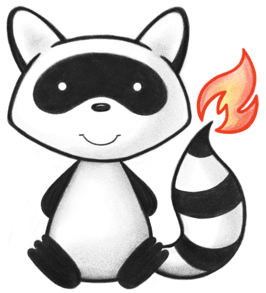
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * Record of delivery of what is supplied. 047 */ 048@ResourceDef(name = "SupplyDelivery", profile = "http://hl7.org/fhir/StructureDefinition/SupplyDelivery") 049public class SupplyDelivery extends DomainResource { 050 051 public enum SupplyDeliveryStatus { 052 /** 053 * Supply has been requested, but not delivered. 054 */ 055 INPROGRESS, 056 /** 057 * Supply has been delivered ("completed"). 058 */ 059 COMPLETED, 060 /** 061 * Delivery was not completed. 062 */ 063 ABANDONED, 064 /** 065 * This electronic record should never have existed, though it is possible that 066 * real-world decisions were based on it. (If real-world activity has occurred, 067 * the status should be "abandoned" rather than "entered-in-error".). 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 075 public static SupplyDeliveryStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("in-progress".equals(codeString)) 079 return INPROGRESS; 080 if ("completed".equals(codeString)) 081 return COMPLETED; 082 if ("abandoned".equals(codeString)) 083 return ABANDONED; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case INPROGRESS: 095 return "in-progress"; 096 case COMPLETED: 097 return "completed"; 098 case ABANDONED: 099 return "abandoned"; 100 case ENTEREDINERROR: 101 return "entered-in-error"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case INPROGRESS: 112 return "http://hl7.org/fhir/supplydelivery-status"; 113 case COMPLETED: 114 return "http://hl7.org/fhir/supplydelivery-status"; 115 case ABANDONED: 116 return "http://hl7.org/fhir/supplydelivery-status"; 117 case ENTEREDINERROR: 118 return "http://hl7.org/fhir/supplydelivery-status"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case INPROGRESS: 129 return "Supply has been requested, but not delivered."; 130 case COMPLETED: 131 return "Supply has been delivered (\"completed\")."; 132 case ABANDONED: 133 return "Delivery was not completed."; 134 case ENTEREDINERROR: 135 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"abandoned\" rather than \"entered-in-error\".)."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case INPROGRESS: 146 return "In Progress"; 147 case COMPLETED: 148 return "Delivered"; 149 case ABANDONED: 150 return "Abandoned"; 151 case ENTEREDINERROR: 152 return "Entered In Error"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class SupplyDeliveryStatusEnumFactory implements EnumFactory<SupplyDeliveryStatus> { 162 public SupplyDeliveryStatus fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("in-progress".equals(codeString)) 167 return SupplyDeliveryStatus.INPROGRESS; 168 if ("completed".equals(codeString)) 169 return SupplyDeliveryStatus.COMPLETED; 170 if ("abandoned".equals(codeString)) 171 return SupplyDeliveryStatus.ABANDONED; 172 if ("entered-in-error".equals(codeString)) 173 return SupplyDeliveryStatus.ENTEREDINERROR; 174 throw new IllegalArgumentException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 175 } 176 177 public Enumeration<SupplyDeliveryStatus> fromType(PrimitiveType<?> code) throws FHIRException { 178 if (code == null) 179 return null; 180 if (code.isEmpty()) 181 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.NULL, code); 182 String codeString = code.asStringValue(); 183 if (codeString == null || "".equals(codeString)) 184 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.NULL, code); 185 if ("in-progress".equals(codeString)) 186 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.INPROGRESS, code); 187 if ("completed".equals(codeString)) 188 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.COMPLETED, code); 189 if ("abandoned".equals(codeString)) 190 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ABANDONED, code); 191 if ("entered-in-error".equals(codeString)) 192 return new Enumeration<SupplyDeliveryStatus>(this, SupplyDeliveryStatus.ENTEREDINERROR, code); 193 throw new FHIRException("Unknown SupplyDeliveryStatus code '" + codeString + "'"); 194 } 195 196 public String toCode(SupplyDeliveryStatus code) { 197 if (code == SupplyDeliveryStatus.NULL) 198 return null; 199 if (code == SupplyDeliveryStatus.INPROGRESS) 200 return "in-progress"; 201 if (code == SupplyDeliveryStatus.COMPLETED) 202 return "completed"; 203 if (code == SupplyDeliveryStatus.ABANDONED) 204 return "abandoned"; 205 if (code == SupplyDeliveryStatus.ENTEREDINERROR) 206 return "entered-in-error"; 207 return "?"; 208 } 209 210 public String toSystem(SupplyDeliveryStatus code) { 211 return code.getSystem(); 212 } 213 } 214 215 @Block() 216 public static class SupplyDeliverySuppliedItemComponent extends BackboneElement implements IBaseBackboneElement { 217 /** 218 * The amount of supply that has been dispensed. Includes unit of measure. 219 */ 220 @Child(name = "quantity", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 221 @Description(shortDefinition = "Amount dispensed", formalDefinition = "The amount of supply that has been dispensed. Includes unit of measure.") 222 protected Quantity quantity; 223 224 /** 225 * Identifies the medication, substance or device being dispensed. This is 226 * either a link to a resource representing the details of the item or a code 227 * that identifies the item from a known list. 228 */ 229 @Child(name = "item", type = { CodeableConcept.class, Medication.class, Substance.class, 230 Device.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 231 @Description(shortDefinition = "Medication, Substance, or Device supplied", formalDefinition = "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.") 232 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supply-item") 233 protected Type item; 234 235 private static final long serialVersionUID = 1628109307L; 236 237 /** 238 * Constructor 239 */ 240 public SupplyDeliverySuppliedItemComponent() { 241 super(); 242 } 243 244 /** 245 * @return {@link #quantity} (The amount of supply that has been dispensed. 246 * Includes unit of measure.) 247 */ 248 public Quantity getQuantity() { 249 if (this.quantity == null) 250 if (Configuration.errorOnAutoCreate()) 251 throw new Error("Attempt to auto-create SupplyDeliverySuppliedItemComponent.quantity"); 252 else if (Configuration.doAutoCreate()) 253 this.quantity = new Quantity(); // cc 254 return this.quantity; 255 } 256 257 public boolean hasQuantity() { 258 return this.quantity != null && !this.quantity.isEmpty(); 259 } 260 261 /** 262 * @param value {@link #quantity} (The amount of supply that has been dispensed. 263 * Includes unit of measure.) 264 */ 265 public SupplyDeliverySuppliedItemComponent setQuantity(Quantity value) { 266 this.quantity = value; 267 return this; 268 } 269 270 /** 271 * @return {@link #item} (Identifies the medication, substance or device being 272 * dispensed. This is either a link to a resource representing the 273 * details of the item or a code that identifies the item from a known 274 * list.) 275 */ 276 public Type getItem() { 277 return this.item; 278 } 279 280 /** 281 * @return {@link #item} (Identifies the medication, substance or device being 282 * dispensed. This is either a link to a resource representing the 283 * details of the item or a code that identifies the item from a known 284 * list.) 285 */ 286 public CodeableConcept getItemCodeableConcept() throws FHIRException { 287 if (this.item == null) 288 this.item = new CodeableConcept(); 289 if (!(this.item instanceof CodeableConcept)) 290 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 291 + this.item.getClass().getName() + " was encountered"); 292 return (CodeableConcept) this.item; 293 } 294 295 public boolean hasItemCodeableConcept() { 296 return this.item instanceof CodeableConcept; 297 } 298 299 /** 300 * @return {@link #item} (Identifies the medication, substance or device being 301 * dispensed. This is either a link to a resource representing the 302 * details of the item or a code that identifies the item from a known 303 * list.) 304 */ 305 public Reference getItemReference() throws FHIRException { 306 if (this.item == null) 307 this.item = new Reference(); 308 if (!(this.item instanceof Reference)) 309 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() 310 + " was encountered"); 311 return (Reference) this.item; 312 } 313 314 public boolean hasItemReference() { 315 return this.item instanceof Reference; 316 } 317 318 public boolean hasItem() { 319 return this.item != null && !this.item.isEmpty(); 320 } 321 322 /** 323 * @param value {@link #item} (Identifies the medication, substance or device 324 * being dispensed. This is either a link to a resource 325 * representing the details of the item or a code that identifies 326 * the item from a known list.) 327 */ 328 public SupplyDeliverySuppliedItemComponent setItem(Type value) { 329 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 330 throw new Error("Not the right type for SupplyDelivery.suppliedItem.item[x]: " + value.fhirType()); 331 this.item = value; 332 return this; 333 } 334 335 protected void listChildren(List<Property> children) { 336 super.listChildren(children); 337 children.add(new Property("quantity", "SimpleQuantity", 338 "The amount of supply that has been dispensed. Includes unit of measure.", 0, 1, quantity)); 339 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 340 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 341 0, 1, item)); 342 } 343 344 @Override 345 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 346 switch (_hash) { 347 case -1285004149: 348 /* quantity */ return new Property("quantity", "SimpleQuantity", 349 "The amount of supply that has been dispensed. Includes unit of measure.", 0, 1, quantity); 350 case 2116201613: 351 /* item[x] */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 352 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 353 0, 1, item); 354 case 3242771: 355 /* item */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 356 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 357 0, 1, item); 358 case 106644494: 359 /* itemCodeableConcept */ return new Property("item[x]", 360 "CodeableConcept|Reference(Medication|Substance|Device)", 361 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 362 0, 1, item); 363 case 1376364920: 364 /* itemReference */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 365 "Identifies the medication, substance or device being dispensed. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 366 0, 1, item); 367 default: 368 return super.getNamedProperty(_hash, _name, _checkValid); 369 } 370 371 } 372 373 @Override 374 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 375 switch (hash) { 376 case -1285004149: 377 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 378 case 3242771: 379 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 380 default: 381 return super.getProperty(hash, name, checkValid); 382 } 383 384 } 385 386 @Override 387 public Base setProperty(int hash, String name, Base value) throws FHIRException { 388 switch (hash) { 389 case -1285004149: // quantity 390 this.quantity = castToQuantity(value); // Quantity 391 return value; 392 case 3242771: // item 393 this.item = castToType(value); // Type 394 return value; 395 default: 396 return super.setProperty(hash, name, value); 397 } 398 399 } 400 401 @Override 402 public Base setProperty(String name, Base value) throws FHIRException { 403 if (name.equals("quantity")) { 404 this.quantity = castToQuantity(value); // Quantity 405 } else if (name.equals("item[x]")) { 406 this.item = castToType(value); // Type 407 } else 408 return super.setProperty(name, value); 409 return value; 410 } 411 412 @Override 413 public void removeChild(String name, Base value) throws FHIRException { 414 if (name.equals("quantity")) { 415 this.quantity = null; 416 } else if (name.equals("item[x]")) { 417 this.item = null; 418 } else 419 super.removeChild(name, value); 420 421 } 422 423 @Override 424 public Base makeProperty(int hash, String name) throws FHIRException { 425 switch (hash) { 426 case -1285004149: 427 return getQuantity(); 428 case 2116201613: 429 return getItem(); 430 case 3242771: 431 return getItem(); 432 default: 433 return super.makeProperty(hash, name); 434 } 435 436 } 437 438 @Override 439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 440 switch (hash) { 441 case -1285004149: 442 /* quantity */ return new String[] { "SimpleQuantity" }; 443 case 3242771: 444 /* item */ return new String[] { "CodeableConcept", "Reference" }; 445 default: 446 return super.getTypesForProperty(hash, name); 447 } 448 449 } 450 451 @Override 452 public Base addChild(String name) throws FHIRException { 453 if (name.equals("quantity")) { 454 this.quantity = new Quantity(); 455 return this.quantity; 456 } else if (name.equals("itemCodeableConcept")) { 457 this.item = new CodeableConcept(); 458 return this.item; 459 } else if (name.equals("itemReference")) { 460 this.item = new Reference(); 461 return this.item; 462 } else 463 return super.addChild(name); 464 } 465 466 public SupplyDeliverySuppliedItemComponent copy() { 467 SupplyDeliverySuppliedItemComponent dst = new SupplyDeliverySuppliedItemComponent(); 468 copyValues(dst); 469 return dst; 470 } 471 472 public void copyValues(SupplyDeliverySuppliedItemComponent dst) { 473 super.copyValues(dst); 474 dst.quantity = quantity == null ? null : quantity.copy(); 475 dst.item = item == null ? null : item.copy(); 476 } 477 478 @Override 479 public boolean equalsDeep(Base other_) { 480 if (!super.equalsDeep(other_)) 481 return false; 482 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 483 return false; 484 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 485 return compareDeep(quantity, o.quantity, true) && compareDeep(item, o.item, true); 486 } 487 488 @Override 489 public boolean equalsShallow(Base other_) { 490 if (!super.equalsShallow(other_)) 491 return false; 492 if (!(other_ instanceof SupplyDeliverySuppliedItemComponent)) 493 return false; 494 SupplyDeliverySuppliedItemComponent o = (SupplyDeliverySuppliedItemComponent) other_; 495 return true; 496 } 497 498 public boolean isEmpty() { 499 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, item); 500 } 501 502 public String fhirType() { 503 return "SupplyDelivery.suppliedItem"; 504 505 } 506 507 } 508 509 /** 510 * Identifier for the supply delivery event that is used to identify it across 511 * multiple disparate systems. 512 */ 513 @Child(name = "identifier", type = { 514 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 515 @Description(shortDefinition = "External identifier", formalDefinition = "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.") 516 protected List<Identifier> identifier; 517 518 /** 519 * A plan, proposal or order that is fulfilled in whole or in part by this 520 * event. 521 */ 522 @Child(name = "basedOn", type = { 523 SupplyRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 524 @Description(shortDefinition = "Fulfills plan, proposal or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this event.") 525 protected List<Reference> basedOn; 526 /** 527 * The actual objects that are the target of the reference (A plan, proposal or 528 * order that is fulfilled in whole or in part by this event.) 529 */ 530 protected List<SupplyRequest> basedOnTarget; 531 532 /** 533 * A larger event of which this particular event is a component or step. 534 */ 535 @Child(name = "partOf", type = { SupplyDelivery.class, 536 Contract.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 537 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular event is a component or step.") 538 protected List<Reference> partOf; 539 /** 540 * The actual objects that are the target of the reference (A larger event of 541 * which this particular event is a component or step.) 542 */ 543 protected List<Resource> partOfTarget; 544 545 /** 546 * A code specifying the state of the dispense event. 547 */ 548 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 549 @Description(shortDefinition = "in-progress | completed | abandoned | entered-in-error", formalDefinition = "A code specifying the state of the dispense event.") 550 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplydelivery-status") 551 protected Enumeration<SupplyDeliveryStatus> status; 552 553 /** 554 * A link to a resource representing the person whom the delivered item is for. 555 */ 556 @Child(name = "patient", type = { Patient.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 557 @Description(shortDefinition = "Patient for whom the item is supplied", formalDefinition = "A link to a resource representing the person whom the delivered item is for.") 558 protected Reference patient; 559 560 /** 561 * The actual object that is the target of the reference (A link to a resource 562 * representing the person whom the delivered item is for.) 563 */ 564 protected Patient patientTarget; 565 566 /** 567 * Indicates the type of dispensing event that is performed. Examples include: 568 * Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc. 569 */ 570 @Child(name = "type", type = { 571 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 572 @Description(shortDefinition = "Category of dispense event", formalDefinition = "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.") 573 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplydelivery-type") 574 protected CodeableConcept type; 575 576 /** 577 * The item that is being delivered or has been supplied. 578 */ 579 @Child(name = "suppliedItem", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = false) 580 @Description(shortDefinition = "The item that is delivered or supplied", formalDefinition = "The item that is being delivered or has been supplied.") 581 protected SupplyDeliverySuppliedItemComponent suppliedItem; 582 583 /** 584 * The date or time(s) the activity occurred. 585 */ 586 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 587 Timing.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 588 @Description(shortDefinition = "When event occurred", formalDefinition = "The date or time(s) the activity occurred.") 589 protected Type occurrence; 590 591 /** 592 * The individual responsible for dispensing the medication, supplier or device. 593 */ 594 @Child(name = "supplier", type = { Practitioner.class, PractitionerRole.class, 595 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 596 @Description(shortDefinition = "Dispenser", formalDefinition = "The individual responsible for dispensing the medication, supplier or device.") 597 protected Reference supplier; 598 599 /** 600 * The actual object that is the target of the reference (The individual 601 * responsible for dispensing the medication, supplier or device.) 602 */ 603 protected Resource supplierTarget; 604 605 /** 606 * Identification of the facility/location where the Supply was shipped to, as 607 * part of the dispense event. 608 */ 609 @Child(name = "destination", type = { 610 Location.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 611 @Description(shortDefinition = "Where the Supply was sent", formalDefinition = "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.") 612 protected Reference destination; 613 614 /** 615 * The actual object that is the target of the reference (Identification of the 616 * facility/location where the Supply was shipped to, as part of the dispense 617 * event.) 618 */ 619 protected Location destinationTarget; 620 621 /** 622 * Identifies the person who picked up the Supply. 623 */ 624 @Child(name = "receiver", type = { Practitioner.class, 625 PractitionerRole.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 626 @Description(shortDefinition = "Who collected the Supply", formalDefinition = "Identifies the person who picked up the Supply.") 627 protected List<Reference> receiver; 628 /** 629 * The actual objects that are the target of the reference (Identifies the 630 * person who picked up the Supply.) 631 */ 632 protected List<Resource> receiverTarget; 633 634 private static final long serialVersionUID = -750389806L; 635 636 /** 637 * Constructor 638 */ 639 public SupplyDelivery() { 640 super(); 641 } 642 643 /** 644 * @return {@link #identifier} (Identifier for the supply delivery event that is 645 * used to identify it across multiple disparate systems.) 646 */ 647 public List<Identifier> getIdentifier() { 648 if (this.identifier == null) 649 this.identifier = new ArrayList<Identifier>(); 650 return this.identifier; 651 } 652 653 /** 654 * @return Returns a reference to <code>this</code> for easy method chaining 655 */ 656 public SupplyDelivery setIdentifier(List<Identifier> theIdentifier) { 657 this.identifier = theIdentifier; 658 return this; 659 } 660 661 public boolean hasIdentifier() { 662 if (this.identifier == null) 663 return false; 664 for (Identifier item : this.identifier) 665 if (!item.isEmpty()) 666 return true; 667 return false; 668 } 669 670 public Identifier addIdentifier() { // 3 671 Identifier t = new Identifier(); 672 if (this.identifier == null) 673 this.identifier = new ArrayList<Identifier>(); 674 this.identifier.add(t); 675 return t; 676 } 677 678 public SupplyDelivery addIdentifier(Identifier t) { // 3 679 if (t == null) 680 return this; 681 if (this.identifier == null) 682 this.identifier = new ArrayList<Identifier>(); 683 this.identifier.add(t); 684 return this; 685 } 686 687 /** 688 * @return The first repetition of repeating field {@link #identifier}, creating 689 * it if it does not already exist 690 */ 691 public Identifier getIdentifierFirstRep() { 692 if (getIdentifier().isEmpty()) { 693 addIdentifier(); 694 } 695 return getIdentifier().get(0); 696 } 697 698 /** 699 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 700 * whole or in part by this event.) 701 */ 702 public List<Reference> getBasedOn() { 703 if (this.basedOn == null) 704 this.basedOn = new ArrayList<Reference>(); 705 return this.basedOn; 706 } 707 708 /** 709 * @return Returns a reference to <code>this</code> for easy method chaining 710 */ 711 public SupplyDelivery setBasedOn(List<Reference> theBasedOn) { 712 this.basedOn = theBasedOn; 713 return this; 714 } 715 716 public boolean hasBasedOn() { 717 if (this.basedOn == null) 718 return false; 719 for (Reference item : this.basedOn) 720 if (!item.isEmpty()) 721 return true; 722 return false; 723 } 724 725 public Reference addBasedOn() { // 3 726 Reference t = new Reference(); 727 if (this.basedOn == null) 728 this.basedOn = new ArrayList<Reference>(); 729 this.basedOn.add(t); 730 return t; 731 } 732 733 public SupplyDelivery addBasedOn(Reference t) { // 3 734 if (t == null) 735 return this; 736 if (this.basedOn == null) 737 this.basedOn = new ArrayList<Reference>(); 738 this.basedOn.add(t); 739 return this; 740 } 741 742 /** 743 * @return The first repetition of repeating field {@link #basedOn}, creating it 744 * if it does not already exist 745 */ 746 public Reference getBasedOnFirstRep() { 747 if (getBasedOn().isEmpty()) { 748 addBasedOn(); 749 } 750 return getBasedOn().get(0); 751 } 752 753 /** 754 * @deprecated Use Reference#setResource(IBaseResource) instead 755 */ 756 @Deprecated 757 public List<SupplyRequest> getBasedOnTarget() { 758 if (this.basedOnTarget == null) 759 this.basedOnTarget = new ArrayList<SupplyRequest>(); 760 return this.basedOnTarget; 761 } 762 763 /** 764 * @deprecated Use Reference#setResource(IBaseResource) instead 765 */ 766 @Deprecated 767 public SupplyRequest addBasedOnTarget() { 768 SupplyRequest r = new SupplyRequest(); 769 if (this.basedOnTarget == null) 770 this.basedOnTarget = new ArrayList<SupplyRequest>(); 771 this.basedOnTarget.add(r); 772 return r; 773 } 774 775 /** 776 * @return {@link #partOf} (A larger event of which this particular event is a 777 * component or step.) 778 */ 779 public List<Reference> getPartOf() { 780 if (this.partOf == null) 781 this.partOf = new ArrayList<Reference>(); 782 return this.partOf; 783 } 784 785 /** 786 * @return Returns a reference to <code>this</code> for easy method chaining 787 */ 788 public SupplyDelivery setPartOf(List<Reference> thePartOf) { 789 this.partOf = thePartOf; 790 return this; 791 } 792 793 public boolean hasPartOf() { 794 if (this.partOf == null) 795 return false; 796 for (Reference item : this.partOf) 797 if (!item.isEmpty()) 798 return true; 799 return false; 800 } 801 802 public Reference addPartOf() { // 3 803 Reference t = new Reference(); 804 if (this.partOf == null) 805 this.partOf = new ArrayList<Reference>(); 806 this.partOf.add(t); 807 return t; 808 } 809 810 public SupplyDelivery addPartOf(Reference t) { // 3 811 if (t == null) 812 return this; 813 if (this.partOf == null) 814 this.partOf = new ArrayList<Reference>(); 815 this.partOf.add(t); 816 return this; 817 } 818 819 /** 820 * @return The first repetition of repeating field {@link #partOf}, creating it 821 * if it does not already exist 822 */ 823 public Reference getPartOfFirstRep() { 824 if (getPartOf().isEmpty()) { 825 addPartOf(); 826 } 827 return getPartOf().get(0); 828 } 829 830 /** 831 * @deprecated Use Reference#setResource(IBaseResource) instead 832 */ 833 @Deprecated 834 public List<Resource> getPartOfTarget() { 835 if (this.partOfTarget == null) 836 this.partOfTarget = new ArrayList<Resource>(); 837 return this.partOfTarget; 838 } 839 840 /** 841 * @return {@link #status} (A code specifying the state of the dispense event.). 842 * This is the underlying object with id, value and extensions. The 843 * accessor "getStatus" gives direct access to the value 844 */ 845 public Enumeration<SupplyDeliveryStatus> getStatusElement() { 846 if (this.status == null) 847 if (Configuration.errorOnAutoCreate()) 848 throw new Error("Attempt to auto-create SupplyDelivery.status"); 849 else if (Configuration.doAutoCreate()) 850 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); // bb 851 return this.status; 852 } 853 854 public boolean hasStatusElement() { 855 return this.status != null && !this.status.isEmpty(); 856 } 857 858 public boolean hasStatus() { 859 return this.status != null && !this.status.isEmpty(); 860 } 861 862 /** 863 * @param value {@link #status} (A code specifying the state of the dispense 864 * event.). This is the underlying object with id, value and 865 * extensions. The accessor "getStatus" gives direct access to the 866 * value 867 */ 868 public SupplyDelivery setStatusElement(Enumeration<SupplyDeliveryStatus> value) { 869 this.status = value; 870 return this; 871 } 872 873 /** 874 * @return A code specifying the state of the dispense event. 875 */ 876 public SupplyDeliveryStatus getStatus() { 877 return this.status == null ? null : this.status.getValue(); 878 } 879 880 /** 881 * @param value A code specifying the state of the dispense event. 882 */ 883 public SupplyDelivery setStatus(SupplyDeliveryStatus value) { 884 if (value == null) 885 this.status = null; 886 else { 887 if (this.status == null) 888 this.status = new Enumeration<SupplyDeliveryStatus>(new SupplyDeliveryStatusEnumFactory()); 889 this.status.setValue(value); 890 } 891 return this; 892 } 893 894 /** 895 * @return {@link #patient} (A link to a resource representing the person whom 896 * the delivered item is for.) 897 */ 898 public Reference getPatient() { 899 if (this.patient == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 902 else if (Configuration.doAutoCreate()) 903 this.patient = new Reference(); // cc 904 return this.patient; 905 } 906 907 public boolean hasPatient() { 908 return this.patient != null && !this.patient.isEmpty(); 909 } 910 911 /** 912 * @param value {@link #patient} (A link to a resource representing the person 913 * whom the delivered item is for.) 914 */ 915 public SupplyDelivery setPatient(Reference value) { 916 this.patient = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #patient} The actual object that is the target of the 922 * reference. The reference library doesn't populate this, but you can 923 * use it to hold the resource if you resolve it. (A link to a resource 924 * representing the person whom the delivered item is for.) 925 */ 926 public Patient getPatientTarget() { 927 if (this.patientTarget == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create SupplyDelivery.patient"); 930 else if (Configuration.doAutoCreate()) 931 this.patientTarget = new Patient(); // aa 932 return this.patientTarget; 933 } 934 935 /** 936 * @param value {@link #patient} The actual object that is the target of the 937 * reference. The reference library doesn't use these, but you can 938 * use it to hold the resource if you resolve it. (A link to a 939 * resource representing the person whom the delivered item is 940 * for.) 941 */ 942 public SupplyDelivery setPatientTarget(Patient value) { 943 this.patientTarget = value; 944 return this; 945 } 946 947 /** 948 * @return {@link #type} (Indicates the type of dispensing event that is 949 * performed. Examples include: Trial Fill, Completion of Trial, Partial 950 * Fill, Emergency Fill, Samples, etc.) 951 */ 952 public CodeableConcept getType() { 953 if (this.type == null) 954 if (Configuration.errorOnAutoCreate()) 955 throw new Error("Attempt to auto-create SupplyDelivery.type"); 956 else if (Configuration.doAutoCreate()) 957 this.type = new CodeableConcept(); // cc 958 return this.type; 959 } 960 961 public boolean hasType() { 962 return this.type != null && !this.type.isEmpty(); 963 } 964 965 /** 966 * @param value {@link #type} (Indicates the type of dispensing event that is 967 * performed. Examples include: Trial Fill, Completion of Trial, 968 * Partial Fill, Emergency Fill, Samples, etc.) 969 */ 970 public SupplyDelivery setType(CodeableConcept value) { 971 this.type = value; 972 return this; 973 } 974 975 /** 976 * @return {@link #suppliedItem} (The item that is being delivered or has been 977 * supplied.) 978 */ 979 public SupplyDeliverySuppliedItemComponent getSuppliedItem() { 980 if (this.suppliedItem == null) 981 if (Configuration.errorOnAutoCreate()) 982 throw new Error("Attempt to auto-create SupplyDelivery.suppliedItem"); 983 else if (Configuration.doAutoCreate()) 984 this.suppliedItem = new SupplyDeliverySuppliedItemComponent(); // cc 985 return this.suppliedItem; 986 } 987 988 public boolean hasSuppliedItem() { 989 return this.suppliedItem != null && !this.suppliedItem.isEmpty(); 990 } 991 992 /** 993 * @param value {@link #suppliedItem} (The item that is being delivered or has 994 * been supplied.) 995 */ 996 public SupplyDelivery setSuppliedItem(SupplyDeliverySuppliedItemComponent value) { 997 this.suppliedItem = value; 998 return this; 999 } 1000 1001 /** 1002 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 1003 */ 1004 public Type getOccurrence() { 1005 return this.occurrence; 1006 } 1007 1008 /** 1009 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 1010 */ 1011 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1012 if (this.occurrence == null) 1013 this.occurrence = new DateTimeType(); 1014 if (!(this.occurrence instanceof DateTimeType)) 1015 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1016 + this.occurrence.getClass().getName() + " was encountered"); 1017 return (DateTimeType) this.occurrence; 1018 } 1019 1020 public boolean hasOccurrenceDateTimeType() { 1021 return this.occurrence instanceof DateTimeType; 1022 } 1023 1024 /** 1025 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 1026 */ 1027 public Period getOccurrencePeriod() throws FHIRException { 1028 if (this.occurrence == null) 1029 this.occurrence = new Period(); 1030 if (!(this.occurrence instanceof Period)) 1031 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1032 + " was encountered"); 1033 return (Period) this.occurrence; 1034 } 1035 1036 public boolean hasOccurrencePeriod() { 1037 return this.occurrence instanceof Period; 1038 } 1039 1040 /** 1041 * @return {@link #occurrence} (The date or time(s) the activity occurred.) 1042 */ 1043 public Timing getOccurrenceTiming() throws FHIRException { 1044 if (this.occurrence == null) 1045 this.occurrence = new Timing(); 1046 if (!(this.occurrence instanceof Timing)) 1047 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.occurrence.getClass().getName() 1048 + " was encountered"); 1049 return (Timing) this.occurrence; 1050 } 1051 1052 public boolean hasOccurrenceTiming() { 1053 return this.occurrence instanceof Timing; 1054 } 1055 1056 public boolean hasOccurrence() { 1057 return this.occurrence != null && !this.occurrence.isEmpty(); 1058 } 1059 1060 /** 1061 * @param value {@link #occurrence} (The date or time(s) the activity occurred.) 1062 */ 1063 public SupplyDelivery setOccurrence(Type value) { 1064 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1065 throw new Error("Not the right type for SupplyDelivery.occurrence[x]: " + value.fhirType()); 1066 this.occurrence = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #supplier} (The individual responsible for dispensing the 1072 * medication, supplier or device.) 1073 */ 1074 public Reference getSupplier() { 1075 if (this.supplier == null) 1076 if (Configuration.errorOnAutoCreate()) 1077 throw new Error("Attempt to auto-create SupplyDelivery.supplier"); 1078 else if (Configuration.doAutoCreate()) 1079 this.supplier = new Reference(); // cc 1080 return this.supplier; 1081 } 1082 1083 public boolean hasSupplier() { 1084 return this.supplier != null && !this.supplier.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #supplier} (The individual responsible for dispensing the 1089 * medication, supplier or device.) 1090 */ 1091 public SupplyDelivery setSupplier(Reference value) { 1092 this.supplier = value; 1093 return this; 1094 } 1095 1096 /** 1097 * @return {@link #supplier} The actual object that is the target of the 1098 * reference. The reference library doesn't populate this, but you can 1099 * use it to hold the resource if you resolve it. (The individual 1100 * responsible for dispensing the medication, supplier or device.) 1101 */ 1102 public Resource getSupplierTarget() { 1103 return this.supplierTarget; 1104 } 1105 1106 /** 1107 * @param value {@link #supplier} The actual object that is the target of the 1108 * reference. The reference library doesn't use these, but you can 1109 * use it to hold the resource if you resolve it. (The individual 1110 * responsible for dispensing the medication, supplier or device.) 1111 */ 1112 public SupplyDelivery setSupplierTarget(Resource value) { 1113 this.supplierTarget = value; 1114 return this; 1115 } 1116 1117 /** 1118 * @return {@link #destination} (Identification of the facility/location where 1119 * the Supply was shipped to, as part of the dispense event.) 1120 */ 1121 public Reference getDestination() { 1122 if (this.destination == null) 1123 if (Configuration.errorOnAutoCreate()) 1124 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 1125 else if (Configuration.doAutoCreate()) 1126 this.destination = new Reference(); // cc 1127 return this.destination; 1128 } 1129 1130 public boolean hasDestination() { 1131 return this.destination != null && !this.destination.isEmpty(); 1132 } 1133 1134 /** 1135 * @param value {@link #destination} (Identification of the facility/location 1136 * where the Supply was shipped to, as part of the dispense event.) 1137 */ 1138 public SupplyDelivery setDestination(Reference value) { 1139 this.destination = value; 1140 return this; 1141 } 1142 1143 /** 1144 * @return {@link #destination} The actual object that is the target of the 1145 * reference. The reference library doesn't populate this, but you can 1146 * use it to hold the resource if you resolve it. (Identification of the 1147 * facility/location where the Supply was shipped to, as part of the 1148 * dispense event.) 1149 */ 1150 public Location getDestinationTarget() { 1151 if (this.destinationTarget == null) 1152 if (Configuration.errorOnAutoCreate()) 1153 throw new Error("Attempt to auto-create SupplyDelivery.destination"); 1154 else if (Configuration.doAutoCreate()) 1155 this.destinationTarget = new Location(); // aa 1156 return this.destinationTarget; 1157 } 1158 1159 /** 1160 * @param value {@link #destination} The actual object that is the target of the 1161 * reference. The reference library doesn't use these, but you can 1162 * use it to hold the resource if you resolve it. (Identification 1163 * of the facility/location where the Supply was shipped to, as 1164 * part of the dispense event.) 1165 */ 1166 public SupplyDelivery setDestinationTarget(Location value) { 1167 this.destinationTarget = value; 1168 return this; 1169 } 1170 1171 /** 1172 * @return {@link #receiver} (Identifies the person who picked up the Supply.) 1173 */ 1174 public List<Reference> getReceiver() { 1175 if (this.receiver == null) 1176 this.receiver = new ArrayList<Reference>(); 1177 return this.receiver; 1178 } 1179 1180 /** 1181 * @return Returns a reference to <code>this</code> for easy method chaining 1182 */ 1183 public SupplyDelivery setReceiver(List<Reference> theReceiver) { 1184 this.receiver = theReceiver; 1185 return this; 1186 } 1187 1188 public boolean hasReceiver() { 1189 if (this.receiver == null) 1190 return false; 1191 for (Reference item : this.receiver) 1192 if (!item.isEmpty()) 1193 return true; 1194 return false; 1195 } 1196 1197 public Reference addReceiver() { // 3 1198 Reference t = new Reference(); 1199 if (this.receiver == null) 1200 this.receiver = new ArrayList<Reference>(); 1201 this.receiver.add(t); 1202 return t; 1203 } 1204 1205 public SupplyDelivery addReceiver(Reference t) { // 3 1206 if (t == null) 1207 return this; 1208 if (this.receiver == null) 1209 this.receiver = new ArrayList<Reference>(); 1210 this.receiver.add(t); 1211 return this; 1212 } 1213 1214 /** 1215 * @return The first repetition of repeating field {@link #receiver}, creating 1216 * it if it does not already exist 1217 */ 1218 public Reference getReceiverFirstRep() { 1219 if (getReceiver().isEmpty()) { 1220 addReceiver(); 1221 } 1222 return getReceiver().get(0); 1223 } 1224 1225 /** 1226 * @deprecated Use Reference#setResource(IBaseResource) instead 1227 */ 1228 @Deprecated 1229 public List<Resource> getReceiverTarget() { 1230 if (this.receiverTarget == null) 1231 this.receiverTarget = new ArrayList<Resource>(); 1232 return this.receiverTarget; 1233 } 1234 1235 protected void listChildren(List<Property> children) { 1236 super.listChildren(children); 1237 children.add(new Property("identifier", "Identifier", 1238 "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.", 0, 1239 java.lang.Integer.MAX_VALUE, identifier)); 1240 children.add(new Property("basedOn", "Reference(SupplyRequest)", 1241 "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, 1242 java.lang.Integer.MAX_VALUE, basedOn)); 1243 children.add(new Property("partOf", "Reference(SupplyDelivery|Contract)", 1244 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1245 partOf)); 1246 children.add(new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, status)); 1247 children.add(new Property("patient", "Reference(Patient)", 1248 "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient)); 1249 children.add(new Property("type", "CodeableConcept", 1250 "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 1251 0, 1, type)); 1252 children.add( 1253 new Property("suppliedItem", "", "The item that is being delivered or has been supplied.", 0, 1, suppliedItem)); 1254 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "The date or time(s) the activity occurred.", 1255 0, 1, occurrence)); 1256 children.add(new Property("supplier", "Reference(Practitioner|PractitionerRole|Organization)", 1257 "The individual responsible for dispensing the medication, supplier or device.", 0, 1, supplier)); 1258 children.add(new Property("destination", "Reference(Location)", 1259 "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.", 0, 1, 1260 destination)); 1261 children.add(new Property("receiver", "Reference(Practitioner|PractitionerRole)", 1262 "Identifies the person who picked up the Supply.", 0, java.lang.Integer.MAX_VALUE, receiver)); 1263 } 1264 1265 @Override 1266 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1267 switch (_hash) { 1268 case -1618432855: 1269 /* identifier */ return new Property("identifier", "Identifier", 1270 "Identifier for the supply delivery event that is used to identify it across multiple disparate systems.", 0, 1271 java.lang.Integer.MAX_VALUE, identifier); 1272 case -332612366: 1273 /* basedOn */ return new Property("basedOn", "Reference(SupplyRequest)", 1274 "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, 1275 java.lang.Integer.MAX_VALUE, basedOn); 1276 case -995410646: 1277 /* partOf */ return new Property("partOf", "Reference(SupplyDelivery|Contract)", 1278 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1279 partOf); 1280 case -892481550: 1281 /* status */ return new Property("status", "code", "A code specifying the state of the dispense event.", 0, 1, 1282 status); 1283 case -791418107: 1284 /* patient */ return new Property("patient", "Reference(Patient)", 1285 "A link to a resource representing the person whom the delivered item is for.", 0, 1, patient); 1286 case 3575610: 1287 /* type */ return new Property("type", "CodeableConcept", 1288 "Indicates the type of dispensing event that is performed. Examples include: Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 1289 0, 1, type); 1290 case 1993333233: 1291 /* suppliedItem */ return new Property("suppliedItem", "", 1292 "The item that is being delivered or has been supplied.", 0, 1, suppliedItem); 1293 case -2022646513: 1294 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1295 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1296 case 1687874001: 1297 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1298 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1299 case -298443636: 1300 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1301 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1302 case 1397156594: 1303 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1304 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1305 case 1515218299: 1306 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1307 "The date or time(s) the activity occurred.", 0, 1, occurrence); 1308 case -1663305268: 1309 /* supplier */ return new Property("supplier", "Reference(Practitioner|PractitionerRole|Organization)", 1310 "The individual responsible for dispensing the medication, supplier or device.", 0, 1, supplier); 1311 case -1429847026: 1312 /* destination */ return new Property("destination", "Reference(Location)", 1313 "Identification of the facility/location where the Supply was shipped to, as part of the dispense event.", 0, 1314 1, destination); 1315 case -808719889: 1316 /* receiver */ return new Property("receiver", "Reference(Practitioner|PractitionerRole)", 1317 "Identifies the person who picked up the Supply.", 0, java.lang.Integer.MAX_VALUE, receiver); 1318 default: 1319 return super.getNamedProperty(_hash, _name, _checkValid); 1320 } 1321 1322 } 1323 1324 @Override 1325 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1326 switch (hash) { 1327 case -1618432855: 1328 /* identifier */ return this.identifier == null ? new Base[0] 1329 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1330 case -332612366: 1331 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1332 case -995410646: 1333 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1334 case -892481550: 1335 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<SupplyDeliveryStatus> 1336 case -791418107: 1337 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 1338 case 3575610: 1339 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1340 case 1993333233: 1341 /* suppliedItem */ return this.suppliedItem == null ? new Base[0] : new Base[] { this.suppliedItem }; // SupplyDeliverySuppliedItemComponent 1342 case 1687874001: 1343 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 1344 case -1663305268: 1345 /* supplier */ return this.supplier == null ? new Base[0] : new Base[] { this.supplier }; // Reference 1346 case -1429847026: 1347 /* destination */ return this.destination == null ? new Base[0] : new Base[] { this.destination }; // Reference 1348 case -808719889: 1349 /* receiver */ return this.receiver == null ? new Base[0] : this.receiver.toArray(new Base[this.receiver.size()]); // Reference 1350 default: 1351 return super.getProperty(hash, name, checkValid); 1352 } 1353 1354 } 1355 1356 @Override 1357 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1358 switch (hash) { 1359 case -1618432855: // identifier 1360 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1361 return value; 1362 case -332612366: // basedOn 1363 this.getBasedOn().add(castToReference(value)); // Reference 1364 return value; 1365 case -995410646: // partOf 1366 this.getPartOf().add(castToReference(value)); // Reference 1367 return value; 1368 case -892481550: // status 1369 value = new SupplyDeliveryStatusEnumFactory().fromType(castToCode(value)); 1370 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1371 return value; 1372 case -791418107: // patient 1373 this.patient = castToReference(value); // Reference 1374 return value; 1375 case 3575610: // type 1376 this.type = castToCodeableConcept(value); // CodeableConcept 1377 return value; 1378 case 1993333233: // suppliedItem 1379 this.suppliedItem = (SupplyDeliverySuppliedItemComponent) value; // SupplyDeliverySuppliedItemComponent 1380 return value; 1381 case 1687874001: // occurrence 1382 this.occurrence = castToType(value); // Type 1383 return value; 1384 case -1663305268: // supplier 1385 this.supplier = castToReference(value); // Reference 1386 return value; 1387 case -1429847026: // destination 1388 this.destination = castToReference(value); // Reference 1389 return value; 1390 case -808719889: // receiver 1391 this.getReceiver().add(castToReference(value)); // Reference 1392 return value; 1393 default: 1394 return super.setProperty(hash, name, value); 1395 } 1396 1397 } 1398 1399 @Override 1400 public Base setProperty(String name, Base value) throws FHIRException { 1401 if (name.equals("identifier")) { 1402 this.getIdentifier().add(castToIdentifier(value)); 1403 } else if (name.equals("basedOn")) { 1404 this.getBasedOn().add(castToReference(value)); 1405 } else if (name.equals("partOf")) { 1406 this.getPartOf().add(castToReference(value)); 1407 } else if (name.equals("status")) { 1408 value = new SupplyDeliveryStatusEnumFactory().fromType(castToCode(value)); 1409 this.status = (Enumeration) value; // Enumeration<SupplyDeliveryStatus> 1410 } else if (name.equals("patient")) { 1411 this.patient = castToReference(value); // Reference 1412 } else if (name.equals("type")) { 1413 this.type = castToCodeableConcept(value); // CodeableConcept 1414 } else if (name.equals("suppliedItem")) { 1415 this.suppliedItem = (SupplyDeliverySuppliedItemComponent) value; // SupplyDeliverySuppliedItemComponent 1416 } else if (name.equals("occurrence[x]")) { 1417 this.occurrence = castToType(value); // Type 1418 } else if (name.equals("supplier")) { 1419 this.supplier = castToReference(value); // Reference 1420 } else if (name.equals("destination")) { 1421 this.destination = castToReference(value); // Reference 1422 } else if (name.equals("receiver")) { 1423 this.getReceiver().add(castToReference(value)); 1424 } else 1425 return super.setProperty(name, value); 1426 return value; 1427 } 1428 1429 @Override 1430 public void removeChild(String name, Base value) throws FHIRException { 1431 if (name.equals("identifier")) { 1432 this.getIdentifier().remove(castToIdentifier(value)); 1433 } else if (name.equals("basedOn")) { 1434 this.getBasedOn().remove(castToReference(value)); 1435 } else if (name.equals("partOf")) { 1436 this.getPartOf().remove(castToReference(value)); 1437 } else if (name.equals("status")) { 1438 this.status = null; 1439 } else if (name.equals("patient")) { 1440 this.patient = null; 1441 } else if (name.equals("type")) { 1442 this.type = null; 1443 } else if (name.equals("suppliedItem")) { 1444 this.suppliedItem = (SupplyDeliverySuppliedItemComponent) value; // SupplyDeliverySuppliedItemComponent 1445 } else if (name.equals("occurrence[x]")) { 1446 this.occurrence = null; 1447 } else if (name.equals("supplier")) { 1448 this.supplier = null; 1449 } else if (name.equals("destination")) { 1450 this.destination = null; 1451 } else if (name.equals("receiver")) { 1452 this.getReceiver().remove(castToReference(value)); 1453 } else 1454 super.removeChild(name, value); 1455 1456 } 1457 1458 @Override 1459 public Base makeProperty(int hash, String name) throws FHIRException { 1460 switch (hash) { 1461 case -1618432855: 1462 return addIdentifier(); 1463 case -332612366: 1464 return addBasedOn(); 1465 case -995410646: 1466 return addPartOf(); 1467 case -892481550: 1468 return getStatusElement(); 1469 case -791418107: 1470 return getPatient(); 1471 case 3575610: 1472 return getType(); 1473 case 1993333233: 1474 return getSuppliedItem(); 1475 case -2022646513: 1476 return getOccurrence(); 1477 case 1687874001: 1478 return getOccurrence(); 1479 case -1663305268: 1480 return getSupplier(); 1481 case -1429847026: 1482 return getDestination(); 1483 case -808719889: 1484 return addReceiver(); 1485 default: 1486 return super.makeProperty(hash, name); 1487 } 1488 1489 } 1490 1491 @Override 1492 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1493 switch (hash) { 1494 case -1618432855: 1495 /* identifier */ return new String[] { "Identifier" }; 1496 case -332612366: 1497 /* basedOn */ return new String[] { "Reference" }; 1498 case -995410646: 1499 /* partOf */ return new String[] { "Reference" }; 1500 case -892481550: 1501 /* status */ return new String[] { "code" }; 1502 case -791418107: 1503 /* patient */ return new String[] { "Reference" }; 1504 case 3575610: 1505 /* type */ return new String[] { "CodeableConcept" }; 1506 case 1993333233: 1507 /* suppliedItem */ return new String[] {}; 1508 case 1687874001: 1509 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 1510 case -1663305268: 1511 /* supplier */ return new String[] { "Reference" }; 1512 case -1429847026: 1513 /* destination */ return new String[] { "Reference" }; 1514 case -808719889: 1515 /* receiver */ return new String[] { "Reference" }; 1516 default: 1517 return super.getTypesForProperty(hash, name); 1518 } 1519 1520 } 1521 1522 @Override 1523 public Base addChild(String name) throws FHIRException { 1524 if (name.equals("identifier")) { 1525 return addIdentifier(); 1526 } else if (name.equals("basedOn")) { 1527 return addBasedOn(); 1528 } else if (name.equals("partOf")) { 1529 return addPartOf(); 1530 } else if (name.equals("status")) { 1531 throw new FHIRException("Cannot call addChild on a singleton property SupplyDelivery.status"); 1532 } else if (name.equals("patient")) { 1533 this.patient = new Reference(); 1534 return this.patient; 1535 } else if (name.equals("type")) { 1536 this.type = new CodeableConcept(); 1537 return this.type; 1538 } else if (name.equals("suppliedItem")) { 1539 this.suppliedItem = new SupplyDeliverySuppliedItemComponent(); 1540 return this.suppliedItem; 1541 } else if (name.equals("occurrenceDateTime")) { 1542 this.occurrence = new DateTimeType(); 1543 return this.occurrence; 1544 } else if (name.equals("occurrencePeriod")) { 1545 this.occurrence = new Period(); 1546 return this.occurrence; 1547 } else if (name.equals("occurrenceTiming")) { 1548 this.occurrence = new Timing(); 1549 return this.occurrence; 1550 } else if (name.equals("supplier")) { 1551 this.supplier = new Reference(); 1552 return this.supplier; 1553 } else if (name.equals("destination")) { 1554 this.destination = new Reference(); 1555 return this.destination; 1556 } else if (name.equals("receiver")) { 1557 return addReceiver(); 1558 } else 1559 return super.addChild(name); 1560 } 1561 1562 public String fhirType() { 1563 return "SupplyDelivery"; 1564 1565 } 1566 1567 public SupplyDelivery copy() { 1568 SupplyDelivery dst = new SupplyDelivery(); 1569 copyValues(dst); 1570 return dst; 1571 } 1572 1573 public void copyValues(SupplyDelivery dst) { 1574 super.copyValues(dst); 1575 if (identifier != null) { 1576 dst.identifier = new ArrayList<Identifier>(); 1577 for (Identifier i : identifier) 1578 dst.identifier.add(i.copy()); 1579 } 1580 ; 1581 if (basedOn != null) { 1582 dst.basedOn = new ArrayList<Reference>(); 1583 for (Reference i : basedOn) 1584 dst.basedOn.add(i.copy()); 1585 } 1586 ; 1587 if (partOf != null) { 1588 dst.partOf = new ArrayList<Reference>(); 1589 for (Reference i : partOf) 1590 dst.partOf.add(i.copy()); 1591 } 1592 ; 1593 dst.status = status == null ? null : status.copy(); 1594 dst.patient = patient == null ? null : patient.copy(); 1595 dst.type = type == null ? null : type.copy(); 1596 dst.suppliedItem = suppliedItem == null ? null : suppliedItem.copy(); 1597 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1598 dst.supplier = supplier == null ? null : supplier.copy(); 1599 dst.destination = destination == null ? null : destination.copy(); 1600 if (receiver != null) { 1601 dst.receiver = new ArrayList<Reference>(); 1602 for (Reference i : receiver) 1603 dst.receiver.add(i.copy()); 1604 } 1605 ; 1606 } 1607 1608 protected SupplyDelivery typedCopy() { 1609 return copy(); 1610 } 1611 1612 @Override 1613 public boolean equalsDeep(Base other_) { 1614 if (!super.equalsDeep(other_)) 1615 return false; 1616 if (!(other_ instanceof SupplyDelivery)) 1617 return false; 1618 SupplyDelivery o = (SupplyDelivery) other_; 1619 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 1620 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 1621 && compareDeep(patient, o.patient, true) && compareDeep(type, o.type, true) 1622 && compareDeep(suppliedItem, o.suppliedItem, true) && compareDeep(occurrence, o.occurrence, true) 1623 && compareDeep(supplier, o.supplier, true) && compareDeep(destination, o.destination, true) 1624 && compareDeep(receiver, o.receiver, true); 1625 } 1626 1627 @Override 1628 public boolean equalsShallow(Base other_) { 1629 if (!super.equalsShallow(other_)) 1630 return false; 1631 if (!(other_ instanceof SupplyDelivery)) 1632 return false; 1633 SupplyDelivery o = (SupplyDelivery) other_; 1634 return compareValues(status, o.status, true); 1635 } 1636 1637 public boolean isEmpty() { 1638 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, status, patient, type, 1639 suppliedItem, occurrence, supplier, destination, receiver); 1640 } 1641 1642 @Override 1643 public ResourceType getResourceType() { 1644 return ResourceType.SupplyDelivery; 1645 } 1646 1647 /** 1648 * Search parameter: <b>identifier</b> 1649 * <p> 1650 * Description: <b>External identifier</b><br> 1651 * Type: <b>token</b><br> 1652 * Path: <b>SupplyDelivery.identifier</b><br> 1653 * </p> 1654 */ 1655 @SearchParamDefinition(name = "identifier", path = "SupplyDelivery.identifier", description = "External identifier", type = "token") 1656 public static final String SP_IDENTIFIER = "identifier"; 1657 /** 1658 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1659 * <p> 1660 * Description: <b>External identifier</b><br> 1661 * Type: <b>token</b><br> 1662 * Path: <b>SupplyDelivery.identifier</b><br> 1663 * </p> 1664 */ 1665 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1666 SP_IDENTIFIER); 1667 1668 /** 1669 * Search parameter: <b>receiver</b> 1670 * <p> 1671 * Description: <b>Who collected the Supply</b><br> 1672 * Type: <b>reference</b><br> 1673 * Path: <b>SupplyDelivery.receiver</b><br> 1674 * </p> 1675 */ 1676 @SearchParamDefinition(name = "receiver", path = "SupplyDelivery.receiver", description = "Who collected the Supply", type = "reference", providesMembershipIn = { 1677 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 1678 PractitionerRole.class }) 1679 public static final String SP_RECEIVER = "receiver"; 1680 /** 1681 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 1682 * <p> 1683 * Description: <b>Who collected the Supply</b><br> 1684 * Type: <b>reference</b><br> 1685 * Path: <b>SupplyDelivery.receiver</b><br> 1686 * </p> 1687 */ 1688 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1689 SP_RECEIVER); 1690 1691 /** 1692 * Constant for fluent queries to be used to add include statements. Specifies 1693 * the path value of "<b>SupplyDelivery:receiver</b>". 1694 */ 1695 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include( 1696 "SupplyDelivery:receiver").toLocked(); 1697 1698 /** 1699 * Search parameter: <b>patient</b> 1700 * <p> 1701 * Description: <b>Patient for whom the item is supplied</b><br> 1702 * Type: <b>reference</b><br> 1703 * Path: <b>SupplyDelivery.patient</b><br> 1704 * </p> 1705 */ 1706 @SearchParamDefinition(name = "patient", path = "SupplyDelivery.patient", description = "Patient for whom the item is supplied", type = "reference", providesMembershipIn = { 1707 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 1708 public static final String SP_PATIENT = "patient"; 1709 /** 1710 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1711 * <p> 1712 * Description: <b>Patient for whom the item is supplied</b><br> 1713 * Type: <b>reference</b><br> 1714 * Path: <b>SupplyDelivery.patient</b><br> 1715 * </p> 1716 */ 1717 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1718 SP_PATIENT); 1719 1720 /** 1721 * Constant for fluent queries to be used to add include statements. Specifies 1722 * the path value of "<b>SupplyDelivery:patient</b>". 1723 */ 1724 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1725 "SupplyDelivery:patient").toLocked(); 1726 1727 /** 1728 * Search parameter: <b>supplier</b> 1729 * <p> 1730 * Description: <b>Dispenser</b><br> 1731 * Type: <b>reference</b><br> 1732 * Path: <b>SupplyDelivery.supplier</b><br> 1733 * </p> 1734 */ 1735 @SearchParamDefinition(name = "supplier", path = "SupplyDelivery.supplier", description = "Dispenser", type = "reference", providesMembershipIn = { 1736 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 1737 Practitioner.class, PractitionerRole.class }) 1738 public static final String SP_SUPPLIER = "supplier"; 1739 /** 1740 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 1741 * <p> 1742 * Description: <b>Dispenser</b><br> 1743 * Type: <b>reference</b><br> 1744 * Path: <b>SupplyDelivery.supplier</b><br> 1745 * </p> 1746 */ 1747 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1748 SP_SUPPLIER); 1749 1750 /** 1751 * Constant for fluent queries to be used to add include statements. Specifies 1752 * the path value of "<b>SupplyDelivery:supplier</b>". 1753 */ 1754 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include( 1755 "SupplyDelivery:supplier").toLocked(); 1756 1757 /** 1758 * Search parameter: <b>status</b> 1759 * <p> 1760 * Description: <b>in-progress | completed | abandoned | 1761 * entered-in-error</b><br> 1762 * Type: <b>token</b><br> 1763 * Path: <b>SupplyDelivery.status</b><br> 1764 * </p> 1765 */ 1766 @SearchParamDefinition(name = "status", path = "SupplyDelivery.status", description = "in-progress | completed | abandoned | entered-in-error", type = "token") 1767 public static final String SP_STATUS = "status"; 1768 /** 1769 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1770 * <p> 1771 * Description: <b>in-progress | completed | abandoned | 1772 * entered-in-error</b><br> 1773 * Type: <b>token</b><br> 1774 * Path: <b>SupplyDelivery.status</b><br> 1775 * </p> 1776 */ 1777 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1778 SP_STATUS); 1779 1780}