3431 : new Base[] { this.patientInstruction }; // StringType 3432 case 1538891575: 3433 /* relevantHistory */ return this.relevantHistory == null ? new Base[0] 3434 : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 3435 default: 3436 return super.getProperty(hash, name, checkValid); 3437 } 3438 3439 } 3440 3441 @Override 3442 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3443 switch (hash) { 3444 case -1618432855: // identifier 3445 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3446 return value; 3447 case 8911915: // instantiatesCanonical 3448 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 3449 return value; 3450 case -1926393373: // instantiatesUri 3451 this.getInstantiatesUri().add(castToUri(value)); // UriType 3452 return value; 3453 case -332612366: // basedOn 3454 this.getBasedOn().add(castToReference(value)); // Reference 3455 return value; 3456 case -430332865: // replaces 3457 this.getReplaces().add(castToReference(value)); // Reference 3458 return value; 3459 case 395923612: // requisition 3460 this.requisition = castToIdentifier(value); // Identifier 3461 return value; 3462 case -892481550: // status 3463 value = new ServiceRequestStatusEnumFactory().fromType(castToCode(value)); 3464 this.status = (Enumeration) value; // Enumeration<ServiceRequestStatus> 3465 return value; 3466 case -1183762788: // intent 3467 value = new ServiceRequestIntentEnumFactory().fromType(castToCode(value)); 3468 this.intent = (Enumeration) value; // Enumeration<ServiceRequestIntent> 3469 return value; 3470 case 50511102: // category 3471 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3472 return value; 3473 case -1165461084: // priority 3474 value = new ServiceRequestPriorityEnumFactory().fromType(castToCode(value)); 3475 this.priority = (Enumeration) value; // Enumeration<ServiceRequestPriority> 3476 return value; 3477 case -1788508167: // doNotPerform 3478 this.doNotPerform = castToBoolean(value); // BooleanType 3479 return value; 3480 case 3059181: // code 3481 this.code = castToCodeableConcept(value); // CodeableConcept 3482 return value; 3483 case 1187338559: // orderDetail 3484 this.getOrderDetail().add(castToCodeableConcept(value)); // CodeableConcept 3485 return value; 3486 case -1285004149: // quantity 3487 this.quantity = castToType(value); // Type 3488 return value; 3489 case -1867885268: // subject 3490 this.subject = castToReference(value); // Reference 3491 return value; 3492 case 1524132147: // encounter 3493 this.encounter = castToReference(value); // Reference 3494 return value; 3495 case 1687874001: // occurrence 3496 this.occurrence = castToType(value); // Type 3497 return value; 3498 case -1432923513: // asNeeded 3499 this.asNeeded = castToType(value); // Type 3500 return value; 3501 case -1500852503: // authoredOn 3502 this.authoredOn = castToDateTime(value); // DateTimeType 3503 return value; 3504 case 693933948: // requester 3505 this.requester = castToReference(value); // Reference 3506 return value; 3507 case -901444568: // performerType 3508 this.performerType = castToCodeableConcept(value); // CodeableConcept 3509 return value; 3510 case 481140686: // performer 3511 this.getPerformer().add(castToReference(value)); // Reference 3512 return value; 3513 case -58794174: // locationCode 3514 this.getLocationCode().add(castToCodeableConcept(value)); // CodeableConcept 3515 return value; 3516 case 755866390: // locationReference 3517 this.getLocationReference().add(castToReference(value)); // Reference 3518 return value; 3519 case 722137681: // reasonCode 3520 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 3521 return value; 3522 case -1146218137: // reasonReference 3523 this.getReasonReference().add(castToReference(value)); // Reference 3524 return value; 3525 case 73049818: // insurance 3526 this.getInsurance().add(castToReference(value)); // Reference 3527 return value; 3528 case 1922406657: // supportingInfo 3529 this.getSupportingInfo().add(castToReference(value)); // Reference 3530 return value; 3531 case -2132868344: // specimen 3532 this.getSpecimen().add(castToReference(value)); // Reference 3533 return value; 3534 case 1702620169: // bodySite 3535 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 3536 return value; 3537 case 3387378: // note 3538 this.getNote().add(castToAnnotation(value)); // Annotation 3539 return value; 3540 case 737543241: // patientInstruction 3541 this.patientInstruction = castToString(value); // StringType 3542 return value; 3543 case 1538891575: // relevantHistory 3544 this.getRelevantHistory().add(castToReference(value)); // Reference 3545 return value; 3546 default: 3547 return super.setProperty(hash, name, value); 3548 } 3549 3550 } 3551 3552 @Override 3553 public Base setProperty(String name, Base value) throws FHIRException { 3554 if (name.equals("identifier")) { 3555 this.getIdentifier().add(castToIdentifier(value)); 3556 } else if (name.equals("instantiatesCanonical")) { 3557 this.getInstantiatesCanonical().add(castToCanonical(value)); 3558 } else if (name.equals("instantiatesUri")) { 3559 this.getInstantiatesUri().add(castToUri(value)); 3560 } else if (name.equals("basedOn")) { 3561 this.getBasedOn().add(castToReference(value)); 3562 } else if (name.equals("replaces")) { 3563 this.getReplaces().add(castToReference(value)); 3564 } else if (name.equals("requisition")) { 3565 this.requisition = castToIdentifier(value); // Identifier 3566 } else if (name.equals("status")) { 3567 value = new ServiceRequestStatusEnumFactory().fromType(castToCode(value)); 3568 this.status = (Enumeration) value; // Enumeration<ServiceRequestStatus> 3569 } else if (name.equals("intent")) { 3570 value = new ServiceRequestIntentEnumFactory().fromType(castToCode(value)); 3571 this.intent = (Enumeration) value; // Enumeration<ServiceRequestIntent> 3572 } else if (name.equals("category")) { 3573 this.getCategory().add(castToCodeableConcept(value)); 3574 } else if (name.equals("priority")) { 3575 value = new ServiceRequestPriorityEnumFactory().fromType(castToCode(value)); 3576 this.priority = (Enumeration) value; // Enumeration<ServiceRequestPriority> 3577 } else if (name.equals("doNotPerform")) { 3578 this.doNotPerform = castToBoolean(value); // BooleanType 3579 } else if (name.equals("code")) { 3580 this.code = castToCodeableConcept(value); // CodeableConcept 3581 } else if (name.equals("orderDetail")) { 3582 this.getOrderDetail().add(castToCodeableConcept(value)); 3583 } else if (name.equals("quantity[x]")) { 3584 this.quantity = castToType(value); // Type 3585 } else if (name.equals("subject")) { 3586 this.subject = castToReference(value); // Reference 3587 } else if (name.equals("encounter")) { 3588 this.encounter = castToReference(value); // Reference 3589 } else if (name.equals("occurrence[x]")) { 3590 this.occurrence = castToType(value); // Type 3591 } else if (name.equals("asNeeded[x]")) { 3592 this.asNeeded = castToType(value); // Type 3593 } else if (name.equals("authoredOn")) { 3594 this.authoredOn = castToDateTime(value); // DateTimeType 3595 } else if (name.equals("requester")) { 3596 this.requester = castToReference(value); // Reference 3597 } else if (name.equals("performerType")) { 3598 this.performerType = castToCodeableConcept(value); // CodeableConcept 3599 } else if (name.equals("performer")) { 3600 this.getPerformer().add(castToReference(value)); 3601 } else if (name.equals("locationCode")) { 3602 this.getLocationCode().add(castToCodeableConcept(value)); 3603 } else if (name.equals("locationReference")) { 3604 this.getLocationReference().add(castToReference(value)); 3605 } else if (name.equals("reasonCode")) { 3606 this.getReasonCode().add(castToCodeableConcept(value)); 3607 } else if (name.equals("reasonReference")) { 3608 this.getReasonReference().add(castToReference(value)); 3609 } else if (name.equals("insurance")) { 3610 this.getInsurance().add(castToReference(value)); 3611 } else if (name.equals("supportingInfo")) { 3612 this.getSupportingInfo().add(castToReference(value)); 3613 } else if (name.equals("specimen")) { 3614 this.getSpecimen().add(castToReference(value)); 3615 } else if (name.equals("bodySite")) { 3616 this.getBodySite().add(castToCodeableConcept(value)); 3617 } else if (name.equals("note")) { 3618 this.getNote().add(castToAnnotation(value)); 3619 } else if (name.equals("patientInstruction")) { 3620 this.patientInstruction = castToString(value); // StringType 3621 } else if (name.equals("relevantHistory")) { 3622 this.getRelevantHistory().add(castToReference(value)); 3623 } else 3624 return super.setProperty(name, value); 3625 return value; 3626 } 3627 3628 @Override 3629 public void removeChild(String name, Base value) throws FHIRException { 3630 if (name.equals("identifier")) { 3631 this.getIdentifier().remove(castToIdentifier(value)); 3632 } else if (name.equals("instantiatesCanonical")) { 3633 this.getInstantiatesCanonical().remove(castToCanonical(value)); 3634 } else if (name.equals("instantiatesUri")) { 3635 this.getInstantiatesUri().remove(castToUri(value)); 3636 } else if (name.equals("basedOn")) { 3637 this.getBasedOn().remove(castToReference(value)); 3638 } else if (name.equals("replaces")) { 3639 this.getReplaces().remove(castToReference(value)); 3640 } else if (name.equals("requisition")) { 3641 this.requisition = null; 3642 } else if (name.equals("status")) { 3643 this.status = null; 3644 } else if (name.equals("intent")) { 3645 this.intent = null; 3646 } else if (name.equals("category")) { 3647 this.getCategory().remove(castToCodeableConcept(value)); 3648 } else if (name.equals("priority")) { 3649 this.priority = null; 3650 } else if (name.equals("doNotPerform")) { 3651 this.doNotPerform = null; 3652 } else if (name.equals("code")) { 3653 this.code = null; 3654 } else if (name.equals("orderDetail")) { 3655 this.getOrderDetail().remove(castToCodeableConcept(value)); 3656 } else if (name.equals("quantity[x]")) { 3657 this.quantity = null; 3658 } else if (name.equals("subject")) { 3659 this.subject = null; 3660 } else if (name.equals("encounter")) { 3661 this.encounter = null; 3662 } else if (name.equals("occurrence[x]")) { 3663 this.occurrence = null; 3664 } else if (name.equals("asNeeded[x]")) { 3665 this.asNeeded = null; 3666 } else if (name.equals("authoredOn")) { 3667 this.authoredOn = null; 3668 } else if (name.equals("requester")) { 3669 this.requester = null; 3670 } else if (name.equals("performerType")) { 3671 this.performerType = null; 3672 } else if (name.equals("performer")) { 3673 this.getPerformer().remove(castToReference(value)); 3674 } else if (name.equals("locationCode")) { 3675 this.getLocationCode().remove(castToCodeableConcept(value)); 3676 } else if (name.equals("locationReference")) { 3677 this.getLocationReference().remove(castToReference(value)); 3678 } else if (name.equals("reasonCode")) { 3679 this.getReasonCode().remove(castToCodeableConcept(value)); 3680 } else if (name.equals("reasonReference")) { 3681 this.getReasonReference().remove(castToReference(value)); 3682 } else if (name.equals("insurance")) { 3683 this.getInsurance().remove(castToReference(value)); 3684 } else if (name.equals("supportingInfo")) { 3685 this.getSupportingInfo().remove(castToReference(value)); 3686 } else if (name.equals("specimen")) { 3687 this.getSpecimen().remove(castToReference(value)); 3688 } else if (name.equals("bodySite")) { 3689 this.getBodySite().remove(castToCodeableConcept(value)); 3690 } else if (name.equals("note")) { 3691 this.getNote().remove(castToAnnotation(value)); 3692 } else if (name.equals("patientInstruction")) { 3693 this.patientInstruction = null; 3694 } else if (name.equals("relevantHistory")) { 3695 this.getRelevantHistory().remove(castToReference(value)); 3696 } else 3697 super.removeChild(name, value); 3698 3699 } 3700 3701 @Override 3702 public Base makeProperty(int hash, String name) throws FHIRException { 3703 switch (hash) { 3704 case -1618432855: 3705 return addIdentifier(); 3706 case 8911915: 3707 return addInstantiatesCanonicalElement(); 3708 case -1926393373: 3709 return addInstantiatesUriElement(); 3710 case -332612366: 3711 return addBasedOn(); 3712 case -430332865: 3713 return addReplaces(); 3714 case 395923612: 3715 return getRequisition(); 3716 case -892481550: 3717 return getStatusElement(); 3718 case -1183762788: 3719 return getIntentElement(); 3720 case 50511102: 3721 return addCategory(); 3722 case -1165461084: 3723 return getPriorityElement(); 3724 case -1788508167: 3725 return getDoNotPerformElement(); 3726 case 3059181: 3727 return getCode(); 3728 case 1187338559: 3729 return addOrderDetail(); 3730 case -515002347: 3731 return getQuantity(); 3732 case -1285004149: 3733 return getQuantity(); 3734 case -1867885268: 3735 return getSubject(); 3736 case 1524132147: 3737 return getEncounter(); 3738 case -2022646513: 3739 return getOccurrence(); 3740 case 1687874001: 3741 return getOccurrence(); 3742 case -544329575: 3743 return getAsNeeded(); 3744 case -1432923513: 3745 return getAsNeeded(); 3746 case -1500852503: 3747 return getAuthoredOnElement(); 3748 case 693933948: 3749 return getRequester(); 3750 case -901444568: 3751 return getPerformerType(); 3752 case 481140686: 3753 return addPerformer(); 3754 case -58794174: 3755 return addLocationCode(); 3756 case 755866390: 3757 return addLocationReference(); 3758 case 722137681: 3759 return addReasonCode(); 3760 case -1146218137: 3761 return addReasonReference(); 3762 case 73049818: 3763 return addInsurance(); 3764 case 1922406657: 3765 return addSupportingInfo(); 3766 case -2132868344: 3767 return addSpecimen(); 3768 case 1702620169: 3769 return addBodySite(); 3770 case 3387378: 3771 return addNote(); 3772 case 737543241: 3773 return getPatientInstructionElement(); 3774 case 1538891575: 3775 return addRelevantHistory(); 3776 default: 3777 return super.makeProperty(hash, name); 3778 } 3779 3780 } 3781 3782 @Override 3783 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3784 switch (hash) { 3785 case -1618432855: 3786 /* identifier */ return new String[] { "Identifier" }; 3787 case 8911915: 3788 /* instantiatesCanonical */ return new String[] { "canonical" }; 3789 case -1926393373: 3790 /* instantiatesUri */ return new String[] { "uri" }; 3791 case -332612366: 3792 /* basedOn */ return new String[] { "Reference" }; 3793 case -430332865: 3794 /* replaces */ return new String[] { "Reference" }; 3795 case 395923612: 3796 /* requisition */ return new String[] { "Identifier" }; 3797 case -892481550: 3798 /* status */ return new String[] { "code" }; 3799 case -1183762788: 3800 /* intent */ return new String[] { "code" }; 3801 case 50511102: 3802 /* category */ return new String[] { "CodeableConcept" }; 3803 case -1165461084: 3804 /* priority */ return new String[] { "code" }; 3805 case -1788508167: 3806 /* doNotPerform */ return new String[] { "boolean" }; 3807 case 3059181: 3808 /* code */ return new String[] { "CodeableConcept" }; 3809 case 1187338559: 3810 /* orderDetail */ return new String[] { "CodeableConcept" }; 3811 case -1285004149: 3812 /* quantity */ return new String[] { "Quantity", "Ratio", "Range" }; 3813 case -1867885268: 3814 /* subject */ return new String[] { "Reference" }; 3815 case 1524132147: 3816 /* encounter */ return new String[] { "Reference" }; 3817 case 1687874001: 3818 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 3819 case -1432923513: 3820 /* asNeeded */ return new String[] { "boolean", "CodeableConcept" }; 3821 case -1500852503: 3822 /* authoredOn */ return new String[] { "dateTime" }; 3823 case 693933948: 3824 /* requester */ return new String[] { "Reference" }; 3825 case -901444568: 3826 /* performerType */ return new String[] { "CodeableConcept" }; 3827 case 481140686: 3828 /* performer */ return new String[] { "Reference" }; 3829 case -58794174: 3830 /* locationCode */ return new String[] { "CodeableConcept" }; 3831 case 755866390: 3832 /* locationReference */ return new String[] { "Reference" }; 3833 case 722137681: 3834 /* reasonCode */ return new String[] { "CodeableConcept" }; 3835 case -1146218137: 3836 /* reasonReference */ return new String[] { "Reference" }; 3837 case 73049818: 3838 /* insurance */ return new String[] { "Reference" }; 3839 case 1922406657: 3840 /* supportingInfo */ return new String[] { "Reference" }; 3841 case -2132868344: 3842 /* specimen */ return new String[] { "Reference" }; 3843 case 1702620169: 3844 /* bodySite */ return new String[] { "CodeableConcept" }; 3845 case 3387378: 3846 /* note */ return new String[] { "Annotation" }; 3847 case 737543241: 3848 /* patientInstruction */ return new String[] { "string" }; 3849 case 1538891575: 3850 /* relevantHistory */ return new String[] { "Reference" }; 3851 default: 3852 return super.getTypesForProperty(hash, name); 3853 } 3854 3855 } 3856 3857 @Override 3858 public Base addChild(String name) throws FHIRException { 3859 if (name.equals("identifier")) { 3860 return addIdentifier(); 3861 } else if (name.equals("instantiatesCanonical")) { 3862 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.instantiatesCanonical"); 3863 } else if (name.equals("instantiatesUri")) { 3864 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.instantiatesUri"); 3865 } else if (name.equals("basedOn")) { 3866 return addBasedOn(); 3867 } else if (name.equals("replaces")) { 3868 return addReplaces(); 3869 } else if (name.equals("requisition")) { 3870 this.requisition = new Identifier(); 3871 return this.requisition; 3872 } else if (name.equals("status")) { 3873 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.status"); 3874 } else if (name.equals("intent")) { 3875 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.intent"); 3876 } else if (name.equals("category")) { 3877 return addCategory(); 3878 } else if (name.equals("priority")) { 3879 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.priority"); 3880 } else if (name.equals("doNotPerform")) { 3881 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.doNotPerform"); 3882 } else if (name.equals("code")) { 3883 this.code = new CodeableConcept(); 3884 return this.code; 3885 } else if (name.equals("orderDetail")) { 3886 return addOrderDetail(); 3887 } else if (name.equals("quantityQuantity")) { 3888 this.quantity = new Quantity(); 3889 return this.quantity; 3890 } else if (name.equals("quantityRatio")) { 3891 this.quantity = new Ratio(); 3892 return this.quantity; 3893 } else if (name.equals("quantityRange")) { 3894 this.quantity = new Range(); 3895 return this.quantity; 3896 } else if (name.equals("subject")) { 3897 this.subject = new Reference(); 3898 return this.subject; 3899 } else if (name.equals("encounter")) { 3900 this.encounter = new Reference(); 3901 return this.encounter; 3902 } else if (name.equals("occurrenceDateTime")) { 3903 this.occurrence = new DateTimeType(); 3904 return this.occurrence; 3905 } else if (name.equals("occurrencePeriod")) { 3906 this.occurrence = new Period(); 3907 return this.occurrence; 3908 } else if (name.equals("occurrenceTiming")) { 3909 this.occurrence = new Timing(); 3910 return this.occurrence; 3911 } else if (name.equals("asNeededBoolean")) { 3912 this.asNeeded = new BooleanType(); 3913 return this.asNeeded; 3914 } else if (name.equals("asNeededCodeableConcept")) { 3915 this.asNeeded = new CodeableConcept(); 3916 return this.asNeeded; 3917 } else if (name.equals("authoredOn")) { 3918 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.authoredOn"); 3919 } else if (name.equals("requester")) { 3920 this.requester = new Reference(); 3921 return this.requester; 3922 } else if (name.equals("performerType")) { 3923 this.performerType = new CodeableConcept(); 3924 return this.performerType; 3925 } else if (name.equals("performer")) { 3926 return addPerformer(); 3927 } else if (name.equals("locationCode")) { 3928 return addLocationCode(); 3929 } else if (name.equals("locationReference")) { 3930 return addLocationReference(); 3931 } else if (name.equals("reasonCode")) { 3932 return addReasonCode(); 3933 } else if (name.equals("reasonReference")) { 3934 return addReasonReference(); 3935 } else if (name.equals("insurance")) { 3936 return addInsurance(); 3937 } else if (name.equals("supportingInfo")) { 3938 return addSupportingInfo(); 3939 } else if (name.equals("specimen")) { 3940 return addSpecimen(); 3941 } else if (name.equals("bodySite")) { 3942 return addBodySite(); 3943 } else if (name.equals("note")) { 3944 return addNote(); 3945 } else if (name.equals("patientInstruction")) { 3946 throw new FHIRException("Cannot call addChild on a singleton property ServiceRequest.patientInstruction"); 3947 } else if (name.equals("relevantHistory")) { 3948 return addRelevantHistory(); 3949 } else 3950 return super.addChild(name); 3951 } 3952 3953 public String fhirType() { 3954 return "ServiceRequest"; 3955 3956 } 3957 3958 public ServiceRequest copy() { 3959 ServiceRequest dst = new ServiceRequest(); 3960 copyValues(dst); 3961 return dst; 3962 } 3963 3964 public void copyValues(ServiceRequest dst) { 3965 super.copyValues(dst); 3966 if (identifier != null) { 3967 dst.identifier = new ArrayList<Identifier>(); 3968 for (Identifier i : identifier) 3969 dst.identifier.add(i.copy()); 3970 } 3971 ; 3972 if (instantiatesCanonical != null) { 3973 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 3974 for (CanonicalType i : instantiatesCanonical) 3975 dst.instantiatesCanonical.add(i.copy()); 3976 } 3977 ; 3978 if (instantiatesUri != null) { 3979 dst.instantiatesUri = new ArrayList<UriType>(); 3980 for (UriType i : instantiatesUri) 3981 dst.instantiatesUri.add(i.copy()); 3982 } 3983 ; 3984 if (basedOn != null) { 3985 dst.basedOn = new ArrayList<Reference>(); 3986 for (Reference i : basedOn) 3987 dst.basedOn.add(i.copy()); 3988 } 3989 ; 3990 if (replaces != null) { 3991 dst.replaces = new ArrayList<Reference>(); 3992 for (Reference i : replaces) 3993 dst.replaces.add(i.copy()); 3994 } 3995 ; 3996 dst.requisition = requisition == null ? null : requisition.copy(); 3997 dst.status = status == null ? null : status.copy(); 3998 dst.intent = intent == null ? null : intent.copy(); 3999 if (category != null) { 4000 dst.category = new ArrayList<CodeableConcept>(); 4001 for (CodeableConcept i : category) 4002 dst.category.add(i.copy()); 4003 } 4004 ; 4005 dst.priority = priority == null ? null : priority.copy(); 4006 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 4007 dst.code = code == null ? null : code.copy(); 4008 if (orderDetail != null) { 4009 dst.orderDetail = new ArrayList<CodeableConcept>(); 4010 for (CodeableConcept i : orderDetail) 4011 dst.orderDetail.add(i.copy()); 4012 } 4013 ; 4014 dst.quantity = quantity == null ? null : quantity.copy(); 4015 dst.subject = subject == null ? null : subject.copy(); 4016 dst.encounter = encounter == null ? null : encounter.copy(); 4017 dst.occurrence = occurrence == null ? null : occurrence.copy(); 4018 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 4019 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 4020 dst.requester = requester == null ? null : requester.copy(); 4021 dst.performerType = performerType == null ? null : performerType.copy(); 4022 if (performer != null) { 4023 dst.performer = new ArrayList<Reference>(); 4024 for (Reference i : performer) 4025 dst.performer.add(i.copy()); 4026 } 4027 ; 4028 if (locationCode != null) { 4029 dst.locationCode = new ArrayList<CodeableConcept>(); 4030 for (CodeableConcept i : locationCode) 4031 dst.locationCode.add(i.copy()); 4032 } 4033 ; 4034 if (locationReference != null) { 4035 dst.locationReference = new ArrayList<Reference>(); 4036 for (Reference i : locationReference) 4037 dst.locationReference.add(i.copy()); 4038 } 4039 ; 4040 if (reasonCode != null) { 4041 dst.reasonCode = new ArrayList<CodeableConcept>(); 4042 for (CodeableConcept i : reasonCode) 4043 dst.reasonCode.add(i.copy()); 4044 } 4045 ; 4046 if (reasonReference != null) { 4047 dst.reasonReference = new ArrayList<Reference>(); 4048 for (Reference i : reasonReference) 4049 dst.reasonReference.add(i.copy()); 4050 } 4051 ; 4052 if (insurance != null) { 4053 dst.insurance = new ArrayList<Reference>(); 4054 for (Reference i : insurance) 4055 dst.insurance.add(i.copy()); 4056 } 4057 ; 4058 if (supportingInfo != null) { 4059 dst.supportingInfo = new ArrayList<Reference>(); 4060 for (Reference i : supportingInfo) 4061 dst.supportingInfo.add(i.copy()); 4062 } 4063 ; 4064 if (specimen != null) { 4065 dst.specimen = new ArrayList<Reference>(); 4066 for (Reference i : specimen) 4067 dst.specimen.add(i.copy()); 4068 } 4069 ; 4070 if (bodySite != null) { 4071 dst.bodySite = new ArrayList<CodeableConcept>(); 4072 for (CodeableConcept i : bodySite) 4073 dst.bodySite.add(i.copy()); 4074 } 4075 ; 4076 if (note != null) { 4077 dst.note = new ArrayList<Annotation>(); 4078 for (Annotation i : note) 4079 dst.note.add(i.copy()); 4080 } 4081 ; 4082 dst.patientInstruction = patientInstruction == null ? null : patientInstruction.copy(); 4083 if (relevantHistory != null) { 4084 dst.relevantHistory = new ArrayList<Reference>(); 4085 for (Reference i : relevantHistory) 4086 dst.relevantHistory.add(i.copy()); 4087 } 4088 ; 4089 } 4090 4091 protected ServiceRequest typedCopy() { 4092 return copy(); 4093 } 4094 4095 @Override 4096 public boolean equalsDeep(Base other_) { 4097 if (!super.equalsDeep(other_)) 4098 return false; 4099 if (!(other_ instanceof ServiceRequest)) 4100 return false; 4101 ServiceRequest o = (ServiceRequest) other_; 4102 return compareDeep(identifier, o.identifier, true) 4103 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 4104 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 4105 && compareDeep(replaces, o.replaces, true) && compareDeep(requisition, o.requisition, true) 4106 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) 4107 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 4108 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(code, o.code, true) 4109 && compareDeep(orderDetail, o.orderDetail, true) && compareDeep(quantity, o.quantity, true) 4110 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 4111 && compareDeep(occurrence, o.occurrence, true) && compareDeep(asNeeded, o.asNeeded, true) 4112 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 4113 && compareDeep(performerType, o.performerType, true) && compareDeep(performer, o.performer, true) 4114 && compareDeep(locationCode, o.locationCode, true) && compareDeep(locationReference, o.locationReference, true) 4115 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 4116 && compareDeep(insurance, o.insurance, true) && compareDeep(supportingInfo, o.supportingInfo, true) 4117 && compareDeep(specimen, o.specimen, true) && compareDeep(bodySite, o.bodySite, true) 4118 && compareDeep(note, o.note, true) && compareDeep(patientInstruction, o.patientInstruction, true) 4119 && compareDeep(relevantHistory, o.relevantHistory, true); 4120 } 4121 4122 @Override 4123 public boolean equalsShallow(Base other_) { 4124 if (!super.equalsShallow(other_)) 4125 return false; 4126 if (!(other_ instanceof ServiceRequest)) 4127 return false; 4128 ServiceRequest o = (ServiceRequest) other_; 4129 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 4130 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 4131 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(authoredOn, o.authoredOn, true) 4132 && compareValues(patientInstruction, o.patientInstruction, true); 4133 } 4134 4135 public boolean isEmpty() { 4136 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 4137 basedOn, replaces, requisition, status, intent, category, priority, doNotPerform, code, orderDetail, quantity, 4138 subject, encounter, occurrence, asNeeded, authoredOn, requester, performerType, performer, locationCode, 4139 locationReference, reasonCode, reasonReference, insurance, supportingInfo, specimen, bodySite, note, 4140 patientInstruction, relevantHistory); 4141 } 4142 4143 @Override 4144 public ResourceType getResourceType() { 4145 return ResourceType.ServiceRequest; 4146 } 4147 4148 /** 4149 * Search parameter: <b>authored</b> 4150 * <p> 4151 * Description: <b>Date request signed</b><br> 4152 * Type: <b>date</b><br> 4153 * Path: <b>ServiceRequest.authoredOn</b><br> 4154 * </p> 4155 */ 4156 @SearchParamDefinition(name = "authored", path = "ServiceRequest.authoredOn", description = "Date request signed", type = "date") 4157 public static final String SP_AUTHORED = "authored"; 4158 /** 4159 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 4160 * <p> 4161 * Description: <b>Date request signed</b><br> 4162 * Type: <b>date</b><br> 4163 * Path: <b>ServiceRequest.authoredOn</b><br> 4164 * </p> 4165 */ 4166 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam( 4167 SP_AUTHORED); 4168 4169 /** 4170 * Search parameter: <b>requester</b> 4171 * <p> 4172 * Description: <b>Who/what is requesting service</b><br> 4173 * Type: <b>reference</b><br> 4174 * Path: <b>ServiceRequest.requester</b><br> 4175 * </p> 4176 */ 4177 @SearchParamDefinition(name = "requester", path = "ServiceRequest.requester", description = "Who/what is requesting service", type = "reference", providesMembershipIn = { 4178 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 4179 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 4180 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4181 public static final String SP_REQUESTER = "requester"; 4182 /** 4183 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 4184 * <p> 4185 * Description: <b>Who/what is requesting service</b><br> 4186 * Type: <b>reference</b><br> 4187 * Path: <b>ServiceRequest.requester</b><br> 4188 * </p> 4189 */ 4190 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4191 SP_REQUESTER); 4192 4193 /** 4194 * Constant for fluent queries to be used to add include statements. Specifies 4195 * the path value of "<b>ServiceRequest:requester</b>". 4196 */ 4197 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 4198 "ServiceRequest:requester").toLocked(); 4199 4200 /** 4201 * Search parameter: <b>identifier</b> 4202 * <p> 4203 * Description: <b>Identifiers assigned to this order</b><br> 4204 * Type: <b>token</b><br> 4205 * Path: <b>ServiceRequest.identifier</b><br> 4206 * </p> 4207 */ 4208 @SearchParamDefinition(name = "identifier", path = "ServiceRequest.identifier", description = "Identifiers assigned to this order", type = "token") 4209 public static final String SP_IDENTIFIER = "identifier"; 4210 /** 4211 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4212 * <p> 4213 * Description: <b>Identifiers assigned to this order</b><br> 4214 * Type: <b>token</b><br> 4215 * Path: <b>ServiceRequest.identifier</b><br> 4216 * </p> 4217 */ 4218 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4219 SP_IDENTIFIER); 4220 4221 /** 4222 * Search parameter: <b>code</b> 4223 * <p> 4224 * Description: <b>What is being requested/ordered</b><br> 4225 * Type: <b>token</b><br> 4226 * Path: <b>ServiceRequest.code</b><br> 4227 * </p> 4228 */ 4229 @SearchParamDefinition(name = "code", path = "ServiceRequest.code", description = "What is being requested/ordered", type = "token") 4230 public static final String SP_CODE = "code"; 4231 /** 4232 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4233 * <p> 4234 * Description: <b>What is being requested/ordered</b><br> 4235 * Type: <b>token</b><br> 4236 * Path: <b>ServiceRequest.code</b><br> 4237 * </p> 4238 */ 4239 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4240 SP_CODE); 4241 4242 /** 4243 * Search parameter: <b>performer</b> 4244 * <p> 4245 * Description: <b>Requested performer</b><br> 4246 * Type: <b>reference</b><br> 4247 * Path: <b>ServiceRequest.performer</b><br> 4248 * </p> 4249 */ 4250 @SearchParamDefinition(name = "performer", path = "ServiceRequest.performer", description = "Requested performer", type = "reference", providesMembershipIn = { 4251 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 4252 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4253 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 4254 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 4255 HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 4256 RelatedPerson.class }) 4257 public static final String SP_PERFORMER = "performer"; 4258 /** 4259 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4260 * <p> 4261 * Description: <b>Requested performer</b><br> 4262 * Type: <b>reference</b><br> 4263 * Path: <b>ServiceRequest.performer</b><br> 4264 * </p> 4265 */ 4266 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4267 SP_PERFORMER); 4268 4269 /** 4270 * Constant for fluent queries to be used to add include statements. Specifies 4271 * the path value of "<b>ServiceRequest:performer</b>". 4272 */ 4273 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 4274 "ServiceRequest:performer").toLocked(); 4275 4276 /** 4277 * Search parameter: <b>requisition</b> 4278 * <p> 4279 * Description: <b>Composite Request ID</b><br> 4280 * Type: <b>token</b><br> 4281 * Path: <b>ServiceRequest.requisition</b><br> 4282 * </p> 4283 */ 4284 @SearchParamDefinition(name = "requisition", path = "ServiceRequest.requisition", description = "Composite Request ID", type = "token") 4285 public static final String SP_REQUISITION = "requisition"; 4286 /** 4287 * <b>Fluent Client</b> search parameter constant for <b>requisition</b> 4288 * <p> 4289 * Description: <b>Composite Request ID</b><br> 4290 * Type: <b>token</b><br> 4291 * Path: <b>ServiceRequest.requisition</b><br> 4292 * </p> 4293 */ 4294 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REQUISITION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4295 SP_REQUISITION); 4296 4297 /** 4298 * Search parameter: <b>replaces</b> 4299 * <p> 4300 * Description: <b>What request replaces</b><br> 4301 * Type: <b>reference</b><br> 4302 * Path: <b>ServiceRequest.replaces</b><br> 4303 * </p> 4304 */ 4305 @SearchParamDefinition(name = "replaces", path = "ServiceRequest.replaces", description = "What request replaces", type = "reference", target = { 4306 ServiceRequest.class }) 4307 public static final String SP_REPLACES = "replaces"; 4308 /** 4309 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 4310 * <p> 4311 * Description: <b>What request replaces</b><br> 4312 * Type: <b>reference</b><br> 4313 * Path: <b>ServiceRequest.replaces</b><br> 4314 * </p> 4315 */ 4316 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4317 SP_REPLACES); 4318 4319 /** 4320 * Constant for fluent queries to be used to add include statements. Specifies 4321 * the path value of "<b>ServiceRequest:replaces</b>". 4322 */ 4323 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include( 4324 "ServiceRequest:replaces").toLocked(); 4325 4326 /** 4327 * Search parameter: <b>subject</b> 4328 * <p> 4329 * Description: <b>Search by subject</b><br> 4330 * Type: <b>reference</b><br> 4331 * Path: <b>ServiceRequest.subject</b><br> 4332 * </p> 4333 */ 4334 @SearchParamDefinition(name = "subject", path = "ServiceRequest.subject", description = "Search by subject", type = "reference", providesMembershipIn = { 4335 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Device.class, Group.class, 4336 Location.class, Patient.class }) 4337 public static final String SP_SUBJECT = "subject"; 4338 /** 4339 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4340 * <p> 4341 * Description: <b>Search by subject</b><br> 4342 * Type: <b>reference</b><br> 4343 * Path: <b>ServiceRequest.subject</b><br> 4344 * </p> 4345 */ 4346 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4347 SP_SUBJECT); 4348 4349 /** 4350 * Constant for fluent queries to be used to add include statements. Specifies 4351 * the path value of "<b>ServiceRequest:subject</b>". 4352 */ 4353 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 4354 "ServiceRequest:subject").toLocked(); 4355 4356 /** 4357 * Search parameter: <b>instantiates-canonical</b> 4358 * <p> 4359 * Description: <b>Instantiates FHIR protocol or definition</b><br> 4360 * Type: <b>reference</b><br> 4361 * Path: <b>ServiceRequest.instantiatesCanonical</b><br> 4362 * </p> 4363 */ 4364 @SearchParamDefinition(name = "instantiates-canonical", path = "ServiceRequest.instantiatesCanonical", description = "Instantiates FHIR protocol or definition", type = "reference", target = { 4365 ActivityDefinition.class, PlanDefinition.class }) 4366 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 4367 /** 4368 * <b>Fluent Client</b> search parameter constant for 4369 * <b>instantiates-canonical</b> 4370 * <p> 4371 * Description: <b>Instantiates FHIR protocol or definition</b><br> 4372 * Type: <b>reference</b><br> 4373 * Path: <b>ServiceRequest.instantiatesCanonical</b><br> 4374 * </p> 4375 */ 4376 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4377 SP_INSTANTIATES_CANONICAL); 4378 4379 /** 4380 * Constant for fluent queries to be used to add include statements. Specifies 4381 * the path value of "<b>ServiceRequest:instantiates-canonical</b>". 4382 */ 4383 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 4384 "ServiceRequest:instantiates-canonical").toLocked(); 4385 4386 /** 4387 * Search parameter: <b>encounter</b> 4388 * <p> 4389 * Description: <b>An encounter in which this request is made</b><br> 4390 * Type: <b>reference</b><br> 4391 * Path: <b>ServiceRequest.encounter</b><br> 4392 * </p> 4393 */ 4394 @SearchParamDefinition(name = "encounter", path = "ServiceRequest.encounter", description = "An encounter in which this request is made", type = "reference", providesMembershipIn = { 4395 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 4396 public static final String SP_ENCOUNTER = "encounter"; 4397 /** 4398 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4399 * <p> 4400 * Description: <b>An encounter in which this request is made</b><br> 4401 * Type: <b>reference</b><br> 4402 * Path: <b>ServiceRequest.encounter</b><br> 4403 * </p> 4404 */ 4405 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4406 SP_ENCOUNTER); 4407 4408 /** 4409 * Constant for fluent queries to be used to add include statements. Specifies 4410 * the path value of "<b>ServiceRequest:encounter</b>". 4411 */ 4412 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 4413 "ServiceRequest:encounter").toLocked(); 4414 4415 /** 4416 * Search parameter: <b>occurrence</b> 4417 * <p> 4418 * Description: <b>When service should occur</b><br> 4419 * Type: <b>date</b><br> 4420 * Path: <b>ServiceRequest.occurrence[x]</b><br> 4421 * </p> 4422 */ 4423 @SearchParamDefinition(name = "occurrence", path = "ServiceRequest.occurrence", description = "When service should occur", type = "date") 4424 public static final String SP_OCCURRENCE = "occurrence"; 4425 /** 4426 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 4427 * <p> 4428 * Description: <b>When service should occur</b><br> 4429 * Type: <b>date</b><br> 4430 * Path: <b>ServiceRequest.occurrence[x]</b><br> 4431 * </p> 4432 */ 4433 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4434 SP_OCCURRENCE); 4435 4436 /** 4437 * Search parameter: <b>priority</b> 4438 * <p> 4439 * Description: <b>routine | urgent | asap | stat</b><br> 4440 * Type: <b>token</b><br> 4441 * Path: <b>ServiceRequest.priority</b><br> 4442 * </p> 4443 */ 4444 @SearchParamDefinition(name = "priority", path = "ServiceRequest.priority", description = "routine | urgent | asap | stat", type = "token") 4445 public static final String SP_PRIORITY = "priority"; 4446 /** 4447 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 4448 * <p> 4449 * Description: <b>routine | urgent | asap | stat</b><br> 4450 * Type: <b>token</b><br> 4451 * Path: <b>ServiceRequest.priority</b><br> 4452 * </p> 4453 */ 4454 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4455 SP_PRIORITY); 4456 4457 /** 4458 * Search parameter: <b>intent</b> 4459 * <p> 4460 * Description: <b>proposal | plan | directive | order | original-order | 4461 * reflex-order | filler-order | instance-order | option</b><br> 4462 * Type: <b>token</b><br> 4463 * Path: <b>ServiceRequest.intent</b><br> 4464 * </p> 4465 */ 4466 @SearchParamDefinition(name = "intent", path = "ServiceRequest.intent", description = "proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", type = "token") 4467 public static final String SP_INTENT = "intent"; 4468 /** 4469 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 4470 * <p> 4471 * Description: <b>proposal | plan | directive | order | original-order | 4472 * reflex-order | filler-order | instance-order | option</b><br> 4473 * Type: <b>token</b><br> 4474 * Path: <b>ServiceRequest.intent</b><br> 4475 * </p> 4476 */ 4477 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4478 SP_INTENT); 4479 4480 /** 4481 * Search parameter: <b>performer-type</b> 4482 * <p> 4483 * Description: <b>Performer role</b><br> 4484 * Type: <b>token</b><br> 4485 * Path: <b>ServiceRequest.performerType</b><br> 4486 * </p> 4487 */ 4488 @SearchParamDefinition(name = "performer-type", path = "ServiceRequest.performerType", description = "Performer role", type = "token") 4489 public static final String SP_PERFORMER_TYPE = "performer-type"; 4490 /** 4491 * <b>Fluent Client</b> search parameter constant for <b>performer-type</b> 4492 * <p> 4493 * Description: <b>Performer role</b><br> 4494 * Type: <b>token</b><br> 4495 * Path: <b>ServiceRequest.performerType</b><br> 4496 * </p> 4497 */ 4498 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4499 SP_PERFORMER_TYPE); 4500 4501 /** 4502 * Search parameter: <b>based-on</b> 4503 * <p> 4504 * Description: <b>What request fulfills</b><br> 4505 * Type: <b>reference</b><br> 4506 * Path: <b>ServiceRequest.basedOn</b><br> 4507 * </p> 4508 */ 4509 @SearchParamDefinition(name = "based-on", path = "ServiceRequest.basedOn", description = "What request fulfills", type = "reference", target = { 4510 CarePlan.class, MedicationRequest.class, ServiceRequest.class }) 4511 public static final String SP_BASED_ON = "based-on"; 4512 /** 4513 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 4514 * <p> 4515 * Description: <b>What request fulfills</b><br> 4516 * Type: <b>reference</b><br> 4517 * Path: <b>ServiceRequest.basedOn</b><br> 4518 * </p> 4519 */ 4520 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4521 SP_BASED_ON); 4522 4523 /** 4524 * Constant for fluent queries to be used to add include statements. Specifies 4525 * the path value of "<b>ServiceRequest:based-on</b>". 4526 */ 4527 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 4528 "ServiceRequest:based-on").toLocked(); 4529 4530 /** 4531 * Search parameter: <b>patient</b> 4532 * <p> 4533 * Description: <b>Search by subject - a patient</b><br> 4534 * Type: <b>reference</b><br> 4535 * Path: <b>ServiceRequest.subject</b><br> 4536 * </p> 4537 */ 4538 @SearchParamDefinition(name = "patient", path = "ServiceRequest.subject.where(resolve() is Patient)", description = "Search by subject - a patient", type = "reference", target = { 4539 Patient.class }) 4540 public static final String SP_PATIENT = "patient"; 4541 /** 4542 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4543 * <p> 4544 * Description: <b>Search by subject - a patient</b><br> 4545 * Type: <b>reference</b><br> 4546 * Path: <b>ServiceRequest.subject</b><br> 4547 * </p> 4548 */ 4549 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4550 SP_PATIENT); 4551 4552 /** 4553 * Constant for fluent queries to be used to add include statements. Specifies 4554 * the path value of "<b>ServiceRequest:patient</b>". 4555 */ 4556 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4557 "ServiceRequest:patient").toLocked(); 4558 4559 /** 4560 * Search parameter: <b>specimen</b> 4561 * <p> 4562 * Description: <b>Specimen to be tested</b><br> 4563 * Type: <b>reference</b><br> 4564 * Path: <b>ServiceRequest.specimen</b><br> 4565 * </p> 4566 */ 4567 @SearchParamDefinition(name = "specimen", path = "ServiceRequest.specimen", description = "Specimen to be tested", type = "reference", target = { 4568 Specimen.class }) 4569 public static final String SP_SPECIMEN = "specimen"; 4570 /** 4571 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 4572 * <p> 4573 * Description: <b>Specimen to be tested</b><br> 4574 * Type: <b>reference</b><br> 4575 * Path: <b>ServiceRequest.specimen</b><br> 4576 * </p> 4577 */ 4578 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4579 SP_SPECIMEN); 4580 4581 /** 4582 * Constant for fluent queries to be used to add include statements. Specifies 4583 * the path value of "<b>ServiceRequest:specimen</b>". 4584 */ 4585 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include( 4586 "ServiceRequest:specimen").toLocked(); 4587 4588 /** 4589 * Search parameter: <b>instantiates-uri</b> 4590 * <p> 4591 * Description: <b>Instantiates external protocol or definition</b><br> 4592 * Type: <b>uri</b><br> 4593 * Path: <b>ServiceRequest.instantiatesUri</b><br> 4594 * </p> 4595 */ 4596 @SearchParamDefinition(name = "instantiates-uri", path = "ServiceRequest.instantiatesUri", description = "Instantiates external protocol or definition", type = "uri") 4597 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 4598 /** 4599 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 4600 * <p> 4601 * Description: <b>Instantiates external protocol or definition</b><br> 4602 * Type: <b>uri</b><br> 4603 * Path: <b>ServiceRequest.instantiatesUri</b><br> 4604 * </p> 4605 */ 4606 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 4607 SP_INSTANTIATES_URI); 4608 4609 /** 4610 * Search parameter: <b>body-site</b> 4611 * <p> 4612 * Description: <b>Where procedure is going to be done</b><br> 4613 * Type: <b>token</b><br> 4614 * Path: <b>ServiceRequest.bodySite</b><br> 4615 * </p> 4616 */ 4617 @SearchParamDefinition(name = "body-site", path = "ServiceRequest.bodySite", description = "Where procedure is going to be done", type = "token") 4618 public static final String SP_BODY_SITE = "body-site"; 4619 /** 4620 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 4621 * <p> 4622 * Description: <b>Where procedure is going to be done</b><br> 4623 * Type: <b>token</b><br> 4624 * Path: <b>ServiceRequest.bodySite</b><br> 4625 * </p> 4626 */ 4627 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4628 SP_BODY_SITE); 4629 4630 /** 4631 * Search parameter: <b>category</b> 4632 * <p> 4633 * Description: <b>Classification of service</b><br> 4634 * Type: <b>token</b><br> 4635 * Path: <b>ServiceRequest.category</b><br> 4636 * </p> 4637 */ 4638 @SearchParamDefinition(name = "category", path = "ServiceRequest.category", description = "Classification of service", type = "token") 4639 public static final String SP_CATEGORY = "category"; 4640 /** 4641 * <b>Fluent Client</b> search parameter constant for <b>category</b> 4642 * <p> 4643 * Description: <b>Classification of service</b><br> 4644 * Type: <b>token</b><br> 4645 * Path: <b>ServiceRequest.category</b><br> 4646 * </p> 4647 */ 4648 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4649 SP_CATEGORY); 4650 4651 /** 4652 * Search parameter: <b>status</b> 4653 * <p> 4654 * Description: <b>draft | active | on-hold | revoked | completed | 4655 * entered-in-error | unknown</b><br> 4656 * Type: <b>token</b><br> 4657 * Path: <b>ServiceRequest.status</b><br> 4658 * </p> 4659 */ 4660 @SearchParamDefinition(name = "status", path = "ServiceRequest.status", description = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", type = "token") 4661 public static final String SP_STATUS = "status"; 4662 /** 4663 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4664 * <p> 4665 * Description: <b>draft | active | on-hold | revoked | completed | 4666 * entered-in-error | unknown</b><br> 4667 * Type: <b>token</b><br> 4668 * Path: <b>ServiceRequest.status</b><br> 4669 * </p> 4670 */ 4671 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4672 SP_STATUS); 4673 4674}