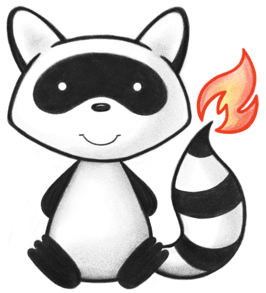
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044 045/** 046 * Todo. 047 */ 048@ResourceDef(name = "SubstancePolymer", profile = "http://hl7.org/fhir/StructureDefinition/SubstancePolymer") 049public class SubstancePolymer extends DomainResource { 050 051 @Block() 052 public static class SubstancePolymerMonomerSetComponent extends BackboneElement implements IBaseBackboneElement { 053 /** 054 * Todo. 055 */ 056 @Child(name = "ratioType", type = { 057 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 058 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 059 protected CodeableConcept ratioType; 060 061 /** 062 * Todo. 063 */ 064 @Child(name = "startingMaterial", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 065 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 066 protected List<SubstancePolymerMonomerSetStartingMaterialComponent> startingMaterial; 067 068 private static final long serialVersionUID = -933825014L; 069 070 /** 071 * Constructor 072 */ 073 public SubstancePolymerMonomerSetComponent() { 074 super(); 075 } 076 077 /** 078 * @return {@link #ratioType} (Todo.) 079 */ 080 public CodeableConcept getRatioType() { 081 if (this.ratioType == null) 082 if (Configuration.errorOnAutoCreate()) 083 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetComponent.ratioType"); 084 else if (Configuration.doAutoCreate()) 085 this.ratioType = new CodeableConcept(); // cc 086 return this.ratioType; 087 } 088 089 public boolean hasRatioType() { 090 return this.ratioType != null && !this.ratioType.isEmpty(); 091 } 092 093 /** 094 * @param value {@link #ratioType} (Todo.) 095 */ 096 public SubstancePolymerMonomerSetComponent setRatioType(CodeableConcept value) { 097 this.ratioType = value; 098 return this; 099 } 100 101 /** 102 * @return {@link #startingMaterial} (Todo.) 103 */ 104 public List<SubstancePolymerMonomerSetStartingMaterialComponent> getStartingMaterial() { 105 if (this.startingMaterial == null) 106 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 107 return this.startingMaterial; 108 } 109 110 /** 111 * @return Returns a reference to <code>this</code> for easy method chaining 112 */ 113 public SubstancePolymerMonomerSetComponent setStartingMaterial( 114 List<SubstancePolymerMonomerSetStartingMaterialComponent> theStartingMaterial) { 115 this.startingMaterial = theStartingMaterial; 116 return this; 117 } 118 119 public boolean hasStartingMaterial() { 120 if (this.startingMaterial == null) 121 return false; 122 for (SubstancePolymerMonomerSetStartingMaterialComponent item : this.startingMaterial) 123 if (!item.isEmpty()) 124 return true; 125 return false; 126 } 127 128 public SubstancePolymerMonomerSetStartingMaterialComponent addStartingMaterial() { // 3 129 SubstancePolymerMonomerSetStartingMaterialComponent t = new SubstancePolymerMonomerSetStartingMaterialComponent(); 130 if (this.startingMaterial == null) 131 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 132 this.startingMaterial.add(t); 133 return t; 134 } 135 136 public SubstancePolymerMonomerSetComponent addStartingMaterial( 137 SubstancePolymerMonomerSetStartingMaterialComponent t) { // 3 138 if (t == null) 139 return this; 140 if (this.startingMaterial == null) 141 this.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 142 this.startingMaterial.add(t); 143 return this; 144 } 145 146 /** 147 * @return The first repetition of repeating field {@link #startingMaterial}, 148 * creating it if it does not already exist 149 */ 150 public SubstancePolymerMonomerSetStartingMaterialComponent getStartingMaterialFirstRep() { 151 if (getStartingMaterial().isEmpty()) { 152 addStartingMaterial(); 153 } 154 return getStartingMaterial().get(0); 155 } 156 157 protected void listChildren(List<Property> children) { 158 super.listChildren(children); 159 children.add(new Property("ratioType", "CodeableConcept", "Todo.", 0, 1, ratioType)); 160 children.add(new Property("startingMaterial", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, startingMaterial)); 161 } 162 163 @Override 164 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 165 switch (_hash) { 166 case 344937957: 167 /* ratioType */ return new Property("ratioType", "CodeableConcept", "Todo.", 0, 1, ratioType); 168 case 442919303: 169 /* startingMaterial */ return new Property("startingMaterial", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, 170 startingMaterial); 171 default: 172 return super.getNamedProperty(_hash, _name, _checkValid); 173 } 174 175 } 176 177 @Override 178 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 179 switch (hash) { 180 case 344937957: 181 /* ratioType */ return this.ratioType == null ? new Base[0] : new Base[] { this.ratioType }; // CodeableConcept 182 case 442919303: 183 /* startingMaterial */ return this.startingMaterial == null ? new Base[0] 184 : this.startingMaterial.toArray(new Base[this.startingMaterial.size()]); // SubstancePolymerMonomerSetStartingMaterialComponent 185 default: 186 return super.getProperty(hash, name, checkValid); 187 } 188 189 } 190 191 @Override 192 public Base setProperty(int hash, String name, Base value) throws FHIRException { 193 switch (hash) { 194 case 344937957: // ratioType 195 this.ratioType = castToCodeableConcept(value); // CodeableConcept 196 return value; 197 case 442919303: // startingMaterial 198 this.getStartingMaterial().add((SubstancePolymerMonomerSetStartingMaterialComponent) value); // SubstancePolymerMonomerSetStartingMaterialComponent 199 return value; 200 default: 201 return super.setProperty(hash, name, value); 202 } 203 204 } 205 206 @Override 207 public Base setProperty(String name, Base value) throws FHIRException { 208 if (name.equals("ratioType")) { 209 this.ratioType = castToCodeableConcept(value); // CodeableConcept 210 } else if (name.equals("startingMaterial")) { 211 this.getStartingMaterial().add((SubstancePolymerMonomerSetStartingMaterialComponent) value); 212 } else 213 return super.setProperty(name, value); 214 return value; 215 } 216 217 @Override 218 public Base makeProperty(int hash, String name) throws FHIRException { 219 switch (hash) { 220 case 344937957: 221 return getRatioType(); 222 case 442919303: 223 return addStartingMaterial(); 224 default: 225 return super.makeProperty(hash, name); 226 } 227 228 } 229 230 @Override 231 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 232 switch (hash) { 233 case 344937957: 234 /* ratioType */ return new String[] { "CodeableConcept" }; 235 case 442919303: 236 /* startingMaterial */ return new String[] {}; 237 default: 238 return super.getTypesForProperty(hash, name); 239 } 240 241 } 242 243 @Override 244 public Base addChild(String name) throws FHIRException { 245 if (name.equals("ratioType")) { 246 this.ratioType = new CodeableConcept(); 247 return this.ratioType; 248 } else if (name.equals("startingMaterial")) { 249 return addStartingMaterial(); 250 } else 251 return super.addChild(name); 252 } 253 254 public SubstancePolymerMonomerSetComponent copy() { 255 SubstancePolymerMonomerSetComponent dst = new SubstancePolymerMonomerSetComponent(); 256 copyValues(dst); 257 return dst; 258 } 259 260 public void copyValues(SubstancePolymerMonomerSetComponent dst) { 261 super.copyValues(dst); 262 dst.ratioType = ratioType == null ? null : ratioType.copy(); 263 if (startingMaterial != null) { 264 dst.startingMaterial = new ArrayList<SubstancePolymerMonomerSetStartingMaterialComponent>(); 265 for (SubstancePolymerMonomerSetStartingMaterialComponent i : startingMaterial) 266 dst.startingMaterial.add(i.copy()); 267 } 268 ; 269 } 270 271 @Override 272 public boolean equalsDeep(Base other_) { 273 if (!super.equalsDeep(other_)) 274 return false; 275 if (!(other_ instanceof SubstancePolymerMonomerSetComponent)) 276 return false; 277 SubstancePolymerMonomerSetComponent o = (SubstancePolymerMonomerSetComponent) other_; 278 return compareDeep(ratioType, o.ratioType, true) && compareDeep(startingMaterial, o.startingMaterial, true); 279 } 280 281 @Override 282 public boolean equalsShallow(Base other_) { 283 if (!super.equalsShallow(other_)) 284 return false; 285 if (!(other_ instanceof SubstancePolymerMonomerSetComponent)) 286 return false; 287 SubstancePolymerMonomerSetComponent o = (SubstancePolymerMonomerSetComponent) other_; 288 return true; 289 } 290 291 public boolean isEmpty() { 292 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(ratioType, startingMaterial); 293 } 294 295 public String fhirType() { 296 return "SubstancePolymer.monomerSet"; 297 298 } 299 300 } 301 302 @Block() 303 public static class SubstancePolymerMonomerSetStartingMaterialComponent extends BackboneElement 304 implements IBaseBackboneElement { 305 /** 306 * Todo. 307 */ 308 @Child(name = "material", type = { 309 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 310 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 311 protected CodeableConcept material; 312 313 /** 314 * Todo. 315 */ 316 @Child(name = "type", type = { 317 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 318 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 319 protected CodeableConcept type; 320 321 /** 322 * Todo. 323 */ 324 @Child(name = "isDefining", type = { 325 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 326 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 327 protected BooleanType isDefining; 328 329 /** 330 * Todo. 331 */ 332 @Child(name = "amount", type = { 333 SubstanceAmount.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 334 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 335 protected SubstanceAmount amount; 336 337 private static final long serialVersionUID = 589614045L; 338 339 /** 340 * Constructor 341 */ 342 public SubstancePolymerMonomerSetStartingMaterialComponent() { 343 super(); 344 } 345 346 /** 347 * @return {@link #material} (Todo.) 348 */ 349 public CodeableConcept getMaterial() { 350 if (this.material == null) 351 if (Configuration.errorOnAutoCreate()) 352 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.material"); 353 else if (Configuration.doAutoCreate()) 354 this.material = new CodeableConcept(); // cc 355 return this.material; 356 } 357 358 public boolean hasMaterial() { 359 return this.material != null && !this.material.isEmpty(); 360 } 361 362 /** 363 * @param value {@link #material} (Todo.) 364 */ 365 public SubstancePolymerMonomerSetStartingMaterialComponent setMaterial(CodeableConcept value) { 366 this.material = value; 367 return this; 368 } 369 370 /** 371 * @return {@link #type} (Todo.) 372 */ 373 public CodeableConcept getType() { 374 if (this.type == null) 375 if (Configuration.errorOnAutoCreate()) 376 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.type"); 377 else if (Configuration.doAutoCreate()) 378 this.type = new CodeableConcept(); // cc 379 return this.type; 380 } 381 382 public boolean hasType() { 383 return this.type != null && !this.type.isEmpty(); 384 } 385 386 /** 387 * @param value {@link #type} (Todo.) 388 */ 389 public SubstancePolymerMonomerSetStartingMaterialComponent setType(CodeableConcept value) { 390 this.type = value; 391 return this; 392 } 393 394 /** 395 * @return {@link #isDefining} (Todo.). This is the underlying object with id, 396 * value and extensions. The accessor "getIsDefining" gives direct 397 * access to the value 398 */ 399 public BooleanType getIsDefiningElement() { 400 if (this.isDefining == null) 401 if (Configuration.errorOnAutoCreate()) 402 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.isDefining"); 403 else if (Configuration.doAutoCreate()) 404 this.isDefining = new BooleanType(); // bb 405 return this.isDefining; 406 } 407 408 public boolean hasIsDefiningElement() { 409 return this.isDefining != null && !this.isDefining.isEmpty(); 410 } 411 412 public boolean hasIsDefining() { 413 return this.isDefining != null && !this.isDefining.isEmpty(); 414 } 415 416 /** 417 * @param value {@link #isDefining} (Todo.). This is the underlying object with 418 * id, value and extensions. The accessor "getIsDefining" gives 419 * direct access to the value 420 */ 421 public SubstancePolymerMonomerSetStartingMaterialComponent setIsDefiningElement(BooleanType value) { 422 this.isDefining = value; 423 return this; 424 } 425 426 /** 427 * @return Todo. 428 */ 429 public boolean getIsDefining() { 430 return this.isDefining == null || this.isDefining.isEmpty() ? false : this.isDefining.getValue(); 431 } 432 433 /** 434 * @param value Todo. 435 */ 436 public SubstancePolymerMonomerSetStartingMaterialComponent setIsDefining(boolean value) { 437 if (this.isDefining == null) 438 this.isDefining = new BooleanType(); 439 this.isDefining.setValue(value); 440 return this; 441 } 442 443 /** 444 * @return {@link #amount} (Todo.) 445 */ 446 public SubstanceAmount getAmount() { 447 if (this.amount == null) 448 if (Configuration.errorOnAutoCreate()) 449 throw new Error("Attempt to auto-create SubstancePolymerMonomerSetStartingMaterialComponent.amount"); 450 else if (Configuration.doAutoCreate()) 451 this.amount = new SubstanceAmount(); // cc 452 return this.amount; 453 } 454 455 public boolean hasAmount() { 456 return this.amount != null && !this.amount.isEmpty(); 457 } 458 459 /** 460 * @param value {@link #amount} (Todo.) 461 */ 462 public SubstancePolymerMonomerSetStartingMaterialComponent setAmount(SubstanceAmount value) { 463 this.amount = value; 464 return this; 465 } 466 467 protected void listChildren(List<Property> children) { 468 super.listChildren(children); 469 children.add(new Property("material", "CodeableConcept", "Todo.", 0, 1, material)); 470 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 471 children.add(new Property("isDefining", "boolean", "Todo.", 0, 1, isDefining)); 472 children.add(new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount)); 473 } 474 475 @Override 476 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 477 switch (_hash) { 478 case 299066663: 479 /* material */ return new Property("material", "CodeableConcept", "Todo.", 0, 1, material); 480 case 3575610: 481 /* type */ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 482 case -141812990: 483 /* isDefining */ return new Property("isDefining", "boolean", "Todo.", 0, 1, isDefining); 484 case -1413853096: 485 /* amount */ return new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount); 486 default: 487 return super.getNamedProperty(_hash, _name, _checkValid); 488 } 489 490 } 491 492 @Override 493 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 494 switch (hash) { 495 case 299066663: 496 /* material */ return this.material == null ? new Base[0] : new Base[] { this.material }; // CodeableConcept 497 case 3575610: 498 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 499 case -141812990: 500 /* isDefining */ return this.isDefining == null ? new Base[0] : new Base[] { this.isDefining }; // BooleanType 501 case -1413853096: 502 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // SubstanceAmount 503 default: 504 return super.getProperty(hash, name, checkValid); 505 } 506 507 } 508 509 @Override 510 public Base setProperty(int hash, String name, Base value) throws FHIRException { 511 switch (hash) { 512 case 299066663: // material 513 this.material = castToCodeableConcept(value); // CodeableConcept 514 return value; 515 case 3575610: // type 516 this.type = castToCodeableConcept(value); // CodeableConcept 517 return value; 518 case -141812990: // isDefining 519 this.isDefining = castToBoolean(value); // BooleanType 520 return value; 521 case -1413853096: // amount 522 this.amount = castToSubstanceAmount(value); // SubstanceAmount 523 return value; 524 default: 525 return super.setProperty(hash, name, value); 526 } 527 528 } 529 530 @Override 531 public Base setProperty(String name, Base value) throws FHIRException { 532 if (name.equals("material")) { 533 this.material = castToCodeableConcept(value); // CodeableConcept 534 } else if (name.equals("type")) { 535 this.type = castToCodeableConcept(value); // CodeableConcept 536 } else if (name.equals("isDefining")) { 537 this.isDefining = castToBoolean(value); // BooleanType 538 } else if (name.equals("amount")) { 539 this.amount = castToSubstanceAmount(value); // SubstanceAmount 540 } else 541 return super.setProperty(name, value); 542 return value; 543 } 544 545 @Override 546 public Base makeProperty(int hash, String name) throws FHIRException { 547 switch (hash) { 548 case 299066663: 549 return getMaterial(); 550 case 3575610: 551 return getType(); 552 case -141812990: 553 return getIsDefiningElement(); 554 case -1413853096: 555 return getAmount(); 556 default: 557 return super.makeProperty(hash, name); 558 } 559 560 } 561 562 @Override 563 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 564 switch (hash) { 565 case 299066663: 566 /* material */ return new String[] { "CodeableConcept" }; 567 case 3575610: 568 /* type */ return new String[] { "CodeableConcept" }; 569 case -141812990: 570 /* isDefining */ return new String[] { "boolean" }; 571 case -1413853096: 572 /* amount */ return new String[] { "SubstanceAmount" }; 573 default: 574 return super.getTypesForProperty(hash, name); 575 } 576 577 } 578 579 @Override 580 public Base addChild(String name) throws FHIRException { 581 if (name.equals("material")) { 582 this.material = new CodeableConcept(); 583 return this.material; 584 } else if (name.equals("type")) { 585 this.type = new CodeableConcept(); 586 return this.type; 587 } else if (name.equals("isDefining")) { 588 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.isDefining"); 589 } else if (name.equals("amount")) { 590 this.amount = new SubstanceAmount(); 591 return this.amount; 592 } else 593 return super.addChild(name); 594 } 595 596 public SubstancePolymerMonomerSetStartingMaterialComponent copy() { 597 SubstancePolymerMonomerSetStartingMaterialComponent dst = new SubstancePolymerMonomerSetStartingMaterialComponent(); 598 copyValues(dst); 599 return dst; 600 } 601 602 public void copyValues(SubstancePolymerMonomerSetStartingMaterialComponent dst) { 603 super.copyValues(dst); 604 dst.material = material == null ? null : material.copy(); 605 dst.type = type == null ? null : type.copy(); 606 dst.isDefining = isDefining == null ? null : isDefining.copy(); 607 dst.amount = amount == null ? null : amount.copy(); 608 } 609 610 @Override 611 public boolean equalsDeep(Base other_) { 612 if (!super.equalsDeep(other_)) 613 return false; 614 if (!(other_ instanceof SubstancePolymerMonomerSetStartingMaterialComponent)) 615 return false; 616 SubstancePolymerMonomerSetStartingMaterialComponent o = (SubstancePolymerMonomerSetStartingMaterialComponent) other_; 617 return compareDeep(material, o.material, true) && compareDeep(type, o.type, true) 618 && compareDeep(isDefining, o.isDefining, true) && compareDeep(amount, o.amount, true); 619 } 620 621 @Override 622 public boolean equalsShallow(Base other_) { 623 if (!super.equalsShallow(other_)) 624 return false; 625 if (!(other_ instanceof SubstancePolymerMonomerSetStartingMaterialComponent)) 626 return false; 627 SubstancePolymerMonomerSetStartingMaterialComponent o = (SubstancePolymerMonomerSetStartingMaterialComponent) other_; 628 return compareValues(isDefining, o.isDefining, true); 629 } 630 631 public boolean isEmpty() { 632 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(material, type, isDefining, amount); 633 } 634 635 public String fhirType() { 636 return "SubstancePolymer.monomerSet.startingMaterial"; 637 638 } 639 640 } 641 642 @Block() 643 public static class SubstancePolymerRepeatComponent extends BackboneElement implements IBaseBackboneElement { 644 /** 645 * Todo. 646 */ 647 @Child(name = "numberOfUnits", type = { 648 IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 649 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 650 protected IntegerType numberOfUnits; 651 652 /** 653 * Todo. 654 */ 655 @Child(name = "averageMolecularFormula", type = { 656 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 657 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 658 protected StringType averageMolecularFormula; 659 660 /** 661 * Todo. 662 */ 663 @Child(name = "repeatUnitAmountType", type = { 664 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 665 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 666 protected CodeableConcept repeatUnitAmountType; 667 668 /** 669 * Todo. 670 */ 671 @Child(name = "repeatUnit", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 672 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 673 protected List<SubstancePolymerRepeatRepeatUnitComponent> repeatUnit; 674 675 private static final long serialVersionUID = -988147059L; 676 677 /** 678 * Constructor 679 */ 680 public SubstancePolymerRepeatComponent() { 681 super(); 682 } 683 684 /** 685 * @return {@link #numberOfUnits} (Todo.). This is the underlying object with 686 * id, value and extensions. The accessor "getNumberOfUnits" gives 687 * direct access to the value 688 */ 689 public IntegerType getNumberOfUnitsElement() { 690 if (this.numberOfUnits == null) 691 if (Configuration.errorOnAutoCreate()) 692 throw new Error("Attempt to auto-create SubstancePolymerRepeatComponent.numberOfUnits"); 693 else if (Configuration.doAutoCreate()) 694 this.numberOfUnits = new IntegerType(); // bb 695 return this.numberOfUnits; 696 } 697 698 public boolean hasNumberOfUnitsElement() { 699 return this.numberOfUnits != null && !this.numberOfUnits.isEmpty(); 700 } 701 702 public boolean hasNumberOfUnits() { 703 return this.numberOfUnits != null && !this.numberOfUnits.isEmpty(); 704 } 705 706 /** 707 * @param value {@link #numberOfUnits} (Todo.). This is the underlying object 708 * with id, value and extensions. The accessor "getNumberOfUnits" 709 * gives direct access to the value 710 */ 711 public SubstancePolymerRepeatComponent setNumberOfUnitsElement(IntegerType value) { 712 this.numberOfUnits = value; 713 return this; 714 } 715 716 /** 717 * @return Todo. 718 */ 719 public int getNumberOfUnits() { 720 return this.numberOfUnits == null || this.numberOfUnits.isEmpty() ? 0 : this.numberOfUnits.getValue(); 721 } 722 723 /** 724 * @param value Todo. 725 */ 726 public SubstancePolymerRepeatComponent setNumberOfUnits(int value) { 727 if (this.numberOfUnits == null) 728 this.numberOfUnits = new IntegerType(); 729 this.numberOfUnits.setValue(value); 730 return this; 731 } 732 733 /** 734 * @return {@link #averageMolecularFormula} (Todo.). This is the underlying 735 * object with id, value and extensions. The accessor 736 * "getAverageMolecularFormula" gives direct access to the value 737 */ 738 public StringType getAverageMolecularFormulaElement() { 739 if (this.averageMolecularFormula == null) 740 if (Configuration.errorOnAutoCreate()) 741 throw new Error("Attempt to auto-create SubstancePolymerRepeatComponent.averageMolecularFormula"); 742 else if (Configuration.doAutoCreate()) 743 this.averageMolecularFormula = new StringType(); // bb 744 return this.averageMolecularFormula; 745 } 746 747 public boolean hasAverageMolecularFormulaElement() { 748 return this.averageMolecularFormula != null && !this.averageMolecularFormula.isEmpty(); 749 } 750 751 public boolean hasAverageMolecularFormula() { 752 return this.averageMolecularFormula != null && !this.averageMolecularFormula.isEmpty(); 753 } 754 755 /** 756 * @param value {@link #averageMolecularFormula} (Todo.). This is the underlying 757 * object with id, value and extensions. The accessor 758 * "getAverageMolecularFormula" gives direct access to the value 759 */ 760 public SubstancePolymerRepeatComponent setAverageMolecularFormulaElement(StringType value) { 761 this.averageMolecularFormula = value; 762 return this; 763 } 764 765 /** 766 * @return Todo. 767 */ 768 public String getAverageMolecularFormula() { 769 return this.averageMolecularFormula == null ? null : this.averageMolecularFormula.getValue(); 770 } 771 772 /** 773 * @param value Todo. 774 */ 775 public SubstancePolymerRepeatComponent setAverageMolecularFormula(String value) { 776 if (Utilities.noString(value)) 777 this.averageMolecularFormula = null; 778 else { 779 if (this.averageMolecularFormula == null) 780 this.averageMolecularFormula = new StringType(); 781 this.averageMolecularFormula.setValue(value); 782 } 783 return this; 784 } 785 786 /** 787 * @return {@link #repeatUnitAmountType} (Todo.) 788 */ 789 public CodeableConcept getRepeatUnitAmountType() { 790 if (this.repeatUnitAmountType == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create SubstancePolymerRepeatComponent.repeatUnitAmountType"); 793 else if (Configuration.doAutoCreate()) 794 this.repeatUnitAmountType = new CodeableConcept(); // cc 795 return this.repeatUnitAmountType; 796 } 797 798 public boolean hasRepeatUnitAmountType() { 799 return this.repeatUnitAmountType != null && !this.repeatUnitAmountType.isEmpty(); 800 } 801 802 /** 803 * @param value {@link #repeatUnitAmountType} (Todo.) 804 */ 805 public SubstancePolymerRepeatComponent setRepeatUnitAmountType(CodeableConcept value) { 806 this.repeatUnitAmountType = value; 807 return this; 808 } 809 810 /** 811 * @return {@link #repeatUnit} (Todo.) 812 */ 813 public List<SubstancePolymerRepeatRepeatUnitComponent> getRepeatUnit() { 814 if (this.repeatUnit == null) 815 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 816 return this.repeatUnit; 817 } 818 819 /** 820 * @return Returns a reference to <code>this</code> for easy method chaining 821 */ 822 public SubstancePolymerRepeatComponent setRepeatUnit( 823 List<SubstancePolymerRepeatRepeatUnitComponent> theRepeatUnit) { 824 this.repeatUnit = theRepeatUnit; 825 return this; 826 } 827 828 public boolean hasRepeatUnit() { 829 if (this.repeatUnit == null) 830 return false; 831 for (SubstancePolymerRepeatRepeatUnitComponent item : this.repeatUnit) 832 if (!item.isEmpty()) 833 return true; 834 return false; 835 } 836 837 public SubstancePolymerRepeatRepeatUnitComponent addRepeatUnit() { // 3 838 SubstancePolymerRepeatRepeatUnitComponent t = new SubstancePolymerRepeatRepeatUnitComponent(); 839 if (this.repeatUnit == null) 840 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 841 this.repeatUnit.add(t); 842 return t; 843 } 844 845 public SubstancePolymerRepeatComponent addRepeatUnit(SubstancePolymerRepeatRepeatUnitComponent t) { // 3 846 if (t == null) 847 return this; 848 if (this.repeatUnit == null) 849 this.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 850 this.repeatUnit.add(t); 851 return this; 852 } 853 854 /** 855 * @return The first repetition of repeating field {@link #repeatUnit}, creating 856 * it if it does not already exist 857 */ 858 public SubstancePolymerRepeatRepeatUnitComponent getRepeatUnitFirstRep() { 859 if (getRepeatUnit().isEmpty()) { 860 addRepeatUnit(); 861 } 862 return getRepeatUnit().get(0); 863 } 864 865 protected void listChildren(List<Property> children) { 866 super.listChildren(children); 867 children.add(new Property("numberOfUnits", "integer", "Todo.", 0, 1, numberOfUnits)); 868 children.add(new Property("averageMolecularFormula", "string", "Todo.", 0, 1, averageMolecularFormula)); 869 children.add(new Property("repeatUnitAmountType", "CodeableConcept", "Todo.", 0, 1, repeatUnitAmountType)); 870 children.add(new Property("repeatUnit", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, repeatUnit)); 871 } 872 873 @Override 874 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 875 switch (_hash) { 876 case -1321430961: 877 /* numberOfUnits */ return new Property("numberOfUnits", "integer", "Todo.", 0, 1, numberOfUnits); 878 case 111461715: 879 /* averageMolecularFormula */ return new Property("averageMolecularFormula", "string", "Todo.", 0, 1, 880 averageMolecularFormula); 881 case -1994025263: 882 /* repeatUnitAmountType */ return new Property("repeatUnitAmountType", "CodeableConcept", "Todo.", 0, 1, 883 repeatUnitAmountType); 884 case 1159607743: 885 /* repeatUnit */ return new Property("repeatUnit", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, repeatUnit); 886 default: 887 return super.getNamedProperty(_hash, _name, _checkValid); 888 } 889 890 } 891 892 @Override 893 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 894 switch (hash) { 895 case -1321430961: 896 /* numberOfUnits */ return this.numberOfUnits == null ? new Base[0] : new Base[] { this.numberOfUnits }; // IntegerType 897 case 111461715: 898 /* averageMolecularFormula */ return this.averageMolecularFormula == null ? new Base[0] 899 : new Base[] { this.averageMolecularFormula }; // StringType 900 case -1994025263: 901 /* repeatUnitAmountType */ return this.repeatUnitAmountType == null ? new Base[0] 902 : new Base[] { this.repeatUnitAmountType }; // CodeableConcept 903 case 1159607743: 904 /* repeatUnit */ return this.repeatUnit == null ? new Base[0] 905 : this.repeatUnit.toArray(new Base[this.repeatUnit.size()]); // SubstancePolymerRepeatRepeatUnitComponent 906 default: 907 return super.getProperty(hash, name, checkValid); 908 } 909 910 } 911 912 @Override 913 public Base setProperty(int hash, String name, Base value) throws FHIRException { 914 switch (hash) { 915 case -1321430961: // numberOfUnits 916 this.numberOfUnits = castToInteger(value); // IntegerType 917 return value; 918 case 111461715: // averageMolecularFormula 919 this.averageMolecularFormula = castToString(value); // StringType 920 return value; 921 case -1994025263: // repeatUnitAmountType 922 this.repeatUnitAmountType = castToCodeableConcept(value); // CodeableConcept 923 return value; 924 case 1159607743: // repeatUnit 925 this.getRepeatUnit().add((SubstancePolymerRepeatRepeatUnitComponent) value); // SubstancePolymerRepeatRepeatUnitComponent 926 return value; 927 default: 928 return super.setProperty(hash, name, value); 929 } 930 931 } 932 933 @Override 934 public Base setProperty(String name, Base value) throws FHIRException { 935 if (name.equals("numberOfUnits")) { 936 this.numberOfUnits = castToInteger(value); // IntegerType 937 } else if (name.equals("averageMolecularFormula")) { 938 this.averageMolecularFormula = castToString(value); // StringType 939 } else if (name.equals("repeatUnitAmountType")) { 940 this.repeatUnitAmountType = castToCodeableConcept(value); // CodeableConcept 941 } else if (name.equals("repeatUnit")) { 942 this.getRepeatUnit().add((SubstancePolymerRepeatRepeatUnitComponent) value); 943 } else 944 return super.setProperty(name, value); 945 return value; 946 } 947 948 @Override 949 public Base makeProperty(int hash, String name) throws FHIRException { 950 switch (hash) { 951 case -1321430961: 952 return getNumberOfUnitsElement(); 953 case 111461715: 954 return getAverageMolecularFormulaElement(); 955 case -1994025263: 956 return getRepeatUnitAmountType(); 957 case 1159607743: 958 return addRepeatUnit(); 959 default: 960 return super.makeProperty(hash, name); 961 } 962 963 } 964 965 @Override 966 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 967 switch (hash) { 968 case -1321430961: 969 /* numberOfUnits */ return new String[] { "integer" }; 970 case 111461715: 971 /* averageMolecularFormula */ return new String[] { "string" }; 972 case -1994025263: 973 /* repeatUnitAmountType */ return new String[] { "CodeableConcept" }; 974 case 1159607743: 975 /* repeatUnit */ return new String[] {}; 976 default: 977 return super.getTypesForProperty(hash, name); 978 } 979 980 } 981 982 @Override 983 public Base addChild(String name) throws FHIRException { 984 if (name.equals("numberOfUnits")) { 985 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.numberOfUnits"); 986 } else if (name.equals("averageMolecularFormula")) { 987 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.averageMolecularFormula"); 988 } else if (name.equals("repeatUnitAmountType")) { 989 this.repeatUnitAmountType = new CodeableConcept(); 990 return this.repeatUnitAmountType; 991 } else if (name.equals("repeatUnit")) { 992 return addRepeatUnit(); 993 } else 994 return super.addChild(name); 995 } 996 997 public SubstancePolymerRepeatComponent copy() { 998 SubstancePolymerRepeatComponent dst = new SubstancePolymerRepeatComponent(); 999 copyValues(dst); 1000 return dst; 1001 } 1002 1003 public void copyValues(SubstancePolymerRepeatComponent dst) { 1004 super.copyValues(dst); 1005 dst.numberOfUnits = numberOfUnits == null ? null : numberOfUnits.copy(); 1006 dst.averageMolecularFormula = averageMolecularFormula == null ? null : averageMolecularFormula.copy(); 1007 dst.repeatUnitAmountType = repeatUnitAmountType == null ? null : repeatUnitAmountType.copy(); 1008 if (repeatUnit != null) { 1009 dst.repeatUnit = new ArrayList<SubstancePolymerRepeatRepeatUnitComponent>(); 1010 for (SubstancePolymerRepeatRepeatUnitComponent i : repeatUnit) 1011 dst.repeatUnit.add(i.copy()); 1012 } 1013 ; 1014 } 1015 1016 @Override 1017 public boolean equalsDeep(Base other_) { 1018 if (!super.equalsDeep(other_)) 1019 return false; 1020 if (!(other_ instanceof SubstancePolymerRepeatComponent)) 1021 return false; 1022 SubstancePolymerRepeatComponent o = (SubstancePolymerRepeatComponent) other_; 1023 return compareDeep(numberOfUnits, o.numberOfUnits, true) 1024 && compareDeep(averageMolecularFormula, o.averageMolecularFormula, true) 1025 && compareDeep(repeatUnitAmountType, o.repeatUnitAmountType, true) 1026 && compareDeep(repeatUnit, o.repeatUnit, true); 1027 } 1028 1029 @Override 1030 public boolean equalsShallow(Base other_) { 1031 if (!super.equalsShallow(other_)) 1032 return false; 1033 if (!(other_ instanceof SubstancePolymerRepeatComponent)) 1034 return false; 1035 SubstancePolymerRepeatComponent o = (SubstancePolymerRepeatComponent) other_; 1036 return compareValues(numberOfUnits, o.numberOfUnits, true) 1037 && compareValues(averageMolecularFormula, o.averageMolecularFormula, true); 1038 } 1039 1040 public boolean isEmpty() { 1041 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(numberOfUnits, averageMolecularFormula, 1042 repeatUnitAmountType, repeatUnit); 1043 } 1044 1045 public String fhirType() { 1046 return "SubstancePolymer.repeat"; 1047 1048 } 1049 1050 } 1051 1052 @Block() 1053 public static class SubstancePolymerRepeatRepeatUnitComponent extends BackboneElement 1054 implements IBaseBackboneElement { 1055 /** 1056 * Todo. 1057 */ 1058 @Child(name = "orientationOfPolymerisation", type = { 1059 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1060 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1061 protected CodeableConcept orientationOfPolymerisation; 1062 1063 /** 1064 * Todo. 1065 */ 1066 @Child(name = "repeatUnit", type = { 1067 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1068 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1069 protected StringType repeatUnit; 1070 1071 /** 1072 * Todo. 1073 */ 1074 @Child(name = "amount", type = { 1075 SubstanceAmount.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1076 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1077 protected SubstanceAmount amount; 1078 1079 /** 1080 * Todo. 1081 */ 1082 @Child(name = "degreeOfPolymerisation", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1083 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1084 protected List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> degreeOfPolymerisation; 1085 1086 /** 1087 * Todo. 1088 */ 1089 @Child(name = "structuralRepresentation", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1090 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1091 protected List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> structuralRepresentation; 1092 1093 private static final long serialVersionUID = -1823741061L; 1094 1095 /** 1096 * Constructor 1097 */ 1098 public SubstancePolymerRepeatRepeatUnitComponent() { 1099 super(); 1100 } 1101 1102 /** 1103 * @return {@link #orientationOfPolymerisation} (Todo.) 1104 */ 1105 public CodeableConcept getOrientationOfPolymerisation() { 1106 if (this.orientationOfPolymerisation == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error( 1109 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.orientationOfPolymerisation"); 1110 else if (Configuration.doAutoCreate()) 1111 this.orientationOfPolymerisation = new CodeableConcept(); // cc 1112 return this.orientationOfPolymerisation; 1113 } 1114 1115 public boolean hasOrientationOfPolymerisation() { 1116 return this.orientationOfPolymerisation != null && !this.orientationOfPolymerisation.isEmpty(); 1117 } 1118 1119 /** 1120 * @param value {@link #orientationOfPolymerisation} (Todo.) 1121 */ 1122 public SubstancePolymerRepeatRepeatUnitComponent setOrientationOfPolymerisation(CodeableConcept value) { 1123 this.orientationOfPolymerisation = value; 1124 return this; 1125 } 1126 1127 /** 1128 * @return {@link #repeatUnit} (Todo.). This is the underlying object with id, 1129 * value and extensions. The accessor "getRepeatUnit" gives direct 1130 * access to the value 1131 */ 1132 public StringType getRepeatUnitElement() { 1133 if (this.repeatUnit == null) 1134 if (Configuration.errorOnAutoCreate()) 1135 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.repeatUnit"); 1136 else if (Configuration.doAutoCreate()) 1137 this.repeatUnit = new StringType(); // bb 1138 return this.repeatUnit; 1139 } 1140 1141 public boolean hasRepeatUnitElement() { 1142 return this.repeatUnit != null && !this.repeatUnit.isEmpty(); 1143 } 1144 1145 public boolean hasRepeatUnit() { 1146 return this.repeatUnit != null && !this.repeatUnit.isEmpty(); 1147 } 1148 1149 /** 1150 * @param value {@link #repeatUnit} (Todo.). This is the underlying object with 1151 * id, value and extensions. The accessor "getRepeatUnit" gives 1152 * direct access to the value 1153 */ 1154 public SubstancePolymerRepeatRepeatUnitComponent setRepeatUnitElement(StringType value) { 1155 this.repeatUnit = value; 1156 return this; 1157 } 1158 1159 /** 1160 * @return Todo. 1161 */ 1162 public String getRepeatUnit() { 1163 return this.repeatUnit == null ? null : this.repeatUnit.getValue(); 1164 } 1165 1166 /** 1167 * @param value Todo. 1168 */ 1169 public SubstancePolymerRepeatRepeatUnitComponent setRepeatUnit(String value) { 1170 if (Utilities.noString(value)) 1171 this.repeatUnit = null; 1172 else { 1173 if (this.repeatUnit == null) 1174 this.repeatUnit = new StringType(); 1175 this.repeatUnit.setValue(value); 1176 } 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #amount} (Todo.) 1182 */ 1183 public SubstanceAmount getAmount() { 1184 if (this.amount == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create SubstancePolymerRepeatRepeatUnitComponent.amount"); 1187 else if (Configuration.doAutoCreate()) 1188 this.amount = new SubstanceAmount(); // cc 1189 return this.amount; 1190 } 1191 1192 public boolean hasAmount() { 1193 return this.amount != null && !this.amount.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #amount} (Todo.) 1198 */ 1199 public SubstancePolymerRepeatRepeatUnitComponent setAmount(SubstanceAmount value) { 1200 this.amount = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #degreeOfPolymerisation} (Todo.) 1206 */ 1207 public List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> getDegreeOfPolymerisation() { 1208 if (this.degreeOfPolymerisation == null) 1209 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1210 return this.degreeOfPolymerisation; 1211 } 1212 1213 /** 1214 * @return Returns a reference to <code>this</code> for easy method chaining 1215 */ 1216 public SubstancePolymerRepeatRepeatUnitComponent setDegreeOfPolymerisation( 1217 List<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent> theDegreeOfPolymerisation) { 1218 this.degreeOfPolymerisation = theDegreeOfPolymerisation; 1219 return this; 1220 } 1221 1222 public boolean hasDegreeOfPolymerisation() { 1223 if (this.degreeOfPolymerisation == null) 1224 return false; 1225 for (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent item : this.degreeOfPolymerisation) 1226 if (!item.isEmpty()) 1227 return true; 1228 return false; 1229 } 1230 1231 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent addDegreeOfPolymerisation() { // 3 1232 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent t = new SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(); 1233 if (this.degreeOfPolymerisation == null) 1234 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1235 this.degreeOfPolymerisation.add(t); 1236 return t; 1237 } 1238 1239 public SubstancePolymerRepeatRepeatUnitComponent addDegreeOfPolymerisation( 1240 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent t) { // 3 1241 if (t == null) 1242 return this; 1243 if (this.degreeOfPolymerisation == null) 1244 this.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1245 this.degreeOfPolymerisation.add(t); 1246 return this; 1247 } 1248 1249 /** 1250 * @return The first repetition of repeating field 1251 * {@link #degreeOfPolymerisation}, creating it if it does not already 1252 * exist 1253 */ 1254 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent getDegreeOfPolymerisationFirstRep() { 1255 if (getDegreeOfPolymerisation().isEmpty()) { 1256 addDegreeOfPolymerisation(); 1257 } 1258 return getDegreeOfPolymerisation().get(0); 1259 } 1260 1261 /** 1262 * @return {@link #structuralRepresentation} (Todo.) 1263 */ 1264 public List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> getStructuralRepresentation() { 1265 if (this.structuralRepresentation == null) 1266 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1267 return this.structuralRepresentation; 1268 } 1269 1270 /** 1271 * @return Returns a reference to <code>this</code> for easy method chaining 1272 */ 1273 public SubstancePolymerRepeatRepeatUnitComponent setStructuralRepresentation( 1274 List<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent> theStructuralRepresentation) { 1275 this.structuralRepresentation = theStructuralRepresentation; 1276 return this; 1277 } 1278 1279 public boolean hasStructuralRepresentation() { 1280 if (this.structuralRepresentation == null) 1281 return false; 1282 for (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent item : this.structuralRepresentation) 1283 if (!item.isEmpty()) 1284 return true; 1285 return false; 1286 } 1287 1288 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent addStructuralRepresentation() { // 3 1289 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent t = new SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(); 1290 if (this.structuralRepresentation == null) 1291 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1292 this.structuralRepresentation.add(t); 1293 return t; 1294 } 1295 1296 public SubstancePolymerRepeatRepeatUnitComponent addStructuralRepresentation( 1297 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent t) { // 3 1298 if (t == null) 1299 return this; 1300 if (this.structuralRepresentation == null) 1301 this.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1302 this.structuralRepresentation.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field 1308 * {@link #structuralRepresentation}, creating it if it does not already 1309 * exist 1310 */ 1311 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent getStructuralRepresentationFirstRep() { 1312 if (getStructuralRepresentation().isEmpty()) { 1313 addStructuralRepresentation(); 1314 } 1315 return getStructuralRepresentation().get(0); 1316 } 1317 1318 protected void listChildren(List<Property> children) { 1319 super.listChildren(children); 1320 children.add( 1321 new Property("orientationOfPolymerisation", "CodeableConcept", "Todo.", 0, 1, orientationOfPolymerisation)); 1322 children.add(new Property("repeatUnit", "string", "Todo.", 0, 1, repeatUnit)); 1323 children.add(new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount)); 1324 children.add( 1325 new Property("degreeOfPolymerisation", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, degreeOfPolymerisation)); 1326 children.add(new Property("structuralRepresentation", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, 1327 structuralRepresentation)); 1328 } 1329 1330 @Override 1331 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1332 switch (_hash) { 1333 case 1795817828: 1334 /* orientationOfPolymerisation */ return new Property("orientationOfPolymerisation", "CodeableConcept", "Todo.", 1335 0, 1, orientationOfPolymerisation); 1336 case 1159607743: 1337 /* repeatUnit */ return new Property("repeatUnit", "string", "Todo.", 0, 1, repeatUnit); 1338 case -1413853096: 1339 /* amount */ return new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount); 1340 case -159251872: 1341 /* degreeOfPolymerisation */ return new Property("degreeOfPolymerisation", "", "Todo.", 0, 1342 java.lang.Integer.MAX_VALUE, degreeOfPolymerisation); 1343 case 14311178: 1344 /* structuralRepresentation */ return new Property("structuralRepresentation", "", "Todo.", 0, 1345 java.lang.Integer.MAX_VALUE, structuralRepresentation); 1346 default: 1347 return super.getNamedProperty(_hash, _name, _checkValid); 1348 } 1349 1350 } 1351 1352 @Override 1353 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1354 switch (hash) { 1355 case 1795817828: 1356 /* orientationOfPolymerisation */ return this.orientationOfPolymerisation == null ? new Base[0] 1357 : new Base[] { this.orientationOfPolymerisation }; // CodeableConcept 1358 case 1159607743: 1359 /* repeatUnit */ return this.repeatUnit == null ? new Base[0] : new Base[] { this.repeatUnit }; // StringType 1360 case -1413853096: 1361 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // SubstanceAmount 1362 case -159251872: 1363 /* degreeOfPolymerisation */ return this.degreeOfPolymerisation == null ? new Base[0] 1364 : this.degreeOfPolymerisation.toArray(new Base[this.degreeOfPolymerisation.size()]); // SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent 1365 case 14311178: 1366 /* structuralRepresentation */ return this.structuralRepresentation == null ? new Base[0] 1367 : this.structuralRepresentation.toArray(new Base[this.structuralRepresentation.size()]); // SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent 1368 default: 1369 return super.getProperty(hash, name, checkValid); 1370 } 1371 1372 } 1373 1374 @Override 1375 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1376 switch (hash) { 1377 case 1795817828: // orientationOfPolymerisation 1378 this.orientationOfPolymerisation = castToCodeableConcept(value); // CodeableConcept 1379 return value; 1380 case 1159607743: // repeatUnit 1381 this.repeatUnit = castToString(value); // StringType 1382 return value; 1383 case -1413853096: // amount 1384 this.amount = castToSubstanceAmount(value); // SubstanceAmount 1385 return value; 1386 case -159251872: // degreeOfPolymerisation 1387 this.getDegreeOfPolymerisation().add((SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) value); // SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent 1388 return value; 1389 case 14311178: // structuralRepresentation 1390 this.getStructuralRepresentation() 1391 .add((SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) value); // SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent 1392 return value; 1393 default: 1394 return super.setProperty(hash, name, value); 1395 } 1396 1397 } 1398 1399 @Override 1400 public Base setProperty(String name, Base value) throws FHIRException { 1401 if (name.equals("orientationOfPolymerisation")) { 1402 this.orientationOfPolymerisation = castToCodeableConcept(value); // CodeableConcept 1403 } else if (name.equals("repeatUnit")) { 1404 this.repeatUnit = castToString(value); // StringType 1405 } else if (name.equals("amount")) { 1406 this.amount = castToSubstanceAmount(value); // SubstanceAmount 1407 } else if (name.equals("degreeOfPolymerisation")) { 1408 this.getDegreeOfPolymerisation().add((SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) value); 1409 } else if (name.equals("structuralRepresentation")) { 1410 this.getStructuralRepresentation() 1411 .add((SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) value); 1412 } else 1413 return super.setProperty(name, value); 1414 return value; 1415 } 1416 1417 @Override 1418 public Base makeProperty(int hash, String name) throws FHIRException { 1419 switch (hash) { 1420 case 1795817828: 1421 return getOrientationOfPolymerisation(); 1422 case 1159607743: 1423 return getRepeatUnitElement(); 1424 case -1413853096: 1425 return getAmount(); 1426 case -159251872: 1427 return addDegreeOfPolymerisation(); 1428 case 14311178: 1429 return addStructuralRepresentation(); 1430 default: 1431 return super.makeProperty(hash, name); 1432 } 1433 1434 } 1435 1436 @Override 1437 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1438 switch (hash) { 1439 case 1795817828: 1440 /* orientationOfPolymerisation */ return new String[] { "CodeableConcept" }; 1441 case 1159607743: 1442 /* repeatUnit */ return new String[] { "string" }; 1443 case -1413853096: 1444 /* amount */ return new String[] { "SubstanceAmount" }; 1445 case -159251872: 1446 /* degreeOfPolymerisation */ return new String[] {}; 1447 case 14311178: 1448 /* structuralRepresentation */ return new String[] {}; 1449 default: 1450 return super.getTypesForProperty(hash, name); 1451 } 1452 1453 } 1454 1455 @Override 1456 public Base addChild(String name) throws FHIRException { 1457 if (name.equals("orientationOfPolymerisation")) { 1458 this.orientationOfPolymerisation = new CodeableConcept(); 1459 return this.orientationOfPolymerisation; 1460 } else if (name.equals("repeatUnit")) { 1461 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.repeatUnit"); 1462 } else if (name.equals("amount")) { 1463 this.amount = new SubstanceAmount(); 1464 return this.amount; 1465 } else if (name.equals("degreeOfPolymerisation")) { 1466 return addDegreeOfPolymerisation(); 1467 } else if (name.equals("structuralRepresentation")) { 1468 return addStructuralRepresentation(); 1469 } else 1470 return super.addChild(name); 1471 } 1472 1473 public SubstancePolymerRepeatRepeatUnitComponent copy() { 1474 SubstancePolymerRepeatRepeatUnitComponent dst = new SubstancePolymerRepeatRepeatUnitComponent(); 1475 copyValues(dst); 1476 return dst; 1477 } 1478 1479 public void copyValues(SubstancePolymerRepeatRepeatUnitComponent dst) { 1480 super.copyValues(dst); 1481 dst.orientationOfPolymerisation = orientationOfPolymerisation == null ? null : orientationOfPolymerisation.copy(); 1482 dst.repeatUnit = repeatUnit == null ? null : repeatUnit.copy(); 1483 dst.amount = amount == null ? null : amount.copy(); 1484 if (degreeOfPolymerisation != null) { 1485 dst.degreeOfPolymerisation = new ArrayList<SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent>(); 1486 for (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent i : degreeOfPolymerisation) 1487 dst.degreeOfPolymerisation.add(i.copy()); 1488 } 1489 ; 1490 if (structuralRepresentation != null) { 1491 dst.structuralRepresentation = new ArrayList<SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent>(); 1492 for (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent i : structuralRepresentation) 1493 dst.structuralRepresentation.add(i.copy()); 1494 } 1495 ; 1496 } 1497 1498 @Override 1499 public boolean equalsDeep(Base other_) { 1500 if (!super.equalsDeep(other_)) 1501 return false; 1502 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitComponent)) 1503 return false; 1504 SubstancePolymerRepeatRepeatUnitComponent o = (SubstancePolymerRepeatRepeatUnitComponent) other_; 1505 return compareDeep(orientationOfPolymerisation, o.orientationOfPolymerisation, true) 1506 && compareDeep(repeatUnit, o.repeatUnit, true) && compareDeep(amount, o.amount, true) 1507 && compareDeep(degreeOfPolymerisation, o.degreeOfPolymerisation, true) 1508 && compareDeep(structuralRepresentation, o.structuralRepresentation, true); 1509 } 1510 1511 @Override 1512 public boolean equalsShallow(Base other_) { 1513 if (!super.equalsShallow(other_)) 1514 return false; 1515 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitComponent)) 1516 return false; 1517 SubstancePolymerRepeatRepeatUnitComponent o = (SubstancePolymerRepeatRepeatUnitComponent) other_; 1518 return compareValues(repeatUnit, o.repeatUnit, true); 1519 } 1520 1521 public boolean isEmpty() { 1522 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(orientationOfPolymerisation, repeatUnit, amount, 1523 degreeOfPolymerisation, structuralRepresentation); 1524 } 1525 1526 public String fhirType() { 1527 return "SubstancePolymer.repeat.repeatUnit"; 1528 1529 } 1530 1531 } 1532 1533 @Block() 1534 public static class SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent extends BackboneElement 1535 implements IBaseBackboneElement { 1536 /** 1537 * Todo. 1538 */ 1539 @Child(name = "degree", type = { 1540 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1541 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1542 protected CodeableConcept degree; 1543 1544 /** 1545 * Todo. 1546 */ 1547 @Child(name = "amount", type = { 1548 SubstanceAmount.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1549 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1550 protected SubstanceAmount amount; 1551 1552 private static final long serialVersionUID = -1487452773L; 1553 1554 /** 1555 * Constructor 1556 */ 1557 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent() { 1558 super(); 1559 } 1560 1561 /** 1562 * @return {@link #degree} (Todo.) 1563 */ 1564 public CodeableConcept getDegree() { 1565 if (this.degree == null) 1566 if (Configuration.errorOnAutoCreate()) 1567 throw new Error( 1568 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent.degree"); 1569 else if (Configuration.doAutoCreate()) 1570 this.degree = new CodeableConcept(); // cc 1571 return this.degree; 1572 } 1573 1574 public boolean hasDegree() { 1575 return this.degree != null && !this.degree.isEmpty(); 1576 } 1577 1578 /** 1579 * @param value {@link #degree} (Todo.) 1580 */ 1581 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setDegree(CodeableConcept value) { 1582 this.degree = value; 1583 return this; 1584 } 1585 1586 /** 1587 * @return {@link #amount} (Todo.) 1588 */ 1589 public SubstanceAmount getAmount() { 1590 if (this.amount == null) 1591 if (Configuration.errorOnAutoCreate()) 1592 throw new Error( 1593 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent.amount"); 1594 else if (Configuration.doAutoCreate()) 1595 this.amount = new SubstanceAmount(); // cc 1596 return this.amount; 1597 } 1598 1599 public boolean hasAmount() { 1600 return this.amount != null && !this.amount.isEmpty(); 1601 } 1602 1603 /** 1604 * @param value {@link #amount} (Todo.) 1605 */ 1606 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent setAmount(SubstanceAmount value) { 1607 this.amount = value; 1608 return this; 1609 } 1610 1611 protected void listChildren(List<Property> children) { 1612 super.listChildren(children); 1613 children.add(new Property("degree", "CodeableConcept", "Todo.", 0, 1, degree)); 1614 children.add(new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount)); 1615 } 1616 1617 @Override 1618 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1619 switch (_hash) { 1620 case -1335595316: 1621 /* degree */ return new Property("degree", "CodeableConcept", "Todo.", 0, 1, degree); 1622 case -1413853096: 1623 /* amount */ return new Property("amount", "SubstanceAmount", "Todo.", 0, 1, amount); 1624 default: 1625 return super.getNamedProperty(_hash, _name, _checkValid); 1626 } 1627 1628 } 1629 1630 @Override 1631 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1632 switch (hash) { 1633 case -1335595316: 1634 /* degree */ return this.degree == null ? new Base[0] : new Base[] { this.degree }; // CodeableConcept 1635 case -1413853096: 1636 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // SubstanceAmount 1637 default: 1638 return super.getProperty(hash, name, checkValid); 1639 } 1640 1641 } 1642 1643 @Override 1644 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1645 switch (hash) { 1646 case -1335595316: // degree 1647 this.degree = castToCodeableConcept(value); // CodeableConcept 1648 return value; 1649 case -1413853096: // amount 1650 this.amount = castToSubstanceAmount(value); // SubstanceAmount 1651 return value; 1652 default: 1653 return super.setProperty(hash, name, value); 1654 } 1655 1656 } 1657 1658 @Override 1659 public Base setProperty(String name, Base value) throws FHIRException { 1660 if (name.equals("degree")) { 1661 this.degree = castToCodeableConcept(value); // CodeableConcept 1662 } else if (name.equals("amount")) { 1663 this.amount = castToSubstanceAmount(value); // SubstanceAmount 1664 } else 1665 return super.setProperty(name, value); 1666 return value; 1667 } 1668 1669 @Override 1670 public Base makeProperty(int hash, String name) throws FHIRException { 1671 switch (hash) { 1672 case -1335595316: 1673 return getDegree(); 1674 case -1413853096: 1675 return getAmount(); 1676 default: 1677 return super.makeProperty(hash, name); 1678 } 1679 1680 } 1681 1682 @Override 1683 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1684 switch (hash) { 1685 case -1335595316: 1686 /* degree */ return new String[] { "CodeableConcept" }; 1687 case -1413853096: 1688 /* amount */ return new String[] { "SubstanceAmount" }; 1689 default: 1690 return super.getTypesForProperty(hash, name); 1691 } 1692 1693 } 1694 1695 @Override 1696 public Base addChild(String name) throws FHIRException { 1697 if (name.equals("degree")) { 1698 this.degree = new CodeableConcept(); 1699 return this.degree; 1700 } else if (name.equals("amount")) { 1701 this.amount = new SubstanceAmount(); 1702 return this.amount; 1703 } else 1704 return super.addChild(name); 1705 } 1706 1707 public SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent copy() { 1708 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent dst = new SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(); 1709 copyValues(dst); 1710 return dst; 1711 } 1712 1713 public void copyValues(SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent dst) { 1714 super.copyValues(dst); 1715 dst.degree = degree == null ? null : degree.copy(); 1716 dst.amount = amount == null ? null : amount.copy(); 1717 } 1718 1719 @Override 1720 public boolean equalsDeep(Base other_) { 1721 if (!super.equalsDeep(other_)) 1722 return false; 1723 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent)) 1724 return false; 1725 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent o = (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) other_; 1726 return compareDeep(degree, o.degree, true) && compareDeep(amount, o.amount, true); 1727 } 1728 1729 @Override 1730 public boolean equalsShallow(Base other_) { 1731 if (!super.equalsShallow(other_)) 1732 return false; 1733 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent)) 1734 return false; 1735 SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent o = (SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent) other_; 1736 return true; 1737 } 1738 1739 public boolean isEmpty() { 1740 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(degree, amount); 1741 } 1742 1743 public String fhirType() { 1744 return "SubstancePolymer.repeat.repeatUnit.degreeOfPolymerisation"; 1745 1746 } 1747 1748 } 1749 1750 @Block() 1751 public static class SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent extends BackboneElement 1752 implements IBaseBackboneElement { 1753 /** 1754 * Todo. 1755 */ 1756 @Child(name = "type", type = { 1757 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1758 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1759 protected CodeableConcept type; 1760 1761 /** 1762 * Todo. 1763 */ 1764 @Child(name = "representation", type = { 1765 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1766 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1767 protected StringType representation; 1768 1769 /** 1770 * Todo. 1771 */ 1772 @Child(name = "attachment", type = { 1773 Attachment.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1774 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1775 protected Attachment attachment; 1776 1777 private static final long serialVersionUID = 167954495L; 1778 1779 /** 1780 * Constructor 1781 */ 1782 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent() { 1783 super(); 1784 } 1785 1786 /** 1787 * @return {@link #type} (Todo.) 1788 */ 1789 public CodeableConcept getType() { 1790 if (this.type == null) 1791 if (Configuration.errorOnAutoCreate()) 1792 throw new Error( 1793 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.type"); 1794 else if (Configuration.doAutoCreate()) 1795 this.type = new CodeableConcept(); // cc 1796 return this.type; 1797 } 1798 1799 public boolean hasType() { 1800 return this.type != null && !this.type.isEmpty(); 1801 } 1802 1803 /** 1804 * @param value {@link #type} (Todo.) 1805 */ 1806 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setType(CodeableConcept value) { 1807 this.type = value; 1808 return this; 1809 } 1810 1811 /** 1812 * @return {@link #representation} (Todo.). This is the underlying object with 1813 * id, value and extensions. The accessor "getRepresentation" gives 1814 * direct access to the value 1815 */ 1816 public StringType getRepresentationElement() { 1817 if (this.representation == null) 1818 if (Configuration.errorOnAutoCreate()) 1819 throw new Error( 1820 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.representation"); 1821 else if (Configuration.doAutoCreate()) 1822 this.representation = new StringType(); // bb 1823 return this.representation; 1824 } 1825 1826 public boolean hasRepresentationElement() { 1827 return this.representation != null && !this.representation.isEmpty(); 1828 } 1829 1830 public boolean hasRepresentation() { 1831 return this.representation != null && !this.representation.isEmpty(); 1832 } 1833 1834 /** 1835 * @param value {@link #representation} (Todo.). This is the underlying object 1836 * with id, value and extensions. The accessor "getRepresentation" 1837 * gives direct access to the value 1838 */ 1839 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setRepresentationElement( 1840 StringType value) { 1841 this.representation = value; 1842 return this; 1843 } 1844 1845 /** 1846 * @return Todo. 1847 */ 1848 public String getRepresentation() { 1849 return this.representation == null ? null : this.representation.getValue(); 1850 } 1851 1852 /** 1853 * @param value Todo. 1854 */ 1855 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setRepresentation(String value) { 1856 if (Utilities.noString(value)) 1857 this.representation = null; 1858 else { 1859 if (this.representation == null) 1860 this.representation = new StringType(); 1861 this.representation.setValue(value); 1862 } 1863 return this; 1864 } 1865 1866 /** 1867 * @return {@link #attachment} (Todo.) 1868 */ 1869 public Attachment getAttachment() { 1870 if (this.attachment == null) 1871 if (Configuration.errorOnAutoCreate()) 1872 throw new Error( 1873 "Attempt to auto-create SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent.attachment"); 1874 else if (Configuration.doAutoCreate()) 1875 this.attachment = new Attachment(); // cc 1876 return this.attachment; 1877 } 1878 1879 public boolean hasAttachment() { 1880 return this.attachment != null && !this.attachment.isEmpty(); 1881 } 1882 1883 /** 1884 * @param value {@link #attachment} (Todo.) 1885 */ 1886 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent setAttachment(Attachment value) { 1887 this.attachment = value; 1888 return this; 1889 } 1890 1891 protected void listChildren(List<Property> children) { 1892 super.listChildren(children); 1893 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 1894 children.add(new Property("representation", "string", "Todo.", 0, 1, representation)); 1895 children.add(new Property("attachment", "Attachment", "Todo.", 0, 1, attachment)); 1896 } 1897 1898 @Override 1899 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1900 switch (_hash) { 1901 case 3575610: 1902 /* type */ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 1903 case -671065907: 1904 /* representation */ return new Property("representation", "string", "Todo.", 0, 1, representation); 1905 case -1963501277: 1906 /* attachment */ return new Property("attachment", "Attachment", "Todo.", 0, 1, attachment); 1907 default: 1908 return super.getNamedProperty(_hash, _name, _checkValid); 1909 } 1910 1911 } 1912 1913 @Override 1914 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1915 switch (hash) { 1916 case 3575610: 1917 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1918 case -671065907: 1919 /* representation */ return this.representation == null ? new Base[0] : new Base[] { this.representation }; // StringType 1920 case -1963501277: 1921 /* attachment */ return this.attachment == null ? new Base[0] : new Base[] { this.attachment }; // Attachment 1922 default: 1923 return super.getProperty(hash, name, checkValid); 1924 } 1925 1926 } 1927 1928 @Override 1929 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1930 switch (hash) { 1931 case 3575610: // type 1932 this.type = castToCodeableConcept(value); // CodeableConcept 1933 return value; 1934 case -671065907: // representation 1935 this.representation = castToString(value); // StringType 1936 return value; 1937 case -1963501277: // attachment 1938 this.attachment = castToAttachment(value); // Attachment 1939 return value; 1940 default: 1941 return super.setProperty(hash, name, value); 1942 } 1943 1944 } 1945 1946 @Override 1947 public Base setProperty(String name, Base value) throws FHIRException { 1948 if (name.equals("type")) { 1949 this.type = castToCodeableConcept(value); // CodeableConcept 1950 } else if (name.equals("representation")) { 1951 this.representation = castToString(value); // StringType 1952 } else if (name.equals("attachment")) { 1953 this.attachment = castToAttachment(value); // Attachment 1954 } else 1955 return super.setProperty(name, value); 1956 return value; 1957 } 1958 1959 @Override 1960 public Base makeProperty(int hash, String name) throws FHIRException { 1961 switch (hash) { 1962 case 3575610: 1963 return getType(); 1964 case -671065907: 1965 return getRepresentationElement(); 1966 case -1963501277: 1967 return getAttachment(); 1968 default: 1969 return super.makeProperty(hash, name); 1970 } 1971 1972 } 1973 1974 @Override 1975 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1976 switch (hash) { 1977 case 3575610: 1978 /* type */ return new String[] { "CodeableConcept" }; 1979 case -671065907: 1980 /* representation */ return new String[] { "string" }; 1981 case -1963501277: 1982 /* attachment */ return new String[] { "Attachment" }; 1983 default: 1984 return super.getTypesForProperty(hash, name); 1985 } 1986 1987 } 1988 1989 @Override 1990 public Base addChild(String name) throws FHIRException { 1991 if (name.equals("type")) { 1992 this.type = new CodeableConcept(); 1993 return this.type; 1994 } else if (name.equals("representation")) { 1995 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.representation"); 1996 } else if (name.equals("attachment")) { 1997 this.attachment = new Attachment(); 1998 return this.attachment; 1999 } else 2000 return super.addChild(name); 2001 } 2002 2003 public SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent copy() { 2004 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent dst = new SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(); 2005 copyValues(dst); 2006 return dst; 2007 } 2008 2009 public void copyValues(SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent dst) { 2010 super.copyValues(dst); 2011 dst.type = type == null ? null : type.copy(); 2012 dst.representation = representation == null ? null : representation.copy(); 2013 dst.attachment = attachment == null ? null : attachment.copy(); 2014 } 2015 2016 @Override 2017 public boolean equalsDeep(Base other_) { 2018 if (!super.equalsDeep(other_)) 2019 return false; 2020 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent)) 2021 return false; 2022 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent o = (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) other_; 2023 return compareDeep(type, o.type, true) && compareDeep(representation, o.representation, true) 2024 && compareDeep(attachment, o.attachment, true); 2025 } 2026 2027 @Override 2028 public boolean equalsShallow(Base other_) { 2029 if (!super.equalsShallow(other_)) 2030 return false; 2031 if (!(other_ instanceof SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent)) 2032 return false; 2033 SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent o = (SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent) other_; 2034 return compareValues(representation, o.representation, true); 2035 } 2036 2037 public boolean isEmpty() { 2038 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, representation, attachment); 2039 } 2040 2041 public String fhirType() { 2042 return "SubstancePolymer.repeat.repeatUnit.structuralRepresentation"; 2043 2044 } 2045 2046 } 2047 2048 /** 2049 * Todo. 2050 */ 2051 @Child(name = "class", type = { 2052 CodeableConcept.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 2053 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2054 protected CodeableConcept class_; 2055 2056 /** 2057 * Todo. 2058 */ 2059 @Child(name = "geometry", type = { 2060 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2061 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2062 protected CodeableConcept geometry; 2063 2064 /** 2065 * Todo. 2066 */ 2067 @Child(name = "copolymerConnectivity", type = { 2068 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2069 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2070 protected List<CodeableConcept> copolymerConnectivity; 2071 2072 /** 2073 * Todo. 2074 */ 2075 @Child(name = "modification", type = { 2076 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2077 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2078 protected List<StringType> modification; 2079 2080 /** 2081 * Todo. 2082 */ 2083 @Child(name = "monomerSet", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2084 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2085 protected List<SubstancePolymerMonomerSetComponent> monomerSet; 2086 2087 /** 2088 * Todo. 2089 */ 2090 @Child(name = "repeat", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2091 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 2092 protected List<SubstancePolymerRepeatComponent> repeat; 2093 2094 private static final long serialVersionUID = -58301650L; 2095 2096 /** 2097 * Constructor 2098 */ 2099 public SubstancePolymer() { 2100 super(); 2101 } 2102 2103 /** 2104 * @return {@link #class_} (Todo.) 2105 */ 2106 public CodeableConcept getClass_() { 2107 if (this.class_ == null) 2108 if (Configuration.errorOnAutoCreate()) 2109 throw new Error("Attempt to auto-create SubstancePolymer.class_"); 2110 else if (Configuration.doAutoCreate()) 2111 this.class_ = new CodeableConcept(); // cc 2112 return this.class_; 2113 } 2114 2115 public boolean hasClass_() { 2116 return this.class_ != null && !this.class_.isEmpty(); 2117 } 2118 2119 /** 2120 * @param value {@link #class_} (Todo.) 2121 */ 2122 public SubstancePolymer setClass_(CodeableConcept value) { 2123 this.class_ = value; 2124 return this; 2125 } 2126 2127 /** 2128 * @return {@link #geometry} (Todo.) 2129 */ 2130 public CodeableConcept getGeometry() { 2131 if (this.geometry == null) 2132 if (Configuration.errorOnAutoCreate()) 2133 throw new Error("Attempt to auto-create SubstancePolymer.geometry"); 2134 else if (Configuration.doAutoCreate()) 2135 this.geometry = new CodeableConcept(); // cc 2136 return this.geometry; 2137 } 2138 2139 public boolean hasGeometry() { 2140 return this.geometry != null && !this.geometry.isEmpty(); 2141 } 2142 2143 /** 2144 * @param value {@link #geometry} (Todo.) 2145 */ 2146 public SubstancePolymer setGeometry(CodeableConcept value) { 2147 this.geometry = value; 2148 return this; 2149 } 2150 2151 /** 2152 * @return {@link #copolymerConnectivity} (Todo.) 2153 */ 2154 public List<CodeableConcept> getCopolymerConnectivity() { 2155 if (this.copolymerConnectivity == null) 2156 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2157 return this.copolymerConnectivity; 2158 } 2159 2160 /** 2161 * @return Returns a reference to <code>this</code> for easy method chaining 2162 */ 2163 public SubstancePolymer setCopolymerConnectivity(List<CodeableConcept> theCopolymerConnectivity) { 2164 this.copolymerConnectivity = theCopolymerConnectivity; 2165 return this; 2166 } 2167 2168 public boolean hasCopolymerConnectivity() { 2169 if (this.copolymerConnectivity == null) 2170 return false; 2171 for (CodeableConcept item : this.copolymerConnectivity) 2172 if (!item.isEmpty()) 2173 return true; 2174 return false; 2175 } 2176 2177 public CodeableConcept addCopolymerConnectivity() { // 3 2178 CodeableConcept t = new CodeableConcept(); 2179 if (this.copolymerConnectivity == null) 2180 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2181 this.copolymerConnectivity.add(t); 2182 return t; 2183 } 2184 2185 public SubstancePolymer addCopolymerConnectivity(CodeableConcept t) { // 3 2186 if (t == null) 2187 return this; 2188 if (this.copolymerConnectivity == null) 2189 this.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2190 this.copolymerConnectivity.add(t); 2191 return this; 2192 } 2193 2194 /** 2195 * @return The first repetition of repeating field 2196 * {@link #copolymerConnectivity}, creating it if it does not already 2197 * exist 2198 */ 2199 public CodeableConcept getCopolymerConnectivityFirstRep() { 2200 if (getCopolymerConnectivity().isEmpty()) { 2201 addCopolymerConnectivity(); 2202 } 2203 return getCopolymerConnectivity().get(0); 2204 } 2205 2206 /** 2207 * @return {@link #modification} (Todo.) 2208 */ 2209 public List<StringType> getModification() { 2210 if (this.modification == null) 2211 this.modification = new ArrayList<StringType>(); 2212 return this.modification; 2213 } 2214 2215 /** 2216 * @return Returns a reference to <code>this</code> for easy method chaining 2217 */ 2218 public SubstancePolymer setModification(List<StringType> theModification) { 2219 this.modification = theModification; 2220 return this; 2221 } 2222 2223 public boolean hasModification() { 2224 if (this.modification == null) 2225 return false; 2226 for (StringType item : this.modification) 2227 if (!item.isEmpty()) 2228 return true; 2229 return false; 2230 } 2231 2232 /** 2233 * @return {@link #modification} (Todo.) 2234 */ 2235 public StringType addModificationElement() {// 2 2236 StringType t = new StringType(); 2237 if (this.modification == null) 2238 this.modification = new ArrayList<StringType>(); 2239 this.modification.add(t); 2240 return t; 2241 } 2242 2243 /** 2244 * @param value {@link #modification} (Todo.) 2245 */ 2246 public SubstancePolymer addModification(String value) { // 1 2247 StringType t = new StringType(); 2248 t.setValue(value); 2249 if (this.modification == null) 2250 this.modification = new ArrayList<StringType>(); 2251 this.modification.add(t); 2252 return this; 2253 } 2254 2255 /** 2256 * @param value {@link #modification} (Todo.) 2257 */ 2258 public boolean hasModification(String value) { 2259 if (this.modification == null) 2260 return false; 2261 for (StringType v : this.modification) 2262 if (v.getValue().equals(value)) // string 2263 return true; 2264 return false; 2265 } 2266 2267 /** 2268 * @return {@link #monomerSet} (Todo.) 2269 */ 2270 public List<SubstancePolymerMonomerSetComponent> getMonomerSet() { 2271 if (this.monomerSet == null) 2272 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2273 return this.monomerSet; 2274 } 2275 2276 /** 2277 * @return Returns a reference to <code>this</code> for easy method chaining 2278 */ 2279 public SubstancePolymer setMonomerSet(List<SubstancePolymerMonomerSetComponent> theMonomerSet) { 2280 this.monomerSet = theMonomerSet; 2281 return this; 2282 } 2283 2284 public boolean hasMonomerSet() { 2285 if (this.monomerSet == null) 2286 return false; 2287 for (SubstancePolymerMonomerSetComponent item : this.monomerSet) 2288 if (!item.isEmpty()) 2289 return true; 2290 return false; 2291 } 2292 2293 public SubstancePolymerMonomerSetComponent addMonomerSet() { // 3 2294 SubstancePolymerMonomerSetComponent t = new SubstancePolymerMonomerSetComponent(); 2295 if (this.monomerSet == null) 2296 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2297 this.monomerSet.add(t); 2298 return t; 2299 } 2300 2301 public SubstancePolymer addMonomerSet(SubstancePolymerMonomerSetComponent t) { // 3 2302 if (t == null) 2303 return this; 2304 if (this.monomerSet == null) 2305 this.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2306 this.monomerSet.add(t); 2307 return this; 2308 } 2309 2310 /** 2311 * @return The first repetition of repeating field {@link #monomerSet}, creating 2312 * it if it does not already exist 2313 */ 2314 public SubstancePolymerMonomerSetComponent getMonomerSetFirstRep() { 2315 if (getMonomerSet().isEmpty()) { 2316 addMonomerSet(); 2317 } 2318 return getMonomerSet().get(0); 2319 } 2320 2321 /** 2322 * @return {@link #repeat} (Todo.) 2323 */ 2324 public List<SubstancePolymerRepeatComponent> getRepeat() { 2325 if (this.repeat == null) 2326 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2327 return this.repeat; 2328 } 2329 2330 /** 2331 * @return Returns a reference to <code>this</code> for easy method chaining 2332 */ 2333 public SubstancePolymer setRepeat(List<SubstancePolymerRepeatComponent> theRepeat) { 2334 this.repeat = theRepeat; 2335 return this; 2336 } 2337 2338 public boolean hasRepeat() { 2339 if (this.repeat == null) 2340 return false; 2341 for (SubstancePolymerRepeatComponent item : this.repeat) 2342 if (!item.isEmpty()) 2343 return true; 2344 return false; 2345 } 2346 2347 public SubstancePolymerRepeatComponent addRepeat() { // 3 2348 SubstancePolymerRepeatComponent t = new SubstancePolymerRepeatComponent(); 2349 if (this.repeat == null) 2350 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2351 this.repeat.add(t); 2352 return t; 2353 } 2354 2355 public SubstancePolymer addRepeat(SubstancePolymerRepeatComponent t) { // 3 2356 if (t == null) 2357 return this; 2358 if (this.repeat == null) 2359 this.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2360 this.repeat.add(t); 2361 return this; 2362 } 2363 2364 /** 2365 * @return The first repetition of repeating field {@link #repeat}, creating it 2366 * if it does not already exist 2367 */ 2368 public SubstancePolymerRepeatComponent getRepeatFirstRep() { 2369 if (getRepeat().isEmpty()) { 2370 addRepeat(); 2371 } 2372 return getRepeat().get(0); 2373 } 2374 2375 protected void listChildren(List<Property> children) { 2376 super.listChildren(children); 2377 children.add(new Property("class", "CodeableConcept", "Todo.", 0, 1, class_)); 2378 children.add(new Property("geometry", "CodeableConcept", "Todo.", 0, 1, geometry)); 2379 children.add(new Property("copolymerConnectivity", "CodeableConcept", "Todo.", 0, java.lang.Integer.MAX_VALUE, 2380 copolymerConnectivity)); 2381 children.add(new Property("modification", "string", "Todo.", 0, java.lang.Integer.MAX_VALUE, modification)); 2382 children.add(new Property("monomerSet", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, monomerSet)); 2383 children.add(new Property("repeat", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, repeat)); 2384 } 2385 2386 @Override 2387 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2388 switch (_hash) { 2389 case 94742904: 2390 /* class */ return new Property("class", "CodeableConcept", "Todo.", 0, 1, class_); 2391 case 1846020210: 2392 /* geometry */ return new Property("geometry", "CodeableConcept", "Todo.", 0, 1, geometry); 2393 case 997107577: 2394 /* copolymerConnectivity */ return new Property("copolymerConnectivity", "CodeableConcept", "Todo.", 0, 2395 java.lang.Integer.MAX_VALUE, copolymerConnectivity); 2396 case -684600932: 2397 /* modification */ return new Property("modification", "string", "Todo.", 0, java.lang.Integer.MAX_VALUE, 2398 modification); 2399 case -1622483765: 2400 /* monomerSet */ return new Property("monomerSet", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, monomerSet); 2401 case -934531685: 2402 /* repeat */ return new Property("repeat", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, repeat); 2403 default: 2404 return super.getNamedProperty(_hash, _name, _checkValid); 2405 } 2406 2407 } 2408 2409 @Override 2410 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2411 switch (hash) { 2412 case 94742904: 2413 /* class */ return this.class_ == null ? new Base[0] : new Base[] { this.class_ }; // CodeableConcept 2414 case 1846020210: 2415 /* geometry */ return this.geometry == null ? new Base[0] : new Base[] { this.geometry }; // CodeableConcept 2416 case 997107577: 2417 /* copolymerConnectivity */ return this.copolymerConnectivity == null ? new Base[0] 2418 : this.copolymerConnectivity.toArray(new Base[this.copolymerConnectivity.size()]); // CodeableConcept 2419 case -684600932: 2420 /* modification */ return this.modification == null ? new Base[0] 2421 : this.modification.toArray(new Base[this.modification.size()]); // StringType 2422 case -1622483765: 2423 /* monomerSet */ return this.monomerSet == null ? new Base[0] 2424 : this.monomerSet.toArray(new Base[this.monomerSet.size()]); // SubstancePolymerMonomerSetComponent 2425 case -934531685: 2426 /* repeat */ return this.repeat == null ? new Base[0] : this.repeat.toArray(new Base[this.repeat.size()]); // SubstancePolymerRepeatComponent 2427 default: 2428 return super.getProperty(hash, name, checkValid); 2429 } 2430 2431 } 2432 2433 @Override 2434 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2435 switch (hash) { 2436 case 94742904: // class 2437 this.class_ = castToCodeableConcept(value); // CodeableConcept 2438 return value; 2439 case 1846020210: // geometry 2440 this.geometry = castToCodeableConcept(value); // CodeableConcept 2441 return value; 2442 case 997107577: // copolymerConnectivity 2443 this.getCopolymerConnectivity().add(castToCodeableConcept(value)); // CodeableConcept 2444 return value; 2445 case -684600932: // modification 2446 this.getModification().add(castToString(value)); // StringType 2447 return value; 2448 case -1622483765: // monomerSet 2449 this.getMonomerSet().add((SubstancePolymerMonomerSetComponent) value); // SubstancePolymerMonomerSetComponent 2450 return value; 2451 case -934531685: // repeat 2452 this.getRepeat().add((SubstancePolymerRepeatComponent) value); // SubstancePolymerRepeatComponent 2453 return value; 2454 default: 2455 return super.setProperty(hash, name, value); 2456 } 2457 2458 } 2459 2460 @Override 2461 public Base setProperty(String name, Base value) throws FHIRException { 2462 if (name.equals("class")) { 2463 this.class_ = castToCodeableConcept(value); // CodeableConcept 2464 } else if (name.equals("geometry")) { 2465 this.geometry = castToCodeableConcept(value); // CodeableConcept 2466 } else if (name.equals("copolymerConnectivity")) { 2467 this.getCopolymerConnectivity().add(castToCodeableConcept(value)); 2468 } else if (name.equals("modification")) { 2469 this.getModification().add(castToString(value)); 2470 } else if (name.equals("monomerSet")) { 2471 this.getMonomerSet().add((SubstancePolymerMonomerSetComponent) value); 2472 } else if (name.equals("repeat")) { 2473 this.getRepeat().add((SubstancePolymerRepeatComponent) value); 2474 } else 2475 return super.setProperty(name, value); 2476 return value; 2477 } 2478 2479 @Override 2480 public Base makeProperty(int hash, String name) throws FHIRException { 2481 switch (hash) { 2482 case 94742904: 2483 return getClass_(); 2484 case 1846020210: 2485 return getGeometry(); 2486 case 997107577: 2487 return addCopolymerConnectivity(); 2488 case -684600932: 2489 return addModificationElement(); 2490 case -1622483765: 2491 return addMonomerSet(); 2492 case -934531685: 2493 return addRepeat(); 2494 default: 2495 return super.makeProperty(hash, name); 2496 } 2497 2498 } 2499 2500 @Override 2501 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2502 switch (hash) { 2503 case 94742904: 2504 /* class */ return new String[] { "CodeableConcept" }; 2505 case 1846020210: 2506 /* geometry */ return new String[] { "CodeableConcept" }; 2507 case 997107577: 2508 /* copolymerConnectivity */ return new String[] { "CodeableConcept" }; 2509 case -684600932: 2510 /* modification */ return new String[] { "string" }; 2511 case -1622483765: 2512 /* monomerSet */ return new String[] {}; 2513 case -934531685: 2514 /* repeat */ return new String[] {}; 2515 default: 2516 return super.getTypesForProperty(hash, name); 2517 } 2518 2519 } 2520 2521 @Override 2522 public Base addChild(String name) throws FHIRException { 2523 if (name.equals("class")) { 2524 this.class_ = new CodeableConcept(); 2525 return this.class_; 2526 } else if (name.equals("geometry")) { 2527 this.geometry = new CodeableConcept(); 2528 return this.geometry; 2529 } else if (name.equals("copolymerConnectivity")) { 2530 return addCopolymerConnectivity(); 2531 } else if (name.equals("modification")) { 2532 throw new FHIRException("Cannot call addChild on a singleton property SubstancePolymer.modification"); 2533 } else if (name.equals("monomerSet")) { 2534 return addMonomerSet(); 2535 } else if (name.equals("repeat")) { 2536 return addRepeat(); 2537 } else 2538 return super.addChild(name); 2539 } 2540 2541 public String fhirType() { 2542 return "SubstancePolymer"; 2543 2544 } 2545 2546 public SubstancePolymer copy() { 2547 SubstancePolymer dst = new SubstancePolymer(); 2548 copyValues(dst); 2549 return dst; 2550 } 2551 2552 public void copyValues(SubstancePolymer dst) { 2553 super.copyValues(dst); 2554 dst.class_ = class_ == null ? null : class_.copy(); 2555 dst.geometry = geometry == null ? null : geometry.copy(); 2556 if (copolymerConnectivity != null) { 2557 dst.copolymerConnectivity = new ArrayList<CodeableConcept>(); 2558 for (CodeableConcept i : copolymerConnectivity) 2559 dst.copolymerConnectivity.add(i.copy()); 2560 } 2561 ; 2562 if (modification != null) { 2563 dst.modification = new ArrayList<StringType>(); 2564 for (StringType i : modification) 2565 dst.modification.add(i.copy()); 2566 } 2567 ; 2568 if (monomerSet != null) { 2569 dst.monomerSet = new ArrayList<SubstancePolymerMonomerSetComponent>(); 2570 for (SubstancePolymerMonomerSetComponent i : monomerSet) 2571 dst.monomerSet.add(i.copy()); 2572 } 2573 ; 2574 if (repeat != null) { 2575 dst.repeat = new ArrayList<SubstancePolymerRepeatComponent>(); 2576 for (SubstancePolymerRepeatComponent i : repeat) 2577 dst.repeat.add(i.copy()); 2578 } 2579 ; 2580 } 2581 2582 protected SubstancePolymer typedCopy() { 2583 return copy(); 2584 } 2585 2586 @Override 2587 public boolean equalsDeep(Base other_) { 2588 if (!super.equalsDeep(other_)) 2589 return false; 2590 if (!(other_ instanceof SubstancePolymer)) 2591 return false; 2592 SubstancePolymer o = (SubstancePolymer) other_; 2593 return compareDeep(class_, o.class_, true) && compareDeep(geometry, o.geometry, true) 2594 && compareDeep(copolymerConnectivity, o.copolymerConnectivity, true) 2595 && compareDeep(modification, o.modification, true) && compareDeep(monomerSet, o.monomerSet, true) 2596 && compareDeep(repeat, o.repeat, true); 2597 } 2598 2599 @Override 2600 public boolean equalsShallow(Base other_) { 2601 if (!super.equalsShallow(other_)) 2602 return false; 2603 if (!(other_ instanceof SubstancePolymer)) 2604 return false; 2605 SubstancePolymer o = (SubstancePolymer) other_; 2606 return compareValues(modification, o.modification, true); 2607 } 2608 2609 public boolean isEmpty() { 2610 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(class_, geometry, copolymerConnectivity, 2611 modification, monomerSet, repeat); 2612 } 2613 2614 @Override 2615 public ResourceType getResourceType() { 2616 return ResourceType.SubstancePolymer; 2617 } 2618 2619}