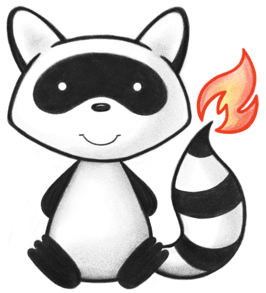
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044 045/** 046 * Nucleic acids are defined by three distinct elements: the base, sugar and 047 * linkage. Individual substance/moiety IDs will be created for each of these 048 * elements. The nucleotide sequence will be always entered in the 5?-3? 049 * direction. 050 */ 051@ResourceDef(name = "SubstanceNucleicAcid", profile = "http://hl7.org/fhir/StructureDefinition/SubstanceNucleicAcid") 052public class SubstanceNucleicAcid extends DomainResource { 053 054 @Block() 055 public static class SubstanceNucleicAcidSubunitComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * Index of linear sequences of nucleic acids in order of decreasing length. 058 * Sequences of the same length will be ordered by molecular weight. Subunits 059 * that have identical sequences will be repeated and have sequential 060 * subscripts. 061 */ 062 @Child(name = "subunit", type = { 063 IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 064 @Description(shortDefinition = "Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts", formalDefinition = "Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.") 065 protected IntegerType subunit; 066 067 /** 068 * Actual nucleotide sequence notation from 5' to 3' end using standard single 069 * letter codes. In addition to the base sequence, sugar and type of phosphate 070 * or non-phosphate linkage should also be captured. 071 */ 072 @Child(name = "sequence", type = { 073 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 074 @Description(shortDefinition = "Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured", formalDefinition = "Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured.") 075 protected StringType sequence; 076 077 /** 078 * The length of the sequence shall be captured. 079 */ 080 @Child(name = "length", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 081 @Description(shortDefinition = "The length of the sequence shall be captured", formalDefinition = "The length of the sequence shall be captured.") 082 protected IntegerType length; 083 084 /** 085 * (TBC). 086 */ 087 @Child(name = "sequenceAttachment", type = { 088 Attachment.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 089 @Description(shortDefinition = "(TBC)", formalDefinition = "(TBC).") 090 protected Attachment sequenceAttachment; 091 092 /** 093 * The nucleotide present at the 5? terminal shall be specified based on a 094 * controlled vocabulary. Since the sequence is represented from the 5' to the 095 * 3' end, the 5? prime nucleotide is the letter at the first position in the 096 * sequence. A separate representation would be redundant. 097 */ 098 @Child(name = "fivePrime", type = { 099 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 100 @Description(shortDefinition = "The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant", formalDefinition = "The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant.") 101 protected CodeableConcept fivePrime; 102 103 /** 104 * The nucleotide present at the 3? terminal shall be specified based on a 105 * controlled vocabulary. Since the sequence is represented from the 5' to the 106 * 3' end, the 5? prime nucleotide is the letter at the last position in the 107 * sequence. A separate representation would be redundant. 108 */ 109 @Child(name = "threePrime", type = { 110 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 111 @Description(shortDefinition = "The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant", formalDefinition = "The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant.") 112 protected CodeableConcept threePrime; 113 114 /** 115 * The linkages between sugar residues will also be captured. 116 */ 117 @Child(name = "linkage", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 118 @Description(shortDefinition = "The linkages between sugar residues will also be captured", formalDefinition = "The linkages between sugar residues will also be captured.") 119 protected List<SubstanceNucleicAcidSubunitLinkageComponent> linkage; 120 121 /** 122 * 5.3.6.8.1 Sugar ID (Mandatory). 123 */ 124 @Child(name = "sugar", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 125 @Description(shortDefinition = "5.3.6.8.1 Sugar ID (Mandatory)", formalDefinition = "5.3.6.8.1 Sugar ID (Mandatory).") 126 protected List<SubstanceNucleicAcidSubunitSugarComponent> sugar; 127 128 private static final long serialVersionUID = 1835593659L; 129 130 /** 131 * Constructor 132 */ 133 public SubstanceNucleicAcidSubunitComponent() { 134 super(); 135 } 136 137 /** 138 * @return {@link #subunit} (Index of linear sequences of nucleic acids in order 139 * of decreasing length. Sequences of the same length will be ordered by 140 * molecular weight. Subunits that have identical sequences will be 141 * repeated and have sequential subscripts.). This is the underlying 142 * object with id, value and extensions. The accessor "getSubunit" gives 143 * direct access to the value 144 */ 145 public IntegerType getSubunitElement() { 146 if (this.subunit == null) 147 if (Configuration.errorOnAutoCreate()) 148 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.subunit"); 149 else if (Configuration.doAutoCreate()) 150 this.subunit = new IntegerType(); // bb 151 return this.subunit; 152 } 153 154 public boolean hasSubunitElement() { 155 return this.subunit != null && !this.subunit.isEmpty(); 156 } 157 158 public boolean hasSubunit() { 159 return this.subunit != null && !this.subunit.isEmpty(); 160 } 161 162 /** 163 * @param value {@link #subunit} (Index of linear sequences of nucleic acids in 164 * order of decreasing length. Sequences of the same length will be 165 * ordered by molecular weight. Subunits that have identical 166 * sequences will be repeated and have sequential subscripts.). 167 * This is the underlying object with id, value and extensions. The 168 * accessor "getSubunit" gives direct access to the value 169 */ 170 public SubstanceNucleicAcidSubunitComponent setSubunitElement(IntegerType value) { 171 this.subunit = value; 172 return this; 173 } 174 175 /** 176 * @return Index of linear sequences of nucleic acids in order of decreasing 177 * length. Sequences of the same length will be ordered by molecular 178 * weight. Subunits that have identical sequences will be repeated and 179 * have sequential subscripts. 180 */ 181 public int getSubunit() { 182 return this.subunit == null || this.subunit.isEmpty() ? 0 : this.subunit.getValue(); 183 } 184 185 /** 186 * @param value Index of linear sequences of nucleic acids in order of 187 * decreasing length. Sequences of the same length will be ordered 188 * by molecular weight. Subunits that have identical sequences will 189 * be repeated and have sequential subscripts. 190 */ 191 public SubstanceNucleicAcidSubunitComponent setSubunit(int value) { 192 if (this.subunit == null) 193 this.subunit = new IntegerType(); 194 this.subunit.setValue(value); 195 return this; 196 } 197 198 /** 199 * @return {@link #sequence} (Actual nucleotide sequence notation from 5' to 3' 200 * end using standard single letter codes. In addition to the base 201 * sequence, sugar and type of phosphate or non-phosphate linkage should 202 * also be captured.). This is the underlying object with id, value and 203 * extensions. The accessor "getSequence" gives direct access to the 204 * value 205 */ 206 public StringType getSequenceElement() { 207 if (this.sequence == null) 208 if (Configuration.errorOnAutoCreate()) 209 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.sequence"); 210 else if (Configuration.doAutoCreate()) 211 this.sequence = new StringType(); // bb 212 return this.sequence; 213 } 214 215 public boolean hasSequenceElement() { 216 return this.sequence != null && !this.sequence.isEmpty(); 217 } 218 219 public boolean hasSequence() { 220 return this.sequence != null && !this.sequence.isEmpty(); 221 } 222 223 /** 224 * @param value {@link #sequence} (Actual nucleotide sequence notation from 5' 225 * to 3' end using standard single letter codes. In addition to the 226 * base sequence, sugar and type of phosphate or non-phosphate 227 * linkage should also be captured.). This is the underlying object 228 * with id, value and extensions. The accessor "getSequence" gives 229 * direct access to the value 230 */ 231 public SubstanceNucleicAcidSubunitComponent setSequenceElement(StringType value) { 232 this.sequence = value; 233 return this; 234 } 235 236 /** 237 * @return Actual nucleotide sequence notation from 5' to 3' end using standard 238 * single letter codes. In addition to the base sequence, sugar and type 239 * of phosphate or non-phosphate linkage should also be captured. 240 */ 241 public String getSequence() { 242 return this.sequence == null ? null : this.sequence.getValue(); 243 } 244 245 /** 246 * @param value Actual nucleotide sequence notation from 5' to 3' end using 247 * standard single letter codes. In addition to the base sequence, 248 * sugar and type of phosphate or non-phosphate linkage should also 249 * be captured. 250 */ 251 public SubstanceNucleicAcidSubunitComponent setSequence(String value) { 252 if (Utilities.noString(value)) 253 this.sequence = null; 254 else { 255 if (this.sequence == null) 256 this.sequence = new StringType(); 257 this.sequence.setValue(value); 258 } 259 return this; 260 } 261 262 /** 263 * @return {@link #length} (The length of the sequence shall be captured.). This 264 * is the underlying object with id, value and extensions. The accessor 265 * "getLength" gives direct access to the value 266 */ 267 public IntegerType getLengthElement() { 268 if (this.length == null) 269 if (Configuration.errorOnAutoCreate()) 270 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.length"); 271 else if (Configuration.doAutoCreate()) 272 this.length = new IntegerType(); // bb 273 return this.length; 274 } 275 276 public boolean hasLengthElement() { 277 return this.length != null && !this.length.isEmpty(); 278 } 279 280 public boolean hasLength() { 281 return this.length != null && !this.length.isEmpty(); 282 } 283 284 /** 285 * @param value {@link #length} (The length of the sequence shall be captured.). 286 * This is the underlying object with id, value and extensions. The 287 * accessor "getLength" gives direct access to the value 288 */ 289 public SubstanceNucleicAcidSubunitComponent setLengthElement(IntegerType value) { 290 this.length = value; 291 return this; 292 } 293 294 /** 295 * @return The length of the sequence shall be captured. 296 */ 297 public int getLength() { 298 return this.length == null || this.length.isEmpty() ? 0 : this.length.getValue(); 299 } 300 301 /** 302 * @param value The length of the sequence shall be captured. 303 */ 304 public SubstanceNucleicAcidSubunitComponent setLength(int value) { 305 if (this.length == null) 306 this.length = new IntegerType(); 307 this.length.setValue(value); 308 return this; 309 } 310 311 /** 312 * @return {@link #sequenceAttachment} ((TBC).) 313 */ 314 public Attachment getSequenceAttachment() { 315 if (this.sequenceAttachment == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.sequenceAttachment"); 318 else if (Configuration.doAutoCreate()) 319 this.sequenceAttachment = new Attachment(); // cc 320 return this.sequenceAttachment; 321 } 322 323 public boolean hasSequenceAttachment() { 324 return this.sequenceAttachment != null && !this.sequenceAttachment.isEmpty(); 325 } 326 327 /** 328 * @param value {@link #sequenceAttachment} ((TBC).) 329 */ 330 public SubstanceNucleicAcidSubunitComponent setSequenceAttachment(Attachment value) { 331 this.sequenceAttachment = value; 332 return this; 333 } 334 335 /** 336 * @return {@link #fivePrime} (The nucleotide present at the 5? terminal shall 337 * be specified based on a controlled vocabulary. Since the sequence is 338 * represented from the 5' to the 3' end, the 5? prime nucleotide is the 339 * letter at the first position in the sequence. A separate 340 * representation would be redundant.) 341 */ 342 public CodeableConcept getFivePrime() { 343 if (this.fivePrime == null) 344 if (Configuration.errorOnAutoCreate()) 345 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.fivePrime"); 346 else if (Configuration.doAutoCreate()) 347 this.fivePrime = new CodeableConcept(); // cc 348 return this.fivePrime; 349 } 350 351 public boolean hasFivePrime() { 352 return this.fivePrime != null && !this.fivePrime.isEmpty(); 353 } 354 355 /** 356 * @param value {@link #fivePrime} (The nucleotide present at the 5? terminal 357 * shall be specified based on a controlled vocabulary. Since the 358 * sequence is represented from the 5' to the 3' end, the 5? prime 359 * nucleotide is the letter at the first position in the sequence. 360 * A separate representation would be redundant.) 361 */ 362 public SubstanceNucleicAcidSubunitComponent setFivePrime(CodeableConcept value) { 363 this.fivePrime = value; 364 return this; 365 } 366 367 /** 368 * @return {@link #threePrime} (The nucleotide present at the 3? terminal shall 369 * be specified based on a controlled vocabulary. Since the sequence is 370 * represented from the 5' to the 3' end, the 5? prime nucleotide is the 371 * letter at the last position in the sequence. A separate 372 * representation would be redundant.) 373 */ 374 public CodeableConcept getThreePrime() { 375 if (this.threePrime == null) 376 if (Configuration.errorOnAutoCreate()) 377 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitComponent.threePrime"); 378 else if (Configuration.doAutoCreate()) 379 this.threePrime = new CodeableConcept(); // cc 380 return this.threePrime; 381 } 382 383 public boolean hasThreePrime() { 384 return this.threePrime != null && !this.threePrime.isEmpty(); 385 } 386 387 /** 388 * @param value {@link #threePrime} (The nucleotide present at the 3? terminal 389 * shall be specified based on a controlled vocabulary. Since the 390 * sequence is represented from the 5' to the 3' end, the 5? prime 391 * nucleotide is the letter at the last position in the sequence. A 392 * separate representation would be redundant.) 393 */ 394 public SubstanceNucleicAcidSubunitComponent setThreePrime(CodeableConcept value) { 395 this.threePrime = value; 396 return this; 397 } 398 399 /** 400 * @return {@link #linkage} (The linkages between sugar residues will also be 401 * captured.) 402 */ 403 public List<SubstanceNucleicAcidSubunitLinkageComponent> getLinkage() { 404 if (this.linkage == null) 405 this.linkage = new ArrayList<SubstanceNucleicAcidSubunitLinkageComponent>(); 406 return this.linkage; 407 } 408 409 /** 410 * @return Returns a reference to <code>this</code> for easy method chaining 411 */ 412 public SubstanceNucleicAcidSubunitComponent setLinkage( 413 List<SubstanceNucleicAcidSubunitLinkageComponent> theLinkage) { 414 this.linkage = theLinkage; 415 return this; 416 } 417 418 public boolean hasLinkage() { 419 if (this.linkage == null) 420 return false; 421 for (SubstanceNucleicAcidSubunitLinkageComponent item : this.linkage) 422 if (!item.isEmpty()) 423 return true; 424 return false; 425 } 426 427 public SubstanceNucleicAcidSubunitLinkageComponent addLinkage() { // 3 428 SubstanceNucleicAcidSubunitLinkageComponent t = new SubstanceNucleicAcidSubunitLinkageComponent(); 429 if (this.linkage == null) 430 this.linkage = new ArrayList<SubstanceNucleicAcidSubunitLinkageComponent>(); 431 this.linkage.add(t); 432 return t; 433 } 434 435 public SubstanceNucleicAcidSubunitComponent addLinkage(SubstanceNucleicAcidSubunitLinkageComponent t) { // 3 436 if (t == null) 437 return this; 438 if (this.linkage == null) 439 this.linkage = new ArrayList<SubstanceNucleicAcidSubunitLinkageComponent>(); 440 this.linkage.add(t); 441 return this; 442 } 443 444 /** 445 * @return The first repetition of repeating field {@link #linkage}, creating it 446 * if it does not already exist 447 */ 448 public SubstanceNucleicAcidSubunitLinkageComponent getLinkageFirstRep() { 449 if (getLinkage().isEmpty()) { 450 addLinkage(); 451 } 452 return getLinkage().get(0); 453 } 454 455 /** 456 * @return {@link #sugar} (5.3.6.8.1 Sugar ID (Mandatory).) 457 */ 458 public List<SubstanceNucleicAcidSubunitSugarComponent> getSugar() { 459 if (this.sugar == null) 460 this.sugar = new ArrayList<SubstanceNucleicAcidSubunitSugarComponent>(); 461 return this.sugar; 462 } 463 464 /** 465 * @return Returns a reference to <code>this</code> for easy method chaining 466 */ 467 public SubstanceNucleicAcidSubunitComponent setSugar(List<SubstanceNucleicAcidSubunitSugarComponent> theSugar) { 468 this.sugar = theSugar; 469 return this; 470 } 471 472 public boolean hasSugar() { 473 if (this.sugar == null) 474 return false; 475 for (SubstanceNucleicAcidSubunitSugarComponent item : this.sugar) 476 if (!item.isEmpty()) 477 return true; 478 return false; 479 } 480 481 public SubstanceNucleicAcidSubunitSugarComponent addSugar() { // 3 482 SubstanceNucleicAcidSubunitSugarComponent t = new SubstanceNucleicAcidSubunitSugarComponent(); 483 if (this.sugar == null) 484 this.sugar = new ArrayList<SubstanceNucleicAcidSubunitSugarComponent>(); 485 this.sugar.add(t); 486 return t; 487 } 488 489 public SubstanceNucleicAcidSubunitComponent addSugar(SubstanceNucleicAcidSubunitSugarComponent t) { // 3 490 if (t == null) 491 return this; 492 if (this.sugar == null) 493 this.sugar = new ArrayList<SubstanceNucleicAcidSubunitSugarComponent>(); 494 this.sugar.add(t); 495 return this; 496 } 497 498 /** 499 * @return The first repetition of repeating field {@link #sugar}, creating it 500 * if it does not already exist 501 */ 502 public SubstanceNucleicAcidSubunitSugarComponent getSugarFirstRep() { 503 if (getSugar().isEmpty()) { 504 addSugar(); 505 } 506 return getSugar().get(0); 507 } 508 509 protected void listChildren(List<Property> children) { 510 super.listChildren(children); 511 children.add(new Property("subunit", "integer", 512 "Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.", 513 0, 1, subunit)); 514 children.add(new Property("sequence", "string", 515 "Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured.", 516 0, 1, sequence)); 517 children.add(new Property("length", "integer", "The length of the sequence shall be captured.", 0, 1, length)); 518 children.add(new Property("sequenceAttachment", "Attachment", "(TBC).", 0, 1, sequenceAttachment)); 519 children.add(new Property("fivePrime", "CodeableConcept", 520 "The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant.", 521 0, 1, fivePrime)); 522 children.add(new Property("threePrime", "CodeableConcept", 523 "The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant.", 524 0, 1, threePrime)); 525 children.add(new Property("linkage", "", "The linkages between sugar residues will also be captured.", 0, 526 java.lang.Integer.MAX_VALUE, linkage)); 527 children.add(new Property("sugar", "", "5.3.6.8.1 Sugar ID (Mandatory).", 0, java.lang.Integer.MAX_VALUE, sugar)); 528 } 529 530 @Override 531 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 532 switch (_hash) { 533 case -1867548732: 534 /* subunit */ return new Property("subunit", "integer", 535 "Index of linear sequences of nucleic acids in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.", 536 0, 1, subunit); 537 case 1349547969: 538 /* sequence */ return new Property("sequence", "string", 539 "Actual nucleotide sequence notation from 5' to 3' end using standard single letter codes. In addition to the base sequence, sugar and type of phosphate or non-phosphate linkage should also be captured.", 540 0, 1, sequence); 541 case -1106363674: 542 /* length */ return new Property("length", "integer", "The length of the sequence shall be captured.", 0, 1, 543 length); 544 case 364621764: 545 /* sequenceAttachment */ return new Property("sequenceAttachment", "Attachment", "(TBC).", 0, 1, 546 sequenceAttachment); 547 case -1045091603: 548 /* fivePrime */ return new Property("fivePrime", "CodeableConcept", 549 "The nucleotide present at the 5? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the first position in the sequence. A separate representation would be redundant.", 550 0, 1, fivePrime); 551 case -1088032895: 552 /* threePrime */ return new Property("threePrime", "CodeableConcept", 553 "The nucleotide present at the 3? terminal shall be specified based on a controlled vocabulary. Since the sequence is represented from the 5' to the 3' end, the 5? prime nucleotide is the letter at the last position in the sequence. A separate representation would be redundant.", 554 0, 1, threePrime); 555 case 177082053: 556 /* linkage */ return new Property("linkage", "", "The linkages between sugar residues will also be captured.", 557 0, java.lang.Integer.MAX_VALUE, linkage); 558 case 109792566: 559 /* sugar */ return new Property("sugar", "", "5.3.6.8.1 Sugar ID (Mandatory).", 0, java.lang.Integer.MAX_VALUE, 560 sugar); 561 default: 562 return super.getNamedProperty(_hash, _name, _checkValid); 563 } 564 565 } 566 567 @Override 568 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 569 switch (hash) { 570 case -1867548732: 571 /* subunit */ return this.subunit == null ? new Base[0] : new Base[] { this.subunit }; // IntegerType 572 case 1349547969: 573 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // StringType 574 case -1106363674: 575 /* length */ return this.length == null ? new Base[0] : new Base[] { this.length }; // IntegerType 576 case 364621764: 577 /* sequenceAttachment */ return this.sequenceAttachment == null ? new Base[0] 578 : new Base[] { this.sequenceAttachment }; // Attachment 579 case -1045091603: 580 /* fivePrime */ return this.fivePrime == null ? new Base[0] : new Base[] { this.fivePrime }; // CodeableConcept 581 case -1088032895: 582 /* threePrime */ return this.threePrime == null ? new Base[0] : new Base[] { this.threePrime }; // CodeableConcept 583 case 177082053: 584 /* linkage */ return this.linkage == null ? new Base[0] : this.linkage.toArray(new Base[this.linkage.size()]); // SubstanceNucleicAcidSubunitLinkageComponent 585 case 109792566: 586 /* sugar */ return this.sugar == null ? new Base[0] : this.sugar.toArray(new Base[this.sugar.size()]); // SubstanceNucleicAcidSubunitSugarComponent 587 default: 588 return super.getProperty(hash, name, checkValid); 589 } 590 591 } 592 593 @Override 594 public Base setProperty(int hash, String name, Base value) throws FHIRException { 595 switch (hash) { 596 case -1867548732: // subunit 597 this.subunit = castToInteger(value); // IntegerType 598 return value; 599 case 1349547969: // sequence 600 this.sequence = castToString(value); // StringType 601 return value; 602 case -1106363674: // length 603 this.length = castToInteger(value); // IntegerType 604 return value; 605 case 364621764: // sequenceAttachment 606 this.sequenceAttachment = castToAttachment(value); // Attachment 607 return value; 608 case -1045091603: // fivePrime 609 this.fivePrime = castToCodeableConcept(value); // CodeableConcept 610 return value; 611 case -1088032895: // threePrime 612 this.threePrime = castToCodeableConcept(value); // CodeableConcept 613 return value; 614 case 177082053: // linkage 615 this.getLinkage().add((SubstanceNucleicAcidSubunitLinkageComponent) value); // SubstanceNucleicAcidSubunitLinkageComponent 616 return value; 617 case 109792566: // sugar 618 this.getSugar().add((SubstanceNucleicAcidSubunitSugarComponent) value); // SubstanceNucleicAcidSubunitSugarComponent 619 return value; 620 default: 621 return super.setProperty(hash, name, value); 622 } 623 624 } 625 626 @Override 627 public Base setProperty(String name, Base value) throws FHIRException { 628 if (name.equals("subunit")) { 629 this.subunit = castToInteger(value); // IntegerType 630 } else if (name.equals("sequence")) { 631 this.sequence = castToString(value); // StringType 632 } else if (name.equals("length")) { 633 this.length = castToInteger(value); // IntegerType 634 } else if (name.equals("sequenceAttachment")) { 635 this.sequenceAttachment = castToAttachment(value); // Attachment 636 } else if (name.equals("fivePrime")) { 637 this.fivePrime = castToCodeableConcept(value); // CodeableConcept 638 } else if (name.equals("threePrime")) { 639 this.threePrime = castToCodeableConcept(value); // CodeableConcept 640 } else if (name.equals("linkage")) { 641 this.getLinkage().add((SubstanceNucleicAcidSubunitLinkageComponent) value); 642 } else if (name.equals("sugar")) { 643 this.getSugar().add((SubstanceNucleicAcidSubunitSugarComponent) value); 644 } else 645 return super.setProperty(name, value); 646 return value; 647 } 648 649 @Override 650 public void removeChild(String name, Base value) throws FHIRException { 651 if (name.equals("subunit")) { 652 this.subunit = null; 653 } else if (name.equals("sequence")) { 654 this.sequence = null; 655 } else if (name.equals("length")) { 656 this.length = null; 657 } else if (name.equals("sequenceAttachment")) { 658 this.sequenceAttachment = null; 659 } else if (name.equals("fivePrime")) { 660 this.fivePrime = null; 661 } else if (name.equals("threePrime")) { 662 this.threePrime = null; 663 } else if (name.equals("linkage")) { 664 this.getLinkage().remove((SubstanceNucleicAcidSubunitLinkageComponent) value); 665 } else if (name.equals("sugar")) { 666 this.getSugar().remove((SubstanceNucleicAcidSubunitSugarComponent) value); 667 } else 668 super.removeChild(name, value); 669 670 } 671 672 @Override 673 public Base makeProperty(int hash, String name) throws FHIRException { 674 switch (hash) { 675 case -1867548732: 676 return getSubunitElement(); 677 case 1349547969: 678 return getSequenceElement(); 679 case -1106363674: 680 return getLengthElement(); 681 case 364621764: 682 return getSequenceAttachment(); 683 case -1045091603: 684 return getFivePrime(); 685 case -1088032895: 686 return getThreePrime(); 687 case 177082053: 688 return addLinkage(); 689 case 109792566: 690 return addSugar(); 691 default: 692 return super.makeProperty(hash, name); 693 } 694 695 } 696 697 @Override 698 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 699 switch (hash) { 700 case -1867548732: 701 /* subunit */ return new String[] { "integer" }; 702 case 1349547969: 703 /* sequence */ return new String[] { "string" }; 704 case -1106363674: 705 /* length */ return new String[] { "integer" }; 706 case 364621764: 707 /* sequenceAttachment */ return new String[] { "Attachment" }; 708 case -1045091603: 709 /* fivePrime */ return new String[] { "CodeableConcept" }; 710 case -1088032895: 711 /* threePrime */ return new String[] { "CodeableConcept" }; 712 case 177082053: 713 /* linkage */ return new String[] {}; 714 case 109792566: 715 /* sugar */ return new String[] {}; 716 default: 717 return super.getTypesForProperty(hash, name); 718 } 719 720 } 721 722 @Override 723 public Base addChild(String name) throws FHIRException { 724 if (name.equals("subunit")) { 725 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.subunit"); 726 } else if (name.equals("sequence")) { 727 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.sequence"); 728 } else if (name.equals("length")) { 729 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.length"); 730 } else if (name.equals("sequenceAttachment")) { 731 this.sequenceAttachment = new Attachment(); 732 return this.sequenceAttachment; 733 } else if (name.equals("fivePrime")) { 734 this.fivePrime = new CodeableConcept(); 735 return this.fivePrime; 736 } else if (name.equals("threePrime")) { 737 this.threePrime = new CodeableConcept(); 738 return this.threePrime; 739 } else if (name.equals("linkage")) { 740 return addLinkage(); 741 } else if (name.equals("sugar")) { 742 return addSugar(); 743 } else 744 return super.addChild(name); 745 } 746 747 public SubstanceNucleicAcidSubunitComponent copy() { 748 SubstanceNucleicAcidSubunitComponent dst = new SubstanceNucleicAcidSubunitComponent(); 749 copyValues(dst); 750 return dst; 751 } 752 753 public void copyValues(SubstanceNucleicAcidSubunitComponent dst) { 754 super.copyValues(dst); 755 dst.subunit = subunit == null ? null : subunit.copy(); 756 dst.sequence = sequence == null ? null : sequence.copy(); 757 dst.length = length == null ? null : length.copy(); 758 dst.sequenceAttachment = sequenceAttachment == null ? null : sequenceAttachment.copy(); 759 dst.fivePrime = fivePrime == null ? null : fivePrime.copy(); 760 dst.threePrime = threePrime == null ? null : threePrime.copy(); 761 if (linkage != null) { 762 dst.linkage = new ArrayList<SubstanceNucleicAcidSubunitLinkageComponent>(); 763 for (SubstanceNucleicAcidSubunitLinkageComponent i : linkage) 764 dst.linkage.add(i.copy()); 765 } 766 ; 767 if (sugar != null) { 768 dst.sugar = new ArrayList<SubstanceNucleicAcidSubunitSugarComponent>(); 769 for (SubstanceNucleicAcidSubunitSugarComponent i : sugar) 770 dst.sugar.add(i.copy()); 771 } 772 ; 773 } 774 775 @Override 776 public boolean equalsDeep(Base other_) { 777 if (!super.equalsDeep(other_)) 778 return false; 779 if (!(other_ instanceof SubstanceNucleicAcidSubunitComponent)) 780 return false; 781 SubstanceNucleicAcidSubunitComponent o = (SubstanceNucleicAcidSubunitComponent) other_; 782 return compareDeep(subunit, o.subunit, true) && compareDeep(sequence, o.sequence, true) 783 && compareDeep(length, o.length, true) && compareDeep(sequenceAttachment, o.sequenceAttachment, true) 784 && compareDeep(fivePrime, o.fivePrime, true) && compareDeep(threePrime, o.threePrime, true) 785 && compareDeep(linkage, o.linkage, true) && compareDeep(sugar, o.sugar, true); 786 } 787 788 @Override 789 public boolean equalsShallow(Base other_) { 790 if (!super.equalsShallow(other_)) 791 return false; 792 if (!(other_ instanceof SubstanceNucleicAcidSubunitComponent)) 793 return false; 794 SubstanceNucleicAcidSubunitComponent o = (SubstanceNucleicAcidSubunitComponent) other_; 795 return compareValues(subunit, o.subunit, true) && compareValues(sequence, o.sequence, true) 796 && compareValues(length, o.length, true); 797 } 798 799 public boolean isEmpty() { 800 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subunit, sequence, length, sequenceAttachment, 801 fivePrime, threePrime, linkage, sugar); 802 } 803 804 public String fhirType() { 805 return "SubstanceNucleicAcid.subunit"; 806 807 } 808 809 } 810 811 @Block() 812 public static class SubstanceNucleicAcidSubunitLinkageComponent extends BackboneElement 813 implements IBaseBackboneElement { 814 /** 815 * The entity that links the sugar residues together should also be captured for 816 * nearly all naturally occurring nucleic acid the linkage is a phosphate group. 817 * For many synthetic oligonucleotides phosphorothioate linkages are often seen. 818 * Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? 819 * or 5?-5? this should be specified. 820 */ 821 @Child(name = "connectivity", type = { 822 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 823 @Description(shortDefinition = "The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified", formalDefinition = "The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified.") 824 protected StringType connectivity; 825 826 /** 827 * Each linkage will be registered as a fragment and have an ID. 828 */ 829 @Child(name = "identifier", type = { 830 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 831 @Description(shortDefinition = "Each linkage will be registered as a fragment and have an ID", formalDefinition = "Each linkage will be registered as a fragment and have an ID.") 832 protected Identifier identifier; 833 834 /** 835 * Each linkage will be registered as a fragment and have at least one name. A 836 * single name shall be assigned to each linkage. 837 */ 838 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 839 @Description(shortDefinition = "Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage", formalDefinition = "Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage.") 840 protected StringType name; 841 842 /** 843 * Residues shall be captured as described in 5.3.6.8.3. 844 */ 845 @Child(name = "residueSite", type = { 846 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 847 @Description(shortDefinition = "Residues shall be captured as described in 5.3.6.8.3", formalDefinition = "Residues shall be captured as described in 5.3.6.8.3.") 848 protected StringType residueSite; 849 850 private static final long serialVersionUID = 1392155799L; 851 852 /** 853 * Constructor 854 */ 855 public SubstanceNucleicAcidSubunitLinkageComponent() { 856 super(); 857 } 858 859 /** 860 * @return {@link #connectivity} (The entity that links the sugar residues 861 * together should also be captured for nearly all naturally occurring 862 * nucleic acid the linkage is a phosphate group. For many synthetic 863 * oligonucleotides phosphorothioate linkages are often seen. Linkage 864 * connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? 865 * or 5?-5? this should be specified.). This is the underlying object 866 * with id, value and extensions. The accessor "getConnectivity" gives 867 * direct access to the value 868 */ 869 public StringType getConnectivityElement() { 870 if (this.connectivity == null) 871 if (Configuration.errorOnAutoCreate()) 872 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitLinkageComponent.connectivity"); 873 else if (Configuration.doAutoCreate()) 874 this.connectivity = new StringType(); // bb 875 return this.connectivity; 876 } 877 878 public boolean hasConnectivityElement() { 879 return this.connectivity != null && !this.connectivity.isEmpty(); 880 } 881 882 public boolean hasConnectivity() { 883 return this.connectivity != null && !this.connectivity.isEmpty(); 884 } 885 886 /** 887 * @param value {@link #connectivity} (The entity that links the sugar residues 888 * together should also be captured for nearly all naturally 889 * occurring nucleic acid the linkage is a phosphate group. For 890 * many synthetic oligonucleotides phosphorothioate linkages are 891 * often seen. Linkage connectivity is assumed to be 3?-5?. If the 892 * linkage is either 3?-3? or 5?-5? this should be specified.). 893 * This is the underlying object with id, value and extensions. The 894 * accessor "getConnectivity" gives direct access to the value 895 */ 896 public SubstanceNucleicAcidSubunitLinkageComponent setConnectivityElement(StringType value) { 897 this.connectivity = value; 898 return this; 899 } 900 901 /** 902 * @return The entity that links the sugar residues together should also be 903 * captured for nearly all naturally occurring nucleic acid the linkage 904 * is a phosphate group. For many synthetic oligonucleotides 905 * phosphorothioate linkages are often seen. Linkage connectivity is 906 * assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this 907 * should be specified. 908 */ 909 public String getConnectivity() { 910 return this.connectivity == null ? null : this.connectivity.getValue(); 911 } 912 913 /** 914 * @param value The entity that links the sugar residues together should also be 915 * captured for nearly all naturally occurring nucleic acid the 916 * linkage is a phosphate group. For many synthetic 917 * oligonucleotides phosphorothioate linkages are often seen. 918 * Linkage connectivity is assumed to be 3?-5?. If the linkage is 919 * either 3?-3? or 5?-5? this should be specified. 920 */ 921 public SubstanceNucleicAcidSubunitLinkageComponent setConnectivity(String value) { 922 if (Utilities.noString(value)) 923 this.connectivity = null; 924 else { 925 if (this.connectivity == null) 926 this.connectivity = new StringType(); 927 this.connectivity.setValue(value); 928 } 929 return this; 930 } 931 932 /** 933 * @return {@link #identifier} (Each linkage will be registered as a fragment 934 * and have an ID.) 935 */ 936 public Identifier getIdentifier() { 937 if (this.identifier == null) 938 if (Configuration.errorOnAutoCreate()) 939 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitLinkageComponent.identifier"); 940 else if (Configuration.doAutoCreate()) 941 this.identifier = new Identifier(); // cc 942 return this.identifier; 943 } 944 945 public boolean hasIdentifier() { 946 return this.identifier != null && !this.identifier.isEmpty(); 947 } 948 949 /** 950 * @param value {@link #identifier} (Each linkage will be registered as a 951 * fragment and have an ID.) 952 */ 953 public SubstanceNucleicAcidSubunitLinkageComponent setIdentifier(Identifier value) { 954 this.identifier = value; 955 return this; 956 } 957 958 /** 959 * @return {@link #name} (Each linkage will be registered as a fragment and have 960 * at least one name. A single name shall be assigned to each linkage.). 961 * This is the underlying object with id, value and extensions. The 962 * accessor "getName" gives direct access to the value 963 */ 964 public StringType getNameElement() { 965 if (this.name == null) 966 if (Configuration.errorOnAutoCreate()) 967 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitLinkageComponent.name"); 968 else if (Configuration.doAutoCreate()) 969 this.name = new StringType(); // bb 970 return this.name; 971 } 972 973 public boolean hasNameElement() { 974 return this.name != null && !this.name.isEmpty(); 975 } 976 977 public boolean hasName() { 978 return this.name != null && !this.name.isEmpty(); 979 } 980 981 /** 982 * @param value {@link #name} (Each linkage will be registered as a fragment and 983 * have at least one name. A single name shall be assigned to each 984 * linkage.). This is the underlying object with id, value and 985 * extensions. The accessor "getName" gives direct access to the 986 * value 987 */ 988 public SubstanceNucleicAcidSubunitLinkageComponent setNameElement(StringType value) { 989 this.name = value; 990 return this; 991 } 992 993 /** 994 * @return Each linkage will be registered as a fragment and have at least one 995 * name. A single name shall be assigned to each linkage. 996 */ 997 public String getName() { 998 return this.name == null ? null : this.name.getValue(); 999 } 1000 1001 /** 1002 * @param value Each linkage will be registered as a fragment and have at least 1003 * one name. A single name shall be assigned to each linkage. 1004 */ 1005 public SubstanceNucleicAcidSubunitLinkageComponent setName(String value) { 1006 if (Utilities.noString(value)) 1007 this.name = null; 1008 else { 1009 if (this.name == null) 1010 this.name = new StringType(); 1011 this.name.setValue(value); 1012 } 1013 return this; 1014 } 1015 1016 /** 1017 * @return {@link #residueSite} (Residues shall be captured as described in 1018 * 5.3.6.8.3.). This is the underlying object with id, value and 1019 * extensions. The accessor "getResidueSite" gives direct access to the 1020 * value 1021 */ 1022 public StringType getResidueSiteElement() { 1023 if (this.residueSite == null) 1024 if (Configuration.errorOnAutoCreate()) 1025 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitLinkageComponent.residueSite"); 1026 else if (Configuration.doAutoCreate()) 1027 this.residueSite = new StringType(); // bb 1028 return this.residueSite; 1029 } 1030 1031 public boolean hasResidueSiteElement() { 1032 return this.residueSite != null && !this.residueSite.isEmpty(); 1033 } 1034 1035 public boolean hasResidueSite() { 1036 return this.residueSite != null && !this.residueSite.isEmpty(); 1037 } 1038 1039 /** 1040 * @param value {@link #residueSite} (Residues shall be captured as described in 1041 * 5.3.6.8.3.). This is the underlying object with id, value and 1042 * extensions. The accessor "getResidueSite" gives direct access to 1043 * the value 1044 */ 1045 public SubstanceNucleicAcidSubunitLinkageComponent setResidueSiteElement(StringType value) { 1046 this.residueSite = value; 1047 return this; 1048 } 1049 1050 /** 1051 * @return Residues shall be captured as described in 5.3.6.8.3. 1052 */ 1053 public String getResidueSite() { 1054 return this.residueSite == null ? null : this.residueSite.getValue(); 1055 } 1056 1057 /** 1058 * @param value Residues shall be captured as described in 5.3.6.8.3. 1059 */ 1060 public SubstanceNucleicAcidSubunitLinkageComponent setResidueSite(String value) { 1061 if (Utilities.noString(value)) 1062 this.residueSite = null; 1063 else { 1064 if (this.residueSite == null) 1065 this.residueSite = new StringType(); 1066 this.residueSite.setValue(value); 1067 } 1068 return this; 1069 } 1070 1071 protected void listChildren(List<Property> children) { 1072 super.listChildren(children); 1073 children.add(new Property("connectivity", "string", 1074 "The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified.", 1075 0, 1, connectivity)); 1076 children.add(new Property("identifier", "Identifier", 1077 "Each linkage will be registered as a fragment and have an ID.", 0, 1, identifier)); 1078 children.add(new Property("name", "string", 1079 "Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage.", 1080 0, 1, name)); 1081 children.add(new Property("residueSite", "string", "Residues shall be captured as described in 5.3.6.8.3.", 0, 1, 1082 residueSite)); 1083 } 1084 1085 @Override 1086 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1087 switch (_hash) { 1088 case 1923312055: 1089 /* connectivity */ return new Property("connectivity", "string", 1090 "The entity that links the sugar residues together should also be captured for nearly all naturally occurring nucleic acid the linkage is a phosphate group. For many synthetic oligonucleotides phosphorothioate linkages are often seen. Linkage connectivity is assumed to be 3?-5?. If the linkage is either 3?-3? or 5?-5? this should be specified.", 1091 0, 1, connectivity); 1092 case -1618432855: 1093 /* identifier */ return new Property("identifier", "Identifier", 1094 "Each linkage will be registered as a fragment and have an ID.", 0, 1, identifier); 1095 case 3373707: 1096 /* name */ return new Property("name", "string", 1097 "Each linkage will be registered as a fragment and have at least one name. A single name shall be assigned to each linkage.", 1098 0, 1, name); 1099 case 1547124594: 1100 /* residueSite */ return new Property("residueSite", "string", 1101 "Residues shall be captured as described in 5.3.6.8.3.", 0, 1, residueSite); 1102 default: 1103 return super.getNamedProperty(_hash, _name, _checkValid); 1104 } 1105 1106 } 1107 1108 @Override 1109 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1110 switch (hash) { 1111 case 1923312055: 1112 /* connectivity */ return this.connectivity == null ? new Base[0] : new Base[] { this.connectivity }; // StringType 1113 case -1618432855: 1114 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 1115 case 3373707: 1116 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1117 case 1547124594: 1118 /* residueSite */ return this.residueSite == null ? new Base[0] : new Base[] { this.residueSite }; // StringType 1119 default: 1120 return super.getProperty(hash, name, checkValid); 1121 } 1122 1123 } 1124 1125 @Override 1126 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1127 switch (hash) { 1128 case 1923312055: // connectivity 1129 this.connectivity = castToString(value); // StringType 1130 return value; 1131 case -1618432855: // identifier 1132 this.identifier = castToIdentifier(value); // Identifier 1133 return value; 1134 case 3373707: // name 1135 this.name = castToString(value); // StringType 1136 return value; 1137 case 1547124594: // residueSite 1138 this.residueSite = castToString(value); // StringType 1139 return value; 1140 default: 1141 return super.setProperty(hash, name, value); 1142 } 1143 1144 } 1145 1146 @Override 1147 public Base setProperty(String name, Base value) throws FHIRException { 1148 if (name.equals("connectivity")) { 1149 this.connectivity = castToString(value); // StringType 1150 } else if (name.equals("identifier")) { 1151 this.identifier = castToIdentifier(value); // Identifier 1152 } else if (name.equals("name")) { 1153 this.name = castToString(value); // StringType 1154 } else if (name.equals("residueSite")) { 1155 this.residueSite = castToString(value); // StringType 1156 } else 1157 return super.setProperty(name, value); 1158 return value; 1159 } 1160 1161 @Override 1162 public void removeChild(String name, Base value) throws FHIRException { 1163 if (name.equals("connectivity")) { 1164 this.connectivity = null; 1165 } else if (name.equals("identifier")) { 1166 this.identifier = null; 1167 } else if (name.equals("name")) { 1168 this.name = null; 1169 } else if (name.equals("residueSite")) { 1170 this.residueSite = null; 1171 } else 1172 super.removeChild(name, value); 1173 1174 } 1175 1176 @Override 1177 public Base makeProperty(int hash, String name) throws FHIRException { 1178 switch (hash) { 1179 case 1923312055: 1180 return getConnectivityElement(); 1181 case -1618432855: 1182 return getIdentifier(); 1183 case 3373707: 1184 return getNameElement(); 1185 case 1547124594: 1186 return getResidueSiteElement(); 1187 default: 1188 return super.makeProperty(hash, name); 1189 } 1190 1191 } 1192 1193 @Override 1194 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1195 switch (hash) { 1196 case 1923312055: 1197 /* connectivity */ return new String[] { "string" }; 1198 case -1618432855: 1199 /* identifier */ return new String[] { "Identifier" }; 1200 case 3373707: 1201 /* name */ return new String[] { "string" }; 1202 case 1547124594: 1203 /* residueSite */ return new String[] { "string" }; 1204 default: 1205 return super.getTypesForProperty(hash, name); 1206 } 1207 1208 } 1209 1210 @Override 1211 public Base addChild(String name) throws FHIRException { 1212 if (name.equals("connectivity")) { 1213 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.connectivity"); 1214 } else if (name.equals("identifier")) { 1215 this.identifier = new Identifier(); 1216 return this.identifier; 1217 } else if (name.equals("name")) { 1218 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.name"); 1219 } else if (name.equals("residueSite")) { 1220 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.residueSite"); 1221 } else 1222 return super.addChild(name); 1223 } 1224 1225 public SubstanceNucleicAcidSubunitLinkageComponent copy() { 1226 SubstanceNucleicAcidSubunitLinkageComponent dst = new SubstanceNucleicAcidSubunitLinkageComponent(); 1227 copyValues(dst); 1228 return dst; 1229 } 1230 1231 public void copyValues(SubstanceNucleicAcidSubunitLinkageComponent dst) { 1232 super.copyValues(dst); 1233 dst.connectivity = connectivity == null ? null : connectivity.copy(); 1234 dst.identifier = identifier == null ? null : identifier.copy(); 1235 dst.name = name == null ? null : name.copy(); 1236 dst.residueSite = residueSite == null ? null : residueSite.copy(); 1237 } 1238 1239 @Override 1240 public boolean equalsDeep(Base other_) { 1241 if (!super.equalsDeep(other_)) 1242 return false; 1243 if (!(other_ instanceof SubstanceNucleicAcidSubunitLinkageComponent)) 1244 return false; 1245 SubstanceNucleicAcidSubunitLinkageComponent o = (SubstanceNucleicAcidSubunitLinkageComponent) other_; 1246 return compareDeep(connectivity, o.connectivity, true) && compareDeep(identifier, o.identifier, true) 1247 && compareDeep(name, o.name, true) && compareDeep(residueSite, o.residueSite, true); 1248 } 1249 1250 @Override 1251 public boolean equalsShallow(Base other_) { 1252 if (!super.equalsShallow(other_)) 1253 return false; 1254 if (!(other_ instanceof SubstanceNucleicAcidSubunitLinkageComponent)) 1255 return false; 1256 SubstanceNucleicAcidSubunitLinkageComponent o = (SubstanceNucleicAcidSubunitLinkageComponent) other_; 1257 return compareValues(connectivity, o.connectivity, true) && compareValues(name, o.name, true) 1258 && compareValues(residueSite, o.residueSite, true); 1259 } 1260 1261 public boolean isEmpty() { 1262 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(connectivity, identifier, name, residueSite); 1263 } 1264 1265 public String fhirType() { 1266 return "SubstanceNucleicAcid.subunit.linkage"; 1267 1268 } 1269 1270 } 1271 1272 @Block() 1273 public static class SubstanceNucleicAcidSubunitSugarComponent extends BackboneElement 1274 implements IBaseBackboneElement { 1275 /** 1276 * The Substance ID of the sugar or sugar-like component that make up the 1277 * nucleotide. 1278 */ 1279 @Child(name = "identifier", type = { 1280 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1281 @Description(shortDefinition = "The Substance ID of the sugar or sugar-like component that make up the nucleotide", formalDefinition = "The Substance ID of the sugar or sugar-like component that make up the nucleotide.") 1282 protected Identifier identifier; 1283 1284 /** 1285 * The name of the sugar or sugar-like component that make up the nucleotide. 1286 */ 1287 @Child(name = "name", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1288 @Description(shortDefinition = "The name of the sugar or sugar-like component that make up the nucleotide", formalDefinition = "The name of the sugar or sugar-like component that make up the nucleotide.") 1289 protected StringType name; 1290 1291 /** 1292 * The residues that contain a given sugar will be captured. The order of given 1293 * residues will be captured in the 5?-3?direction consistent with the base 1294 * sequences listed above. 1295 */ 1296 @Child(name = "residueSite", type = { 1297 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1298 @Description(shortDefinition = "The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above", formalDefinition = "The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above.") 1299 protected StringType residueSite; 1300 1301 private static final long serialVersionUID = 1933713781L; 1302 1303 /** 1304 * Constructor 1305 */ 1306 public SubstanceNucleicAcidSubunitSugarComponent() { 1307 super(); 1308 } 1309 1310 /** 1311 * @return {@link #identifier} (The Substance ID of the sugar or sugar-like 1312 * component that make up the nucleotide.) 1313 */ 1314 public Identifier getIdentifier() { 1315 if (this.identifier == null) 1316 if (Configuration.errorOnAutoCreate()) 1317 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitSugarComponent.identifier"); 1318 else if (Configuration.doAutoCreate()) 1319 this.identifier = new Identifier(); // cc 1320 return this.identifier; 1321 } 1322 1323 public boolean hasIdentifier() { 1324 return this.identifier != null && !this.identifier.isEmpty(); 1325 } 1326 1327 /** 1328 * @param value {@link #identifier} (The Substance ID of the sugar or sugar-like 1329 * component that make up the nucleotide.) 1330 */ 1331 public SubstanceNucleicAcidSubunitSugarComponent setIdentifier(Identifier value) { 1332 this.identifier = value; 1333 return this; 1334 } 1335 1336 /** 1337 * @return {@link #name} (The name of the sugar or sugar-like component that 1338 * make up the nucleotide.). This is the underlying object with id, 1339 * value and extensions. The accessor "getName" gives direct access to 1340 * the value 1341 */ 1342 public StringType getNameElement() { 1343 if (this.name == null) 1344 if (Configuration.errorOnAutoCreate()) 1345 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitSugarComponent.name"); 1346 else if (Configuration.doAutoCreate()) 1347 this.name = new StringType(); // bb 1348 return this.name; 1349 } 1350 1351 public boolean hasNameElement() { 1352 return this.name != null && !this.name.isEmpty(); 1353 } 1354 1355 public boolean hasName() { 1356 return this.name != null && !this.name.isEmpty(); 1357 } 1358 1359 /** 1360 * @param value {@link #name} (The name of the sugar or sugar-like component 1361 * that make up the nucleotide.). This is the underlying object 1362 * with id, value and extensions. The accessor "getName" gives 1363 * direct access to the value 1364 */ 1365 public SubstanceNucleicAcidSubunitSugarComponent setNameElement(StringType value) { 1366 this.name = value; 1367 return this; 1368 } 1369 1370 /** 1371 * @return The name of the sugar or sugar-like component that make up the 1372 * nucleotide. 1373 */ 1374 public String getName() { 1375 return this.name == null ? null : this.name.getValue(); 1376 } 1377 1378 /** 1379 * @param value The name of the sugar or sugar-like component that make up the 1380 * nucleotide. 1381 */ 1382 public SubstanceNucleicAcidSubunitSugarComponent setName(String value) { 1383 if (Utilities.noString(value)) 1384 this.name = null; 1385 else { 1386 if (this.name == null) 1387 this.name = new StringType(); 1388 this.name.setValue(value); 1389 } 1390 return this; 1391 } 1392 1393 /** 1394 * @return {@link #residueSite} (The residues that contain a given sugar will be 1395 * captured. The order of given residues will be captured in the 1396 * 5?-3?direction consistent with the base sequences listed above.). 1397 * This is the underlying object with id, value and extensions. The 1398 * accessor "getResidueSite" gives direct access to the value 1399 */ 1400 public StringType getResidueSiteElement() { 1401 if (this.residueSite == null) 1402 if (Configuration.errorOnAutoCreate()) 1403 throw new Error("Attempt to auto-create SubstanceNucleicAcidSubunitSugarComponent.residueSite"); 1404 else if (Configuration.doAutoCreate()) 1405 this.residueSite = new StringType(); // bb 1406 return this.residueSite; 1407 } 1408 1409 public boolean hasResidueSiteElement() { 1410 return this.residueSite != null && !this.residueSite.isEmpty(); 1411 } 1412 1413 public boolean hasResidueSite() { 1414 return this.residueSite != null && !this.residueSite.isEmpty(); 1415 } 1416 1417 /** 1418 * @param value {@link #residueSite} (The residues that contain a given sugar 1419 * will be captured. The order of given residues will be captured 1420 * in the 5?-3?direction consistent with the base sequences listed 1421 * above.). This is the underlying object with id, value and 1422 * extensions. The accessor "getResidueSite" gives direct access to 1423 * the value 1424 */ 1425 public SubstanceNucleicAcidSubunitSugarComponent setResidueSiteElement(StringType value) { 1426 this.residueSite = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return The residues that contain a given sugar will be captured. The order 1432 * of given residues will be captured in the 5?-3?direction consistent 1433 * with the base sequences listed above. 1434 */ 1435 public String getResidueSite() { 1436 return this.residueSite == null ? null : this.residueSite.getValue(); 1437 } 1438 1439 /** 1440 * @param value The residues that contain a given sugar will be captured. The 1441 * order of given residues will be captured in the 5?-3?direction 1442 * consistent with the base sequences listed above. 1443 */ 1444 public SubstanceNucleicAcidSubunitSugarComponent setResidueSite(String value) { 1445 if (Utilities.noString(value)) 1446 this.residueSite = null; 1447 else { 1448 if (this.residueSite == null) 1449 this.residueSite = new StringType(); 1450 this.residueSite.setValue(value); 1451 } 1452 return this; 1453 } 1454 1455 protected void listChildren(List<Property> children) { 1456 super.listChildren(children); 1457 children.add(new Property("identifier", "Identifier", 1458 "The Substance ID of the sugar or sugar-like component that make up the nucleotide.", 0, 1, identifier)); 1459 children.add(new Property("name", "string", 1460 "The name of the sugar or sugar-like component that make up the nucleotide.", 0, 1, name)); 1461 children.add(new Property("residueSite", "string", 1462 "The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above.", 1463 0, 1, residueSite)); 1464 } 1465 1466 @Override 1467 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1468 switch (_hash) { 1469 case -1618432855: 1470 /* identifier */ return new Property("identifier", "Identifier", 1471 "The Substance ID of the sugar or sugar-like component that make up the nucleotide.", 0, 1, identifier); 1472 case 3373707: 1473 /* name */ return new Property("name", "string", 1474 "The name of the sugar or sugar-like component that make up the nucleotide.", 0, 1, name); 1475 case 1547124594: 1476 /* residueSite */ return new Property("residueSite", "string", 1477 "The residues that contain a given sugar will be captured. The order of given residues will be captured in the 5?-3?direction consistent with the base sequences listed above.", 1478 0, 1, residueSite); 1479 default: 1480 return super.getNamedProperty(_hash, _name, _checkValid); 1481 } 1482 1483 } 1484 1485 @Override 1486 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1487 switch (hash) { 1488 case -1618432855: 1489 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 1490 case 3373707: 1491 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1492 case 1547124594: 1493 /* residueSite */ return this.residueSite == null ? new Base[0] : new Base[] { this.residueSite }; // StringType 1494 default: 1495 return super.getProperty(hash, name, checkValid); 1496 } 1497 1498 } 1499 1500 @Override 1501 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1502 switch (hash) { 1503 case -1618432855: // identifier 1504 this.identifier = castToIdentifier(value); // Identifier 1505 return value; 1506 case 3373707: // name 1507 this.name = castToString(value); // StringType 1508 return value; 1509 case 1547124594: // residueSite 1510 this.residueSite = castToString(value); // StringType 1511 return value; 1512 default: 1513 return super.setProperty(hash, name, value); 1514 } 1515 1516 } 1517 1518 @Override 1519 public Base setProperty(String name, Base value) throws FHIRException { 1520 if (name.equals("identifier")) { 1521 this.identifier = castToIdentifier(value); // Identifier 1522 } else if (name.equals("name")) { 1523 this.name = castToString(value); // StringType 1524 } else if (name.equals("residueSite")) { 1525 this.residueSite = castToString(value); // StringType 1526 } else 1527 return super.setProperty(name, value); 1528 return value; 1529 } 1530 1531 @Override 1532 public void removeChild(String name, Base value) throws FHIRException { 1533 if (name.equals("identifier")) { 1534 this.identifier = null; 1535 } else if (name.equals("name")) { 1536 this.name = null; 1537 } else if (name.equals("residueSite")) { 1538 this.residueSite = null; 1539 } else 1540 super.removeChild(name, value); 1541 1542 } 1543 1544 @Override 1545 public Base makeProperty(int hash, String name) throws FHIRException { 1546 switch (hash) { 1547 case -1618432855: 1548 return getIdentifier(); 1549 case 3373707: 1550 return getNameElement(); 1551 case 1547124594: 1552 return getResidueSiteElement(); 1553 default: 1554 return super.makeProperty(hash, name); 1555 } 1556 1557 } 1558 1559 @Override 1560 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1561 switch (hash) { 1562 case -1618432855: 1563 /* identifier */ return new String[] { "Identifier" }; 1564 case 3373707: 1565 /* name */ return new String[] { "string" }; 1566 case 1547124594: 1567 /* residueSite */ return new String[] { "string" }; 1568 default: 1569 return super.getTypesForProperty(hash, name); 1570 } 1571 1572 } 1573 1574 @Override 1575 public Base addChild(String name) throws FHIRException { 1576 if (name.equals("identifier")) { 1577 this.identifier = new Identifier(); 1578 return this.identifier; 1579 } else if (name.equals("name")) { 1580 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.name"); 1581 } else if (name.equals("residueSite")) { 1582 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.residueSite"); 1583 } else 1584 return super.addChild(name); 1585 } 1586 1587 public SubstanceNucleicAcidSubunitSugarComponent copy() { 1588 SubstanceNucleicAcidSubunitSugarComponent dst = new SubstanceNucleicAcidSubunitSugarComponent(); 1589 copyValues(dst); 1590 return dst; 1591 } 1592 1593 public void copyValues(SubstanceNucleicAcidSubunitSugarComponent dst) { 1594 super.copyValues(dst); 1595 dst.identifier = identifier == null ? null : identifier.copy(); 1596 dst.name = name == null ? null : name.copy(); 1597 dst.residueSite = residueSite == null ? null : residueSite.copy(); 1598 } 1599 1600 @Override 1601 public boolean equalsDeep(Base other_) { 1602 if (!super.equalsDeep(other_)) 1603 return false; 1604 if (!(other_ instanceof SubstanceNucleicAcidSubunitSugarComponent)) 1605 return false; 1606 SubstanceNucleicAcidSubunitSugarComponent o = (SubstanceNucleicAcidSubunitSugarComponent) other_; 1607 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 1608 && compareDeep(residueSite, o.residueSite, true); 1609 } 1610 1611 @Override 1612 public boolean equalsShallow(Base other_) { 1613 if (!super.equalsShallow(other_)) 1614 return false; 1615 if (!(other_ instanceof SubstanceNucleicAcidSubunitSugarComponent)) 1616 return false; 1617 SubstanceNucleicAcidSubunitSugarComponent o = (SubstanceNucleicAcidSubunitSugarComponent) other_; 1618 return compareValues(name, o.name, true) && compareValues(residueSite, o.residueSite, true); 1619 } 1620 1621 public boolean isEmpty() { 1622 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, residueSite); 1623 } 1624 1625 public String fhirType() { 1626 return "SubstanceNucleicAcid.subunit.sugar"; 1627 1628 } 1629 1630 } 1631 1632 /** 1633 * The type of the sequence shall be specified based on a controlled vocabulary. 1634 */ 1635 @Child(name = "sequenceType", type = { 1636 CodeableConcept.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1637 @Description(shortDefinition = "The type of the sequence shall be specified based on a controlled vocabulary", formalDefinition = "The type of the sequence shall be specified based on a controlled vocabulary.") 1638 protected CodeableConcept sequenceType; 1639 1640 /** 1641 * The number of linear sequences of nucleotides linked through phosphodiester 1642 * bonds shall be described. Subunits would be strands of nucleic acids that are 1643 * tightly associated typically through Watson-Crick base pairing. NOTE: If not 1644 * specified in the reference source, the assumption is that there is 1 subunit. 1645 */ 1646 @Child(name = "numberOfSubunits", type = { 1647 IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1648 @Description(shortDefinition = "The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit", formalDefinition = "The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit.") 1649 protected IntegerType numberOfSubunits; 1650 1651 /** 1652 * The area of hybridisation shall be described if applicable for double 1653 * stranded RNA or DNA. The number associated with the subunit followed by the 1654 * number associated to the residue shall be specified in increasing order. The 1655 * underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?. 1656 */ 1657 @Child(name = "areaOfHybridisation", type = { 1658 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1659 @Description(shortDefinition = "The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?", formalDefinition = "The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?.") 1660 protected StringType areaOfHybridisation; 1661 1662 /** 1663 * (TBC). 1664 */ 1665 @Child(name = "oligoNucleotideType", type = { 1666 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1667 @Description(shortDefinition = "(TBC)", formalDefinition = "(TBC).") 1668 protected CodeableConcept oligoNucleotideType; 1669 1670 /** 1671 * Subunits are listed in order of decreasing length; sequences of the same 1672 * length will be ordered by molecular weight; subunits that have identical 1673 * sequences will be repeated multiple times. 1674 */ 1675 @Child(name = "subunit", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1676 @Description(shortDefinition = "Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times", formalDefinition = "Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times.") 1677 protected List<SubstanceNucleicAcidSubunitComponent> subunit; 1678 1679 private static final long serialVersionUID = -1906822433L; 1680 1681 /** 1682 * Constructor 1683 */ 1684 public SubstanceNucleicAcid() { 1685 super(); 1686 } 1687 1688 /** 1689 * @return {@link #sequenceType} (The type of the sequence shall be specified 1690 * based on a controlled vocabulary.) 1691 */ 1692 public CodeableConcept getSequenceType() { 1693 if (this.sequenceType == null) 1694 if (Configuration.errorOnAutoCreate()) 1695 throw new Error("Attempt to auto-create SubstanceNucleicAcid.sequenceType"); 1696 else if (Configuration.doAutoCreate()) 1697 this.sequenceType = new CodeableConcept(); // cc 1698 return this.sequenceType; 1699 } 1700 1701 public boolean hasSequenceType() { 1702 return this.sequenceType != null && !this.sequenceType.isEmpty(); 1703 } 1704 1705 /** 1706 * @param value {@link #sequenceType} (The type of the sequence shall be 1707 * specified based on a controlled vocabulary.) 1708 */ 1709 public SubstanceNucleicAcid setSequenceType(CodeableConcept value) { 1710 this.sequenceType = value; 1711 return this; 1712 } 1713 1714 /** 1715 * @return {@link #numberOfSubunits} (The number of linear sequences of 1716 * nucleotides linked through phosphodiester bonds shall be described. 1717 * Subunits would be strands of nucleic acids that are tightly 1718 * associated typically through Watson-Crick base pairing. NOTE: If not 1719 * specified in the reference source, the assumption is that there is 1 1720 * subunit.). This is the underlying object with id, value and 1721 * extensions. The accessor "getNumberOfSubunits" gives direct access to 1722 * the value 1723 */ 1724 public IntegerType getNumberOfSubunitsElement() { 1725 if (this.numberOfSubunits == null) 1726 if (Configuration.errorOnAutoCreate()) 1727 throw new Error("Attempt to auto-create SubstanceNucleicAcid.numberOfSubunits"); 1728 else if (Configuration.doAutoCreate()) 1729 this.numberOfSubunits = new IntegerType(); // bb 1730 return this.numberOfSubunits; 1731 } 1732 1733 public boolean hasNumberOfSubunitsElement() { 1734 return this.numberOfSubunits != null && !this.numberOfSubunits.isEmpty(); 1735 } 1736 1737 public boolean hasNumberOfSubunits() { 1738 return this.numberOfSubunits != null && !this.numberOfSubunits.isEmpty(); 1739 } 1740 1741 /** 1742 * @param value {@link #numberOfSubunits} (The number of linear sequences of 1743 * nucleotides linked through phosphodiester bonds shall be 1744 * described. Subunits would be strands of nucleic acids that are 1745 * tightly associated typically through Watson-Crick base pairing. 1746 * NOTE: If not specified in the reference source, the assumption 1747 * is that there is 1 subunit.). This is the underlying object with 1748 * id, value and extensions. The accessor "getNumberOfSubunits" 1749 * gives direct access to the value 1750 */ 1751 public SubstanceNucleicAcid setNumberOfSubunitsElement(IntegerType value) { 1752 this.numberOfSubunits = value; 1753 return this; 1754 } 1755 1756 /** 1757 * @return The number of linear sequences of nucleotides linked through 1758 * phosphodiester bonds shall be described. Subunits would be strands of 1759 * nucleic acids that are tightly associated typically through 1760 * Watson-Crick base pairing. NOTE: If not specified in the reference 1761 * source, the assumption is that there is 1 subunit. 1762 */ 1763 public int getNumberOfSubunits() { 1764 return this.numberOfSubunits == null || this.numberOfSubunits.isEmpty() ? 0 : this.numberOfSubunits.getValue(); 1765 } 1766 1767 /** 1768 * @param value The number of linear sequences of nucleotides linked through 1769 * phosphodiester bonds shall be described. Subunits would be 1770 * strands of nucleic acids that are tightly associated typically 1771 * through Watson-Crick base pairing. NOTE: If not specified in the 1772 * reference source, the assumption is that there is 1 subunit. 1773 */ 1774 public SubstanceNucleicAcid setNumberOfSubunits(int value) { 1775 if (this.numberOfSubunits == null) 1776 this.numberOfSubunits = new IntegerType(); 1777 this.numberOfSubunits.setValue(value); 1778 return this; 1779 } 1780 1781 /** 1782 * @return {@link #areaOfHybridisation} (The area of hybridisation shall be 1783 * described if applicable for double stranded RNA or DNA. The number 1784 * associated with the subunit followed by the number associated to the 1785 * residue shall be specified in increasing order. The underscore ?? 1786 * shall be used as separator as follows: ?Subunitnumber Residue?.). 1787 * This is the underlying object with id, value and extensions. The 1788 * accessor "getAreaOfHybridisation" gives direct access to the value 1789 */ 1790 public StringType getAreaOfHybridisationElement() { 1791 if (this.areaOfHybridisation == null) 1792 if (Configuration.errorOnAutoCreate()) 1793 throw new Error("Attempt to auto-create SubstanceNucleicAcid.areaOfHybridisation"); 1794 else if (Configuration.doAutoCreate()) 1795 this.areaOfHybridisation = new StringType(); // bb 1796 return this.areaOfHybridisation; 1797 } 1798 1799 public boolean hasAreaOfHybridisationElement() { 1800 return this.areaOfHybridisation != null && !this.areaOfHybridisation.isEmpty(); 1801 } 1802 1803 public boolean hasAreaOfHybridisation() { 1804 return this.areaOfHybridisation != null && !this.areaOfHybridisation.isEmpty(); 1805 } 1806 1807 /** 1808 * @param value {@link #areaOfHybridisation} (The area of hybridisation shall be 1809 * described if applicable for double stranded RNA or DNA. The 1810 * number associated with the subunit followed by the number 1811 * associated to the residue shall be specified in increasing 1812 * order. The underscore ?? shall be used as separator as follows: 1813 * ?Subunitnumber Residue?.). This is the underlying object with 1814 * id, value and extensions. The accessor "getAreaOfHybridisation" 1815 * gives direct access to the value 1816 */ 1817 public SubstanceNucleicAcid setAreaOfHybridisationElement(StringType value) { 1818 this.areaOfHybridisation = value; 1819 return this; 1820 } 1821 1822 /** 1823 * @return The area of hybridisation shall be described if applicable for double 1824 * stranded RNA or DNA. The number associated with the subunit followed 1825 * by the number associated to the residue shall be specified in 1826 * increasing order. The underscore ?? shall be used as separator as 1827 * follows: ?Subunitnumber Residue?. 1828 */ 1829 public String getAreaOfHybridisation() { 1830 return this.areaOfHybridisation == null ? null : this.areaOfHybridisation.getValue(); 1831 } 1832 1833 /** 1834 * @param value The area of hybridisation shall be described if applicable for 1835 * double stranded RNA or DNA. The number associated with the 1836 * subunit followed by the number associated to the residue shall 1837 * be specified in increasing order. The underscore ?? shall be 1838 * used as separator as follows: ?Subunitnumber Residue?. 1839 */ 1840 public SubstanceNucleicAcid setAreaOfHybridisation(String value) { 1841 if (Utilities.noString(value)) 1842 this.areaOfHybridisation = null; 1843 else { 1844 if (this.areaOfHybridisation == null) 1845 this.areaOfHybridisation = new StringType(); 1846 this.areaOfHybridisation.setValue(value); 1847 } 1848 return this; 1849 } 1850 1851 /** 1852 * @return {@link #oligoNucleotideType} ((TBC).) 1853 */ 1854 public CodeableConcept getOligoNucleotideType() { 1855 if (this.oligoNucleotideType == null) 1856 if (Configuration.errorOnAutoCreate()) 1857 throw new Error("Attempt to auto-create SubstanceNucleicAcid.oligoNucleotideType"); 1858 else if (Configuration.doAutoCreate()) 1859 this.oligoNucleotideType = new CodeableConcept(); // cc 1860 return this.oligoNucleotideType; 1861 } 1862 1863 public boolean hasOligoNucleotideType() { 1864 return this.oligoNucleotideType != null && !this.oligoNucleotideType.isEmpty(); 1865 } 1866 1867 /** 1868 * @param value {@link #oligoNucleotideType} ((TBC).) 1869 */ 1870 public SubstanceNucleicAcid setOligoNucleotideType(CodeableConcept value) { 1871 this.oligoNucleotideType = value; 1872 return this; 1873 } 1874 1875 /** 1876 * @return {@link #subunit} (Subunits are listed in order of decreasing length; 1877 * sequences of the same length will be ordered by molecular weight; 1878 * subunits that have identical sequences will be repeated multiple 1879 * times.) 1880 */ 1881 public List<SubstanceNucleicAcidSubunitComponent> getSubunit() { 1882 if (this.subunit == null) 1883 this.subunit = new ArrayList<SubstanceNucleicAcidSubunitComponent>(); 1884 return this.subunit; 1885 } 1886 1887 /** 1888 * @return Returns a reference to <code>this</code> for easy method chaining 1889 */ 1890 public SubstanceNucleicAcid setSubunit(List<SubstanceNucleicAcidSubunitComponent> theSubunit) { 1891 this.subunit = theSubunit; 1892 return this; 1893 } 1894 1895 public boolean hasSubunit() { 1896 if (this.subunit == null) 1897 return false; 1898 for (SubstanceNucleicAcidSubunitComponent item : this.subunit) 1899 if (!item.isEmpty()) 1900 return true; 1901 return false; 1902 } 1903 1904 public SubstanceNucleicAcidSubunitComponent addSubunit() { // 3 1905 SubstanceNucleicAcidSubunitComponent t = new SubstanceNucleicAcidSubunitComponent(); 1906 if (this.subunit == null) 1907 this.subunit = new ArrayList<SubstanceNucleicAcidSubunitComponent>(); 1908 this.subunit.add(t); 1909 return t; 1910 } 1911 1912 public SubstanceNucleicAcid addSubunit(SubstanceNucleicAcidSubunitComponent t) { // 3 1913 if (t == null) 1914 return this; 1915 if (this.subunit == null) 1916 this.subunit = new ArrayList<SubstanceNucleicAcidSubunitComponent>(); 1917 this.subunit.add(t); 1918 return this; 1919 } 1920 1921 /** 1922 * @return The first repetition of repeating field {@link #subunit}, creating it 1923 * if it does not already exist 1924 */ 1925 public SubstanceNucleicAcidSubunitComponent getSubunitFirstRep() { 1926 if (getSubunit().isEmpty()) { 1927 addSubunit(); 1928 } 1929 return getSubunit().get(0); 1930 } 1931 1932 protected void listChildren(List<Property> children) { 1933 super.listChildren(children); 1934 children.add(new Property("sequenceType", "CodeableConcept", 1935 "The type of the sequence shall be specified based on a controlled vocabulary.", 0, 1, sequenceType)); 1936 children.add(new Property("numberOfSubunits", "integer", 1937 "The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit.", 1938 0, 1, numberOfSubunits)); 1939 children.add(new Property("areaOfHybridisation", "string", 1940 "The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?.", 1941 0, 1, areaOfHybridisation)); 1942 children.add(new Property("oligoNucleotideType", "CodeableConcept", "(TBC).", 0, 1, oligoNucleotideType)); 1943 children.add(new Property("subunit", "", 1944 "Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times.", 1945 0, java.lang.Integer.MAX_VALUE, subunit)); 1946 } 1947 1948 @Override 1949 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1950 switch (_hash) { 1951 case 807711387: 1952 /* sequenceType */ return new Property("sequenceType", "CodeableConcept", 1953 "The type of the sequence shall be specified based on a controlled vocabulary.", 0, 1, sequenceType); 1954 case -847111089: 1955 /* numberOfSubunits */ return new Property("numberOfSubunits", "integer", 1956 "The number of linear sequences of nucleotides linked through phosphodiester bonds shall be described. Subunits would be strands of nucleic acids that are tightly associated typically through Watson-Crick base pairing. NOTE: If not specified in the reference source, the assumption is that there is 1 subunit.", 1957 0, 1, numberOfSubunits); 1958 case -617269845: 1959 /* areaOfHybridisation */ return new Property("areaOfHybridisation", "string", 1960 "The area of hybridisation shall be described if applicable for double stranded RNA or DNA. The number associated with the subunit followed by the number associated to the residue shall be specified in increasing order. The underscore ?? shall be used as separator as follows: ?Subunitnumber Residue?.", 1961 0, 1, areaOfHybridisation); 1962 case -1526251938: 1963 /* oligoNucleotideType */ return new Property("oligoNucleotideType", "CodeableConcept", "(TBC).", 0, 1, 1964 oligoNucleotideType); 1965 case -1867548732: 1966 /* subunit */ return new Property("subunit", "", 1967 "Subunits are listed in order of decreasing length; sequences of the same length will be ordered by molecular weight; subunits that have identical sequences will be repeated multiple times.", 1968 0, java.lang.Integer.MAX_VALUE, subunit); 1969 default: 1970 return super.getNamedProperty(_hash, _name, _checkValid); 1971 } 1972 1973 } 1974 1975 @Override 1976 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1977 switch (hash) { 1978 case 807711387: 1979 /* sequenceType */ return this.sequenceType == null ? new Base[0] : new Base[] { this.sequenceType }; // CodeableConcept 1980 case -847111089: 1981 /* numberOfSubunits */ return this.numberOfSubunits == null ? new Base[0] : new Base[] { this.numberOfSubunits }; // IntegerType 1982 case -617269845: 1983 /* areaOfHybridisation */ return this.areaOfHybridisation == null ? new Base[0] 1984 : new Base[] { this.areaOfHybridisation }; // StringType 1985 case -1526251938: 1986 /* oligoNucleotideType */ return this.oligoNucleotideType == null ? new Base[0] 1987 : new Base[] { this.oligoNucleotideType }; // CodeableConcept 1988 case -1867548732: 1989 /* subunit */ return this.subunit == null ? new Base[0] : this.subunit.toArray(new Base[this.subunit.size()]); // SubstanceNucleicAcidSubunitComponent 1990 default: 1991 return super.getProperty(hash, name, checkValid); 1992 } 1993 1994 } 1995 1996 @Override 1997 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1998 switch (hash) { 1999 case 807711387: // sequenceType 2000 this.sequenceType = castToCodeableConcept(value); // CodeableConcept 2001 return value; 2002 case -847111089: // numberOfSubunits 2003 this.numberOfSubunits = castToInteger(value); // IntegerType 2004 return value; 2005 case -617269845: // areaOfHybridisation 2006 this.areaOfHybridisation = castToString(value); // StringType 2007 return value; 2008 case -1526251938: // oligoNucleotideType 2009 this.oligoNucleotideType = castToCodeableConcept(value); // CodeableConcept 2010 return value; 2011 case -1867548732: // subunit 2012 this.getSubunit().add((SubstanceNucleicAcidSubunitComponent) value); // SubstanceNucleicAcidSubunitComponent 2013 return value; 2014 default: 2015 return super.setProperty(hash, name, value); 2016 } 2017 2018 } 2019 2020 @Override 2021 public Base setProperty(String name, Base value) throws FHIRException { 2022 if (name.equals("sequenceType")) { 2023 this.sequenceType = castToCodeableConcept(value); // CodeableConcept 2024 } else if (name.equals("numberOfSubunits")) { 2025 this.numberOfSubunits = castToInteger(value); // IntegerType 2026 } else if (name.equals("areaOfHybridisation")) { 2027 this.areaOfHybridisation = castToString(value); // StringType 2028 } else if (name.equals("oligoNucleotideType")) { 2029 this.oligoNucleotideType = castToCodeableConcept(value); // CodeableConcept 2030 } else if (name.equals("subunit")) { 2031 this.getSubunit().add((SubstanceNucleicAcidSubunitComponent) value); 2032 } else 2033 return super.setProperty(name, value); 2034 return value; 2035 } 2036 2037 @Override 2038 public void removeChild(String name, Base value) throws FHIRException { 2039 if (name.equals("sequenceType")) { 2040 this.sequenceType = null; 2041 } else if (name.equals("numberOfSubunits")) { 2042 this.numberOfSubunits = null; 2043 } else if (name.equals("areaOfHybridisation")) { 2044 this.areaOfHybridisation = null; 2045 } else if (name.equals("oligoNucleotideType")) { 2046 this.oligoNucleotideType = null; 2047 } else if (name.equals("subunit")) { 2048 this.getSubunit().remove((SubstanceNucleicAcidSubunitComponent) value); 2049 } else 2050 super.removeChild(name, value); 2051 2052 } 2053 2054 @Override 2055 public Base makeProperty(int hash, String name) throws FHIRException { 2056 switch (hash) { 2057 case 807711387: 2058 return getSequenceType(); 2059 case -847111089: 2060 return getNumberOfSubunitsElement(); 2061 case -617269845: 2062 return getAreaOfHybridisationElement(); 2063 case -1526251938: 2064 return getOligoNucleotideType(); 2065 case -1867548732: 2066 return addSubunit(); 2067 default: 2068 return super.makeProperty(hash, name); 2069 } 2070 2071 } 2072 2073 @Override 2074 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2075 switch (hash) { 2076 case 807711387: 2077 /* sequenceType */ return new String[] { "CodeableConcept" }; 2078 case -847111089: 2079 /* numberOfSubunits */ return new String[] { "integer" }; 2080 case -617269845: 2081 /* areaOfHybridisation */ return new String[] { "string" }; 2082 case -1526251938: 2083 /* oligoNucleotideType */ return new String[] { "CodeableConcept" }; 2084 case -1867548732: 2085 /* subunit */ return new String[] {}; 2086 default: 2087 return super.getTypesForProperty(hash, name); 2088 } 2089 2090 } 2091 2092 @Override 2093 public Base addChild(String name) throws FHIRException { 2094 if (name.equals("sequenceType")) { 2095 this.sequenceType = new CodeableConcept(); 2096 return this.sequenceType; 2097 } else if (name.equals("numberOfSubunits")) { 2098 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.numberOfSubunits"); 2099 } else if (name.equals("areaOfHybridisation")) { 2100 throw new FHIRException("Cannot call addChild on a singleton property SubstanceNucleicAcid.areaOfHybridisation"); 2101 } else if (name.equals("oligoNucleotideType")) { 2102 this.oligoNucleotideType = new CodeableConcept(); 2103 return this.oligoNucleotideType; 2104 } else if (name.equals("subunit")) { 2105 return addSubunit(); 2106 } else 2107 return super.addChild(name); 2108 } 2109 2110 public String fhirType() { 2111 return "SubstanceNucleicAcid"; 2112 2113 } 2114 2115 public SubstanceNucleicAcid copy() { 2116 SubstanceNucleicAcid dst = new SubstanceNucleicAcid(); 2117 copyValues(dst); 2118 return dst; 2119 } 2120 2121 public void copyValues(SubstanceNucleicAcid dst) { 2122 super.copyValues(dst); 2123 dst.sequenceType = sequenceType == null ? null : sequenceType.copy(); 2124 dst.numberOfSubunits = numberOfSubunits == null ? null : numberOfSubunits.copy(); 2125 dst.areaOfHybridisation = areaOfHybridisation == null ? null : areaOfHybridisation.copy(); 2126 dst.oligoNucleotideType = oligoNucleotideType == null ? null : oligoNucleotideType.copy(); 2127 if (subunit != null) { 2128 dst.subunit = new ArrayList<SubstanceNucleicAcidSubunitComponent>(); 2129 for (SubstanceNucleicAcidSubunitComponent i : subunit) 2130 dst.subunit.add(i.copy()); 2131 } 2132 ; 2133 } 2134 2135 protected SubstanceNucleicAcid typedCopy() { 2136 return copy(); 2137 } 2138 2139 @Override 2140 public boolean equalsDeep(Base other_) { 2141 if (!super.equalsDeep(other_)) 2142 return false; 2143 if (!(other_ instanceof SubstanceNucleicAcid)) 2144 return false; 2145 SubstanceNucleicAcid o = (SubstanceNucleicAcid) other_; 2146 return compareDeep(sequenceType, o.sequenceType, true) && compareDeep(numberOfSubunits, o.numberOfSubunits, true) 2147 && compareDeep(areaOfHybridisation, o.areaOfHybridisation, true) 2148 && compareDeep(oligoNucleotideType, o.oligoNucleotideType, true) && compareDeep(subunit, o.subunit, true); 2149 } 2150 2151 @Override 2152 public boolean equalsShallow(Base other_) { 2153 if (!super.equalsShallow(other_)) 2154 return false; 2155 if (!(other_ instanceof SubstanceNucleicAcid)) 2156 return false; 2157 SubstanceNucleicAcid o = (SubstanceNucleicAcid) other_; 2158 return compareValues(numberOfSubunits, o.numberOfSubunits, true) 2159 && compareValues(areaOfHybridisation, o.areaOfHybridisation, true); 2160 } 2161 2162 public boolean isEmpty() { 2163 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceType, numberOfSubunits, areaOfHybridisation, 2164 oligoNucleotideType, subunit); 2165 } 2166 2167 @Override 2168 public ResourceType getResourceType() { 2169 return ResourceType.SubstanceNucleicAcid; 2170 } 2171 2172}