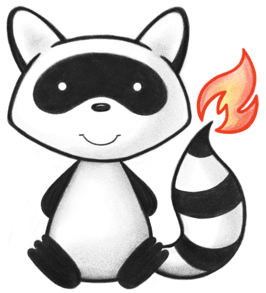
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * The detailed description of a substance, typically at a level beyond what is 049 * used for prescribing. 050 */ 051@ResourceDef(name = "SubstanceSpecification", profile = "http://hl7.org/fhir/StructureDefinition/SubstanceSpecification") 052public class SubstanceSpecification extends DomainResource { 053 054 @Block() 055 public static class SubstanceSpecificationMoietyComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * Role that the moiety is playing. 058 */ 059 @Child(name = "role", type = { 060 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "Role that the moiety is playing", formalDefinition = "Role that the moiety is playing.") 062 protected CodeableConcept role; 063 064 /** 065 * Identifier by which this moiety substance is known. 066 */ 067 @Child(name = "identifier", type = { 068 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 069 @Description(shortDefinition = "Identifier by which this moiety substance is known", formalDefinition = "Identifier by which this moiety substance is known.") 070 protected Identifier identifier; 071 072 /** 073 * Textual name for this moiety substance. 074 */ 075 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 076 @Description(shortDefinition = "Textual name for this moiety substance", formalDefinition = "Textual name for this moiety substance.") 077 protected StringType name; 078 079 /** 080 * Stereochemistry type. 081 */ 082 @Child(name = "stereochemistry", type = { 083 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 084 @Description(shortDefinition = "Stereochemistry type", formalDefinition = "Stereochemistry type.") 085 protected CodeableConcept stereochemistry; 086 087 /** 088 * Optical activity type. 089 */ 090 @Child(name = "opticalActivity", type = { 091 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 092 @Description(shortDefinition = "Optical activity type", formalDefinition = "Optical activity type.") 093 protected CodeableConcept opticalActivity; 094 095 /** 096 * Molecular formula. 097 */ 098 @Child(name = "molecularFormula", type = { 099 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 100 @Description(shortDefinition = "Molecular formula", formalDefinition = "Molecular formula.") 101 protected StringType molecularFormula; 102 103 /** 104 * Quantitative value for this moiety. 105 */ 106 @Child(name = "amount", type = { Quantity.class, 107 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 108 @Description(shortDefinition = "Quantitative value for this moiety", formalDefinition = "Quantitative value for this moiety.") 109 protected Type amount; 110 111 private static final long serialVersionUID = -505630417L; 112 113 /** 114 * Constructor 115 */ 116 public SubstanceSpecificationMoietyComponent() { 117 super(); 118 } 119 120 /** 121 * @return {@link #role} (Role that the moiety is playing.) 122 */ 123 public CodeableConcept getRole() { 124 if (this.role == null) 125 if (Configuration.errorOnAutoCreate()) 126 throw new Error("Attempt to auto-create SubstanceSpecificationMoietyComponent.role"); 127 else if (Configuration.doAutoCreate()) 128 this.role = new CodeableConcept(); // cc 129 return this.role; 130 } 131 132 public boolean hasRole() { 133 return this.role != null && !this.role.isEmpty(); 134 } 135 136 /** 137 * @param value {@link #role} (Role that the moiety is playing.) 138 */ 139 public SubstanceSpecificationMoietyComponent setRole(CodeableConcept value) { 140 this.role = value; 141 return this; 142 } 143 144 /** 145 * @return {@link #identifier} (Identifier by which this moiety substance is 146 * known.) 147 */ 148 public Identifier getIdentifier() { 149 if (this.identifier == null) 150 if (Configuration.errorOnAutoCreate()) 151 throw new Error("Attempt to auto-create SubstanceSpecificationMoietyComponent.identifier"); 152 else if (Configuration.doAutoCreate()) 153 this.identifier = new Identifier(); // cc 154 return this.identifier; 155 } 156 157 public boolean hasIdentifier() { 158 return this.identifier != null && !this.identifier.isEmpty(); 159 } 160 161 /** 162 * @param value {@link #identifier} (Identifier by which this moiety substance 163 * is known.) 164 */ 165 public SubstanceSpecificationMoietyComponent setIdentifier(Identifier value) { 166 this.identifier = value; 167 return this; 168 } 169 170 /** 171 * @return {@link #name} (Textual name for this moiety substance.). This is the 172 * underlying object with id, value and extensions. The accessor 173 * "getName" gives direct access to the value 174 */ 175 public StringType getNameElement() { 176 if (this.name == null) 177 if (Configuration.errorOnAutoCreate()) 178 throw new Error("Attempt to auto-create SubstanceSpecificationMoietyComponent.name"); 179 else if (Configuration.doAutoCreate()) 180 this.name = new StringType(); // bb 181 return this.name; 182 } 183 184 public boolean hasNameElement() { 185 return this.name != null && !this.name.isEmpty(); 186 } 187 188 public boolean hasName() { 189 return this.name != null && !this.name.isEmpty(); 190 } 191 192 /** 193 * @param value {@link #name} (Textual name for this moiety substance.). This is 194 * the underlying object with id, value and extensions. The 195 * accessor "getName" gives direct access to the value 196 */ 197 public SubstanceSpecificationMoietyComponent setNameElement(StringType value) { 198 this.name = value; 199 return this; 200 } 201 202 /** 203 * @return Textual name for this moiety substance. 204 */ 205 public String getName() { 206 return this.name == null ? null : this.name.getValue(); 207 } 208 209 /** 210 * @param value Textual name for this moiety substance. 211 */ 212 public SubstanceSpecificationMoietyComponent setName(String value) { 213 if (Utilities.noString(value)) 214 this.name = null; 215 else { 216 if (this.name == null) 217 this.name = new StringType(); 218 this.name.setValue(value); 219 } 220 return this; 221 } 222 223 /** 224 * @return {@link #stereochemistry} (Stereochemistry type.) 225 */ 226 public CodeableConcept getStereochemistry() { 227 if (this.stereochemistry == null) 228 if (Configuration.errorOnAutoCreate()) 229 throw new Error("Attempt to auto-create SubstanceSpecificationMoietyComponent.stereochemistry"); 230 else if (Configuration.doAutoCreate()) 231 this.stereochemistry = new CodeableConcept(); // cc 232 return this.stereochemistry; 233 } 234 235 public boolean hasStereochemistry() { 236 return this.stereochemistry != null && !this.stereochemistry.isEmpty(); 237 } 238 239 /** 240 * @param value {@link #stereochemistry} (Stereochemistry type.) 241 */ 242 public SubstanceSpecificationMoietyComponent setStereochemistry(CodeableConcept value) { 243 this.stereochemistry = value; 244 return this; 245 } 246 247 /** 248 * @return {@link #opticalActivity} (Optical activity type.) 249 */ 250 public CodeableConcept getOpticalActivity() { 251 if (this.opticalActivity == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create SubstanceSpecificationMoietyComponent.opticalActivity"); 254 else if (Configuration.doAutoCreate()) 255 this.opticalActivity = new CodeableConcept(); // cc 256 return this.opticalActivity; 257 } 258 259 public boolean hasOpticalActivity() { 260 return this.opticalActivity != null && !this.opticalActivity.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #opticalActivity} (Optical activity type.) 265 */ 266 public SubstanceSpecificationMoietyComponent setOpticalActivity(CodeableConcept value) { 267 this.opticalActivity = value; 268 return this; 269 } 270 271 /** 272 * @return {@link #molecularFormula} (Molecular formula.). This is the 273 * underlying object with id, value and extensions. The accessor 274 * "getMolecularFormula" gives direct access to the value 275 */ 276 public StringType getMolecularFormulaElement() { 277 if (this.molecularFormula == null) 278 if (Configuration.errorOnAutoCreate()) 279 throw new Error("Attempt to auto-create SubstanceSpecificationMoietyComponent.molecularFormula"); 280 else if (Configuration.doAutoCreate()) 281 this.molecularFormula = new StringType(); // bb 282 return this.molecularFormula; 283 } 284 285 public boolean hasMolecularFormulaElement() { 286 return this.molecularFormula != null && !this.molecularFormula.isEmpty(); 287 } 288 289 public boolean hasMolecularFormula() { 290 return this.molecularFormula != null && !this.molecularFormula.isEmpty(); 291 } 292 293 /** 294 * @param value {@link #molecularFormula} (Molecular formula.). This is the 295 * underlying object with id, value and extensions. The accessor 296 * "getMolecularFormula" gives direct access to the value 297 */ 298 public SubstanceSpecificationMoietyComponent setMolecularFormulaElement(StringType value) { 299 this.molecularFormula = value; 300 return this; 301 } 302 303 /** 304 * @return Molecular formula. 305 */ 306 public String getMolecularFormula() { 307 return this.molecularFormula == null ? null : this.molecularFormula.getValue(); 308 } 309 310 /** 311 * @param value Molecular formula. 312 */ 313 public SubstanceSpecificationMoietyComponent setMolecularFormula(String value) { 314 if (Utilities.noString(value)) 315 this.molecularFormula = null; 316 else { 317 if (this.molecularFormula == null) 318 this.molecularFormula = new StringType(); 319 this.molecularFormula.setValue(value); 320 } 321 return this; 322 } 323 324 /** 325 * @return {@link #amount} (Quantitative value for this moiety.) 326 */ 327 public Type getAmount() { 328 return this.amount; 329 } 330 331 /** 332 * @return {@link #amount} (Quantitative value for this moiety.) 333 */ 334 public Quantity getAmountQuantity() throws FHIRException { 335 if (this.amount == null) 336 this.amount = new Quantity(); 337 if (!(this.amount instanceof Quantity)) 338 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.amount.getClass().getName() 339 + " was encountered"); 340 return (Quantity) this.amount; 341 } 342 343 public boolean hasAmountQuantity() { 344 return this != null && this.amount instanceof Quantity; 345 } 346 347 /** 348 * @return {@link #amount} (Quantitative value for this moiety.) 349 */ 350 public StringType getAmountStringType() throws FHIRException { 351 if (this.amount == null) 352 this.amount = new StringType(); 353 if (!(this.amount instanceof StringType)) 354 throw new FHIRException("Type mismatch: the type StringType was expected, but " 355 + this.amount.getClass().getName() + " was encountered"); 356 return (StringType) this.amount; 357 } 358 359 public boolean hasAmountStringType() { 360 return this != null && this.amount instanceof StringType; 361 } 362 363 public boolean hasAmount() { 364 return this.amount != null && !this.amount.isEmpty(); 365 } 366 367 /** 368 * @param value {@link #amount} (Quantitative value for this moiety.) 369 */ 370 public SubstanceSpecificationMoietyComponent setAmount(Type value) { 371 if (value != null && !(value instanceof Quantity || value instanceof StringType)) 372 throw new Error("Not the right type for SubstanceSpecification.moiety.amount[x]: " + value.fhirType()); 373 this.amount = value; 374 return this; 375 } 376 377 protected void listChildren(List<Property> children) { 378 super.listChildren(children); 379 children.add(new Property("role", "CodeableConcept", "Role that the moiety is playing.", 0, 1, role)); 380 children.add(new Property("identifier", "Identifier", "Identifier by which this moiety substance is known.", 0, 1, 381 identifier)); 382 children.add(new Property("name", "string", "Textual name for this moiety substance.", 0, 1, name)); 383 children.add(new Property("stereochemistry", "CodeableConcept", "Stereochemistry type.", 0, 1, stereochemistry)); 384 children.add(new Property("opticalActivity", "CodeableConcept", "Optical activity type.", 0, 1, opticalActivity)); 385 children.add(new Property("molecularFormula", "string", "Molecular formula.", 0, 1, molecularFormula)); 386 children.add(new Property("amount[x]", "Quantity|string", "Quantitative value for this moiety.", 0, 1, amount)); 387 } 388 389 @Override 390 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 391 switch (_hash) { 392 case 3506294: 393 /* role */ return new Property("role", "CodeableConcept", "Role that the moiety is playing.", 0, 1, role); 394 case -1618432855: 395 /* identifier */ return new Property("identifier", "Identifier", 396 "Identifier by which this moiety substance is known.", 0, 1, identifier); 397 case 3373707: 398 /* name */ return new Property("name", "string", "Textual name for this moiety substance.", 0, 1, name); 399 case 263475116: 400 /* stereochemistry */ return new Property("stereochemistry", "CodeableConcept", "Stereochemistry type.", 0, 1, 401 stereochemistry); 402 case 1420900135: 403 /* opticalActivity */ return new Property("opticalActivity", "CodeableConcept", "Optical activity type.", 0, 1, 404 opticalActivity); 405 case 616660246: 406 /* molecularFormula */ return new Property("molecularFormula", "string", "Molecular formula.", 0, 1, 407 molecularFormula); 408 case 646780200: 409 /* amount[x] */ return new Property("amount[x]", "Quantity|string", "Quantitative value for this moiety.", 0, 1, 410 amount); 411 case -1413853096: 412 /* amount */ return new Property("amount[x]", "Quantity|string", "Quantitative value for this moiety.", 0, 1, 413 amount); 414 case 1664303363: 415 /* amountQuantity */ return new Property("amount[x]", "Quantity|string", "Quantitative value for this moiety.", 416 0, 1, amount); 417 case 773651081: 418 /* amountString */ return new Property("amount[x]", "Quantity|string", "Quantitative value for this moiety.", 0, 419 1, amount); 420 default: 421 return super.getNamedProperty(_hash, _name, _checkValid); 422 } 423 424 } 425 426 @Override 427 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 428 switch (hash) { 429 case 3506294: 430 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 431 case -1618432855: 432 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 433 case 3373707: 434 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 435 case 263475116: 436 /* stereochemistry */ return this.stereochemistry == null ? new Base[0] : new Base[] { this.stereochemistry }; // CodeableConcept 437 case 1420900135: 438 /* opticalActivity */ return this.opticalActivity == null ? new Base[0] : new Base[] { this.opticalActivity }; // CodeableConcept 439 case 616660246: 440 /* molecularFormula */ return this.molecularFormula == null ? new Base[0] 441 : new Base[] { this.molecularFormula }; // StringType 442 case -1413853096: 443 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Type 444 default: 445 return super.getProperty(hash, name, checkValid); 446 } 447 448 } 449 450 @Override 451 public Base setProperty(int hash, String name, Base value) throws FHIRException { 452 switch (hash) { 453 case 3506294: // role 454 this.role = castToCodeableConcept(value); // CodeableConcept 455 return value; 456 case -1618432855: // identifier 457 this.identifier = castToIdentifier(value); // Identifier 458 return value; 459 case 3373707: // name 460 this.name = castToString(value); // StringType 461 return value; 462 case 263475116: // stereochemistry 463 this.stereochemistry = castToCodeableConcept(value); // CodeableConcept 464 return value; 465 case 1420900135: // opticalActivity 466 this.opticalActivity = castToCodeableConcept(value); // CodeableConcept 467 return value; 468 case 616660246: // molecularFormula 469 this.molecularFormula = castToString(value); // StringType 470 return value; 471 case -1413853096: // amount 472 this.amount = castToType(value); // Type 473 return value; 474 default: 475 return super.setProperty(hash, name, value); 476 } 477 478 } 479 480 @Override 481 public Base setProperty(String name, Base value) throws FHIRException { 482 if (name.equals("role")) { 483 this.role = castToCodeableConcept(value); // CodeableConcept 484 } else if (name.equals("identifier")) { 485 this.identifier = castToIdentifier(value); // Identifier 486 } else if (name.equals("name")) { 487 this.name = castToString(value); // StringType 488 } else if (name.equals("stereochemistry")) { 489 this.stereochemistry = castToCodeableConcept(value); // CodeableConcept 490 } else if (name.equals("opticalActivity")) { 491 this.opticalActivity = castToCodeableConcept(value); // CodeableConcept 492 } else if (name.equals("molecularFormula")) { 493 this.molecularFormula = castToString(value); // StringType 494 } else if (name.equals("amount[x]")) { 495 this.amount = castToType(value); // Type 496 } else 497 return super.setProperty(name, value); 498 return value; 499 } 500 501 @Override 502 public Base makeProperty(int hash, String name) throws FHIRException { 503 switch (hash) { 504 case 3506294: 505 return getRole(); 506 case -1618432855: 507 return getIdentifier(); 508 case 3373707: 509 return getNameElement(); 510 case 263475116: 511 return getStereochemistry(); 512 case 1420900135: 513 return getOpticalActivity(); 514 case 616660246: 515 return getMolecularFormulaElement(); 516 case 646780200: 517 return getAmount(); 518 case -1413853096: 519 return getAmount(); 520 default: 521 return super.makeProperty(hash, name); 522 } 523 524 } 525 526 @Override 527 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 528 switch (hash) { 529 case 3506294: 530 /* role */ return new String[] { "CodeableConcept" }; 531 case -1618432855: 532 /* identifier */ return new String[] { "Identifier" }; 533 case 3373707: 534 /* name */ return new String[] { "string" }; 535 case 263475116: 536 /* stereochemistry */ return new String[] { "CodeableConcept" }; 537 case 1420900135: 538 /* opticalActivity */ return new String[] { "CodeableConcept" }; 539 case 616660246: 540 /* molecularFormula */ return new String[] { "string" }; 541 case -1413853096: 542 /* amount */ return new String[] { "Quantity", "string" }; 543 default: 544 return super.getTypesForProperty(hash, name); 545 } 546 547 } 548 549 @Override 550 public Base addChild(String name) throws FHIRException { 551 if (name.equals("role")) { 552 this.role = new CodeableConcept(); 553 return this.role; 554 } else if (name.equals("identifier")) { 555 this.identifier = new Identifier(); 556 return this.identifier; 557 } else if (name.equals("name")) { 558 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.name"); 559 } else if (name.equals("stereochemistry")) { 560 this.stereochemistry = new CodeableConcept(); 561 return this.stereochemistry; 562 } else if (name.equals("opticalActivity")) { 563 this.opticalActivity = new CodeableConcept(); 564 return this.opticalActivity; 565 } else if (name.equals("molecularFormula")) { 566 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.molecularFormula"); 567 } else if (name.equals("amountQuantity")) { 568 this.amount = new Quantity(); 569 return this.amount; 570 } else if (name.equals("amountString")) { 571 this.amount = new StringType(); 572 return this.amount; 573 } else 574 return super.addChild(name); 575 } 576 577 public SubstanceSpecificationMoietyComponent copy() { 578 SubstanceSpecificationMoietyComponent dst = new SubstanceSpecificationMoietyComponent(); 579 copyValues(dst); 580 return dst; 581 } 582 583 public void copyValues(SubstanceSpecificationMoietyComponent dst) { 584 super.copyValues(dst); 585 dst.role = role == null ? null : role.copy(); 586 dst.identifier = identifier == null ? null : identifier.copy(); 587 dst.name = name == null ? null : name.copy(); 588 dst.stereochemistry = stereochemistry == null ? null : stereochemistry.copy(); 589 dst.opticalActivity = opticalActivity == null ? null : opticalActivity.copy(); 590 dst.molecularFormula = molecularFormula == null ? null : molecularFormula.copy(); 591 dst.amount = amount == null ? null : amount.copy(); 592 } 593 594 @Override 595 public boolean equalsDeep(Base other_) { 596 if (!super.equalsDeep(other_)) 597 return false; 598 if (!(other_ instanceof SubstanceSpecificationMoietyComponent)) 599 return false; 600 SubstanceSpecificationMoietyComponent o = (SubstanceSpecificationMoietyComponent) other_; 601 return compareDeep(role, o.role, true) && compareDeep(identifier, o.identifier, true) 602 && compareDeep(name, o.name, true) && compareDeep(stereochemistry, o.stereochemistry, true) 603 && compareDeep(opticalActivity, o.opticalActivity, true) 604 && compareDeep(molecularFormula, o.molecularFormula, true) && compareDeep(amount, o.amount, true); 605 } 606 607 @Override 608 public boolean equalsShallow(Base other_) { 609 if (!super.equalsShallow(other_)) 610 return false; 611 if (!(other_ instanceof SubstanceSpecificationMoietyComponent)) 612 return false; 613 SubstanceSpecificationMoietyComponent o = (SubstanceSpecificationMoietyComponent) other_; 614 return compareValues(name, o.name, true) && compareValues(molecularFormula, o.molecularFormula, true); 615 } 616 617 public boolean isEmpty() { 618 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, identifier, name, stereochemistry, 619 opticalActivity, molecularFormula, amount); 620 } 621 622 public String fhirType() { 623 return "SubstanceSpecification.moiety"; 624 625 } 626 627 } 628 629 @Block() 630 public static class SubstanceSpecificationPropertyComponent extends BackboneElement implements IBaseBackboneElement { 631 /** 632 * A category for this property, e.g. Physical, Chemical, Enzymatic. 633 */ 634 @Child(name = "category", type = { 635 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 636 @Description(shortDefinition = "A category for this property, e.g. Physical, Chemical, Enzymatic", formalDefinition = "A category for this property, e.g. Physical, Chemical, Enzymatic.") 637 protected CodeableConcept category; 638 639 /** 640 * Property type e.g. viscosity, pH, isoelectric point. 641 */ 642 @Child(name = "code", type = { 643 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 644 @Description(shortDefinition = "Property type e.g. viscosity, pH, isoelectric point", formalDefinition = "Property type e.g. viscosity, pH, isoelectric point.") 645 protected CodeableConcept code; 646 647 /** 648 * Parameters that were used in the measurement of a property (e.g. for 649 * viscosity: measured at 20C with a pH of 7.1). 650 */ 651 @Child(name = "parameters", type = { 652 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 653 @Description(shortDefinition = "Parameters that were used in the measurement of a property (e.g. for viscosity: measured at 20C with a pH of 7.1)", formalDefinition = "Parameters that were used in the measurement of a property (e.g. for viscosity: measured at 20C with a pH of 7.1).") 654 protected StringType parameters; 655 656 /** 657 * A substance upon which a defining property depends (e.g. for solubility: in 658 * water, in alcohol). 659 */ 660 @Child(name = "definingSubstance", type = { SubstanceSpecification.class, Substance.class, 661 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 662 @Description(shortDefinition = "A substance upon which a defining property depends (e.g. for solubility: in water, in alcohol)", formalDefinition = "A substance upon which a defining property depends (e.g. for solubility: in water, in alcohol).") 663 protected Type definingSubstance; 664 665 /** 666 * Quantitative value for this property. 667 */ 668 @Child(name = "amount", type = { Quantity.class, 669 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 670 @Description(shortDefinition = "Quantitative value for this property", formalDefinition = "Quantitative value for this property.") 671 protected Type amount; 672 673 private static final long serialVersionUID = 556834916L; 674 675 /** 676 * Constructor 677 */ 678 public SubstanceSpecificationPropertyComponent() { 679 super(); 680 } 681 682 /** 683 * @return {@link #category} (A category for this property, e.g. Physical, 684 * Chemical, Enzymatic.) 685 */ 686 public CodeableConcept getCategory() { 687 if (this.category == null) 688 if (Configuration.errorOnAutoCreate()) 689 throw new Error("Attempt to auto-create SubstanceSpecificationPropertyComponent.category"); 690 else if (Configuration.doAutoCreate()) 691 this.category = new CodeableConcept(); // cc 692 return this.category; 693 } 694 695 public boolean hasCategory() { 696 return this.category != null && !this.category.isEmpty(); 697 } 698 699 /** 700 * @param value {@link #category} (A category for this property, e.g. Physical, 701 * Chemical, Enzymatic.) 702 */ 703 public SubstanceSpecificationPropertyComponent setCategory(CodeableConcept value) { 704 this.category = value; 705 return this; 706 } 707 708 /** 709 * @return {@link #code} (Property type e.g. viscosity, pH, isoelectric point.) 710 */ 711 public CodeableConcept getCode() { 712 if (this.code == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create SubstanceSpecificationPropertyComponent.code"); 715 else if (Configuration.doAutoCreate()) 716 this.code = new CodeableConcept(); // cc 717 return this.code; 718 } 719 720 public boolean hasCode() { 721 return this.code != null && !this.code.isEmpty(); 722 } 723 724 /** 725 * @param value {@link #code} (Property type e.g. viscosity, pH, isoelectric 726 * point.) 727 */ 728 public SubstanceSpecificationPropertyComponent setCode(CodeableConcept value) { 729 this.code = value; 730 return this; 731 } 732 733 /** 734 * @return {@link #parameters} (Parameters that were used in the measurement of 735 * a property (e.g. for viscosity: measured at 20C with a pH of 7.1).). 736 * This is the underlying object with id, value and extensions. The 737 * accessor "getParameters" gives direct access to the value 738 */ 739 public StringType getParametersElement() { 740 if (this.parameters == null) 741 if (Configuration.errorOnAutoCreate()) 742 throw new Error("Attempt to auto-create SubstanceSpecificationPropertyComponent.parameters"); 743 else if (Configuration.doAutoCreate()) 744 this.parameters = new StringType(); // bb 745 return this.parameters; 746 } 747 748 public boolean hasParametersElement() { 749 return this.parameters != null && !this.parameters.isEmpty(); 750 } 751 752 public boolean hasParameters() { 753 return this.parameters != null && !this.parameters.isEmpty(); 754 } 755 756 /** 757 * @param value {@link #parameters} (Parameters that were used in the 758 * measurement of a property (e.g. for viscosity: measured at 20C 759 * with a pH of 7.1).). This is the underlying object with id, 760 * value and extensions. The accessor "getParameters" gives direct 761 * access to the value 762 */ 763 public SubstanceSpecificationPropertyComponent setParametersElement(StringType value) { 764 this.parameters = value; 765 return this; 766 } 767 768 /** 769 * @return Parameters that were used in the measurement of a property (e.g. for 770 * viscosity: measured at 20C with a pH of 7.1). 771 */ 772 public String getParameters() { 773 return this.parameters == null ? null : this.parameters.getValue(); 774 } 775 776 /** 777 * @param value Parameters that were used in the measurement of a property (e.g. 778 * for viscosity: measured at 20C with a pH of 7.1). 779 */ 780 public SubstanceSpecificationPropertyComponent setParameters(String value) { 781 if (Utilities.noString(value)) 782 this.parameters = null; 783 else { 784 if (this.parameters == null) 785 this.parameters = new StringType(); 786 this.parameters.setValue(value); 787 } 788 return this; 789 } 790 791 /** 792 * @return {@link #definingSubstance} (A substance upon which a defining 793 * property depends (e.g. for solubility: in water, in alcohol).) 794 */ 795 public Type getDefiningSubstance() { 796 return this.definingSubstance; 797 } 798 799 /** 800 * @return {@link #definingSubstance} (A substance upon which a defining 801 * property depends (e.g. for solubility: in water, in alcohol).) 802 */ 803 public Reference getDefiningSubstanceReference() throws FHIRException { 804 if (this.definingSubstance == null) 805 this.definingSubstance = new Reference(); 806 if (!(this.definingSubstance instanceof Reference)) 807 throw new FHIRException("Type mismatch: the type Reference was expected, but " 808 + this.definingSubstance.getClass().getName() + " was encountered"); 809 return (Reference) this.definingSubstance; 810 } 811 812 public boolean hasDefiningSubstanceReference() { 813 return this != null && this.definingSubstance instanceof Reference; 814 } 815 816 /** 817 * @return {@link #definingSubstance} (A substance upon which a defining 818 * property depends (e.g. for solubility: in water, in alcohol).) 819 */ 820 public CodeableConcept getDefiningSubstanceCodeableConcept() throws FHIRException { 821 if (this.definingSubstance == null) 822 this.definingSubstance = new CodeableConcept(); 823 if (!(this.definingSubstance instanceof CodeableConcept)) 824 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 825 + this.definingSubstance.getClass().getName() + " was encountered"); 826 return (CodeableConcept) this.definingSubstance; 827 } 828 829 public boolean hasDefiningSubstanceCodeableConcept() { 830 return this != null && this.definingSubstance instanceof CodeableConcept; 831 } 832 833 public boolean hasDefiningSubstance() { 834 return this.definingSubstance != null && !this.definingSubstance.isEmpty(); 835 } 836 837 /** 838 * @param value {@link #definingSubstance} (A substance upon which a defining 839 * property depends (e.g. for solubility: in water, in alcohol).) 840 */ 841 public SubstanceSpecificationPropertyComponent setDefiningSubstance(Type value) { 842 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 843 throw new Error( 844 "Not the right type for SubstanceSpecification.property.definingSubstance[x]: " + value.fhirType()); 845 this.definingSubstance = value; 846 return this; 847 } 848 849 /** 850 * @return {@link #amount} (Quantitative value for this property.) 851 */ 852 public Type getAmount() { 853 return this.amount; 854 } 855 856 /** 857 * @return {@link #amount} (Quantitative value for this property.) 858 */ 859 public Quantity getAmountQuantity() throws FHIRException { 860 if (this.amount == null) 861 this.amount = new Quantity(); 862 if (!(this.amount instanceof Quantity)) 863 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.amount.getClass().getName() 864 + " was encountered"); 865 return (Quantity) this.amount; 866 } 867 868 public boolean hasAmountQuantity() { 869 return this != null && this.amount instanceof Quantity; 870 } 871 872 /** 873 * @return {@link #amount} (Quantitative value for this property.) 874 */ 875 public StringType getAmountStringType() throws FHIRException { 876 if (this.amount == null) 877 this.amount = new StringType(); 878 if (!(this.amount instanceof StringType)) 879 throw new FHIRException("Type mismatch: the type StringType was expected, but " 880 + this.amount.getClass().getName() + " was encountered"); 881 return (StringType) this.amount; 882 } 883 884 public boolean hasAmountStringType() { 885 return this != null && this.amount instanceof StringType; 886 } 887 888 public boolean hasAmount() { 889 return this.amount != null && !this.amount.isEmpty(); 890 } 891 892 /** 893 * @param value {@link #amount} (Quantitative value for this property.) 894 */ 895 public SubstanceSpecificationPropertyComponent setAmount(Type value) { 896 if (value != null && !(value instanceof Quantity || value instanceof StringType)) 897 throw new Error("Not the right type for SubstanceSpecification.property.amount[x]: " + value.fhirType()); 898 this.amount = value; 899 return this; 900 } 901 902 protected void listChildren(List<Property> children) { 903 super.listChildren(children); 904 children.add(new Property("category", "CodeableConcept", 905 "A category for this property, e.g. Physical, Chemical, Enzymatic.", 0, 1, category)); 906 children.add( 907 new Property("code", "CodeableConcept", "Property type e.g. viscosity, pH, isoelectric point.", 0, 1, code)); 908 children.add(new Property("parameters", "string", 909 "Parameters that were used in the measurement of a property (e.g. for viscosity: measured at 20C with a pH of 7.1).", 910 0, 1, parameters)); 911 children.add(new Property("definingSubstance[x]", "Reference(SubstanceSpecification|Substance)|CodeableConcept", 912 "A substance upon which a defining property depends (e.g. for solubility: in water, in alcohol).", 0, 1, 913 definingSubstance)); 914 children.add(new Property("amount[x]", "Quantity|string", "Quantitative value for this property.", 0, 1, amount)); 915 } 916 917 @Override 918 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 919 switch (_hash) { 920 case 50511102: 921 /* category */ return new Property("category", "CodeableConcept", 922 "A category for this property, e.g. Physical, Chemical, Enzymatic.", 0, 1, category); 923 case 3059181: 924 /* code */ return new Property("code", "CodeableConcept", 925 "Property type e.g. viscosity, pH, isoelectric point.", 0, 1, code); 926 case 458736106: 927 /* parameters */ return new Property("parameters", "string", 928 "Parameters that were used in the measurement of a property (e.g. for viscosity: measured at 20C with a pH of 7.1).", 929 0, 1, parameters); 930 case 1535270120: 931 /* definingSubstance[x] */ return new Property("definingSubstance[x]", 932 "Reference(SubstanceSpecification|Substance)|CodeableConcept", 933 "A substance upon which a defining property depends (e.g. for solubility: in water, in alcohol).", 0, 1, 934 definingSubstance); 935 case 1901076632: 936 /* definingSubstance */ return new Property("definingSubstance[x]", 937 "Reference(SubstanceSpecification|Substance)|CodeableConcept", 938 "A substance upon which a defining property depends (e.g. for solubility: in water, in alcohol).", 0, 1, 939 definingSubstance); 940 case -2101581421: 941 /* definingSubstanceReference */ return new Property("definingSubstance[x]", 942 "Reference(SubstanceSpecification|Substance)|CodeableConcept", 943 "A substance upon which a defining property depends (e.g. for solubility: in water, in alcohol).", 0, 1, 944 definingSubstance); 945 case -1438235671: 946 /* definingSubstanceCodeableConcept */ return new Property("definingSubstance[x]", 947 "Reference(SubstanceSpecification|Substance)|CodeableConcept", 948 "A substance upon which a defining property depends (e.g. for solubility: in water, in alcohol).", 0, 1, 949 definingSubstance); 950 case 646780200: 951 /* amount[x] */ return new Property("amount[x]", "Quantity|string", "Quantitative value for this property.", 0, 952 1, amount); 953 case -1413853096: 954 /* amount */ return new Property("amount[x]", "Quantity|string", "Quantitative value for this property.", 0, 1, 955 amount); 956 case 1664303363: 957 /* amountQuantity */ return new Property("amount[x]", "Quantity|string", 958 "Quantitative value for this property.", 0, 1, amount); 959 case 773651081: 960 /* amountString */ return new Property("amount[x]", "Quantity|string", "Quantitative value for this property.", 961 0, 1, amount); 962 default: 963 return super.getNamedProperty(_hash, _name, _checkValid); 964 } 965 966 } 967 968 @Override 969 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 970 switch (hash) { 971 case 50511102: 972 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 973 case 3059181: 974 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 975 case 458736106: 976 /* parameters */ return this.parameters == null ? new Base[0] : new Base[] { this.parameters }; // StringType 977 case 1901076632: 978 /* definingSubstance */ return this.definingSubstance == null ? new Base[0] 979 : new Base[] { this.definingSubstance }; // Type 980 case -1413853096: 981 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Type 982 default: 983 return super.getProperty(hash, name, checkValid); 984 } 985 986 } 987 988 @Override 989 public Base setProperty(int hash, String name, Base value) throws FHIRException { 990 switch (hash) { 991 case 50511102: // category 992 this.category = castToCodeableConcept(value); // CodeableConcept 993 return value; 994 case 3059181: // code 995 this.code = castToCodeableConcept(value); // CodeableConcept 996 return value; 997 case 458736106: // parameters 998 this.parameters = castToString(value); // StringType 999 return value; 1000 case 1901076632: // definingSubstance 1001 this.definingSubstance = castToType(value); // Type 1002 return value; 1003 case -1413853096: // amount 1004 this.amount = castToType(value); // Type 1005 return value; 1006 default: 1007 return super.setProperty(hash, name, value); 1008 } 1009 1010 } 1011 1012 @Override 1013 public Base setProperty(String name, Base value) throws FHIRException { 1014 if (name.equals("category")) { 1015 this.category = castToCodeableConcept(value); // CodeableConcept 1016 } else if (name.equals("code")) { 1017 this.code = castToCodeableConcept(value); // CodeableConcept 1018 } else if (name.equals("parameters")) { 1019 this.parameters = castToString(value); // StringType 1020 } else if (name.equals("definingSubstance[x]")) { 1021 this.definingSubstance = castToType(value); // Type 1022 } else if (name.equals("amount[x]")) { 1023 this.amount = castToType(value); // Type 1024 } else 1025 return super.setProperty(name, value); 1026 return value; 1027 } 1028 1029 @Override 1030 public Base makeProperty(int hash, String name) throws FHIRException { 1031 switch (hash) { 1032 case 50511102: 1033 return getCategory(); 1034 case 3059181: 1035 return getCode(); 1036 case 458736106: 1037 return getParametersElement(); 1038 case 1535270120: 1039 return getDefiningSubstance(); 1040 case 1901076632: 1041 return getDefiningSubstance(); 1042 case 646780200: 1043 return getAmount(); 1044 case -1413853096: 1045 return getAmount(); 1046 default: 1047 return super.makeProperty(hash, name); 1048 } 1049 1050 } 1051 1052 @Override 1053 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1054 switch (hash) { 1055 case 50511102: 1056 /* category */ return new String[] { "CodeableConcept" }; 1057 case 3059181: 1058 /* code */ return new String[] { "CodeableConcept" }; 1059 case 458736106: 1060 /* parameters */ return new String[] { "string" }; 1061 case 1901076632: 1062 /* definingSubstance */ return new String[] { "Reference", "CodeableConcept" }; 1063 case -1413853096: 1064 /* amount */ return new String[] { "Quantity", "string" }; 1065 default: 1066 return super.getTypesForProperty(hash, name); 1067 } 1068 1069 } 1070 1071 @Override 1072 public Base addChild(String name) throws FHIRException { 1073 if (name.equals("category")) { 1074 this.category = new CodeableConcept(); 1075 return this.category; 1076 } else if (name.equals("code")) { 1077 this.code = new CodeableConcept(); 1078 return this.code; 1079 } else if (name.equals("parameters")) { 1080 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.parameters"); 1081 } else if (name.equals("definingSubstanceReference")) { 1082 this.definingSubstance = new Reference(); 1083 return this.definingSubstance; 1084 } else if (name.equals("definingSubstanceCodeableConcept")) { 1085 this.definingSubstance = new CodeableConcept(); 1086 return this.definingSubstance; 1087 } else if (name.equals("amountQuantity")) { 1088 this.amount = new Quantity(); 1089 return this.amount; 1090 } else if (name.equals("amountString")) { 1091 this.amount = new StringType(); 1092 return this.amount; 1093 } else 1094 return super.addChild(name); 1095 } 1096 1097 public SubstanceSpecificationPropertyComponent copy() { 1098 SubstanceSpecificationPropertyComponent dst = new SubstanceSpecificationPropertyComponent(); 1099 copyValues(dst); 1100 return dst; 1101 } 1102 1103 public void copyValues(SubstanceSpecificationPropertyComponent dst) { 1104 super.copyValues(dst); 1105 dst.category = category == null ? null : category.copy(); 1106 dst.code = code == null ? null : code.copy(); 1107 dst.parameters = parameters == null ? null : parameters.copy(); 1108 dst.definingSubstance = definingSubstance == null ? null : definingSubstance.copy(); 1109 dst.amount = amount == null ? null : amount.copy(); 1110 } 1111 1112 @Override 1113 public boolean equalsDeep(Base other_) { 1114 if (!super.equalsDeep(other_)) 1115 return false; 1116 if (!(other_ instanceof SubstanceSpecificationPropertyComponent)) 1117 return false; 1118 SubstanceSpecificationPropertyComponent o = (SubstanceSpecificationPropertyComponent) other_; 1119 return compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1120 && compareDeep(parameters, o.parameters, true) && compareDeep(definingSubstance, o.definingSubstance, true) 1121 && compareDeep(amount, o.amount, true); 1122 } 1123 1124 @Override 1125 public boolean equalsShallow(Base other_) { 1126 if (!super.equalsShallow(other_)) 1127 return false; 1128 if (!(other_ instanceof SubstanceSpecificationPropertyComponent)) 1129 return false; 1130 SubstanceSpecificationPropertyComponent o = (SubstanceSpecificationPropertyComponent) other_; 1131 return compareValues(parameters, o.parameters, true); 1132 } 1133 1134 public boolean isEmpty() { 1135 return super.isEmpty() 1136 && ca.uhn.fhir.util.ElementUtil.isEmpty(category, code, parameters, definingSubstance, amount); 1137 } 1138 1139 public String fhirType() { 1140 return "SubstanceSpecification.property"; 1141 1142 } 1143 1144 } 1145 1146 @Block() 1147 public static class SubstanceSpecificationStructureComponent extends BackboneElement implements IBaseBackboneElement { 1148 /** 1149 * Stereochemistry type. 1150 */ 1151 @Child(name = "stereochemistry", type = { 1152 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1153 @Description(shortDefinition = "Stereochemistry type", formalDefinition = "Stereochemistry type.") 1154 protected CodeableConcept stereochemistry; 1155 1156 /** 1157 * Optical activity type. 1158 */ 1159 @Child(name = "opticalActivity", type = { 1160 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1161 @Description(shortDefinition = "Optical activity type", formalDefinition = "Optical activity type.") 1162 protected CodeableConcept opticalActivity; 1163 1164 /** 1165 * Molecular formula. 1166 */ 1167 @Child(name = "molecularFormula", type = { 1168 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1169 @Description(shortDefinition = "Molecular formula", formalDefinition = "Molecular formula.") 1170 protected StringType molecularFormula; 1171 1172 /** 1173 * Specified per moiety according to the Hill system, i.e. first C, then H, then 1174 * alphabetical, each moiety separated by a dot. 1175 */ 1176 @Child(name = "molecularFormulaByMoiety", type = { 1177 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1178 @Description(shortDefinition = "Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot", formalDefinition = "Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot.") 1179 protected StringType molecularFormulaByMoiety; 1180 1181 /** 1182 * Applicable for single substances that contain a radionuclide or a non-natural 1183 * isotopic ratio. 1184 */ 1185 @Child(name = "isotope", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1186 @Description(shortDefinition = "Applicable for single substances that contain a radionuclide or a non-natural isotopic ratio", formalDefinition = "Applicable for single substances that contain a radionuclide or a non-natural isotopic ratio.") 1187 protected List<SubstanceSpecificationStructureIsotopeComponent> isotope; 1188 1189 /** 1190 * The molecular weight or weight range (for proteins, polymers or nucleic 1191 * acids). 1192 */ 1193 @Child(name = "molecularWeight", type = { 1194 SubstanceSpecificationStructureIsotopeMolecularWeightComponent.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1195 @Description(shortDefinition = "The molecular weight or weight range (for proteins, polymers or nucleic acids)", formalDefinition = "The molecular weight or weight range (for proteins, polymers or nucleic acids).") 1196 protected SubstanceSpecificationStructureIsotopeMolecularWeightComponent molecularWeight; 1197 1198 /** 1199 * Supporting literature. 1200 */ 1201 @Child(name = "source", type = { 1202 DocumentReference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1203 @Description(shortDefinition = "Supporting literature", formalDefinition = "Supporting literature.") 1204 protected List<Reference> source; 1205 /** 1206 * The actual objects that are the target of the reference (Supporting 1207 * literature.) 1208 */ 1209 protected List<DocumentReference> sourceTarget; 1210 1211 /** 1212 * Molecular structural representation. 1213 */ 1214 @Child(name = "representation", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1215 @Description(shortDefinition = "Molecular structural representation", formalDefinition = "Molecular structural representation.") 1216 protected List<SubstanceSpecificationStructureRepresentationComponent> representation; 1217 1218 private static final long serialVersionUID = -851521497L; 1219 1220 /** 1221 * Constructor 1222 */ 1223 public SubstanceSpecificationStructureComponent() { 1224 super(); 1225 } 1226 1227 /** 1228 * @return {@link #stereochemistry} (Stereochemistry type.) 1229 */ 1230 public CodeableConcept getStereochemistry() { 1231 if (this.stereochemistry == null) 1232 if (Configuration.errorOnAutoCreate()) 1233 throw new Error("Attempt to auto-create SubstanceSpecificationStructureComponent.stereochemistry"); 1234 else if (Configuration.doAutoCreate()) 1235 this.stereochemistry = new CodeableConcept(); // cc 1236 return this.stereochemistry; 1237 } 1238 1239 public boolean hasStereochemistry() { 1240 return this.stereochemistry != null && !this.stereochemistry.isEmpty(); 1241 } 1242 1243 /** 1244 * @param value {@link #stereochemistry} (Stereochemistry type.) 1245 */ 1246 public SubstanceSpecificationStructureComponent setStereochemistry(CodeableConcept value) { 1247 this.stereochemistry = value; 1248 return this; 1249 } 1250 1251 /** 1252 * @return {@link #opticalActivity} (Optical activity type.) 1253 */ 1254 public CodeableConcept getOpticalActivity() { 1255 if (this.opticalActivity == null) 1256 if (Configuration.errorOnAutoCreate()) 1257 throw new Error("Attempt to auto-create SubstanceSpecificationStructureComponent.opticalActivity"); 1258 else if (Configuration.doAutoCreate()) 1259 this.opticalActivity = new CodeableConcept(); // cc 1260 return this.opticalActivity; 1261 } 1262 1263 public boolean hasOpticalActivity() { 1264 return this.opticalActivity != null && !this.opticalActivity.isEmpty(); 1265 } 1266 1267 /** 1268 * @param value {@link #opticalActivity} (Optical activity type.) 1269 */ 1270 public SubstanceSpecificationStructureComponent setOpticalActivity(CodeableConcept value) { 1271 this.opticalActivity = value; 1272 return this; 1273 } 1274 1275 /** 1276 * @return {@link #molecularFormula} (Molecular formula.). This is the 1277 * underlying object with id, value and extensions. The accessor 1278 * "getMolecularFormula" gives direct access to the value 1279 */ 1280 public StringType getMolecularFormulaElement() { 1281 if (this.molecularFormula == null) 1282 if (Configuration.errorOnAutoCreate()) 1283 throw new Error("Attempt to auto-create SubstanceSpecificationStructureComponent.molecularFormula"); 1284 else if (Configuration.doAutoCreate()) 1285 this.molecularFormula = new StringType(); // bb 1286 return this.molecularFormula; 1287 } 1288 1289 public boolean hasMolecularFormulaElement() { 1290 return this.molecularFormula != null && !this.molecularFormula.isEmpty(); 1291 } 1292 1293 public boolean hasMolecularFormula() { 1294 return this.molecularFormula != null && !this.molecularFormula.isEmpty(); 1295 } 1296 1297 /** 1298 * @param value {@link #molecularFormula} (Molecular formula.). This is the 1299 * underlying object with id, value and extensions. The accessor 1300 * "getMolecularFormula" gives direct access to the value 1301 */ 1302 public SubstanceSpecificationStructureComponent setMolecularFormulaElement(StringType value) { 1303 this.molecularFormula = value; 1304 return this; 1305 } 1306 1307 /** 1308 * @return Molecular formula. 1309 */ 1310 public String getMolecularFormula() { 1311 return this.molecularFormula == null ? null : this.molecularFormula.getValue(); 1312 } 1313 1314 /** 1315 * @param value Molecular formula. 1316 */ 1317 public SubstanceSpecificationStructureComponent setMolecularFormula(String value) { 1318 if (Utilities.noString(value)) 1319 this.molecularFormula = null; 1320 else { 1321 if (this.molecularFormula == null) 1322 this.molecularFormula = new StringType(); 1323 this.molecularFormula.setValue(value); 1324 } 1325 return this; 1326 } 1327 1328 /** 1329 * @return {@link #molecularFormulaByMoiety} (Specified per moiety according to 1330 * the Hill system, i.e. first C, then H, then alphabetical, each moiety 1331 * separated by a dot.). This is the underlying object with id, value 1332 * and extensions. The accessor "getMolecularFormulaByMoiety" gives 1333 * direct access to the value 1334 */ 1335 public StringType getMolecularFormulaByMoietyElement() { 1336 if (this.molecularFormulaByMoiety == null) 1337 if (Configuration.errorOnAutoCreate()) 1338 throw new Error("Attempt to auto-create SubstanceSpecificationStructureComponent.molecularFormulaByMoiety"); 1339 else if (Configuration.doAutoCreate()) 1340 this.molecularFormulaByMoiety = new StringType(); // bb 1341 return this.molecularFormulaByMoiety; 1342 } 1343 1344 public boolean hasMolecularFormulaByMoietyElement() { 1345 return this.molecularFormulaByMoiety != null && !this.molecularFormulaByMoiety.isEmpty(); 1346 } 1347 1348 public boolean hasMolecularFormulaByMoiety() { 1349 return this.molecularFormulaByMoiety != null && !this.molecularFormulaByMoiety.isEmpty(); 1350 } 1351 1352 /** 1353 * @param value {@link #molecularFormulaByMoiety} (Specified per moiety 1354 * according to the Hill system, i.e. first C, then H, then 1355 * alphabetical, each moiety separated by a dot.). This is the 1356 * underlying object with id, value and extensions. The accessor 1357 * "getMolecularFormulaByMoiety" gives direct access to the value 1358 */ 1359 public SubstanceSpecificationStructureComponent setMolecularFormulaByMoietyElement(StringType value) { 1360 this.molecularFormulaByMoiety = value; 1361 return this; 1362 } 1363 1364 /** 1365 * @return Specified per moiety according to the Hill system, i.e. first C, then 1366 * H, then alphabetical, each moiety separated by a dot. 1367 */ 1368 public String getMolecularFormulaByMoiety() { 1369 return this.molecularFormulaByMoiety == null ? null : this.molecularFormulaByMoiety.getValue(); 1370 } 1371 1372 /** 1373 * @param value Specified per moiety according to the Hill system, i.e. first C, 1374 * then H, then alphabetical, each moiety separated by a dot. 1375 */ 1376 public SubstanceSpecificationStructureComponent setMolecularFormulaByMoiety(String value) { 1377 if (Utilities.noString(value)) 1378 this.molecularFormulaByMoiety = null; 1379 else { 1380 if (this.molecularFormulaByMoiety == null) 1381 this.molecularFormulaByMoiety = new StringType(); 1382 this.molecularFormulaByMoiety.setValue(value); 1383 } 1384 return this; 1385 } 1386 1387 /** 1388 * @return {@link #isotope} (Applicable for single substances that contain a 1389 * radionuclide or a non-natural isotopic ratio.) 1390 */ 1391 public List<SubstanceSpecificationStructureIsotopeComponent> getIsotope() { 1392 if (this.isotope == null) 1393 this.isotope = new ArrayList<SubstanceSpecificationStructureIsotopeComponent>(); 1394 return this.isotope; 1395 } 1396 1397 /** 1398 * @return Returns a reference to <code>this</code> for easy method chaining 1399 */ 1400 public SubstanceSpecificationStructureComponent setIsotope( 1401 List<SubstanceSpecificationStructureIsotopeComponent> theIsotope) { 1402 this.isotope = theIsotope; 1403 return this; 1404 } 1405 1406 public boolean hasIsotope() { 1407 if (this.isotope == null) 1408 return false; 1409 for (SubstanceSpecificationStructureIsotopeComponent item : this.isotope) 1410 if (!item.isEmpty()) 1411 return true; 1412 return false; 1413 } 1414 1415 public SubstanceSpecificationStructureIsotopeComponent addIsotope() { // 3 1416 SubstanceSpecificationStructureIsotopeComponent t = new SubstanceSpecificationStructureIsotopeComponent(); 1417 if (this.isotope == null) 1418 this.isotope = new ArrayList<SubstanceSpecificationStructureIsotopeComponent>(); 1419 this.isotope.add(t); 1420 return t; 1421 } 1422 1423 public SubstanceSpecificationStructureComponent addIsotope(SubstanceSpecificationStructureIsotopeComponent t) { // 3 1424 if (t == null) 1425 return this; 1426 if (this.isotope == null) 1427 this.isotope = new ArrayList<SubstanceSpecificationStructureIsotopeComponent>(); 1428 this.isotope.add(t); 1429 return this; 1430 } 1431 1432 /** 1433 * @return The first repetition of repeating field {@link #isotope}, creating it 1434 * if it does not already exist 1435 */ 1436 public SubstanceSpecificationStructureIsotopeComponent getIsotopeFirstRep() { 1437 if (getIsotope().isEmpty()) { 1438 addIsotope(); 1439 } 1440 return getIsotope().get(0); 1441 } 1442 1443 /** 1444 * @return {@link #molecularWeight} (The molecular weight or weight range (for 1445 * proteins, polymers or nucleic acids).) 1446 */ 1447 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent getMolecularWeight() { 1448 if (this.molecularWeight == null) 1449 if (Configuration.errorOnAutoCreate()) 1450 throw new Error("Attempt to auto-create SubstanceSpecificationStructureComponent.molecularWeight"); 1451 else if (Configuration.doAutoCreate()) 1452 this.molecularWeight = new SubstanceSpecificationStructureIsotopeMolecularWeightComponent(); // cc 1453 return this.molecularWeight; 1454 } 1455 1456 public boolean hasMolecularWeight() { 1457 return this.molecularWeight != null && !this.molecularWeight.isEmpty(); 1458 } 1459 1460 /** 1461 * @param value {@link #molecularWeight} (The molecular weight or weight range 1462 * (for proteins, polymers or nucleic acids).) 1463 */ 1464 public SubstanceSpecificationStructureComponent setMolecularWeight( 1465 SubstanceSpecificationStructureIsotopeMolecularWeightComponent value) { 1466 this.molecularWeight = value; 1467 return this; 1468 } 1469 1470 /** 1471 * @return {@link #source} (Supporting literature.) 1472 */ 1473 public List<Reference> getSource() { 1474 if (this.source == null) 1475 this.source = new ArrayList<Reference>(); 1476 return this.source; 1477 } 1478 1479 /** 1480 * @return Returns a reference to <code>this</code> for easy method chaining 1481 */ 1482 public SubstanceSpecificationStructureComponent setSource(List<Reference> theSource) { 1483 this.source = theSource; 1484 return this; 1485 } 1486 1487 public boolean hasSource() { 1488 if (this.source == null) 1489 return false; 1490 for (Reference item : this.source) 1491 if (!item.isEmpty()) 1492 return true; 1493 return false; 1494 } 1495 1496 public Reference addSource() { // 3 1497 Reference t = new Reference(); 1498 if (this.source == null) 1499 this.source = new ArrayList<Reference>(); 1500 this.source.add(t); 1501 return t; 1502 } 1503 1504 public SubstanceSpecificationStructureComponent addSource(Reference t) { // 3 1505 if (t == null) 1506 return this; 1507 if (this.source == null) 1508 this.source = new ArrayList<Reference>(); 1509 this.source.add(t); 1510 return this; 1511 } 1512 1513 /** 1514 * @return The first repetition of repeating field {@link #source}, creating it 1515 * if it does not already exist 1516 */ 1517 public Reference getSourceFirstRep() { 1518 if (getSource().isEmpty()) { 1519 addSource(); 1520 } 1521 return getSource().get(0); 1522 } 1523 1524 /** 1525 * @deprecated Use Reference#setResource(IBaseResource) instead 1526 */ 1527 @Deprecated 1528 public List<DocumentReference> getSourceTarget() { 1529 if (this.sourceTarget == null) 1530 this.sourceTarget = new ArrayList<DocumentReference>(); 1531 return this.sourceTarget; 1532 } 1533 1534 /** 1535 * @deprecated Use Reference#setResource(IBaseResource) instead 1536 */ 1537 @Deprecated 1538 public DocumentReference addSourceTarget() { 1539 DocumentReference r = new DocumentReference(); 1540 if (this.sourceTarget == null) 1541 this.sourceTarget = new ArrayList<DocumentReference>(); 1542 this.sourceTarget.add(r); 1543 return r; 1544 } 1545 1546 /** 1547 * @return {@link #representation} (Molecular structural representation.) 1548 */ 1549 public List<SubstanceSpecificationStructureRepresentationComponent> getRepresentation() { 1550 if (this.representation == null) 1551 this.representation = new ArrayList<SubstanceSpecificationStructureRepresentationComponent>(); 1552 return this.representation; 1553 } 1554 1555 /** 1556 * @return Returns a reference to <code>this</code> for easy method chaining 1557 */ 1558 public SubstanceSpecificationStructureComponent setRepresentation( 1559 List<SubstanceSpecificationStructureRepresentationComponent> theRepresentation) { 1560 this.representation = theRepresentation; 1561 return this; 1562 } 1563 1564 public boolean hasRepresentation() { 1565 if (this.representation == null) 1566 return false; 1567 for (SubstanceSpecificationStructureRepresentationComponent item : this.representation) 1568 if (!item.isEmpty()) 1569 return true; 1570 return false; 1571 } 1572 1573 public SubstanceSpecificationStructureRepresentationComponent addRepresentation() { // 3 1574 SubstanceSpecificationStructureRepresentationComponent t = new SubstanceSpecificationStructureRepresentationComponent(); 1575 if (this.representation == null) 1576 this.representation = new ArrayList<SubstanceSpecificationStructureRepresentationComponent>(); 1577 this.representation.add(t); 1578 return t; 1579 } 1580 1581 public SubstanceSpecificationStructureComponent addRepresentation( 1582 SubstanceSpecificationStructureRepresentationComponent t) { // 3 1583 if (t == null) 1584 return this; 1585 if (this.representation == null) 1586 this.representation = new ArrayList<SubstanceSpecificationStructureRepresentationComponent>(); 1587 this.representation.add(t); 1588 return this; 1589 } 1590 1591 /** 1592 * @return The first repetition of repeating field {@link #representation}, 1593 * creating it if it does not already exist 1594 */ 1595 public SubstanceSpecificationStructureRepresentationComponent getRepresentationFirstRep() { 1596 if (getRepresentation().isEmpty()) { 1597 addRepresentation(); 1598 } 1599 return getRepresentation().get(0); 1600 } 1601 1602 protected void listChildren(List<Property> children) { 1603 super.listChildren(children); 1604 children.add(new Property("stereochemistry", "CodeableConcept", "Stereochemistry type.", 0, 1, stereochemistry)); 1605 children.add(new Property("opticalActivity", "CodeableConcept", "Optical activity type.", 0, 1, opticalActivity)); 1606 children.add(new Property("molecularFormula", "string", "Molecular formula.", 0, 1, molecularFormula)); 1607 children.add(new Property("molecularFormulaByMoiety", "string", 1608 "Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot.", 1609 0, 1, molecularFormulaByMoiety)); 1610 children.add(new Property("isotope", "", 1611 "Applicable for single substances that contain a radionuclide or a non-natural isotopic ratio.", 0, 1612 java.lang.Integer.MAX_VALUE, isotope)); 1613 children.add(new Property("molecularWeight", "@SubstanceSpecification.structure.isotope.molecularWeight", 1614 "The molecular weight or weight range (for proteins, polymers or nucleic acids).", 0, 1, molecularWeight)); 1615 children.add(new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 1616 java.lang.Integer.MAX_VALUE, source)); 1617 children.add(new Property("representation", "", "Molecular structural representation.", 0, 1618 java.lang.Integer.MAX_VALUE, representation)); 1619 } 1620 1621 @Override 1622 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1623 switch (_hash) { 1624 case 263475116: 1625 /* stereochemistry */ return new Property("stereochemistry", "CodeableConcept", "Stereochemistry type.", 0, 1, 1626 stereochemistry); 1627 case 1420900135: 1628 /* opticalActivity */ return new Property("opticalActivity", "CodeableConcept", "Optical activity type.", 0, 1, 1629 opticalActivity); 1630 case 616660246: 1631 /* molecularFormula */ return new Property("molecularFormula", "string", "Molecular formula.", 0, 1, 1632 molecularFormula); 1633 case 1315452848: 1634 /* molecularFormulaByMoiety */ return new Property("molecularFormulaByMoiety", "string", 1635 "Specified per moiety according to the Hill system, i.e. first C, then H, then alphabetical, each moiety separated by a dot.", 1636 0, 1, molecularFormulaByMoiety); 1637 case 2097035189: 1638 /* isotope */ return new Property("isotope", "", 1639 "Applicable for single substances that contain a radionuclide or a non-natural isotopic ratio.", 0, 1640 java.lang.Integer.MAX_VALUE, isotope); 1641 case 635625672: 1642 /* molecularWeight */ return new Property("molecularWeight", 1643 "@SubstanceSpecification.structure.isotope.molecularWeight", 1644 "The molecular weight or weight range (for proteins, polymers or nucleic acids).", 0, 1, molecularWeight); 1645 case -896505829: 1646 /* source */ return new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 1647 java.lang.Integer.MAX_VALUE, source); 1648 case -671065907: 1649 /* representation */ return new Property("representation", "", "Molecular structural representation.", 0, 1650 java.lang.Integer.MAX_VALUE, representation); 1651 default: 1652 return super.getNamedProperty(_hash, _name, _checkValid); 1653 } 1654 1655 } 1656 1657 @Override 1658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1659 switch (hash) { 1660 case 263475116: 1661 /* stereochemistry */ return this.stereochemistry == null ? new Base[0] : new Base[] { this.stereochemistry }; // CodeableConcept 1662 case 1420900135: 1663 /* opticalActivity */ return this.opticalActivity == null ? new Base[0] : new Base[] { this.opticalActivity }; // CodeableConcept 1664 case 616660246: 1665 /* molecularFormula */ return this.molecularFormula == null ? new Base[0] 1666 : new Base[] { this.molecularFormula }; // StringType 1667 case 1315452848: 1668 /* molecularFormulaByMoiety */ return this.molecularFormulaByMoiety == null ? new Base[0] 1669 : new Base[] { this.molecularFormulaByMoiety }; // StringType 1670 case 2097035189: 1671 /* isotope */ return this.isotope == null ? new Base[0] : this.isotope.toArray(new Base[this.isotope.size()]); // SubstanceSpecificationStructureIsotopeComponent 1672 case 635625672: 1673 /* molecularWeight */ return this.molecularWeight == null ? new Base[0] : new Base[] { this.molecularWeight }; // SubstanceSpecificationStructureIsotopeMolecularWeightComponent 1674 case -896505829: 1675 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 1676 case -671065907: 1677 /* representation */ return this.representation == null ? new Base[0] 1678 : this.representation.toArray(new Base[this.representation.size()]); // SubstanceSpecificationStructureRepresentationComponent 1679 default: 1680 return super.getProperty(hash, name, checkValid); 1681 } 1682 1683 } 1684 1685 @Override 1686 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1687 switch (hash) { 1688 case 263475116: // stereochemistry 1689 this.stereochemistry = castToCodeableConcept(value); // CodeableConcept 1690 return value; 1691 case 1420900135: // opticalActivity 1692 this.opticalActivity = castToCodeableConcept(value); // CodeableConcept 1693 return value; 1694 case 616660246: // molecularFormula 1695 this.molecularFormula = castToString(value); // StringType 1696 return value; 1697 case 1315452848: // molecularFormulaByMoiety 1698 this.molecularFormulaByMoiety = castToString(value); // StringType 1699 return value; 1700 case 2097035189: // isotope 1701 this.getIsotope().add((SubstanceSpecificationStructureIsotopeComponent) value); // SubstanceSpecificationStructureIsotopeComponent 1702 return value; 1703 case 635625672: // molecularWeight 1704 this.molecularWeight = (SubstanceSpecificationStructureIsotopeMolecularWeightComponent) value; // SubstanceSpecificationStructureIsotopeMolecularWeightComponent 1705 return value; 1706 case -896505829: // source 1707 this.getSource().add(castToReference(value)); // Reference 1708 return value; 1709 case -671065907: // representation 1710 this.getRepresentation().add((SubstanceSpecificationStructureRepresentationComponent) value); // SubstanceSpecificationStructureRepresentationComponent 1711 return value; 1712 default: 1713 return super.setProperty(hash, name, value); 1714 } 1715 1716 } 1717 1718 @Override 1719 public Base setProperty(String name, Base value) throws FHIRException { 1720 if (name.equals("stereochemistry")) { 1721 this.stereochemistry = castToCodeableConcept(value); // CodeableConcept 1722 } else if (name.equals("opticalActivity")) { 1723 this.opticalActivity = castToCodeableConcept(value); // CodeableConcept 1724 } else if (name.equals("molecularFormula")) { 1725 this.molecularFormula = castToString(value); // StringType 1726 } else if (name.equals("molecularFormulaByMoiety")) { 1727 this.molecularFormulaByMoiety = castToString(value); // StringType 1728 } else if (name.equals("isotope")) { 1729 this.getIsotope().add((SubstanceSpecificationStructureIsotopeComponent) value); 1730 } else if (name.equals("molecularWeight")) { 1731 this.molecularWeight = (SubstanceSpecificationStructureIsotopeMolecularWeightComponent) value; // SubstanceSpecificationStructureIsotopeMolecularWeightComponent 1732 } else if (name.equals("source")) { 1733 this.getSource().add(castToReference(value)); 1734 } else if (name.equals("representation")) { 1735 this.getRepresentation().add((SubstanceSpecificationStructureRepresentationComponent) value); 1736 } else 1737 return super.setProperty(name, value); 1738 return value; 1739 } 1740 1741 @Override 1742 public Base makeProperty(int hash, String name) throws FHIRException { 1743 switch (hash) { 1744 case 263475116: 1745 return getStereochemistry(); 1746 case 1420900135: 1747 return getOpticalActivity(); 1748 case 616660246: 1749 return getMolecularFormulaElement(); 1750 case 1315452848: 1751 return getMolecularFormulaByMoietyElement(); 1752 case 2097035189: 1753 return addIsotope(); 1754 case 635625672: 1755 return getMolecularWeight(); 1756 case -896505829: 1757 return addSource(); 1758 case -671065907: 1759 return addRepresentation(); 1760 default: 1761 return super.makeProperty(hash, name); 1762 } 1763 1764 } 1765 1766 @Override 1767 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1768 switch (hash) { 1769 case 263475116: 1770 /* stereochemistry */ return new String[] { "CodeableConcept" }; 1771 case 1420900135: 1772 /* opticalActivity */ return new String[] { "CodeableConcept" }; 1773 case 616660246: 1774 /* molecularFormula */ return new String[] { "string" }; 1775 case 1315452848: 1776 /* molecularFormulaByMoiety */ return new String[] { "string" }; 1777 case 2097035189: 1778 /* isotope */ return new String[] {}; 1779 case 635625672: 1780 /* molecularWeight */ return new String[] { "@SubstanceSpecification.structure.isotope.molecularWeight" }; 1781 case -896505829: 1782 /* source */ return new String[] { "Reference" }; 1783 case -671065907: 1784 /* representation */ return new String[] {}; 1785 default: 1786 return super.getTypesForProperty(hash, name); 1787 } 1788 1789 } 1790 1791 @Override 1792 public Base addChild(String name) throws FHIRException { 1793 if (name.equals("stereochemistry")) { 1794 this.stereochemistry = new CodeableConcept(); 1795 return this.stereochemistry; 1796 } else if (name.equals("opticalActivity")) { 1797 this.opticalActivity = new CodeableConcept(); 1798 return this.opticalActivity; 1799 } else if (name.equals("molecularFormula")) { 1800 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.molecularFormula"); 1801 } else if (name.equals("molecularFormulaByMoiety")) { 1802 throw new FHIRException( 1803 "Cannot call addChild on a singleton property SubstanceSpecification.molecularFormulaByMoiety"); 1804 } else if (name.equals("isotope")) { 1805 return addIsotope(); 1806 } else if (name.equals("molecularWeight")) { 1807 this.molecularWeight = new SubstanceSpecificationStructureIsotopeMolecularWeightComponent(); 1808 return this.molecularWeight; 1809 } else if (name.equals("source")) { 1810 return addSource(); 1811 } else if (name.equals("representation")) { 1812 return addRepresentation(); 1813 } else 1814 return super.addChild(name); 1815 } 1816 1817 public SubstanceSpecificationStructureComponent copy() { 1818 SubstanceSpecificationStructureComponent dst = new SubstanceSpecificationStructureComponent(); 1819 copyValues(dst); 1820 return dst; 1821 } 1822 1823 public void copyValues(SubstanceSpecificationStructureComponent dst) { 1824 super.copyValues(dst); 1825 dst.stereochemistry = stereochemistry == null ? null : stereochemistry.copy(); 1826 dst.opticalActivity = opticalActivity == null ? null : opticalActivity.copy(); 1827 dst.molecularFormula = molecularFormula == null ? null : molecularFormula.copy(); 1828 dst.molecularFormulaByMoiety = molecularFormulaByMoiety == null ? null : molecularFormulaByMoiety.copy(); 1829 if (isotope != null) { 1830 dst.isotope = new ArrayList<SubstanceSpecificationStructureIsotopeComponent>(); 1831 for (SubstanceSpecificationStructureIsotopeComponent i : isotope) 1832 dst.isotope.add(i.copy()); 1833 } 1834 ; 1835 dst.molecularWeight = molecularWeight == null ? null : molecularWeight.copy(); 1836 if (source != null) { 1837 dst.source = new ArrayList<Reference>(); 1838 for (Reference i : source) 1839 dst.source.add(i.copy()); 1840 } 1841 ; 1842 if (representation != null) { 1843 dst.representation = new ArrayList<SubstanceSpecificationStructureRepresentationComponent>(); 1844 for (SubstanceSpecificationStructureRepresentationComponent i : representation) 1845 dst.representation.add(i.copy()); 1846 } 1847 ; 1848 } 1849 1850 @Override 1851 public boolean equalsDeep(Base other_) { 1852 if (!super.equalsDeep(other_)) 1853 return false; 1854 if (!(other_ instanceof SubstanceSpecificationStructureComponent)) 1855 return false; 1856 SubstanceSpecificationStructureComponent o = (SubstanceSpecificationStructureComponent) other_; 1857 return compareDeep(stereochemistry, o.stereochemistry, true) 1858 && compareDeep(opticalActivity, o.opticalActivity, true) 1859 && compareDeep(molecularFormula, o.molecularFormula, true) 1860 && compareDeep(molecularFormulaByMoiety, o.molecularFormulaByMoiety, true) 1861 && compareDeep(isotope, o.isotope, true) && compareDeep(molecularWeight, o.molecularWeight, true) 1862 && compareDeep(source, o.source, true) && compareDeep(representation, o.representation, true); 1863 } 1864 1865 @Override 1866 public boolean equalsShallow(Base other_) { 1867 if (!super.equalsShallow(other_)) 1868 return false; 1869 if (!(other_ instanceof SubstanceSpecificationStructureComponent)) 1870 return false; 1871 SubstanceSpecificationStructureComponent o = (SubstanceSpecificationStructureComponent) other_; 1872 return compareValues(molecularFormula, o.molecularFormula, true) 1873 && compareValues(molecularFormulaByMoiety, o.molecularFormulaByMoiety, true); 1874 } 1875 1876 public boolean isEmpty() { 1877 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(stereochemistry, opticalActivity, molecularFormula, 1878 molecularFormulaByMoiety, isotope, molecularWeight, source, representation); 1879 } 1880 1881 public String fhirType() { 1882 return "SubstanceSpecification.structure"; 1883 1884 } 1885 1886 } 1887 1888 @Block() 1889 public static class SubstanceSpecificationStructureIsotopeComponent extends BackboneElement 1890 implements IBaseBackboneElement { 1891 /** 1892 * Substance identifier for each non-natural or radioisotope. 1893 */ 1894 @Child(name = "identifier", type = { 1895 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1896 @Description(shortDefinition = "Substance identifier for each non-natural or radioisotope", formalDefinition = "Substance identifier for each non-natural or radioisotope.") 1897 protected Identifier identifier; 1898 1899 /** 1900 * Substance name for each non-natural or radioisotope. 1901 */ 1902 @Child(name = "name", type = { 1903 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1904 @Description(shortDefinition = "Substance name for each non-natural or radioisotope", formalDefinition = "Substance name for each non-natural or radioisotope.") 1905 protected CodeableConcept name; 1906 1907 /** 1908 * The type of isotopic substitution present in a single substance. 1909 */ 1910 @Child(name = "substitution", type = { 1911 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1912 @Description(shortDefinition = "The type of isotopic substitution present in a single substance", formalDefinition = "The type of isotopic substitution present in a single substance.") 1913 protected CodeableConcept substitution; 1914 1915 /** 1916 * Half life - for a non-natural nuclide. 1917 */ 1918 @Child(name = "halfLife", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1919 @Description(shortDefinition = "Half life - for a non-natural nuclide", formalDefinition = "Half life - for a non-natural nuclide.") 1920 protected Quantity halfLife; 1921 1922 /** 1923 * The molecular weight or weight range (for proteins, polymers or nucleic 1924 * acids). 1925 */ 1926 @Child(name = "molecularWeight", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 1927 @Description(shortDefinition = "The molecular weight or weight range (for proteins, polymers or nucleic acids)", formalDefinition = "The molecular weight or weight range (for proteins, polymers or nucleic acids).") 1928 protected SubstanceSpecificationStructureIsotopeMolecularWeightComponent molecularWeight; 1929 1930 private static final long serialVersionUID = -531167114L; 1931 1932 /** 1933 * Constructor 1934 */ 1935 public SubstanceSpecificationStructureIsotopeComponent() { 1936 super(); 1937 } 1938 1939 /** 1940 * @return {@link #identifier} (Substance identifier for each non-natural or 1941 * radioisotope.) 1942 */ 1943 public Identifier getIdentifier() { 1944 if (this.identifier == null) 1945 if (Configuration.errorOnAutoCreate()) 1946 throw new Error("Attempt to auto-create SubstanceSpecificationStructureIsotopeComponent.identifier"); 1947 else if (Configuration.doAutoCreate()) 1948 this.identifier = new Identifier(); // cc 1949 return this.identifier; 1950 } 1951 1952 public boolean hasIdentifier() { 1953 return this.identifier != null && !this.identifier.isEmpty(); 1954 } 1955 1956 /** 1957 * @param value {@link #identifier} (Substance identifier for each non-natural 1958 * or radioisotope.) 1959 */ 1960 public SubstanceSpecificationStructureIsotopeComponent setIdentifier(Identifier value) { 1961 this.identifier = value; 1962 return this; 1963 } 1964 1965 /** 1966 * @return {@link #name} (Substance name for each non-natural or radioisotope.) 1967 */ 1968 public CodeableConcept getName() { 1969 if (this.name == null) 1970 if (Configuration.errorOnAutoCreate()) 1971 throw new Error("Attempt to auto-create SubstanceSpecificationStructureIsotopeComponent.name"); 1972 else if (Configuration.doAutoCreate()) 1973 this.name = new CodeableConcept(); // cc 1974 return this.name; 1975 } 1976 1977 public boolean hasName() { 1978 return this.name != null && !this.name.isEmpty(); 1979 } 1980 1981 /** 1982 * @param value {@link #name} (Substance name for each non-natural or 1983 * radioisotope.) 1984 */ 1985 public SubstanceSpecificationStructureIsotopeComponent setName(CodeableConcept value) { 1986 this.name = value; 1987 return this; 1988 } 1989 1990 /** 1991 * @return {@link #substitution} (The type of isotopic substitution present in a 1992 * single substance.) 1993 */ 1994 public CodeableConcept getSubstitution() { 1995 if (this.substitution == null) 1996 if (Configuration.errorOnAutoCreate()) 1997 throw new Error("Attempt to auto-create SubstanceSpecificationStructureIsotopeComponent.substitution"); 1998 else if (Configuration.doAutoCreate()) 1999 this.substitution = new CodeableConcept(); // cc 2000 return this.substitution; 2001 } 2002 2003 public boolean hasSubstitution() { 2004 return this.substitution != null && !this.substitution.isEmpty(); 2005 } 2006 2007 /** 2008 * @param value {@link #substitution} (The type of isotopic substitution present 2009 * in a single substance.) 2010 */ 2011 public SubstanceSpecificationStructureIsotopeComponent setSubstitution(CodeableConcept value) { 2012 this.substitution = value; 2013 return this; 2014 } 2015 2016 /** 2017 * @return {@link #halfLife} (Half life - for a non-natural nuclide.) 2018 */ 2019 public Quantity getHalfLife() { 2020 if (this.halfLife == null) 2021 if (Configuration.errorOnAutoCreate()) 2022 throw new Error("Attempt to auto-create SubstanceSpecificationStructureIsotopeComponent.halfLife"); 2023 else if (Configuration.doAutoCreate()) 2024 this.halfLife = new Quantity(); // cc 2025 return this.halfLife; 2026 } 2027 2028 public boolean hasHalfLife() { 2029 return this.halfLife != null && !this.halfLife.isEmpty(); 2030 } 2031 2032 /** 2033 * @param value {@link #halfLife} (Half life - for a non-natural nuclide.) 2034 */ 2035 public SubstanceSpecificationStructureIsotopeComponent setHalfLife(Quantity value) { 2036 this.halfLife = value; 2037 return this; 2038 } 2039 2040 /** 2041 * @return {@link #molecularWeight} (The molecular weight or weight range (for 2042 * proteins, polymers or nucleic acids).) 2043 */ 2044 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent getMolecularWeight() { 2045 if (this.molecularWeight == null) 2046 if (Configuration.errorOnAutoCreate()) 2047 throw new Error("Attempt to auto-create SubstanceSpecificationStructureIsotopeComponent.molecularWeight"); 2048 else if (Configuration.doAutoCreate()) 2049 this.molecularWeight = new SubstanceSpecificationStructureIsotopeMolecularWeightComponent(); // cc 2050 return this.molecularWeight; 2051 } 2052 2053 public boolean hasMolecularWeight() { 2054 return this.molecularWeight != null && !this.molecularWeight.isEmpty(); 2055 } 2056 2057 /** 2058 * @param value {@link #molecularWeight} (The molecular weight or weight range 2059 * (for proteins, polymers or nucleic acids).) 2060 */ 2061 public SubstanceSpecificationStructureIsotopeComponent setMolecularWeight( 2062 SubstanceSpecificationStructureIsotopeMolecularWeightComponent value) { 2063 this.molecularWeight = value; 2064 return this; 2065 } 2066 2067 protected void listChildren(List<Property> children) { 2068 super.listChildren(children); 2069 children.add(new Property("identifier", "Identifier", 2070 "Substance identifier for each non-natural or radioisotope.", 0, 1, identifier)); 2071 children.add( 2072 new Property("name", "CodeableConcept", "Substance name for each non-natural or radioisotope.", 0, 1, name)); 2073 children.add(new Property("substitution", "CodeableConcept", 2074 "The type of isotopic substitution present in a single substance.", 0, 1, substitution)); 2075 children.add(new Property("halfLife", "Quantity", "Half life - for a non-natural nuclide.", 0, 1, halfLife)); 2076 children.add(new Property("molecularWeight", "", 2077 "The molecular weight or weight range (for proteins, polymers or nucleic acids).", 0, 1, molecularWeight)); 2078 } 2079 2080 @Override 2081 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2082 switch (_hash) { 2083 case -1618432855: 2084 /* identifier */ return new Property("identifier", "Identifier", 2085 "Substance identifier for each non-natural or radioisotope.", 0, 1, identifier); 2086 case 3373707: 2087 /* name */ return new Property("name", "CodeableConcept", 2088 "Substance name for each non-natural or radioisotope.", 0, 1, name); 2089 case 826147581: 2090 /* substitution */ return new Property("substitution", "CodeableConcept", 2091 "The type of isotopic substitution present in a single substance.", 0, 1, substitution); 2092 case -54292017: 2093 /* halfLife */ return new Property("halfLife", "Quantity", "Half life - for a non-natural nuclide.", 0, 1, 2094 halfLife); 2095 case 635625672: 2096 /* molecularWeight */ return new Property("molecularWeight", "", 2097 "The molecular weight or weight range (for proteins, polymers or nucleic acids).", 0, 1, molecularWeight); 2098 default: 2099 return super.getNamedProperty(_hash, _name, _checkValid); 2100 } 2101 2102 } 2103 2104 @Override 2105 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2106 switch (hash) { 2107 case -1618432855: 2108 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 2109 case 3373707: 2110 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // CodeableConcept 2111 case 826147581: 2112 /* substitution */ return this.substitution == null ? new Base[0] : new Base[] { this.substitution }; // CodeableConcept 2113 case -54292017: 2114 /* halfLife */ return this.halfLife == null ? new Base[0] : new Base[] { this.halfLife }; // Quantity 2115 case 635625672: 2116 /* molecularWeight */ return this.molecularWeight == null ? new Base[0] : new Base[] { this.molecularWeight }; // SubstanceSpecificationStructureIsotopeMolecularWeightComponent 2117 default: 2118 return super.getProperty(hash, name, checkValid); 2119 } 2120 2121 } 2122 2123 @Override 2124 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2125 switch (hash) { 2126 case -1618432855: // identifier 2127 this.identifier = castToIdentifier(value); // Identifier 2128 return value; 2129 case 3373707: // name 2130 this.name = castToCodeableConcept(value); // CodeableConcept 2131 return value; 2132 case 826147581: // substitution 2133 this.substitution = castToCodeableConcept(value); // CodeableConcept 2134 return value; 2135 case -54292017: // halfLife 2136 this.halfLife = castToQuantity(value); // Quantity 2137 return value; 2138 case 635625672: // molecularWeight 2139 this.molecularWeight = (SubstanceSpecificationStructureIsotopeMolecularWeightComponent) value; // SubstanceSpecificationStructureIsotopeMolecularWeightComponent 2140 return value; 2141 default: 2142 return super.setProperty(hash, name, value); 2143 } 2144 2145 } 2146 2147 @Override 2148 public Base setProperty(String name, Base value) throws FHIRException { 2149 if (name.equals("identifier")) { 2150 this.identifier = castToIdentifier(value); // Identifier 2151 } else if (name.equals("name")) { 2152 this.name = castToCodeableConcept(value); // CodeableConcept 2153 } else if (name.equals("substitution")) { 2154 this.substitution = castToCodeableConcept(value); // CodeableConcept 2155 } else if (name.equals("halfLife")) { 2156 this.halfLife = castToQuantity(value); // Quantity 2157 } else if (name.equals("molecularWeight")) { 2158 this.molecularWeight = (SubstanceSpecificationStructureIsotopeMolecularWeightComponent) value; // SubstanceSpecificationStructureIsotopeMolecularWeightComponent 2159 } else 2160 return super.setProperty(name, value); 2161 return value; 2162 } 2163 2164 @Override 2165 public Base makeProperty(int hash, String name) throws FHIRException { 2166 switch (hash) { 2167 case -1618432855: 2168 return getIdentifier(); 2169 case 3373707: 2170 return getName(); 2171 case 826147581: 2172 return getSubstitution(); 2173 case -54292017: 2174 return getHalfLife(); 2175 case 635625672: 2176 return getMolecularWeight(); 2177 default: 2178 return super.makeProperty(hash, name); 2179 } 2180 2181 } 2182 2183 @Override 2184 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2185 switch (hash) { 2186 case -1618432855: 2187 /* identifier */ return new String[] { "Identifier" }; 2188 case 3373707: 2189 /* name */ return new String[] { "CodeableConcept" }; 2190 case 826147581: 2191 /* substitution */ return new String[] { "CodeableConcept" }; 2192 case -54292017: 2193 /* halfLife */ return new String[] { "Quantity" }; 2194 case 635625672: 2195 /* molecularWeight */ return new String[] {}; 2196 default: 2197 return super.getTypesForProperty(hash, name); 2198 } 2199 2200 } 2201 2202 @Override 2203 public Base addChild(String name) throws FHIRException { 2204 if (name.equals("identifier")) { 2205 this.identifier = new Identifier(); 2206 return this.identifier; 2207 } else if (name.equals("name")) { 2208 this.name = new CodeableConcept(); 2209 return this.name; 2210 } else if (name.equals("substitution")) { 2211 this.substitution = new CodeableConcept(); 2212 return this.substitution; 2213 } else if (name.equals("halfLife")) { 2214 this.halfLife = new Quantity(); 2215 return this.halfLife; 2216 } else if (name.equals("molecularWeight")) { 2217 this.molecularWeight = new SubstanceSpecificationStructureIsotopeMolecularWeightComponent(); 2218 return this.molecularWeight; 2219 } else 2220 return super.addChild(name); 2221 } 2222 2223 public SubstanceSpecificationStructureIsotopeComponent copy() { 2224 SubstanceSpecificationStructureIsotopeComponent dst = new SubstanceSpecificationStructureIsotopeComponent(); 2225 copyValues(dst); 2226 return dst; 2227 } 2228 2229 public void copyValues(SubstanceSpecificationStructureIsotopeComponent dst) { 2230 super.copyValues(dst); 2231 dst.identifier = identifier == null ? null : identifier.copy(); 2232 dst.name = name == null ? null : name.copy(); 2233 dst.substitution = substitution == null ? null : substitution.copy(); 2234 dst.halfLife = halfLife == null ? null : halfLife.copy(); 2235 dst.molecularWeight = molecularWeight == null ? null : molecularWeight.copy(); 2236 } 2237 2238 @Override 2239 public boolean equalsDeep(Base other_) { 2240 if (!super.equalsDeep(other_)) 2241 return false; 2242 if (!(other_ instanceof SubstanceSpecificationStructureIsotopeComponent)) 2243 return false; 2244 SubstanceSpecificationStructureIsotopeComponent o = (SubstanceSpecificationStructureIsotopeComponent) other_; 2245 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 2246 && compareDeep(substitution, o.substitution, true) && compareDeep(halfLife, o.halfLife, true) 2247 && compareDeep(molecularWeight, o.molecularWeight, true); 2248 } 2249 2250 @Override 2251 public boolean equalsShallow(Base other_) { 2252 if (!super.equalsShallow(other_)) 2253 return false; 2254 if (!(other_ instanceof SubstanceSpecificationStructureIsotopeComponent)) 2255 return false; 2256 SubstanceSpecificationStructureIsotopeComponent o = (SubstanceSpecificationStructureIsotopeComponent) other_; 2257 return true; 2258 } 2259 2260 public boolean isEmpty() { 2261 return super.isEmpty() 2262 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, substitution, halfLife, molecularWeight); 2263 } 2264 2265 public String fhirType() { 2266 return "SubstanceSpecification.structure.isotope"; 2267 2268 } 2269 2270 } 2271 2272 @Block() 2273 public static class SubstanceSpecificationStructureIsotopeMolecularWeightComponent extends BackboneElement 2274 implements IBaseBackboneElement { 2275 /** 2276 * The method by which the molecular weight was determined. 2277 */ 2278 @Child(name = "method", type = { 2279 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2280 @Description(shortDefinition = "The method by which the molecular weight was determined", formalDefinition = "The method by which the molecular weight was determined.") 2281 protected CodeableConcept method; 2282 2283 /** 2284 * Type of molecular weight such as exact, average (also known as. number 2285 * average), weight average. 2286 */ 2287 @Child(name = "type", type = { 2288 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2289 @Description(shortDefinition = "Type of molecular weight such as exact, average (also known as. number average), weight average", formalDefinition = "Type of molecular weight such as exact, average (also known as. number average), weight average.") 2290 protected CodeableConcept type; 2291 2292 /** 2293 * Used to capture quantitative values for a variety of elements. If only limits 2294 * are given, the arithmetic mean would be the average. If only a single 2295 * definite value for a given element is given, it would be captured in this 2296 * field. 2297 */ 2298 @Child(name = "amount", type = { Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2299 @Description(shortDefinition = "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field", formalDefinition = "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.") 2300 protected Quantity amount; 2301 2302 private static final long serialVersionUID = 805939780L; 2303 2304 /** 2305 * Constructor 2306 */ 2307 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent() { 2308 super(); 2309 } 2310 2311 /** 2312 * @return {@link #method} (The method by which the molecular weight was 2313 * determined.) 2314 */ 2315 public CodeableConcept getMethod() { 2316 if (this.method == null) 2317 if (Configuration.errorOnAutoCreate()) 2318 throw new Error( 2319 "Attempt to auto-create SubstanceSpecificationStructureIsotopeMolecularWeightComponent.method"); 2320 else if (Configuration.doAutoCreate()) 2321 this.method = new CodeableConcept(); // cc 2322 return this.method; 2323 } 2324 2325 public boolean hasMethod() { 2326 return this.method != null && !this.method.isEmpty(); 2327 } 2328 2329 /** 2330 * @param value {@link #method} (The method by which the molecular weight was 2331 * determined.) 2332 */ 2333 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent setMethod(CodeableConcept value) { 2334 this.method = value; 2335 return this; 2336 } 2337 2338 /** 2339 * @return {@link #type} (Type of molecular weight such as exact, average (also 2340 * known as. number average), weight average.) 2341 */ 2342 public CodeableConcept getType() { 2343 if (this.type == null) 2344 if (Configuration.errorOnAutoCreate()) 2345 throw new Error("Attempt to auto-create SubstanceSpecificationStructureIsotopeMolecularWeightComponent.type"); 2346 else if (Configuration.doAutoCreate()) 2347 this.type = new CodeableConcept(); // cc 2348 return this.type; 2349 } 2350 2351 public boolean hasType() { 2352 return this.type != null && !this.type.isEmpty(); 2353 } 2354 2355 /** 2356 * @param value {@link #type} (Type of molecular weight such as exact, average 2357 * (also known as. number average), weight average.) 2358 */ 2359 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent setType(CodeableConcept value) { 2360 this.type = value; 2361 return this; 2362 } 2363 2364 /** 2365 * @return {@link #amount} (Used to capture quantitative values for a variety of 2366 * elements. If only limits are given, the arithmetic mean would be the 2367 * average. If only a single definite value for a given element is 2368 * given, it would be captured in this field.) 2369 */ 2370 public Quantity getAmount() { 2371 if (this.amount == null) 2372 if (Configuration.errorOnAutoCreate()) 2373 throw new Error( 2374 "Attempt to auto-create SubstanceSpecificationStructureIsotopeMolecularWeightComponent.amount"); 2375 else if (Configuration.doAutoCreate()) 2376 this.amount = new Quantity(); // cc 2377 return this.amount; 2378 } 2379 2380 public boolean hasAmount() { 2381 return this.amount != null && !this.amount.isEmpty(); 2382 } 2383 2384 /** 2385 * @param value {@link #amount} (Used to capture quantitative values for a 2386 * variety of elements. If only limits are given, the arithmetic 2387 * mean would be the average. If only a single definite value for a 2388 * given element is given, it would be captured in this field.) 2389 */ 2390 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent setAmount(Quantity value) { 2391 this.amount = value; 2392 return this; 2393 } 2394 2395 protected void listChildren(List<Property> children) { 2396 super.listChildren(children); 2397 children.add(new Property("method", "CodeableConcept", "The method by which the molecular weight was determined.", 2398 0, 1, method)); 2399 children.add(new Property("type", "CodeableConcept", 2400 "Type of molecular weight such as exact, average (also known as. number average), weight average.", 0, 1, 2401 type)); 2402 children.add(new Property("amount", "Quantity", 2403 "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 2404 0, 1, amount)); 2405 } 2406 2407 @Override 2408 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2409 switch (_hash) { 2410 case -1077554975: 2411 /* method */ return new Property("method", "CodeableConcept", 2412 "The method by which the molecular weight was determined.", 0, 1, method); 2413 case 3575610: 2414 /* type */ return new Property("type", "CodeableConcept", 2415 "Type of molecular weight such as exact, average (also known as. number average), weight average.", 0, 1, 2416 type); 2417 case -1413853096: 2418 /* amount */ return new Property("amount", "Quantity", 2419 "Used to capture quantitative values for a variety of elements. If only limits are given, the arithmetic mean would be the average. If only a single definite value for a given element is given, it would be captured in this field.", 2420 0, 1, amount); 2421 default: 2422 return super.getNamedProperty(_hash, _name, _checkValid); 2423 } 2424 2425 } 2426 2427 @Override 2428 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2429 switch (hash) { 2430 case -1077554975: 2431 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 2432 case 3575610: 2433 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2434 case -1413853096: 2435 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Quantity 2436 default: 2437 return super.getProperty(hash, name, checkValid); 2438 } 2439 2440 } 2441 2442 @Override 2443 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2444 switch (hash) { 2445 case -1077554975: // method 2446 this.method = castToCodeableConcept(value); // CodeableConcept 2447 return value; 2448 case 3575610: // type 2449 this.type = castToCodeableConcept(value); // CodeableConcept 2450 return value; 2451 case -1413853096: // amount 2452 this.amount = castToQuantity(value); // Quantity 2453 return value; 2454 default: 2455 return super.setProperty(hash, name, value); 2456 } 2457 2458 } 2459 2460 @Override 2461 public Base setProperty(String name, Base value) throws FHIRException { 2462 if (name.equals("method")) { 2463 this.method = castToCodeableConcept(value); // CodeableConcept 2464 } else if (name.equals("type")) { 2465 this.type = castToCodeableConcept(value); // CodeableConcept 2466 } else if (name.equals("amount")) { 2467 this.amount = castToQuantity(value); // Quantity 2468 } else 2469 return super.setProperty(name, value); 2470 return value; 2471 } 2472 2473 @Override 2474 public Base makeProperty(int hash, String name) throws FHIRException { 2475 switch (hash) { 2476 case -1077554975: 2477 return getMethod(); 2478 case 3575610: 2479 return getType(); 2480 case -1413853096: 2481 return getAmount(); 2482 default: 2483 return super.makeProperty(hash, name); 2484 } 2485 2486 } 2487 2488 @Override 2489 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2490 switch (hash) { 2491 case -1077554975: 2492 /* method */ return new String[] { "CodeableConcept" }; 2493 case 3575610: 2494 /* type */ return new String[] { "CodeableConcept" }; 2495 case -1413853096: 2496 /* amount */ return new String[] { "Quantity" }; 2497 default: 2498 return super.getTypesForProperty(hash, name); 2499 } 2500 2501 } 2502 2503 @Override 2504 public Base addChild(String name) throws FHIRException { 2505 if (name.equals("method")) { 2506 this.method = new CodeableConcept(); 2507 return this.method; 2508 } else if (name.equals("type")) { 2509 this.type = new CodeableConcept(); 2510 return this.type; 2511 } else if (name.equals("amount")) { 2512 this.amount = new Quantity(); 2513 return this.amount; 2514 } else 2515 return super.addChild(name); 2516 } 2517 2518 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent copy() { 2519 SubstanceSpecificationStructureIsotopeMolecularWeightComponent dst = new SubstanceSpecificationStructureIsotopeMolecularWeightComponent(); 2520 copyValues(dst); 2521 return dst; 2522 } 2523 2524 public void copyValues(SubstanceSpecificationStructureIsotopeMolecularWeightComponent dst) { 2525 super.copyValues(dst); 2526 dst.method = method == null ? null : method.copy(); 2527 dst.type = type == null ? null : type.copy(); 2528 dst.amount = amount == null ? null : amount.copy(); 2529 } 2530 2531 @Override 2532 public boolean equalsDeep(Base other_) { 2533 if (!super.equalsDeep(other_)) 2534 return false; 2535 if (!(other_ instanceof SubstanceSpecificationStructureIsotopeMolecularWeightComponent)) 2536 return false; 2537 SubstanceSpecificationStructureIsotopeMolecularWeightComponent o = (SubstanceSpecificationStructureIsotopeMolecularWeightComponent) other_; 2538 return compareDeep(method, o.method, true) && compareDeep(type, o.type, true) 2539 && compareDeep(amount, o.amount, true); 2540 } 2541 2542 @Override 2543 public boolean equalsShallow(Base other_) { 2544 if (!super.equalsShallow(other_)) 2545 return false; 2546 if (!(other_ instanceof SubstanceSpecificationStructureIsotopeMolecularWeightComponent)) 2547 return false; 2548 SubstanceSpecificationStructureIsotopeMolecularWeightComponent o = (SubstanceSpecificationStructureIsotopeMolecularWeightComponent) other_; 2549 return true; 2550 } 2551 2552 public boolean isEmpty() { 2553 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(method, type, amount); 2554 } 2555 2556 public String fhirType() { 2557 return "SubstanceSpecification.structure.isotope.molecularWeight"; 2558 2559 } 2560 2561 } 2562 2563 @Block() 2564 public static class SubstanceSpecificationStructureRepresentationComponent extends BackboneElement 2565 implements IBaseBackboneElement { 2566 /** 2567 * The type of structure (e.g. Full, Partial, Representative). 2568 */ 2569 @Child(name = "type", type = { 2570 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2571 @Description(shortDefinition = "The type of structure (e.g. Full, Partial, Representative)", formalDefinition = "The type of structure (e.g. Full, Partial, Representative).") 2572 protected CodeableConcept type; 2573 2574 /** 2575 * The structural representation as text string in a format e.g. InChI, SMILES, 2576 * MOLFILE, CDX. 2577 */ 2578 @Child(name = "representation", type = { 2579 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2580 @Description(shortDefinition = "The structural representation as text string in a format e.g. InChI, SMILES, MOLFILE, CDX", formalDefinition = "The structural representation as text string in a format e.g. InChI, SMILES, MOLFILE, CDX.") 2581 protected StringType representation; 2582 2583 /** 2584 * An attached file with the structural representation. 2585 */ 2586 @Child(name = "attachment", type = { 2587 Attachment.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2588 @Description(shortDefinition = "An attached file with the structural representation", formalDefinition = "An attached file with the structural representation.") 2589 protected Attachment attachment; 2590 2591 private static final long serialVersionUID = 167954495L; 2592 2593 /** 2594 * Constructor 2595 */ 2596 public SubstanceSpecificationStructureRepresentationComponent() { 2597 super(); 2598 } 2599 2600 /** 2601 * @return {@link #type} (The type of structure (e.g. Full, Partial, 2602 * Representative).) 2603 */ 2604 public CodeableConcept getType() { 2605 if (this.type == null) 2606 if (Configuration.errorOnAutoCreate()) 2607 throw new Error("Attempt to auto-create SubstanceSpecificationStructureRepresentationComponent.type"); 2608 else if (Configuration.doAutoCreate()) 2609 this.type = new CodeableConcept(); // cc 2610 return this.type; 2611 } 2612 2613 public boolean hasType() { 2614 return this.type != null && !this.type.isEmpty(); 2615 } 2616 2617 /** 2618 * @param value {@link #type} (The type of structure (e.g. Full, Partial, 2619 * Representative).) 2620 */ 2621 public SubstanceSpecificationStructureRepresentationComponent setType(CodeableConcept value) { 2622 this.type = value; 2623 return this; 2624 } 2625 2626 /** 2627 * @return {@link #representation} (The structural representation as text string 2628 * in a format e.g. InChI, SMILES, MOLFILE, CDX.). This is the 2629 * underlying object with id, value and extensions. The accessor 2630 * "getRepresentation" gives direct access to the value 2631 */ 2632 public StringType getRepresentationElement() { 2633 if (this.representation == null) 2634 if (Configuration.errorOnAutoCreate()) 2635 throw new Error( 2636 "Attempt to auto-create SubstanceSpecificationStructureRepresentationComponent.representation"); 2637 else if (Configuration.doAutoCreate()) 2638 this.representation = new StringType(); // bb 2639 return this.representation; 2640 } 2641 2642 public boolean hasRepresentationElement() { 2643 return this.representation != null && !this.representation.isEmpty(); 2644 } 2645 2646 public boolean hasRepresentation() { 2647 return this.representation != null && !this.representation.isEmpty(); 2648 } 2649 2650 /** 2651 * @param value {@link #representation} (The structural representation as text 2652 * string in a format e.g. InChI, SMILES, MOLFILE, CDX.). This is 2653 * the underlying object with id, value and extensions. The 2654 * accessor "getRepresentation" gives direct access to the value 2655 */ 2656 public SubstanceSpecificationStructureRepresentationComponent setRepresentationElement(StringType value) { 2657 this.representation = value; 2658 return this; 2659 } 2660 2661 /** 2662 * @return The structural representation as text string in a format e.g. InChI, 2663 * SMILES, MOLFILE, CDX. 2664 */ 2665 public String getRepresentation() { 2666 return this.representation == null ? null : this.representation.getValue(); 2667 } 2668 2669 /** 2670 * @param value The structural representation as text string in a format e.g. 2671 * InChI, SMILES, MOLFILE, CDX. 2672 */ 2673 public SubstanceSpecificationStructureRepresentationComponent setRepresentation(String value) { 2674 if (Utilities.noString(value)) 2675 this.representation = null; 2676 else { 2677 if (this.representation == null) 2678 this.representation = new StringType(); 2679 this.representation.setValue(value); 2680 } 2681 return this; 2682 } 2683 2684 /** 2685 * @return {@link #attachment} (An attached file with the structural 2686 * representation.) 2687 */ 2688 public Attachment getAttachment() { 2689 if (this.attachment == null) 2690 if (Configuration.errorOnAutoCreate()) 2691 throw new Error("Attempt to auto-create SubstanceSpecificationStructureRepresentationComponent.attachment"); 2692 else if (Configuration.doAutoCreate()) 2693 this.attachment = new Attachment(); // cc 2694 return this.attachment; 2695 } 2696 2697 public boolean hasAttachment() { 2698 return this.attachment != null && !this.attachment.isEmpty(); 2699 } 2700 2701 /** 2702 * @param value {@link #attachment} (An attached file with the structural 2703 * representation.) 2704 */ 2705 public SubstanceSpecificationStructureRepresentationComponent setAttachment(Attachment value) { 2706 this.attachment = value; 2707 return this; 2708 } 2709 2710 protected void listChildren(List<Property> children) { 2711 super.listChildren(children); 2712 children.add(new Property("type", "CodeableConcept", 2713 "The type of structure (e.g. Full, Partial, Representative).", 0, 1, type)); 2714 children.add(new Property("representation", "string", 2715 "The structural representation as text string in a format e.g. InChI, SMILES, MOLFILE, CDX.", 0, 1, 2716 representation)); 2717 children.add(new Property("attachment", "Attachment", "An attached file with the structural representation.", 0, 2718 1, attachment)); 2719 } 2720 2721 @Override 2722 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2723 switch (_hash) { 2724 case 3575610: 2725 /* type */ return new Property("type", "CodeableConcept", 2726 "The type of structure (e.g. Full, Partial, Representative).", 0, 1, type); 2727 case -671065907: 2728 /* representation */ return new Property("representation", "string", 2729 "The structural representation as text string in a format e.g. InChI, SMILES, MOLFILE, CDX.", 0, 1, 2730 representation); 2731 case -1963501277: 2732 /* attachment */ return new Property("attachment", "Attachment", 2733 "An attached file with the structural representation.", 0, 1, attachment); 2734 default: 2735 return super.getNamedProperty(_hash, _name, _checkValid); 2736 } 2737 2738 } 2739 2740 @Override 2741 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2742 switch (hash) { 2743 case 3575610: 2744 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2745 case -671065907: 2746 /* representation */ return this.representation == null ? new Base[0] : new Base[] { this.representation }; // StringType 2747 case -1963501277: 2748 /* attachment */ return this.attachment == null ? new Base[0] : new Base[] { this.attachment }; // Attachment 2749 default: 2750 return super.getProperty(hash, name, checkValid); 2751 } 2752 2753 } 2754 2755 @Override 2756 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2757 switch (hash) { 2758 case 3575610: // type 2759 this.type = castToCodeableConcept(value); // CodeableConcept 2760 return value; 2761 case -671065907: // representation 2762 this.representation = castToString(value); // StringType 2763 return value; 2764 case -1963501277: // attachment 2765 this.attachment = castToAttachment(value); // Attachment 2766 return value; 2767 default: 2768 return super.setProperty(hash, name, value); 2769 } 2770 2771 } 2772 2773 @Override 2774 public Base setProperty(String name, Base value) throws FHIRException { 2775 if (name.equals("type")) { 2776 this.type = castToCodeableConcept(value); // CodeableConcept 2777 } else if (name.equals("representation")) { 2778 this.representation = castToString(value); // StringType 2779 } else if (name.equals("attachment")) { 2780 this.attachment = castToAttachment(value); // Attachment 2781 } else 2782 return super.setProperty(name, value); 2783 return value; 2784 } 2785 2786 @Override 2787 public Base makeProperty(int hash, String name) throws FHIRException { 2788 switch (hash) { 2789 case 3575610: 2790 return getType(); 2791 case -671065907: 2792 return getRepresentationElement(); 2793 case -1963501277: 2794 return getAttachment(); 2795 default: 2796 return super.makeProperty(hash, name); 2797 } 2798 2799 } 2800 2801 @Override 2802 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2803 switch (hash) { 2804 case 3575610: 2805 /* type */ return new String[] { "CodeableConcept" }; 2806 case -671065907: 2807 /* representation */ return new String[] { "string" }; 2808 case -1963501277: 2809 /* attachment */ return new String[] { "Attachment" }; 2810 default: 2811 return super.getTypesForProperty(hash, name); 2812 } 2813 2814 } 2815 2816 @Override 2817 public Base addChild(String name) throws FHIRException { 2818 if (name.equals("type")) { 2819 this.type = new CodeableConcept(); 2820 return this.type; 2821 } else if (name.equals("representation")) { 2822 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.representation"); 2823 } else if (name.equals("attachment")) { 2824 this.attachment = new Attachment(); 2825 return this.attachment; 2826 } else 2827 return super.addChild(name); 2828 } 2829 2830 public SubstanceSpecificationStructureRepresentationComponent copy() { 2831 SubstanceSpecificationStructureRepresentationComponent dst = new SubstanceSpecificationStructureRepresentationComponent(); 2832 copyValues(dst); 2833 return dst; 2834 } 2835 2836 public void copyValues(SubstanceSpecificationStructureRepresentationComponent dst) { 2837 super.copyValues(dst); 2838 dst.type = type == null ? null : type.copy(); 2839 dst.representation = representation == null ? null : representation.copy(); 2840 dst.attachment = attachment == null ? null : attachment.copy(); 2841 } 2842 2843 @Override 2844 public boolean equalsDeep(Base other_) { 2845 if (!super.equalsDeep(other_)) 2846 return false; 2847 if (!(other_ instanceof SubstanceSpecificationStructureRepresentationComponent)) 2848 return false; 2849 SubstanceSpecificationStructureRepresentationComponent o = (SubstanceSpecificationStructureRepresentationComponent) other_; 2850 return compareDeep(type, o.type, true) && compareDeep(representation, o.representation, true) 2851 && compareDeep(attachment, o.attachment, true); 2852 } 2853 2854 @Override 2855 public boolean equalsShallow(Base other_) { 2856 if (!super.equalsShallow(other_)) 2857 return false; 2858 if (!(other_ instanceof SubstanceSpecificationStructureRepresentationComponent)) 2859 return false; 2860 SubstanceSpecificationStructureRepresentationComponent o = (SubstanceSpecificationStructureRepresentationComponent) other_; 2861 return compareValues(representation, o.representation, true); 2862 } 2863 2864 public boolean isEmpty() { 2865 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, representation, attachment); 2866 } 2867 2868 public String fhirType() { 2869 return "SubstanceSpecification.structure.representation"; 2870 2871 } 2872 2873 } 2874 2875 @Block() 2876 public static class SubstanceSpecificationCodeComponent extends BackboneElement implements IBaseBackboneElement { 2877 /** 2878 * The specific code. 2879 */ 2880 @Child(name = "code", type = { 2881 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2882 @Description(shortDefinition = "The specific code", formalDefinition = "The specific code.") 2883 protected CodeableConcept code; 2884 2885 /** 2886 * Status of the code assignment. 2887 */ 2888 @Child(name = "status", type = { 2889 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2890 @Description(shortDefinition = "Status of the code assignment", formalDefinition = "Status of the code assignment.") 2891 protected CodeableConcept status; 2892 2893 /** 2894 * The date at which the code status is changed as part of the terminology 2895 * maintenance. 2896 */ 2897 @Child(name = "statusDate", type = { 2898 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2899 @Description(shortDefinition = "The date at which the code status is changed as part of the terminology maintenance", formalDefinition = "The date at which the code status is changed as part of the terminology maintenance.") 2900 protected DateTimeType statusDate; 2901 2902 /** 2903 * Any comment can be provided in this field, if necessary. 2904 */ 2905 @Child(name = "comment", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2906 @Description(shortDefinition = "Any comment can be provided in this field, if necessary", formalDefinition = "Any comment can be provided in this field, if necessary.") 2907 protected StringType comment; 2908 2909 /** 2910 * Supporting literature. 2911 */ 2912 @Child(name = "source", type = { 2913 DocumentReference.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2914 @Description(shortDefinition = "Supporting literature", formalDefinition = "Supporting literature.") 2915 protected List<Reference> source; 2916 /** 2917 * The actual objects that are the target of the reference (Supporting 2918 * literature.) 2919 */ 2920 protected List<DocumentReference> sourceTarget; 2921 2922 private static final long serialVersionUID = -1629693460L; 2923 2924 /** 2925 * Constructor 2926 */ 2927 public SubstanceSpecificationCodeComponent() { 2928 super(); 2929 } 2930 2931 /** 2932 * @return {@link #code} (The specific code.) 2933 */ 2934 public CodeableConcept getCode() { 2935 if (this.code == null) 2936 if (Configuration.errorOnAutoCreate()) 2937 throw new Error("Attempt to auto-create SubstanceSpecificationCodeComponent.code"); 2938 else if (Configuration.doAutoCreate()) 2939 this.code = new CodeableConcept(); // cc 2940 return this.code; 2941 } 2942 2943 public boolean hasCode() { 2944 return this.code != null && !this.code.isEmpty(); 2945 } 2946 2947 /** 2948 * @param value {@link #code} (The specific code.) 2949 */ 2950 public SubstanceSpecificationCodeComponent setCode(CodeableConcept value) { 2951 this.code = value; 2952 return this; 2953 } 2954 2955 /** 2956 * @return {@link #status} (Status of the code assignment.) 2957 */ 2958 public CodeableConcept getStatus() { 2959 if (this.status == null) 2960 if (Configuration.errorOnAutoCreate()) 2961 throw new Error("Attempt to auto-create SubstanceSpecificationCodeComponent.status"); 2962 else if (Configuration.doAutoCreate()) 2963 this.status = new CodeableConcept(); // cc 2964 return this.status; 2965 } 2966 2967 public boolean hasStatus() { 2968 return this.status != null && !this.status.isEmpty(); 2969 } 2970 2971 /** 2972 * @param value {@link #status} (Status of the code assignment.) 2973 */ 2974 public SubstanceSpecificationCodeComponent setStatus(CodeableConcept value) { 2975 this.status = value; 2976 return this; 2977 } 2978 2979 /** 2980 * @return {@link #statusDate} (The date at which the code status is changed as 2981 * part of the terminology maintenance.). This is the underlying object 2982 * with id, value and extensions. The accessor "getStatusDate" gives 2983 * direct access to the value 2984 */ 2985 public DateTimeType getStatusDateElement() { 2986 if (this.statusDate == null) 2987 if (Configuration.errorOnAutoCreate()) 2988 throw new Error("Attempt to auto-create SubstanceSpecificationCodeComponent.statusDate"); 2989 else if (Configuration.doAutoCreate()) 2990 this.statusDate = new DateTimeType(); // bb 2991 return this.statusDate; 2992 } 2993 2994 public boolean hasStatusDateElement() { 2995 return this.statusDate != null && !this.statusDate.isEmpty(); 2996 } 2997 2998 public boolean hasStatusDate() { 2999 return this.statusDate != null && !this.statusDate.isEmpty(); 3000 } 3001 3002 /** 3003 * @param value {@link #statusDate} (The date at which the code status is 3004 * changed as part of the terminology maintenance.). This is the 3005 * underlying object with id, value and extensions. The accessor 3006 * "getStatusDate" gives direct access to the value 3007 */ 3008 public SubstanceSpecificationCodeComponent setStatusDateElement(DateTimeType value) { 3009 this.statusDate = value; 3010 return this; 3011 } 3012 3013 /** 3014 * @return The date at which the code status is changed as part of the 3015 * terminology maintenance. 3016 */ 3017 public Date getStatusDate() { 3018 return this.statusDate == null ? null : this.statusDate.getValue(); 3019 } 3020 3021 /** 3022 * @param value The date at which the code status is changed as part of the 3023 * terminology maintenance. 3024 */ 3025 public SubstanceSpecificationCodeComponent setStatusDate(Date value) { 3026 if (value == null) 3027 this.statusDate = null; 3028 else { 3029 if (this.statusDate == null) 3030 this.statusDate = new DateTimeType(); 3031 this.statusDate.setValue(value); 3032 } 3033 return this; 3034 } 3035 3036 /** 3037 * @return {@link #comment} (Any comment can be provided in this field, if 3038 * necessary.). This is the underlying object with id, value and 3039 * extensions. The accessor "getComment" gives direct access to the 3040 * value 3041 */ 3042 public StringType getCommentElement() { 3043 if (this.comment == null) 3044 if (Configuration.errorOnAutoCreate()) 3045 throw new Error("Attempt to auto-create SubstanceSpecificationCodeComponent.comment"); 3046 else if (Configuration.doAutoCreate()) 3047 this.comment = new StringType(); // bb 3048 return this.comment; 3049 } 3050 3051 public boolean hasCommentElement() { 3052 return this.comment != null && !this.comment.isEmpty(); 3053 } 3054 3055 public boolean hasComment() { 3056 return this.comment != null && !this.comment.isEmpty(); 3057 } 3058 3059 /** 3060 * @param value {@link #comment} (Any comment can be provided in this field, if 3061 * necessary.). This is the underlying object with id, value and 3062 * extensions. The accessor "getComment" gives direct access to the 3063 * value 3064 */ 3065 public SubstanceSpecificationCodeComponent setCommentElement(StringType value) { 3066 this.comment = value; 3067 return this; 3068 } 3069 3070 /** 3071 * @return Any comment can be provided in this field, if necessary. 3072 */ 3073 public String getComment() { 3074 return this.comment == null ? null : this.comment.getValue(); 3075 } 3076 3077 /** 3078 * @param value Any comment can be provided in this field, if necessary. 3079 */ 3080 public SubstanceSpecificationCodeComponent setComment(String value) { 3081 if (Utilities.noString(value)) 3082 this.comment = null; 3083 else { 3084 if (this.comment == null) 3085 this.comment = new StringType(); 3086 this.comment.setValue(value); 3087 } 3088 return this; 3089 } 3090 3091 /** 3092 * @return {@link #source} (Supporting literature.) 3093 */ 3094 public List<Reference> getSource() { 3095 if (this.source == null) 3096 this.source = new ArrayList<Reference>(); 3097 return this.source; 3098 } 3099 3100 /** 3101 * @return Returns a reference to <code>this</code> for easy method chaining 3102 */ 3103 public SubstanceSpecificationCodeComponent setSource(List<Reference> theSource) { 3104 this.source = theSource; 3105 return this; 3106 } 3107 3108 public boolean hasSource() { 3109 if (this.source == null) 3110 return false; 3111 for (Reference item : this.source) 3112 if (!item.isEmpty()) 3113 return true; 3114 return false; 3115 } 3116 3117 public Reference addSource() { // 3 3118 Reference t = new Reference(); 3119 if (this.source == null) 3120 this.source = new ArrayList<Reference>(); 3121 this.source.add(t); 3122 return t; 3123 } 3124 3125 public SubstanceSpecificationCodeComponent addSource(Reference t) { // 3 3126 if (t == null) 3127 return this; 3128 if (this.source == null) 3129 this.source = new ArrayList<Reference>(); 3130 this.source.add(t); 3131 return this; 3132 } 3133 3134 /** 3135 * @return The first repetition of repeating field {@link #source}, creating it 3136 * if it does not already exist 3137 */ 3138 public Reference getSourceFirstRep() { 3139 if (getSource().isEmpty()) { 3140 addSource(); 3141 } 3142 return getSource().get(0); 3143 } 3144 3145 /** 3146 * @deprecated Use Reference#setResource(IBaseResource) instead 3147 */ 3148 @Deprecated 3149 public List<DocumentReference> getSourceTarget() { 3150 if (this.sourceTarget == null) 3151 this.sourceTarget = new ArrayList<DocumentReference>(); 3152 return this.sourceTarget; 3153 } 3154 3155 /** 3156 * @deprecated Use Reference#setResource(IBaseResource) instead 3157 */ 3158 @Deprecated 3159 public DocumentReference addSourceTarget() { 3160 DocumentReference r = new DocumentReference(); 3161 if (this.sourceTarget == null) 3162 this.sourceTarget = new ArrayList<DocumentReference>(); 3163 this.sourceTarget.add(r); 3164 return r; 3165 } 3166 3167 protected void listChildren(List<Property> children) { 3168 super.listChildren(children); 3169 children.add(new Property("code", "CodeableConcept", "The specific code.", 0, 1, code)); 3170 children.add(new Property("status", "CodeableConcept", "Status of the code assignment.", 0, 1, status)); 3171 children.add(new Property("statusDate", "dateTime", 3172 "The date at which the code status is changed as part of the terminology maintenance.", 0, 1, statusDate)); 3173 children.add( 3174 new Property("comment", "string", "Any comment can be provided in this field, if necessary.", 0, 1, comment)); 3175 children.add(new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 3176 java.lang.Integer.MAX_VALUE, source)); 3177 } 3178 3179 @Override 3180 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3181 switch (_hash) { 3182 case 3059181: 3183 /* code */ return new Property("code", "CodeableConcept", "The specific code.", 0, 1, code); 3184 case -892481550: 3185 /* status */ return new Property("status", "CodeableConcept", "Status of the code assignment.", 0, 1, status); 3186 case 247524032: 3187 /* statusDate */ return new Property("statusDate", "dateTime", 3188 "The date at which the code status is changed as part of the terminology maintenance.", 0, 1, statusDate); 3189 case 950398559: 3190 /* comment */ return new Property("comment", "string", 3191 "Any comment can be provided in this field, if necessary.", 0, 1, comment); 3192 case -896505829: 3193 /* source */ return new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 3194 java.lang.Integer.MAX_VALUE, source); 3195 default: 3196 return super.getNamedProperty(_hash, _name, _checkValid); 3197 } 3198 3199 } 3200 3201 @Override 3202 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3203 switch (hash) { 3204 case 3059181: 3205 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 3206 case -892481550: 3207 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 3208 case 247524032: 3209 /* statusDate */ return this.statusDate == null ? new Base[0] : new Base[] { this.statusDate }; // DateTimeType 3210 case 950398559: 3211 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 3212 case -896505829: 3213 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 3214 default: 3215 return super.getProperty(hash, name, checkValid); 3216 } 3217 3218 } 3219 3220 @Override 3221 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3222 switch (hash) { 3223 case 3059181: // code 3224 this.code = castToCodeableConcept(value); // CodeableConcept 3225 return value; 3226 case -892481550: // status 3227 this.status = castToCodeableConcept(value); // CodeableConcept 3228 return value; 3229 case 247524032: // statusDate 3230 this.statusDate = castToDateTime(value); // DateTimeType 3231 return value; 3232 case 950398559: // comment 3233 this.comment = castToString(value); // StringType 3234 return value; 3235 case -896505829: // source 3236 this.getSource().add(castToReference(value)); // Reference 3237 return value; 3238 default: 3239 return super.setProperty(hash, name, value); 3240 } 3241 3242 } 3243 3244 @Override 3245 public Base setProperty(String name, Base value) throws FHIRException { 3246 if (name.equals("code")) { 3247 this.code = castToCodeableConcept(value); // CodeableConcept 3248 } else if (name.equals("status")) { 3249 this.status = castToCodeableConcept(value); // CodeableConcept 3250 } else if (name.equals("statusDate")) { 3251 this.statusDate = castToDateTime(value); // DateTimeType 3252 } else if (name.equals("comment")) { 3253 this.comment = castToString(value); // StringType 3254 } else if (name.equals("source")) { 3255 this.getSource().add(castToReference(value)); 3256 } else 3257 return super.setProperty(name, value); 3258 return value; 3259 } 3260 3261 @Override 3262 public Base makeProperty(int hash, String name) throws FHIRException { 3263 switch (hash) { 3264 case 3059181: 3265 return getCode(); 3266 case -892481550: 3267 return getStatus(); 3268 case 247524032: 3269 return getStatusDateElement(); 3270 case 950398559: 3271 return getCommentElement(); 3272 case -896505829: 3273 return addSource(); 3274 default: 3275 return super.makeProperty(hash, name); 3276 } 3277 3278 } 3279 3280 @Override 3281 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3282 switch (hash) { 3283 case 3059181: 3284 /* code */ return new String[] { "CodeableConcept" }; 3285 case -892481550: 3286 /* status */ return new String[] { "CodeableConcept" }; 3287 case 247524032: 3288 /* statusDate */ return new String[] { "dateTime" }; 3289 case 950398559: 3290 /* comment */ return new String[] { "string" }; 3291 case -896505829: 3292 /* source */ return new String[] { "Reference" }; 3293 default: 3294 return super.getTypesForProperty(hash, name); 3295 } 3296 3297 } 3298 3299 @Override 3300 public Base addChild(String name) throws FHIRException { 3301 if (name.equals("code")) { 3302 this.code = new CodeableConcept(); 3303 return this.code; 3304 } else if (name.equals("status")) { 3305 this.status = new CodeableConcept(); 3306 return this.status; 3307 } else if (name.equals("statusDate")) { 3308 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.statusDate"); 3309 } else if (name.equals("comment")) { 3310 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.comment"); 3311 } else if (name.equals("source")) { 3312 return addSource(); 3313 } else 3314 return super.addChild(name); 3315 } 3316 3317 public SubstanceSpecificationCodeComponent copy() { 3318 SubstanceSpecificationCodeComponent dst = new SubstanceSpecificationCodeComponent(); 3319 copyValues(dst); 3320 return dst; 3321 } 3322 3323 public void copyValues(SubstanceSpecificationCodeComponent dst) { 3324 super.copyValues(dst); 3325 dst.code = code == null ? null : code.copy(); 3326 dst.status = status == null ? null : status.copy(); 3327 dst.statusDate = statusDate == null ? null : statusDate.copy(); 3328 dst.comment = comment == null ? null : comment.copy(); 3329 if (source != null) { 3330 dst.source = new ArrayList<Reference>(); 3331 for (Reference i : source) 3332 dst.source.add(i.copy()); 3333 } 3334 ; 3335 } 3336 3337 @Override 3338 public boolean equalsDeep(Base other_) { 3339 if (!super.equalsDeep(other_)) 3340 return false; 3341 if (!(other_ instanceof SubstanceSpecificationCodeComponent)) 3342 return false; 3343 SubstanceSpecificationCodeComponent o = (SubstanceSpecificationCodeComponent) other_; 3344 return compareDeep(code, o.code, true) && compareDeep(status, o.status, true) 3345 && compareDeep(statusDate, o.statusDate, true) && compareDeep(comment, o.comment, true) 3346 && compareDeep(source, o.source, true); 3347 } 3348 3349 @Override 3350 public boolean equalsShallow(Base other_) { 3351 if (!super.equalsShallow(other_)) 3352 return false; 3353 if (!(other_ instanceof SubstanceSpecificationCodeComponent)) 3354 return false; 3355 SubstanceSpecificationCodeComponent o = (SubstanceSpecificationCodeComponent) other_; 3356 return compareValues(statusDate, o.statusDate, true) && compareValues(comment, o.comment, true); 3357 } 3358 3359 public boolean isEmpty() { 3360 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, status, statusDate, comment, source); 3361 } 3362 3363 public String fhirType() { 3364 return "SubstanceSpecification.code"; 3365 3366 } 3367 3368 } 3369 3370 @Block() 3371 public static class SubstanceSpecificationNameComponent extends BackboneElement implements IBaseBackboneElement { 3372 /** 3373 * The actual name. 3374 */ 3375 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3376 @Description(shortDefinition = "The actual name", formalDefinition = "The actual name.") 3377 protected StringType name; 3378 3379 /** 3380 * Name type. 3381 */ 3382 @Child(name = "type", type = { 3383 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3384 @Description(shortDefinition = "Name type", formalDefinition = "Name type.") 3385 protected CodeableConcept type; 3386 3387 /** 3388 * The status of the name. 3389 */ 3390 @Child(name = "status", type = { 3391 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3392 @Description(shortDefinition = "The status of the name", formalDefinition = "The status of the name.") 3393 protected CodeableConcept status; 3394 3395 /** 3396 * If this is the preferred name for this substance. 3397 */ 3398 @Child(name = "preferred", type = { 3399 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 3400 @Description(shortDefinition = "If this is the preferred name for this substance", formalDefinition = "If this is the preferred name for this substance.") 3401 protected BooleanType preferred; 3402 3403 /** 3404 * Language of the name. 3405 */ 3406 @Child(name = "language", type = { 3407 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3408 @Description(shortDefinition = "Language of the name", formalDefinition = "Language of the name.") 3409 protected List<CodeableConcept> language; 3410 3411 /** 3412 * The use context of this name for example if there is a different name a drug 3413 * active ingredient as opposed to a food colour additive. 3414 */ 3415 @Child(name = "domain", type = { 3416 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3417 @Description(shortDefinition = "The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive", formalDefinition = "The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive.") 3418 protected List<CodeableConcept> domain; 3419 3420 /** 3421 * The jurisdiction where this name applies. 3422 */ 3423 @Child(name = "jurisdiction", type = { 3424 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3425 @Description(shortDefinition = "The jurisdiction where this name applies", formalDefinition = "The jurisdiction where this name applies.") 3426 protected List<CodeableConcept> jurisdiction; 3427 3428 /** 3429 * A synonym of this name. 3430 */ 3431 @Child(name = "synonym", type = { 3432 SubstanceSpecificationNameComponent.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3433 @Description(shortDefinition = "A synonym of this name", formalDefinition = "A synonym of this name.") 3434 protected List<SubstanceSpecificationNameComponent> synonym; 3435 3436 /** 3437 * A translation for this name. 3438 */ 3439 @Child(name = "translation", type = { 3440 SubstanceSpecificationNameComponent.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3441 @Description(shortDefinition = "A translation for this name", formalDefinition = "A translation for this name.") 3442 protected List<SubstanceSpecificationNameComponent> translation; 3443 3444 /** 3445 * Details of the official nature of this name. 3446 */ 3447 @Child(name = "official", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3448 @Description(shortDefinition = "Details of the official nature of this name", formalDefinition = "Details of the official nature of this name.") 3449 protected List<SubstanceSpecificationNameOfficialComponent> official; 3450 3451 /** 3452 * Supporting literature. 3453 */ 3454 @Child(name = "source", type = { 3455 DocumentReference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3456 @Description(shortDefinition = "Supporting literature", formalDefinition = "Supporting literature.") 3457 protected List<Reference> source; 3458 /** 3459 * The actual objects that are the target of the reference (Supporting 3460 * literature.) 3461 */ 3462 protected List<DocumentReference> sourceTarget; 3463 3464 private static final long serialVersionUID = 1547107852L; 3465 3466 /** 3467 * Constructor 3468 */ 3469 public SubstanceSpecificationNameComponent() { 3470 super(); 3471 } 3472 3473 /** 3474 * Constructor 3475 */ 3476 public SubstanceSpecificationNameComponent(StringType name) { 3477 super(); 3478 this.name = name; 3479 } 3480 3481 /** 3482 * @return {@link #name} (The actual name.). This is the underlying object with 3483 * id, value and extensions. The accessor "getName" gives direct access 3484 * to the value 3485 */ 3486 public StringType getNameElement() { 3487 if (this.name == null) 3488 if (Configuration.errorOnAutoCreate()) 3489 throw new Error("Attempt to auto-create SubstanceSpecificationNameComponent.name"); 3490 else if (Configuration.doAutoCreate()) 3491 this.name = new StringType(); // bb 3492 return this.name; 3493 } 3494 3495 public boolean hasNameElement() { 3496 return this.name != null && !this.name.isEmpty(); 3497 } 3498 3499 public boolean hasName() { 3500 return this.name != null && !this.name.isEmpty(); 3501 } 3502 3503 /** 3504 * @param value {@link #name} (The actual name.). This is the underlying object 3505 * with id, value and extensions. The accessor "getName" gives 3506 * direct access to the value 3507 */ 3508 public SubstanceSpecificationNameComponent setNameElement(StringType value) { 3509 this.name = value; 3510 return this; 3511 } 3512 3513 /** 3514 * @return The actual name. 3515 */ 3516 public String getName() { 3517 return this.name == null ? null : this.name.getValue(); 3518 } 3519 3520 /** 3521 * @param value The actual name. 3522 */ 3523 public SubstanceSpecificationNameComponent setName(String value) { 3524 if (this.name == null) 3525 this.name = new StringType(); 3526 this.name.setValue(value); 3527 return this; 3528 } 3529 3530 /** 3531 * @return {@link #type} (Name type.) 3532 */ 3533 public CodeableConcept getType() { 3534 if (this.type == null) 3535 if (Configuration.errorOnAutoCreate()) 3536 throw new Error("Attempt to auto-create SubstanceSpecificationNameComponent.type"); 3537 else if (Configuration.doAutoCreate()) 3538 this.type = new CodeableConcept(); // cc 3539 return this.type; 3540 } 3541 3542 public boolean hasType() { 3543 return this.type != null && !this.type.isEmpty(); 3544 } 3545 3546 /** 3547 * @param value {@link #type} (Name type.) 3548 */ 3549 public SubstanceSpecificationNameComponent setType(CodeableConcept value) { 3550 this.type = value; 3551 return this; 3552 } 3553 3554 /** 3555 * @return {@link #status} (The status of the name.) 3556 */ 3557 public CodeableConcept getStatus() { 3558 if (this.status == null) 3559 if (Configuration.errorOnAutoCreate()) 3560 throw new Error("Attempt to auto-create SubstanceSpecificationNameComponent.status"); 3561 else if (Configuration.doAutoCreate()) 3562 this.status = new CodeableConcept(); // cc 3563 return this.status; 3564 } 3565 3566 public boolean hasStatus() { 3567 return this.status != null && !this.status.isEmpty(); 3568 } 3569 3570 /** 3571 * @param value {@link #status} (The status of the name.) 3572 */ 3573 public SubstanceSpecificationNameComponent setStatus(CodeableConcept value) { 3574 this.status = value; 3575 return this; 3576 } 3577 3578 /** 3579 * @return {@link #preferred} (If this is the preferred name for this 3580 * substance.). This is the underlying object with id, value and 3581 * extensions. The accessor "getPreferred" gives direct access to the 3582 * value 3583 */ 3584 public BooleanType getPreferredElement() { 3585 if (this.preferred == null) 3586 if (Configuration.errorOnAutoCreate()) 3587 throw new Error("Attempt to auto-create SubstanceSpecificationNameComponent.preferred"); 3588 else if (Configuration.doAutoCreate()) 3589 this.preferred = new BooleanType(); // bb 3590 return this.preferred; 3591 } 3592 3593 public boolean hasPreferredElement() { 3594 return this.preferred != null && !this.preferred.isEmpty(); 3595 } 3596 3597 public boolean hasPreferred() { 3598 return this.preferred != null && !this.preferred.isEmpty(); 3599 } 3600 3601 /** 3602 * @param value {@link #preferred} (If this is the preferred name for this 3603 * substance.). This is the underlying object with id, value and 3604 * extensions. The accessor "getPreferred" gives direct access to 3605 * the value 3606 */ 3607 public SubstanceSpecificationNameComponent setPreferredElement(BooleanType value) { 3608 this.preferred = value; 3609 return this; 3610 } 3611 3612 /** 3613 * @return If this is the preferred name for this substance. 3614 */ 3615 public boolean getPreferred() { 3616 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 3617 } 3618 3619 /** 3620 * @param value If this is the preferred name for this substance. 3621 */ 3622 public SubstanceSpecificationNameComponent setPreferred(boolean value) { 3623 if (this.preferred == null) 3624 this.preferred = new BooleanType(); 3625 this.preferred.setValue(value); 3626 return this; 3627 } 3628 3629 /** 3630 * @return {@link #language} (Language of the name.) 3631 */ 3632 public List<CodeableConcept> getLanguage() { 3633 if (this.language == null) 3634 this.language = new ArrayList<CodeableConcept>(); 3635 return this.language; 3636 } 3637 3638 /** 3639 * @return Returns a reference to <code>this</code> for easy method chaining 3640 */ 3641 public SubstanceSpecificationNameComponent setLanguage(List<CodeableConcept> theLanguage) { 3642 this.language = theLanguage; 3643 return this; 3644 } 3645 3646 public boolean hasLanguage() { 3647 if (this.language == null) 3648 return false; 3649 for (CodeableConcept item : this.language) 3650 if (!item.isEmpty()) 3651 return true; 3652 return false; 3653 } 3654 3655 public CodeableConcept addLanguage() { // 3 3656 CodeableConcept t = new CodeableConcept(); 3657 if (this.language == null) 3658 this.language = new ArrayList<CodeableConcept>(); 3659 this.language.add(t); 3660 return t; 3661 } 3662 3663 public SubstanceSpecificationNameComponent addLanguage(CodeableConcept t) { // 3 3664 if (t == null) 3665 return this; 3666 if (this.language == null) 3667 this.language = new ArrayList<CodeableConcept>(); 3668 this.language.add(t); 3669 return this; 3670 } 3671 3672 /** 3673 * @return The first repetition of repeating field {@link #language}, creating 3674 * it if it does not already exist 3675 */ 3676 public CodeableConcept getLanguageFirstRep() { 3677 if (getLanguage().isEmpty()) { 3678 addLanguage(); 3679 } 3680 return getLanguage().get(0); 3681 } 3682 3683 /** 3684 * @return {@link #domain} (The use context of this name for example if there is 3685 * a different name a drug active ingredient as opposed to a food colour 3686 * additive.) 3687 */ 3688 public List<CodeableConcept> getDomain() { 3689 if (this.domain == null) 3690 this.domain = new ArrayList<CodeableConcept>(); 3691 return this.domain; 3692 } 3693 3694 /** 3695 * @return Returns a reference to <code>this</code> for easy method chaining 3696 */ 3697 public SubstanceSpecificationNameComponent setDomain(List<CodeableConcept> theDomain) { 3698 this.domain = theDomain; 3699 return this; 3700 } 3701 3702 public boolean hasDomain() { 3703 if (this.domain == null) 3704 return false; 3705 for (CodeableConcept item : this.domain) 3706 if (!item.isEmpty()) 3707 return true; 3708 return false; 3709 } 3710 3711 public CodeableConcept addDomain() { // 3 3712 CodeableConcept t = new CodeableConcept(); 3713 if (this.domain == null) 3714 this.domain = new ArrayList<CodeableConcept>(); 3715 this.domain.add(t); 3716 return t; 3717 } 3718 3719 public SubstanceSpecificationNameComponent addDomain(CodeableConcept t) { // 3 3720 if (t == null) 3721 return this; 3722 if (this.domain == null) 3723 this.domain = new ArrayList<CodeableConcept>(); 3724 this.domain.add(t); 3725 return this; 3726 } 3727 3728 /** 3729 * @return The first repetition of repeating field {@link #domain}, creating it 3730 * if it does not already exist 3731 */ 3732 public CodeableConcept getDomainFirstRep() { 3733 if (getDomain().isEmpty()) { 3734 addDomain(); 3735 } 3736 return getDomain().get(0); 3737 } 3738 3739 /** 3740 * @return {@link #jurisdiction} (The jurisdiction where this name applies.) 3741 */ 3742 public List<CodeableConcept> getJurisdiction() { 3743 if (this.jurisdiction == null) 3744 this.jurisdiction = new ArrayList<CodeableConcept>(); 3745 return this.jurisdiction; 3746 } 3747 3748 /** 3749 * @return Returns a reference to <code>this</code> for easy method chaining 3750 */ 3751 public SubstanceSpecificationNameComponent setJurisdiction(List<CodeableConcept> theJurisdiction) { 3752 this.jurisdiction = theJurisdiction; 3753 return this; 3754 } 3755 3756 public boolean hasJurisdiction() { 3757 if (this.jurisdiction == null) 3758 return false; 3759 for (CodeableConcept item : this.jurisdiction) 3760 if (!item.isEmpty()) 3761 return true; 3762 return false; 3763 } 3764 3765 public CodeableConcept addJurisdiction() { // 3 3766 CodeableConcept t = new CodeableConcept(); 3767 if (this.jurisdiction == null) 3768 this.jurisdiction = new ArrayList<CodeableConcept>(); 3769 this.jurisdiction.add(t); 3770 return t; 3771 } 3772 3773 public SubstanceSpecificationNameComponent addJurisdiction(CodeableConcept t) { // 3 3774 if (t == null) 3775 return this; 3776 if (this.jurisdiction == null) 3777 this.jurisdiction = new ArrayList<CodeableConcept>(); 3778 this.jurisdiction.add(t); 3779 return this; 3780 } 3781 3782 /** 3783 * @return The first repetition of repeating field {@link #jurisdiction}, 3784 * creating it if it does not already exist 3785 */ 3786 public CodeableConcept getJurisdictionFirstRep() { 3787 if (getJurisdiction().isEmpty()) { 3788 addJurisdiction(); 3789 } 3790 return getJurisdiction().get(0); 3791 } 3792 3793 /** 3794 * @return {@link #synonym} (A synonym of this name.) 3795 */ 3796 public List<SubstanceSpecificationNameComponent> getSynonym() { 3797 if (this.synonym == null) 3798 this.synonym = new ArrayList<SubstanceSpecificationNameComponent>(); 3799 return this.synonym; 3800 } 3801 3802 /** 3803 * @return Returns a reference to <code>this</code> for easy method chaining 3804 */ 3805 public SubstanceSpecificationNameComponent setSynonym(List<SubstanceSpecificationNameComponent> theSynonym) { 3806 this.synonym = theSynonym; 3807 return this; 3808 } 3809 3810 public boolean hasSynonym() { 3811 if (this.synonym == null) 3812 return false; 3813 for (SubstanceSpecificationNameComponent item : this.synonym) 3814 if (!item.isEmpty()) 3815 return true; 3816 return false; 3817 } 3818 3819 public SubstanceSpecificationNameComponent addSynonym() { // 3 3820 SubstanceSpecificationNameComponent t = new SubstanceSpecificationNameComponent(); 3821 if (this.synonym == null) 3822 this.synonym = new ArrayList<SubstanceSpecificationNameComponent>(); 3823 this.synonym.add(t); 3824 return t; 3825 } 3826 3827 public SubstanceSpecificationNameComponent addSynonym(SubstanceSpecificationNameComponent t) { // 3 3828 if (t == null) 3829 return this; 3830 if (this.synonym == null) 3831 this.synonym = new ArrayList<SubstanceSpecificationNameComponent>(); 3832 this.synonym.add(t); 3833 return this; 3834 } 3835 3836 /** 3837 * @return The first repetition of repeating field {@link #synonym}, creating it 3838 * if it does not already exist 3839 */ 3840 public SubstanceSpecificationNameComponent getSynonymFirstRep() { 3841 if (getSynonym().isEmpty()) { 3842 addSynonym(); 3843 } 3844 return getSynonym().get(0); 3845 } 3846 3847 /** 3848 * @return {@link #translation} (A translation for this name.) 3849 */ 3850 public List<SubstanceSpecificationNameComponent> getTranslation() { 3851 if (this.translation == null) 3852 this.translation = new ArrayList<SubstanceSpecificationNameComponent>(); 3853 return this.translation; 3854 } 3855 3856 /** 3857 * @return Returns a reference to <code>this</code> for easy method chaining 3858 */ 3859 public SubstanceSpecificationNameComponent setTranslation( 3860 List<SubstanceSpecificationNameComponent> theTranslation) { 3861 this.translation = theTranslation; 3862 return this; 3863 } 3864 3865 public boolean hasTranslation() { 3866 if (this.translation == null) 3867 return false; 3868 for (SubstanceSpecificationNameComponent item : this.translation) 3869 if (!item.isEmpty()) 3870 return true; 3871 return false; 3872 } 3873 3874 public SubstanceSpecificationNameComponent addTranslation() { // 3 3875 SubstanceSpecificationNameComponent t = new SubstanceSpecificationNameComponent(); 3876 if (this.translation == null) 3877 this.translation = new ArrayList<SubstanceSpecificationNameComponent>(); 3878 this.translation.add(t); 3879 return t; 3880 } 3881 3882 public SubstanceSpecificationNameComponent addTranslation(SubstanceSpecificationNameComponent t) { // 3 3883 if (t == null) 3884 return this; 3885 if (this.translation == null) 3886 this.translation = new ArrayList<SubstanceSpecificationNameComponent>(); 3887 this.translation.add(t); 3888 return this; 3889 } 3890 3891 /** 3892 * @return The first repetition of repeating field {@link #translation}, 3893 * creating it if it does not already exist 3894 */ 3895 public SubstanceSpecificationNameComponent getTranslationFirstRep() { 3896 if (getTranslation().isEmpty()) { 3897 addTranslation(); 3898 } 3899 return getTranslation().get(0); 3900 } 3901 3902 /** 3903 * @return {@link #official} (Details of the official nature of this name.) 3904 */ 3905 public List<SubstanceSpecificationNameOfficialComponent> getOfficial() { 3906 if (this.official == null) 3907 this.official = new ArrayList<SubstanceSpecificationNameOfficialComponent>(); 3908 return this.official; 3909 } 3910 3911 /** 3912 * @return Returns a reference to <code>this</code> for easy method chaining 3913 */ 3914 public SubstanceSpecificationNameComponent setOfficial( 3915 List<SubstanceSpecificationNameOfficialComponent> theOfficial) { 3916 this.official = theOfficial; 3917 return this; 3918 } 3919 3920 public boolean hasOfficial() { 3921 if (this.official == null) 3922 return false; 3923 for (SubstanceSpecificationNameOfficialComponent item : this.official) 3924 if (!item.isEmpty()) 3925 return true; 3926 return false; 3927 } 3928 3929 public SubstanceSpecificationNameOfficialComponent addOfficial() { // 3 3930 SubstanceSpecificationNameOfficialComponent t = new SubstanceSpecificationNameOfficialComponent(); 3931 if (this.official == null) 3932 this.official = new ArrayList<SubstanceSpecificationNameOfficialComponent>(); 3933 this.official.add(t); 3934 return t; 3935 } 3936 3937 public SubstanceSpecificationNameComponent addOfficial(SubstanceSpecificationNameOfficialComponent t) { // 3 3938 if (t == null) 3939 return this; 3940 if (this.official == null) 3941 this.official = new ArrayList<SubstanceSpecificationNameOfficialComponent>(); 3942 this.official.add(t); 3943 return this; 3944 } 3945 3946 /** 3947 * @return The first repetition of repeating field {@link #official}, creating 3948 * it if it does not already exist 3949 */ 3950 public SubstanceSpecificationNameOfficialComponent getOfficialFirstRep() { 3951 if (getOfficial().isEmpty()) { 3952 addOfficial(); 3953 } 3954 return getOfficial().get(0); 3955 } 3956 3957 /** 3958 * @return {@link #source} (Supporting literature.) 3959 */ 3960 public List<Reference> getSource() { 3961 if (this.source == null) 3962 this.source = new ArrayList<Reference>(); 3963 return this.source; 3964 } 3965 3966 /** 3967 * @return Returns a reference to <code>this</code> for easy method chaining 3968 */ 3969 public SubstanceSpecificationNameComponent setSource(List<Reference> theSource) { 3970 this.source = theSource; 3971 return this; 3972 } 3973 3974 public boolean hasSource() { 3975 if (this.source == null) 3976 return false; 3977 for (Reference item : this.source) 3978 if (!item.isEmpty()) 3979 return true; 3980 return false; 3981 } 3982 3983 public Reference addSource() { // 3 3984 Reference t = new Reference(); 3985 if (this.source == null) 3986 this.source = new ArrayList<Reference>(); 3987 this.source.add(t); 3988 return t; 3989 } 3990 3991 public SubstanceSpecificationNameComponent addSource(Reference t) { // 3 3992 if (t == null) 3993 return this; 3994 if (this.source == null) 3995 this.source = new ArrayList<Reference>(); 3996 this.source.add(t); 3997 return this; 3998 } 3999 4000 /** 4001 * @return The first repetition of repeating field {@link #source}, creating it 4002 * if it does not already exist 4003 */ 4004 public Reference getSourceFirstRep() { 4005 if (getSource().isEmpty()) { 4006 addSource(); 4007 } 4008 return getSource().get(0); 4009 } 4010 4011 /** 4012 * @deprecated Use Reference#setResource(IBaseResource) instead 4013 */ 4014 @Deprecated 4015 public List<DocumentReference> getSourceTarget() { 4016 if (this.sourceTarget == null) 4017 this.sourceTarget = new ArrayList<DocumentReference>(); 4018 return this.sourceTarget; 4019 } 4020 4021 /** 4022 * @deprecated Use Reference#setResource(IBaseResource) instead 4023 */ 4024 @Deprecated 4025 public DocumentReference addSourceTarget() { 4026 DocumentReference r = new DocumentReference(); 4027 if (this.sourceTarget == null) 4028 this.sourceTarget = new ArrayList<DocumentReference>(); 4029 this.sourceTarget.add(r); 4030 return r; 4031 } 4032 4033 protected void listChildren(List<Property> children) { 4034 super.listChildren(children); 4035 children.add(new Property("name", "string", "The actual name.", 0, 1, name)); 4036 children.add(new Property("type", "CodeableConcept", "Name type.", 0, 1, type)); 4037 children.add(new Property("status", "CodeableConcept", "The status of the name.", 0, 1, status)); 4038 children.add( 4039 new Property("preferred", "boolean", "If this is the preferred name for this substance.", 0, 1, preferred)); 4040 children.add(new Property("language", "CodeableConcept", "Language of the name.", 0, java.lang.Integer.MAX_VALUE, 4041 language)); 4042 children.add(new Property("domain", "CodeableConcept", 4043 "The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive.", 4044 0, java.lang.Integer.MAX_VALUE, domain)); 4045 children.add(new Property("jurisdiction", "CodeableConcept", "The jurisdiction where this name applies.", 0, 4046 java.lang.Integer.MAX_VALUE, jurisdiction)); 4047 children.add(new Property("synonym", "@SubstanceSpecification.name", "A synonym of this name.", 0, 4048 java.lang.Integer.MAX_VALUE, synonym)); 4049 children.add(new Property("translation", "@SubstanceSpecification.name", "A translation for this name.", 0, 4050 java.lang.Integer.MAX_VALUE, translation)); 4051 children.add(new Property("official", "", "Details of the official nature of this name.", 0, 4052 java.lang.Integer.MAX_VALUE, official)); 4053 children.add(new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 4054 java.lang.Integer.MAX_VALUE, source)); 4055 } 4056 4057 @Override 4058 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4059 switch (_hash) { 4060 case 3373707: 4061 /* name */ return new Property("name", "string", "The actual name.", 0, 1, name); 4062 case 3575610: 4063 /* type */ return new Property("type", "CodeableConcept", "Name type.", 0, 1, type); 4064 case -892481550: 4065 /* status */ return new Property("status", "CodeableConcept", "The status of the name.", 0, 1, status); 4066 case -1294005119: 4067 /* preferred */ return new Property("preferred", "boolean", "If this is the preferred name for this substance.", 4068 0, 1, preferred); 4069 case -1613589672: 4070 /* language */ return new Property("language", "CodeableConcept", "Language of the name.", 0, 4071 java.lang.Integer.MAX_VALUE, language); 4072 case -1326197564: 4073 /* domain */ return new Property("domain", "CodeableConcept", 4074 "The use context of this name for example if there is a different name a drug active ingredient as opposed to a food colour additive.", 4075 0, java.lang.Integer.MAX_VALUE, domain); 4076 case -507075711: 4077 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4078 "The jurisdiction where this name applies.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 4079 case -1742128133: 4080 /* synonym */ return new Property("synonym", "@SubstanceSpecification.name", "A synonym of this name.", 0, 4081 java.lang.Integer.MAX_VALUE, synonym); 4082 case -1840647503: 4083 /* translation */ return new Property("translation", "@SubstanceSpecification.name", 4084 "A translation for this name.", 0, java.lang.Integer.MAX_VALUE, translation); 4085 case -765289749: 4086 /* official */ return new Property("official", "", "Details of the official nature of this name.", 0, 4087 java.lang.Integer.MAX_VALUE, official); 4088 case -896505829: 4089 /* source */ return new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 4090 java.lang.Integer.MAX_VALUE, source); 4091 default: 4092 return super.getNamedProperty(_hash, _name, _checkValid); 4093 } 4094 4095 } 4096 4097 @Override 4098 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4099 switch (hash) { 4100 case 3373707: 4101 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4102 case 3575610: 4103 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4104 case -892481550: 4105 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 4106 case -1294005119: 4107 /* preferred */ return this.preferred == null ? new Base[0] : new Base[] { this.preferred }; // BooleanType 4108 case -1613589672: 4109 /* language */ return this.language == null ? new Base[0] 4110 : this.language.toArray(new Base[this.language.size()]); // CodeableConcept 4111 case -1326197564: 4112 /* domain */ return this.domain == null ? new Base[0] : this.domain.toArray(new Base[this.domain.size()]); // CodeableConcept 4113 case -507075711: 4114 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 4115 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4116 case -1742128133: 4117 /* synonym */ return this.synonym == null ? new Base[0] : this.synonym.toArray(new Base[this.synonym.size()]); // SubstanceSpecificationNameComponent 4118 case -1840647503: 4119 /* translation */ return this.translation == null ? new Base[0] 4120 : this.translation.toArray(new Base[this.translation.size()]); // SubstanceSpecificationNameComponent 4121 case -765289749: 4122 /* official */ return this.official == null ? new Base[0] 4123 : this.official.toArray(new Base[this.official.size()]); // SubstanceSpecificationNameOfficialComponent 4124 case -896505829: 4125 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 4126 default: 4127 return super.getProperty(hash, name, checkValid); 4128 } 4129 4130 } 4131 4132 @Override 4133 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4134 switch (hash) { 4135 case 3373707: // name 4136 this.name = castToString(value); // StringType 4137 return value; 4138 case 3575610: // type 4139 this.type = castToCodeableConcept(value); // CodeableConcept 4140 return value; 4141 case -892481550: // status 4142 this.status = castToCodeableConcept(value); // CodeableConcept 4143 return value; 4144 case -1294005119: // preferred 4145 this.preferred = castToBoolean(value); // BooleanType 4146 return value; 4147 case -1613589672: // language 4148 this.getLanguage().add(castToCodeableConcept(value)); // CodeableConcept 4149 return value; 4150 case -1326197564: // domain 4151 this.getDomain().add(castToCodeableConcept(value)); // CodeableConcept 4152 return value; 4153 case -507075711: // jurisdiction 4154 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4155 return value; 4156 case -1742128133: // synonym 4157 this.getSynonym().add((SubstanceSpecificationNameComponent) value); // SubstanceSpecificationNameComponent 4158 return value; 4159 case -1840647503: // translation 4160 this.getTranslation().add((SubstanceSpecificationNameComponent) value); // SubstanceSpecificationNameComponent 4161 return value; 4162 case -765289749: // official 4163 this.getOfficial().add((SubstanceSpecificationNameOfficialComponent) value); // SubstanceSpecificationNameOfficialComponent 4164 return value; 4165 case -896505829: // source 4166 this.getSource().add(castToReference(value)); // Reference 4167 return value; 4168 default: 4169 return super.setProperty(hash, name, value); 4170 } 4171 4172 } 4173 4174 @Override 4175 public Base setProperty(String name, Base value) throws FHIRException { 4176 if (name.equals("name")) { 4177 this.name = castToString(value); // StringType 4178 } else if (name.equals("type")) { 4179 this.type = castToCodeableConcept(value); // CodeableConcept 4180 } else if (name.equals("status")) { 4181 this.status = castToCodeableConcept(value); // CodeableConcept 4182 } else if (name.equals("preferred")) { 4183 this.preferred = castToBoolean(value); // BooleanType 4184 } else if (name.equals("language")) { 4185 this.getLanguage().add(castToCodeableConcept(value)); 4186 } else if (name.equals("domain")) { 4187 this.getDomain().add(castToCodeableConcept(value)); 4188 } else if (name.equals("jurisdiction")) { 4189 this.getJurisdiction().add(castToCodeableConcept(value)); 4190 } else if (name.equals("synonym")) { 4191 this.getSynonym().add((SubstanceSpecificationNameComponent) value); 4192 } else if (name.equals("translation")) { 4193 this.getTranslation().add((SubstanceSpecificationNameComponent) value); 4194 } else if (name.equals("official")) { 4195 this.getOfficial().add((SubstanceSpecificationNameOfficialComponent) value); 4196 } else if (name.equals("source")) { 4197 this.getSource().add(castToReference(value)); 4198 } else 4199 return super.setProperty(name, value); 4200 return value; 4201 } 4202 4203 @Override 4204 public Base makeProperty(int hash, String name) throws FHIRException { 4205 switch (hash) { 4206 case 3373707: 4207 return getNameElement(); 4208 case 3575610: 4209 return getType(); 4210 case -892481550: 4211 return getStatus(); 4212 case -1294005119: 4213 return getPreferredElement(); 4214 case -1613589672: 4215 return addLanguage(); 4216 case -1326197564: 4217 return addDomain(); 4218 case -507075711: 4219 return addJurisdiction(); 4220 case -1742128133: 4221 return addSynonym(); 4222 case -1840647503: 4223 return addTranslation(); 4224 case -765289749: 4225 return addOfficial(); 4226 case -896505829: 4227 return addSource(); 4228 default: 4229 return super.makeProperty(hash, name); 4230 } 4231 4232 } 4233 4234 @Override 4235 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4236 switch (hash) { 4237 case 3373707: 4238 /* name */ return new String[] { "string" }; 4239 case 3575610: 4240 /* type */ return new String[] { "CodeableConcept" }; 4241 case -892481550: 4242 /* status */ return new String[] { "CodeableConcept" }; 4243 case -1294005119: 4244 /* preferred */ return new String[] { "boolean" }; 4245 case -1613589672: 4246 /* language */ return new String[] { "CodeableConcept" }; 4247 case -1326197564: 4248 /* domain */ return new String[] { "CodeableConcept" }; 4249 case -507075711: 4250 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4251 case -1742128133: 4252 /* synonym */ return new String[] { "@SubstanceSpecification.name" }; 4253 case -1840647503: 4254 /* translation */ return new String[] { "@SubstanceSpecification.name" }; 4255 case -765289749: 4256 /* official */ return new String[] {}; 4257 case -896505829: 4258 /* source */ return new String[] { "Reference" }; 4259 default: 4260 return super.getTypesForProperty(hash, name); 4261 } 4262 4263 } 4264 4265 @Override 4266 public Base addChild(String name) throws FHIRException { 4267 if (name.equals("name")) { 4268 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.name"); 4269 } else if (name.equals("type")) { 4270 this.type = new CodeableConcept(); 4271 return this.type; 4272 } else if (name.equals("status")) { 4273 this.status = new CodeableConcept(); 4274 return this.status; 4275 } else if (name.equals("preferred")) { 4276 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.preferred"); 4277 } else if (name.equals("language")) { 4278 return addLanguage(); 4279 } else if (name.equals("domain")) { 4280 return addDomain(); 4281 } else if (name.equals("jurisdiction")) { 4282 return addJurisdiction(); 4283 } else if (name.equals("synonym")) { 4284 return addSynonym(); 4285 } else if (name.equals("translation")) { 4286 return addTranslation(); 4287 } else if (name.equals("official")) { 4288 return addOfficial(); 4289 } else if (name.equals("source")) { 4290 return addSource(); 4291 } else 4292 return super.addChild(name); 4293 } 4294 4295 public SubstanceSpecificationNameComponent copy() { 4296 SubstanceSpecificationNameComponent dst = new SubstanceSpecificationNameComponent(); 4297 copyValues(dst); 4298 return dst; 4299 } 4300 4301 public void copyValues(SubstanceSpecificationNameComponent dst) { 4302 super.copyValues(dst); 4303 dst.name = name == null ? null : name.copy(); 4304 dst.type = type == null ? null : type.copy(); 4305 dst.status = status == null ? null : status.copy(); 4306 dst.preferred = preferred == null ? null : preferred.copy(); 4307 if (language != null) { 4308 dst.language = new ArrayList<CodeableConcept>(); 4309 for (CodeableConcept i : language) 4310 dst.language.add(i.copy()); 4311 } 4312 ; 4313 if (domain != null) { 4314 dst.domain = new ArrayList<CodeableConcept>(); 4315 for (CodeableConcept i : domain) 4316 dst.domain.add(i.copy()); 4317 } 4318 ; 4319 if (jurisdiction != null) { 4320 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4321 for (CodeableConcept i : jurisdiction) 4322 dst.jurisdiction.add(i.copy()); 4323 } 4324 ; 4325 if (synonym != null) { 4326 dst.synonym = new ArrayList<SubstanceSpecificationNameComponent>(); 4327 for (SubstanceSpecificationNameComponent i : synonym) 4328 dst.synonym.add(i.copy()); 4329 } 4330 ; 4331 if (translation != null) { 4332 dst.translation = new ArrayList<SubstanceSpecificationNameComponent>(); 4333 for (SubstanceSpecificationNameComponent i : translation) 4334 dst.translation.add(i.copy()); 4335 } 4336 ; 4337 if (official != null) { 4338 dst.official = new ArrayList<SubstanceSpecificationNameOfficialComponent>(); 4339 for (SubstanceSpecificationNameOfficialComponent i : official) 4340 dst.official.add(i.copy()); 4341 } 4342 ; 4343 if (source != null) { 4344 dst.source = new ArrayList<Reference>(); 4345 for (Reference i : source) 4346 dst.source.add(i.copy()); 4347 } 4348 ; 4349 } 4350 4351 @Override 4352 public boolean equalsDeep(Base other_) { 4353 if (!super.equalsDeep(other_)) 4354 return false; 4355 if (!(other_ instanceof SubstanceSpecificationNameComponent)) 4356 return false; 4357 SubstanceSpecificationNameComponent o = (SubstanceSpecificationNameComponent) other_; 4358 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true) && compareDeep(status, o.status, true) 4359 && compareDeep(preferred, o.preferred, true) && compareDeep(language, o.language, true) 4360 && compareDeep(domain, o.domain, true) && compareDeep(jurisdiction, o.jurisdiction, true) 4361 && compareDeep(synonym, o.synonym, true) && compareDeep(translation, o.translation, true) 4362 && compareDeep(official, o.official, true) && compareDeep(source, o.source, true); 4363 } 4364 4365 @Override 4366 public boolean equalsShallow(Base other_) { 4367 if (!super.equalsShallow(other_)) 4368 return false; 4369 if (!(other_ instanceof SubstanceSpecificationNameComponent)) 4370 return false; 4371 SubstanceSpecificationNameComponent o = (SubstanceSpecificationNameComponent) other_; 4372 return compareValues(name, o.name, true) && compareValues(preferred, o.preferred, true); 4373 } 4374 4375 public boolean isEmpty() { 4376 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type, status, preferred, language, domain, 4377 jurisdiction, synonym, translation, official, source); 4378 } 4379 4380 public String fhirType() { 4381 return "SubstanceSpecification.name"; 4382 4383 } 4384 4385 } 4386 4387 @Block() 4388 public static class SubstanceSpecificationNameOfficialComponent extends BackboneElement 4389 implements IBaseBackboneElement { 4390 /** 4391 * Which authority uses this official name. 4392 */ 4393 @Child(name = "authority", type = { 4394 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 4395 @Description(shortDefinition = "Which authority uses this official name", formalDefinition = "Which authority uses this official name.") 4396 protected CodeableConcept authority; 4397 4398 /** 4399 * The status of the official name. 4400 */ 4401 @Child(name = "status", type = { 4402 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4403 @Description(shortDefinition = "The status of the official name", formalDefinition = "The status of the official name.") 4404 protected CodeableConcept status; 4405 4406 /** 4407 * Date of official name change. 4408 */ 4409 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4410 @Description(shortDefinition = "Date of official name change", formalDefinition = "Date of official name change.") 4411 protected DateTimeType date; 4412 4413 private static final long serialVersionUID = -2040011008L; 4414 4415 /** 4416 * Constructor 4417 */ 4418 public SubstanceSpecificationNameOfficialComponent() { 4419 super(); 4420 } 4421 4422 /** 4423 * @return {@link #authority} (Which authority uses this official name.) 4424 */ 4425 public CodeableConcept getAuthority() { 4426 if (this.authority == null) 4427 if (Configuration.errorOnAutoCreate()) 4428 throw new Error("Attempt to auto-create SubstanceSpecificationNameOfficialComponent.authority"); 4429 else if (Configuration.doAutoCreate()) 4430 this.authority = new CodeableConcept(); // cc 4431 return this.authority; 4432 } 4433 4434 public boolean hasAuthority() { 4435 return this.authority != null && !this.authority.isEmpty(); 4436 } 4437 4438 /** 4439 * @param value {@link #authority} (Which authority uses this official name.) 4440 */ 4441 public SubstanceSpecificationNameOfficialComponent setAuthority(CodeableConcept value) { 4442 this.authority = value; 4443 return this; 4444 } 4445 4446 /** 4447 * @return {@link #status} (The status of the official name.) 4448 */ 4449 public CodeableConcept getStatus() { 4450 if (this.status == null) 4451 if (Configuration.errorOnAutoCreate()) 4452 throw new Error("Attempt to auto-create SubstanceSpecificationNameOfficialComponent.status"); 4453 else if (Configuration.doAutoCreate()) 4454 this.status = new CodeableConcept(); // cc 4455 return this.status; 4456 } 4457 4458 public boolean hasStatus() { 4459 return this.status != null && !this.status.isEmpty(); 4460 } 4461 4462 /** 4463 * @param value {@link #status} (The status of the official name.) 4464 */ 4465 public SubstanceSpecificationNameOfficialComponent setStatus(CodeableConcept value) { 4466 this.status = value; 4467 return this; 4468 } 4469 4470 /** 4471 * @return {@link #date} (Date of official name change.). This is the underlying 4472 * object with id, value and extensions. The accessor "getDate" gives 4473 * direct access to the value 4474 */ 4475 public DateTimeType getDateElement() { 4476 if (this.date == null) 4477 if (Configuration.errorOnAutoCreate()) 4478 throw new Error("Attempt to auto-create SubstanceSpecificationNameOfficialComponent.date"); 4479 else if (Configuration.doAutoCreate()) 4480 this.date = new DateTimeType(); // bb 4481 return this.date; 4482 } 4483 4484 public boolean hasDateElement() { 4485 return this.date != null && !this.date.isEmpty(); 4486 } 4487 4488 public boolean hasDate() { 4489 return this.date != null && !this.date.isEmpty(); 4490 } 4491 4492 /** 4493 * @param value {@link #date} (Date of official name change.). This is the 4494 * underlying object with id, value and extensions. The accessor 4495 * "getDate" gives direct access to the value 4496 */ 4497 public SubstanceSpecificationNameOfficialComponent setDateElement(DateTimeType value) { 4498 this.date = value; 4499 return this; 4500 } 4501 4502 /** 4503 * @return Date of official name change. 4504 */ 4505 public Date getDate() { 4506 return this.date == null ? null : this.date.getValue(); 4507 } 4508 4509 /** 4510 * @param value Date of official name change. 4511 */ 4512 public SubstanceSpecificationNameOfficialComponent setDate(Date value) { 4513 if (value == null) 4514 this.date = null; 4515 else { 4516 if (this.date == null) 4517 this.date = new DateTimeType(); 4518 this.date.setValue(value); 4519 } 4520 return this; 4521 } 4522 4523 protected void listChildren(List<Property> children) { 4524 super.listChildren(children); 4525 children.add( 4526 new Property("authority", "CodeableConcept", "Which authority uses this official name.", 0, 1, authority)); 4527 children.add(new Property("status", "CodeableConcept", "The status of the official name.", 0, 1, status)); 4528 children.add(new Property("date", "dateTime", "Date of official name change.", 0, 1, date)); 4529 } 4530 4531 @Override 4532 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4533 switch (_hash) { 4534 case 1475610435: 4535 /* authority */ return new Property("authority", "CodeableConcept", "Which authority uses this official name.", 4536 0, 1, authority); 4537 case -892481550: 4538 /* status */ return new Property("status", "CodeableConcept", "The status of the official name.", 0, 1, status); 4539 case 3076014: 4540 /* date */ return new Property("date", "dateTime", "Date of official name change.", 0, 1, date); 4541 default: 4542 return super.getNamedProperty(_hash, _name, _checkValid); 4543 } 4544 4545 } 4546 4547 @Override 4548 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4549 switch (hash) { 4550 case 1475610435: 4551 /* authority */ return this.authority == null ? new Base[0] : new Base[] { this.authority }; // CodeableConcept 4552 case -892481550: 4553 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 4554 case 3076014: 4555 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4556 default: 4557 return super.getProperty(hash, name, checkValid); 4558 } 4559 4560 } 4561 4562 @Override 4563 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4564 switch (hash) { 4565 case 1475610435: // authority 4566 this.authority = castToCodeableConcept(value); // CodeableConcept 4567 return value; 4568 case -892481550: // status 4569 this.status = castToCodeableConcept(value); // CodeableConcept 4570 return value; 4571 case 3076014: // date 4572 this.date = castToDateTime(value); // DateTimeType 4573 return value; 4574 default: 4575 return super.setProperty(hash, name, value); 4576 } 4577 4578 } 4579 4580 @Override 4581 public Base setProperty(String name, Base value) throws FHIRException { 4582 if (name.equals("authority")) { 4583 this.authority = castToCodeableConcept(value); // CodeableConcept 4584 } else if (name.equals("status")) { 4585 this.status = castToCodeableConcept(value); // CodeableConcept 4586 } else if (name.equals("date")) { 4587 this.date = castToDateTime(value); // DateTimeType 4588 } else 4589 return super.setProperty(name, value); 4590 return value; 4591 } 4592 4593 @Override 4594 public Base makeProperty(int hash, String name) throws FHIRException { 4595 switch (hash) { 4596 case 1475610435: 4597 return getAuthority(); 4598 case -892481550: 4599 return getStatus(); 4600 case 3076014: 4601 return getDateElement(); 4602 default: 4603 return super.makeProperty(hash, name); 4604 } 4605 4606 } 4607 4608 @Override 4609 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4610 switch (hash) { 4611 case 1475610435: 4612 /* authority */ return new String[] { "CodeableConcept" }; 4613 case -892481550: 4614 /* status */ return new String[] { "CodeableConcept" }; 4615 case 3076014: 4616 /* date */ return new String[] { "dateTime" }; 4617 default: 4618 return super.getTypesForProperty(hash, name); 4619 } 4620 4621 } 4622 4623 @Override 4624 public Base addChild(String name) throws FHIRException { 4625 if (name.equals("authority")) { 4626 this.authority = new CodeableConcept(); 4627 return this.authority; 4628 } else if (name.equals("status")) { 4629 this.status = new CodeableConcept(); 4630 return this.status; 4631 } else if (name.equals("date")) { 4632 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.date"); 4633 } else 4634 return super.addChild(name); 4635 } 4636 4637 public SubstanceSpecificationNameOfficialComponent copy() { 4638 SubstanceSpecificationNameOfficialComponent dst = new SubstanceSpecificationNameOfficialComponent(); 4639 copyValues(dst); 4640 return dst; 4641 } 4642 4643 public void copyValues(SubstanceSpecificationNameOfficialComponent dst) { 4644 super.copyValues(dst); 4645 dst.authority = authority == null ? null : authority.copy(); 4646 dst.status = status == null ? null : status.copy(); 4647 dst.date = date == null ? null : date.copy(); 4648 } 4649 4650 @Override 4651 public boolean equalsDeep(Base other_) { 4652 if (!super.equalsDeep(other_)) 4653 return false; 4654 if (!(other_ instanceof SubstanceSpecificationNameOfficialComponent)) 4655 return false; 4656 SubstanceSpecificationNameOfficialComponent o = (SubstanceSpecificationNameOfficialComponent) other_; 4657 return compareDeep(authority, o.authority, true) && compareDeep(status, o.status, true) 4658 && compareDeep(date, o.date, true); 4659 } 4660 4661 @Override 4662 public boolean equalsShallow(Base other_) { 4663 if (!super.equalsShallow(other_)) 4664 return false; 4665 if (!(other_ instanceof SubstanceSpecificationNameOfficialComponent)) 4666 return false; 4667 SubstanceSpecificationNameOfficialComponent o = (SubstanceSpecificationNameOfficialComponent) other_; 4668 return compareValues(date, o.date, true); 4669 } 4670 4671 public boolean isEmpty() { 4672 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(authority, status, date); 4673 } 4674 4675 public String fhirType() { 4676 return "SubstanceSpecification.name.official"; 4677 4678 } 4679 4680 } 4681 4682 @Block() 4683 public static class SubstanceSpecificationRelationshipComponent extends BackboneElement 4684 implements IBaseBackboneElement { 4685 /** 4686 * A pointer to another substance, as a resource or just a representational 4687 * code. 4688 */ 4689 @Child(name = "substance", type = { SubstanceSpecification.class, 4690 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 4691 @Description(shortDefinition = "A pointer to another substance, as a resource or just a representational code", formalDefinition = "A pointer to another substance, as a resource or just a representational code.") 4692 protected Type substance; 4693 4694 /** 4695 * For example "salt to parent", "active moiety", "starting material". 4696 */ 4697 @Child(name = "relationship", type = { 4698 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4699 @Description(shortDefinition = "For example \"salt to parent\", \"active moiety\", \"starting material\"", formalDefinition = "For example \"salt to parent\", \"active moiety\", \"starting material\".") 4700 protected CodeableConcept relationship; 4701 4702 /** 4703 * For example where an enzyme strongly bonds with a particular substance, this 4704 * is a defining relationship for that enzyme, out of several possible substance 4705 * relationships. 4706 */ 4707 @Child(name = "isDefining", type = { 4708 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4709 @Description(shortDefinition = "For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships", formalDefinition = "For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships.") 4710 protected BooleanType isDefining; 4711 4712 /** 4713 * A numeric factor for the relationship, for instance to express that the salt 4714 * of a substance has some percentage of the active substance in relation to 4715 * some other. 4716 */ 4717 @Child(name = "amount", type = { Quantity.class, Range.class, Ratio.class, 4718 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 4719 @Description(shortDefinition = "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other", formalDefinition = "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.") 4720 protected Type amount; 4721 4722 /** 4723 * For use when the numeric. 4724 */ 4725 @Child(name = "amountRatioLowLimit", type = { 4726 Ratio.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 4727 @Description(shortDefinition = "For use when the numeric", formalDefinition = "For use when the numeric.") 4728 protected Ratio amountRatioLowLimit; 4729 4730 /** 4731 * An operator for the amount, for example "average", "approximately", "less 4732 * than". 4733 */ 4734 @Child(name = "amountType", type = { 4735 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 4736 @Description(shortDefinition = "An operator for the amount, for example \"average\", \"approximately\", \"less than\"", formalDefinition = "An operator for the amount, for example \"average\", \"approximately\", \"less than\".") 4737 protected CodeableConcept amountType; 4738 4739 /** 4740 * Supporting literature. 4741 */ 4742 @Child(name = "source", type = { 4743 DocumentReference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4744 @Description(shortDefinition = "Supporting literature", formalDefinition = "Supporting literature.") 4745 protected List<Reference> source; 4746 /** 4747 * The actual objects that are the target of the reference (Supporting 4748 * literature.) 4749 */ 4750 protected List<DocumentReference> sourceTarget; 4751 4752 private static final long serialVersionUID = -1277419269L; 4753 4754 /** 4755 * Constructor 4756 */ 4757 public SubstanceSpecificationRelationshipComponent() { 4758 super(); 4759 } 4760 4761 /** 4762 * @return {@link #substance} (A pointer to another substance, as a resource or 4763 * just a representational code.) 4764 */ 4765 public Type getSubstance() { 4766 return this.substance; 4767 } 4768 4769 /** 4770 * @return {@link #substance} (A pointer to another substance, as a resource or 4771 * just a representational code.) 4772 */ 4773 public Reference getSubstanceReference() throws FHIRException { 4774 if (this.substance == null) 4775 this.substance = new Reference(); 4776 if (!(this.substance instanceof Reference)) 4777 throw new FHIRException("Type mismatch: the type Reference was expected, but " 4778 + this.substance.getClass().getName() + " was encountered"); 4779 return (Reference) this.substance; 4780 } 4781 4782 public boolean hasSubstanceReference() { 4783 return this != null && this.substance instanceof Reference; 4784 } 4785 4786 /** 4787 * @return {@link #substance} (A pointer to another substance, as a resource or 4788 * just a representational code.) 4789 */ 4790 public CodeableConcept getSubstanceCodeableConcept() throws FHIRException { 4791 if (this.substance == null) 4792 this.substance = new CodeableConcept(); 4793 if (!(this.substance instanceof CodeableConcept)) 4794 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 4795 + this.substance.getClass().getName() + " was encountered"); 4796 return (CodeableConcept) this.substance; 4797 } 4798 4799 public boolean hasSubstanceCodeableConcept() { 4800 return this != null && this.substance instanceof CodeableConcept; 4801 } 4802 4803 public boolean hasSubstance() { 4804 return this.substance != null && !this.substance.isEmpty(); 4805 } 4806 4807 /** 4808 * @param value {@link #substance} (A pointer to another substance, as a 4809 * resource or just a representational code.) 4810 */ 4811 public SubstanceSpecificationRelationshipComponent setSubstance(Type value) { 4812 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 4813 throw new Error("Not the right type for SubstanceSpecification.relationship.substance[x]: " + value.fhirType()); 4814 this.substance = value; 4815 return this; 4816 } 4817 4818 /** 4819 * @return {@link #relationship} (For example "salt to parent", "active moiety", 4820 * "starting material".) 4821 */ 4822 public CodeableConcept getRelationship() { 4823 if (this.relationship == null) 4824 if (Configuration.errorOnAutoCreate()) 4825 throw new Error("Attempt to auto-create SubstanceSpecificationRelationshipComponent.relationship"); 4826 else if (Configuration.doAutoCreate()) 4827 this.relationship = new CodeableConcept(); // cc 4828 return this.relationship; 4829 } 4830 4831 public boolean hasRelationship() { 4832 return this.relationship != null && !this.relationship.isEmpty(); 4833 } 4834 4835 /** 4836 * @param value {@link #relationship} (For example "salt to parent", "active 4837 * moiety", "starting material".) 4838 */ 4839 public SubstanceSpecificationRelationshipComponent setRelationship(CodeableConcept value) { 4840 this.relationship = value; 4841 return this; 4842 } 4843 4844 /** 4845 * @return {@link #isDefining} (For example where an enzyme strongly bonds with 4846 * a particular substance, this is a defining relationship for that 4847 * enzyme, out of several possible substance relationships.). This is 4848 * the underlying object with id, value and extensions. The accessor 4849 * "getIsDefining" gives direct access to the value 4850 */ 4851 public BooleanType getIsDefiningElement() { 4852 if (this.isDefining == null) 4853 if (Configuration.errorOnAutoCreate()) 4854 throw new Error("Attempt to auto-create SubstanceSpecificationRelationshipComponent.isDefining"); 4855 else if (Configuration.doAutoCreate()) 4856 this.isDefining = new BooleanType(); // bb 4857 return this.isDefining; 4858 } 4859 4860 public boolean hasIsDefiningElement() { 4861 return this.isDefining != null && !this.isDefining.isEmpty(); 4862 } 4863 4864 public boolean hasIsDefining() { 4865 return this.isDefining != null && !this.isDefining.isEmpty(); 4866 } 4867 4868 /** 4869 * @param value {@link #isDefining} (For example where an enzyme strongly bonds 4870 * with a particular substance, this is a defining relationship for 4871 * that enzyme, out of several possible substance relationships.). 4872 * This is the underlying object with id, value and extensions. The 4873 * accessor "getIsDefining" gives direct access to the value 4874 */ 4875 public SubstanceSpecificationRelationshipComponent setIsDefiningElement(BooleanType value) { 4876 this.isDefining = value; 4877 return this; 4878 } 4879 4880 /** 4881 * @return For example where an enzyme strongly bonds with a particular 4882 * substance, this is a defining relationship for that enzyme, out of 4883 * several possible substance relationships. 4884 */ 4885 public boolean getIsDefining() { 4886 return this.isDefining == null || this.isDefining.isEmpty() ? false : this.isDefining.getValue(); 4887 } 4888 4889 /** 4890 * @param value For example where an enzyme strongly bonds with a particular 4891 * substance, this is a defining relationship for that enzyme, out 4892 * of several possible substance relationships. 4893 */ 4894 public SubstanceSpecificationRelationshipComponent setIsDefining(boolean value) { 4895 if (this.isDefining == null) 4896 this.isDefining = new BooleanType(); 4897 this.isDefining.setValue(value); 4898 return this; 4899 } 4900 4901 /** 4902 * @return {@link #amount} (A numeric factor for the relationship, for instance 4903 * to express that the salt of a substance has some percentage of the 4904 * active substance in relation to some other.) 4905 */ 4906 public Type getAmount() { 4907 return this.amount; 4908 } 4909 4910 /** 4911 * @return {@link #amount} (A numeric factor for the relationship, for instance 4912 * to express that the salt of a substance has some percentage of the 4913 * active substance in relation to some other.) 4914 */ 4915 public Quantity getAmountQuantity() throws FHIRException { 4916 if (this.amount == null) 4917 this.amount = new Quantity(); 4918 if (!(this.amount instanceof Quantity)) 4919 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.amount.getClass().getName() 4920 + " was encountered"); 4921 return (Quantity) this.amount; 4922 } 4923 4924 public boolean hasAmountQuantity() { 4925 return this != null && this.amount instanceof Quantity; 4926 } 4927 4928 /** 4929 * @return {@link #amount} (A numeric factor for the relationship, for instance 4930 * to express that the salt of a substance has some percentage of the 4931 * active substance in relation to some other.) 4932 */ 4933 public Range getAmountRange() throws FHIRException { 4934 if (this.amount == null) 4935 this.amount = new Range(); 4936 if (!(this.amount instanceof Range)) 4937 throw new FHIRException( 4938 "Type mismatch: the type Range was expected, but " + this.amount.getClass().getName() + " was encountered"); 4939 return (Range) this.amount; 4940 } 4941 4942 public boolean hasAmountRange() { 4943 return this != null && this.amount instanceof Range; 4944 } 4945 4946 /** 4947 * @return {@link #amount} (A numeric factor for the relationship, for instance 4948 * to express that the salt of a substance has some percentage of the 4949 * active substance in relation to some other.) 4950 */ 4951 public Ratio getAmountRatio() throws FHIRException { 4952 if (this.amount == null) 4953 this.amount = new Ratio(); 4954 if (!(this.amount instanceof Ratio)) 4955 throw new FHIRException( 4956 "Type mismatch: the type Ratio was expected, but " + this.amount.getClass().getName() + " was encountered"); 4957 return (Ratio) this.amount; 4958 } 4959 4960 public boolean hasAmountRatio() { 4961 return this != null && this.amount instanceof Ratio; 4962 } 4963 4964 /** 4965 * @return {@link #amount} (A numeric factor for the relationship, for instance 4966 * to express that the salt of a substance has some percentage of the 4967 * active substance in relation to some other.) 4968 */ 4969 public StringType getAmountStringType() throws FHIRException { 4970 if (this.amount == null) 4971 this.amount = new StringType(); 4972 if (!(this.amount instanceof StringType)) 4973 throw new FHIRException("Type mismatch: the type StringType was expected, but " 4974 + this.amount.getClass().getName() + " was encountered"); 4975 return (StringType) this.amount; 4976 } 4977 4978 public boolean hasAmountStringType() { 4979 return this != null && this.amount instanceof StringType; 4980 } 4981 4982 public boolean hasAmount() { 4983 return this.amount != null && !this.amount.isEmpty(); 4984 } 4985 4986 /** 4987 * @param value {@link #amount} (A numeric factor for the relationship, for 4988 * instance to express that the salt of a substance has some 4989 * percentage of the active substance in relation to some other.) 4990 */ 4991 public SubstanceSpecificationRelationshipComponent setAmount(Type value) { 4992 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof Ratio 4993 || value instanceof StringType)) 4994 throw new Error("Not the right type for SubstanceSpecification.relationship.amount[x]: " + value.fhirType()); 4995 this.amount = value; 4996 return this; 4997 } 4998 4999 /** 5000 * @return {@link #amountRatioLowLimit} (For use when the numeric.) 5001 */ 5002 public Ratio getAmountRatioLowLimit() { 5003 if (this.amountRatioLowLimit == null) 5004 if (Configuration.errorOnAutoCreate()) 5005 throw new Error("Attempt to auto-create SubstanceSpecificationRelationshipComponent.amountRatioLowLimit"); 5006 else if (Configuration.doAutoCreate()) 5007 this.amountRatioLowLimit = new Ratio(); // cc 5008 return this.amountRatioLowLimit; 5009 } 5010 5011 public boolean hasAmountRatioLowLimit() { 5012 return this.amountRatioLowLimit != null && !this.amountRatioLowLimit.isEmpty(); 5013 } 5014 5015 /** 5016 * @param value {@link #amountRatioLowLimit} (For use when the numeric.) 5017 */ 5018 public SubstanceSpecificationRelationshipComponent setAmountRatioLowLimit(Ratio value) { 5019 this.amountRatioLowLimit = value; 5020 return this; 5021 } 5022 5023 /** 5024 * @return {@link #amountType} (An operator for the amount, for example 5025 * "average", "approximately", "less than".) 5026 */ 5027 public CodeableConcept getAmountType() { 5028 if (this.amountType == null) 5029 if (Configuration.errorOnAutoCreate()) 5030 throw new Error("Attempt to auto-create SubstanceSpecificationRelationshipComponent.amountType"); 5031 else if (Configuration.doAutoCreate()) 5032 this.amountType = new CodeableConcept(); // cc 5033 return this.amountType; 5034 } 5035 5036 public boolean hasAmountType() { 5037 return this.amountType != null && !this.amountType.isEmpty(); 5038 } 5039 5040 /** 5041 * @param value {@link #amountType} (An operator for the amount, for example 5042 * "average", "approximately", "less than".) 5043 */ 5044 public SubstanceSpecificationRelationshipComponent setAmountType(CodeableConcept value) { 5045 this.amountType = value; 5046 return this; 5047 } 5048 5049 /** 5050 * @return {@link #source} (Supporting literature.) 5051 */ 5052 public List<Reference> getSource() { 5053 if (this.source == null) 5054 this.source = new ArrayList<Reference>(); 5055 return this.source; 5056 } 5057 5058 /** 5059 * @return Returns a reference to <code>this</code> for easy method chaining 5060 */ 5061 public SubstanceSpecificationRelationshipComponent setSource(List<Reference> theSource) { 5062 this.source = theSource; 5063 return this; 5064 } 5065 5066 public boolean hasSource() { 5067 if (this.source == null) 5068 return false; 5069 for (Reference item : this.source) 5070 if (!item.isEmpty()) 5071 return true; 5072 return false; 5073 } 5074 5075 public Reference addSource() { // 3 5076 Reference t = new Reference(); 5077 if (this.source == null) 5078 this.source = new ArrayList<Reference>(); 5079 this.source.add(t); 5080 return t; 5081 } 5082 5083 public SubstanceSpecificationRelationshipComponent addSource(Reference t) { // 3 5084 if (t == null) 5085 return this; 5086 if (this.source == null) 5087 this.source = new ArrayList<Reference>(); 5088 this.source.add(t); 5089 return this; 5090 } 5091 5092 /** 5093 * @return The first repetition of repeating field {@link #source}, creating it 5094 * if it does not already exist 5095 */ 5096 public Reference getSourceFirstRep() { 5097 if (getSource().isEmpty()) { 5098 addSource(); 5099 } 5100 return getSource().get(0); 5101 } 5102 5103 /** 5104 * @deprecated Use Reference#setResource(IBaseResource) instead 5105 */ 5106 @Deprecated 5107 public List<DocumentReference> getSourceTarget() { 5108 if (this.sourceTarget == null) 5109 this.sourceTarget = new ArrayList<DocumentReference>(); 5110 return this.sourceTarget; 5111 } 5112 5113 /** 5114 * @deprecated Use Reference#setResource(IBaseResource) instead 5115 */ 5116 @Deprecated 5117 public DocumentReference addSourceTarget() { 5118 DocumentReference r = new DocumentReference(); 5119 if (this.sourceTarget == null) 5120 this.sourceTarget = new ArrayList<DocumentReference>(); 5121 this.sourceTarget.add(r); 5122 return r; 5123 } 5124 5125 protected void listChildren(List<Property> children) { 5126 super.listChildren(children); 5127 children.add(new Property("substance[x]", "Reference(SubstanceSpecification)|CodeableConcept", 5128 "A pointer to another substance, as a resource or just a representational code.", 0, 1, substance)); 5129 children.add(new Property("relationship", "CodeableConcept", 5130 "For example \"salt to parent\", \"active moiety\", \"starting material\".", 0, 1, relationship)); 5131 children.add(new Property("isDefining", "boolean", 5132 "For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships.", 5133 0, 1, isDefining)); 5134 children.add(new Property("amount[x]", "Quantity|Range|Ratio|string", 5135 "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 5136 0, 1, amount)); 5137 children 5138 .add(new Property("amountRatioLowLimit", "Ratio", "For use when the numeric.", 0, 1, amountRatioLowLimit)); 5139 children.add(new Property("amountType", "CodeableConcept", 5140 "An operator for the amount, for example \"average\", \"approximately\", \"less than\".", 0, 1, amountType)); 5141 children.add(new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 5142 java.lang.Integer.MAX_VALUE, source)); 5143 } 5144 5145 @Override 5146 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5147 switch (_hash) { 5148 case 2127194384: 5149 /* substance[x] */ return new Property("substance[x]", "Reference(SubstanceSpecification)|CodeableConcept", 5150 "A pointer to another substance, as a resource or just a representational code.", 0, 1, substance); 5151 case 530040176: 5152 /* substance */ return new Property("substance[x]", "Reference(SubstanceSpecification)|CodeableConcept", 5153 "A pointer to another substance, as a resource or just a representational code.", 0, 1, substance); 5154 case 516208571: 5155 /* substanceReference */ return new Property("substance[x]", 5156 "Reference(SubstanceSpecification)|CodeableConcept", 5157 "A pointer to another substance, as a resource or just a representational code.", 0, 1, substance); 5158 case -1974119407: 5159 /* substanceCodeableConcept */ return new Property("substance[x]", 5160 "Reference(SubstanceSpecification)|CodeableConcept", 5161 "A pointer to another substance, as a resource or just a representational code.", 0, 1, substance); 5162 case -261851592: 5163 /* relationship */ return new Property("relationship", "CodeableConcept", 5164 "For example \"salt to parent\", \"active moiety\", \"starting material\".", 0, 1, relationship); 5165 case -141812990: 5166 /* isDefining */ return new Property("isDefining", "boolean", 5167 "For example where an enzyme strongly bonds with a particular substance, this is a defining relationship for that enzyme, out of several possible substance relationships.", 5168 0, 1, isDefining); 5169 case 646780200: 5170 /* amount[x] */ return new Property("amount[x]", "Quantity|Range|Ratio|string", 5171 "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 5172 0, 1, amount); 5173 case -1413853096: 5174 /* amount */ return new Property("amount[x]", "Quantity|Range|Ratio|string", 5175 "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 5176 0, 1, amount); 5177 case 1664303363: 5178 /* amountQuantity */ return new Property("amount[x]", "Quantity|Range|Ratio|string", 5179 "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 5180 0, 1, amount); 5181 case -1223462971: 5182 /* amountRange */ return new Property("amount[x]", "Quantity|Range|Ratio|string", 5183 "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 5184 0, 1, amount); 5185 case -1223457133: 5186 /* amountRatio */ return new Property("amount[x]", "Quantity|Range|Ratio|string", 5187 "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 5188 0, 1, amount); 5189 case 773651081: 5190 /* amountString */ return new Property("amount[x]", "Quantity|Range|Ratio|string", 5191 "A numeric factor for the relationship, for instance to express that the salt of a substance has some percentage of the active substance in relation to some other.", 5192 0, 1, amount); 5193 case 2140623994: 5194 /* amountRatioLowLimit */ return new Property("amountRatioLowLimit", "Ratio", "For use when the numeric.", 0, 1, 5195 amountRatioLowLimit); 5196 case -1424857166: 5197 /* amountType */ return new Property("amountType", "CodeableConcept", 5198 "An operator for the amount, for example \"average\", \"approximately\", \"less than\".", 0, 1, amountType); 5199 case -896505829: 5200 /* source */ return new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 5201 java.lang.Integer.MAX_VALUE, source); 5202 default: 5203 return super.getNamedProperty(_hash, _name, _checkValid); 5204 } 5205 5206 } 5207 5208 @Override 5209 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5210 switch (hash) { 5211 case 530040176: 5212 /* substance */ return this.substance == null ? new Base[0] : new Base[] { this.substance }; // Type 5213 case -261851592: 5214 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // CodeableConcept 5215 case -141812990: 5216 /* isDefining */ return this.isDefining == null ? new Base[0] : new Base[] { this.isDefining }; // BooleanType 5217 case -1413853096: 5218 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Type 5219 case 2140623994: 5220 /* amountRatioLowLimit */ return this.amountRatioLowLimit == null ? new Base[0] 5221 : new Base[] { this.amountRatioLowLimit }; // Ratio 5222 case -1424857166: 5223 /* amountType */ return this.amountType == null ? new Base[0] : new Base[] { this.amountType }; // CodeableConcept 5224 case -896505829: 5225 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 5226 default: 5227 return super.getProperty(hash, name, checkValid); 5228 } 5229 5230 } 5231 5232 @Override 5233 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5234 switch (hash) { 5235 case 530040176: // substance 5236 this.substance = castToType(value); // Type 5237 return value; 5238 case -261851592: // relationship 5239 this.relationship = castToCodeableConcept(value); // CodeableConcept 5240 return value; 5241 case -141812990: // isDefining 5242 this.isDefining = castToBoolean(value); // BooleanType 5243 return value; 5244 case -1413853096: // amount 5245 this.amount = castToType(value); // Type 5246 return value; 5247 case 2140623994: // amountRatioLowLimit 5248 this.amountRatioLowLimit = castToRatio(value); // Ratio 5249 return value; 5250 case -1424857166: // amountType 5251 this.amountType = castToCodeableConcept(value); // CodeableConcept 5252 return value; 5253 case -896505829: // source 5254 this.getSource().add(castToReference(value)); // Reference 5255 return value; 5256 default: 5257 return super.setProperty(hash, name, value); 5258 } 5259 5260 } 5261 5262 @Override 5263 public Base setProperty(String name, Base value) throws FHIRException { 5264 if (name.equals("substance[x]")) { 5265 this.substance = castToType(value); // Type 5266 } else if (name.equals("relationship")) { 5267 this.relationship = castToCodeableConcept(value); // CodeableConcept 5268 } else if (name.equals("isDefining")) { 5269 this.isDefining = castToBoolean(value); // BooleanType 5270 } else if (name.equals("amount[x]")) { 5271 this.amount = castToType(value); // Type 5272 } else if (name.equals("amountRatioLowLimit")) { 5273 this.amountRatioLowLimit = castToRatio(value); // Ratio 5274 } else if (name.equals("amountType")) { 5275 this.amountType = castToCodeableConcept(value); // CodeableConcept 5276 } else if (name.equals("source")) { 5277 this.getSource().add(castToReference(value)); 5278 } else 5279 return super.setProperty(name, value); 5280 return value; 5281 } 5282 5283 @Override 5284 public Base makeProperty(int hash, String name) throws FHIRException { 5285 switch (hash) { 5286 case 2127194384: 5287 return getSubstance(); 5288 case 530040176: 5289 return getSubstance(); 5290 case -261851592: 5291 return getRelationship(); 5292 case -141812990: 5293 return getIsDefiningElement(); 5294 case 646780200: 5295 return getAmount(); 5296 case -1413853096: 5297 return getAmount(); 5298 case 2140623994: 5299 return getAmountRatioLowLimit(); 5300 case -1424857166: 5301 return getAmountType(); 5302 case -896505829: 5303 return addSource(); 5304 default: 5305 return super.makeProperty(hash, name); 5306 } 5307 5308 } 5309 5310 @Override 5311 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5312 switch (hash) { 5313 case 530040176: 5314 /* substance */ return new String[] { "Reference", "CodeableConcept" }; 5315 case -261851592: 5316 /* relationship */ return new String[] { "CodeableConcept" }; 5317 case -141812990: 5318 /* isDefining */ return new String[] { "boolean" }; 5319 case -1413853096: 5320 /* amount */ return new String[] { "Quantity", "Range", "Ratio", "string" }; 5321 case 2140623994: 5322 /* amountRatioLowLimit */ return new String[] { "Ratio" }; 5323 case -1424857166: 5324 /* amountType */ return new String[] { "CodeableConcept" }; 5325 case -896505829: 5326 /* source */ return new String[] { "Reference" }; 5327 default: 5328 return super.getTypesForProperty(hash, name); 5329 } 5330 5331 } 5332 5333 @Override 5334 public Base addChild(String name) throws FHIRException { 5335 if (name.equals("substanceReference")) { 5336 this.substance = new Reference(); 5337 return this.substance; 5338 } else if (name.equals("substanceCodeableConcept")) { 5339 this.substance = new CodeableConcept(); 5340 return this.substance; 5341 } else if (name.equals("relationship")) { 5342 this.relationship = new CodeableConcept(); 5343 return this.relationship; 5344 } else if (name.equals("isDefining")) { 5345 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.isDefining"); 5346 } else if (name.equals("amountQuantity")) { 5347 this.amount = new Quantity(); 5348 return this.amount; 5349 } else if (name.equals("amountRange")) { 5350 this.amount = new Range(); 5351 return this.amount; 5352 } else if (name.equals("amountRatio")) { 5353 this.amount = new Ratio(); 5354 return this.amount; 5355 } else if (name.equals("amountString")) { 5356 this.amount = new StringType(); 5357 return this.amount; 5358 } else if (name.equals("amountRatioLowLimit")) { 5359 this.amountRatioLowLimit = new Ratio(); 5360 return this.amountRatioLowLimit; 5361 } else if (name.equals("amountType")) { 5362 this.amountType = new CodeableConcept(); 5363 return this.amountType; 5364 } else if (name.equals("source")) { 5365 return addSource(); 5366 } else 5367 return super.addChild(name); 5368 } 5369 5370 public SubstanceSpecificationRelationshipComponent copy() { 5371 SubstanceSpecificationRelationshipComponent dst = new SubstanceSpecificationRelationshipComponent(); 5372 copyValues(dst); 5373 return dst; 5374 } 5375 5376 public void copyValues(SubstanceSpecificationRelationshipComponent dst) { 5377 super.copyValues(dst); 5378 dst.substance = substance == null ? null : substance.copy(); 5379 dst.relationship = relationship == null ? null : relationship.copy(); 5380 dst.isDefining = isDefining == null ? null : isDefining.copy(); 5381 dst.amount = amount == null ? null : amount.copy(); 5382 dst.amountRatioLowLimit = amountRatioLowLimit == null ? null : amountRatioLowLimit.copy(); 5383 dst.amountType = amountType == null ? null : amountType.copy(); 5384 if (source != null) { 5385 dst.source = new ArrayList<Reference>(); 5386 for (Reference i : source) 5387 dst.source.add(i.copy()); 5388 } 5389 ; 5390 } 5391 5392 @Override 5393 public boolean equalsDeep(Base other_) { 5394 if (!super.equalsDeep(other_)) 5395 return false; 5396 if (!(other_ instanceof SubstanceSpecificationRelationshipComponent)) 5397 return false; 5398 SubstanceSpecificationRelationshipComponent o = (SubstanceSpecificationRelationshipComponent) other_; 5399 return compareDeep(substance, o.substance, true) && compareDeep(relationship, o.relationship, true) 5400 && compareDeep(isDefining, o.isDefining, true) && compareDeep(amount, o.amount, true) 5401 && compareDeep(amountRatioLowLimit, o.amountRatioLowLimit, true) 5402 && compareDeep(amountType, o.amountType, true) && compareDeep(source, o.source, true); 5403 } 5404 5405 @Override 5406 public boolean equalsShallow(Base other_) { 5407 if (!super.equalsShallow(other_)) 5408 return false; 5409 if (!(other_ instanceof SubstanceSpecificationRelationshipComponent)) 5410 return false; 5411 SubstanceSpecificationRelationshipComponent o = (SubstanceSpecificationRelationshipComponent) other_; 5412 return compareValues(isDefining, o.isDefining, true); 5413 } 5414 5415 public boolean isEmpty() { 5416 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, relationship, isDefining, amount, 5417 amountRatioLowLimit, amountType, source); 5418 } 5419 5420 public String fhirType() { 5421 return "SubstanceSpecification.relationship"; 5422 5423 } 5424 5425 } 5426 5427 /** 5428 * Identifier by which this substance is known. 5429 */ 5430 @Child(name = "identifier", type = { 5431 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 5432 @Description(shortDefinition = "Identifier by which this substance is known", formalDefinition = "Identifier by which this substance is known.") 5433 protected Identifier identifier; 5434 5435 /** 5436 * High level categorization, e.g. polymer or nucleic acid. 5437 */ 5438 @Child(name = "type", type = { CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 5439 @Description(shortDefinition = "High level categorization, e.g. polymer or nucleic acid", formalDefinition = "High level categorization, e.g. polymer or nucleic acid.") 5440 protected CodeableConcept type; 5441 5442 /** 5443 * Status of substance within the catalogue e.g. approved. 5444 */ 5445 @Child(name = "status", type = { 5446 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 5447 @Description(shortDefinition = "Status of substance within the catalogue e.g. approved", formalDefinition = "Status of substance within the catalogue e.g. approved.") 5448 protected CodeableConcept status; 5449 5450 /** 5451 * If the substance applies to only human or veterinary use. 5452 */ 5453 @Child(name = "domain", type = { 5454 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 5455 @Description(shortDefinition = "If the substance applies to only human or veterinary use", formalDefinition = "If the substance applies to only human or veterinary use.") 5456 protected CodeableConcept domain; 5457 5458 /** 5459 * Textual description of the substance. 5460 */ 5461 @Child(name = "description", type = { 5462 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 5463 @Description(shortDefinition = "Textual description of the substance", formalDefinition = "Textual description of the substance.") 5464 protected StringType description; 5465 5466 /** 5467 * Supporting literature. 5468 */ 5469 @Child(name = "source", type = { 5470 DocumentReference.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5471 @Description(shortDefinition = "Supporting literature", formalDefinition = "Supporting literature.") 5472 protected List<Reference> source; 5473 /** 5474 * The actual objects that are the target of the reference (Supporting 5475 * literature.) 5476 */ 5477 protected List<DocumentReference> sourceTarget; 5478 5479 /** 5480 * Textual comment about this record of a substance. 5481 */ 5482 @Child(name = "comment", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 5483 @Description(shortDefinition = "Textual comment about this record of a substance", formalDefinition = "Textual comment about this record of a substance.") 5484 protected StringType comment; 5485 5486 /** 5487 * Moiety, for structural modifications. 5488 */ 5489 @Child(name = "moiety", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5490 @Description(shortDefinition = "Moiety, for structural modifications", formalDefinition = "Moiety, for structural modifications.") 5491 protected List<SubstanceSpecificationMoietyComponent> moiety; 5492 5493 /** 5494 * General specifications for this substance, including how it is related to 5495 * other substances. 5496 */ 5497 @Child(name = "property", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5498 @Description(shortDefinition = "General specifications for this substance, including how it is related to other substances", formalDefinition = "General specifications for this substance, including how it is related to other substances.") 5499 protected List<SubstanceSpecificationPropertyComponent> property; 5500 5501 /** 5502 * General information detailing this substance. 5503 */ 5504 @Child(name = "referenceInformation", type = { 5505 SubstanceReferenceInformation.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 5506 @Description(shortDefinition = "General information detailing this substance", formalDefinition = "General information detailing this substance.") 5507 protected Reference referenceInformation; 5508 5509 /** 5510 * The actual object that is the target of the reference (General information 5511 * detailing this substance.) 5512 */ 5513 protected SubstanceReferenceInformation referenceInformationTarget; 5514 5515 /** 5516 * Structural information. 5517 */ 5518 @Child(name = "structure", type = {}, order = 10, min = 0, max = 1, modifier = false, summary = true) 5519 @Description(shortDefinition = "Structural information", formalDefinition = "Structural information.") 5520 protected SubstanceSpecificationStructureComponent structure; 5521 5522 /** 5523 * Codes associated with the substance. 5524 */ 5525 @Child(name = "code", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5526 @Description(shortDefinition = "Codes associated with the substance", formalDefinition = "Codes associated with the substance.") 5527 protected List<SubstanceSpecificationCodeComponent> code; 5528 5529 /** 5530 * Names applicable to this substance. 5531 */ 5532 @Child(name = "name", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5533 @Description(shortDefinition = "Names applicable to this substance", formalDefinition = "Names applicable to this substance.") 5534 protected List<SubstanceSpecificationNameComponent> name; 5535 5536 /** 5537 * The molecular weight or weight range (for proteins, polymers or nucleic 5538 * acids). 5539 */ 5540 @Child(name = "molecularWeight", type = { 5541 SubstanceSpecificationStructureIsotopeMolecularWeightComponent.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5542 @Description(shortDefinition = "The molecular weight or weight range (for proteins, polymers or nucleic acids)", formalDefinition = "The molecular weight or weight range (for proteins, polymers or nucleic acids).") 5543 protected List<SubstanceSpecificationStructureIsotopeMolecularWeightComponent> molecularWeight; 5544 5545 /** 5546 * A link between this substance and another, with details of the relationship. 5547 */ 5548 @Child(name = "relationship", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5549 @Description(shortDefinition = "A link between this substance and another, with details of the relationship", formalDefinition = "A link between this substance and another, with details of the relationship.") 5550 protected List<SubstanceSpecificationRelationshipComponent> relationship; 5551 5552 /** 5553 * Data items specific to nucleic acids. 5554 */ 5555 @Child(name = "nucleicAcid", type = { 5556 SubstanceNucleicAcid.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 5557 @Description(shortDefinition = "Data items specific to nucleic acids", formalDefinition = "Data items specific to nucleic acids.") 5558 protected Reference nucleicAcid; 5559 5560 /** 5561 * The actual object that is the target of the reference (Data items specific to 5562 * nucleic acids.) 5563 */ 5564 protected SubstanceNucleicAcid nucleicAcidTarget; 5565 5566 /** 5567 * Data items specific to polymers. 5568 */ 5569 @Child(name = "polymer", type = { 5570 SubstancePolymer.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 5571 @Description(shortDefinition = "Data items specific to polymers", formalDefinition = "Data items specific to polymers.") 5572 protected Reference polymer; 5573 5574 /** 5575 * The actual object that is the target of the reference (Data items specific to 5576 * polymers.) 5577 */ 5578 protected SubstancePolymer polymerTarget; 5579 5580 /** 5581 * Data items specific to proteins. 5582 */ 5583 @Child(name = "protein", type = { 5584 SubstanceProtein.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 5585 @Description(shortDefinition = "Data items specific to proteins", formalDefinition = "Data items specific to proteins.") 5586 protected Reference protein; 5587 5588 /** 5589 * The actual object that is the target of the reference (Data items specific to 5590 * proteins.) 5591 */ 5592 protected SubstanceProtein proteinTarget; 5593 5594 /** 5595 * Material or taxonomic/anatomical source for the substance. 5596 */ 5597 @Child(name = "sourceMaterial", type = { 5598 SubstanceSourceMaterial.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 5599 @Description(shortDefinition = "Material or taxonomic/anatomical source for the substance", formalDefinition = "Material or taxonomic/anatomical source for the substance.") 5600 protected Reference sourceMaterial; 5601 5602 /** 5603 * The actual object that is the target of the reference (Material or 5604 * taxonomic/anatomical source for the substance.) 5605 */ 5606 protected SubstanceSourceMaterial sourceMaterialTarget; 5607 5608 private static final long serialVersionUID = 1782072718L; 5609 5610 /** 5611 * Constructor 5612 */ 5613 public SubstanceSpecification() { 5614 super(); 5615 } 5616 5617 /** 5618 * @return {@link #identifier} (Identifier by which this substance is known.) 5619 */ 5620 public Identifier getIdentifier() { 5621 if (this.identifier == null) 5622 if (Configuration.errorOnAutoCreate()) 5623 throw new Error("Attempt to auto-create SubstanceSpecification.identifier"); 5624 else if (Configuration.doAutoCreate()) 5625 this.identifier = new Identifier(); // cc 5626 return this.identifier; 5627 } 5628 5629 public boolean hasIdentifier() { 5630 return this.identifier != null && !this.identifier.isEmpty(); 5631 } 5632 5633 /** 5634 * @param value {@link #identifier} (Identifier by which this substance is 5635 * known.) 5636 */ 5637 public SubstanceSpecification setIdentifier(Identifier value) { 5638 this.identifier = value; 5639 return this; 5640 } 5641 5642 /** 5643 * @return {@link #type} (High level categorization, e.g. polymer or nucleic 5644 * acid.) 5645 */ 5646 public CodeableConcept getType() { 5647 if (this.type == null) 5648 if (Configuration.errorOnAutoCreate()) 5649 throw new Error("Attempt to auto-create SubstanceSpecification.type"); 5650 else if (Configuration.doAutoCreate()) 5651 this.type = new CodeableConcept(); // cc 5652 return this.type; 5653 } 5654 5655 public boolean hasType() { 5656 return this.type != null && !this.type.isEmpty(); 5657 } 5658 5659 /** 5660 * @param value {@link #type} (High level categorization, e.g. polymer or 5661 * nucleic acid.) 5662 */ 5663 public SubstanceSpecification setType(CodeableConcept value) { 5664 this.type = value; 5665 return this; 5666 } 5667 5668 /** 5669 * @return {@link #status} (Status of substance within the catalogue e.g. 5670 * approved.) 5671 */ 5672 public CodeableConcept getStatus() { 5673 if (this.status == null) 5674 if (Configuration.errorOnAutoCreate()) 5675 throw new Error("Attempt to auto-create SubstanceSpecification.status"); 5676 else if (Configuration.doAutoCreate()) 5677 this.status = new CodeableConcept(); // cc 5678 return this.status; 5679 } 5680 5681 public boolean hasStatus() { 5682 return this.status != null && !this.status.isEmpty(); 5683 } 5684 5685 /** 5686 * @param value {@link #status} (Status of substance within the catalogue e.g. 5687 * approved.) 5688 */ 5689 public SubstanceSpecification setStatus(CodeableConcept value) { 5690 this.status = value; 5691 return this; 5692 } 5693 5694 /** 5695 * @return {@link #domain} (If the substance applies to only human or veterinary 5696 * use.) 5697 */ 5698 public CodeableConcept getDomain() { 5699 if (this.domain == null) 5700 if (Configuration.errorOnAutoCreate()) 5701 throw new Error("Attempt to auto-create SubstanceSpecification.domain"); 5702 else if (Configuration.doAutoCreate()) 5703 this.domain = new CodeableConcept(); // cc 5704 return this.domain; 5705 } 5706 5707 public boolean hasDomain() { 5708 return this.domain != null && !this.domain.isEmpty(); 5709 } 5710 5711 /** 5712 * @param value {@link #domain} (If the substance applies to only human or 5713 * veterinary use.) 5714 */ 5715 public SubstanceSpecification setDomain(CodeableConcept value) { 5716 this.domain = value; 5717 return this; 5718 } 5719 5720 /** 5721 * @return {@link #description} (Textual description of the substance.). This is 5722 * the underlying object with id, value and extensions. The accessor 5723 * "getDescription" gives direct access to the value 5724 */ 5725 public StringType getDescriptionElement() { 5726 if (this.description == null) 5727 if (Configuration.errorOnAutoCreate()) 5728 throw new Error("Attempt to auto-create SubstanceSpecification.description"); 5729 else if (Configuration.doAutoCreate()) 5730 this.description = new StringType(); // bb 5731 return this.description; 5732 } 5733 5734 public boolean hasDescriptionElement() { 5735 return this.description != null && !this.description.isEmpty(); 5736 } 5737 5738 public boolean hasDescription() { 5739 return this.description != null && !this.description.isEmpty(); 5740 } 5741 5742 /** 5743 * @param value {@link #description} (Textual description of the substance.). 5744 * This is the underlying object with id, value and extensions. The 5745 * accessor "getDescription" gives direct access to the value 5746 */ 5747 public SubstanceSpecification setDescriptionElement(StringType value) { 5748 this.description = value; 5749 return this; 5750 } 5751 5752 /** 5753 * @return Textual description of the substance. 5754 */ 5755 public String getDescription() { 5756 return this.description == null ? null : this.description.getValue(); 5757 } 5758 5759 /** 5760 * @param value Textual description of the substance. 5761 */ 5762 public SubstanceSpecification setDescription(String value) { 5763 if (Utilities.noString(value)) 5764 this.description = null; 5765 else { 5766 if (this.description == null) 5767 this.description = new StringType(); 5768 this.description.setValue(value); 5769 } 5770 return this; 5771 } 5772 5773 /** 5774 * @return {@link #source} (Supporting literature.) 5775 */ 5776 public List<Reference> getSource() { 5777 if (this.source == null) 5778 this.source = new ArrayList<Reference>(); 5779 return this.source; 5780 } 5781 5782 /** 5783 * @return Returns a reference to <code>this</code> for easy method chaining 5784 */ 5785 public SubstanceSpecification setSource(List<Reference> theSource) { 5786 this.source = theSource; 5787 return this; 5788 } 5789 5790 public boolean hasSource() { 5791 if (this.source == null) 5792 return false; 5793 for (Reference item : this.source) 5794 if (!item.isEmpty()) 5795 return true; 5796 return false; 5797 } 5798 5799 public Reference addSource() { // 3 5800 Reference t = new Reference(); 5801 if (this.source == null) 5802 this.source = new ArrayList<Reference>(); 5803 this.source.add(t); 5804 return t; 5805 } 5806 5807 public SubstanceSpecification addSource(Reference t) { // 3 5808 if (t == null) 5809 return this; 5810 if (this.source == null) 5811 this.source = new ArrayList<Reference>(); 5812 this.source.add(t); 5813 return this; 5814 } 5815 5816 /** 5817 * @return The first repetition of repeating field {@link #source}, creating it 5818 * if it does not already exist 5819 */ 5820 public Reference getSourceFirstRep() { 5821 if (getSource().isEmpty()) { 5822 addSource(); 5823 } 5824 return getSource().get(0); 5825 } 5826 5827 /** 5828 * @deprecated Use Reference#setResource(IBaseResource) instead 5829 */ 5830 @Deprecated 5831 public List<DocumentReference> getSourceTarget() { 5832 if (this.sourceTarget == null) 5833 this.sourceTarget = new ArrayList<DocumentReference>(); 5834 return this.sourceTarget; 5835 } 5836 5837 /** 5838 * @deprecated Use Reference#setResource(IBaseResource) instead 5839 */ 5840 @Deprecated 5841 public DocumentReference addSourceTarget() { 5842 DocumentReference r = new DocumentReference(); 5843 if (this.sourceTarget == null) 5844 this.sourceTarget = new ArrayList<DocumentReference>(); 5845 this.sourceTarget.add(r); 5846 return r; 5847 } 5848 5849 /** 5850 * @return {@link #comment} (Textual comment about this record of a substance.). 5851 * This is the underlying object with id, value and extensions. The 5852 * accessor "getComment" gives direct access to the value 5853 */ 5854 public StringType getCommentElement() { 5855 if (this.comment == null) 5856 if (Configuration.errorOnAutoCreate()) 5857 throw new Error("Attempt to auto-create SubstanceSpecification.comment"); 5858 else if (Configuration.doAutoCreate()) 5859 this.comment = new StringType(); // bb 5860 return this.comment; 5861 } 5862 5863 public boolean hasCommentElement() { 5864 return this.comment != null && !this.comment.isEmpty(); 5865 } 5866 5867 public boolean hasComment() { 5868 return this.comment != null && !this.comment.isEmpty(); 5869 } 5870 5871 /** 5872 * @param value {@link #comment} (Textual comment about this record of a 5873 * substance.). This is the underlying object with id, value and 5874 * extensions. The accessor "getComment" gives direct access to the 5875 * value 5876 */ 5877 public SubstanceSpecification setCommentElement(StringType value) { 5878 this.comment = value; 5879 return this; 5880 } 5881 5882 /** 5883 * @return Textual comment about this record of a substance. 5884 */ 5885 public String getComment() { 5886 return this.comment == null ? null : this.comment.getValue(); 5887 } 5888 5889 /** 5890 * @param value Textual comment about this record of a substance. 5891 */ 5892 public SubstanceSpecification setComment(String value) { 5893 if (Utilities.noString(value)) 5894 this.comment = null; 5895 else { 5896 if (this.comment == null) 5897 this.comment = new StringType(); 5898 this.comment.setValue(value); 5899 } 5900 return this; 5901 } 5902 5903 /** 5904 * @return {@link #moiety} (Moiety, for structural modifications.) 5905 */ 5906 public List<SubstanceSpecificationMoietyComponent> getMoiety() { 5907 if (this.moiety == null) 5908 this.moiety = new ArrayList<SubstanceSpecificationMoietyComponent>(); 5909 return this.moiety; 5910 } 5911 5912 /** 5913 * @return Returns a reference to <code>this</code> for easy method chaining 5914 */ 5915 public SubstanceSpecification setMoiety(List<SubstanceSpecificationMoietyComponent> theMoiety) { 5916 this.moiety = theMoiety; 5917 return this; 5918 } 5919 5920 public boolean hasMoiety() { 5921 if (this.moiety == null) 5922 return false; 5923 for (SubstanceSpecificationMoietyComponent item : this.moiety) 5924 if (!item.isEmpty()) 5925 return true; 5926 return false; 5927 } 5928 5929 public SubstanceSpecificationMoietyComponent addMoiety() { // 3 5930 SubstanceSpecificationMoietyComponent t = new SubstanceSpecificationMoietyComponent(); 5931 if (this.moiety == null) 5932 this.moiety = new ArrayList<SubstanceSpecificationMoietyComponent>(); 5933 this.moiety.add(t); 5934 return t; 5935 } 5936 5937 public SubstanceSpecification addMoiety(SubstanceSpecificationMoietyComponent t) { // 3 5938 if (t == null) 5939 return this; 5940 if (this.moiety == null) 5941 this.moiety = new ArrayList<SubstanceSpecificationMoietyComponent>(); 5942 this.moiety.add(t); 5943 return this; 5944 } 5945 5946 /** 5947 * @return The first repetition of repeating field {@link #moiety}, creating it 5948 * if it does not already exist 5949 */ 5950 public SubstanceSpecificationMoietyComponent getMoietyFirstRep() { 5951 if (getMoiety().isEmpty()) { 5952 addMoiety(); 5953 } 5954 return getMoiety().get(0); 5955 } 5956 5957 /** 5958 * @return {@link #property} (General specifications for this substance, 5959 * including how it is related to other substances.) 5960 */ 5961 public List<SubstanceSpecificationPropertyComponent> getProperty() { 5962 if (this.property == null) 5963 this.property = new ArrayList<SubstanceSpecificationPropertyComponent>(); 5964 return this.property; 5965 } 5966 5967 /** 5968 * @return Returns a reference to <code>this</code> for easy method chaining 5969 */ 5970 public SubstanceSpecification setProperty(List<SubstanceSpecificationPropertyComponent> theProperty) { 5971 this.property = theProperty; 5972 return this; 5973 } 5974 5975 public boolean hasProperty() { 5976 if (this.property == null) 5977 return false; 5978 for (SubstanceSpecificationPropertyComponent item : this.property) 5979 if (!item.isEmpty()) 5980 return true; 5981 return false; 5982 } 5983 5984 public SubstanceSpecificationPropertyComponent addProperty() { // 3 5985 SubstanceSpecificationPropertyComponent t = new SubstanceSpecificationPropertyComponent(); 5986 if (this.property == null) 5987 this.property = new ArrayList<SubstanceSpecificationPropertyComponent>(); 5988 this.property.add(t); 5989 return t; 5990 } 5991 5992 public SubstanceSpecification addProperty(SubstanceSpecificationPropertyComponent t) { // 3 5993 if (t == null) 5994 return this; 5995 if (this.property == null) 5996 this.property = new ArrayList<SubstanceSpecificationPropertyComponent>(); 5997 this.property.add(t); 5998 return this; 5999 } 6000 6001 /** 6002 * @return The first repetition of repeating field {@link #property}, creating 6003 * it if it does not already exist 6004 */ 6005 public SubstanceSpecificationPropertyComponent getPropertyFirstRep() { 6006 if (getProperty().isEmpty()) { 6007 addProperty(); 6008 } 6009 return getProperty().get(0); 6010 } 6011 6012 /** 6013 * @return {@link #referenceInformation} (General information detailing this 6014 * substance.) 6015 */ 6016 public Reference getReferenceInformation() { 6017 if (this.referenceInformation == null) 6018 if (Configuration.errorOnAutoCreate()) 6019 throw new Error("Attempt to auto-create SubstanceSpecification.referenceInformation"); 6020 else if (Configuration.doAutoCreate()) 6021 this.referenceInformation = new Reference(); // cc 6022 return this.referenceInformation; 6023 } 6024 6025 public boolean hasReferenceInformation() { 6026 return this.referenceInformation != null && !this.referenceInformation.isEmpty(); 6027 } 6028 6029 /** 6030 * @param value {@link #referenceInformation} (General information detailing 6031 * this substance.) 6032 */ 6033 public SubstanceSpecification setReferenceInformation(Reference value) { 6034 this.referenceInformation = value; 6035 return this; 6036 } 6037 6038 /** 6039 * @return {@link #referenceInformation} The actual object that is the target of 6040 * the reference. The reference library doesn't populate this, but you 6041 * can use it to hold the resource if you resolve it. (General 6042 * information detailing this substance.) 6043 */ 6044 public SubstanceReferenceInformation getReferenceInformationTarget() { 6045 if (this.referenceInformationTarget == null) 6046 if (Configuration.errorOnAutoCreate()) 6047 throw new Error("Attempt to auto-create SubstanceSpecification.referenceInformation"); 6048 else if (Configuration.doAutoCreate()) 6049 this.referenceInformationTarget = new SubstanceReferenceInformation(); // aa 6050 return this.referenceInformationTarget; 6051 } 6052 6053 /** 6054 * @param value {@link #referenceInformation} The actual object that is the 6055 * target of the reference. The reference library doesn't use 6056 * these, but you can use it to hold the resource if you resolve 6057 * it. (General information detailing this substance.) 6058 */ 6059 public SubstanceSpecification setReferenceInformationTarget(SubstanceReferenceInformation value) { 6060 this.referenceInformationTarget = value; 6061 return this; 6062 } 6063 6064 /** 6065 * @return {@link #structure} (Structural information.) 6066 */ 6067 public SubstanceSpecificationStructureComponent getStructure() { 6068 if (this.structure == null) 6069 if (Configuration.errorOnAutoCreate()) 6070 throw new Error("Attempt to auto-create SubstanceSpecification.structure"); 6071 else if (Configuration.doAutoCreate()) 6072 this.structure = new SubstanceSpecificationStructureComponent(); // cc 6073 return this.structure; 6074 } 6075 6076 public boolean hasStructure() { 6077 return this.structure != null && !this.structure.isEmpty(); 6078 } 6079 6080 /** 6081 * @param value {@link #structure} (Structural information.) 6082 */ 6083 public SubstanceSpecification setStructure(SubstanceSpecificationStructureComponent value) { 6084 this.structure = value; 6085 return this; 6086 } 6087 6088 /** 6089 * @return {@link #code} (Codes associated with the substance.) 6090 */ 6091 public List<SubstanceSpecificationCodeComponent> getCode() { 6092 if (this.code == null) 6093 this.code = new ArrayList<SubstanceSpecificationCodeComponent>(); 6094 return this.code; 6095 } 6096 6097 /** 6098 * @return Returns a reference to <code>this</code> for easy method chaining 6099 */ 6100 public SubstanceSpecification setCode(List<SubstanceSpecificationCodeComponent> theCode) { 6101 this.code = theCode; 6102 return this; 6103 } 6104 6105 public boolean hasCode() { 6106 if (this.code == null) 6107 return false; 6108 for (SubstanceSpecificationCodeComponent item : this.code) 6109 if (!item.isEmpty()) 6110 return true; 6111 return false; 6112 } 6113 6114 public SubstanceSpecificationCodeComponent addCode() { // 3 6115 SubstanceSpecificationCodeComponent t = new SubstanceSpecificationCodeComponent(); 6116 if (this.code == null) 6117 this.code = new ArrayList<SubstanceSpecificationCodeComponent>(); 6118 this.code.add(t); 6119 return t; 6120 } 6121 6122 public SubstanceSpecification addCode(SubstanceSpecificationCodeComponent t) { // 3 6123 if (t == null) 6124 return this; 6125 if (this.code == null) 6126 this.code = new ArrayList<SubstanceSpecificationCodeComponent>(); 6127 this.code.add(t); 6128 return this; 6129 } 6130 6131 /** 6132 * @return The first repetition of repeating field {@link #code}, creating it if 6133 * it does not already exist 6134 */ 6135 public SubstanceSpecificationCodeComponent getCodeFirstRep() { 6136 if (getCode().isEmpty()) { 6137 addCode(); 6138 } 6139 return getCode().get(0); 6140 } 6141 6142 /** 6143 * @return {@link #name} (Names applicable to this substance.) 6144 */ 6145 public List<SubstanceSpecificationNameComponent> getName() { 6146 if (this.name == null) 6147 this.name = new ArrayList<SubstanceSpecificationNameComponent>(); 6148 return this.name; 6149 } 6150 6151 /** 6152 * @return Returns a reference to <code>this</code> for easy method chaining 6153 */ 6154 public SubstanceSpecification setName(List<SubstanceSpecificationNameComponent> theName) { 6155 this.name = theName; 6156 return this; 6157 } 6158 6159 public boolean hasName() { 6160 if (this.name == null) 6161 return false; 6162 for (SubstanceSpecificationNameComponent item : this.name) 6163 if (!item.isEmpty()) 6164 return true; 6165 return false; 6166 } 6167 6168 public SubstanceSpecificationNameComponent addName() { // 3 6169 SubstanceSpecificationNameComponent t = new SubstanceSpecificationNameComponent(); 6170 if (this.name == null) 6171 this.name = new ArrayList<SubstanceSpecificationNameComponent>(); 6172 this.name.add(t); 6173 return t; 6174 } 6175 6176 public SubstanceSpecification addName(SubstanceSpecificationNameComponent t) { // 3 6177 if (t == null) 6178 return this; 6179 if (this.name == null) 6180 this.name = new ArrayList<SubstanceSpecificationNameComponent>(); 6181 this.name.add(t); 6182 return this; 6183 } 6184 6185 /** 6186 * @return The first repetition of repeating field {@link #name}, creating it if 6187 * it does not already exist 6188 */ 6189 public SubstanceSpecificationNameComponent getNameFirstRep() { 6190 if (getName().isEmpty()) { 6191 addName(); 6192 } 6193 return getName().get(0); 6194 } 6195 6196 /** 6197 * @return {@link #molecularWeight} (The molecular weight or weight range (for 6198 * proteins, polymers or nucleic acids).) 6199 */ 6200 public List<SubstanceSpecificationStructureIsotopeMolecularWeightComponent> getMolecularWeight() { 6201 if (this.molecularWeight == null) 6202 this.molecularWeight = new ArrayList<SubstanceSpecificationStructureIsotopeMolecularWeightComponent>(); 6203 return this.molecularWeight; 6204 } 6205 6206 /** 6207 * @return Returns a reference to <code>this</code> for easy method chaining 6208 */ 6209 public SubstanceSpecification setMolecularWeight( 6210 List<SubstanceSpecificationStructureIsotopeMolecularWeightComponent> theMolecularWeight) { 6211 this.molecularWeight = theMolecularWeight; 6212 return this; 6213 } 6214 6215 public boolean hasMolecularWeight() { 6216 if (this.molecularWeight == null) 6217 return false; 6218 for (SubstanceSpecificationStructureIsotopeMolecularWeightComponent item : this.molecularWeight) 6219 if (!item.isEmpty()) 6220 return true; 6221 return false; 6222 } 6223 6224 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent addMolecularWeight() { // 3 6225 SubstanceSpecificationStructureIsotopeMolecularWeightComponent t = new SubstanceSpecificationStructureIsotopeMolecularWeightComponent(); 6226 if (this.molecularWeight == null) 6227 this.molecularWeight = new ArrayList<SubstanceSpecificationStructureIsotopeMolecularWeightComponent>(); 6228 this.molecularWeight.add(t); 6229 return t; 6230 } 6231 6232 public SubstanceSpecification addMolecularWeight(SubstanceSpecificationStructureIsotopeMolecularWeightComponent t) { // 3 6233 if (t == null) 6234 return this; 6235 if (this.molecularWeight == null) 6236 this.molecularWeight = new ArrayList<SubstanceSpecificationStructureIsotopeMolecularWeightComponent>(); 6237 this.molecularWeight.add(t); 6238 return this; 6239 } 6240 6241 /** 6242 * @return The first repetition of repeating field {@link #molecularWeight}, 6243 * creating it if it does not already exist 6244 */ 6245 public SubstanceSpecificationStructureIsotopeMolecularWeightComponent getMolecularWeightFirstRep() { 6246 if (getMolecularWeight().isEmpty()) { 6247 addMolecularWeight(); 6248 } 6249 return getMolecularWeight().get(0); 6250 } 6251 6252 /** 6253 * @return {@link #relationship} (A link between this substance and another, 6254 * with details of the relationship.) 6255 */ 6256 public List<SubstanceSpecificationRelationshipComponent> getRelationship() { 6257 if (this.relationship == null) 6258 this.relationship = new ArrayList<SubstanceSpecificationRelationshipComponent>(); 6259 return this.relationship; 6260 } 6261 6262 /** 6263 * @return Returns a reference to <code>this</code> for easy method chaining 6264 */ 6265 public SubstanceSpecification setRelationship(List<SubstanceSpecificationRelationshipComponent> theRelationship) { 6266 this.relationship = theRelationship; 6267 return this; 6268 } 6269 6270 public boolean hasRelationship() { 6271 if (this.relationship == null) 6272 return false; 6273 for (SubstanceSpecificationRelationshipComponent item : this.relationship) 6274 if (!item.isEmpty()) 6275 return true; 6276 return false; 6277 } 6278 6279 public SubstanceSpecificationRelationshipComponent addRelationship() { // 3 6280 SubstanceSpecificationRelationshipComponent t = new SubstanceSpecificationRelationshipComponent(); 6281 if (this.relationship == null) 6282 this.relationship = new ArrayList<SubstanceSpecificationRelationshipComponent>(); 6283 this.relationship.add(t); 6284 return t; 6285 } 6286 6287 public SubstanceSpecification addRelationship(SubstanceSpecificationRelationshipComponent t) { // 3 6288 if (t == null) 6289 return this; 6290 if (this.relationship == null) 6291 this.relationship = new ArrayList<SubstanceSpecificationRelationshipComponent>(); 6292 this.relationship.add(t); 6293 return this; 6294 } 6295 6296 /** 6297 * @return The first repetition of repeating field {@link #relationship}, 6298 * creating it if it does not already exist 6299 */ 6300 public SubstanceSpecificationRelationshipComponent getRelationshipFirstRep() { 6301 if (getRelationship().isEmpty()) { 6302 addRelationship(); 6303 } 6304 return getRelationship().get(0); 6305 } 6306 6307 /** 6308 * @return {@link #nucleicAcid} (Data items specific to nucleic acids.) 6309 */ 6310 public Reference getNucleicAcid() { 6311 if (this.nucleicAcid == null) 6312 if (Configuration.errorOnAutoCreate()) 6313 throw new Error("Attempt to auto-create SubstanceSpecification.nucleicAcid"); 6314 else if (Configuration.doAutoCreate()) 6315 this.nucleicAcid = new Reference(); // cc 6316 return this.nucleicAcid; 6317 } 6318 6319 public boolean hasNucleicAcid() { 6320 return this.nucleicAcid != null && !this.nucleicAcid.isEmpty(); 6321 } 6322 6323 /** 6324 * @param value {@link #nucleicAcid} (Data items specific to nucleic acids.) 6325 */ 6326 public SubstanceSpecification setNucleicAcid(Reference value) { 6327 this.nucleicAcid = value; 6328 return this; 6329 } 6330 6331 /** 6332 * @return {@link #nucleicAcid} The actual object that is the target of the 6333 * reference. The reference library doesn't populate this, but you can 6334 * use it to hold the resource if you resolve it. (Data items specific 6335 * to nucleic acids.) 6336 */ 6337 public SubstanceNucleicAcid getNucleicAcidTarget() { 6338 if (this.nucleicAcidTarget == null) 6339 if (Configuration.errorOnAutoCreate()) 6340 throw new Error("Attempt to auto-create SubstanceSpecification.nucleicAcid"); 6341 else if (Configuration.doAutoCreate()) 6342 this.nucleicAcidTarget = new SubstanceNucleicAcid(); // aa 6343 return this.nucleicAcidTarget; 6344 } 6345 6346 /** 6347 * @param value {@link #nucleicAcid} The actual object that is the target of the 6348 * reference. The reference library doesn't use these, but you can 6349 * use it to hold the resource if you resolve it. (Data items 6350 * specific to nucleic acids.) 6351 */ 6352 public SubstanceSpecification setNucleicAcidTarget(SubstanceNucleicAcid value) { 6353 this.nucleicAcidTarget = value; 6354 return this; 6355 } 6356 6357 /** 6358 * @return {@link #polymer} (Data items specific to polymers.) 6359 */ 6360 public Reference getPolymer() { 6361 if (this.polymer == null) 6362 if (Configuration.errorOnAutoCreate()) 6363 throw new Error("Attempt to auto-create SubstanceSpecification.polymer"); 6364 else if (Configuration.doAutoCreate()) 6365 this.polymer = new Reference(); // cc 6366 return this.polymer; 6367 } 6368 6369 public boolean hasPolymer() { 6370 return this.polymer != null && !this.polymer.isEmpty(); 6371 } 6372 6373 /** 6374 * @param value {@link #polymer} (Data items specific to polymers.) 6375 */ 6376 public SubstanceSpecification setPolymer(Reference value) { 6377 this.polymer = value; 6378 return this; 6379 } 6380 6381 /** 6382 * @return {@link #polymer} The actual object that is the target of the 6383 * reference. The reference library doesn't populate this, but you can 6384 * use it to hold the resource if you resolve it. (Data items specific 6385 * to polymers.) 6386 */ 6387 public SubstancePolymer getPolymerTarget() { 6388 if (this.polymerTarget == null) 6389 if (Configuration.errorOnAutoCreate()) 6390 throw new Error("Attempt to auto-create SubstanceSpecification.polymer"); 6391 else if (Configuration.doAutoCreate()) 6392 this.polymerTarget = new SubstancePolymer(); // aa 6393 return this.polymerTarget; 6394 } 6395 6396 /** 6397 * @param value {@link #polymer} The actual object that is the target of the 6398 * reference. The reference library doesn't use these, but you can 6399 * use it to hold the resource if you resolve it. (Data items 6400 * specific to polymers.) 6401 */ 6402 public SubstanceSpecification setPolymerTarget(SubstancePolymer value) { 6403 this.polymerTarget = value; 6404 return this; 6405 } 6406 6407 /** 6408 * @return {@link #protein} (Data items specific to proteins.) 6409 */ 6410 public Reference getProtein() { 6411 if (this.protein == null) 6412 if (Configuration.errorOnAutoCreate()) 6413 throw new Error("Attempt to auto-create SubstanceSpecification.protein"); 6414 else if (Configuration.doAutoCreate()) 6415 this.protein = new Reference(); // cc 6416 return this.protein; 6417 } 6418 6419 public boolean hasProtein() { 6420 return this.protein != null && !this.protein.isEmpty(); 6421 } 6422 6423 /** 6424 * @param value {@link #protein} (Data items specific to proteins.) 6425 */ 6426 public SubstanceSpecification setProtein(Reference value) { 6427 this.protein = value; 6428 return this; 6429 } 6430 6431 /** 6432 * @return {@link #protein} The actual object that is the target of the 6433 * reference. The reference library doesn't populate this, but you can 6434 * use it to hold the resource if you resolve it. (Data items specific 6435 * to proteins.) 6436 */ 6437 public SubstanceProtein getProteinTarget() { 6438 if (this.proteinTarget == null) 6439 if (Configuration.errorOnAutoCreate()) 6440 throw new Error("Attempt to auto-create SubstanceSpecification.protein"); 6441 else if (Configuration.doAutoCreate()) 6442 this.proteinTarget = new SubstanceProtein(); // aa 6443 return this.proteinTarget; 6444 } 6445 6446 /** 6447 * @param value {@link #protein} The actual object that is the target of the 6448 * reference. The reference library doesn't use these, but you can 6449 * use it to hold the resource if you resolve it. (Data items 6450 * specific to proteins.) 6451 */ 6452 public SubstanceSpecification setProteinTarget(SubstanceProtein value) { 6453 this.proteinTarget = value; 6454 return this; 6455 } 6456 6457 /** 6458 * @return {@link #sourceMaterial} (Material or taxonomic/anatomical source for 6459 * the substance.) 6460 */ 6461 public Reference getSourceMaterial() { 6462 if (this.sourceMaterial == null) 6463 if (Configuration.errorOnAutoCreate()) 6464 throw new Error("Attempt to auto-create SubstanceSpecification.sourceMaterial"); 6465 else if (Configuration.doAutoCreate()) 6466 this.sourceMaterial = new Reference(); // cc 6467 return this.sourceMaterial; 6468 } 6469 6470 public boolean hasSourceMaterial() { 6471 return this.sourceMaterial != null && !this.sourceMaterial.isEmpty(); 6472 } 6473 6474 /** 6475 * @param value {@link #sourceMaterial} (Material or taxonomic/anatomical source 6476 * for the substance.) 6477 */ 6478 public SubstanceSpecification setSourceMaterial(Reference value) { 6479 this.sourceMaterial = value; 6480 return this; 6481 } 6482 6483 /** 6484 * @return {@link #sourceMaterial} The actual object that is the target of the 6485 * reference. The reference library doesn't populate this, but you can 6486 * use it to hold the resource if you resolve it. (Material or 6487 * taxonomic/anatomical source for the substance.) 6488 */ 6489 public SubstanceSourceMaterial getSourceMaterialTarget() { 6490 if (this.sourceMaterialTarget == null) 6491 if (Configuration.errorOnAutoCreate()) 6492 throw new Error("Attempt to auto-create SubstanceSpecification.sourceMaterial"); 6493 else if (Configuration.doAutoCreate()) 6494 this.sourceMaterialTarget = new SubstanceSourceMaterial(); // aa 6495 return this.sourceMaterialTarget; 6496 } 6497 6498 /** 6499 * @param value {@link #sourceMaterial} The actual object that is the target of 6500 * the reference. The reference library doesn't use these, but you 6501 * can use it to hold the resource if you resolve it. (Material or 6502 * taxonomic/anatomical source for the substance.) 6503 */ 6504 public SubstanceSpecification setSourceMaterialTarget(SubstanceSourceMaterial value) { 6505 this.sourceMaterialTarget = value; 6506 return this; 6507 } 6508 6509 protected void listChildren(List<Property> children) { 6510 super.listChildren(children); 6511 children.add( 6512 new Property("identifier", "Identifier", "Identifier by which this substance is known.", 0, 1, identifier)); 6513 children.add(new Property("type", "CodeableConcept", "High level categorization, e.g. polymer or nucleic acid.", 0, 6514 1, type)); 6515 children.add(new Property("status", "CodeableConcept", "Status of substance within the catalogue e.g. approved.", 0, 6516 1, status)); 6517 children.add(new Property("domain", "CodeableConcept", "If the substance applies to only human or veterinary use.", 6518 0, 1, domain)); 6519 children.add(new Property("description", "string", "Textual description of the substance.", 0, 1, description)); 6520 children.add(new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 6521 java.lang.Integer.MAX_VALUE, source)); 6522 children.add(new Property("comment", "string", "Textual comment about this record of a substance.", 0, 1, comment)); 6523 children.add( 6524 new Property("moiety", "", "Moiety, for structural modifications.", 0, java.lang.Integer.MAX_VALUE, moiety)); 6525 children.add(new Property("property", "", 6526 "General specifications for this substance, including how it is related to other substances.", 0, 6527 java.lang.Integer.MAX_VALUE, property)); 6528 children.add(new Property("referenceInformation", "Reference(SubstanceReferenceInformation)", 6529 "General information detailing this substance.", 0, 1, referenceInformation)); 6530 children.add(new Property("structure", "", "Structural information.", 0, 1, structure)); 6531 children 6532 .add(new Property("code", "", "Codes associated with the substance.", 0, java.lang.Integer.MAX_VALUE, code)); 6533 children.add(new Property("name", "", "Names applicable to this substance.", 0, java.lang.Integer.MAX_VALUE, name)); 6534 children.add(new Property("molecularWeight", "@SubstanceSpecification.structure.isotope.molecularWeight", 6535 "The molecular weight or weight range (for proteins, polymers or nucleic acids).", 0, 6536 java.lang.Integer.MAX_VALUE, molecularWeight)); 6537 children.add( 6538 new Property("relationship", "", "A link between this substance and another, with details of the relationship.", 6539 0, java.lang.Integer.MAX_VALUE, relationship)); 6540 children.add(new Property("nucleicAcid", "Reference(SubstanceNucleicAcid)", "Data items specific to nucleic acids.", 6541 0, 1, nucleicAcid)); 6542 children 6543 .add(new Property("polymer", "Reference(SubstancePolymer)", "Data items specific to polymers.", 0, 1, polymer)); 6544 children 6545 .add(new Property("protein", "Reference(SubstanceProtein)", "Data items specific to proteins.", 0, 1, protein)); 6546 children.add(new Property("sourceMaterial", "Reference(SubstanceSourceMaterial)", 6547 "Material or taxonomic/anatomical source for the substance.", 0, 1, sourceMaterial)); 6548 } 6549 6550 @Override 6551 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6552 switch (_hash) { 6553 case -1618432855: 6554 /* identifier */ return new Property("identifier", "Identifier", "Identifier by which this substance is known.", 6555 0, 1, identifier); 6556 case 3575610: 6557 /* type */ return new Property("type", "CodeableConcept", 6558 "High level categorization, e.g. polymer or nucleic acid.", 0, 1, type); 6559 case -892481550: 6560 /* status */ return new Property("status", "CodeableConcept", 6561 "Status of substance within the catalogue e.g. approved.", 0, 1, status); 6562 case -1326197564: 6563 /* domain */ return new Property("domain", "CodeableConcept", 6564 "If the substance applies to only human or veterinary use.", 0, 1, domain); 6565 case -1724546052: 6566 /* description */ return new Property("description", "string", "Textual description of the substance.", 0, 1, 6567 description); 6568 case -896505829: 6569 /* source */ return new Property("source", "Reference(DocumentReference)", "Supporting literature.", 0, 6570 java.lang.Integer.MAX_VALUE, source); 6571 case 950398559: 6572 /* comment */ return new Property("comment", "string", "Textual comment about this record of a substance.", 0, 1, 6573 comment); 6574 case -1068650173: 6575 /* moiety */ return new Property("moiety", "", "Moiety, for structural modifications.", 0, 6576 java.lang.Integer.MAX_VALUE, moiety); 6577 case -993141291: 6578 /* property */ return new Property("property", "", 6579 "General specifications for this substance, including how it is related to other substances.", 0, 6580 java.lang.Integer.MAX_VALUE, property); 6581 case -2117930783: 6582 /* referenceInformation */ return new Property("referenceInformation", "Reference(SubstanceReferenceInformation)", 6583 "General information detailing this substance.", 0, 1, referenceInformation); 6584 case 144518515: 6585 /* structure */ return new Property("structure", "", "Structural information.", 0, 1, structure); 6586 case 3059181: 6587 /* code */ return new Property("code", "", "Codes associated with the substance.", 0, java.lang.Integer.MAX_VALUE, 6588 code); 6589 case 3373707: 6590 /* name */ return new Property("name", "", "Names applicable to this substance.", 0, java.lang.Integer.MAX_VALUE, 6591 name); 6592 case 635625672: 6593 /* molecularWeight */ return new Property("molecularWeight", 6594 "@SubstanceSpecification.structure.isotope.molecularWeight", 6595 "The molecular weight or weight range (for proteins, polymers or nucleic acids).", 0, 6596 java.lang.Integer.MAX_VALUE, molecularWeight); 6597 case -261851592: 6598 /* relationship */ return new Property("relationship", "", 6599 "A link between this substance and another, with details of the relationship.", 0, 6600 java.lang.Integer.MAX_VALUE, relationship); 6601 case 1625275180: 6602 /* nucleicAcid */ return new Property("nucleicAcid", "Reference(SubstanceNucleicAcid)", 6603 "Data items specific to nucleic acids.", 0, 1, nucleicAcid); 6604 case -397514098: 6605 /* polymer */ return new Property("polymer", "Reference(SubstancePolymer)", "Data items specific to polymers.", 0, 6606 1, polymer); 6607 case -309012605: 6608 /* protein */ return new Property("protein", "Reference(SubstanceProtein)", "Data items specific to proteins.", 0, 6609 1, protein); 6610 case -1064442270: 6611 /* sourceMaterial */ return new Property("sourceMaterial", "Reference(SubstanceSourceMaterial)", 6612 "Material or taxonomic/anatomical source for the substance.", 0, 1, sourceMaterial); 6613 default: 6614 return super.getNamedProperty(_hash, _name, _checkValid); 6615 } 6616 6617 } 6618 6619 @Override 6620 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6621 switch (hash) { 6622 case -1618432855: 6623 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 6624 case 3575610: 6625 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 6626 case -892481550: 6627 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 6628 case -1326197564: 6629 /* domain */ return this.domain == null ? new Base[0] : new Base[] { this.domain }; // CodeableConcept 6630 case -1724546052: 6631 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 6632 case -896505829: 6633 /* source */ return this.source == null ? new Base[0] : this.source.toArray(new Base[this.source.size()]); // Reference 6634 case 950398559: 6635 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 6636 case -1068650173: 6637 /* moiety */ return this.moiety == null ? new Base[0] : this.moiety.toArray(new Base[this.moiety.size()]); // SubstanceSpecificationMoietyComponent 6638 case -993141291: 6639 /* property */ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // SubstanceSpecificationPropertyComponent 6640 case -2117930783: 6641 /* referenceInformation */ return this.referenceInformation == null ? new Base[0] 6642 : new Base[] { this.referenceInformation }; // Reference 6643 case 144518515: 6644 /* structure */ return this.structure == null ? new Base[0] : new Base[] { this.structure }; // SubstanceSpecificationStructureComponent 6645 case 3059181: 6646 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // SubstanceSpecificationCodeComponent 6647 case 3373707: 6648 /* name */ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // SubstanceSpecificationNameComponent 6649 case 635625672: 6650 /* molecularWeight */ return this.molecularWeight == null ? new Base[0] 6651 : this.molecularWeight.toArray(new Base[this.molecularWeight.size()]); // SubstanceSpecificationStructureIsotopeMolecularWeightComponent 6652 case -261851592: 6653 /* relationship */ return this.relationship == null ? new Base[0] 6654 : this.relationship.toArray(new Base[this.relationship.size()]); // SubstanceSpecificationRelationshipComponent 6655 case 1625275180: 6656 /* nucleicAcid */ return this.nucleicAcid == null ? new Base[0] : new Base[] { this.nucleicAcid }; // Reference 6657 case -397514098: 6658 /* polymer */ return this.polymer == null ? new Base[0] : new Base[] { this.polymer }; // Reference 6659 case -309012605: 6660 /* protein */ return this.protein == null ? new Base[0] : new Base[] { this.protein }; // Reference 6661 case -1064442270: 6662 /* sourceMaterial */ return this.sourceMaterial == null ? new Base[0] : new Base[] { this.sourceMaterial }; // Reference 6663 default: 6664 return super.getProperty(hash, name, checkValid); 6665 } 6666 6667 } 6668 6669 @Override 6670 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6671 switch (hash) { 6672 case -1618432855: // identifier 6673 this.identifier = castToIdentifier(value); // Identifier 6674 return value; 6675 case 3575610: // type 6676 this.type = castToCodeableConcept(value); // CodeableConcept 6677 return value; 6678 case -892481550: // status 6679 this.status = castToCodeableConcept(value); // CodeableConcept 6680 return value; 6681 case -1326197564: // domain 6682 this.domain = castToCodeableConcept(value); // CodeableConcept 6683 return value; 6684 case -1724546052: // description 6685 this.description = castToString(value); // StringType 6686 return value; 6687 case -896505829: // source 6688 this.getSource().add(castToReference(value)); // Reference 6689 return value; 6690 case 950398559: // comment 6691 this.comment = castToString(value); // StringType 6692 return value; 6693 case -1068650173: // moiety 6694 this.getMoiety().add((SubstanceSpecificationMoietyComponent) value); // SubstanceSpecificationMoietyComponent 6695 return value; 6696 case -993141291: // property 6697 this.getProperty().add((SubstanceSpecificationPropertyComponent) value); // SubstanceSpecificationPropertyComponent 6698 return value; 6699 case -2117930783: // referenceInformation 6700 this.referenceInformation = castToReference(value); // Reference 6701 return value; 6702 case 144518515: // structure 6703 this.structure = (SubstanceSpecificationStructureComponent) value; // SubstanceSpecificationStructureComponent 6704 return value; 6705 case 3059181: // code 6706 this.getCode().add((SubstanceSpecificationCodeComponent) value); // SubstanceSpecificationCodeComponent 6707 return value; 6708 case 3373707: // name 6709 this.getName().add((SubstanceSpecificationNameComponent) value); // SubstanceSpecificationNameComponent 6710 return value; 6711 case 635625672: // molecularWeight 6712 this.getMolecularWeight().add((SubstanceSpecificationStructureIsotopeMolecularWeightComponent) value); // SubstanceSpecificationStructureIsotopeMolecularWeightComponent 6713 return value; 6714 case -261851592: // relationship 6715 this.getRelationship().add((SubstanceSpecificationRelationshipComponent) value); // SubstanceSpecificationRelationshipComponent 6716 return value; 6717 case 1625275180: // nucleicAcid 6718 this.nucleicAcid = castToReference(value); // Reference 6719 return value; 6720 case -397514098: // polymer 6721 this.polymer = castToReference(value); // Reference 6722 return value; 6723 case -309012605: // protein 6724 this.protein = castToReference(value); // Reference 6725 return value; 6726 case -1064442270: // sourceMaterial 6727 this.sourceMaterial = castToReference(value); // Reference 6728 return value; 6729 default: 6730 return super.setProperty(hash, name, value); 6731 } 6732 6733 } 6734 6735 @Override 6736 public Base setProperty(String name, Base value) throws FHIRException { 6737 if (name.equals("identifier")) { 6738 this.identifier = castToIdentifier(value); // Identifier 6739 } else if (name.equals("type")) { 6740 this.type = castToCodeableConcept(value); // CodeableConcept 6741 } else if (name.equals("status")) { 6742 this.status = castToCodeableConcept(value); // CodeableConcept 6743 } else if (name.equals("domain")) { 6744 this.domain = castToCodeableConcept(value); // CodeableConcept 6745 } else if (name.equals("description")) { 6746 this.description = castToString(value); // StringType 6747 } else if (name.equals("source")) { 6748 this.getSource().add(castToReference(value)); 6749 } else if (name.equals("comment")) { 6750 this.comment = castToString(value); // StringType 6751 } else if (name.equals("moiety")) { 6752 this.getMoiety().add((SubstanceSpecificationMoietyComponent) value); 6753 } else if (name.equals("property")) { 6754 this.getProperty().add((SubstanceSpecificationPropertyComponent) value); 6755 } else if (name.equals("referenceInformation")) { 6756 this.referenceInformation = castToReference(value); // Reference 6757 } else if (name.equals("structure")) { 6758 this.structure = (SubstanceSpecificationStructureComponent) value; // SubstanceSpecificationStructureComponent 6759 } else if (name.equals("code")) { 6760 this.getCode().add((SubstanceSpecificationCodeComponent) value); 6761 } else if (name.equals("name")) { 6762 this.getName().add((SubstanceSpecificationNameComponent) value); 6763 } else if (name.equals("molecularWeight")) { 6764 this.getMolecularWeight().add((SubstanceSpecificationStructureIsotopeMolecularWeightComponent) value); 6765 } else if (name.equals("relationship")) { 6766 this.getRelationship().add((SubstanceSpecificationRelationshipComponent) value); 6767 } else if (name.equals("nucleicAcid")) { 6768 this.nucleicAcid = castToReference(value); // Reference 6769 } else if (name.equals("polymer")) { 6770 this.polymer = castToReference(value); // Reference 6771 } else if (name.equals("protein")) { 6772 this.protein = castToReference(value); // Reference 6773 } else if (name.equals("sourceMaterial")) { 6774 this.sourceMaterial = castToReference(value); // Reference 6775 } else 6776 return super.setProperty(name, value); 6777 return value; 6778 } 6779 6780 @Override 6781 public Base makeProperty(int hash, String name) throws FHIRException { 6782 switch (hash) { 6783 case -1618432855: 6784 return getIdentifier(); 6785 case 3575610: 6786 return getType(); 6787 case -892481550: 6788 return getStatus(); 6789 case -1326197564: 6790 return getDomain(); 6791 case -1724546052: 6792 return getDescriptionElement(); 6793 case -896505829: 6794 return addSource(); 6795 case 950398559: 6796 return getCommentElement(); 6797 case -1068650173: 6798 return addMoiety(); 6799 case -993141291: 6800 return addProperty(); 6801 case -2117930783: 6802 return getReferenceInformation(); 6803 case 144518515: 6804 return getStructure(); 6805 case 3059181: 6806 return addCode(); 6807 case 3373707: 6808 return addName(); 6809 case 635625672: 6810 return addMolecularWeight(); 6811 case -261851592: 6812 return addRelationship(); 6813 case 1625275180: 6814 return getNucleicAcid(); 6815 case -397514098: 6816 return getPolymer(); 6817 case -309012605: 6818 return getProtein(); 6819 case -1064442270: 6820 return getSourceMaterial(); 6821 default: 6822 return super.makeProperty(hash, name); 6823 } 6824 6825 } 6826 6827 @Override 6828 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6829 switch (hash) { 6830 case -1618432855: 6831 /* identifier */ return new String[] { "Identifier" }; 6832 case 3575610: 6833 /* type */ return new String[] { "CodeableConcept" }; 6834 case -892481550: 6835 /* status */ return new String[] { "CodeableConcept" }; 6836 case -1326197564: 6837 /* domain */ return new String[] { "CodeableConcept" }; 6838 case -1724546052: 6839 /* description */ return new String[] { "string" }; 6840 case -896505829: 6841 /* source */ return new String[] { "Reference" }; 6842 case 950398559: 6843 /* comment */ return new String[] { "string" }; 6844 case -1068650173: 6845 /* moiety */ return new String[] {}; 6846 case -993141291: 6847 /* property */ return new String[] {}; 6848 case -2117930783: 6849 /* referenceInformation */ return new String[] { "Reference" }; 6850 case 144518515: 6851 /* structure */ return new String[] {}; 6852 case 3059181: 6853 /* code */ return new String[] {}; 6854 case 3373707: 6855 /* name */ return new String[] {}; 6856 case 635625672: 6857 /* molecularWeight */ return new String[] { "@SubstanceSpecification.structure.isotope.molecularWeight" }; 6858 case -261851592: 6859 /* relationship */ return new String[] {}; 6860 case 1625275180: 6861 /* nucleicAcid */ return new String[] { "Reference" }; 6862 case -397514098: 6863 /* polymer */ return new String[] { "Reference" }; 6864 case -309012605: 6865 /* protein */ return new String[] { "Reference" }; 6866 case -1064442270: 6867 /* sourceMaterial */ return new String[] { "Reference" }; 6868 default: 6869 return super.getTypesForProperty(hash, name); 6870 } 6871 6872 } 6873 6874 @Override 6875 public Base addChild(String name) throws FHIRException { 6876 if (name.equals("identifier")) { 6877 this.identifier = new Identifier(); 6878 return this.identifier; 6879 } else if (name.equals("type")) { 6880 this.type = new CodeableConcept(); 6881 return this.type; 6882 } else if (name.equals("status")) { 6883 this.status = new CodeableConcept(); 6884 return this.status; 6885 } else if (name.equals("domain")) { 6886 this.domain = new CodeableConcept(); 6887 return this.domain; 6888 } else if (name.equals("description")) { 6889 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.description"); 6890 } else if (name.equals("source")) { 6891 return addSource(); 6892 } else if (name.equals("comment")) { 6893 throw new FHIRException("Cannot call addChild on a singleton property SubstanceSpecification.comment"); 6894 } else if (name.equals("moiety")) { 6895 return addMoiety(); 6896 } else if (name.equals("property")) { 6897 return addProperty(); 6898 } else if (name.equals("referenceInformation")) { 6899 this.referenceInformation = new Reference(); 6900 return this.referenceInformation; 6901 } else if (name.equals("structure")) { 6902 this.structure = new SubstanceSpecificationStructureComponent(); 6903 return this.structure; 6904 } else if (name.equals("code")) { 6905 return addCode(); 6906 } else if (name.equals("name")) { 6907 return addName(); 6908 } else if (name.equals("molecularWeight")) { 6909 return addMolecularWeight(); 6910 } else if (name.equals("relationship")) { 6911 return addRelationship(); 6912 } else if (name.equals("nucleicAcid")) { 6913 this.nucleicAcid = new Reference(); 6914 return this.nucleicAcid; 6915 } else if (name.equals("polymer")) { 6916 this.polymer = new Reference(); 6917 return this.polymer; 6918 } else if (name.equals("protein")) { 6919 this.protein = new Reference(); 6920 return this.protein; 6921 } else if (name.equals("sourceMaterial")) { 6922 this.sourceMaterial = new Reference(); 6923 return this.sourceMaterial; 6924 } else 6925 return super.addChild(name); 6926 } 6927 6928 public String fhirType() { 6929 return "SubstanceSpecification"; 6930 6931 } 6932 6933 public SubstanceSpecification copy() { 6934 SubstanceSpecification dst = new SubstanceSpecification(); 6935 copyValues(dst); 6936 return dst; 6937 } 6938 6939 public void copyValues(SubstanceSpecification dst) { 6940 super.copyValues(dst); 6941 dst.identifier = identifier == null ? null : identifier.copy(); 6942 dst.type = type == null ? null : type.copy(); 6943 dst.status = status == null ? null : status.copy(); 6944 dst.domain = domain == null ? null : domain.copy(); 6945 dst.description = description == null ? null : description.copy(); 6946 if (source != null) { 6947 dst.source = new ArrayList<Reference>(); 6948 for (Reference i : source) 6949 dst.source.add(i.copy()); 6950 } 6951 ; 6952 dst.comment = comment == null ? null : comment.copy(); 6953 if (moiety != null) { 6954 dst.moiety = new ArrayList<SubstanceSpecificationMoietyComponent>(); 6955 for (SubstanceSpecificationMoietyComponent i : moiety) 6956 dst.moiety.add(i.copy()); 6957 } 6958 ; 6959 if (property != null) { 6960 dst.property = new ArrayList<SubstanceSpecificationPropertyComponent>(); 6961 for (SubstanceSpecificationPropertyComponent i : property) 6962 dst.property.add(i.copy()); 6963 } 6964 ; 6965 dst.referenceInformation = referenceInformation == null ? null : referenceInformation.copy(); 6966 dst.structure = structure == null ? null : structure.copy(); 6967 if (code != null) { 6968 dst.code = new ArrayList<SubstanceSpecificationCodeComponent>(); 6969 for (SubstanceSpecificationCodeComponent i : code) 6970 dst.code.add(i.copy()); 6971 } 6972 ; 6973 if (name != null) { 6974 dst.name = new ArrayList<SubstanceSpecificationNameComponent>(); 6975 for (SubstanceSpecificationNameComponent i : name) 6976 dst.name.add(i.copy()); 6977 } 6978 ; 6979 if (molecularWeight != null) { 6980 dst.molecularWeight = new ArrayList<SubstanceSpecificationStructureIsotopeMolecularWeightComponent>(); 6981 for (SubstanceSpecificationStructureIsotopeMolecularWeightComponent i : molecularWeight) 6982 dst.molecularWeight.add(i.copy()); 6983 } 6984 ; 6985 if (relationship != null) { 6986 dst.relationship = new ArrayList<SubstanceSpecificationRelationshipComponent>(); 6987 for (SubstanceSpecificationRelationshipComponent i : relationship) 6988 dst.relationship.add(i.copy()); 6989 } 6990 ; 6991 dst.nucleicAcid = nucleicAcid == null ? null : nucleicAcid.copy(); 6992 dst.polymer = polymer == null ? null : polymer.copy(); 6993 dst.protein = protein == null ? null : protein.copy(); 6994 dst.sourceMaterial = sourceMaterial == null ? null : sourceMaterial.copy(); 6995 } 6996 6997 protected SubstanceSpecification typedCopy() { 6998 return copy(); 6999 } 7000 7001 @Override 7002 public boolean equalsDeep(Base other_) { 7003 if (!super.equalsDeep(other_)) 7004 return false; 7005 if (!(other_ instanceof SubstanceSpecification)) 7006 return false; 7007 SubstanceSpecification o = (SubstanceSpecification) other_; 7008 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 7009 && compareDeep(status, o.status, true) && compareDeep(domain, o.domain, true) 7010 && compareDeep(description, o.description, true) && compareDeep(source, o.source, true) 7011 && compareDeep(comment, o.comment, true) && compareDeep(moiety, o.moiety, true) 7012 && compareDeep(property, o.property, true) && compareDeep(referenceInformation, o.referenceInformation, true) 7013 && compareDeep(structure, o.structure, true) && compareDeep(code, o.code, true) 7014 && compareDeep(name, o.name, true) && compareDeep(molecularWeight, o.molecularWeight, true) 7015 && compareDeep(relationship, o.relationship, true) && compareDeep(nucleicAcid, o.nucleicAcid, true) 7016 && compareDeep(polymer, o.polymer, true) && compareDeep(protein, o.protein, true) 7017 && compareDeep(sourceMaterial, o.sourceMaterial, true); 7018 } 7019 7020 @Override 7021 public boolean equalsShallow(Base other_) { 7022 if (!super.equalsShallow(other_)) 7023 return false; 7024 if (!(other_ instanceof SubstanceSpecification)) 7025 return false; 7026 SubstanceSpecification o = (SubstanceSpecification) other_; 7027 return compareValues(description, o.description, true) && compareValues(comment, o.comment, true); 7028 } 7029 7030 public boolean isEmpty() { 7031 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, status, domain, description, 7032 source, comment, moiety, property, referenceInformation, structure, code, name, molecularWeight, relationship, 7033 nucleicAcid, polymer, protein, sourceMaterial); 7034 } 7035 7036 @Override 7037 public ResourceType getResourceType() { 7038 return ResourceType.SubstanceSpecification; 7039 } 7040 7041 /** 7042 * Search parameter: <b>code</b> 7043 * <p> 7044 * Description: <b>The specific code</b><br> 7045 * Type: <b>token</b><br> 7046 * Path: <b>SubstanceSpecification.code.code</b><br> 7047 * </p> 7048 */ 7049 @SearchParamDefinition(name = "code", path = "SubstanceSpecification.code.code", description = "The specific code", type = "token") 7050 public static final String SP_CODE = "code"; 7051 /** 7052 * <b>Fluent Client</b> search parameter constant for <b>code</b> 7053 * <p> 7054 * Description: <b>The specific code</b><br> 7055 * Type: <b>token</b><br> 7056 * Path: <b>SubstanceSpecification.code.code</b><br> 7057 * </p> 7058 */ 7059 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7060 SP_CODE); 7061 7062}