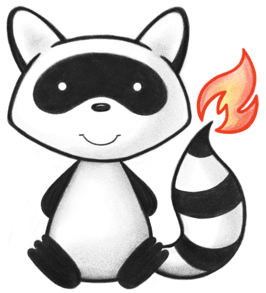
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * Chemical substances are a single substance type whose primary defining 047 * element is the molecular structure. Chemical substances shall be defined on 048 * the basis of their complete covalent molecular structure; the presence of a 049 * salt (counter-ion) and/or solvates (water, alcohols) is also captured. 050 * Purity, grade, physical form or particle size are not taken into account in 051 * the definition of a chemical substance or in the assignment of a Substance 052 * ID. 053 */ 054@DatatypeDef(name = "SubstanceAmount") 055public class SubstanceAmount extends BackboneType implements ICompositeType { 056 057 @Block() 058 public static class SubstanceAmountReferenceRangeComponent extends Element implements IBaseDatatypeElement { 059 /** 060 * Lower limit possible or expected. 061 */ 062 @Child(name = "lowLimit", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "Lower limit possible or expected", formalDefinition = "Lower limit possible or expected.") 064 protected Quantity lowLimit; 065 066 /** 067 * Upper limit possible or expected. 068 */ 069 @Child(name = "highLimit", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 070 @Description(shortDefinition = "Upper limit possible or expected", formalDefinition = "Upper limit possible or expected.") 071 protected Quantity highLimit; 072 073 private static final long serialVersionUID = -193230412L; 074 075 /** 076 * Constructor 077 */ 078 public SubstanceAmountReferenceRangeComponent() { 079 super(); 080 } 081 082 /** 083 * @return {@link #lowLimit} (Lower limit possible or expected.) 084 */ 085 public Quantity getLowLimit() { 086 if (this.lowLimit == null) 087 if (Configuration.errorOnAutoCreate()) 088 throw new Error("Attempt to auto-create SubstanceAmountReferenceRangeComponent.lowLimit"); 089 else if (Configuration.doAutoCreate()) 090 this.lowLimit = new Quantity(); // cc 091 return this.lowLimit; 092 } 093 094 public boolean hasLowLimit() { 095 return this.lowLimit != null && !this.lowLimit.isEmpty(); 096 } 097 098 /** 099 * @param value {@link #lowLimit} (Lower limit possible or expected.) 100 */ 101 public SubstanceAmountReferenceRangeComponent setLowLimit(Quantity value) { 102 this.lowLimit = value; 103 return this; 104 } 105 106 /** 107 * @return {@link #highLimit} (Upper limit possible or expected.) 108 */ 109 public Quantity getHighLimit() { 110 if (this.highLimit == null) 111 if (Configuration.errorOnAutoCreate()) 112 throw new Error("Attempt to auto-create SubstanceAmountReferenceRangeComponent.highLimit"); 113 else if (Configuration.doAutoCreate()) 114 this.highLimit = new Quantity(); // cc 115 return this.highLimit; 116 } 117 118 public boolean hasHighLimit() { 119 return this.highLimit != null && !this.highLimit.isEmpty(); 120 } 121 122 /** 123 * @param value {@link #highLimit} (Upper limit possible or expected.) 124 */ 125 public SubstanceAmountReferenceRangeComponent setHighLimit(Quantity value) { 126 this.highLimit = value; 127 return this; 128 } 129 130 protected void listChildren(List<Property> children) { 131 super.listChildren(children); 132 children.add(new Property("lowLimit", "Quantity", "Lower limit possible or expected.", 0, 1, lowLimit)); 133 children.add(new Property("highLimit", "Quantity", "Upper limit possible or expected.", 0, 1, highLimit)); 134 } 135 136 @Override 137 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 138 switch (_hash) { 139 case -1841058617: 140 /* lowLimit */ return new Property("lowLimit", "Quantity", "Lower limit possible or expected.", 0, 1, lowLimit); 141 case -710757575: 142 /* highLimit */ return new Property("highLimit", "Quantity", "Upper limit possible or expected.", 0, 1, 143 highLimit); 144 default: 145 return super.getNamedProperty(_hash, _name, _checkValid); 146 } 147 148 } 149 150 @Override 151 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 152 switch (hash) { 153 case -1841058617: 154 /* lowLimit */ return this.lowLimit == null ? new Base[0] : new Base[] { this.lowLimit }; // Quantity 155 case -710757575: 156 /* highLimit */ return this.highLimit == null ? new Base[0] : new Base[] { this.highLimit }; // Quantity 157 default: 158 return super.getProperty(hash, name, checkValid); 159 } 160 161 } 162 163 @Override 164 public Base setProperty(int hash, String name, Base value) throws FHIRException { 165 switch (hash) { 166 case -1841058617: // lowLimit 167 this.lowLimit = castToQuantity(value); // Quantity 168 return value; 169 case -710757575: // highLimit 170 this.highLimit = castToQuantity(value); // Quantity 171 return value; 172 default: 173 return super.setProperty(hash, name, value); 174 } 175 176 } 177 178 @Override 179 public Base setProperty(String name, Base value) throws FHIRException { 180 if (name.equals("lowLimit")) { 181 this.lowLimit = castToQuantity(value); // Quantity 182 } else if (name.equals("highLimit")) { 183 this.highLimit = castToQuantity(value); // Quantity 184 } else 185 return super.setProperty(name, value); 186 return value; 187 } 188 189 @Override 190 public void removeChild(String name, Base value) throws FHIRException { 191 if (name.equals("lowLimit")) { 192 this.lowLimit = null; 193 } else if (name.equals("highLimit")) { 194 this.highLimit = null; 195 } else 196 super.removeChild(name, value); 197 198 } 199 200 @Override 201 public Base makeProperty(int hash, String name) throws FHIRException { 202 switch (hash) { 203 case -1841058617: 204 return getLowLimit(); 205 case -710757575: 206 return getHighLimit(); 207 default: 208 return super.makeProperty(hash, name); 209 } 210 211 } 212 213 @Override 214 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 215 switch (hash) { 216 case -1841058617: 217 /* lowLimit */ return new String[] { "Quantity" }; 218 case -710757575: 219 /* highLimit */ return new String[] { "Quantity" }; 220 default: 221 return super.getTypesForProperty(hash, name); 222 } 223 224 } 225 226 @Override 227 public Base addChild(String name) throws FHIRException { 228 if (name.equals("lowLimit")) { 229 this.lowLimit = new Quantity(); 230 return this.lowLimit; 231 } else if (name.equals("highLimit")) { 232 this.highLimit = new Quantity(); 233 return this.highLimit; 234 } else 235 return super.addChild(name); 236 } 237 238 public SubstanceAmountReferenceRangeComponent copy() { 239 SubstanceAmountReferenceRangeComponent dst = new SubstanceAmountReferenceRangeComponent(); 240 copyValues(dst); 241 return dst; 242 } 243 244 public void copyValues(SubstanceAmountReferenceRangeComponent dst) { 245 super.copyValues(dst); 246 dst.lowLimit = lowLimit == null ? null : lowLimit.copy(); 247 dst.highLimit = highLimit == null ? null : highLimit.copy(); 248 } 249 250 @Override 251 public boolean equalsDeep(Base other_) { 252 if (!super.equalsDeep(other_)) 253 return false; 254 if (!(other_ instanceof SubstanceAmountReferenceRangeComponent)) 255 return false; 256 SubstanceAmountReferenceRangeComponent o = (SubstanceAmountReferenceRangeComponent) other_; 257 return compareDeep(lowLimit, o.lowLimit, true) && compareDeep(highLimit, o.highLimit, true); 258 } 259 260 @Override 261 public boolean equalsShallow(Base other_) { 262 if (!super.equalsShallow(other_)) 263 return false; 264 if (!(other_ instanceof SubstanceAmountReferenceRangeComponent)) 265 return false; 266 SubstanceAmountReferenceRangeComponent o = (SubstanceAmountReferenceRangeComponent) other_; 267 return true; 268 } 269 270 public boolean isEmpty() { 271 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lowLimit, highLimit); 272 } 273 274 public String fhirType() { 275 return "SubstanceAmount.referenceRange"; 276 277 } 278 279 } 280 281 /** 282 * Used to capture quantitative values for a variety of elements. If only limits 283 * are given, the arithmetic mean would be the average. If only a single 284 * definite value for a given element is given, it would be captured in this 285 * field. 286 */ 287 @Child(name = "amount", type = { Quantity.class, Range.class, 288 StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 289 @Description(shortDefinition = "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field", formalDefinition = "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.") 290 protected Type amount; 291 292 /** 293 * Most elements that require a quantitative value will also have a field called 294 * amount type. Amount type should always be specified because the actual value 295 * of the amount is often dependent on it. EXAMPLE: In capturing the actual 296 * relative amounts of substances or molecular fragments it is essential to 297 * indicate whether the amount refers to a mole ratio or weight ratio. For any 298 * given element an effort should be made to use same the amount type for all 299 * related definitional elements. 300 */ 301 @Child(name = "amountType", type = { 302 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 303 @Description(shortDefinition = "Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements", formalDefinition = "Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements.") 304 protected CodeableConcept amountType; 305 306 /** 307 * A textual comment on a numeric value. 308 */ 309 @Child(name = "amountText", type = { 310 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 311 @Description(shortDefinition = "A textual comment on a numeric value", formalDefinition = "A textual comment on a numeric value.") 312 protected StringType amountText; 313 314 /** 315 * Reference range of possible or expected values. 316 */ 317 @Child(name = "referenceRange", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = true) 318 @Description(shortDefinition = "Reference range of possible or expected values", formalDefinition = "Reference range of possible or expected values.") 319 protected SubstanceAmountReferenceRangeComponent referenceRange; 320 321 private static final long serialVersionUID = -174997548L; 322 323 /** 324 * Constructor 325 */ 326 public SubstanceAmount() { 327 super(); 328 } 329 330 /** 331 * @return {@link #amount} (Used to capture quantitative values for a variety of 332 * elements. If only limits are given, the arithmetic mean would be the 333 * average. If only a single definite value for a given element is 334 * given, it would be captured in this field.) 335 */ 336 public Type getAmount() { 337 return this.amount; 338 } 339 340 /** 341 * @return {@link #amount} (Used to capture quantitative values for a variety of 342 * elements. If only limits are given, the arithmetic mean would be the 343 * average. If only a single definite value for a given element is 344 * given, it would be captured in this field.) 345 */ 346 public Quantity getAmountQuantity() throws FHIRException { 347 if (this.amount == null) 348 this.amount = new Quantity(); 349 if (!(this.amount instanceof Quantity)) 350 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.amount.getClass().getName() 351 + " was encountered"); 352 return (Quantity) this.amount; 353 } 354 355 public boolean hasAmountQuantity() { 356 return this.amount instanceof Quantity; 357 } 358 359 /** 360 * @return {@link #amount} (Used to capture quantitative values for a variety of 361 * elements. If only limits are given, the arithmetic mean would be the 362 * average. If only a single definite value for a given element is 363 * given, it would be captured in this field.) 364 */ 365 public Range getAmountRange() throws FHIRException { 366 if (this.amount == null) 367 this.amount = new Range(); 368 if (!(this.amount instanceof Range)) 369 throw new FHIRException( 370 "Type mismatch: the type Range was expected, but " + this.amount.getClass().getName() + " was encountered"); 371 return (Range) this.amount; 372 } 373 374 public boolean hasAmountRange() { 375 return this.amount instanceof Range; 376 } 377 378 /** 379 * @return {@link #amount} (Used to capture quantitative values for a variety of 380 * elements. If only limits are given, the arithmetic mean would be the 381 * average. If only a single definite value for a given element is 382 * given, it would be captured in this field.) 383 */ 384 public StringType getAmountStringType() throws FHIRException { 385 if (this.amount == null) 386 this.amount = new StringType(); 387 if (!(this.amount instanceof StringType)) 388 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.amount.getClass().getName() 389 + " was encountered"); 390 return (StringType) this.amount; 391 } 392 393 public boolean hasAmountStringType() { 394 return this.amount instanceof StringType; 395 } 396 397 public boolean hasAmount() { 398 return this.amount != null && !this.amount.isEmpty(); 399 } 400 401 /** 402 * @param value {@link #amount} (Used to capture quantitative values for a 403 * variety of elements. If only limits are given, the arithmetic 404 * mean would be the average. If only a single definite value for a 405 * given element is given, it would be captured in this field.) 406 */ 407 public SubstanceAmount setAmount(Type value) { 408 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof StringType)) 409 throw new Error("Not the right type for SubstanceAmount.amount[x]: " + value.fhirType()); 410 this.amount = value; 411 return this; 412 } 413 414 /** 415 * @return {@link #amountType} (Most elements that require a quantitative value 416 * will also have a field called amount type. Amount type should always 417 * be specified because the actual value of the amount is often 418 * dependent on it. EXAMPLE: In capturing the actual relative amounts of 419 * substances or molecular fragments it is essential to indicate whether 420 * the amount refers to a mole ratio or weight ratio. For any given 421 * element an effort should be made to use same the amount type for all 422 * related definitional elements.) 423 */ 424 public CodeableConcept getAmountType() { 425 if (this.amountType == null) 426 if (Configuration.errorOnAutoCreate()) 427 throw new Error("Attempt to auto-create SubstanceAmount.amountType"); 428 else if (Configuration.doAutoCreate()) 429 this.amountType = new CodeableConcept(); // cc 430 return this.amountType; 431 } 432 433 public boolean hasAmountType() { 434 return this.amountType != null && !this.amountType.isEmpty(); 435 } 436 437 /** 438 * @param value {@link #amountType} (Most elements that require a quantitative 439 * value will also have a field called amount type. Amount type 440 * should always be specified because the actual value of the 441 * amount is often dependent on it. EXAMPLE: In capturing the 442 * actual relative amounts of substances or molecular fragments it 443 * is essential to indicate whether the amount refers to a mole 444 * ratio or weight ratio. For any given element an effort should be 445 * made to use same the amount type for all related definitional 446 * elements.) 447 */ 448 public SubstanceAmount setAmountType(CodeableConcept value) { 449 this.amountType = value; 450 return this; 451 } 452 453 /** 454 * @return {@link #amountText} (A textual comment on a numeric value.). This is 455 * the underlying object with id, value and extensions. The accessor 456 * "getAmountText" gives direct access to the value 457 */ 458 public StringType getAmountTextElement() { 459 if (this.amountText == null) 460 if (Configuration.errorOnAutoCreate()) 461 throw new Error("Attempt to auto-create SubstanceAmount.amountText"); 462 else if (Configuration.doAutoCreate()) 463 this.amountText = new StringType(); // bb 464 return this.amountText; 465 } 466 467 public boolean hasAmountTextElement() { 468 return this.amountText != null && !this.amountText.isEmpty(); 469 } 470 471 public boolean hasAmountText() { 472 return this.amountText != null && !this.amountText.isEmpty(); 473 } 474 475 /** 476 * @param value {@link #amountText} (A textual comment on a numeric value.). 477 * This is the underlying object with id, value and extensions. The 478 * accessor "getAmountText" gives direct access to the value 479 */ 480 public SubstanceAmount setAmountTextElement(StringType value) { 481 this.amountText = value; 482 return this; 483 } 484 485 /** 486 * @return A textual comment on a numeric value. 487 */ 488 public String getAmountText() { 489 return this.amountText == null ? null : this.amountText.getValue(); 490 } 491 492 /** 493 * @param value A textual comment on a numeric value. 494 */ 495 public SubstanceAmount setAmountText(String value) { 496 if (Utilities.noString(value)) 497 this.amountText = null; 498 else { 499 if (this.amountText == null) 500 this.amountText = new StringType(); 501 this.amountText.setValue(value); 502 } 503 return this; 504 } 505 506 /** 507 * @return {@link #referenceRange} (Reference range of possible or expected 508 * values.) 509 */ 510 public SubstanceAmountReferenceRangeComponent getReferenceRange() { 511 if (this.referenceRange == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create SubstanceAmount.referenceRange"); 514 else if (Configuration.doAutoCreate()) 515 this.referenceRange = new SubstanceAmountReferenceRangeComponent(); // cc 516 return this.referenceRange; 517 } 518 519 public boolean hasReferenceRange() { 520 return this.referenceRange != null && !this.referenceRange.isEmpty(); 521 } 522 523 /** 524 * @param value {@link #referenceRange} (Reference range of possible or expected 525 * values.) 526 */ 527 public SubstanceAmount setReferenceRange(SubstanceAmountReferenceRangeComponent value) { 528 this.referenceRange = value; 529 return this; 530 } 531 532 protected void listChildren(List<Property> children) { 533 super.listChildren(children); 534 children.add(new Property("amount[x]", "Quantity|Range|string", 535 "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 536 0, 1, amount)); 537 children.add(new Property("amountType", "CodeableConcept", 538 "Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements.", 539 0, 1, amountType)); 540 children.add(new Property("amountText", "string", "A textual comment on a numeric value.", 0, 1, amountText)); 541 children.add( 542 new Property("referenceRange", "", "Reference range of possible or expected values.", 0, 1, referenceRange)); 543 } 544 545 @Override 546 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 547 switch (_hash) { 548 case 646780200: 549 /* amount[x] */ return new Property("amount[x]", "Quantity|Range|string", 550 "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 551 0, 1, amount); 552 case -1413853096: 553 /* amount */ return new Property("amount[x]", "Quantity|Range|string", 554 "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 555 0, 1, amount); 556 case 1664303363: 557 /* amountQuantity */ return new Property("amount[x]", "Quantity|Range|string", 558 "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 559 0, 1, amount); 560 case -1223462971: 561 /* amountRange */ return new Property("amount[x]", "Quantity|Range|string", 562 "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 563 0, 1, amount); 564 case 773651081: 565 /* amountString */ return new Property("amount[x]", "Quantity|Range|string", 566 "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 567 0, 1, amount); 568 case -1424857166: 569 /* amountType */ return new Property("amountType", "CodeableConcept", 570 "Most elements that require a quantitative value will also have a field called amount type. Amount type should always be specified because the actual value of the amount is often dependent on it. EXAMPLE: In capturing the actual relative amounts of substances or molecular fragments it is essential to indicate whether the amount refers to a mole ratio or weight ratio. For any given element an effort should be made to use same the amount type for all related definitional elements.", 571 0, 1, amountType); 572 case -1424876123: 573 /* amountText */ return new Property("amountText", "string", "A textual comment on a numeric value.", 0, 1, 574 amountText); 575 case -1912545102: 576 /* referenceRange */ return new Property("referenceRange", "", "Reference range of possible or expected values.", 577 0, 1, referenceRange); 578 default: 579 return super.getNamedProperty(_hash, _name, _checkValid); 580 } 581 582 } 583 584 @Override 585 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 586 switch (hash) { 587 case -1413853096: 588 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Type 589 case -1424857166: 590 /* amountType */ return this.amountType == null ? new Base[0] : new Base[] { this.amountType }; // CodeableConcept 591 case -1424876123: 592 /* amountText */ return this.amountText == null ? new Base[0] : new Base[] { this.amountText }; // StringType 593 case -1912545102: 594 /* referenceRange */ return this.referenceRange == null ? new Base[0] : new Base[] { this.referenceRange }; // SubstanceAmountReferenceRangeComponent 595 default: 596 return super.getProperty(hash, name, checkValid); 597 } 598 599 } 600 601 @Override 602 public Base setProperty(int hash, String name, Base value) throws FHIRException { 603 switch (hash) { 604 case -1413853096: // amount 605 this.amount = castToType(value); // Type 606 return value; 607 case -1424857166: // amountType 608 this.amountType = castToCodeableConcept(value); // CodeableConcept 609 return value; 610 case -1424876123: // amountText 611 this.amountText = castToString(value); // StringType 612 return value; 613 case -1912545102: // referenceRange 614 this.referenceRange = (SubstanceAmountReferenceRangeComponent) value; // SubstanceAmountReferenceRangeComponent 615 return value; 616 default: 617 return super.setProperty(hash, name, value); 618 } 619 620 } 621 622 @Override 623 public Base setProperty(String name, Base value) throws FHIRException { 624 if (name.equals("amount[x]")) { 625 this.amount = castToType(value); // Type 626 } else if (name.equals("amountType")) { 627 this.amountType = castToCodeableConcept(value); // CodeableConcept 628 } else if (name.equals("amountText")) { 629 this.amountText = castToString(value); // StringType 630 } else if (name.equals("referenceRange")) { 631 this.referenceRange = (SubstanceAmountReferenceRangeComponent) value; // SubstanceAmountReferenceRangeComponent 632 } else 633 return super.setProperty(name, value); 634 return value; 635 } 636 637 @Override 638 public void removeChild(String name, Base value) throws FHIRException { 639 if (name.equals("amount[x]")) { 640 this.amount = null; 641 } else if (name.equals("amountType")) { 642 this.amountType = null; 643 } else if (name.equals("amountText")) { 644 this.amountText = null; 645 } else if (name.equals("referenceRange")) { 646 this.referenceRange = (SubstanceAmountReferenceRangeComponent) value; // SubstanceAmountReferenceRangeComponent 647 } else 648 super.removeChild(name, value); 649 650 } 651 652 @Override 653 public Base makeProperty(int hash, String name) throws FHIRException { 654 switch (hash) { 655 case 646780200: 656 return getAmount(); 657 case -1413853096: 658 return getAmount(); 659 case -1424857166: 660 return getAmountType(); 661 case -1424876123: 662 return getAmountTextElement(); 663 case -1912545102: 664 return getReferenceRange(); 665 default: 666 return super.makeProperty(hash, name); 667 } 668 669 } 670 671 @Override 672 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 673 switch (hash) { 674 case -1413853096: 675 /* amount */ return new String[] { "Quantity", "Range", "string" }; 676 case -1424857166: 677 /* amountType */ return new String[] { "CodeableConcept" }; 678 case -1424876123: 679 /* amountText */ return new String[] { "string" }; 680 case -1912545102: 681 /* referenceRange */ return new String[] {}; 682 default: 683 return super.getTypesForProperty(hash, name); 684 } 685 686 } 687 688 @Override 689 public Base addChild(String name) throws FHIRException { 690 if (name.equals("amountQuantity")) { 691 this.amount = new Quantity(); 692 return this.amount; 693 } else if (name.equals("amountRange")) { 694 this.amount = new Range(); 695 return this.amount; 696 } else if (name.equals("amountString")) { 697 this.amount = new StringType(); 698 return this.amount; 699 } else if (name.equals("amountType")) { 700 this.amountType = new CodeableConcept(); 701 return this.amountType; 702 } else if (name.equals("amountText")) { 703 throw new FHIRException("Cannot call addChild on a singleton property SubstanceAmount.amountText"); 704 } else if (name.equals("referenceRange")) { 705 this.referenceRange = new SubstanceAmountReferenceRangeComponent(); 706 return this.referenceRange; 707 } else 708 return super.addChild(name); 709 } 710 711 public String fhirType() { 712 return "SubstanceAmount"; 713 714 } 715 716 public SubstanceAmount copy() { 717 SubstanceAmount dst = new SubstanceAmount(); 718 copyValues(dst); 719 return dst; 720 } 721 722 public void copyValues(SubstanceAmount dst) { 723 super.copyValues(dst); 724 dst.amount = amount == null ? null : amount.copy(); 725 dst.amountType = amountType == null ? null : amountType.copy(); 726 dst.amountText = amountText == null ? null : amountText.copy(); 727 dst.referenceRange = referenceRange == null ? null : referenceRange.copy(); 728 } 729 730 protected SubstanceAmount typedCopy() { 731 return copy(); 732 } 733 734 @Override 735 public boolean equalsDeep(Base other_) { 736 if (!super.equalsDeep(other_)) 737 return false; 738 if (!(other_ instanceof SubstanceAmount)) 739 return false; 740 SubstanceAmount o = (SubstanceAmount) other_; 741 return compareDeep(amount, o.amount, true) && compareDeep(amountType, o.amountType, true) 742 && compareDeep(amountText, o.amountText, true) && compareDeep(referenceRange, o.referenceRange, true); 743 } 744 745 @Override 746 public boolean equalsShallow(Base other_) { 747 if (!super.equalsShallow(other_)) 748 return false; 749 if (!(other_ instanceof SubstanceAmount)) 750 return false; 751 SubstanceAmount o = (SubstanceAmount) other_; 752 return compareValues(amountText, o.amountText, true); 753 } 754 755 public boolean isEmpty() { 756 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(amount, amountType, amountText, referenceRange); 757 } 758 759}