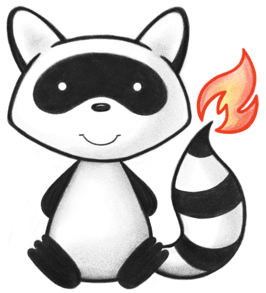
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041// added from java-adornments.txt: 042import org.hl7.fhir.r4.utils.StructureMapUtilities; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052// end addition 053/** 054 * A Map of relationships between 2 structures that can be used to transform 055 * data. 056 */ 057@ResourceDef(name = "StructureMap", profile = "http://hl7.org/fhir/StructureDefinition/StructureMap") 058@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", 059 "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "structure", "import", "group" }) 060public class StructureMap extends MetadataResource { 061 062 public enum StructureMapModelMode { 063 /** 064 * This structure describes an instance passed to the mapping engine that is 065 * used a source of data. 066 */ 067 SOURCE, 068 /** 069 * This structure describes an instance that the mapping engine may ask for that 070 * is used a source of data. 071 */ 072 QUERIED, 073 /** 074 * This structure describes an instance passed to the mapping engine that is 075 * used a target of data. 076 */ 077 TARGET, 078 /** 079 * This structure describes an instance that the mapping engine may ask to 080 * create that is used a target of data. 081 */ 082 PRODUCED, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 088 public static StructureMapModelMode fromCode(String codeString) throws FHIRException { 089 if (codeString == null || "".equals(codeString)) 090 return null; 091 if ("source".equals(codeString)) 092 return SOURCE; 093 if ("queried".equals(codeString)) 094 return QUERIED; 095 if ("target".equals(codeString)) 096 return TARGET; 097 if ("produced".equals(codeString)) 098 return PRODUCED; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown StructureMapModelMode code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case SOURCE: 108 return "source"; 109 case QUERIED: 110 return "queried"; 111 case TARGET: 112 return "target"; 113 case PRODUCED: 114 return "produced"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case SOURCE: 125 return "http://hl7.org/fhir/map-model-mode"; 126 case QUERIED: 127 return "http://hl7.org/fhir/map-model-mode"; 128 case TARGET: 129 return "http://hl7.org/fhir/map-model-mode"; 130 case PRODUCED: 131 return "http://hl7.org/fhir/map-model-mode"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case SOURCE: 142 return "This structure describes an instance passed to the mapping engine that is used a source of data."; 143 case QUERIED: 144 return "This structure describes an instance that the mapping engine may ask for that is used a source of data."; 145 case TARGET: 146 return "This structure describes an instance passed to the mapping engine that is used a target of data."; 147 case PRODUCED: 148 return "This structure describes an instance that the mapping engine may ask to create that is used a target of data."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case SOURCE: 159 return "Source Structure Definition"; 160 case QUERIED: 161 return "Queried Structure Definition"; 162 case TARGET: 163 return "Target Structure Definition"; 164 case PRODUCED: 165 return "Produced Structure Definition"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 } 173 174 public static class StructureMapModelModeEnumFactory implements EnumFactory<StructureMapModelMode> { 175 public StructureMapModelMode fromCode(String codeString) throws IllegalArgumentException { 176 if (codeString == null || "".equals(codeString)) 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("source".equals(codeString)) 180 return StructureMapModelMode.SOURCE; 181 if ("queried".equals(codeString)) 182 return StructureMapModelMode.QUERIED; 183 if ("target".equals(codeString)) 184 return StructureMapModelMode.TARGET; 185 if ("produced".equals(codeString)) 186 return StructureMapModelMode.PRODUCED; 187 throw new IllegalArgumentException("Unknown StructureMapModelMode code '" + codeString + "'"); 188 } 189 190 public Enumeration<StructureMapModelMode> fromType(PrimitiveType<?> code) throws FHIRException { 191 if (code == null) 192 return null; 193 if (code.isEmpty()) 194 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.NULL, code); 195 String codeString = code.asStringValue(); 196 if (codeString == null || "".equals(codeString)) 197 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.NULL, code); 198 if ("source".equals(codeString)) 199 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.SOURCE, code); 200 if ("queried".equals(codeString)) 201 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.QUERIED, code); 202 if ("target".equals(codeString)) 203 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.TARGET, code); 204 if ("produced".equals(codeString)) 205 return new Enumeration<StructureMapModelMode>(this, StructureMapModelMode.PRODUCED, code); 206 throw new FHIRException("Unknown StructureMapModelMode code '" + codeString + "'"); 207 } 208 209 public String toCode(StructureMapModelMode code) { 210 if (code == StructureMapModelMode.NULL) 211 return null; 212 if (code == StructureMapModelMode.SOURCE) 213 return "source"; 214 if (code == StructureMapModelMode.QUERIED) 215 return "queried"; 216 if (code == StructureMapModelMode.TARGET) 217 return "target"; 218 if (code == StructureMapModelMode.PRODUCED) 219 return "produced"; 220 return "?"; 221 } 222 223 public String toSystem(StructureMapModelMode code) { 224 return code.getSystem(); 225 } 226 } 227 228 public enum StructureMapGroupTypeMode { 229 /** 230 * This group is not a default group for the types. 231 */ 232 NONE, 233 /** 234 * This group is a default mapping group for the specified types and for the 235 * primary source type. 236 */ 237 TYPES, 238 /** 239 * This group is a default mapping group for the specified types. 240 */ 241 TYPEANDTYPES, 242 /** 243 * added to help the parsers with the generic types 244 */ 245 NULL; 246 247 public static StructureMapGroupTypeMode fromCode(String codeString) throws FHIRException { 248 if (codeString == null || "".equals(codeString)) 249 return null; 250 if ("none".equals(codeString)) 251 return NONE; 252 if ("types".equals(codeString)) 253 return TYPES; 254 if ("type-and-types".equals(codeString)) 255 return TYPEANDTYPES; 256 if (Configuration.isAcceptInvalidEnums()) 257 return null; 258 else 259 throw new FHIRException("Unknown StructureMapGroupTypeMode code '" + codeString + "'"); 260 } 261 262 public String toCode() { 263 switch (this) { 264 case NONE: 265 return "none"; 266 case TYPES: 267 return "types"; 268 case TYPEANDTYPES: 269 return "type-and-types"; 270 case NULL: 271 return null; 272 default: 273 return "?"; 274 } 275 } 276 277 public String getSystem() { 278 switch (this) { 279 case NONE: 280 return "http://hl7.org/fhir/map-group-type-mode"; 281 case TYPES: 282 return "http://hl7.org/fhir/map-group-type-mode"; 283 case TYPEANDTYPES: 284 return "http://hl7.org/fhir/map-group-type-mode"; 285 case NULL: 286 return null; 287 default: 288 return "?"; 289 } 290 } 291 292 public String getDefinition() { 293 switch (this) { 294 case NONE: 295 return "This group is not a default group for the types."; 296 case TYPES: 297 return "This group is a default mapping group for the specified types and for the primary source type."; 298 case TYPEANDTYPES: 299 return "This group is a default mapping group for the specified types."; 300 case NULL: 301 return null; 302 default: 303 return "?"; 304 } 305 } 306 307 public String getDisplay() { 308 switch (this) { 309 case NONE: 310 return "Not a Default"; 311 case TYPES: 312 return "Default for Type Combination"; 313 case TYPEANDTYPES: 314 return "Default for type + combination"; 315 case NULL: 316 return null; 317 default: 318 return "?"; 319 } 320 } 321 } 322 323 public static class StructureMapGroupTypeModeEnumFactory implements EnumFactory<StructureMapGroupTypeMode> { 324 public StructureMapGroupTypeMode fromCode(String codeString) throws IllegalArgumentException { 325 if (codeString == null || "".equals(codeString)) 326 if (codeString == null || "".equals(codeString)) 327 return null; 328 if ("none".equals(codeString)) 329 return StructureMapGroupTypeMode.NONE; 330 if ("types".equals(codeString)) 331 return StructureMapGroupTypeMode.TYPES; 332 if ("type-and-types".equals(codeString)) 333 return StructureMapGroupTypeMode.TYPEANDTYPES; 334 throw new IllegalArgumentException("Unknown StructureMapGroupTypeMode code '" + codeString + "'"); 335 } 336 337 public Enumeration<StructureMapGroupTypeMode> fromType(PrimitiveType<?> code) throws FHIRException { 338 if (code == null) 339 return null; 340 if (code.isEmpty()) 341 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.NULL, code); 342 String codeString = code.asStringValue(); 343 if (codeString == null || "".equals(codeString)) 344 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.NULL, code); 345 if ("none".equals(codeString)) 346 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.NONE, code); 347 if ("types".equals(codeString)) 348 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.TYPES, code); 349 if ("type-and-types".equals(codeString)) 350 return new Enumeration<StructureMapGroupTypeMode>(this, StructureMapGroupTypeMode.TYPEANDTYPES, code); 351 throw new FHIRException("Unknown StructureMapGroupTypeMode code '" + codeString + "'"); 352 } 353 354 public String toCode(StructureMapGroupTypeMode code) { 355 if (code == StructureMapGroupTypeMode.NULL) 356 return null; 357 if (code == StructureMapGroupTypeMode.NONE) 358 return "none"; 359 if (code == StructureMapGroupTypeMode.TYPES) 360 return "types"; 361 if (code == StructureMapGroupTypeMode.TYPEANDTYPES) 362 return "type-and-types"; 363 return "?"; 364 } 365 366 public String toSystem(StructureMapGroupTypeMode code) { 367 return code.getSystem(); 368 } 369 } 370 371 public enum StructureMapInputMode { 372 /** 373 * Names an input instance used a source for mapping. 374 */ 375 SOURCE, 376 /** 377 * Names an instance that is being populated. 378 */ 379 TARGET, 380 /** 381 * added to help the parsers with the generic types 382 */ 383 NULL; 384 385 public static StructureMapInputMode fromCode(String codeString) throws FHIRException { 386 if (codeString == null || "".equals(codeString)) 387 return null; 388 if ("source".equals(codeString)) 389 return SOURCE; 390 if ("target".equals(codeString)) 391 return TARGET; 392 if (Configuration.isAcceptInvalidEnums()) 393 return null; 394 else 395 throw new FHIRException("Unknown StructureMapInputMode code '" + codeString + "'"); 396 } 397 398 public String toCode() { 399 switch (this) { 400 case SOURCE: 401 return "source"; 402 case TARGET: 403 return "target"; 404 case NULL: 405 return null; 406 default: 407 return "?"; 408 } 409 } 410 411 public String getSystem() { 412 switch (this) { 413 case SOURCE: 414 return "http://hl7.org/fhir/map-input-mode"; 415 case TARGET: 416 return "http://hl7.org/fhir/map-input-mode"; 417 case NULL: 418 return null; 419 default: 420 return "?"; 421 } 422 } 423 424 public String getDefinition() { 425 switch (this) { 426 case SOURCE: 427 return "Names an input instance used a source for mapping."; 428 case TARGET: 429 return "Names an instance that is being populated."; 430 case NULL: 431 return null; 432 default: 433 return "?"; 434 } 435 } 436 437 public String getDisplay() { 438 switch (this) { 439 case SOURCE: 440 return "Source Instance"; 441 case TARGET: 442 return "Target Instance"; 443 case NULL: 444 return null; 445 default: 446 return "?"; 447 } 448 } 449 } 450 451 public static class StructureMapInputModeEnumFactory implements EnumFactory<StructureMapInputMode> { 452 public StructureMapInputMode fromCode(String codeString) throws IllegalArgumentException { 453 if (codeString == null || "".equals(codeString)) 454 if (codeString == null || "".equals(codeString)) 455 return null; 456 if ("source".equals(codeString)) 457 return StructureMapInputMode.SOURCE; 458 if ("target".equals(codeString)) 459 return StructureMapInputMode.TARGET; 460 throw new IllegalArgumentException("Unknown StructureMapInputMode code '" + codeString + "'"); 461 } 462 463 public Enumeration<StructureMapInputMode> fromType(PrimitiveType<?> code) throws FHIRException { 464 if (code == null) 465 return null; 466 if (code.isEmpty()) 467 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.NULL, code); 468 String codeString = code.asStringValue(); 469 if (codeString == null || "".equals(codeString)) 470 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.NULL, code); 471 if ("source".equals(codeString)) 472 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.SOURCE, code); 473 if ("target".equals(codeString)) 474 return new Enumeration<StructureMapInputMode>(this, StructureMapInputMode.TARGET, code); 475 throw new FHIRException("Unknown StructureMapInputMode code '" + codeString + "'"); 476 } 477 478 public String toCode(StructureMapInputMode code) { 479 if (code == StructureMapInputMode.NULL) 480 return null; 481 if (code == StructureMapInputMode.SOURCE) 482 return "source"; 483 if (code == StructureMapInputMode.TARGET) 484 return "target"; 485 return "?"; 486 } 487 488 public String toSystem(StructureMapInputMode code) { 489 return code.getSystem(); 490 } 491 } 492 493 public enum StructureMapSourceListMode { 494 /** 495 * Only process this rule for the first in the list. 496 */ 497 FIRST, 498 /** 499 * Process this rule for all but the first. 500 */ 501 NOTFIRST, 502 /** 503 * Only process this rule for the last in the list. 504 */ 505 LAST, 506 /** 507 * Process this rule for all but the last. 508 */ 509 NOTLAST, 510 /** 511 * Only process this rule is there is only item. 512 */ 513 ONLYONE, 514 /** 515 * added to help the parsers with the generic types 516 */ 517 NULL; 518 519 public static StructureMapSourceListMode fromCode(String codeString) throws FHIRException { 520 if (codeString == null || "".equals(codeString)) 521 return null; 522 if ("first".equals(codeString)) 523 return FIRST; 524 if ("not_first".equals(codeString)) 525 return NOTFIRST; 526 if ("last".equals(codeString)) 527 return LAST; 528 if ("not_last".equals(codeString)) 529 return NOTLAST; 530 if ("only_one".equals(codeString)) 531 return ONLYONE; 532 if (Configuration.isAcceptInvalidEnums()) 533 return null; 534 else 535 throw new FHIRException("Unknown StructureMapSourceListMode code '" + codeString + "'"); 536 } 537 538 public String toCode() { 539 switch (this) { 540 case FIRST: 541 return "first"; 542 case NOTFIRST: 543 return "not_first"; 544 case LAST: 545 return "last"; 546 case NOTLAST: 547 return "not_last"; 548 case ONLYONE: 549 return "only_one"; 550 case NULL: 551 return null; 552 default: 553 return "?"; 554 } 555 } 556 557 public String getSystem() { 558 switch (this) { 559 case FIRST: 560 return "http://hl7.org/fhir/map-source-list-mode"; 561 case NOTFIRST: 562 return "http://hl7.org/fhir/map-source-list-mode"; 563 case LAST: 564 return "http://hl7.org/fhir/map-source-list-mode"; 565 case NOTLAST: 566 return "http://hl7.org/fhir/map-source-list-mode"; 567 case ONLYONE: 568 return "http://hl7.org/fhir/map-source-list-mode"; 569 case NULL: 570 return null; 571 default: 572 return "?"; 573 } 574 } 575 576 public String getDefinition() { 577 switch (this) { 578 case FIRST: 579 return "Only process this rule for the first in the list."; 580 case NOTFIRST: 581 return "Process this rule for all but the first."; 582 case LAST: 583 return "Only process this rule for the last in the list."; 584 case NOTLAST: 585 return "Process this rule for all but the last."; 586 case ONLYONE: 587 return "Only process this rule is there is only item."; 588 case NULL: 589 return null; 590 default: 591 return "?"; 592 } 593 } 594 595 public String getDisplay() { 596 switch (this) { 597 case FIRST: 598 return "First"; 599 case NOTFIRST: 600 return "All but the first"; 601 case LAST: 602 return "Last"; 603 case NOTLAST: 604 return "All but the last"; 605 case ONLYONE: 606 return "Enforce only one"; 607 case NULL: 608 return null; 609 default: 610 return "?"; 611 } 612 } 613 } 614 615 public static class StructureMapSourceListModeEnumFactory implements EnumFactory<StructureMapSourceListMode> { 616 public StructureMapSourceListMode fromCode(String codeString) throws IllegalArgumentException { 617 if (codeString == null || "".equals(codeString)) 618 if (codeString == null || "".equals(codeString)) 619 return null; 620 if ("first".equals(codeString)) 621 return StructureMapSourceListMode.FIRST; 622 if ("not_first".equals(codeString)) 623 return StructureMapSourceListMode.NOTFIRST; 624 if ("last".equals(codeString)) 625 return StructureMapSourceListMode.LAST; 626 if ("not_last".equals(codeString)) 627 return StructureMapSourceListMode.NOTLAST; 628 if ("only_one".equals(codeString)) 629 return StructureMapSourceListMode.ONLYONE; 630 throw new IllegalArgumentException("Unknown StructureMapSourceListMode code '" + codeString + "'"); 631 } 632 633 public Enumeration<StructureMapSourceListMode> fromType(PrimitiveType<?> code) throws FHIRException { 634 if (code == null) 635 return null; 636 if (code.isEmpty()) 637 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NULL, code); 638 String codeString = code.asStringValue(); 639 if (codeString == null || "".equals(codeString)) 640 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NULL, code); 641 if ("first".equals(codeString)) 642 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.FIRST, code); 643 if ("not_first".equals(codeString)) 644 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NOTFIRST, code); 645 if ("last".equals(codeString)) 646 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.LAST, code); 647 if ("not_last".equals(codeString)) 648 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.NOTLAST, code); 649 if ("only_one".equals(codeString)) 650 return new Enumeration<StructureMapSourceListMode>(this, StructureMapSourceListMode.ONLYONE, code); 651 throw new FHIRException("Unknown StructureMapSourceListMode code '" + codeString + "'"); 652 } 653 654 public String toCode(StructureMapSourceListMode code) { 655 if (code == StructureMapSourceListMode.NULL) 656 return null; 657 if (code == StructureMapSourceListMode.FIRST) 658 return "first"; 659 if (code == StructureMapSourceListMode.NOTFIRST) 660 return "not_first"; 661 if (code == StructureMapSourceListMode.LAST) 662 return "last"; 663 if (code == StructureMapSourceListMode.NOTLAST) 664 return "not_last"; 665 if (code == StructureMapSourceListMode.ONLYONE) 666 return "only_one"; 667 return "?"; 668 } 669 670 public String toSystem(StructureMapSourceListMode code) { 671 return code.getSystem(); 672 } 673 } 674 675 public enum StructureMapContextType { 676 /** 677 * The context specifies a type. 678 */ 679 TYPE, 680 /** 681 * The context specifies a variable. 682 */ 683 VARIABLE, 684 /** 685 * added to help the parsers with the generic types 686 */ 687 NULL; 688 689 public static StructureMapContextType fromCode(String codeString) throws FHIRException { 690 if (codeString == null || "".equals(codeString)) 691 return null; 692 if ("type".equals(codeString)) 693 return TYPE; 694 if ("variable".equals(codeString)) 695 return VARIABLE; 696 if (Configuration.isAcceptInvalidEnums()) 697 return null; 698 else 699 throw new FHIRException("Unknown StructureMapContextType code '" + codeString + "'"); 700 } 701 702 public String toCode() { 703 switch (this) { 704 case TYPE: 705 return "type"; 706 case VARIABLE: 707 return "variable"; 708 case NULL: 709 return null; 710 default: 711 return "?"; 712 } 713 } 714 715 public String getSystem() { 716 switch (this) { 717 case TYPE: 718 return "http://hl7.org/fhir/map-context-type"; 719 case VARIABLE: 720 return "http://hl7.org/fhir/map-context-type"; 721 case NULL: 722 return null; 723 default: 724 return "?"; 725 } 726 } 727 728 public String getDefinition() { 729 switch (this) { 730 case TYPE: 731 return "The context specifies a type."; 732 case VARIABLE: 733 return "The context specifies a variable."; 734 case NULL: 735 return null; 736 default: 737 return "?"; 738 } 739 } 740 741 public String getDisplay() { 742 switch (this) { 743 case TYPE: 744 return "Type"; 745 case VARIABLE: 746 return "Variable"; 747 case NULL: 748 return null; 749 default: 750 return "?"; 751 } 752 } 753 } 754 755 public static class StructureMapContextTypeEnumFactory implements EnumFactory<StructureMapContextType> { 756 public StructureMapContextType fromCode(String codeString) throws IllegalArgumentException { 757 if (codeString == null || "".equals(codeString)) 758 if (codeString == null || "".equals(codeString)) 759 return null; 760 if ("type".equals(codeString)) 761 return StructureMapContextType.TYPE; 762 if ("variable".equals(codeString)) 763 return StructureMapContextType.VARIABLE; 764 throw new IllegalArgumentException("Unknown StructureMapContextType code '" + codeString + "'"); 765 } 766 767 public Enumeration<StructureMapContextType> fromType(PrimitiveType<?> code) throws FHIRException { 768 if (code == null) 769 return null; 770 if (code.isEmpty()) 771 return new Enumeration<StructureMapContextType>(this, StructureMapContextType.NULL, code); 772 String codeString = code.asStringValue(); 773 if (codeString == null || "".equals(codeString)) 774 return new Enumeration<StructureMapContextType>(this, StructureMapContextType.NULL, code); 775 if ("type".equals(codeString)) 776 return new Enumeration<StructureMapContextType>(this, StructureMapContextType.TYPE, code); 777 if ("variable".equals(codeString)) 778 return new Enumeration<StructureMapContextType>(this, StructureMapContextType.VARIABLE, code); 779 throw new FHIRException("Unknown StructureMapContextType code '" + codeString + "'"); 780 } 781 782 public String toCode(StructureMapContextType code) { 783 if (code == StructureMapContextType.NULL) 784 return null; 785 if (code == StructureMapContextType.TYPE) 786 return "type"; 787 if (code == StructureMapContextType.VARIABLE) 788 return "variable"; 789 return "?"; 790 } 791 792 public String toSystem(StructureMapContextType code) { 793 return code.getSystem(); 794 } 795 } 796 797 public enum StructureMapTargetListMode { 798 /** 799 * when the target list is being assembled, the items for this rule go first. If 800 * more than one rule defines a first item (for a given instance of mapping) 801 * then this is an error. 802 */ 803 FIRST, 804 /** 805 * the target instance is shared with the target instances generated by another 806 * rule (up to the first common n items, then create new ones). 807 */ 808 SHARE, 809 /** 810 * when the target list is being assembled, the items for this rule go last. If 811 * more than one rule defines a last item (for a given instance of mapping) then 812 * this is an error. 813 */ 814 LAST, 815 /** 816 * re-use the first item in the list, and keep adding content to it. 817 */ 818 COLLATE, 819 /** 820 * added to help the parsers with the generic types 821 */ 822 NULL; 823 824 public static StructureMapTargetListMode fromCode(String codeString) throws FHIRException { 825 if (codeString == null || "".equals(codeString)) 826 return null; 827 if ("first".equals(codeString)) 828 return FIRST; 829 if ("share".equals(codeString)) 830 return SHARE; 831 if ("last".equals(codeString)) 832 return LAST; 833 if ("collate".equals(codeString)) 834 return COLLATE; 835 if (Configuration.isAcceptInvalidEnums()) 836 return null; 837 else 838 throw new FHIRException("Unknown StructureMapTargetListMode code '" + codeString + "'"); 839 } 840 841 public String toCode() { 842 switch (this) { 843 case FIRST: 844 return "first"; 845 case SHARE: 846 return "share"; 847 case LAST: 848 return "last"; 849 case COLLATE: 850 return "collate"; 851 case NULL: 852 return null; 853 default: 854 return "?"; 855 } 856 } 857 858 public String getSystem() { 859 switch (this) { 860 case FIRST: 861 return "http://hl7.org/fhir/map-target-list-mode"; 862 case SHARE: 863 return "http://hl7.org/fhir/map-target-list-mode"; 864 case LAST: 865 return "http://hl7.org/fhir/map-target-list-mode"; 866 case COLLATE: 867 return "http://hl7.org/fhir/map-target-list-mode"; 868 case NULL: 869 return null; 870 default: 871 return "?"; 872 } 873 } 874 875 public String getDefinition() { 876 switch (this) { 877 case FIRST: 878 return "when the target list is being assembled, the items for this rule go first. If more than one rule defines a first item (for a given instance of mapping) then this is an error."; 879 case SHARE: 880 return "the target instance is shared with the target instances generated by another rule (up to the first common n items, then create new ones)."; 881 case LAST: 882 return "when the target list is being assembled, the items for this rule go last. If more than one rule defines a last item (for a given instance of mapping) then this is an error."; 883 case COLLATE: 884 return "re-use the first item in the list, and keep adding content to it."; 885 case NULL: 886 return null; 887 default: 888 return "?"; 889 } 890 } 891 892 public String getDisplay() { 893 switch (this) { 894 case FIRST: 895 return "First"; 896 case SHARE: 897 return "Share"; 898 case LAST: 899 return "Last"; 900 case COLLATE: 901 return "Collate"; 902 case NULL: 903 return null; 904 default: 905 return "?"; 906 } 907 } 908 } 909 910 public static class StructureMapTargetListModeEnumFactory implements EnumFactory<StructureMapTargetListMode> { 911 public StructureMapTargetListMode fromCode(String codeString) throws IllegalArgumentException { 912 if (codeString == null || "".equals(codeString)) 913 if (codeString == null || "".equals(codeString)) 914 return null; 915 if ("first".equals(codeString)) 916 return StructureMapTargetListMode.FIRST; 917 if ("share".equals(codeString)) 918 return StructureMapTargetListMode.SHARE; 919 if ("last".equals(codeString)) 920 return StructureMapTargetListMode.LAST; 921 if ("collate".equals(codeString)) 922 return StructureMapTargetListMode.COLLATE; 923 throw new IllegalArgumentException("Unknown StructureMapTargetListMode code '" + codeString + "'"); 924 } 925 926 public Enumeration<StructureMapTargetListMode> fromType(PrimitiveType<?> code) throws FHIRException { 927 if (code == null) 928 return null; 929 if (code.isEmpty()) 930 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.NULL, code); 931 String codeString = code.asStringValue(); 932 if (codeString == null || "".equals(codeString)) 933 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.NULL, code); 934 if ("first".equals(codeString)) 935 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.FIRST, code); 936 if ("share".equals(codeString)) 937 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.SHARE, code); 938 if ("last".equals(codeString)) 939 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.LAST, code); 940 if ("collate".equals(codeString)) 941 return new Enumeration<StructureMapTargetListMode>(this, StructureMapTargetListMode.COLLATE, code); 942 throw new FHIRException("Unknown StructureMapTargetListMode code '" + codeString + "'"); 943 } 944 945 public String toCode(StructureMapTargetListMode code) { 946 if (code == StructureMapTargetListMode.NULL) 947 return null; 948 if (code == StructureMapTargetListMode.FIRST) 949 return "first"; 950 if (code == StructureMapTargetListMode.SHARE) 951 return "share"; 952 if (code == StructureMapTargetListMode.LAST) 953 return "last"; 954 if (code == StructureMapTargetListMode.COLLATE) 955 return "collate"; 956 return "?"; 957 } 958 959 public String toSystem(StructureMapTargetListMode code) { 960 return code.getSystem(); 961 } 962 } 963 964 public enum StructureMapTransform { 965 /** 966 * create(type : string) - type is passed through to the application on the 967 * standard API, and must be known by it. 968 */ 969 CREATE, 970 /** 971 * copy(source). 972 */ 973 COPY, 974 /** 975 * truncate(source, length) - source must be stringy type. 976 */ 977 TRUNCATE, 978 /** 979 * escape(source, fmt1, fmt2) - change source from one kind of escaping to 980 * another (plain, java, xml, json). note that this is for when the string 981 * itself is escaped. 982 */ 983 ESCAPE, 984 /** 985 * cast(source, type?) - case source from one type to another. target type can 986 * be left as implicit if there is one and only one target type known. 987 */ 988 CAST, 989 /** 990 * append(source...) - source is element or string. 991 */ 992 APPEND, 993 /** 994 * translate(source, uri_of_map) - use the translate operation. 995 */ 996 TRANSLATE, 997 /** 998 * reference(source : object) - return a string that references the provided 999 * tree properly. 1000 */ 1001 REFERENCE, 1002 /** 1003 * Perform a date operation. *Parameters to be documented*. 1004 */ 1005 DATEOP, 1006 /** 1007 * Generate a random UUID (in lowercase). No Parameters. 1008 */ 1009 UUID, 1010 /** 1011 * Return the appropriate string to put in a reference that refers to the 1012 * resource provided as a parameter. 1013 */ 1014 POINTER, 1015 /** 1016 * Execute the supplied FHIRPath expression and use the value returned by that. 1017 */ 1018 EVALUATE, 1019 /** 1020 * Create a CodeableConcept. Parameters = (text) or (system. Code[, display]). 1021 */ 1022 CC, 1023 /** 1024 * Create a Coding. Parameters = (system. Code[, display]). 1025 */ 1026 C, 1027 /** 1028 * Create a quantity. Parameters = (text) or (value, unit, [system, code]) where 1029 * text is the natural representation e.g. [comparator]value[space]unit. 1030 */ 1031 QTY, 1032 /** 1033 * Create an identifier. Parameters = (system, value[, type]) where type is a 1034 * code from the identifier type value set. 1035 */ 1036 ID, 1037 /** 1038 * Create a contact details. Parameters = (value) or (system, value). If no 1039 * system is provided, the system should be inferred from the content of the 1040 * value. 1041 */ 1042 CP, 1043 /** 1044 * added to help the parsers with the generic types 1045 */ 1046 NULL; 1047 1048 public static StructureMapTransform fromCode(String codeString) throws FHIRException { 1049 if (codeString == null || "".equals(codeString)) 1050 return null; 1051 if ("create".equals(codeString)) 1052 return CREATE; 1053 if ("copy".equals(codeString)) 1054 return COPY; 1055 if ("truncate".equals(codeString)) 1056 return TRUNCATE; 1057 if ("escape".equals(codeString)) 1058 return ESCAPE; 1059 if ("cast".equals(codeString)) 1060 return CAST; 1061 if ("append".equals(codeString)) 1062 return APPEND; 1063 if ("translate".equals(codeString)) 1064 return TRANSLATE; 1065 if ("reference".equals(codeString)) 1066 return REFERENCE; 1067 if ("dateOp".equals(codeString)) 1068 return DATEOP; 1069 if ("uuid".equals(codeString)) 1070 return UUID; 1071 if ("pointer".equals(codeString)) 1072 return POINTER; 1073 if ("evaluate".equals(codeString)) 1074 return EVALUATE; 1075 if ("cc".equals(codeString)) 1076 return CC; 1077 if ("c".equals(codeString)) 1078 return C; 1079 if ("qty".equals(codeString)) 1080 return QTY; 1081 if ("id".equals(codeString)) 1082 return ID; 1083 if ("cp".equals(codeString)) 1084 return CP; 1085 if (Configuration.isAcceptInvalidEnums()) 1086 return null; 1087 else 1088 throw new FHIRException("Unknown StructureMapTransform code '" + codeString + "'"); 1089 } 1090 1091 public String toCode() { 1092 switch (this) { 1093 case CREATE: 1094 return "create"; 1095 case COPY: 1096 return "copy"; 1097 case TRUNCATE: 1098 return "truncate"; 1099 case ESCAPE: 1100 return "escape"; 1101 case CAST: 1102 return "cast"; 1103 case APPEND: 1104 return "append"; 1105 case TRANSLATE: 1106 return "translate"; 1107 case REFERENCE: 1108 return "reference"; 1109 case DATEOP: 1110 return "dateOp"; 1111 case UUID: 1112 return "uuid"; 1113 case POINTER: 1114 return "pointer"; 1115 case EVALUATE: 1116 return "evaluate"; 1117 case CC: 1118 return "cc"; 1119 case C: 1120 return "c"; 1121 case QTY: 1122 return "qty"; 1123 case ID: 1124 return "id"; 1125 case CP: 1126 return "cp"; 1127 case NULL: 1128 return null; 1129 default: 1130 return "?"; 1131 } 1132 } 1133 1134 public String getSystem() { 1135 switch (this) { 1136 case CREATE: 1137 return "http://hl7.org/fhir/map-transform"; 1138 case COPY: 1139 return "http://hl7.org/fhir/map-transform"; 1140 case TRUNCATE: 1141 return "http://hl7.org/fhir/map-transform"; 1142 case ESCAPE: 1143 return "http://hl7.org/fhir/map-transform"; 1144 case CAST: 1145 return "http://hl7.org/fhir/map-transform"; 1146 case APPEND: 1147 return "http://hl7.org/fhir/map-transform"; 1148 case TRANSLATE: 1149 return "http://hl7.org/fhir/map-transform"; 1150 case REFERENCE: 1151 return "http://hl7.org/fhir/map-transform"; 1152 case DATEOP: 1153 return "http://hl7.org/fhir/map-transform"; 1154 case UUID: 1155 return "http://hl7.org/fhir/map-transform"; 1156 case POINTER: 1157 return "http://hl7.org/fhir/map-transform"; 1158 case EVALUATE: 1159 return "http://hl7.org/fhir/map-transform"; 1160 case CC: 1161 return "http://hl7.org/fhir/map-transform"; 1162 case C: 1163 return "http://hl7.org/fhir/map-transform"; 1164 case QTY: 1165 return "http://hl7.org/fhir/map-transform"; 1166 case ID: 1167 return "http://hl7.org/fhir/map-transform"; 1168 case CP: 1169 return "http://hl7.org/fhir/map-transform"; 1170 case NULL: 1171 return null; 1172 default: 1173 return "?"; 1174 } 1175 } 1176 1177 public String getDefinition() { 1178 switch (this) { 1179 case CREATE: 1180 return "create(type : string) - type is passed through to the application on the standard API, and must be known by it."; 1181 case COPY: 1182 return "copy(source)."; 1183 case TRUNCATE: 1184 return "truncate(source, length) - source must be stringy type."; 1185 case ESCAPE: 1186 return "escape(source, fmt1, fmt2) - change source from one kind of escaping to another (plain, java, xml, json). note that this is for when the string itself is escaped."; 1187 case CAST: 1188 return "cast(source, type?) - case source from one type to another. target type can be left as implicit if there is one and only one target type known."; 1189 case APPEND: 1190 return "append(source...) - source is element or string."; 1191 case TRANSLATE: 1192 return "translate(source, uri_of_map) - use the translate operation."; 1193 case REFERENCE: 1194 return "reference(source : object) - return a string that references the provided tree properly."; 1195 case DATEOP: 1196 return "Perform a date operation. *Parameters to be documented*."; 1197 case UUID: 1198 return "Generate a random UUID (in lowercase). No Parameters."; 1199 case POINTER: 1200 return "Return the appropriate string to put in a reference that refers to the resource provided as a parameter."; 1201 case EVALUATE: 1202 return "Execute the supplied FHIRPath expression and use the value returned by that."; 1203 case CC: 1204 return "Create a CodeableConcept. Parameters = (text) or (system. Code[, display])."; 1205 case C: 1206 return "Create a Coding. Parameters = (system. Code[, display])."; 1207 case QTY: 1208 return "Create a quantity. Parameters = (text) or (value, unit, [system, code]) where text is the natural representation e.g. [comparator]value[space]unit."; 1209 case ID: 1210 return "Create an identifier. Parameters = (system, value[, type]) where type is a code from the identifier type value set."; 1211 case CP: 1212 return "Create a contact details. Parameters = (value) or (system, value). If no system is provided, the system should be inferred from the content of the value."; 1213 case NULL: 1214 return null; 1215 default: 1216 return "?"; 1217 } 1218 } 1219 1220 public String getDisplay() { 1221 switch (this) { 1222 case CREATE: 1223 return "create"; 1224 case COPY: 1225 return "copy"; 1226 case TRUNCATE: 1227 return "truncate"; 1228 case ESCAPE: 1229 return "escape"; 1230 case CAST: 1231 return "cast"; 1232 case APPEND: 1233 return "append"; 1234 case TRANSLATE: 1235 return "translate"; 1236 case REFERENCE: 1237 return "reference"; 1238 case DATEOP: 1239 return "dateOp"; 1240 case UUID: 1241 return "uuid"; 1242 case POINTER: 1243 return "pointer"; 1244 case EVALUATE: 1245 return "evaluate"; 1246 case CC: 1247 return "cc"; 1248 case C: 1249 return "c"; 1250 case QTY: 1251 return "qty"; 1252 case ID: 1253 return "id"; 1254 case CP: 1255 return "cp"; 1256 case NULL: 1257 return null; 1258 default: 1259 return "?"; 1260 } 1261 } 1262 } 1263 1264 public static class StructureMapTransformEnumFactory implements EnumFactory<StructureMapTransform> { 1265 public StructureMapTransform fromCode(String codeString) throws IllegalArgumentException { 1266 if (codeString == null || "".equals(codeString)) 1267 if (codeString == null || "".equals(codeString)) 1268 return null; 1269 if ("create".equals(codeString)) 1270 return StructureMapTransform.CREATE; 1271 if ("copy".equals(codeString)) 1272 return StructureMapTransform.COPY; 1273 if ("truncate".equals(codeString)) 1274 return StructureMapTransform.TRUNCATE; 1275 if ("escape".equals(codeString)) 1276 return StructureMapTransform.ESCAPE; 1277 if ("cast".equals(codeString)) 1278 return StructureMapTransform.CAST; 1279 if ("append".equals(codeString)) 1280 return StructureMapTransform.APPEND; 1281 if ("translate".equals(codeString)) 1282 return StructureMapTransform.TRANSLATE; 1283 if ("reference".equals(codeString)) 1284 return StructureMapTransform.REFERENCE; 1285 if ("dateOp".equals(codeString)) 1286 return StructureMapTransform.DATEOP; 1287 if ("uuid".equals(codeString)) 1288 return StructureMapTransform.UUID; 1289 if ("pointer".equals(codeString)) 1290 return StructureMapTransform.POINTER; 1291 if ("evaluate".equals(codeString)) 1292 return StructureMapTransform.EVALUATE; 1293 if ("cc".equals(codeString)) 1294 return StructureMapTransform.CC; 1295 if ("c".equals(codeString)) 1296 return StructureMapTransform.C; 1297 if ("qty".equals(codeString)) 1298 return StructureMapTransform.QTY; 1299 if ("id".equals(codeString)) 1300 return StructureMapTransform.ID; 1301 if ("cp".equals(codeString)) 1302 return StructureMapTransform.CP; 1303 throw new IllegalArgumentException("Unknown StructureMapTransform code '" + codeString + "'"); 1304 } 1305 1306 public Enumeration<StructureMapTransform> fromType(PrimitiveType<?> code) throws FHIRException { 1307 if (code == null) 1308 return null; 1309 if (code.isEmpty()) 1310 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.NULL, code); 1311 String codeString = code.asStringValue(); 1312 if (codeString == null || "".equals(codeString)) 1313 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.NULL, code); 1314 if ("create".equals(codeString)) 1315 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CREATE, code); 1316 if ("copy".equals(codeString)) 1317 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.COPY, code); 1318 if ("truncate".equals(codeString)) 1319 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.TRUNCATE, code); 1320 if ("escape".equals(codeString)) 1321 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.ESCAPE, code); 1322 if ("cast".equals(codeString)) 1323 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CAST, code); 1324 if ("append".equals(codeString)) 1325 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.APPEND, code); 1326 if ("translate".equals(codeString)) 1327 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.TRANSLATE, code); 1328 if ("reference".equals(codeString)) 1329 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.REFERENCE, code); 1330 if ("dateOp".equals(codeString)) 1331 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.DATEOP, code); 1332 if ("uuid".equals(codeString)) 1333 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.UUID, code); 1334 if ("pointer".equals(codeString)) 1335 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.POINTER, code); 1336 if ("evaluate".equals(codeString)) 1337 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.EVALUATE, code); 1338 if ("cc".equals(codeString)) 1339 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CC, code); 1340 if ("c".equals(codeString)) 1341 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.C, code); 1342 if ("qty".equals(codeString)) 1343 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.QTY, code); 1344 if ("id".equals(codeString)) 1345 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.ID, code); 1346 if ("cp".equals(codeString)) 1347 return new Enumeration<StructureMapTransform>(this, StructureMapTransform.CP, code); 1348 throw new FHIRException("Unknown StructureMapTransform code '" + codeString + "'"); 1349 } 1350 1351 public String toCode(StructureMapTransform code) { 1352 if (code == StructureMapTransform.NULL) 1353 return null; 1354 if (code == StructureMapTransform.CREATE) 1355 return "create"; 1356 if (code == StructureMapTransform.COPY) 1357 return "copy"; 1358 if (code == StructureMapTransform.TRUNCATE) 1359 return "truncate"; 1360 if (code == StructureMapTransform.ESCAPE) 1361 return "escape"; 1362 if (code == StructureMapTransform.CAST) 1363 return "cast"; 1364 if (code == StructureMapTransform.APPEND) 1365 return "append"; 1366 if (code == StructureMapTransform.TRANSLATE) 1367 return "translate"; 1368 if (code == StructureMapTransform.REFERENCE) 1369 return "reference"; 1370 if (code == StructureMapTransform.DATEOP) 1371 return "dateOp"; 1372 if (code == StructureMapTransform.UUID) 1373 return "uuid"; 1374 if (code == StructureMapTransform.POINTER) 1375 return "pointer"; 1376 if (code == StructureMapTransform.EVALUATE) 1377 return "evaluate"; 1378 if (code == StructureMapTransform.CC) 1379 return "cc"; 1380 if (code == StructureMapTransform.C) 1381 return "c"; 1382 if (code == StructureMapTransform.QTY) 1383 return "qty"; 1384 if (code == StructureMapTransform.ID) 1385 return "id"; 1386 if (code == StructureMapTransform.CP) 1387 return "cp"; 1388 return "?"; 1389 } 1390 1391 public String toSystem(StructureMapTransform code) { 1392 return code.getSystem(); 1393 } 1394 } 1395 1396 @Block() 1397 public static class StructureMapStructureComponent extends BackboneElement implements IBaseBackboneElement { 1398 /** 1399 * The canonical reference to the structure. 1400 */ 1401 @Child(name = "url", type = { CanonicalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1402 @Description(shortDefinition = "Canonical reference to structure definition", formalDefinition = "The canonical reference to the structure.") 1403 protected CanonicalType url; 1404 1405 /** 1406 * How the referenced structure is used in this mapping. 1407 */ 1408 @Child(name = "mode", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1409 @Description(shortDefinition = "source | queried | target | produced", formalDefinition = "How the referenced structure is used in this mapping.") 1410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/map-model-mode") 1411 protected Enumeration<StructureMapModelMode> mode; 1412 1413 /** 1414 * The name used for this type in the map. 1415 */ 1416 @Child(name = "alias", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1417 @Description(shortDefinition = "Name for type in this map", formalDefinition = "The name used for this type in the map.") 1418 protected StringType alias; 1419 1420 /** 1421 * Documentation that describes how the structure is used in the mapping. 1422 */ 1423 @Child(name = "documentation", type = { 1424 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1425 @Description(shortDefinition = "Documentation on use of structure", formalDefinition = "Documentation that describes how the structure is used in the mapping.") 1426 protected StringType documentation; 1427 1428 private static final long serialVersionUID = 364750586L; 1429 1430 /** 1431 * Constructor 1432 */ 1433 public StructureMapStructureComponent() { 1434 super(); 1435 } 1436 1437 /** 1438 * Constructor 1439 */ 1440 public StructureMapStructureComponent(CanonicalType url, Enumeration<StructureMapModelMode> mode) { 1441 super(); 1442 this.url = url; 1443 this.mode = mode; 1444 } 1445 1446 /** 1447 * @return {@link #url} (The canonical reference to the structure.). This is the 1448 * underlying object with id, value and extensions. The accessor 1449 * "getUrl" gives direct access to the value 1450 */ 1451 public CanonicalType getUrlElement() { 1452 if (this.url == null) 1453 if (Configuration.errorOnAutoCreate()) 1454 throw new Error("Attempt to auto-create StructureMapStructureComponent.url"); 1455 else if (Configuration.doAutoCreate()) 1456 this.url = new CanonicalType(); // bb 1457 return this.url; 1458 } 1459 1460 public boolean hasUrlElement() { 1461 return this.url != null && !this.url.isEmpty(); 1462 } 1463 1464 public boolean hasUrl() { 1465 return this.url != null && !this.url.isEmpty(); 1466 } 1467 1468 /** 1469 * @param value {@link #url} (The canonical reference to the structure.). This 1470 * is the underlying object with id, value and extensions. The 1471 * accessor "getUrl" gives direct access to the value 1472 */ 1473 public StructureMapStructureComponent setUrlElement(CanonicalType value) { 1474 this.url = value; 1475 return this; 1476 } 1477 1478 /** 1479 * @return The canonical reference to the structure. 1480 */ 1481 public String getUrl() { 1482 return this.url == null ? null : this.url.getValue(); 1483 } 1484 1485 /** 1486 * @param value The canonical reference to the structure. 1487 */ 1488 public StructureMapStructureComponent setUrl(String value) { 1489 if (this.url == null) 1490 this.url = new CanonicalType(); 1491 this.url.setValue(value); 1492 return this; 1493 } 1494 1495 /** 1496 * @return {@link #mode} (How the referenced structure is used in this 1497 * mapping.). This is the underlying object with id, value and 1498 * extensions. The accessor "getMode" gives direct access to the value 1499 */ 1500 public Enumeration<StructureMapModelMode> getModeElement() { 1501 if (this.mode == null) 1502 if (Configuration.errorOnAutoCreate()) 1503 throw new Error("Attempt to auto-create StructureMapStructureComponent.mode"); 1504 else if (Configuration.doAutoCreate()) 1505 this.mode = new Enumeration<StructureMapModelMode>(new StructureMapModelModeEnumFactory()); // bb 1506 return this.mode; 1507 } 1508 1509 public boolean hasModeElement() { 1510 return this.mode != null && !this.mode.isEmpty(); 1511 } 1512 1513 public boolean hasMode() { 1514 return this.mode != null && !this.mode.isEmpty(); 1515 } 1516 1517 /** 1518 * @param value {@link #mode} (How the referenced structure is used in this 1519 * mapping.). This is the underlying object with id, value and 1520 * extensions. The accessor "getMode" gives direct access to the 1521 * value 1522 */ 1523 public StructureMapStructureComponent setModeElement(Enumeration<StructureMapModelMode> value) { 1524 this.mode = value; 1525 return this; 1526 } 1527 1528 /** 1529 * @return How the referenced structure is used in this mapping. 1530 */ 1531 public StructureMapModelMode getMode() { 1532 return this.mode == null ? null : this.mode.getValue(); 1533 } 1534 1535 /** 1536 * @param value How the referenced structure is used in this mapping. 1537 */ 1538 public StructureMapStructureComponent setMode(StructureMapModelMode value) { 1539 if (this.mode == null) 1540 this.mode = new Enumeration<StructureMapModelMode>(new StructureMapModelModeEnumFactory()); 1541 this.mode.setValue(value); 1542 return this; 1543 } 1544 1545 /** 1546 * @return {@link #alias} (The name used for this type in the map.). This is the 1547 * underlying object with id, value and extensions. The accessor 1548 * "getAlias" gives direct access to the value 1549 */ 1550 public StringType getAliasElement() { 1551 if (this.alias == null) 1552 if (Configuration.errorOnAutoCreate()) 1553 throw new Error("Attempt to auto-create StructureMapStructureComponent.alias"); 1554 else if (Configuration.doAutoCreate()) 1555 this.alias = new StringType(); // bb 1556 return this.alias; 1557 } 1558 1559 public boolean hasAliasElement() { 1560 return this.alias != null && !this.alias.isEmpty(); 1561 } 1562 1563 public boolean hasAlias() { 1564 return this.alias != null && !this.alias.isEmpty(); 1565 } 1566 1567 /** 1568 * @param value {@link #alias} (The name used for this type in the map.). This 1569 * is the underlying object with id, value and extensions. The 1570 * accessor "getAlias" gives direct access to the value 1571 */ 1572 public StructureMapStructureComponent setAliasElement(StringType value) { 1573 this.alias = value; 1574 return this; 1575 } 1576 1577 /** 1578 * @return The name used for this type in the map. 1579 */ 1580 public String getAlias() { 1581 return this.alias == null ? null : this.alias.getValue(); 1582 } 1583 1584 /** 1585 * @param value The name used for this type in the map. 1586 */ 1587 public StructureMapStructureComponent setAlias(String value) { 1588 if (Utilities.noString(value)) 1589 this.alias = null; 1590 else { 1591 if (this.alias == null) 1592 this.alias = new StringType(); 1593 this.alias.setValue(value); 1594 } 1595 return this; 1596 } 1597 1598 /** 1599 * @return {@link #documentation} (Documentation that describes how the 1600 * structure is used in the mapping.). This is the underlying object 1601 * with id, value and extensions. The accessor "getDocumentation" gives 1602 * direct access to the value 1603 */ 1604 public StringType getDocumentationElement() { 1605 if (this.documentation == null) 1606 if (Configuration.errorOnAutoCreate()) 1607 throw new Error("Attempt to auto-create StructureMapStructureComponent.documentation"); 1608 else if (Configuration.doAutoCreate()) 1609 this.documentation = new StringType(); // bb 1610 return this.documentation; 1611 } 1612 1613 public boolean hasDocumentationElement() { 1614 return this.documentation != null && !this.documentation.isEmpty(); 1615 } 1616 1617 public boolean hasDocumentation() { 1618 return this.documentation != null && !this.documentation.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #documentation} (Documentation that describes how the 1623 * structure is used in the mapping.). This is the underlying 1624 * object with id, value and extensions. The accessor 1625 * "getDocumentation" gives direct access to the value 1626 */ 1627 public StructureMapStructureComponent setDocumentationElement(StringType value) { 1628 this.documentation = value; 1629 return this; 1630 } 1631 1632 /** 1633 * @return Documentation that describes how the structure is used in the 1634 * mapping. 1635 */ 1636 public String getDocumentation() { 1637 return this.documentation == null ? null : this.documentation.getValue(); 1638 } 1639 1640 /** 1641 * @param value Documentation that describes how the structure is used in the 1642 * mapping. 1643 */ 1644 public StructureMapStructureComponent setDocumentation(String value) { 1645 if (Utilities.noString(value)) 1646 this.documentation = null; 1647 else { 1648 if (this.documentation == null) 1649 this.documentation = new StringType(); 1650 this.documentation.setValue(value); 1651 } 1652 return this; 1653 } 1654 1655 protected void listChildren(List<Property> children) { 1656 super.listChildren(children); 1657 children.add(new Property("url", "canonical(StructureDefinition)", "The canonical reference to the structure.", 0, 1658 1, url)); 1659 children.add(new Property("mode", "code", "How the referenced structure is used in this mapping.", 0, 1, mode)); 1660 children.add(new Property("alias", "string", "The name used for this type in the map.", 0, 1, alias)); 1661 children.add(new Property("documentation", "string", 1662 "Documentation that describes how the structure is used in the mapping.", 0, 1, documentation)); 1663 } 1664 1665 @Override 1666 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1667 switch (_hash) { 1668 case 116079: 1669 /* url */ return new Property("url", "canonical(StructureDefinition)", 1670 "The canonical reference to the structure.", 0, 1, url); 1671 case 3357091: 1672 /* mode */ return new Property("mode", "code", "How the referenced structure is used in this mapping.", 0, 1, 1673 mode); 1674 case 92902992: 1675 /* alias */ return new Property("alias", "string", "The name used for this type in the map.", 0, 1, alias); 1676 case 1587405498: 1677 /* documentation */ return new Property("documentation", "string", 1678 "Documentation that describes how the structure is used in the mapping.", 0, 1, documentation); 1679 default: 1680 return super.getNamedProperty(_hash, _name, _checkValid); 1681 } 1682 1683 } 1684 1685 @Override 1686 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1687 switch (hash) { 1688 case 116079: 1689 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // CanonicalType 1690 case 3357091: 1691 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<StructureMapModelMode> 1692 case 92902992: 1693 /* alias */ return this.alias == null ? new Base[0] : new Base[] { this.alias }; // StringType 1694 case 1587405498: 1695 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 1696 default: 1697 return super.getProperty(hash, name, checkValid); 1698 } 1699 1700 } 1701 1702 @Override 1703 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1704 switch (hash) { 1705 case 116079: // url 1706 this.url = castToCanonical(value); // CanonicalType 1707 return value; 1708 case 3357091: // mode 1709 value = new StructureMapModelModeEnumFactory().fromType(castToCode(value)); 1710 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1711 return value; 1712 case 92902992: // alias 1713 this.alias = castToString(value); // StringType 1714 return value; 1715 case 1587405498: // documentation 1716 this.documentation = castToString(value); // StringType 1717 return value; 1718 default: 1719 return super.setProperty(hash, name, value); 1720 } 1721 1722 } 1723 1724 @Override 1725 public Base setProperty(String name, Base value) throws FHIRException { 1726 if (name.equals("url")) { 1727 this.url = castToCanonical(value); // CanonicalType 1728 } else if (name.equals("mode")) { 1729 value = new StructureMapModelModeEnumFactory().fromType(castToCode(value)); 1730 this.mode = (Enumeration) value; // Enumeration<StructureMapModelMode> 1731 } else if (name.equals("alias")) { 1732 this.alias = castToString(value); // StringType 1733 } else if (name.equals("documentation")) { 1734 this.documentation = castToString(value); // StringType 1735 } else 1736 return super.setProperty(name, value); 1737 return value; 1738 } 1739 1740 @Override 1741 public void removeChild(String name, Base value) throws FHIRException { 1742 if (name.equals("url")) { 1743 this.url = null; 1744 } else if (name.equals("mode")) { 1745 this.mode = null; 1746 } else if (name.equals("alias")) { 1747 this.alias = null; 1748 } else if (name.equals("documentation")) { 1749 this.documentation = null; 1750 } else 1751 super.removeChild(name, value); 1752 1753 } 1754 1755 @Override 1756 public Base makeProperty(int hash, String name) throws FHIRException { 1757 switch (hash) { 1758 case 116079: 1759 return getUrlElement(); 1760 case 3357091: 1761 return getModeElement(); 1762 case 92902992: 1763 return getAliasElement(); 1764 case 1587405498: 1765 return getDocumentationElement(); 1766 default: 1767 return super.makeProperty(hash, name); 1768 } 1769 1770 } 1771 1772 @Override 1773 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1774 switch (hash) { 1775 case 116079: 1776 /* url */ return new String[] { "canonical" }; 1777 case 3357091: 1778 /* mode */ return new String[] { "code" }; 1779 case 92902992: 1780 /* alias */ return new String[] { "string" }; 1781 case 1587405498: 1782 /* documentation */ return new String[] { "string" }; 1783 default: 1784 return super.getTypesForProperty(hash, name); 1785 } 1786 1787 } 1788 1789 @Override 1790 public Base addChild(String name) throws FHIRException { 1791 if (name.equals("url")) { 1792 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.url"); 1793 } else if (name.equals("mode")) { 1794 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.mode"); 1795 } else if (name.equals("alias")) { 1796 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.alias"); 1797 } else if (name.equals("documentation")) { 1798 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.documentation"); 1799 } else 1800 return super.addChild(name); 1801 } 1802 1803 public StructureMapStructureComponent copy() { 1804 StructureMapStructureComponent dst = new StructureMapStructureComponent(); 1805 copyValues(dst); 1806 return dst; 1807 } 1808 1809 public void copyValues(StructureMapStructureComponent dst) { 1810 super.copyValues(dst); 1811 dst.url = url == null ? null : url.copy(); 1812 dst.mode = mode == null ? null : mode.copy(); 1813 dst.alias = alias == null ? null : alias.copy(); 1814 dst.documentation = documentation == null ? null : documentation.copy(); 1815 } 1816 1817 @Override 1818 public boolean equalsDeep(Base other_) { 1819 if (!super.equalsDeep(other_)) 1820 return false; 1821 if (!(other_ instanceof StructureMapStructureComponent)) 1822 return false; 1823 StructureMapStructureComponent o = (StructureMapStructureComponent) other_; 1824 return compareDeep(url, o.url, true) && compareDeep(mode, o.mode, true) && compareDeep(alias, o.alias, true) 1825 && compareDeep(documentation, o.documentation, true); 1826 } 1827 1828 @Override 1829 public boolean equalsShallow(Base other_) { 1830 if (!super.equalsShallow(other_)) 1831 return false; 1832 if (!(other_ instanceof StructureMapStructureComponent)) 1833 return false; 1834 StructureMapStructureComponent o = (StructureMapStructureComponent) other_; 1835 return compareValues(mode, o.mode, true) && compareValues(alias, o.alias, true) 1836 && compareValues(documentation, o.documentation, true); 1837 } 1838 1839 public boolean isEmpty() { 1840 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, mode, alias, documentation); 1841 } 1842 1843 public String fhirType() { 1844 return "StructureMap.structure"; 1845 1846 } 1847 1848 } 1849 1850 @Block() 1851 public static class StructureMapGroupComponent extends BackboneElement implements IBaseBackboneElement { 1852 /** 1853 * A unique name for the group for the convenience of human readers. 1854 */ 1855 @Child(name = "name", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1856 @Description(shortDefinition = "Human-readable label", formalDefinition = "A unique name for the group for the convenience of human readers.") 1857 protected IdType name; 1858 1859 /** 1860 * Another group that this group adds rules to. 1861 */ 1862 @Child(name = "extends", type = { IdType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1863 @Description(shortDefinition = "Another group that this group adds rules to", formalDefinition = "Another group that this group adds rules to.") 1864 protected IdType extends_; 1865 1866 /** 1867 * If this is the default rule set to apply for the source type or this 1868 * combination of types. 1869 */ 1870 @Child(name = "typeMode", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1871 @Description(shortDefinition = "none | types | type-and-types", formalDefinition = "If this is the default rule set to apply for the source type or this combination of types.") 1872 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/map-group-type-mode") 1873 protected Enumeration<StructureMapGroupTypeMode> typeMode; 1874 1875 /** 1876 * Additional supporting documentation that explains the purpose of the group 1877 * and the types of mappings within it. 1878 */ 1879 @Child(name = "documentation", type = { 1880 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1881 @Description(shortDefinition = "Additional description/explanation for group", formalDefinition = "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.") 1882 protected StringType documentation; 1883 1884 /** 1885 * A name assigned to an instance of data. The instance must be provided when 1886 * the mapping is invoked. 1887 */ 1888 @Child(name = "input", type = {}, order = 5, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1889 @Description(shortDefinition = "Named instance provided when invoking the map", formalDefinition = "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.") 1890 protected List<StructureMapGroupInputComponent> input; 1891 1892 /** 1893 * Transform Rule from source to target. 1894 */ 1895 @Child(name = "rule", type = {}, order = 6, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1896 @Description(shortDefinition = "Transform Rule from source to target", formalDefinition = "Transform Rule from source to target.") 1897 protected List<StructureMapGroupRuleComponent> rule; 1898 1899 private static final long serialVersionUID = -1474595081L; 1900 1901 /** 1902 * Constructor 1903 */ 1904 public StructureMapGroupComponent() { 1905 super(); 1906 } 1907 1908 /** 1909 * Constructor 1910 */ 1911 public StructureMapGroupComponent(IdType name, Enumeration<StructureMapGroupTypeMode> typeMode) { 1912 super(); 1913 this.name = name; 1914 this.typeMode = typeMode; 1915 } 1916 1917 /** 1918 * @return {@link #name} (A unique name for the group for the convenience of 1919 * human readers.). This is the underlying object with id, value and 1920 * extensions. The accessor "getName" gives direct access to the value 1921 */ 1922 public IdType getNameElement() { 1923 if (this.name == null) 1924 if (Configuration.errorOnAutoCreate()) 1925 throw new Error("Attempt to auto-create StructureMapGroupComponent.name"); 1926 else if (Configuration.doAutoCreate()) 1927 this.name = new IdType(); // bb 1928 return this.name; 1929 } 1930 1931 public boolean hasNameElement() { 1932 return this.name != null && !this.name.isEmpty(); 1933 } 1934 1935 public boolean hasName() { 1936 return this.name != null && !this.name.isEmpty(); 1937 } 1938 1939 /** 1940 * @param value {@link #name} (A unique name for the group for the convenience 1941 * of human readers.). This is the underlying object with id, value 1942 * and extensions. The accessor "getName" gives direct access to 1943 * the value 1944 */ 1945 public StructureMapGroupComponent setNameElement(IdType value) { 1946 this.name = value; 1947 return this; 1948 } 1949 1950 /** 1951 * @return A unique name for the group for the convenience of human readers. 1952 */ 1953 public String getName() { 1954 return this.name == null ? null : this.name.getValue(); 1955 } 1956 1957 /** 1958 * @param value A unique name for the group for the convenience of human 1959 * readers. 1960 */ 1961 public StructureMapGroupComponent setName(String value) { 1962 if (this.name == null) 1963 this.name = new IdType(); 1964 this.name.setValue(value); 1965 return this; 1966 } 1967 1968 /** 1969 * @return {@link #extends_} (Another group that this group adds rules to.). 1970 * This is the underlying object with id, value and extensions. The 1971 * accessor "getExtends" gives direct access to the value 1972 */ 1973 public IdType getExtendsElement() { 1974 if (this.extends_ == null) 1975 if (Configuration.errorOnAutoCreate()) 1976 throw new Error("Attempt to auto-create StructureMapGroupComponent.extends_"); 1977 else if (Configuration.doAutoCreate()) 1978 this.extends_ = new IdType(); // bb 1979 return this.extends_; 1980 } 1981 1982 public boolean hasExtendsElement() { 1983 return this.extends_ != null && !this.extends_.isEmpty(); 1984 } 1985 1986 public boolean hasExtends() { 1987 return this.extends_ != null && !this.extends_.isEmpty(); 1988 } 1989 1990 /** 1991 * @param value {@link #extends_} (Another group that this group adds rules 1992 * to.). This is the underlying object with id, value and 1993 * extensions. The accessor "getExtends" gives direct access to the 1994 * value 1995 */ 1996 public StructureMapGroupComponent setExtendsElement(IdType value) { 1997 this.extends_ = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return Another group that this group adds rules to. 2003 */ 2004 public String getExtends() { 2005 return this.extends_ == null ? null : this.extends_.getValue(); 2006 } 2007 2008 /** 2009 * @param value Another group that this group adds rules to. 2010 */ 2011 public StructureMapGroupComponent setExtends(String value) { 2012 if (Utilities.noString(value)) 2013 this.extends_ = null; 2014 else { 2015 if (this.extends_ == null) 2016 this.extends_ = new IdType(); 2017 this.extends_.setValue(value); 2018 } 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #typeMode} (If this is the default rule set to apply for the 2024 * source type or this combination of types.). This is the underlying 2025 * object with id, value and extensions. The accessor "getTypeMode" 2026 * gives direct access to the value 2027 */ 2028 public Enumeration<StructureMapGroupTypeMode> getTypeModeElement() { 2029 if (this.typeMode == null) 2030 if (Configuration.errorOnAutoCreate()) 2031 throw new Error("Attempt to auto-create StructureMapGroupComponent.typeMode"); 2032 else if (Configuration.doAutoCreate()) 2033 this.typeMode = new Enumeration<StructureMapGroupTypeMode>(new StructureMapGroupTypeModeEnumFactory()); // bb 2034 return this.typeMode; 2035 } 2036 2037 public boolean hasTypeModeElement() { 2038 return this.typeMode != null && !this.typeMode.isEmpty(); 2039 } 2040 2041 public boolean hasTypeMode() { 2042 return this.typeMode != null && !this.typeMode.isEmpty(); 2043 } 2044 2045 /** 2046 * @param value {@link #typeMode} (If this is the default rule set to apply for 2047 * the source type or this combination of types.). This is the 2048 * underlying object with id, value and extensions. The accessor 2049 * "getTypeMode" gives direct access to the value 2050 */ 2051 public StructureMapGroupComponent setTypeModeElement(Enumeration<StructureMapGroupTypeMode> value) { 2052 this.typeMode = value; 2053 return this; 2054 } 2055 2056 /** 2057 * @return If this is the default rule set to apply for the source type or this 2058 * combination of types. 2059 */ 2060 public StructureMapGroupTypeMode getTypeMode() { 2061 return this.typeMode == null ? null : this.typeMode.getValue(); 2062 } 2063 2064 /** 2065 * @param value If this is the default rule set to apply for the source type or 2066 * this combination of types. 2067 */ 2068 public StructureMapGroupComponent setTypeMode(StructureMapGroupTypeMode value) { 2069 if (this.typeMode == null) 2070 this.typeMode = new Enumeration<StructureMapGroupTypeMode>(new StructureMapGroupTypeModeEnumFactory()); 2071 this.typeMode.setValue(value); 2072 return this; 2073 } 2074 2075 /** 2076 * @return {@link #documentation} (Additional supporting documentation that 2077 * explains the purpose of the group and the types of mappings within 2078 * it.). This is the underlying object with id, value and extensions. 2079 * The accessor "getDocumentation" gives direct access to the value 2080 */ 2081 public StringType getDocumentationElement() { 2082 if (this.documentation == null) 2083 if (Configuration.errorOnAutoCreate()) 2084 throw new Error("Attempt to auto-create StructureMapGroupComponent.documentation"); 2085 else if (Configuration.doAutoCreate()) 2086 this.documentation = new StringType(); // bb 2087 return this.documentation; 2088 } 2089 2090 public boolean hasDocumentationElement() { 2091 return this.documentation != null && !this.documentation.isEmpty(); 2092 } 2093 2094 public boolean hasDocumentation() { 2095 return this.documentation != null && !this.documentation.isEmpty(); 2096 } 2097 2098 /** 2099 * @param value {@link #documentation} (Additional supporting documentation that 2100 * explains the purpose of the group and the types of mappings 2101 * within it.). This is the underlying object with id, value and 2102 * extensions. The accessor "getDocumentation" gives direct access 2103 * to the value 2104 */ 2105 public StructureMapGroupComponent setDocumentationElement(StringType value) { 2106 this.documentation = value; 2107 return this; 2108 } 2109 2110 /** 2111 * @return Additional supporting documentation that explains the purpose of the 2112 * group and the types of mappings within it. 2113 */ 2114 public String getDocumentation() { 2115 return this.documentation == null ? null : this.documentation.getValue(); 2116 } 2117 2118 /** 2119 * @param value Additional supporting documentation that explains the purpose of 2120 * the group and the types of mappings within it. 2121 */ 2122 public StructureMapGroupComponent setDocumentation(String value) { 2123 if (Utilities.noString(value)) 2124 this.documentation = null; 2125 else { 2126 if (this.documentation == null) 2127 this.documentation = new StringType(); 2128 this.documentation.setValue(value); 2129 } 2130 return this; 2131 } 2132 2133 /** 2134 * @return {@link #input} (A name assigned to an instance of data. The instance 2135 * must be provided when the mapping is invoked.) 2136 */ 2137 public List<StructureMapGroupInputComponent> getInput() { 2138 if (this.input == null) 2139 this.input = new ArrayList<StructureMapGroupInputComponent>(); 2140 return this.input; 2141 } 2142 2143 /** 2144 * @return Returns a reference to <code>this</code> for easy method chaining 2145 */ 2146 public StructureMapGroupComponent setInput(List<StructureMapGroupInputComponent> theInput) { 2147 this.input = theInput; 2148 return this; 2149 } 2150 2151 public boolean hasInput() { 2152 if (this.input == null) 2153 return false; 2154 for (StructureMapGroupInputComponent item : this.input) 2155 if (!item.isEmpty()) 2156 return true; 2157 return false; 2158 } 2159 2160 public StructureMapGroupInputComponent addInput() { // 3 2161 StructureMapGroupInputComponent t = new StructureMapGroupInputComponent(); 2162 if (this.input == null) 2163 this.input = new ArrayList<StructureMapGroupInputComponent>(); 2164 this.input.add(t); 2165 return t; 2166 } 2167 2168 public StructureMapGroupComponent addInput(StructureMapGroupInputComponent t) { // 3 2169 if (t == null) 2170 return this; 2171 if (this.input == null) 2172 this.input = new ArrayList<StructureMapGroupInputComponent>(); 2173 this.input.add(t); 2174 return this; 2175 } 2176 2177 /** 2178 * @return The first repetition of repeating field {@link #input}, creating it 2179 * if it does not already exist 2180 */ 2181 public StructureMapGroupInputComponent getInputFirstRep() { 2182 if (getInput().isEmpty()) { 2183 addInput(); 2184 } 2185 return getInput().get(0); 2186 } 2187 2188 /** 2189 * @return {@link #rule} (Transform Rule from source to target.) 2190 */ 2191 public List<StructureMapGroupRuleComponent> getRule() { 2192 if (this.rule == null) 2193 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2194 return this.rule; 2195 } 2196 2197 /** 2198 * @return Returns a reference to <code>this</code> for easy method chaining 2199 */ 2200 public StructureMapGroupComponent setRule(List<StructureMapGroupRuleComponent> theRule) { 2201 this.rule = theRule; 2202 return this; 2203 } 2204 2205 public boolean hasRule() { 2206 if (this.rule == null) 2207 return false; 2208 for (StructureMapGroupRuleComponent item : this.rule) 2209 if (!item.isEmpty()) 2210 return true; 2211 return false; 2212 } 2213 2214 public StructureMapGroupRuleComponent addRule() { // 3 2215 StructureMapGroupRuleComponent t = new StructureMapGroupRuleComponent(); 2216 if (this.rule == null) 2217 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2218 this.rule.add(t); 2219 return t; 2220 } 2221 2222 public StructureMapGroupComponent addRule(StructureMapGroupRuleComponent t) { // 3 2223 if (t == null) 2224 return this; 2225 if (this.rule == null) 2226 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2227 this.rule.add(t); 2228 return this; 2229 } 2230 2231 /** 2232 * @return The first repetition of repeating field {@link #rule}, creating it if 2233 * it does not already exist 2234 */ 2235 public StructureMapGroupRuleComponent getRuleFirstRep() { 2236 if (getRule().isEmpty()) { 2237 addRule(); 2238 } 2239 return getRule().get(0); 2240 } 2241 2242 protected void listChildren(List<Property> children) { 2243 super.listChildren(children); 2244 children.add( 2245 new Property("name", "id", "A unique name for the group for the convenience of human readers.", 0, 1, name)); 2246 children.add(new Property("extends", "id", "Another group that this group adds rules to.", 0, 1, extends_)); 2247 children.add(new Property("typeMode", "code", 2248 "If this is the default rule set to apply for the source type or this combination of types.", 0, 1, 2249 typeMode)); 2250 children.add(new Property("documentation", "string", 2251 "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.", 2252 0, 1, documentation)); 2253 children.add(new Property("input", "", 2254 "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.", 0, 2255 java.lang.Integer.MAX_VALUE, input)); 2256 children 2257 .add(new Property("rule", "", "Transform Rule from source to target.", 0, java.lang.Integer.MAX_VALUE, rule)); 2258 } 2259 2260 @Override 2261 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2262 switch (_hash) { 2263 case 3373707: 2264 /* name */ return new Property("name", "id", 2265 "A unique name for the group for the convenience of human readers.", 0, 1, name); 2266 case -1305664359: 2267 /* extends */ return new Property("extends", "id", "Another group that this group adds rules to.", 0, 1, 2268 extends_); 2269 case -676524035: 2270 /* typeMode */ return new Property("typeMode", "code", 2271 "If this is the default rule set to apply for the source type or this combination of types.", 0, 1, 2272 typeMode); 2273 case 1587405498: 2274 /* documentation */ return new Property("documentation", "string", 2275 "Additional supporting documentation that explains the purpose of the group and the types of mappings within it.", 2276 0, 1, documentation); 2277 case 100358090: 2278 /* input */ return new Property("input", "", 2279 "A name assigned to an instance of data. The instance must be provided when the mapping is invoked.", 0, 2280 java.lang.Integer.MAX_VALUE, input); 2281 case 3512060: 2282 /* rule */ return new Property("rule", "", "Transform Rule from source to target.", 0, 2283 java.lang.Integer.MAX_VALUE, rule); 2284 default: 2285 return super.getNamedProperty(_hash, _name, _checkValid); 2286 } 2287 2288 } 2289 2290 @Override 2291 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2292 switch (hash) { 2293 case 3373707: 2294 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // IdType 2295 case -1305664359: 2296 /* extends */ return this.extends_ == null ? new Base[0] : new Base[] { this.extends_ }; // IdType 2297 case -676524035: 2298 /* typeMode */ return this.typeMode == null ? new Base[0] : new Base[] { this.typeMode }; // Enumeration<StructureMapGroupTypeMode> 2299 case 1587405498: 2300 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 2301 case 100358090: 2302 /* input */ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // StructureMapGroupInputComponent 2303 case 3512060: 2304 /* rule */ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // StructureMapGroupRuleComponent 2305 default: 2306 return super.getProperty(hash, name, checkValid); 2307 } 2308 2309 } 2310 2311 @Override 2312 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2313 switch (hash) { 2314 case 3373707: // name 2315 this.name = castToId(value); // IdType 2316 return value; 2317 case -1305664359: // extends 2318 this.extends_ = castToId(value); // IdType 2319 return value; 2320 case -676524035: // typeMode 2321 value = new StructureMapGroupTypeModeEnumFactory().fromType(castToCode(value)); 2322 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 2323 return value; 2324 case 1587405498: // documentation 2325 this.documentation = castToString(value); // StringType 2326 return value; 2327 case 100358090: // input 2328 this.getInput().add((StructureMapGroupInputComponent) value); // StructureMapGroupInputComponent 2329 return value; 2330 case 3512060: // rule 2331 this.getRule().add((StructureMapGroupRuleComponent) value); // StructureMapGroupRuleComponent 2332 return value; 2333 default: 2334 return super.setProperty(hash, name, value); 2335 } 2336 2337 } 2338 2339 @Override 2340 public Base setProperty(String name, Base value) throws FHIRException { 2341 if (name.equals("name")) { 2342 this.name = castToId(value); // IdType 2343 } else if (name.equals("extends")) { 2344 this.extends_ = castToId(value); // IdType 2345 } else if (name.equals("typeMode")) { 2346 value = new StructureMapGroupTypeModeEnumFactory().fromType(castToCode(value)); 2347 this.typeMode = (Enumeration) value; // Enumeration<StructureMapGroupTypeMode> 2348 } else if (name.equals("documentation")) { 2349 this.documentation = castToString(value); // StringType 2350 } else if (name.equals("input")) { 2351 this.getInput().add((StructureMapGroupInputComponent) value); 2352 } else if (name.equals("rule")) { 2353 this.getRule().add((StructureMapGroupRuleComponent) value); 2354 } else 2355 return super.setProperty(name, value); 2356 return value; 2357 } 2358 2359 @Override 2360 public void removeChild(String name, Base value) throws FHIRException { 2361 if (name.equals("name")) { 2362 this.name = null; 2363 } else if (name.equals("extends")) { 2364 this.extends_ = null; 2365 } else if (name.equals("typeMode")) { 2366 this.typeMode = null; 2367 } else if (name.equals("documentation")) { 2368 this.documentation = null; 2369 } else if (name.equals("input")) { 2370 this.getInput().remove((StructureMapGroupInputComponent) value); 2371 } else if (name.equals("rule")) { 2372 this.getRule().remove((StructureMapGroupRuleComponent) value); 2373 } else 2374 super.removeChild(name, value); 2375 2376 } 2377 2378 @Override 2379 public Base makeProperty(int hash, String name) throws FHIRException { 2380 switch (hash) { 2381 case 3373707: 2382 return getNameElement(); 2383 case -1305664359: 2384 return getExtendsElement(); 2385 case -676524035: 2386 return getTypeModeElement(); 2387 case 1587405498: 2388 return getDocumentationElement(); 2389 case 100358090: 2390 return addInput(); 2391 case 3512060: 2392 return addRule(); 2393 default: 2394 return super.makeProperty(hash, name); 2395 } 2396 2397 } 2398 2399 @Override 2400 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2401 switch (hash) { 2402 case 3373707: 2403 /* name */ return new String[] { "id" }; 2404 case -1305664359: 2405 /* extends */ return new String[] { "id" }; 2406 case -676524035: 2407 /* typeMode */ return new String[] { "code" }; 2408 case 1587405498: 2409 /* documentation */ return new String[] { "string" }; 2410 case 100358090: 2411 /* input */ return new String[] {}; 2412 case 3512060: 2413 /* rule */ return new String[] {}; 2414 default: 2415 return super.getTypesForProperty(hash, name); 2416 } 2417 2418 } 2419 2420 @Override 2421 public Base addChild(String name) throws FHIRException { 2422 if (name.equals("name")) { 2423 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 2424 } else if (name.equals("extends")) { 2425 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.extends"); 2426 } else if (name.equals("typeMode")) { 2427 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.typeMode"); 2428 } else if (name.equals("documentation")) { 2429 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.documentation"); 2430 } else if (name.equals("input")) { 2431 return addInput(); 2432 } else if (name.equals("rule")) { 2433 return addRule(); 2434 } else 2435 return super.addChild(name); 2436 } 2437 2438 public StructureMapGroupComponent copy() { 2439 StructureMapGroupComponent dst = new StructureMapGroupComponent(); 2440 copyValues(dst); 2441 return dst; 2442 } 2443 2444 public void copyValues(StructureMapGroupComponent dst) { 2445 super.copyValues(dst); 2446 dst.name = name == null ? null : name.copy(); 2447 dst.extends_ = extends_ == null ? null : extends_.copy(); 2448 dst.typeMode = typeMode == null ? null : typeMode.copy(); 2449 dst.documentation = documentation == null ? null : documentation.copy(); 2450 if (input != null) { 2451 dst.input = new ArrayList<StructureMapGroupInputComponent>(); 2452 for (StructureMapGroupInputComponent i : input) 2453 dst.input.add(i.copy()); 2454 } 2455 ; 2456 if (rule != null) { 2457 dst.rule = new ArrayList<StructureMapGroupRuleComponent>(); 2458 for (StructureMapGroupRuleComponent i : rule) 2459 dst.rule.add(i.copy()); 2460 } 2461 ; 2462 } 2463 2464 @Override 2465 public boolean equalsDeep(Base other_) { 2466 if (!super.equalsDeep(other_)) 2467 return false; 2468 if (!(other_ instanceof StructureMapGroupComponent)) 2469 return false; 2470 StructureMapGroupComponent o = (StructureMapGroupComponent) other_; 2471 return compareDeep(name, o.name, true) && compareDeep(extends_, o.extends_, true) 2472 && compareDeep(typeMode, o.typeMode, true) && compareDeep(documentation, o.documentation, true) 2473 && compareDeep(input, o.input, true) && compareDeep(rule, o.rule, true); 2474 } 2475 2476 @Override 2477 public boolean equalsShallow(Base other_) { 2478 if (!super.equalsShallow(other_)) 2479 return false; 2480 if (!(other_ instanceof StructureMapGroupComponent)) 2481 return false; 2482 StructureMapGroupComponent o = (StructureMapGroupComponent) other_; 2483 return compareValues(name, o.name, true) && compareValues(extends_, o.extends_, true) 2484 && compareValues(typeMode, o.typeMode, true) && compareValues(documentation, o.documentation, true); 2485 } 2486 2487 public boolean isEmpty() { 2488 return super.isEmpty() 2489 && ca.uhn.fhir.util.ElementUtil.isEmpty(name, extends_, typeMode, documentation, input, rule); 2490 } 2491 2492 public String fhirType() { 2493 return "StructureMap.group"; 2494 2495 } 2496 2497// added from java-adornments.txt: 2498 2499 public String toString() { 2500 return StructureMapUtilities.groupToString(this); 2501 } 2502 2503// end addition 2504 } 2505 2506 @Block() 2507 public static class StructureMapGroupInputComponent extends BackboneElement implements IBaseBackboneElement { 2508 /** 2509 * Name for this instance of data. 2510 */ 2511 @Child(name = "name", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2512 @Description(shortDefinition = "Name for this instance of data", formalDefinition = "Name for this instance of data.") 2513 protected IdType name; 2514 2515 /** 2516 * Type for this instance of data. 2517 */ 2518 @Child(name = "type", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2519 @Description(shortDefinition = "Type for this instance of data", formalDefinition = "Type for this instance of data.") 2520 protected StringType type; 2521 2522 /** 2523 * Mode for this instance of data. 2524 */ 2525 @Child(name = "mode", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2526 @Description(shortDefinition = "source | target", formalDefinition = "Mode for this instance of data.") 2527 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/map-input-mode") 2528 protected Enumeration<StructureMapInputMode> mode; 2529 2530 /** 2531 * Documentation for this instance of data. 2532 */ 2533 @Child(name = "documentation", type = { 2534 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2535 @Description(shortDefinition = "Documentation for this instance of data", formalDefinition = "Documentation for this instance of data.") 2536 protected StringType documentation; 2537 2538 private static final long serialVersionUID = -25050724L; 2539 2540 /** 2541 * Constructor 2542 */ 2543 public StructureMapGroupInputComponent() { 2544 super(); 2545 } 2546 2547 /** 2548 * Constructor 2549 */ 2550 public StructureMapGroupInputComponent(IdType name, Enumeration<StructureMapInputMode> mode) { 2551 super(); 2552 this.name = name; 2553 this.mode = mode; 2554 } 2555 2556 /** 2557 * @return {@link #name} (Name for this instance of data.). This is the 2558 * underlying object with id, value and extensions. The accessor 2559 * "getName" gives direct access to the value 2560 */ 2561 public IdType getNameElement() { 2562 if (this.name == null) 2563 if (Configuration.errorOnAutoCreate()) 2564 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.name"); 2565 else if (Configuration.doAutoCreate()) 2566 this.name = new IdType(); // bb 2567 return this.name; 2568 } 2569 2570 public boolean hasNameElement() { 2571 return this.name != null && !this.name.isEmpty(); 2572 } 2573 2574 public boolean hasName() { 2575 return this.name != null && !this.name.isEmpty(); 2576 } 2577 2578 /** 2579 * @param value {@link #name} (Name for this instance of data.). This is the 2580 * underlying object with id, value and extensions. The accessor 2581 * "getName" gives direct access to the value 2582 */ 2583 public StructureMapGroupInputComponent setNameElement(IdType value) { 2584 this.name = value; 2585 return this; 2586 } 2587 2588 /** 2589 * @return Name for this instance of data. 2590 */ 2591 public String getName() { 2592 return this.name == null ? null : this.name.getValue(); 2593 } 2594 2595 /** 2596 * @param value Name for this instance of data. 2597 */ 2598 public StructureMapGroupInputComponent setName(String value) { 2599 if (this.name == null) 2600 this.name = new IdType(); 2601 this.name.setValue(value); 2602 return this; 2603 } 2604 2605 /** 2606 * @return {@link #type} (Type for this instance of data.). This is the 2607 * underlying object with id, value and extensions. The accessor 2608 * "getType" gives direct access to the value 2609 */ 2610 public StringType getTypeElement() { 2611 if (this.type == null) 2612 if (Configuration.errorOnAutoCreate()) 2613 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.type"); 2614 else if (Configuration.doAutoCreate()) 2615 this.type = new StringType(); // bb 2616 return this.type; 2617 } 2618 2619 public boolean hasTypeElement() { 2620 return this.type != null && !this.type.isEmpty(); 2621 } 2622 2623 public boolean hasType() { 2624 return this.type != null && !this.type.isEmpty(); 2625 } 2626 2627 /** 2628 * @param value {@link #type} (Type for this instance of data.). This is the 2629 * underlying object with id, value and extensions. The accessor 2630 * "getType" gives direct access to the value 2631 */ 2632 public StructureMapGroupInputComponent setTypeElement(StringType value) { 2633 this.type = value; 2634 return this; 2635 } 2636 2637 /** 2638 * @return Type for this instance of data. 2639 */ 2640 public String getType() { 2641 return this.type == null ? null : this.type.getValue(); 2642 } 2643 2644 /** 2645 * @param value Type for this instance of data. 2646 */ 2647 public StructureMapGroupInputComponent setType(String value) { 2648 if (Utilities.noString(value)) 2649 this.type = null; 2650 else { 2651 if (this.type == null) 2652 this.type = new StringType(); 2653 this.type.setValue(value); 2654 } 2655 return this; 2656 } 2657 2658 /** 2659 * @return {@link #mode} (Mode for this instance of data.). This is the 2660 * underlying object with id, value and extensions. The accessor 2661 * "getMode" gives direct access to the value 2662 */ 2663 public Enumeration<StructureMapInputMode> getModeElement() { 2664 if (this.mode == null) 2665 if (Configuration.errorOnAutoCreate()) 2666 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.mode"); 2667 else if (Configuration.doAutoCreate()) 2668 this.mode = new Enumeration<StructureMapInputMode>(new StructureMapInputModeEnumFactory()); // bb 2669 return this.mode; 2670 } 2671 2672 public boolean hasModeElement() { 2673 return this.mode != null && !this.mode.isEmpty(); 2674 } 2675 2676 public boolean hasMode() { 2677 return this.mode != null && !this.mode.isEmpty(); 2678 } 2679 2680 /** 2681 * @param value {@link #mode} (Mode for this instance of data.). This is the 2682 * underlying object with id, value and extensions. The accessor 2683 * "getMode" gives direct access to the value 2684 */ 2685 public StructureMapGroupInputComponent setModeElement(Enumeration<StructureMapInputMode> value) { 2686 this.mode = value; 2687 return this; 2688 } 2689 2690 /** 2691 * @return Mode for this instance of data. 2692 */ 2693 public StructureMapInputMode getMode() { 2694 return this.mode == null ? null : this.mode.getValue(); 2695 } 2696 2697 /** 2698 * @param value Mode for this instance of data. 2699 */ 2700 public StructureMapGroupInputComponent setMode(StructureMapInputMode value) { 2701 if (this.mode == null) 2702 this.mode = new Enumeration<StructureMapInputMode>(new StructureMapInputModeEnumFactory()); 2703 this.mode.setValue(value); 2704 return this; 2705 } 2706 2707 /** 2708 * @return {@link #documentation} (Documentation for this instance of data.). 2709 * This is the underlying object with id, value and extensions. The 2710 * accessor "getDocumentation" gives direct access to the value 2711 */ 2712 public StringType getDocumentationElement() { 2713 if (this.documentation == null) 2714 if (Configuration.errorOnAutoCreate()) 2715 throw new Error("Attempt to auto-create StructureMapGroupInputComponent.documentation"); 2716 else if (Configuration.doAutoCreate()) 2717 this.documentation = new StringType(); // bb 2718 return this.documentation; 2719 } 2720 2721 public boolean hasDocumentationElement() { 2722 return this.documentation != null && !this.documentation.isEmpty(); 2723 } 2724 2725 public boolean hasDocumentation() { 2726 return this.documentation != null && !this.documentation.isEmpty(); 2727 } 2728 2729 /** 2730 * @param value {@link #documentation} (Documentation for this instance of 2731 * data.). This is the underlying object with id, value and 2732 * extensions. The accessor "getDocumentation" gives direct access 2733 * to the value 2734 */ 2735 public StructureMapGroupInputComponent setDocumentationElement(StringType value) { 2736 this.documentation = value; 2737 return this; 2738 } 2739 2740 /** 2741 * @return Documentation for this instance of data. 2742 */ 2743 public String getDocumentation() { 2744 return this.documentation == null ? null : this.documentation.getValue(); 2745 } 2746 2747 /** 2748 * @param value Documentation for this instance of data. 2749 */ 2750 public StructureMapGroupInputComponent setDocumentation(String value) { 2751 if (Utilities.noString(value)) 2752 this.documentation = null; 2753 else { 2754 if (this.documentation == null) 2755 this.documentation = new StringType(); 2756 this.documentation.setValue(value); 2757 } 2758 return this; 2759 } 2760 2761 protected void listChildren(List<Property> children) { 2762 super.listChildren(children); 2763 children.add(new Property("name", "id", "Name for this instance of data.", 0, 1, name)); 2764 children.add(new Property("type", "string", "Type for this instance of data.", 0, 1, type)); 2765 children.add(new Property("mode", "code", "Mode for this instance of data.", 0, 1, mode)); 2766 children.add( 2767 new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation)); 2768 } 2769 2770 @Override 2771 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2772 switch (_hash) { 2773 case 3373707: 2774 /* name */ return new Property("name", "id", "Name for this instance of data.", 0, 1, name); 2775 case 3575610: 2776 /* type */ return new Property("type", "string", "Type for this instance of data.", 0, 1, type); 2777 case 3357091: 2778 /* mode */ return new Property("mode", "code", "Mode for this instance of data.", 0, 1, mode); 2779 case 1587405498: 2780 /* documentation */ return new Property("documentation", "string", "Documentation for this instance of data.", 2781 0, 1, documentation); 2782 default: 2783 return super.getNamedProperty(_hash, _name, _checkValid); 2784 } 2785 2786 } 2787 2788 @Override 2789 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2790 switch (hash) { 2791 case 3373707: 2792 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // IdType 2793 case 3575610: 2794 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // StringType 2795 case 3357091: 2796 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<StructureMapInputMode> 2797 case 1587405498: 2798 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 2799 default: 2800 return super.getProperty(hash, name, checkValid); 2801 } 2802 2803 } 2804 2805 @Override 2806 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2807 switch (hash) { 2808 case 3373707: // name 2809 this.name = castToId(value); // IdType 2810 return value; 2811 case 3575610: // type 2812 this.type = castToString(value); // StringType 2813 return value; 2814 case 3357091: // mode 2815 value = new StructureMapInputModeEnumFactory().fromType(castToCode(value)); 2816 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2817 return value; 2818 case 1587405498: // documentation 2819 this.documentation = castToString(value); // StringType 2820 return value; 2821 default: 2822 return super.setProperty(hash, name, value); 2823 } 2824 2825 } 2826 2827 @Override 2828 public Base setProperty(String name, Base value) throws FHIRException { 2829 if (name.equals("name")) { 2830 this.name = castToId(value); // IdType 2831 } else if (name.equals("type")) { 2832 this.type = castToString(value); // StringType 2833 } else if (name.equals("mode")) { 2834 value = new StructureMapInputModeEnumFactory().fromType(castToCode(value)); 2835 this.mode = (Enumeration) value; // Enumeration<StructureMapInputMode> 2836 } else if (name.equals("documentation")) { 2837 this.documentation = castToString(value); // StringType 2838 } else 2839 return super.setProperty(name, value); 2840 return value; 2841 } 2842 2843 @Override 2844 public void removeChild(String name, Base value) throws FHIRException { 2845 if (name.equals("name")) { 2846 this.name = null; 2847 } else if (name.equals("type")) { 2848 this.type = null; 2849 } else if (name.equals("mode")) { 2850 this.mode = null; 2851 } else if (name.equals("documentation")) { 2852 this.documentation = null; 2853 } else 2854 super.removeChild(name, value); 2855 2856 } 2857 2858 @Override 2859 public Base makeProperty(int hash, String name) throws FHIRException { 2860 switch (hash) { 2861 case 3373707: 2862 return getNameElement(); 2863 case 3575610: 2864 return getTypeElement(); 2865 case 3357091: 2866 return getModeElement(); 2867 case 1587405498: 2868 return getDocumentationElement(); 2869 default: 2870 return super.makeProperty(hash, name); 2871 } 2872 2873 } 2874 2875 @Override 2876 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2877 switch (hash) { 2878 case 3373707: 2879 /* name */ return new String[] { "id" }; 2880 case 3575610: 2881 /* type */ return new String[] { "string" }; 2882 case 3357091: 2883 /* mode */ return new String[] { "code" }; 2884 case 1587405498: 2885 /* documentation */ return new String[] { "string" }; 2886 default: 2887 return super.getTypesForProperty(hash, name); 2888 } 2889 2890 } 2891 2892 @Override 2893 public Base addChild(String name) throws FHIRException { 2894 if (name.equals("name")) { 2895 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 2896 } else if (name.equals("type")) { 2897 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.type"); 2898 } else if (name.equals("mode")) { 2899 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.mode"); 2900 } else if (name.equals("documentation")) { 2901 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.documentation"); 2902 } else 2903 return super.addChild(name); 2904 } 2905 2906 public StructureMapGroupInputComponent copy() { 2907 StructureMapGroupInputComponent dst = new StructureMapGroupInputComponent(); 2908 copyValues(dst); 2909 return dst; 2910 } 2911 2912 public void copyValues(StructureMapGroupInputComponent dst) { 2913 super.copyValues(dst); 2914 dst.name = name == null ? null : name.copy(); 2915 dst.type = type == null ? null : type.copy(); 2916 dst.mode = mode == null ? null : mode.copy(); 2917 dst.documentation = documentation == null ? null : documentation.copy(); 2918 } 2919 2920 @Override 2921 public boolean equalsDeep(Base other_) { 2922 if (!super.equalsDeep(other_)) 2923 return false; 2924 if (!(other_ instanceof StructureMapGroupInputComponent)) 2925 return false; 2926 StructureMapGroupInputComponent o = (StructureMapGroupInputComponent) other_; 2927 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(mode, o.mode, true) 2928 && compareDeep(documentation, o.documentation, true); 2929 } 2930 2931 @Override 2932 public boolean equalsShallow(Base other_) { 2933 if (!super.equalsShallow(other_)) 2934 return false; 2935 if (!(other_ instanceof StructureMapGroupInputComponent)) 2936 return false; 2937 StructureMapGroupInputComponent o = (StructureMapGroupInputComponent) other_; 2938 return compareValues(name, o.name, true) && compareValues(type, o.type, true) && compareValues(mode, o.mode, true) 2939 && compareValues(documentation, o.documentation, true); 2940 } 2941 2942 public boolean isEmpty() { 2943 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, mode, documentation); 2944 } 2945 2946 public String fhirType() { 2947 return "StructureMap.group.input"; 2948 2949 } 2950 2951 } 2952 2953 @Block() 2954 public static class StructureMapGroupRuleComponent extends BackboneElement implements IBaseBackboneElement { 2955 /** 2956 * Name of the rule for internal references. 2957 */ 2958 @Child(name = "name", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2959 @Description(shortDefinition = "Name of the rule for internal references", formalDefinition = "Name of the rule for internal references.") 2960 protected IdType name; 2961 2962 /** 2963 * Source inputs to the mapping. 2964 */ 2965 @Child(name = "source", type = {}, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2966 @Description(shortDefinition = "Source inputs to the mapping", formalDefinition = "Source inputs to the mapping.") 2967 protected List<StructureMapGroupRuleSourceComponent> source; 2968 2969 /** 2970 * Content to create because of this mapping rule. 2971 */ 2972 @Child(name = "target", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2973 @Description(shortDefinition = "Content to create because of this mapping rule", formalDefinition = "Content to create because of this mapping rule.") 2974 protected List<StructureMapGroupRuleTargetComponent> target; 2975 2976 /** 2977 * Rules contained in this rule. 2978 */ 2979 @Child(name = "rule", type = { 2980 StructureMapGroupRuleComponent.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2981 @Description(shortDefinition = "Rules contained in this rule", formalDefinition = "Rules contained in this rule.") 2982 protected List<StructureMapGroupRuleComponent> rule; 2983 2984 /** 2985 * Which other rules to apply in the context of this rule. 2986 */ 2987 @Child(name = "dependent", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2988 @Description(shortDefinition = "Which other rules to apply in the context of this rule", formalDefinition = "Which other rules to apply in the context of this rule.") 2989 protected List<StructureMapGroupRuleDependentComponent> dependent; 2990 2991 /** 2992 * Documentation for this instance of data. 2993 */ 2994 @Child(name = "documentation", type = { 2995 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2996 @Description(shortDefinition = "Documentation for this instance of data", formalDefinition = "Documentation for this instance of data.") 2997 protected StringType documentation; 2998 2999 private static final long serialVersionUID = 773925517L; 3000 3001 /** 3002 * Constructor 3003 */ 3004 public StructureMapGroupRuleComponent() { 3005 super(); 3006 } 3007 3008 /** 3009 * Constructor 3010 */ 3011 public StructureMapGroupRuleComponent(IdType name) { 3012 super(); 3013 this.name = name; 3014 } 3015 3016 /** 3017 * @return {@link #name} (Name of the rule for internal references.). This is 3018 * the underlying object with id, value and extensions. The accessor 3019 * "getName" gives direct access to the value 3020 */ 3021 public IdType getNameElement() { 3022 if (this.name == null) 3023 if (Configuration.errorOnAutoCreate()) 3024 throw new Error("Attempt to auto-create StructureMapGroupRuleComponent.name"); 3025 else if (Configuration.doAutoCreate()) 3026 this.name = new IdType(); // bb 3027 return this.name; 3028 } 3029 3030 public boolean hasNameElement() { 3031 return this.name != null && !this.name.isEmpty(); 3032 } 3033 3034 public boolean hasName() { 3035 return this.name != null && !this.name.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #name} (Name of the rule for internal references.). This 3040 * is the underlying object with id, value and extensions. The 3041 * accessor "getName" gives direct access to the value 3042 */ 3043 public StructureMapGroupRuleComponent setNameElement(IdType value) { 3044 this.name = value; 3045 return this; 3046 } 3047 3048 /** 3049 * @return Name of the rule for internal references. 3050 */ 3051 public String getName() { 3052 return this.name == null ? null : this.name.getValue(); 3053 } 3054 3055 /** 3056 * @param value Name of the rule for internal references. 3057 */ 3058 public StructureMapGroupRuleComponent setName(String value) { 3059 if (this.name == null) 3060 this.name = new IdType(); 3061 this.name.setValue(value); 3062 return this; 3063 } 3064 3065 /** 3066 * @return {@link #source} (Source inputs to the mapping.) 3067 */ 3068 public List<StructureMapGroupRuleSourceComponent> getSource() { 3069 if (this.source == null) 3070 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 3071 return this.source; 3072 } 3073 3074 /** 3075 * @return Returns a reference to <code>this</code> for easy method chaining 3076 */ 3077 public StructureMapGroupRuleComponent setSource(List<StructureMapGroupRuleSourceComponent> theSource) { 3078 this.source = theSource; 3079 return this; 3080 } 3081 3082 public boolean hasSource() { 3083 if (this.source == null) 3084 return false; 3085 for (StructureMapGroupRuleSourceComponent item : this.source) 3086 if (!item.isEmpty()) 3087 return true; 3088 return false; 3089 } 3090 3091 public StructureMapGroupRuleSourceComponent addSource() { // 3 3092 StructureMapGroupRuleSourceComponent t = new StructureMapGroupRuleSourceComponent(); 3093 if (this.source == null) 3094 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 3095 this.source.add(t); 3096 return t; 3097 } 3098 3099 public StructureMapGroupRuleComponent addSource(StructureMapGroupRuleSourceComponent t) { // 3 3100 if (t == null) 3101 return this; 3102 if (this.source == null) 3103 this.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 3104 this.source.add(t); 3105 return this; 3106 } 3107 3108 /** 3109 * @return The first repetition of repeating field {@link #source}, creating it 3110 * if it does not already exist 3111 */ 3112 public StructureMapGroupRuleSourceComponent getSourceFirstRep() { 3113 if (getSource().isEmpty()) { 3114 addSource(); 3115 } 3116 return getSource().get(0); 3117 } 3118 3119 /** 3120 * @return {@link #target} (Content to create because of this mapping rule.) 3121 */ 3122 public List<StructureMapGroupRuleTargetComponent> getTarget() { 3123 if (this.target == null) 3124 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 3125 return this.target; 3126 } 3127 3128 /** 3129 * @return Returns a reference to <code>this</code> for easy method chaining 3130 */ 3131 public StructureMapGroupRuleComponent setTarget(List<StructureMapGroupRuleTargetComponent> theTarget) { 3132 this.target = theTarget; 3133 return this; 3134 } 3135 3136 public boolean hasTarget() { 3137 if (this.target == null) 3138 return false; 3139 for (StructureMapGroupRuleTargetComponent item : this.target) 3140 if (!item.isEmpty()) 3141 return true; 3142 return false; 3143 } 3144 3145 public StructureMapGroupRuleTargetComponent addTarget() { // 3 3146 StructureMapGroupRuleTargetComponent t = new StructureMapGroupRuleTargetComponent(); 3147 if (this.target == null) 3148 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 3149 this.target.add(t); 3150 return t; 3151 } 3152 3153 public StructureMapGroupRuleComponent addTarget(StructureMapGroupRuleTargetComponent t) { // 3 3154 if (t == null) 3155 return this; 3156 if (this.target == null) 3157 this.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 3158 this.target.add(t); 3159 return this; 3160 } 3161 3162 /** 3163 * @return The first repetition of repeating field {@link #target}, creating it 3164 * if it does not already exist 3165 */ 3166 public StructureMapGroupRuleTargetComponent getTargetFirstRep() { 3167 if (getTarget().isEmpty()) { 3168 addTarget(); 3169 } 3170 return getTarget().get(0); 3171 } 3172 3173 /** 3174 * @return {@link #rule} (Rules contained in this rule.) 3175 */ 3176 public List<StructureMapGroupRuleComponent> getRule() { 3177 if (this.rule == null) 3178 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 3179 return this.rule; 3180 } 3181 3182 /** 3183 * @return Returns a reference to <code>this</code> for easy method chaining 3184 */ 3185 public StructureMapGroupRuleComponent setRule(List<StructureMapGroupRuleComponent> theRule) { 3186 this.rule = theRule; 3187 return this; 3188 } 3189 3190 public boolean hasRule() { 3191 if (this.rule == null) 3192 return false; 3193 for (StructureMapGroupRuleComponent item : this.rule) 3194 if (!item.isEmpty()) 3195 return true; 3196 return false; 3197 } 3198 3199 public StructureMapGroupRuleComponent addRule() { // 3 3200 StructureMapGroupRuleComponent t = new StructureMapGroupRuleComponent(); 3201 if (this.rule == null) 3202 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 3203 this.rule.add(t); 3204 return t; 3205 } 3206 3207 public StructureMapGroupRuleComponent addRule(StructureMapGroupRuleComponent t) { // 3 3208 if (t == null) 3209 return this; 3210 if (this.rule == null) 3211 this.rule = new ArrayList<StructureMapGroupRuleComponent>(); 3212 this.rule.add(t); 3213 return this; 3214 } 3215 3216 /** 3217 * @return The first repetition of repeating field {@link #rule}, creating it if 3218 * it does not already exist 3219 */ 3220 public StructureMapGroupRuleComponent getRuleFirstRep() { 3221 if (getRule().isEmpty()) { 3222 addRule(); 3223 } 3224 return getRule().get(0); 3225 } 3226 3227 /** 3228 * @return {@link #dependent} (Which other rules to apply in the context of this 3229 * rule.) 3230 */ 3231 public List<StructureMapGroupRuleDependentComponent> getDependent() { 3232 if (this.dependent == null) 3233 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 3234 return this.dependent; 3235 } 3236 3237 /** 3238 * @return Returns a reference to <code>this</code> for easy method chaining 3239 */ 3240 public StructureMapGroupRuleComponent setDependent(List<StructureMapGroupRuleDependentComponent> theDependent) { 3241 this.dependent = theDependent; 3242 return this; 3243 } 3244 3245 public boolean hasDependent() { 3246 if (this.dependent == null) 3247 return false; 3248 for (StructureMapGroupRuleDependentComponent item : this.dependent) 3249 if (!item.isEmpty()) 3250 return true; 3251 return false; 3252 } 3253 3254 public StructureMapGroupRuleDependentComponent addDependent() { // 3 3255 StructureMapGroupRuleDependentComponent t = new StructureMapGroupRuleDependentComponent(); 3256 if (this.dependent == null) 3257 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 3258 this.dependent.add(t); 3259 return t; 3260 } 3261 3262 public StructureMapGroupRuleComponent addDependent(StructureMapGroupRuleDependentComponent t) { // 3 3263 if (t == null) 3264 return this; 3265 if (this.dependent == null) 3266 this.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 3267 this.dependent.add(t); 3268 return this; 3269 } 3270 3271 /** 3272 * @return The first repetition of repeating field {@link #dependent}, creating 3273 * it if it does not already exist 3274 */ 3275 public StructureMapGroupRuleDependentComponent getDependentFirstRep() { 3276 if (getDependent().isEmpty()) { 3277 addDependent(); 3278 } 3279 return getDependent().get(0); 3280 } 3281 3282 /** 3283 * @return {@link #documentation} (Documentation for this instance of data.). 3284 * This is the underlying object with id, value and extensions. The 3285 * accessor "getDocumentation" gives direct access to the value 3286 */ 3287 public StringType getDocumentationElement() { 3288 if (this.documentation == null) 3289 if (Configuration.errorOnAutoCreate()) 3290 throw new Error("Attempt to auto-create StructureMapGroupRuleComponent.documentation"); 3291 else if (Configuration.doAutoCreate()) 3292 this.documentation = new StringType(); // bb 3293 return this.documentation; 3294 } 3295 3296 public boolean hasDocumentationElement() { 3297 return this.documentation != null && !this.documentation.isEmpty(); 3298 } 3299 3300 public boolean hasDocumentation() { 3301 return this.documentation != null && !this.documentation.isEmpty(); 3302 } 3303 3304 /** 3305 * @param value {@link #documentation} (Documentation for this instance of 3306 * data.). This is the underlying object with id, value and 3307 * extensions. The accessor "getDocumentation" gives direct access 3308 * to the value 3309 */ 3310 public StructureMapGroupRuleComponent setDocumentationElement(StringType value) { 3311 this.documentation = value; 3312 return this; 3313 } 3314 3315 /** 3316 * @return Documentation for this instance of data. 3317 */ 3318 public String getDocumentation() { 3319 return this.documentation == null ? null : this.documentation.getValue(); 3320 } 3321 3322 /** 3323 * @param value Documentation for this instance of data. 3324 */ 3325 public StructureMapGroupRuleComponent setDocumentation(String value) { 3326 if (Utilities.noString(value)) 3327 this.documentation = null; 3328 else { 3329 if (this.documentation == null) 3330 this.documentation = new StringType(); 3331 this.documentation.setValue(value); 3332 } 3333 return this; 3334 } 3335 3336 protected void listChildren(List<Property> children) { 3337 super.listChildren(children); 3338 children.add(new Property("name", "id", "Name of the rule for internal references.", 0, 1, name)); 3339 children.add(new Property("source", "", "Source inputs to the mapping.", 0, java.lang.Integer.MAX_VALUE, source)); 3340 children.add(new Property("target", "", "Content to create because of this mapping rule.", 0, 3341 java.lang.Integer.MAX_VALUE, target)); 3342 children.add(new Property("rule", "@StructureMap.group.rule", "Rules contained in this rule.", 0, 3343 java.lang.Integer.MAX_VALUE, rule)); 3344 children.add(new Property("dependent", "", "Which other rules to apply in the context of this rule.", 0, 3345 java.lang.Integer.MAX_VALUE, dependent)); 3346 children.add( 3347 new Property("documentation", "string", "Documentation for this instance of data.", 0, 1, documentation)); 3348 } 3349 3350 @Override 3351 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3352 switch (_hash) { 3353 case 3373707: 3354 /* name */ return new Property("name", "id", "Name of the rule for internal references.", 0, 1, name); 3355 case -896505829: 3356 /* source */ return new Property("source", "", "Source inputs to the mapping.", 0, java.lang.Integer.MAX_VALUE, 3357 source); 3358 case -880905839: 3359 /* target */ return new Property("target", "", "Content to create because of this mapping rule.", 0, 3360 java.lang.Integer.MAX_VALUE, target); 3361 case 3512060: 3362 /* rule */ return new Property("rule", "@StructureMap.group.rule", "Rules contained in this rule.", 0, 3363 java.lang.Integer.MAX_VALUE, rule); 3364 case -1109226753: 3365 /* dependent */ return new Property("dependent", "", "Which other rules to apply in the context of this rule.", 3366 0, java.lang.Integer.MAX_VALUE, dependent); 3367 case 1587405498: 3368 /* documentation */ return new Property("documentation", "string", "Documentation for this instance of data.", 3369 0, 1, documentation); 3370 default: 3371 return super.getNamedProperty(_hash, _name, _checkValid); 3372 } 3373 3374 } 3375 3376 @Override 3377 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3378 switch (hash) { 3379 case 3373707: 3380 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // IdType 3381 case -896505829: 3382 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // StructureMapGroupRuleSourceComponent 3383 case -880905839: 3384 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // StructureMapGroupRuleTargetComponent 3385 case 3512060: 3386 /* rule */ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // StructureMapGroupRuleComponent 3387 case -1109226753: 3388 /* dependent */ return this.dependent == null ? new Base[0] 3389 : this.dependent.toArray(new Base[this.dependent.size()]); // StructureMapGroupRuleDependentComponent 3390 case 1587405498: 3391 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 3392 default: 3393 return super.getProperty(hash, name, checkValid); 3394 } 3395 3396 } 3397 3398 @Override 3399 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3400 switch (hash) { 3401 case 3373707: // name 3402 this.name = castToId(value); // IdType 3403 return value; 3404 case -896505829: // source 3405 this.getSource().add((StructureMapGroupRuleSourceComponent) value); // StructureMapGroupRuleSourceComponent 3406 return value; 3407 case -880905839: // target 3408 this.getTarget().add((StructureMapGroupRuleTargetComponent) value); // StructureMapGroupRuleTargetComponent 3409 return value; 3410 case 3512060: // rule 3411 this.getRule().add((StructureMapGroupRuleComponent) value); // StructureMapGroupRuleComponent 3412 return value; 3413 case -1109226753: // dependent 3414 this.getDependent().add((StructureMapGroupRuleDependentComponent) value); // StructureMapGroupRuleDependentComponent 3415 return value; 3416 case 1587405498: // documentation 3417 this.documentation = castToString(value); // StringType 3418 return value; 3419 default: 3420 return super.setProperty(hash, name, value); 3421 } 3422 3423 } 3424 3425 @Override 3426 public Base setProperty(String name, Base value) throws FHIRException { 3427 if (name.equals("name")) { 3428 this.name = castToId(value); // IdType 3429 } else if (name.equals("source")) { 3430 this.getSource().add((StructureMapGroupRuleSourceComponent) value); 3431 } else if (name.equals("target")) { 3432 this.getTarget().add((StructureMapGroupRuleTargetComponent) value); 3433 } else if (name.equals("rule")) { 3434 this.getRule().add((StructureMapGroupRuleComponent) value); 3435 } else if (name.equals("dependent")) { 3436 this.getDependent().add((StructureMapGroupRuleDependentComponent) value); 3437 } else if (name.equals("documentation")) { 3438 this.documentation = castToString(value); // StringType 3439 } else 3440 return super.setProperty(name, value); 3441 return value; 3442 } 3443 3444 @Override 3445 public void removeChild(String name, Base value) throws FHIRException { 3446 if (name.equals("name")) { 3447 this.name = null; 3448 } else if (name.equals("source")) { 3449 this.getSource().remove((StructureMapGroupRuleSourceComponent) value); 3450 } else if (name.equals("target")) { 3451 this.getTarget().remove((StructureMapGroupRuleTargetComponent) value); 3452 } else if (name.equals("rule")) { 3453 this.getRule().remove((StructureMapGroupRuleComponent) value); 3454 } else if (name.equals("dependent")) { 3455 this.getDependent().remove((StructureMapGroupRuleDependentComponent) value); 3456 } else if (name.equals("documentation")) { 3457 this.documentation = null; 3458 } else 3459 super.removeChild(name, value); 3460 3461 } 3462 3463 @Override 3464 public Base makeProperty(int hash, String name) throws FHIRException { 3465 switch (hash) { 3466 case 3373707: 3467 return getNameElement(); 3468 case -896505829: 3469 return addSource(); 3470 case -880905839: 3471 return addTarget(); 3472 case 3512060: 3473 return addRule(); 3474 case -1109226753: 3475 return addDependent(); 3476 case 1587405498: 3477 return getDocumentationElement(); 3478 default: 3479 return super.makeProperty(hash, name); 3480 } 3481 3482 } 3483 3484 @Override 3485 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3486 switch (hash) { 3487 case 3373707: 3488 /* name */ return new String[] { "id" }; 3489 case -896505829: 3490 /* source */ return new String[] {}; 3491 case -880905839: 3492 /* target */ return new String[] {}; 3493 case 3512060: 3494 /* rule */ return new String[] { "@StructureMap.group.rule" }; 3495 case -1109226753: 3496 /* dependent */ return new String[] {}; 3497 case 1587405498: 3498 /* documentation */ return new String[] { "string" }; 3499 default: 3500 return super.getTypesForProperty(hash, name); 3501 } 3502 3503 } 3504 3505 @Override 3506 public Base addChild(String name) throws FHIRException { 3507 if (name.equals("name")) { 3508 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 3509 } else if (name.equals("source")) { 3510 return addSource(); 3511 } else if (name.equals("target")) { 3512 return addTarget(); 3513 } else if (name.equals("rule")) { 3514 return addRule(); 3515 } else if (name.equals("dependent")) { 3516 return addDependent(); 3517 } else if (name.equals("documentation")) { 3518 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.documentation"); 3519 } else 3520 return super.addChild(name); 3521 } 3522 3523 public StructureMapGroupRuleComponent copy() { 3524 StructureMapGroupRuleComponent dst = new StructureMapGroupRuleComponent(); 3525 copyValues(dst); 3526 return dst; 3527 } 3528 3529 public void copyValues(StructureMapGroupRuleComponent dst) { 3530 super.copyValues(dst); 3531 dst.name = name == null ? null : name.copy(); 3532 if (source != null) { 3533 dst.source = new ArrayList<StructureMapGroupRuleSourceComponent>(); 3534 for (StructureMapGroupRuleSourceComponent i : source) 3535 dst.source.add(i.copy()); 3536 } 3537 ; 3538 if (target != null) { 3539 dst.target = new ArrayList<StructureMapGroupRuleTargetComponent>(); 3540 for (StructureMapGroupRuleTargetComponent i : target) 3541 dst.target.add(i.copy()); 3542 } 3543 ; 3544 if (rule != null) { 3545 dst.rule = new ArrayList<StructureMapGroupRuleComponent>(); 3546 for (StructureMapGroupRuleComponent i : rule) 3547 dst.rule.add(i.copy()); 3548 } 3549 ; 3550 if (dependent != null) { 3551 dst.dependent = new ArrayList<StructureMapGroupRuleDependentComponent>(); 3552 for (StructureMapGroupRuleDependentComponent i : dependent) 3553 dst.dependent.add(i.copy()); 3554 } 3555 ; 3556 dst.documentation = documentation == null ? null : documentation.copy(); 3557 } 3558 3559 @Override 3560 public boolean equalsDeep(Base other_) { 3561 if (!super.equalsDeep(other_)) 3562 return false; 3563 if (!(other_ instanceof StructureMapGroupRuleComponent)) 3564 return false; 3565 StructureMapGroupRuleComponent o = (StructureMapGroupRuleComponent) other_; 3566 return compareDeep(name, o.name, true) && compareDeep(source, o.source, true) 3567 && compareDeep(target, o.target, true) && compareDeep(rule, o.rule, true) 3568 && compareDeep(dependent, o.dependent, true) && compareDeep(documentation, o.documentation, true); 3569 } 3570 3571 @Override 3572 public boolean equalsShallow(Base other_) { 3573 if (!super.equalsShallow(other_)) 3574 return false; 3575 if (!(other_ instanceof StructureMapGroupRuleComponent)) 3576 return false; 3577 StructureMapGroupRuleComponent o = (StructureMapGroupRuleComponent) other_; 3578 return compareValues(name, o.name, true) && compareValues(documentation, o.documentation, true); 3579 } 3580 3581 public boolean isEmpty() { 3582 return super.isEmpty() 3583 && ca.uhn.fhir.util.ElementUtil.isEmpty(name, source, target, rule, dependent, documentation); 3584 } 3585 3586 public String fhirType() { 3587 return "StructureMap.group.rule"; 3588 3589 } 3590 3591// added from java-adornments.txt: 3592 3593 public String toString() { 3594 return StructureMapUtilities.ruleToString(this); 3595 } 3596 3597// end addition 3598 } 3599 3600 @Block() 3601 public static class StructureMapGroupRuleSourceComponent extends BackboneElement implements IBaseBackboneElement { 3602 /** 3603 * Type or variable this rule applies to. 3604 */ 3605 @Child(name = "context", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3606 @Description(shortDefinition = "Type or variable this rule applies to", formalDefinition = "Type or variable this rule applies to.") 3607 protected IdType context; 3608 3609 /** 3610 * Specified minimum cardinality for the element. This is optional; if present, 3611 * it acts an implicit check on the input content. 3612 */ 3613 @Child(name = "min", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3614 @Description(shortDefinition = "Specified minimum cardinality", formalDefinition = "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.") 3615 protected IntegerType min; 3616 3617 /** 3618 * Specified maximum cardinality for the element - a number or a "*". This is 3619 * optional; if present, it acts an implicit check on the input content (* just 3620 * serves as documentation; it's the default value). 3621 */ 3622 @Child(name = "max", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3623 @Description(shortDefinition = "Specified maximum cardinality (number or *)", formalDefinition = "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).") 3624 protected StringType max; 3625 3626 /** 3627 * Specified type for the element. This works as a condition on the mapping - 3628 * use for polymorphic elements. 3629 */ 3630 @Child(name = "type", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 3631 @Description(shortDefinition = "Rule only applies if source has this type", formalDefinition = "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.") 3632 protected StringType type; 3633 3634 /** 3635 * A value to use if there is no existing value in the source object. 3636 */ 3637 @Child(name = "defaultValue", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 3638 @Description(shortDefinition = "Default value if no value exists", formalDefinition = "A value to use if there is no existing value in the source object.") 3639 protected org.hl7.fhir.r4.model.Type defaultValue; 3640 3641 /** 3642 * Optional field for this source. 3643 */ 3644 @Child(name = "element", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3645 @Description(shortDefinition = "Optional field for this source", formalDefinition = "Optional field for this source.") 3646 protected StringType element; 3647 3648 /** 3649 * How to handle the list mode for this element. 3650 */ 3651 @Child(name = "listMode", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3652 @Description(shortDefinition = "first | not_first | last | not_last | only_one", formalDefinition = "How to handle the list mode for this element.") 3653 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/map-source-list-mode") 3654 protected Enumeration<StructureMapSourceListMode> listMode; 3655 3656 /** 3657 * Named context for field, if a field is specified. 3658 */ 3659 @Child(name = "variable", type = { IdType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 3660 @Description(shortDefinition = "Named context for field, if a field is specified", formalDefinition = "Named context for field, if a field is specified.") 3661 protected IdType variable; 3662 3663 /** 3664 * FHIRPath expression - must be true or the rule does not apply. 3665 */ 3666 @Child(name = "condition", type = { 3667 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3668 @Description(shortDefinition = "FHIRPath expression - must be true or the rule does not apply", formalDefinition = "FHIRPath expression - must be true or the rule does not apply.") 3669 protected StringType condition; 3670 3671 /** 3672 * FHIRPath expression - must be true or the mapping engine throws an error 3673 * instead of completing. 3674 */ 3675 @Child(name = "check", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 3676 @Description(shortDefinition = "FHIRPath expression - must be true or the mapping engine throws an error instead of completing", formalDefinition = "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.") 3677 protected StringType check; 3678 3679 /** 3680 * A FHIRPath expression which specifies a message to put in the transform log 3681 * when content matching the source rule is found. 3682 */ 3683 @Child(name = "logMessage", type = { 3684 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 3685 @Description(shortDefinition = "Message to put in log if source exists (FHIRPath)", formalDefinition = "A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.") 3686 protected StringType logMessage; 3687 3688 private static final long serialVersionUID = 736427977L; 3689 3690 /** 3691 * Constructor 3692 */ 3693 public StructureMapGroupRuleSourceComponent() { 3694 super(); 3695 } 3696 3697 /** 3698 * Constructor 3699 */ 3700 public StructureMapGroupRuleSourceComponent(IdType context) { 3701 super(); 3702 this.context = context; 3703 } 3704 3705 /** 3706 * @return {@link #context} (Type or variable this rule applies to.). This is 3707 * the underlying object with id, value and extensions. The accessor 3708 * "getContext" gives direct access to the value 3709 */ 3710 public IdType getContextElement() { 3711 if (this.context == null) 3712 if (Configuration.errorOnAutoCreate()) 3713 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.context"); 3714 else if (Configuration.doAutoCreate()) 3715 this.context = new IdType(); // bb 3716 return this.context; 3717 } 3718 3719 public boolean hasContextElement() { 3720 return this.context != null && !this.context.isEmpty(); 3721 } 3722 3723 public boolean hasContext() { 3724 return this.context != null && !this.context.isEmpty(); 3725 } 3726 3727 /** 3728 * @param value {@link #context} (Type or variable this rule applies to.). This 3729 * is the underlying object with id, value and extensions. The 3730 * accessor "getContext" gives direct access to the value 3731 */ 3732 public StructureMapGroupRuleSourceComponent setContextElement(IdType value) { 3733 this.context = value; 3734 return this; 3735 } 3736 3737 /** 3738 * @return Type or variable this rule applies to. 3739 */ 3740 public String getContext() { 3741 return this.context == null ? null : this.context.getValue(); 3742 } 3743 3744 /** 3745 * @param value Type or variable this rule applies to. 3746 */ 3747 public StructureMapGroupRuleSourceComponent setContext(String value) { 3748 if (this.context == null) 3749 this.context = new IdType(); 3750 this.context.setValue(value); 3751 return this; 3752 } 3753 3754 /** 3755 * @return {@link #min} (Specified minimum cardinality for the element. This is 3756 * optional; if present, it acts an implicit check on the input 3757 * content.). This is the underlying object with id, value and 3758 * extensions. The accessor "getMin" gives direct access to the value 3759 */ 3760 public IntegerType getMinElement() { 3761 if (this.min == null) 3762 if (Configuration.errorOnAutoCreate()) 3763 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.min"); 3764 else if (Configuration.doAutoCreate()) 3765 this.min = new IntegerType(); // bb 3766 return this.min; 3767 } 3768 3769 public boolean hasMinElement() { 3770 return this.min != null && !this.min.isEmpty(); 3771 } 3772 3773 public boolean hasMin() { 3774 return this.min != null && !this.min.isEmpty(); 3775 } 3776 3777 /** 3778 * @param value {@link #min} (Specified minimum cardinality for the element. 3779 * This is optional; if present, it acts an implicit check on the 3780 * input content.). This is the underlying object with id, value 3781 * and extensions. The accessor "getMin" gives direct access to the 3782 * value 3783 */ 3784 public StructureMapGroupRuleSourceComponent setMinElement(IntegerType value) { 3785 this.min = value; 3786 return this; 3787 } 3788 3789 /** 3790 * @return Specified minimum cardinality for the element. This is optional; if 3791 * present, it acts an implicit check on the input content. 3792 */ 3793 public int getMin() { 3794 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 3795 } 3796 3797 /** 3798 * @param value Specified minimum cardinality for the element. This is optional; 3799 * if present, it acts an implicit check on the input content. 3800 */ 3801 public StructureMapGroupRuleSourceComponent setMin(int value) { 3802 if (this.min == null) 3803 this.min = new IntegerType(); 3804 this.min.setValue(value); 3805 return this; 3806 } 3807 3808 /** 3809 * @return {@link #max} (Specified maximum cardinality for the element - a 3810 * number or a "*". This is optional; if present, it acts an implicit 3811 * check on the input content (* just serves as documentation; it's the 3812 * default value).). This is the underlying object with id, value and 3813 * extensions. The accessor "getMax" gives direct access to the value 3814 */ 3815 public StringType getMaxElement() { 3816 if (this.max == null) 3817 if (Configuration.errorOnAutoCreate()) 3818 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.max"); 3819 else if (Configuration.doAutoCreate()) 3820 this.max = new StringType(); // bb 3821 return this.max; 3822 } 3823 3824 public boolean hasMaxElement() { 3825 return this.max != null && !this.max.isEmpty(); 3826 } 3827 3828 public boolean hasMax() { 3829 return this.max != null && !this.max.isEmpty(); 3830 } 3831 3832 /** 3833 * @param value {@link #max} (Specified maximum cardinality for the element - a 3834 * number or a "*". This is optional; if present, it acts an 3835 * implicit check on the input content (* just serves as 3836 * documentation; it's the default value).). This is the underlying 3837 * object with id, value and extensions. The accessor "getMax" 3838 * gives direct access to the value 3839 */ 3840 public StructureMapGroupRuleSourceComponent setMaxElement(StringType value) { 3841 this.max = value; 3842 return this; 3843 } 3844 3845 /** 3846 * @return Specified maximum cardinality for the element - a number or a "*". 3847 * This is optional; if present, it acts an implicit check on the input 3848 * content (* just serves as documentation; it's the default value). 3849 */ 3850 public String getMax() { 3851 return this.max == null ? null : this.max.getValue(); 3852 } 3853 3854 /** 3855 * @param value Specified maximum cardinality for the element - a number or a 3856 * "*". This is optional; if present, it acts an implicit check on 3857 * the input content (* just serves as documentation; it's the 3858 * default value). 3859 */ 3860 public StructureMapGroupRuleSourceComponent setMax(String value) { 3861 if (Utilities.noString(value)) 3862 this.max = null; 3863 else { 3864 if (this.max == null) 3865 this.max = new StringType(); 3866 this.max.setValue(value); 3867 } 3868 return this; 3869 } 3870 3871 /** 3872 * @return {@link #type} (Specified type for the element. This works as a 3873 * condition on the mapping - use for polymorphic elements.). This is 3874 * the underlying object with id, value and extensions. The accessor 3875 * "getType" gives direct access to the value 3876 */ 3877 public StringType getTypeElement() { 3878 if (this.type == null) 3879 if (Configuration.errorOnAutoCreate()) 3880 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.type"); 3881 else if (Configuration.doAutoCreate()) 3882 this.type = new StringType(); // bb 3883 return this.type; 3884 } 3885 3886 public boolean hasTypeElement() { 3887 return this.type != null && !this.type.isEmpty(); 3888 } 3889 3890 public boolean hasType() { 3891 return this.type != null && !this.type.isEmpty(); 3892 } 3893 3894 /** 3895 * @param value {@link #type} (Specified type for the element. This works as a 3896 * condition on the mapping - use for polymorphic elements.). This 3897 * is the underlying object with id, value and extensions. The 3898 * accessor "getType" gives direct access to the value 3899 */ 3900 public StructureMapGroupRuleSourceComponent setTypeElement(StringType value) { 3901 this.type = value; 3902 return this; 3903 } 3904 3905 /** 3906 * @return Specified type for the element. This works as a condition on the 3907 * mapping - use for polymorphic elements. 3908 */ 3909 public String getType() { 3910 return this.type == null ? null : this.type.getValue(); 3911 } 3912 3913 /** 3914 * @param value Specified type for the element. This works as a condition on the 3915 * mapping - use for polymorphic elements. 3916 */ 3917 public StructureMapGroupRuleSourceComponent setType(String value) { 3918 if (Utilities.noString(value)) 3919 this.type = null; 3920 else { 3921 if (this.type == null) 3922 this.type = new StringType(); 3923 this.type.setValue(value); 3924 } 3925 return this; 3926 } 3927 3928 /** 3929 * @return {@link #defaultValue} (A value to use if there is no existing value 3930 * in the source object.) 3931 */ 3932 public org.hl7.fhir.r4.model.Type getDefaultValue() { 3933 return this.defaultValue; 3934 } 3935 3936 public boolean hasDefaultValue() { 3937 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3938 } 3939 3940 /** 3941 * @param value {@link #defaultValue} (A value to use if there is no existing 3942 * value in the source object.) 3943 */ 3944 public StructureMapGroupRuleSourceComponent setDefaultValue(org.hl7.fhir.r4.model.Type value) { 3945 this.defaultValue = value; 3946 return this; 3947 } 3948 3949 /** 3950 * @return {@link #element} (Optional field for this source.). This is the 3951 * underlying object with id, value and extensions. The accessor 3952 * "getElement" gives direct access to the value 3953 */ 3954 public StringType getElementElement() { 3955 if (this.element == null) 3956 if (Configuration.errorOnAutoCreate()) 3957 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.element"); 3958 else if (Configuration.doAutoCreate()) 3959 this.element = new StringType(); // bb 3960 return this.element; 3961 } 3962 3963 public boolean hasElementElement() { 3964 return this.element != null && !this.element.isEmpty(); 3965 } 3966 3967 public boolean hasElement() { 3968 return this.element != null && !this.element.isEmpty(); 3969 } 3970 3971 /** 3972 * @param value {@link #element} (Optional field for this source.). This is the 3973 * underlying object with id, value and extensions. The accessor 3974 * "getElement" gives direct access to the value 3975 */ 3976 public StructureMapGroupRuleSourceComponent setElementElement(StringType value) { 3977 this.element = value; 3978 return this; 3979 } 3980 3981 /** 3982 * @return Optional field for this source. 3983 */ 3984 public String getElement() { 3985 return this.element == null ? null : this.element.getValue(); 3986 } 3987 3988 /** 3989 * @param value Optional field for this source. 3990 */ 3991 public StructureMapGroupRuleSourceComponent setElement(String value) { 3992 if (Utilities.noString(value)) 3993 this.element = null; 3994 else { 3995 if (this.element == null) 3996 this.element = new StringType(); 3997 this.element.setValue(value); 3998 } 3999 return this; 4000 } 4001 4002 /** 4003 * @return {@link #listMode} (How to handle the list mode for this element.). 4004 * This is the underlying object with id, value and extensions. The 4005 * accessor "getListMode" gives direct access to the value 4006 */ 4007 public Enumeration<StructureMapSourceListMode> getListModeElement() { 4008 if (this.listMode == null) 4009 if (Configuration.errorOnAutoCreate()) 4010 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.listMode"); 4011 else if (Configuration.doAutoCreate()) 4012 this.listMode = new Enumeration<StructureMapSourceListMode>(new StructureMapSourceListModeEnumFactory()); // bb 4013 return this.listMode; 4014 } 4015 4016 public boolean hasListModeElement() { 4017 return this.listMode != null && !this.listMode.isEmpty(); 4018 } 4019 4020 public boolean hasListMode() { 4021 return this.listMode != null && !this.listMode.isEmpty(); 4022 } 4023 4024 /** 4025 * @param value {@link #listMode} (How to handle the list mode for this 4026 * element.). This is the underlying object with id, value and 4027 * extensions. The accessor "getListMode" gives direct access to 4028 * the value 4029 */ 4030 public StructureMapGroupRuleSourceComponent setListModeElement(Enumeration<StructureMapSourceListMode> value) { 4031 this.listMode = value; 4032 return this; 4033 } 4034 4035 /** 4036 * @return How to handle the list mode for this element. 4037 */ 4038 public StructureMapSourceListMode getListMode() { 4039 return this.listMode == null ? null : this.listMode.getValue(); 4040 } 4041 4042 /** 4043 * @param value How to handle the list mode for this element. 4044 */ 4045 public StructureMapGroupRuleSourceComponent setListMode(StructureMapSourceListMode value) { 4046 if (value == null) 4047 this.listMode = null; 4048 else { 4049 if (this.listMode == null) 4050 this.listMode = new Enumeration<StructureMapSourceListMode>(new StructureMapSourceListModeEnumFactory()); 4051 this.listMode.setValue(value); 4052 } 4053 return this; 4054 } 4055 4056 /** 4057 * @return {@link #variable} (Named context for field, if a field is 4058 * specified.). This is the underlying object with id, value and 4059 * extensions. The accessor "getVariable" gives direct access to the 4060 * value 4061 */ 4062 public IdType getVariableElement() { 4063 if (this.variable == null) 4064 if (Configuration.errorOnAutoCreate()) 4065 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.variable"); 4066 else if (Configuration.doAutoCreate()) 4067 this.variable = new IdType(); // bb 4068 return this.variable; 4069 } 4070 4071 public boolean hasVariableElement() { 4072 return this.variable != null && !this.variable.isEmpty(); 4073 } 4074 4075 public boolean hasVariable() { 4076 return this.variable != null && !this.variable.isEmpty(); 4077 } 4078 4079 /** 4080 * @param value {@link #variable} (Named context for field, if a field is 4081 * specified.). This is the underlying object with id, value and 4082 * extensions. The accessor "getVariable" gives direct access to 4083 * the value 4084 */ 4085 public StructureMapGroupRuleSourceComponent setVariableElement(IdType value) { 4086 this.variable = value; 4087 return this; 4088 } 4089 4090 /** 4091 * @return Named context for field, if a field is specified. 4092 */ 4093 public String getVariable() { 4094 return this.variable == null ? null : this.variable.getValue(); 4095 } 4096 4097 /** 4098 * @param value Named context for field, if a field is specified. 4099 */ 4100 public StructureMapGroupRuleSourceComponent setVariable(String value) { 4101 if (Utilities.noString(value)) 4102 this.variable = null; 4103 else { 4104 if (this.variable == null) 4105 this.variable = new IdType(); 4106 this.variable.setValue(value); 4107 } 4108 return this; 4109 } 4110 4111 /** 4112 * @return {@link #condition} (FHIRPath expression - must be true or the rule 4113 * does not apply.). This is the underlying object with id, value and 4114 * extensions. The accessor "getCondition" gives direct access to the 4115 * value 4116 */ 4117 public StringType getConditionElement() { 4118 if (this.condition == null) 4119 if (Configuration.errorOnAutoCreate()) 4120 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.condition"); 4121 else if (Configuration.doAutoCreate()) 4122 this.condition = new StringType(); // bb 4123 return this.condition; 4124 } 4125 4126 public boolean hasConditionElement() { 4127 return this.condition != null && !this.condition.isEmpty(); 4128 } 4129 4130 public boolean hasCondition() { 4131 return this.condition != null && !this.condition.isEmpty(); 4132 } 4133 4134 /** 4135 * @param value {@link #condition} (FHIRPath expression - must be true or the 4136 * rule does not apply.). This is the underlying object with id, 4137 * value and extensions. The accessor "getCondition" gives direct 4138 * access to the value 4139 */ 4140 public StructureMapGroupRuleSourceComponent setConditionElement(StringType value) { 4141 this.condition = value; 4142 return this; 4143 } 4144 4145 /** 4146 * @return FHIRPath expression - must be true or the rule does not apply. 4147 */ 4148 public String getCondition() { 4149 return this.condition == null ? null : this.condition.getValue(); 4150 } 4151 4152 /** 4153 * @param value FHIRPath expression - must be true or the rule does not apply. 4154 */ 4155 public StructureMapGroupRuleSourceComponent setCondition(String value) { 4156 if (Utilities.noString(value)) 4157 this.condition = null; 4158 else { 4159 if (this.condition == null) 4160 this.condition = new StringType(); 4161 this.condition.setValue(value); 4162 } 4163 return this; 4164 } 4165 4166 /** 4167 * @return {@link #check} (FHIRPath expression - must be true or the mapping 4168 * engine throws an error instead of completing.). This is the 4169 * underlying object with id, value and extensions. The accessor 4170 * "getCheck" gives direct access to the value 4171 */ 4172 public StringType getCheckElement() { 4173 if (this.check == null) 4174 if (Configuration.errorOnAutoCreate()) 4175 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.check"); 4176 else if (Configuration.doAutoCreate()) 4177 this.check = new StringType(); // bb 4178 return this.check; 4179 } 4180 4181 public boolean hasCheckElement() { 4182 return this.check != null && !this.check.isEmpty(); 4183 } 4184 4185 public boolean hasCheck() { 4186 return this.check != null && !this.check.isEmpty(); 4187 } 4188 4189 /** 4190 * @param value {@link #check} (FHIRPath expression - must be true or the 4191 * mapping engine throws an error instead of completing.). This is 4192 * the underlying object with id, value and extensions. The 4193 * accessor "getCheck" gives direct access to the value 4194 */ 4195 public StructureMapGroupRuleSourceComponent setCheckElement(StringType value) { 4196 this.check = value; 4197 return this; 4198 } 4199 4200 /** 4201 * @return FHIRPath expression - must be true or the mapping engine throws an 4202 * error instead of completing. 4203 */ 4204 public String getCheck() { 4205 return this.check == null ? null : this.check.getValue(); 4206 } 4207 4208 /** 4209 * @param value FHIRPath expression - must be true or the mapping engine throws 4210 * an error instead of completing. 4211 */ 4212 public StructureMapGroupRuleSourceComponent setCheck(String value) { 4213 if (Utilities.noString(value)) 4214 this.check = null; 4215 else { 4216 if (this.check == null) 4217 this.check = new StringType(); 4218 this.check.setValue(value); 4219 } 4220 return this; 4221 } 4222 4223 /** 4224 * @return {@link #logMessage} (A FHIRPath expression which specifies a message 4225 * to put in the transform log when content matching the source rule is 4226 * found.). This is the underlying object with id, value and extensions. 4227 * The accessor "getLogMessage" gives direct access to the value 4228 */ 4229 public StringType getLogMessageElement() { 4230 if (this.logMessage == null) 4231 if (Configuration.errorOnAutoCreate()) 4232 throw new Error("Attempt to auto-create StructureMapGroupRuleSourceComponent.logMessage"); 4233 else if (Configuration.doAutoCreate()) 4234 this.logMessage = new StringType(); // bb 4235 return this.logMessage; 4236 } 4237 4238 public boolean hasLogMessageElement() { 4239 return this.logMessage != null && !this.logMessage.isEmpty(); 4240 } 4241 4242 public boolean hasLogMessage() { 4243 return this.logMessage != null && !this.logMessage.isEmpty(); 4244 } 4245 4246 /** 4247 * @param value {@link #logMessage} (A FHIRPath expression which specifies a 4248 * message to put in the transform log when content matching the 4249 * source rule is found.). This is the underlying object with id, 4250 * value and extensions. The accessor "getLogMessage" gives direct 4251 * access to the value 4252 */ 4253 public StructureMapGroupRuleSourceComponent setLogMessageElement(StringType value) { 4254 this.logMessage = value; 4255 return this; 4256 } 4257 4258 /** 4259 * @return A FHIRPath expression which specifies a message to put in the 4260 * transform log when content matching the source rule is found. 4261 */ 4262 public String getLogMessage() { 4263 return this.logMessage == null ? null : this.logMessage.getValue(); 4264 } 4265 4266 /** 4267 * @param value A FHIRPath expression which specifies a message to put in the 4268 * transform log when content matching the source rule is found. 4269 */ 4270 public StructureMapGroupRuleSourceComponent setLogMessage(String value) { 4271 if (Utilities.noString(value)) 4272 this.logMessage = null; 4273 else { 4274 if (this.logMessage == null) 4275 this.logMessage = new StringType(); 4276 this.logMessage.setValue(value); 4277 } 4278 return this; 4279 } 4280 4281 protected void listChildren(List<Property> children) { 4282 super.listChildren(children); 4283 children.add(new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context)); 4284 children.add(new Property("min", "integer", 4285 "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.", 4286 0, 1, min)); 4287 children.add(new Property("max", "string", 4288 "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).", 4289 0, 1, max)); 4290 children.add(new Property("type", "string", 4291 "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.", 0, 4292 1, type)); 4293 children.add(new Property("defaultValue[x]", "*", 4294 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue)); 4295 children.add(new Property("element", "string", "Optional field for this source.", 0, 1, element)); 4296 children.add(new Property("listMode", "code", "How to handle the list mode for this element.", 0, 1, listMode)); 4297 children.add(new Property("variable", "id", "Named context for field, if a field is specified.", 0, 1, variable)); 4298 children.add(new Property("condition", "string", 4299 "FHIRPath expression - must be true or the rule does not apply.", 0, 1, condition)); 4300 children.add(new Property("check", "string", 4301 "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.", 0, 1, 4302 check)); 4303 children.add(new Property("logMessage", "string", 4304 "A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.", 4305 0, 1, logMessage)); 4306 } 4307 4308 @Override 4309 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4310 switch (_hash) { 4311 case 951530927: 4312 /* context */ return new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context); 4313 case 108114: 4314 /* min */ return new Property("min", "integer", 4315 "Specified minimum cardinality for the element. This is optional; if present, it acts an implicit check on the input content.", 4316 0, 1, min); 4317 case 107876: 4318 /* max */ return new Property("max", "string", 4319 "Specified maximum cardinality for the element - a number or a \"*\". This is optional; if present, it acts an implicit check on the input content (* just serves as documentation; it's the default value).", 4320 0, 1, max); 4321 case 3575610: 4322 /* type */ return new Property("type", "string", 4323 "Specified type for the element. This works as a condition on the mapping - use for polymorphic elements.", 4324 0, 1, type); 4325 case 587922128: 4326 /* defaultValue[x] */ return new Property("defaultValue[x]", "*", 4327 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4328 case -659125328: 4329 /* defaultValue */ return new Property("defaultValue[x]", "*", 4330 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4331 case 1470297600: 4332 /* defaultValueBase64Binary */ return new Property("defaultValue[x]", "*", 4333 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4334 case 600437336: 4335 /* defaultValueBoolean */ return new Property("defaultValue[x]", "*", 4336 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4337 case 264593188: 4338 /* defaultValueCanonical */ return new Property("defaultValue[x]", "*", 4339 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4340 case 1044993469: 4341 /* defaultValueCode */ return new Property("defaultValue[x]", "*", 4342 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4343 case 1045010302: 4344 /* defaultValueDate */ return new Property("defaultValue[x]", "*", 4345 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4346 case 1220374379: 4347 /* defaultValueDateTime */ return new Property("defaultValue[x]", "*", 4348 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4349 case 2077989249: 4350 /* defaultValueDecimal */ return new Property("defaultValue[x]", "*", 4351 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4352 case -2059245333: 4353 /* defaultValueId */ return new Property("defaultValue[x]", "*", 4354 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4355 case -1801671663: 4356 /* defaultValueInstant */ return new Property("defaultValue[x]", "*", 4357 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4358 case -1801189522: 4359 /* defaultValueInteger */ return new Property("defaultValue[x]", "*", 4360 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4361 case -325436225: 4362 /* defaultValueMarkdown */ return new Property("defaultValue[x]", "*", 4363 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4364 case 587910138: 4365 /* defaultValueOid */ return new Property("defaultValue[x]", "*", 4366 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4367 case -737344154: 4368 /* defaultValuePositiveInt */ return new Property("defaultValue[x]", "*", 4369 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4370 case -320515103: 4371 /* defaultValueString */ return new Property("defaultValue[x]", "*", 4372 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4373 case 1045494429: 4374 /* defaultValueTime */ return new Property("defaultValue[x]", "*", 4375 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4376 case 539117290: 4377 /* defaultValueUnsignedInt */ return new Property("defaultValue[x]", "*", 4378 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4379 case 587916188: 4380 /* defaultValueUri */ return new Property("defaultValue[x]", "*", 4381 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4382 case 587916191: 4383 /* defaultValueUrl */ return new Property("defaultValue[x]", "*", 4384 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4385 case 1045535627: 4386 /* defaultValueUuid */ return new Property("defaultValue[x]", "*", 4387 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4388 case -611966428: 4389 /* defaultValueAddress */ return new Property("defaultValue[x]", "*", 4390 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4391 case -1851689217: 4392 /* defaultValueAnnotation */ return new Property("defaultValue[x]", "*", 4393 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4394 case 2034820339: 4395 /* defaultValueAttachment */ return new Property("defaultValue[x]", "*", 4396 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4397 case -410434095: 4398 /* defaultValueCodeableConcept */ return new Property("defaultValue[x]", "*", 4399 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4400 case -783616198: 4401 /* defaultValueCoding */ return new Property("defaultValue[x]", "*", 4402 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4403 case -344740576: 4404 /* defaultValueContactPoint */ return new Property("defaultValue[x]", "*", 4405 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4406 case -975393912: 4407 /* defaultValueHumanName */ return new Property("defaultValue[x]", "*", 4408 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4409 case -1915078535: 4410 /* defaultValueIdentifier */ return new Property("defaultValue[x]", "*", 4411 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4412 case -420255343: 4413 /* defaultValuePeriod */ return new Property("defaultValue[x]", "*", 4414 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4415 case -1857379237: 4416 /* defaultValueQuantity */ return new Property("defaultValue[x]", "*", 4417 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4418 case -1951495315: 4419 /* defaultValueRange */ return new Property("defaultValue[x]", "*", 4420 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4421 case -1951489477: 4422 /* defaultValueRatio */ return new Property("defaultValue[x]", "*", 4423 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4424 case -1488914053: 4425 /* defaultValueReference */ return new Property("defaultValue[x]", "*", 4426 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4427 case -449641228: 4428 /* defaultValueSampledData */ return new Property("defaultValue[x]", "*", 4429 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4430 case 509825768: 4431 /* defaultValueSignature */ return new Property("defaultValue[x]", "*", 4432 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4433 case -302193638: 4434 /* defaultValueTiming */ return new Property("defaultValue[x]", "*", 4435 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4436 case -754548089: 4437 /* defaultValueDosage */ return new Property("defaultValue[x]", "*", 4438 "A value to use if there is no existing value in the source object.", 0, 1, defaultValue); 4439 case -1662836996: 4440 /* element */ return new Property("element", "string", "Optional field for this source.", 0, 1, element); 4441 case 1345445729: 4442 /* listMode */ return new Property("listMode", "code", "How to handle the list mode for this element.", 0, 1, 4443 listMode); 4444 case -1249586564: 4445 /* variable */ return new Property("variable", "id", "Named context for field, if a field is specified.", 0, 1, 4446 variable); 4447 case -861311717: 4448 /* condition */ return new Property("condition", "string", 4449 "FHIRPath expression - must be true or the rule does not apply.", 0, 1, condition); 4450 case 94627080: 4451 /* check */ return new Property("check", "string", 4452 "FHIRPath expression - must be true or the mapping engine throws an error instead of completing.", 0, 1, 4453 check); 4454 case -1067155421: 4455 /* logMessage */ return new Property("logMessage", "string", 4456 "A FHIRPath expression which specifies a message to put in the transform log when content matching the source rule is found.", 4457 0, 1, logMessage); 4458 default: 4459 return super.getNamedProperty(_hash, _name, _checkValid); 4460 } 4461 4462 } 4463 4464 @Override 4465 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4466 switch (hash) { 4467 case 951530927: 4468 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // IdType 4469 case 108114: 4470 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // IntegerType 4471 case 107876: 4472 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 4473 case 3575610: 4474 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // StringType 4475 case -659125328: 4476 /* defaultValue */ return this.defaultValue == null ? new Base[0] : new Base[] { this.defaultValue }; // org.hl7.fhir.r4.model.Type 4477 case -1662836996: 4478 /* element */ return this.element == null ? new Base[0] : new Base[] { this.element }; // StringType 4479 case 1345445729: 4480 /* listMode */ return this.listMode == null ? new Base[0] : new Base[] { this.listMode }; // Enumeration<StructureMapSourceListMode> 4481 case -1249586564: 4482 /* variable */ return this.variable == null ? new Base[0] : new Base[] { this.variable }; // IdType 4483 case -861311717: 4484 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // StringType 4485 case 94627080: 4486 /* check */ return this.check == null ? new Base[0] : new Base[] { this.check }; // StringType 4487 case -1067155421: 4488 /* logMessage */ return this.logMessage == null ? new Base[0] : new Base[] { this.logMessage }; // StringType 4489 default: 4490 return super.getProperty(hash, name, checkValid); 4491 } 4492 4493 } 4494 4495 @Override 4496 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4497 switch (hash) { 4498 case 951530927: // context 4499 this.context = castToId(value); // IdType 4500 return value; 4501 case 108114: // min 4502 this.min = castToInteger(value); // IntegerType 4503 return value; 4504 case 107876: // max 4505 this.max = castToString(value); // StringType 4506 return value; 4507 case 3575610: // type 4508 this.type = castToString(value); // StringType 4509 return value; 4510 case -659125328: // defaultValue 4511 this.defaultValue = castToType(value); // org.hl7.fhir.r4.model.Type 4512 return value; 4513 case -1662836996: // element 4514 this.element = castToString(value); // StringType 4515 return value; 4516 case 1345445729: // listMode 4517 value = new StructureMapSourceListModeEnumFactory().fromType(castToCode(value)); 4518 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 4519 return value; 4520 case -1249586564: // variable 4521 this.variable = castToId(value); // IdType 4522 return value; 4523 case -861311717: // condition 4524 this.condition = castToString(value); // StringType 4525 return value; 4526 case 94627080: // check 4527 this.check = castToString(value); // StringType 4528 return value; 4529 case -1067155421: // logMessage 4530 this.logMessage = castToString(value); // StringType 4531 return value; 4532 default: 4533 return super.setProperty(hash, name, value); 4534 } 4535 4536 } 4537 4538 @Override 4539 public Base setProperty(String name, Base value) throws FHIRException { 4540 if (name.equals("context")) { 4541 this.context = castToId(value); // IdType 4542 } else if (name.equals("min")) { 4543 this.min = castToInteger(value); // IntegerType 4544 } else if (name.equals("max")) { 4545 this.max = castToString(value); // StringType 4546 } else if (name.equals("type")) { 4547 this.type = castToString(value); // StringType 4548 } else if (name.equals("defaultValue[x]")) { 4549 this.defaultValue = castToType(value); // org.hl7.fhir.r4.model.Type 4550 } else if (name.equals("element")) { 4551 this.element = castToString(value); // StringType 4552 } else if (name.equals("listMode")) { 4553 value = new StructureMapSourceListModeEnumFactory().fromType(castToCode(value)); 4554 this.listMode = (Enumeration) value; // Enumeration<StructureMapSourceListMode> 4555 } else if (name.equals("variable")) { 4556 this.variable = castToId(value); // IdType 4557 } else if (name.equals("condition")) { 4558 this.condition = castToString(value); // StringType 4559 } else if (name.equals("check")) { 4560 this.check = castToString(value); // StringType 4561 } else if (name.equals("logMessage")) { 4562 this.logMessage = castToString(value); // StringType 4563 } else 4564 return super.setProperty(name, value); 4565 return value; 4566 } 4567 4568 @Override 4569 public void removeChild(String name, Base value) throws FHIRException { 4570 if (name.equals("context")) { 4571 this.context = null; 4572 } else if (name.equals("min")) { 4573 this.min = null; 4574 } else if (name.equals("max")) { 4575 this.max = null; 4576 } else if (name.equals("type")) { 4577 this.type = null; 4578 } else if (name.equals("defaultValue[x]")) { 4579 this.defaultValue = null; 4580 } else if (name.equals("element")) { 4581 this.element = null; 4582 } else if (name.equals("listMode")) { 4583 this.listMode = null; 4584 } else if (name.equals("variable")) { 4585 this.variable = null; 4586 } else if (name.equals("condition")) { 4587 this.condition = null; 4588 } else if (name.equals("check")) { 4589 this.check = null; 4590 } else if (name.equals("logMessage")) { 4591 this.logMessage = null; 4592 } else 4593 super.removeChild(name, value); 4594 4595 } 4596 4597 @Override 4598 public Base makeProperty(int hash, String name) throws FHIRException { 4599 switch (hash) { 4600 case 951530927: 4601 return getContextElement(); 4602 case 108114: 4603 return getMinElement(); 4604 case 107876: 4605 return getMaxElement(); 4606 case 3575610: 4607 return getTypeElement(); 4608 case 587922128: 4609 return getDefaultValue(); 4610 case -659125328: 4611 return getDefaultValue(); 4612 case -1662836996: 4613 return getElementElement(); 4614 case 1345445729: 4615 return getListModeElement(); 4616 case -1249586564: 4617 return getVariableElement(); 4618 case -861311717: 4619 return getConditionElement(); 4620 case 94627080: 4621 return getCheckElement(); 4622 case -1067155421: 4623 return getLogMessageElement(); 4624 default: 4625 return super.makeProperty(hash, name); 4626 } 4627 4628 } 4629 4630 @Override 4631 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4632 switch (hash) { 4633 case 951530927: 4634 /* context */ return new String[] { "id" }; 4635 case 108114: 4636 /* min */ return new String[] { "integer" }; 4637 case 107876: 4638 /* max */ return new String[] { "string" }; 4639 case 3575610: 4640 /* type */ return new String[] { "string" }; 4641 case -659125328: 4642 /* defaultValue */ return new String[] { "*" }; 4643 case -1662836996: 4644 /* element */ return new String[] { "string" }; 4645 case 1345445729: 4646 /* listMode */ return new String[] { "code" }; 4647 case -1249586564: 4648 /* variable */ return new String[] { "id" }; 4649 case -861311717: 4650 /* condition */ return new String[] { "string" }; 4651 case 94627080: 4652 /* check */ return new String[] { "string" }; 4653 case -1067155421: 4654 /* logMessage */ return new String[] { "string" }; 4655 default: 4656 return super.getTypesForProperty(hash, name); 4657 } 4658 4659 } 4660 4661 @Override 4662 public Base addChild(String name) throws FHIRException { 4663 if (name.equals("context")) { 4664 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.context"); 4665 } else if (name.equals("min")) { 4666 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.min"); 4667 } else if (name.equals("max")) { 4668 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.max"); 4669 } else if (name.equals("type")) { 4670 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.type"); 4671 } else if (name.equals("defaultValueBase64Binary")) { 4672 this.defaultValue = new Base64BinaryType(); 4673 return this.defaultValue; 4674 } else if (name.equals("defaultValueBoolean")) { 4675 this.defaultValue = new BooleanType(); 4676 return this.defaultValue; 4677 } else if (name.equals("defaultValueCanonical")) { 4678 this.defaultValue = new CanonicalType(); 4679 return this.defaultValue; 4680 } else if (name.equals("defaultValueCode")) { 4681 this.defaultValue = new CodeType(); 4682 return this.defaultValue; 4683 } else if (name.equals("defaultValueDate")) { 4684 this.defaultValue = new DateType(); 4685 return this.defaultValue; 4686 } else if (name.equals("defaultValueDateTime")) { 4687 this.defaultValue = new DateTimeType(); 4688 return this.defaultValue; 4689 } else if (name.equals("defaultValueDecimal")) { 4690 this.defaultValue = new DecimalType(); 4691 return this.defaultValue; 4692 } else if (name.equals("defaultValueId")) { 4693 this.defaultValue = new IdType(); 4694 return this.defaultValue; 4695 } else if (name.equals("defaultValueInstant")) { 4696 this.defaultValue = new InstantType(); 4697 return this.defaultValue; 4698 } else if (name.equals("defaultValueInteger")) { 4699 this.defaultValue = new IntegerType(); 4700 return this.defaultValue; 4701 } else if (name.equals("defaultValueMarkdown")) { 4702 this.defaultValue = new MarkdownType(); 4703 return this.defaultValue; 4704 } else if (name.equals("defaultValueOid")) { 4705 this.defaultValue = new OidType(); 4706 return this.defaultValue; 4707 } else if (name.equals("defaultValuePositiveInt")) { 4708 this.defaultValue = new PositiveIntType(); 4709 return this.defaultValue; 4710 } else if (name.equals("defaultValueString")) { 4711 this.defaultValue = new StringType(); 4712 return this.defaultValue; 4713 } else if (name.equals("defaultValueTime")) { 4714 this.defaultValue = new TimeType(); 4715 return this.defaultValue; 4716 } else if (name.equals("defaultValueUnsignedInt")) { 4717 this.defaultValue = new UnsignedIntType(); 4718 return this.defaultValue; 4719 } else if (name.equals("defaultValueUri")) { 4720 this.defaultValue = new UriType(); 4721 return this.defaultValue; 4722 } else if (name.equals("defaultValueUrl")) { 4723 this.defaultValue = new UrlType(); 4724 return this.defaultValue; 4725 } else if (name.equals("defaultValueUuid")) { 4726 this.defaultValue = new UuidType(); 4727 return this.defaultValue; 4728 } else if (name.equals("defaultValueAddress")) { 4729 this.defaultValue = new Address(); 4730 return this.defaultValue; 4731 } else if (name.equals("defaultValueAge")) { 4732 this.defaultValue = new Age(); 4733 return this.defaultValue; 4734 } else if (name.equals("defaultValueAnnotation")) { 4735 this.defaultValue = new Annotation(); 4736 return this.defaultValue; 4737 } else if (name.equals("defaultValueAttachment")) { 4738 this.defaultValue = new Attachment(); 4739 return this.defaultValue; 4740 } else if (name.equals("defaultValueCodeableConcept")) { 4741 this.defaultValue = new CodeableConcept(); 4742 return this.defaultValue; 4743 } else if (name.equals("defaultValueCoding")) { 4744 this.defaultValue = new Coding(); 4745 return this.defaultValue; 4746 } else if (name.equals("defaultValueContactPoint")) { 4747 this.defaultValue = new ContactPoint(); 4748 return this.defaultValue; 4749 } else if (name.equals("defaultValueCount")) { 4750 this.defaultValue = new Count(); 4751 return this.defaultValue; 4752 } else if (name.equals("defaultValueDistance")) { 4753 this.defaultValue = new Distance(); 4754 return this.defaultValue; 4755 } else if (name.equals("defaultValueDuration")) { 4756 this.defaultValue = new Duration(); 4757 return this.defaultValue; 4758 } else if (name.equals("defaultValueHumanName")) { 4759 this.defaultValue = new HumanName(); 4760 return this.defaultValue; 4761 } else if (name.equals("defaultValueIdentifier")) { 4762 this.defaultValue = new Identifier(); 4763 return this.defaultValue; 4764 } else if (name.equals("defaultValueMoney")) { 4765 this.defaultValue = new Money(); 4766 return this.defaultValue; 4767 } else if (name.equals("defaultValuePeriod")) { 4768 this.defaultValue = new Period(); 4769 return this.defaultValue; 4770 } else if (name.equals("defaultValueQuantity")) { 4771 this.defaultValue = new Quantity(); 4772 return this.defaultValue; 4773 } else if (name.equals("defaultValueRange")) { 4774 this.defaultValue = new Range(); 4775 return this.defaultValue; 4776 } else if (name.equals("defaultValueRatio")) { 4777 this.defaultValue = new Ratio(); 4778 return this.defaultValue; 4779 } else if (name.equals("defaultValueReference")) { 4780 this.defaultValue = new Reference(); 4781 return this.defaultValue; 4782 } else if (name.equals("defaultValueSampledData")) { 4783 this.defaultValue = new SampledData(); 4784 return this.defaultValue; 4785 } else if (name.equals("defaultValueSignature")) { 4786 this.defaultValue = new Signature(); 4787 return this.defaultValue; 4788 } else if (name.equals("defaultValueTiming")) { 4789 this.defaultValue = new Timing(); 4790 return this.defaultValue; 4791 } else if (name.equals("defaultValueContactDetail")) { 4792 this.defaultValue = new ContactDetail(); 4793 return this.defaultValue; 4794 } else if (name.equals("defaultValueContributor")) { 4795 this.defaultValue = new Contributor(); 4796 return this.defaultValue; 4797 } else if (name.equals("defaultValueDataRequirement")) { 4798 this.defaultValue = new DataRequirement(); 4799 return this.defaultValue; 4800 } else if (name.equals("defaultValueExpression")) { 4801 this.defaultValue = new Expression(); 4802 return this.defaultValue; 4803 } else if (name.equals("defaultValueParameterDefinition")) { 4804 this.defaultValue = new ParameterDefinition(); 4805 return this.defaultValue; 4806 } else if (name.equals("defaultValueRelatedArtifact")) { 4807 this.defaultValue = new RelatedArtifact(); 4808 return this.defaultValue; 4809 } else if (name.equals("defaultValueTriggerDefinition")) { 4810 this.defaultValue = new TriggerDefinition(); 4811 return this.defaultValue; 4812 } else if (name.equals("defaultValueUsageContext")) { 4813 this.defaultValue = new UsageContext(); 4814 return this.defaultValue; 4815 } else if (name.equals("defaultValueDosage")) { 4816 this.defaultValue = new Dosage(); 4817 return this.defaultValue; 4818 } else if (name.equals("defaultValueMeta")) { 4819 this.defaultValue = new Meta(); 4820 return this.defaultValue; 4821 } else if (name.equals("element")) { 4822 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.element"); 4823 } else if (name.equals("listMode")) { 4824 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.listMode"); 4825 } else if (name.equals("variable")) { 4826 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.variable"); 4827 } else if (name.equals("condition")) { 4828 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.condition"); 4829 } else if (name.equals("check")) { 4830 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.check"); 4831 } else if (name.equals("logMessage")) { 4832 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.logMessage"); 4833 } else 4834 return super.addChild(name); 4835 } 4836 4837 public StructureMapGroupRuleSourceComponent copy() { 4838 StructureMapGroupRuleSourceComponent dst = new StructureMapGroupRuleSourceComponent(); 4839 copyValues(dst); 4840 return dst; 4841 } 4842 4843 public void copyValues(StructureMapGroupRuleSourceComponent dst) { 4844 super.copyValues(dst); 4845 dst.context = context == null ? null : context.copy(); 4846 dst.min = min == null ? null : min.copy(); 4847 dst.max = max == null ? null : max.copy(); 4848 dst.type = type == null ? null : type.copy(); 4849 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 4850 dst.element = element == null ? null : element.copy(); 4851 dst.listMode = listMode == null ? null : listMode.copy(); 4852 dst.variable = variable == null ? null : variable.copy(); 4853 dst.condition = condition == null ? null : condition.copy(); 4854 dst.check = check == null ? null : check.copy(); 4855 dst.logMessage = logMessage == null ? null : logMessage.copy(); 4856 } 4857 4858 @Override 4859 public boolean equalsDeep(Base other_) { 4860 if (!super.equalsDeep(other_)) 4861 return false; 4862 if (!(other_ instanceof StructureMapGroupRuleSourceComponent)) 4863 return false; 4864 StructureMapGroupRuleSourceComponent o = (StructureMapGroupRuleSourceComponent) other_; 4865 return compareDeep(context, o.context, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 4866 && compareDeep(type, o.type, true) && compareDeep(defaultValue, o.defaultValue, true) 4867 && compareDeep(element, o.element, true) && compareDeep(listMode, o.listMode, true) 4868 && compareDeep(variable, o.variable, true) && compareDeep(condition, o.condition, true) 4869 && compareDeep(check, o.check, true) && compareDeep(logMessage, o.logMessage, true); 4870 } 4871 4872 @Override 4873 public boolean equalsShallow(Base other_) { 4874 if (!super.equalsShallow(other_)) 4875 return false; 4876 if (!(other_ instanceof StructureMapGroupRuleSourceComponent)) 4877 return false; 4878 StructureMapGroupRuleSourceComponent o = (StructureMapGroupRuleSourceComponent) other_; 4879 return compareValues(context, o.context, true) && compareValues(min, o.min, true) 4880 && compareValues(max, o.max, true) && compareValues(type, o.type, true) 4881 && compareValues(element, o.element, true) && compareValues(listMode, o.listMode, true) 4882 && compareValues(variable, o.variable, true) && compareValues(condition, o.condition, true) 4883 && compareValues(check, o.check, true) && compareValues(logMessage, o.logMessage, true); 4884 } 4885 4886 public boolean isEmpty() { 4887 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, min, max, type, defaultValue, element, 4888 listMode, variable, condition, check, logMessage); 4889 } 4890 4891 public String fhirType() { 4892 return "StructureMap.group.rule.source"; 4893 4894 } 4895 4896// added from java-adornments.txt: 4897 4898 public String toString() { 4899 return StructureMapUtilities.sourceToString(this); 4900 } 4901 4902// end addition 4903 } 4904 4905 @Block() 4906 public static class StructureMapGroupRuleTargetComponent extends BackboneElement implements IBaseBackboneElement { 4907 /** 4908 * Type or variable this rule applies to. 4909 */ 4910 @Child(name = "context", type = { IdType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 4911 @Description(shortDefinition = "Type or variable this rule applies to", formalDefinition = "Type or variable this rule applies to.") 4912 protected IdType context; 4913 4914 /** 4915 * How to interpret the context. 4916 */ 4917 @Child(name = "contextType", type = { 4918 CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4919 @Description(shortDefinition = "type | variable", formalDefinition = "How to interpret the context.") 4920 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/map-context-type") 4921 protected Enumeration<StructureMapContextType> contextType; 4922 4923 /** 4924 * Field to create in the context. 4925 */ 4926 @Child(name = "element", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4927 @Description(shortDefinition = "Field to create in the context", formalDefinition = "Field to create in the context.") 4928 protected StringType element; 4929 4930 /** 4931 * Named context for field, if desired, and a field is specified. 4932 */ 4933 @Child(name = "variable", type = { IdType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 4934 @Description(shortDefinition = "Named context for field, if desired, and a field is specified", formalDefinition = "Named context for field, if desired, and a field is specified.") 4935 protected IdType variable; 4936 4937 /** 4938 * If field is a list, how to manage the list. 4939 */ 4940 @Child(name = "listMode", type = { 4941 CodeType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4942 @Description(shortDefinition = "first | share | last | collate", formalDefinition = "If field is a list, how to manage the list.") 4943 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/map-target-list-mode") 4944 protected List<Enumeration<StructureMapTargetListMode>> listMode; 4945 4946 /** 4947 * Internal rule reference for shared list items. 4948 */ 4949 @Child(name = "listRuleId", type = { IdType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 4950 @Description(shortDefinition = "Internal rule reference for shared list items", formalDefinition = "Internal rule reference for shared list items.") 4951 protected IdType listRuleId; 4952 4953 /** 4954 * How the data is copied / created. 4955 */ 4956 @Child(name = "transform", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 4957 @Description(shortDefinition = "create | copy +", formalDefinition = "How the data is copied / created.") 4958 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/map-transform") 4959 protected Enumeration<StructureMapTransform> transform; 4960 4961 /** 4962 * Parameters to the transform. 4963 */ 4964 @Child(name = "parameter", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4965 @Description(shortDefinition = "Parameters to the transform", formalDefinition = "Parameters to the transform.") 4966 protected List<StructureMapGroupRuleTargetParameterComponent> parameter; 4967 4968 private static final long serialVersionUID = -1441766429L; 4969 4970 /** 4971 * Constructor 4972 */ 4973 public StructureMapGroupRuleTargetComponent() { 4974 super(); 4975 } 4976 4977 /** 4978 * @return {@link #context} (Type or variable this rule applies to.). This is 4979 * the underlying object with id, value and extensions. The accessor 4980 * "getContext" gives direct access to the value 4981 */ 4982 public IdType getContextElement() { 4983 if (this.context == null) 4984 if (Configuration.errorOnAutoCreate()) 4985 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.context"); 4986 else if (Configuration.doAutoCreate()) 4987 this.context = new IdType(); // bb 4988 return this.context; 4989 } 4990 4991 public boolean hasContextElement() { 4992 return this.context != null && !this.context.isEmpty(); 4993 } 4994 4995 public boolean hasContext() { 4996 return this.context != null && !this.context.isEmpty(); 4997 } 4998 4999 /** 5000 * @param value {@link #context} (Type or variable this rule applies to.). This 5001 * is the underlying object with id, value and extensions. The 5002 * accessor "getContext" gives direct access to the value 5003 */ 5004 public StructureMapGroupRuleTargetComponent setContextElement(IdType value) { 5005 this.context = value; 5006 return this; 5007 } 5008 5009 /** 5010 * @return Type or variable this rule applies to. 5011 */ 5012 public String getContext() { 5013 return this.context == null ? null : this.context.getValue(); 5014 } 5015 5016 /** 5017 * @param value Type or variable this rule applies to. 5018 */ 5019 public StructureMapGroupRuleTargetComponent setContext(String value) { 5020 if (Utilities.noString(value)) 5021 this.context = null; 5022 else { 5023 if (this.context == null) 5024 this.context = new IdType(); 5025 this.context.setValue(value); 5026 } 5027 return this; 5028 } 5029 5030 /** 5031 * @return {@link #contextType} (How to interpret the context.). This is the 5032 * underlying object with id, value and extensions. The accessor 5033 * "getContextType" gives direct access to the value 5034 */ 5035 public Enumeration<StructureMapContextType> getContextTypeElement() { 5036 if (this.contextType == null) 5037 if (Configuration.errorOnAutoCreate()) 5038 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.contextType"); 5039 else if (Configuration.doAutoCreate()) 5040 this.contextType = new Enumeration<StructureMapContextType>(new StructureMapContextTypeEnumFactory()); // bb 5041 return this.contextType; 5042 } 5043 5044 public boolean hasContextTypeElement() { 5045 return this.contextType != null && !this.contextType.isEmpty(); 5046 } 5047 5048 public boolean hasContextType() { 5049 return this.contextType != null && !this.contextType.isEmpty(); 5050 } 5051 5052 /** 5053 * @param value {@link #contextType} (How to interpret the context.). This is 5054 * the underlying object with id, value and extensions. The 5055 * accessor "getContextType" gives direct access to the value 5056 */ 5057 public StructureMapGroupRuleTargetComponent setContextTypeElement(Enumeration<StructureMapContextType> value) { 5058 this.contextType = value; 5059 return this; 5060 } 5061 5062 /** 5063 * @return How to interpret the context. 5064 */ 5065 public StructureMapContextType getContextType() { 5066 return this.contextType == null ? null : this.contextType.getValue(); 5067 } 5068 5069 /** 5070 * @param value How to interpret the context. 5071 */ 5072 public StructureMapGroupRuleTargetComponent setContextType(StructureMapContextType value) { 5073 if (value == null) 5074 this.contextType = null; 5075 else { 5076 if (this.contextType == null) 5077 this.contextType = new Enumeration<StructureMapContextType>(new StructureMapContextTypeEnumFactory()); 5078 this.contextType.setValue(value); 5079 } 5080 return this; 5081 } 5082 5083 /** 5084 * @return {@link #element} (Field to create in the context.). This is the 5085 * underlying object with id, value and extensions. The accessor 5086 * "getElement" gives direct access to the value 5087 */ 5088 public StringType getElementElement() { 5089 if (this.element == null) 5090 if (Configuration.errorOnAutoCreate()) 5091 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.element"); 5092 else if (Configuration.doAutoCreate()) 5093 this.element = new StringType(); // bb 5094 return this.element; 5095 } 5096 5097 public boolean hasElementElement() { 5098 return this.element != null && !this.element.isEmpty(); 5099 } 5100 5101 public boolean hasElement() { 5102 return this.element != null && !this.element.isEmpty(); 5103 } 5104 5105 /** 5106 * @param value {@link #element} (Field to create in the context.). This is the 5107 * underlying object with id, value and extensions. The accessor 5108 * "getElement" gives direct access to the value 5109 */ 5110 public StructureMapGroupRuleTargetComponent setElementElement(StringType value) { 5111 this.element = value; 5112 return this; 5113 } 5114 5115 /** 5116 * @return Field to create in the context. 5117 */ 5118 public String getElement() { 5119 return this.element == null ? null : this.element.getValue(); 5120 } 5121 5122 /** 5123 * @param value Field to create in the context. 5124 */ 5125 public StructureMapGroupRuleTargetComponent setElement(String value) { 5126 if (Utilities.noString(value)) 5127 this.element = null; 5128 else { 5129 if (this.element == null) 5130 this.element = new StringType(); 5131 this.element.setValue(value); 5132 } 5133 return this; 5134 } 5135 5136 /** 5137 * @return {@link #variable} (Named context for field, if desired, and a field 5138 * is specified.). This is the underlying object with id, value and 5139 * extensions. The accessor "getVariable" gives direct access to the 5140 * value 5141 */ 5142 public IdType getVariableElement() { 5143 if (this.variable == null) 5144 if (Configuration.errorOnAutoCreate()) 5145 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.variable"); 5146 else if (Configuration.doAutoCreate()) 5147 this.variable = new IdType(); // bb 5148 return this.variable; 5149 } 5150 5151 public boolean hasVariableElement() { 5152 return this.variable != null && !this.variable.isEmpty(); 5153 } 5154 5155 public boolean hasVariable() { 5156 return this.variable != null && !this.variable.isEmpty(); 5157 } 5158 5159 /** 5160 * @param value {@link #variable} (Named context for field, if desired, and a 5161 * field is specified.). This is the underlying object with id, 5162 * value and extensions. The accessor "getVariable" gives direct 5163 * access to the value 5164 */ 5165 public StructureMapGroupRuleTargetComponent setVariableElement(IdType value) { 5166 this.variable = value; 5167 return this; 5168 } 5169 5170 /** 5171 * @return Named context for field, if desired, and a field is specified. 5172 */ 5173 public String getVariable() { 5174 return this.variable == null ? null : this.variable.getValue(); 5175 } 5176 5177 /** 5178 * @param value Named context for field, if desired, and a field is specified. 5179 */ 5180 public StructureMapGroupRuleTargetComponent setVariable(String value) { 5181 if (Utilities.noString(value)) 5182 this.variable = null; 5183 else { 5184 if (this.variable == null) 5185 this.variable = new IdType(); 5186 this.variable.setValue(value); 5187 } 5188 return this; 5189 } 5190 5191 /** 5192 * @return {@link #listMode} (If field is a list, how to manage the list.) 5193 */ 5194 public List<Enumeration<StructureMapTargetListMode>> getListMode() { 5195 if (this.listMode == null) 5196 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 5197 return this.listMode; 5198 } 5199 5200 /** 5201 * @return Returns a reference to <code>this</code> for easy method chaining 5202 */ 5203 public StructureMapGroupRuleTargetComponent setListMode(List<Enumeration<StructureMapTargetListMode>> theListMode) { 5204 this.listMode = theListMode; 5205 return this; 5206 } 5207 5208 public boolean hasListMode() { 5209 if (this.listMode == null) 5210 return false; 5211 for (Enumeration<StructureMapTargetListMode> item : this.listMode) 5212 if (!item.isEmpty()) 5213 return true; 5214 return false; 5215 } 5216 5217 /** 5218 * @return {@link #listMode} (If field is a list, how to manage the list.) 5219 */ 5220 public Enumeration<StructureMapTargetListMode> addListModeElement() {// 2 5221 Enumeration<StructureMapTargetListMode> t = new Enumeration<StructureMapTargetListMode>( 5222 new StructureMapTargetListModeEnumFactory()); 5223 if (this.listMode == null) 5224 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 5225 this.listMode.add(t); 5226 return t; 5227 } 5228 5229 /** 5230 * @param value {@link #listMode} (If field is a list, how to manage the list.) 5231 */ 5232 public StructureMapGroupRuleTargetComponent addListMode(StructureMapTargetListMode value) { // 1 5233 Enumeration<StructureMapTargetListMode> t = new Enumeration<StructureMapTargetListMode>( 5234 new StructureMapTargetListModeEnumFactory()); 5235 t.setValue(value); 5236 if (this.listMode == null) 5237 this.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 5238 this.listMode.add(t); 5239 return this; 5240 } 5241 5242 /** 5243 * @param value {@link #listMode} (If field is a list, how to manage the list.) 5244 */ 5245 public boolean hasListMode(StructureMapTargetListMode value) { 5246 if (this.listMode == null) 5247 return false; 5248 for (Enumeration<StructureMapTargetListMode> v : this.listMode) 5249 if (v.getValue().equals(value)) // code 5250 return true; 5251 return false; 5252 } 5253 5254 /** 5255 * @return {@link #listRuleId} (Internal rule reference for shared list items.). 5256 * This is the underlying object with id, value and extensions. The 5257 * accessor "getListRuleId" gives direct access to the value 5258 */ 5259 public IdType getListRuleIdElement() { 5260 if (this.listRuleId == null) 5261 if (Configuration.errorOnAutoCreate()) 5262 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.listRuleId"); 5263 else if (Configuration.doAutoCreate()) 5264 this.listRuleId = new IdType(); // bb 5265 return this.listRuleId; 5266 } 5267 5268 public boolean hasListRuleIdElement() { 5269 return this.listRuleId != null && !this.listRuleId.isEmpty(); 5270 } 5271 5272 public boolean hasListRuleId() { 5273 return this.listRuleId != null && !this.listRuleId.isEmpty(); 5274 } 5275 5276 /** 5277 * @param value {@link #listRuleId} (Internal rule reference for shared list 5278 * items.). This is the underlying object with id, value and 5279 * extensions. The accessor "getListRuleId" gives direct access to 5280 * the value 5281 */ 5282 public StructureMapGroupRuleTargetComponent setListRuleIdElement(IdType value) { 5283 this.listRuleId = value; 5284 return this; 5285 } 5286 5287 /** 5288 * @return Internal rule reference for shared list items. 5289 */ 5290 public String getListRuleId() { 5291 return this.listRuleId == null ? null : this.listRuleId.getValue(); 5292 } 5293 5294 /** 5295 * @param value Internal rule reference for shared list items. 5296 */ 5297 public StructureMapGroupRuleTargetComponent setListRuleId(String value) { 5298 if (Utilities.noString(value)) 5299 this.listRuleId = null; 5300 else { 5301 if (this.listRuleId == null) 5302 this.listRuleId = new IdType(); 5303 this.listRuleId.setValue(value); 5304 } 5305 return this; 5306 } 5307 5308 /** 5309 * @return {@link #transform} (How the data is copied / created.). This is the 5310 * underlying object with id, value and extensions. The accessor 5311 * "getTransform" gives direct access to the value 5312 */ 5313 public Enumeration<StructureMapTransform> getTransformElement() { 5314 if (this.transform == null) 5315 if (Configuration.errorOnAutoCreate()) 5316 throw new Error("Attempt to auto-create StructureMapGroupRuleTargetComponent.transform"); 5317 else if (Configuration.doAutoCreate()) 5318 this.transform = new Enumeration<StructureMapTransform>(new StructureMapTransformEnumFactory()); // bb 5319 return this.transform; 5320 } 5321 5322 public boolean hasTransformElement() { 5323 return this.transform != null && !this.transform.isEmpty(); 5324 } 5325 5326 public boolean hasTransform() { 5327 return this.transform != null && !this.transform.isEmpty(); 5328 } 5329 5330 /** 5331 * @param value {@link #transform} (How the data is copied / created.). This is 5332 * the underlying object with id, value and extensions. The 5333 * accessor "getTransform" gives direct access to the value 5334 */ 5335 public StructureMapGroupRuleTargetComponent setTransformElement(Enumeration<StructureMapTransform> value) { 5336 this.transform = value; 5337 return this; 5338 } 5339 5340 /** 5341 * @return How the data is copied / created. 5342 */ 5343 public StructureMapTransform getTransform() { 5344 return this.transform == null ? null : this.transform.getValue(); 5345 } 5346 5347 /** 5348 * @param value How the data is copied / created. 5349 */ 5350 public StructureMapGroupRuleTargetComponent setTransform(StructureMapTransform value) { 5351 if (value == null) 5352 this.transform = null; 5353 else { 5354 if (this.transform == null) 5355 this.transform = new Enumeration<StructureMapTransform>(new StructureMapTransformEnumFactory()); 5356 this.transform.setValue(value); 5357 } 5358 return this; 5359 } 5360 5361 /** 5362 * @return {@link #parameter} (Parameters to the transform.) 5363 */ 5364 public List<StructureMapGroupRuleTargetParameterComponent> getParameter() { 5365 if (this.parameter == null) 5366 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5367 return this.parameter; 5368 } 5369 5370 /** 5371 * @return Returns a reference to <code>this</code> for easy method chaining 5372 */ 5373 public StructureMapGroupRuleTargetComponent setParameter( 5374 List<StructureMapGroupRuleTargetParameterComponent> theParameter) { 5375 this.parameter = theParameter; 5376 return this; 5377 } 5378 5379 public boolean hasParameter() { 5380 if (this.parameter == null) 5381 return false; 5382 for (StructureMapGroupRuleTargetParameterComponent item : this.parameter) 5383 if (!item.isEmpty()) 5384 return true; 5385 return false; 5386 } 5387 5388 public StructureMapGroupRuleTargetParameterComponent addParameter() { // 3 5389 StructureMapGroupRuleTargetParameterComponent t = new StructureMapGroupRuleTargetParameterComponent(); 5390 if (this.parameter == null) 5391 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5392 this.parameter.add(t); 5393 return t; 5394 } 5395 5396 public StructureMapGroupRuleTargetComponent addParameter(StructureMapGroupRuleTargetParameterComponent t) { // 3 5397 if (t == null) 5398 return this; 5399 if (this.parameter == null) 5400 this.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5401 this.parameter.add(t); 5402 return this; 5403 } 5404 5405 /** 5406 * @return The first repetition of repeating field {@link #parameter}, creating 5407 * it if it does not already exist 5408 */ 5409 public StructureMapGroupRuleTargetParameterComponent getParameterFirstRep() { 5410 if (getParameter().isEmpty()) { 5411 addParameter(); 5412 } 5413 return getParameter().get(0); 5414 } 5415 5416 protected void listChildren(List<Property> children) { 5417 super.listChildren(children); 5418 children.add(new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context)); 5419 children.add(new Property("contextType", "code", "How to interpret the context.", 0, 1, contextType)); 5420 children.add(new Property("element", "string", "Field to create in the context.", 0, 1, element)); 5421 children.add(new Property("variable", "id", "Named context for field, if desired, and a field is specified.", 0, 5422 1, variable)); 5423 children.add(new Property("listMode", "code", "If field is a list, how to manage the list.", 0, 5424 java.lang.Integer.MAX_VALUE, listMode)); 5425 children 5426 .add(new Property("listRuleId", "id", "Internal rule reference for shared list items.", 0, 1, listRuleId)); 5427 children.add(new Property("transform", "code", "How the data is copied / created.", 0, 1, transform)); 5428 children.add( 5429 new Property("parameter", "", "Parameters to the transform.", 0, java.lang.Integer.MAX_VALUE, parameter)); 5430 } 5431 5432 @Override 5433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5434 switch (_hash) { 5435 case 951530927: 5436 /* context */ return new Property("context", "id", "Type or variable this rule applies to.", 0, 1, context); 5437 case -102839927: 5438 /* contextType */ return new Property("contextType", "code", "How to interpret the context.", 0, 1, 5439 contextType); 5440 case -1662836996: 5441 /* element */ return new Property("element", "string", "Field to create in the context.", 0, 1, element); 5442 case -1249586564: 5443 /* variable */ return new Property("variable", "id", 5444 "Named context for field, if desired, and a field is specified.", 0, 1, variable); 5445 case 1345445729: 5446 /* listMode */ return new Property("listMode", "code", "If field is a list, how to manage the list.", 0, 5447 java.lang.Integer.MAX_VALUE, listMode); 5448 case 337117045: 5449 /* listRuleId */ return new Property("listRuleId", "id", "Internal rule reference for shared list items.", 0, 1, 5450 listRuleId); 5451 case 1052666732: 5452 /* transform */ return new Property("transform", "code", "How the data is copied / created.", 0, 1, transform); 5453 case 1954460585: 5454 /* parameter */ return new Property("parameter", "", "Parameters to the transform.", 0, 5455 java.lang.Integer.MAX_VALUE, parameter); 5456 default: 5457 return super.getNamedProperty(_hash, _name, _checkValid); 5458 } 5459 5460 } 5461 5462 @Override 5463 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5464 switch (hash) { 5465 case 951530927: 5466 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // IdType 5467 case -102839927: 5468 /* contextType */ return this.contextType == null ? new Base[0] : new Base[] { this.contextType }; // Enumeration<StructureMapContextType> 5469 case -1662836996: 5470 /* element */ return this.element == null ? new Base[0] : new Base[] { this.element }; // StringType 5471 case -1249586564: 5472 /* variable */ return this.variable == null ? new Base[0] : new Base[] { this.variable }; // IdType 5473 case 1345445729: 5474 /* listMode */ return this.listMode == null ? new Base[0] 5475 : this.listMode.toArray(new Base[this.listMode.size()]); // Enumeration<StructureMapTargetListMode> 5476 case 337117045: 5477 /* listRuleId */ return this.listRuleId == null ? new Base[0] : new Base[] { this.listRuleId }; // IdType 5478 case 1052666732: 5479 /* transform */ return this.transform == null ? new Base[0] : new Base[] { this.transform }; // Enumeration<StructureMapTransform> 5480 case 1954460585: 5481 /* parameter */ return this.parameter == null ? new Base[0] 5482 : this.parameter.toArray(new Base[this.parameter.size()]); // StructureMapGroupRuleTargetParameterComponent 5483 default: 5484 return super.getProperty(hash, name, checkValid); 5485 } 5486 5487 } 5488 5489 @Override 5490 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5491 switch (hash) { 5492 case 951530927: // context 5493 this.context = castToId(value); // IdType 5494 return value; 5495 case -102839927: // contextType 5496 value = new StructureMapContextTypeEnumFactory().fromType(castToCode(value)); 5497 this.contextType = (Enumeration) value; // Enumeration<StructureMapContextType> 5498 return value; 5499 case -1662836996: // element 5500 this.element = castToString(value); // StringType 5501 return value; 5502 case -1249586564: // variable 5503 this.variable = castToId(value); // IdType 5504 return value; 5505 case 1345445729: // listMode 5506 value = new StructureMapTargetListModeEnumFactory().fromType(castToCode(value)); 5507 this.getListMode().add((Enumeration) value); // Enumeration<StructureMapTargetListMode> 5508 return value; 5509 case 337117045: // listRuleId 5510 this.listRuleId = castToId(value); // IdType 5511 return value; 5512 case 1052666732: // transform 5513 value = new StructureMapTransformEnumFactory().fromType(castToCode(value)); 5514 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 5515 return value; 5516 case 1954460585: // parameter 5517 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); // StructureMapGroupRuleTargetParameterComponent 5518 return value; 5519 default: 5520 return super.setProperty(hash, name, value); 5521 } 5522 5523 } 5524 5525 @Override 5526 public Base setProperty(String name, Base value) throws FHIRException { 5527 if (name.equals("context")) { 5528 this.context = castToId(value); // IdType 5529 } else if (name.equals("contextType")) { 5530 value = new StructureMapContextTypeEnumFactory().fromType(castToCode(value)); 5531 this.contextType = (Enumeration) value; // Enumeration<StructureMapContextType> 5532 } else if (name.equals("element")) { 5533 this.element = castToString(value); // StringType 5534 } else if (name.equals("variable")) { 5535 this.variable = castToId(value); // IdType 5536 } else if (name.equals("listMode")) { 5537 value = new StructureMapTargetListModeEnumFactory().fromType(castToCode(value)); 5538 this.getListMode().add((Enumeration) value); 5539 } else if (name.equals("listRuleId")) { 5540 this.listRuleId = castToId(value); // IdType 5541 } else if (name.equals("transform")) { 5542 value = new StructureMapTransformEnumFactory().fromType(castToCode(value)); 5543 this.transform = (Enumeration) value; // Enumeration<StructureMapTransform> 5544 } else if (name.equals("parameter")) { 5545 this.getParameter().add((StructureMapGroupRuleTargetParameterComponent) value); 5546 } else 5547 return super.setProperty(name, value); 5548 return value; 5549 } 5550 5551 @Override 5552 public void removeChild(String name, Base value) throws FHIRException { 5553 if (name.equals("context")) { 5554 this.context = null; 5555 } else if (name.equals("contextType")) { 5556 this.contextType = null; 5557 } else if (name.equals("element")) { 5558 this.element = null; 5559 } else if (name.equals("variable")) { 5560 this.variable = null; 5561 } else if (name.equals("listMode")) { 5562 this.getListMode().remove((Enumeration) value); 5563 } else if (name.equals("listRuleId")) { 5564 this.listRuleId = null; 5565 } else if (name.equals("transform")) { 5566 this.transform = null; 5567 } else if (name.equals("parameter")) { 5568 this.getParameter().remove((StructureMapGroupRuleTargetParameterComponent) value); 5569 } else 5570 super.removeChild(name, value); 5571 5572 } 5573 5574 @Override 5575 public Base makeProperty(int hash, String name) throws FHIRException { 5576 switch (hash) { 5577 case 951530927: 5578 return getContextElement(); 5579 case -102839927: 5580 return getContextTypeElement(); 5581 case -1662836996: 5582 return getElementElement(); 5583 case -1249586564: 5584 return getVariableElement(); 5585 case 1345445729: 5586 return addListModeElement(); 5587 case 337117045: 5588 return getListRuleIdElement(); 5589 case 1052666732: 5590 return getTransformElement(); 5591 case 1954460585: 5592 return addParameter(); 5593 default: 5594 return super.makeProperty(hash, name); 5595 } 5596 5597 } 5598 5599 @Override 5600 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5601 switch (hash) { 5602 case 951530927: 5603 /* context */ return new String[] { "id" }; 5604 case -102839927: 5605 /* contextType */ return new String[] { "code" }; 5606 case -1662836996: 5607 /* element */ return new String[] { "string" }; 5608 case -1249586564: 5609 /* variable */ return new String[] { "id" }; 5610 case 1345445729: 5611 /* listMode */ return new String[] { "code" }; 5612 case 337117045: 5613 /* listRuleId */ return new String[] { "id" }; 5614 case 1052666732: 5615 /* transform */ return new String[] { "code" }; 5616 case 1954460585: 5617 /* parameter */ return new String[] {}; 5618 default: 5619 return super.getTypesForProperty(hash, name); 5620 } 5621 5622 } 5623 5624 @Override 5625 public Base addChild(String name) throws FHIRException { 5626 if (name.equals("context")) { 5627 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.context"); 5628 } else if (name.equals("contextType")) { 5629 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.contextType"); 5630 } else if (name.equals("element")) { 5631 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.element"); 5632 } else if (name.equals("variable")) { 5633 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.variable"); 5634 } else if (name.equals("listMode")) { 5635 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.listMode"); 5636 } else if (name.equals("listRuleId")) { 5637 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.listRuleId"); 5638 } else if (name.equals("transform")) { 5639 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.transform"); 5640 } else if (name.equals("parameter")) { 5641 return addParameter(); 5642 } else 5643 return super.addChild(name); 5644 } 5645 5646 public StructureMapGroupRuleTargetComponent copy() { 5647 StructureMapGroupRuleTargetComponent dst = new StructureMapGroupRuleTargetComponent(); 5648 copyValues(dst); 5649 return dst; 5650 } 5651 5652 public void copyValues(StructureMapGroupRuleTargetComponent dst) { 5653 super.copyValues(dst); 5654 dst.context = context == null ? null : context.copy(); 5655 dst.contextType = contextType == null ? null : contextType.copy(); 5656 dst.element = element == null ? null : element.copy(); 5657 dst.variable = variable == null ? null : variable.copy(); 5658 if (listMode != null) { 5659 dst.listMode = new ArrayList<Enumeration<StructureMapTargetListMode>>(); 5660 for (Enumeration<StructureMapTargetListMode> i : listMode) 5661 dst.listMode.add(i.copy()); 5662 } 5663 ; 5664 dst.listRuleId = listRuleId == null ? null : listRuleId.copy(); 5665 dst.transform = transform == null ? null : transform.copy(); 5666 if (parameter != null) { 5667 dst.parameter = new ArrayList<StructureMapGroupRuleTargetParameterComponent>(); 5668 for (StructureMapGroupRuleTargetParameterComponent i : parameter) 5669 dst.parameter.add(i.copy()); 5670 } 5671 ; 5672 } 5673 5674 @Override 5675 public boolean equalsDeep(Base other_) { 5676 if (!super.equalsDeep(other_)) 5677 return false; 5678 if (!(other_ instanceof StructureMapGroupRuleTargetComponent)) 5679 return false; 5680 StructureMapGroupRuleTargetComponent o = (StructureMapGroupRuleTargetComponent) other_; 5681 return compareDeep(context, o.context, true) && compareDeep(contextType, o.contextType, true) 5682 && compareDeep(element, o.element, true) && compareDeep(variable, o.variable, true) 5683 && compareDeep(listMode, o.listMode, true) && compareDeep(listRuleId, o.listRuleId, true) 5684 && compareDeep(transform, o.transform, true) && compareDeep(parameter, o.parameter, true); 5685 } 5686 5687 @Override 5688 public boolean equalsShallow(Base other_) { 5689 if (!super.equalsShallow(other_)) 5690 return false; 5691 if (!(other_ instanceof StructureMapGroupRuleTargetComponent)) 5692 return false; 5693 StructureMapGroupRuleTargetComponent o = (StructureMapGroupRuleTargetComponent) other_; 5694 return compareValues(context, o.context, true) && compareValues(contextType, o.contextType, true) 5695 && compareValues(element, o.element, true) && compareValues(variable, o.variable, true) 5696 && compareValues(listMode, o.listMode, true) && compareValues(listRuleId, o.listRuleId, true) 5697 && compareValues(transform, o.transform, true); 5698 } 5699 5700 public boolean isEmpty() { 5701 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(context, contextType, element, variable, listMode, 5702 listRuleId, transform, parameter); 5703 } 5704 5705 public String fhirType() { 5706 return "StructureMap.group.rule.target"; 5707 5708 } 5709 5710// added from java-adornments.txt: 5711 5712 public String toString() { 5713 return StructureMapUtilities.targetToString(this); 5714 } 5715 5716// end addition 5717 } 5718 5719 @Block() 5720 public static class StructureMapGroupRuleTargetParameterComponent extends BackboneElement 5721 implements IBaseBackboneElement { 5722 /** 5723 * Parameter value - variable or literal. 5724 */ 5725 @Child(name = "value", type = { IdType.class, StringType.class, BooleanType.class, IntegerType.class, 5726 DecimalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 5727 @Description(shortDefinition = "Parameter value - variable or literal", formalDefinition = "Parameter value - variable or literal.") 5728 protected Type value; 5729 5730 private static final long serialVersionUID = -732981989L; 5731 5732 /** 5733 * Constructor 5734 */ 5735 public StructureMapGroupRuleTargetParameterComponent() { 5736 super(); 5737 } 5738 5739 /** 5740 * Constructor 5741 */ 5742 public StructureMapGroupRuleTargetParameterComponent(Type value) { 5743 super(); 5744 this.value = value; 5745 } 5746 5747 /** 5748 * @return {@link #value} (Parameter value - variable or literal.) 5749 */ 5750 public Type getValue() { 5751 return this.value; 5752 } 5753 5754 /** 5755 * @return {@link #value} (Parameter value - variable or literal.) 5756 */ 5757 public IdType getValueIdType() throws FHIRException { 5758 if (this.value == null) 5759 this.value = new IdType(); 5760 if (!(this.value instanceof IdType)) 5761 throw new FHIRException( 5762 "Type mismatch: the type IdType was expected, but " + this.value.getClass().getName() + " was encountered"); 5763 return (IdType) this.value; 5764 } 5765 5766 public boolean hasValueIdType() { 5767 return this.value instanceof IdType; 5768 } 5769 5770 /** 5771 * @return {@link #value} (Parameter value - variable or literal.) 5772 */ 5773 public StringType getValueStringType() throws FHIRException { 5774 if (this.value == null) 5775 this.value = new StringType(); 5776 if (!(this.value instanceof StringType)) 5777 throw new FHIRException("Type mismatch: the type StringType was expected, but " 5778 + this.value.getClass().getName() + " was encountered"); 5779 return (StringType) this.value; 5780 } 5781 5782 public boolean hasValueStringType() { 5783 return this.value instanceof StringType; 5784 } 5785 5786 /** 5787 * @return {@link #value} (Parameter value - variable or literal.) 5788 */ 5789 public BooleanType getValueBooleanType() throws FHIRException { 5790 if (this.value == null) 5791 this.value = new BooleanType(); 5792 if (!(this.value instanceof BooleanType)) 5793 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 5794 + this.value.getClass().getName() + " was encountered"); 5795 return (BooleanType) this.value; 5796 } 5797 5798 public boolean hasValueBooleanType() { 5799 return this.value instanceof BooleanType; 5800 } 5801 5802 /** 5803 * @return {@link #value} (Parameter value - variable or literal.) 5804 */ 5805 public IntegerType getValueIntegerType() throws FHIRException { 5806 if (this.value == null) 5807 this.value = new IntegerType(); 5808 if (!(this.value instanceof IntegerType)) 5809 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 5810 + this.value.getClass().getName() + " was encountered"); 5811 return (IntegerType) this.value; 5812 } 5813 5814 public boolean hasValueIntegerType() { 5815 return this.value instanceof IntegerType; 5816 } 5817 5818 /** 5819 * @return {@link #value} (Parameter value - variable or literal.) 5820 */ 5821 public DecimalType getValueDecimalType() throws FHIRException { 5822 if (this.value == null) 5823 this.value = new DecimalType(); 5824 if (!(this.value instanceof DecimalType)) 5825 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 5826 + this.value.getClass().getName() + " was encountered"); 5827 return (DecimalType) this.value; 5828 } 5829 5830 public boolean hasValueDecimalType() { 5831 return this.value instanceof DecimalType; 5832 } 5833 5834 public boolean hasValue() { 5835 return this.value != null && !this.value.isEmpty(); 5836 } 5837 5838 /** 5839 * @param value {@link #value} (Parameter value - variable or literal.) 5840 */ 5841 public StructureMapGroupRuleTargetParameterComponent setValue(Type value) { 5842 if (value != null && !(value instanceof IdType || value instanceof StringType || value instanceof BooleanType 5843 || value instanceof IntegerType || value instanceof DecimalType)) 5844 throw new Error( 5845 "Not the right type for StructureMap.group.rule.target.parameter.value[x]: " + value.fhirType()); 5846 this.value = value; 5847 return this; 5848 } 5849 5850 protected void listChildren(List<Property> children) { 5851 super.listChildren(children); 5852 children.add(new Property("value[x]", "id|string|boolean|integer|decimal", 5853 "Parameter value - variable or literal.", 0, 1, value)); 5854 } 5855 5856 @Override 5857 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5858 switch (_hash) { 5859 case -1410166417: 5860 /* value[x] */ return new Property("value[x]", "id|string|boolean|integer|decimal", 5861 "Parameter value - variable or literal.", 0, 1, value); 5862 case 111972721: 5863 /* value */ return new Property("value[x]", "id|string|boolean|integer|decimal", 5864 "Parameter value - variable or literal.", 0, 1, value); 5865 case 231604844: 5866 /* valueId */ return new Property("value[x]", "id|string|boolean|integer|decimal", 5867 "Parameter value - variable or literal.", 0, 1, value); 5868 case -1424603934: 5869 /* valueString */ return new Property("value[x]", "id|string|boolean|integer|decimal", 5870 "Parameter value - variable or literal.", 0, 1, value); 5871 case 733421943: 5872 /* valueBoolean */ return new Property("value[x]", "id|string|boolean|integer|decimal", 5873 "Parameter value - variable or literal.", 0, 1, value); 5874 case -1668204915: 5875 /* valueInteger */ return new Property("value[x]", "id|string|boolean|integer|decimal", 5876 "Parameter value - variable or literal.", 0, 1, value); 5877 case -2083993440: 5878 /* valueDecimal */ return new Property("value[x]", "id|string|boolean|integer|decimal", 5879 "Parameter value - variable or literal.", 0, 1, value); 5880 default: 5881 return super.getNamedProperty(_hash, _name, _checkValid); 5882 } 5883 5884 } 5885 5886 @Override 5887 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5888 switch (hash) { 5889 case 111972721: 5890 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 5891 default: 5892 return super.getProperty(hash, name, checkValid); 5893 } 5894 5895 } 5896 5897 @Override 5898 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5899 switch (hash) { 5900 case 111972721: // value 5901 this.value = castToType(value); // Type 5902 return value; 5903 default: 5904 return super.setProperty(hash, name, value); 5905 } 5906 5907 } 5908 5909 @Override 5910 public Base setProperty(String name, Base value) throws FHIRException { 5911 if (name.equals("value[x]")) { 5912 this.value = castToType(value); // Type 5913 } else 5914 return super.setProperty(name, value); 5915 return value; 5916 } 5917 5918 @Override 5919 public void removeChild(String name, Base value) throws FHIRException { 5920 if (name.equals("value[x]")) { 5921 this.value = null; 5922 } else 5923 super.removeChild(name, value); 5924 5925 } 5926 5927 @Override 5928 public Base makeProperty(int hash, String name) throws FHIRException { 5929 switch (hash) { 5930 case -1410166417: 5931 return getValue(); 5932 case 111972721: 5933 return getValue(); 5934 default: 5935 return super.makeProperty(hash, name); 5936 } 5937 5938 } 5939 5940 @Override 5941 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5942 switch (hash) { 5943 case 111972721: 5944 /* value */ return new String[] { "id", "string", "boolean", "integer", "decimal" }; 5945 default: 5946 return super.getTypesForProperty(hash, name); 5947 } 5948 5949 } 5950 5951 @Override 5952 public Base addChild(String name) throws FHIRException { 5953 if (name.equals("valueId")) { 5954 this.value = new IdType(); 5955 return this.value; 5956 } else if (name.equals("valueString")) { 5957 this.value = new StringType(); 5958 return this.value; 5959 } else if (name.equals("valueBoolean")) { 5960 this.value = new BooleanType(); 5961 return this.value; 5962 } else if (name.equals("valueInteger")) { 5963 this.value = new IntegerType(); 5964 return this.value; 5965 } else if (name.equals("valueDecimal")) { 5966 this.value = new DecimalType(); 5967 return this.value; 5968 } else 5969 return super.addChild(name); 5970 } 5971 5972 public StructureMapGroupRuleTargetParameterComponent copy() { 5973 StructureMapGroupRuleTargetParameterComponent dst = new StructureMapGroupRuleTargetParameterComponent(); 5974 copyValues(dst); 5975 return dst; 5976 } 5977 5978 public void copyValues(StructureMapGroupRuleTargetParameterComponent dst) { 5979 super.copyValues(dst); 5980 dst.value = value == null ? null : value.copy(); 5981 } 5982 5983 @Override 5984 public boolean equalsDeep(Base other_) { 5985 if (!super.equalsDeep(other_)) 5986 return false; 5987 if (!(other_ instanceof StructureMapGroupRuleTargetParameterComponent)) 5988 return false; 5989 StructureMapGroupRuleTargetParameterComponent o = (StructureMapGroupRuleTargetParameterComponent) other_; 5990 return compareDeep(value, o.value, true); 5991 } 5992 5993 @Override 5994 public boolean equalsShallow(Base other_) { 5995 if (!super.equalsShallow(other_)) 5996 return false; 5997 if (!(other_ instanceof StructureMapGroupRuleTargetParameterComponent)) 5998 return false; 5999 StructureMapGroupRuleTargetParameterComponent o = (StructureMapGroupRuleTargetParameterComponent) other_; 6000 return true; 6001 } 6002 6003 public boolean isEmpty() { 6004 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 6005 } 6006 6007 public String fhirType() { 6008 return "StructureMap.group.rule.target.parameter"; 6009 6010 } 6011 6012// added from java-adornments.txt: 6013 6014 public String toString() { 6015 return value == null ? "null!" : value.toString(); 6016 } 6017 6018// end addition 6019 } 6020 6021 @Block() 6022 public static class StructureMapGroupRuleDependentComponent extends BackboneElement implements IBaseBackboneElement { 6023 /** 6024 * Name of a rule or group to apply. 6025 */ 6026 @Child(name = "name", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 6027 @Description(shortDefinition = "Name of a rule or group to apply", formalDefinition = "Name of a rule or group to apply.") 6028 protected IdType name; 6029 6030 /** 6031 * Variable to pass to the rule or group. 6032 */ 6033 @Child(name = "variable", type = { 6034 StringType.class }, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6035 @Description(shortDefinition = "Variable to pass to the rule or group", formalDefinition = "Variable to pass to the rule or group.") 6036 protected List<StringType> variable; 6037 6038 private static final long serialVersionUID = 1021661591L; 6039 6040 /** 6041 * Constructor 6042 */ 6043 public StructureMapGroupRuleDependentComponent() { 6044 super(); 6045 } 6046 6047 /** 6048 * Constructor 6049 */ 6050 public StructureMapGroupRuleDependentComponent(IdType name) { 6051 super(); 6052 this.name = name; 6053 } 6054 6055 /** 6056 * @return {@link #name} (Name of a rule or group to apply.). This is the 6057 * underlying object with id, value and extensions. The accessor 6058 * "getName" gives direct access to the value 6059 */ 6060 public IdType getNameElement() { 6061 if (this.name == null) 6062 if (Configuration.errorOnAutoCreate()) 6063 throw new Error("Attempt to auto-create StructureMapGroupRuleDependentComponent.name"); 6064 else if (Configuration.doAutoCreate()) 6065 this.name = new IdType(); // bb 6066 return this.name; 6067 } 6068 6069 public boolean hasNameElement() { 6070 return this.name != null && !this.name.isEmpty(); 6071 } 6072 6073 public boolean hasName() { 6074 return this.name != null && !this.name.isEmpty(); 6075 } 6076 6077 /** 6078 * @param value {@link #name} (Name of a rule or group to apply.). This is the 6079 * underlying object with id, value and extensions. The accessor 6080 * "getName" gives direct access to the value 6081 */ 6082 public StructureMapGroupRuleDependentComponent setNameElement(IdType value) { 6083 this.name = value; 6084 return this; 6085 } 6086 6087 /** 6088 * @return Name of a rule or group to apply. 6089 */ 6090 public String getName() { 6091 return this.name == null ? null : this.name.getValue(); 6092 } 6093 6094 /** 6095 * @param value Name of a rule or group to apply. 6096 */ 6097 public StructureMapGroupRuleDependentComponent setName(String value) { 6098 if (this.name == null) 6099 this.name = new IdType(); 6100 this.name.setValue(value); 6101 return this; 6102 } 6103 6104 /** 6105 * @return {@link #variable} (Variable to pass to the rule or group.) 6106 */ 6107 public List<StringType> getVariable() { 6108 if (this.variable == null) 6109 this.variable = new ArrayList<StringType>(); 6110 return this.variable; 6111 } 6112 6113 /** 6114 * @return Returns a reference to <code>this</code> for easy method chaining 6115 */ 6116 public StructureMapGroupRuleDependentComponent setVariable(List<StringType> theVariable) { 6117 this.variable = theVariable; 6118 return this; 6119 } 6120 6121 public boolean hasVariable() { 6122 if (this.variable == null) 6123 return false; 6124 for (StringType item : this.variable) 6125 if (!item.isEmpty()) 6126 return true; 6127 return false; 6128 } 6129 6130 /** 6131 * @return {@link #variable} (Variable to pass to the rule or group.) 6132 */ 6133 public StringType addVariableElement() {// 2 6134 StringType t = new StringType(); 6135 if (this.variable == null) 6136 this.variable = new ArrayList<StringType>(); 6137 this.variable.add(t); 6138 return t; 6139 } 6140 6141 /** 6142 * @param value {@link #variable} (Variable to pass to the rule or group.) 6143 */ 6144 public StructureMapGroupRuleDependentComponent addVariable(String value) { // 1 6145 StringType t = new StringType(); 6146 t.setValue(value); 6147 if (this.variable == null) 6148 this.variable = new ArrayList<StringType>(); 6149 this.variable.add(t); 6150 return this; 6151 } 6152 6153 /** 6154 * @param value {@link #variable} (Variable to pass to the rule or group.) 6155 */ 6156 public boolean hasVariable(String value) { 6157 if (this.variable == null) 6158 return false; 6159 for (StringType v : this.variable) 6160 if (v.getValue().equals(value)) // string 6161 return true; 6162 return false; 6163 } 6164 6165 protected void listChildren(List<Property> children) { 6166 super.listChildren(children); 6167 children.add(new Property("name", "id", "Name of a rule or group to apply.", 0, 1, name)); 6168 children.add(new Property("variable", "string", "Variable to pass to the rule or group.", 0, 6169 java.lang.Integer.MAX_VALUE, variable)); 6170 } 6171 6172 @Override 6173 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6174 switch (_hash) { 6175 case 3373707: 6176 /* name */ return new Property("name", "id", "Name of a rule or group to apply.", 0, 1, name); 6177 case -1249586564: 6178 /* variable */ return new Property("variable", "string", "Variable to pass to the rule or group.", 0, 6179 java.lang.Integer.MAX_VALUE, variable); 6180 default: 6181 return super.getNamedProperty(_hash, _name, _checkValid); 6182 } 6183 6184 } 6185 6186 @Override 6187 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6188 switch (hash) { 6189 case 3373707: 6190 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // IdType 6191 case -1249586564: 6192 /* variable */ return this.variable == null ? new Base[0] 6193 : this.variable.toArray(new Base[this.variable.size()]); // StringType 6194 default: 6195 return super.getProperty(hash, name, checkValid); 6196 } 6197 6198 } 6199 6200 @Override 6201 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6202 switch (hash) { 6203 case 3373707: // name 6204 this.name = castToId(value); // IdType 6205 return value; 6206 case -1249586564: // variable 6207 this.getVariable().add(castToString(value)); // StringType 6208 return value; 6209 default: 6210 return super.setProperty(hash, name, value); 6211 } 6212 6213 } 6214 6215 @Override 6216 public Base setProperty(String name, Base value) throws FHIRException { 6217 if (name.equals("name")) { 6218 this.name = castToId(value); // IdType 6219 } else if (name.equals("variable")) { 6220 this.getVariable().add(castToString(value)); 6221 } else 6222 return super.setProperty(name, value); 6223 return value; 6224 } 6225 6226 @Override 6227 public void removeChild(String name, Base value) throws FHIRException { 6228 if (name.equals("name")) { 6229 this.name = null; 6230 } else if (name.equals("variable")) { 6231 this.getVariable().remove(castToString(value)); 6232 } else 6233 super.removeChild(name, value); 6234 6235 } 6236 6237 @Override 6238 public Base makeProperty(int hash, String name) throws FHIRException { 6239 switch (hash) { 6240 case 3373707: 6241 return getNameElement(); 6242 case -1249586564: 6243 return addVariableElement(); 6244 default: 6245 return super.makeProperty(hash, name); 6246 } 6247 6248 } 6249 6250 @Override 6251 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6252 switch (hash) { 6253 case 3373707: 6254 /* name */ return new String[] { "id" }; 6255 case -1249586564: 6256 /* variable */ return new String[] { "string" }; 6257 default: 6258 return super.getTypesForProperty(hash, name); 6259 } 6260 6261 } 6262 6263 @Override 6264 public Base addChild(String name) throws FHIRException { 6265 if (name.equals("name")) { 6266 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 6267 } else if (name.equals("variable")) { 6268 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.variable"); 6269 } else 6270 return super.addChild(name); 6271 } 6272 6273 public StructureMapGroupRuleDependentComponent copy() { 6274 StructureMapGroupRuleDependentComponent dst = new StructureMapGroupRuleDependentComponent(); 6275 copyValues(dst); 6276 return dst; 6277 } 6278 6279 public void copyValues(StructureMapGroupRuleDependentComponent dst) { 6280 super.copyValues(dst); 6281 dst.name = name == null ? null : name.copy(); 6282 if (variable != null) { 6283 dst.variable = new ArrayList<StringType>(); 6284 for (StringType i : variable) 6285 dst.variable.add(i.copy()); 6286 } 6287 ; 6288 } 6289 6290 @Override 6291 public boolean equalsDeep(Base other_) { 6292 if (!super.equalsDeep(other_)) 6293 return false; 6294 if (!(other_ instanceof StructureMapGroupRuleDependentComponent)) 6295 return false; 6296 StructureMapGroupRuleDependentComponent o = (StructureMapGroupRuleDependentComponent) other_; 6297 return compareDeep(name, o.name, true) && compareDeep(variable, o.variable, true); 6298 } 6299 6300 @Override 6301 public boolean equalsShallow(Base other_) { 6302 if (!super.equalsShallow(other_)) 6303 return false; 6304 if (!(other_ instanceof StructureMapGroupRuleDependentComponent)) 6305 return false; 6306 StructureMapGroupRuleDependentComponent o = (StructureMapGroupRuleDependentComponent) other_; 6307 return compareValues(name, o.name, true) && compareValues(variable, o.variable, true); 6308 } 6309 6310 public boolean isEmpty() { 6311 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, variable); 6312 } 6313 6314 public String fhirType() { 6315 return "StructureMap.group.rule.dependent"; 6316 6317 } 6318 6319 } 6320 6321 /** 6322 * A formal identifier that is used to identify this structure map when it is 6323 * represented in other formats, or referenced in a specification, model, design 6324 * or an instance. 6325 */ 6326 @Child(name = "identifier", type = { 6327 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6328 @Description(shortDefinition = "Additional identifier for the structure map", formalDefinition = "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.") 6329 protected List<Identifier> identifier; 6330 6331 /** 6332 * Explanation of why this structure map is needed and why it has been designed 6333 * as it has. 6334 */ 6335 @Child(name = "purpose", type = { 6336 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6337 @Description(shortDefinition = "Why this structure map is defined", formalDefinition = "Explanation of why this structure map is needed and why it has been designed as it has.") 6338 protected MarkdownType purpose; 6339 6340 /** 6341 * A copyright statement relating to the structure map and/or its contents. 6342 * Copyright statements are generally legal restrictions on the use and 6343 * publishing of the structure map. 6344 */ 6345 @Child(name = "copyright", type = { 6346 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6347 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.") 6348 protected MarkdownType copyright; 6349 6350 /** 6351 * A structure definition used by this map. The structure definition may 6352 * describe instances that are converted, or the instances that are produced. 6353 */ 6354 @Child(name = "structure", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6355 @Description(shortDefinition = "Structure Definition used by this map", formalDefinition = "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.") 6356 protected List<StructureMapStructureComponent> structure; 6357 6358 /** 6359 * Other maps used by this map (canonical URLs). 6360 */ 6361 @Child(name = "import", type = { 6362 CanonicalType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6363 @Description(shortDefinition = "Other maps used by this map (canonical URLs)", formalDefinition = "Other maps used by this map (canonical URLs).") 6364 protected List<CanonicalType> import_; 6365 6366 /** 6367 * Organizes the mapping into manageable chunks for human review/ease of 6368 * maintenance. 6369 */ 6370 @Child(name = "group", type = {}, order = 5, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6371 @Description(shortDefinition = "Named sections for reader convenience", formalDefinition = "Organizes the mapping into manageable chunks for human review/ease of maintenance.") 6372 protected List<StructureMapGroupComponent> group; 6373 6374 private static final long serialVersionUID = 263060597L; 6375 6376 /** 6377 * Constructor 6378 */ 6379 public StructureMap() { 6380 super(); 6381 } 6382 6383 /** 6384 * Constructor 6385 */ 6386 public StructureMap(UriType url, StringType name, Enumeration<PublicationStatus> status) { 6387 super(); 6388 this.url = url; 6389 this.name = name; 6390 this.status = status; 6391 } 6392 6393 /** 6394 * @return {@link #url} (An absolute URI that is used to identify this structure 6395 * map when it is referenced in a specification, model, design or an 6396 * instance; also called its canonical identifier. This SHOULD be 6397 * globally unique and SHOULD be a literal address at which at which an 6398 * authoritative instance of this structure map is (or will be) 6399 * published. This URL can be the target of a canonical reference. It 6400 * SHALL remain the same when the structure map is stored on different 6401 * servers.). This is the underlying object with id, value and 6402 * extensions. The accessor "getUrl" gives direct access to the value 6403 */ 6404 public UriType getUrlElement() { 6405 if (this.url == null) 6406 if (Configuration.errorOnAutoCreate()) 6407 throw new Error("Attempt to auto-create StructureMap.url"); 6408 else if (Configuration.doAutoCreate()) 6409 this.url = new UriType(); // bb 6410 return this.url; 6411 } 6412 6413 public boolean hasUrlElement() { 6414 return this.url != null && !this.url.isEmpty(); 6415 } 6416 6417 public boolean hasUrl() { 6418 return this.url != null && !this.url.isEmpty(); 6419 } 6420 6421 /** 6422 * @param value {@link #url} (An absolute URI that is used to identify this 6423 * structure map when it is referenced in a specification, model, 6424 * design or an instance; also called its canonical identifier. 6425 * This SHOULD be globally unique and SHOULD be a literal address 6426 * at which at which an authoritative instance of this structure 6427 * map is (or will be) published. This URL can be the target of a 6428 * canonical reference. It SHALL remain the same when the structure 6429 * map is stored on different servers.). This is the underlying 6430 * object with id, value and extensions. The accessor "getUrl" 6431 * gives direct access to the value 6432 */ 6433 public StructureMap setUrlElement(UriType value) { 6434 this.url = value; 6435 return this; 6436 } 6437 6438 /** 6439 * @return An absolute URI that is used to identify this structure map when it 6440 * is referenced in a specification, model, design or an instance; also 6441 * called its canonical identifier. This SHOULD be globally unique and 6442 * SHOULD be a literal address at which at which an authoritative 6443 * instance of this structure map is (or will be) published. This URL 6444 * can be the target of a canonical reference. It SHALL remain the same 6445 * when the structure map is stored on different servers. 6446 */ 6447 public String getUrl() { 6448 return this.url == null ? null : this.url.getValue(); 6449 } 6450 6451 /** 6452 * @param value An absolute URI that is used to identify this structure map when 6453 * it is referenced in a specification, model, design or an 6454 * instance; also called its canonical identifier. This SHOULD be 6455 * globally unique and SHOULD be a literal address at which at 6456 * which an authoritative instance of this structure map is (or 6457 * will be) published. This URL can be the target of a canonical 6458 * reference. It SHALL remain the same when the structure map is 6459 * stored on different servers. 6460 */ 6461 public StructureMap setUrl(String value) { 6462 if (this.url == null) 6463 this.url = new UriType(); 6464 this.url.setValue(value); 6465 return this; 6466 } 6467 6468 /** 6469 * @return {@link #identifier} (A formal identifier that is used to identify 6470 * this structure map when it is represented in other formats, or 6471 * referenced in a specification, model, design or an instance.) 6472 */ 6473 public List<Identifier> getIdentifier() { 6474 if (this.identifier == null) 6475 this.identifier = new ArrayList<Identifier>(); 6476 return this.identifier; 6477 } 6478 6479 /** 6480 * @return Returns a reference to <code>this</code> for easy method chaining 6481 */ 6482 public StructureMap setIdentifier(List<Identifier> theIdentifier) { 6483 this.identifier = theIdentifier; 6484 return this; 6485 } 6486 6487 public boolean hasIdentifier() { 6488 if (this.identifier == null) 6489 return false; 6490 for (Identifier item : this.identifier) 6491 if (!item.isEmpty()) 6492 return true; 6493 return false; 6494 } 6495 6496 public Identifier addIdentifier() { // 3 6497 Identifier t = new Identifier(); 6498 if (this.identifier == null) 6499 this.identifier = new ArrayList<Identifier>(); 6500 this.identifier.add(t); 6501 return t; 6502 } 6503 6504 public StructureMap addIdentifier(Identifier t) { // 3 6505 if (t == null) 6506 return this; 6507 if (this.identifier == null) 6508 this.identifier = new ArrayList<Identifier>(); 6509 this.identifier.add(t); 6510 return this; 6511 } 6512 6513 /** 6514 * @return The first repetition of repeating field {@link #identifier}, creating 6515 * it if it does not already exist 6516 */ 6517 public Identifier getIdentifierFirstRep() { 6518 if (getIdentifier().isEmpty()) { 6519 addIdentifier(); 6520 } 6521 return getIdentifier().get(0); 6522 } 6523 6524 /** 6525 * @return {@link #version} (The identifier that is used to identify this 6526 * version of the structure map when it is referenced in a 6527 * specification, model, design or instance. This is an arbitrary value 6528 * managed by the structure map author and is not expected to be 6529 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 6530 * if a managed version is not available. There is also no expectation 6531 * that versions can be placed in a lexicographical sequence.). This is 6532 * the underlying object with id, value and extensions. The accessor 6533 * "getVersion" gives direct access to the value 6534 */ 6535 public StringType getVersionElement() { 6536 if (this.version == null) 6537 if (Configuration.errorOnAutoCreate()) 6538 throw new Error("Attempt to auto-create StructureMap.version"); 6539 else if (Configuration.doAutoCreate()) 6540 this.version = new StringType(); // bb 6541 return this.version; 6542 } 6543 6544 public boolean hasVersionElement() { 6545 return this.version != null && !this.version.isEmpty(); 6546 } 6547 6548 public boolean hasVersion() { 6549 return this.version != null && !this.version.isEmpty(); 6550 } 6551 6552 /** 6553 * @param value {@link #version} (The identifier that is used to identify this 6554 * version of the structure map when it is referenced in a 6555 * specification, model, design or instance. This is an arbitrary 6556 * value managed by the structure map author and is not expected to 6557 * be globally unique. For example, it might be a timestamp (e.g. 6558 * yyyymmdd) if a managed version is not available. There is also 6559 * no expectation that versions can be placed in a lexicographical 6560 * sequence.). This is the underlying object with id, value and 6561 * extensions. The accessor "getVersion" gives direct access to the 6562 * value 6563 */ 6564 public StructureMap setVersionElement(StringType value) { 6565 this.version = value; 6566 return this; 6567 } 6568 6569 /** 6570 * @return The identifier that is used to identify this version of the structure 6571 * map when it is referenced in a specification, model, design or 6572 * instance. This is an arbitrary value managed by the structure map 6573 * author and is not expected to be globally unique. For example, it 6574 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 6575 * available. There is also no expectation that versions can be placed 6576 * in a lexicographical sequence. 6577 */ 6578 public String getVersion() { 6579 return this.version == null ? null : this.version.getValue(); 6580 } 6581 6582 /** 6583 * @param value The identifier that is used to identify this version of the 6584 * structure map when it is referenced in a specification, model, 6585 * design or instance. This is an arbitrary value managed by the 6586 * structure map author and is not expected to be globally unique. 6587 * For example, it might be a timestamp (e.g. yyyymmdd) if a 6588 * managed version is not available. There is also no expectation 6589 * that versions can be placed in a lexicographical sequence. 6590 */ 6591 public StructureMap setVersion(String value) { 6592 if (Utilities.noString(value)) 6593 this.version = null; 6594 else { 6595 if (this.version == null) 6596 this.version = new StringType(); 6597 this.version.setValue(value); 6598 } 6599 return this; 6600 } 6601 6602 /** 6603 * @return {@link #name} (A natural language name identifying the structure map. 6604 * This name should be usable as an identifier for the module by machine 6605 * processing applications such as code generation.). This is the 6606 * underlying object with id, value and extensions. The accessor 6607 * "getName" gives direct access to the value 6608 */ 6609 public StringType getNameElement() { 6610 if (this.name == null) 6611 if (Configuration.errorOnAutoCreate()) 6612 throw new Error("Attempt to auto-create StructureMap.name"); 6613 else if (Configuration.doAutoCreate()) 6614 this.name = new StringType(); // bb 6615 return this.name; 6616 } 6617 6618 public boolean hasNameElement() { 6619 return this.name != null && !this.name.isEmpty(); 6620 } 6621 6622 public boolean hasName() { 6623 return this.name != null && !this.name.isEmpty(); 6624 } 6625 6626 /** 6627 * @param value {@link #name} (A natural language name identifying the structure 6628 * map. This name should be usable as an identifier for the module 6629 * by machine processing applications such as code generation.). 6630 * This is the underlying object with id, value and extensions. The 6631 * accessor "getName" gives direct access to the value 6632 */ 6633 public StructureMap setNameElement(StringType value) { 6634 this.name = value; 6635 return this; 6636 } 6637 6638 /** 6639 * @return A natural language name identifying the structure map. This name 6640 * should be usable as an identifier for the module by machine 6641 * processing applications such as code generation. 6642 */ 6643 public String getName() { 6644 return this.name == null ? null : this.name.getValue(); 6645 } 6646 6647 /** 6648 * @param value A natural language name identifying the structure map. This name 6649 * should be usable as an identifier for the module by machine 6650 * processing applications such as code generation. 6651 */ 6652 public StructureMap setName(String value) { 6653 if (this.name == null) 6654 this.name = new StringType(); 6655 this.name.setValue(value); 6656 return this; 6657 } 6658 6659 /** 6660 * @return {@link #title} (A short, descriptive, user-friendly title for the 6661 * structure map.). This is the underlying object with id, value and 6662 * extensions. The accessor "getTitle" gives direct access to the value 6663 */ 6664 public StringType getTitleElement() { 6665 if (this.title == null) 6666 if (Configuration.errorOnAutoCreate()) 6667 throw new Error("Attempt to auto-create StructureMap.title"); 6668 else if (Configuration.doAutoCreate()) 6669 this.title = new StringType(); // bb 6670 return this.title; 6671 } 6672 6673 public boolean hasTitleElement() { 6674 return this.title != null && !this.title.isEmpty(); 6675 } 6676 6677 public boolean hasTitle() { 6678 return this.title != null && !this.title.isEmpty(); 6679 } 6680 6681 /** 6682 * @param value {@link #title} (A short, descriptive, user-friendly title for 6683 * the structure map.). This is the underlying object with id, 6684 * value and extensions. The accessor "getTitle" gives direct 6685 * access to the value 6686 */ 6687 public StructureMap setTitleElement(StringType value) { 6688 this.title = value; 6689 return this; 6690 } 6691 6692 /** 6693 * @return A short, descriptive, user-friendly title for the structure map. 6694 */ 6695 public String getTitle() { 6696 return this.title == null ? null : this.title.getValue(); 6697 } 6698 6699 /** 6700 * @param value A short, descriptive, user-friendly title for the structure map. 6701 */ 6702 public StructureMap setTitle(String value) { 6703 if (Utilities.noString(value)) 6704 this.title = null; 6705 else { 6706 if (this.title == null) 6707 this.title = new StringType(); 6708 this.title.setValue(value); 6709 } 6710 return this; 6711 } 6712 6713 /** 6714 * @return {@link #status} (The status of this structure map. Enables tracking 6715 * the life-cycle of the content.). This is the underlying object with 6716 * id, value and extensions. The accessor "getStatus" gives direct 6717 * access to the value 6718 */ 6719 public Enumeration<PublicationStatus> getStatusElement() { 6720 if (this.status == null) 6721 if (Configuration.errorOnAutoCreate()) 6722 throw new Error("Attempt to auto-create StructureMap.status"); 6723 else if (Configuration.doAutoCreate()) 6724 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 6725 return this.status; 6726 } 6727 6728 public boolean hasStatusElement() { 6729 return this.status != null && !this.status.isEmpty(); 6730 } 6731 6732 public boolean hasStatus() { 6733 return this.status != null && !this.status.isEmpty(); 6734 } 6735 6736 /** 6737 * @param value {@link #status} (The status of this structure map. Enables 6738 * tracking the life-cycle of the content.). This is the underlying 6739 * object with id, value and extensions. The accessor "getStatus" 6740 * gives direct access to the value 6741 */ 6742 public StructureMap setStatusElement(Enumeration<PublicationStatus> value) { 6743 this.status = value; 6744 return this; 6745 } 6746 6747 /** 6748 * @return The status of this structure map. Enables tracking the life-cycle of 6749 * the content. 6750 */ 6751 public PublicationStatus getStatus() { 6752 return this.status == null ? null : this.status.getValue(); 6753 } 6754 6755 /** 6756 * @param value The status of this structure map. Enables tracking the 6757 * life-cycle of the content. 6758 */ 6759 public StructureMap setStatus(PublicationStatus value) { 6760 if (this.status == null) 6761 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 6762 this.status.setValue(value); 6763 return this; 6764 } 6765 6766 /** 6767 * @return {@link #experimental} (A Boolean value to indicate that this 6768 * structure map is authored for testing purposes (or 6769 * education/evaluation/marketing) and is not intended to be used for 6770 * genuine usage.). This is the underlying object with id, value and 6771 * extensions. The accessor "getExperimental" gives direct access to the 6772 * value 6773 */ 6774 public BooleanType getExperimentalElement() { 6775 if (this.experimental == null) 6776 if (Configuration.errorOnAutoCreate()) 6777 throw new Error("Attempt to auto-create StructureMap.experimental"); 6778 else if (Configuration.doAutoCreate()) 6779 this.experimental = new BooleanType(); // bb 6780 return this.experimental; 6781 } 6782 6783 public boolean hasExperimentalElement() { 6784 return this.experimental != null && !this.experimental.isEmpty(); 6785 } 6786 6787 public boolean hasExperimental() { 6788 return this.experimental != null && !this.experimental.isEmpty(); 6789 } 6790 6791 /** 6792 * @param value {@link #experimental} (A Boolean value to indicate that this 6793 * structure map is authored for testing purposes (or 6794 * education/evaluation/marketing) and is not intended to be used 6795 * for genuine usage.). This is the underlying object with id, 6796 * value and extensions. The accessor "getExperimental" gives 6797 * direct access to the value 6798 */ 6799 public StructureMap setExperimentalElement(BooleanType value) { 6800 this.experimental = value; 6801 return this; 6802 } 6803 6804 /** 6805 * @return A Boolean value to indicate that this structure map is authored for 6806 * testing purposes (or education/evaluation/marketing) and is not 6807 * intended to be used for genuine usage. 6808 */ 6809 public boolean getExperimental() { 6810 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 6811 } 6812 6813 /** 6814 * @param value A Boolean value to indicate that this structure map is authored 6815 * for testing purposes (or education/evaluation/marketing) and is 6816 * not intended to be used for genuine usage. 6817 */ 6818 public StructureMap setExperimental(boolean value) { 6819 if (this.experimental == null) 6820 this.experimental = new BooleanType(); 6821 this.experimental.setValue(value); 6822 return this; 6823 } 6824 6825 /** 6826 * @return {@link #date} (The date (and optionally time) when the structure map 6827 * was published. The date must change when the business version changes 6828 * and it must change if the status code changes. In addition, it should 6829 * change when the substantive content of the structure map changes.). 6830 * This is the underlying object with id, value and extensions. The 6831 * accessor "getDate" gives direct access to the value 6832 */ 6833 public DateTimeType getDateElement() { 6834 if (this.date == null) 6835 if (Configuration.errorOnAutoCreate()) 6836 throw new Error("Attempt to auto-create StructureMap.date"); 6837 else if (Configuration.doAutoCreate()) 6838 this.date = new DateTimeType(); // bb 6839 return this.date; 6840 } 6841 6842 public boolean hasDateElement() { 6843 return this.date != null && !this.date.isEmpty(); 6844 } 6845 6846 public boolean hasDate() { 6847 return this.date != null && !this.date.isEmpty(); 6848 } 6849 6850 /** 6851 * @param value {@link #date} (The date (and optionally time) when the structure 6852 * map was published. The date must change when the business 6853 * version changes and it must change if the status code changes. 6854 * In addition, it should change when the substantive content of 6855 * the structure map changes.). This is the underlying object with 6856 * id, value and extensions. The accessor "getDate" gives direct 6857 * access to the value 6858 */ 6859 public StructureMap setDateElement(DateTimeType value) { 6860 this.date = value; 6861 return this; 6862 } 6863 6864 /** 6865 * @return The date (and optionally time) when the structure map was published. 6866 * The date must change when the business version changes and it must 6867 * change if the status code changes. In addition, it should change when 6868 * the substantive content of the structure map changes. 6869 */ 6870 public Date getDate() { 6871 return this.date == null ? null : this.date.getValue(); 6872 } 6873 6874 /** 6875 * @param value The date (and optionally time) when the structure map was 6876 * published. The date must change when the business version 6877 * changes and it must change if the status code changes. In 6878 * addition, it should change when the substantive content of the 6879 * structure map changes. 6880 */ 6881 public StructureMap setDate(Date value) { 6882 if (value == null) 6883 this.date = null; 6884 else { 6885 if (this.date == null) 6886 this.date = new DateTimeType(); 6887 this.date.setValue(value); 6888 } 6889 return this; 6890 } 6891 6892 /** 6893 * @return {@link #publisher} (The name of the organization or individual that 6894 * published the structure map.). This is the underlying object with id, 6895 * value and extensions. The accessor "getPublisher" gives direct access 6896 * to the value 6897 */ 6898 public StringType getPublisherElement() { 6899 if (this.publisher == null) 6900 if (Configuration.errorOnAutoCreate()) 6901 throw new Error("Attempt to auto-create StructureMap.publisher"); 6902 else if (Configuration.doAutoCreate()) 6903 this.publisher = new StringType(); // bb 6904 return this.publisher; 6905 } 6906 6907 public boolean hasPublisherElement() { 6908 return this.publisher != null && !this.publisher.isEmpty(); 6909 } 6910 6911 public boolean hasPublisher() { 6912 return this.publisher != null && !this.publisher.isEmpty(); 6913 } 6914 6915 /** 6916 * @param value {@link #publisher} (The name of the organization or individual 6917 * that published the structure map.). This is the underlying 6918 * object with id, value and extensions. The accessor 6919 * "getPublisher" gives direct access to the value 6920 */ 6921 public StructureMap setPublisherElement(StringType value) { 6922 this.publisher = value; 6923 return this; 6924 } 6925 6926 /** 6927 * @return The name of the organization or individual that published the 6928 * structure map. 6929 */ 6930 public String getPublisher() { 6931 return this.publisher == null ? null : this.publisher.getValue(); 6932 } 6933 6934 /** 6935 * @param value The name of the organization or individual that published the 6936 * structure map. 6937 */ 6938 public StructureMap setPublisher(String value) { 6939 if (Utilities.noString(value)) 6940 this.publisher = null; 6941 else { 6942 if (this.publisher == null) 6943 this.publisher = new StringType(); 6944 this.publisher.setValue(value); 6945 } 6946 return this; 6947 } 6948 6949 /** 6950 * @return {@link #contact} (Contact details to assist a user in finding and 6951 * communicating with the publisher.) 6952 */ 6953 public List<ContactDetail> getContact() { 6954 if (this.contact == null) 6955 this.contact = new ArrayList<ContactDetail>(); 6956 return this.contact; 6957 } 6958 6959 /** 6960 * @return Returns a reference to <code>this</code> for easy method chaining 6961 */ 6962 public StructureMap setContact(List<ContactDetail> theContact) { 6963 this.contact = theContact; 6964 return this; 6965 } 6966 6967 public boolean hasContact() { 6968 if (this.contact == null) 6969 return false; 6970 for (ContactDetail item : this.contact) 6971 if (!item.isEmpty()) 6972 return true; 6973 return false; 6974 } 6975 6976 public ContactDetail addContact() { // 3 6977 ContactDetail t = new ContactDetail(); 6978 if (this.contact == null) 6979 this.contact = new ArrayList<ContactDetail>(); 6980 this.contact.add(t); 6981 return t; 6982 } 6983 6984 public StructureMap addContact(ContactDetail t) { // 3 6985 if (t == null) 6986 return this; 6987 if (this.contact == null) 6988 this.contact = new ArrayList<ContactDetail>(); 6989 this.contact.add(t); 6990 return this; 6991 } 6992 6993 /** 6994 * @return The first repetition of repeating field {@link #contact}, creating it 6995 * if it does not already exist 6996 */ 6997 public ContactDetail getContactFirstRep() { 6998 if (getContact().isEmpty()) { 6999 addContact(); 7000 } 7001 return getContact().get(0); 7002 } 7003 7004 /** 7005 * @return {@link #description} (A free text natural language description of the 7006 * structure map from a consumer's perspective.). This is the underlying 7007 * object with id, value and extensions. The accessor "getDescription" 7008 * gives direct access to the value 7009 */ 7010 public MarkdownType getDescriptionElement() { 7011 if (this.description == null) 7012 if (Configuration.errorOnAutoCreate()) 7013 throw new Error("Attempt to auto-create StructureMap.description"); 7014 else if (Configuration.doAutoCreate()) 7015 this.description = new MarkdownType(); // bb 7016 return this.description; 7017 } 7018 7019 public boolean hasDescriptionElement() { 7020 return this.description != null && !this.description.isEmpty(); 7021 } 7022 7023 public boolean hasDescription() { 7024 return this.description != null && !this.description.isEmpty(); 7025 } 7026 7027 /** 7028 * @param value {@link #description} (A free text natural language description 7029 * of the structure map from a consumer's perspective.). This is 7030 * the underlying object with id, value and extensions. The 7031 * accessor "getDescription" gives direct access to the value 7032 */ 7033 public StructureMap setDescriptionElement(MarkdownType value) { 7034 this.description = value; 7035 return this; 7036 } 7037 7038 /** 7039 * @return A free text natural language description of the structure map from a 7040 * consumer's perspective. 7041 */ 7042 public String getDescription() { 7043 return this.description == null ? null : this.description.getValue(); 7044 } 7045 7046 /** 7047 * @param value A free text natural language description of the structure map 7048 * from a consumer's perspective. 7049 */ 7050 public StructureMap setDescription(String value) { 7051 if (value == null) 7052 this.description = null; 7053 else { 7054 if (this.description == null) 7055 this.description = new MarkdownType(); 7056 this.description.setValue(value); 7057 } 7058 return this; 7059 } 7060 7061 /** 7062 * @return {@link #useContext} (The content was developed with a focus and 7063 * intent of supporting the contexts that are listed. These contexts may 7064 * be general categories (gender, age, ...) or may be references to 7065 * specific programs (insurance plans, studies, ...) and may be used to 7066 * assist with indexing and searching for appropriate structure map 7067 * instances.) 7068 */ 7069 public List<UsageContext> getUseContext() { 7070 if (this.useContext == null) 7071 this.useContext = new ArrayList<UsageContext>(); 7072 return this.useContext; 7073 } 7074 7075 /** 7076 * @return Returns a reference to <code>this</code> for easy method chaining 7077 */ 7078 public StructureMap setUseContext(List<UsageContext> theUseContext) { 7079 this.useContext = theUseContext; 7080 return this; 7081 } 7082 7083 public boolean hasUseContext() { 7084 if (this.useContext == null) 7085 return false; 7086 for (UsageContext item : this.useContext) 7087 if (!item.isEmpty()) 7088 return true; 7089 return false; 7090 } 7091 7092 public UsageContext addUseContext() { // 3 7093 UsageContext t = new UsageContext(); 7094 if (this.useContext == null) 7095 this.useContext = new ArrayList<UsageContext>(); 7096 this.useContext.add(t); 7097 return t; 7098 } 7099 7100 public StructureMap addUseContext(UsageContext t) { // 3 7101 if (t == null) 7102 return this; 7103 if (this.useContext == null) 7104 this.useContext = new ArrayList<UsageContext>(); 7105 this.useContext.add(t); 7106 return this; 7107 } 7108 7109 /** 7110 * @return The first repetition of repeating field {@link #useContext}, creating 7111 * it if it does not already exist 7112 */ 7113 public UsageContext getUseContextFirstRep() { 7114 if (getUseContext().isEmpty()) { 7115 addUseContext(); 7116 } 7117 return getUseContext().get(0); 7118 } 7119 7120 /** 7121 * @return {@link #jurisdiction} (A legal or geographic region in which the 7122 * structure map is intended to be used.) 7123 */ 7124 public List<CodeableConcept> getJurisdiction() { 7125 if (this.jurisdiction == null) 7126 this.jurisdiction = new ArrayList<CodeableConcept>(); 7127 return this.jurisdiction; 7128 } 7129 7130 /** 7131 * @return Returns a reference to <code>this</code> for easy method chaining 7132 */ 7133 public StructureMap setJurisdiction(List<CodeableConcept> theJurisdiction) { 7134 this.jurisdiction = theJurisdiction; 7135 return this; 7136 } 7137 7138 public boolean hasJurisdiction() { 7139 if (this.jurisdiction == null) 7140 return false; 7141 for (CodeableConcept item : this.jurisdiction) 7142 if (!item.isEmpty()) 7143 return true; 7144 return false; 7145 } 7146 7147 public CodeableConcept addJurisdiction() { // 3 7148 CodeableConcept t = new CodeableConcept(); 7149 if (this.jurisdiction == null) 7150 this.jurisdiction = new ArrayList<CodeableConcept>(); 7151 this.jurisdiction.add(t); 7152 return t; 7153 } 7154 7155 public StructureMap addJurisdiction(CodeableConcept t) { // 3 7156 if (t == null) 7157 return this; 7158 if (this.jurisdiction == null) 7159 this.jurisdiction = new ArrayList<CodeableConcept>(); 7160 this.jurisdiction.add(t); 7161 return this; 7162 } 7163 7164 /** 7165 * @return The first repetition of repeating field {@link #jurisdiction}, 7166 * creating it if it does not already exist 7167 */ 7168 public CodeableConcept getJurisdictionFirstRep() { 7169 if (getJurisdiction().isEmpty()) { 7170 addJurisdiction(); 7171 } 7172 return getJurisdiction().get(0); 7173 } 7174 7175 /** 7176 * @return {@link #purpose} (Explanation of why this structure map is needed and 7177 * why it has been designed as it has.). This is the underlying object 7178 * with id, value and extensions. The accessor "getPurpose" gives direct 7179 * access to the value 7180 */ 7181 public MarkdownType getPurposeElement() { 7182 if (this.purpose == null) 7183 if (Configuration.errorOnAutoCreate()) 7184 throw new Error("Attempt to auto-create StructureMap.purpose"); 7185 else if (Configuration.doAutoCreate()) 7186 this.purpose = new MarkdownType(); // bb 7187 return this.purpose; 7188 } 7189 7190 public boolean hasPurposeElement() { 7191 return this.purpose != null && !this.purpose.isEmpty(); 7192 } 7193 7194 public boolean hasPurpose() { 7195 return this.purpose != null && !this.purpose.isEmpty(); 7196 } 7197 7198 /** 7199 * @param value {@link #purpose} (Explanation of why this structure map is 7200 * needed and why it has been designed as it has.). This is the 7201 * underlying object with id, value and extensions. The accessor 7202 * "getPurpose" gives direct access to the value 7203 */ 7204 public StructureMap setPurposeElement(MarkdownType value) { 7205 this.purpose = value; 7206 return this; 7207 } 7208 7209 /** 7210 * @return Explanation of why this structure map is needed and why it has been 7211 * designed as it has. 7212 */ 7213 public String getPurpose() { 7214 return this.purpose == null ? null : this.purpose.getValue(); 7215 } 7216 7217 /** 7218 * @param value Explanation of why this structure map is needed and why it has 7219 * been designed as it has. 7220 */ 7221 public StructureMap setPurpose(String value) { 7222 if (value == null) 7223 this.purpose = null; 7224 else { 7225 if (this.purpose == null) 7226 this.purpose = new MarkdownType(); 7227 this.purpose.setValue(value); 7228 } 7229 return this; 7230 } 7231 7232 /** 7233 * @return {@link #copyright} (A copyright statement relating to the structure 7234 * map and/or its contents. Copyright statements are generally legal 7235 * restrictions on the use and publishing of the structure map.). This 7236 * is the underlying object with id, value and extensions. The accessor 7237 * "getCopyright" gives direct access to the value 7238 */ 7239 public MarkdownType getCopyrightElement() { 7240 if (this.copyright == null) 7241 if (Configuration.errorOnAutoCreate()) 7242 throw new Error("Attempt to auto-create StructureMap.copyright"); 7243 else if (Configuration.doAutoCreate()) 7244 this.copyright = new MarkdownType(); // bb 7245 return this.copyright; 7246 } 7247 7248 public boolean hasCopyrightElement() { 7249 return this.copyright != null && !this.copyright.isEmpty(); 7250 } 7251 7252 public boolean hasCopyright() { 7253 return this.copyright != null && !this.copyright.isEmpty(); 7254 } 7255 7256 /** 7257 * @param value {@link #copyright} (A copyright statement relating to the 7258 * structure map and/or its contents. Copyright statements are 7259 * generally legal restrictions on the use and publishing of the 7260 * structure map.). This is the underlying object with id, value 7261 * and extensions. The accessor "getCopyright" gives direct access 7262 * to the value 7263 */ 7264 public StructureMap setCopyrightElement(MarkdownType value) { 7265 this.copyright = value; 7266 return this; 7267 } 7268 7269 /** 7270 * @return A copyright statement relating to the structure map and/or its 7271 * contents. Copyright statements are generally legal restrictions on 7272 * the use and publishing of the structure map. 7273 */ 7274 public String getCopyright() { 7275 return this.copyright == null ? null : this.copyright.getValue(); 7276 } 7277 7278 /** 7279 * @param value A copyright statement relating to the structure map and/or its 7280 * contents. Copyright statements are generally legal restrictions 7281 * on the use and publishing of the structure map. 7282 */ 7283 public StructureMap setCopyright(String value) { 7284 if (value == null) 7285 this.copyright = null; 7286 else { 7287 if (this.copyright == null) 7288 this.copyright = new MarkdownType(); 7289 this.copyright.setValue(value); 7290 } 7291 return this; 7292 } 7293 7294 /** 7295 * @return {@link #structure} (A structure definition used by this map. The 7296 * structure definition may describe instances that are converted, or 7297 * the instances that are produced.) 7298 */ 7299 public List<StructureMapStructureComponent> getStructure() { 7300 if (this.structure == null) 7301 this.structure = new ArrayList<StructureMapStructureComponent>(); 7302 return this.structure; 7303 } 7304 7305 /** 7306 * @return Returns a reference to <code>this</code> for easy method chaining 7307 */ 7308 public StructureMap setStructure(List<StructureMapStructureComponent> theStructure) { 7309 this.structure = theStructure; 7310 return this; 7311 } 7312 7313 public boolean hasStructure() { 7314 if (this.structure == null) 7315 return false; 7316 for (StructureMapStructureComponent item : this.structure) 7317 if (!item.isEmpty()) 7318 return true; 7319 return false; 7320 } 7321 7322 public StructureMapStructureComponent addStructure() { // 3 7323 StructureMapStructureComponent t = new StructureMapStructureComponent(); 7324 if (this.structure == null) 7325 this.structure = new ArrayList<StructureMapStructureComponent>(); 7326 this.structure.add(t); 7327 return t; 7328 } 7329 7330 public StructureMap addStructure(StructureMapStructureComponent t) { // 3 7331 if (t == null) 7332 return this; 7333 if (this.structure == null) 7334 this.structure = new ArrayList<StructureMapStructureComponent>(); 7335 this.structure.add(t); 7336 return this; 7337 } 7338 7339 /** 7340 * @return The first repetition of repeating field {@link #structure}, creating 7341 * it if it does not already exist 7342 */ 7343 public StructureMapStructureComponent getStructureFirstRep() { 7344 if (getStructure().isEmpty()) { 7345 addStructure(); 7346 } 7347 return getStructure().get(0); 7348 } 7349 7350 /** 7351 * @return {@link #import_} (Other maps used by this map (canonical URLs).) 7352 */ 7353 public List<CanonicalType> getImport() { 7354 if (this.import_ == null) 7355 this.import_ = new ArrayList<CanonicalType>(); 7356 return this.import_; 7357 } 7358 7359 /** 7360 * @return Returns a reference to <code>this</code> for easy method chaining 7361 */ 7362 public StructureMap setImport(List<CanonicalType> theImport) { 7363 this.import_ = theImport; 7364 return this; 7365 } 7366 7367 public boolean hasImport() { 7368 if (this.import_ == null) 7369 return false; 7370 for (CanonicalType item : this.import_) 7371 if (!item.isEmpty()) 7372 return true; 7373 return false; 7374 } 7375 7376 /** 7377 * @return {@link #import_} (Other maps used by this map (canonical URLs).) 7378 */ 7379 public CanonicalType addImportElement() {// 2 7380 CanonicalType t = new CanonicalType(); 7381 if (this.import_ == null) 7382 this.import_ = new ArrayList<CanonicalType>(); 7383 this.import_.add(t); 7384 return t; 7385 } 7386 7387 /** 7388 * @param value {@link #import_} (Other maps used by this map (canonical URLs).) 7389 */ 7390 public StructureMap addImport(String value) { // 1 7391 CanonicalType t = new CanonicalType(); 7392 t.setValue(value); 7393 if (this.import_ == null) 7394 this.import_ = new ArrayList<CanonicalType>(); 7395 this.import_.add(t); 7396 return this; 7397 } 7398 7399 /** 7400 * @param value {@link #import_} (Other maps used by this map (canonical URLs).) 7401 */ 7402 public boolean hasImport(String value) { 7403 if (this.import_ == null) 7404 return false; 7405 for (CanonicalType v : this.import_) 7406 if (v.getValue().equals(value)) // canonical(StructureMap) 7407 return true; 7408 return false; 7409 } 7410 7411 /** 7412 * @return {@link #group} (Organizes the mapping into manageable chunks for 7413 * human review/ease of maintenance.) 7414 */ 7415 public List<StructureMapGroupComponent> getGroup() { 7416 if (this.group == null) 7417 this.group = new ArrayList<StructureMapGroupComponent>(); 7418 return this.group; 7419 } 7420 7421 /** 7422 * @return Returns a reference to <code>this</code> for easy method chaining 7423 */ 7424 public StructureMap setGroup(List<StructureMapGroupComponent> theGroup) { 7425 this.group = theGroup; 7426 return this; 7427 } 7428 7429 public boolean hasGroup() { 7430 if (this.group == null) 7431 return false; 7432 for (StructureMapGroupComponent item : this.group) 7433 if (!item.isEmpty()) 7434 return true; 7435 return false; 7436 } 7437 7438 public StructureMapGroupComponent addGroup() { // 3 7439 StructureMapGroupComponent t = new StructureMapGroupComponent(); 7440 if (this.group == null) 7441 this.group = new ArrayList<StructureMapGroupComponent>(); 7442 this.group.add(t); 7443 return t; 7444 } 7445 7446 public StructureMap addGroup(StructureMapGroupComponent t) { // 3 7447 if (t == null) 7448 return this; 7449 if (this.group == null) 7450 this.group = new ArrayList<StructureMapGroupComponent>(); 7451 this.group.add(t); 7452 return this; 7453 } 7454 7455 /** 7456 * @return The first repetition of repeating field {@link #group}, creating it 7457 * if it does not already exist 7458 */ 7459 public StructureMapGroupComponent getGroupFirstRep() { 7460 if (getGroup().isEmpty()) { 7461 addGroup(); 7462 } 7463 return getGroup().get(0); 7464 } 7465 7466 protected void listChildren(List<Property> children) { 7467 super.listChildren(children); 7468 children.add(new Property("url", "uri", 7469 "An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.", 7470 0, 1, url)); 7471 children.add(new Property("identifier", "Identifier", 7472 "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 7473 0, java.lang.Integer.MAX_VALUE, identifier)); 7474 children.add(new Property("version", "string", 7475 "The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 7476 0, 1, version)); 7477 children.add(new Property("name", "string", 7478 "A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 7479 0, 1, name)); 7480 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the structure map.", 0, 7481 1, title)); 7482 children.add(new Property("status", "code", 7483 "The status of this structure map. Enables tracking the life-cycle of the content.", 0, 1, status)); 7484 children.add(new Property("experimental", "boolean", 7485 "A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 7486 0, 1, experimental)); 7487 children.add(new Property("date", "dateTime", 7488 "The date (and optionally time) when the structure map was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.", 7489 0, 1, date)); 7490 children.add(new Property("publisher", "string", 7491 "The name of the organization or individual that published the structure map.", 0, 1, publisher)); 7492 children.add(new Property("contact", "ContactDetail", 7493 "Contact details to assist a user in finding and communicating with the publisher.", 0, 7494 java.lang.Integer.MAX_VALUE, contact)); 7495 children.add(new Property("description", "markdown", 7496 "A free text natural language description of the structure map from a consumer's perspective.", 0, 1, 7497 description)); 7498 children.add(new Property("useContext", "UsageContext", 7499 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances.", 7500 0, java.lang.Integer.MAX_VALUE, useContext)); 7501 children.add(new Property("jurisdiction", "CodeableConcept", 7502 "A legal or geographic region in which the structure map is intended to be used.", 0, 7503 java.lang.Integer.MAX_VALUE, jurisdiction)); 7504 children.add(new Property("purpose", "markdown", 7505 "Explanation of why this structure map is needed and why it has been designed as it has.", 0, 1, purpose)); 7506 children.add(new Property("copyright", "markdown", 7507 "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.", 7508 0, 1, copyright)); 7509 children.add(new Property("structure", "", 7510 "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.", 7511 0, java.lang.Integer.MAX_VALUE, structure)); 7512 children.add(new Property("import", "canonical(StructureMap)", "Other maps used by this map (canonical URLs).", 0, 7513 java.lang.Integer.MAX_VALUE, import_)); 7514 children.add( 7515 new Property("group", "", "Organizes the mapping into manageable chunks for human review/ease of maintenance.", 7516 0, java.lang.Integer.MAX_VALUE, group)); 7517 } 7518 7519 @Override 7520 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7521 switch (_hash) { 7522 case 116079: 7523 /* url */ return new Property("url", "uri", 7524 "An absolute URI that is used to identify this structure map when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this structure map is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the structure map is stored on different servers.", 7525 0, 1, url); 7526 case -1618432855: 7527 /* identifier */ return new Property("identifier", "Identifier", 7528 "A formal identifier that is used to identify this structure map when it is represented in other formats, or referenced in a specification, model, design or an instance.", 7529 0, java.lang.Integer.MAX_VALUE, identifier); 7530 case 351608024: 7531 /* version */ return new Property("version", "string", 7532 "The identifier that is used to identify this version of the structure map when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the structure map author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 7533 0, 1, version); 7534 case 3373707: 7535 /* name */ return new Property("name", "string", 7536 "A natural language name identifying the structure map. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 7537 0, 1, name); 7538 case 110371416: 7539 /* title */ return new Property("title", "string", 7540 "A short, descriptive, user-friendly title for the structure map.", 0, 1, title); 7541 case -892481550: 7542 /* status */ return new Property("status", "code", 7543 "The status of this structure map. Enables tracking the life-cycle of the content.", 0, 1, status); 7544 case -404562712: 7545 /* experimental */ return new Property("experimental", "boolean", 7546 "A Boolean value to indicate that this structure map is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 7547 0, 1, experimental); 7548 case 3076014: 7549 /* date */ return new Property("date", "dateTime", 7550 "The date (and optionally time) when the structure map was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the structure map changes.", 7551 0, 1, date); 7552 case 1447404028: 7553 /* publisher */ return new Property("publisher", "string", 7554 "The name of the organization or individual that published the structure map.", 0, 1, publisher); 7555 case 951526432: 7556 /* contact */ return new Property("contact", "ContactDetail", 7557 "Contact details to assist a user in finding and communicating with the publisher.", 0, 7558 java.lang.Integer.MAX_VALUE, contact); 7559 case -1724546052: 7560 /* description */ return new Property("description", "markdown", 7561 "A free text natural language description of the structure map from a consumer's perspective.", 0, 1, 7562 description); 7563 case -669707736: 7564 /* useContext */ return new Property("useContext", "UsageContext", 7565 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate structure map instances.", 7566 0, java.lang.Integer.MAX_VALUE, useContext); 7567 case -507075711: 7568 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 7569 "A legal or geographic region in which the structure map is intended to be used.", 0, 7570 java.lang.Integer.MAX_VALUE, jurisdiction); 7571 case -220463842: 7572 /* purpose */ return new Property("purpose", "markdown", 7573 "Explanation of why this structure map is needed and why it has been designed as it has.", 0, 1, purpose); 7574 case 1522889671: 7575 /* copyright */ return new Property("copyright", "markdown", 7576 "A copyright statement relating to the structure map and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the structure map.", 7577 0, 1, copyright); 7578 case 144518515: 7579 /* structure */ return new Property("structure", "", 7580 "A structure definition used by this map. The structure definition may describe instances that are converted, or the instances that are produced.", 7581 0, java.lang.Integer.MAX_VALUE, structure); 7582 case -1184795739: 7583 /* import */ return new Property("import", "canonical(StructureMap)", 7584 "Other maps used by this map (canonical URLs).", 0, java.lang.Integer.MAX_VALUE, import_); 7585 case 98629247: 7586 /* group */ return new Property("group", "", 7587 "Organizes the mapping into manageable chunks for human review/ease of maintenance.", 0, 7588 java.lang.Integer.MAX_VALUE, group); 7589 default: 7590 return super.getNamedProperty(_hash, _name, _checkValid); 7591 } 7592 7593 } 7594 7595 @Override 7596 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7597 switch (hash) { 7598 case 116079: 7599 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 7600 case -1618432855: 7601 /* identifier */ return this.identifier == null ? new Base[0] 7602 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 7603 case 351608024: 7604 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 7605 case 3373707: 7606 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 7607 case 110371416: 7608 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 7609 case -892481550: 7610 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 7611 case -404562712: 7612 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 7613 case 3076014: 7614 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 7615 case 1447404028: 7616 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 7617 case 951526432: 7618 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 7619 case -1724546052: 7620 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 7621 case -669707736: 7622 /* useContext */ return this.useContext == null ? new Base[0] 7623 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 7624 case -507075711: 7625 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 7626 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 7627 case -220463842: 7628 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 7629 case 1522889671: 7630 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 7631 case 144518515: 7632 /* structure */ return this.structure == null ? new Base[0] 7633 : this.structure.toArray(new Base[this.structure.size()]); // StructureMapStructureComponent 7634 case -1184795739: 7635 /* import */ return this.import_ == null ? new Base[0] : this.import_.toArray(new Base[this.import_.size()]); // CanonicalType 7636 case 98629247: 7637 /* group */ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // StructureMapGroupComponent 7638 default: 7639 return super.getProperty(hash, name, checkValid); 7640 } 7641 7642 } 7643 7644 @Override 7645 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7646 switch (hash) { 7647 case 116079: // url 7648 this.url = castToUri(value); // UriType 7649 return value; 7650 case -1618432855: // identifier 7651 this.getIdentifier().add(castToIdentifier(value)); // Identifier 7652 return value; 7653 case 351608024: // version 7654 this.version = castToString(value); // StringType 7655 return value; 7656 case 3373707: // name 7657 this.name = castToString(value); // StringType 7658 return value; 7659 case 110371416: // title 7660 this.title = castToString(value); // StringType 7661 return value; 7662 case -892481550: // status 7663 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 7664 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 7665 return value; 7666 case -404562712: // experimental 7667 this.experimental = castToBoolean(value); // BooleanType 7668 return value; 7669 case 3076014: // date 7670 this.date = castToDateTime(value); // DateTimeType 7671 return value; 7672 case 1447404028: // publisher 7673 this.publisher = castToString(value); // StringType 7674 return value; 7675 case 951526432: // contact 7676 this.getContact().add(castToContactDetail(value)); // ContactDetail 7677 return value; 7678 case -1724546052: // description 7679 this.description = castToMarkdown(value); // MarkdownType 7680 return value; 7681 case -669707736: // useContext 7682 this.getUseContext().add(castToUsageContext(value)); // UsageContext 7683 return value; 7684 case -507075711: // jurisdiction 7685 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 7686 return value; 7687 case -220463842: // purpose 7688 this.purpose = castToMarkdown(value); // MarkdownType 7689 return value; 7690 case 1522889671: // copyright 7691 this.copyright = castToMarkdown(value); // MarkdownType 7692 return value; 7693 case 144518515: // structure 7694 this.getStructure().add((StructureMapStructureComponent) value); // StructureMapStructureComponent 7695 return value; 7696 case -1184795739: // import 7697 this.getImport().add(castToCanonical(value)); // CanonicalType 7698 return value; 7699 case 98629247: // group 7700 this.getGroup().add((StructureMapGroupComponent) value); // StructureMapGroupComponent 7701 return value; 7702 default: 7703 return super.setProperty(hash, name, value); 7704 } 7705 7706 } 7707 7708 @Override 7709 public Base setProperty(String name, Base value) throws FHIRException { 7710 if (name.equals("url")) { 7711 this.url = castToUri(value); // UriType 7712 } else if (name.equals("identifier")) { 7713 this.getIdentifier().add(castToIdentifier(value)); 7714 } else if (name.equals("version")) { 7715 this.version = castToString(value); // StringType 7716 } else if (name.equals("name")) { 7717 this.name = castToString(value); // StringType 7718 } else if (name.equals("title")) { 7719 this.title = castToString(value); // StringType 7720 } else if (name.equals("status")) { 7721 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 7722 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 7723 } else if (name.equals("experimental")) { 7724 this.experimental = castToBoolean(value); // BooleanType 7725 } else if (name.equals("date")) { 7726 this.date = castToDateTime(value); // DateTimeType 7727 } else if (name.equals("publisher")) { 7728 this.publisher = castToString(value); // StringType 7729 } else if (name.equals("contact")) { 7730 this.getContact().add(castToContactDetail(value)); 7731 } else if (name.equals("description")) { 7732 this.description = castToMarkdown(value); // MarkdownType 7733 } else if (name.equals("useContext")) { 7734 this.getUseContext().add(castToUsageContext(value)); 7735 } else if (name.equals("jurisdiction")) { 7736 this.getJurisdiction().add(castToCodeableConcept(value)); 7737 } else if (name.equals("purpose")) { 7738 this.purpose = castToMarkdown(value); // MarkdownType 7739 } else if (name.equals("copyright")) { 7740 this.copyright = castToMarkdown(value); // MarkdownType 7741 } else if (name.equals("structure")) { 7742 this.getStructure().add((StructureMapStructureComponent) value); 7743 } else if (name.equals("import")) { 7744 this.getImport().add(castToCanonical(value)); 7745 } else if (name.equals("group")) { 7746 this.getGroup().add((StructureMapGroupComponent) value); 7747 } else 7748 return super.setProperty(name, value); 7749 return value; 7750 } 7751 7752 @Override 7753 public void removeChild(String name, Base value) throws FHIRException { 7754 if (name.equals("url")) { 7755 this.url = null; 7756 } else if (name.equals("identifier")) { 7757 this.getIdentifier().remove(castToIdentifier(value)); 7758 } else if (name.equals("version")) { 7759 this.version = null; 7760 } else if (name.equals("name")) { 7761 this.name = null; 7762 } else if (name.equals("title")) { 7763 this.title = null; 7764 } else if (name.equals("status")) { 7765 this.status = null; 7766 } else if (name.equals("experimental")) { 7767 this.experimental = null; 7768 } else if (name.equals("date")) { 7769 this.date = null; 7770 } else if (name.equals("publisher")) { 7771 this.publisher = null; 7772 } else if (name.equals("contact")) { 7773 this.getContact().remove(castToContactDetail(value)); 7774 } else if (name.equals("description")) { 7775 this.description = null; 7776 } else if (name.equals("useContext")) { 7777 this.getUseContext().remove(castToUsageContext(value)); 7778 } else if (name.equals("jurisdiction")) { 7779 this.getJurisdiction().remove(castToCodeableConcept(value)); 7780 } else if (name.equals("purpose")) { 7781 this.purpose = null; 7782 } else if (name.equals("copyright")) { 7783 this.copyright = null; 7784 } else if (name.equals("structure")) { 7785 this.getStructure().remove((StructureMapStructureComponent) value); 7786 } else if (name.equals("import")) { 7787 this.getImport().remove(castToCanonical(value)); 7788 } else if (name.equals("group")) { 7789 this.getGroup().remove((StructureMapGroupComponent) value); 7790 } else 7791 super.removeChild(name, value); 7792 7793 } 7794 7795 @Override 7796 public Base makeProperty(int hash, String name) throws FHIRException { 7797 switch (hash) { 7798 case 116079: 7799 return getUrlElement(); 7800 case -1618432855: 7801 return addIdentifier(); 7802 case 351608024: 7803 return getVersionElement(); 7804 case 3373707: 7805 return getNameElement(); 7806 case 110371416: 7807 return getTitleElement(); 7808 case -892481550: 7809 return getStatusElement(); 7810 case -404562712: 7811 return getExperimentalElement(); 7812 case 3076014: 7813 return getDateElement(); 7814 case 1447404028: 7815 return getPublisherElement(); 7816 case 951526432: 7817 return addContact(); 7818 case -1724546052: 7819 return getDescriptionElement(); 7820 case -669707736: 7821 return addUseContext(); 7822 case -507075711: 7823 return addJurisdiction(); 7824 case -220463842: 7825 return getPurposeElement(); 7826 case 1522889671: 7827 return getCopyrightElement(); 7828 case 144518515: 7829 return addStructure(); 7830 case -1184795739: 7831 return addImportElement(); 7832 case 98629247: 7833 return addGroup(); 7834 default: 7835 return super.makeProperty(hash, name); 7836 } 7837 7838 } 7839 7840 @Override 7841 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7842 switch (hash) { 7843 case 116079: 7844 /* url */ return new String[] { "uri" }; 7845 case -1618432855: 7846 /* identifier */ return new String[] { "Identifier" }; 7847 case 351608024: 7848 /* version */ return new String[] { "string" }; 7849 case 3373707: 7850 /* name */ return new String[] { "string" }; 7851 case 110371416: 7852 /* title */ return new String[] { "string" }; 7853 case -892481550: 7854 /* status */ return new String[] { "code" }; 7855 case -404562712: 7856 /* experimental */ return new String[] { "boolean" }; 7857 case 3076014: 7858 /* date */ return new String[] { "dateTime" }; 7859 case 1447404028: 7860 /* publisher */ return new String[] { "string" }; 7861 case 951526432: 7862 /* contact */ return new String[] { "ContactDetail" }; 7863 case -1724546052: 7864 /* description */ return new String[] { "markdown" }; 7865 case -669707736: 7866 /* useContext */ return new String[] { "UsageContext" }; 7867 case -507075711: 7868 /* jurisdiction */ return new String[] { "CodeableConcept" }; 7869 case -220463842: 7870 /* purpose */ return new String[] { "markdown" }; 7871 case 1522889671: 7872 /* copyright */ return new String[] { "markdown" }; 7873 case 144518515: 7874 /* structure */ return new String[] {}; 7875 case -1184795739: 7876 /* import */ return new String[] { "canonical" }; 7877 case 98629247: 7878 /* group */ return new String[] {}; 7879 default: 7880 return super.getTypesForProperty(hash, name); 7881 } 7882 7883 } 7884 7885 @Override 7886 public Base addChild(String name) throws FHIRException { 7887 if (name.equals("url")) { 7888 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.url"); 7889 } else if (name.equals("identifier")) { 7890 return addIdentifier(); 7891 } else if (name.equals("version")) { 7892 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.version"); 7893 } else if (name.equals("name")) { 7894 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.name"); 7895 } else if (name.equals("title")) { 7896 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.title"); 7897 } else if (name.equals("status")) { 7898 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.status"); 7899 } else if (name.equals("experimental")) { 7900 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.experimental"); 7901 } else if (name.equals("date")) { 7902 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.date"); 7903 } else if (name.equals("publisher")) { 7904 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.publisher"); 7905 } else if (name.equals("contact")) { 7906 return addContact(); 7907 } else if (name.equals("description")) { 7908 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.description"); 7909 } else if (name.equals("useContext")) { 7910 return addUseContext(); 7911 } else if (name.equals("jurisdiction")) { 7912 return addJurisdiction(); 7913 } else if (name.equals("purpose")) { 7914 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.purpose"); 7915 } else if (name.equals("copyright")) { 7916 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.copyright"); 7917 } else if (name.equals("structure")) { 7918 return addStructure(); 7919 } else if (name.equals("import")) { 7920 throw new FHIRException("Cannot call addChild on a singleton property StructureMap.import"); 7921 } else if (name.equals("group")) { 7922 return addGroup(); 7923 } else 7924 return super.addChild(name); 7925 } 7926 7927 public String fhirType() { 7928 return "StructureMap"; 7929 7930 } 7931 7932 public StructureMap copy() { 7933 StructureMap dst = new StructureMap(); 7934 copyValues(dst); 7935 return dst; 7936 } 7937 7938 public void copyValues(StructureMap dst) { 7939 super.copyValues(dst); 7940 dst.url = url == null ? null : url.copy(); 7941 if (identifier != null) { 7942 dst.identifier = new ArrayList<Identifier>(); 7943 for (Identifier i : identifier) 7944 dst.identifier.add(i.copy()); 7945 } 7946 ; 7947 dst.version = version == null ? null : version.copy(); 7948 dst.name = name == null ? null : name.copy(); 7949 dst.title = title == null ? null : title.copy(); 7950 dst.status = status == null ? null : status.copy(); 7951 dst.experimental = experimental == null ? null : experimental.copy(); 7952 dst.date = date == null ? null : date.copy(); 7953 dst.publisher = publisher == null ? null : publisher.copy(); 7954 if (contact != null) { 7955 dst.contact = new ArrayList<ContactDetail>(); 7956 for (ContactDetail i : contact) 7957 dst.contact.add(i.copy()); 7958 } 7959 ; 7960 dst.description = description == null ? null : description.copy(); 7961 if (useContext != null) { 7962 dst.useContext = new ArrayList<UsageContext>(); 7963 for (UsageContext i : useContext) 7964 dst.useContext.add(i.copy()); 7965 } 7966 ; 7967 if (jurisdiction != null) { 7968 dst.jurisdiction = new ArrayList<CodeableConcept>(); 7969 for (CodeableConcept i : jurisdiction) 7970 dst.jurisdiction.add(i.copy()); 7971 } 7972 ; 7973 dst.purpose = purpose == null ? null : purpose.copy(); 7974 dst.copyright = copyright == null ? null : copyright.copy(); 7975 if (structure != null) { 7976 dst.structure = new ArrayList<StructureMapStructureComponent>(); 7977 for (StructureMapStructureComponent i : structure) 7978 dst.structure.add(i.copy()); 7979 } 7980 ; 7981 if (import_ != null) { 7982 dst.import_ = new ArrayList<CanonicalType>(); 7983 for (CanonicalType i : import_) 7984 dst.import_.add(i.copy()); 7985 } 7986 ; 7987 if (group != null) { 7988 dst.group = new ArrayList<StructureMapGroupComponent>(); 7989 for (StructureMapGroupComponent i : group) 7990 dst.group.add(i.copy()); 7991 } 7992 ; 7993 } 7994 7995 protected StructureMap typedCopy() { 7996 return copy(); 7997 } 7998 7999 @Override 8000 public boolean equalsDeep(Base other_) { 8001 if (!super.equalsDeep(other_)) 8002 return false; 8003 if (!(other_ instanceof StructureMap)) 8004 return false; 8005 StructureMap o = (StructureMap) other_; 8006 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) 8007 && compareDeep(copyright, o.copyright, true) && compareDeep(structure, o.structure, true) 8008 && compareDeep(import_, o.import_, true) && compareDeep(group, o.group, true); 8009 } 8010 8011 @Override 8012 public boolean equalsShallow(Base other_) { 8013 if (!super.equalsShallow(other_)) 8014 return false; 8015 if (!(other_ instanceof StructureMap)) 8016 return false; 8017 StructureMap o = (StructureMap) other_; 8018 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true); 8019 } 8020 8021 public boolean isEmpty() { 8022 return super.isEmpty() 8023 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright, structure, import_, group); 8024 } 8025 8026 @Override 8027 public ResourceType getResourceType() { 8028 return ResourceType.StructureMap; 8029 } 8030 8031 /** 8032 * Search parameter: <b>date</b> 8033 * <p> 8034 * Description: <b>The structure map publication date</b><br> 8035 * Type: <b>date</b><br> 8036 * Path: <b>StructureMap.date</b><br> 8037 * </p> 8038 */ 8039 @SearchParamDefinition(name = "date", path = "StructureMap.date", description = "The structure map publication date", type = "date") 8040 public static final String SP_DATE = "date"; 8041 /** 8042 * <b>Fluent Client</b> search parameter constant for <b>date</b> 8043 * <p> 8044 * Description: <b>The structure map publication date</b><br> 8045 * Type: <b>date</b><br> 8046 * Path: <b>StructureMap.date</b><br> 8047 * </p> 8048 */ 8049 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 8050 SP_DATE); 8051 8052 /** 8053 * Search parameter: <b>identifier</b> 8054 * <p> 8055 * Description: <b>External identifier for the structure map</b><br> 8056 * Type: <b>token</b><br> 8057 * Path: <b>StructureMap.identifier</b><br> 8058 * </p> 8059 */ 8060 @SearchParamDefinition(name = "identifier", path = "StructureMap.identifier", description = "External identifier for the structure map", type = "token") 8061 public static final String SP_IDENTIFIER = "identifier"; 8062 /** 8063 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 8064 * <p> 8065 * Description: <b>External identifier for the structure map</b><br> 8066 * Type: <b>token</b><br> 8067 * Path: <b>StructureMap.identifier</b><br> 8068 * </p> 8069 */ 8070 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8071 SP_IDENTIFIER); 8072 8073 /** 8074 * Search parameter: <b>context-type-value</b> 8075 * <p> 8076 * Description: <b>A use context type and value assigned to the structure 8077 * map</b><br> 8078 * Type: <b>composite</b><br> 8079 * Path: <b></b><br> 8080 * </p> 8081 */ 8082 @SearchParamDefinition(name = "context-type-value", path = "StructureMap.useContext", description = "A use context type and value assigned to the structure map", type = "composite", compositeOf = { 8083 "context-type", "context" }) 8084 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 8085 /** 8086 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 8087 * <p> 8088 * Description: <b>A use context type and value assigned to the structure 8089 * map</b><br> 8090 * Type: <b>composite</b><br> 8091 * Path: <b></b><br> 8092 * </p> 8093 */ 8094 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 8095 SP_CONTEXT_TYPE_VALUE); 8096 8097 /** 8098 * Search parameter: <b>jurisdiction</b> 8099 * <p> 8100 * Description: <b>Intended jurisdiction for the structure map</b><br> 8101 * Type: <b>token</b><br> 8102 * Path: <b>StructureMap.jurisdiction</b><br> 8103 * </p> 8104 */ 8105 @SearchParamDefinition(name = "jurisdiction", path = "StructureMap.jurisdiction", description = "Intended jurisdiction for the structure map", type = "token") 8106 public static final String SP_JURISDICTION = "jurisdiction"; 8107 /** 8108 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 8109 * <p> 8110 * Description: <b>Intended jurisdiction for the structure map</b><br> 8111 * Type: <b>token</b><br> 8112 * Path: <b>StructureMap.jurisdiction</b><br> 8113 * </p> 8114 */ 8115 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8116 SP_JURISDICTION); 8117 8118 /** 8119 * Search parameter: <b>description</b> 8120 * <p> 8121 * Description: <b>The description of the structure map</b><br> 8122 * Type: <b>string</b><br> 8123 * Path: <b>StructureMap.description</b><br> 8124 * </p> 8125 */ 8126 @SearchParamDefinition(name = "description", path = "StructureMap.description", description = "The description of the structure map", type = "string") 8127 public static final String SP_DESCRIPTION = "description"; 8128 /** 8129 * <b>Fluent Client</b> search parameter constant for <b>description</b> 8130 * <p> 8131 * Description: <b>The description of the structure map</b><br> 8132 * Type: <b>string</b><br> 8133 * Path: <b>StructureMap.description</b><br> 8134 * </p> 8135 */ 8136 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 8137 SP_DESCRIPTION); 8138 8139 /** 8140 * Search parameter: <b>context-type</b> 8141 * <p> 8142 * Description: <b>A type of use context assigned to the structure map</b><br> 8143 * Type: <b>token</b><br> 8144 * Path: <b>StructureMap.useContext.code</b><br> 8145 * </p> 8146 */ 8147 @SearchParamDefinition(name = "context-type", path = "StructureMap.useContext.code", description = "A type of use context assigned to the structure map", type = "token") 8148 public static final String SP_CONTEXT_TYPE = "context-type"; 8149 /** 8150 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 8151 * <p> 8152 * Description: <b>A type of use context assigned to the structure map</b><br> 8153 * Type: <b>token</b><br> 8154 * Path: <b>StructureMap.useContext.code</b><br> 8155 * </p> 8156 */ 8157 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8158 SP_CONTEXT_TYPE); 8159 8160 /** 8161 * Search parameter: <b>title</b> 8162 * <p> 8163 * Description: <b>The human-friendly name of the structure map</b><br> 8164 * Type: <b>string</b><br> 8165 * Path: <b>StructureMap.title</b><br> 8166 * </p> 8167 */ 8168 @SearchParamDefinition(name = "title", path = "StructureMap.title", description = "The human-friendly name of the structure map", type = "string") 8169 public static final String SP_TITLE = "title"; 8170 /** 8171 * <b>Fluent Client</b> search parameter constant for <b>title</b> 8172 * <p> 8173 * Description: <b>The human-friendly name of the structure map</b><br> 8174 * Type: <b>string</b><br> 8175 * Path: <b>StructureMap.title</b><br> 8176 * </p> 8177 */ 8178 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 8179 SP_TITLE); 8180 8181 /** 8182 * Search parameter: <b>version</b> 8183 * <p> 8184 * Description: <b>The business version of the structure map</b><br> 8185 * Type: <b>token</b><br> 8186 * Path: <b>StructureMap.version</b><br> 8187 * </p> 8188 */ 8189 @SearchParamDefinition(name = "version", path = "StructureMap.version", description = "The business version of the structure map", type = "token") 8190 public static final String SP_VERSION = "version"; 8191 /** 8192 * <b>Fluent Client</b> search parameter constant for <b>version</b> 8193 * <p> 8194 * Description: <b>The business version of the structure map</b><br> 8195 * Type: <b>token</b><br> 8196 * Path: <b>StructureMap.version</b><br> 8197 * </p> 8198 */ 8199 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8200 SP_VERSION); 8201 8202 /** 8203 * Search parameter: <b>url</b> 8204 * <p> 8205 * Description: <b>The uri that identifies the structure map</b><br> 8206 * Type: <b>uri</b><br> 8207 * Path: <b>StructureMap.url</b><br> 8208 * </p> 8209 */ 8210 @SearchParamDefinition(name = "url", path = "StructureMap.url", description = "The uri that identifies the structure map", type = "uri") 8211 public static final String SP_URL = "url"; 8212 /** 8213 * <b>Fluent Client</b> search parameter constant for <b>url</b> 8214 * <p> 8215 * Description: <b>The uri that identifies the structure map</b><br> 8216 * Type: <b>uri</b><br> 8217 * Path: <b>StructureMap.url</b><br> 8218 * </p> 8219 */ 8220 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 8221 8222 /** 8223 * Search parameter: <b>context-quantity</b> 8224 * <p> 8225 * Description: <b>A quantity- or range-valued use context assigned to the 8226 * structure map</b><br> 8227 * Type: <b>quantity</b><br> 8228 * Path: <b>StructureMap.useContext.valueQuantity, 8229 * StructureMap.useContext.valueRange</b><br> 8230 * </p> 8231 */ 8232 @SearchParamDefinition(name = "context-quantity", path = "(StructureMap.useContext.value as Quantity) | (StructureMap.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the structure map", type = "quantity") 8233 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 8234 /** 8235 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 8236 * <p> 8237 * Description: <b>A quantity- or range-valued use context assigned to the 8238 * structure map</b><br> 8239 * Type: <b>quantity</b><br> 8240 * Path: <b>StructureMap.useContext.valueQuantity, 8241 * StructureMap.useContext.valueRange</b><br> 8242 * </p> 8243 */ 8244 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 8245 SP_CONTEXT_QUANTITY); 8246 8247 /** 8248 * Search parameter: <b>name</b> 8249 * <p> 8250 * Description: <b>Computationally friendly name of the structure map</b><br> 8251 * Type: <b>string</b><br> 8252 * Path: <b>StructureMap.name</b><br> 8253 * </p> 8254 */ 8255 @SearchParamDefinition(name = "name", path = "StructureMap.name", description = "Computationally friendly name of the structure map", type = "string") 8256 public static final String SP_NAME = "name"; 8257 /** 8258 * <b>Fluent Client</b> search parameter constant for <b>name</b> 8259 * <p> 8260 * Description: <b>Computationally friendly name of the structure map</b><br> 8261 * Type: <b>string</b><br> 8262 * Path: <b>StructureMap.name</b><br> 8263 * </p> 8264 */ 8265 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 8266 SP_NAME); 8267 8268 /** 8269 * Search parameter: <b>context</b> 8270 * <p> 8271 * Description: <b>A use context assigned to the structure map</b><br> 8272 * Type: <b>token</b><br> 8273 * Path: <b>StructureMap.useContext.valueCodeableConcept</b><br> 8274 * </p> 8275 */ 8276 @SearchParamDefinition(name = "context", path = "(StructureMap.useContext.value as CodeableConcept)", description = "A use context assigned to the structure map", type = "token") 8277 public static final String SP_CONTEXT = "context"; 8278 /** 8279 * <b>Fluent Client</b> search parameter constant for <b>context</b> 8280 * <p> 8281 * Description: <b>A use context assigned to the structure map</b><br> 8282 * Type: <b>token</b><br> 8283 * Path: <b>StructureMap.useContext.valueCodeableConcept</b><br> 8284 * </p> 8285 */ 8286 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8287 SP_CONTEXT); 8288 8289 /** 8290 * Search parameter: <b>publisher</b> 8291 * <p> 8292 * Description: <b>Name of the publisher of the structure map</b><br> 8293 * Type: <b>string</b><br> 8294 * Path: <b>StructureMap.publisher</b><br> 8295 * </p> 8296 */ 8297 @SearchParamDefinition(name = "publisher", path = "StructureMap.publisher", description = "Name of the publisher of the structure map", type = "string") 8298 public static final String SP_PUBLISHER = "publisher"; 8299 /** 8300 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 8301 * <p> 8302 * Description: <b>Name of the publisher of the structure map</b><br> 8303 * Type: <b>string</b><br> 8304 * Path: <b>StructureMap.publisher</b><br> 8305 * </p> 8306 */ 8307 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 8308 SP_PUBLISHER); 8309 8310 /** 8311 * Search parameter: <b>context-type-quantity</b> 8312 * <p> 8313 * Description: <b>A use context type and quantity- or range-based value 8314 * assigned to the structure map</b><br> 8315 * Type: <b>composite</b><br> 8316 * Path: <b></b><br> 8317 * </p> 8318 */ 8319 @SearchParamDefinition(name = "context-type-quantity", path = "StructureMap.useContext", description = "A use context type and quantity- or range-based value assigned to the structure map", type = "composite", compositeOf = { 8320 "context-type", "context-quantity" }) 8321 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 8322 /** 8323 * <b>Fluent Client</b> search parameter constant for 8324 * <b>context-type-quantity</b> 8325 * <p> 8326 * Description: <b>A use context type and quantity- or range-based value 8327 * assigned to the structure map</b><br> 8328 * Type: <b>composite</b><br> 8329 * Path: <b></b><br> 8330 * </p> 8331 */ 8332 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 8333 SP_CONTEXT_TYPE_QUANTITY); 8334 8335 /** 8336 * Search parameter: <b>status</b> 8337 * <p> 8338 * Description: <b>The current status of the structure map</b><br> 8339 * Type: <b>token</b><br> 8340 * Path: <b>StructureMap.status</b><br> 8341 * </p> 8342 */ 8343 @SearchParamDefinition(name = "status", path = "StructureMap.status", description = "The current status of the structure map", type = "token") 8344 public static final String SP_STATUS = "status"; 8345 /** 8346 * <b>Fluent Client</b> search parameter constant for <b>status</b> 8347 * <p> 8348 * Description: <b>The current status of the structure map</b><br> 8349 * Type: <b>token</b><br> 8350 * Path: <b>StructureMap.status</b><br> 8351 * </p> 8352 */ 8353 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8354 SP_STATUS); 8355 8356// added from java-adornments.txt: 8357 8358 public String toString() { 8359 return StructureMapUtilities.render(this); 8360 } 8361 8362// end addition 8363 8364}