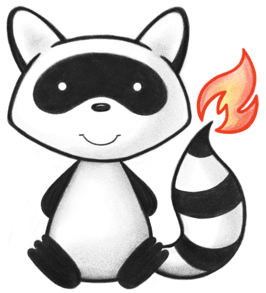
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A homogeneous material with a definite composition. 049 */ 050@ResourceDef(name = "Substance", profile = "http://hl7.org/fhir/StructureDefinition/Substance") 051public class Substance extends DomainResource { 052 053 public enum FHIRSubstanceStatus { 054 /** 055 * The substance is considered for use or reference. 056 */ 057 ACTIVE, 058 /** 059 * The substance is considered for reference, but not for use. 060 */ 061 INACTIVE, 062 /** 063 * The substance was entered in error. 064 */ 065 ENTEREDINERROR, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 071 public static FHIRSubstanceStatus fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("active".equals(codeString)) 075 return ACTIVE; 076 if ("inactive".equals(codeString)) 077 return INACTIVE; 078 if ("entered-in-error".equals(codeString)) 079 return ENTEREDINERROR; 080 if (Configuration.isAcceptInvalidEnums()) 081 return null; 082 else 083 throw new FHIRException("Unknown FHIRSubstanceStatus code '" + codeString + "'"); 084 } 085 086 public String toCode() { 087 switch (this) { 088 case ACTIVE: 089 return "active"; 090 case INACTIVE: 091 return "inactive"; 092 case ENTEREDINERROR: 093 return "entered-in-error"; 094 case NULL: 095 return null; 096 default: 097 return "?"; 098 } 099 } 100 101 public String getSystem() { 102 switch (this) { 103 case ACTIVE: 104 return "http://hl7.org/fhir/substance-status"; 105 case INACTIVE: 106 return "http://hl7.org/fhir/substance-status"; 107 case ENTEREDINERROR: 108 return "http://hl7.org/fhir/substance-status"; 109 case NULL: 110 return null; 111 default: 112 return "?"; 113 } 114 } 115 116 public String getDefinition() { 117 switch (this) { 118 case ACTIVE: 119 return "The substance is considered for use or reference."; 120 case INACTIVE: 121 return "The substance is considered for reference, but not for use."; 122 case ENTEREDINERROR: 123 return "The substance was entered in error."; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDisplay() { 132 switch (this) { 133 case ACTIVE: 134 return "Active"; 135 case INACTIVE: 136 return "Inactive"; 137 case ENTEREDINERROR: 138 return "Entered in Error"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 } 146 147 public static class FHIRSubstanceStatusEnumFactory implements EnumFactory<FHIRSubstanceStatus> { 148 public FHIRSubstanceStatus fromCode(String codeString) throws IllegalArgumentException { 149 if (codeString == null || "".equals(codeString)) 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("active".equals(codeString)) 153 return FHIRSubstanceStatus.ACTIVE; 154 if ("inactive".equals(codeString)) 155 return FHIRSubstanceStatus.INACTIVE; 156 if ("entered-in-error".equals(codeString)) 157 return FHIRSubstanceStatus.ENTEREDINERROR; 158 throw new IllegalArgumentException("Unknown FHIRSubstanceStatus code '" + codeString + "'"); 159 } 160 161 public Enumeration<FHIRSubstanceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 162 if (code == null) 163 return null; 164 if (code.isEmpty()) 165 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.NULL, code); 166 String codeString = code.asStringValue(); 167 if (codeString == null || "".equals(codeString)) 168 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.NULL, code); 169 if ("active".equals(codeString)) 170 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.ACTIVE, code); 171 if ("inactive".equals(codeString)) 172 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.INACTIVE, code); 173 if ("entered-in-error".equals(codeString)) 174 return new Enumeration<FHIRSubstanceStatus>(this, FHIRSubstanceStatus.ENTEREDINERROR, code); 175 throw new FHIRException("Unknown FHIRSubstanceStatus code '" + codeString + "'"); 176 } 177 178 public String toCode(FHIRSubstanceStatus code) { 179 if (code == FHIRSubstanceStatus.NULL) 180 return null; 181 if (code == FHIRSubstanceStatus.ACTIVE) 182 return "active"; 183 if (code == FHIRSubstanceStatus.INACTIVE) 184 return "inactive"; 185 if (code == FHIRSubstanceStatus.ENTEREDINERROR) 186 return "entered-in-error"; 187 return "?"; 188 } 189 190 public String toSystem(FHIRSubstanceStatus code) { 191 return code.getSystem(); 192 } 193 } 194 195 @Block() 196 public static class SubstanceInstanceComponent extends BackboneElement implements IBaseBackboneElement { 197 /** 198 * Identifier associated with the package/container (usually a label affixed 199 * directly). 200 */ 201 @Child(name = "identifier", type = { 202 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 203 @Description(shortDefinition = "Identifier of the package/container", formalDefinition = "Identifier associated with the package/container (usually a label affixed directly).") 204 protected Identifier identifier; 205 206 /** 207 * When the substance is no longer valid to use. For some substances, a single 208 * arbitrary date is used for expiry. 209 */ 210 @Child(name = "expiry", type = { 211 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 212 @Description(shortDefinition = "When no longer valid to use", formalDefinition = "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.") 213 protected DateTimeType expiry; 214 215 /** 216 * The amount of the substance. 217 */ 218 @Child(name = "quantity", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 219 @Description(shortDefinition = "Amount of substance in the package", formalDefinition = "The amount of the substance.") 220 protected Quantity quantity; 221 222 private static final long serialVersionUID = -1474380480L; 223 224 /** 225 * Constructor 226 */ 227 public SubstanceInstanceComponent() { 228 super(); 229 } 230 231 /** 232 * @return {@link #identifier} (Identifier associated with the package/container 233 * (usually a label affixed directly).) 234 */ 235 public Identifier getIdentifier() { 236 if (this.identifier == null) 237 if (Configuration.errorOnAutoCreate()) 238 throw new Error("Attempt to auto-create SubstanceInstanceComponent.identifier"); 239 else if (Configuration.doAutoCreate()) 240 this.identifier = new Identifier(); // cc 241 return this.identifier; 242 } 243 244 public boolean hasIdentifier() { 245 return this.identifier != null && !this.identifier.isEmpty(); 246 } 247 248 /** 249 * @param value {@link #identifier} (Identifier associated with the 250 * package/container (usually a label affixed directly).) 251 */ 252 public SubstanceInstanceComponent setIdentifier(Identifier value) { 253 this.identifier = value; 254 return this; 255 } 256 257 /** 258 * @return {@link #expiry} (When the substance is no longer valid to use. For 259 * some substances, a single arbitrary date is used for expiry.). This 260 * is the underlying object with id, value and extensions. The accessor 261 * "getExpiry" gives direct access to the value 262 */ 263 public DateTimeType getExpiryElement() { 264 if (this.expiry == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create SubstanceInstanceComponent.expiry"); 267 else if (Configuration.doAutoCreate()) 268 this.expiry = new DateTimeType(); // bb 269 return this.expiry; 270 } 271 272 public boolean hasExpiryElement() { 273 return this.expiry != null && !this.expiry.isEmpty(); 274 } 275 276 public boolean hasExpiry() { 277 return this.expiry != null && !this.expiry.isEmpty(); 278 } 279 280 /** 281 * @param value {@link #expiry} (When the substance is no longer valid to use. 282 * For some substances, a single arbitrary date is used for 283 * expiry.). This is the underlying object with id, value and 284 * extensions. The accessor "getExpiry" gives direct access to the 285 * value 286 */ 287 public SubstanceInstanceComponent setExpiryElement(DateTimeType value) { 288 this.expiry = value; 289 return this; 290 } 291 292 /** 293 * @return When the substance is no longer valid to use. For some substances, a 294 * single arbitrary date is used for expiry. 295 */ 296 public Date getExpiry() { 297 return this.expiry == null ? null : this.expiry.getValue(); 298 } 299 300 /** 301 * @param value When the substance is no longer valid to use. For some 302 * substances, a single arbitrary date is used for expiry. 303 */ 304 public SubstanceInstanceComponent setExpiry(Date value) { 305 if (value == null) 306 this.expiry = null; 307 else { 308 if (this.expiry == null) 309 this.expiry = new DateTimeType(); 310 this.expiry.setValue(value); 311 } 312 return this; 313 } 314 315 /** 316 * @return {@link #quantity} (The amount of the substance.) 317 */ 318 public Quantity getQuantity() { 319 if (this.quantity == null) 320 if (Configuration.errorOnAutoCreate()) 321 throw new Error("Attempt to auto-create SubstanceInstanceComponent.quantity"); 322 else if (Configuration.doAutoCreate()) 323 this.quantity = new Quantity(); // cc 324 return this.quantity; 325 } 326 327 public boolean hasQuantity() { 328 return this.quantity != null && !this.quantity.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #quantity} (The amount of the substance.) 333 */ 334 public SubstanceInstanceComponent setQuantity(Quantity value) { 335 this.quantity = value; 336 return this; 337 } 338 339 protected void listChildren(List<Property> children) { 340 super.listChildren(children); 341 children.add(new Property("identifier", "Identifier", 342 "Identifier associated with the package/container (usually a label affixed directly).", 0, 1, identifier)); 343 children.add(new Property("expiry", "dateTime", 344 "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 345 0, 1, expiry)); 346 children.add(new Property("quantity", "SimpleQuantity", "The amount of the substance.", 0, 1, quantity)); 347 } 348 349 @Override 350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 351 switch (_hash) { 352 case -1618432855: 353 /* identifier */ return new Property("identifier", "Identifier", 354 "Identifier associated with the package/container (usually a label affixed directly).", 0, 1, identifier); 355 case -1289159373: 356 /* expiry */ return new Property("expiry", "dateTime", 357 "When the substance is no longer valid to use. For some substances, a single arbitrary date is used for expiry.", 358 0, 1, expiry); 359 case -1285004149: 360 /* quantity */ return new Property("quantity", "SimpleQuantity", "The amount of the substance.", 0, 1, 361 quantity); 362 default: 363 return super.getNamedProperty(_hash, _name, _checkValid); 364 } 365 366 } 367 368 @Override 369 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 370 switch (hash) { 371 case -1618432855: 372 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 373 case -1289159373: 374 /* expiry */ return this.expiry == null ? new Base[0] : new Base[] { this.expiry }; // DateTimeType 375 case -1285004149: 376 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 377 default: 378 return super.getProperty(hash, name, checkValid); 379 } 380 381 } 382 383 @Override 384 public Base setProperty(int hash, String name, Base value) throws FHIRException { 385 switch (hash) { 386 case -1618432855: // identifier 387 this.identifier = castToIdentifier(value); // Identifier 388 return value; 389 case -1289159373: // expiry 390 this.expiry = castToDateTime(value); // DateTimeType 391 return value; 392 case -1285004149: // quantity 393 this.quantity = castToQuantity(value); // Quantity 394 return value; 395 default: 396 return super.setProperty(hash, name, value); 397 } 398 399 } 400 401 @Override 402 public Base setProperty(String name, Base value) throws FHIRException { 403 if (name.equals("identifier")) { 404 this.identifier = castToIdentifier(value); // Identifier 405 } else if (name.equals("expiry")) { 406 this.expiry = castToDateTime(value); // DateTimeType 407 } else if (name.equals("quantity")) { 408 this.quantity = castToQuantity(value); // Quantity 409 } else 410 return super.setProperty(name, value); 411 return value; 412 } 413 414 @Override 415 public void removeChild(String name, Base value) throws FHIRException { 416 if (name.equals("identifier")) { 417 this.identifier = null; 418 } else if (name.equals("expiry")) { 419 this.expiry = null; 420 } else if (name.equals("quantity")) { 421 this.quantity = null; 422 } else 423 super.removeChild(name, value); 424 425 } 426 427 @Override 428 public Base makeProperty(int hash, String name) throws FHIRException { 429 switch (hash) { 430 case -1618432855: 431 return getIdentifier(); 432 case -1289159373: 433 return getExpiryElement(); 434 case -1285004149: 435 return getQuantity(); 436 default: 437 return super.makeProperty(hash, name); 438 } 439 440 } 441 442 @Override 443 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 444 switch (hash) { 445 case -1618432855: 446 /* identifier */ return new String[] { "Identifier" }; 447 case -1289159373: 448 /* expiry */ return new String[] { "dateTime" }; 449 case -1285004149: 450 /* quantity */ return new String[] { "SimpleQuantity" }; 451 default: 452 return super.getTypesForProperty(hash, name); 453 } 454 455 } 456 457 @Override 458 public Base addChild(String name) throws FHIRException { 459 if (name.equals("identifier")) { 460 this.identifier = new Identifier(); 461 return this.identifier; 462 } else if (name.equals("expiry")) { 463 throw new FHIRException("Cannot call addChild on a singleton property Substance.expiry"); 464 } else if (name.equals("quantity")) { 465 this.quantity = new Quantity(); 466 return this.quantity; 467 } else 468 return super.addChild(name); 469 } 470 471 public SubstanceInstanceComponent copy() { 472 SubstanceInstanceComponent dst = new SubstanceInstanceComponent(); 473 copyValues(dst); 474 return dst; 475 } 476 477 public void copyValues(SubstanceInstanceComponent dst) { 478 super.copyValues(dst); 479 dst.identifier = identifier == null ? null : identifier.copy(); 480 dst.expiry = expiry == null ? null : expiry.copy(); 481 dst.quantity = quantity == null ? null : quantity.copy(); 482 } 483 484 @Override 485 public boolean equalsDeep(Base other_) { 486 if (!super.equalsDeep(other_)) 487 return false; 488 if (!(other_ instanceof SubstanceInstanceComponent)) 489 return false; 490 SubstanceInstanceComponent o = (SubstanceInstanceComponent) other_; 491 return compareDeep(identifier, o.identifier, true) && compareDeep(expiry, o.expiry, true) 492 && compareDeep(quantity, o.quantity, true); 493 } 494 495 @Override 496 public boolean equalsShallow(Base other_) { 497 if (!super.equalsShallow(other_)) 498 return false; 499 if (!(other_ instanceof SubstanceInstanceComponent)) 500 return false; 501 SubstanceInstanceComponent o = (SubstanceInstanceComponent) other_; 502 return compareValues(expiry, o.expiry, true); 503 } 504 505 public boolean isEmpty() { 506 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, expiry, quantity); 507 } 508 509 public String fhirType() { 510 return "Substance.instance"; 511 512 } 513 514 } 515 516 @Block() 517 public static class SubstanceIngredientComponent extends BackboneElement implements IBaseBackboneElement { 518 /** 519 * The amount of the ingredient in the substance - a concentration ratio. 520 */ 521 @Child(name = "quantity", type = { Ratio.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 522 @Description(shortDefinition = "Optional amount (concentration)", formalDefinition = "The amount of the ingredient in the substance - a concentration ratio.") 523 protected Ratio quantity; 524 525 /** 526 * Another substance that is a component of this substance. 527 */ 528 @Child(name = "substance", type = { CodeableConcept.class, 529 Substance.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 530 @Description(shortDefinition = "A component of the substance", formalDefinition = "Another substance that is a component of this substance.") 531 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/substance-code") 532 protected Type substance; 533 534 private static final long serialVersionUID = -469805322L; 535 536 /** 537 * Constructor 538 */ 539 public SubstanceIngredientComponent() { 540 super(); 541 } 542 543 /** 544 * Constructor 545 */ 546 public SubstanceIngredientComponent(Type substance) { 547 super(); 548 this.substance = substance; 549 } 550 551 /** 552 * @return {@link #quantity} (The amount of the ingredient in the substance - a 553 * concentration ratio.) 554 */ 555 public Ratio getQuantity() { 556 if (this.quantity == null) 557 if (Configuration.errorOnAutoCreate()) 558 throw new Error("Attempt to auto-create SubstanceIngredientComponent.quantity"); 559 else if (Configuration.doAutoCreate()) 560 this.quantity = new Ratio(); // cc 561 return this.quantity; 562 } 563 564 public boolean hasQuantity() { 565 return this.quantity != null && !this.quantity.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #quantity} (The amount of the ingredient in the substance 570 * - a concentration ratio.) 571 */ 572 public SubstanceIngredientComponent setQuantity(Ratio value) { 573 this.quantity = value; 574 return this; 575 } 576 577 /** 578 * @return {@link #substance} (Another substance that is a component of this 579 * substance.) 580 */ 581 public Type getSubstance() { 582 return this.substance; 583 } 584 585 /** 586 * @return {@link #substance} (Another substance that is a component of this 587 * substance.) 588 */ 589 public CodeableConcept getSubstanceCodeableConcept() throws FHIRException { 590 if (this.substance == null) 591 this.substance = new CodeableConcept(); 592 if (!(this.substance instanceof CodeableConcept)) 593 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 594 + this.substance.getClass().getName() + " was encountered"); 595 return (CodeableConcept) this.substance; 596 } 597 598 public boolean hasSubstanceCodeableConcept() { 599 return this.substance instanceof CodeableConcept; 600 } 601 602 /** 603 * @return {@link #substance} (Another substance that is a component of this 604 * substance.) 605 */ 606 public Reference getSubstanceReference() throws FHIRException { 607 if (this.substance == null) 608 this.substance = new Reference(); 609 if (!(this.substance instanceof Reference)) 610 throw new FHIRException("Type mismatch: the type Reference was expected, but " 611 + this.substance.getClass().getName() + " was encountered"); 612 return (Reference) this.substance; 613 } 614 615 public boolean hasSubstanceReference() { 616 return this.substance instanceof Reference; 617 } 618 619 public boolean hasSubstance() { 620 return this.substance != null && !this.substance.isEmpty(); 621 } 622 623 /** 624 * @param value {@link #substance} (Another substance that is a component of 625 * this substance.) 626 */ 627 public SubstanceIngredientComponent setSubstance(Type value) { 628 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 629 throw new Error("Not the right type for Substance.ingredient.substance[x]: " + value.fhirType()); 630 this.substance = value; 631 return this; 632 } 633 634 protected void listChildren(List<Property> children) { 635 super.listChildren(children); 636 children.add(new Property("quantity", "Ratio", 637 "The amount of the ingredient in the substance - a concentration ratio.", 0, 1, quantity)); 638 children.add(new Property("substance[x]", "CodeableConcept|Reference(Substance)", 639 "Another substance that is a component of this substance.", 0, 1, substance)); 640 } 641 642 @Override 643 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 644 switch (_hash) { 645 case -1285004149: 646 /* quantity */ return new Property("quantity", "Ratio", 647 "The amount of the ingredient in the substance - a concentration ratio.", 0, 1, quantity); 648 case 2127194384: 649 /* substance[x] */ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", 650 "Another substance that is a component of this substance.", 0, 1, substance); 651 case 530040176: 652 /* substance */ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", 653 "Another substance that is a component of this substance.", 0, 1, substance); 654 case -1974119407: 655 /* substanceCodeableConcept */ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", 656 "Another substance that is a component of this substance.", 0, 1, substance); 657 case 516208571: 658 /* substanceReference */ return new Property("substance[x]", "CodeableConcept|Reference(Substance)", 659 "Another substance that is a component of this substance.", 0, 1, substance); 660 default: 661 return super.getNamedProperty(_hash, _name, _checkValid); 662 } 663 664 } 665 666 @Override 667 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 668 switch (hash) { 669 case -1285004149: 670 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Ratio 671 case 530040176: 672 /* substance */ return this.substance == null ? new Base[0] : new Base[] { this.substance }; // Type 673 default: 674 return super.getProperty(hash, name, checkValid); 675 } 676 677 } 678 679 @Override 680 public Base setProperty(int hash, String name, Base value) throws FHIRException { 681 switch (hash) { 682 case -1285004149: // quantity 683 this.quantity = castToRatio(value); // Ratio 684 return value; 685 case 530040176: // substance 686 this.substance = castToType(value); // Type 687 return value; 688 default: 689 return super.setProperty(hash, name, value); 690 } 691 692 } 693 694 @Override 695 public Base setProperty(String name, Base value) throws FHIRException { 696 if (name.equals("quantity")) { 697 this.quantity = castToRatio(value); // Ratio 698 } else if (name.equals("substance[x]")) { 699 this.substance = castToType(value); // Type 700 } else 701 return super.setProperty(name, value); 702 return value; 703 } 704 705 @Override 706 public void removeChild(String name, Base value) throws FHIRException { 707 if (name.equals("quantity")) { 708 this.quantity = null; 709 } else if (name.equals("substance[x]")) { 710 this.substance = null; 711 } else 712 super.removeChild(name, value); 713 714 } 715 716 @Override 717 public Base makeProperty(int hash, String name) throws FHIRException { 718 switch (hash) { 719 case -1285004149: 720 return getQuantity(); 721 case 2127194384: 722 return getSubstance(); 723 case 530040176: 724 return getSubstance(); 725 default: 726 return super.makeProperty(hash, name); 727 } 728 729 } 730 731 @Override 732 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 733 switch (hash) { 734 case -1285004149: 735 /* quantity */ return new String[] { "Ratio" }; 736 case 530040176: 737 /* substance */ return new String[] { "CodeableConcept", "Reference" }; 738 default: 739 return super.getTypesForProperty(hash, name); 740 } 741 742 } 743 744 @Override 745 public Base addChild(String name) throws FHIRException { 746 if (name.equals("quantity")) { 747 this.quantity = new Ratio(); 748 return this.quantity; 749 } else if (name.equals("substanceCodeableConcept")) { 750 this.substance = new CodeableConcept(); 751 return this.substance; 752 } else if (name.equals("substanceReference")) { 753 this.substance = new Reference(); 754 return this.substance; 755 } else 756 return super.addChild(name); 757 } 758 759 public SubstanceIngredientComponent copy() { 760 SubstanceIngredientComponent dst = new SubstanceIngredientComponent(); 761 copyValues(dst); 762 return dst; 763 } 764 765 public void copyValues(SubstanceIngredientComponent dst) { 766 super.copyValues(dst); 767 dst.quantity = quantity == null ? null : quantity.copy(); 768 dst.substance = substance == null ? null : substance.copy(); 769 } 770 771 @Override 772 public boolean equalsDeep(Base other_) { 773 if (!super.equalsDeep(other_)) 774 return false; 775 if (!(other_ instanceof SubstanceIngredientComponent)) 776 return false; 777 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other_; 778 return compareDeep(quantity, o.quantity, true) && compareDeep(substance, o.substance, true); 779 } 780 781 @Override 782 public boolean equalsShallow(Base other_) { 783 if (!super.equalsShallow(other_)) 784 return false; 785 if (!(other_ instanceof SubstanceIngredientComponent)) 786 return false; 787 SubstanceIngredientComponent o = (SubstanceIngredientComponent) other_; 788 return true; 789 } 790 791 public boolean isEmpty() { 792 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, substance); 793 } 794 795 public String fhirType() { 796 return "Substance.ingredient"; 797 798 } 799 800 } 801 802 /** 803 * Unique identifier for the substance. 804 */ 805 @Child(name = "identifier", type = { 806 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 807 @Description(shortDefinition = "Unique identifier", formalDefinition = "Unique identifier for the substance.") 808 protected List<Identifier> identifier; 809 810 /** 811 * A code to indicate if the substance is actively used. 812 */ 813 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 814 @Description(shortDefinition = "active | inactive | entered-in-error", formalDefinition = "A code to indicate if the substance is actively used.") 815 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/substance-status") 816 protected Enumeration<FHIRSubstanceStatus> status; 817 818 /** 819 * A code that classifies the general type of substance. This is used for 820 * searching, sorting and display purposes. 821 */ 822 @Child(name = "category", type = { 823 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 824 @Description(shortDefinition = "What class/type of substance this is", formalDefinition = "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.") 825 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/substance-category") 826 protected List<CodeableConcept> category; 827 828 /** 829 * A code (or set of codes) that identify this substance. 830 */ 831 @Child(name = "code", type = { CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 832 @Description(shortDefinition = "What substance this is", formalDefinition = "A code (or set of codes) that identify this substance.") 833 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/substance-code") 834 protected CodeableConcept code; 835 836 /** 837 * A description of the substance - its appearance, handling requirements, and 838 * other usage notes. 839 */ 840 @Child(name = "description", type = { 841 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 842 @Description(shortDefinition = "Textual description of the substance, comments", formalDefinition = "A description of the substance - its appearance, handling requirements, and other usage notes.") 843 protected StringType description; 844 845 /** 846 * Substance may be used to describe a kind of substance, or a specific 847 * package/container of the substance: an instance. 848 */ 849 @Child(name = "instance", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 850 @Description(shortDefinition = "If this describes a specific package/container of the substance", formalDefinition = "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.") 851 protected List<SubstanceInstanceComponent> instance; 852 853 /** 854 * A substance can be composed of other substances. 855 */ 856 @Child(name = "ingredient", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 857 @Description(shortDefinition = "Composition information about the substance", formalDefinition = "A substance can be composed of other substances.") 858 protected List<SubstanceIngredientComponent> ingredient; 859 860 private static final long serialVersionUID = -1467626602L; 861 862 /** 863 * Constructor 864 */ 865 public Substance() { 866 super(); 867 } 868 869 /** 870 * Constructor 871 */ 872 public Substance(CodeableConcept code) { 873 super(); 874 this.code = code; 875 } 876 877 /** 878 * @return {@link #identifier} (Unique identifier for the substance.) 879 */ 880 public List<Identifier> getIdentifier() { 881 if (this.identifier == null) 882 this.identifier = new ArrayList<Identifier>(); 883 return this.identifier; 884 } 885 886 /** 887 * @return Returns a reference to <code>this</code> for easy method chaining 888 */ 889 public Substance setIdentifier(List<Identifier> theIdentifier) { 890 this.identifier = theIdentifier; 891 return this; 892 } 893 894 public boolean hasIdentifier() { 895 if (this.identifier == null) 896 return false; 897 for (Identifier item : this.identifier) 898 if (!item.isEmpty()) 899 return true; 900 return false; 901 } 902 903 public Identifier addIdentifier() { // 3 904 Identifier t = new Identifier(); 905 if (this.identifier == null) 906 this.identifier = new ArrayList<Identifier>(); 907 this.identifier.add(t); 908 return t; 909 } 910 911 public Substance addIdentifier(Identifier t) { // 3 912 if (t == null) 913 return this; 914 if (this.identifier == null) 915 this.identifier = new ArrayList<Identifier>(); 916 this.identifier.add(t); 917 return this; 918 } 919 920 /** 921 * @return The first repetition of repeating field {@link #identifier}, creating 922 * it if it does not already exist 923 */ 924 public Identifier getIdentifierFirstRep() { 925 if (getIdentifier().isEmpty()) { 926 addIdentifier(); 927 } 928 return getIdentifier().get(0); 929 } 930 931 /** 932 * @return {@link #status} (A code to indicate if the substance is actively 933 * used.). This is the underlying object with id, value and extensions. 934 * The accessor "getStatus" gives direct access to the value 935 */ 936 public Enumeration<FHIRSubstanceStatus> getStatusElement() { 937 if (this.status == null) 938 if (Configuration.errorOnAutoCreate()) 939 throw new Error("Attempt to auto-create Substance.status"); 940 else if (Configuration.doAutoCreate()) 941 this.status = new Enumeration<FHIRSubstanceStatus>(new FHIRSubstanceStatusEnumFactory()); // bb 942 return this.status; 943 } 944 945 public boolean hasStatusElement() { 946 return this.status != null && !this.status.isEmpty(); 947 } 948 949 public boolean hasStatus() { 950 return this.status != null && !this.status.isEmpty(); 951 } 952 953 /** 954 * @param value {@link #status} (A code to indicate if the substance is actively 955 * used.). This is the underlying object with id, value and 956 * extensions. The accessor "getStatus" gives direct access to the 957 * value 958 */ 959 public Substance setStatusElement(Enumeration<FHIRSubstanceStatus> value) { 960 this.status = value; 961 return this; 962 } 963 964 /** 965 * @return A code to indicate if the substance is actively used. 966 */ 967 public FHIRSubstanceStatus getStatus() { 968 return this.status == null ? null : this.status.getValue(); 969 } 970 971 /** 972 * @param value A code to indicate if the substance is actively used. 973 */ 974 public Substance setStatus(FHIRSubstanceStatus value) { 975 if (value == null) 976 this.status = null; 977 else { 978 if (this.status == null) 979 this.status = new Enumeration<FHIRSubstanceStatus>(new FHIRSubstanceStatusEnumFactory()); 980 this.status.setValue(value); 981 } 982 return this; 983 } 984 985 /** 986 * @return {@link #category} (A code that classifies the general type of 987 * substance. This is used for searching, sorting and display purposes.) 988 */ 989 public List<CodeableConcept> getCategory() { 990 if (this.category == null) 991 this.category = new ArrayList<CodeableConcept>(); 992 return this.category; 993 } 994 995 /** 996 * @return Returns a reference to <code>this</code> for easy method chaining 997 */ 998 public Substance setCategory(List<CodeableConcept> theCategory) { 999 this.category = theCategory; 1000 return this; 1001 } 1002 1003 public boolean hasCategory() { 1004 if (this.category == null) 1005 return false; 1006 for (CodeableConcept item : this.category) 1007 if (!item.isEmpty()) 1008 return true; 1009 return false; 1010 } 1011 1012 public CodeableConcept addCategory() { // 3 1013 CodeableConcept t = new CodeableConcept(); 1014 if (this.category == null) 1015 this.category = new ArrayList<CodeableConcept>(); 1016 this.category.add(t); 1017 return t; 1018 } 1019 1020 public Substance addCategory(CodeableConcept t) { // 3 1021 if (t == null) 1022 return this; 1023 if (this.category == null) 1024 this.category = new ArrayList<CodeableConcept>(); 1025 this.category.add(t); 1026 return this; 1027 } 1028 1029 /** 1030 * @return The first repetition of repeating field {@link #category}, creating 1031 * it if it does not already exist 1032 */ 1033 public CodeableConcept getCategoryFirstRep() { 1034 if (getCategory().isEmpty()) { 1035 addCategory(); 1036 } 1037 return getCategory().get(0); 1038 } 1039 1040 /** 1041 * @return {@link #code} (A code (or set of codes) that identify this 1042 * substance.) 1043 */ 1044 public CodeableConcept getCode() { 1045 if (this.code == null) 1046 if (Configuration.errorOnAutoCreate()) 1047 throw new Error("Attempt to auto-create Substance.code"); 1048 else if (Configuration.doAutoCreate()) 1049 this.code = new CodeableConcept(); // cc 1050 return this.code; 1051 } 1052 1053 public boolean hasCode() { 1054 return this.code != null && !this.code.isEmpty(); 1055 } 1056 1057 /** 1058 * @param value {@link #code} (A code (or set of codes) that identify this 1059 * substance.) 1060 */ 1061 public Substance setCode(CodeableConcept value) { 1062 this.code = value; 1063 return this; 1064 } 1065 1066 /** 1067 * @return {@link #description} (A description of the substance - its 1068 * appearance, handling requirements, and other usage notes.). This is 1069 * the underlying object with id, value and extensions. The accessor 1070 * "getDescription" gives direct access to the value 1071 */ 1072 public StringType getDescriptionElement() { 1073 if (this.description == null) 1074 if (Configuration.errorOnAutoCreate()) 1075 throw new Error("Attempt to auto-create Substance.description"); 1076 else if (Configuration.doAutoCreate()) 1077 this.description = new StringType(); // bb 1078 return this.description; 1079 } 1080 1081 public boolean hasDescriptionElement() { 1082 return this.description != null && !this.description.isEmpty(); 1083 } 1084 1085 public boolean hasDescription() { 1086 return this.description != null && !this.description.isEmpty(); 1087 } 1088 1089 /** 1090 * @param value {@link #description} (A description of the substance - its 1091 * appearance, handling requirements, and other usage notes.). This 1092 * is the underlying object with id, value and extensions. The 1093 * accessor "getDescription" gives direct access to the value 1094 */ 1095 public Substance setDescriptionElement(StringType value) { 1096 this.description = value; 1097 return this; 1098 } 1099 1100 /** 1101 * @return A description of the substance - its appearance, handling 1102 * requirements, and other usage notes. 1103 */ 1104 public String getDescription() { 1105 return this.description == null ? null : this.description.getValue(); 1106 } 1107 1108 /** 1109 * @param value A description of the substance - its appearance, handling 1110 * requirements, and other usage notes. 1111 */ 1112 public Substance setDescription(String value) { 1113 if (Utilities.noString(value)) 1114 this.description = null; 1115 else { 1116 if (this.description == null) 1117 this.description = new StringType(); 1118 this.description.setValue(value); 1119 } 1120 return this; 1121 } 1122 1123 /** 1124 * @return {@link #instance} (Substance may be used to describe a kind of 1125 * substance, or a specific package/container of the substance: an 1126 * instance.) 1127 */ 1128 public List<SubstanceInstanceComponent> getInstance() { 1129 if (this.instance == null) 1130 this.instance = new ArrayList<SubstanceInstanceComponent>(); 1131 return this.instance; 1132 } 1133 1134 /** 1135 * @return Returns a reference to <code>this</code> for easy method chaining 1136 */ 1137 public Substance setInstance(List<SubstanceInstanceComponent> theInstance) { 1138 this.instance = theInstance; 1139 return this; 1140 } 1141 1142 public boolean hasInstance() { 1143 if (this.instance == null) 1144 return false; 1145 for (SubstanceInstanceComponent item : this.instance) 1146 if (!item.isEmpty()) 1147 return true; 1148 return false; 1149 } 1150 1151 public SubstanceInstanceComponent addInstance() { // 3 1152 SubstanceInstanceComponent t = new SubstanceInstanceComponent(); 1153 if (this.instance == null) 1154 this.instance = new ArrayList<SubstanceInstanceComponent>(); 1155 this.instance.add(t); 1156 return t; 1157 } 1158 1159 public Substance addInstance(SubstanceInstanceComponent t) { // 3 1160 if (t == null) 1161 return this; 1162 if (this.instance == null) 1163 this.instance = new ArrayList<SubstanceInstanceComponent>(); 1164 this.instance.add(t); 1165 return this; 1166 } 1167 1168 /** 1169 * @return The first repetition of repeating field {@link #instance}, creating 1170 * it if it does not already exist 1171 */ 1172 public SubstanceInstanceComponent getInstanceFirstRep() { 1173 if (getInstance().isEmpty()) { 1174 addInstance(); 1175 } 1176 return getInstance().get(0); 1177 } 1178 1179 /** 1180 * @return {@link #ingredient} (A substance can be composed of other 1181 * substances.) 1182 */ 1183 public List<SubstanceIngredientComponent> getIngredient() { 1184 if (this.ingredient == null) 1185 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1186 return this.ingredient; 1187 } 1188 1189 /** 1190 * @return Returns a reference to <code>this</code> for easy method chaining 1191 */ 1192 public Substance setIngredient(List<SubstanceIngredientComponent> theIngredient) { 1193 this.ingredient = theIngredient; 1194 return this; 1195 } 1196 1197 public boolean hasIngredient() { 1198 if (this.ingredient == null) 1199 return false; 1200 for (SubstanceIngredientComponent item : this.ingredient) 1201 if (!item.isEmpty()) 1202 return true; 1203 return false; 1204 } 1205 1206 public SubstanceIngredientComponent addIngredient() { // 3 1207 SubstanceIngredientComponent t = new SubstanceIngredientComponent(); 1208 if (this.ingredient == null) 1209 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1210 this.ingredient.add(t); 1211 return t; 1212 } 1213 1214 public Substance addIngredient(SubstanceIngredientComponent t) { // 3 1215 if (t == null) 1216 return this; 1217 if (this.ingredient == null) 1218 this.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1219 this.ingredient.add(t); 1220 return this; 1221 } 1222 1223 /** 1224 * @return The first repetition of repeating field {@link #ingredient}, creating 1225 * it if it does not already exist 1226 */ 1227 public SubstanceIngredientComponent getIngredientFirstRep() { 1228 if (getIngredient().isEmpty()) { 1229 addIngredient(); 1230 } 1231 return getIngredient().get(0); 1232 } 1233 1234 protected void listChildren(List<Property> children) { 1235 super.listChildren(children); 1236 children.add(new Property("identifier", "Identifier", "Unique identifier for the substance.", 0, 1237 java.lang.Integer.MAX_VALUE, identifier)); 1238 children.add(new Property("status", "code", "A code to indicate if the substance is actively used.", 0, 1, status)); 1239 children.add(new Property("category", "CodeableConcept", 1240 "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 1241 0, java.lang.Integer.MAX_VALUE, category)); 1242 children.add( 1243 new Property("code", "CodeableConcept", "A code (or set of codes) that identify this substance.", 0, 1, code)); 1244 children.add(new Property("description", "string", 1245 "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 1, 1246 description)); 1247 children.add(new Property("instance", "", 1248 "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.", 1249 0, java.lang.Integer.MAX_VALUE, instance)); 1250 children.add(new Property("ingredient", "", "A substance can be composed of other substances.", 0, 1251 java.lang.Integer.MAX_VALUE, ingredient)); 1252 } 1253 1254 @Override 1255 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1256 switch (_hash) { 1257 case -1618432855: 1258 /* identifier */ return new Property("identifier", "Identifier", "Unique identifier for the substance.", 0, 1259 java.lang.Integer.MAX_VALUE, identifier); 1260 case -892481550: 1261 /* status */ return new Property("status", "code", "A code to indicate if the substance is actively used.", 0, 1, 1262 status); 1263 case 50511102: 1264 /* category */ return new Property("category", "CodeableConcept", 1265 "A code that classifies the general type of substance. This is used for searching, sorting and display purposes.", 1266 0, java.lang.Integer.MAX_VALUE, category); 1267 case 3059181: 1268 /* code */ return new Property("code", "CodeableConcept", 1269 "A code (or set of codes) that identify this substance.", 0, 1, code); 1270 case -1724546052: 1271 /* description */ return new Property("description", "string", 1272 "A description of the substance - its appearance, handling requirements, and other usage notes.", 0, 1, 1273 description); 1274 case 555127957: 1275 /* instance */ return new Property("instance", "", 1276 "Substance may be used to describe a kind of substance, or a specific package/container of the substance: an instance.", 1277 0, java.lang.Integer.MAX_VALUE, instance); 1278 case -206409263: 1279 /* ingredient */ return new Property("ingredient", "", "A substance can be composed of other substances.", 0, 1280 java.lang.Integer.MAX_VALUE, ingredient); 1281 default: 1282 return super.getNamedProperty(_hash, _name, _checkValid); 1283 } 1284 1285 } 1286 1287 @Override 1288 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1289 switch (hash) { 1290 case -1618432855: 1291 /* identifier */ return this.identifier == null ? new Base[0] 1292 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1293 case -892481550: 1294 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<FHIRSubstanceStatus> 1295 case 50511102: 1296 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1297 case 3059181: 1298 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1299 case -1724546052: 1300 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1301 case 555127957: 1302 /* instance */ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // SubstanceInstanceComponent 1303 case -206409263: 1304 /* ingredient */ return this.ingredient == null ? new Base[0] 1305 : this.ingredient.toArray(new Base[this.ingredient.size()]); // SubstanceIngredientComponent 1306 default: 1307 return super.getProperty(hash, name, checkValid); 1308 } 1309 1310 } 1311 1312 @Override 1313 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1314 switch (hash) { 1315 case -1618432855: // identifier 1316 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1317 return value; 1318 case -892481550: // status 1319 value = new FHIRSubstanceStatusEnumFactory().fromType(castToCode(value)); 1320 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 1321 return value; 1322 case 50511102: // category 1323 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1324 return value; 1325 case 3059181: // code 1326 this.code = castToCodeableConcept(value); // CodeableConcept 1327 return value; 1328 case -1724546052: // description 1329 this.description = castToString(value); // StringType 1330 return value; 1331 case 555127957: // instance 1332 this.getInstance().add((SubstanceInstanceComponent) value); // SubstanceInstanceComponent 1333 return value; 1334 case -206409263: // ingredient 1335 this.getIngredient().add((SubstanceIngredientComponent) value); // SubstanceIngredientComponent 1336 return value; 1337 default: 1338 return super.setProperty(hash, name, value); 1339 } 1340 1341 } 1342 1343 @Override 1344 public Base setProperty(String name, Base value) throws FHIRException { 1345 if (name.equals("identifier")) { 1346 this.getIdentifier().add(castToIdentifier(value)); 1347 } else if (name.equals("status")) { 1348 value = new FHIRSubstanceStatusEnumFactory().fromType(castToCode(value)); 1349 this.status = (Enumeration) value; // Enumeration<FHIRSubstanceStatus> 1350 } else if (name.equals("category")) { 1351 this.getCategory().add(castToCodeableConcept(value)); 1352 } else if (name.equals("code")) { 1353 this.code = castToCodeableConcept(value); // CodeableConcept 1354 } else if (name.equals("description")) { 1355 this.description = castToString(value); // StringType 1356 } else if (name.equals("instance")) { 1357 this.getInstance().add((SubstanceInstanceComponent) value); 1358 } else if (name.equals("ingredient")) { 1359 this.getIngredient().add((SubstanceIngredientComponent) value); 1360 } else 1361 return super.setProperty(name, value); 1362 return value; 1363 } 1364 1365 @Override 1366 public void removeChild(String name, Base value) throws FHIRException { 1367 if (name.equals("identifier")) { 1368 this.getIdentifier().remove(castToIdentifier(value)); 1369 } else if (name.equals("status")) { 1370 this.status = null; 1371 } else if (name.equals("category")) { 1372 this.getCategory().remove(castToCodeableConcept(value)); 1373 } else if (name.equals("code")) { 1374 this.code = null; 1375 } else if (name.equals("description")) { 1376 this.description = null; 1377 } else if (name.equals("instance")) { 1378 this.getInstance().remove((SubstanceInstanceComponent) value); 1379 } else if (name.equals("ingredient")) { 1380 this.getIngredient().remove((SubstanceIngredientComponent) value); 1381 } else 1382 super.removeChild(name, value); 1383 1384 } 1385 1386 @Override 1387 public Base makeProperty(int hash, String name) throws FHIRException { 1388 switch (hash) { 1389 case -1618432855: 1390 return addIdentifier(); 1391 case -892481550: 1392 return getStatusElement(); 1393 case 50511102: 1394 return addCategory(); 1395 case 3059181: 1396 return getCode(); 1397 case -1724546052: 1398 return getDescriptionElement(); 1399 case 555127957: 1400 return addInstance(); 1401 case -206409263: 1402 return addIngredient(); 1403 default: 1404 return super.makeProperty(hash, name); 1405 } 1406 1407 } 1408 1409 @Override 1410 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1411 switch (hash) { 1412 case -1618432855: 1413 /* identifier */ return new String[] { "Identifier" }; 1414 case -892481550: 1415 /* status */ return new String[] { "code" }; 1416 case 50511102: 1417 /* category */ return new String[] { "CodeableConcept" }; 1418 case 3059181: 1419 /* code */ return new String[] { "CodeableConcept" }; 1420 case -1724546052: 1421 /* description */ return new String[] { "string" }; 1422 case 555127957: 1423 /* instance */ return new String[] {}; 1424 case -206409263: 1425 /* ingredient */ return new String[] {}; 1426 default: 1427 return super.getTypesForProperty(hash, name); 1428 } 1429 1430 } 1431 1432 @Override 1433 public Base addChild(String name) throws FHIRException { 1434 if (name.equals("identifier")) { 1435 return addIdentifier(); 1436 } else if (name.equals("status")) { 1437 throw new FHIRException("Cannot call addChild on a singleton property Substance.status"); 1438 } else if (name.equals("category")) { 1439 return addCategory(); 1440 } else if (name.equals("code")) { 1441 this.code = new CodeableConcept(); 1442 return this.code; 1443 } else if (name.equals("description")) { 1444 throw new FHIRException("Cannot call addChild on a singleton property Substance.description"); 1445 } else if (name.equals("instance")) { 1446 return addInstance(); 1447 } else if (name.equals("ingredient")) { 1448 return addIngredient(); 1449 } else 1450 return super.addChild(name); 1451 } 1452 1453 public String fhirType() { 1454 return "Substance"; 1455 1456 } 1457 1458 public Substance copy() { 1459 Substance dst = new Substance(); 1460 copyValues(dst); 1461 return dst; 1462 } 1463 1464 public void copyValues(Substance dst) { 1465 super.copyValues(dst); 1466 if (identifier != null) { 1467 dst.identifier = new ArrayList<Identifier>(); 1468 for (Identifier i : identifier) 1469 dst.identifier.add(i.copy()); 1470 } 1471 ; 1472 dst.status = status == null ? null : status.copy(); 1473 if (category != null) { 1474 dst.category = new ArrayList<CodeableConcept>(); 1475 for (CodeableConcept i : category) 1476 dst.category.add(i.copy()); 1477 } 1478 ; 1479 dst.code = code == null ? null : code.copy(); 1480 dst.description = description == null ? null : description.copy(); 1481 if (instance != null) { 1482 dst.instance = new ArrayList<SubstanceInstanceComponent>(); 1483 for (SubstanceInstanceComponent i : instance) 1484 dst.instance.add(i.copy()); 1485 } 1486 ; 1487 if (ingredient != null) { 1488 dst.ingredient = new ArrayList<SubstanceIngredientComponent>(); 1489 for (SubstanceIngredientComponent i : ingredient) 1490 dst.ingredient.add(i.copy()); 1491 } 1492 ; 1493 } 1494 1495 protected Substance typedCopy() { 1496 return copy(); 1497 } 1498 1499 @Override 1500 public boolean equalsDeep(Base other_) { 1501 if (!super.equalsDeep(other_)) 1502 return false; 1503 if (!(other_ instanceof Substance)) 1504 return false; 1505 Substance o = (Substance) other_; 1506 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1507 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1508 && compareDeep(description, o.description, true) && compareDeep(instance, o.instance, true) 1509 && compareDeep(ingredient, o.ingredient, true); 1510 } 1511 1512 @Override 1513 public boolean equalsShallow(Base other_) { 1514 if (!super.equalsShallow(other_)) 1515 return false; 1516 if (!(other_ instanceof Substance)) 1517 return false; 1518 Substance o = (Substance) other_; 1519 return compareValues(status, o.status, true) && compareValues(description, o.description, true); 1520 } 1521 1522 public boolean isEmpty() { 1523 return super.isEmpty() 1524 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category, code, description, instance, ingredient); 1525 } 1526 1527 @Override 1528 public ResourceType getResourceType() { 1529 return ResourceType.Substance; 1530 } 1531 1532 /** 1533 * Search parameter: <b>identifier</b> 1534 * <p> 1535 * Description: <b>Unique identifier for the substance</b><br> 1536 * Type: <b>token</b><br> 1537 * Path: <b>Substance.identifier</b><br> 1538 * </p> 1539 */ 1540 @SearchParamDefinition(name = "identifier", path = "Substance.identifier", description = "Unique identifier for the substance", type = "token") 1541 public static final String SP_IDENTIFIER = "identifier"; 1542 /** 1543 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1544 * <p> 1545 * Description: <b>Unique identifier for the substance</b><br> 1546 * Type: <b>token</b><br> 1547 * Path: <b>Substance.identifier</b><br> 1548 * </p> 1549 */ 1550 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1551 SP_IDENTIFIER); 1552 1553 /** 1554 * Search parameter: <b>container-identifier</b> 1555 * <p> 1556 * Description: <b>Identifier of the package/container</b><br> 1557 * Type: <b>token</b><br> 1558 * Path: <b>Substance.instance.identifier</b><br> 1559 * </p> 1560 */ 1561 @SearchParamDefinition(name = "container-identifier", path = "Substance.instance.identifier", description = "Identifier of the package/container", type = "token") 1562 public static final String SP_CONTAINER_IDENTIFIER = "container-identifier"; 1563 /** 1564 * <b>Fluent Client</b> search parameter constant for 1565 * <b>container-identifier</b> 1566 * <p> 1567 * Description: <b>Identifier of the package/container</b><br> 1568 * Type: <b>token</b><br> 1569 * Path: <b>Substance.instance.identifier</b><br> 1570 * </p> 1571 */ 1572 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1573 SP_CONTAINER_IDENTIFIER); 1574 1575 /** 1576 * Search parameter: <b>code</b> 1577 * <p> 1578 * Description: <b>The code of the substance or ingredient</b><br> 1579 * Type: <b>token</b><br> 1580 * Path: <b>Substance.code, 1581 * Substance.ingredient.substanceCodeableConcept</b><br> 1582 * </p> 1583 */ 1584 @SearchParamDefinition(name = "code", path = "Substance.code | (Substance.ingredient.substance as CodeableConcept)", description = "The code of the substance or ingredient", type = "token") 1585 public static final String SP_CODE = "code"; 1586 /** 1587 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1588 * <p> 1589 * Description: <b>The code of the substance or ingredient</b><br> 1590 * Type: <b>token</b><br> 1591 * Path: <b>Substance.code, 1592 * Substance.ingredient.substanceCodeableConcept</b><br> 1593 * </p> 1594 */ 1595 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1596 SP_CODE); 1597 1598 /** 1599 * Search parameter: <b>quantity</b> 1600 * <p> 1601 * Description: <b>Amount of substance in the package</b><br> 1602 * Type: <b>quantity</b><br> 1603 * Path: <b>Substance.instance.quantity</b><br> 1604 * </p> 1605 */ 1606 @SearchParamDefinition(name = "quantity", path = "Substance.instance.quantity", description = "Amount of substance in the package", type = "quantity") 1607 public static final String SP_QUANTITY = "quantity"; 1608 /** 1609 * <b>Fluent Client</b> search parameter constant for <b>quantity</b> 1610 * <p> 1611 * Description: <b>Amount of substance in the package</b><br> 1612 * Type: <b>quantity</b><br> 1613 * Path: <b>Substance.instance.quantity</b><br> 1614 * </p> 1615 */ 1616 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 1617 SP_QUANTITY); 1618 1619 /** 1620 * Search parameter: <b>substance-reference</b> 1621 * <p> 1622 * Description: <b>A component of the substance</b><br> 1623 * Type: <b>reference</b><br> 1624 * Path: <b>Substance.ingredient.substanceReference</b><br> 1625 * </p> 1626 */ 1627 @SearchParamDefinition(name = "substance-reference", path = "(Substance.ingredient.substance as Reference)", description = "A component of the substance", type = "reference", target = { 1628 Substance.class }) 1629 public static final String SP_SUBSTANCE_REFERENCE = "substance-reference"; 1630 /** 1631 * <b>Fluent Client</b> search parameter constant for <b>substance-reference</b> 1632 * <p> 1633 * Description: <b>A component of the substance</b><br> 1634 * Type: <b>reference</b><br> 1635 * Path: <b>Substance.ingredient.substanceReference</b><br> 1636 * </p> 1637 */ 1638 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1639 SP_SUBSTANCE_REFERENCE); 1640 1641 /** 1642 * Constant for fluent queries to be used to add include statements. Specifies 1643 * the path value of "<b>Substance:substance-reference</b>". 1644 */ 1645 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE_REFERENCE = new ca.uhn.fhir.model.api.Include( 1646 "Substance:substance-reference").toLocked(); 1647 1648 /** 1649 * Search parameter: <b>expiry</b> 1650 * <p> 1651 * Description: <b>Expiry date of package or container of substance</b><br> 1652 * Type: <b>date</b><br> 1653 * Path: <b>Substance.instance.expiry</b><br> 1654 * </p> 1655 */ 1656 @SearchParamDefinition(name = "expiry", path = "Substance.instance.expiry", description = "Expiry date of package or container of substance", type = "date") 1657 public static final String SP_EXPIRY = "expiry"; 1658 /** 1659 * <b>Fluent Client</b> search parameter constant for <b>expiry</b> 1660 * <p> 1661 * Description: <b>Expiry date of package or container of substance</b><br> 1662 * Type: <b>date</b><br> 1663 * Path: <b>Substance.instance.expiry</b><br> 1664 * </p> 1665 */ 1666 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRY = new ca.uhn.fhir.rest.gclient.DateClientParam( 1667 SP_EXPIRY); 1668 1669 /** 1670 * Search parameter: <b>category</b> 1671 * <p> 1672 * Description: <b>The category of the substance</b><br> 1673 * Type: <b>token</b><br> 1674 * Path: <b>Substance.category</b><br> 1675 * </p> 1676 */ 1677 @SearchParamDefinition(name = "category", path = "Substance.category", description = "The category of the substance", type = "token") 1678 public static final String SP_CATEGORY = "category"; 1679 /** 1680 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1681 * <p> 1682 * Description: <b>The category of the substance</b><br> 1683 * Type: <b>token</b><br> 1684 * Path: <b>Substance.category</b><br> 1685 * </p> 1686 */ 1687 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1688 SP_CATEGORY); 1689 1690 /** 1691 * Search parameter: <b>status</b> 1692 * <p> 1693 * Description: <b>active | inactive | entered-in-error</b><br> 1694 * Type: <b>token</b><br> 1695 * Path: <b>Substance.status</b><br> 1696 * </p> 1697 */ 1698 @SearchParamDefinition(name = "status", path = "Substance.status", description = "active | inactive | entered-in-error", type = "token") 1699 public static final String SP_STATUS = "status"; 1700 /** 1701 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1702 * <p> 1703 * Description: <b>active | inactive | entered-in-error</b><br> 1704 * Type: <b>token</b><br> 1705 * Path: <b>Substance.status</b><br> 1706 * </p> 1707 */ 1708 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1709 SP_STATUS); 1710 1711}