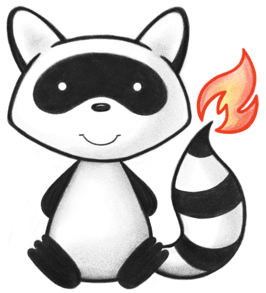
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044 045/** 046 * A SubstanceProtein is defined as a single unit of a linear amino acid 047 * sequence, or a combination of subunits that are either covalently linked or 048 * have a defined invariant stoichiometric relationship. This includes all 049 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 050 * whether the use is therapeutic or prophylactic. This set of elements will be 051 * used to describe albumins, coagulation factors, cytokines, growth factors, 052 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 053 * vaccines, and immunomodulators. 054 */ 055@ResourceDef(name = "SubstanceProtein", profile = "http://hl7.org/fhir/StructureDefinition/SubstanceProtein") 056public class SubstanceProtein extends DomainResource { 057 058 @Block() 059 public static class SubstanceProteinSubunitComponent extends BackboneElement implements IBaseBackboneElement { 060 /** 061 * Index of primary sequences of amino acids linked through peptide bonds in 062 * order of decreasing length. Sequences of the same length will be ordered by 063 * molecular weight. Subunits that have identical sequences will be repeated and 064 * have sequential subscripts. 065 */ 066 @Child(name = "subunit", type = { 067 IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts", formalDefinition = "Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.") 069 protected IntegerType subunit; 070 071 /** 072 * The sequence information shall be provided enumerating the amino acids from 073 * N- to C-terminal end using standard single-letter amino acid codes. Uppercase 074 * shall be used for L-amino acids and lowercase for D-amino acids. Transcribed 075 * SubstanceProteins will always be described using the translated sequence; for 076 * synthetic peptide containing amino acids that are not represented with a 077 * single letter code an X should be used within the sequence. The modified 078 * amino acids will be distinguished by their position in the sequence. 079 */ 080 @Child(name = "sequence", type = { 081 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 082 @Description(shortDefinition = "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence", formalDefinition = "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.") 083 protected StringType sequence; 084 085 /** 086 * Length of linear sequences of amino acids contained in the subunit. 087 */ 088 @Child(name = "length", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 089 @Description(shortDefinition = "Length of linear sequences of amino acids contained in the subunit", formalDefinition = "Length of linear sequences of amino acids contained in the subunit.") 090 protected IntegerType length; 091 092 /** 093 * The sequence information shall be provided enumerating the amino acids from 094 * N- to C-terminal end using standard single-letter amino acid codes. Uppercase 095 * shall be used for L-amino acids and lowercase for D-amino acids. Transcribed 096 * SubstanceProteins will always be described using the translated sequence; for 097 * synthetic peptide containing amino acids that are not represented with a 098 * single letter code an X should be used within the sequence. The modified 099 * amino acids will be distinguished by their position in the sequence. 100 */ 101 @Child(name = "sequenceAttachment", type = { 102 Attachment.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 103 @Description(shortDefinition = "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence", formalDefinition = "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.") 104 protected Attachment sequenceAttachment; 105 106 /** 107 * Unique identifier for molecular fragment modification based on the ISO 11238 108 * Substance ID. 109 */ 110 @Child(name = "nTerminalModificationId", type = { 111 Identifier.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 112 @Description(shortDefinition = "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID", formalDefinition = "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.") 113 protected Identifier nTerminalModificationId; 114 115 /** 116 * The name of the fragment modified at the N-terminal of the SubstanceProtein 117 * shall be specified. 118 */ 119 @Child(name = "nTerminalModification", type = { 120 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 121 @Description(shortDefinition = "The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified", formalDefinition = "The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified.") 122 protected StringType nTerminalModification; 123 124 /** 125 * Unique identifier for molecular fragment modification based on the ISO 11238 126 * Substance ID. 127 */ 128 @Child(name = "cTerminalModificationId", type = { 129 Identifier.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 130 @Description(shortDefinition = "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID", formalDefinition = "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.") 131 protected Identifier cTerminalModificationId; 132 133 /** 134 * The modification at the C-terminal shall be specified. 135 */ 136 @Child(name = "cTerminalModification", type = { 137 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 138 @Description(shortDefinition = "The modification at the C-terminal shall be specified", formalDefinition = "The modification at the C-terminal shall be specified.") 139 protected StringType cTerminalModification; 140 141 private static final long serialVersionUID = 99973841L; 142 143 /** 144 * Constructor 145 */ 146 public SubstanceProteinSubunitComponent() { 147 super(); 148 } 149 150 /** 151 * @return {@link #subunit} (Index of primary sequences of amino acids linked 152 * through peptide bonds in order of decreasing length. Sequences of the 153 * same length will be ordered by molecular weight. Subunits that have 154 * identical sequences will be repeated and have sequential 155 * subscripts.). This is the underlying object with id, value and 156 * extensions. The accessor "getSubunit" gives direct access to the 157 * value 158 */ 159 public IntegerType getSubunitElement() { 160 if (this.subunit == null) 161 if (Configuration.errorOnAutoCreate()) 162 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.subunit"); 163 else if (Configuration.doAutoCreate()) 164 this.subunit = new IntegerType(); // bb 165 return this.subunit; 166 } 167 168 public boolean hasSubunitElement() { 169 return this.subunit != null && !this.subunit.isEmpty(); 170 } 171 172 public boolean hasSubunit() { 173 return this.subunit != null && !this.subunit.isEmpty(); 174 } 175 176 /** 177 * @param value {@link #subunit} (Index of primary sequences of amino acids 178 * linked through peptide bonds in order of decreasing length. 179 * Sequences of the same length will be ordered by molecular 180 * weight. Subunits that have identical sequences will be repeated 181 * and have sequential subscripts.). This is the underlying object 182 * with id, value and extensions. The accessor "getSubunit" gives 183 * direct access to the value 184 */ 185 public SubstanceProteinSubunitComponent setSubunitElement(IntegerType value) { 186 this.subunit = value; 187 return this; 188 } 189 190 /** 191 * @return Index of primary sequences of amino acids linked through peptide 192 * bonds in order of decreasing length. Sequences of the same length 193 * will be ordered by molecular weight. Subunits that have identical 194 * sequences will be repeated and have sequential subscripts. 195 */ 196 public int getSubunit() { 197 return this.subunit == null || this.subunit.isEmpty() ? 0 : this.subunit.getValue(); 198 } 199 200 /** 201 * @param value Index of primary sequences of amino acids linked through peptide 202 * bonds in order of decreasing length. Sequences of the same 203 * length will be ordered by molecular weight. Subunits that have 204 * identical sequences will be repeated and have sequential 205 * subscripts. 206 */ 207 public SubstanceProteinSubunitComponent setSubunit(int value) { 208 if (this.subunit == null) 209 this.subunit = new IntegerType(); 210 this.subunit.setValue(value); 211 return this; 212 } 213 214 /** 215 * @return {@link #sequence} (The sequence information shall be provided 216 * enumerating the amino acids from N- to C-terminal end using standard 217 * single-letter amino acid codes. Uppercase shall be used for L-amino 218 * acids and lowercase for D-amino acids. Transcribed SubstanceProteins 219 * will always be described using the translated sequence; for synthetic 220 * peptide containing amino acids that are not represented with a single 221 * letter code an X should be used within the sequence. The modified 222 * amino acids will be distinguished by their position in the 223 * sequence.). This is the underlying object with id, value and 224 * extensions. The accessor "getSequence" gives direct access to the 225 * value 226 */ 227 public StringType getSequenceElement() { 228 if (this.sequence == null) 229 if (Configuration.errorOnAutoCreate()) 230 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.sequence"); 231 else if (Configuration.doAutoCreate()) 232 this.sequence = new StringType(); // bb 233 return this.sequence; 234 } 235 236 public boolean hasSequenceElement() { 237 return this.sequence != null && !this.sequence.isEmpty(); 238 } 239 240 public boolean hasSequence() { 241 return this.sequence != null && !this.sequence.isEmpty(); 242 } 243 244 /** 245 * @param value {@link #sequence} (The sequence information shall be provided 246 * enumerating the amino acids from N- to C-terminal end using 247 * standard single-letter amino acid codes. Uppercase shall be used 248 * for L-amino acids and lowercase for D-amino acids. Transcribed 249 * SubstanceProteins will always be described using the translated 250 * sequence; for synthetic peptide containing amino acids that are 251 * not represented with a single letter code an X should be used 252 * within the sequence. The modified amino acids will be 253 * distinguished by their position in the sequence.). This is the 254 * underlying object with id, value and extensions. The accessor 255 * "getSequence" gives direct access to the value 256 */ 257 public SubstanceProteinSubunitComponent setSequenceElement(StringType value) { 258 this.sequence = value; 259 return this; 260 } 261 262 /** 263 * @return The sequence information shall be provided enumerating the amino 264 * acids from N- to C-terminal end using standard single-letter amino 265 * acid codes. Uppercase shall be used for L-amino acids and lowercase 266 * for D-amino acids. Transcribed SubstanceProteins will always be 267 * described using the translated sequence; for synthetic peptide 268 * containing amino acids that are not represented with a single letter 269 * code an X should be used within the sequence. The modified amino 270 * acids will be distinguished by their position in the sequence. 271 */ 272 public String getSequence() { 273 return this.sequence == null ? null : this.sequence.getValue(); 274 } 275 276 /** 277 * @param value The sequence information shall be provided enumerating the amino 278 * acids from N- to C-terminal end using standard single-letter 279 * amino acid codes. Uppercase shall be used for L-amino acids and 280 * lowercase for D-amino acids. Transcribed SubstanceProteins will 281 * always be described using the translated sequence; for synthetic 282 * peptide containing amino acids that are not represented with a 283 * single letter code an X should be used within the sequence. The 284 * modified amino acids will be distinguished by their position in 285 * the sequence. 286 */ 287 public SubstanceProteinSubunitComponent setSequence(String value) { 288 if (Utilities.noString(value)) 289 this.sequence = null; 290 else { 291 if (this.sequence == null) 292 this.sequence = new StringType(); 293 this.sequence.setValue(value); 294 } 295 return this; 296 } 297 298 /** 299 * @return {@link #length} (Length of linear sequences of amino acids contained 300 * in the subunit.). This is the underlying object with id, value and 301 * extensions. The accessor "getLength" gives direct access to the value 302 */ 303 public IntegerType getLengthElement() { 304 if (this.length == null) 305 if (Configuration.errorOnAutoCreate()) 306 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.length"); 307 else if (Configuration.doAutoCreate()) 308 this.length = new IntegerType(); // bb 309 return this.length; 310 } 311 312 public boolean hasLengthElement() { 313 return this.length != null && !this.length.isEmpty(); 314 } 315 316 public boolean hasLength() { 317 return this.length != null && !this.length.isEmpty(); 318 } 319 320 /** 321 * @param value {@link #length} (Length of linear sequences of amino acids 322 * contained in the subunit.). This is the underlying object with 323 * id, value and extensions. The accessor "getLength" gives direct 324 * access to the value 325 */ 326 public SubstanceProteinSubunitComponent setLengthElement(IntegerType value) { 327 this.length = value; 328 return this; 329 } 330 331 /** 332 * @return Length of linear sequences of amino acids contained in the subunit. 333 */ 334 public int getLength() { 335 return this.length == null || this.length.isEmpty() ? 0 : this.length.getValue(); 336 } 337 338 /** 339 * @param value Length of linear sequences of amino acids contained in the 340 * subunit. 341 */ 342 public SubstanceProteinSubunitComponent setLength(int value) { 343 if (this.length == null) 344 this.length = new IntegerType(); 345 this.length.setValue(value); 346 return this; 347 } 348 349 /** 350 * @return {@link #sequenceAttachment} (The sequence information shall be 351 * provided enumerating the amino acids from N- to C-terminal end using 352 * standard single-letter amino acid codes. Uppercase shall be used for 353 * L-amino acids and lowercase for D-amino acids. Transcribed 354 * SubstanceProteins will always be described using the translated 355 * sequence; for synthetic peptide containing amino acids that are not 356 * represented with a single letter code an X should be used within the 357 * sequence. The modified amino acids will be distinguished by their 358 * position in the sequence.) 359 */ 360 public Attachment getSequenceAttachment() { 361 if (this.sequenceAttachment == null) 362 if (Configuration.errorOnAutoCreate()) 363 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.sequenceAttachment"); 364 else if (Configuration.doAutoCreate()) 365 this.sequenceAttachment = new Attachment(); // cc 366 return this.sequenceAttachment; 367 } 368 369 public boolean hasSequenceAttachment() { 370 return this.sequenceAttachment != null && !this.sequenceAttachment.isEmpty(); 371 } 372 373 /** 374 * @param value {@link #sequenceAttachment} (The sequence information shall be 375 * provided enumerating the amino acids from N- to C-terminal end 376 * using standard single-letter amino acid codes. Uppercase shall 377 * be used for L-amino acids and lowercase for D-amino acids. 378 * Transcribed SubstanceProteins will always be described using the 379 * translated sequence; for synthetic peptide containing amino 380 * acids that are not represented with a single letter code an X 381 * should be used within the sequence. The modified amino acids 382 * will be distinguished by their position in the sequence.) 383 */ 384 public SubstanceProteinSubunitComponent setSequenceAttachment(Attachment value) { 385 this.sequenceAttachment = value; 386 return this; 387 } 388 389 /** 390 * @return {@link #nTerminalModificationId} (Unique identifier for molecular 391 * fragment modification based on the ISO 11238 Substance ID.) 392 */ 393 public Identifier getNTerminalModificationId() { 394 if (this.nTerminalModificationId == null) 395 if (Configuration.errorOnAutoCreate()) 396 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.nTerminalModificationId"); 397 else if (Configuration.doAutoCreate()) 398 this.nTerminalModificationId = new Identifier(); // cc 399 return this.nTerminalModificationId; 400 } 401 402 public boolean hasNTerminalModificationId() { 403 return this.nTerminalModificationId != null && !this.nTerminalModificationId.isEmpty(); 404 } 405 406 /** 407 * @param value {@link #nTerminalModificationId} (Unique identifier for 408 * molecular fragment modification based on the ISO 11238 Substance 409 * ID.) 410 */ 411 public SubstanceProteinSubunitComponent setNTerminalModificationId(Identifier value) { 412 this.nTerminalModificationId = value; 413 return this; 414 } 415 416 /** 417 * @return {@link #nTerminalModification} (The name of the fragment modified at 418 * the N-terminal of the SubstanceProtein shall be specified.). This is 419 * the underlying object with id, value and extensions. The accessor 420 * "getNTerminalModification" gives direct access to the value 421 */ 422 public StringType getNTerminalModificationElement() { 423 if (this.nTerminalModification == null) 424 if (Configuration.errorOnAutoCreate()) 425 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.nTerminalModification"); 426 else if (Configuration.doAutoCreate()) 427 this.nTerminalModification = new StringType(); // bb 428 return this.nTerminalModification; 429 } 430 431 public boolean hasNTerminalModificationElement() { 432 return this.nTerminalModification != null && !this.nTerminalModification.isEmpty(); 433 } 434 435 public boolean hasNTerminalModification() { 436 return this.nTerminalModification != null && !this.nTerminalModification.isEmpty(); 437 } 438 439 /** 440 * @param value {@link #nTerminalModification} (The name of the fragment 441 * modified at the N-terminal of the SubstanceProtein shall be 442 * specified.). This is the underlying object with id, value and 443 * extensions. The accessor "getNTerminalModification" gives direct 444 * access to the value 445 */ 446 public SubstanceProteinSubunitComponent setNTerminalModificationElement(StringType value) { 447 this.nTerminalModification = value; 448 return this; 449 } 450 451 /** 452 * @return The name of the fragment modified at the N-terminal of the 453 * SubstanceProtein shall be specified. 454 */ 455 public String getNTerminalModification() { 456 return this.nTerminalModification == null ? null : this.nTerminalModification.getValue(); 457 } 458 459 /** 460 * @param value The name of the fragment modified at the N-terminal of the 461 * SubstanceProtein shall be specified. 462 */ 463 public SubstanceProteinSubunitComponent setNTerminalModification(String value) { 464 if (Utilities.noString(value)) 465 this.nTerminalModification = null; 466 else { 467 if (this.nTerminalModification == null) 468 this.nTerminalModification = new StringType(); 469 this.nTerminalModification.setValue(value); 470 } 471 return this; 472 } 473 474 /** 475 * @return {@link #cTerminalModificationId} (Unique identifier for molecular 476 * fragment modification based on the ISO 11238 Substance ID.) 477 */ 478 public Identifier getCTerminalModificationId() { 479 if (this.cTerminalModificationId == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.cTerminalModificationId"); 482 else if (Configuration.doAutoCreate()) 483 this.cTerminalModificationId = new Identifier(); // cc 484 return this.cTerminalModificationId; 485 } 486 487 public boolean hasCTerminalModificationId() { 488 return this.cTerminalModificationId != null && !this.cTerminalModificationId.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #cTerminalModificationId} (Unique identifier for 493 * molecular fragment modification based on the ISO 11238 Substance 494 * ID.) 495 */ 496 public SubstanceProteinSubunitComponent setCTerminalModificationId(Identifier value) { 497 this.cTerminalModificationId = value; 498 return this; 499 } 500 501 /** 502 * @return {@link #cTerminalModification} (The modification at the C-terminal 503 * shall be specified.). This is the underlying object with id, value 504 * and extensions. The accessor "getCTerminalModification" gives direct 505 * access to the value 506 */ 507 public StringType getCTerminalModificationElement() { 508 if (this.cTerminalModification == null) 509 if (Configuration.errorOnAutoCreate()) 510 throw new Error("Attempt to auto-create SubstanceProteinSubunitComponent.cTerminalModification"); 511 else if (Configuration.doAutoCreate()) 512 this.cTerminalModification = new StringType(); // bb 513 return this.cTerminalModification; 514 } 515 516 public boolean hasCTerminalModificationElement() { 517 return this.cTerminalModification != null && !this.cTerminalModification.isEmpty(); 518 } 519 520 public boolean hasCTerminalModification() { 521 return this.cTerminalModification != null && !this.cTerminalModification.isEmpty(); 522 } 523 524 /** 525 * @param value {@link #cTerminalModification} (The modification at the 526 * C-terminal shall be specified.). This is the underlying object 527 * with id, value and extensions. The accessor 528 * "getCTerminalModification" gives direct access to the value 529 */ 530 public SubstanceProteinSubunitComponent setCTerminalModificationElement(StringType value) { 531 this.cTerminalModification = value; 532 return this; 533 } 534 535 /** 536 * @return The modification at the C-terminal shall be specified. 537 */ 538 public String getCTerminalModification() { 539 return this.cTerminalModification == null ? null : this.cTerminalModification.getValue(); 540 } 541 542 /** 543 * @param value The modification at the C-terminal shall be specified. 544 */ 545 public SubstanceProteinSubunitComponent setCTerminalModification(String value) { 546 if (Utilities.noString(value)) 547 this.cTerminalModification = null; 548 else { 549 if (this.cTerminalModification == null) 550 this.cTerminalModification = new StringType(); 551 this.cTerminalModification.setValue(value); 552 } 553 return this; 554 } 555 556 protected void listChildren(List<Property> children) { 557 super.listChildren(children); 558 children.add(new Property("subunit", "integer", 559 "Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.", 560 0, 1, subunit)); 561 children.add(new Property("sequence", "string", 562 "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.", 563 0, 1, sequence)); 564 children.add(new Property("length", "integer", 565 "Length of linear sequences of amino acids contained in the subunit.", 0, 1, length)); 566 children.add(new Property("sequenceAttachment", "Attachment", 567 "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.", 568 0, 1, sequenceAttachment)); 569 children.add(new Property("nTerminalModificationId", "Identifier", 570 "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.", 0, 1, 571 nTerminalModificationId)); 572 children.add(new Property("nTerminalModification", "string", 573 "The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified.", 0, 1, 574 nTerminalModification)); 575 children.add(new Property("cTerminalModificationId", "Identifier", 576 "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.", 0, 1, 577 cTerminalModificationId)); 578 children.add(new Property("cTerminalModification", "string", 579 "The modification at the C-terminal shall be specified.", 0, 1, cTerminalModification)); 580 } 581 582 @Override 583 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 584 switch (_hash) { 585 case -1867548732: 586 /* subunit */ return new Property("subunit", "integer", 587 "Index of primary sequences of amino acids linked through peptide bonds in order of decreasing length. Sequences of the same length will be ordered by molecular weight. Subunits that have identical sequences will be repeated and have sequential subscripts.", 588 0, 1, subunit); 589 case 1349547969: 590 /* sequence */ return new Property("sequence", "string", 591 "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.", 592 0, 1, sequence); 593 case -1106363674: 594 /* length */ return new Property("length", "integer", 595 "Length of linear sequences of amino acids contained in the subunit.", 0, 1, length); 596 case 364621764: 597 /* sequenceAttachment */ return new Property("sequenceAttachment", "Attachment", 598 "The sequence information shall be provided enumerating the amino acids from N- to C-terminal end using standard single-letter amino acid codes. Uppercase shall be used for L-amino acids and lowercase for D-amino acids. Transcribed SubstanceProteins will always be described using the translated sequence; for synthetic peptide containing amino acids that are not represented with a single letter code an X should be used within the sequence. The modified amino acids will be distinguished by their position in the sequence.", 599 0, 1, sequenceAttachment); 600 case -182796415: 601 /* nTerminalModificationId */ return new Property("nTerminalModificationId", "Identifier", 602 "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.", 0, 1, 603 nTerminalModificationId); 604 case -1497395258: 605 /* nTerminalModification */ return new Property("nTerminalModification", "string", 606 "The name of the fragment modified at the N-terminal of the SubstanceProtein shall be specified.", 0, 1, 607 nTerminalModification); 608 case -990303818: 609 /* cTerminalModificationId */ return new Property("cTerminalModificationId", "Identifier", 610 "Unique identifier for molecular fragment modification based on the ISO 11238 Substance ID.", 0, 1, 611 cTerminalModificationId); 612 case 472711995: 613 /* cTerminalModification */ return new Property("cTerminalModification", "string", 614 "The modification at the C-terminal shall be specified.", 0, 1, cTerminalModification); 615 default: 616 return super.getNamedProperty(_hash, _name, _checkValid); 617 } 618 619 } 620 621 @Override 622 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 623 switch (hash) { 624 case -1867548732: 625 /* subunit */ return this.subunit == null ? new Base[0] : new Base[] { this.subunit }; // IntegerType 626 case 1349547969: 627 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // StringType 628 case -1106363674: 629 /* length */ return this.length == null ? new Base[0] : new Base[] { this.length }; // IntegerType 630 case 364621764: 631 /* sequenceAttachment */ return this.sequenceAttachment == null ? new Base[0] 632 : new Base[] { this.sequenceAttachment }; // Attachment 633 case -182796415: 634 /* nTerminalModificationId */ return this.nTerminalModificationId == null ? new Base[0] 635 : new Base[] { this.nTerminalModificationId }; // Identifier 636 case -1497395258: 637 /* nTerminalModification */ return this.nTerminalModification == null ? new Base[0] 638 : new Base[] { this.nTerminalModification }; // StringType 639 case -990303818: 640 /* cTerminalModificationId */ return this.cTerminalModificationId == null ? new Base[0] 641 : new Base[] { this.cTerminalModificationId }; // Identifier 642 case 472711995: 643 /* cTerminalModification */ return this.cTerminalModification == null ? new Base[0] 644 : new Base[] { this.cTerminalModification }; // StringType 645 default: 646 return super.getProperty(hash, name, checkValid); 647 } 648 649 } 650 651 @Override 652 public Base setProperty(int hash, String name, Base value) throws FHIRException { 653 switch (hash) { 654 case -1867548732: // subunit 655 this.subunit = castToInteger(value); // IntegerType 656 return value; 657 case 1349547969: // sequence 658 this.sequence = castToString(value); // StringType 659 return value; 660 case -1106363674: // length 661 this.length = castToInteger(value); // IntegerType 662 return value; 663 case 364621764: // sequenceAttachment 664 this.sequenceAttachment = castToAttachment(value); // Attachment 665 return value; 666 case -182796415: // nTerminalModificationId 667 this.nTerminalModificationId = castToIdentifier(value); // Identifier 668 return value; 669 case -1497395258: // nTerminalModification 670 this.nTerminalModification = castToString(value); // StringType 671 return value; 672 case -990303818: // cTerminalModificationId 673 this.cTerminalModificationId = castToIdentifier(value); // Identifier 674 return value; 675 case 472711995: // cTerminalModification 676 this.cTerminalModification = castToString(value); // StringType 677 return value; 678 default: 679 return super.setProperty(hash, name, value); 680 } 681 682 } 683 684 @Override 685 public Base setProperty(String name, Base value) throws FHIRException { 686 if (name.equals("subunit")) { 687 this.subunit = castToInteger(value); // IntegerType 688 } else if (name.equals("sequence")) { 689 this.sequence = castToString(value); // StringType 690 } else if (name.equals("length")) { 691 this.length = castToInteger(value); // IntegerType 692 } else if (name.equals("sequenceAttachment")) { 693 this.sequenceAttachment = castToAttachment(value); // Attachment 694 } else if (name.equals("nTerminalModificationId")) { 695 this.nTerminalModificationId = castToIdentifier(value); // Identifier 696 } else if (name.equals("nTerminalModification")) { 697 this.nTerminalModification = castToString(value); // StringType 698 } else if (name.equals("cTerminalModificationId")) { 699 this.cTerminalModificationId = castToIdentifier(value); // Identifier 700 } else if (name.equals("cTerminalModification")) { 701 this.cTerminalModification = castToString(value); // StringType 702 } else 703 return super.setProperty(name, value); 704 return value; 705 } 706 707 @Override 708 public void removeChild(String name, Base value) throws FHIRException { 709 if (name.equals("subunit")) { 710 this.subunit = null; 711 } else if (name.equals("sequence")) { 712 this.sequence = null; 713 } else if (name.equals("length")) { 714 this.length = null; 715 } else if (name.equals("sequenceAttachment")) { 716 this.sequenceAttachment = null; 717 } else if (name.equals("nTerminalModificationId")) { 718 this.nTerminalModificationId = null; 719 } else if (name.equals("nTerminalModification")) { 720 this.nTerminalModification = null; 721 } else if (name.equals("cTerminalModificationId")) { 722 this.cTerminalModificationId = null; 723 } else if (name.equals("cTerminalModification")) { 724 this.cTerminalModification = null; 725 } else 726 super.removeChild(name, value); 727 728 } 729 730 @Override 731 public Base makeProperty(int hash, String name) throws FHIRException { 732 switch (hash) { 733 case -1867548732: 734 return getSubunitElement(); 735 case 1349547969: 736 return getSequenceElement(); 737 case -1106363674: 738 return getLengthElement(); 739 case 364621764: 740 return getSequenceAttachment(); 741 case -182796415: 742 return getNTerminalModificationId(); 743 case -1497395258: 744 return getNTerminalModificationElement(); 745 case -990303818: 746 return getCTerminalModificationId(); 747 case 472711995: 748 return getCTerminalModificationElement(); 749 default: 750 return super.makeProperty(hash, name); 751 } 752 753 } 754 755 @Override 756 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 757 switch (hash) { 758 case -1867548732: 759 /* subunit */ return new String[] { "integer" }; 760 case 1349547969: 761 /* sequence */ return new String[] { "string" }; 762 case -1106363674: 763 /* length */ return new String[] { "integer" }; 764 case 364621764: 765 /* sequenceAttachment */ return new String[] { "Attachment" }; 766 case -182796415: 767 /* nTerminalModificationId */ return new String[] { "Identifier" }; 768 case -1497395258: 769 /* nTerminalModification */ return new String[] { "string" }; 770 case -990303818: 771 /* cTerminalModificationId */ return new String[] { "Identifier" }; 772 case 472711995: 773 /* cTerminalModification */ return new String[] { "string" }; 774 default: 775 return super.getTypesForProperty(hash, name); 776 } 777 778 } 779 780 @Override 781 public Base addChild(String name) throws FHIRException { 782 if (name.equals("subunit")) { 783 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.subunit"); 784 } else if (name.equals("sequence")) { 785 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.sequence"); 786 } else if (name.equals("length")) { 787 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.length"); 788 } else if (name.equals("sequenceAttachment")) { 789 this.sequenceAttachment = new Attachment(); 790 return this.sequenceAttachment; 791 } else if (name.equals("nTerminalModificationId")) { 792 this.nTerminalModificationId = new Identifier(); 793 return this.nTerminalModificationId; 794 } else if (name.equals("nTerminalModification")) { 795 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.nTerminalModification"); 796 } else if (name.equals("cTerminalModificationId")) { 797 this.cTerminalModificationId = new Identifier(); 798 return this.cTerminalModificationId; 799 } else if (name.equals("cTerminalModification")) { 800 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.cTerminalModification"); 801 } else 802 return super.addChild(name); 803 } 804 805 public SubstanceProteinSubunitComponent copy() { 806 SubstanceProteinSubunitComponent dst = new SubstanceProteinSubunitComponent(); 807 copyValues(dst); 808 return dst; 809 } 810 811 public void copyValues(SubstanceProteinSubunitComponent dst) { 812 super.copyValues(dst); 813 dst.subunit = subunit == null ? null : subunit.copy(); 814 dst.sequence = sequence == null ? null : sequence.copy(); 815 dst.length = length == null ? null : length.copy(); 816 dst.sequenceAttachment = sequenceAttachment == null ? null : sequenceAttachment.copy(); 817 dst.nTerminalModificationId = nTerminalModificationId == null ? null : nTerminalModificationId.copy(); 818 dst.nTerminalModification = nTerminalModification == null ? null : nTerminalModification.copy(); 819 dst.cTerminalModificationId = cTerminalModificationId == null ? null : cTerminalModificationId.copy(); 820 dst.cTerminalModification = cTerminalModification == null ? null : cTerminalModification.copy(); 821 } 822 823 @Override 824 public boolean equalsDeep(Base other_) { 825 if (!super.equalsDeep(other_)) 826 return false; 827 if (!(other_ instanceof SubstanceProteinSubunitComponent)) 828 return false; 829 SubstanceProteinSubunitComponent o = (SubstanceProteinSubunitComponent) other_; 830 return compareDeep(subunit, o.subunit, true) && compareDeep(sequence, o.sequence, true) 831 && compareDeep(length, o.length, true) && compareDeep(sequenceAttachment, o.sequenceAttachment, true) 832 && compareDeep(nTerminalModificationId, o.nTerminalModificationId, true) 833 && compareDeep(nTerminalModification, o.nTerminalModification, true) 834 && compareDeep(cTerminalModificationId, o.cTerminalModificationId, true) 835 && compareDeep(cTerminalModification, o.cTerminalModification, true); 836 } 837 838 @Override 839 public boolean equalsShallow(Base other_) { 840 if (!super.equalsShallow(other_)) 841 return false; 842 if (!(other_ instanceof SubstanceProteinSubunitComponent)) 843 return false; 844 SubstanceProteinSubunitComponent o = (SubstanceProteinSubunitComponent) other_; 845 return compareValues(subunit, o.subunit, true) && compareValues(sequence, o.sequence, true) 846 && compareValues(length, o.length, true) 847 && compareValues(nTerminalModification, o.nTerminalModification, true) 848 && compareValues(cTerminalModification, o.cTerminalModification, true); 849 } 850 851 public boolean isEmpty() { 852 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subunit, sequence, length, sequenceAttachment, 853 nTerminalModificationId, nTerminalModification, cTerminalModificationId, cTerminalModification); 854 } 855 856 public String fhirType() { 857 return "SubstanceProtein.subunit"; 858 859 } 860 861 } 862 863 /** 864 * The SubstanceProtein descriptive elements will only be used when a complete 865 * or partial amino acid sequence is available or derivable from a nucleic acid 866 * sequence. 867 */ 868 @Child(name = "sequenceType", type = { 869 CodeableConcept.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 870 @Description(shortDefinition = "The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence", formalDefinition = "The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence.") 871 protected CodeableConcept sequenceType; 872 873 /** 874 * Number of linear sequences of amino acids linked through peptide bonds. The 875 * number of subunits constituting the SubstanceProtein shall be described. It 876 * is possible that the number of subunits can be variable. 877 */ 878 @Child(name = "numberOfSubunits", type = { 879 IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 880 @Description(shortDefinition = "Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable", formalDefinition = "Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable.") 881 protected IntegerType numberOfSubunits; 882 883 /** 884 * The disulphide bond between two cysteine residues either on the same subunit 885 * or on two different subunits shall be described. The position of the 886 * disulfide bonds in the SubstanceProtein shall be listed in increasing order 887 * of subunit number and position within subunit followed by the abbreviation of 888 * the amino acids involved. The disulfide linkage positions shall actually 889 * contain the amino acid Cysteine at the respective positions. 890 */ 891 @Child(name = "disulfideLinkage", type = { 892 StringType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 893 @Description(shortDefinition = "The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions", formalDefinition = "The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.") 894 protected List<StringType> disulfideLinkage; 895 896 /** 897 * This subclause refers to the description of each subunit constituting the 898 * SubstanceProtein. A subunit is a linear sequence of amino acids linked 899 * through peptide bonds. The Subunit information shall be provided when the 900 * finished SubstanceProtein is a complex of multiple sequences; subunits are 901 * not used to delineate domains within a single sequence. Subunits are listed 902 * in order of decreasing length; sequences of the same length will be ordered 903 * by decreasing molecular weight; subunits that have identical sequences will 904 * be repeated multiple times. 905 */ 906 @Child(name = "subunit", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 907 @Description(shortDefinition = "This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times", formalDefinition = "This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times.") 908 protected List<SubstanceProteinSubunitComponent> subunit; 909 910 private static final long serialVersionUID = 469786856L; 911 912 /** 913 * Constructor 914 */ 915 public SubstanceProtein() { 916 super(); 917 } 918 919 /** 920 * @return {@link #sequenceType} (The SubstanceProtein descriptive elements will 921 * only be used when a complete or partial amino acid sequence is 922 * available or derivable from a nucleic acid sequence.) 923 */ 924 public CodeableConcept getSequenceType() { 925 if (this.sequenceType == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error("Attempt to auto-create SubstanceProtein.sequenceType"); 928 else if (Configuration.doAutoCreate()) 929 this.sequenceType = new CodeableConcept(); // cc 930 return this.sequenceType; 931 } 932 933 public boolean hasSequenceType() { 934 return this.sequenceType != null && !this.sequenceType.isEmpty(); 935 } 936 937 /** 938 * @param value {@link #sequenceType} (The SubstanceProtein descriptive elements 939 * will only be used when a complete or partial amino acid sequence 940 * is available or derivable from a nucleic acid sequence.) 941 */ 942 public SubstanceProtein setSequenceType(CodeableConcept value) { 943 this.sequenceType = value; 944 return this; 945 } 946 947 /** 948 * @return {@link #numberOfSubunits} (Number of linear sequences of amino acids 949 * linked through peptide bonds. The number of subunits constituting the 950 * SubstanceProtein shall be described. It is possible that the number 951 * of subunits can be variable.). This is the underlying object with id, 952 * value and extensions. The accessor "getNumberOfSubunits" gives direct 953 * access to the value 954 */ 955 public IntegerType getNumberOfSubunitsElement() { 956 if (this.numberOfSubunits == null) 957 if (Configuration.errorOnAutoCreate()) 958 throw new Error("Attempt to auto-create SubstanceProtein.numberOfSubunits"); 959 else if (Configuration.doAutoCreate()) 960 this.numberOfSubunits = new IntegerType(); // bb 961 return this.numberOfSubunits; 962 } 963 964 public boolean hasNumberOfSubunitsElement() { 965 return this.numberOfSubunits != null && !this.numberOfSubunits.isEmpty(); 966 } 967 968 public boolean hasNumberOfSubunits() { 969 return this.numberOfSubunits != null && !this.numberOfSubunits.isEmpty(); 970 } 971 972 /** 973 * @param value {@link #numberOfSubunits} (Number of linear sequences of amino 974 * acids linked through peptide bonds. The number of subunits 975 * constituting the SubstanceProtein shall be described. It is 976 * possible that the number of subunits can be variable.). This is 977 * the underlying object with id, value and extensions. The 978 * accessor "getNumberOfSubunits" gives direct access to the value 979 */ 980 public SubstanceProtein setNumberOfSubunitsElement(IntegerType value) { 981 this.numberOfSubunits = value; 982 return this; 983 } 984 985 /** 986 * @return Number of linear sequences of amino acids linked through peptide 987 * bonds. The number of subunits constituting the SubstanceProtein shall 988 * be described. It is possible that the number of subunits can be 989 * variable. 990 */ 991 public int getNumberOfSubunits() { 992 return this.numberOfSubunits == null || this.numberOfSubunits.isEmpty() ? 0 : this.numberOfSubunits.getValue(); 993 } 994 995 /** 996 * @param value Number of linear sequences of amino acids linked through peptide 997 * bonds. The number of subunits constituting the SubstanceProtein 998 * shall be described. It is possible that the number of subunits 999 * can be variable. 1000 */ 1001 public SubstanceProtein setNumberOfSubunits(int value) { 1002 if (this.numberOfSubunits == null) 1003 this.numberOfSubunits = new IntegerType(); 1004 this.numberOfSubunits.setValue(value); 1005 return this; 1006 } 1007 1008 /** 1009 * @return {@link #disulfideLinkage} (The disulphide bond between two cysteine 1010 * residues either on the same subunit or on two different subunits 1011 * shall be described. The position of the disulfide bonds in the 1012 * SubstanceProtein shall be listed in increasing order of subunit 1013 * number and position within subunit followed by the abbreviation of 1014 * the amino acids involved. The disulfide linkage positions shall 1015 * actually contain the amino acid Cysteine at the respective 1016 * positions.) 1017 */ 1018 public List<StringType> getDisulfideLinkage() { 1019 if (this.disulfideLinkage == null) 1020 this.disulfideLinkage = new ArrayList<StringType>(); 1021 return this.disulfideLinkage; 1022 } 1023 1024 /** 1025 * @return Returns a reference to <code>this</code> for easy method chaining 1026 */ 1027 public SubstanceProtein setDisulfideLinkage(List<StringType> theDisulfideLinkage) { 1028 this.disulfideLinkage = theDisulfideLinkage; 1029 return this; 1030 } 1031 1032 public boolean hasDisulfideLinkage() { 1033 if (this.disulfideLinkage == null) 1034 return false; 1035 for (StringType item : this.disulfideLinkage) 1036 if (!item.isEmpty()) 1037 return true; 1038 return false; 1039 } 1040 1041 /** 1042 * @return {@link #disulfideLinkage} (The disulphide bond between two cysteine 1043 * residues either on the same subunit or on two different subunits 1044 * shall be described. The position of the disulfide bonds in the 1045 * SubstanceProtein shall be listed in increasing order of subunit 1046 * number and position within subunit followed by the abbreviation of 1047 * the amino acids involved. The disulfide linkage positions shall 1048 * actually contain the amino acid Cysteine at the respective 1049 * positions.) 1050 */ 1051 public StringType addDisulfideLinkageElement() {// 2 1052 StringType t = new StringType(); 1053 if (this.disulfideLinkage == null) 1054 this.disulfideLinkage = new ArrayList<StringType>(); 1055 this.disulfideLinkage.add(t); 1056 return t; 1057 } 1058 1059 /** 1060 * @param value {@link #disulfideLinkage} (The disulphide bond between two 1061 * cysteine residues either on the same subunit or on two different 1062 * subunits shall be described. The position of the disulfide bonds 1063 * in the SubstanceProtein shall be listed in increasing order of 1064 * subunit number and position within subunit followed by the 1065 * abbreviation of the amino acids involved. The disulfide linkage 1066 * positions shall actually contain the amino acid Cysteine at the 1067 * respective positions.) 1068 */ 1069 public SubstanceProtein addDisulfideLinkage(String value) { // 1 1070 StringType t = new StringType(); 1071 t.setValue(value); 1072 if (this.disulfideLinkage == null) 1073 this.disulfideLinkage = new ArrayList<StringType>(); 1074 this.disulfideLinkage.add(t); 1075 return this; 1076 } 1077 1078 /** 1079 * @param value {@link #disulfideLinkage} (The disulphide bond between two 1080 * cysteine residues either on the same subunit or on two different 1081 * subunits shall be described. The position of the disulfide bonds 1082 * in the SubstanceProtein shall be listed in increasing order of 1083 * subunit number and position within subunit followed by the 1084 * abbreviation of the amino acids involved. The disulfide linkage 1085 * positions shall actually contain the amino acid Cysteine at the 1086 * respective positions.) 1087 */ 1088 public boolean hasDisulfideLinkage(String value) { 1089 if (this.disulfideLinkage == null) 1090 return false; 1091 for (StringType v : this.disulfideLinkage) 1092 if (v.getValue().equals(value)) // string 1093 return true; 1094 return false; 1095 } 1096 1097 /** 1098 * @return {@link #subunit} (This subclause refers to the description of each 1099 * subunit constituting the SubstanceProtein. A subunit is a linear 1100 * sequence of amino acids linked through peptide bonds. The Subunit 1101 * information shall be provided when the finished SubstanceProtein is a 1102 * complex of multiple sequences; subunits are not used to delineate 1103 * domains within a single sequence. Subunits are listed in order of 1104 * decreasing length; sequences of the same length will be ordered by 1105 * decreasing molecular weight; subunits that have identical sequences 1106 * will be repeated multiple times.) 1107 */ 1108 public List<SubstanceProteinSubunitComponent> getSubunit() { 1109 if (this.subunit == null) 1110 this.subunit = new ArrayList<SubstanceProteinSubunitComponent>(); 1111 return this.subunit; 1112 } 1113 1114 /** 1115 * @return Returns a reference to <code>this</code> for easy method chaining 1116 */ 1117 public SubstanceProtein setSubunit(List<SubstanceProteinSubunitComponent> theSubunit) { 1118 this.subunit = theSubunit; 1119 return this; 1120 } 1121 1122 public boolean hasSubunit() { 1123 if (this.subunit == null) 1124 return false; 1125 for (SubstanceProteinSubunitComponent item : this.subunit) 1126 if (!item.isEmpty()) 1127 return true; 1128 return false; 1129 } 1130 1131 public SubstanceProteinSubunitComponent addSubunit() { // 3 1132 SubstanceProteinSubunitComponent t = new SubstanceProteinSubunitComponent(); 1133 if (this.subunit == null) 1134 this.subunit = new ArrayList<SubstanceProteinSubunitComponent>(); 1135 this.subunit.add(t); 1136 return t; 1137 } 1138 1139 public SubstanceProtein addSubunit(SubstanceProteinSubunitComponent t) { // 3 1140 if (t == null) 1141 return this; 1142 if (this.subunit == null) 1143 this.subunit = new ArrayList<SubstanceProteinSubunitComponent>(); 1144 this.subunit.add(t); 1145 return this; 1146 } 1147 1148 /** 1149 * @return The first repetition of repeating field {@link #subunit}, creating it 1150 * if it does not already exist 1151 */ 1152 public SubstanceProteinSubunitComponent getSubunitFirstRep() { 1153 if (getSubunit().isEmpty()) { 1154 addSubunit(); 1155 } 1156 return getSubunit().get(0); 1157 } 1158 1159 protected void listChildren(List<Property> children) { 1160 super.listChildren(children); 1161 children.add(new Property("sequenceType", "CodeableConcept", 1162 "The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence.", 1163 0, 1, sequenceType)); 1164 children.add(new Property("numberOfSubunits", "integer", 1165 "Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable.", 1166 0, 1, numberOfSubunits)); 1167 children.add(new Property("disulfideLinkage", "string", 1168 "The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.", 1169 0, java.lang.Integer.MAX_VALUE, disulfideLinkage)); 1170 children.add(new Property("subunit", "", 1171 "This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times.", 1172 0, java.lang.Integer.MAX_VALUE, subunit)); 1173 } 1174 1175 @Override 1176 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1177 switch (_hash) { 1178 case 807711387: 1179 /* sequenceType */ return new Property("sequenceType", "CodeableConcept", 1180 "The SubstanceProtein descriptive elements will only be used when a complete or partial amino acid sequence is available or derivable from a nucleic acid sequence.", 1181 0, 1, sequenceType); 1182 case -847111089: 1183 /* numberOfSubunits */ return new Property("numberOfSubunits", "integer", 1184 "Number of linear sequences of amino acids linked through peptide bonds. The number of subunits constituting the SubstanceProtein shall be described. It is possible that the number of subunits can be variable.", 1185 0, 1, numberOfSubunits); 1186 case -1996102436: 1187 /* disulfideLinkage */ return new Property("disulfideLinkage", "string", 1188 "The disulphide bond between two cysteine residues either on the same subunit or on two different subunits shall be described. The position of the disulfide bonds in the SubstanceProtein shall be listed in increasing order of subunit number and position within subunit followed by the abbreviation of the amino acids involved. The disulfide linkage positions shall actually contain the amino acid Cysteine at the respective positions.", 1189 0, java.lang.Integer.MAX_VALUE, disulfideLinkage); 1190 case -1867548732: 1191 /* subunit */ return new Property("subunit", "", 1192 "This subclause refers to the description of each subunit constituting the SubstanceProtein. A subunit is a linear sequence of amino acids linked through peptide bonds. The Subunit information shall be provided when the finished SubstanceProtein is a complex of multiple sequences; subunits are not used to delineate domains within a single sequence. Subunits are listed in order of decreasing length; sequences of the same length will be ordered by decreasing molecular weight; subunits that have identical sequences will be repeated multiple times.", 1193 0, java.lang.Integer.MAX_VALUE, subunit); 1194 default: 1195 return super.getNamedProperty(_hash, _name, _checkValid); 1196 } 1197 1198 } 1199 1200 @Override 1201 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1202 switch (hash) { 1203 case 807711387: 1204 /* sequenceType */ return this.sequenceType == null ? new Base[0] : new Base[] { this.sequenceType }; // CodeableConcept 1205 case -847111089: 1206 /* numberOfSubunits */ return this.numberOfSubunits == null ? new Base[0] : new Base[] { this.numberOfSubunits }; // IntegerType 1207 case -1996102436: 1208 /* disulfideLinkage */ return this.disulfideLinkage == null ? new Base[0] 1209 : this.disulfideLinkage.toArray(new Base[this.disulfideLinkage.size()]); // StringType 1210 case -1867548732: 1211 /* subunit */ return this.subunit == null ? new Base[0] : this.subunit.toArray(new Base[this.subunit.size()]); // SubstanceProteinSubunitComponent 1212 default: 1213 return super.getProperty(hash, name, checkValid); 1214 } 1215 1216 } 1217 1218 @Override 1219 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1220 switch (hash) { 1221 case 807711387: // sequenceType 1222 this.sequenceType = castToCodeableConcept(value); // CodeableConcept 1223 return value; 1224 case -847111089: // numberOfSubunits 1225 this.numberOfSubunits = castToInteger(value); // IntegerType 1226 return value; 1227 case -1996102436: // disulfideLinkage 1228 this.getDisulfideLinkage().add(castToString(value)); // StringType 1229 return value; 1230 case -1867548732: // subunit 1231 this.getSubunit().add((SubstanceProteinSubunitComponent) value); // SubstanceProteinSubunitComponent 1232 return value; 1233 default: 1234 return super.setProperty(hash, name, value); 1235 } 1236 1237 } 1238 1239 @Override 1240 public Base setProperty(String name, Base value) throws FHIRException { 1241 if (name.equals("sequenceType")) { 1242 this.sequenceType = castToCodeableConcept(value); // CodeableConcept 1243 } else if (name.equals("numberOfSubunits")) { 1244 this.numberOfSubunits = castToInteger(value); // IntegerType 1245 } else if (name.equals("disulfideLinkage")) { 1246 this.getDisulfideLinkage().add(castToString(value)); 1247 } else if (name.equals("subunit")) { 1248 this.getSubunit().add((SubstanceProteinSubunitComponent) value); 1249 } else 1250 return super.setProperty(name, value); 1251 return value; 1252 } 1253 1254 @Override 1255 public void removeChild(String name, Base value) throws FHIRException { 1256 if (name.equals("sequenceType")) { 1257 this.sequenceType = null; 1258 } else if (name.equals("numberOfSubunits")) { 1259 this.numberOfSubunits = null; 1260 } else if (name.equals("disulfideLinkage")) { 1261 this.getDisulfideLinkage().remove(castToString(value)); 1262 } else if (name.equals("subunit")) { 1263 this.getSubunit().remove((SubstanceProteinSubunitComponent) value); 1264 } else 1265 super.removeChild(name, value); 1266 1267 } 1268 1269 @Override 1270 public Base makeProperty(int hash, String name) throws FHIRException { 1271 switch (hash) { 1272 case 807711387: 1273 return getSequenceType(); 1274 case -847111089: 1275 return getNumberOfSubunitsElement(); 1276 case -1996102436: 1277 return addDisulfideLinkageElement(); 1278 case -1867548732: 1279 return addSubunit(); 1280 default: 1281 return super.makeProperty(hash, name); 1282 } 1283 1284 } 1285 1286 @Override 1287 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1288 switch (hash) { 1289 case 807711387: 1290 /* sequenceType */ return new String[] { "CodeableConcept" }; 1291 case -847111089: 1292 /* numberOfSubunits */ return new String[] { "integer" }; 1293 case -1996102436: 1294 /* disulfideLinkage */ return new String[] { "string" }; 1295 case -1867548732: 1296 /* subunit */ return new String[] {}; 1297 default: 1298 return super.getTypesForProperty(hash, name); 1299 } 1300 1301 } 1302 1303 @Override 1304 public Base addChild(String name) throws FHIRException { 1305 if (name.equals("sequenceType")) { 1306 this.sequenceType = new CodeableConcept(); 1307 return this.sequenceType; 1308 } else if (name.equals("numberOfSubunits")) { 1309 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.numberOfSubunits"); 1310 } else if (name.equals("disulfideLinkage")) { 1311 throw new FHIRException("Cannot call addChild on a singleton property SubstanceProtein.disulfideLinkage"); 1312 } else if (name.equals("subunit")) { 1313 return addSubunit(); 1314 } else 1315 return super.addChild(name); 1316 } 1317 1318 public String fhirType() { 1319 return "SubstanceProtein"; 1320 1321 } 1322 1323 public SubstanceProtein copy() { 1324 SubstanceProtein dst = new SubstanceProtein(); 1325 copyValues(dst); 1326 return dst; 1327 } 1328 1329 public void copyValues(SubstanceProtein dst) { 1330 super.copyValues(dst); 1331 dst.sequenceType = sequenceType == null ? null : sequenceType.copy(); 1332 dst.numberOfSubunits = numberOfSubunits == null ? null : numberOfSubunits.copy(); 1333 if (disulfideLinkage != null) { 1334 dst.disulfideLinkage = new ArrayList<StringType>(); 1335 for (StringType i : disulfideLinkage) 1336 dst.disulfideLinkage.add(i.copy()); 1337 } 1338 ; 1339 if (subunit != null) { 1340 dst.subunit = new ArrayList<SubstanceProteinSubunitComponent>(); 1341 for (SubstanceProteinSubunitComponent i : subunit) 1342 dst.subunit.add(i.copy()); 1343 } 1344 ; 1345 } 1346 1347 protected SubstanceProtein typedCopy() { 1348 return copy(); 1349 } 1350 1351 @Override 1352 public boolean equalsDeep(Base other_) { 1353 if (!super.equalsDeep(other_)) 1354 return false; 1355 if (!(other_ instanceof SubstanceProtein)) 1356 return false; 1357 SubstanceProtein o = (SubstanceProtein) other_; 1358 return compareDeep(sequenceType, o.sequenceType, true) && compareDeep(numberOfSubunits, o.numberOfSubunits, true) 1359 && compareDeep(disulfideLinkage, o.disulfideLinkage, true) && compareDeep(subunit, o.subunit, true); 1360 } 1361 1362 @Override 1363 public boolean equalsShallow(Base other_) { 1364 if (!super.equalsShallow(other_)) 1365 return false; 1366 if (!(other_ instanceof SubstanceProtein)) 1367 return false; 1368 SubstanceProtein o = (SubstanceProtein) other_; 1369 return compareValues(numberOfSubunits, o.numberOfSubunits, true) 1370 && compareValues(disulfideLinkage, o.disulfideLinkage, true); 1371 } 1372 1373 public boolean isEmpty() { 1374 return super.isEmpty() 1375 && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceType, numberOfSubunits, disulfideLinkage, subunit); 1376 } 1377 1378 @Override 1379 public ResourceType getResourceType() { 1380 return ResourceType.SubstanceProtein; 1381 } 1382 1383}