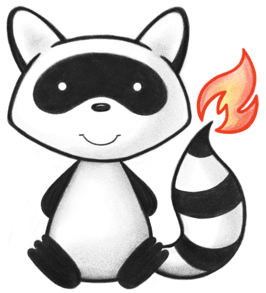
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044 045/** 046 * Todo. 047 */ 048@ResourceDef(name = "SubstancePolymer", profile = "http://hl7.org/fhir/StructureDefinition/SubstancePolymer") 049public class SubstancePolymer extends DomainResource { 050 051 @Block() 052 public static class SubstancePolymerMonomerSetComponent extends BackboneElement implements IBaseBackboneElement { 053 /** 054 * Todo. 055 */ 056 @Child(name = "ratioType", type = { 057 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 058 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 059 protected CodeableConcept ratioType; 060 061 /** 062 * Todo. 063 */ 064 @Child(name = "startingMaterial", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 065 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 066 protected List<SubstancePolymerMonomerSetStartingMaterialComponent> startingMaterial; 067 068 private static final long serialVersionUID = -933825014L; 069 070 /** 071 * Constructor 072 */ 073 public SubstancePolymerMonomerSetComponent() { 074 super(); 075 } 076 077 /** 078 * @return {@link #ratioType} (Todo.) 079 */ 080 public CodeableConcept getRatioType() { 081 if (this.ratioType == null) 082 if (Configuration.errorOnAutoCreate()) 083 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetComponent.ratioType"); 084 else if (Configuration.doAutoCreate()) 085 this.ratioType = new CodeableConcept(); // cc 086 return this.ratioType; 087 } 088 089 public boolean hasRatioType() { 090 return this.ratioType != null && !this.ratioType.isEmpty(); 091 } 092 093 /** 094 * @param value {@link #ratioType} (Todo.) 095 */ 096 public SubstancePolymerMonomerSetComponent setRatioType(CodeableConcept value) { 097 this.ratioType = value; 098 return this; 099 } 100 101 /** 102 * @return {@link #startingMaterial} (Todo.) 103 */ 104 public List<SubstancePolymerMonomerSetStartingMaterialComponent> getStartingMaterial() { 105 if (this.startingMaterial == null) 106 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 107 return this.startingMaterial; 108 } 109 110 /** 111 * @return Returns a reference to <code>this</code> for easy method chaining 112 */ 113 public SubstancePolymerMonomerSetComponent setStartingMaterial( 114 List<SubstancePolymerMonomerSetStartingMaterialComponent> theStartingMaterial) { 115 this.startingMaterial = theStartingMaterial; 116 return this; 117 } 118 119 public boolean hasStartingMaterial() { 120 if (this.startingMaterial == null) 121 return false; 122 for (SubstancePolymerMonomerSetStartingMaterialComponent item : this.startingMaterial) 123 if (!item.isEmpty()) 124 return true; 125 return false; 126 } 127 128 public SubstancePolymerMonomerSetStartingMaterialComponent addStartingMaterial() { // 3 129 SubstancePolymerMonomerSetStartingMaterialComponent t = new SubstancePolymerMonomerSetStartingMaterialComponent(); 130 if (this.startingMaterial == null) 131 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 132 this.startingMaterial.add(t); 133 return t; 134 } 135 136 public SubstancePolymerMonomerSetComponent addStartingMaterial( 137 SubstancePolymerMonomerSetStartingMaterialComponent t) { // 3 138 if (t == null) 139 return this; 140 if (this.startingMaterial == null) 141 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 142 this.startingMaterial.add(t); 143 return this; 144 } 145 146 /** 147 * @return The first repetition of repeating field {@link #startingMaterial}, 148 * creating it if it does not already exist 149 */ 150 public SubstancePolymerMonomerSetStartingMaterialComponent getStartingMaterialFirstRep() { 151 if (getStartingMaterial().isEmpty()) { 152 addStartingMaterial(); 153 } 154 return getStartingMaterial().get(0); 155 } 156 157 protected void listChildren(List<Property> children) { 158 super.listChildren(children); 159 children.add(new Property("ratioType", "CodeableConcept", "Todo.", 0, 1, ratioType)); 160 children.add(new Property("startingMaterial", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, startingMaterial)); 161 } 162 163 @Override 164 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 165 switch (_hash) { 166 case 344937957: 167 /* ratioType */ return new Property("ratioType", "CodeableConcept", "Todo.", 0, 1, ratioType); 168 case 442919303: 169 /* startingMaterial */ return new Property("startingMaterial", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, 170 startingMaterial); 171 default: 172 return super.getNamedProperty(_hash, _name, _checkValid); 173 } 174 175 } 176 177 @Override 178 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 179 switch (hash) { 180 case 344937957: 181 /* ratioType */ return this.ratioType == null ? new Base[0] : new Base[] { this.ratioType }; // CodeableConcept 182 case 442919303: 183 /* startingMaterial */ return this.startingMaterial == null ? new Base[0] 184 : this.startingMaterial.toArray(new Base[this.startingMaterial.size()]); // SubstancePolymerMonomerSetStartingMaterialComponent 185 default: 186 return super.getProperty(hash, name, checkValid); 187 } 188 189 } 190 191 @Override 192 public Base setProperty(int hash, String name, Base value) throws FHIRException { 193 switch (hash) { 194 case 344937957: // ratioType 195 this.ratioType = castToCodeableConcept(value); // CodeableConcept 196 return value; 197 case 442919303: // startingMaterial 198 this.getStartingMaterial().add((SubstancePolymerMonomerSetStartingMaterialComponent) value); // SubstancePolymerMonomerSetStartingMaterialComponent 199 return value; 200 default: 201 return super.setProperty(hash, name, value); 202 } 203 204 } 205 206 @Override 207 public Base setProperty(String name, Base value) throws FHIRException { 208 if (name.equals("ratioType")) { 209 this.ratioType = castToCodeableConcept(value); // CodeableConcept 210 } else if (name.equals("startingMaterial")) { 211 this.getStartingMaterial().add((SubstancePolymerMonomerSetStartingMaterialComponent) value); 212 } else 213 return super.setProperty(name, value); 214 return value; 215 } 216 217 @Override 218 public void removeChild(String name, Base value) throws FHIRException { 219 if (name.equals("ratioType")) { 220 this.ratioType = null; 221 } else if (name.equals("startingMaterial")) { 222 this.getStartingMaterial().remove((SubstancePolymerMonomerSetStartingMaterialComponent) value); 223 } else 224 super.removeChild(name, value); 225 226 } 227 228 @Override 229 public Base makeProperty(int hash, String name) throws FHIRException { 230 switch (hash) { 231 case 344937957: 232 return getRatioType(); 233 case 442919303: 234 return addStartingMaterial(); 235 default: 236 return super.makeProperty(hash, name); 237 } 238 239 } 240 241 @Override 242 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 243 switch (hash) { 244 case 344937957: 245 /* ratioType */ return new String[] { "CodeableConcept" }; 246 case 442919303: 247 /* startingMaterial */ return new String[] {}; 248 default: 249 return super.getTypesForProperty(hash, name); 250 } 251 252 } 253 254 @Override 255 public Base addChild(String name) throws FHIRException { 256 if (name.equals("ratioType")) { 257 this.ratioType = new CodeableConcept(); 258 return this.ratioType; 259 } else if (name.equals("startingMaterial")) { 260 return addStartingMaterial(); 261 } else 262 return super.addChild(name); 263 } 264 265 public SubstancePolymerMonomerSetComponent copy() { 266 SubstancePolymerMonomerSetComponent dst = new SubstancePolymerMonomerSetComponent(); 267 copyValues(dst); 268 return dst; 269 } 270 271 public void copyValues(SubstancePolymerMonomerSetComponent dst) { 272 super.copyValues(dst); 273 dst.ratioType = ratioType == null ? null : ratioType.copy(); 274 if (startingMaterial != null) { 275 dst.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 276 for (SubstancePolymerMonomerSetStartingMaterialComponent i : startingMaterial) 277 dst.startingMaterial.add(i.copy()); 278 } 279 ; 280 } 281 282 @Override 283 public boolean equalsDeep(Base other_) { 284 if (!super.equalsDeep(other_)) 285 return false; 286 if (!(other_ instanceof SubstancePolymerMonomerSetComponent)) 287 return false; 288 SubstancePolymerMonomerSetComponent o = (SubstancePolymerMonomerSetComponent) other_; 289 return compareDeep(ratioType, o.ratioType, true) && compareDeep(startingMaterial, o.startingMaterial, true); 290 } 291 292 @Override 293 public boolean equalsShallow(Base other_) { 294 if (!super.equalsShallow(other_)) 295 return false; 296 if (!(other_ instanceof SubstancePolymerMonomerSetComponent)) 297 return false; 298 SubstancePolymerMonomerSetComponent o = (SubstancePolymerMonomerSetComponent) other_; 299 return true; 300 } 301 302 public boolean isEmpty() { 303 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(ratioType, startingMaterial); 304 } 305 306 public String fhirType() { 307 return "SubstancePolymer.monomerSet"; 308 309 } 310 311 } 312 313 @Block() 314 public static class SubstancePolymerMonomerSetStartingMaterialComponent extends BackboneElement 315 implements IBaseBackboneElement { 316 /** 317 * Todo. 318 */ 319 @Child(name = "material", type = { 320 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 321 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 322 protected CodeableConcept material; 323 324 /** 325 * Todo. 326 */ 327 @Child(name = "type", type = { 328 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 329 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 330 protected CodeableConcept type; 331 332 /** 333 * Todo. 334 */ 335 @Child(name = "isDefining", type = { 336 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 337 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 338 protected BooleanType isDefining; 339 340 /** 341 * Todo. 342 */ 343 @Child(name = "amount", type = { 344 SubstanceAmount.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 345 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 346 protected SubstanceAmount amount; 347 348 private static final long serialVersionUID = 589614045L; 349 350 /** 351 * Constructor 352 */ 353 public SubstancePolymerMonomerSetStartingMaterialComponent() { 354 super(); 355 } 356 357 /** 358 * @return {@link #material} (Todo.) 359 */ 360 public CodeableConcept getMaterial() { 361 if (this.material == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.material"); 364 else if (Configuration.doAutoCreate()) 365 this.material = new CodeableConcept(); // cc 366 return this.material; 367 } 368 369 public boolean hasMaterial() { 370 return this.material != null && !this.material.isEmpty(); 371 } 372 373 /** 374 * @param value {@link #material} (Todo.) 375 */ 376 public SubstancePolymerMonomerSetStartingMaterialComponent setMaterial(CodeableConcept value) { 377 this.material = value; 378 return this; 379 } 380 381 /** 382 * @return {@link #type} (Todo.) 383 */ 384 public CodeableConcept getType() { 385 if (this.type == null) 386 if (Configuration.errorOnAutoCreate()) 387 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.type"); 388 else if (Configuration.doAutoCreate()) 389 this.type = new CodeableConcept(); // cc 390 return this.type; 391 } 392 393 public boolean hasType() { 394 return this.type != null && !this.type.isEmpty(); 395 } 396 397 /** 398 * @param value {@link #type} (Todo.) 399 */ 400 public SubstancePolymerMonomerSetStartingMaterialComponent setType(CodeableConcept value) { 401 this.type = value; 402 return this; 403 } 404 405 /** 406 * @return {@link #isDefining} (Todo.). This is the underlying object with id, 407 * value and extensions. The accessor "getIsDefining" gives direct 408 * access to the value 409 */ 410 public BooleanType getIsDefiningElement() { 411 if (this.isDefining == null) 412 if (Configuration.errorOnAutoCreate()) 413 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.isDefining"); 414 else if (Configuration.doAutoCreate()) 415 this.isDefining = new BooleanType(); // bb 416 return this.isDefining; 417 } 418 419 public boolean hasIsDefiningElement() { 420 return this.isDefining != null && !this.isDefining.isEmpty(); 421 } 422 423 public boolean hasIsDefining() { 424 return this.isDefining != null && !this.isDefining.isEmpty(); 425 } 426 427 /** 428 * @param value {@link #isDefining} (Todo.). This is the underlying object with 429 * id, value and extensions. The accessor "getIsDefining" gives 430 * direct access to the value 431 */ 432 public SubstancePolymerMonomerSetStartingMaterialComponent setIsDefiningElement(BooleanType value) { 433 this.isDefining = value; 434 return this; 435 } 436 437 /** 438 * @return Todo. 439 */ 440 public boolean getIsDefining() { 441 return this.isDefining == null || this.isDefining.isEmpty() ? false : this.isDefining.getValue(); 442 } 443 444 /** 445 * @param value Todo. 446 */ 447 public SubstancePolymerMonomerSetStartingMaterialComponent setIsDefining(boolean value) { 448 if (this.isDefining == null) 449 this.isDefining = new BooleanType(); 450 this.isDefining.setValue(value); 451 return this; 452 } 453 454 /** 455 * @return {@link #amount} (Todo.) 456 */ 457 public SubstanceAmount getAmount() { 458 if (this.amount == null) 459 if (Configuration.errorOnAutoCreate()) 460 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.amount"); 461 else if (Configuration.doAutoCreate()) 462 this.amount = new SubstanceAmount(); // cc 463 return this.amount; 464 } 465 466 public boolean hasAmount() { 467 return this.amount != null && !this.amount.isEmpty(); 468 } 469 470 /** 471 * @param value {@link #amount} (Todo.) 472 */ 473 public SubstancePolymerMonomerSetStartingMaterialComponent setAmount(SubstanceAmount value) { 474 this.amount = value; 475 return this; 476 } 477 478 protected void listChildren(List<Property> children) { 479 super.listChildren(children); 480 children.add(new Property("material", "CodeableConcept", "Todo.", 0, 1, material)); 481 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 482 children.add(new Property("isDefining", "boolean", "Todo.", 0, 1, isDefining)); 483 children.add(new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount)); 484 } 485 486 @Override 487 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 488 switch (_hash) { 489 case 299066663: 490 /* material */ return new Property("material", "CodeableConcept", "Todo.", 0, 1, material); 491 case 3575610: 492 /* type */ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 493 case -141812990: 494 /* isDefining */ return new Property("isDefining", "boolean", "Todo.", 0, 1, isDefining); 495 case -1413853096: 496 /* amount */ return new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount); 497 default: 498 return super.getNamedProperty(_hash, _name, _checkValid); 499 } 500 501 } 502 503 @Override 504 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 505 switch (hash) { 506 case 299066663: 507 /* material */ return this.material == null ? new Base[0] : new Base[] { this.material }; // CodeableConcept 508 case 3575610: 509 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 510 case -141812990: 511 /* isDefining */ return this.isDefining == null ? new Base[0] : new Base[] { this.isDefining }; // BooleanType 512 case -1413853096: 513 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // SubstanceAmount 514 default: 515 return super.getProperty(hash, name, checkValid); 516 } 517 518 } 519 520 @Override 521 public Base setProperty(int hash, String name, Base value) throws FHIRException { 522 switch (hash) { 523 case 299066663: // material 524 this.material = castToCodeableConcept(value); // CodeableConcept 525 return value; 526 case 3575610: // type 527 this.type = castToCodeableConcept(value); // CodeableConcept 528 return value; 529 case -141812990: // isDefining 530 this.isDefining = castToBoolean(value); // BooleanType 531 return value; 532 case -1413853096: // amount 533 this.amount = castToSubstanceAmount(value); // SubstanceAmount 534 return value; 535 default: 536 return super.setProperty(hash, name, value); 537 } 538 539 } 540 541 @Override 542 public Base setProperty(String name, Base value) throws FHIRException { 543 if (name.equals("material")) { 544 this.material = castToCodeableConcept(value); // CodeableConcept 545 } else if (name.equals("type")) { 546 this.type = castToCodeableConcept(value); // CodeableConcept 547 } else if (name.equals("isDefining")) { 548 this.isDefining = castToBoolean(value); // BooleanType 549 } else if (name.equals("amount")) { 550 this.amount = castToSubstanceAmount(value); // SubstanceAmount 551 } else 552 return super.setProperty(name, value); 553 return value; 554 } 555 556 @Override 557 public void removeChild(String name, Base value) throws FHIRException { 558 if (name.equals("material")) { 559 this.material = null; 560 } else if (name.equals("type")) { 561 this.type = null; 562 } else if (name.equals("isDefining")) { 563 this.isDefining = null; 564 } else if (name.equals("amount")) { 565 this.amount = null; 566 } else 567 super.removeChild(name, value); 568 569 } 570 571 @Override 572 public Base makeProperty(int hash, String name) throws FHIRException { 573 switch (hash) { 574 case 299066663: 575 return getMaterial(); 576 case 3575610: 577 return getType(); 578 case -141812990: 579 return getIsDefiningElement(); 580 case -1413853096: 581 return getAmount(); 582 default: 583 return super.makeProperty(hash, name); 584 } 585 586 } 587 588 @Override 589 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 590 switch (hash) { 591 case 299066663: 592 /* material */ return new String[] { "CodeableConcept" }; 593 case 3575610: 594 /* type */ return new String[] { "CodeableConcept" }; 595 case -141812990: 596 /* isDefining */ return new String[] { "boolean" }; 597 case -1413853096: 598 /* amount */ return new String[] { "SubstanceAmount" }; 599 default: 600 return super.getTypesForProperty(hash, name); 601 } 602 603 } 604 605 @Override 606 public Base addChild(String name) throws FHIRException { 607 if (name.equals("material")) { 608 this.material = new CodeableConcept(); 609 return this.material; 610 } else if (name.equals("type")) { 611 this.type = new CodeableConcept(); 612 return this.type; 613 } else if (name.equals("isDefining")) { 614 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.isDefining"); 615 } else if (name.equals("amount")) { 616 this.amount = new SubstanceAmount(); 617 return this.amount; 618 } else 619 return super.addChild(name); 620 } 621 622 public SubstancePolymerMonomerSetStartingMaterialComponent copy() { 623 SubstancePolymerMonomerSetStartingMaterialComponent dst = new SubstancePolymerMonomerSetStartingMaterialComponent(); 624 copyValues(dst); 625 return dst; 626 } 627 628 public void copyValues(SubstancePolymerMonomerSetStartingMaterialComponent dst) { 629 super.copyValues(dst); 630 dst.material = material == null ? null : material.copy(); 631 dst.type = type == null ? null : type.copy(); 632 dst.isDefining = isDefining == null ? null : isDefining.copy(); 633 dst.amount = amount == null ? null : amount.copy(); 634 } 635 636 @Override 637 public boolean equalsDeep(Base other_) { 638 if (!super.equalsDeep(other_)) 639 return false; 640 if (!(other_ instanceof SubstancePolymerMonomerSetStartingMaterialComponent)) 641 return false; 642 SubstancePolymerMonomerSetStartingMaterialComponent o = (SubstancePolymerMonomerSetStartingMaterialComponent) other_; 643 return compareDeep(material, o.material, true) && compareDeep(type, o.type, true) 644 && compareDeep(isDefining, o.isDefining, true) && compareDeep(amount, o.amount, true); 645 } 646 647 @Override 648 public boolean equalsShallow(Base other_) { 649 if (!super.equalsShallow(other_)) 650 return false; 651 if (!(other_ instanceof SubstancePolymerMonomerSetStartingMaterialComponent)) 652 return false; 653 SubstancePolymerMonomerSetStartingMaterialComponent o = (SubstancePolymerMonomerSetStartingMaterialComponent) other_; 654 return compareValues(isDefining, o.isDefining, true); 655 } 656 657 public boolean isEmpty() { 658 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(material, type, isDefining, amount); 659 } 660 661 public String fhirType() { 662 return "SubstancePolymer.monomerSet.startingMaterial"; 663 664 } 665 666 } 667 668 @Block() 669 public static class SubstancePolymerRepeatComponent extends BackboneElement implements IBaseBackboneElement { 670 /** 671 * Todo. 672 */ 673 @Child(name = "numberOfUnits", type = { 674 IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 675 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 676 protected IntegerType numberOfUnits; 677 678 /** 679 * Todo. 680 */ 681 @Child(name = "averageMolecularFormula", type = { 682 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 683 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 684 protected StringType averageMolecularFormula; 685 686 /** 687 * Todo. 688 */ 689 @Child(name = "repeatUnitAmountType", type = { 690 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 691 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 692 protected CodeableConcept repeatUnitAmountType; 693 694 /** 695 * Todo. 696 */ 697 @Child(name = "repeatUnit", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 698 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 699 protected List<SubstancePolymerRepeatRepeatUnitComponent> repeatUnit; 700 701 private static final long serialVersionUID = -988147059L; 702 703 /** 704 * Constructor 705 */ 706 public SubstancePolymerRepeatComponent() { 707 super(); 708 } 709 710 /** 711 * @return {@link #numberOfUnits} (Todo.). This is the underlying object with 712 * id, value and extensions. The accessor "getNumberOfUnits" gives 713 * direct access to the value 714 */ 715 public IntegerType getNumberOfUnitsElement() { 716 if (this.numberOfUnits == null) 717 if (Configuration.errorOnAutoCreate()) 718 throw new Error("Attempt to auto-create SubstancePolymerRepeatComponent.numberOfUnits"); 719 else if (Configuration.doAutoCreate()) 720 this.numberOfUnits = new IntegerType(); // bb 721 return this.numberOfUnits; 722 } 723 724 public boolean hasNumberOfUnitsElement() { 725 return this.numberOfUnits != null && !this.numberOfUnits.isEmpty(); 726 } 727 728 public boolean hasNumberOfUnits() { 729 return this.numberOfUnits != null && !this.numberOfUnits.isEmpty(); 730 } 731 732 /** 733 * @param value {@link #numberOfUnits} (Todo.). This is the underlying object 734 * with id, value and extensions. The accessor "getNumberOfUnits" 735 * gives direct access to the value 736 */ 737 public SubstancePolymerRepeatComponent setNumberOfUnitsElement(IntegerType value) { 738 this.numberOfUnits = value; 739 return this; 740 } 741 742 /** 743 * @return Todo. 744 */ 745 public int getNumberOfUnits() { 746 return this.numberOfUnits == null || this.numberOfUnits.isEmpty() ? 0 : this.numberOfUnits.getValue(); 747 } 748 749 /** 750 * @param value Todo. 751 */ 752 public SubstancePolymerRepeatComponent setNumberOfUnits(int value) { 753 if (this.numberOfUnits == null) 754 this.numberOfUnits = new IntegerType(); 755 this.numberOfUnits.setValue(value); 756 return this; 757 } 758 759 /** 760 * @return {@link #averageMolecularFormula} (Todo.). This is the underlying 761 * object with id, value and extensions. The accessor 762 * "getAverageMolecularFormula" gives direct access to the value 763 */ 764 public StringType getAverageMolecularFormulaElement() { 765 if (this.averageMolecularFormula == null) 766 if (Configuration.errorOnAutoCreate()) 767 throw new Error("Attempt to auto-create SubstancePolymerRepeatComponent.averageMolecularFormula"); 768 else if (Configuration.doAutoCreate()) 769 this.averageMolecularFormula = new StringType(); // bb 770 return this.averageMolecularFormula; 771 } 772 773 public boolean hasAverageMolecularFormulaElement() { 774 return this.averageMolecularFormula != null && !this.averageMolecularFormula.isEmpty(); 775 } 776 777 public boolean hasAverageMolecularFormula() { 778 return this.averageMolecularFormula != null && !this.averageMolecularFormula.isEmpty(); 779 } 780 781 /** 782 * @param value {@link #averageMolecularFormula} (Todo.). This is the underlying 783 * object with id, value and extensions. The accessor 784 * "getAverageMolecularFormula" gives direct access to the value 785 */ 786 public SubstancePolymerRepeatComponent setAverageMolecularFormulaElement(StringType value) { 787 this.averageMolecularFormula = value; 788 return this; 789 } 790 791 /** 792 * @return Todo. 793 */ 794 public String getAverageMolecularFormula() { 795 return this.averageMolecularFormula == null ? null : this.averageMolecularFormula.getValue(); 796 } 797 798 /** 799 * @param value Todo. 800 */ 801 public SubstancePolymerRepeatComponent setAverageMolecularFormula(String value) { 802 if (Utilities.noString(value)) 803 this.averageMolecularFormula = null; 804 else { 805 if (this.averageMolecularFormula == null) 806 this.averageMolecularFormula = new StringType(); 807 this.averageMolecularFormula.setValue(value); 808 } 809 return this; 810 } 811 812 /** 813 * @return {@link #repeatUnitAmountType} (Todo.) 814 */ 815 public CodeableConcept getRepeatUnitAmountType() { 816 if (this.repeatUnitAmountType == null) 817 if (Configuration.errorOnAutoCreate()) 818 throw new Error("Attempt to auto-create SubstancePolymerRepeatComponent.repeatUnitAmountType"); 819 else if (Configuration.doAutoCreate()) 820 this.repeatUnitAmountType = new CodeableConcept(); // cc 821 return this.repeatUnitAmountType; 822 } 823 824 public boolean hasRepeatUnitAmountType() { 825 return this.repeatUnitAmountType != null && !this.repeatUnitAmountType.isEmpty(); 826 } 827 828 /** 829 * @param value {@link #repeatUnitAmountType} (Todo.) 830 */ 831 public SubstancePolymerRepeatComponent setRepeatUnitAmountType(CodeableConcept value) { 832 this.repeatUnitAmountType = value; 833 return this; 834 } 835 836 /** 837 * @return {@link #repeatUnit} (Todo.) 838 */ 839 public List<SubstancePolymerRepeatRepeatUnitComponent> getRepeatUnit() { 840 if (this.repeatUnit == null) 841 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 842 return this.repeatUnit; 843 } 844 845 /** 846 * @return Returns a reference to <code>this</code> for easy method chaining 847 */ 848 public SubstancePolymerRepeatComponent setRepeatUnit( 849 List<SubstancePolymerRepeatRepeatUnitComponent> theRepeatUnit) { 850 this.repeatUnit = theRepeatUnit; 851 return this; 852 } 853 854 public boolean hasRepeatUnit() { 855 if (this.repeatUnit == null) 856 return false; 857 for (SubstancePolymerRepeatRepeatUnitComponent item : this.repeatUnit) 858 if (!item.isEmpty()) 859 return true; 860 return false; 861 } 862 863 public SubstancePolymerRepeatRepeatUnitComponent addRepeatUnit() { // 3 864 SubstancePolymerRepeatRepeatUnitComponent t = new SubstancePolymerRepeatRepeatUnitComponent(); 865 if (this.repeatUnit == null) 866 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 867 this.repeatUnit.add(t); 868 return t; 869 } 870 871 public SubstancePolymerRepeatComponent addRepeatUnit(SubstancePolymerRepeatRepeatUnitComponent t) { // 3 872 if (t == null) 873 return this; 874 if (this.repeatUnit == null) 875 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 876 this.repeatUnit.add(t); 877 return this; 878 } 879 880 /** 881 * @return The first repetition of repeating field {@link #repeatUnit}, creating 882 * it if it does not already exist 883 */ 884 public SubstancePolymerRepeatRepeatUnitComponent getRepeatUnitFirstRep() { 885 if (getRepeatUnit().isEmpty()) { 886 addRepeatUnit(); 887 } 888 return getRepeatUnit().get(0); 889 } 890 891 protected void listChildren(List<Property> children) { 892 super.listChildren(children); 893 children.add(new Property("numberOfUnits", "integer", "Todo.", 0, 1, numberOfUnits)); 894 children.add(new Property("averageMolecularFormula", "string", "Todo.", 0, 1, averageMolecularFormula)); 895 children.add(new Property("repeatUnitAmountType", "CodeableConcept", "Todo.", 0, 1, repeatUnitAmountType)); 896 children.add(new Property("repeatUnit", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, repeatUnit)); 897 } 898 899 @Override 900 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 901 switch (_hash) { 902 case -1321430961: 903 /* numberOfUnits */ return new Property("numberOfUnits", "integer", "Todo.", 0, 1, numberOfUnits); 904 case 111461715: 905 /* averageMolecularFormula */ return new Property("averageMolecularFormula", "string", "Todo.", 0, 1, 906 averageMolecularFormula); 907 case -1994025263: 908 /* repeatUnitAmountType */ return new Property("repeatUnitAmountType", "CodeableConcept", "Todo.", 0, 1, 909 repeatUnitAmountType); 910 case 1159607743: 911 /* repeatUnit */ return new Property("repeatUnit", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, repeatUnit); 912 default: 913 return super.getNamedProperty(_hash, _name, _checkValid); 914 } 915 916 } 917 918 @Override 919 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 920 switch (hash) { 921 case -1321430961: 922 /* numberOfUnits */ return this.numberOfUnits == null ? new Base[0] : new Base[] { this.numberOfUnits }; // IntegerType 923 case 111461715: 924 /* averageMolecularFormula */ return this.averageMolecularFormula == null ? new Base[0] 925 : new Base[] { this.averageMolecularFormula }; // StringType 926 case -1994025263: 927 /* repeatUnitAmountType */ return this.repeatUnitAmountType == null ? new Base[0] 928 : new Base[] { this.repeatUnitAmountType }; // CodeableConcept 929 case 1159607743: 930 /* repeatUnit */ return this.repeatUnit == null ? new Base[0] 931 : this.repeatUnit.toArray(new Base[this.repeatUnit.size()]); // SubstancePolymerRepeatRepeatUnitComponent 932 default: 933 return super.getProperty(hash, name, checkValid); 934 } 935 936 } 937 938 @Override 939 public Base setProperty(int hash, String name, Base value) throws FHIRException { 940 switch (hash) { 941 case -1321430961: // numberOfUnits 942 this.numberOfUnits = castToInteger(value); // IntegerType 943 return value; 944 case 111461715: // averageMolecularFormula 945 this.averageMolecularFormula = castToString(value); // StringType 946 return value; 947 case -1994025263: // repeatUnitAmountType 948 this.repeatUnitAmountType = castToCodeableConcept(value); // CodeableConcept 949 return value; 950 case 1159607743: // repeatUnit 951 this.getRepeatUnit().add((SubstancePolymerRepeatRepeatUnitComponent) value); // SubstancePolymerRepeatRepeatUnitComponent 952 return value; 953 default: 954 return super.setProperty(hash, name, value); 955 } 956 957 } 958 959 @Override 960 public Base setProperty(String name, Base value) throws FHIRException { 961 if (name.equals("numberOfUnits")) { 962 this.numberOfUnits = castToInteger(value); // IntegerType 963 } else if (name.equals("averageMolecularFormula")) { 964 this.averageMolecularFormula = castToString(value); // StringType 965 } else if (name.equals("repeatUnitAmountType")) { 966 this.repeatUnitAmountType = castToCodeableConcept(value); // CodeableConcept 967 } else if (name.equals("repeatUnit")) { 968 this.getRepeatUnit().add((SubstancePolymerRepeatRepeatUnitComponent) value); 969 } else 970 return super.setProperty(name, value); 971 return value; 972 } 973 974 @Override 975 public void removeChild(String name, Base value) throws FHIRException { 976 if (name.equals("numberOfUnits")) { 977 this.numberOfUnits = null; 978 } else if (name.equals("averageMolecularFormula")) { 979 this.averageMolecularFormula = null; 980 } else if (name.equals("repeatUnitAmountType")) { 981 this.repeatUnitAmountType = null; 982 } else if (name.equals("repeatUnit")) { 983 this.getRepeatUnit().remove((SubstancePolymerRepeatRepeatUnitComponent) value); 984 } else 985 super.removeChild(name, value); 986 987 } 988 989 @Override 990 public Base makeProperty(int hash, String name) throws FHIRException { 991 switch (hash) { 992 case -1321430961: 993 return getNumberOfUnitsElement(); 994 case 111461715: 995 return getAverageMolecularFormulaElement(); 996 case -1994025263: 997 return getRepeatUnitAmountType(); 998 case 1159607743: 999 return addRepeatUnit(); 1000 default: 1001 return super.makeProperty(hash, name); 1002 } 1003 1004 } 1005 1006 @Override 1007 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1008 switch (hash) { 1009 case -1321430961: 1010 /* numberOfUnits */ return new String[] { "integer" }; 1011 case 111461715: 1012 /* averageMolecularFormula */ return new String[] { "string" }; 1013 case -1994025263: 1014 /* repeatUnitAmountType */ return new String[] { "CodeableConcept" }; 1015 case 1159607743: 1016 /* repeatUnit */ return new String[] {}; 1017 default: 1018 return super.getTypesForProperty(hash, name); 1019 } 1020 1021 } 1022 1023 @Override 1024 public Base addChild(String name) throws FHIRException { 1025 if (name.equals("numberOfUnits")) { 1026 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.numberOfUnits"); 1027 } else if (name.equals("averageMolecularFormula")) { 1028 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.averageMolecularFormula"); 1029 } else if (name.equals("repeatUnitAmountType")) { 1030 this.repeatUnitAmountType = new CodeableConcept(); 1031 return this.repeatUnitAmountType; 1032 } else if (name.equals("repeatUnit")) { 1033 return addRepeatUnit(); 1034 } else 1035 return super.addChild(name); 1036 } 1037 1038 public SubstancePolymerRepeatComponent copy() { 1039 SubstancePolymerRepeatComponent dst = new SubstancePolymerRepeatComponent(); 1040 copyValues(dst); 1041 return dst; 1042 } 1043 1044 public void copyValues(SubstancePolymerRepeatComponent dst) { 1045 super.copyValues(dst); 1046 dst.numberOfUnits = numberOfUnits == null ? null : numberOfUnits.copy(); 1047 dst.averageMolecularFormula = averageMolecularFormula == null ? null : averageMolecularFormula.copy(); 1048 dst.repeatUnitAmountType = repeatUnitAmountType == null ? null : repeatUnitAmountType.copy(); 1049 if (repeatUnit != null) { 1050 dst.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 1051 for (SubstancePolymerRepeatRepeatUnitComponent i : repeatUnit) 1052 dst.repeatUnit.add(i.copy()); 1053 } 1054 ; 1055 } 1056 1057 @Override 1058 public boolean equalsDeep(Base other_) { 1059 if (!super.equalsDeep(other_)) 1060 return false; 1061 if (!(other_ instanceof SubstancePolymerRepeatComponent)) 1062 return false; 1063 SubstancePolymerRepeatComponent o = (SubstancePolymerRepeatComponent) other_; 1064 return compareDeep(numberOfUnits, o.numberOfUnits, true) 1065 && compareDeep(averageMolecularFormula, o.averageMolecularFormula, true) 1066 && compareDeep(repeatUnitAmountType, o.repeatUnitAmountType, true) 1067 && compareDeep(repeatUnit, o.repeatUnit, true); 1068 } 1069 1070 @Override 1071 public boolean equalsShallow(Base other_) { 1072 if (!super.equalsShallow(other_)) 1073 return false; 1074 if (!(other_ instanceof SubstancePolymerRepeatComponent)) 1075 return false; 1076 SubstancePolymerRepeatComponent o = (SubstancePolymerRepeatComponent) other_; 1077 return compareValues(numberOfUnits, o.numberOfUnits, true) 1078 && compareValues(averageMolecularFormula, o.averageMolecularFormula, true); 1079 } 1080 1081 public boolean isEmpty() { 1082 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(numberOfUnits, averageMolecularFormula, 1083 repeatUnitAmountType, repeatUnit); 1084 } 1085 1086 public String fhirType() { 1087 return "SubstancePolymer.repeat"; 1088 1089 } 1090 1091 } 1092 1093 @Block() 1094 public static class SubstancePolymerRepeatRepeatUnitComponent extends BackboneElement 1095 implements IBaseBackboneElement { 1096 /** 1097 * Todo. 1098 */ 1099 @Child(name = "orientationOfPolymerisation", type = { 1100 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1101 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1102 protected CodeableConcept orientationOfPolymerisation; 1103 1104 /** 1105 * Todo. 1106 */ 1107 @Child(name = "repeatUnit", type = { 1108 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1109 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1110 protected StringType repeatUnit; 1111 1112 /** 1113 * Todo. 1114 */ 1115 @Child(name = "amount", type = { 1116 SubstanceAmount.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1117 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1118 protected SubstanceAmount amount; 1119 1120 /** 1121 * Todo. 1122 */ 1123 @Child(name = "degreeOfPolymerisation", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1124 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1125 protected List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> degreeOfPolymerisation; 1126 1127 /** 1128 * Todo. 1129 */ 1130 @Child(name = "structuralRepresentation", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1131 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1132 protected List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> structuralRepresentation; 1133 1134 private static final long serialVersionUID = -1823741061L; 1135 1136 /** 1137 * Constructor 1138 */ 1139 public SubstancePolymerRepeatRepeatUnitComponent() { 1140 super(); 1141 } 1142 1143 /** 1144 * @return {@link #orientationOfPolymerisation} (Todo.) 1145 */ 1146 public CodeableConcept getOrientationOfPolymerisation() { 1147 if (this.orientationOfPolymerisation == null) 1148 if (Configuration.errorOnAutoCreate()) 1149 throw new Error( 1150 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.orientationOfPolymerisation"); 1151 else if (Configuration.doAutoCreate()) 1152 this.orientationOfPolymerisation = new CodeableConcept(); // cc 1153 return this.orientationOfPolymerisation; 1154 } 1155 1156 public boolean hasOrientationOfPolymerisation() { 1157 return this.orientationOfPolymerisation != null && !this.orientationOfPolymerisation.isEmpty(); 1158 } 1159 1160 /** 1161 * @param value {@link #orientationOfPolymerisation} (Todo.) 1162 */ 1163 public SubstancePolymerRepeatRepeatUnitComponent setOrientationOfPolymerisation(CodeableConcept value) { 1164 this.orientationOfPolymerisation = value; 1165 return this; 1166 } 1167 1168 /** 1169 * @return {@link #repeatUnit} (Todo.). This is the underlying object with id, 1170 * value and extensions. The accessor "getRepeatUnit" gives direct 1171 * access to the value 1172 */ 1173 public StringType getRepeatUnitElement() { 1174 if (this.repeatUnit == null) 1175 if (Configuration.errorOnAutoCreate()) 1176 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.repeatUnit"); 1177 else if (Configuration.doAutoCreate()) 1178 this.repeatUnit = new StringType(); // bb 1179 return this.repeatUnit; 1180 } 1181 1182 public boolean hasRepeatUnitElement() { 1183 return this.repeatUnit != null && !this.repeatUnit.isEmpty(); 1184 } 1185 1186 public boolean hasRepeatUnit() { 1187 return this.repeatUnit != null && !this.repeatUnit.isEmpty(); 1188 } 1189 1190 /** 1191 * @param value {@link #repeatUnit} (Todo.). This is the underlying object with 1192 * id, value and extensions. The accessor "getRepeatUnit" gives 1193 * direct access to the value 1194 */ 1195 public SubstancePolymerRepeatRepeatUnitComponent setRepeatUnitElement(StringType value) { 1196 this.repeatUnit = value; 1197 return this; 1198 } 1199 1200 /** 1201 * @return Todo. 1202 */ 1203 public String getRepeatUnit() { 1204 return this.repeatUnit == null ? null : this.repeatUnit.getValue(); 1205 } 1206 1207 /** 1208 * @param value Todo. 1209 */ 1210 public SubstancePolymerRepeatRepeatUnitComponent setRepeatUnit(String value) { 1211 if (Utilities.noString(value)) 1212 this.repeatUnit = null; 1213 else { 1214 if (this.repeatUnit == null) 1215 this.repeatUnit = new StringType(); 1216 this.repeatUnit.setValue(value); 1217 } 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #amount} (Todo.) 1223 */ 1224 public SubstanceAmount getAmount() { 1225 if (this.amount == null) 1226 if (Configuration.errorOnAutoCreate()) 1227 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.amount"); 1228 else if (Configuration.doAutoCreate()) 1229 this.amount = new SubstanceAmount(); // cc 1230 return this.amount; 1231 } 1232 1233 public boolean hasAmount() { 1234 return this.amount != null && !this.amount.isEmpty(); 1235 } 1236 1237 /** 1238 * @param value {@link #amount} (Todo.) 1239 */ 1240 public SubstancePolymerRepeatRepeatUnitComponent setAmount(SubstanceAmount value) { 1241 this.amount = value; 1242 return this; 1243 } 1244 1245 /** 1246 * @return {@link #degreeOfPolymerisation} (Todo.) 1247 */ 1248 public List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> getDegreeOfPolymerisation() { 1249 if (this.degreeOfPolymerisation == null) 1250 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1251 return this.degreeOfPolymerisation; 1252 } 1253 1254 /** 1255 * @return Returns a reference to <code>this</code> for easy method chaining 1256 */ 1257 public SubstancePolymerRepeatRepeatUnitComponent setDegreeOfPolymerisation( 1258 List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> theDegreeOfPolymerisation) { 1259 this.degreeOfPolymerisation = theDegreeOfPolymerisation; 1260 return this; 1261 } 1262 1263 public boolean hasDegreeOfPolymerisation() { 1264 if (this.degreeOfPolymerisation == null) 1265 return false; 1266 for (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent item : this.degreeOfPolymerisation) 1267 if (!item.isEmpty()) 1268 return true; 1269 return false; 1270 } 1271 1272 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent addDegreeOfPolymerisation() { // 3 1273 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent t = new SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(); 1274 if (this.degreeOfPolymerisation == null) 1275 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1276 this.degreeOfPolymerisation.add(t); 1277 return t; 1278 } 1279 1280 public SubstancePolymerRepeatRepeatUnitComponent addDegreeOfPolymerisation( 1281 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent t) { // 3 1282 if (t == null) 1283 return this; 1284 if (this.degreeOfPolymerisation == null) 1285 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1286 this.degreeOfPolymerisation.add(t); 1287 return this; 1288 } 1289 1290 /** 1291 * @return The first repetition of repeating field 1292 * {@link #degreeOfPolymerisation}, creating it if it does not already 1293 * exist 1294 */ 1295 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent getDegreeOfPolymerisationFirstRep() { 1296 if (getDegreeOfPolymerisation().isEmpty()) { 1297 addDegreeOfPolymerisation(); 1298 } 1299 return getDegreeOfPolymerisation().get(0); 1300 } 1301 1302 /** 1303 * @return {@link #structuralRepresentation} (Todo.) 1304 */ 1305 public List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> getStructuralRepresentation() { 1306 if (this.structuralRepresentation == null) 1307 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1308 return this.structuralRepresentation; 1309 } 1310 1311 /** 1312 * @return Returns a reference to <code>this</code> for easy method chaining 1313 */ 1314 public SubstancePolymerRepeatRepeatUnitComponent setStructuralRepresentation( 1315 List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> theStructuralRepresentation) { 1316 this.structuralRepresentation = theStructuralRepresentation; 1317 return this; 1318 } 1319 1320 public boolean hasStructuralRepresentation() { 1321 if (this.structuralRepresentation == null) 1322 return false; 1323 for (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent item : this.structuralRepresentation) 1324 if (!item.isEmpty()) 1325 return true; 1326 return false; 1327 } 1328 1329 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent addStructuralRepresentation() { // 3 1330 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent t = new SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(); 1331 if (this.structuralRepresentation == null) 1332 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1333 this.structuralRepresentation.add(t); 1334 return t; 1335 } 1336 1337 public SubstancePolymerRepeatRepeatUnitComponent addStructuralRepresentation( 1338 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent t) { // 3 1339 if (t == null) 1340 return this; 1341 if (this.structuralRepresentation == null) 1342 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1343 this.structuralRepresentation.add(t); 1344 return this; 1345 } 1346 1347 /** 1348 * @return The first repetition of repeating field 1349 * {@link #structuralRepresentation}, creating it if it does not already 1350 * exist 1351 */ 1352 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent getStructuralRepresentationFirstRep() { 1353 if (getStructuralRepresentation().isEmpty()) { 1354 addStructuralRepresentation(); 1355 } 1356 return getStructuralRepresentation().get(0); 1357 } 1358 1359 protected void listChildren(List<Property> children) { 1360 super.listChildren(children); 1361 children.add( 1362 new Property("orientationOfPolymerisation", "CodeableConcept", "Todo.", 0, 1, orientationOfPolymerisation)); 1363 children.add(new Property("repeatUnit", "string", "Todo.", 0, 1, repeatUnit)); 1364 children.add(new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount)); 1365 children.add( 1366 new Property("degreeOfPolymerisation", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, degreeOfPolymerisation)); 1367 children.add(new Property("structuralRepresentation", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, 1368 structuralRepresentation)); 1369 } 1370 1371 @Override 1372 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1373 switch (_hash) { 1374 case 1795817828: 1375 /* orientationOfPolymerisation */ return new Property("orientationOfPolymerisation", "CodeableConcept", "Todo.", 1376 0, 1, orientationOfPolymerisation); 1377 case 1159607743: 1378 /* repeatUnit */ return new Property("repeatUnit", "string", "Todo.", 0, 1, repeatUnit); 1379 case -1413853096: 1380 /* amount */ return new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount); 1381 case -159251872: 1382 /* degreeOfPolymerisation */ return new Property("degreeOfPolymerisation", "", "Todo.", 0, 1383 java.lang.Integer.MAX_VALUE, degreeOfPolymerisation); 1384 case 14311178: 1385 /* structuralRepresentation */ return new Property("structuralRepresentation", "", "Todo.", 0, 1386 java.lang.Integer.MAX_VALUE, structuralRepresentation); 1387 default: 1388 return super.getNamedProperty(_hash, _name, _checkValid); 1389 } 1390 1391 } 1392 1393 @Override 1394 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1395 switch (hash) { 1396 case 1795817828: 1397 /* orientationOfPolymerisation */ return this.orientationOfPolymerisation == null ? new Base[0] 1398 : new Base[] { this.orientationOfPolymerisation }; // CodeableConcept 1399 case 1159607743: 1400 /* repeatUnit */ return this.repeatUnit == null ? new Base[0] : new Base[] { this.repeatUnit }; // StringType 1401 case -1413853096: 1402 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // SubstanceAmount 1403 case -159251872: 1404 /* degreeOfPolymerisation */ return this.degreeOfPolymerisation == null ? new Base[0] 1405 : this.degreeOfPolymerisation.toArray(new Base[this.degreeOfPolymerisation.size()]); // SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent 1406 case 14311178: 1407 /* structuralRepresentation */ return this.structuralRepresentation == null ? new Base[0] 1408 : this.structuralRepresentation.toArray(new Base[this.structuralRepresentation.size()]); // SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent 1409 default: 1410 return super.getProperty(hash, name, checkValid); 1411 } 1412 1413 } 1414 1415 @Override 1416 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1417 switch (hash) { 1418 case 1795817828: // orientationOfPolymerisation 1419 this.orientationOfPolymerisation = castToCodeableConcept(value); // CodeableConcept 1420 return value; 1421 case 1159607743: // repeatUnit 1422 this.repeatUnit = castToString(value); // StringType 1423 return value; 1424 case -1413853096: // amount 1425 this.amount = castToSubstanceAmount(value); // SubstanceAmount 1426 return value; 1427 case -159251872: // degreeOfPolymerisation 1428 this.getDegreeOfPolymerisation().add((SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) value); // SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent 1429 return value; 1430 case 14311178: // structuralRepresentation 1431 this.getStructuralRepresentation() 1432 .add((SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) value); // SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent 1433 return value; 1434 default: 1435 return super.setProperty(hash, name, value); 1436 } 1437 1438 } 1439 1440 @Override 1441 public Base setProperty(String name, Base value) throws FHIRException { 1442 if (name.equals("orientationOfPolymerisation")) { 1443 this.orientationOfPolymerisation = castToCodeableConcept(value); // CodeableConcept 1444 } else if (name.equals("repeatUnit")) { 1445 this.repeatUnit = castToString(value); // StringType 1446 } else if (name.equals("amount")) { 1447 this.amount = castToSubstanceAmount(value); // SubstanceAmount 1448 } else if (name.equals("degreeOfPolymerisation")) { 1449 this.getDegreeOfPolymerisation().add((SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) value); 1450 } else if (name.equals("structuralRepresentation")) { 1451 this.getStructuralRepresentation() 1452 .add((SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) value); 1453 } else 1454 return super.setProperty(name, value); 1455 return value; 1456 } 1457 1458 @Override 1459 public void removeChild(String name, Base value) throws FHIRException { 1460 if (name.equals("orientationOfPolymerisation")) { 1461 this.orientationOfPolymerisation = null; 1462 } else if (name.equals("repeatUnit")) { 1463 this.repeatUnit = null; 1464 } else if (name.equals("amount")) { 1465 this.amount = null; 1466 } else if (name.equals("degreeOfPolymerisation")) { 1467 this.getDegreeOfPolymerisation().remove((SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) value); 1468 } else if (name.equals("structuralRepresentation")) { 1469 this.getStructuralRepresentation() 1470 .remove((SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) value); 1471 } else 1472 super.removeChild(name, value); 1473 1474 } 1475 1476 @Override 1477 public Base makeProperty(int hash, String name) throws FHIRException { 1478 switch (hash) { 1479 case 1795817828: 1480 return getOrientationOfPolymerisation(); 1481 case 1159607743: 1482 return getRepeatUnitElement(); 1483 case -1413853096: 1484 return getAmount(); 1485 case -159251872: 1486 return addDegreeOfPolymerisation(); 1487 case 14311178: 1488 return addStructuralRepresentation(); 1489 default: 1490 return super.makeProperty(hash, name); 1491 } 1492 1493 } 1494 1495 @Override 1496 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1497 switch (hash) { 1498 case 1795817828: 1499 /* orientationOfPolymerisation */ return new String[] { "CodeableConcept" }; 1500 case 1159607743: 1501 /* repeatUnit */ return new String[] { "string" }; 1502 case -1413853096: 1503 /* amount */ return new String[] { "SubstanceAmount" }; 1504 case -159251872: 1505 /* degreeOfPolymerisation */ return new String[] {}; 1506 case 14311178: 1507 /* structuralRepresentation */ return new String[] {}; 1508 default: 1509 return super.getTypesForProperty(hash, name); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base addChild(String name) throws FHIRException { 1516 if (name.equals("orientationOfPolymerisation")) { 1517 this.orientationOfPolymerisation = new CodeableConcept(); 1518 return this.orientationOfPolymerisation; 1519 } else if (name.equals("repeatUnit")) { 1520 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeatUnit"); 1521 } else if (name.equals("amount")) { 1522 this.amount = new SubstanceAmount(); 1523 return this.amount; 1524 } else if (name.equals("degreeOfPolymerisation")) { 1525 return addDegreeOfPolymerisation(); 1526 } else if (name.equals("structuralRepresentation")) { 1527 return addStructuralRepresentation(); 1528 } else 1529 return super.addChild(name); 1530 } 1531 1532 public SubstancePolymerRepeatRepeatUnitComponent copy() { 1533 SubstancePolymerRepeatRepeatUnitComponent dst = new SubstancePolymerRepeatRepeatUnitComponent(); 1534 copyValues(dst); 1535 return dst; 1536 } 1537 1538 public void copyValues(SubstancePolymerRepeatRepeatUnitComponent dst) { 1539 super.copyValues(dst); 1540 dst.orientationOfPolymerisation = orientationOfPolymerisation == null ? null : orientationOfPolymerisation.copy(); 1541 dst.repeatUnit = repeatUnit == null ? null : repeatUnit.copy(); 1542 dst.amount = amount == null ? null : amount.copy(); 1543 if (degreeOfPolymerisation != null) { 1544 dst.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1545 for (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent i : degreeOfPolymerisation) 1546 dst.degreeOfPolymerisation.add(i.copy()); 1547 } 1548 ; 1549 if (structuralRepresentation != null) { 1550 dst.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1551 for (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent i : structuralRepresentation) 1552 dst.structuralRepresentation.add(i.copy()); 1553 } 1554 ; 1555 } 1556 1557 @Override 1558 public boolean equalsDeep(Base other_) { 1559 if (!super.equalsDeep(other_)) 1560 return false; 1561 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitComponent)) 1562 return false; 1563 SubstancePolymerRepeatRepeatUnitComponent o = (SubstancePolymerRepeatRepeatUnitComponent) other_; 1564 return compareDeep(orientationOfPolymerisation, o.orientationOfPolymerisation, true) 1565 && compareDeep(repeatUnit, o.repeatUnit, true) && compareDeep(amount, o.amount, true) 1566 && compareDeep(degreeOfPolymerisation, o.degreeOfPolymerisation, true) 1567 && compareDeep(structuralRepresentation, o.structuralRepresentation, true); 1568 } 1569 1570 @Override 1571 public boolean equalsShallow(Base other_) { 1572 if (!super.equalsShallow(other_)) 1573 return false; 1574 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitComponent)) 1575 return false; 1576 SubstancePolymerRepeatRepeatUnitComponent o = (SubstancePolymerRepeatRepeatUnitComponent) other_; 1577 return compareValues(repeatUnit, o.repeatUnit, true); 1578 } 1579 1580 public boolean isEmpty() { 1581 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(orientationOfPolymerisation, repeatUnit, amount, 1582 degreeOfPolymerisation, structuralRepresentation); 1583 } 1584 1585 public String fhirType() { 1586 return "SubstancePolymer.repeat.repeatUnit"; 1587 1588 } 1589 1590 } 1591 1592 @Block() 1593 public static class SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent extends BackboneElement 1594 implements IBaseBackboneElement { 1595 /** 1596 * Todo. 1597 */ 1598 @Child(name = "degree", type = { 1599 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1600 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1601 protected CodeableConcept degree; 1602 1603 /** 1604 * Todo. 1605 */ 1606 @Child(name = "amount", type = { 1607 SubstanceAmount.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1608 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1609 protected SubstanceAmount amount; 1610 1611 private static final long serialVersionUID = -1487452773L; 1612 1613 /** 1614 * Constructor 1615 */ 1616 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent() { 1617 super(); 1618 } 1619 1620 /** 1621 * @return {@link #degree} (Todo.) 1622 */ 1623 public CodeableConcept getDegree() { 1624 if (this.degree == null) 1625 if (Configuration.errorOnAutoCreate()) 1626 throw new Error( 1627 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent.degree"); 1628 else if (Configuration.doAutoCreate()) 1629 this.degree = new CodeableConcept(); // cc 1630 return this.degree; 1631 } 1632 1633 public boolean hasDegree() { 1634 return this.degree != null && !this.degree.isEmpty(); 1635 } 1636 1637 /** 1638 * @param value {@link #degree} (Todo.) 1639 */ 1640 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setDegree(CodeableConcept value) { 1641 this.degree = value; 1642 return this; 1643 } 1644 1645 /** 1646 * @return {@link #amount} (Todo.) 1647 */ 1648 public SubstanceAmount getAmount() { 1649 if (this.amount == null) 1650 if (Configuration.errorOnAutoCreate()) 1651 throw new Error( 1652 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent.amount"); 1653 else if (Configuration.doAutoCreate()) 1654 this.amount = new SubstanceAmount(); // cc 1655 return this.amount; 1656 } 1657 1658 public boolean hasAmount() { 1659 return this.amount != null && !this.amount.isEmpty(); 1660 } 1661 1662 /** 1663 * @param value {@link #amount} (Todo.) 1664 */ 1665 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setAmount(SubstanceAmount value) { 1666 this.amount = value; 1667 return this; 1668 } 1669 1670 protected void listChildren(List<Property> children) { 1671 super.listChildren(children); 1672 children.add(new Property("degree", "CodeableConcept", "Todo.", 0, 1, degree)); 1673 children.add(new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount)); 1674 } 1675 1676 @Override 1677 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1678 switch (_hash) { 1679 case -1335595316: 1680 /* degree */ return new Property("degree", "CodeableConcept", "Todo.", 0, 1, degree); 1681 case -1413853096: 1682 /* amount */ return new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount); 1683 default: 1684 return super.getNamedProperty(_hash, _name, _checkValid); 1685 } 1686 1687 } 1688 1689 @Override 1690 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1691 switch (hash) { 1692 case -1335595316: 1693 /* degree */ return this.degree == null ? new Base[0] : new Base[] { this.degree }; // CodeableConcept 1694 case -1413853096: 1695 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // SubstanceAmount 1696 default: 1697 return super.getProperty(hash, name, checkValid); 1698 } 1699 1700 } 1701 1702 @Override 1703 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1704 switch (hash) { 1705 case -1335595316: // degree 1706 this.degree = castToCodeableConcept(value); // CodeableConcept 1707 return value; 1708 case -1413853096: // amount 1709 this.amount = castToSubstanceAmount(value); // SubstanceAmount 1710 return value; 1711 default: 1712 return super.setProperty(hash, name, value); 1713 } 1714 1715 } 1716 1717 @Override 1718 public Base setProperty(String name, Base value) throws FHIRException { 1719 if (name.equals("degree")) { 1720 this.degree = castToCodeableConcept(value); // CodeableConcept 1721 } else if (name.equals("amount")) { 1722 this.amount = castToSubstanceAmount(value); // SubstanceAmount 1723 } else 1724 return super.setProperty(name, value); 1725 return value; 1726 } 1727 1728 @Override 1729 public void removeChild(String name, Base value) throws FHIRException { 1730 if (name.equals("degree")) { 1731 this.degree = null; 1732 } else if (name.equals("amount")) { 1733 this.amount = null; 1734 } else 1735 super.removeChild(name, value); 1736 1737 } 1738 1739 @Override 1740 public Base makeProperty(int hash, String name) throws FHIRException { 1741 switch (hash) { 1742 case -1335595316: 1743 return getDegree(); 1744 case -1413853096: 1745 return getAmount(); 1746 default: 1747 return super.makeProperty(hash, name); 1748 } 1749 1750 } 1751 1752 @Override 1753 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1754 switch (hash) { 1755 case -1335595316: 1756 /* degree */ return new String[] { "CodeableConcept" }; 1757 case -1413853096: 1758 /* amount */ return new String[] { "SubstanceAmount" }; 1759 default: 1760 return super.getTypesForProperty(hash, name); 1761 } 1762 1763 } 1764 1765 @Override 1766 public Base addChild(String name) throws FHIRException { 1767 if (name.equals("degree")) { 1768 this.degree = new CodeableConcept(); 1769 return this.degree; 1770 } else if (name.equals("amount")) { 1771 this.amount = new SubstanceAmount(); 1772 return this.amount; 1773 } else 1774 return super.addChild(name); 1775 } 1776 1777 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent copy() { 1778 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent dst = new SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(); 1779 copyValues(dst); 1780 return dst; 1781 } 1782 1783 public void copyValues(SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent dst) { 1784 super.copyValues(dst); 1785 dst.degree = degree == null ? null : degree.copy(); 1786 dst.amount = amount == null ? null : amount.copy(); 1787 } 1788 1789 @Override 1790 public boolean equalsDeep(Base other_) { 1791 if (!super.equalsDeep(other_)) 1792 return false; 1793 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent)) 1794 return false; 1795 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent o = (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) other_; 1796 return compareDeep(degree, o.degree, true) && compareDeep(amount, o.amount, true); 1797 } 1798 1799 @Override 1800 public boolean equalsShallow(Base other_) { 1801 if (!super.equalsShallow(other_)) 1802 return false; 1803 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent)) 1804 return false; 1805 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent o = (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) other_; 1806 return true; 1807 } 1808 1809 public boolean isEmpty() { 1810 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(degree, amount); 1811 } 1812 1813 public String fhirType() { 1814 return "SubstancePolymer.repeat.repeatUnit.degreeOfPolymerisation"; 1815 1816 } 1817 1818 } 1819 1820 @Block() 1821 public static class SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent extends BackboneElement 1822 implements IBaseBackboneElement { 1823 /** 1824 * Todo. 1825 */ 1826 @Child(name = "type", type = { 1827 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1828 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1829 protected CodeableConcept type; 1830 1831 /** 1832 * Todo. 1833 */ 1834 @Child(name = "representation", type = { 1835 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1836 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1837 protected StringType representation; 1838 1839 /** 1840 * Todo. 1841 */ 1842 @Child(name = "attachment", type = { 1843 Attachment.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1844 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1845 protected Attachment attachment; 1846 1847 private static final long serialVersionUID = 167954495L; 1848 1849 /** 1850 * Constructor 1851 */ 1852 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent() { 1853 super(); 1854 } 1855 1856 /** 1857 * @return {@link #type} (Todo.) 1858 */ 1859 public CodeableConcept getType() { 1860 if (this.type == null) 1861 if (Configuration.errorOnAutoCreate()) 1862 throw new Error( 1863 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.type"); 1864 else if (Configuration.doAutoCreate()) 1865 this.type = new CodeableConcept(); // cc 1866 return this.type; 1867 } 1868 1869 public boolean hasType() { 1870 return this.type != null && !this.type.isEmpty(); 1871 } 1872 1873 /** 1874 * @param value {@link #type} (Todo.) 1875 */ 1876 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setType(CodeableConcept value) { 1877 this.type = value; 1878 return this; 1879 } 1880 1881 /** 1882 * @return {@link #representation} (Todo.). This is the underlying object with 1883 * id, value and extensions. The accessor "getRepresentation" gives 1884 * direct access to the value 1885 */ 1886 public StringType getRepresentationElement() { 1887 if (this.representation == null) 1888 if (Configuration.errorOnAutoCreate()) 1889 throw new Error( 1890 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.representation"); 1891 else if (Configuration.doAutoCreate()) 1892 this.representation = new StringType(); // bb 1893 return this.representation; 1894 } 1895 1896 public boolean hasRepresentationElement() { 1897 return this.representation != null && !this.representation.isEmpty(); 1898 } 1899 1900 public boolean hasRepresentation() { 1901 return this.representation != null && !this.representation.isEmpty(); 1902 } 1903 1904 /** 1905 * @param value {@link #representation} (Todo.). This is the underlying object 1906 * with id, value and extensions. The accessor "getRepresentation" 1907 * gives direct access to the value 1908 */ 1909 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setRepresentationElement( 1910 StringType value) { 1911 this.representation = value; 1912 return this; 1913 } 1914 1915 /** 1916 * @return Todo. 1917 */ 1918 public String getRepresentation() { 1919 return this.representation == null ? null : this.representation.getValue(); 1920 } 1921 1922 /** 1923 * @param value Todo. 1924 */ 1925 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setRepresentation(String value) { 1926 if (Utilities.noString(value)) 1927 this.representation = null; 1928 else { 1929 if (this.representation == null) 1930 this.representation = new StringType(); 1931 this.representation.setValue(value); 1932 } 1933 return this; 1934 } 1935 1936 /** 1937 * @return {@link #attachment} (Todo.) 1938 */ 1939 public Attachment getAttachment() { 1940 if (this.attachment == null) 1941 if (Configuration.errorOnAutoCreate()) 1942 throw new Error( 1943 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.attachment"); 1944 else if (Configuration.doAutoCreate()) 1945 this.attachment = new Attachment(); // cc 1946 return this.attachment; 1947 } 1948 1949 public boolean hasAttachment() { 1950 return this.attachment != null && !this.attachment.isEmpty(); 1951 } 1952 1953 /** 1954 * @param value {@link #attachment} (Todo.) 1955 */ 1956 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setAttachment(Attachment value) { 1957 this.attachment = value; 1958 return this; 1959 } 1960 1961 protected void listChildren(List<Property> children) { 1962 super.listChildren(children); 1963 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 1964 children.add(new Property("representation", "string", "Todo.", 0, 1, representation)); 1965 children.add(new Property("attachment", "Attachment", "Todo.", 0, 1, attachment)); 1966 } 1967 1968 @Override 1969 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1970 switch (_hash) { 1971 case 3575610: 1972 /* type */ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 1973 case -671065907: 1974 /* representation */ return new Property("representation", "string", "Todo.", 0, 1, representation); 1975 case -1963501277: 1976 /* attachment */ return new Property("attachment", "Attachment", "Todo.", 0, 1, attachment); 1977 default: 1978 return super.getNamedProperty(_hash, _name, _checkValid); 1979 } 1980 1981 } 1982 1983 @Override 1984 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1985 switch (hash) { 1986 case 3575610: 1987 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1988 case -671065907: 1989 /* representation */ return this.representation == null ? new Base[0] : new Base[] { this.representation }; // StringType 1990 case -1963501277: 1991 /* attachment */ return this.attachment == null ? new Base[0] : new Base[] { this.attachment }; // Attachment 1992 default: 1993 return super.getProperty(hash, name, checkValid); 1994 } 1995 1996 } 1997 1998 @Override 1999 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2000 switch (hash) { 2001 case 3575610: // type 2002 this.type = castToCodeableConcept(value); // CodeableConcept 2003 return value; 2004 case -671065907: // representation 2005 this.representation = castToString(value); // StringType 2006 return value; 2007 case -1963501277: // attachment 2008 this.attachment = castToAttachment(value); // Attachment 2009 return value; 2010 default: 2011 return super.setProperty(hash, name, value); 2012 } 2013 2014 } 2015 2016 @Override 2017 public Base setProperty(String name, Base value) throws FHIRException { 2018 if (name.equals("type")) { 2019 this.type = castToCodeableConcept(value); // CodeableConcept 2020 } else if (name.equals("representation")) { 2021 this.representation = castToString(value); // StringType 2022 } else if (name.equals("attachment")) { 2023 this.attachment = castToAttachment(value); // Attachment 2024 } else 2025 return super.setProperty(name, value); 2026 return value; 2027 } 2028 2029 @Override 2030 public void removeChild(String name, Base value) throws FHIRException { 2031 if (name.equals("type")) { 2032 this.type = null; 2033 } else if (name.equals("representation")) { 2034 this.representation = null; 2035 } else if (name.equals("attachment")) { 2036 this.attachment = null; 2037 } else 2038 super.removeChild(name, value); 2039 2040 } 2041 2042 @Override 2043 public Base makeProperty(int hash, String name) throws FHIRException { 2044 switch (hash) { 2045 case 3575610: 2046 return getType(); 2047 case -671065907: 2048 return getRepresentationElement(); 2049 case -1963501277: 2050 return getAttachment(); 2051 default: 2052 return super.makeProperty(hash, name); 2053 } 2054 2055 } 2056 2057 @Override 2058 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2059 switch (hash) { 2060 case 3575610: 2061 /* type */ return new String[] { "CodeableConcept" }; 2062 case -671065907: 2063 /* representation */ return new String[] { "string" }; 2064 case -1963501277: 2065 /* attachment */ return new String[] { "Attachment" }; 2066 default: 2067 return super.getTypesForProperty(hash, name); 2068 } 2069 2070 } 2071 2072 @Override 2073 public Base addChild(String name) throws FHIRException { 2074 if (name.equals("type")) { 2075 this.type = new CodeableConcept(); 2076 return this.type; 2077 } else if (name.equals("representation")) { 2078 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.representation"); 2079 } else if (name.equals("attachment")) { 2080 this.attachment = new Attachment(); 2081 return this.attachment; 2082 } else 2083 return super.addChild(name); 2084 } 2085 2086 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent copy() { 2087 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent dst = new SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(); 2088 copyValues(dst); 2089 return dst; 2090 } 2091 2092 public void copyValues(SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent dst) { 2093 super.copyValues(dst); 2094 dst.type = type == null ? null : type.copy(); 2095 dst.representation = representation == null ? null : representation.copy(); 2096 dst.attachment = attachment == null ? null : attachment.copy(); 2097 } 2098 2099 @Override 2100 public boolean equalsDeep(Base other_) { 2101 if (!super.equalsDeep(other_)) 2102 return false; 2103 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent)) 2104 return false; 2105 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent o = (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) other_; 2106 return compareDeep(type, o.type, true) && compareDeep(representation, o.representation, true) 2107 && compareDeep(attachment, o.attachment, true); 2108 } 2109 2110 @Override 2111 public boolean equalsShallow(Base other_) { 2112 if (!super.equalsShallow(other_)) 2113 return false; 2114 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent)) 2115 return false; 2116 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent o = (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) other_; 2117 return compareValues(representation, o.representation, true); 2118 } 2119 2120 public boolean isEmpty() { 2121 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, representation, attachment); 2122 } 2123 2124 public String fhirType() { 2125 return "SubstancePolymer.repeat.repeatUnit.structuralRepresentation"; 2126 2127 } 2128 2129 } 2130 2131 /** 2132 * Todo. 2133 */ 2134 @Child(name = "class", type = { 2135 CodeableConcept.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 2136 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2137 protected CodeableConcept class_; 2138 2139 /** 2140 * Todo. 2141 */ 2142 @Child(name = "geometry", type = { 2143 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2144 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2145 protected CodeableConcept geometry; 2146 2147 /** 2148 * Todo. 2149 */ 2150 @Child(name = "copolymerConnectivity", type = { 2151 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2152 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2153 protected List<CodeableConcept> copolymerConnectivity; 2154 2155 /** 2156 * Todo. 2157 */ 2158 @Child(name = "modification", type = { 2159 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2160 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2161 protected List<StringType> modification; 2162 2163 /** 2164 * Todo. 2165 */ 2166 @Child(name = "monomerSet", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2167 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2168 protected List<SubstancePolymerMonomerSetComponent> monomerSet; 2169 2170 /** 2171 * Todo. 2172 */ 2173 @Child(name = "repeat", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2174 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2175 protected List<SubstancePolymerRepeatComponent> repeat; 2176 2177 private static final long serialVersionUID = -58301650L; 2178 2179 /** 2180 * Constructor 2181 */ 2182 public SubstancePolymer() { 2183 super(); 2184 } 2185 2186 /** 2187 * @return {@link #class_} (Todo.) 2188 */ 2189 public CodeableConcept getClass_() { 2190 if (this.class_ == null) 2191 if (Configuration.errorOnAutoCreate()) 2192 throw new Error("Attempt to auto-create SubstancePolymer.class_"); 2193 else if (Configuration.doAutoCreate()) 2194 this.class_ = new CodeableConcept(); // cc 2195 return this.class_; 2196 } 2197 2198 public boolean hasClass_() { 2199 return this.class_ != null && !this.class_.isEmpty(); 2200 } 2201 2202 /** 2203 * @param value {@link #class_} (Todo.) 2204 */ 2205 public SubstancePolymer setClass_(CodeableConcept value) { 2206 this.class_ = value; 2207 return this; 2208 } 2209 2210 /** 2211 * @return {@link #geometry} (Todo.) 2212 */ 2213 public CodeableConcept getGeometry() { 2214 if (this.geometry == null) 2215 if (Configuration.errorOnAutoCreate()) 2216 throw new Error("Attempt to auto-create SubstancePolymer.geometry"); 2217 else if (Configuration.doAutoCreate()) 2218 this.geometry = new CodeableConcept(); // cc 2219 return this.geometry; 2220 } 2221 2222 public boolean hasGeometry() { 2223 return this.geometry != null && !this.geometry.isEmpty(); 2224 } 2225 2226 /** 2227 * @param value {@link #geometry} (Todo.) 2228 */ 2229 public SubstancePolymer setGeometry(CodeableConcept value) { 2230 this.geometry = value; 2231 return this; 2232 } 2233 2234 /** 2235 * @return {@link #copolymerConnectivity} (Todo.) 2236 */ 2237 public List<CodeableConcept> getCopolymerConnectivity() { 2238 if (this.copolymerConnectivity == null) 2239 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2240 return this.copolymerConnectivity; 2241 } 2242 2243 /** 2244 * @return Returns a reference to <code>this</code> for easy method chaining 2245 */ 2246 public SubstancePolymer setCopolymerConnectivity(List<CodeableConcept> theCopolymerConnectivity) { 2247 this.copolymerConnectivity = theCopolymerConnectivity; 2248 return this; 2249 } 2250 2251 public boolean hasCopolymerConnectivity() { 2252 if (this.copolymerConnectivity == null) 2253 return false; 2254 for (CodeableConcept item : this.copolymerConnectivity) 2255 if (!item.isEmpty()) 2256 return true; 2257 return false; 2258 } 2259 2260 public CodeableConcept addCopolymerConnectivity() { // 3 2261 CodeableConcept t = new CodeableConcept(); 2262 if (this.copolymerConnectivity == null) 2263 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2264 this.copolymerConnectivity.add(t); 2265 return t; 2266 } 2267 2268 public SubstancePolymer addCopolymerConnectivity(CodeableConcept t) { // 3 2269 if (t == null) 2270 return this; 2271 if (this.copolymerConnectivity == null) 2272 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2273 this.copolymerConnectivity.add(t); 2274 return this; 2275 } 2276 2277 /** 2278 * @return The first repetition of repeating field 2279 * {@link #copolymerConnectivity}, creating it if it does not already 2280 * exist 2281 */ 2282 public CodeableConcept getCopolymerConnectivityFirstRep() { 2283 if (getCopolymerConnectivity().isEmpty()) { 2284 addCopolymerConnectivity(); 2285 } 2286 return getCopolymerConnectivity().get(0); 2287 } 2288 2289 /** 2290 * @return {@link #modification} (Todo.) 2291 */ 2292 public List<StringType> getModification() { 2293 if (this.modification == null) 2294 this.modification = new ArrayList<StringType>(); 2295 return this.modification; 2296 } 2297 2298 /** 2299 * @return Returns a reference to <code>this</code> for easy method chaining 2300 */ 2301 public SubstancePolymer setModification(List<StringType> theModification) { 2302 this.modification = theModification; 2303 return this; 2304 } 2305 2306 public boolean hasModification() { 2307 if (this.modification == null) 2308 return false; 2309 for (StringType item : this.modification) 2310 if (!item.isEmpty()) 2311 return true; 2312 return false; 2313 } 2314 2315 /** 2316 * @return {@link #modification} (Todo.) 2317 */ 2318 public StringType addModificationElement() {// 2 2319 StringType t = new StringType(); 2320 if (this.modification == null) 2321 this.modification = new ArrayList<StringType>(); 2322 this.modification.add(t); 2323 return t; 2324 } 2325 2326 /** 2327 * @param value {@link #modification} (Todo.) 2328 */ 2329 public SubstancePolymer addModification(String value) { // 1 2330 StringType t = new StringType(); 2331 t.setValue(value); 2332 if (this.modification == null) 2333 this.modification = new ArrayList<StringType>(); 2334 this.modification.add(t); 2335 return this; 2336 } 2337 2338 /** 2339 * @param value {@link #modification} (Todo.) 2340 */ 2341 public boolean hasModification(String value) { 2342 if (this.modification == null) 2343 return false; 2344 for (StringType v : this.modification) 2345 if (v.getValue().equals(value)) // string 2346 return true; 2347 return false; 2348 } 2349 2350 /** 2351 * @return {@link #monomerSet} (Todo.) 2352 */ 2353 public List<SubstancePolymerMonomerSetComponent> getMonomerSet() { 2354 if (this.monomerSet == null) 2355 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2356 return this.monomerSet; 2357 } 2358 2359 /** 2360 * @return Returns a reference to <code>this</code> for easy method chaining 2361 */ 2362 public SubstancePolymer setMonomerSet(List<SubstancePolymerMonomerSetComponent> theMonomerSet) { 2363 this.monomerSet = theMonomerSet; 2364 return this; 2365 } 2366 2367 public boolean hasMonomerSet() { 2368 if (this.monomerSet == null) 2369 return false; 2370 for (SubstancePolymerMonomerSetComponent item : this.monomerSet) 2371 if (!item.isEmpty()) 2372 return true; 2373 return false; 2374 } 2375 2376 public SubstancePolymerMonomerSetComponent addMonomerSet() { // 3 2377 SubstancePolymerMonomerSetComponent t = new SubstancePolymerMonomerSetComponent(); 2378 if (this.monomerSet == null) 2379 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2380 this.monomerSet.add(t); 2381 return t; 2382 } 2383 2384 public SubstancePolymer addMonomerSet(SubstancePolymerMonomerSetComponent t) { // 3 2385 if (t == null) 2386 return this; 2387 if (this.monomerSet == null) 2388 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2389 this.monomerSet.add(t); 2390 return this; 2391 } 2392 2393 /** 2394 * @return The first repetition of repeating field {@link #monomerSet}, creating 2395 * it if it does not already exist 2396 */ 2397 public SubstancePolymerMonomerSetComponent getMonomerSetFirstRep() { 2398 if (getMonomerSet().isEmpty()) { 2399 addMonomerSet(); 2400 } 2401 return getMonomerSet().get(0); 2402 } 2403 2404 /** 2405 * @return {@link #repeat} (Todo.) 2406 */ 2407 public List<SubstancePolymerRepeatComponent> getRepeat() { 2408 if (this.repeat == null) 2409 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2410 return this.repeat; 2411 } 2412 2413 /** 2414 * @return Returns a reference to <code>this</code> for easy method chaining 2415 */ 2416 public SubstancePolymer setRepeat(List<SubstancePolymerRepeatComponent> theRepeat) { 2417 this.repeat = theRepeat; 2418 return this; 2419 } 2420 2421 public boolean hasRepeat() { 2422 if (this.repeat == null) 2423 return false; 2424 for (SubstancePolymerRepeatComponent item : this.repeat) 2425 if (!item.isEmpty()) 2426 return true; 2427 return false; 2428 } 2429 2430 public SubstancePolymerRepeatComponent addRepeat() { // 3 2431 SubstancePolymerRepeatComponent t = new SubstancePolymerRepeatComponent(); 2432 if (this.repeat == null) 2433 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2434 this.repeat.add(t); 2435 return t; 2436 } 2437 2438 public SubstancePolymer addRepeat(SubstancePolymerRepeatComponent t) { // 3 2439 if (t == null) 2440 return this; 2441 if (this.repeat == null) 2442 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2443 this.repeat.add(t); 2444 return this; 2445 } 2446 2447 /** 2448 * @return The first repetition of repeating field {@link #repeat}, creating it 2449 * if it does not already exist 2450 */ 2451 public SubstancePolymerRepeatComponent getRepeatFirstRep() { 2452 if (getRepeat().isEmpty()) { 2453 addRepeat(); 2454 } 2455 return getRepeat().get(0); 2456 } 2457 2458 protected void listChildren(List<Property> children) { 2459 super.listChildren(children); 2460 children.add(new Property("class", "CodeableConcept", "Todo.", 0, 1, class_)); 2461 children.add(new Property("geometry", "CodeableConcept", "Todo.", 0, 1, geometry)); 2462 children.add(new Property("copolymerConnectivity", "CodeableConcept", "Todo.", 0, java.lang.Integer.MAX_VALUE, 2463 copolymerConnectivity)); 2464 children.add(new Property("modification", "string", "Todo.", 0, java.lang.Integer.MAX_VALUE, modification)); 2465 children.add(new Property("monomerSet", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, monomerSet)); 2466 children.add(new Property("repeat", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, repeat)); 2467 } 2468 2469 @Override 2470 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2471 switch (_hash) { 2472 case 94742904: 2473 /* class */ return new Property("class", "CodeableConcept", "Todo.", 0, 1, class_); 2474 case 1846020210: 2475 /* geometry */ return new Property("geometry", "CodeableConcept", "Todo.", 0, 1, geometry); 2476 case 997107577: 2477 /* copolymerConnectivity */ return new Property("copolymerConnectivity", "CodeableConcept", "Todo.", 0, 2478 java.lang.Integer.MAX_VALUE, copolymerConnectivity); 2479 case -684600932: 2480 /* modification */ return new Property("modification", "string", "Todo.", 0, java.lang.Integer.MAX_VALUE, 2481 modification); 2482 case -1622483765: 2483 /* monomerSet */ return new Property("monomerSet", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, monomerSet); 2484 case -934531685: 2485 /* repeat */ return new Property("repeat", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, repeat); 2486 default: 2487 return super.getNamedProperty(_hash, _name, _checkValid); 2488 } 2489 2490 } 2491 2492 @Override 2493 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2494 switch (hash) { 2495 case 94742904: 2496 /* class */ return this.class_ == null ? new Base[0] : new Base[] { this.class_ }; // CodeableConcept 2497 case 1846020210: 2498 /* geometry */ return this.geometry == null ? new Base[0] : new Base[] { this.geometry }; // CodeableConcept 2499 case 997107577: 2500 /* copolymerConnectivity */ return this.copolymerConnectivity == null ? new Base[0] 2501 : this.copolymerConnectivity.toArray(new Base[this.copolymerConnectivity.size()]); // CodeableConcept 2502 case -684600932: 2503 /* modification */ return this.modification == null ? new Base[0] 2504 : this.modification.toArray(new Base[this.modification.size()]); // StringType 2505 case -1622483765: 2506 /* monomerSet */ return this.monomerSet == null ? new Base[0] 2507 : this.monomerSet.toArray(new Base[this.monomerSet.size()]); // SubstancePolymerMonomerSetComponent 2508 case -934531685: 2509 /* repeat */ return this.repeat == null ? new Base[0] : this.repeat.toArray(new Base[this.repeat.size()]); // SubstancePolymerRepeatComponent 2510 default: 2511 return super.getProperty(hash, name, checkValid); 2512 } 2513 2514 } 2515 2516 @Override 2517 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2518 switch (hash) { 2519 case 94742904: // class 2520 this.class_ = castToCodeableConcept(value); // CodeableConcept 2521 return value; 2522 case 1846020210: // geometry 2523 this.geometry = castToCodeableConcept(value); // CodeableConcept 2524 return value; 2525 case 997107577: // copolymerConnectivity 2526 this.getCopolymerConnectivity().add(castToCodeableConcept(value)); // CodeableConcept 2527 return value; 2528 case -684600932: // modification 2529 this.getModification().add(castToString(value)); // StringType 2530 return value; 2531 case -1622483765: // monomerSet 2532 this.getMonomerSet().add((SubstancePolymerMonomerSetComponent) value); // SubstancePolymerMonomerSetComponent 2533 return value; 2534 case -934531685: // repeat 2535 this.getRepeat().add((SubstancePolymerRepeatComponent) value); // SubstancePolymerRepeatComponent 2536 return value; 2537 default: 2538 return super.setProperty(hash, name, value); 2539 } 2540 2541 } 2542 2543 @Override 2544 public Base setProperty(String name, Base value) throws FHIRException { 2545 if (name.equals("class")) { 2546 this.class_ = castToCodeableConcept(value); // CodeableConcept 2547 } else if (name.equals("geometry")) { 2548 this.geometry = castToCodeableConcept(value); // CodeableConcept 2549 } else if (name.equals("copolymerConnectivity")) { 2550 this.getCopolymerConnectivity().add(castToCodeableConcept(value)); 2551 } else if (name.equals("modification")) { 2552 this.getModification().add(castToString(value)); 2553 } else if (name.equals("monomerSet")) { 2554 this.getMonomerSet().add((SubstancePolymerMonomerSetComponent) value); 2555 } else if (name.equals("repeat")) { 2556 this.getRepeat().add((SubstancePolymerRepeatComponent) value); 2557 } else 2558 return super.setProperty(name, value); 2559 return value; 2560 } 2561 2562 @Override 2563 public void removeChild(String name, Base value) throws FHIRException { 2564 if (name.equals("class")) { 2565 this.class_ = null; 2566 } else if (name.equals("geometry")) { 2567 this.geometry = null; 2568 } else if (name.equals("copolymerConnectivity")) { 2569 this.getCopolymerConnectivity().remove(castToCodeableConcept(value)); 2570 } else if (name.equals("modification")) { 2571 this.getModification().remove(castToString(value)); 2572 } else if (name.equals("monomerSet")) { 2573 this.getMonomerSet().remove((SubstancePolymerMonomerSetComponent) value); 2574 } else if (name.equals("repeat")) { 2575 this.getRepeat().remove((SubstancePolymerRepeatComponent) value); 2576 } else 2577 super.removeChild(name, value); 2578 2579 } 2580 2581 @Override 2582 public Base makeProperty(int hash, String name) throws FHIRException { 2583 switch (hash) { 2584 case 94742904: 2585 return getClass_(); 2586 case 1846020210: 2587 return getGeometry(); 2588 case 997107577: 2589 return addCopolymerConnectivity(); 2590 case -684600932: 2591 return addModificationElement(); 2592 case -1622483765: 2593 return addMonomerSet(); 2594 case -934531685: 2595 return addRepeat(); 2596 default: 2597 return super.makeProperty(hash, name); 2598 } 2599 2600 } 2601 2602 @Override 2603 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2604 switch (hash) { 2605 case 94742904: 2606 /* class */ return new String[] { "CodeableConcept" }; 2607 case 1846020210: 2608 /* geometry */ return new String[] { "CodeableConcept" }; 2609 case 997107577: 2610 /* copolymerConnectivity */ return new String[] { "CodeableConcept" }; 2611 case -684600932: 2612 /* modification */ return new String[] { "string" }; 2613 case -1622483765: 2614 /* monomerSet */ return new String[] {}; 2615 case -934531685: 2616 /* repeat */ return new String[] {}; 2617 default: 2618 return super.getTypesForProperty(hash, name); 2619 } 2620 2621 } 2622 2623 @Override 2624 public Base addChild(String name) throws FHIRException { 2625 if (name.equals("class")) { 2626 this.class_ = new CodeableConcept(); 2627 return this.class_; 2628 } else if (name.equals("geometry")) { 2629 this.geometry = new CodeableConcept(); 2630 return this.geometry; 2631 } else if (name.equals("copolymerConnectivity")) { 2632 return addCopolymerConnectivity(); 2633 } else if (name.equals("modification")) { 2634 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.modification"); 2635 } else if (name.equals("monomerSet")) { 2636 return addMonomerSet(); 2637 } else if (name.equals("repeat")) { 2638 return addRepeat(); 2639 } else 2640 return super.addChild(name); 2641 } 2642 2643 public String fhirType() { 2644 return "SubstancePolymer"; 2645 2646 } 2647 2648 public SubstancePolymer copy() { 2649 SubstancePolymer dst = new SubstancePolymer(); 2650 copyValues(dst); 2651 return dst; 2652 } 2653 2654 public void copyValues(SubstancePolymer dst) { 2655 super.copyValues(dst); 2656 dst.class_ = class_ == null ? null : class_.copy(); 2657 dst.geometry = geometry == null ? null : geometry.copy(); 2658 if (copolymerConnectivity != null) { 2659 dst.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2660 for (CodeableConcept i : copolymerConnectivity) 2661 dst.copolymerConnectivity.add(i.copy()); 2662 } 2663 ; 2664 if (modification != null) { 2665 dst.modification = new ArrayList<StringType>(); 2666 for (StringType i : modification) 2667 dst.modification.add(i.copy()); 2668 } 2669 ; 2670 if (monomerSet != null) { 2671 dst.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2672 for (SubstancePolymerMonomerSetComponent i : monomerSet) 2673 dst.monomerSet.add(i.copy()); 2674 } 2675 ; 2676 if (repeat != null) { 2677 dst.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2678 for (SubstancePolymerRepeatComponent i : repeat) 2679 dst.repeat.add(i.copy()); 2680 } 2681 ; 2682 } 2683 2684 protected SubstancePolymer typedCopy() { 2685 return copy(); 2686 } 2687 2688 @Override 2689 public boolean equalsDeep(Base other_) { 2690 if (!super.equalsDeep(other_)) 2691 return false; 2692 if (!(other_ instanceof SubstancePolymer)) 2693 return false; 2694 SubstancePolymer o = (SubstancePolymer) other_; 2695 return compareDeep(class_, o.class_, true) && compareDeep(geometry, o.geometry, true) 2696 && compareDeep(copolymerConnectivity, o.copolymerConnectivity, true) 2697 && compareDeep(modification, o.modification, true) && compareDeep(monomerSet, o.monomerSet, true) 2698 && compareDeep(repeat, o.repeat, true); 2699 } 2700 2701 @Override 2702 public boolean equalsShallow(Base other_) { 2703 if (!super.equalsShallow(other_)) 2704 return false; 2705 if (!(other_ instanceof SubstancePolymer)) 2706 return false; 2707 SubstancePolymer o = (SubstancePolymer) other_; 2708 return compareValues(modification, o.modification, true); 2709 } 2710 2711 public boolean isEmpty() { 2712 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(class_, geometry, copolymerConnectivity, 2713 modification, monomerSet, repeat); 2714 } 2715 2716 @Override 2717 public ResourceType getResourceType() { 2718 return ResourceType.SubstancePolymer; 2719 } 2720 2721}