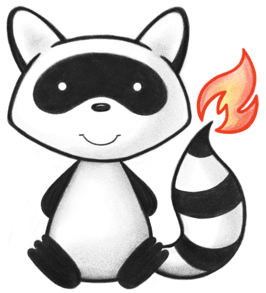
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044 045/** 046 * Source material shall capture information on the taxonomic and anatomical 047 * origins as well as the fraction of a material that can result in or can be 048 * modified to form a substance. This set of data elements shall be used to 049 * define polymer substances isolated from biological matrices. Taxonomic and 050 * anatomical origins shall be described using a controlled vocabulary as 051 * required. This information is captured for naturally derived polymers ( . 052 * starch) and structurally diverse substances. For Organisms belonging to the 053 * Kingdom Plantae the Substance level defines the fresh material of a single 054 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 055 * Herbal preparations, the fraction information will be captured at the 056 * Substance information level and additional information for herbal extracts 057 * will be captured at the Specified Substance Group 1 information level. See 058 * for further explanation the Substance Class: Structurally Diverse and the 059 * herbal annex. 060 */ 061@ResourceDef(name = "SubstanceSourceMaterial", profile = "http://hl7.org/fhir/StructureDefinition/SubstanceSourceMaterial") 062public class SubstanceSourceMaterial extends DomainResource { 063 064 @Block() 065 public static class SubstanceSourceMaterialFractionDescriptionComponent extends BackboneElement 066 implements IBaseBackboneElement { 067 /** 068 * This element is capturing information about the fraction of a plant part, or 069 * human plasma for fractionation. 070 */ 071 @Child(name = "fraction", type = { 072 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 073 @Description(shortDefinition = "This element is capturing information about the fraction of a plant part, or human plasma for fractionation", formalDefinition = "This element is capturing information about the fraction of a plant part, or human plasma for fractionation.") 074 protected StringType fraction; 075 076 /** 077 * The specific type of the material constituting the component. For Herbal 078 * preparations the particulars of the extracts (liquid/dry) is described in 079 * Specified Substance Group 1. 080 */ 081 @Child(name = "materialType", type = { 082 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 083 @Description(shortDefinition = "The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1", formalDefinition = "The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1.") 084 protected CodeableConcept materialType; 085 086 private static final long serialVersionUID = -1118226733L; 087 088 /** 089 * Constructor 090 */ 091 public SubstanceSourceMaterialFractionDescriptionComponent() { 092 super(); 093 } 094 095 /** 096 * @return {@link #fraction} (This element is capturing information about the 097 * fraction of a plant part, or human plasma for fractionation.). This 098 * is the underlying object with id, value and extensions. The accessor 099 * "getFraction" gives direct access to the value 100 */ 101 public StringType getFractionElement() { 102 if (this.fraction == null) 103 if (Configuration.errorOnAutoCreate()) 104 throw new Error("Attempt to auto-create SubstanceSourceMaterialFractionDescriptionComponent.fraction"); 105 else if (Configuration.doAutoCreate()) 106 this.fraction = new StringType(); // bb 107 return this.fraction; 108 } 109 110 public boolean hasFractionElement() { 111 return this.fraction != null && !this.fraction.isEmpty(); 112 } 113 114 public boolean hasFraction() { 115 return this.fraction != null && !this.fraction.isEmpty(); 116 } 117 118 /** 119 * @param value {@link #fraction} (This element is capturing information about 120 * the fraction of a plant part, or human plasma for 121 * fractionation.). This is the underlying object with id, value 122 * and extensions. The accessor "getFraction" gives direct access 123 * to the value 124 */ 125 public SubstanceSourceMaterialFractionDescriptionComponent setFractionElement(StringType value) { 126 this.fraction = value; 127 return this; 128 } 129 130 /** 131 * @return This element is capturing information about the fraction of a plant 132 * part, or human plasma for fractionation. 133 */ 134 public String getFraction() { 135 return this.fraction == null ? null : this.fraction.getValue(); 136 } 137 138 /** 139 * @param value This element is capturing information about the fraction of a 140 * plant part, or human plasma for fractionation. 141 */ 142 public SubstanceSourceMaterialFractionDescriptionComponent setFraction(String value) { 143 if (Utilities.noString(value)) 144 this.fraction = null; 145 else { 146 if (this.fraction == null) 147 this.fraction = new StringType(); 148 this.fraction.setValue(value); 149 } 150 return this; 151 } 152 153 /** 154 * @return {@link #materialType} (The specific type of the material constituting 155 * the component. For Herbal preparations the particulars of the 156 * extracts (liquid/dry) is described in Specified Substance Group 1.) 157 */ 158 public CodeableConcept getMaterialType() { 159 if (this.materialType == null) 160 if (Configuration.errorOnAutoCreate()) 161 throw new Error("Attempt to auto-create SubstanceSourceMaterialFractionDescriptionComponent.materialType"); 162 else if (Configuration.doAutoCreate()) 163 this.materialType = new CodeableConcept(); // cc 164 return this.materialType; 165 } 166 167 public boolean hasMaterialType() { 168 return this.materialType != null && !this.materialType.isEmpty(); 169 } 170 171 /** 172 * @param value {@link #materialType} (The specific type of the material 173 * constituting the component. For Herbal preparations the 174 * particulars of the extracts (liquid/dry) is described in 175 * Specified Substance Group 1.) 176 */ 177 public SubstanceSourceMaterialFractionDescriptionComponent setMaterialType(CodeableConcept value) { 178 this.materialType = value; 179 return this; 180 } 181 182 protected void listChildren(List<Property> children) { 183 super.listChildren(children); 184 children.add(new Property("fraction", "string", 185 "This element is capturing information about the fraction of a plant part, or human plasma for fractionation.", 186 0, 1, fraction)); 187 children.add(new Property("materialType", "CodeableConcept", 188 "The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1.", 189 0, 1, materialType)); 190 } 191 192 @Override 193 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 194 switch (_hash) { 195 case -1653751294: 196 /* fraction */ return new Property("fraction", "string", 197 "This element is capturing information about the fraction of a plant part, or human plasma for fractionation.", 198 0, 1, fraction); 199 case -2115601151: 200 /* materialType */ return new Property("materialType", "CodeableConcept", 201 "The specific type of the material constituting the component. For Herbal preparations the particulars of the extracts (liquid/dry) is described in Specified Substance Group 1.", 202 0, 1, materialType); 203 default: 204 return super.getNamedProperty(_hash, _name, _checkValid); 205 } 206 207 } 208 209 @Override 210 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 211 switch (hash) { 212 case -1653751294: 213 /* fraction */ return this.fraction == null ? new Base[0] : new Base[] { this.fraction }; // StringType 214 case -2115601151: 215 /* materialType */ return this.materialType == null ? new Base[0] : new Base[] { this.materialType }; // CodeableConcept 216 default: 217 return super.getProperty(hash, name, checkValid); 218 } 219 220 } 221 222 @Override 223 public Base setProperty(int hash, String name, Base value) throws FHIRException { 224 switch (hash) { 225 case -1653751294: // fraction 226 this.fraction = castToString(value); // StringType 227 return value; 228 case -2115601151: // materialType 229 this.materialType = castToCodeableConcept(value); // CodeableConcept 230 return value; 231 default: 232 return super.setProperty(hash, name, value); 233 } 234 235 } 236 237 @Override 238 public Base setProperty(String name, Base value) throws FHIRException { 239 if (name.equals("fraction")) { 240 this.fraction = castToString(value); // StringType 241 } else if (name.equals("materialType")) { 242 this.materialType = castToCodeableConcept(value); // CodeableConcept 243 } else 244 return super.setProperty(name, value); 245 return value; 246 } 247 248 @Override 249 public void removeChild(String name, Base value) throws FHIRException { 250 if (name.equals("fraction")) { 251 this.fraction = null; 252 } else if (name.equals("materialType")) { 253 this.materialType = null; 254 } else 255 super.removeChild(name, value); 256 257 } 258 259 @Override 260 public Base makeProperty(int hash, String name) throws FHIRException { 261 switch (hash) { 262 case -1653751294: 263 return getFractionElement(); 264 case -2115601151: 265 return getMaterialType(); 266 default: 267 return super.makeProperty(hash, name); 268 } 269 270 } 271 272 @Override 273 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 274 switch (hash) { 275 case -1653751294: 276 /* fraction */ return new String[] { "string" }; 277 case -2115601151: 278 /* materialType */ return new String[] { "CodeableConcept" }; 279 default: 280 return super.getTypesForProperty(hash, name); 281 } 282 283 } 284 285 @Override 286 public Base addChild(String name) throws FHIRException { 287 if (name.equals("fraction")) { 288 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.fraction"); 289 } else if (name.equals("materialType")) { 290 this.materialType = new CodeableConcept(); 291 return this.materialType; 292 } else 293 return super.addChild(name); 294 } 295 296 public SubstanceSourceMaterialFractionDescriptionComponent copy() { 297 SubstanceSourceMaterialFractionDescriptionComponent dst = new SubstanceSourceMaterialFractionDescriptionComponent(); 298 copyValues(dst); 299 return dst; 300 } 301 302 public void copyValues(SubstanceSourceMaterialFractionDescriptionComponent dst) { 303 super.copyValues(dst); 304 dst.fraction = fraction == null ? null : fraction.copy(); 305 dst.materialType = materialType == null ? null : materialType.copy(); 306 } 307 308 @Override 309 public boolean equalsDeep(Base other_) { 310 if (!super.equalsDeep(other_)) 311 return false; 312 if (!(other_ instanceof SubstanceSourceMaterialFractionDescriptionComponent)) 313 return false; 314 SubstanceSourceMaterialFractionDescriptionComponent o = (SubstanceSourceMaterialFractionDescriptionComponent) other_; 315 return compareDeep(fraction, o.fraction, true) && compareDeep(materialType, o.materialType, true); 316 } 317 318 @Override 319 public boolean equalsShallow(Base other_) { 320 if (!super.equalsShallow(other_)) 321 return false; 322 if (!(other_ instanceof SubstanceSourceMaterialFractionDescriptionComponent)) 323 return false; 324 SubstanceSourceMaterialFractionDescriptionComponent o = (SubstanceSourceMaterialFractionDescriptionComponent) other_; 325 return compareValues(fraction, o.fraction, true); 326 } 327 328 public boolean isEmpty() { 329 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(fraction, materialType); 330 } 331 332 public String fhirType() { 333 return "SubstanceSourceMaterial.fractionDescription"; 334 335 } 336 337 } 338 339 @Block() 340 public static class SubstanceSourceMaterialOrganismComponent extends BackboneElement implements IBaseBackboneElement { 341 /** 342 * The family of an organism shall be specified. 343 */ 344 @Child(name = "family", type = { 345 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 346 @Description(shortDefinition = "The family of an organism shall be specified", formalDefinition = "The family of an organism shall be specified.") 347 protected CodeableConcept family; 348 349 /** 350 * The genus of an organism shall be specified; refers to the Latin epithet of 351 * the genus element of the plant/animal scientific name; it is present in names 352 * for genera, species and infraspecies. 353 */ 354 @Child(name = "genus", type = { 355 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 356 @Description(shortDefinition = "The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies", formalDefinition = "The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies.") 357 protected CodeableConcept genus; 358 359 /** 360 * The species of an organism shall be specified; refers to the Latin epithet of 361 * the species of the plant/animal; it is present in names for species and 362 * infraspecies. 363 */ 364 @Child(name = "species", type = { 365 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 366 @Description(shortDefinition = "The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies", formalDefinition = "The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies.") 367 protected CodeableConcept species; 368 369 /** 370 * The Intraspecific type of an organism shall be specified. 371 */ 372 @Child(name = "intraspecificType", type = { 373 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 374 @Description(shortDefinition = "The Intraspecific type of an organism shall be specified", formalDefinition = "The Intraspecific type of an organism shall be specified.") 375 protected CodeableConcept intraspecificType; 376 377 /** 378 * The intraspecific description of an organism shall be specified based on a 379 * controlled vocabulary. For Influenza Vaccine, the intraspecific description 380 * shall contain the syntax of the antigen in line with the WHO convention. 381 */ 382 @Child(name = "intraspecificDescription", type = { 383 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 384 @Description(shortDefinition = "The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention", formalDefinition = "The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention.") 385 protected StringType intraspecificDescription; 386 387 /** 388 * 4.9.13.6.1 Author type (Conditional). 389 */ 390 @Child(name = "author", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 391 @Description(shortDefinition = "4.9.13.6.1 Author type (Conditional)", formalDefinition = "4.9.13.6.1 Author type (Conditional).") 392 protected List<SubstanceSourceMaterialOrganismAuthorComponent> author; 393 394 /** 395 * 4.9.13.8.1 Hybrid species maternal organism ID (Optional). 396 */ 397 @Child(name = "hybrid", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = true) 398 @Description(shortDefinition = "4.9.13.8.1 Hybrid species maternal organism ID (Optional)", formalDefinition = "4.9.13.8.1 Hybrid species maternal organism ID (Optional).") 399 protected SubstanceSourceMaterialOrganismHybridComponent hybrid; 400 401 /** 402 * 4.9.13.7.1 Kingdom (Conditional). 403 */ 404 @Child(name = "organismGeneral", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = true) 405 @Description(shortDefinition = "4.9.13.7.1 Kingdom (Conditional)", formalDefinition = "4.9.13.7.1 Kingdom (Conditional).") 406 protected SubstanceSourceMaterialOrganismOrganismGeneralComponent organismGeneral; 407 408 private static final long serialVersionUID = 941648312L; 409 410 /** 411 * Constructor 412 */ 413 public SubstanceSourceMaterialOrganismComponent() { 414 super(); 415 } 416 417 /** 418 * @return {@link #family} (The family of an organism shall be specified.) 419 */ 420 public CodeableConcept getFamily() { 421 if (this.family == null) 422 if (Configuration.errorOnAutoCreate()) 423 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.family"); 424 else if (Configuration.doAutoCreate()) 425 this.family = new CodeableConcept(); // cc 426 return this.family; 427 } 428 429 public boolean hasFamily() { 430 return this.family != null && !this.family.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #family} (The family of an organism shall be specified.) 435 */ 436 public SubstanceSourceMaterialOrganismComponent setFamily(CodeableConcept value) { 437 this.family = value; 438 return this; 439 } 440 441 /** 442 * @return {@link #genus} (The genus of an organism shall be specified; refers 443 * to the Latin epithet of the genus element of the plant/animal 444 * scientific name; it is present in names for genera, species and 445 * infraspecies.) 446 */ 447 public CodeableConcept getGenus() { 448 if (this.genus == null) 449 if (Configuration.errorOnAutoCreate()) 450 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.genus"); 451 else if (Configuration.doAutoCreate()) 452 this.genus = new CodeableConcept(); // cc 453 return this.genus; 454 } 455 456 public boolean hasGenus() { 457 return this.genus != null && !this.genus.isEmpty(); 458 } 459 460 /** 461 * @param value {@link #genus} (The genus of an organism shall be specified; 462 * refers to the Latin epithet of the genus element of the 463 * plant/animal scientific name; it is present in names for genera, 464 * species and infraspecies.) 465 */ 466 public SubstanceSourceMaterialOrganismComponent setGenus(CodeableConcept value) { 467 this.genus = value; 468 return this; 469 } 470 471 /** 472 * @return {@link #species} (The species of an organism shall be specified; 473 * refers to the Latin epithet of the species of the plant/animal; it is 474 * present in names for species and infraspecies.) 475 */ 476 public CodeableConcept getSpecies() { 477 if (this.species == null) 478 if (Configuration.errorOnAutoCreate()) 479 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.species"); 480 else if (Configuration.doAutoCreate()) 481 this.species = new CodeableConcept(); // cc 482 return this.species; 483 } 484 485 public boolean hasSpecies() { 486 return this.species != null && !this.species.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #species} (The species of an organism shall be specified; 491 * refers to the Latin epithet of the species of the plant/animal; 492 * it is present in names for species and infraspecies.) 493 */ 494 public SubstanceSourceMaterialOrganismComponent setSpecies(CodeableConcept value) { 495 this.species = value; 496 return this; 497 } 498 499 /** 500 * @return {@link #intraspecificType} (The Intraspecific type of an organism 501 * shall be specified.) 502 */ 503 public CodeableConcept getIntraspecificType() { 504 if (this.intraspecificType == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.intraspecificType"); 507 else if (Configuration.doAutoCreate()) 508 this.intraspecificType = new CodeableConcept(); // cc 509 return this.intraspecificType; 510 } 511 512 public boolean hasIntraspecificType() { 513 return this.intraspecificType != null && !this.intraspecificType.isEmpty(); 514 } 515 516 /** 517 * @param value {@link #intraspecificType} (The Intraspecific type of an 518 * organism shall be specified.) 519 */ 520 public SubstanceSourceMaterialOrganismComponent setIntraspecificType(CodeableConcept value) { 521 this.intraspecificType = value; 522 return this; 523 } 524 525 /** 526 * @return {@link #intraspecificDescription} (The intraspecific description of 527 * an organism shall be specified based on a controlled vocabulary. For 528 * Influenza Vaccine, the intraspecific description shall contain the 529 * syntax of the antigen in line with the WHO convention.). This is the 530 * underlying object with id, value and extensions. The accessor 531 * "getIntraspecificDescription" gives direct access to the value 532 */ 533 public StringType getIntraspecificDescriptionElement() { 534 if (this.intraspecificDescription == null) 535 if (Configuration.errorOnAutoCreate()) 536 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.intraspecificDescription"); 537 else if (Configuration.doAutoCreate()) 538 this.intraspecificDescription = new StringType(); // bb 539 return this.intraspecificDescription; 540 } 541 542 public boolean hasIntraspecificDescriptionElement() { 543 return this.intraspecificDescription != null && !this.intraspecificDescription.isEmpty(); 544 } 545 546 public boolean hasIntraspecificDescription() { 547 return this.intraspecificDescription != null && !this.intraspecificDescription.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #intraspecificDescription} (The intraspecific description 552 * of an organism shall be specified based on a controlled 553 * vocabulary. For Influenza Vaccine, the intraspecific description 554 * shall contain the syntax of the antigen in line with the WHO 555 * convention.). This is the underlying object with id, value and 556 * extensions. The accessor "getIntraspecificDescription" gives 557 * direct access to the value 558 */ 559 public SubstanceSourceMaterialOrganismComponent setIntraspecificDescriptionElement(StringType value) { 560 this.intraspecificDescription = value; 561 return this; 562 } 563 564 /** 565 * @return The intraspecific description of an organism shall be specified based 566 * on a controlled vocabulary. For Influenza Vaccine, the intraspecific 567 * description shall contain the syntax of the antigen in line with the 568 * WHO convention. 569 */ 570 public String getIntraspecificDescription() { 571 return this.intraspecificDescription == null ? null : this.intraspecificDescription.getValue(); 572 } 573 574 /** 575 * @param value The intraspecific description of an organism shall be specified 576 * based on a controlled vocabulary. For Influenza Vaccine, the 577 * intraspecific description shall contain the syntax of the 578 * antigen in line with the WHO convention. 579 */ 580 public SubstanceSourceMaterialOrganismComponent setIntraspecificDescription(String value) { 581 if (Utilities.noString(value)) 582 this.intraspecificDescription = null; 583 else { 584 if (this.intraspecificDescription == null) 585 this.intraspecificDescription = new StringType(); 586 this.intraspecificDescription.setValue(value); 587 } 588 return this; 589 } 590 591 /** 592 * @return {@link #author} (4.9.13.6.1 Author type (Conditional).) 593 */ 594 public List<SubstanceSourceMaterialOrganismAuthorComponent> getAuthor() { 595 if (this.author == null) 596 this.author = new ArrayList<SubstanceSourceMaterialOrganismAuthorComponent>(); 597 return this.author; 598 } 599 600 /** 601 * @return Returns a reference to <code>this</code> for easy method chaining 602 */ 603 public SubstanceSourceMaterialOrganismComponent setAuthor( 604 List<SubstanceSourceMaterialOrganismAuthorComponent> theAuthor) { 605 this.author = theAuthor; 606 return this; 607 } 608 609 public boolean hasAuthor() { 610 if (this.author == null) 611 return false; 612 for (SubstanceSourceMaterialOrganismAuthorComponent item : this.author) 613 if (!item.isEmpty()) 614 return true; 615 return false; 616 } 617 618 public SubstanceSourceMaterialOrganismAuthorComponent addAuthor() { // 3 619 SubstanceSourceMaterialOrganismAuthorComponent t = new SubstanceSourceMaterialOrganismAuthorComponent(); 620 if (this.author == null) 621 this.author = new ArrayList<SubstanceSourceMaterialOrganismAuthorComponent>(); 622 this.author.add(t); 623 return t; 624 } 625 626 public SubstanceSourceMaterialOrganismComponent addAuthor(SubstanceSourceMaterialOrganismAuthorComponent t) { // 3 627 if (t == null) 628 return this; 629 if (this.author == null) 630 this.author = new ArrayList<SubstanceSourceMaterialOrganismAuthorComponent>(); 631 this.author.add(t); 632 return this; 633 } 634 635 /** 636 * @return The first repetition of repeating field {@link #author}, creating it 637 * if it does not already exist 638 */ 639 public SubstanceSourceMaterialOrganismAuthorComponent getAuthorFirstRep() { 640 if (getAuthor().isEmpty()) { 641 addAuthor(); 642 } 643 return getAuthor().get(0); 644 } 645 646 /** 647 * @return {@link #hybrid} (4.9.13.8.1 Hybrid species maternal organism ID 648 * (Optional).) 649 */ 650 public SubstanceSourceMaterialOrganismHybridComponent getHybrid() { 651 if (this.hybrid == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.hybrid"); 654 else if (Configuration.doAutoCreate()) 655 this.hybrid = new SubstanceSourceMaterialOrganismHybridComponent(); // cc 656 return this.hybrid; 657 } 658 659 public boolean hasHybrid() { 660 return this.hybrid != null && !this.hybrid.isEmpty(); 661 } 662 663 /** 664 * @param value {@link #hybrid} (4.9.13.8.1 Hybrid species maternal organism ID 665 * (Optional).) 666 */ 667 public SubstanceSourceMaterialOrganismComponent setHybrid(SubstanceSourceMaterialOrganismHybridComponent value) { 668 this.hybrid = value; 669 return this; 670 } 671 672 /** 673 * @return {@link #organismGeneral} (4.9.13.7.1 Kingdom (Conditional).) 674 */ 675 public SubstanceSourceMaterialOrganismOrganismGeneralComponent getOrganismGeneral() { 676 if (this.organismGeneral == null) 677 if (Configuration.errorOnAutoCreate()) 678 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismComponent.organismGeneral"); 679 else if (Configuration.doAutoCreate()) 680 this.organismGeneral = new SubstanceSourceMaterialOrganismOrganismGeneralComponent(); // cc 681 return this.organismGeneral; 682 } 683 684 public boolean hasOrganismGeneral() { 685 return this.organismGeneral != null && !this.organismGeneral.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #organismGeneral} (4.9.13.7.1 Kingdom (Conditional).) 690 */ 691 public SubstanceSourceMaterialOrganismComponent setOrganismGeneral( 692 SubstanceSourceMaterialOrganismOrganismGeneralComponent value) { 693 this.organismGeneral = value; 694 return this; 695 } 696 697 protected void listChildren(List<Property> children) { 698 super.listChildren(children); 699 children.add( 700 new Property("family", "CodeableConcept", "The family of an organism shall be specified.", 0, 1, family)); 701 children.add(new Property("genus", "CodeableConcept", 702 "The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies.", 703 0, 1, genus)); 704 children.add(new Property("species", "CodeableConcept", 705 "The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies.", 706 0, 1, species)); 707 children.add(new Property("intraspecificType", "CodeableConcept", 708 "The Intraspecific type of an organism shall be specified.", 0, 1, intraspecificType)); 709 children.add(new Property("intraspecificDescription", "string", 710 "The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention.", 711 0, 1, intraspecificDescription)); 712 children.add( 713 new Property("author", "", "4.9.13.6.1 Author type (Conditional).", 0, java.lang.Integer.MAX_VALUE, author)); 714 children 715 .add(new Property("hybrid", "", "4.9.13.8.1 Hybrid species maternal organism ID (Optional).", 0, 1, hybrid)); 716 children.add(new Property("organismGeneral", "", "4.9.13.7.1 Kingdom (Conditional).", 0, 1, organismGeneral)); 717 } 718 719 @Override 720 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 721 switch (_hash) { 722 case -1281860764: 723 /* family */ return new Property("family", "CodeableConcept", "The family of an organism shall be specified.", 724 0, 1, family); 725 case 98241006: 726 /* genus */ return new Property("genus", "CodeableConcept", 727 "The genus of an organism shall be specified; refers to the Latin epithet of the genus element of the plant/animal scientific name; it is present in names for genera, species and infraspecies.", 728 0, 1, genus); 729 case -2008465092: 730 /* species */ return new Property("species", "CodeableConcept", 731 "The species of an organism shall be specified; refers to the Latin epithet of the species of the plant/animal; it is present in names for species and infraspecies.", 732 0, 1, species); 733 case 1717161194: 734 /* intraspecificType */ return new Property("intraspecificType", "CodeableConcept", 735 "The Intraspecific type of an organism shall be specified.", 0, 1, intraspecificType); 736 case -1473085364: 737 /* intraspecificDescription */ return new Property("intraspecificDescription", "string", 738 "The intraspecific description of an organism shall be specified based on a controlled vocabulary. For Influenza Vaccine, the intraspecific description shall contain the syntax of the antigen in line with the WHO convention.", 739 0, 1, intraspecificDescription); 740 case -1406328437: 741 /* author */ return new Property("author", "", "4.9.13.6.1 Author type (Conditional).", 0, 742 java.lang.Integer.MAX_VALUE, author); 743 case -1202757124: 744 /* hybrid */ return new Property("hybrid", "", "4.9.13.8.1 Hybrid species maternal organism ID (Optional).", 0, 745 1, hybrid); 746 case -865996874: 747 /* organismGeneral */ return new Property("organismGeneral", "", "4.9.13.7.1 Kingdom (Conditional).", 0, 1, 748 organismGeneral); 749 default: 750 return super.getNamedProperty(_hash, _name, _checkValid); 751 } 752 753 } 754 755 @Override 756 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 757 switch (hash) { 758 case -1281860764: 759 /* family */ return this.family == null ? new Base[0] : new Base[] { this.family }; // CodeableConcept 760 case 98241006: 761 /* genus */ return this.genus == null ? new Base[0] : new Base[] { this.genus }; // CodeableConcept 762 case -2008465092: 763 /* species */ return this.species == null ? new Base[0] : new Base[] { this.species }; // CodeableConcept 764 case 1717161194: 765 /* intraspecificType */ return this.intraspecificType == null ? new Base[0] 766 : new Base[] { this.intraspecificType }; // CodeableConcept 767 case -1473085364: 768 /* intraspecificDescription */ return this.intraspecificDescription == null ? new Base[0] 769 : new Base[] { this.intraspecificDescription }; // StringType 770 case -1406328437: 771 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // SubstanceSourceMaterialOrganismAuthorComponent 772 case -1202757124: 773 /* hybrid */ return this.hybrid == null ? new Base[0] : new Base[] { this.hybrid }; // SubstanceSourceMaterialOrganismHybridComponent 774 case -865996874: 775 /* organismGeneral */ return this.organismGeneral == null ? new Base[0] : new Base[] { this.organismGeneral }; // SubstanceSourceMaterialOrganismOrganismGeneralComponent 776 default: 777 return super.getProperty(hash, name, checkValid); 778 } 779 780 } 781 782 @Override 783 public Base setProperty(int hash, String name, Base value) throws FHIRException { 784 switch (hash) { 785 case -1281860764: // family 786 this.family = castToCodeableConcept(value); // CodeableConcept 787 return value; 788 case 98241006: // genus 789 this.genus = castToCodeableConcept(value); // CodeableConcept 790 return value; 791 case -2008465092: // species 792 this.species = castToCodeableConcept(value); // CodeableConcept 793 return value; 794 case 1717161194: // intraspecificType 795 this.intraspecificType = castToCodeableConcept(value); // CodeableConcept 796 return value; 797 case -1473085364: // intraspecificDescription 798 this.intraspecificDescription = castToString(value); // StringType 799 return value; 800 case -1406328437: // author 801 this.getAuthor().add((SubstanceSourceMaterialOrganismAuthorComponent) value); // SubstanceSourceMaterialOrganismAuthorComponent 802 return value; 803 case -1202757124: // hybrid 804 this.hybrid = (SubstanceSourceMaterialOrganismHybridComponent) value; // SubstanceSourceMaterialOrganismHybridComponent 805 return value; 806 case -865996874: // organismGeneral 807 this.organismGeneral = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) value; // SubstanceSourceMaterialOrganismOrganismGeneralComponent 808 return value; 809 default: 810 return super.setProperty(hash, name, value); 811 } 812 813 } 814 815 @Override 816 public Base setProperty(String name, Base value) throws FHIRException { 817 if (name.equals("family")) { 818 this.family = castToCodeableConcept(value); // CodeableConcept 819 } else if (name.equals("genus")) { 820 this.genus = castToCodeableConcept(value); // CodeableConcept 821 } else if (name.equals("species")) { 822 this.species = castToCodeableConcept(value); // CodeableConcept 823 } else if (name.equals("intraspecificType")) { 824 this.intraspecificType = castToCodeableConcept(value); // CodeableConcept 825 } else if (name.equals("intraspecificDescription")) { 826 this.intraspecificDescription = castToString(value); // StringType 827 } else if (name.equals("author")) { 828 this.getAuthor().add((SubstanceSourceMaterialOrganismAuthorComponent) value); 829 } else if (name.equals("hybrid")) { 830 this.hybrid = (SubstanceSourceMaterialOrganismHybridComponent) value; // SubstanceSourceMaterialOrganismHybridComponent 831 } else if (name.equals("organismGeneral")) { 832 this.organismGeneral = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) value; // SubstanceSourceMaterialOrganismOrganismGeneralComponent 833 } else 834 return super.setProperty(name, value); 835 return value; 836 } 837 838 @Override 839 public void removeChild(String name, Base value) throws FHIRException { 840 if (name.equals("family")) { 841 this.family = null; 842 } else if (name.equals("genus")) { 843 this.genus = null; 844 } else if (name.equals("species")) { 845 this.species = null; 846 } else if (name.equals("intraspecificType")) { 847 this.intraspecificType = null; 848 } else if (name.equals("intraspecificDescription")) { 849 this.intraspecificDescription = null; 850 } else if (name.equals("author")) { 851 this.getAuthor().remove((SubstanceSourceMaterialOrganismAuthorComponent) value); 852 } else if (name.equals("hybrid")) { 853 this.hybrid = (SubstanceSourceMaterialOrganismHybridComponent) value; // SubstanceSourceMaterialOrganismHybridComponent 854 } else if (name.equals("organismGeneral")) { 855 this.organismGeneral = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) value; // SubstanceSourceMaterialOrganismOrganismGeneralComponent 856 } else 857 super.removeChild(name, value); 858 859 } 860 861 @Override 862 public Base makeProperty(int hash, String name) throws FHIRException { 863 switch (hash) { 864 case -1281860764: 865 return getFamily(); 866 case 98241006: 867 return getGenus(); 868 case -2008465092: 869 return getSpecies(); 870 case 1717161194: 871 return getIntraspecificType(); 872 case -1473085364: 873 return getIntraspecificDescriptionElement(); 874 case -1406328437: 875 return addAuthor(); 876 case -1202757124: 877 return getHybrid(); 878 case -865996874: 879 return getOrganismGeneral(); 880 default: 881 return super.makeProperty(hash, name); 882 } 883 884 } 885 886 @Override 887 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 888 switch (hash) { 889 case -1281860764: 890 /* family */ return new String[] { "CodeableConcept" }; 891 case 98241006: 892 /* genus */ return new String[] { "CodeableConcept" }; 893 case -2008465092: 894 /* species */ return new String[] { "CodeableConcept" }; 895 case 1717161194: 896 /* intraspecificType */ return new String[] { "CodeableConcept" }; 897 case -1473085364: 898 /* intraspecificDescription */ return new String[] { "string" }; 899 case -1406328437: 900 /* author */ return new String[] {}; 901 case -1202757124: 902 /* hybrid */ return new String[] {}; 903 case -865996874: 904 /* organismGeneral */ return new String[] {}; 905 default: 906 return super.getTypesForProperty(hash, name); 907 } 908 909 } 910 911 @Override 912 public Base addChild(String name) throws FHIRException { 913 if (name.equals("family")) { 914 this.family = new CodeableConcept(); 915 return this.family; 916 } else if (name.equals("genus")) { 917 this.genus = new CodeableConcept(); 918 return this.genus; 919 } else if (name.equals("species")) { 920 this.species = new CodeableConcept(); 921 return this.species; 922 } else if (name.equals("intraspecificType")) { 923 this.intraspecificType = new CodeableConcept(); 924 return this.intraspecificType; 925 } else if (name.equals("intraspecificDescription")) { 926 throw new FHIRException( 927 "Cannot call addChild on a singleton property SubstanceSourceMaterial.intraspecificDescription"); 928 } else if (name.equals("author")) { 929 return addAuthor(); 930 } else if (name.equals("hybrid")) { 931 this.hybrid = new SubstanceSourceMaterialOrganismHybridComponent(); 932 return this.hybrid; 933 } else if (name.equals("organismGeneral")) { 934 this.organismGeneral = new SubstanceSourceMaterialOrganismOrganismGeneralComponent(); 935 return this.organismGeneral; 936 } else 937 return super.addChild(name); 938 } 939 940 public SubstanceSourceMaterialOrganismComponent copy() { 941 SubstanceSourceMaterialOrganismComponent dst = new SubstanceSourceMaterialOrganismComponent(); 942 copyValues(dst); 943 return dst; 944 } 945 946 public void copyValues(SubstanceSourceMaterialOrganismComponent dst) { 947 super.copyValues(dst); 948 dst.family = family == null ? null : family.copy(); 949 dst.genus = genus == null ? null : genus.copy(); 950 dst.species = species == null ? null : species.copy(); 951 dst.intraspecificType = intraspecificType == null ? null : intraspecificType.copy(); 952 dst.intraspecificDescription = intraspecificDescription == null ? null : intraspecificDescription.copy(); 953 if (author != null) { 954 dst.author = new ArrayList<SubstanceSourceMaterialOrganismAuthorComponent>(); 955 for (SubstanceSourceMaterialOrganismAuthorComponent i : author) 956 dst.author.add(i.copy()); 957 } 958 ; 959 dst.hybrid = hybrid == null ? null : hybrid.copy(); 960 dst.organismGeneral = organismGeneral == null ? null : organismGeneral.copy(); 961 } 962 963 @Override 964 public boolean equalsDeep(Base other_) { 965 if (!super.equalsDeep(other_)) 966 return false; 967 if (!(other_ instanceof SubstanceSourceMaterialOrganismComponent)) 968 return false; 969 SubstanceSourceMaterialOrganismComponent o = (SubstanceSourceMaterialOrganismComponent) other_; 970 return compareDeep(family, o.family, true) && compareDeep(genus, o.genus, true) 971 && compareDeep(species, o.species, true) && compareDeep(intraspecificType, o.intraspecificType, true) 972 && compareDeep(intraspecificDescription, o.intraspecificDescription, true) 973 && compareDeep(author, o.author, true) && compareDeep(hybrid, o.hybrid, true) 974 && compareDeep(organismGeneral, o.organismGeneral, true); 975 } 976 977 @Override 978 public boolean equalsShallow(Base other_) { 979 if (!super.equalsShallow(other_)) 980 return false; 981 if (!(other_ instanceof SubstanceSourceMaterialOrganismComponent)) 982 return false; 983 SubstanceSourceMaterialOrganismComponent o = (SubstanceSourceMaterialOrganismComponent) other_; 984 return compareValues(intraspecificDescription, o.intraspecificDescription, true); 985 } 986 987 public boolean isEmpty() { 988 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(family, genus, species, intraspecificType, 989 intraspecificDescription, author, hybrid, organismGeneral); 990 } 991 992 public String fhirType() { 993 return "SubstanceSourceMaterial.organism"; 994 995 } 996 997 } 998 999 @Block() 1000 public static class SubstanceSourceMaterialOrganismAuthorComponent extends BackboneElement 1001 implements IBaseBackboneElement { 1002 /** 1003 * The type of author of an organism species shall be specified. The 1004 * parenthetical author of an organism species refers to the first author who 1005 * published the plant/animal name (of any rank). The primary author of an 1006 * organism species refers to the first author(s), who validly published the 1007 * plant/animal name. 1008 */ 1009 @Child(name = "authorType", type = { 1010 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1011 @Description(shortDefinition = "The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name", formalDefinition = "The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name.") 1012 protected CodeableConcept authorType; 1013 1014 /** 1015 * The author of an organism species shall be specified. The author year of an 1016 * organism shall also be specified when applicable; refers to the year in which 1017 * the first author(s) published the infraspecific plant/animal name (of any 1018 * rank). 1019 */ 1020 @Child(name = "authorDescription", type = { 1021 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1022 @Description(shortDefinition = "The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank)", formalDefinition = "The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank).") 1023 protected StringType authorDescription; 1024 1025 private static final long serialVersionUID = 1429770120L; 1026 1027 /** 1028 * Constructor 1029 */ 1030 public SubstanceSourceMaterialOrganismAuthorComponent() { 1031 super(); 1032 } 1033 1034 /** 1035 * @return {@link #authorType} (The type of author of an organism species shall 1036 * be specified. The parenthetical author of an organism species refers 1037 * to the first author who published the plant/animal name (of any 1038 * rank). The primary author of an organism species refers to the first 1039 * author(s), who validly published the plant/animal name.) 1040 */ 1041 public CodeableConcept getAuthorType() { 1042 if (this.authorType == null) 1043 if (Configuration.errorOnAutoCreate()) 1044 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismAuthorComponent.authorType"); 1045 else if (Configuration.doAutoCreate()) 1046 this.authorType = new CodeableConcept(); // cc 1047 return this.authorType; 1048 } 1049 1050 public boolean hasAuthorType() { 1051 return this.authorType != null && !this.authorType.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #authorType} (The type of author of an organism species 1056 * shall be specified. The parenthetical author of an organism 1057 * species refers to the first author who published the 1058 * plant/animal name (of any rank). The primary author of an 1059 * organism species refers to the first author(s), who validly 1060 * published the plant/animal name.) 1061 */ 1062 public SubstanceSourceMaterialOrganismAuthorComponent setAuthorType(CodeableConcept value) { 1063 this.authorType = value; 1064 return this; 1065 } 1066 1067 /** 1068 * @return {@link #authorDescription} (The author of an organism species shall 1069 * be specified. The author year of an organism shall also be specified 1070 * when applicable; refers to the year in which the first author(s) 1071 * published the infraspecific plant/animal name (of any rank).). This 1072 * is the underlying object with id, value and extensions. The accessor 1073 * "getAuthorDescription" gives direct access to the value 1074 */ 1075 public StringType getAuthorDescriptionElement() { 1076 if (this.authorDescription == null) 1077 if (Configuration.errorOnAutoCreate()) 1078 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismAuthorComponent.authorDescription"); 1079 else if (Configuration.doAutoCreate()) 1080 this.authorDescription = new StringType(); // bb 1081 return this.authorDescription; 1082 } 1083 1084 public boolean hasAuthorDescriptionElement() { 1085 return this.authorDescription != null && !this.authorDescription.isEmpty(); 1086 } 1087 1088 public boolean hasAuthorDescription() { 1089 return this.authorDescription != null && !this.authorDescription.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #authorDescription} (The author of an organism species 1094 * shall be specified. The author year of an organism shall also be 1095 * specified when applicable; refers to the year in which the first 1096 * author(s) published the infraspecific plant/animal name (of any 1097 * rank).). This is the underlying object with id, value and 1098 * extensions. The accessor "getAuthorDescription" gives direct 1099 * access to the value 1100 */ 1101 public SubstanceSourceMaterialOrganismAuthorComponent setAuthorDescriptionElement(StringType value) { 1102 this.authorDescription = value; 1103 return this; 1104 } 1105 1106 /** 1107 * @return The author of an organism species shall be specified. The author year 1108 * of an organism shall also be specified when applicable; refers to the 1109 * year in which the first author(s) published the infraspecific 1110 * plant/animal name (of any rank). 1111 */ 1112 public String getAuthorDescription() { 1113 return this.authorDescription == null ? null : this.authorDescription.getValue(); 1114 } 1115 1116 /** 1117 * @param value The author of an organism species shall be specified. The author 1118 * year of an organism shall also be specified when applicable; 1119 * refers to the year in which the first author(s) published the 1120 * infraspecific plant/animal name (of any rank). 1121 */ 1122 public SubstanceSourceMaterialOrganismAuthorComponent setAuthorDescription(String value) { 1123 if (Utilities.noString(value)) 1124 this.authorDescription = null; 1125 else { 1126 if (this.authorDescription == null) 1127 this.authorDescription = new StringType(); 1128 this.authorDescription.setValue(value); 1129 } 1130 return this; 1131 } 1132 1133 protected void listChildren(List<Property> children) { 1134 super.listChildren(children); 1135 children.add(new Property("authorType", "CodeableConcept", 1136 "The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name.", 1137 0, 1, authorType)); 1138 children.add(new Property("authorDescription", "string", 1139 "The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank).", 1140 0, 1, authorDescription)); 1141 } 1142 1143 @Override 1144 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1145 switch (_hash) { 1146 case -1501337755: 1147 /* authorType */ return new Property("authorType", "CodeableConcept", 1148 "The type of author of an organism species shall be specified. The parenthetical author of an organism species refers to the first author who published the plant/animal name (of any rank). The primary author of an organism species refers to the first author(s), who validly published the plant/animal name.", 1149 0, 1, authorType); 1150 case -166185615: 1151 /* authorDescription */ return new Property("authorDescription", "string", 1152 "The author of an organism species shall be specified. The author year of an organism shall also be specified when applicable; refers to the year in which the first author(s) published the infraspecific plant/animal name (of any rank).", 1153 0, 1, authorDescription); 1154 default: 1155 return super.getNamedProperty(_hash, _name, _checkValid); 1156 } 1157 1158 } 1159 1160 @Override 1161 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1162 switch (hash) { 1163 case -1501337755: 1164 /* authorType */ return this.authorType == null ? new Base[0] : new Base[] { this.authorType }; // CodeableConcept 1165 case -166185615: 1166 /* authorDescription */ return this.authorDescription == null ? new Base[0] 1167 : new Base[] { this.authorDescription }; // StringType 1168 default: 1169 return super.getProperty(hash, name, checkValid); 1170 } 1171 1172 } 1173 1174 @Override 1175 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1176 switch (hash) { 1177 case -1501337755: // authorType 1178 this.authorType = castToCodeableConcept(value); // CodeableConcept 1179 return value; 1180 case -166185615: // authorDescription 1181 this.authorDescription = castToString(value); // StringType 1182 return value; 1183 default: 1184 return super.setProperty(hash, name, value); 1185 } 1186 1187 } 1188 1189 @Override 1190 public Base setProperty(String name, Base value) throws FHIRException { 1191 if (name.equals("authorType")) { 1192 this.authorType = castToCodeableConcept(value); // CodeableConcept 1193 } else if (name.equals("authorDescription")) { 1194 this.authorDescription = castToString(value); // StringType 1195 } else 1196 return super.setProperty(name, value); 1197 return value; 1198 } 1199 1200 @Override 1201 public void removeChild(String name, Base value) throws FHIRException { 1202 if (name.equals("authorType")) { 1203 this.authorType = null; 1204 } else if (name.equals("authorDescription")) { 1205 this.authorDescription = null; 1206 } else 1207 super.removeChild(name, value); 1208 1209 } 1210 1211 @Override 1212 public Base makeProperty(int hash, String name) throws FHIRException { 1213 switch (hash) { 1214 case -1501337755: 1215 return getAuthorType(); 1216 case -166185615: 1217 return getAuthorDescriptionElement(); 1218 default: 1219 return super.makeProperty(hash, name); 1220 } 1221 1222 } 1223 1224 @Override 1225 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1226 switch (hash) { 1227 case -1501337755: 1228 /* authorType */ return new String[] { "CodeableConcept" }; 1229 case -166185615: 1230 /* authorDescription */ return new String[] { "string" }; 1231 default: 1232 return super.getTypesForProperty(hash, name); 1233 } 1234 1235 } 1236 1237 @Override 1238 public Base addChild(String name) throws FHIRException { 1239 if (name.equals("authorType")) { 1240 this.authorType = new CodeableConcept(); 1241 return this.authorType; 1242 } else if (name.equals("authorDescription")) { 1243 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.authorDescription"); 1244 } else 1245 return super.addChild(name); 1246 } 1247 1248 public SubstanceSourceMaterialOrganismAuthorComponent copy() { 1249 SubstanceSourceMaterialOrganismAuthorComponent dst = new SubstanceSourceMaterialOrganismAuthorComponent(); 1250 copyValues(dst); 1251 return dst; 1252 } 1253 1254 public void copyValues(SubstanceSourceMaterialOrganismAuthorComponent dst) { 1255 super.copyValues(dst); 1256 dst.authorType = authorType == null ? null : authorType.copy(); 1257 dst.authorDescription = authorDescription == null ? null : authorDescription.copy(); 1258 } 1259 1260 @Override 1261 public boolean equalsDeep(Base other_) { 1262 if (!super.equalsDeep(other_)) 1263 return false; 1264 if (!(other_ instanceof SubstanceSourceMaterialOrganismAuthorComponent)) 1265 return false; 1266 SubstanceSourceMaterialOrganismAuthorComponent o = (SubstanceSourceMaterialOrganismAuthorComponent) other_; 1267 return compareDeep(authorType, o.authorType, true) && compareDeep(authorDescription, o.authorDescription, true); 1268 } 1269 1270 @Override 1271 public boolean equalsShallow(Base other_) { 1272 if (!super.equalsShallow(other_)) 1273 return false; 1274 if (!(other_ instanceof SubstanceSourceMaterialOrganismAuthorComponent)) 1275 return false; 1276 SubstanceSourceMaterialOrganismAuthorComponent o = (SubstanceSourceMaterialOrganismAuthorComponent) other_; 1277 return compareValues(authorDescription, o.authorDescription, true); 1278 } 1279 1280 public boolean isEmpty() { 1281 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(authorType, authorDescription); 1282 } 1283 1284 public String fhirType() { 1285 return "SubstanceSourceMaterial.organism.author"; 1286 1287 } 1288 1289 } 1290 1291 @Block() 1292 public static class SubstanceSourceMaterialOrganismHybridComponent extends BackboneElement 1293 implements IBaseBackboneElement { 1294 /** 1295 * The identifier of the maternal species constituting the hybrid organism shall 1296 * be specified based on a controlled vocabulary. For plants, the parents aren?t 1297 * always known, and it is unlikely that it will be known which is maternal and 1298 * which is paternal. 1299 */ 1300 @Child(name = "maternalOrganismId", type = { 1301 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1302 @Description(shortDefinition = "The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal", formalDefinition = "The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.") 1303 protected StringType maternalOrganismId; 1304 1305 /** 1306 * The name of the maternal species constituting the hybrid organism shall be 1307 * specified. For plants, the parents aren?t always known, and it is unlikely 1308 * that it will be known which is maternal and which is paternal. 1309 */ 1310 @Child(name = "maternalOrganismName", type = { 1311 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1312 @Description(shortDefinition = "The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal", formalDefinition = "The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.") 1313 protected StringType maternalOrganismName; 1314 1315 /** 1316 * The identifier of the paternal species constituting the hybrid organism shall 1317 * be specified based on a controlled vocabulary. 1318 */ 1319 @Child(name = "paternalOrganismId", type = { 1320 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1321 @Description(shortDefinition = "The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary", formalDefinition = "The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary.") 1322 protected StringType paternalOrganismId; 1323 1324 /** 1325 * The name of the paternal species constituting the hybrid organism shall be 1326 * specified. 1327 */ 1328 @Child(name = "paternalOrganismName", type = { 1329 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1330 @Description(shortDefinition = "The name of the paternal species constituting the hybrid organism shall be specified", formalDefinition = "The name of the paternal species constituting the hybrid organism shall be specified.") 1331 protected StringType paternalOrganismName; 1332 1333 /** 1334 * The hybrid type of an organism shall be specified. 1335 */ 1336 @Child(name = "hybridType", type = { 1337 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1338 @Description(shortDefinition = "The hybrid type of an organism shall be specified", formalDefinition = "The hybrid type of an organism shall be specified.") 1339 protected CodeableConcept hybridType; 1340 1341 private static final long serialVersionUID = 1981189787L; 1342 1343 /** 1344 * Constructor 1345 */ 1346 public SubstanceSourceMaterialOrganismHybridComponent() { 1347 super(); 1348 } 1349 1350 /** 1351 * @return {@link #maternalOrganismId} (The identifier of the maternal species 1352 * constituting the hybrid organism shall be specified based on a 1353 * controlled vocabulary. For plants, the parents aren?t always known, 1354 * and it is unlikely that it will be known which is maternal and which 1355 * is paternal.). This is the underlying object with id, value and 1356 * extensions. The accessor "getMaternalOrganismId" gives direct access 1357 * to the value 1358 */ 1359 public StringType getMaternalOrganismIdElement() { 1360 if (this.maternalOrganismId == null) 1361 if (Configuration.errorOnAutoCreate()) 1362 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.maternalOrganismId"); 1363 else if (Configuration.doAutoCreate()) 1364 this.maternalOrganismId = new StringType(); // bb 1365 return this.maternalOrganismId; 1366 } 1367 1368 public boolean hasMaternalOrganismIdElement() { 1369 return this.maternalOrganismId != null && !this.maternalOrganismId.isEmpty(); 1370 } 1371 1372 public boolean hasMaternalOrganismId() { 1373 return this.maternalOrganismId != null && !this.maternalOrganismId.isEmpty(); 1374 } 1375 1376 /** 1377 * @param value {@link #maternalOrganismId} (The identifier of the maternal 1378 * species constituting the hybrid organism shall be specified 1379 * based on a controlled vocabulary. For plants, the parents aren?t 1380 * always known, and it is unlikely that it will be known which is 1381 * maternal and which is paternal.). This is the underlying object 1382 * with id, value and extensions. The accessor 1383 * "getMaternalOrganismId" gives direct access to the value 1384 */ 1385 public SubstanceSourceMaterialOrganismHybridComponent setMaternalOrganismIdElement(StringType value) { 1386 this.maternalOrganismId = value; 1387 return this; 1388 } 1389 1390 /** 1391 * @return The identifier of the maternal species constituting the hybrid 1392 * organism shall be specified based on a controlled vocabulary. For 1393 * plants, the parents aren?t always known, and it is unlikely that it 1394 * will be known which is maternal and which is paternal. 1395 */ 1396 public String getMaternalOrganismId() { 1397 return this.maternalOrganismId == null ? null : this.maternalOrganismId.getValue(); 1398 } 1399 1400 /** 1401 * @param value The identifier of the maternal species constituting the hybrid 1402 * organism shall be specified based on a controlled vocabulary. 1403 * For plants, the parents aren?t always known, and it is unlikely 1404 * that it will be known which is maternal and which is paternal. 1405 */ 1406 public SubstanceSourceMaterialOrganismHybridComponent setMaternalOrganismId(String value) { 1407 if (Utilities.noString(value)) 1408 this.maternalOrganismId = null; 1409 else { 1410 if (this.maternalOrganismId == null) 1411 this.maternalOrganismId = new StringType(); 1412 this.maternalOrganismId.setValue(value); 1413 } 1414 return this; 1415 } 1416 1417 /** 1418 * @return {@link #maternalOrganismName} (The name of the maternal species 1419 * constituting the hybrid organism shall be specified. For plants, the 1420 * parents aren?t always known, and it is unlikely that it will be known 1421 * which is maternal and which is paternal.). This is the underlying 1422 * object with id, value and extensions. The accessor 1423 * "getMaternalOrganismName" gives direct access to the value 1424 */ 1425 public StringType getMaternalOrganismNameElement() { 1426 if (this.maternalOrganismName == null) 1427 if (Configuration.errorOnAutoCreate()) 1428 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.maternalOrganismName"); 1429 else if (Configuration.doAutoCreate()) 1430 this.maternalOrganismName = new StringType(); // bb 1431 return this.maternalOrganismName; 1432 } 1433 1434 public boolean hasMaternalOrganismNameElement() { 1435 return this.maternalOrganismName != null && !this.maternalOrganismName.isEmpty(); 1436 } 1437 1438 public boolean hasMaternalOrganismName() { 1439 return this.maternalOrganismName != null && !this.maternalOrganismName.isEmpty(); 1440 } 1441 1442 /** 1443 * @param value {@link #maternalOrganismName} (The name of the maternal species 1444 * constituting the hybrid organism shall be specified. For plants, 1445 * the parents aren?t always known, and it is unlikely that it will 1446 * be known which is maternal and which is paternal.). This is the 1447 * underlying object with id, value and extensions. The accessor 1448 * "getMaternalOrganismName" gives direct access to the value 1449 */ 1450 public SubstanceSourceMaterialOrganismHybridComponent setMaternalOrganismNameElement(StringType value) { 1451 this.maternalOrganismName = value; 1452 return this; 1453 } 1454 1455 /** 1456 * @return The name of the maternal species constituting the hybrid organism 1457 * shall be specified. For plants, the parents aren?t always known, and 1458 * it is unlikely that it will be known which is maternal and which is 1459 * paternal. 1460 */ 1461 public String getMaternalOrganismName() { 1462 return this.maternalOrganismName == null ? null : this.maternalOrganismName.getValue(); 1463 } 1464 1465 /** 1466 * @param value The name of the maternal species constituting the hybrid 1467 * organism shall be specified. For plants, the parents aren?t 1468 * always known, and it is unlikely that it will be known which is 1469 * maternal and which is paternal. 1470 */ 1471 public SubstanceSourceMaterialOrganismHybridComponent setMaternalOrganismName(String value) { 1472 if (Utilities.noString(value)) 1473 this.maternalOrganismName = null; 1474 else { 1475 if (this.maternalOrganismName == null) 1476 this.maternalOrganismName = new StringType(); 1477 this.maternalOrganismName.setValue(value); 1478 } 1479 return this; 1480 } 1481 1482 /** 1483 * @return {@link #paternalOrganismId} (The identifier of the paternal species 1484 * constituting the hybrid organism shall be specified based on a 1485 * controlled vocabulary.). This is the underlying object with id, value 1486 * and extensions. The accessor "getPaternalOrganismId" gives direct 1487 * access to the value 1488 */ 1489 public StringType getPaternalOrganismIdElement() { 1490 if (this.paternalOrganismId == null) 1491 if (Configuration.errorOnAutoCreate()) 1492 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.paternalOrganismId"); 1493 else if (Configuration.doAutoCreate()) 1494 this.paternalOrganismId = new StringType(); // bb 1495 return this.paternalOrganismId; 1496 } 1497 1498 public boolean hasPaternalOrganismIdElement() { 1499 return this.paternalOrganismId != null && !this.paternalOrganismId.isEmpty(); 1500 } 1501 1502 public boolean hasPaternalOrganismId() { 1503 return this.paternalOrganismId != null && !this.paternalOrganismId.isEmpty(); 1504 } 1505 1506 /** 1507 * @param value {@link #paternalOrganismId} (The identifier of the paternal 1508 * species constituting the hybrid organism shall be specified 1509 * based on a controlled vocabulary.). This is the underlying 1510 * object with id, value and extensions. The accessor 1511 * "getPaternalOrganismId" gives direct access to the value 1512 */ 1513 public SubstanceSourceMaterialOrganismHybridComponent setPaternalOrganismIdElement(StringType value) { 1514 this.paternalOrganismId = value; 1515 return this; 1516 } 1517 1518 /** 1519 * @return The identifier of the paternal species constituting the hybrid 1520 * organism shall be specified based on a controlled vocabulary. 1521 */ 1522 public String getPaternalOrganismId() { 1523 return this.paternalOrganismId == null ? null : this.paternalOrganismId.getValue(); 1524 } 1525 1526 /** 1527 * @param value The identifier of the paternal species constituting the hybrid 1528 * organism shall be specified based on a controlled vocabulary. 1529 */ 1530 public SubstanceSourceMaterialOrganismHybridComponent setPaternalOrganismId(String value) { 1531 if (Utilities.noString(value)) 1532 this.paternalOrganismId = null; 1533 else { 1534 if (this.paternalOrganismId == null) 1535 this.paternalOrganismId = new StringType(); 1536 this.paternalOrganismId.setValue(value); 1537 } 1538 return this; 1539 } 1540 1541 /** 1542 * @return {@link #paternalOrganismName} (The name of the paternal species 1543 * constituting the hybrid organism shall be specified.). This is the 1544 * underlying object with id, value and extensions. The accessor 1545 * "getPaternalOrganismName" gives direct access to the value 1546 */ 1547 public StringType getPaternalOrganismNameElement() { 1548 if (this.paternalOrganismName == null) 1549 if (Configuration.errorOnAutoCreate()) 1550 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.paternalOrganismName"); 1551 else if (Configuration.doAutoCreate()) 1552 this.paternalOrganismName = new StringType(); // bb 1553 return this.paternalOrganismName; 1554 } 1555 1556 public boolean hasPaternalOrganismNameElement() { 1557 return this.paternalOrganismName != null && !this.paternalOrganismName.isEmpty(); 1558 } 1559 1560 public boolean hasPaternalOrganismName() { 1561 return this.paternalOrganismName != null && !this.paternalOrganismName.isEmpty(); 1562 } 1563 1564 /** 1565 * @param value {@link #paternalOrganismName} (The name of the paternal species 1566 * constituting the hybrid organism shall be specified.). This is 1567 * the underlying object with id, value and extensions. The 1568 * accessor "getPaternalOrganismName" gives direct access to the 1569 * value 1570 */ 1571 public SubstanceSourceMaterialOrganismHybridComponent setPaternalOrganismNameElement(StringType value) { 1572 this.paternalOrganismName = value; 1573 return this; 1574 } 1575 1576 /** 1577 * @return The name of the paternal species constituting the hybrid organism 1578 * shall be specified. 1579 */ 1580 public String getPaternalOrganismName() { 1581 return this.paternalOrganismName == null ? null : this.paternalOrganismName.getValue(); 1582 } 1583 1584 /** 1585 * @param value The name of the paternal species constituting the hybrid 1586 * organism shall be specified. 1587 */ 1588 public SubstanceSourceMaterialOrganismHybridComponent setPaternalOrganismName(String value) { 1589 if (Utilities.noString(value)) 1590 this.paternalOrganismName = null; 1591 else { 1592 if (this.paternalOrganismName == null) 1593 this.paternalOrganismName = new StringType(); 1594 this.paternalOrganismName.setValue(value); 1595 } 1596 return this; 1597 } 1598 1599 /** 1600 * @return {@link #hybridType} (The hybrid type of an organism shall be 1601 * specified.) 1602 */ 1603 public CodeableConcept getHybridType() { 1604 if (this.hybridType == null) 1605 if (Configuration.errorOnAutoCreate()) 1606 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismHybridComponent.hybridType"); 1607 else if (Configuration.doAutoCreate()) 1608 this.hybridType = new CodeableConcept(); // cc 1609 return this.hybridType; 1610 } 1611 1612 public boolean hasHybridType() { 1613 return this.hybridType != null && !this.hybridType.isEmpty(); 1614 } 1615 1616 /** 1617 * @param value {@link #hybridType} (The hybrid type of an organism shall be 1618 * specified.) 1619 */ 1620 public SubstanceSourceMaterialOrganismHybridComponent setHybridType(CodeableConcept value) { 1621 this.hybridType = value; 1622 return this; 1623 } 1624 1625 protected void listChildren(List<Property> children) { 1626 super.listChildren(children); 1627 children.add(new Property("maternalOrganismId", "string", 1628 "The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.", 1629 0, 1, maternalOrganismId)); 1630 children.add(new Property("maternalOrganismName", "string", 1631 "The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.", 1632 0, 1, maternalOrganismName)); 1633 children.add(new Property("paternalOrganismId", "string", 1634 "The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary.", 1635 0, 1, paternalOrganismId)); 1636 children.add(new Property("paternalOrganismName", "string", 1637 "The name of the paternal species constituting the hybrid organism shall be specified.", 0, 1, 1638 paternalOrganismName)); 1639 children.add(new Property("hybridType", "CodeableConcept", "The hybrid type of an organism shall be specified.", 1640 0, 1, hybridType)); 1641 } 1642 1643 @Override 1644 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1645 switch (_hash) { 1646 case -1179977063: 1647 /* maternalOrganismId */ return new Property("maternalOrganismId", "string", 1648 "The identifier of the maternal species constituting the hybrid organism shall be specified based on a controlled vocabulary. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.", 1649 0, 1, maternalOrganismId); 1650 case -86441847: 1651 /* maternalOrganismName */ return new Property("maternalOrganismName", "string", 1652 "The name of the maternal species constituting the hybrid organism shall be specified. For plants, the parents aren?t always known, and it is unlikely that it will be known which is maternal and which is paternal.", 1653 0, 1, maternalOrganismName); 1654 case 123773174: 1655 /* paternalOrganismId */ return new Property("paternalOrganismId", "string", 1656 "The identifier of the paternal species constituting the hybrid organism shall be specified based on a controlled vocabulary.", 1657 0, 1, paternalOrganismId); 1658 case -1312914522: 1659 /* paternalOrganismName */ return new Property("paternalOrganismName", "string", 1660 "The name of the paternal species constituting the hybrid organism shall be specified.", 0, 1, 1661 paternalOrganismName); 1662 case 1572734806: 1663 /* hybridType */ return new Property("hybridType", "CodeableConcept", 1664 "The hybrid type of an organism shall be specified.", 0, 1, hybridType); 1665 default: 1666 return super.getNamedProperty(_hash, _name, _checkValid); 1667 } 1668 1669 } 1670 1671 @Override 1672 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1673 switch (hash) { 1674 case -1179977063: 1675 /* maternalOrganismId */ return this.maternalOrganismId == null ? new Base[0] 1676 : new Base[] { this.maternalOrganismId }; // StringType 1677 case -86441847: 1678 /* maternalOrganismName */ return this.maternalOrganismName == null ? new Base[0] 1679 : new Base[] { this.maternalOrganismName }; // StringType 1680 case 123773174: 1681 /* paternalOrganismId */ return this.paternalOrganismId == null ? new Base[0] 1682 : new Base[] { this.paternalOrganismId }; // StringType 1683 case -1312914522: 1684 /* paternalOrganismName */ return this.paternalOrganismName == null ? new Base[0] 1685 : new Base[] { this.paternalOrganismName }; // StringType 1686 case 1572734806: 1687 /* hybridType */ return this.hybridType == null ? new Base[0] : new Base[] { this.hybridType }; // CodeableConcept 1688 default: 1689 return super.getProperty(hash, name, checkValid); 1690 } 1691 1692 } 1693 1694 @Override 1695 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1696 switch (hash) { 1697 case -1179977063: // maternalOrganismId 1698 this.maternalOrganismId = castToString(value); // StringType 1699 return value; 1700 case -86441847: // maternalOrganismName 1701 this.maternalOrganismName = castToString(value); // StringType 1702 return value; 1703 case 123773174: // paternalOrganismId 1704 this.paternalOrganismId = castToString(value); // StringType 1705 return value; 1706 case -1312914522: // paternalOrganismName 1707 this.paternalOrganismName = castToString(value); // StringType 1708 return value; 1709 case 1572734806: // hybridType 1710 this.hybridType = castToCodeableConcept(value); // CodeableConcept 1711 return value; 1712 default: 1713 return super.setProperty(hash, name, value); 1714 } 1715 1716 } 1717 1718 @Override 1719 public Base setProperty(String name, Base value) throws FHIRException { 1720 if (name.equals("maternalOrganismId")) { 1721 this.maternalOrganismId = castToString(value); // StringType 1722 } else if (name.equals("maternalOrganismName")) { 1723 this.maternalOrganismName = castToString(value); // StringType 1724 } else if (name.equals("paternalOrganismId")) { 1725 this.paternalOrganismId = castToString(value); // StringType 1726 } else if (name.equals("paternalOrganismName")) { 1727 this.paternalOrganismName = castToString(value); // StringType 1728 } else if (name.equals("hybridType")) { 1729 this.hybridType = castToCodeableConcept(value); // CodeableConcept 1730 } else 1731 return super.setProperty(name, value); 1732 return value; 1733 } 1734 1735 @Override 1736 public void removeChild(String name, Base value) throws FHIRException { 1737 if (name.equals("maternalOrganismId")) { 1738 this.maternalOrganismId = null; 1739 } else if (name.equals("maternalOrganismName")) { 1740 this.maternalOrganismName = null; 1741 } else if (name.equals("paternalOrganismId")) { 1742 this.paternalOrganismId = null; 1743 } else if (name.equals("paternalOrganismName")) { 1744 this.paternalOrganismName = null; 1745 } else if (name.equals("hybridType")) { 1746 this.hybridType = null; 1747 } else 1748 super.removeChild(name, value); 1749 1750 } 1751 1752 @Override 1753 public Base makeProperty(int hash, String name) throws FHIRException { 1754 switch (hash) { 1755 case -1179977063: 1756 return getMaternalOrganismIdElement(); 1757 case -86441847: 1758 return getMaternalOrganismNameElement(); 1759 case 123773174: 1760 return getPaternalOrganismIdElement(); 1761 case -1312914522: 1762 return getPaternalOrganismNameElement(); 1763 case 1572734806: 1764 return getHybridType(); 1765 default: 1766 return super.makeProperty(hash, name); 1767 } 1768 1769 } 1770 1771 @Override 1772 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1773 switch (hash) { 1774 case -1179977063: 1775 /* maternalOrganismId */ return new String[] { "string" }; 1776 case -86441847: 1777 /* maternalOrganismName */ return new String[] { "string" }; 1778 case 123773174: 1779 /* paternalOrganismId */ return new String[] { "string" }; 1780 case -1312914522: 1781 /* paternalOrganismName */ return new String[] { "string" }; 1782 case 1572734806: 1783 /* hybridType */ return new String[] { "CodeableConcept" }; 1784 default: 1785 return super.getTypesForProperty(hash, name); 1786 } 1787 1788 } 1789 1790 @Override 1791 public Base addChild(String name) throws FHIRException { 1792 if (name.equals("maternalOrganismId")) { 1793 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.maternalOrganismId"); 1794 } else if (name.equals("maternalOrganismName")) { 1795 throw new FHIRException( 1796 "Cannot call addChild on a singleton property SubstanceSourceMaterial.maternalOrganismName"); 1797 } else if (name.equals("paternalOrganismId")) { 1798 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.paternalOrganismId"); 1799 } else if (name.equals("paternalOrganismName")) { 1800 throw new FHIRException( 1801 "Cannot call addChild on a singleton property SubstanceSourceMaterial.paternalOrganismName"); 1802 } else if (name.equals("hybridType")) { 1803 this.hybridType = new CodeableConcept(); 1804 return this.hybridType; 1805 } else 1806 return super.addChild(name); 1807 } 1808 1809 public SubstanceSourceMaterialOrganismHybridComponent copy() { 1810 SubstanceSourceMaterialOrganismHybridComponent dst = new SubstanceSourceMaterialOrganismHybridComponent(); 1811 copyValues(dst); 1812 return dst; 1813 } 1814 1815 public void copyValues(SubstanceSourceMaterialOrganismHybridComponent dst) { 1816 super.copyValues(dst); 1817 dst.maternalOrganismId = maternalOrganismId == null ? null : maternalOrganismId.copy(); 1818 dst.maternalOrganismName = maternalOrganismName == null ? null : maternalOrganismName.copy(); 1819 dst.paternalOrganismId = paternalOrganismId == null ? null : paternalOrganismId.copy(); 1820 dst.paternalOrganismName = paternalOrganismName == null ? null : paternalOrganismName.copy(); 1821 dst.hybridType = hybridType == null ? null : hybridType.copy(); 1822 } 1823 1824 @Override 1825 public boolean equalsDeep(Base other_) { 1826 if (!super.equalsDeep(other_)) 1827 return false; 1828 if (!(other_ instanceof SubstanceSourceMaterialOrganismHybridComponent)) 1829 return false; 1830 SubstanceSourceMaterialOrganismHybridComponent o = (SubstanceSourceMaterialOrganismHybridComponent) other_; 1831 return compareDeep(maternalOrganismId, o.maternalOrganismId, true) 1832 && compareDeep(maternalOrganismName, o.maternalOrganismName, true) 1833 && compareDeep(paternalOrganismId, o.paternalOrganismId, true) 1834 && compareDeep(paternalOrganismName, o.paternalOrganismName, true) 1835 && compareDeep(hybridType, o.hybridType, true); 1836 } 1837 1838 @Override 1839 public boolean equalsShallow(Base other_) { 1840 if (!super.equalsShallow(other_)) 1841 return false; 1842 if (!(other_ instanceof SubstanceSourceMaterialOrganismHybridComponent)) 1843 return false; 1844 SubstanceSourceMaterialOrganismHybridComponent o = (SubstanceSourceMaterialOrganismHybridComponent) other_; 1845 return compareValues(maternalOrganismId, o.maternalOrganismId, true) 1846 && compareValues(maternalOrganismName, o.maternalOrganismName, true) 1847 && compareValues(paternalOrganismId, o.paternalOrganismId, true) 1848 && compareValues(paternalOrganismName, o.paternalOrganismName, true); 1849 } 1850 1851 public boolean isEmpty() { 1852 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(maternalOrganismId, maternalOrganismName, 1853 paternalOrganismId, paternalOrganismName, hybridType); 1854 } 1855 1856 public String fhirType() { 1857 return "SubstanceSourceMaterial.organism.hybrid"; 1858 1859 } 1860 1861 } 1862 1863 @Block() 1864 public static class SubstanceSourceMaterialOrganismOrganismGeneralComponent extends BackboneElement 1865 implements IBaseBackboneElement { 1866 /** 1867 * The kingdom of an organism shall be specified. 1868 */ 1869 @Child(name = "kingdom", type = { 1870 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1871 @Description(shortDefinition = "The kingdom of an organism shall be specified", formalDefinition = "The kingdom of an organism shall be specified.") 1872 protected CodeableConcept kingdom; 1873 1874 /** 1875 * The phylum of an organism shall be specified. 1876 */ 1877 @Child(name = "phylum", type = { 1878 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1879 @Description(shortDefinition = "The phylum of an organism shall be specified", formalDefinition = "The phylum of an organism shall be specified.") 1880 protected CodeableConcept phylum; 1881 1882 /** 1883 * The class of an organism shall be specified. 1884 */ 1885 @Child(name = "class", type = { 1886 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1887 @Description(shortDefinition = "The class of an organism shall be specified", formalDefinition = "The class of an organism shall be specified.") 1888 protected CodeableConcept class_; 1889 1890 /** 1891 * The order of an organism shall be specified,. 1892 */ 1893 @Child(name = "order", type = { 1894 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1895 @Description(shortDefinition = "The order of an organism shall be specified,", formalDefinition = "The order of an organism shall be specified,.") 1896 protected CodeableConcept order; 1897 1898 private static final long serialVersionUID = 659838613L; 1899 1900 /** 1901 * Constructor 1902 */ 1903 public SubstanceSourceMaterialOrganismOrganismGeneralComponent() { 1904 super(); 1905 } 1906 1907 /** 1908 * @return {@link #kingdom} (The kingdom of an organism shall be specified.) 1909 */ 1910 public CodeableConcept getKingdom() { 1911 if (this.kingdom == null) 1912 if (Configuration.errorOnAutoCreate()) 1913 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismOrganismGeneralComponent.kingdom"); 1914 else if (Configuration.doAutoCreate()) 1915 this.kingdom = new CodeableConcept(); // cc 1916 return this.kingdom; 1917 } 1918 1919 public boolean hasKingdom() { 1920 return this.kingdom != null && !this.kingdom.isEmpty(); 1921 } 1922 1923 /** 1924 * @param value {@link #kingdom} (The kingdom of an organism shall be 1925 * specified.) 1926 */ 1927 public SubstanceSourceMaterialOrganismOrganismGeneralComponent setKingdom(CodeableConcept value) { 1928 this.kingdom = value; 1929 return this; 1930 } 1931 1932 /** 1933 * @return {@link #phylum} (The phylum of an organism shall be specified.) 1934 */ 1935 public CodeableConcept getPhylum() { 1936 if (this.phylum == null) 1937 if (Configuration.errorOnAutoCreate()) 1938 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismOrganismGeneralComponent.phylum"); 1939 else if (Configuration.doAutoCreate()) 1940 this.phylum = new CodeableConcept(); // cc 1941 return this.phylum; 1942 } 1943 1944 public boolean hasPhylum() { 1945 return this.phylum != null && !this.phylum.isEmpty(); 1946 } 1947 1948 /** 1949 * @param value {@link #phylum} (The phylum of an organism shall be specified.) 1950 */ 1951 public SubstanceSourceMaterialOrganismOrganismGeneralComponent setPhylum(CodeableConcept value) { 1952 this.phylum = value; 1953 return this; 1954 } 1955 1956 /** 1957 * @return {@link #class_} (The class of an organism shall be specified.) 1958 */ 1959 public CodeableConcept getClass_() { 1960 if (this.class_ == null) 1961 if (Configuration.errorOnAutoCreate()) 1962 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismOrganismGeneralComponent.class_"); 1963 else if (Configuration.doAutoCreate()) 1964 this.class_ = new CodeableConcept(); // cc 1965 return this.class_; 1966 } 1967 1968 public boolean hasClass_() { 1969 return this.class_ != null && !this.class_.isEmpty(); 1970 } 1971 1972 /** 1973 * @param value {@link #class_} (The class of an organism shall be specified.) 1974 */ 1975 public SubstanceSourceMaterialOrganismOrganismGeneralComponent setClass_(CodeableConcept value) { 1976 this.class_ = value; 1977 return this; 1978 } 1979 1980 /** 1981 * @return {@link #order} (The order of an organism shall be specified,.) 1982 */ 1983 public CodeableConcept getOrder() { 1984 if (this.order == null) 1985 if (Configuration.errorOnAutoCreate()) 1986 throw new Error("Attempt to auto-create SubstanceSourceMaterialOrganismOrganismGeneralComponent.order"); 1987 else if (Configuration.doAutoCreate()) 1988 this.order = new CodeableConcept(); // cc 1989 return this.order; 1990 } 1991 1992 public boolean hasOrder() { 1993 return this.order != null && !this.order.isEmpty(); 1994 } 1995 1996 /** 1997 * @param value {@link #order} (The order of an organism shall be specified,.) 1998 */ 1999 public SubstanceSourceMaterialOrganismOrganismGeneralComponent setOrder(CodeableConcept value) { 2000 this.order = value; 2001 return this; 2002 } 2003 2004 protected void listChildren(List<Property> children) { 2005 super.listChildren(children); 2006 children.add( 2007 new Property("kingdom", "CodeableConcept", "The kingdom of an organism shall be specified.", 0, 1, kingdom)); 2008 children.add( 2009 new Property("phylum", "CodeableConcept", "The phylum of an organism shall be specified.", 0, 1, phylum)); 2010 children 2011 .add(new Property("class", "CodeableConcept", "The class of an organism shall be specified.", 0, 1, class_)); 2012 children 2013 .add(new Property("order", "CodeableConcept", "The order of an organism shall be specified,.", 0, 1, order)); 2014 } 2015 2016 @Override 2017 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2018 switch (_hash) { 2019 case -710537653: 2020 /* kingdom */ return new Property("kingdom", "CodeableConcept", 2021 "The kingdom of an organism shall be specified.", 0, 1, kingdom); 2022 case -988743965: 2023 /* phylum */ return new Property("phylum", "CodeableConcept", "The phylum of an organism shall be specified.", 2024 0, 1, phylum); 2025 case 94742904: 2026 /* class */ return new Property("class", "CodeableConcept", "The class of an organism shall be specified.", 0, 2027 1, class_); 2028 case 106006350: 2029 /* order */ return new Property("order", "CodeableConcept", "The order of an organism shall be specified,.", 0, 2030 1, order); 2031 default: 2032 return super.getNamedProperty(_hash, _name, _checkValid); 2033 } 2034 2035 } 2036 2037 @Override 2038 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2039 switch (hash) { 2040 case -710537653: 2041 /* kingdom */ return this.kingdom == null ? new Base[0] : new Base[] { this.kingdom }; // CodeableConcept 2042 case -988743965: 2043 /* phylum */ return this.phylum == null ? new Base[0] : new Base[] { this.phylum }; // CodeableConcept 2044 case 94742904: 2045 /* class */ return this.class_ == null ? new Base[0] : new Base[] { this.class_ }; // CodeableConcept 2046 case 106006350: 2047 /* order */ return this.order == null ? new Base[0] : new Base[] { this.order }; // CodeableConcept 2048 default: 2049 return super.getProperty(hash, name, checkValid); 2050 } 2051 2052 } 2053 2054 @Override 2055 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2056 switch (hash) { 2057 case -710537653: // kingdom 2058 this.kingdom = castToCodeableConcept(value); // CodeableConcept 2059 return value; 2060 case -988743965: // phylum 2061 this.phylum = castToCodeableConcept(value); // CodeableConcept 2062 return value; 2063 case 94742904: // class 2064 this.class_ = castToCodeableConcept(value); // CodeableConcept 2065 return value; 2066 case 106006350: // order 2067 this.order = castToCodeableConcept(value); // CodeableConcept 2068 return value; 2069 default: 2070 return super.setProperty(hash, name, value); 2071 } 2072 2073 } 2074 2075 @Override 2076 public Base setProperty(String name, Base value) throws FHIRException { 2077 if (name.equals("kingdom")) { 2078 this.kingdom = castToCodeableConcept(value); // CodeableConcept 2079 } else if (name.equals("phylum")) { 2080 this.phylum = castToCodeableConcept(value); // CodeableConcept 2081 } else if (name.equals("class")) { 2082 this.class_ = castToCodeableConcept(value); // CodeableConcept 2083 } else if (name.equals("order")) { 2084 this.order = castToCodeableConcept(value); // CodeableConcept 2085 } else 2086 return super.setProperty(name, value); 2087 return value; 2088 } 2089 2090 @Override 2091 public void removeChild(String name, Base value) throws FHIRException { 2092 if (name.equals("kingdom")) { 2093 this.kingdom = null; 2094 } else if (name.equals("phylum")) { 2095 this.phylum = null; 2096 } else if (name.equals("class")) { 2097 this.class_ = null; 2098 } else if (name.equals("order")) { 2099 this.order = null; 2100 } else 2101 super.removeChild(name, value); 2102 2103 } 2104 2105 @Override 2106 public Base makeProperty(int hash, String name) throws FHIRException { 2107 switch (hash) { 2108 case -710537653: 2109 return getKingdom(); 2110 case -988743965: 2111 return getPhylum(); 2112 case 94742904: 2113 return getClass_(); 2114 case 106006350: 2115 return getOrder(); 2116 default: 2117 return super.makeProperty(hash, name); 2118 } 2119 2120 } 2121 2122 @Override 2123 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2124 switch (hash) { 2125 case -710537653: 2126 /* kingdom */ return new String[] { "CodeableConcept" }; 2127 case -988743965: 2128 /* phylum */ return new String[] { "CodeableConcept" }; 2129 case 94742904: 2130 /* class */ return new String[] { "CodeableConcept" }; 2131 case 106006350: 2132 /* order */ return new String[] { "CodeableConcept" }; 2133 default: 2134 return super.getTypesForProperty(hash, name); 2135 } 2136 2137 } 2138 2139 @Override 2140 public Base addChild(String name) throws FHIRException { 2141 if (name.equals("kingdom")) { 2142 this.kingdom = new CodeableConcept(); 2143 return this.kingdom; 2144 } else if (name.equals("phylum")) { 2145 this.phylum = new CodeableConcept(); 2146 return this.phylum; 2147 } else if (name.equals("class")) { 2148 this.class_ = new CodeableConcept(); 2149 return this.class_; 2150 } else if (name.equals("order")) { 2151 this.order = new CodeableConcept(); 2152 return this.order; 2153 } else 2154 return super.addChild(name); 2155 } 2156 2157 public SubstanceSourceMaterialOrganismOrganismGeneralComponent copy() { 2158 SubstanceSourceMaterialOrganismOrganismGeneralComponent dst = new SubstanceSourceMaterialOrganismOrganismGeneralComponent(); 2159 copyValues(dst); 2160 return dst; 2161 } 2162 2163 public void copyValues(SubstanceSourceMaterialOrganismOrganismGeneralComponent dst) { 2164 super.copyValues(dst); 2165 dst.kingdom = kingdom == null ? null : kingdom.copy(); 2166 dst.phylum = phylum == null ? null : phylum.copy(); 2167 dst.class_ = class_ == null ? null : class_.copy(); 2168 dst.order = order == null ? null : order.copy(); 2169 } 2170 2171 @Override 2172 public boolean equalsDeep(Base other_) { 2173 if (!super.equalsDeep(other_)) 2174 return false; 2175 if (!(other_ instanceof SubstanceSourceMaterialOrganismOrganismGeneralComponent)) 2176 return false; 2177 SubstanceSourceMaterialOrganismOrganismGeneralComponent o = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) other_; 2178 return compareDeep(kingdom, o.kingdom, true) && compareDeep(phylum, o.phylum, true) 2179 && compareDeep(class_, o.class_, true) && compareDeep(order, o.order, true); 2180 } 2181 2182 @Override 2183 public boolean equalsShallow(Base other_) { 2184 if (!super.equalsShallow(other_)) 2185 return false; 2186 if (!(other_ instanceof SubstanceSourceMaterialOrganismOrganismGeneralComponent)) 2187 return false; 2188 SubstanceSourceMaterialOrganismOrganismGeneralComponent o = (SubstanceSourceMaterialOrganismOrganismGeneralComponent) other_; 2189 return true; 2190 } 2191 2192 public boolean isEmpty() { 2193 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kingdom, phylum, class_, order); 2194 } 2195 2196 public String fhirType() { 2197 return "SubstanceSourceMaterial.organism.organismGeneral"; 2198 2199 } 2200 2201 } 2202 2203 @Block() 2204 public static class SubstanceSourceMaterialPartDescriptionComponent extends BackboneElement 2205 implements IBaseBackboneElement { 2206 /** 2207 * Entity of anatomical origin of source material within an organism. 2208 */ 2209 @Child(name = "part", type = { 2210 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2211 @Description(shortDefinition = "Entity of anatomical origin of source material within an organism", formalDefinition = "Entity of anatomical origin of source material within an organism.") 2212 protected CodeableConcept part; 2213 2214 /** 2215 * The detailed anatomic location when the part can be extracted from different 2216 * anatomical locations of the organism. Multiple alternative locations may 2217 * apply. 2218 */ 2219 @Child(name = "partLocation", type = { 2220 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2221 @Description(shortDefinition = "The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply", formalDefinition = "The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply.") 2222 protected CodeableConcept partLocation; 2223 2224 private static final long serialVersionUID = 308875915L; 2225 2226 /** 2227 * Constructor 2228 */ 2229 public SubstanceSourceMaterialPartDescriptionComponent() { 2230 super(); 2231 } 2232 2233 /** 2234 * @return {@link #part} (Entity of anatomical origin of source material within 2235 * an organism.) 2236 */ 2237 public CodeableConcept getPart() { 2238 if (this.part == null) 2239 if (Configuration.errorOnAutoCreate()) 2240 throw new Error("Attempt to auto-create SubstanceSourceMaterialPartDescriptionComponent.part"); 2241 else if (Configuration.doAutoCreate()) 2242 this.part = new CodeableConcept(); // cc 2243 return this.part; 2244 } 2245 2246 public boolean hasPart() { 2247 return this.part != null && !this.part.isEmpty(); 2248 } 2249 2250 /** 2251 * @param value {@link #part} (Entity of anatomical origin of source material 2252 * within an organism.) 2253 */ 2254 public SubstanceSourceMaterialPartDescriptionComponent setPart(CodeableConcept value) { 2255 this.part = value; 2256 return this; 2257 } 2258 2259 /** 2260 * @return {@link #partLocation} (The detailed anatomic location when the part 2261 * can be extracted from different anatomical locations of the organism. 2262 * Multiple alternative locations may apply.) 2263 */ 2264 public CodeableConcept getPartLocation() { 2265 if (this.partLocation == null) 2266 if (Configuration.errorOnAutoCreate()) 2267 throw new Error("Attempt to auto-create SubstanceSourceMaterialPartDescriptionComponent.partLocation"); 2268 else if (Configuration.doAutoCreate()) 2269 this.partLocation = new CodeableConcept(); // cc 2270 return this.partLocation; 2271 } 2272 2273 public boolean hasPartLocation() { 2274 return this.partLocation != null && !this.partLocation.isEmpty(); 2275 } 2276 2277 /** 2278 * @param value {@link #partLocation} (The detailed anatomic location when the 2279 * part can be extracted from different anatomical locations of the 2280 * organism. Multiple alternative locations may apply.) 2281 */ 2282 public SubstanceSourceMaterialPartDescriptionComponent setPartLocation(CodeableConcept value) { 2283 this.partLocation = value; 2284 return this; 2285 } 2286 2287 protected void listChildren(List<Property> children) { 2288 super.listChildren(children); 2289 children.add(new Property("part", "CodeableConcept", 2290 "Entity of anatomical origin of source material within an organism.", 0, 1, part)); 2291 children.add(new Property("partLocation", "CodeableConcept", 2292 "The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply.", 2293 0, 1, partLocation)); 2294 } 2295 2296 @Override 2297 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2298 switch (_hash) { 2299 case 3433459: 2300 /* part */ return new Property("part", "CodeableConcept", 2301 "Entity of anatomical origin of source material within an organism.", 0, 1, part); 2302 case 893437128: 2303 /* partLocation */ return new Property("partLocation", "CodeableConcept", 2304 "The detailed anatomic location when the part can be extracted from different anatomical locations of the organism. Multiple alternative locations may apply.", 2305 0, 1, partLocation); 2306 default: 2307 return super.getNamedProperty(_hash, _name, _checkValid); 2308 } 2309 2310 } 2311 2312 @Override 2313 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2314 switch (hash) { 2315 case 3433459: 2316 /* part */ return this.part == null ? new Base[0] : new Base[] { this.part }; // CodeableConcept 2317 case 893437128: 2318 /* partLocation */ return this.partLocation == null ? new Base[0] : new Base[] { this.partLocation }; // CodeableConcept 2319 default: 2320 return super.getProperty(hash, name, checkValid); 2321 } 2322 2323 } 2324 2325 @Override 2326 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2327 switch (hash) { 2328 case 3433459: // part 2329 this.part = castToCodeableConcept(value); // CodeableConcept 2330 return value; 2331 case 893437128: // partLocation 2332 this.partLocation = castToCodeableConcept(value); // CodeableConcept 2333 return value; 2334 default: 2335 return super.setProperty(hash, name, value); 2336 } 2337 2338 } 2339 2340 @Override 2341 public Base setProperty(String name, Base value) throws FHIRException { 2342 if (name.equals("part")) { 2343 this.part = castToCodeableConcept(value); // CodeableConcept 2344 } else if (name.equals("partLocation")) { 2345 this.partLocation = castToCodeableConcept(value); // CodeableConcept 2346 } else 2347 return super.setProperty(name, value); 2348 return value; 2349 } 2350 2351 @Override 2352 public void removeChild(String name, Base value) throws FHIRException { 2353 if (name.equals("part")) { 2354 this.part = null; 2355 } else if (name.equals("partLocation")) { 2356 this.partLocation = null; 2357 } else 2358 super.removeChild(name, value); 2359 2360 } 2361 2362 @Override 2363 public Base makeProperty(int hash, String name) throws FHIRException { 2364 switch (hash) { 2365 case 3433459: 2366 return getPart(); 2367 case 893437128: 2368 return getPartLocation(); 2369 default: 2370 return super.makeProperty(hash, name); 2371 } 2372 2373 } 2374 2375 @Override 2376 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2377 switch (hash) { 2378 case 3433459: 2379 /* part */ return new String[] { "CodeableConcept" }; 2380 case 893437128: 2381 /* partLocation */ return new String[] { "CodeableConcept" }; 2382 default: 2383 return super.getTypesForProperty(hash, name); 2384 } 2385 2386 } 2387 2388 @Override 2389 public Base addChild(String name) throws FHIRException { 2390 if (name.equals("part")) { 2391 this.part = new CodeableConcept(); 2392 return this.part; 2393 } else if (name.equals("partLocation")) { 2394 this.partLocation = new CodeableConcept(); 2395 return this.partLocation; 2396 } else 2397 return super.addChild(name); 2398 } 2399 2400 public SubstanceSourceMaterialPartDescriptionComponent copy() { 2401 SubstanceSourceMaterialPartDescriptionComponent dst = new SubstanceSourceMaterialPartDescriptionComponent(); 2402 copyValues(dst); 2403 return dst; 2404 } 2405 2406 public void copyValues(SubstanceSourceMaterialPartDescriptionComponent dst) { 2407 super.copyValues(dst); 2408 dst.part = part == null ? null : part.copy(); 2409 dst.partLocation = partLocation == null ? null : partLocation.copy(); 2410 } 2411 2412 @Override 2413 public boolean equalsDeep(Base other_) { 2414 if (!super.equalsDeep(other_)) 2415 return false; 2416 if (!(other_ instanceof SubstanceSourceMaterialPartDescriptionComponent)) 2417 return false; 2418 SubstanceSourceMaterialPartDescriptionComponent o = (SubstanceSourceMaterialPartDescriptionComponent) other_; 2419 return compareDeep(part, o.part, true) && compareDeep(partLocation, o.partLocation, true); 2420 } 2421 2422 @Override 2423 public boolean equalsShallow(Base other_) { 2424 if (!super.equalsShallow(other_)) 2425 return false; 2426 if (!(other_ instanceof SubstanceSourceMaterialPartDescriptionComponent)) 2427 return false; 2428 SubstanceSourceMaterialPartDescriptionComponent o = (SubstanceSourceMaterialPartDescriptionComponent) other_; 2429 return true; 2430 } 2431 2432 public boolean isEmpty() { 2433 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(part, partLocation); 2434 } 2435 2436 public String fhirType() { 2437 return "SubstanceSourceMaterial.partDescription"; 2438 2439 } 2440 2441 } 2442 2443 /** 2444 * General high level classification of the source material specific to the 2445 * origin of the material. 2446 */ 2447 @Child(name = "sourceMaterialClass", type = { 2448 CodeableConcept.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 2449 @Description(shortDefinition = "General high level classification of the source material specific to the origin of the material", formalDefinition = "General high level classification of the source material specific to the origin of the material.") 2450 protected CodeableConcept sourceMaterialClass; 2451 2452 /** 2453 * The type of the source material shall be specified based on a controlled 2454 * vocabulary. For vaccines, this subclause refers to the class of infectious 2455 * agent. 2456 */ 2457 @Child(name = "sourceMaterialType", type = { 2458 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2459 @Description(shortDefinition = "The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent", formalDefinition = "The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent.") 2460 protected CodeableConcept sourceMaterialType; 2461 2462 /** 2463 * The state of the source material when extracted. 2464 */ 2465 @Child(name = "sourceMaterialState", type = { 2466 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2467 @Description(shortDefinition = "The state of the source material when extracted", formalDefinition = "The state of the source material when extracted.") 2468 protected CodeableConcept sourceMaterialState; 2469 2470 /** 2471 * The unique identifier associated with the source material parent organism 2472 * shall be specified. 2473 */ 2474 @Child(name = "organismId", type = { 2475 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2476 @Description(shortDefinition = "The unique identifier associated with the source material parent organism shall be specified", formalDefinition = "The unique identifier associated with the source material parent organism shall be specified.") 2477 protected Identifier organismId; 2478 2479 /** 2480 * The organism accepted Scientific name shall be provided based on the organism 2481 * taxonomy. 2482 */ 2483 @Child(name = "organismName", type = { 2484 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2485 @Description(shortDefinition = "The organism accepted Scientific name shall be provided based on the organism taxonomy", formalDefinition = "The organism accepted Scientific name shall be provided based on the organism taxonomy.") 2486 protected StringType organismName; 2487 2488 /** 2489 * The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the 2490 * substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant). 2491 */ 2492 @Child(name = "parentSubstanceId", type = { 2493 Identifier.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2494 @Description(shortDefinition = "The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant)", formalDefinition = "The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant).") 2495 protected List<Identifier> parentSubstanceId; 2496 2497 /** 2498 * The parent substance of the Herbal Drug, or Herbal preparation. 2499 */ 2500 @Child(name = "parentSubstanceName", type = { 2501 StringType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2502 @Description(shortDefinition = "The parent substance of the Herbal Drug, or Herbal preparation", formalDefinition = "The parent substance of the Herbal Drug, or Herbal preparation.") 2503 protected List<StringType> parentSubstanceName; 2504 2505 /** 2506 * The country where the plant material is harvested or the countries where the 2507 * plasma is sourced from as laid down in accordance with the Plasma Master 2508 * File. For ?Plasma-derived substances? the attribute country of origin 2509 * provides information about the countries used for the manufacturing of the 2510 * Cryopoor plama or Crioprecipitate. 2511 */ 2512 @Child(name = "countryOfOrigin", type = { 2513 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2514 @Description(shortDefinition = "The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate", formalDefinition = "The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate.") 2515 protected List<CodeableConcept> countryOfOrigin; 2516 2517 /** 2518 * The place/region where the plant is harvested or the places/regions where the 2519 * animal source material has its habitat. 2520 */ 2521 @Child(name = "geographicalLocation", type = { 2522 StringType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2523 @Description(shortDefinition = "The place/region where the plant is harvested or the places/regions where the animal source material has its habitat", formalDefinition = "The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.") 2524 protected List<StringType> geographicalLocation; 2525 2526 /** 2527 * Stage of life for animals, plants, insects and microorganisms. This 2528 * information shall be provided only when the substance is significantly 2529 * different in these stages (e.g. foetal bovine serum). 2530 */ 2531 @Child(name = "developmentStage", type = { 2532 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 2533 @Description(shortDefinition = "Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum)", formalDefinition = "Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum).") 2534 protected CodeableConcept developmentStage; 2535 2536 /** 2537 * Many complex materials are fractions of parts of plants, animals, or 2538 * minerals. Fraction elements are often necessary to define both Substances and 2539 * Specified Group 1 Substances. For substances derived from Plants, fraction 2540 * information will be captured at the Substance information level ( . Oils, 2541 * Juices and Exudates). Additional information for Extracts, such as extraction 2542 * solvent composition, will be captured at the Specified Substance Group 1 2543 * information level. For plasma-derived products fraction information will be 2544 * captured at the Substance and the Specified Substance Group 1 levels. 2545 */ 2546 @Child(name = "fractionDescription", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2547 @Description(shortDefinition = "Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels", formalDefinition = "Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels.") 2548 protected List<SubstanceSourceMaterialFractionDescriptionComponent> fractionDescription; 2549 2550 /** 2551 * This subclause describes the organism which the substance is derived from. 2552 * For vaccines, the parent organism shall be specified based on these subclause 2553 * elements. As an example, full taxonomy will be described for the Substance 2554 * Name: ., Leaf. 2555 */ 2556 @Child(name = "organism", type = {}, order = 11, min = 0, max = 1, modifier = false, summary = true) 2557 @Description(shortDefinition = "This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf", formalDefinition = "This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf.") 2558 protected SubstanceSourceMaterialOrganismComponent organism; 2559 2560 /** 2561 * To do. 2562 */ 2563 @Child(name = "partDescription", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2564 @Description(shortDefinition = "To do", formalDefinition = "To do.") 2565 protected List<SubstanceSourceMaterialPartDescriptionComponent> partDescription; 2566 2567 private static final long serialVersionUID = 442657667L; 2568 2569 /** 2570 * Constructor 2571 */ 2572 public SubstanceSourceMaterial() { 2573 super(); 2574 } 2575 2576 /** 2577 * @return {@link #sourceMaterialClass} (General high level classification of 2578 * the source material specific to the origin of the material.) 2579 */ 2580 public CodeableConcept getSourceMaterialClass() { 2581 if (this.sourceMaterialClass == null) 2582 if (Configuration.errorOnAutoCreate()) 2583 throw new Error("Attempt to auto-create SubstanceSourceMaterial.sourceMaterialClass"); 2584 else if (Configuration.doAutoCreate()) 2585 this.sourceMaterialClass = new CodeableConcept(); // cc 2586 return this.sourceMaterialClass; 2587 } 2588 2589 public boolean hasSourceMaterialClass() { 2590 return this.sourceMaterialClass != null && !this.sourceMaterialClass.isEmpty(); 2591 } 2592 2593 /** 2594 * @param value {@link #sourceMaterialClass} (General high level classification 2595 * of the source material specific to the origin of the material.) 2596 */ 2597 public SubstanceSourceMaterial setSourceMaterialClass(CodeableConcept value) { 2598 this.sourceMaterialClass = value; 2599 return this; 2600 } 2601 2602 /** 2603 * @return {@link #sourceMaterialType} (The type of the source material shall be 2604 * specified based on a controlled vocabulary. For vaccines, this 2605 * subclause refers to the class of infectious agent.) 2606 */ 2607 public CodeableConcept getSourceMaterialType() { 2608 if (this.sourceMaterialType == null) 2609 if (Configuration.errorOnAutoCreate()) 2610 throw new Error("Attempt to auto-create SubstanceSourceMaterial.sourceMaterialType"); 2611 else if (Configuration.doAutoCreate()) 2612 this.sourceMaterialType = new CodeableConcept(); // cc 2613 return this.sourceMaterialType; 2614 } 2615 2616 public boolean hasSourceMaterialType() { 2617 return this.sourceMaterialType != null && !this.sourceMaterialType.isEmpty(); 2618 } 2619 2620 /** 2621 * @param value {@link #sourceMaterialType} (The type of the source material 2622 * shall be specified based on a controlled vocabulary. For 2623 * vaccines, this subclause refers to the class of infectious 2624 * agent.) 2625 */ 2626 public SubstanceSourceMaterial setSourceMaterialType(CodeableConcept value) { 2627 this.sourceMaterialType = value; 2628 return this; 2629 } 2630 2631 /** 2632 * @return {@link #sourceMaterialState} (The state of the source material when 2633 * extracted.) 2634 */ 2635 public CodeableConcept getSourceMaterialState() { 2636 if (this.sourceMaterialState == null) 2637 if (Configuration.errorOnAutoCreate()) 2638 throw new Error("Attempt to auto-create SubstanceSourceMaterial.sourceMaterialState"); 2639 else if (Configuration.doAutoCreate()) 2640 this.sourceMaterialState = new CodeableConcept(); // cc 2641 return this.sourceMaterialState; 2642 } 2643 2644 public boolean hasSourceMaterialState() { 2645 return this.sourceMaterialState != null && !this.sourceMaterialState.isEmpty(); 2646 } 2647 2648 /** 2649 * @param value {@link #sourceMaterialState} (The state of the source material 2650 * when extracted.) 2651 */ 2652 public SubstanceSourceMaterial setSourceMaterialState(CodeableConcept value) { 2653 this.sourceMaterialState = value; 2654 return this; 2655 } 2656 2657 /** 2658 * @return {@link #organismId} (The unique identifier associated with the source 2659 * material parent organism shall be specified.) 2660 */ 2661 public Identifier getOrganismId() { 2662 if (this.organismId == null) 2663 if (Configuration.errorOnAutoCreate()) 2664 throw new Error("Attempt to auto-create SubstanceSourceMaterial.organismId"); 2665 else if (Configuration.doAutoCreate()) 2666 this.organismId = new Identifier(); // cc 2667 return this.organismId; 2668 } 2669 2670 public boolean hasOrganismId() { 2671 return this.organismId != null && !this.organismId.isEmpty(); 2672 } 2673 2674 /** 2675 * @param value {@link #organismId} (The unique identifier associated with the 2676 * source material parent organism shall be specified.) 2677 */ 2678 public SubstanceSourceMaterial setOrganismId(Identifier value) { 2679 this.organismId = value; 2680 return this; 2681 } 2682 2683 /** 2684 * @return {@link #organismName} (The organism accepted Scientific name shall be 2685 * provided based on the organism taxonomy.). This is the underlying 2686 * object with id, value and extensions. The accessor "getOrganismName" 2687 * gives direct access to the value 2688 */ 2689 public StringType getOrganismNameElement() { 2690 if (this.organismName == null) 2691 if (Configuration.errorOnAutoCreate()) 2692 throw new Error("Attempt to auto-create SubstanceSourceMaterial.organismName"); 2693 else if (Configuration.doAutoCreate()) 2694 this.organismName = new StringType(); // bb 2695 return this.organismName; 2696 } 2697 2698 public boolean hasOrganismNameElement() { 2699 return this.organismName != null && !this.organismName.isEmpty(); 2700 } 2701 2702 public boolean hasOrganismName() { 2703 return this.organismName != null && !this.organismName.isEmpty(); 2704 } 2705 2706 /** 2707 * @param value {@link #organismName} (The organism accepted Scientific name 2708 * shall be provided based on the organism taxonomy.). This is the 2709 * underlying object with id, value and extensions. The accessor 2710 * "getOrganismName" gives direct access to the value 2711 */ 2712 public SubstanceSourceMaterial setOrganismNameElement(StringType value) { 2713 this.organismName = value; 2714 return this; 2715 } 2716 2717 /** 2718 * @return The organism accepted Scientific name shall be provided based on the 2719 * organism taxonomy. 2720 */ 2721 public String getOrganismName() { 2722 return this.organismName == null ? null : this.organismName.getValue(); 2723 } 2724 2725 /** 2726 * @param value The organism accepted Scientific name shall be provided based on 2727 * the organism taxonomy. 2728 */ 2729 public SubstanceSourceMaterial setOrganismName(String value) { 2730 if (Utilities.noString(value)) 2731 this.organismName = null; 2732 else { 2733 if (this.organismName == null) 2734 this.organismName = new StringType(); 2735 this.organismName.setValue(value); 2736 } 2737 return this; 2738 } 2739 2740 /** 2741 * @return {@link #parentSubstanceId} (The parent of the herbal drug Ginkgo 2742 * biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo 2743 * biloba L. or Ginkgo biloba L. (Whole plant).) 2744 */ 2745 public List<Identifier> getParentSubstanceId() { 2746 if (this.parentSubstanceId == null) 2747 this.parentSubstanceId = new ArrayList<Identifier>(); 2748 return this.parentSubstanceId; 2749 } 2750 2751 /** 2752 * @return Returns a reference to <code>this</code> for easy method chaining 2753 */ 2754 public SubstanceSourceMaterial setParentSubstanceId(List<Identifier> theParentSubstanceId) { 2755 this.parentSubstanceId = theParentSubstanceId; 2756 return this; 2757 } 2758 2759 public boolean hasParentSubstanceId() { 2760 if (this.parentSubstanceId == null) 2761 return false; 2762 for (Identifier item : this.parentSubstanceId) 2763 if (!item.isEmpty()) 2764 return true; 2765 return false; 2766 } 2767 2768 public Identifier addParentSubstanceId() { // 3 2769 Identifier t = new Identifier(); 2770 if (this.parentSubstanceId == null) 2771 this.parentSubstanceId = new ArrayList<Identifier>(); 2772 this.parentSubstanceId.add(t); 2773 return t; 2774 } 2775 2776 public SubstanceSourceMaterial addParentSubstanceId(Identifier t) { // 3 2777 if (t == null) 2778 return this; 2779 if (this.parentSubstanceId == null) 2780 this.parentSubstanceId = new ArrayList<Identifier>(); 2781 this.parentSubstanceId.add(t); 2782 return this; 2783 } 2784 2785 /** 2786 * @return The first repetition of repeating field {@link #parentSubstanceId}, 2787 * creating it if it does not already exist 2788 */ 2789 public Identifier getParentSubstanceIdFirstRep() { 2790 if (getParentSubstanceId().isEmpty()) { 2791 addParentSubstanceId(); 2792 } 2793 return getParentSubstanceId().get(0); 2794 } 2795 2796 /** 2797 * @return {@link #parentSubstanceName} (The parent substance of the Herbal 2798 * Drug, or Herbal preparation.) 2799 */ 2800 public List<StringType> getParentSubstanceName() { 2801 if (this.parentSubstanceName == null) 2802 this.parentSubstanceName = new ArrayList<StringType>(); 2803 return this.parentSubstanceName; 2804 } 2805 2806 /** 2807 * @return Returns a reference to <code>this</code> for easy method chaining 2808 */ 2809 public SubstanceSourceMaterial setParentSubstanceName(List<StringType> theParentSubstanceName) { 2810 this.parentSubstanceName = theParentSubstanceName; 2811 return this; 2812 } 2813 2814 public boolean hasParentSubstanceName() { 2815 if (this.parentSubstanceName == null) 2816 return false; 2817 for (StringType item : this.parentSubstanceName) 2818 if (!item.isEmpty()) 2819 return true; 2820 return false; 2821 } 2822 2823 /** 2824 * @return {@link #parentSubstanceName} (The parent substance of the Herbal 2825 * Drug, or Herbal preparation.) 2826 */ 2827 public StringType addParentSubstanceNameElement() {// 2 2828 StringType t = new StringType(); 2829 if (this.parentSubstanceName == null) 2830 this.parentSubstanceName = new ArrayList<StringType>(); 2831 this.parentSubstanceName.add(t); 2832 return t; 2833 } 2834 2835 /** 2836 * @param value {@link #parentSubstanceName} (The parent substance of the Herbal 2837 * Drug, or Herbal preparation.) 2838 */ 2839 public SubstanceSourceMaterial addParentSubstanceName(String value) { // 1 2840 StringType t = new StringType(); 2841 t.setValue(value); 2842 if (this.parentSubstanceName == null) 2843 this.parentSubstanceName = new ArrayList<StringType>(); 2844 this.parentSubstanceName.add(t); 2845 return this; 2846 } 2847 2848 /** 2849 * @param value {@link #parentSubstanceName} (The parent substance of the Herbal 2850 * Drug, or Herbal preparation.) 2851 */ 2852 public boolean hasParentSubstanceName(String value) { 2853 if (this.parentSubstanceName == null) 2854 return false; 2855 for (StringType v : this.parentSubstanceName) 2856 if (v.getValue().equals(value)) // string 2857 return true; 2858 return false; 2859 } 2860 2861 /** 2862 * @return {@link #countryOfOrigin} (The country where the plant material is 2863 * harvested or the countries where the plasma is sourced from as laid 2864 * down in accordance with the Plasma Master File. For ?Plasma-derived 2865 * substances? the attribute country of origin provides information 2866 * about the countries used for the manufacturing of the Cryopoor plama 2867 * or Crioprecipitate.) 2868 */ 2869 public List<CodeableConcept> getCountryOfOrigin() { 2870 if (this.countryOfOrigin == null) 2871 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 2872 return this.countryOfOrigin; 2873 } 2874 2875 /** 2876 * @return Returns a reference to <code>this</code> for easy method chaining 2877 */ 2878 public SubstanceSourceMaterial setCountryOfOrigin(List<CodeableConcept> theCountryOfOrigin) { 2879 this.countryOfOrigin = theCountryOfOrigin; 2880 return this; 2881 } 2882 2883 public boolean hasCountryOfOrigin() { 2884 if (this.countryOfOrigin == null) 2885 return false; 2886 for (CodeableConcept item : this.countryOfOrigin) 2887 if (!item.isEmpty()) 2888 return true; 2889 return false; 2890 } 2891 2892 public CodeableConcept addCountryOfOrigin() { // 3 2893 CodeableConcept t = new CodeableConcept(); 2894 if (this.countryOfOrigin == null) 2895 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 2896 this.countryOfOrigin.add(t); 2897 return t; 2898 } 2899 2900 public SubstanceSourceMaterial addCountryOfOrigin(CodeableConcept t) { // 3 2901 if (t == null) 2902 return this; 2903 if (this.countryOfOrigin == null) 2904 this.countryOfOrigin = new ArrayList<CodeableConcept>(); 2905 this.countryOfOrigin.add(t); 2906 return this; 2907 } 2908 2909 /** 2910 * @return The first repetition of repeating field {@link #countryOfOrigin}, 2911 * creating it if it does not already exist 2912 */ 2913 public CodeableConcept getCountryOfOriginFirstRep() { 2914 if (getCountryOfOrigin().isEmpty()) { 2915 addCountryOfOrigin(); 2916 } 2917 return getCountryOfOrigin().get(0); 2918 } 2919 2920 /** 2921 * @return {@link #geographicalLocation} (The place/region where the plant is 2922 * harvested or the places/regions where the animal source material has 2923 * its habitat.) 2924 */ 2925 public List<StringType> getGeographicalLocation() { 2926 if (this.geographicalLocation == null) 2927 this.geographicalLocation = new ArrayList<StringType>(); 2928 return this.geographicalLocation; 2929 } 2930 2931 /** 2932 * @return Returns a reference to <code>this</code> for easy method chaining 2933 */ 2934 public SubstanceSourceMaterial setGeographicalLocation(List<StringType> theGeographicalLocation) { 2935 this.geographicalLocation = theGeographicalLocation; 2936 return this; 2937 } 2938 2939 public boolean hasGeographicalLocation() { 2940 if (this.geographicalLocation == null) 2941 return false; 2942 for (StringType item : this.geographicalLocation) 2943 if (!item.isEmpty()) 2944 return true; 2945 return false; 2946 } 2947 2948 /** 2949 * @return {@link #geographicalLocation} (The place/region where the plant is 2950 * harvested or the places/regions where the animal source material has 2951 * its habitat.) 2952 */ 2953 public StringType addGeographicalLocationElement() {// 2 2954 StringType t = new StringType(); 2955 if (this.geographicalLocation == null) 2956 this.geographicalLocation = new ArrayList<StringType>(); 2957 this.geographicalLocation.add(t); 2958 return t; 2959 } 2960 2961 /** 2962 * @param value {@link #geographicalLocation} (The place/region where the plant 2963 * is harvested or the places/regions where the animal source 2964 * material has its habitat.) 2965 */ 2966 public SubstanceSourceMaterial addGeographicalLocation(String value) { // 1 2967 StringType t = new StringType(); 2968 t.setValue(value); 2969 if (this.geographicalLocation == null) 2970 this.geographicalLocation = new ArrayList<StringType>(); 2971 this.geographicalLocation.add(t); 2972 return this; 2973 } 2974 2975 /** 2976 * @param value {@link #geographicalLocation} (The place/region where the plant 2977 * is harvested or the places/regions where the animal source 2978 * material has its habitat.) 2979 */ 2980 public boolean hasGeographicalLocation(String value) { 2981 if (this.geographicalLocation == null) 2982 return false; 2983 for (StringType v : this.geographicalLocation) 2984 if (v.getValue().equals(value)) // string 2985 return true; 2986 return false; 2987 } 2988 2989 /** 2990 * @return {@link #developmentStage} (Stage of life for animals, plants, insects 2991 * and microorganisms. This information shall be provided only when the 2992 * substance is significantly different in these stages (e.g. foetal 2993 * bovine serum).) 2994 */ 2995 public CodeableConcept getDevelopmentStage() { 2996 if (this.developmentStage == null) 2997 if (Configuration.errorOnAutoCreate()) 2998 throw new Error("Attempt to auto-create SubstanceSourceMaterial.developmentStage"); 2999 else if (Configuration.doAutoCreate()) 3000 this.developmentStage = new CodeableConcept(); // cc 3001 return this.developmentStage; 3002 } 3003 3004 public boolean hasDevelopmentStage() { 3005 return this.developmentStage != null && !this.developmentStage.isEmpty(); 3006 } 3007 3008 /** 3009 * @param value {@link #developmentStage} (Stage of life for animals, plants, 3010 * insects and microorganisms. This information shall be provided 3011 * only when the substance is significantly different in these 3012 * stages (e.g. foetal bovine serum).) 3013 */ 3014 public SubstanceSourceMaterial setDevelopmentStage(CodeableConcept value) { 3015 this.developmentStage = value; 3016 return this; 3017 } 3018 3019 /** 3020 * @return {@link #fractionDescription} (Many complex materials are fractions of 3021 * parts of plants, animals, or minerals. Fraction elements are often 3022 * necessary to define both Substances and Specified Group 1 Substances. 3023 * For substances derived from Plants, fraction information will be 3024 * captured at the Substance information level ( . Oils, Juices and 3025 * Exudates). Additional information for Extracts, such as extraction 3026 * solvent composition, will be captured at the Specified Substance 3027 * Group 1 information level. For plasma-derived products fraction 3028 * information will be captured at the Substance and the Specified 3029 * Substance Group 1 levels.) 3030 */ 3031 public List<SubstanceSourceMaterialFractionDescriptionComponent> getFractionDescription() { 3032 if (this.fractionDescription == null) 3033 this.fractionDescription = new ArrayList<SubstanceSourceMaterialFractionDescriptionComponent>(); 3034 return this.fractionDescription; 3035 } 3036 3037 /** 3038 * @return Returns a reference to <code>this</code> for easy method chaining 3039 */ 3040 public SubstanceSourceMaterial setFractionDescription( 3041 List<SubstanceSourceMaterialFractionDescriptionComponent> theFractionDescription) { 3042 this.fractionDescription = theFractionDescription; 3043 return this; 3044 } 3045 3046 public boolean hasFractionDescription() { 3047 if (this.fractionDescription == null) 3048 return false; 3049 for (SubstanceSourceMaterialFractionDescriptionComponent item : this.fractionDescription) 3050 if (!item.isEmpty()) 3051 return true; 3052 return false; 3053 } 3054 3055 public SubstanceSourceMaterialFractionDescriptionComponent addFractionDescription() { // 3 3056 SubstanceSourceMaterialFractionDescriptionComponent t = new SubstanceSourceMaterialFractionDescriptionComponent(); 3057 if (this.fractionDescription == null) 3058 this.fractionDescription = new ArrayList<SubstanceSourceMaterialFractionDescriptionComponent>(); 3059 this.fractionDescription.add(t); 3060 return t; 3061 } 3062 3063 public SubstanceSourceMaterial addFractionDescription(SubstanceSourceMaterialFractionDescriptionComponent t) { // 3 3064 if (t == null) 3065 return this; 3066 if (this.fractionDescription == null) 3067 this.fractionDescription = new ArrayList<SubstanceSourceMaterialFractionDescriptionComponent>(); 3068 this.fractionDescription.add(t); 3069 return this; 3070 } 3071 3072 /** 3073 * @return The first repetition of repeating field {@link #fractionDescription}, 3074 * creating it if it does not already exist 3075 */ 3076 public SubstanceSourceMaterialFractionDescriptionComponent getFractionDescriptionFirstRep() { 3077 if (getFractionDescription().isEmpty()) { 3078 addFractionDescription(); 3079 } 3080 return getFractionDescription().get(0); 3081 } 3082 3083 /** 3084 * @return {@link #organism} (This subclause describes the organism which the 3085 * substance is derived from. For vaccines, the parent organism shall be 3086 * specified based on these subclause elements. As an example, full 3087 * taxonomy will be described for the Substance Name: ., Leaf.) 3088 */ 3089 public SubstanceSourceMaterialOrganismComponent getOrganism() { 3090 if (this.organism == null) 3091 if (Configuration.errorOnAutoCreate()) 3092 throw new Error("Attempt to auto-create SubstanceSourceMaterial.organism"); 3093 else if (Configuration.doAutoCreate()) 3094 this.organism = new SubstanceSourceMaterialOrganismComponent(); // cc 3095 return this.organism; 3096 } 3097 3098 public boolean hasOrganism() { 3099 return this.organism != null && !this.organism.isEmpty(); 3100 } 3101 3102 /** 3103 * @param value {@link #organism} (This subclause describes the organism which 3104 * the substance is derived from. For vaccines, the parent organism 3105 * shall be specified based on these subclause elements. As an 3106 * example, full taxonomy will be described for the Substance Name: 3107 * ., Leaf.) 3108 */ 3109 public SubstanceSourceMaterial setOrganism(SubstanceSourceMaterialOrganismComponent value) { 3110 this.organism = value; 3111 return this; 3112 } 3113 3114 /** 3115 * @return {@link #partDescription} (To do.) 3116 */ 3117 public List<SubstanceSourceMaterialPartDescriptionComponent> getPartDescription() { 3118 if (this.partDescription == null) 3119 this.partDescription = new ArrayList<SubstanceSourceMaterialPartDescriptionComponent>(); 3120 return this.partDescription; 3121 } 3122 3123 /** 3124 * @return Returns a reference to <code>this</code> for easy method chaining 3125 */ 3126 public SubstanceSourceMaterial setPartDescription( 3127 List<SubstanceSourceMaterialPartDescriptionComponent> thePartDescription) { 3128 this.partDescription = thePartDescription; 3129 return this; 3130 } 3131 3132 public boolean hasPartDescription() { 3133 if (this.partDescription == null) 3134 return false; 3135 for (SubstanceSourceMaterialPartDescriptionComponent item : this.partDescription) 3136 if (!item.isEmpty()) 3137 return true; 3138 return false; 3139 } 3140 3141 public SubstanceSourceMaterialPartDescriptionComponent addPartDescription() { // 3 3142 SubstanceSourceMaterialPartDescriptionComponent t = new SubstanceSourceMaterialPartDescriptionComponent(); 3143 if (this.partDescription == null) 3144 this.partDescription = new ArrayList<SubstanceSourceMaterialPartDescriptionComponent>(); 3145 this.partDescription.add(t); 3146 return t; 3147 } 3148 3149 public SubstanceSourceMaterial addPartDescription(SubstanceSourceMaterialPartDescriptionComponent t) { // 3 3150 if (t == null) 3151 return this; 3152 if (this.partDescription == null) 3153 this.partDescription = new ArrayList<SubstanceSourceMaterialPartDescriptionComponent>(); 3154 this.partDescription.add(t); 3155 return this; 3156 } 3157 3158 /** 3159 * @return The first repetition of repeating field {@link #partDescription}, 3160 * creating it if it does not already exist 3161 */ 3162 public SubstanceSourceMaterialPartDescriptionComponent getPartDescriptionFirstRep() { 3163 if (getPartDescription().isEmpty()) { 3164 addPartDescription(); 3165 } 3166 return getPartDescription().get(0); 3167 } 3168 3169 protected void listChildren(List<Property> children) { 3170 super.listChildren(children); 3171 children.add(new Property("sourceMaterialClass", "CodeableConcept", 3172 "General high level classification of the source material specific to the origin of the material.", 0, 1, 3173 sourceMaterialClass)); 3174 children.add(new Property("sourceMaterialType", "CodeableConcept", 3175 "The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent.", 3176 0, 1, sourceMaterialType)); 3177 children.add(new Property("sourceMaterialState", "CodeableConcept", 3178 "The state of the source material when extracted.", 0, 1, sourceMaterialState)); 3179 children.add(new Property("organismId", "Identifier", 3180 "The unique identifier associated with the source material parent organism shall be specified.", 0, 1, 3181 organismId)); 3182 children.add(new Property("organismName", "string", 3183 "The organism accepted Scientific name shall be provided based on the organism taxonomy.", 0, 1, organismName)); 3184 children.add(new Property("parentSubstanceId", "Identifier", 3185 "The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant).", 3186 0, java.lang.Integer.MAX_VALUE, parentSubstanceId)); 3187 children.add( 3188 new Property("parentSubstanceName", "string", "The parent substance of the Herbal Drug, or Herbal preparation.", 3189 0, java.lang.Integer.MAX_VALUE, parentSubstanceName)); 3190 children.add(new Property("countryOfOrigin", "CodeableConcept", 3191 "The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate.", 3192 0, java.lang.Integer.MAX_VALUE, countryOfOrigin)); 3193 children.add(new Property("geographicalLocation", "string", 3194 "The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.", 3195 0, java.lang.Integer.MAX_VALUE, geographicalLocation)); 3196 children.add(new Property("developmentStage", "CodeableConcept", 3197 "Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum).", 3198 0, 1, developmentStage)); 3199 children.add(new Property("fractionDescription", "", 3200 "Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels.", 3201 0, java.lang.Integer.MAX_VALUE, fractionDescription)); 3202 children.add(new Property("organism", "", 3203 "This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf.", 3204 0, 1, organism)); 3205 children.add(new Property("partDescription", "", "To do.", 0, java.lang.Integer.MAX_VALUE, partDescription)); 3206 } 3207 3208 @Override 3209 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3210 switch (_hash) { 3211 case -1253081034: 3212 /* sourceMaterialClass */ return new Property("sourceMaterialClass", "CodeableConcept", 3213 "General high level classification of the source material specific to the origin of the material.", 0, 1, 3214 sourceMaterialClass); 3215 case 1622665404: 3216 /* sourceMaterialType */ return new Property("sourceMaterialType", "CodeableConcept", 3217 "The type of the source material shall be specified based on a controlled vocabulary. For vaccines, this subclause refers to the class of infectious agent.", 3218 0, 1, sourceMaterialType); 3219 case -1238066353: 3220 /* sourceMaterialState */ return new Property("sourceMaterialState", "CodeableConcept", 3221 "The state of the source material when extracted.", 0, 1, sourceMaterialState); 3222 case -1965449843: 3223 /* organismId */ return new Property("organismId", "Identifier", 3224 "The unique identifier associated with the source material parent organism shall be specified.", 0, 1, 3225 organismId); 3226 case 988460669: 3227 /* organismName */ return new Property("organismName", "string", 3228 "The organism accepted Scientific name shall be provided based on the organism taxonomy.", 0, 1, 3229 organismName); 3230 case -675437663: 3231 /* parentSubstanceId */ return new Property("parentSubstanceId", "Identifier", 3232 "The parent of the herbal drug Ginkgo biloba, Leaf is the substance ID of the substance (fresh) of Ginkgo biloba L. or Ginkgo biloba L. (Whole plant).", 3233 0, java.lang.Integer.MAX_VALUE, parentSubstanceId); 3234 case -555382895: 3235 /* parentSubstanceName */ return new Property("parentSubstanceName", "string", 3236 "The parent substance of the Herbal Drug, or Herbal preparation.", 0, java.lang.Integer.MAX_VALUE, 3237 parentSubstanceName); 3238 case 57176467: 3239 /* countryOfOrigin */ return new Property("countryOfOrigin", "CodeableConcept", 3240 "The country where the plant material is harvested or the countries where the plasma is sourced from as laid down in accordance with the Plasma Master File. For ?Plasma-derived substances? the attribute country of origin provides information about the countries used for the manufacturing of the Cryopoor plama or Crioprecipitate.", 3241 0, java.lang.Integer.MAX_VALUE, countryOfOrigin); 3242 case -1988836681: 3243 /* geographicalLocation */ return new Property("geographicalLocation", "string", 3244 "The place/region where the plant is harvested or the places/regions where the animal source material has its habitat.", 3245 0, java.lang.Integer.MAX_VALUE, geographicalLocation); 3246 case 391529091: 3247 /* developmentStage */ return new Property("developmentStage", "CodeableConcept", 3248 "Stage of life for animals, plants, insects and microorganisms. This information shall be provided only when the substance is significantly different in these stages (e.g. foetal bovine serum).", 3249 0, 1, developmentStage); 3250 case 1472689306: 3251 /* fractionDescription */ return new Property("fractionDescription", "", 3252 "Many complex materials are fractions of parts of plants, animals, or minerals. Fraction elements are often necessary to define both Substances and Specified Group 1 Substances. For substances derived from Plants, fraction information will be captured at the Substance information level ( . Oils, Juices and Exudates). Additional information for Extracts, such as extraction solvent composition, will be captured at the Specified Substance Group 1 information level. For plasma-derived products fraction information will be captured at the Substance and the Specified Substance Group 1 levels.", 3253 0, java.lang.Integer.MAX_VALUE, fractionDescription); 3254 case 1316389074: 3255 /* organism */ return new Property("organism", "", 3256 "This subclause describes the organism which the substance is derived from. For vaccines, the parent organism shall be specified based on these subclause elements. As an example, full taxonomy will be described for the Substance Name: ., Leaf.", 3257 0, 1, organism); 3258 case -1803623927: 3259 /* partDescription */ return new Property("partDescription", "", "To do.", 0, java.lang.Integer.MAX_VALUE, 3260 partDescription); 3261 default: 3262 return super.getNamedProperty(_hash, _name, _checkValid); 3263 } 3264 3265 } 3266 3267 @Override 3268 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3269 switch (hash) { 3270 case -1253081034: 3271 /* sourceMaterialClass */ return this.sourceMaterialClass == null ? new Base[0] 3272 : new Base[] { this.sourceMaterialClass }; // CodeableConcept 3273 case 1622665404: 3274 /* sourceMaterialType */ return this.sourceMaterialType == null ? new Base[0] 3275 : new Base[] { this.sourceMaterialType }; // CodeableConcept 3276 case -1238066353: 3277 /* sourceMaterialState */ return this.sourceMaterialState == null ? new Base[0] 3278 : new Base[] { this.sourceMaterialState }; // CodeableConcept 3279 case -1965449843: 3280 /* organismId */ return this.organismId == null ? new Base[0] : new Base[] { this.organismId }; // Identifier 3281 case 988460669: 3282 /* organismName */ return this.organismName == null ? new Base[0] : new Base[] { this.organismName }; // StringType 3283 case -675437663: 3284 /* parentSubstanceId */ return this.parentSubstanceId == null ? new Base[0] 3285 : this.parentSubstanceId.toArray(new Base[this.parentSubstanceId.size()]); // Identifier 3286 case -555382895: 3287 /* parentSubstanceName */ return this.parentSubstanceName == null ? new Base[0] 3288 : this.parentSubstanceName.toArray(new Base[this.parentSubstanceName.size()]); // StringType 3289 case 57176467: 3290 /* countryOfOrigin */ return this.countryOfOrigin == null ? new Base[0] 3291 : this.countryOfOrigin.toArray(new Base[this.countryOfOrigin.size()]); // CodeableConcept 3292 case -1988836681: 3293 /* geographicalLocation */ return this.geographicalLocation == null ? new Base[0] 3294 : this.geographicalLocation.toArray(new Base[this.geographicalLocation.size()]); // StringType 3295 case 391529091: 3296 /* developmentStage */ return this.developmentStage == null ? new Base[0] : new Base[] { this.developmentStage }; // CodeableConcept 3297 case 1472689306: 3298 /* fractionDescription */ return this.fractionDescription == null ? new Base[0] 3299 : this.fractionDescription.toArray(new Base[this.fractionDescription.size()]); // SubstanceSourceMaterialFractionDescriptionComponent 3300 case 1316389074: 3301 /* organism */ return this.organism == null ? new Base[0] : new Base[] { this.organism }; // SubstanceSourceMaterialOrganismComponent 3302 case -1803623927: 3303 /* partDescription */ return this.partDescription == null ? new Base[0] 3304 : this.partDescription.toArray(new Base[this.partDescription.size()]); // SubstanceSourceMaterialPartDescriptionComponent 3305 default: 3306 return super.getProperty(hash, name, checkValid); 3307 } 3308 3309 } 3310 3311 @Override 3312 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3313 switch (hash) { 3314 case -1253081034: // sourceMaterialClass 3315 this.sourceMaterialClass = castToCodeableConcept(value); // CodeableConcept 3316 return value; 3317 case 1622665404: // sourceMaterialType 3318 this.sourceMaterialType = castToCodeableConcept(value); // CodeableConcept 3319 return value; 3320 case -1238066353: // sourceMaterialState 3321 this.sourceMaterialState = castToCodeableConcept(value); // CodeableConcept 3322 return value; 3323 case -1965449843: // organismId 3324 this.organismId = castToIdentifier(value); // Identifier 3325 return value; 3326 case 988460669: // organismName 3327 this.organismName = castToString(value); // StringType 3328 return value; 3329 case -675437663: // parentSubstanceId 3330 this.getParentSubstanceId().add(castToIdentifier(value)); // Identifier 3331 return value; 3332 case -555382895: // parentSubstanceName 3333 this.getParentSubstanceName().add(castToString(value)); // StringType 3334 return value; 3335 case 57176467: // countryOfOrigin 3336 this.getCountryOfOrigin().add(castToCodeableConcept(value)); // CodeableConcept 3337 return value; 3338 case -1988836681: // geographicalLocation 3339 this.getGeographicalLocation().add(castToString(value)); // StringType 3340 return value; 3341 case 391529091: // developmentStage 3342 this.developmentStage = castToCodeableConcept(value); // CodeableConcept 3343 return value; 3344 case 1472689306: // fractionDescription 3345 this.getFractionDescription().add((SubstanceSourceMaterialFractionDescriptionComponent) value); // SubstanceSourceMaterialFractionDescriptionComponent 3346 return value; 3347 case 1316389074: // organism 3348 this.organism = (SubstanceSourceMaterialOrganismComponent) value; // SubstanceSourceMaterialOrganismComponent 3349 return value; 3350 case -1803623927: // partDescription 3351 this.getPartDescription().add((SubstanceSourceMaterialPartDescriptionComponent) value); // SubstanceSourceMaterialPartDescriptionComponent 3352 return value; 3353 default: 3354 return super.setProperty(hash, name, value); 3355 } 3356 3357 } 3358 3359 @Override 3360 public Base setProperty(String name, Base value) throws FHIRException { 3361 if (name.equals("sourceMaterialClass")) { 3362 this.sourceMaterialClass = castToCodeableConcept(value); // CodeableConcept 3363 } else if (name.equals("sourceMaterialType")) { 3364 this.sourceMaterialType = castToCodeableConcept(value); // CodeableConcept 3365 } else if (name.equals("sourceMaterialState")) { 3366 this.sourceMaterialState = castToCodeableConcept(value); // CodeableConcept 3367 } else if (name.equals("organismId")) { 3368 this.organismId = castToIdentifier(value); // Identifier 3369 } else if (name.equals("organismName")) { 3370 this.organismName = castToString(value); // StringType 3371 } else if (name.equals("parentSubstanceId")) { 3372 this.getParentSubstanceId().add(castToIdentifier(value)); 3373 } else if (name.equals("parentSubstanceName")) { 3374 this.getParentSubstanceName().add(castToString(value)); 3375 } else if (name.equals("countryOfOrigin")) { 3376 this.getCountryOfOrigin().add(castToCodeableConcept(value)); 3377 } else if (name.equals("geographicalLocation")) { 3378 this.getGeographicalLocation().add(castToString(value)); 3379 } else if (name.equals("developmentStage")) { 3380 this.developmentStage = castToCodeableConcept(value); // CodeableConcept 3381 } else if (name.equals("fractionDescription")) { 3382 this.getFractionDescription().add((SubstanceSourceMaterialFractionDescriptionComponent) value); 3383 } else if (name.equals("organism")) { 3384 this.organism = (SubstanceSourceMaterialOrganismComponent) value; // SubstanceSourceMaterialOrganismComponent 3385 } else if (name.equals("partDescription")) { 3386 this.getPartDescription().add((SubstanceSourceMaterialPartDescriptionComponent) value); 3387 } else 3388 return super.setProperty(name, value); 3389 return value; 3390 } 3391 3392 @Override 3393 public void removeChild(String name, Base value) throws FHIRException { 3394 if (name.equals("sourceMaterialClass")) { 3395 this.sourceMaterialClass = null; 3396 } else if (name.equals("sourceMaterialType")) { 3397 this.sourceMaterialType = null; 3398 } else if (name.equals("sourceMaterialState")) { 3399 this.sourceMaterialState = null; 3400 } else if (name.equals("organismId")) { 3401 this.organismId = null; 3402 } else if (name.equals("organismName")) { 3403 this.organismName = null; 3404 } else if (name.equals("parentSubstanceId")) { 3405 this.getParentSubstanceId().remove(castToIdentifier(value)); 3406 } else if (name.equals("parentSubstanceName")) { 3407 this.getParentSubstanceName().remove(castToString(value)); 3408 } else if (name.equals("countryOfOrigin")) { 3409 this.getCountryOfOrigin().remove(castToCodeableConcept(value)); 3410 } else if (name.equals("geographicalLocation")) { 3411 this.getGeographicalLocation().remove(castToString(value)); 3412 } else if (name.equals("developmentStage")) { 3413 this.developmentStage = null; 3414 } else if (name.equals("fractionDescription")) { 3415 this.getFractionDescription().remove((SubstanceSourceMaterialFractionDescriptionComponent) value); 3416 } else if (name.equals("organism")) { 3417 this.organism = (SubstanceSourceMaterialOrganismComponent) value; // SubstanceSourceMaterialOrganismComponent 3418 } else if (name.equals("partDescription")) { 3419 this.getPartDescription().remove((SubstanceSourceMaterialPartDescriptionComponent) value); 3420 } else 3421 super.removeChild(name, value); 3422 3423 } 3424 3425 @Override 3426 public Base makeProperty(int hash, String name) throws FHIRException { 3427 switch (hash) { 3428 case -1253081034: 3429 return getSourceMaterialClass(); 3430 case 1622665404: 3431 return getSourceMaterialType(); 3432 case -1238066353: 3433 return getSourceMaterialState(); 3434 case -1965449843: 3435 return getOrganismId(); 3436 case 988460669: 3437 return getOrganismNameElement(); 3438 case -675437663: 3439 return addParentSubstanceId(); 3440 case -555382895: 3441 return addParentSubstanceNameElement(); 3442 case 57176467: 3443 return addCountryOfOrigin(); 3444 case -1988836681: 3445 return addGeographicalLocationElement(); 3446 case 391529091: 3447 return getDevelopmentStage(); 3448 case 1472689306: 3449 return addFractionDescription(); 3450 case 1316389074: 3451 return getOrganism(); 3452 case -1803623927: 3453 return addPartDescription(); 3454 default: 3455 return super.makeProperty(hash, name); 3456 } 3457 3458 } 3459 3460 @Override 3461 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3462 switch (hash) { 3463 case -1253081034: 3464 /* sourceMaterialClass */ return new String[] { "CodeableConcept" }; 3465 case 1622665404: 3466 /* sourceMaterialType */ return new String[] { "CodeableConcept" }; 3467 case -1238066353: 3468 /* sourceMaterialState */ return new String[] { "CodeableConcept" }; 3469 case -1965449843: 3470 /* organismId */ return new String[] { "Identifier" }; 3471 case 988460669: 3472 /* organismName */ return new String[] { "string" }; 3473 case -675437663: 3474 /* parentSubstanceId */ return new String[] { "Identifier" }; 3475 case -555382895: 3476 /* parentSubstanceName */ return new String[] { "string" }; 3477 case 57176467: 3478 /* countryOfOrigin */ return new String[] { "CodeableConcept" }; 3479 case -1988836681: 3480 /* geographicalLocation */ return new String[] { "string" }; 3481 case 391529091: 3482 /* developmentStage */ return new String[] { "CodeableConcept" }; 3483 case 1472689306: 3484 /* fractionDescription */ return new String[] {}; 3485 case 1316389074: 3486 /* organism */ return new String[] {}; 3487 case -1803623927: 3488 /* partDescription */ return new String[] {}; 3489 default: 3490 return super.getTypesForProperty(hash, name); 3491 } 3492 3493 } 3494 3495 @Override 3496 public Base addChild(String name) throws FHIRException { 3497 if (name.equals("sourceMaterialClass")) { 3498 this.sourceMaterialClass = new CodeableConcept(); 3499 return this.sourceMaterialClass; 3500 } else if (name.equals("sourceMaterialType")) { 3501 this.sourceMaterialType = new CodeableConcept(); 3502 return this.sourceMaterialType; 3503 } else if (name.equals("sourceMaterialState")) { 3504 this.sourceMaterialState = new CodeableConcept(); 3505 return this.sourceMaterialState; 3506 } else if (name.equals("organismId")) { 3507 this.organismId = new Identifier(); 3508 return this.organismId; 3509 } else if (name.equals("organismName")) { 3510 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.organismName"); 3511 } else if (name.equals("parentSubstanceId")) { 3512 return addParentSubstanceId(); 3513 } else if (name.equals("parentSubstanceName")) { 3514 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.parentSubstanceName"); 3515 } else if (name.equals("countryOfOrigin")) { 3516 return addCountryOfOrigin(); 3517 } else if (name.equals("geographicalLocation")) { 3518 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSourceMaterial.geographicalLocation"); 3519 } else if (name.equals("developmentStage")) { 3520 this.developmentStage = new CodeableConcept(); 3521 return this.developmentStage; 3522 } else if (name.equals("fractionDescription")) { 3523 return addFractionDescription(); 3524 } else if (name.equals("organism")) { 3525 this.organism = new SubstanceSourceMaterialOrganismComponent(); 3526 return this.organism; 3527 } else if (name.equals("partDescription")) { 3528 return addPartDescription(); 3529 } else 3530 return super.addChild(name); 3531 } 3532 3533 public String fhirType() { 3534 return "SubstanceSourceMaterial"; 3535 3536 } 3537 3538 public SubstanceSourceMaterial copy() { 3539 SubstanceSourceMaterial dst = new SubstanceSourceMaterial(); 3540 copyValues(dst); 3541 return dst; 3542 } 3543 3544 public void copyValues(SubstanceSourceMaterial dst) { 3545 super.copyValues(dst); 3546 dst.sourceMaterialClass = sourceMaterialClass == null ? null : sourceMaterialClass.copy(); 3547 dst.sourceMaterialType = sourceMaterialType == null ? null : sourceMaterialType.copy(); 3548 dst.sourceMaterialState = sourceMaterialState == null ? null : sourceMaterialState.copy(); 3549 dst.organismId = organismId == null ? null : organismId.copy(); 3550 dst.organismName = organismName == null ? null : organismName.copy(); 3551 if (parentSubstanceId != null) { 3552 dst.parentSubstanceId = new ArrayList<Identifier>(); 3553 for (Identifier i : parentSubstanceId) 3554 dst.parentSubstanceId.add(i.copy()); 3555 } 3556 ; 3557 if (parentSubstanceName != null) { 3558 dst.parentSubstanceName = new ArrayList<StringType>(); 3559 for (StringType i : parentSubstanceName) 3560 dst.parentSubstanceName.add(i.copy()); 3561 } 3562 ; 3563 if (countryOfOrigin != null) { 3564 dst.countryOfOrigin = new ArrayList<CodeableConcept>(); 3565 for (CodeableConcept i : countryOfOrigin) 3566 dst.countryOfOrigin.add(i.copy()); 3567 } 3568 ; 3569 if (geographicalLocation != null) { 3570 dst.geographicalLocation = new ArrayList<StringType>(); 3571 for (StringType i : geographicalLocation) 3572 dst.geographicalLocation.add(i.copy()); 3573 } 3574 ; 3575 dst.developmentStage = developmentStage == null ? null : developmentStage.copy(); 3576 if (fractionDescription != null) { 3577 dst.fractionDescription = new ArrayList<SubstanceSourceMaterialFractionDescriptionComponent>(); 3578 for (SubstanceSourceMaterialFractionDescriptionComponent i : fractionDescription) 3579 dst.fractionDescription.add(i.copy()); 3580 } 3581 ; 3582 dst.organism = organism == null ? null : organism.copy(); 3583 if (partDescription != null) { 3584 dst.partDescription = new ArrayList<SubstanceSourceMaterialPartDescriptionComponent>(); 3585 for (SubstanceSourceMaterialPartDescriptionComponent i : partDescription) 3586 dst.partDescription.add(i.copy()); 3587 } 3588 ; 3589 } 3590 3591 protected SubstanceSourceMaterial typedCopy() { 3592 return copy(); 3593 } 3594 3595 @Override 3596 public boolean equalsDeep(Base other_) { 3597 if (!super.equalsDeep(other_)) 3598 return false; 3599 if (!(other_ instanceof SubstanceSourceMaterial)) 3600 return false; 3601 SubstanceSourceMaterial o = (SubstanceSourceMaterial) other_; 3602 return compareDeep(sourceMaterialClass, o.sourceMaterialClass, true) 3603 && compareDeep(sourceMaterialType, o.sourceMaterialType, true) 3604 && compareDeep(sourceMaterialState, o.sourceMaterialState, true) && compareDeep(organismId, o.organismId, true) 3605 && compareDeep(organismName, o.organismName, true) && compareDeep(parentSubstanceId, o.parentSubstanceId, true) 3606 && compareDeep(parentSubstanceName, o.parentSubstanceName, true) 3607 && compareDeep(countryOfOrigin, o.countryOfOrigin, true) 3608 && compareDeep(geographicalLocation, o.geographicalLocation, true) 3609 && compareDeep(developmentStage, o.developmentStage, true) 3610 && compareDeep(fractionDescription, o.fractionDescription, true) && compareDeep(organism, o.organism, true) 3611 && compareDeep(partDescription, o.partDescription, true); 3612 } 3613 3614 @Override 3615 public boolean equalsShallow(Base other_) { 3616 if (!super.equalsShallow(other_)) 3617 return false; 3618 if (!(other_ instanceof SubstanceSourceMaterial)) 3619 return false; 3620 SubstanceSourceMaterial o = (SubstanceSourceMaterial) other_; 3621 return compareValues(organismName, o.organismName, true) 3622 && compareValues(parentSubstanceName, o.parentSubstanceName, true) 3623 && compareValues(geographicalLocation, o.geographicalLocation, true); 3624 } 3625 3626 public boolean isEmpty() { 3627 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sourceMaterialClass, sourceMaterialType, 3628 sourceMaterialState, organismId, organismName, parentSubstanceId, parentSubstanceName, countryOfOrigin, 3629 geographicalLocation, developmentStage, fractionDescription, organism, partDescription); 3630 } 3631 3632 @Override 3633 public ResourceType getResourceType() { 3634 return ResourceType.SubstanceSourceMaterial; 3635 } 3636 3637}