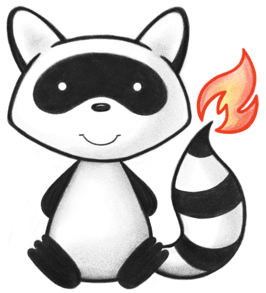
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044 045/** 046 * Todo. 047 */ 048@ResourceDef(name = "SubstanceReferenceInformation", profile = "http://hl7.org/fhir/StructureDefinition/SubstanceReferenceInformation") 049public class SubstanceReferenceInformation extends DomainResource { 050 051 @Block() 052 public static class SubstanceReferenceInformationGeneComponent extends BackboneElement 053 implements IBaseBackboneElement { 054 /** 055 * Todo. 056 */ 057 @Child(name = "geneSequenceOrigin", type = { 058 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 060 protected CodeableConcept geneSequenceOrigin; 061 062 /** 063 * Todo. 064 */ 065 @Child(name = "gene", type = { 066 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 067 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 068 protected CodeableConcept gene; 069 070 /** 071 * Todo. 072 */ 073 @Child(name = "source", type = { 074 DocumentReference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 075 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 076 protected List<Reference> source; 077 /** 078 * The actual objects that are the target of the reference (Todo.) 079 */ 080 protected List<DocumentReference> sourceTarget; 081 082 private static final long serialVersionUID = 1615185105L; 083 084 /** 085 * Constructor 086 */ 087 public SubstanceReferenceInformationGeneComponent() { 088 super(); 089 } 090 091 /** 092 * @return {@link #geneSequenceOrigin} (Todo.) 093 */ 094 public CodeableConcept getGeneSequenceOrigin() { 095 if (this.geneSequenceOrigin == null) 096 if (Configuration.errorOnAutoCreate()) 097 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneComponent.geneSequenceOrigin"); 098 else if (Configuration.doAutoCreate()) 099 this.geneSequenceOrigin = new CodeableConcept(); // cc 100 return this.geneSequenceOrigin; 101 } 102 103 public boolean hasGeneSequenceOrigin() { 104 return this.geneSequenceOrigin != null && !this.geneSequenceOrigin.isEmpty(); 105 } 106 107 /** 108 * @param value {@link #geneSequenceOrigin} (Todo.) 109 */ 110 public SubstanceReferenceInformationGeneComponent setGeneSequenceOrigin(CodeableConcept value) { 111 this.geneSequenceOrigin = value; 112 return this; 113 } 114 115 /** 116 * @return {@link #gene} (Todo.) 117 */ 118 public CodeableConcept getGene() { 119 if (this.gene == null) 120 if (Configuration.errorOnAutoCreate()) 121 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneComponent.gene"); 122 else if (Configuration.doAutoCreate()) 123 this.gene = new CodeableConcept(); // cc 124 return this.gene; 125 } 126 127 public boolean hasGene() { 128 return this.gene != null && !this.gene.isEmpty(); 129 } 130 131 /** 132 * @param value {@link #gene} (Todo.) 133 */ 134 public SubstanceReferenceInformationGeneComponent setGene(CodeableConcept value) { 135 this.gene = value; 136 return this; 137 } 138 139 /** 140 * @return {@link #source} (Todo.) 141 */ 142 public List<Reference> getSource() { 143 if (this.source == null) 144 this.source = new ArrayList<Reference>(); 145 return this.source; 146 } 147 148 /** 149 * @return Returns a reference to <code>this</code> for easy method chaining 150 */ 151 public SubstanceReferenceInformationGeneComponent setSource(List<Reference> theSource) { 152 this.source = theSource; 153 return this; 154 } 155 156 public boolean hasSource() { 157 if (this.source == null) 158 return false; 159 for (Reference item : this.source) 160 if (!item.isEmpty()) 161 return true; 162 return false; 163 } 164 165 public Reference addSource() { // 3 166 Reference t = new Reference(); 167 if (this.source == null) 168 this.source = new ArrayList<Reference>(); 169 this.source.add(t); 170 return t; 171 } 172 173 public SubstanceReferenceInformationGeneComponent addSource(Reference t) { // 3 174 if (t == null) 175 return this; 176 if (this.source == null) 177 this.source = new ArrayList<Reference>(); 178 this.source.add(t); 179 return this; 180 } 181 182 /** 183 * @return The first repetition of repeating field {@link #source}, creating it 184 * if it does not already exist 185 */ 186 public Reference getSourceFirstRep() { 187 if (getSource().isEmpty()) { 188 addSource(); 189 } 190 return getSource().get(0); 191 } 192 193 /** 194 * @deprecated Use Reference#setResource(IBaseResource) instead 195 */ 196 @Deprecated 197 public List<DocumentReference> getSourceTarget() { 198 if (this.sourceTarget == null) 199 this.sourceTarget = new ArrayList<DocumentReference>(); 200 return this.sourceTarget; 201 } 202 203 /** 204 * @deprecated Use Reference#setResource(IBaseResource) instead 205 */ 206 @Deprecated 207 public DocumentReference addSourceTarget() { 208 DocumentReference r = new DocumentReference(); 209 if (this.sourceTarget == null) 210 this.sourceTarget = new ArrayList<DocumentReference>(); 211 this.sourceTarget.add(r); 212 return r; 213 } 214 215 protected void listChildren(List<Property> children) { 216 super.listChildren(children); 217 children.add(new Property("geneSequenceOrigin", "CodeableConcept", "Todo.", 0, 1, geneSequenceOrigin)); 218 children.add(new Property("gene", "CodeableConcept", "Todo.", 0, 1, gene)); 219 children 220 .add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 221 } 222 223 @Override 224 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 225 switch (_hash) { 226 case -1089463108: 227 /* geneSequenceOrigin */ return new Property("geneSequenceOrigin", "CodeableConcept", "Todo.", 0, 1, 228 geneSequenceOrigin); 229 case 3169045: 230 /* gene */ return new Property("gene", "CodeableConcept", "Todo.", 0, 1, gene); 231 case -896505829: 232 /* source */ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, 233 java.lang.Integer.MAX_VALUE, source); 234 default: 235 return super.getNamedProperty(_hash, _name, _checkValid); 236 } 237 238 } 239 240 @Override 241 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 242 switch (hash) { 243 case -1089463108: 244 /* geneSequenceOrigin */ return this.geneSequenceOrigin == null ? new Base[0] 245 : new Base[] { this.geneSequenceOrigin }; // CodeableConcept 246 case 3169045: 247 /* gene */ return this.gene == null ? new Base[0] : new Base[] { this.gene }; // CodeableConcept 248 case -896505829: 249 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 250 default: 251 return super.getProperty(hash, name, checkValid); 252 } 253 254 } 255 256 @Override 257 public Base setProperty(int hash, String name, Base value) throws FHIRException { 258 switch (hash) { 259 case -1089463108: // geneSequenceOrigin 260 this.geneSequenceOrigin = castToCodeableConcept(value); // CodeableConcept 261 return value; 262 case 3169045: // gene 263 this.gene = castToCodeableConcept(value); // CodeableConcept 264 return value; 265 case -896505829: // source 266 this.getSource().add(castToReference(value)); // Reference 267 return value; 268 default: 269 return super.setProperty(hash, name, value); 270 } 271 272 } 273 274 @Override 275 public Base setProperty(String name, Base value) throws FHIRException { 276 if (name.equals("geneSequenceOrigin")) { 277 this.geneSequenceOrigin = castToCodeableConcept(value); // CodeableConcept 278 } else if (name.equals("gene")) { 279 this.gene = castToCodeableConcept(value); // CodeableConcept 280 } else if (name.equals("source")) { 281 this.getSource().add(castToReference(value)); 282 } else 283 return super.setProperty(name, value); 284 return value; 285 } 286 287 @Override 288 public void removeChild(String name, Base value) throws FHIRException { 289 if (name.equals("geneSequenceOrigin")) { 290 this.geneSequenceOrigin = null; 291 } else if (name.equals("gene")) { 292 this.gene = null; 293 } else if (name.equals("source")) { 294 this.getSource().remove(castToReference(value)); 295 } else 296 super.removeChild(name, value); 297 298 } 299 300 @Override 301 public Base makeProperty(int hash, String name) throws FHIRException { 302 switch (hash) { 303 case -1089463108: 304 return getGeneSequenceOrigin(); 305 case 3169045: 306 return getGene(); 307 case -896505829: 308 return addSource(); 309 default: 310 return super.makeProperty(hash, name); 311 } 312 313 } 314 315 @Override 316 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 317 switch (hash) { 318 case -1089463108: 319 /* geneSequenceOrigin */ return new String[] { "CodeableConcept" }; 320 case 3169045: 321 /* gene */ return new String[] { "CodeableConcept" }; 322 case -896505829: 323 /* source */ return new String[] { "Reference" }; 324 default: 325 return super.getTypesForProperty(hash, name); 326 } 327 328 } 329 330 @Override 331 public Base addChild(String name) throws FHIRException { 332 if (name.equals("geneSequenceOrigin")) { 333 this.geneSequenceOrigin = new CodeableConcept(); 334 return this.geneSequenceOrigin; 335 } else if (name.equals("gene")) { 336 this.gene = new CodeableConcept(); 337 return this.gene; 338 } else if (name.equals("source")) { 339 return addSource(); 340 } else 341 return super.addChild(name); 342 } 343 344 public SubstanceReferenceInformationGeneComponent copy() { 345 SubstanceReferenceInformationGeneComponent dst = new SubstanceReferenceInformationGeneComponent(); 346 copyValues(dst); 347 return dst; 348 } 349 350 public void copyValues(SubstanceReferenceInformationGeneComponent dst) { 351 super.copyValues(dst); 352 dst.geneSequenceOrigin = geneSequenceOrigin == null ? null : geneSequenceOrigin.copy(); 353 dst.gene = gene == null ? null : gene.copy(); 354 if (source != null) { 355 dst.source = new ArrayList<Reference>(); 356 for (Reference i : source) 357 dst.source.add(i.copy()); 358 } 359 ; 360 } 361 362 @Override 363 public boolean equalsDeep(Base other_) { 364 if (!super.equalsDeep(other_)) 365 return false; 366 if (!(other_ instanceof SubstanceReferenceInformationGeneComponent)) 367 return false; 368 SubstanceReferenceInformationGeneComponent o = (SubstanceReferenceInformationGeneComponent) other_; 369 return compareDeep(geneSequenceOrigin, o.geneSequenceOrigin, true) && compareDeep(gene, o.gene, true) 370 && compareDeep(source, o.source, true); 371 } 372 373 @Override 374 public boolean equalsShallow(Base other_) { 375 if (!super.equalsShallow(other_)) 376 return false; 377 if (!(other_ instanceof SubstanceReferenceInformationGeneComponent)) 378 return false; 379 SubstanceReferenceInformationGeneComponent o = (SubstanceReferenceInformationGeneComponent) other_; 380 return true; 381 } 382 383 public boolean isEmpty() { 384 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(geneSequenceOrigin, gene, source); 385 } 386 387 public String fhirType() { 388 return "SubstanceReferenceInformation.gene"; 389 390 } 391 392 } 393 394 @Block() 395 public static class SubstanceReferenceInformationGeneElementComponent extends BackboneElement 396 implements IBaseBackboneElement { 397 /** 398 * Todo. 399 */ 400 @Child(name = "type", type = { 401 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 402 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 403 protected CodeableConcept type; 404 405 /** 406 * Todo. 407 */ 408 @Child(name = "element", type = { Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 409 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 410 protected Identifier element; 411 412 /** 413 * Todo. 414 */ 415 @Child(name = "source", type = { 416 DocumentReference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 417 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 418 protected List<Reference> source; 419 /** 420 * The actual objects that are the target of the reference (Todo.) 421 */ 422 protected List<DocumentReference> sourceTarget; 423 424 private static final long serialVersionUID = 2055145950L; 425 426 /** 427 * Constructor 428 */ 429 public SubstanceReferenceInformationGeneElementComponent() { 430 super(); 431 } 432 433 /** 434 * @return {@link #type} (Todo.) 435 */ 436 public CodeableConcept getType() { 437 if (this.type == null) 438 if (Configuration.errorOnAutoCreate()) 439 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneElementComponent.type"); 440 else if (Configuration.doAutoCreate()) 441 this.type = new CodeableConcept(); // cc 442 return this.type; 443 } 444 445 public boolean hasType() { 446 return this.type != null && !this.type.isEmpty(); 447 } 448 449 /** 450 * @param value {@link #type} (Todo.) 451 */ 452 public SubstanceReferenceInformationGeneElementComponent setType(CodeableConcept value) { 453 this.type = value; 454 return this; 455 } 456 457 /** 458 * @return {@link #element} (Todo.) 459 */ 460 public Identifier getElement() { 461 if (this.element == null) 462 if (Configuration.errorOnAutoCreate()) 463 throw new Error("Attempt to auto-create SubstanceReferenceInformationGeneElementComponent.element"); 464 else if (Configuration.doAutoCreate()) 465 this.element = new Identifier(); // cc 466 return this.element; 467 } 468 469 public boolean hasElement() { 470 return this.element != null && !this.element.isEmpty(); 471 } 472 473 /** 474 * @param value {@link #element} (Todo.) 475 */ 476 public SubstanceReferenceInformationGeneElementComponent setElement(Identifier value) { 477 this.element = value; 478 return this; 479 } 480 481 /** 482 * @return {@link #source} (Todo.) 483 */ 484 public List<Reference> getSource() { 485 if (this.source == null) 486 this.source = new ArrayList<Reference>(); 487 return this.source; 488 } 489 490 /** 491 * @return Returns a reference to <code>this</code> for easy method chaining 492 */ 493 public SubstanceReferenceInformationGeneElementComponent setSource(List<Reference> theSource) { 494 this.source = theSource; 495 return this; 496 } 497 498 public boolean hasSource() { 499 if (this.source == null) 500 return false; 501 for (Reference item : this.source) 502 if (!item.isEmpty()) 503 return true; 504 return false; 505 } 506 507 public Reference addSource() { // 3 508 Reference t = new Reference(); 509 if (this.source == null) 510 this.source = new ArrayList<Reference>(); 511 this.source.add(t); 512 return t; 513 } 514 515 public SubstanceReferenceInformationGeneElementComponent addSource(Reference t) { // 3 516 if (t == null) 517 return this; 518 if (this.source == null) 519 this.source = new ArrayList<Reference>(); 520 this.source.add(t); 521 return this; 522 } 523 524 /** 525 * @return The first repetition of repeating field {@link #source}, creating it 526 * if it does not already exist 527 */ 528 public Reference getSourceFirstRep() { 529 if (getSource().isEmpty()) { 530 addSource(); 531 } 532 return getSource().get(0); 533 } 534 535 /** 536 * @deprecated Use Reference#setResource(IBaseResource) instead 537 */ 538 @Deprecated 539 public List<DocumentReference> getSourceTarget() { 540 if (this.sourceTarget == null) 541 this.sourceTarget = new ArrayList<DocumentReference>(); 542 return this.sourceTarget; 543 } 544 545 /** 546 * @deprecated Use Reference#setResource(IBaseResource) instead 547 */ 548 @Deprecated 549 public DocumentReference addSourceTarget() { 550 DocumentReference r = new DocumentReference(); 551 if (this.sourceTarget == null) 552 this.sourceTarget = new ArrayList<DocumentReference>(); 553 this.sourceTarget.add(r); 554 return r; 555 } 556 557 protected void listChildren(List<Property> children) { 558 super.listChildren(children); 559 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 560 children.add(new Property("element", "Identifier", "Todo.", 0, 1, element)); 561 children 562 .add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 563 } 564 565 @Override 566 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 567 switch (_hash) { 568 case 3575610: 569 /* type */ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 570 case -1662836996: 571 /* element */ return new Property("element", "Identifier", "Todo.", 0, 1, element); 572 case -896505829: 573 /* source */ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, 574 java.lang.Integer.MAX_VALUE, source); 575 default: 576 return super.getNamedProperty(_hash, _name, _checkValid); 577 } 578 579 } 580 581 @Override 582 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 583 switch (hash) { 584 case 3575610: 585 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 586 case -1662836996: 587 /* element */ return this.element == null ? new Base[0] : new Base[] { this.element }; // Identifier 588 case -896505829: 589 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 590 default: 591 return super.getProperty(hash, name, checkValid); 592 } 593 594 } 595 596 @Override 597 public Base setProperty(int hash, String name, Base value) throws FHIRException { 598 switch (hash) { 599 case 3575610: // type 600 this.type = castToCodeableConcept(value); // CodeableConcept 601 return value; 602 case -1662836996: // element 603 this.element = castToIdentifier(value); // Identifier 604 return value; 605 case -896505829: // source 606 this.getSource().add(castToReference(value)); // Reference 607 return value; 608 default: 609 return super.setProperty(hash, name, value); 610 } 611 612 } 613 614 @Override 615 public Base setProperty(String name, Base value) throws FHIRException { 616 if (name.equals("type")) { 617 this.type = castToCodeableConcept(value); // CodeableConcept 618 } else if (name.equals("element")) { 619 this.element = castToIdentifier(value); // Identifier 620 } else if (name.equals("source")) { 621 this.getSource().add(castToReference(value)); 622 } else 623 return super.setProperty(name, value); 624 return value; 625 } 626 627 @Override 628 public void removeChild(String name, Base value) throws FHIRException { 629 if (name.equals("type")) { 630 this.type = null; 631 } else if (name.equals("element")) { 632 this.element = null; 633 } else if (name.equals("source")) { 634 this.getSource().remove(castToReference(value)); 635 } else 636 super.removeChild(name, value); 637 638 } 639 640 @Override 641 public Base makeProperty(int hash, String name) throws FHIRException { 642 switch (hash) { 643 case 3575610: 644 return getType(); 645 case -1662836996: 646 return getElement(); 647 case -896505829: 648 return addSource(); 649 default: 650 return super.makeProperty(hash, name); 651 } 652 653 } 654 655 @Override 656 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 657 switch (hash) { 658 case 3575610: 659 /* type */ return new String[] { "CodeableConcept" }; 660 case -1662836996: 661 /* element */ return new String[] { "Identifier" }; 662 case -896505829: 663 /* source */ return new String[] { "Reference" }; 664 default: 665 return super.getTypesForProperty(hash, name); 666 } 667 668 } 669 670 @Override 671 public Base addChild(String name) throws FHIRException { 672 if (name.equals("type")) { 673 this.type = new CodeableConcept(); 674 return this.type; 675 } else if (name.equals("element")) { 676 this.element = new Identifier(); 677 return this.element; 678 } else if (name.equals("source")) { 679 return addSource(); 680 } else 681 return super.addChild(name); 682 } 683 684 public SubstanceReferenceInformationGeneElementComponent copy() { 685 SubstanceReferenceInformationGeneElementComponent dst = new SubstanceReferenceInformationGeneElementComponent(); 686 copyValues(dst); 687 return dst; 688 } 689 690 public void copyValues(SubstanceReferenceInformationGeneElementComponent dst) { 691 super.copyValues(dst); 692 dst.type = type == null ? null : type.copy(); 693 dst.element = element == null ? null : element.copy(); 694 if (source != null) { 695 dst.source = new ArrayList<Reference>(); 696 for (Reference i : source) 697 dst.source.add(i.copy()); 698 } 699 ; 700 } 701 702 @Override 703 public boolean equalsDeep(Base other_) { 704 if (!super.equalsDeep(other_)) 705 return false; 706 if (!(other_ instanceof SubstanceReferenceInformationGeneElementComponent)) 707 return false; 708 SubstanceReferenceInformationGeneElementComponent o = (SubstanceReferenceInformationGeneElementComponent) other_; 709 return compareDeep(type, o.type, true) && compareDeep(element, o.element, true) 710 && compareDeep(source, o.source, true); 711 } 712 713 @Override 714 public boolean equalsShallow(Base other_) { 715 if (!super.equalsShallow(other_)) 716 return false; 717 if (!(other_ instanceof SubstanceReferenceInformationGeneElementComponent)) 718 return false; 719 SubstanceReferenceInformationGeneElementComponent o = (SubstanceReferenceInformationGeneElementComponent) other_; 720 return true; 721 } 722 723 public boolean isEmpty() { 724 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, element, source); 725 } 726 727 public String fhirType() { 728 return "SubstanceReferenceInformation.geneElement"; 729 730 } 731 732 } 733 734 @Block() 735 public static class SubstanceReferenceInformationClassificationComponent extends BackboneElement 736 implements IBaseBackboneElement { 737 /** 738 * Todo. 739 */ 740 @Child(name = "domain", type = { 741 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 742 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 743 protected CodeableConcept domain; 744 745 /** 746 * Todo. 747 */ 748 @Child(name = "classification", type = { 749 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 750 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 751 protected CodeableConcept classification; 752 753 /** 754 * Todo. 755 */ 756 @Child(name = "subtype", type = { 757 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 758 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 759 protected List<CodeableConcept> subtype; 760 761 /** 762 * Todo. 763 */ 764 @Child(name = "source", type = { 765 DocumentReference.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 766 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 767 protected List<Reference> source; 768 /** 769 * The actual objects that are the target of the reference (Todo.) 770 */ 771 protected List<DocumentReference> sourceTarget; 772 773 private static final long serialVersionUID = -430084579L; 774 775 /** 776 * Constructor 777 */ 778 public SubstanceReferenceInformationClassificationComponent() { 779 super(); 780 } 781 782 /** 783 * @return {@link #domain} (Todo.) 784 */ 785 public CodeableConcept getDomain() { 786 if (this.domain == null) 787 if (Configuration.errorOnAutoCreate()) 788 throw new Error("Attempt to auto-create SubstanceReferenceInformationClassificationComponent.domain"); 789 else if (Configuration.doAutoCreate()) 790 this.domain = new CodeableConcept(); // cc 791 return this.domain; 792 } 793 794 public boolean hasDomain() { 795 return this.domain != null && !this.domain.isEmpty(); 796 } 797 798 /** 799 * @param value {@link #domain} (Todo.) 800 */ 801 public SubstanceReferenceInformationClassificationComponent setDomain(CodeableConcept value) { 802 this.domain = value; 803 return this; 804 } 805 806 /** 807 * @return {@link #classification} (Todo.) 808 */ 809 public CodeableConcept getClassification() { 810 if (this.classification == null) 811 if (Configuration.errorOnAutoCreate()) 812 throw new Error("Attempt to auto-create SubstanceReferenceInformationClassificationComponent.classification"); 813 else if (Configuration.doAutoCreate()) 814 this.classification = new CodeableConcept(); // cc 815 return this.classification; 816 } 817 818 public boolean hasClassification() { 819 return this.classification != null && !this.classification.isEmpty(); 820 } 821 822 /** 823 * @param value {@link #classification} (Todo.) 824 */ 825 public SubstanceReferenceInformationClassificationComponent setClassification(CodeableConcept value) { 826 this.classification = value; 827 return this; 828 } 829 830 /** 831 * @return {@link #subtype} (Todo.) 832 */ 833 public List<CodeableConcept> getSubtype() { 834 if (this.subtype == null) 835 this.subtype = new ArrayList<CodeableConcept>(); 836 return this.subtype; 837 } 838 839 /** 840 * @return Returns a reference to <code>this</code> for easy method chaining 841 */ 842 public SubstanceReferenceInformationClassificationComponent setSubtype(List<CodeableConcept> theSubtype) { 843 this.subtype = theSubtype; 844 return this; 845 } 846 847 public boolean hasSubtype() { 848 if (this.subtype == null) 849 return false; 850 for (CodeableConcept item : this.subtype) 851 if (!item.isEmpty()) 852 return true; 853 return false; 854 } 855 856 public CodeableConcept addSubtype() { // 3 857 CodeableConcept t = new CodeableConcept(); 858 if (this.subtype == null) 859 this.subtype = new ArrayList<CodeableConcept>(); 860 this.subtype.add(t); 861 return t; 862 } 863 864 public SubstanceReferenceInformationClassificationComponent addSubtype(CodeableConcept t) { // 3 865 if (t == null) 866 return this; 867 if (this.subtype == null) 868 this.subtype = new ArrayList<CodeableConcept>(); 869 this.subtype.add(t); 870 return this; 871 } 872 873 /** 874 * @return The first repetition of repeating field {@link #subtype}, creating it 875 * if it does not already exist 876 */ 877 public CodeableConcept getSubtypeFirstRep() { 878 if (getSubtype().isEmpty()) { 879 addSubtype(); 880 } 881 return getSubtype().get(0); 882 } 883 884 /** 885 * @return {@link #source} (Todo.) 886 */ 887 public List<Reference> getSource() { 888 if (this.source == null) 889 this.source = new ArrayList<Reference>(); 890 return this.source; 891 } 892 893 /** 894 * @return Returns a reference to <code>this</code> for easy method chaining 895 */ 896 public SubstanceReferenceInformationClassificationComponent setSource(List<Reference> theSource) { 897 this.source = theSource; 898 return this; 899 } 900 901 public boolean hasSource() { 902 if (this.source == null) 903 return false; 904 for (Reference item : this.source) 905 if (!item.isEmpty()) 906 return true; 907 return false; 908 } 909 910 public Reference addSource() { // 3 911 Reference t = new Reference(); 912 if (this.source == null) 913 this.source = new ArrayList<Reference>(); 914 this.source.add(t); 915 return t; 916 } 917 918 public SubstanceReferenceInformationClassificationComponent addSource(Reference t) { // 3 919 if (t == null) 920 return this; 921 if (this.source == null) 922 this.source = new ArrayList<Reference>(); 923 this.source.add(t); 924 return this; 925 } 926 927 /** 928 * @return The first repetition of repeating field {@link #source}, creating it 929 * if it does not already exist 930 */ 931 public Reference getSourceFirstRep() { 932 if (getSource().isEmpty()) { 933 addSource(); 934 } 935 return getSource().get(0); 936 } 937 938 /** 939 * @deprecated Use Reference#setResource(IBaseResource) instead 940 */ 941 @Deprecated 942 public List<DocumentReference> getSourceTarget() { 943 if (this.sourceTarget == null) 944 this.sourceTarget = new ArrayList<DocumentReference>(); 945 return this.sourceTarget; 946 } 947 948 /** 949 * @deprecated Use Reference#setResource(IBaseResource) instead 950 */ 951 @Deprecated 952 public DocumentReference addSourceTarget() { 953 DocumentReference r = new DocumentReference(); 954 if (this.sourceTarget == null) 955 this.sourceTarget = new ArrayList<DocumentReference>(); 956 this.sourceTarget.add(r); 957 return r; 958 } 959 960 protected void listChildren(List<Property> children) { 961 super.listChildren(children); 962 children.add(new Property("domain", "CodeableConcept", "Todo.", 0, 1, domain)); 963 children.add(new Property("classification", "CodeableConcept", "Todo.", 0, 1, classification)); 964 children.add(new Property("subtype", "CodeableConcept", "Todo.", 0, java.lang.Integer.MAX_VALUE, subtype)); 965 children 966 .add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 967 } 968 969 @Override 970 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 971 switch (_hash) { 972 case -1326197564: 973 /* domain */ return new Property("domain", "CodeableConcept", "Todo.", 0, 1, domain); 974 case 382350310: 975 /* classification */ return new Property("classification", "CodeableConcept", "Todo.", 0, 1, classification); 976 case -1867567750: 977 /* subtype */ return new Property("subtype", "CodeableConcept", "Todo.", 0, java.lang.Integer.MAX_VALUE, 978 subtype); 979 case -896505829: 980 /* source */ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, 981 java.lang.Integer.MAX_VALUE, source); 982 default: 983 return super.getNamedProperty(_hash, _name, _checkValid); 984 } 985 986 } 987 988 @Override 989 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 990 switch (hash) { 991 case -1326197564: 992 /* domain */ return this.domain == null ? new Base[0] : new Base[] { this.domain }; // CodeableConcept 993 case 382350310: 994 /* classification */ return this.classification == null ? new Base[0] : new Base[] { this.classification }; // CodeableConcept 995 case -1867567750: 996 /* subtype */ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // CodeableConcept 997 case -896505829: 998 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 999 default: 1000 return super.getProperty(hash, name, checkValid); 1001 } 1002 1003 } 1004 1005 @Override 1006 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1007 switch (hash) { 1008 case -1326197564: // domain 1009 this.domain = castToCodeableConcept(value); // CodeableConcept 1010 return value; 1011 case 382350310: // classification 1012 this.classification = castToCodeableConcept(value); // CodeableConcept 1013 return value; 1014 case -1867567750: // subtype 1015 this.getSubtype().add(castToCodeableConcept(value)); // CodeableConcept 1016 return value; 1017 case -896505829: // source 1018 this.getSource().add(castToReference(value)); // Reference 1019 return value; 1020 default: 1021 return super.setProperty(hash, name, value); 1022 } 1023 1024 } 1025 1026 @Override 1027 public Base setProperty(String name, Base value) throws FHIRException { 1028 if (name.equals("domain")) { 1029 this.domain = castToCodeableConcept(value); // CodeableConcept 1030 } else if (name.equals("classification")) { 1031 this.classification = castToCodeableConcept(value); // CodeableConcept 1032 } else if (name.equals("subtype")) { 1033 this.getSubtype().add(castToCodeableConcept(value)); 1034 } else if (name.equals("source")) { 1035 this.getSource().add(castToReference(value)); 1036 } else 1037 return super.setProperty(name, value); 1038 return value; 1039 } 1040 1041 @Override 1042 public void removeChild(String name, Base value) throws FHIRException { 1043 if (name.equals("domain")) { 1044 this.domain = null; 1045 } else if (name.equals("classification")) { 1046 this.classification = null; 1047 } else if (name.equals("subtype")) { 1048 this.getSubtype().remove(castToCodeableConcept(value)); 1049 } else if (name.equals("source")) { 1050 this.getSource().remove(castToReference(value)); 1051 } else 1052 super.removeChild(name, value); 1053 1054 } 1055 1056 @Override 1057 public Base makeProperty(int hash, String name) throws FHIRException { 1058 switch (hash) { 1059 case -1326197564: 1060 return getDomain(); 1061 case 382350310: 1062 return getClassification(); 1063 case -1867567750: 1064 return addSubtype(); 1065 case -896505829: 1066 return addSource(); 1067 default: 1068 return super.makeProperty(hash, name); 1069 } 1070 1071 } 1072 1073 @Override 1074 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1075 switch (hash) { 1076 case -1326197564: 1077 /* domain */ return new String[] { "CodeableConcept" }; 1078 case 382350310: 1079 /* classification */ return new String[] { "CodeableConcept" }; 1080 case -1867567750: 1081 /* subtype */ return new String[] { "CodeableConcept" }; 1082 case -896505829: 1083 /* source */ return new String[] { "Reference" }; 1084 default: 1085 return super.getTypesForProperty(hash, name); 1086 } 1087 1088 } 1089 1090 @Override 1091 public Base addChild(String name) throws FHIRException { 1092 if (name.equals("domain")) { 1093 this.domain = new CodeableConcept(); 1094 return this.domain; 1095 } else if (name.equals("classification")) { 1096 this.classification = new CodeableConcept(); 1097 return this.classification; 1098 } else if (name.equals("subtype")) { 1099 return addSubtype(); 1100 } else if (name.equals("source")) { 1101 return addSource(); 1102 } else 1103 return super.addChild(name); 1104 } 1105 1106 public SubstanceReferenceInformationClassificationComponent copy() { 1107 SubstanceReferenceInformationClassificationComponent dst = new SubstanceReferenceInformationClassificationComponent(); 1108 copyValues(dst); 1109 return dst; 1110 } 1111 1112 public void copyValues(SubstanceReferenceInformationClassificationComponent dst) { 1113 super.copyValues(dst); 1114 dst.domain = domain == null ? null : domain.copy(); 1115 dst.classification = classification == null ? null : classification.copy(); 1116 if (subtype != null) { 1117 dst.subtype = new ArrayList<CodeableConcept>(); 1118 for (CodeableConcept i : subtype) 1119 dst.subtype.add(i.copy()); 1120 } 1121 ; 1122 if (source != null) { 1123 dst.source = new ArrayList<Reference>(); 1124 for (Reference i : source) 1125 dst.source.add(i.copy()); 1126 } 1127 ; 1128 } 1129 1130 @Override 1131 public boolean equalsDeep(Base other_) { 1132 if (!super.equalsDeep(other_)) 1133 return false; 1134 if (!(other_ instanceof SubstanceReferenceInformationClassificationComponent)) 1135 return false; 1136 SubstanceReferenceInformationClassificationComponent o = (SubstanceReferenceInformationClassificationComponent) other_; 1137 return compareDeep(domain, o.domain, true) && compareDeep(classification, o.classification, true) 1138 && compareDeep(subtype, o.subtype, true) && compareDeep(source, o.source, true); 1139 } 1140 1141 @Override 1142 public boolean equalsShallow(Base other_) { 1143 if (!super.equalsShallow(other_)) 1144 return false; 1145 if (!(other_ instanceof SubstanceReferenceInformationClassificationComponent)) 1146 return false; 1147 SubstanceReferenceInformationClassificationComponent o = (SubstanceReferenceInformationClassificationComponent) other_; 1148 return true; 1149 } 1150 1151 public boolean isEmpty() { 1152 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(domain, classification, subtype, source); 1153 } 1154 1155 public String fhirType() { 1156 return "SubstanceReferenceInformation.classification"; 1157 1158 } 1159 1160 } 1161 1162 @Block() 1163 public static class SubstanceReferenceInformationTargetComponent extends BackboneElement 1164 implements IBaseBackboneElement { 1165 /** 1166 * Todo. 1167 */ 1168 @Child(name = "target", type = { Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1169 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1170 protected Identifier target; 1171 1172 /** 1173 * Todo. 1174 */ 1175 @Child(name = "type", type = { 1176 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1177 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1178 protected CodeableConcept type; 1179 1180 /** 1181 * Todo. 1182 */ 1183 @Child(name = "interaction", type = { 1184 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1185 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1186 protected CodeableConcept interaction; 1187 1188 /** 1189 * Todo. 1190 */ 1191 @Child(name = "organism", type = { 1192 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1193 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1194 protected CodeableConcept organism; 1195 1196 /** 1197 * Todo. 1198 */ 1199 @Child(name = "organismType", type = { 1200 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1201 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1202 protected CodeableConcept organismType; 1203 1204 /** 1205 * Todo. 1206 */ 1207 @Child(name = "amount", type = { Quantity.class, Range.class, 1208 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1209 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1210 protected Type amount; 1211 1212 /** 1213 * Todo. 1214 */ 1215 @Child(name = "amountType", type = { 1216 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1217 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1218 protected CodeableConcept amountType; 1219 1220 /** 1221 * Todo. 1222 */ 1223 @Child(name = "source", type = { 1224 DocumentReference.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1225 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1226 protected List<Reference> source; 1227 /** 1228 * The actual objects that are the target of the reference (Todo.) 1229 */ 1230 protected List<DocumentReference> sourceTarget; 1231 1232 private static final long serialVersionUID = -1682270197L; 1233 1234 /** 1235 * Constructor 1236 */ 1237 public SubstanceReferenceInformationTargetComponent() { 1238 super(); 1239 } 1240 1241 /** 1242 * @return {@link #target} (Todo.) 1243 */ 1244 public Identifier getTarget() { 1245 if (this.target == null) 1246 if (Configuration.errorOnAutoCreate()) 1247 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.target"); 1248 else if (Configuration.doAutoCreate()) 1249 this.target = new Identifier(); // cc 1250 return this.target; 1251 } 1252 1253 public boolean hasTarget() { 1254 return this.target != null && !this.target.isEmpty(); 1255 } 1256 1257 /** 1258 * @param value {@link #target} (Todo.) 1259 */ 1260 public SubstanceReferenceInformationTargetComponent setTarget(Identifier value) { 1261 this.target = value; 1262 return this; 1263 } 1264 1265 /** 1266 * @return {@link #type} (Todo.) 1267 */ 1268 public CodeableConcept getType() { 1269 if (this.type == null) 1270 if (Configuration.errorOnAutoCreate()) 1271 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.type"); 1272 else if (Configuration.doAutoCreate()) 1273 this.type = new CodeableConcept(); // cc 1274 return this.type; 1275 } 1276 1277 public boolean hasType() { 1278 return this.type != null && !this.type.isEmpty(); 1279 } 1280 1281 /** 1282 * @param value {@link #type} (Todo.) 1283 */ 1284 public SubstanceReferenceInformationTargetComponent setType(CodeableConcept value) { 1285 this.type = value; 1286 return this; 1287 } 1288 1289 /** 1290 * @return {@link #interaction} (Todo.) 1291 */ 1292 public CodeableConcept getInteraction() { 1293 if (this.interaction == null) 1294 if (Configuration.errorOnAutoCreate()) 1295 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.interaction"); 1296 else if (Configuration.doAutoCreate()) 1297 this.interaction = new CodeableConcept(); // cc 1298 return this.interaction; 1299 } 1300 1301 public boolean hasInteraction() { 1302 return this.interaction != null && !this.interaction.isEmpty(); 1303 } 1304 1305 /** 1306 * @param value {@link #interaction} (Todo.) 1307 */ 1308 public SubstanceReferenceInformationTargetComponent setInteraction(CodeableConcept value) { 1309 this.interaction = value; 1310 return this; 1311 } 1312 1313 /** 1314 * @return {@link #organism} (Todo.) 1315 */ 1316 public CodeableConcept getOrganism() { 1317 if (this.organism == null) 1318 if (Configuration.errorOnAutoCreate()) 1319 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.organism"); 1320 else if (Configuration.doAutoCreate()) 1321 this.organism = new CodeableConcept(); // cc 1322 return this.organism; 1323 } 1324 1325 public boolean hasOrganism() { 1326 return this.organism != null && !this.organism.isEmpty(); 1327 } 1328 1329 /** 1330 * @param value {@link #organism} (Todo.) 1331 */ 1332 public SubstanceReferenceInformationTargetComponent setOrganism(CodeableConcept value) { 1333 this.organism = value; 1334 return this; 1335 } 1336 1337 /** 1338 * @return {@link #organismType} (Todo.) 1339 */ 1340 public CodeableConcept getOrganismType() { 1341 if (this.organismType == null) 1342 if (Configuration.errorOnAutoCreate()) 1343 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.organismType"); 1344 else if (Configuration.doAutoCreate()) 1345 this.organismType = new CodeableConcept(); // cc 1346 return this.organismType; 1347 } 1348 1349 public boolean hasOrganismType() { 1350 return this.organismType != null && !this.organismType.isEmpty(); 1351 } 1352 1353 /** 1354 * @param value {@link #organismType} (Todo.) 1355 */ 1356 public SubstanceReferenceInformationTargetComponent setOrganismType(CodeableConcept value) { 1357 this.organismType = value; 1358 return this; 1359 } 1360 1361 /** 1362 * @return {@link #amount} (Todo.) 1363 */ 1364 public Type getAmount() { 1365 return this.amount; 1366 } 1367 1368 /** 1369 * @return {@link #amount} (Todo.) 1370 */ 1371 public Quantity getAmountQuantity() throws FHIRException { 1372 if (this.amount == null) 1373 this.amount = new Quantity(); 1374 if (!(this.amount instanceof Quantity)) 1375 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.amount.getClass().getName() 1376 + " was encountered"); 1377 return (Quantity) this.amount; 1378 } 1379 1380 public boolean hasAmountQuantity() { 1381 return this != null && this.amount instanceof Quantity; 1382 } 1383 1384 /** 1385 * @return {@link #amount} (Todo.) 1386 */ 1387 public Range getAmountRange() throws FHIRException { 1388 if (this.amount == null) 1389 this.amount = new Range(); 1390 if (!(this.amount instanceof Range)) 1391 throw new FHIRException( 1392 "Type mismatch: the type Range was expected, but " + this.amount.getClass().getName() + " was encountered"); 1393 return (Range) this.amount; 1394 } 1395 1396 public boolean hasAmountRange() { 1397 return this != null && this.amount instanceof Range; 1398 } 1399 1400 /** 1401 * @return {@link #amount} (Todo.) 1402 */ 1403 public StringType getAmountStringType() throws FHIRException { 1404 if (this.amount == null) 1405 this.amount = new StringType(); 1406 if (!(this.amount instanceof StringType)) 1407 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1408 + this.amount.getClass().getName() + " was encountered"); 1409 return (StringType) this.amount; 1410 } 1411 1412 public boolean hasAmountStringType() { 1413 return this != null && this.amount instanceof StringType; 1414 } 1415 1416 public boolean hasAmount() { 1417 return this.amount != null && !this.amount.isEmpty(); 1418 } 1419 1420 /** 1421 * @param value {@link #amount} (Todo.) 1422 */ 1423 public SubstanceReferenceInformationTargetComponent setAmount(Type value) { 1424 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof StringType)) 1425 throw new Error("Not the right type for SubstanceReferenceInformation.target.amount[x]: " + value.fhirType()); 1426 this.amount = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return {@link #amountType} (Todo.) 1432 */ 1433 public CodeableConcept getAmountType() { 1434 if (this.amountType == null) 1435 if (Configuration.errorOnAutoCreate()) 1436 throw new Error("Attempt to auto-create SubstanceReferenceInformationTargetComponent.amountType"); 1437 else if (Configuration.doAutoCreate()) 1438 this.amountType = new CodeableConcept(); // cc 1439 return this.amountType; 1440 } 1441 1442 public boolean hasAmountType() { 1443 return this.amountType != null && !this.amountType.isEmpty(); 1444 } 1445 1446 /** 1447 * @param value {@link #amountType} (Todo.) 1448 */ 1449 public SubstanceReferenceInformationTargetComponent setAmountType(CodeableConcept value) { 1450 this.amountType = value; 1451 return this; 1452 } 1453 1454 /** 1455 * @return {@link #source} (Todo.) 1456 */ 1457 public List<Reference> getSource() { 1458 if (this.source == null) 1459 this.source = new ArrayList<Reference>(); 1460 return this.source; 1461 } 1462 1463 /** 1464 * @return Returns a reference to <code>this</code> for easy method chaining 1465 */ 1466 public SubstanceReferenceInformationTargetComponent setSource(List<Reference> theSource) { 1467 this.source = theSource; 1468 return this; 1469 } 1470 1471 public boolean hasSource() { 1472 if (this.source == null) 1473 return false; 1474 for (Reference item : this.source) 1475 if (!item.isEmpty()) 1476 return true; 1477 return false; 1478 } 1479 1480 public Reference addSource() { // 3 1481 Reference t = new Reference(); 1482 if (this.source == null) 1483 this.source = new ArrayList<Reference>(); 1484 this.source.add(t); 1485 return t; 1486 } 1487 1488 public SubstanceReferenceInformationTargetComponent addSource(Reference t) { // 3 1489 if (t == null) 1490 return this; 1491 if (this.source == null) 1492 this.source = new ArrayList<Reference>(); 1493 this.source.add(t); 1494 return this; 1495 } 1496 1497 /** 1498 * @return The first repetition of repeating field {@link #source}, creating it 1499 * if it does not already exist 1500 */ 1501 public Reference getSourceFirstRep() { 1502 if (getSource().isEmpty()) { 1503 addSource(); 1504 } 1505 return getSource().get(0); 1506 } 1507 1508 /** 1509 * @deprecated Use Reference#setResource(IBaseResource) instead 1510 */ 1511 @Deprecated 1512 public List<DocumentReference> getSourceTarget() { 1513 if (this.sourceTarget == null) 1514 this.sourceTarget = new ArrayList<DocumentReference>(); 1515 return this.sourceTarget; 1516 } 1517 1518 /** 1519 * @deprecated Use Reference#setResource(IBaseResource) instead 1520 */ 1521 @Deprecated 1522 public DocumentReference addSourceTarget() { 1523 DocumentReference r = new DocumentReference(); 1524 if (this.sourceTarget == null) 1525 this.sourceTarget = new ArrayList<DocumentReference>(); 1526 this.sourceTarget.add(r); 1527 return r; 1528 } 1529 1530 protected void listChildren(List<Property> children) { 1531 super.listChildren(children); 1532 children.add(new Property("target", "Identifier", "Todo.", 0, 1, target)); 1533 children.add(new Property("type", "CodeableConcept", "Todo.", 0, 1, type)); 1534 children.add(new Property("interaction", "CodeableConcept", "Todo.", 0, 1, interaction)); 1535 children.add(new Property("organism", "CodeableConcept", "Todo.", 0, 1, organism)); 1536 children.add(new Property("organismType", "CodeableConcept", "Todo.", 0, 1, organismType)); 1537 children.add(new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount)); 1538 children.add(new Property("amountType", "CodeableConcept", "Todo.", 0, 1, amountType)); 1539 children 1540 .add(new Property("source", "Reference(DocumentReference)", "Todo.", 0, java.lang.Integer.MAX_VALUE, source)); 1541 } 1542 1543 @Override 1544 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1545 switch (_hash) { 1546 case -880905839: 1547 /* target */ return new Property("target", "Identifier", "Todo.", 0, 1, target); 1548 case 3575610: 1549 /* type */ return new Property("type", "CodeableConcept", "Todo.", 0, 1, type); 1550 case 1844104722: 1551 /* interaction */ return new Property("interaction", "CodeableConcept", "Todo.", 0, 1, interaction); 1552 case 1316389074: 1553 /* organism */ return new Property("organism", "CodeableConcept", "Todo.", 0, 1, organism); 1554 case 988662572: 1555 /* organismType */ return new Property("organismType", "CodeableConcept", "Todo.", 0, 1, organismType); 1556 case 646780200: 1557 /* amount[x] */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1558 case -1413853096: 1559 /* amount */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1560 case 1664303363: 1561 /* amountQuantity */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1562 case -1223462971: 1563 /* amountRange */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1564 case 773651081: 1565 /* amountString */ return new Property("amount[x]", "Quantity|Range|string", "Todo.", 0, 1, amount); 1566 case -1424857166: 1567 /* amountType */ return new Property("amountType", "CodeableConcept", "Todo.", 0, 1, amountType); 1568 case -896505829: 1569 /* source */ return new Property("source", "Reference(DocumentReference)", "Todo.", 0, 1570 java.lang.Integer.MAX_VALUE, source); 1571 default: 1572 return super.getNamedProperty(_hash, _name, _checkValid); 1573 } 1574 1575 } 1576 1577 @Override 1578 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1579 switch (hash) { 1580 case -880905839: 1581 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Identifier 1582 case 3575610: 1583 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1584 case 1844104722: 1585 /* interaction */ return this.interaction == null ? new Base[0] : new Base[] { this.interaction }; // CodeableConcept 1586 case 1316389074: 1587 /* organism */ return this.organism == null ? new Base[0] : new Base[] { this.organism }; // CodeableConcept 1588 case 988662572: 1589 /* organismType */ return this.organismType == null ? new Base[0] : new Base[] { this.organismType }; // CodeableConcept 1590 case -1413853096: 1591 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Type 1592 case -1424857166: 1593 /* amountType */ return this.amountType == null ? new Base[0] : new Base[] { this.amountType }; // CodeableConcept 1594 case -896505829: 1595 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 1596 default: 1597 return super.getProperty(hash, name, checkValid); 1598 } 1599 1600 } 1601 1602 @Override 1603 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1604 switch (hash) { 1605 case -880905839: // target 1606 this.target = castToIdentifier(value); // Identifier 1607 return value; 1608 case 3575610: // type 1609 this.type = castToCodeableConcept(value); // CodeableConcept 1610 return value; 1611 case 1844104722: // interaction 1612 this.interaction = castToCodeableConcept(value); // CodeableConcept 1613 return value; 1614 case 1316389074: // organism 1615 this.organism = castToCodeableConcept(value); // CodeableConcept 1616 return value; 1617 case 988662572: // organismType 1618 this.organismType = castToCodeableConcept(value); // CodeableConcept 1619 return value; 1620 case -1413853096: // amount 1621 this.amount = castToType(value); // Type 1622 return value; 1623 case -1424857166: // amountType 1624 this.amountType = castToCodeableConcept(value); // CodeableConcept 1625 return value; 1626 case -896505829: // source 1627 this.getSource().add(castToReference(value)); // Reference 1628 return value; 1629 default: 1630 return super.setProperty(hash, name, value); 1631 } 1632 1633 } 1634 1635 @Override 1636 public Base setProperty(String name, Base value) throws FHIRException { 1637 if (name.equals("target")) { 1638 this.target = castToIdentifier(value); // Identifier 1639 } else if (name.equals("type")) { 1640 this.type = castToCodeableConcept(value); // CodeableConcept 1641 } else if (name.equals("interaction")) { 1642 this.interaction = castToCodeableConcept(value); // CodeableConcept 1643 } else if (name.equals("organism")) { 1644 this.organism = castToCodeableConcept(value); // CodeableConcept 1645 } else if (name.equals("organismType")) { 1646 this.organismType = castToCodeableConcept(value); // CodeableConcept 1647 } else if (name.equals("amount[x]")) { 1648 this.amount = castToType(value); // Type 1649 } else if (name.equals("amountType")) { 1650 this.amountType = castToCodeableConcept(value); // CodeableConcept 1651 } else if (name.equals("source")) { 1652 this.getSource().add(castToReference(value)); 1653 } else 1654 return super.setProperty(name, value); 1655 return value; 1656 } 1657 1658 @Override 1659 public void removeChild(String name, Base value) throws FHIRException { 1660 if (name.equals("target")) { 1661 this.target = null; 1662 } else if (name.equals("type")) { 1663 this.type = null; 1664 } else if (name.equals("interaction")) { 1665 this.interaction = null; 1666 } else if (name.equals("organism")) { 1667 this.organism = null; 1668 } else if (name.equals("organismType")) { 1669 this.organismType = null; 1670 } else if (name.equals("amount[x]")) { 1671 this.amount = null; 1672 } else if (name.equals("amountType")) { 1673 this.amountType = null; 1674 } else if (name.equals("source")) { 1675 this.getSource().remove(castToReference(value)); 1676 } else 1677 super.removeChild(name, value); 1678 1679 } 1680 1681 @Override 1682 public Base makeProperty(int hash, String name) throws FHIRException { 1683 switch (hash) { 1684 case -880905839: 1685 return getTarget(); 1686 case 3575610: 1687 return getType(); 1688 case 1844104722: 1689 return getInteraction(); 1690 case 1316389074: 1691 return getOrganism(); 1692 case 988662572: 1693 return getOrganismType(); 1694 case 646780200: 1695 return getAmount(); 1696 case -1413853096: 1697 return getAmount(); 1698 case -1424857166: 1699 return getAmountType(); 1700 case -896505829: 1701 return addSource(); 1702 default: 1703 return super.makeProperty(hash, name); 1704 } 1705 1706 } 1707 1708 @Override 1709 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1710 switch (hash) { 1711 case -880905839: 1712 /* target */ return new String[] { "Identifier" }; 1713 case 3575610: 1714 /* type */ return new String[] { "CodeableConcept" }; 1715 case 1844104722: 1716 /* interaction */ return new String[] { "CodeableConcept" }; 1717 case 1316389074: 1718 /* organism */ return new String[] { "CodeableConcept" }; 1719 case 988662572: 1720 /* organismType */ return new String[] { "CodeableConcept" }; 1721 case -1413853096: 1722 /* amount */ return new String[] { "Quantity", "Range", "string" }; 1723 case -1424857166: 1724 /* amountType */ return new String[] { "CodeableConcept" }; 1725 case -896505829: 1726 /* source */ return new String[] { "Reference" }; 1727 default: 1728 return super.getTypesForProperty(hash, name); 1729 } 1730 1731 } 1732 1733 @Override 1734 public Base addChild(String name) throws FHIRException { 1735 if (name.equals("target")) { 1736 this.target = new Identifier(); 1737 return this.target; 1738 } else if (name.equals("type")) { 1739 this.type = new CodeableConcept(); 1740 return this.type; 1741 } else if (name.equals("interaction")) { 1742 this.interaction = new CodeableConcept(); 1743 return this.interaction; 1744 } else if (name.equals("organism")) { 1745 this.organism = new CodeableConcept(); 1746 return this.organism; 1747 } else if (name.equals("organismType")) { 1748 this.organismType = new CodeableConcept(); 1749 return this.organismType; 1750 } else if (name.equals("amountQuantity")) { 1751 this.amount = new Quantity(); 1752 return this.amount; 1753 } else if (name.equals("amountRange")) { 1754 this.amount = new Range(); 1755 return this.amount; 1756 } else if (name.equals("amountString")) { 1757 this.amount = new StringType(); 1758 return this.amount; 1759 } else if (name.equals("amountType")) { 1760 this.amountType = new CodeableConcept(); 1761 return this.amountType; 1762 } else if (name.equals("source")) { 1763 return addSource(); 1764 } else 1765 return super.addChild(name); 1766 } 1767 1768 public SubstanceReferenceInformationTargetComponent copy() { 1769 SubstanceReferenceInformationTargetComponent dst = new SubstanceReferenceInformationTargetComponent(); 1770 copyValues(dst); 1771 return dst; 1772 } 1773 1774 public void copyValues(SubstanceReferenceInformationTargetComponent dst) { 1775 super.copyValues(dst); 1776 dst.target = target == null ? null : target.copy(); 1777 dst.type = type == null ? null : type.copy(); 1778 dst.interaction = interaction == null ? null : interaction.copy(); 1779 dst.organism = organism == null ? null : organism.copy(); 1780 dst.organismType = organismType == null ? null : organismType.copy(); 1781 dst.amount = amount == null ? null : amount.copy(); 1782 dst.amountType = amountType == null ? null : amountType.copy(); 1783 if (source != null) { 1784 dst.source = new ArrayList<Reference>(); 1785 for (Reference i : source) 1786 dst.source.add(i.copy()); 1787 } 1788 ; 1789 } 1790 1791 @Override 1792 public boolean equalsDeep(Base other_) { 1793 if (!super.equalsDeep(other_)) 1794 return false; 1795 if (!(other_ instanceof SubstanceReferenceInformationTargetComponent)) 1796 return false; 1797 SubstanceReferenceInformationTargetComponent o = (SubstanceReferenceInformationTargetComponent) other_; 1798 return compareDeep(target, o.target, true) && compareDeep(type, o.type, true) 1799 && compareDeep(interaction, o.interaction, true) && compareDeep(organism, o.organism, true) 1800 && compareDeep(organismType, o.organismType, true) && compareDeep(amount, o.amount, true) 1801 && compareDeep(amountType, o.amountType, true) && compareDeep(source, o.source, true); 1802 } 1803 1804 @Override 1805 public boolean equalsShallow(Base other_) { 1806 if (!super.equalsShallow(other_)) 1807 return false; 1808 if (!(other_ instanceof SubstanceReferenceInformationTargetComponent)) 1809 return false; 1810 SubstanceReferenceInformationTargetComponent o = (SubstanceReferenceInformationTargetComponent) other_; 1811 return true; 1812 } 1813 1814 public boolean isEmpty() { 1815 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, type, interaction, organism, organismType, 1816 amount, amountType, source); 1817 } 1818 1819 public String fhirType() { 1820 return "SubstanceReferenceInformation.target"; 1821 1822 } 1823 1824 } 1825 1826 /** 1827 * Todo. 1828 */ 1829 @Child(name = "comment", type = { StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1830 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1831 protected StringType comment; 1832 1833 /** 1834 * Todo. 1835 */ 1836 @Child(name = "gene", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1837 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1838 protected List<SubstanceReferenceInformationGeneComponent> gene; 1839 1840 /** 1841 * Todo. 1842 */ 1843 @Child(name = "geneElement", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1844 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1845 protected List<SubstanceReferenceInformationGeneElementComponent> geneElement; 1846 1847 /** 1848 * Todo. 1849 */ 1850 @Child(name = "classification", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1851 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1852 protected List<SubstanceReferenceInformationClassificationComponent> classification; 1853 1854 /** 1855 * Todo. 1856 */ 1857 @Child(name = "target", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1858 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1859 protected List<SubstanceReferenceInformationTargetComponent> target; 1860 1861 private static final long serialVersionUID = 890303332L; 1862 1863 /** 1864 * Constructor 1865 */ 1866 public SubstanceReferenceInformation() { 1867 super(); 1868 } 1869 1870 /** 1871 * @return {@link #comment} (Todo.). This is the underlying object with id, 1872 * value and extensions. The accessor "getComment" gives direct access 1873 * to the value 1874 */ 1875 public StringType getCommentElement() { 1876 if (this.comment == null) 1877 if (Configuration.errorOnAutoCreate()) 1878 throw new Error("Attempt to auto-create SubstanceReferenceInformation.comment"); 1879 else if (Configuration.doAutoCreate()) 1880 this.comment = new StringType(); // bb 1881 return this.comment; 1882 } 1883 1884 public boolean hasCommentElement() { 1885 return this.comment != null && !this.comment.isEmpty(); 1886 } 1887 1888 public boolean hasComment() { 1889 return this.comment != null && !this.comment.isEmpty(); 1890 } 1891 1892 /** 1893 * @param value {@link #comment} (Todo.). This is the underlying object with id, 1894 * value and extensions. The accessor "getComment" gives direct 1895 * access to the value 1896 */ 1897 public SubstanceReferenceInformation setCommentElement(StringType value) { 1898 this.comment = value; 1899 return this; 1900 } 1901 1902 /** 1903 * @return Todo. 1904 */ 1905 public String getComment() { 1906 return this.comment == null ? null : this.comment.getValue(); 1907 } 1908 1909 /** 1910 * @param value Todo. 1911 */ 1912 public SubstanceReferenceInformation setComment(String value) { 1913 if (Utilities.noString(value)) 1914 this.comment = null; 1915 else { 1916 if (this.comment == null) 1917 this.comment = new StringType(); 1918 this.comment.setValue(value); 1919 } 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #gene} (Todo.) 1925 */ 1926 public List<SubstanceReferenceInformationGeneComponent> getGene() { 1927 if (this.gene == null) 1928 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1929 return this.gene; 1930 } 1931 1932 /** 1933 * @return Returns a reference to <code>this</code> for easy method chaining 1934 */ 1935 public SubstanceReferenceInformation setGene(List<SubstanceReferenceInformationGeneComponent> theGene) { 1936 this.gene = theGene; 1937 return this; 1938 } 1939 1940 public boolean hasGene() { 1941 if (this.gene == null) 1942 return false; 1943 for (SubstanceReferenceInformationGeneComponent item : this.gene) 1944 if (!item.isEmpty()) 1945 return true; 1946 return false; 1947 } 1948 1949 public SubstanceReferenceInformationGeneComponent addGene() { // 3 1950 SubstanceReferenceInformationGeneComponent t = new SubstanceReferenceInformationGeneComponent(); 1951 if (this.gene == null) 1952 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1953 this.gene.add(t); 1954 return t; 1955 } 1956 1957 public SubstanceReferenceInformation addGene(SubstanceReferenceInformationGeneComponent t) { // 3 1958 if (t == null) 1959 return this; 1960 if (this.gene == null) 1961 this.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 1962 this.gene.add(t); 1963 return this; 1964 } 1965 1966 /** 1967 * @return The first repetition of repeating field {@link #gene}, creating it if 1968 * it does not already exist 1969 */ 1970 public SubstanceReferenceInformationGeneComponent getGeneFirstRep() { 1971 if (getGene().isEmpty()) { 1972 addGene(); 1973 } 1974 return getGene().get(0); 1975 } 1976 1977 /** 1978 * @return {@link #geneElement} (Todo.) 1979 */ 1980 public List<SubstanceReferenceInformationGeneElementComponent> getGeneElement() { 1981 if (this.geneElement == null) 1982 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 1983 return this.geneElement; 1984 } 1985 1986 /** 1987 * @return Returns a reference to <code>this</code> for easy method chaining 1988 */ 1989 public SubstanceReferenceInformation setGeneElement( 1990 List<SubstanceReferenceInformationGeneElementComponent> theGeneElement) { 1991 this.geneElement = theGeneElement; 1992 return this; 1993 } 1994 1995 public boolean hasGeneElement() { 1996 if (this.geneElement == null) 1997 return false; 1998 for (SubstanceReferenceInformationGeneElementComponent item : this.geneElement) 1999 if (!item.isEmpty()) 2000 return true; 2001 return false; 2002 } 2003 2004 public SubstanceReferenceInformationGeneElementComponent addGeneElement() { // 3 2005 SubstanceReferenceInformationGeneElementComponent t = new SubstanceReferenceInformationGeneElementComponent(); 2006 if (this.geneElement == null) 2007 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 2008 this.geneElement.add(t); 2009 return t; 2010 } 2011 2012 public SubstanceReferenceInformation addGeneElement(SubstanceReferenceInformationGeneElementComponent t) { // 3 2013 if (t == null) 2014 return this; 2015 if (this.geneElement == null) 2016 this.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 2017 this.geneElement.add(t); 2018 return this; 2019 } 2020 2021 /** 2022 * @return The first repetition of repeating field {@link #geneElement}, 2023 * creating it if it does not already exist 2024 */ 2025 public SubstanceReferenceInformationGeneElementComponent getGeneElementFirstRep() { 2026 if (getGeneElement().isEmpty()) { 2027 addGeneElement(); 2028 } 2029 return getGeneElement().get(0); 2030 } 2031 2032 /** 2033 * @return {@link #classification} (Todo.) 2034 */ 2035 public List<SubstanceReferenceInformationClassificationComponent> getClassification() { 2036 if (this.classification == null) 2037 this.classification = new ArrayList<SubstanceReferenceInformationClassificationComponent>(); 2038 return this.classification; 2039 } 2040 2041 /** 2042 * @return Returns a reference to <code>this</code> for easy method chaining 2043 */ 2044 public SubstanceReferenceInformation setClassification( 2045 List<SubstanceReferenceInformationClassificationComponent> theClassification) { 2046 this.classification = theClassification; 2047 return this; 2048 } 2049 2050 public boolean hasClassification() { 2051 if (this.classification == null) 2052 return false; 2053 for (SubstanceReferenceInformationClassificationComponent item : this.classification) 2054 if (!item.isEmpty()) 2055 return true; 2056 return false; 2057 } 2058 2059 public SubstanceReferenceInformationClassificationComponent addClassification() { // 3 2060 SubstanceReferenceInformationClassificationComponent t = new SubstanceReferenceInformationClassificationComponent(); 2061 if (this.classification == null) 2062 this.classification = new ArrayList<SubstanceReferenceInformationClassificationComponent>(); 2063 this.classification.add(t); 2064 return t; 2065 } 2066 2067 public SubstanceReferenceInformation addClassification(SubstanceReferenceInformationClassificationComponent t) { // 3 2068 if (t == null) 2069 return this; 2070 if (this.classification == null) 2071 this.classification = new ArrayList<SubstanceReferenceInformationClassificationComponent>(); 2072 this.classification.add(t); 2073 return this; 2074 } 2075 2076 /** 2077 * @return The first repetition of repeating field {@link #classification}, 2078 * creating it if it does not already exist 2079 */ 2080 public SubstanceReferenceInformationClassificationComponent getClassificationFirstRep() { 2081 if (getClassification().isEmpty()) { 2082 addClassification(); 2083 } 2084 return getClassification().get(0); 2085 } 2086 2087 /** 2088 * @return {@link #target} (Todo.) 2089 */ 2090 public List<SubstanceReferenceInformationTargetComponent> getTarget() { 2091 if (this.target == null) 2092 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 2093 return this.target; 2094 } 2095 2096 /** 2097 * @return Returns a reference to <code>this</code> for easy method chaining 2098 */ 2099 public SubstanceReferenceInformation setTarget(List<SubstanceReferenceInformationTargetComponent> theTarget) { 2100 this.target = theTarget; 2101 return this; 2102 } 2103 2104 public boolean hasTarget() { 2105 if (this.target == null) 2106 return false; 2107 for (SubstanceReferenceInformationTargetComponent item : this.target) 2108 if (!item.isEmpty()) 2109 return true; 2110 return false; 2111 } 2112 2113 public SubstanceReferenceInformationTargetComponent addTarget() { // 3 2114 SubstanceReferenceInformationTargetComponent t = new SubstanceReferenceInformationTargetComponent(); 2115 if (this.target == null) 2116 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 2117 this.target.add(t); 2118 return t; 2119 } 2120 2121 public SubstanceReferenceInformation addTarget(SubstanceReferenceInformationTargetComponent t) { // 3 2122 if (t == null) 2123 return this; 2124 if (this.target == null) 2125 this.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 2126 this.target.add(t); 2127 return this; 2128 } 2129 2130 /** 2131 * @return The first repetition of repeating field {@link #target}, creating it 2132 * if it does not already exist 2133 */ 2134 public SubstanceReferenceInformationTargetComponent getTargetFirstRep() { 2135 if (getTarget().isEmpty()) { 2136 addTarget(); 2137 } 2138 return getTarget().get(0); 2139 } 2140 2141 protected void listChildren(List<Property> children) { 2142 super.listChildren(children); 2143 children.add(new Property("comment", "string", "Todo.", 0, 1, comment)); 2144 children.add(new Property("gene", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, gene)); 2145 children.add(new Property("geneElement", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, geneElement)); 2146 children.add(new Property("classification", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, classification)); 2147 children.add(new Property("target", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, target)); 2148 } 2149 2150 @Override 2151 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2152 switch (_hash) { 2153 case 950398559: 2154 /* comment */ return new Property("comment", "string", "Todo.", 0, 1, comment); 2155 case 3169045: 2156 /* gene */ return new Property("gene", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, gene); 2157 case -94918105: 2158 /* geneElement */ return new Property("geneElement", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, geneElement); 2159 case 382350310: 2160 /* classification */ return new Property("classification", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, 2161 classification); 2162 case -880905839: 2163 /* target */ return new Property("target", "", "Todo.", 0, java.lang.Integer.MAX_VALUE, target); 2164 default: 2165 return super.getNamedProperty(_hash, _name, _checkValid); 2166 } 2167 2168 } 2169 2170 @Override 2171 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2172 switch (hash) { 2173 case 950398559: 2174 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 2175 case 3169045: 2176 /* gene */ return this.gene == null ? new Base[0] : this.gene.toArray(new Base[this.gene.size()]); // SubstanceReferenceInformationGeneComponent 2177 case -94918105: 2178 /* geneElement */ return this.geneElement == null ? new Base[0] 2179 : this.geneElement.toArray(new Base[this.geneElement.size()]); // SubstanceReferenceInformationGeneElementComponent 2180 case 382350310: 2181 /* classification */ return this.classification == null ? new Base[0] 2182 : this.classification.toArray(new Base[this.classification.size()]); // SubstanceReferenceInformationClassificationComponent 2183 case -880905839: 2184 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // SubstanceReferenceInformationTargetComponent 2185 default: 2186 return super.getProperty(hash, name, checkValid); 2187 } 2188 2189 } 2190 2191 @Override 2192 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2193 switch (hash) { 2194 case 950398559: // comment 2195 this.comment = castToString(value); // StringType 2196 return value; 2197 case 3169045: // gene 2198 this.getGene().add((SubstanceReferenceInformationGeneComponent) value); // SubstanceReferenceInformationGeneComponent 2199 return value; 2200 case -94918105: // geneElement 2201 this.getGeneElement().add((SubstanceReferenceInformationGeneElementComponent) value); // SubstanceReferenceInformationGeneElementComponent 2202 return value; 2203 case 382350310: // classification 2204 this.getClassification().add((SubstanceReferenceInformationClassificationComponent) value); // SubstanceReferenceInformationClassificationComponent 2205 return value; 2206 case -880905839: // target 2207 this.getTarget().add((SubstanceReferenceInformationTargetComponent) value); // SubstanceReferenceInformationTargetComponent 2208 return value; 2209 default: 2210 return super.setProperty(hash, name, value); 2211 } 2212 2213 } 2214 2215 @Override 2216 public Base setProperty(String name, Base value) throws FHIRException { 2217 if (name.equals("comment")) { 2218 this.comment = castToString(value); // StringType 2219 } else if (name.equals("gene")) { 2220 this.getGene().add((SubstanceReferenceInformationGeneComponent) value); 2221 } else if (name.equals("geneElement")) { 2222 this.getGeneElement().add((SubstanceReferenceInformationGeneElementComponent) value); 2223 } else if (name.equals("classification")) { 2224 this.getClassification().add((SubstanceReferenceInformationClassificationComponent) value); 2225 } else if (name.equals("target")) { 2226 this.getTarget().add((SubstanceReferenceInformationTargetComponent) value); 2227 } else 2228 return super.setProperty(name, value); 2229 return value; 2230 } 2231 2232 @Override 2233 public void removeChild(String name, Base value) throws FHIRException { 2234 if (name.equals("comment")) { 2235 this.comment = null; 2236 } else if (name.equals("gene")) { 2237 this.getGene().remove((SubstanceReferenceInformationGeneComponent) value); 2238 } else if (name.equals("geneElement")) { 2239 this.getGeneElement().remove((SubstanceReferenceInformationGeneElementComponent) value); 2240 } else if (name.equals("classification")) { 2241 this.getClassification().remove((SubstanceReferenceInformationClassificationComponent) value); 2242 } else if (name.equals("target")) { 2243 this.getTarget().remove((SubstanceReferenceInformationTargetComponent) value); 2244 } else 2245 super.removeChild(name, value); 2246 2247 } 2248 2249 @Override 2250 public Base makeProperty(int hash, String name) throws FHIRException { 2251 switch (hash) { 2252 case 950398559: 2253 return getCommentElement(); 2254 case 3169045: 2255 return addGene(); 2256 case -94918105: 2257 return addGeneElement(); 2258 case 382350310: 2259 return addClassification(); 2260 case -880905839: 2261 return addTarget(); 2262 default: 2263 return super.makeProperty(hash, name); 2264 } 2265 2266 } 2267 2268 @Override 2269 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2270 switch (hash) { 2271 case 950398559: 2272 /* comment */ return new String[] { "string" }; 2273 case 3169045: 2274 /* gene */ return new String[] {}; 2275 case -94918105: 2276 /* geneElement */ return new String[] {}; 2277 case 382350310: 2278 /* classification */ return new String[] {}; 2279 case -880905839: 2280 /* target */ return new String[] {}; 2281 default: 2282 return super.getTypesForProperty(hash, name); 2283 } 2284 2285 } 2286 2287 @Override 2288 public Base addChild(String name) throws FHIRException { 2289 if (name.equals("comment")) { 2290 throw new FHIRException("Cannot call addChild on a singleton property SubstanceReferenceInformation.comment"); 2291 } else if (name.equals("gene")) { 2292 return addGene(); 2293 } else if (name.equals("geneElement")) { 2294 return addGeneElement(); 2295 } else if (name.equals("classification")) { 2296 return addClassification(); 2297 } else if (name.equals("target")) { 2298 return addTarget(); 2299 } else 2300 return super.addChild(name); 2301 } 2302 2303 public String fhirType() { 2304 return "SubstanceReferenceInformation"; 2305 2306 } 2307 2308 public SubstanceReferenceInformation copy() { 2309 SubstanceReferenceInformation dst = new SubstanceReferenceInformation(); 2310 copyValues(dst); 2311 return dst; 2312 } 2313 2314 public void copyValues(SubstanceReferenceInformation dst) { 2315 super.copyValues(dst); 2316 dst.comment = comment == null ? null : comment.copy(); 2317 if (gene != null) { 2318 dst.gene = new ArrayList<SubstanceReferenceInformationGeneComponent>(); 2319 for (SubstanceReferenceInformationGeneComponent i : gene) 2320 dst.gene.add(i.copy()); 2321 } 2322 ; 2323 if (geneElement != null) { 2324 dst.geneElement = new ArrayList<SubstanceReferenceInformationGeneElementComponent>(); 2325 for (SubstanceReferenceInformationGeneElementComponent i : geneElement) 2326 dst.geneElement.add(i.copy()); 2327 } 2328 ; 2329 if (classification != null) { 2330 dst.classification = new ArrayList<SubstanceReferenceInformationClassificationComponent>(); 2331 for (SubstanceReferenceInformationClassificationComponent i : classification) 2332 dst.classification.add(i.copy()); 2333 } 2334 ; 2335 if (target != null) { 2336 dst.target = new ArrayList<SubstanceReferenceInformationTargetComponent>(); 2337 for (SubstanceReferenceInformationTargetComponent i : target) 2338 dst.target.add(i.copy()); 2339 } 2340 ; 2341 } 2342 2343 protected SubstanceReferenceInformation typedCopy() { 2344 return copy(); 2345 } 2346 2347 @Override 2348 public boolean equalsDeep(Base other_) { 2349 if (!super.equalsDeep(other_)) 2350 return false; 2351 if (!(other_ instanceof SubstanceReferenceInformation)) 2352 return false; 2353 SubstanceReferenceInformation o = (SubstanceReferenceInformation) other_; 2354 return compareDeep(comment, o.comment, true) && compareDeep(gene, o.gene, true) 2355 && compareDeep(geneElement, o.geneElement, true) && compareDeep(classification, o.classification, true) 2356 && compareDeep(target, o.target, true); 2357 } 2358 2359 @Override 2360 public boolean equalsShallow(Base other_) { 2361 if (!super.equalsShallow(other_)) 2362 return false; 2363 if (!(other_ instanceof SubstanceReferenceInformation)) 2364 return false; 2365 SubstanceReferenceInformation o = (SubstanceReferenceInformation) other_; 2366 return compareValues(comment, o.comment, true); 2367 } 2368 2369 public boolean isEmpty() { 2370 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(comment, gene, geneElement, classification, target); 2371 } 2372 2373 @Override 2374 public ResourceType getResourceType() { 2375 return ResourceType.SubstanceReferenceInformation; 2376 } 2377 2378}