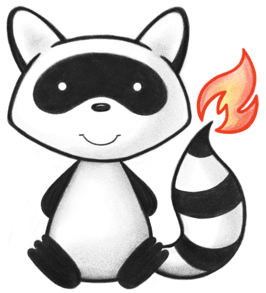
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * A slot of time on a schedule that may be available for booking appointments. 047 */ 048@ResourceDef(name = "Slot", profile = "http://hl7.org/fhir/StructureDefinition/Slot") 049public class Slot extends DomainResource { 050 051 public enum SlotStatus { 052 /** 053 * Indicates that the time interval is busy because one or more events have been 054 * scheduled for that interval. 055 */ 056 BUSY, 057 /** 058 * Indicates that the time interval is free for scheduling. 059 */ 060 FREE, 061 /** 062 * Indicates that the time interval is busy and that the interval cannot be 063 * scheduled. 064 */ 065 BUSYUNAVAILABLE, 066 /** 067 * Indicates that the time interval is busy because one or more events have been 068 * tentatively scheduled for that interval. 069 */ 070 BUSYTENTATIVE, 071 /** 072 * This instance should not have been part of this patient's medical record. 073 */ 074 ENTEREDINERROR, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 080 public static SlotStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("busy".equals(codeString)) 084 return BUSY; 085 if ("free".equals(codeString)) 086 return FREE; 087 if ("busy-unavailable".equals(codeString)) 088 return BUSYUNAVAILABLE; 089 if ("busy-tentative".equals(codeString)) 090 return BUSYTENTATIVE; 091 if ("entered-in-error".equals(codeString)) 092 return ENTEREDINERROR; 093 if (Configuration.isAcceptInvalidEnums()) 094 return null; 095 else 096 throw new FHIRException("Unknown SlotStatus code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case BUSY: 102 return "busy"; 103 case FREE: 104 return "free"; 105 case BUSYUNAVAILABLE: 106 return "busy-unavailable"; 107 case BUSYTENTATIVE: 108 return "busy-tentative"; 109 case ENTEREDINERROR: 110 return "entered-in-error"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getSystem() { 119 switch (this) { 120 case BUSY: 121 return "http://hl7.org/fhir/slotstatus"; 122 case FREE: 123 return "http://hl7.org/fhir/slotstatus"; 124 case BUSYUNAVAILABLE: 125 return "http://hl7.org/fhir/slotstatus"; 126 case BUSYTENTATIVE: 127 return "http://hl7.org/fhir/slotstatus"; 128 case ENTEREDINERROR: 129 return "http://hl7.org/fhir/slotstatus"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case BUSY: 140 return "Indicates that the time interval is busy because one or more events have been scheduled for that interval."; 141 case FREE: 142 return "Indicates that the time interval is free for scheduling."; 143 case BUSYUNAVAILABLE: 144 return "Indicates that the time interval is busy and that the interval cannot be scheduled."; 145 case BUSYTENTATIVE: 146 return "Indicates that the time interval is busy because one or more events have been tentatively scheduled for that interval."; 147 case ENTEREDINERROR: 148 return "This instance should not have been part of this patient's medical record."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case BUSY: 159 return "Busy"; 160 case FREE: 161 return "Free"; 162 case BUSYUNAVAILABLE: 163 return "Busy (Unavailable)"; 164 case BUSYTENTATIVE: 165 return "Busy (Tentative)"; 166 case ENTEREDINERROR: 167 return "Entered in error"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 } 175 176 public static class SlotStatusEnumFactory implements EnumFactory<SlotStatus> { 177 public SlotStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("busy".equals(codeString)) 182 return SlotStatus.BUSY; 183 if ("free".equals(codeString)) 184 return SlotStatus.FREE; 185 if ("busy-unavailable".equals(codeString)) 186 return SlotStatus.BUSYUNAVAILABLE; 187 if ("busy-tentative".equals(codeString)) 188 return SlotStatus.BUSYTENTATIVE; 189 if ("entered-in-error".equals(codeString)) 190 return SlotStatus.ENTEREDINERROR; 191 throw new IllegalArgumentException("Unknown SlotStatus code '" + codeString + "'"); 192 } 193 194 public Enumeration<SlotStatus> fromType(PrimitiveType<?> code) throws FHIRException { 195 if (code == null) 196 return null; 197 if (code.isEmpty()) 198 return new Enumeration<SlotStatus>(this, SlotStatus.NULL, code); 199 String codeString = code.asStringValue(); 200 if (codeString == null || "".equals(codeString)) 201 return new Enumeration<SlotStatus>(this, SlotStatus.NULL, code); 202 if ("busy".equals(codeString)) 203 return new Enumeration<SlotStatus>(this, SlotStatus.BUSY, code); 204 if ("free".equals(codeString)) 205 return new Enumeration<SlotStatus>(this, SlotStatus.FREE, code); 206 if ("busy-unavailable".equals(codeString)) 207 return new Enumeration<SlotStatus>(this, SlotStatus.BUSYUNAVAILABLE, code); 208 if ("busy-tentative".equals(codeString)) 209 return new Enumeration<SlotStatus>(this, SlotStatus.BUSYTENTATIVE, code); 210 if ("entered-in-error".equals(codeString)) 211 return new Enumeration<SlotStatus>(this, SlotStatus.ENTEREDINERROR, code); 212 throw new FHIRException("Unknown SlotStatus code '" + codeString + "'"); 213 } 214 215 public String toCode(SlotStatus code) { 216 if (code == SlotStatus.NULL) 217 return null; 218 if (code == SlotStatus.BUSY) 219 return "busy"; 220 if (code == SlotStatus.FREE) 221 return "free"; 222 if (code == SlotStatus.BUSYUNAVAILABLE) 223 return "busy-unavailable"; 224 if (code == SlotStatus.BUSYTENTATIVE) 225 return "busy-tentative"; 226 if (code == SlotStatus.ENTEREDINERROR) 227 return "entered-in-error"; 228 return "?"; 229 } 230 231 public String toSystem(SlotStatus code) { 232 return code.getSystem(); 233 } 234 } 235 236 /** 237 * External Ids for this item. 238 */ 239 @Child(name = "identifier", type = { 240 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 241 @Description(shortDefinition = "External Ids for this item", formalDefinition = "External Ids for this item.") 242 protected List<Identifier> identifier; 243 244 /** 245 * A broad categorization of the service that is to be performed during this 246 * appointment. 247 */ 248 @Child(name = "serviceCategory", type = { 249 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 250 @Description(shortDefinition = "A broad categorization of the service that is to be performed during this appointment", formalDefinition = "A broad categorization of the service that is to be performed during this appointment.") 251 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-category") 252 protected List<CodeableConcept> serviceCategory; 253 254 /** 255 * The type of appointments that can be booked into this slot (ideally this 256 * would be an identifiable service - which is at a location, rather than the 257 * location itself). If provided then this overrides the value provided on the 258 * availability resource. 259 */ 260 @Child(name = "serviceType", type = { 261 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 262 @Description(shortDefinition = "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource", formalDefinition = "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.") 263 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 264 protected List<CodeableConcept> serviceType; 265 266 /** 267 * The specialty of a practitioner that would be required to perform the service 268 * requested in this appointment. 269 */ 270 @Child(name = "specialty", type = { 271 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 272 @Description(shortDefinition = "The specialty of a practitioner that would be required to perform the service requested in this appointment", formalDefinition = "The specialty of a practitioner that would be required to perform the service requested in this appointment.") 273 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 274 protected List<CodeableConcept> specialty; 275 276 /** 277 * The style of appointment or patient that may be booked in the slot (not 278 * service type). 279 */ 280 @Child(name = "appointmentType", type = { 281 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 282 @Description(shortDefinition = "The style of appointment or patient that may be booked in the slot (not service type)", formalDefinition = "The style of appointment or patient that may be booked in the slot (not service type).") 283 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0276") 284 protected CodeableConcept appointmentType; 285 286 /** 287 * The schedule resource that this slot defines an interval of status 288 * information. 289 */ 290 @Child(name = "schedule", type = { Schedule.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 291 @Description(shortDefinition = "The schedule resource that this slot defines an interval of status information", formalDefinition = "The schedule resource that this slot defines an interval of status information.") 292 protected Reference schedule; 293 294 /** 295 * The actual object that is the target of the reference (The schedule resource 296 * that this slot defines an interval of status information.) 297 */ 298 protected Schedule scheduleTarget; 299 300 /** 301 * busy | free | busy-unavailable | busy-tentative | entered-in-error. 302 */ 303 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 304 @Description(shortDefinition = "busy | free | busy-unavailable | busy-tentative | entered-in-error", formalDefinition = "busy | free | busy-unavailable | busy-tentative | entered-in-error.") 305 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/slotstatus") 306 protected Enumeration<SlotStatus> status; 307 308 /** 309 * Date/Time that the slot is to begin. 310 */ 311 @Child(name = "start", type = { InstantType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 312 @Description(shortDefinition = "Date/Time that the slot is to begin", formalDefinition = "Date/Time that the slot is to begin.") 313 protected InstantType start; 314 315 /** 316 * Date/Time that the slot is to conclude. 317 */ 318 @Child(name = "end", type = { InstantType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 319 @Description(shortDefinition = "Date/Time that the slot is to conclude", formalDefinition = "Date/Time that the slot is to conclude.") 320 protected InstantType end; 321 322 /** 323 * This slot has already been overbooked, appointments are unlikely to be 324 * accepted for this time. 325 */ 326 @Child(name = "overbooked", type = { 327 BooleanType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 328 @Description(shortDefinition = "This slot has already been overbooked, appointments are unlikely to be accepted for this time", formalDefinition = "This slot has already been overbooked, appointments are unlikely to be accepted for this time.") 329 protected BooleanType overbooked; 330 331 /** 332 * Comments on the slot to describe any extended information. Such as custom 333 * constraints on the slot. 334 */ 335 @Child(name = "comment", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 336 @Description(shortDefinition = "Comments on the slot to describe any extended information. Such as custom constraints on the slot", formalDefinition = "Comments on the slot to describe any extended information. Such as custom constraints on the slot.") 337 protected StringType comment; 338 339 private static final long serialVersionUID = 683481856L; 340 341 /** 342 * Constructor 343 */ 344 public Slot() { 345 super(); 346 } 347 348 /** 349 * Constructor 350 */ 351 public Slot(Reference schedule, Enumeration<SlotStatus> status, InstantType start, InstantType end) { 352 super(); 353 this.schedule = schedule; 354 this.status = status; 355 this.start = start; 356 this.end = end; 357 } 358 359 /** 360 * @return {@link #identifier} (External Ids for this item.) 361 */ 362 public List<Identifier> getIdentifier() { 363 if (this.identifier == null) 364 this.identifier = new ArrayList<Identifier>(); 365 return this.identifier; 366 } 367 368 /** 369 * @return Returns a reference to <code>this</code> for easy method chaining 370 */ 371 public Slot setIdentifier(List<Identifier> theIdentifier) { 372 this.identifier = theIdentifier; 373 return this; 374 } 375 376 public boolean hasIdentifier() { 377 if (this.identifier == null) 378 return false; 379 for (Identifier item : this.identifier) 380 if (!item.isEmpty()) 381 return true; 382 return false; 383 } 384 385 public Identifier addIdentifier() { // 3 386 Identifier t = new Identifier(); 387 if (this.identifier == null) 388 this.identifier = new ArrayList<Identifier>(); 389 this.identifier.add(t); 390 return t; 391 } 392 393 public Slot addIdentifier(Identifier t) { // 3 394 if (t == null) 395 return this; 396 if (this.identifier == null) 397 this.identifier = new ArrayList<Identifier>(); 398 this.identifier.add(t); 399 return this; 400 } 401 402 /** 403 * @return The first repetition of repeating field {@link #identifier}, creating 404 * it if it does not already exist 405 */ 406 public Identifier getIdentifierFirstRep() { 407 if (getIdentifier().isEmpty()) { 408 addIdentifier(); 409 } 410 return getIdentifier().get(0); 411 } 412 413 /** 414 * @return {@link #serviceCategory} (A broad categorization of the service that 415 * is to be performed during this appointment.) 416 */ 417 public List<CodeableConcept> getServiceCategory() { 418 if (this.serviceCategory == null) 419 this.serviceCategory = new ArrayList<CodeableConcept>(); 420 return this.serviceCategory; 421 } 422 423 /** 424 * @return Returns a reference to <code>this</code> for easy method chaining 425 */ 426 public Slot setServiceCategory(List<CodeableConcept> theServiceCategory) { 427 this.serviceCategory = theServiceCategory; 428 return this; 429 } 430 431 public boolean hasServiceCategory() { 432 if (this.serviceCategory == null) 433 return false; 434 for (CodeableConcept item : this.serviceCategory) 435 if (!item.isEmpty()) 436 return true; 437 return false; 438 } 439 440 public CodeableConcept addServiceCategory() { // 3 441 CodeableConcept t = new CodeableConcept(); 442 if (this.serviceCategory == null) 443 this.serviceCategory = new ArrayList<CodeableConcept>(); 444 this.serviceCategory.add(t); 445 return t; 446 } 447 448 public Slot addServiceCategory(CodeableConcept t) { // 3 449 if (t == null) 450 return this; 451 if (this.serviceCategory == null) 452 this.serviceCategory = new ArrayList<CodeableConcept>(); 453 this.serviceCategory.add(t); 454 return this; 455 } 456 457 /** 458 * @return The first repetition of repeating field {@link #serviceCategory}, 459 * creating it if it does not already exist 460 */ 461 public CodeableConcept getServiceCategoryFirstRep() { 462 if (getServiceCategory().isEmpty()) { 463 addServiceCategory(); 464 } 465 return getServiceCategory().get(0); 466 } 467 468 /** 469 * @return {@link #serviceType} (The type of appointments that can be booked 470 * into this slot (ideally this would be an identifiable service - which 471 * is at a location, rather than the location itself). If provided then 472 * this overrides the value provided on the availability resource.) 473 */ 474 public List<CodeableConcept> getServiceType() { 475 if (this.serviceType == null) 476 this.serviceType = new ArrayList<CodeableConcept>(); 477 return this.serviceType; 478 } 479 480 /** 481 * @return Returns a reference to <code>this</code> for easy method chaining 482 */ 483 public Slot setServiceType(List<CodeableConcept> theServiceType) { 484 this.serviceType = theServiceType; 485 return this; 486 } 487 488 public boolean hasServiceType() { 489 if (this.serviceType == null) 490 return false; 491 for (CodeableConcept item : this.serviceType) 492 if (!item.isEmpty()) 493 return true; 494 return false; 495 } 496 497 public CodeableConcept addServiceType() { // 3 498 CodeableConcept t = new CodeableConcept(); 499 if (this.serviceType == null) 500 this.serviceType = new ArrayList<CodeableConcept>(); 501 this.serviceType.add(t); 502 return t; 503 } 504 505 public Slot addServiceType(CodeableConcept t) { // 3 506 if (t == null) 507 return this; 508 if (this.serviceType == null) 509 this.serviceType = new ArrayList<CodeableConcept>(); 510 this.serviceType.add(t); 511 return this; 512 } 513 514 /** 515 * @return The first repetition of repeating field {@link #serviceType}, 516 * creating it if it does not already exist 517 */ 518 public CodeableConcept getServiceTypeFirstRep() { 519 if (getServiceType().isEmpty()) { 520 addServiceType(); 521 } 522 return getServiceType().get(0); 523 } 524 525 /** 526 * @return {@link #specialty} (The specialty of a practitioner that would be 527 * required to perform the service requested in this appointment.) 528 */ 529 public List<CodeableConcept> getSpecialty() { 530 if (this.specialty == null) 531 this.specialty = new ArrayList<CodeableConcept>(); 532 return this.specialty; 533 } 534 535 /** 536 * @return Returns a reference to <code>this</code> for easy method chaining 537 */ 538 public Slot setSpecialty(List<CodeableConcept> theSpecialty) { 539 this.specialty = theSpecialty; 540 return this; 541 } 542 543 public boolean hasSpecialty() { 544 if (this.specialty == null) 545 return false; 546 for (CodeableConcept item : this.specialty) 547 if (!item.isEmpty()) 548 return true; 549 return false; 550 } 551 552 public CodeableConcept addSpecialty() { // 3 553 CodeableConcept t = new CodeableConcept(); 554 if (this.specialty == null) 555 this.specialty = new ArrayList<CodeableConcept>(); 556 this.specialty.add(t); 557 return t; 558 } 559 560 public Slot addSpecialty(CodeableConcept t) { // 3 561 if (t == null) 562 return this; 563 if (this.specialty == null) 564 this.specialty = new ArrayList<CodeableConcept>(); 565 this.specialty.add(t); 566 return this; 567 } 568 569 /** 570 * @return The first repetition of repeating field {@link #specialty}, creating 571 * it if it does not already exist 572 */ 573 public CodeableConcept getSpecialtyFirstRep() { 574 if (getSpecialty().isEmpty()) { 575 addSpecialty(); 576 } 577 return getSpecialty().get(0); 578 } 579 580 /** 581 * @return {@link #appointmentType} (The style of appointment or patient that 582 * may be booked in the slot (not service type).) 583 */ 584 public CodeableConcept getAppointmentType() { 585 if (this.appointmentType == null) 586 if (Configuration.errorOnAutoCreate()) 587 throw new Error("Attempt to auto-create Slot.appointmentType"); 588 else if (Configuration.doAutoCreate()) 589 this.appointmentType = new CodeableConcept(); // cc 590 return this.appointmentType; 591 } 592 593 public boolean hasAppointmentType() { 594 return this.appointmentType != null && !this.appointmentType.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #appointmentType} (The style of appointment or patient 599 * that may be booked in the slot (not service type).) 600 */ 601 public Slot setAppointmentType(CodeableConcept value) { 602 this.appointmentType = value; 603 return this; 604 } 605 606 /** 607 * @return {@link #schedule} (The schedule resource that this slot defines an 608 * interval of status information.) 609 */ 610 public Reference getSchedule() { 611 if (this.schedule == null) 612 if (Configuration.errorOnAutoCreate()) 613 throw new Error("Attempt to auto-create Slot.schedule"); 614 else if (Configuration.doAutoCreate()) 615 this.schedule = new Reference(); // cc 616 return this.schedule; 617 } 618 619 public boolean hasSchedule() { 620 return this.schedule != null && !this.schedule.isEmpty(); 621 } 622 623 /** 624 * @param value {@link #schedule} (The schedule resource that this slot defines 625 * an interval of status information.) 626 */ 627 public Slot setSchedule(Reference value) { 628 this.schedule = value; 629 return this; 630 } 631 632 /** 633 * @return {@link #schedule} The actual object that is the target of the 634 * reference. The reference library doesn't populate this, but you can 635 * use it to hold the resource if you resolve it. (The schedule resource 636 * that this slot defines an interval of status information.) 637 */ 638 public Schedule getScheduleTarget() { 639 if (this.scheduleTarget == null) 640 if (Configuration.errorOnAutoCreate()) 641 throw new Error("Attempt to auto-create Slot.schedule"); 642 else if (Configuration.doAutoCreate()) 643 this.scheduleTarget = new Schedule(); // aa 644 return this.scheduleTarget; 645 } 646 647 /** 648 * @param value {@link #schedule} The actual object that is the target of the 649 * reference. The reference library doesn't use these, but you can 650 * use it to hold the resource if you resolve it. (The schedule 651 * resource that this slot defines an interval of status 652 * information.) 653 */ 654 public Slot setScheduleTarget(Schedule value) { 655 this.scheduleTarget = value; 656 return this; 657 } 658 659 /** 660 * @return {@link #status} (busy | free | busy-unavailable | busy-tentative | 661 * entered-in-error.). This is the underlying object with id, value and 662 * extensions. The accessor "getStatus" gives direct access to the value 663 */ 664 public Enumeration<SlotStatus> getStatusElement() { 665 if (this.status == null) 666 if (Configuration.errorOnAutoCreate()) 667 throw new Error("Attempt to auto-create Slot.status"); 668 else if (Configuration.doAutoCreate()) 669 this.status = new Enumeration<SlotStatus>(new SlotStatusEnumFactory()); // bb 670 return this.status; 671 } 672 673 public boolean hasStatusElement() { 674 return this.status != null && !this.status.isEmpty(); 675 } 676 677 public boolean hasStatus() { 678 return this.status != null && !this.status.isEmpty(); 679 } 680 681 /** 682 * @param value {@link #status} (busy | free | busy-unavailable | busy-tentative 683 * | entered-in-error.). This is the underlying object with id, 684 * value and extensions. The accessor "getStatus" gives direct 685 * access to the value 686 */ 687 public Slot setStatusElement(Enumeration<SlotStatus> value) { 688 this.status = value; 689 return this; 690 } 691 692 /** 693 * @return busy | free | busy-unavailable | busy-tentative | entered-in-error. 694 */ 695 public SlotStatus getStatus() { 696 return this.status == null ? null : this.status.getValue(); 697 } 698 699 /** 700 * @param value busy | free | busy-unavailable | busy-tentative | 701 * entered-in-error. 702 */ 703 public Slot setStatus(SlotStatus value) { 704 if (this.status == null) 705 this.status = new Enumeration<SlotStatus>(new SlotStatusEnumFactory()); 706 this.status.setValue(value); 707 return this; 708 } 709 710 /** 711 * @return {@link #start} (Date/Time that the slot is to begin.). This is the 712 * underlying object with id, value and extensions. The accessor 713 * "getStart" gives direct access to the value 714 */ 715 public InstantType getStartElement() { 716 if (this.start == null) 717 if (Configuration.errorOnAutoCreate()) 718 throw new Error("Attempt to auto-create Slot.start"); 719 else if (Configuration.doAutoCreate()) 720 this.start = new InstantType(); // bb 721 return this.start; 722 } 723 724 public boolean hasStartElement() { 725 return this.start != null && !this.start.isEmpty(); 726 } 727 728 public boolean hasStart() { 729 return this.start != null && !this.start.isEmpty(); 730 } 731 732 /** 733 * @param value {@link #start} (Date/Time that the slot is to begin.). This is 734 * the underlying object with id, value and extensions. The 735 * accessor "getStart" gives direct access to the value 736 */ 737 public Slot setStartElement(InstantType value) { 738 this.start = value; 739 return this; 740 } 741 742 /** 743 * @return Date/Time that the slot is to begin. 744 */ 745 public Date getStart() { 746 return this.start == null ? null : this.start.getValue(); 747 } 748 749 /** 750 * @param value Date/Time that the slot is to begin. 751 */ 752 public Slot setStart(Date value) { 753 if (this.start == null) 754 this.start = new InstantType(); 755 this.start.setValue(value); 756 return this; 757 } 758 759 /** 760 * @return {@link #end} (Date/Time that the slot is to conclude.). This is the 761 * underlying object with id, value and extensions. The accessor 762 * "getEnd" gives direct access to the value 763 */ 764 public InstantType getEndElement() { 765 if (this.end == null) 766 if (Configuration.errorOnAutoCreate()) 767 throw new Error("Attempt to auto-create Slot.end"); 768 else if (Configuration.doAutoCreate()) 769 this.end = new InstantType(); // bb 770 return this.end; 771 } 772 773 public boolean hasEndElement() { 774 return this.end != null && !this.end.isEmpty(); 775 } 776 777 public boolean hasEnd() { 778 return this.end != null && !this.end.isEmpty(); 779 } 780 781 /** 782 * @param value {@link #end} (Date/Time that the slot is to conclude.). This is 783 * the underlying object with id, value and extensions. The 784 * accessor "getEnd" gives direct access to the value 785 */ 786 public Slot setEndElement(InstantType value) { 787 this.end = value; 788 return this; 789 } 790 791 /** 792 * @return Date/Time that the slot is to conclude. 793 */ 794 public Date getEnd() { 795 return this.end == null ? null : this.end.getValue(); 796 } 797 798 /** 799 * @param value Date/Time that the slot is to conclude. 800 */ 801 public Slot setEnd(Date value) { 802 if (this.end == null) 803 this.end = new InstantType(); 804 this.end.setValue(value); 805 return this; 806 } 807 808 /** 809 * @return {@link #overbooked} (This slot has already been overbooked, 810 * appointments are unlikely to be accepted for this time.). This is the 811 * underlying object with id, value and extensions. The accessor 812 * "getOverbooked" gives direct access to the value 813 */ 814 public BooleanType getOverbookedElement() { 815 if (this.overbooked == null) 816 if (Configuration.errorOnAutoCreate()) 817 throw new Error("Attempt to auto-create Slot.overbooked"); 818 else if (Configuration.doAutoCreate()) 819 this.overbooked = new BooleanType(); // bb 820 return this.overbooked; 821 } 822 823 public boolean hasOverbookedElement() { 824 return this.overbooked != null && !this.overbooked.isEmpty(); 825 } 826 827 public boolean hasOverbooked() { 828 return this.overbooked != null && !this.overbooked.isEmpty(); 829 } 830 831 /** 832 * @param value {@link #overbooked} (This slot has already been overbooked, 833 * appointments are unlikely to be accepted for this time.). This 834 * is the underlying object with id, value and extensions. The 835 * accessor "getOverbooked" gives direct access to the value 836 */ 837 public Slot setOverbookedElement(BooleanType value) { 838 this.overbooked = value; 839 return this; 840 } 841 842 /** 843 * @return This slot has already been overbooked, appointments are unlikely to 844 * be accepted for this time. 845 */ 846 public boolean getOverbooked() { 847 return this.overbooked == null || this.overbooked.isEmpty() ? false : this.overbooked.getValue(); 848 } 849 850 /** 851 * @param value This slot has already been overbooked, appointments are unlikely 852 * to be accepted for this time. 853 */ 854 public Slot setOverbooked(boolean value) { 855 if (this.overbooked == null) 856 this.overbooked = new BooleanType(); 857 this.overbooked.setValue(value); 858 return this; 859 } 860 861 /** 862 * @return {@link #comment} (Comments on the slot to describe any extended 863 * information. Such as custom constraints on the slot.). This is the 864 * underlying object with id, value and extensions. The accessor 865 * "getComment" gives direct access to the value 866 */ 867 public StringType getCommentElement() { 868 if (this.comment == null) 869 if (Configuration.errorOnAutoCreate()) 870 throw new Error("Attempt to auto-create Slot.comment"); 871 else if (Configuration.doAutoCreate()) 872 this.comment = new StringType(); // bb 873 return this.comment; 874 } 875 876 public boolean hasCommentElement() { 877 return this.comment != null && !this.comment.isEmpty(); 878 } 879 880 public boolean hasComment() { 881 return this.comment != null && !this.comment.isEmpty(); 882 } 883 884 /** 885 * @param value {@link #comment} (Comments on the slot to describe any extended 886 * information. Such as custom constraints on the slot.). This is 887 * the underlying object with id, value and extensions. The 888 * accessor "getComment" gives direct access to the value 889 */ 890 public Slot setCommentElement(StringType value) { 891 this.comment = value; 892 return this; 893 } 894 895 /** 896 * @return Comments on the slot to describe any extended information. Such as 897 * custom constraints on the slot. 898 */ 899 public String getComment() { 900 return this.comment == null ? null : this.comment.getValue(); 901 } 902 903 /** 904 * @param value Comments on the slot to describe any extended information. Such 905 * as custom constraints on the slot. 906 */ 907 public Slot setComment(String value) { 908 if (Utilities.noString(value)) 909 this.comment = null; 910 else { 911 if (this.comment == null) 912 this.comment = new StringType(); 913 this.comment.setValue(value); 914 } 915 return this; 916 } 917 918 protected void listChildren(List<Property> children) { 919 super.listChildren(children); 920 children.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, 921 identifier)); 922 children.add(new Property("serviceCategory", "CodeableConcept", 923 "A broad categorization of the service that is to be performed during this appointment.", 0, 924 java.lang.Integer.MAX_VALUE, serviceCategory)); 925 children.add(new Property("serviceType", "CodeableConcept", 926 "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.", 927 0, java.lang.Integer.MAX_VALUE, serviceType)); 928 children.add(new Property("specialty", "CodeableConcept", 929 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 930 0, java.lang.Integer.MAX_VALUE, specialty)); 931 children.add(new Property("appointmentType", "CodeableConcept", 932 "The style of appointment or patient that may be booked in the slot (not service type).", 0, 1, 933 appointmentType)); 934 children.add(new Property("schedule", "Reference(Schedule)", 935 "The schedule resource that this slot defines an interval of status information.", 0, 1, schedule)); 936 children.add(new Property("status", "code", "busy | free | busy-unavailable | busy-tentative | entered-in-error.", 937 0, 1, status)); 938 children.add(new Property("start", "instant", "Date/Time that the slot is to begin.", 0, 1, start)); 939 children.add(new Property("end", "instant", "Date/Time that the slot is to conclude.", 0, 1, end)); 940 children.add(new Property("overbooked", "boolean", 941 "This slot has already been overbooked, appointments are unlikely to be accepted for this time.", 0, 1, 942 overbooked)); 943 children.add(new Property("comment", "string", 944 "Comments on the slot to describe any extended information. Such as custom constraints on the slot.", 0, 1, 945 comment)); 946 } 947 948 @Override 949 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 950 switch (_hash) { 951 case -1618432855: 952 /* identifier */ return new Property("identifier", "Identifier", "External Ids for this item.", 0, 953 java.lang.Integer.MAX_VALUE, identifier); 954 case 1281188563: 955 /* serviceCategory */ return new Property("serviceCategory", "CodeableConcept", 956 "A broad categorization of the service that is to be performed during this appointment.", 0, 957 java.lang.Integer.MAX_VALUE, serviceCategory); 958 case -1928370289: 959 /* serviceType */ return new Property("serviceType", "CodeableConcept", 960 "The type of appointments that can be booked into this slot (ideally this would be an identifiable service - which is at a location, rather than the location itself). If provided then this overrides the value provided on the availability resource.", 961 0, java.lang.Integer.MAX_VALUE, serviceType); 962 case -1694759682: 963 /* specialty */ return new Property("specialty", "CodeableConcept", 964 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 965 0, java.lang.Integer.MAX_VALUE, specialty); 966 case -1596426375: 967 /* appointmentType */ return new Property("appointmentType", "CodeableConcept", 968 "The style of appointment or patient that may be booked in the slot (not service type).", 0, 1, 969 appointmentType); 970 case -697920873: 971 /* schedule */ return new Property("schedule", "Reference(Schedule)", 972 "The schedule resource that this slot defines an interval of status information.", 0, 1, schedule); 973 case -892481550: 974 /* status */ return new Property("status", "code", 975 "busy | free | busy-unavailable | busy-tentative | entered-in-error.", 0, 1, status); 976 case 109757538: 977 /* start */ return new Property("start", "instant", "Date/Time that the slot is to begin.", 0, 1, start); 978 case 100571: 979 /* end */ return new Property("end", "instant", "Date/Time that the slot is to conclude.", 0, 1, end); 980 case 2068545308: 981 /* overbooked */ return new Property("overbooked", "boolean", 982 "This slot has already been overbooked, appointments are unlikely to be accepted for this time.", 0, 1, 983 overbooked); 984 case 950398559: 985 /* comment */ return new Property("comment", "string", 986 "Comments on the slot to describe any extended information. Such as custom constraints on the slot.", 0, 1, 987 comment); 988 default: 989 return super.getNamedProperty(_hash, _name, _checkValid); 990 } 991 992 } 993 994 @Override 995 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 996 switch (hash) { 997 case -1618432855: 998 /* identifier */ return this.identifier == null ? new Base[0] 999 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1000 case 1281188563: 1001 /* serviceCategory */ return this.serviceCategory == null ? new Base[0] 1002 : this.serviceCategory.toArray(new Base[this.serviceCategory.size()]); // CodeableConcept 1003 case -1928370289: 1004 /* serviceType */ return this.serviceType == null ? new Base[0] 1005 : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableConcept 1006 case -1694759682: 1007 /* specialty */ return this.specialty == null ? new Base[0] 1008 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 1009 case -1596426375: 1010 /* appointmentType */ return this.appointmentType == null ? new Base[0] : new Base[] { this.appointmentType }; // CodeableConcept 1011 case -697920873: 1012 /* schedule */ return this.schedule == null ? new Base[0] : new Base[] { this.schedule }; // Reference 1013 case -892481550: 1014 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<SlotStatus> 1015 case 109757538: 1016 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // InstantType 1017 case 100571: 1018 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // InstantType 1019 case 2068545308: 1020 /* overbooked */ return this.overbooked == null ? new Base[0] : new Base[] { this.overbooked }; // BooleanType 1021 case 950398559: 1022 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 1023 default: 1024 return super.getProperty(hash, name, checkValid); 1025 } 1026 1027 } 1028 1029 @Override 1030 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1031 switch (hash) { 1032 case -1618432855: // identifier 1033 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1034 return value; 1035 case 1281188563: // serviceCategory 1036 this.getServiceCategory().add(castToCodeableConcept(value)); // CodeableConcept 1037 return value; 1038 case -1928370289: // serviceType 1039 this.getServiceType().add(castToCodeableConcept(value)); // CodeableConcept 1040 return value; 1041 case -1694759682: // specialty 1042 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 1043 return value; 1044 case -1596426375: // appointmentType 1045 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 1046 return value; 1047 case -697920873: // schedule 1048 this.schedule = castToReference(value); // Reference 1049 return value; 1050 case -892481550: // status 1051 value = new SlotStatusEnumFactory().fromType(castToCode(value)); 1052 this.status = (Enumeration) value; // Enumeration<SlotStatus> 1053 return value; 1054 case 109757538: // start 1055 this.start = castToInstant(value); // InstantType 1056 return value; 1057 case 100571: // end 1058 this.end = castToInstant(value); // InstantType 1059 return value; 1060 case 2068545308: // overbooked 1061 this.overbooked = castToBoolean(value); // BooleanType 1062 return value; 1063 case 950398559: // comment 1064 this.comment = castToString(value); // StringType 1065 return value; 1066 default: 1067 return super.setProperty(hash, name, value); 1068 } 1069 1070 } 1071 1072 @Override 1073 public Base setProperty(String name, Base value) throws FHIRException { 1074 if (name.equals("identifier")) { 1075 this.getIdentifier().add(castToIdentifier(value)); 1076 } else if (name.equals("serviceCategory")) { 1077 this.getServiceCategory().add(castToCodeableConcept(value)); 1078 } else if (name.equals("serviceType")) { 1079 this.getServiceType().add(castToCodeableConcept(value)); 1080 } else if (name.equals("specialty")) { 1081 this.getSpecialty().add(castToCodeableConcept(value)); 1082 } else if (name.equals("appointmentType")) { 1083 this.appointmentType = castToCodeableConcept(value); // CodeableConcept 1084 } else if (name.equals("schedule")) { 1085 this.schedule = castToReference(value); // Reference 1086 } else if (name.equals("status")) { 1087 value = new SlotStatusEnumFactory().fromType(castToCode(value)); 1088 this.status = (Enumeration) value; // Enumeration<SlotStatus> 1089 } else if (name.equals("start")) { 1090 this.start = castToInstant(value); // InstantType 1091 } else if (name.equals("end")) { 1092 this.end = castToInstant(value); // InstantType 1093 } else if (name.equals("overbooked")) { 1094 this.overbooked = castToBoolean(value); // BooleanType 1095 } else if (name.equals("comment")) { 1096 this.comment = castToString(value); // StringType 1097 } else 1098 return super.setProperty(name, value); 1099 return value; 1100 } 1101 1102 @Override 1103 public void removeChild(String name, Base value) throws FHIRException { 1104 if (name.equals("identifier")) { 1105 this.getIdentifier().remove(castToIdentifier(value)); 1106 } else if (name.equals("serviceCategory")) { 1107 this.getServiceCategory().remove(castToCodeableConcept(value)); 1108 } else if (name.equals("serviceType")) { 1109 this.getServiceType().remove(castToCodeableConcept(value)); 1110 } else if (name.equals("specialty")) { 1111 this.getSpecialty().remove(castToCodeableConcept(value)); 1112 } else if (name.equals("appointmentType")) { 1113 this.appointmentType = null; 1114 } else if (name.equals("schedule")) { 1115 this.schedule = null; 1116 } else if (name.equals("status")) { 1117 this.status = null; 1118 } else if (name.equals("start")) { 1119 this.start = null; 1120 } else if (name.equals("end")) { 1121 this.end = null; 1122 } else if (name.equals("overbooked")) { 1123 this.overbooked = null; 1124 } else if (name.equals("comment")) { 1125 this.comment = null; 1126 } else 1127 super.removeChild(name, value); 1128 1129 } 1130 1131 @Override 1132 public Base makeProperty(int hash, String name) throws FHIRException { 1133 switch (hash) { 1134 case -1618432855: 1135 return addIdentifier(); 1136 case 1281188563: 1137 return addServiceCategory(); 1138 case -1928370289: 1139 return addServiceType(); 1140 case -1694759682: 1141 return addSpecialty(); 1142 case -1596426375: 1143 return getAppointmentType(); 1144 case -697920873: 1145 return getSchedule(); 1146 case -892481550: 1147 return getStatusElement(); 1148 case 109757538: 1149 return getStartElement(); 1150 case 100571: 1151 return getEndElement(); 1152 case 2068545308: 1153 return getOverbookedElement(); 1154 case 950398559: 1155 return getCommentElement(); 1156 default: 1157 return super.makeProperty(hash, name); 1158 } 1159 1160 } 1161 1162 @Override 1163 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1164 switch (hash) { 1165 case -1618432855: 1166 /* identifier */ return new String[] { "Identifier" }; 1167 case 1281188563: 1168 /* serviceCategory */ return new String[] { "CodeableConcept" }; 1169 case -1928370289: 1170 /* serviceType */ return new String[] { "CodeableConcept" }; 1171 case -1694759682: 1172 /* specialty */ return new String[] { "CodeableConcept" }; 1173 case -1596426375: 1174 /* appointmentType */ return new String[] { "CodeableConcept" }; 1175 case -697920873: 1176 /* schedule */ return new String[] { "Reference" }; 1177 case -892481550: 1178 /* status */ return new String[] { "code" }; 1179 case 109757538: 1180 /* start */ return new String[] { "instant" }; 1181 case 100571: 1182 /* end */ return new String[] { "instant" }; 1183 case 2068545308: 1184 /* overbooked */ return new String[] { "boolean" }; 1185 case 950398559: 1186 /* comment */ return new String[] { "string" }; 1187 default: 1188 return super.getTypesForProperty(hash, name); 1189 } 1190 1191 } 1192 1193 @Override 1194 public Base addChild(String name) throws FHIRException { 1195 if (name.equals("identifier")) { 1196 return addIdentifier(); 1197 } else if (name.equals("serviceCategory")) { 1198 return addServiceCategory(); 1199 } else if (name.equals("serviceType")) { 1200 return addServiceType(); 1201 } else if (name.equals("specialty")) { 1202 return addSpecialty(); 1203 } else if (name.equals("appointmentType")) { 1204 this.appointmentType = new CodeableConcept(); 1205 return this.appointmentType; 1206 } else if (name.equals("schedule")) { 1207 this.schedule = new Reference(); 1208 return this.schedule; 1209 } else if (name.equals("status")) { 1210 throw new FHIRException("Cannot call addChild on a singleton property Slot.status"); 1211 } else if (name.equals("start")) { 1212 throw new FHIRException("Cannot call addChild on a singleton property Slot.start"); 1213 } else if (name.equals("end")) { 1214 throw new FHIRException("Cannot call addChild on a singleton property Slot.end"); 1215 } else if (name.equals("overbooked")) { 1216 throw new FHIRException("Cannot call addChild on a singleton property Slot.overbooked"); 1217 } else if (name.equals("comment")) { 1218 throw new FHIRException("Cannot call addChild on a singleton property Slot.comment"); 1219 } else 1220 return super.addChild(name); 1221 } 1222 1223 public String fhirType() { 1224 return "Slot"; 1225 1226 } 1227 1228 public Slot copy() { 1229 Slot dst = new Slot(); 1230 copyValues(dst); 1231 return dst; 1232 } 1233 1234 public void copyValues(Slot dst) { 1235 super.copyValues(dst); 1236 if (identifier != null) { 1237 dst.identifier = new ArrayList<Identifier>(); 1238 for (Identifier i : identifier) 1239 dst.identifier.add(i.copy()); 1240 } 1241 ; 1242 if (serviceCategory != null) { 1243 dst.serviceCategory = new ArrayList<CodeableConcept>(); 1244 for (CodeableConcept i : serviceCategory) 1245 dst.serviceCategory.add(i.copy()); 1246 } 1247 ; 1248 if (serviceType != null) { 1249 dst.serviceType = new ArrayList<CodeableConcept>(); 1250 for (CodeableConcept i : serviceType) 1251 dst.serviceType.add(i.copy()); 1252 } 1253 ; 1254 if (specialty != null) { 1255 dst.specialty = new ArrayList<CodeableConcept>(); 1256 for (CodeableConcept i : specialty) 1257 dst.specialty.add(i.copy()); 1258 } 1259 ; 1260 dst.appointmentType = appointmentType == null ? null : appointmentType.copy(); 1261 dst.schedule = schedule == null ? null : schedule.copy(); 1262 dst.status = status == null ? null : status.copy(); 1263 dst.start = start == null ? null : start.copy(); 1264 dst.end = end == null ? null : end.copy(); 1265 dst.overbooked = overbooked == null ? null : overbooked.copy(); 1266 dst.comment = comment == null ? null : comment.copy(); 1267 } 1268 1269 protected Slot typedCopy() { 1270 return copy(); 1271 } 1272 1273 @Override 1274 public boolean equalsDeep(Base other_) { 1275 if (!super.equalsDeep(other_)) 1276 return false; 1277 if (!(other_ instanceof Slot)) 1278 return false; 1279 Slot o = (Slot) other_; 1280 return compareDeep(identifier, o.identifier, true) && compareDeep(serviceCategory, o.serviceCategory, true) 1281 && compareDeep(serviceType, o.serviceType, true) && compareDeep(specialty, o.specialty, true) 1282 && compareDeep(appointmentType, o.appointmentType, true) && compareDeep(schedule, o.schedule, true) 1283 && compareDeep(status, o.status, true) && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) 1284 && compareDeep(overbooked, o.overbooked, true) && compareDeep(comment, o.comment, true); 1285 } 1286 1287 @Override 1288 public boolean equalsShallow(Base other_) { 1289 if (!super.equalsShallow(other_)) 1290 return false; 1291 if (!(other_ instanceof Slot)) 1292 return false; 1293 Slot o = (Slot) other_; 1294 return compareValues(status, o.status, true) && compareValues(start, o.start, true) 1295 && compareValues(end, o.end, true) && compareValues(overbooked, o.overbooked, true) 1296 && compareValues(comment, o.comment, true); 1297 } 1298 1299 public boolean isEmpty() { 1300 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, serviceCategory, serviceType, specialty, 1301 appointmentType, schedule, status, start, end, overbooked, comment); 1302 } 1303 1304 @Override 1305 public ResourceType getResourceType() { 1306 return ResourceType.Slot; 1307 } 1308 1309 /** 1310 * Search parameter: <b>schedule</b> 1311 * <p> 1312 * Description: <b>The Schedule Resource that we are seeking a slot 1313 * within</b><br> 1314 * Type: <b>reference</b><br> 1315 * Path: <b>Slot.schedule</b><br> 1316 * </p> 1317 */ 1318 @SearchParamDefinition(name = "schedule", path = "Slot.schedule", description = "The Schedule Resource that we are seeking a slot within", type = "reference", target = { 1319 Schedule.class }) 1320 public static final String SP_SCHEDULE = "schedule"; 1321 /** 1322 * <b>Fluent Client</b> search parameter constant for <b>schedule</b> 1323 * <p> 1324 * Description: <b>The Schedule Resource that we are seeking a slot 1325 * within</b><br> 1326 * Type: <b>reference</b><br> 1327 * Path: <b>Slot.schedule</b><br> 1328 * </p> 1329 */ 1330 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SCHEDULE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1331 SP_SCHEDULE); 1332 1333 /** 1334 * Constant for fluent queries to be used to add include statements. Specifies 1335 * the path value of "<b>Slot:schedule</b>". 1336 */ 1337 public static final ca.uhn.fhir.model.api.Include INCLUDE_SCHEDULE = new ca.uhn.fhir.model.api.Include( 1338 "Slot:schedule").toLocked(); 1339 1340 /** 1341 * Search parameter: <b>identifier</b> 1342 * <p> 1343 * Description: <b>A Slot Identifier</b><br> 1344 * Type: <b>token</b><br> 1345 * Path: <b>Slot.identifier</b><br> 1346 * </p> 1347 */ 1348 @SearchParamDefinition(name = "identifier", path = "Slot.identifier", description = "A Slot Identifier", type = "token") 1349 public static final String SP_IDENTIFIER = "identifier"; 1350 /** 1351 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1352 * <p> 1353 * Description: <b>A Slot Identifier</b><br> 1354 * Type: <b>token</b><br> 1355 * Path: <b>Slot.identifier</b><br> 1356 * </p> 1357 */ 1358 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1359 SP_IDENTIFIER); 1360 1361 /** 1362 * Search parameter: <b>specialty</b> 1363 * <p> 1364 * Description: <b>The specialty of a practitioner that would be required to 1365 * perform the service requested in this appointment</b><br> 1366 * Type: <b>token</b><br> 1367 * Path: <b>Slot.specialty</b><br> 1368 * </p> 1369 */ 1370 @SearchParamDefinition(name = "specialty", path = "Slot.specialty", description = "The specialty of a practitioner that would be required to perform the service requested in this appointment", type = "token") 1371 public static final String SP_SPECIALTY = "specialty"; 1372 /** 1373 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 1374 * <p> 1375 * Description: <b>The specialty of a practitioner that would be required to 1376 * perform the service requested in this appointment</b><br> 1377 * Type: <b>token</b><br> 1378 * Path: <b>Slot.specialty</b><br> 1379 * </p> 1380 */ 1381 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1382 SP_SPECIALTY); 1383 1384 /** 1385 * Search parameter: <b>service-category</b> 1386 * <p> 1387 * Description: <b>A broad categorization of the service that is to be performed 1388 * during this appointment</b><br> 1389 * Type: <b>token</b><br> 1390 * Path: <b>Slot.serviceCategory</b><br> 1391 * </p> 1392 */ 1393 @SearchParamDefinition(name = "service-category", path = "Slot.serviceCategory", description = "A broad categorization of the service that is to be performed during this appointment", type = "token") 1394 public static final String SP_SERVICE_CATEGORY = "service-category"; 1395 /** 1396 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 1397 * <p> 1398 * Description: <b>A broad categorization of the service that is to be performed 1399 * during this appointment</b><br> 1400 * Type: <b>token</b><br> 1401 * Path: <b>Slot.serviceCategory</b><br> 1402 * </p> 1403 */ 1404 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1405 SP_SERVICE_CATEGORY); 1406 1407 /** 1408 * Search parameter: <b>appointment-type</b> 1409 * <p> 1410 * Description: <b>The style of appointment or patient that may be booked in the 1411 * slot (not service type)</b><br> 1412 * Type: <b>token</b><br> 1413 * Path: <b>Slot.appointmentType</b><br> 1414 * </p> 1415 */ 1416 @SearchParamDefinition(name = "appointment-type", path = "Slot.appointmentType", description = "The style of appointment or patient that may be booked in the slot (not service type)", type = "token") 1417 public static final String SP_APPOINTMENT_TYPE = "appointment-type"; 1418 /** 1419 * <b>Fluent Client</b> search parameter constant for <b>appointment-type</b> 1420 * <p> 1421 * Description: <b>The style of appointment or patient that may be booked in the 1422 * slot (not service type)</b><br> 1423 * Type: <b>token</b><br> 1424 * Path: <b>Slot.appointmentType</b><br> 1425 * </p> 1426 */ 1427 public static final ca.uhn.fhir.rest.gclient.TokenClientParam APPOINTMENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1428 SP_APPOINTMENT_TYPE); 1429 1430 /** 1431 * Search parameter: <b>service-type</b> 1432 * <p> 1433 * Description: <b>The type of appointments that can be booked into the 1434 * slot</b><br> 1435 * Type: <b>token</b><br> 1436 * Path: <b>Slot.serviceType</b><br> 1437 * </p> 1438 */ 1439 @SearchParamDefinition(name = "service-type", path = "Slot.serviceType", description = "The type of appointments that can be booked into the slot", type = "token") 1440 public static final String SP_SERVICE_TYPE = "service-type"; 1441 /** 1442 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 1443 * <p> 1444 * Description: <b>The type of appointments that can be booked into the 1445 * slot</b><br> 1446 * Type: <b>token</b><br> 1447 * Path: <b>Slot.serviceType</b><br> 1448 * </p> 1449 */ 1450 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1451 SP_SERVICE_TYPE); 1452 1453 /** 1454 * Search parameter: <b>start</b> 1455 * <p> 1456 * Description: <b>Appointment date/time.</b><br> 1457 * Type: <b>date</b><br> 1458 * Path: <b>Slot.start</b><br> 1459 * </p> 1460 */ 1461 @SearchParamDefinition(name = "start", path = "Slot.start", description = "Appointment date/time.", type = "date") 1462 public static final String SP_START = "start"; 1463 /** 1464 * <b>Fluent Client</b> search parameter constant for <b>start</b> 1465 * <p> 1466 * Description: <b>Appointment date/time.</b><br> 1467 * Type: <b>date</b><br> 1468 * Path: <b>Slot.start</b><br> 1469 * </p> 1470 */ 1471 public static final ca.uhn.fhir.rest.gclient.DateClientParam START = new ca.uhn.fhir.rest.gclient.DateClientParam( 1472 SP_START); 1473 1474 /** 1475 * Search parameter: <b>status</b> 1476 * <p> 1477 * Description: <b>The free/busy status of the appointment</b><br> 1478 * Type: <b>token</b><br> 1479 * Path: <b>Slot.status</b><br> 1480 * </p> 1481 */ 1482 @SearchParamDefinition(name = "status", path = "Slot.status", description = "The free/busy status of the appointment", type = "token") 1483 public static final String SP_STATUS = "status"; 1484 /** 1485 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1486 * <p> 1487 * Description: <b>The free/busy status of the appointment</b><br> 1488 * Type: <b>token</b><br> 1489 * Path: <b>Slot.status</b><br> 1490 * </p> 1491 */ 1492 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1493 SP_STATUS); 1494 1495}