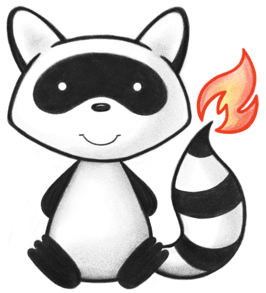
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A record of a request for a medication, substance or device used in the 048 * healthcare setting. 049 */ 050@ResourceDef(name = "SupplyRequest", profile = "http://hl7.org/fhir/StructureDefinition/SupplyRequest") 051public class SupplyRequest extends DomainResource { 052 053 public enum SupplyRequestStatus { 054 /** 055 * The request has been created but is not yet complete or ready for action. 056 */ 057 DRAFT, 058 /** 059 * The request is ready to be acted upon. 060 */ 061 ACTIVE, 062 /** 063 * The authorization/request to act has been temporarily withdrawn but is 064 * expected to resume in the future. 065 */ 066 SUSPENDED, 067 /** 068 * The authorization/request to act has been terminated prior to the full 069 * completion of the intended actions. No further activity should occur. 070 */ 071 CANCELLED, 072 /** 073 * Activity against the request has been sufficiently completed to the 074 * satisfaction of the requester. 075 */ 076 COMPLETED, 077 /** 078 * This electronic record should never have existed, though it is possible that 079 * real-world decisions were based on it. (If real-world activity has occurred, 080 * the status should be "cancelled" rather than "entered-in-error".). 081 */ 082 ENTEREDINERROR, 083 /** 084 * The authoring/source system does not know which of the status values 085 * currently applies for this observation. Note: This concept is not to be used 086 * for "other" - one of the listed statuses is presumed to apply, but the 087 * authoring/source system does not know which. 088 */ 089 UNKNOWN, 090 /** 091 * added to help the parsers with the generic types 092 */ 093 NULL; 094 095 public static SupplyRequestStatus fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("draft".equals(codeString)) 099 return DRAFT; 100 if ("active".equals(codeString)) 101 return ACTIVE; 102 if ("suspended".equals(codeString)) 103 return SUSPENDED; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("completed".equals(codeString)) 107 return COMPLETED; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 if ("unknown".equals(codeString)) 111 return UNKNOWN; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown SupplyRequestStatus code '" + codeString + "'"); 116 } 117 118 public String toCode() { 119 switch (this) { 120 case DRAFT: 121 return "draft"; 122 case ACTIVE: 123 return "active"; 124 case SUSPENDED: 125 return "suspended"; 126 case CANCELLED: 127 return "cancelled"; 128 case COMPLETED: 129 return "completed"; 130 case ENTEREDINERROR: 131 return "entered-in-error"; 132 case UNKNOWN: 133 return "unknown"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getSystem() { 142 switch (this) { 143 case DRAFT: 144 return "http://hl7.org/fhir/supplyrequest-status"; 145 case ACTIVE: 146 return "http://hl7.org/fhir/supplyrequest-status"; 147 case SUSPENDED: 148 return "http://hl7.org/fhir/supplyrequest-status"; 149 case CANCELLED: 150 return "http://hl7.org/fhir/supplyrequest-status"; 151 case COMPLETED: 152 return "http://hl7.org/fhir/supplyrequest-status"; 153 case ENTEREDINERROR: 154 return "http://hl7.org/fhir/supplyrequest-status"; 155 case UNKNOWN: 156 return "http://hl7.org/fhir/supplyrequest-status"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164 public String getDefinition() { 165 switch (this) { 166 case DRAFT: 167 return "The request has been created but is not yet complete or ready for action."; 168 case ACTIVE: 169 return "The request is ready to be acted upon."; 170 case SUSPENDED: 171 return "The authorization/request to act has been temporarily withdrawn but is expected to resume in the future."; 172 case CANCELLED: 173 return "The authorization/request to act has been terminated prior to the full completion of the intended actions. No further activity should occur."; 174 case COMPLETED: 175 return "Activity against the request has been sufficiently completed to the satisfaction of the requester."; 176 case ENTEREDINERROR: 177 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 178 case UNKNOWN: 179 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 180 case NULL: 181 return null; 182 default: 183 return "?"; 184 } 185 } 186 187 public String getDisplay() { 188 switch (this) { 189 case DRAFT: 190 return "Draft"; 191 case ACTIVE: 192 return "Active"; 193 case SUSPENDED: 194 return "Suspended"; 195 case CANCELLED: 196 return "Cancelled"; 197 case COMPLETED: 198 return "Completed"; 199 case ENTEREDINERROR: 200 return "Entered in Error"; 201 case UNKNOWN: 202 return "Unknown"; 203 case NULL: 204 return null; 205 default: 206 return "?"; 207 } 208 } 209 } 210 211 public static class SupplyRequestStatusEnumFactory implements EnumFactory<SupplyRequestStatus> { 212 public SupplyRequestStatus fromCode(String codeString) throws IllegalArgumentException { 213 if (codeString == null || "".equals(codeString)) 214 if (codeString == null || "".equals(codeString)) 215 return null; 216 if ("draft".equals(codeString)) 217 return SupplyRequestStatus.DRAFT; 218 if ("active".equals(codeString)) 219 return SupplyRequestStatus.ACTIVE; 220 if ("suspended".equals(codeString)) 221 return SupplyRequestStatus.SUSPENDED; 222 if ("cancelled".equals(codeString)) 223 return SupplyRequestStatus.CANCELLED; 224 if ("completed".equals(codeString)) 225 return SupplyRequestStatus.COMPLETED; 226 if ("entered-in-error".equals(codeString)) 227 return SupplyRequestStatus.ENTEREDINERROR; 228 if ("unknown".equals(codeString)) 229 return SupplyRequestStatus.UNKNOWN; 230 throw new IllegalArgumentException("Unknown SupplyRequestStatus code '" + codeString + "'"); 231 } 232 233 public Enumeration<SupplyRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 234 if (code == null) 235 return null; 236 if (code.isEmpty()) 237 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.NULL, code); 238 String codeString = code.asStringValue(); 239 if (codeString == null || "".equals(codeString)) 240 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.NULL, code); 241 if ("draft".equals(codeString)) 242 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.DRAFT, code); 243 if ("active".equals(codeString)) 244 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.ACTIVE, code); 245 if ("suspended".equals(codeString)) 246 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.SUSPENDED, code); 247 if ("cancelled".equals(codeString)) 248 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.CANCELLED, code); 249 if ("completed".equals(codeString)) 250 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.COMPLETED, code); 251 if ("entered-in-error".equals(codeString)) 252 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.ENTEREDINERROR, code); 253 if ("unknown".equals(codeString)) 254 return new Enumeration<SupplyRequestStatus>(this, SupplyRequestStatus.UNKNOWN, code); 255 throw new FHIRException("Unknown SupplyRequestStatus code '" + codeString + "'"); 256 } 257 258 public String toCode(SupplyRequestStatus code) { 259 if (code == SupplyRequestStatus.NULL) 260 return null; 261 if (code == SupplyRequestStatus.DRAFT) 262 return "draft"; 263 if (code == SupplyRequestStatus.ACTIVE) 264 return "active"; 265 if (code == SupplyRequestStatus.SUSPENDED) 266 return "suspended"; 267 if (code == SupplyRequestStatus.CANCELLED) 268 return "cancelled"; 269 if (code == SupplyRequestStatus.COMPLETED) 270 return "completed"; 271 if (code == SupplyRequestStatus.ENTEREDINERROR) 272 return "entered-in-error"; 273 if (code == SupplyRequestStatus.UNKNOWN) 274 return "unknown"; 275 return "?"; 276 } 277 278 public String toSystem(SupplyRequestStatus code) { 279 return code.getSystem(); 280 } 281 } 282 283 public enum RequestPriority { 284 /** 285 * The request has normal priority. 286 */ 287 ROUTINE, 288 /** 289 * The request should be actioned promptly - higher priority than routine. 290 */ 291 URGENT, 292 /** 293 * The request should be actioned as soon as possible - higher priority than 294 * urgent. 295 */ 296 ASAP, 297 /** 298 * The request should be actioned immediately - highest possible priority. E.g. 299 * an emergency. 300 */ 301 STAT, 302 /** 303 * added to help the parsers with the generic types 304 */ 305 NULL; 306 307 public static RequestPriority fromCode(String codeString) throws FHIRException { 308 if (codeString == null || "".equals(codeString)) 309 return null; 310 if ("routine".equals(codeString)) 311 return ROUTINE; 312 if ("urgent".equals(codeString)) 313 return URGENT; 314 if ("asap".equals(codeString)) 315 return ASAP; 316 if ("stat".equals(codeString)) 317 return STAT; 318 if (Configuration.isAcceptInvalidEnums()) 319 return null; 320 else 321 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 322 } 323 324 public String toCode() { 325 switch (this) { 326 case ROUTINE: 327 return "routine"; 328 case URGENT: 329 return "urgent"; 330 case ASAP: 331 return "asap"; 332 case STAT: 333 return "stat"; 334 case NULL: 335 return null; 336 default: 337 return "?"; 338 } 339 } 340 341 public String getSystem() { 342 switch (this) { 343 case ROUTINE: 344 return "http://hl7.org/fhir/request-priority"; 345 case URGENT: 346 return "http://hl7.org/fhir/request-priority"; 347 case ASAP: 348 return "http://hl7.org/fhir/request-priority"; 349 case STAT: 350 return "http://hl7.org/fhir/request-priority"; 351 case NULL: 352 return null; 353 default: 354 return "?"; 355 } 356 } 357 358 public String getDefinition() { 359 switch (this) { 360 case ROUTINE: 361 return "The request has normal priority."; 362 case URGENT: 363 return "The request should be actioned promptly - higher priority than routine."; 364 case ASAP: 365 return "The request should be actioned as soon as possible - higher priority than urgent."; 366 case STAT: 367 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 368 case NULL: 369 return null; 370 default: 371 return "?"; 372 } 373 } 374 375 public String getDisplay() { 376 switch (this) { 377 case ROUTINE: 378 return "Routine"; 379 case URGENT: 380 return "Urgent"; 381 case ASAP: 382 return "ASAP"; 383 case STAT: 384 return "STAT"; 385 case NULL: 386 return null; 387 default: 388 return "?"; 389 } 390 } 391 } 392 393 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 394 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 395 if (codeString == null || "".equals(codeString)) 396 if (codeString == null || "".equals(codeString)) 397 return null; 398 if ("routine".equals(codeString)) 399 return RequestPriority.ROUTINE; 400 if ("urgent".equals(codeString)) 401 return RequestPriority.URGENT; 402 if ("asap".equals(codeString)) 403 return RequestPriority.ASAP; 404 if ("stat".equals(codeString)) 405 return RequestPriority.STAT; 406 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 407 } 408 409 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 410 if (code == null) 411 return null; 412 if (code.isEmpty()) 413 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 414 String codeString = code.asStringValue(); 415 if (codeString == null || "".equals(codeString)) 416 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 417 if ("routine".equals(codeString)) 418 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 419 if ("urgent".equals(codeString)) 420 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 421 if ("asap".equals(codeString)) 422 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 423 if ("stat".equals(codeString)) 424 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 425 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 426 } 427 428 public String toCode(RequestPriority code) { 429 if (code == RequestPriority.NULL) 430 return null; 431 if (code == RequestPriority.ROUTINE) 432 return "routine"; 433 if (code == RequestPriority.URGENT) 434 return "urgent"; 435 if (code == RequestPriority.ASAP) 436 return "asap"; 437 if (code == RequestPriority.STAT) 438 return "stat"; 439 return "?"; 440 } 441 442 public String toSystem(RequestPriority code) { 443 return code.getSystem(); 444 } 445 } 446 447 @Block() 448 public static class SupplyRequestParameterComponent extends BackboneElement implements IBaseBackboneElement { 449 /** 450 * A code or string that identifies the device detail being asserted. 451 */ 452 @Child(name = "code", type = { 453 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 454 @Description(shortDefinition = "Item detail", formalDefinition = "A code or string that identifies the device detail being asserted.") 455 protected CodeableConcept code; 456 457 /** 458 * The value of the device detail. 459 */ 460 @Child(name = "value", type = { CodeableConcept.class, Quantity.class, Range.class, 461 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 462 @Description(shortDefinition = "Value of detail", formalDefinition = "The value of the device detail.") 463 protected Type value; 464 465 private static final long serialVersionUID = 884525025L; 466 467 /** 468 * Constructor 469 */ 470 public SupplyRequestParameterComponent() { 471 super(); 472 } 473 474 /** 475 * @return {@link #code} (A code or string that identifies the device detail 476 * being asserted.) 477 */ 478 public CodeableConcept getCode() { 479 if (this.code == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create SupplyRequestParameterComponent.code"); 482 else if (Configuration.doAutoCreate()) 483 this.code = new CodeableConcept(); // cc 484 return this.code; 485 } 486 487 public boolean hasCode() { 488 return this.code != null && !this.code.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #code} (A code or string that identifies the device 493 * detail being asserted.) 494 */ 495 public SupplyRequestParameterComponent setCode(CodeableConcept value) { 496 this.code = value; 497 return this; 498 } 499 500 /** 501 * @return {@link #value} (The value of the device detail.) 502 */ 503 public Type getValue() { 504 return this.value; 505 } 506 507 /** 508 * @return {@link #value} (The value of the device detail.) 509 */ 510 public CodeableConcept getValueCodeableConcept() throws FHIRException { 511 if (this.value == null) 512 this.value = new CodeableConcept(); 513 if (!(this.value instanceof CodeableConcept)) 514 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 515 + this.value.getClass().getName() + " was encountered"); 516 return (CodeableConcept) this.value; 517 } 518 519 public boolean hasValueCodeableConcept() { 520 return this.value instanceof CodeableConcept; 521 } 522 523 /** 524 * @return {@link #value} (The value of the device detail.) 525 */ 526 public Quantity getValueQuantity() throws FHIRException { 527 if (this.value == null) 528 this.value = new Quantity(); 529 if (!(this.value instanceof Quantity)) 530 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 531 + " was encountered"); 532 return (Quantity) this.value; 533 } 534 535 public boolean hasValueQuantity() { 536 return this.value instanceof Quantity; 537 } 538 539 /** 540 * @return {@link #value} (The value of the device detail.) 541 */ 542 public Range getValueRange() throws FHIRException { 543 if (this.value == null) 544 this.value = new Range(); 545 if (!(this.value instanceof Range)) 546 throw new FHIRException( 547 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 548 return (Range) this.value; 549 } 550 551 public boolean hasValueRange() { 552 return this.value instanceof Range; 553 } 554 555 /** 556 * @return {@link #value} (The value of the device detail.) 557 */ 558 public BooleanType getValueBooleanType() throws FHIRException { 559 if (this.value == null) 560 this.value = new BooleanType(); 561 if (!(this.value instanceof BooleanType)) 562 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 563 + this.value.getClass().getName() + " was encountered"); 564 return (BooleanType) this.value; 565 } 566 567 public boolean hasValueBooleanType() { 568 return this.value instanceof BooleanType; 569 } 570 571 public boolean hasValue() { 572 return this.value != null && !this.value.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #value} (The value of the device detail.) 577 */ 578 public SupplyRequestParameterComponent setValue(Type value) { 579 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range 580 || value instanceof BooleanType)) 581 throw new Error("Not the right type for SupplyRequest.parameter.value[x]: " + value.fhirType()); 582 this.value = value; 583 return this; 584 } 585 586 protected void listChildren(List<Property> children) { 587 super.listChildren(children); 588 children.add(new Property("code", "CodeableConcept", 589 "A code or string that identifies the device detail being asserted.", 0, 1, code)); 590 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", "The value of the device detail.", 591 0, 1, value)); 592 } 593 594 @Override 595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 596 switch (_hash) { 597 case 3059181: 598 /* code */ return new Property("code", "CodeableConcept", 599 "A code or string that identifies the device detail being asserted.", 0, 1, code); 600 case -1410166417: 601 /* value[x] */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 602 "The value of the device detail.", 0, 1, value); 603 case 111972721: 604 /* value */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 605 "The value of the device detail.", 0, 1, value); 606 case 924902896: 607 /* valueCodeableConcept */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 608 "The value of the device detail.", 0, 1, value); 609 case -2029823716: 610 /* valueQuantity */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 611 "The value of the device detail.", 0, 1, value); 612 case 2030761548: 613 /* valueRange */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 614 "The value of the device detail.", 0, 1, value); 615 case 733421943: 616 /* valueBoolean */ return new Property("value[x]", "CodeableConcept|Quantity|Range|boolean", 617 "The value of the device detail.", 0, 1, value); 618 default: 619 return super.getNamedProperty(_hash, _name, _checkValid); 620 } 621 622 } 623 624 @Override 625 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 626 switch (hash) { 627 case 3059181: 628 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 629 case 111972721: 630 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 631 default: 632 return super.getProperty(hash, name, checkValid); 633 } 634 635 } 636 637 @Override 638 public Base setProperty(int hash, String name, Base value) throws FHIRException { 639 switch (hash) { 640 case 3059181: // code 641 this.code = castToCodeableConcept(value); // CodeableConcept 642 return value; 643 case 111972721: // value 644 this.value = castToType(value); // Type 645 return value; 646 default: 647 return super.setProperty(hash, name, value); 648 } 649 650 } 651 652 @Override 653 public Base setProperty(String name, Base value) throws FHIRException { 654 if (name.equals("code")) { 655 this.code = castToCodeableConcept(value); // CodeableConcept 656 } else if (name.equals("value[x]")) { 657 this.value = castToType(value); // Type 658 } else 659 return super.setProperty(name, value); 660 return value; 661 } 662 663 @Override 664 public void removeChild(String name, Base value) throws FHIRException { 665 if (name.equals("code")) { 666 this.code = null; 667 } else if (name.equals("value[x]")) { 668 this.value = null; 669 } else 670 super.removeChild(name, value); 671 672 } 673 674 @Override 675 public Base makeProperty(int hash, String name) throws FHIRException { 676 switch (hash) { 677 case 3059181: 678 return getCode(); 679 case -1410166417: 680 return getValue(); 681 case 111972721: 682 return getValue(); 683 default: 684 return super.makeProperty(hash, name); 685 } 686 687 } 688 689 @Override 690 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 691 switch (hash) { 692 case 3059181: 693 /* code */ return new String[] { "CodeableConcept" }; 694 case 111972721: 695 /* value */ return new String[] { "CodeableConcept", "Quantity", "Range", "boolean" }; 696 default: 697 return super.getTypesForProperty(hash, name); 698 } 699 700 } 701 702 @Override 703 public Base addChild(String name) throws FHIRException { 704 if (name.equals("code")) { 705 this.code = new CodeableConcept(); 706 return this.code; 707 } else if (name.equals("valueCodeableConcept")) { 708 this.value = new CodeableConcept(); 709 return this.value; 710 } else if (name.equals("valueQuantity")) { 711 this.value = new Quantity(); 712 return this.value; 713 } else if (name.equals("valueRange")) { 714 this.value = new Range(); 715 return this.value; 716 } else if (name.equals("valueBoolean")) { 717 this.value = new BooleanType(); 718 return this.value; 719 } else 720 return super.addChild(name); 721 } 722 723 public SupplyRequestParameterComponent copy() { 724 SupplyRequestParameterComponent dst = new SupplyRequestParameterComponent(); 725 copyValues(dst); 726 return dst; 727 } 728 729 public void copyValues(SupplyRequestParameterComponent dst) { 730 super.copyValues(dst); 731 dst.code = code == null ? null : code.copy(); 732 dst.value = value == null ? null : value.copy(); 733 } 734 735 @Override 736 public boolean equalsDeep(Base other_) { 737 if (!super.equalsDeep(other_)) 738 return false; 739 if (!(other_ instanceof SupplyRequestParameterComponent)) 740 return false; 741 SupplyRequestParameterComponent o = (SupplyRequestParameterComponent) other_; 742 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 743 } 744 745 @Override 746 public boolean equalsShallow(Base other_) { 747 if (!super.equalsShallow(other_)) 748 return false; 749 if (!(other_ instanceof SupplyRequestParameterComponent)) 750 return false; 751 SupplyRequestParameterComponent o = (SupplyRequestParameterComponent) other_; 752 return true; 753 } 754 755 public boolean isEmpty() { 756 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 757 } 758 759 public String fhirType() { 760 return "SupplyRequest.parameter"; 761 762 } 763 764 } 765 766 /** 767 * Business identifiers assigned to this SupplyRequest by the author and/or 768 * other systems. These identifiers remain constant as the resource is updated 769 * and propagates from server to server. 770 */ 771 @Child(name = "identifier", type = { 772 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 773 @Description(shortDefinition = "Business Identifier for SupplyRequest", formalDefinition = "Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.") 774 protected List<Identifier> identifier; 775 776 /** 777 * Status of the supply request. 778 */ 779 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 780 @Description(shortDefinition = "draft | active | suspended +", formalDefinition = "Status of the supply request.") 781 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplyrequest-status") 782 protected Enumeration<SupplyRequestStatus> status; 783 784 /** 785 * Category of supply, e.g. central, non-stock, etc. This is used to support 786 * work flows associated with the supply process. 787 */ 788 @Child(name = "category", type = { 789 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 790 @Description(shortDefinition = "The kind of supply (central, non-stock, etc.)", formalDefinition = "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.") 791 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplyrequest-kind") 792 protected CodeableConcept category; 793 794 /** 795 * Indicates how quickly this SupplyRequest should be addressed with respect to 796 * other requests. 797 */ 798 @Child(name = "priority", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 799 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.") 800 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 801 protected Enumeration<RequestPriority> priority; 802 803 /** 804 * The item that is requested to be supplied. This is either a link to a 805 * resource representing the details of the item or a code that identifies the 806 * item from a known list. 807 */ 808 @Child(name = "item", type = { CodeableConcept.class, Medication.class, Substance.class, 809 Device.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 810 @Description(shortDefinition = "Medication, Substance, or Device requested to be supplied", formalDefinition = "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.") 811 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supply-item") 812 protected Type item; 813 814 /** 815 * The amount that is being ordered of the indicated item. 816 */ 817 @Child(name = "quantity", type = { Quantity.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 818 @Description(shortDefinition = "The requested amount of the item indicated", formalDefinition = "The amount that is being ordered of the indicated item.") 819 protected Quantity quantity; 820 821 /** 822 * Specific parameters for the ordered item. For example, the size of the 823 * indicated item. 824 */ 825 @Child(name = "parameter", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 826 @Description(shortDefinition = "Ordered item details", formalDefinition = "Specific parameters for the ordered item. For example, the size of the indicated item.") 827 protected List<SupplyRequestParameterComponent> parameter; 828 829 /** 830 * When the request should be fulfilled. 831 */ 832 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 833 Timing.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 834 @Description(shortDefinition = "When the request should be fulfilled", formalDefinition = "When the request should be fulfilled.") 835 protected Type occurrence; 836 837 /** 838 * When the request was made. 839 */ 840 @Child(name = "authoredOn", type = { 841 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 842 @Description(shortDefinition = "When the request was made", formalDefinition = "When the request was made.") 843 protected DateTimeType authoredOn; 844 845 /** 846 * The device, practitioner, etc. who initiated the request. 847 */ 848 @Child(name = "requester", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 849 RelatedPerson.class, Device.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 850 @Description(shortDefinition = "Individual making the request", formalDefinition = "The device, practitioner, etc. who initiated the request.") 851 protected Reference requester; 852 853 /** 854 * The actual object that is the target of the reference (The device, 855 * practitioner, etc. who initiated the request.) 856 */ 857 protected Resource requesterTarget; 858 859 /** 860 * Who is intended to fulfill the request. 861 */ 862 @Child(name = "supplier", type = { Organization.class, 863 HealthcareService.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 864 @Description(shortDefinition = "Who is intended to fulfill the request", formalDefinition = "Who is intended to fulfill the request.") 865 protected List<Reference> supplier; 866 /** 867 * The actual objects that are the target of the reference (Who is intended to 868 * fulfill the request.) 869 */ 870 protected List<Resource> supplierTarget; 871 872 /** 873 * The reason why the supply item was requested. 874 */ 875 @Child(name = "reasonCode", type = { 876 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 877 @Description(shortDefinition = "The reason why the supply item was requested", formalDefinition = "The reason why the supply item was requested.") 878 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplyrequest-reason") 879 protected List<CodeableConcept> reasonCode; 880 881 /** 882 * The reason why the supply item was requested. 883 */ 884 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 885 DocumentReference.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 886 @Description(shortDefinition = "The reason why the supply item was requested", formalDefinition = "The reason why the supply item was requested.") 887 protected List<Reference> reasonReference; 888 /** 889 * The actual objects that are the target of the reference (The reason why the 890 * supply item was requested.) 891 */ 892 protected List<Resource> reasonReferenceTarget; 893 894 /** 895 * Where the supply is expected to come from. 896 */ 897 @Child(name = "deliverFrom", type = { Organization.class, 898 Location.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 899 @Description(shortDefinition = "The origin of the supply", formalDefinition = "Where the supply is expected to come from.") 900 protected Reference deliverFrom; 901 902 /** 903 * The actual object that is the target of the reference (Where the supply is 904 * expected to come from.) 905 */ 906 protected Resource deliverFromTarget; 907 908 /** 909 * Where the supply is destined to go. 910 */ 911 @Child(name = "deliverTo", type = { Organization.class, Location.class, 912 Patient.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 913 @Description(shortDefinition = "The destination of the supply", formalDefinition = "Where the supply is destined to go.") 914 protected Reference deliverTo; 915 916 /** 917 * The actual object that is the target of the reference (Where the supply is 918 * destined to go.) 919 */ 920 protected Resource deliverToTarget; 921 922 private static final long serialVersionUID = 1456312151L; 923 924 /** 925 * Constructor 926 */ 927 public SupplyRequest() { 928 super(); 929 } 930 931 /** 932 * Constructor 933 */ 934 public SupplyRequest(Type item, Quantity quantity) { 935 super(); 936 this.item = item; 937 this.quantity = quantity; 938 } 939 940 /** 941 * @return {@link #identifier} (Business identifiers assigned to this 942 * SupplyRequest by the author and/or other systems. These identifiers 943 * remain constant as the resource is updated and propagates from server 944 * to server.) 945 */ 946 public List<Identifier> getIdentifier() { 947 if (this.identifier == null) 948 this.identifier = new ArrayList<Identifier>(); 949 return this.identifier; 950 } 951 952 /** 953 * @return Returns a reference to <code>this</code> for easy method chaining 954 */ 955 public SupplyRequest setIdentifier(List<Identifier> theIdentifier) { 956 this.identifier = theIdentifier; 957 return this; 958 } 959 960 public boolean hasIdentifier() { 961 if (this.identifier == null) 962 return false; 963 for (Identifier item : this.identifier) 964 if (!item.isEmpty()) 965 return true; 966 return false; 967 } 968 969 public Identifier addIdentifier() { // 3 970 Identifier t = new Identifier(); 971 if (this.identifier == null) 972 this.identifier = new ArrayList<Identifier>(); 973 this.identifier.add(t); 974 return t; 975 } 976 977 public SupplyRequest addIdentifier(Identifier t) { // 3 978 if (t == null) 979 return this; 980 if (this.identifier == null) 981 this.identifier = new ArrayList<Identifier>(); 982 this.identifier.add(t); 983 return this; 984 } 985 986 /** 987 * @return The first repetition of repeating field {@link #identifier}, creating 988 * it if it does not already exist 989 */ 990 public Identifier getIdentifierFirstRep() { 991 if (getIdentifier().isEmpty()) { 992 addIdentifier(); 993 } 994 return getIdentifier().get(0); 995 } 996 997 /** 998 * @return {@link #status} (Status of the supply request.). This is the 999 * underlying object with id, value and extensions. The accessor 1000 * "getStatus" gives direct access to the value 1001 */ 1002 public Enumeration<SupplyRequestStatus> getStatusElement() { 1003 if (this.status == null) 1004 if (Configuration.errorOnAutoCreate()) 1005 throw new Error("Attempt to auto-create SupplyRequest.status"); 1006 else if (Configuration.doAutoCreate()) 1007 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); // bb 1008 return this.status; 1009 } 1010 1011 public boolean hasStatusElement() { 1012 return this.status != null && !this.status.isEmpty(); 1013 } 1014 1015 public boolean hasStatus() { 1016 return this.status != null && !this.status.isEmpty(); 1017 } 1018 1019 /** 1020 * @param value {@link #status} (Status of the supply request.). This is the 1021 * underlying object with id, value and extensions. The accessor 1022 * "getStatus" gives direct access to the value 1023 */ 1024 public SupplyRequest setStatusElement(Enumeration<SupplyRequestStatus> value) { 1025 this.status = value; 1026 return this; 1027 } 1028 1029 /** 1030 * @return Status of the supply request. 1031 */ 1032 public SupplyRequestStatus getStatus() { 1033 return this.status == null ? null : this.status.getValue(); 1034 } 1035 1036 /** 1037 * @param value Status of the supply request. 1038 */ 1039 public SupplyRequest setStatus(SupplyRequestStatus value) { 1040 if (value == null) 1041 this.status = null; 1042 else { 1043 if (this.status == null) 1044 this.status = new Enumeration<SupplyRequestStatus>(new SupplyRequestStatusEnumFactory()); 1045 this.status.setValue(value); 1046 } 1047 return this; 1048 } 1049 1050 /** 1051 * @return {@link #category} (Category of supply, e.g. central, non-stock, etc. 1052 * This is used to support work flows associated with the supply 1053 * process.) 1054 */ 1055 public CodeableConcept getCategory() { 1056 if (this.category == null) 1057 if (Configuration.errorOnAutoCreate()) 1058 throw new Error("Attempt to auto-create SupplyRequest.category"); 1059 else if (Configuration.doAutoCreate()) 1060 this.category = new CodeableConcept(); // cc 1061 return this.category; 1062 } 1063 1064 public boolean hasCategory() { 1065 return this.category != null && !this.category.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #category} (Category of supply, e.g. central, non-stock, 1070 * etc. This is used to support work flows associated with the 1071 * supply process.) 1072 */ 1073 public SupplyRequest setCategory(CodeableConcept value) { 1074 this.category = value; 1075 return this; 1076 } 1077 1078 /** 1079 * @return {@link #priority} (Indicates how quickly this SupplyRequest should be 1080 * addressed with respect to other requests.). This is the underlying 1081 * object with id, value and extensions. The accessor "getPriority" 1082 * gives direct access to the value 1083 */ 1084 public Enumeration<RequestPriority> getPriorityElement() { 1085 if (this.priority == null) 1086 if (Configuration.errorOnAutoCreate()) 1087 throw new Error("Attempt to auto-create SupplyRequest.priority"); 1088 else if (Configuration.doAutoCreate()) 1089 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 1090 return this.priority; 1091 } 1092 1093 public boolean hasPriorityElement() { 1094 return this.priority != null && !this.priority.isEmpty(); 1095 } 1096 1097 public boolean hasPriority() { 1098 return this.priority != null && !this.priority.isEmpty(); 1099 } 1100 1101 /** 1102 * @param value {@link #priority} (Indicates how quickly this SupplyRequest 1103 * should be addressed with respect to other requests.). This is 1104 * the underlying object with id, value and extensions. The 1105 * accessor "getPriority" gives direct access to the value 1106 */ 1107 public SupplyRequest setPriorityElement(Enumeration<RequestPriority> value) { 1108 this.priority = value; 1109 return this; 1110 } 1111 1112 /** 1113 * @return Indicates how quickly this SupplyRequest should be addressed with 1114 * respect to other requests. 1115 */ 1116 public RequestPriority getPriority() { 1117 return this.priority == null ? null : this.priority.getValue(); 1118 } 1119 1120 /** 1121 * @param value Indicates how quickly this SupplyRequest should be addressed 1122 * with respect to other requests. 1123 */ 1124 public SupplyRequest setPriority(RequestPriority value) { 1125 if (value == null) 1126 this.priority = null; 1127 else { 1128 if (this.priority == null) 1129 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 1130 this.priority.setValue(value); 1131 } 1132 return this; 1133 } 1134 1135 /** 1136 * @return {@link #item} (The item that is requested to be supplied. This is 1137 * either a link to a resource representing the details of the item or a 1138 * code that identifies the item from a known list.) 1139 */ 1140 public Type getItem() { 1141 return this.item; 1142 } 1143 1144 /** 1145 * @return {@link #item} (The item that is requested to be supplied. This is 1146 * either a link to a resource representing the details of the item or a 1147 * code that identifies the item from a known list.) 1148 */ 1149 public CodeableConcept getItemCodeableConcept() throws FHIRException { 1150 if (this.item == null) 1151 this.item = new CodeableConcept(); 1152 if (!(this.item instanceof CodeableConcept)) 1153 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1154 + this.item.getClass().getName() + " was encountered"); 1155 return (CodeableConcept) this.item; 1156 } 1157 1158 public boolean hasItemCodeableConcept() { 1159 return this.item instanceof CodeableConcept; 1160 } 1161 1162 /** 1163 * @return {@link #item} (The item that is requested to be supplied. This is 1164 * either a link to a resource representing the details of the item or a 1165 * code that identifies the item from a known list.) 1166 */ 1167 public Reference getItemReference() throws FHIRException { 1168 if (this.item == null) 1169 this.item = new Reference(); 1170 if (!(this.item instanceof Reference)) 1171 throw new FHIRException( 1172 "Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() + " was encountered"); 1173 return (Reference) this.item; 1174 } 1175 1176 public boolean hasItemReference() { 1177 return this.item instanceof Reference; 1178 } 1179 1180 public boolean hasItem() { 1181 return this.item != null && !this.item.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #item} (The item that is requested to be supplied. This 1186 * is either a link to a resource representing the details of the 1187 * item or a code that identifies the item from a known list.) 1188 */ 1189 public SupplyRequest setItem(Type value) { 1190 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1191 throw new Error("Not the right type for SupplyRequest.item[x]: " + value.fhirType()); 1192 this.item = value; 1193 return this; 1194 } 1195 1196 /** 1197 * @return {@link #quantity} (The amount that is being ordered of the indicated 1198 * item.) 1199 */ 1200 public Quantity getQuantity() { 1201 if (this.quantity == null) 1202 if (Configuration.errorOnAutoCreate()) 1203 throw new Error("Attempt to auto-create SupplyRequest.quantity"); 1204 else if (Configuration.doAutoCreate()) 1205 this.quantity = new Quantity(); // cc 1206 return this.quantity; 1207 } 1208 1209 public boolean hasQuantity() { 1210 return this.quantity != null && !this.quantity.isEmpty(); 1211 } 1212 1213 /** 1214 * @param value {@link #quantity} (The amount that is being ordered of the 1215 * indicated item.) 1216 */ 1217 public SupplyRequest setQuantity(Quantity value) { 1218 this.quantity = value; 1219 return this; 1220 } 1221 1222 /** 1223 * @return {@link #parameter} (Specific parameters for the ordered item. For 1224 * example, the size of the indicated item.) 1225 */ 1226 public List<SupplyRequestParameterComponent> getParameter() { 1227 if (this.parameter == null) 1228 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 1229 return this.parameter; 1230 } 1231 1232 /** 1233 * @return Returns a reference to <code>this</code> for easy method chaining 1234 */ 1235 public SupplyRequest setParameter(List<SupplyRequestParameterComponent> theParameter) { 1236 this.parameter = theParameter; 1237 return this; 1238 } 1239 1240 public boolean hasParameter() { 1241 if (this.parameter == null) 1242 return false; 1243 for (SupplyRequestParameterComponent item : this.parameter) 1244 if (!item.isEmpty()) 1245 return true; 1246 return false; 1247 } 1248 1249 public SupplyRequestParameterComponent addParameter() { // 3 1250 SupplyRequestParameterComponent t = new SupplyRequestParameterComponent(); 1251 if (this.parameter == null) 1252 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 1253 this.parameter.add(t); 1254 return t; 1255 } 1256 1257 public SupplyRequest addParameter(SupplyRequestParameterComponent t) { // 3 1258 if (t == null) 1259 return this; 1260 if (this.parameter == null) 1261 this.parameter = new ArrayList<SupplyRequestParameterComponent>(); 1262 this.parameter.add(t); 1263 return this; 1264 } 1265 1266 /** 1267 * @return The first repetition of repeating field {@link #parameter}, creating 1268 * it if it does not already exist 1269 */ 1270 public SupplyRequestParameterComponent getParameterFirstRep() { 1271 if (getParameter().isEmpty()) { 1272 addParameter(); 1273 } 1274 return getParameter().get(0); 1275 } 1276 1277 /** 1278 * @return {@link #occurrence} (When the request should be fulfilled.) 1279 */ 1280 public Type getOccurrence() { 1281 return this.occurrence; 1282 } 1283 1284 /** 1285 * @return {@link #occurrence} (When the request should be fulfilled.) 1286 */ 1287 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1288 if (this.occurrence == null) 1289 this.occurrence = new DateTimeType(); 1290 if (!(this.occurrence instanceof DateTimeType)) 1291 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1292 + this.occurrence.getClass().getName() + " was encountered"); 1293 return (DateTimeType) this.occurrence; 1294 } 1295 1296 public boolean hasOccurrenceDateTimeType() { 1297 return this.occurrence instanceof DateTimeType; 1298 } 1299 1300 /** 1301 * @return {@link #occurrence} (When the request should be fulfilled.) 1302 */ 1303 public Period getOccurrencePeriod() throws FHIRException { 1304 if (this.occurrence == null) 1305 this.occurrence = new Period(); 1306 if (!(this.occurrence instanceof Period)) 1307 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1308 + " was encountered"); 1309 return (Period) this.occurrence; 1310 } 1311 1312 public boolean hasOccurrencePeriod() { 1313 return this.occurrence instanceof Period; 1314 } 1315 1316 /** 1317 * @return {@link #occurrence} (When the request should be fulfilled.) 1318 */ 1319 public Timing getOccurrenceTiming() throws FHIRException { 1320 if (this.occurrence == null) 1321 this.occurrence = new Timing(); 1322 if (!(this.occurrence instanceof Timing)) 1323 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.occurrence.getClass().getName() 1324 + " was encountered"); 1325 return (Timing) this.occurrence; 1326 } 1327 1328 public boolean hasOccurrenceTiming() { 1329 return this.occurrence instanceof Timing; 1330 } 1331 1332 public boolean hasOccurrence() { 1333 return this.occurrence != null && !this.occurrence.isEmpty(); 1334 } 1335 1336 /** 1337 * @param value {@link #occurrence} (When the request should be fulfilled.) 1338 */ 1339 public SupplyRequest setOccurrence(Type value) { 1340 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1341 throw new Error("Not the right type for SupplyRequest.occurrence[x]: " + value.fhirType()); 1342 this.occurrence = value; 1343 return this; 1344 } 1345 1346 /** 1347 * @return {@link #authoredOn} (When the request was made.). This is the 1348 * underlying object with id, value and extensions. The accessor 1349 * "getAuthoredOn" gives direct access to the value 1350 */ 1351 public DateTimeType getAuthoredOnElement() { 1352 if (this.authoredOn == null) 1353 if (Configuration.errorOnAutoCreate()) 1354 throw new Error("Attempt to auto-create SupplyRequest.authoredOn"); 1355 else if (Configuration.doAutoCreate()) 1356 this.authoredOn = new DateTimeType(); // bb 1357 return this.authoredOn; 1358 } 1359 1360 public boolean hasAuthoredOnElement() { 1361 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1362 } 1363 1364 public boolean hasAuthoredOn() { 1365 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1366 } 1367 1368 /** 1369 * @param value {@link #authoredOn} (When the request was made.). This is the 1370 * underlying object with id, value and extensions. The accessor 1371 * "getAuthoredOn" gives direct access to the value 1372 */ 1373 public SupplyRequest setAuthoredOnElement(DateTimeType value) { 1374 this.authoredOn = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return When the request was made. 1380 */ 1381 public Date getAuthoredOn() { 1382 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1383 } 1384 1385 /** 1386 * @param value When the request was made. 1387 */ 1388 public SupplyRequest setAuthoredOn(Date value) { 1389 if (value == null) 1390 this.authoredOn = null; 1391 else { 1392 if (this.authoredOn == null) 1393 this.authoredOn = new DateTimeType(); 1394 this.authoredOn.setValue(value); 1395 } 1396 return this; 1397 } 1398 1399 /** 1400 * @return {@link #requester} (The device, practitioner, etc. who initiated the 1401 * request.) 1402 */ 1403 public Reference getRequester() { 1404 if (this.requester == null) 1405 if (Configuration.errorOnAutoCreate()) 1406 throw new Error("Attempt to auto-create SupplyRequest.requester"); 1407 else if (Configuration.doAutoCreate()) 1408 this.requester = new Reference(); // cc 1409 return this.requester; 1410 } 1411 1412 public boolean hasRequester() { 1413 return this.requester != null && !this.requester.isEmpty(); 1414 } 1415 1416 /** 1417 * @param value {@link #requester} (The device, practitioner, etc. who initiated 1418 * the request.) 1419 */ 1420 public SupplyRequest setRequester(Reference value) { 1421 this.requester = value; 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #requester} The actual object that is the target of the 1427 * reference. The reference library doesn't populate this, but you can 1428 * use it to hold the resource if you resolve it. (The device, 1429 * practitioner, etc. who initiated the request.) 1430 */ 1431 public Resource getRequesterTarget() { 1432 return this.requesterTarget; 1433 } 1434 1435 /** 1436 * @param value {@link #requester} The actual object that is the target of the 1437 * reference. The reference library doesn't use these, but you can 1438 * use it to hold the resource if you resolve it. (The device, 1439 * practitioner, etc. who initiated the request.) 1440 */ 1441 public SupplyRequest setRequesterTarget(Resource value) { 1442 this.requesterTarget = value; 1443 return this; 1444 } 1445 1446 /** 1447 * @return {@link #supplier} (Who is intended to fulfill the request.) 1448 */ 1449 public List<Reference> getSupplier() { 1450 if (this.supplier == null) 1451 this.supplier = new ArrayList<Reference>(); 1452 return this.supplier; 1453 } 1454 1455 /** 1456 * @return Returns a reference to <code>this</code> for easy method chaining 1457 */ 1458 public SupplyRequest setSupplier(List<Reference> theSupplier) { 1459 this.supplier = theSupplier; 1460 return this; 1461 } 1462 1463 public boolean hasSupplier() { 1464 if (this.supplier == null) 1465 return false; 1466 for (Reference item : this.supplier) 1467 if (!item.isEmpty()) 1468 return true; 1469 return false; 1470 } 1471 1472 public Reference addSupplier() { // 3 1473 Reference t = new Reference(); 1474 if (this.supplier == null) 1475 this.supplier = new ArrayList<Reference>(); 1476 this.supplier.add(t); 1477 return t; 1478 } 1479 1480 public SupplyRequest addSupplier(Reference t) { // 3 1481 if (t == null) 1482 return this; 1483 if (this.supplier == null) 1484 this.supplier = new ArrayList<Reference>(); 1485 this.supplier.add(t); 1486 return this; 1487 } 1488 1489 /** 1490 * @return The first repetition of repeating field {@link #supplier}, creating 1491 * it if it does not already exist 1492 */ 1493 public Reference getSupplierFirstRep() { 1494 if (getSupplier().isEmpty()) { 1495 addSupplier(); 1496 } 1497 return getSupplier().get(0); 1498 } 1499 1500 /** 1501 * @return {@link #reasonCode} (The reason why the supply item was requested.) 1502 */ 1503 public List<CodeableConcept> getReasonCode() { 1504 if (this.reasonCode == null) 1505 this.reasonCode = new ArrayList<CodeableConcept>(); 1506 return this.reasonCode; 1507 } 1508 1509 /** 1510 * @return Returns a reference to <code>this</code> for easy method chaining 1511 */ 1512 public SupplyRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1513 this.reasonCode = theReasonCode; 1514 return this; 1515 } 1516 1517 public boolean hasReasonCode() { 1518 if (this.reasonCode == null) 1519 return false; 1520 for (CodeableConcept item : this.reasonCode) 1521 if (!item.isEmpty()) 1522 return true; 1523 return false; 1524 } 1525 1526 public CodeableConcept addReasonCode() { // 3 1527 CodeableConcept t = new CodeableConcept(); 1528 if (this.reasonCode == null) 1529 this.reasonCode = new ArrayList<CodeableConcept>(); 1530 this.reasonCode.add(t); 1531 return t; 1532 } 1533 1534 public SupplyRequest addReasonCode(CodeableConcept t) { // 3 1535 if (t == null) 1536 return this; 1537 if (this.reasonCode == null) 1538 this.reasonCode = new ArrayList<CodeableConcept>(); 1539 this.reasonCode.add(t); 1540 return this; 1541 } 1542 1543 /** 1544 * @return The first repetition of repeating field {@link #reasonCode}, creating 1545 * it if it does not already exist 1546 */ 1547 public CodeableConcept getReasonCodeFirstRep() { 1548 if (getReasonCode().isEmpty()) { 1549 addReasonCode(); 1550 } 1551 return getReasonCode().get(0); 1552 } 1553 1554 /** 1555 * @return {@link #reasonReference} (The reason why the supply item was 1556 * requested.) 1557 */ 1558 public List<Reference> getReasonReference() { 1559 if (this.reasonReference == null) 1560 this.reasonReference = new ArrayList<Reference>(); 1561 return this.reasonReference; 1562 } 1563 1564 /** 1565 * @return Returns a reference to <code>this</code> for easy method chaining 1566 */ 1567 public SupplyRequest setReasonReference(List<Reference> theReasonReference) { 1568 this.reasonReference = theReasonReference; 1569 return this; 1570 } 1571 1572 public boolean hasReasonReference() { 1573 if (this.reasonReference == null) 1574 return false; 1575 for (Reference item : this.reasonReference) 1576 if (!item.isEmpty()) 1577 return true; 1578 return false; 1579 } 1580 1581 public Reference addReasonReference() { // 3 1582 Reference t = new Reference(); 1583 if (this.reasonReference == null) 1584 this.reasonReference = new ArrayList<Reference>(); 1585 this.reasonReference.add(t); 1586 return t; 1587 } 1588 1589 public SupplyRequest addReasonReference(Reference t) { // 3 1590 if (t == null) 1591 return this; 1592 if (this.reasonReference == null) 1593 this.reasonReference = new ArrayList<Reference>(); 1594 this.reasonReference.add(t); 1595 return this; 1596 } 1597 1598 /** 1599 * @return The first repetition of repeating field {@link #reasonReference}, 1600 * creating it if it does not already exist 1601 */ 1602 public Reference getReasonReferenceFirstRep() { 1603 if (getReasonReference().isEmpty()) { 1604 addReasonReference(); 1605 } 1606 return getReasonReference().get(0); 1607 } 1608 1609 /** 1610 * @return {@link #deliverFrom} (Where the supply is expected to come from.) 1611 */ 1612 public Reference getDeliverFrom() { 1613 if (this.deliverFrom == null) 1614 if (Configuration.errorOnAutoCreate()) 1615 throw new Error("Attempt to auto-create SupplyRequest.deliverFrom"); 1616 else if (Configuration.doAutoCreate()) 1617 this.deliverFrom = new Reference(); // cc 1618 return this.deliverFrom; 1619 } 1620 1621 public boolean hasDeliverFrom() { 1622 return this.deliverFrom != null && !this.deliverFrom.isEmpty(); 1623 } 1624 1625 /** 1626 * @param value {@link #deliverFrom} (Where the supply is expected to come 1627 * from.) 1628 */ 1629 public SupplyRequest setDeliverFrom(Reference value) { 1630 this.deliverFrom = value; 1631 return this; 1632 } 1633 1634 /** 1635 * @return {@link #deliverFrom} The actual object that is the target of the 1636 * reference. The reference library doesn't populate this, but you can 1637 * use it to hold the resource if you resolve it. (Where the supply is 1638 * expected to come from.) 1639 */ 1640 public Resource getDeliverFromTarget() { 1641 return this.deliverFromTarget; 1642 } 1643 1644 /** 1645 * @param value {@link #deliverFrom} The actual object that is the target of the 1646 * reference. The reference library doesn't use these, but you can 1647 * use it to hold the resource if you resolve it. (Where the supply 1648 * is expected to come from.) 1649 */ 1650 public SupplyRequest setDeliverFromTarget(Resource value) { 1651 this.deliverFromTarget = value; 1652 return this; 1653 } 1654 1655 /** 1656 * @return {@link #deliverTo} (Where the supply is destined to go.) 1657 */ 1658 public Reference getDeliverTo() { 1659 if (this.deliverTo == null) 1660 if (Configuration.errorOnAutoCreate()) 1661 throw new Error("Attempt to auto-create SupplyRequest.deliverTo"); 1662 else if (Configuration.doAutoCreate()) 1663 this.deliverTo = new Reference(); // cc 1664 return this.deliverTo; 1665 } 1666 1667 public boolean hasDeliverTo() { 1668 return this.deliverTo != null && !this.deliverTo.isEmpty(); 1669 } 1670 1671 /** 1672 * @param value {@link #deliverTo} (Where the supply is destined to go.) 1673 */ 1674 public SupplyRequest setDeliverTo(Reference value) { 1675 this.deliverTo = value; 1676 return this; 1677 } 1678 1679 /** 1680 * @return {@link #deliverTo} The actual object that is the target of the 1681 * reference. The reference library doesn't populate this, but you can 1682 * use it to hold the resource if you resolve it. (Where the supply is 1683 * destined to go.) 1684 */ 1685 public Resource getDeliverToTarget() { 1686 return this.deliverToTarget; 1687 } 1688 1689 /** 1690 * @param value {@link #deliverTo} The actual object that is the target of the 1691 * reference. The reference library doesn't use these, but you can 1692 * use it to hold the resource if you resolve it. (Where the supply 1693 * is destined to go.) 1694 */ 1695 public SupplyRequest setDeliverToTarget(Resource value) { 1696 this.deliverToTarget = value; 1697 return this; 1698 } 1699 1700 protected void listChildren(List<Property> children) { 1701 super.listChildren(children); 1702 children.add(new Property("identifier", "Identifier", 1703 "Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 1704 0, java.lang.Integer.MAX_VALUE, identifier)); 1705 children.add(new Property("status", "code", "Status of the supply request.", 0, 1, status)); 1706 children.add(new Property("category", "CodeableConcept", 1707 "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 1708 0, 1, category)); 1709 children.add(new Property("priority", "code", 1710 "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.", 0, 1, 1711 priority)); 1712 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1713 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1714 0, 1, item)); 1715 children.add(new Property("quantity", "Quantity", "The amount that is being ordered of the indicated item.", 0, 1, 1716 quantity)); 1717 children.add(new Property("parameter", "", 1718 "Specific parameters for the ordered item. For example, the size of the indicated item.", 0, 1719 java.lang.Integer.MAX_VALUE, parameter)); 1720 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "When the request should be fulfilled.", 0, 1, 1721 occurrence)); 1722 children.add(new Property("authoredOn", "dateTime", "When the request was made.", 0, 1, authoredOn)); 1723 children.add( 1724 new Property("requester", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 1725 "The device, practitioner, etc. who initiated the request.", 0, 1, requester)); 1726 children.add(new Property("supplier", "Reference(Organization|HealthcareService)", 1727 "Who is intended to fulfill the request.", 0, java.lang.Integer.MAX_VALUE, supplier)); 1728 children.add(new Property("reasonCode", "CodeableConcept", "The reason why the supply item was requested.", 0, 1729 java.lang.Integer.MAX_VALUE, reasonCode)); 1730 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 1731 "The reason why the supply item was requested.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1732 children.add(new Property("deliverFrom", "Reference(Organization|Location)", 1733 "Where the supply is expected to come from.", 0, 1, deliverFrom)); 1734 children.add(new Property("deliverTo", "Reference(Organization|Location|Patient)", 1735 "Where the supply is destined to go.", 0, 1, deliverTo)); 1736 } 1737 1738 @Override 1739 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1740 switch (_hash) { 1741 case -1618432855: 1742 /* identifier */ return new Property("identifier", "Identifier", 1743 "Business identifiers assigned to this SupplyRequest by the author and/or other systems. These identifiers remain constant as the resource is updated and propagates from server to server.", 1744 0, java.lang.Integer.MAX_VALUE, identifier); 1745 case -892481550: 1746 /* status */ return new Property("status", "code", "Status of the supply request.", 0, 1, status); 1747 case 50511102: 1748 /* category */ return new Property("category", "CodeableConcept", 1749 "Category of supply, e.g. central, non-stock, etc. This is used to support work flows associated with the supply process.", 1750 0, 1, category); 1751 case -1165461084: 1752 /* priority */ return new Property("priority", "code", 1753 "Indicates how quickly this SupplyRequest should be addressed with respect to other requests.", 0, 1, 1754 priority); 1755 case 2116201613: 1756 /* item[x] */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1757 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1758 0, 1, item); 1759 case 3242771: 1760 /* item */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1761 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1762 0, 1, item); 1763 case 106644494: 1764 /* itemCodeableConcept */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1765 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1766 0, 1, item); 1767 case 1376364920: 1768 /* itemReference */ return new Property("item[x]", "CodeableConcept|Reference(Medication|Substance|Device)", 1769 "The item that is requested to be supplied. This is either a link to a resource representing the details of the item or a code that identifies the item from a known list.", 1770 0, 1, item); 1771 case -1285004149: 1772 /* quantity */ return new Property("quantity", "Quantity", 1773 "The amount that is being ordered of the indicated item.", 0, 1, quantity); 1774 case 1954460585: 1775 /* parameter */ return new Property("parameter", "", 1776 "Specific parameters for the ordered item. For example, the size of the indicated item.", 0, 1777 java.lang.Integer.MAX_VALUE, parameter); 1778 case -2022646513: 1779 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1780 "When the request should be fulfilled.", 0, 1, occurrence); 1781 case 1687874001: 1782 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1783 "When the request should be fulfilled.", 0, 1, occurrence); 1784 case -298443636: 1785 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1786 "When the request should be fulfilled.", 0, 1, occurrence); 1787 case 1397156594: 1788 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1789 "When the request should be fulfilled.", 0, 1, occurrence); 1790 case 1515218299: 1791 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", 1792 "When the request should be fulfilled.", 0, 1, occurrence); 1793 case -1500852503: 1794 /* authoredOn */ return new Property("authoredOn", "dateTime", "When the request was made.", 0, 1, authoredOn); 1795 case 693933948: 1796 /* requester */ return new Property("requester", 1797 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 1798 "The device, practitioner, etc. who initiated the request.", 0, 1, requester); 1799 case -1663305268: 1800 /* supplier */ return new Property("supplier", "Reference(Organization|HealthcareService)", 1801 "Who is intended to fulfill the request.", 0, java.lang.Integer.MAX_VALUE, supplier); 1802 case 722137681: 1803 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 1804 "The reason why the supply item was requested.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1805 case -1146218137: 1806 /* reasonReference */ return new Property("reasonReference", 1807 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 1808 "The reason why the supply item was requested.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1809 case -949323153: 1810 /* deliverFrom */ return new Property("deliverFrom", "Reference(Organization|Location)", 1811 "Where the supply is expected to come from.", 0, 1, deliverFrom); 1812 case -242327936: 1813 /* deliverTo */ return new Property("deliverTo", "Reference(Organization|Location|Patient)", 1814 "Where the supply is destined to go.", 0, 1, deliverTo); 1815 default: 1816 return super.getNamedProperty(_hash, _name, _checkValid); 1817 } 1818 1819 } 1820 1821 @Override 1822 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1823 switch (hash) { 1824 case -1618432855: 1825 /* identifier */ return this.identifier == null ? new Base[0] 1826 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1827 case -892481550: 1828 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<SupplyRequestStatus> 1829 case 50511102: 1830 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1831 case -1165461084: 1832 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 1833 case 3242771: 1834 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 1835 case -1285004149: 1836 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 1837 case 1954460585: 1838 /* parameter */ return this.parameter == null ? new Base[0] 1839 : this.parameter.toArray(new Base[this.parameter.size()]); // SupplyRequestParameterComponent 1840 case 1687874001: 1841 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 1842 case -1500852503: 1843 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 1844 case 693933948: 1845 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 1846 case -1663305268: 1847 /* supplier */ return this.supplier == null ? new Base[0] : this.supplier.toArray(new Base[this.supplier.size()]); // Reference 1848 case 722137681: 1849 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1850 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1851 case -1146218137: 1852 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1853 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1854 case -949323153: 1855 /* deliverFrom */ return this.deliverFrom == null ? new Base[0] : new Base[] { this.deliverFrom }; // Reference 1856 case -242327936: 1857 /* deliverTo */ return this.deliverTo == null ? new Base[0] : new Base[] { this.deliverTo }; // Reference 1858 default: 1859 return super.getProperty(hash, name, checkValid); 1860 } 1861 1862 } 1863 1864 @Override 1865 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1866 switch (hash) { 1867 case -1618432855: // identifier 1868 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1869 return value; 1870 case -892481550: // status 1871 value = new SupplyRequestStatusEnumFactory().fromType(castToCode(value)); 1872 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1873 return value; 1874 case 50511102: // category 1875 this.category = castToCodeableConcept(value); // CodeableConcept 1876 return value; 1877 case -1165461084: // priority 1878 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 1879 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1880 return value; 1881 case 3242771: // item 1882 this.item = castToType(value); // Type 1883 return value; 1884 case -1285004149: // quantity 1885 this.quantity = castToQuantity(value); // Quantity 1886 return value; 1887 case 1954460585: // parameter 1888 this.getParameter().add((SupplyRequestParameterComponent) value); // SupplyRequestParameterComponent 1889 return value; 1890 case 1687874001: // occurrence 1891 this.occurrence = castToType(value); // Type 1892 return value; 1893 case -1500852503: // authoredOn 1894 this.authoredOn = castToDateTime(value); // DateTimeType 1895 return value; 1896 case 693933948: // requester 1897 this.requester = castToReference(value); // Reference 1898 return value; 1899 case -1663305268: // supplier 1900 this.getSupplier().add(castToReference(value)); // Reference 1901 return value; 1902 case 722137681: // reasonCode 1903 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1904 return value; 1905 case -1146218137: // reasonReference 1906 this.getReasonReference().add(castToReference(value)); // Reference 1907 return value; 1908 case -949323153: // deliverFrom 1909 this.deliverFrom = castToReference(value); // Reference 1910 return value; 1911 case -242327936: // deliverTo 1912 this.deliverTo = castToReference(value); // Reference 1913 return value; 1914 default: 1915 return super.setProperty(hash, name, value); 1916 } 1917 1918 } 1919 1920 @Override 1921 public Base setProperty(String name, Base value) throws FHIRException { 1922 if (name.equals("identifier")) { 1923 this.getIdentifier().add(castToIdentifier(value)); 1924 } else if (name.equals("status")) { 1925 value = new SupplyRequestStatusEnumFactory().fromType(castToCode(value)); 1926 this.status = (Enumeration) value; // Enumeration<SupplyRequestStatus> 1927 } else if (name.equals("category")) { 1928 this.category = castToCodeableConcept(value); // CodeableConcept 1929 } else if (name.equals("priority")) { 1930 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 1931 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 1932 } else if (name.equals("item[x]")) { 1933 this.item = castToType(value); // Type 1934 } else if (name.equals("quantity")) { 1935 this.quantity = castToQuantity(value); // Quantity 1936 } else if (name.equals("parameter")) { 1937 this.getParameter().add((SupplyRequestParameterComponent) value); 1938 } else if (name.equals("occurrence[x]")) { 1939 this.occurrence = castToType(value); // Type 1940 } else if (name.equals("authoredOn")) { 1941 this.authoredOn = castToDateTime(value); // DateTimeType 1942 } else if (name.equals("requester")) { 1943 this.requester = castToReference(value); // Reference 1944 } else if (name.equals("supplier")) { 1945 this.getSupplier().add(castToReference(value)); 1946 } else if (name.equals("reasonCode")) { 1947 this.getReasonCode().add(castToCodeableConcept(value)); 1948 } else if (name.equals("reasonReference")) { 1949 this.getReasonReference().add(castToReference(value)); 1950 } else if (name.equals("deliverFrom")) { 1951 this.deliverFrom = castToReference(value); // Reference 1952 } else if (name.equals("deliverTo")) { 1953 this.deliverTo = castToReference(value); // Reference 1954 } else 1955 return super.setProperty(name, value); 1956 return value; 1957 } 1958 1959 @Override 1960 public void removeChild(String name, Base value) throws FHIRException { 1961 if (name.equals("identifier")) { 1962 this.getIdentifier().remove(castToIdentifier(value)); 1963 } else if (name.equals("status")) { 1964 this.status = null; 1965 } else if (name.equals("category")) { 1966 this.category = null; 1967 } else if (name.equals("priority")) { 1968 this.priority = null; 1969 } else if (name.equals("item[x]")) { 1970 this.item = null; 1971 } else if (name.equals("quantity")) { 1972 this.quantity = null; 1973 } else if (name.equals("parameter")) { 1974 this.getParameter().remove((SupplyRequestParameterComponent) value); 1975 } else if (name.equals("occurrence[x]")) { 1976 this.occurrence = null; 1977 } else if (name.equals("authoredOn")) { 1978 this.authoredOn = null; 1979 } else if (name.equals("requester")) { 1980 this.requester = null; 1981 } else if (name.equals("supplier")) { 1982 this.getSupplier().remove(castToReference(value)); 1983 } else if (name.equals("reasonCode")) { 1984 this.getReasonCode().remove(castToCodeableConcept(value)); 1985 } else if (name.equals("reasonReference")) { 1986 this.getReasonReference().remove(castToReference(value)); 1987 } else if (name.equals("deliverFrom")) { 1988 this.deliverFrom = null; 1989 } else if (name.equals("deliverTo")) { 1990 this.deliverTo = null; 1991 } else 1992 super.removeChild(name, value); 1993 1994 } 1995 1996 @Override 1997 public Base makeProperty(int hash, String name) throws FHIRException { 1998 switch (hash) { 1999 case -1618432855: 2000 return addIdentifier(); 2001 case -892481550: 2002 return getStatusElement(); 2003 case 50511102: 2004 return getCategory(); 2005 case -1165461084: 2006 return getPriorityElement(); 2007 case 2116201613: 2008 return getItem(); 2009 case 3242771: 2010 return getItem(); 2011 case -1285004149: 2012 return getQuantity(); 2013 case 1954460585: 2014 return addParameter(); 2015 case -2022646513: 2016 return getOccurrence(); 2017 case 1687874001: 2018 return getOccurrence(); 2019 case -1500852503: 2020 return getAuthoredOnElement(); 2021 case 693933948: 2022 return getRequester(); 2023 case -1663305268: 2024 return addSupplier(); 2025 case 722137681: 2026 return addReasonCode(); 2027 case -1146218137: 2028 return addReasonReference(); 2029 case -949323153: 2030 return getDeliverFrom(); 2031 case -242327936: 2032 return getDeliverTo(); 2033 default: 2034 return super.makeProperty(hash, name); 2035 } 2036 2037 } 2038 2039 @Override 2040 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2041 switch (hash) { 2042 case -1618432855: 2043 /* identifier */ return new String[] { "Identifier" }; 2044 case -892481550: 2045 /* status */ return new String[] { "code" }; 2046 case 50511102: 2047 /* category */ return new String[] { "CodeableConcept" }; 2048 case -1165461084: 2049 /* priority */ return new String[] { "code" }; 2050 case 3242771: 2051 /* item */ return new String[] { "CodeableConcept", "Reference" }; 2052 case -1285004149: 2053 /* quantity */ return new String[] { "Quantity" }; 2054 case 1954460585: 2055 /* parameter */ return new String[] {}; 2056 case 1687874001: 2057 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 2058 case -1500852503: 2059 /* authoredOn */ return new String[] { "dateTime" }; 2060 case 693933948: 2061 /* requester */ return new String[] { "Reference" }; 2062 case -1663305268: 2063 /* supplier */ return new String[] { "Reference" }; 2064 case 722137681: 2065 /* reasonCode */ return new String[] { "CodeableConcept" }; 2066 case -1146218137: 2067 /* reasonReference */ return new String[] { "Reference" }; 2068 case -949323153: 2069 /* deliverFrom */ return new String[] { "Reference" }; 2070 case -242327936: 2071 /* deliverTo */ return new String[] { "Reference" }; 2072 default: 2073 return super.getTypesForProperty(hash, name); 2074 } 2075 2076 } 2077 2078 @Override 2079 public Base addChild(String name) throws FHIRException { 2080 if (name.equals("identifier")) { 2081 return addIdentifier(); 2082 } else if (name.equals("status")) { 2083 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.status"); 2084 } else if (name.equals("category")) { 2085 this.category = new CodeableConcept(); 2086 return this.category; 2087 } else if (name.equals("priority")) { 2088 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.priority"); 2089 } else if (name.equals("itemCodeableConcept")) { 2090 this.item = new CodeableConcept(); 2091 return this.item; 2092 } else if (name.equals("itemReference")) { 2093 this.item = new Reference(); 2094 return this.item; 2095 } else if (name.equals("quantity")) { 2096 this.quantity = new Quantity(); 2097 return this.quantity; 2098 } else if (name.equals("parameter")) { 2099 return addParameter(); 2100 } else if (name.equals("occurrenceDateTime")) { 2101 this.occurrence = new DateTimeType(); 2102 return this.occurrence; 2103 } else if (name.equals("occurrencePeriod")) { 2104 this.occurrence = new Period(); 2105 return this.occurrence; 2106 } else if (name.equals("occurrenceTiming")) { 2107 this.occurrence = new Timing(); 2108 return this.occurrence; 2109 } else if (name.equals("authoredOn")) { 2110 throw new FHIRException("Cannot call addChild on a singleton property SupplyRequest.authoredOn"); 2111 } else if (name.equals("requester")) { 2112 this.requester = new Reference(); 2113 return this.requester; 2114 } else if (name.equals("supplier")) { 2115 return addSupplier(); 2116 } else if (name.equals("reasonCode")) { 2117 return addReasonCode(); 2118 } else if (name.equals("reasonReference")) { 2119 return addReasonReference(); 2120 } else if (name.equals("deliverFrom")) { 2121 this.deliverFrom = new Reference(); 2122 return this.deliverFrom; 2123 } else if (name.equals("deliverTo")) { 2124 this.deliverTo = new Reference(); 2125 return this.deliverTo; 2126 } else 2127 return super.addChild(name); 2128 } 2129 2130 public String fhirType() { 2131 return "SupplyRequest"; 2132 2133 } 2134 2135 public SupplyRequest copy() { 2136 SupplyRequest dst = new SupplyRequest(); 2137 copyValues(dst); 2138 return dst; 2139 } 2140 2141 public void copyValues(SupplyRequest dst) { 2142 super.copyValues(dst); 2143 if (identifier != null) { 2144 dst.identifier = new ArrayList<Identifier>(); 2145 for (Identifier i : identifier) 2146 dst.identifier.add(i.copy()); 2147 } 2148 ; 2149 dst.status = status == null ? null : status.copy(); 2150 dst.category = category == null ? null : category.copy(); 2151 dst.priority = priority == null ? null : priority.copy(); 2152 dst.item = item == null ? null : item.copy(); 2153 dst.quantity = quantity == null ? null : quantity.copy(); 2154 if (parameter != null) { 2155 dst.parameter = new ArrayList<SupplyRequestParameterComponent>(); 2156 for (SupplyRequestParameterComponent i : parameter) 2157 dst.parameter.add(i.copy()); 2158 } 2159 ; 2160 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2161 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2162 dst.requester = requester == null ? null : requester.copy(); 2163 if (supplier != null) { 2164 dst.supplier = new ArrayList<Reference>(); 2165 for (Reference i : supplier) 2166 dst.supplier.add(i.copy()); 2167 } 2168 ; 2169 if (reasonCode != null) { 2170 dst.reasonCode = new ArrayList<CodeableConcept>(); 2171 for (CodeableConcept i : reasonCode) 2172 dst.reasonCode.add(i.copy()); 2173 } 2174 ; 2175 if (reasonReference != null) { 2176 dst.reasonReference = new ArrayList<Reference>(); 2177 for (Reference i : reasonReference) 2178 dst.reasonReference.add(i.copy()); 2179 } 2180 ; 2181 dst.deliverFrom = deliverFrom == null ? null : deliverFrom.copy(); 2182 dst.deliverTo = deliverTo == null ? null : deliverTo.copy(); 2183 } 2184 2185 protected SupplyRequest typedCopy() { 2186 return copy(); 2187 } 2188 2189 @Override 2190 public boolean equalsDeep(Base other_) { 2191 if (!super.equalsDeep(other_)) 2192 return false; 2193 if (!(other_ instanceof SupplyRequest)) 2194 return false; 2195 SupplyRequest o = (SupplyRequest) other_; 2196 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2197 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 2198 && compareDeep(item, o.item, true) && compareDeep(quantity, o.quantity, true) 2199 && compareDeep(parameter, o.parameter, true) && compareDeep(occurrence, o.occurrence, true) 2200 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 2201 && compareDeep(supplier, o.supplier, true) && compareDeep(reasonCode, o.reasonCode, true) 2202 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(deliverFrom, o.deliverFrom, true) 2203 && compareDeep(deliverTo, o.deliverTo, true); 2204 } 2205 2206 @Override 2207 public boolean equalsShallow(Base other_) { 2208 if (!super.equalsShallow(other_)) 2209 return false; 2210 if (!(other_ instanceof SupplyRequest)) 2211 return false; 2212 SupplyRequest o = (SupplyRequest) other_; 2213 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) 2214 && compareValues(authoredOn, o.authoredOn, true); 2215 } 2216 2217 public boolean isEmpty() { 2218 return super.isEmpty() 2219 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category, priority, item, quantity, parameter, 2220 occurrence, authoredOn, requester, supplier, reasonCode, reasonReference, deliverFrom, deliverTo); 2221 } 2222 2223 @Override 2224 public ResourceType getResourceType() { 2225 return ResourceType.SupplyRequest; 2226 } 2227 2228 /** 2229 * Search parameter: <b>requester</b> 2230 * <p> 2231 * Description: <b>Individual making the request</b><br> 2232 * Type: <b>reference</b><br> 2233 * Path: <b>SupplyRequest.requester</b><br> 2234 * </p> 2235 */ 2236 @SearchParamDefinition(name = "requester", path = "SupplyRequest.requester", description = "Individual making the request", type = "reference", providesMembershipIn = { 2237 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2238 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2239 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 2240 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2241 public static final String SP_REQUESTER = "requester"; 2242 /** 2243 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2244 * <p> 2245 * Description: <b>Individual making the request</b><br> 2246 * Type: <b>reference</b><br> 2247 * Path: <b>SupplyRequest.requester</b><br> 2248 * </p> 2249 */ 2250 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2251 SP_REQUESTER); 2252 2253 /** 2254 * Constant for fluent queries to be used to add include statements. Specifies 2255 * the path value of "<b>SupplyRequest:requester</b>". 2256 */ 2257 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 2258 "SupplyRequest:requester").toLocked(); 2259 2260 /** 2261 * Search parameter: <b>date</b> 2262 * <p> 2263 * Description: <b>When the request was made</b><br> 2264 * Type: <b>date</b><br> 2265 * Path: <b>SupplyRequest.authoredOn</b><br> 2266 * </p> 2267 */ 2268 @SearchParamDefinition(name = "date", path = "SupplyRequest.authoredOn", description = "When the request was made", type = "date") 2269 public static final String SP_DATE = "date"; 2270 /** 2271 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2272 * <p> 2273 * Description: <b>When the request was made</b><br> 2274 * Type: <b>date</b><br> 2275 * Path: <b>SupplyRequest.authoredOn</b><br> 2276 * </p> 2277 */ 2278 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2279 SP_DATE); 2280 2281 /** 2282 * Search parameter: <b>identifier</b> 2283 * <p> 2284 * Description: <b>Business Identifier for SupplyRequest</b><br> 2285 * Type: <b>token</b><br> 2286 * Path: <b>SupplyRequest.identifier</b><br> 2287 * </p> 2288 */ 2289 @SearchParamDefinition(name = "identifier", path = "SupplyRequest.identifier", description = "Business Identifier for SupplyRequest", type = "token") 2290 public static final String SP_IDENTIFIER = "identifier"; 2291 /** 2292 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2293 * <p> 2294 * Description: <b>Business Identifier for SupplyRequest</b><br> 2295 * Type: <b>token</b><br> 2296 * Path: <b>SupplyRequest.identifier</b><br> 2297 * </p> 2298 */ 2299 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2300 SP_IDENTIFIER); 2301 2302 /** 2303 * Search parameter: <b>subject</b> 2304 * <p> 2305 * Description: <b>The destination of the supply</b><br> 2306 * Type: <b>reference</b><br> 2307 * Path: <b>SupplyRequest.deliverTo</b><br> 2308 * </p> 2309 */ 2310 @SearchParamDefinition(name = "subject", path = "SupplyRequest.deliverTo", description = "The destination of the supply", type = "reference", providesMembershipIn = { 2311 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Location.class, Organization.class, 2312 Patient.class }) 2313 public static final String SP_SUBJECT = "subject"; 2314 /** 2315 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2316 * <p> 2317 * Description: <b>The destination of the supply</b><br> 2318 * Type: <b>reference</b><br> 2319 * Path: <b>SupplyRequest.deliverTo</b><br> 2320 * </p> 2321 */ 2322 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2323 SP_SUBJECT); 2324 2325 /** 2326 * Constant for fluent queries to be used to add include statements. Specifies 2327 * the path value of "<b>SupplyRequest:subject</b>". 2328 */ 2329 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2330 "SupplyRequest:subject").toLocked(); 2331 2332 /** 2333 * Search parameter: <b>supplier</b> 2334 * <p> 2335 * Description: <b>Who is intended to fulfill the request</b><br> 2336 * Type: <b>reference</b><br> 2337 * Path: <b>SupplyRequest.supplier</b><br> 2338 * </p> 2339 */ 2340 @SearchParamDefinition(name = "supplier", path = "SupplyRequest.supplier", description = "Who is intended to fulfill the request", type = "reference", target = { 2341 HealthcareService.class, Organization.class }) 2342 public static final String SP_SUPPLIER = "supplier"; 2343 /** 2344 * <b>Fluent Client</b> search parameter constant for <b>supplier</b> 2345 * <p> 2346 * Description: <b>Who is intended to fulfill the request</b><br> 2347 * Type: <b>reference</b><br> 2348 * Path: <b>SupplyRequest.supplier</b><br> 2349 * </p> 2350 */ 2351 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPLIER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2352 SP_SUPPLIER); 2353 2354 /** 2355 * Constant for fluent queries to be used to add include statements. Specifies 2356 * the path value of "<b>SupplyRequest:supplier</b>". 2357 */ 2358 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPLIER = new ca.uhn.fhir.model.api.Include( 2359 "SupplyRequest:supplier").toLocked(); 2360 2361 /** 2362 * Search parameter: <b>category</b> 2363 * <p> 2364 * Description: <b>The kind of supply (central, non-stock, etc.)</b><br> 2365 * Type: <b>token</b><br> 2366 * Path: <b>SupplyRequest.category</b><br> 2367 * </p> 2368 */ 2369 @SearchParamDefinition(name = "category", path = "SupplyRequest.category", description = "The kind of supply (central, non-stock, etc.)", type = "token") 2370 public static final String SP_CATEGORY = "category"; 2371 /** 2372 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2373 * <p> 2374 * Description: <b>The kind of supply (central, non-stock, etc.)</b><br> 2375 * Type: <b>token</b><br> 2376 * Path: <b>SupplyRequest.category</b><br> 2377 * </p> 2378 */ 2379 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2380 SP_CATEGORY); 2381 2382 /** 2383 * Search parameter: <b>status</b> 2384 * <p> 2385 * Description: <b>draft | active | suspended +</b><br> 2386 * Type: <b>token</b><br> 2387 * Path: <b>SupplyRequest.status</b><br> 2388 * </p> 2389 */ 2390 @SearchParamDefinition(name = "status", path = "SupplyRequest.status", description = "draft | active | suspended +", type = "token") 2391 public static final String SP_STATUS = "status"; 2392 /** 2393 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2394 * <p> 2395 * Description: <b>draft | active | suspended +</b><br> 2396 * Type: <b>token</b><br> 2397 * Path: <b>SupplyRequest.status</b><br> 2398 * </p> 2399 */ 2400 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2401 SP_STATUS); 2402 2403}