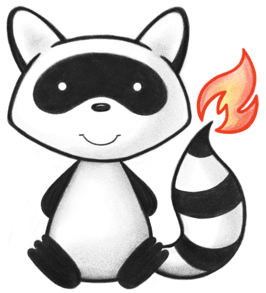
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A task to be performed. 049 */ 050@ResourceDef(name = "Task", profile = "http://hl7.org/fhir/StructureDefinition/Task") 051public class Task extends DomainResource { 052 053 public enum TaskStatus { 054 /** 055 * The task is not yet ready to be acted upon. 056 */ 057 DRAFT, 058 /** 059 * The task is ready to be acted upon and action is sought. 060 */ 061 REQUESTED, 062 /** 063 * A potential performer has claimed ownership of the task and is evaluating 064 * whether to perform it. 065 */ 066 RECEIVED, 067 /** 068 * The potential performer has agreed to execute the task but has not yet 069 * started work. 070 */ 071 ACCEPTED, 072 /** 073 * The potential performer who claimed ownership of the task has decided not to 074 * execute it prior to performing any action. 075 */ 076 REJECTED, 077 /** 078 * The task is ready to be performed, but no action has yet been taken. Used in 079 * place of requested/received/accepted/rejected when request assignment and 080 * acceptance is a given. 081 */ 082 READY, 083 /** 084 * The task was not completed. 085 */ 086 CANCELLED, 087 /** 088 * The task has been started but is not yet complete. 089 */ 090 INPROGRESS, 091 /** 092 * The task has been started but work has been paused. 093 */ 094 ONHOLD, 095 /** 096 * The task was attempted but could not be completed due to some error. 097 */ 098 FAILED, 099 /** 100 * The task has been completed. 101 */ 102 COMPLETED, 103 /** 104 * The task should never have existed and is retained only because of the 105 * possibility it may have used. 106 */ 107 ENTEREDINERROR, 108 /** 109 * added to help the parsers with the generic types 110 */ 111 NULL; 112 113 public static TaskStatus fromCode(String codeString) throws FHIRException { 114 if (codeString == null || "".equals(codeString)) 115 return null; 116 if ("draft".equals(codeString)) 117 return DRAFT; 118 if ("requested".equals(codeString)) 119 return REQUESTED; 120 if ("received".equals(codeString)) 121 return RECEIVED; 122 if ("accepted".equals(codeString)) 123 return ACCEPTED; 124 if ("rejected".equals(codeString)) 125 return REJECTED; 126 if ("ready".equals(codeString)) 127 return READY; 128 if ("cancelled".equals(codeString)) 129 return CANCELLED; 130 if ("in-progress".equals(codeString)) 131 return INPROGRESS; 132 if ("on-hold".equals(codeString)) 133 return ONHOLD; 134 if ("failed".equals(codeString)) 135 return FAILED; 136 if ("completed".equals(codeString)) 137 return COMPLETED; 138 if ("entered-in-error".equals(codeString)) 139 return ENTEREDINERROR; 140 if (Configuration.isAcceptInvalidEnums()) 141 return null; 142 else 143 throw new FHIRException("Unknown TaskStatus code '" + codeString + "'"); 144 } 145 146 public String toCode() { 147 switch (this) { 148 case DRAFT: 149 return "draft"; 150 case REQUESTED: 151 return "requested"; 152 case RECEIVED: 153 return "received"; 154 case ACCEPTED: 155 return "accepted"; 156 case REJECTED: 157 return "rejected"; 158 case READY: 159 return "ready"; 160 case CANCELLED: 161 return "cancelled"; 162 case INPROGRESS: 163 return "in-progress"; 164 case ONHOLD: 165 return "on-hold"; 166 case FAILED: 167 return "failed"; 168 case COMPLETED: 169 return "completed"; 170 case ENTEREDINERROR: 171 return "entered-in-error"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getSystem() { 180 switch (this) { 181 case DRAFT: 182 return "http://hl7.org/fhir/task-status"; 183 case REQUESTED: 184 return "http://hl7.org/fhir/task-status"; 185 case RECEIVED: 186 return "http://hl7.org/fhir/task-status"; 187 case ACCEPTED: 188 return "http://hl7.org/fhir/task-status"; 189 case REJECTED: 190 return "http://hl7.org/fhir/task-status"; 191 case READY: 192 return "http://hl7.org/fhir/task-status"; 193 case CANCELLED: 194 return "http://hl7.org/fhir/task-status"; 195 case INPROGRESS: 196 return "http://hl7.org/fhir/task-status"; 197 case ONHOLD: 198 return "http://hl7.org/fhir/task-status"; 199 case FAILED: 200 return "http://hl7.org/fhir/task-status"; 201 case COMPLETED: 202 return "http://hl7.org/fhir/task-status"; 203 case ENTEREDINERROR: 204 return "http://hl7.org/fhir/task-status"; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212 public String getDefinition() { 213 switch (this) { 214 case DRAFT: 215 return "The task is not yet ready to be acted upon."; 216 case REQUESTED: 217 return "The task is ready to be acted upon and action is sought."; 218 case RECEIVED: 219 return "A potential performer has claimed ownership of the task and is evaluating whether to perform it."; 220 case ACCEPTED: 221 return "The potential performer has agreed to execute the task but has not yet started work."; 222 case REJECTED: 223 return "The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action."; 224 case READY: 225 return "The task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given."; 226 case CANCELLED: 227 return "The task was not completed."; 228 case INPROGRESS: 229 return "The task has been started but is not yet complete."; 230 case ONHOLD: 231 return "The task has been started but work has been paused."; 232 case FAILED: 233 return "The task was attempted but could not be completed due to some error."; 234 case COMPLETED: 235 return "The task has been completed."; 236 case ENTEREDINERROR: 237 return "The task should never have existed and is retained only because of the possibility it may have used."; 238 case NULL: 239 return null; 240 default: 241 return "?"; 242 } 243 } 244 245 public String getDisplay() { 246 switch (this) { 247 case DRAFT: 248 return "Draft"; 249 case REQUESTED: 250 return "Requested"; 251 case RECEIVED: 252 return "Received"; 253 case ACCEPTED: 254 return "Accepted"; 255 case REJECTED: 256 return "Rejected"; 257 case READY: 258 return "Ready"; 259 case CANCELLED: 260 return "Cancelled"; 261 case INPROGRESS: 262 return "In Progress"; 263 case ONHOLD: 264 return "On Hold"; 265 case FAILED: 266 return "Failed"; 267 case COMPLETED: 268 return "Completed"; 269 case ENTEREDINERROR: 270 return "Entered in Error"; 271 case NULL: 272 return null; 273 default: 274 return "?"; 275 } 276 } 277 } 278 279 public static class TaskStatusEnumFactory implements EnumFactory<TaskStatus> { 280 public TaskStatus fromCode(String codeString) throws IllegalArgumentException { 281 if (codeString == null || "".equals(codeString)) 282 if (codeString == null || "".equals(codeString)) 283 return null; 284 if ("draft".equals(codeString)) 285 return TaskStatus.DRAFT; 286 if ("requested".equals(codeString)) 287 return TaskStatus.REQUESTED; 288 if ("received".equals(codeString)) 289 return TaskStatus.RECEIVED; 290 if ("accepted".equals(codeString)) 291 return TaskStatus.ACCEPTED; 292 if ("rejected".equals(codeString)) 293 return TaskStatus.REJECTED; 294 if ("ready".equals(codeString)) 295 return TaskStatus.READY; 296 if ("cancelled".equals(codeString)) 297 return TaskStatus.CANCELLED; 298 if ("in-progress".equals(codeString)) 299 return TaskStatus.INPROGRESS; 300 if ("on-hold".equals(codeString)) 301 return TaskStatus.ONHOLD; 302 if ("failed".equals(codeString)) 303 return TaskStatus.FAILED; 304 if ("completed".equals(codeString)) 305 return TaskStatus.COMPLETED; 306 if ("entered-in-error".equals(codeString)) 307 return TaskStatus.ENTEREDINERROR; 308 throw new IllegalArgumentException("Unknown TaskStatus code '" + codeString + "'"); 309 } 310 311 public Enumeration<TaskStatus> fromType(PrimitiveType<?> code) throws FHIRException { 312 if (code == null) 313 return null; 314 if (code.isEmpty()) 315 return new Enumeration<TaskStatus>(this, TaskStatus.NULL, code); 316 String codeString = code.asStringValue(); 317 if (codeString == null || "".equals(codeString)) 318 return new Enumeration<TaskStatus>(this, TaskStatus.NULL, code); 319 if ("draft".equals(codeString)) 320 return new Enumeration<TaskStatus>(this, TaskStatus.DRAFT, code); 321 if ("requested".equals(codeString)) 322 return new Enumeration<TaskStatus>(this, TaskStatus.REQUESTED, code); 323 if ("received".equals(codeString)) 324 return new Enumeration<TaskStatus>(this, TaskStatus.RECEIVED, code); 325 if ("accepted".equals(codeString)) 326 return new Enumeration<TaskStatus>(this, TaskStatus.ACCEPTED, code); 327 if ("rejected".equals(codeString)) 328 return new Enumeration<TaskStatus>(this, TaskStatus.REJECTED, code); 329 if ("ready".equals(codeString)) 330 return new Enumeration<TaskStatus>(this, TaskStatus.READY, code); 331 if ("cancelled".equals(codeString)) 332 return new Enumeration<TaskStatus>(this, TaskStatus.CANCELLED, code); 333 if ("in-progress".equals(codeString)) 334 return new Enumeration<TaskStatus>(this, TaskStatus.INPROGRESS, code); 335 if ("on-hold".equals(codeString)) 336 return new Enumeration<TaskStatus>(this, TaskStatus.ONHOLD, code); 337 if ("failed".equals(codeString)) 338 return new Enumeration<TaskStatus>(this, TaskStatus.FAILED, code); 339 if ("completed".equals(codeString)) 340 return new Enumeration<TaskStatus>(this, TaskStatus.COMPLETED, code); 341 if ("entered-in-error".equals(codeString)) 342 return new Enumeration<TaskStatus>(this, TaskStatus.ENTEREDINERROR, code); 343 throw new FHIRException("Unknown TaskStatus code '" + codeString + "'"); 344 } 345 346 public String toCode(TaskStatus code) { 347 if (code == TaskStatus.NULL) 348 return null; 349 if (code == TaskStatus.DRAFT) 350 return "draft"; 351 if (code == TaskStatus.REQUESTED) 352 return "requested"; 353 if (code == TaskStatus.RECEIVED) 354 return "received"; 355 if (code == TaskStatus.ACCEPTED) 356 return "accepted"; 357 if (code == TaskStatus.REJECTED) 358 return "rejected"; 359 if (code == TaskStatus.READY) 360 return "ready"; 361 if (code == TaskStatus.CANCELLED) 362 return "cancelled"; 363 if (code == TaskStatus.INPROGRESS) 364 return "in-progress"; 365 if (code == TaskStatus.ONHOLD) 366 return "on-hold"; 367 if (code == TaskStatus.FAILED) 368 return "failed"; 369 if (code == TaskStatus.COMPLETED) 370 return "completed"; 371 if (code == TaskStatus.ENTEREDINERROR) 372 return "entered-in-error"; 373 return "?"; 374 } 375 376 public String toSystem(TaskStatus code) { 377 return code.getSystem(); 378 } 379 } 380 381 public enum TaskIntent { 382 /** 383 * The intent is not known. When dealing with Task, it's not always known (or 384 * relevant) how the task was initiated - i.e. whether it was proposed, planned, 385 * ordered or just done spontaneously. 386 */ 387 UNKNOWN, 388 /** 389 * null 390 */ 391 PROPOSAL, 392 /** 393 * null 394 */ 395 PLAN, 396 /** 397 * null 398 */ 399 ORDER, 400 /** 401 * null 402 */ 403 ORIGINALORDER, 404 /** 405 * null 406 */ 407 REFLEXORDER, 408 /** 409 * null 410 */ 411 FILLERORDER, 412 /** 413 * null 414 */ 415 INSTANCEORDER, 416 /** 417 * null 418 */ 419 OPTION, 420 /** 421 * added to help the parsers with the generic types 422 */ 423 NULL; 424 425 public static TaskIntent fromCode(String codeString) throws FHIRException { 426 if (codeString == null || "".equals(codeString)) 427 return null; 428 if ("unknown".equals(codeString)) 429 return UNKNOWN; 430 if ("proposal".equals(codeString)) 431 return PROPOSAL; 432 if ("plan".equals(codeString)) 433 return PLAN; 434 if ("order".equals(codeString)) 435 return ORDER; 436 if ("original-order".equals(codeString)) 437 return ORIGINALORDER; 438 if ("reflex-order".equals(codeString)) 439 return REFLEXORDER; 440 if ("filler-order".equals(codeString)) 441 return FILLERORDER; 442 if ("instance-order".equals(codeString)) 443 return INSTANCEORDER; 444 if ("option".equals(codeString)) 445 return OPTION; 446 if (Configuration.isAcceptInvalidEnums()) 447 return null; 448 else 449 throw new FHIRException("Unknown TaskIntent code '" + codeString + "'"); 450 } 451 452 public String toCode() { 453 switch (this) { 454 case UNKNOWN: 455 return "unknown"; 456 case PROPOSAL: 457 return "proposal"; 458 case PLAN: 459 return "plan"; 460 case ORDER: 461 return "order"; 462 case ORIGINALORDER: 463 return "original-order"; 464 case REFLEXORDER: 465 return "reflex-order"; 466 case FILLERORDER: 467 return "filler-order"; 468 case INSTANCEORDER: 469 return "instance-order"; 470 case OPTION: 471 return "option"; 472 case NULL: 473 return null; 474 default: 475 return "?"; 476 } 477 } 478 479 public String getSystem() { 480 switch (this) { 481 case UNKNOWN: 482 return "http://hl7.org/fhir/task-intent"; 483 case PROPOSAL: 484 return "http://hl7.org/fhir/request-intent"; 485 case PLAN: 486 return "http://hl7.org/fhir/request-intent"; 487 case ORDER: 488 return "http://hl7.org/fhir/request-intent"; 489 case ORIGINALORDER: 490 return "http://hl7.org/fhir/request-intent"; 491 case REFLEXORDER: 492 return "http://hl7.org/fhir/request-intent"; 493 case FILLERORDER: 494 return "http://hl7.org/fhir/request-intent"; 495 case INSTANCEORDER: 496 return "http://hl7.org/fhir/request-intent"; 497 case OPTION: 498 return "http://hl7.org/fhir/request-intent"; 499 case NULL: 500 return null; 501 default: 502 return "?"; 503 } 504 } 505 506 public String getDefinition() { 507 switch (this) { 508 case UNKNOWN: 509 return "The intent is not known. When dealing with Task, it's not always known (or relevant) how the task was initiated - i.e. whether it was proposed, planned, ordered or just done spontaneously."; 510 case PROPOSAL: 511 return ""; 512 case PLAN: 513 return ""; 514 case ORDER: 515 return ""; 516 case ORIGINALORDER: 517 return ""; 518 case REFLEXORDER: 519 return ""; 520 case FILLERORDER: 521 return ""; 522 case INSTANCEORDER: 523 return ""; 524 case OPTION: 525 return ""; 526 case NULL: 527 return null; 528 default: 529 return "?"; 530 } 531 } 532 533 public String getDisplay() { 534 switch (this) { 535 case UNKNOWN: 536 return "Unknown"; 537 case PROPOSAL: 538 return "proposal"; 539 case PLAN: 540 return "plan"; 541 case ORDER: 542 return "order"; 543 case ORIGINALORDER: 544 return "original-order"; 545 case REFLEXORDER: 546 return "reflex-order"; 547 case FILLERORDER: 548 return "filler-order"; 549 case INSTANCEORDER: 550 return "instance-order"; 551 case OPTION: 552 return "option"; 553 case NULL: 554 return null; 555 default: 556 return "?"; 557 } 558 } 559 } 560 561 public static class TaskIntentEnumFactory implements EnumFactory<TaskIntent> { 562 public TaskIntent fromCode(String codeString) throws IllegalArgumentException { 563 if (codeString == null || "".equals(codeString)) 564 if (codeString == null || "".equals(codeString)) 565 return null; 566 if ("unknown".equals(codeString)) 567 return TaskIntent.UNKNOWN; 568 if ("proposal".equals(codeString)) 569 return TaskIntent.PROPOSAL; 570 if ("plan".equals(codeString)) 571 return TaskIntent.PLAN; 572 if ("order".equals(codeString)) 573 return TaskIntent.ORDER; 574 if ("original-order".equals(codeString)) 575 return TaskIntent.ORIGINALORDER; 576 if ("reflex-order".equals(codeString)) 577 return TaskIntent.REFLEXORDER; 578 if ("filler-order".equals(codeString)) 579 return TaskIntent.FILLERORDER; 580 if ("instance-order".equals(codeString)) 581 return TaskIntent.INSTANCEORDER; 582 if ("option".equals(codeString)) 583 return TaskIntent.OPTION; 584 throw new IllegalArgumentException("Unknown TaskIntent code '" + codeString + "'"); 585 } 586 587 public Enumeration<TaskIntent> fromType(PrimitiveType<?> code) throws FHIRException { 588 if (code == null) 589 return null; 590 if (code.isEmpty()) 591 return new Enumeration<TaskIntent>(this, TaskIntent.NULL, code); 592 String codeString = code.asStringValue(); 593 if (codeString == null || "".equals(codeString)) 594 return new Enumeration<TaskIntent>(this, TaskIntent.NULL, code); 595 if ("unknown".equals(codeString)) 596 return new Enumeration<TaskIntent>(this, TaskIntent.UNKNOWN, code); 597 if ("proposal".equals(codeString)) 598 return new Enumeration<TaskIntent>(this, TaskIntent.PROPOSAL, code); 599 if ("plan".equals(codeString)) 600 return new Enumeration<TaskIntent>(this, TaskIntent.PLAN, code); 601 if ("order".equals(codeString)) 602 return new Enumeration<TaskIntent>(this, TaskIntent.ORDER, code); 603 if ("original-order".equals(codeString)) 604 return new Enumeration<TaskIntent>(this, TaskIntent.ORIGINALORDER, code); 605 if ("reflex-order".equals(codeString)) 606 return new Enumeration<TaskIntent>(this, TaskIntent.REFLEXORDER, code); 607 if ("filler-order".equals(codeString)) 608 return new Enumeration<TaskIntent>(this, TaskIntent.FILLERORDER, code); 609 if ("instance-order".equals(codeString)) 610 return new Enumeration<TaskIntent>(this, TaskIntent.INSTANCEORDER, code); 611 if ("option".equals(codeString)) 612 return new Enumeration<TaskIntent>(this, TaskIntent.OPTION, code); 613 throw new FHIRException("Unknown TaskIntent code '" + codeString + "'"); 614 } 615 616 public String toCode(TaskIntent code) { 617 if (code == TaskIntent.NULL) 618 return null; 619 if (code == TaskIntent.UNKNOWN) 620 return "unknown"; 621 if (code == TaskIntent.PROPOSAL) 622 return "proposal"; 623 if (code == TaskIntent.PLAN) 624 return "plan"; 625 if (code == TaskIntent.ORDER) 626 return "order"; 627 if (code == TaskIntent.ORIGINALORDER) 628 return "original-order"; 629 if (code == TaskIntent.REFLEXORDER) 630 return "reflex-order"; 631 if (code == TaskIntent.FILLERORDER) 632 return "filler-order"; 633 if (code == TaskIntent.INSTANCEORDER) 634 return "instance-order"; 635 if (code == TaskIntent.OPTION) 636 return "option"; 637 return "?"; 638 } 639 640 public String toSystem(TaskIntent code) { 641 return code.getSystem(); 642 } 643 } 644 645 public enum TaskPriority { 646 /** 647 * The request has normal priority. 648 */ 649 ROUTINE, 650 /** 651 * The request should be actioned promptly - higher priority than routine. 652 */ 653 URGENT, 654 /** 655 * The request should be actioned as soon as possible - higher priority than 656 * urgent. 657 */ 658 ASAP, 659 /** 660 * The request should be actioned immediately - highest possible priority. E.g. 661 * an emergency. 662 */ 663 STAT, 664 /** 665 * added to help the parsers with the generic types 666 */ 667 NULL; 668 669 public static TaskPriority fromCode(String codeString) throws FHIRException { 670 if (codeString == null || "".equals(codeString)) 671 return null; 672 if ("routine".equals(codeString)) 673 return ROUTINE; 674 if ("urgent".equals(codeString)) 675 return URGENT; 676 if ("asap".equals(codeString)) 677 return ASAP; 678 if ("stat".equals(codeString)) 679 return STAT; 680 if (Configuration.isAcceptInvalidEnums()) 681 return null; 682 else 683 throw new FHIRException("Unknown TaskPriority code '" + codeString + "'"); 684 } 685 686 public String toCode() { 687 switch (this) { 688 case ROUTINE: 689 return "routine"; 690 case URGENT: 691 return "urgent"; 692 case ASAP: 693 return "asap"; 694 case STAT: 695 return "stat"; 696 case NULL: 697 return null; 698 default: 699 return "?"; 700 } 701 } 702 703 public String getSystem() { 704 switch (this) { 705 case ROUTINE: 706 return "http://hl7.org/fhir/request-priority"; 707 case URGENT: 708 return "http://hl7.org/fhir/request-priority"; 709 case ASAP: 710 return "http://hl7.org/fhir/request-priority"; 711 case STAT: 712 return "http://hl7.org/fhir/request-priority"; 713 case NULL: 714 return null; 715 default: 716 return "?"; 717 } 718 } 719 720 public String getDefinition() { 721 switch (this) { 722 case ROUTINE: 723 return "The request has normal priority."; 724 case URGENT: 725 return "The request should be actioned promptly - higher priority than routine."; 726 case ASAP: 727 return "The request should be actioned as soon as possible - higher priority than urgent."; 728 case STAT: 729 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 730 case NULL: 731 return null; 732 default: 733 return "?"; 734 } 735 } 736 737 public String getDisplay() { 738 switch (this) { 739 case ROUTINE: 740 return "Routine"; 741 case URGENT: 742 return "Urgent"; 743 case ASAP: 744 return "ASAP"; 745 case STAT: 746 return "STAT"; 747 case NULL: 748 return null; 749 default: 750 return "?"; 751 } 752 } 753 } 754 755 public static class TaskPriorityEnumFactory implements EnumFactory<TaskPriority> { 756 public TaskPriority fromCode(String codeString) throws IllegalArgumentException { 757 if (codeString == null || "".equals(codeString)) 758 if (codeString == null || "".equals(codeString)) 759 return null; 760 if ("routine".equals(codeString)) 761 return TaskPriority.ROUTINE; 762 if ("urgent".equals(codeString)) 763 return TaskPriority.URGENT; 764 if ("asap".equals(codeString)) 765 return TaskPriority.ASAP; 766 if ("stat".equals(codeString)) 767 return TaskPriority.STAT; 768 throw new IllegalArgumentException("Unknown TaskPriority code '" + codeString + "'"); 769 } 770 771 public Enumeration<TaskPriority> fromType(PrimitiveType<?> code) throws FHIRException { 772 if (code == null) 773 return null; 774 if (code.isEmpty()) 775 return new Enumeration<TaskPriority>(this, TaskPriority.NULL, code); 776 String codeString = code.asStringValue(); 777 if (codeString == null || "".equals(codeString)) 778 return new Enumeration<TaskPriority>(this, TaskPriority.NULL, code); 779 if ("routine".equals(codeString)) 780 return new Enumeration<TaskPriority>(this, TaskPriority.ROUTINE, code); 781 if ("urgent".equals(codeString)) 782 return new Enumeration<TaskPriority>(this, TaskPriority.URGENT, code); 783 if ("asap".equals(codeString)) 784 return new Enumeration<TaskPriority>(this, TaskPriority.ASAP, code); 785 if ("stat".equals(codeString)) 786 return new Enumeration<TaskPriority>(this, TaskPriority.STAT, code); 787 throw new FHIRException("Unknown TaskPriority code '" + codeString + "'"); 788 } 789 790 public String toCode(TaskPriority code) { 791 if (code == TaskPriority.NULL) 792 return null; 793 if (code == TaskPriority.ROUTINE) 794 return "routine"; 795 if (code == TaskPriority.URGENT) 796 return "urgent"; 797 if (code == TaskPriority.ASAP) 798 return "asap"; 799 if (code == TaskPriority.STAT) 800 return "stat"; 801 return "?"; 802 } 803 804 public String toSystem(TaskPriority code) { 805 return code.getSystem(); 806 } 807 } 808 809 @Block() 810 public static class TaskRestrictionComponent extends BackboneElement implements IBaseBackboneElement { 811 /** 812 * Indicates the number of times the requested action should occur. 813 */ 814 @Child(name = "repetitions", type = { 815 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 816 @Description(shortDefinition = "How many times to repeat", formalDefinition = "Indicates the number of times the requested action should occur.") 817 protected PositiveIntType repetitions; 818 819 /** 820 * Over what time-period is fulfillment sought. 821 */ 822 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 823 @Description(shortDefinition = "When fulfillment sought", formalDefinition = "Over what time-period is fulfillment sought.") 824 protected Period period; 825 826 /** 827 * For requests that are targeted to more than on potential recipient/target, 828 * for whom is fulfillment sought? 829 */ 830 @Child(name = "recipient", type = { Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, 831 Group.class, 832 Organization.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 833 @Description(shortDefinition = "For whom is fulfillment sought?", formalDefinition = "For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?") 834 protected List<Reference> recipient; 835 /** 836 * The actual objects that are the target of the reference (For requests that 837 * are targeted to more than on potential recipient/target, for whom is 838 * fulfillment sought?) 839 */ 840 protected List<Resource> recipientTarget; 841 842 private static final long serialVersionUID = 1503908360L; 843 844 /** 845 * Constructor 846 */ 847 public TaskRestrictionComponent() { 848 super(); 849 } 850 851 /** 852 * @return {@link #repetitions} (Indicates the number of times the requested 853 * action should occur.). This is the underlying object with id, value 854 * and extensions. The accessor "getRepetitions" gives direct access to 855 * the value 856 */ 857 public PositiveIntType getRepetitionsElement() { 858 if (this.repetitions == null) 859 if (Configuration.errorOnAutoCreate()) 860 throw new Error("Attempt to auto-create TaskRestrictionComponent.repetitions"); 861 else if (Configuration.doAutoCreate()) 862 this.repetitions = new PositiveIntType(); // bb 863 return this.repetitions; 864 } 865 866 public boolean hasRepetitionsElement() { 867 return this.repetitions != null && !this.repetitions.isEmpty(); 868 } 869 870 public boolean hasRepetitions() { 871 return this.repetitions != null && !this.repetitions.isEmpty(); 872 } 873 874 /** 875 * @param value {@link #repetitions} (Indicates the number of times the 876 * requested action should occur.). This is the underlying object 877 * with id, value and extensions. The accessor "getRepetitions" 878 * gives direct access to the value 879 */ 880 public TaskRestrictionComponent setRepetitionsElement(PositiveIntType value) { 881 this.repetitions = value; 882 return this; 883 } 884 885 /** 886 * @return Indicates the number of times the requested action should occur. 887 */ 888 public int getRepetitions() { 889 return this.repetitions == null || this.repetitions.isEmpty() ? 0 : this.repetitions.getValue(); 890 } 891 892 /** 893 * @param value Indicates the number of times the requested action should occur. 894 */ 895 public TaskRestrictionComponent setRepetitions(int value) { 896 if (this.repetitions == null) 897 this.repetitions = new PositiveIntType(); 898 this.repetitions.setValue(value); 899 return this; 900 } 901 902 /** 903 * @return {@link #period} (Over what time-period is fulfillment sought.) 904 */ 905 public Period getPeriod() { 906 if (this.period == null) 907 if (Configuration.errorOnAutoCreate()) 908 throw new Error("Attempt to auto-create TaskRestrictionComponent.period"); 909 else if (Configuration.doAutoCreate()) 910 this.period = new Period(); // cc 911 return this.period; 912 } 913 914 public boolean hasPeriod() { 915 return this.period != null && !this.period.isEmpty(); 916 } 917 918 /** 919 * @param value {@link #period} (Over what time-period is fulfillment sought.) 920 */ 921 public TaskRestrictionComponent setPeriod(Period value) { 922 this.period = value; 923 return this; 924 } 925 926 /** 927 * @return {@link #recipient} (For requests that are targeted to more than on 928 * potential recipient/target, for whom is fulfillment sought?) 929 */ 930 public List<Reference> getRecipient() { 931 if (this.recipient == null) 932 this.recipient = new ArrayList<Reference>(); 933 return this.recipient; 934 } 935 936 /** 937 * @return Returns a reference to <code>this</code> for easy method chaining 938 */ 939 public TaskRestrictionComponent setRecipient(List<Reference> theRecipient) { 940 this.recipient = theRecipient; 941 return this; 942 } 943 944 public boolean hasRecipient() { 945 if (this.recipient == null) 946 return false; 947 for (Reference item : this.recipient) 948 if (!item.isEmpty()) 949 return true; 950 return false; 951 } 952 953 public Reference addRecipient() { // 3 954 Reference t = new Reference(); 955 if (this.recipient == null) 956 this.recipient = new ArrayList<Reference>(); 957 this.recipient.add(t); 958 return t; 959 } 960 961 public TaskRestrictionComponent addRecipient(Reference t) { // 3 962 if (t == null) 963 return this; 964 if (this.recipient == null) 965 this.recipient = new ArrayList<Reference>(); 966 this.recipient.add(t); 967 return this; 968 } 969 970 /** 971 * @return The first repetition of repeating field {@link #recipient}, creating 972 * it if it does not already exist 973 */ 974 public Reference getRecipientFirstRep() { 975 if (getRecipient().isEmpty()) { 976 addRecipient(); 977 } 978 return getRecipient().get(0); 979 } 980 981 protected void listChildren(List<Property> children) { 982 super.listChildren(children); 983 children.add(new Property("repetitions", "positiveInt", 984 "Indicates the number of times the requested action should occur.", 0, 1, repetitions)); 985 children.add(new Property("period", "Period", "Over what time-period is fulfillment sought.", 0, 1, period)); 986 children.add(new Property("recipient", 987 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Group|Organization)", 988 "For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?", 989 0, java.lang.Integer.MAX_VALUE, recipient)); 990 } 991 992 @Override 993 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 994 switch (_hash) { 995 case 984367650: 996 /* repetitions */ return new Property("repetitions", "positiveInt", 997 "Indicates the number of times the requested action should occur.", 0, 1, repetitions); 998 case -991726143: 999 /* period */ return new Property("period", "Period", "Over what time-period is fulfillment sought.", 0, 1, 1000 period); 1001 case 820081177: 1002 /* recipient */ return new Property("recipient", 1003 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Group|Organization)", 1004 "For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?", 1005 0, java.lang.Integer.MAX_VALUE, recipient); 1006 default: 1007 return super.getNamedProperty(_hash, _name, _checkValid); 1008 } 1009 1010 } 1011 1012 @Override 1013 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1014 switch (hash) { 1015 case 984367650: 1016 /* repetitions */ return this.repetitions == null ? new Base[0] : new Base[] { this.repetitions }; // PositiveIntType 1017 case -991726143: 1018 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1019 case 820081177: 1020 /* recipient */ return this.recipient == null ? new Base[0] 1021 : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1022 default: 1023 return super.getProperty(hash, name, checkValid); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1030 switch (hash) { 1031 case 984367650: // repetitions 1032 this.repetitions = castToPositiveInt(value); // PositiveIntType 1033 return value; 1034 case -991726143: // period 1035 this.period = castToPeriod(value); // Period 1036 return value; 1037 case 820081177: // recipient 1038 this.getRecipient().add(castToReference(value)); // Reference 1039 return value; 1040 default: 1041 return super.setProperty(hash, name, value); 1042 } 1043 1044 } 1045 1046 @Override 1047 public Base setProperty(String name, Base value) throws FHIRException { 1048 if (name.equals("repetitions")) { 1049 this.repetitions = castToPositiveInt(value); // PositiveIntType 1050 } else if (name.equals("period")) { 1051 this.period = castToPeriod(value); // Period 1052 } else if (name.equals("recipient")) { 1053 this.getRecipient().add(castToReference(value)); 1054 } else 1055 return super.setProperty(name, value); 1056 return value; 1057 } 1058 1059 @Override 1060 public void removeChild(String name, Base value) throws FHIRException { 1061 if (name.equals("repetitions")) { 1062 this.repetitions = null; 1063 } else if (name.equals("period")) { 1064 this.period = null; 1065 } else if (name.equals("recipient")) { 1066 this.getRecipient().remove(castToReference(value)); 1067 } else 1068 super.removeChild(name, value); 1069 1070 } 1071 1072 @Override 1073 public Base makeProperty(int hash, String name) throws FHIRException { 1074 switch (hash) { 1075 case 984367650: 1076 return getRepetitionsElement(); 1077 case -991726143: 1078 return getPeriod(); 1079 case 820081177: 1080 return addRecipient(); 1081 default: 1082 return super.makeProperty(hash, name); 1083 } 1084 1085 } 1086 1087 @Override 1088 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1089 switch (hash) { 1090 case 984367650: 1091 /* repetitions */ return new String[] { "positiveInt" }; 1092 case -991726143: 1093 /* period */ return new String[] { "Period" }; 1094 case 820081177: 1095 /* recipient */ return new String[] { "Reference" }; 1096 default: 1097 return super.getTypesForProperty(hash, name); 1098 } 1099 1100 } 1101 1102 @Override 1103 public Base addChild(String name) throws FHIRException { 1104 if (name.equals("repetitions")) { 1105 throw new FHIRException("Cannot call addChild on a singleton property Task.repetitions"); 1106 } else if (name.equals("period")) { 1107 this.period = new Period(); 1108 return this.period; 1109 } else if (name.equals("recipient")) { 1110 return addRecipient(); 1111 } else 1112 return super.addChild(name); 1113 } 1114 1115 public TaskRestrictionComponent copy() { 1116 TaskRestrictionComponent dst = new TaskRestrictionComponent(); 1117 copyValues(dst); 1118 return dst; 1119 } 1120 1121 public void copyValues(TaskRestrictionComponent dst) { 1122 super.copyValues(dst); 1123 dst.repetitions = repetitions == null ? null : repetitions.copy(); 1124 dst.period = period == null ? null : period.copy(); 1125 if (recipient != null) { 1126 dst.recipient = new ArrayList<Reference>(); 1127 for (Reference i : recipient) 1128 dst.recipient.add(i.copy()); 1129 } 1130 ; 1131 } 1132 1133 @Override 1134 public boolean equalsDeep(Base other_) { 1135 if (!super.equalsDeep(other_)) 1136 return false; 1137 if (!(other_ instanceof TaskRestrictionComponent)) 1138 return false; 1139 TaskRestrictionComponent o = (TaskRestrictionComponent) other_; 1140 return compareDeep(repetitions, o.repetitions, true) && compareDeep(period, o.period, true) 1141 && compareDeep(recipient, o.recipient, true); 1142 } 1143 1144 @Override 1145 public boolean equalsShallow(Base other_) { 1146 if (!super.equalsShallow(other_)) 1147 return false; 1148 if (!(other_ instanceof TaskRestrictionComponent)) 1149 return false; 1150 TaskRestrictionComponent o = (TaskRestrictionComponent) other_; 1151 return compareValues(repetitions, o.repetitions, true); 1152 } 1153 1154 public boolean isEmpty() { 1155 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(repetitions, period, recipient); 1156 } 1157 1158 public String fhirType() { 1159 return "Task.restriction"; 1160 1161 } 1162 1163 } 1164 1165 @Block() 1166 public static class ParameterComponent extends BackboneElement implements IBaseBackboneElement { 1167 /** 1168 * A code or description indicating how the input is intended to be used as part 1169 * of the task execution. 1170 */ 1171 @Child(name = "type", type = { 1172 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1173 @Description(shortDefinition = "Label for the input", formalDefinition = "A code or description indicating how the input is intended to be used as part of the task execution.") 1174 protected CodeableConcept type; 1175 1176 /** 1177 * The value of the input parameter as a basic type. 1178 */ 1179 @Child(name = "value", type = {}, order = 2, min = 1, max = 1, modifier = false, summary = false) 1180 @Description(shortDefinition = "Content to use in performing the task", formalDefinition = "The value of the input parameter as a basic type.") 1181 protected org.hl7.fhir.r4.model.Type value; 1182 1183 private static final long serialVersionUID = -850267045L; 1184 1185 /** 1186 * Constructor 1187 */ 1188 public ParameterComponent() { 1189 super(); 1190 } 1191 1192 /** 1193 * Constructor 1194 */ 1195 public ParameterComponent(CodeableConcept type, org.hl7.fhir.r4.model.Type value) { 1196 super(); 1197 this.type = type; 1198 this.value = value; 1199 } 1200 1201 /** 1202 * @return {@link #type} (A code or description indicating how the input is 1203 * intended to be used as part of the task execution.) 1204 */ 1205 public CodeableConcept getType() { 1206 if (this.type == null) 1207 if (Configuration.errorOnAutoCreate()) 1208 throw new Error("Attempt to auto-create ParameterComponent.type"); 1209 else if (Configuration.doAutoCreate()) 1210 this.type = new CodeableConcept(); // cc 1211 return this.type; 1212 } 1213 1214 public boolean hasType() { 1215 return this.type != null && !this.type.isEmpty(); 1216 } 1217 1218 /** 1219 * @param value {@link #type} (A code or description indicating how the input is 1220 * intended to be used as part of the task execution.) 1221 */ 1222 public ParameterComponent setType(CodeableConcept value) { 1223 this.type = value; 1224 return this; 1225 } 1226 1227 /** 1228 * @return {@link #value} (The value of the input parameter as a basic type.) 1229 */ 1230 public org.hl7.fhir.r4.model.Type getValue() { 1231 return this.value; 1232 } 1233 1234 public boolean hasValue() { 1235 return this.value != null && !this.value.isEmpty(); 1236 } 1237 1238 /** 1239 * @param value {@link #value} (The value of the input parameter as a basic 1240 * type.) 1241 */ 1242 public ParameterComponent setValue(org.hl7.fhir.r4.model.Type value) { 1243 this.value = value; 1244 return this; 1245 } 1246 1247 protected void listChildren(List<Property> children) { 1248 super.listChildren(children); 1249 children.add(new Property("type", "CodeableConcept", 1250 "A code or description indicating how the input is intended to be used as part of the task execution.", 0, 1, 1251 type)); 1252 children.add(new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value)); 1253 } 1254 1255 @Override 1256 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1257 switch (_hash) { 1258 case 3575610: 1259 /* type */ return new Property("type", "CodeableConcept", 1260 "A code or description indicating how the input is intended to be used as part of the task execution.", 0, 1261 1, type); 1262 case -1410166417: 1263 /* value[x] */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1264 value); 1265 case 111972721: 1266 /* value */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1267 value); 1268 case -1535024575: 1269 /* valueBase64Binary */ return new Property("value[x]", "*", 1270 "The value of the input parameter as a basic type.", 0, 1, value); 1271 case 733421943: 1272 /* valueBoolean */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1273 1, value); 1274 case -786218365: 1275 /* valueCanonical */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1276 0, 1, value); 1277 case -766209282: 1278 /* valueCode */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1279 value); 1280 case -766192449: 1281 /* valueDate */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1282 value); 1283 case 1047929900: 1284 /* valueDateTime */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1285 1, value); 1286 case -2083993440: 1287 /* valueDecimal */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1288 1, value); 1289 case 231604844: 1290 /* valueId */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1291 value); 1292 case -1668687056: 1293 /* valueInstant */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1294 1, value); 1295 case -1668204915: 1296 /* valueInteger */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1297 1, value); 1298 case -497880704: 1299 /* valueMarkdown */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1300 1, value); 1301 case -1410178407: 1302 /* valueOid */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1303 value); 1304 case -1249932027: 1305 /* valuePositiveInt */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1306 0, 1, value); 1307 case -1424603934: 1308 /* valueString */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1309 1, value); 1310 case -765708322: 1311 /* valueTime */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1312 value); 1313 case 26529417: 1314 /* valueUnsignedInt */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1315 0, 1, value); 1316 case -1410172357: 1317 /* valueUri */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1318 value); 1319 case -1410172354: 1320 /* valueUrl */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1321 value); 1322 case -765667124: 1323 /* valueUuid */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1324 value); 1325 case -478981821: 1326 /* valueAddress */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1327 1, value); 1328 case -67108992: 1329 /* valueAnnotation */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1330 0, 1, value); 1331 case -475566732: 1332 /* valueAttachment */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1333 0, 1, value); 1334 case 924902896: 1335 /* valueCodeableConcept */ return new Property("value[x]", "*", 1336 "The value of the input parameter as a basic type.", 0, 1, value); 1337 case -1887705029: 1338 /* valueCoding */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1339 1, value); 1340 case 944904545: 1341 /* valueContactPoint */ return new Property("value[x]", "*", 1342 "The value of the input parameter as a basic type.", 0, 1, value); 1343 case -2026205465: 1344 /* valueHumanName */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1345 0, 1, value); 1346 case -130498310: 1347 /* valueIdentifier */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1348 0, 1, value); 1349 case -1524344174: 1350 /* valuePeriod */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1351 1, value); 1352 case -2029823716: 1353 /* valueQuantity */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1354 1, value); 1355 case 2030761548: 1356 /* valueRange */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1357 value); 1358 case 2030767386: 1359 /* valueRatio */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1360 value); 1361 case 1755241690: 1362 /* valueReference */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1363 0, 1, value); 1364 case -962229101: 1365 /* valueSampledData */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1366 0, 1, value); 1367 case -540985785: 1368 /* valueSignature */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1369 0, 1, value); 1370 case -1406282469: 1371 /* valueTiming */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1372 1, value); 1373 case -1858636920: 1374 /* valueDosage */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1375 1, value); 1376 default: 1377 return super.getNamedProperty(_hash, _name, _checkValid); 1378 } 1379 1380 } 1381 1382 @Override 1383 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1384 switch (hash) { 1385 case 3575610: 1386 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1387 case 111972721: 1388 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // org.hl7.fhir.r4.model.Type 1389 default: 1390 return super.getProperty(hash, name, checkValid); 1391 } 1392 1393 } 1394 1395 @Override 1396 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1397 switch (hash) { 1398 case 3575610: // type 1399 this.type = castToCodeableConcept(value); // CodeableConcept 1400 return value; 1401 case 111972721: // value 1402 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 1403 return value; 1404 default: 1405 return super.setProperty(hash, name, value); 1406 } 1407 1408 } 1409 1410 @Override 1411 public Base setProperty(String name, Base value) throws FHIRException { 1412 if (name.equals("type")) { 1413 this.type = castToCodeableConcept(value); // CodeableConcept 1414 } else if (name.equals("value[x]")) { 1415 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 1416 } else 1417 return super.setProperty(name, value); 1418 return value; 1419 } 1420 1421 @Override 1422 public void removeChild(String name, Base value) throws FHIRException { 1423 if (name.equals("type")) { 1424 this.type = null; 1425 } else if (name.equals("value[x]")) { 1426 this.value = null; 1427 } else 1428 super.removeChild(name, value); 1429 1430 } 1431 1432 @Override 1433 public Base makeProperty(int hash, String name) throws FHIRException { 1434 switch (hash) { 1435 case 3575610: 1436 return getType(); 1437 case -1410166417: 1438 return getValue(); 1439 case 111972721: 1440 return getValue(); 1441 default: 1442 return super.makeProperty(hash, name); 1443 } 1444 1445 } 1446 1447 @Override 1448 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1449 switch (hash) { 1450 case 3575610: 1451 /* type */ return new String[] { "CodeableConcept" }; 1452 case 111972721: 1453 /* value */ return new String[] { "*" }; 1454 default: 1455 return super.getTypesForProperty(hash, name); 1456 } 1457 1458 } 1459 1460 @Override 1461 public Base addChild(String name) throws FHIRException { 1462 if (name.equals("type")) { 1463 this.type = new CodeableConcept(); 1464 return this.type; 1465 } else if (name.equals("valueBase64Binary")) { 1466 this.value = new Base64BinaryType(); 1467 return this.value; 1468 } else if (name.equals("valueBoolean")) { 1469 this.value = new BooleanType(); 1470 return this.value; 1471 } else if (name.equals("valueCanonical")) { 1472 this.value = new CanonicalType(); 1473 return this.value; 1474 } else if (name.equals("valueCode")) { 1475 this.value = new CodeType(); 1476 return this.value; 1477 } else if (name.equals("valueDate")) { 1478 this.value = new DateType(); 1479 return this.value; 1480 } else if (name.equals("valueDateTime")) { 1481 this.value = new DateTimeType(); 1482 return this.value; 1483 } else if (name.equals("valueDecimal")) { 1484 this.value = new DecimalType(); 1485 return this.value; 1486 } else if (name.equals("valueId")) { 1487 this.value = new IdType(); 1488 return this.value; 1489 } else if (name.equals("valueInstant")) { 1490 this.value = new InstantType(); 1491 return this.value; 1492 } else if (name.equals("valueInteger")) { 1493 this.value = new IntegerType(); 1494 return this.value; 1495 } else if (name.equals("valueMarkdown")) { 1496 this.value = new MarkdownType(); 1497 return this.value; 1498 } else if (name.equals("valueOid")) { 1499 this.value = new OidType(); 1500 return this.value; 1501 } else if (name.equals("valuePositiveInt")) { 1502 this.value = new PositiveIntType(); 1503 return this.value; 1504 } else if (name.equals("valueString")) { 1505 this.value = new StringType(); 1506 return this.value; 1507 } else if (name.equals("valueTime")) { 1508 this.value = new TimeType(); 1509 return this.value; 1510 } else if (name.equals("valueUnsignedInt")) { 1511 this.value = new UnsignedIntType(); 1512 return this.value; 1513 } else if (name.equals("valueUri")) { 1514 this.value = new UriType(); 1515 return this.value; 1516 } else if (name.equals("valueUrl")) { 1517 this.value = new UrlType(); 1518 return this.value; 1519 } else if (name.equals("valueUuid")) { 1520 this.value = new UuidType(); 1521 return this.value; 1522 } else if (name.equals("valueAddress")) { 1523 this.value = new Address(); 1524 return this.value; 1525 } else if (name.equals("valueAge")) { 1526 this.value = new Age(); 1527 return this.value; 1528 } else if (name.equals("valueAnnotation")) { 1529 this.value = new Annotation(); 1530 return this.value; 1531 } else if (name.equals("valueAttachment")) { 1532 this.value = new Attachment(); 1533 return this.value; 1534 } else if (name.equals("valueCodeableConcept")) { 1535 this.value = new CodeableConcept(); 1536 return this.value; 1537 } else if (name.equals("valueCoding")) { 1538 this.value = new Coding(); 1539 return this.value; 1540 } else if (name.equals("valueContactPoint")) { 1541 this.value = new ContactPoint(); 1542 return this.value; 1543 } else if (name.equals("valueCount")) { 1544 this.value = new Count(); 1545 return this.value; 1546 } else if (name.equals("valueDistance")) { 1547 this.value = new Distance(); 1548 return this.value; 1549 } else if (name.equals("valueDuration")) { 1550 this.value = new Duration(); 1551 return this.value; 1552 } else if (name.equals("valueHumanName")) { 1553 this.value = new HumanName(); 1554 return this.value; 1555 } else if (name.equals("valueIdentifier")) { 1556 this.value = new Identifier(); 1557 return this.value; 1558 } else if (name.equals("valueMoney")) { 1559 this.value = new Money(); 1560 return this.value; 1561 } else if (name.equals("valuePeriod")) { 1562 this.value = new Period(); 1563 return this.value; 1564 } else if (name.equals("valueQuantity")) { 1565 this.value = new Quantity(); 1566 return this.value; 1567 } else if (name.equals("valueRange")) { 1568 this.value = new Range(); 1569 return this.value; 1570 } else if (name.equals("valueRatio")) { 1571 this.value = new Ratio(); 1572 return this.value; 1573 } else if (name.equals("valueReference")) { 1574 this.value = new Reference(); 1575 return this.value; 1576 } else if (name.equals("valueSampledData")) { 1577 this.value = new SampledData(); 1578 return this.value; 1579 } else if (name.equals("valueSignature")) { 1580 this.value = new Signature(); 1581 return this.value; 1582 } else if (name.equals("valueTiming")) { 1583 this.value = new Timing(); 1584 return this.value; 1585 } else if (name.equals("valueContactDetail")) { 1586 this.value = new ContactDetail(); 1587 return this.value; 1588 } else if (name.equals("valueContributor")) { 1589 this.value = new Contributor(); 1590 return this.value; 1591 } else if (name.equals("valueDataRequirement")) { 1592 this.value = new DataRequirement(); 1593 return this.value; 1594 } else if (name.equals("valueExpression")) { 1595 this.value = new Expression(); 1596 return this.value; 1597 } else if (name.equals("valueParameterDefinition")) { 1598 this.value = new ParameterDefinition(); 1599 return this.value; 1600 } else if (name.equals("valueRelatedArtifact")) { 1601 this.value = new RelatedArtifact(); 1602 return this.value; 1603 } else if (name.equals("valueTriggerDefinition")) { 1604 this.value = new TriggerDefinition(); 1605 return this.value; 1606 } else if (name.equals("valueUsageContext")) { 1607 this.value = new UsageContext(); 1608 return this.value; 1609 } else if (name.equals("valueDosage")) { 1610 this.value = new Dosage(); 1611 return this.value; 1612 } else if (name.equals("valueMeta")) { 1613 this.value = new Meta(); 1614 return this.value; 1615 } else 1616 return super.addChild(name); 1617 } 1618 1619 public ParameterComponent copy() { 1620 ParameterComponent dst = new ParameterComponent(); 1621 copyValues(dst); 1622 return dst; 1623 } 1624 1625 public void copyValues(ParameterComponent dst) { 1626 super.copyValues(dst); 1627 dst.type = type == null ? null : type.copy(); 1628 dst.value = value == null ? null : value.copy(); 1629 } 1630 1631 @Override 1632 public boolean equalsDeep(Base other_) { 1633 if (!super.equalsDeep(other_)) 1634 return false; 1635 if (!(other_ instanceof ParameterComponent)) 1636 return false; 1637 ParameterComponent o = (ParameterComponent) other_; 1638 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 1639 } 1640 1641 @Override 1642 public boolean equalsShallow(Base other_) { 1643 if (!super.equalsShallow(other_)) 1644 return false; 1645 if (!(other_ instanceof ParameterComponent)) 1646 return false; 1647 ParameterComponent o = (ParameterComponent) other_; 1648 return true; 1649 } 1650 1651 public boolean isEmpty() { 1652 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 1653 } 1654 1655 public String fhirType() { 1656 return "Task.input"; 1657 1658 } 1659 1660 } 1661 1662 @Block() 1663 public static class TaskOutputComponent extends BackboneElement implements IBaseBackboneElement { 1664 /** 1665 * The name of the Output parameter. 1666 */ 1667 @Child(name = "type", type = { 1668 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1669 @Description(shortDefinition = "Label for output", formalDefinition = "The name of the Output parameter.") 1670 protected CodeableConcept type; 1671 1672 /** 1673 * The value of the Output parameter as a basic type. 1674 */ 1675 @Child(name = "value", type = {}, order = 2, min = 1, max = 1, modifier = false, summary = false) 1676 @Description(shortDefinition = "Result of output", formalDefinition = "The value of the Output parameter as a basic type.") 1677 protected org.hl7.fhir.r4.model.Type value; 1678 1679 private static final long serialVersionUID = -850267045L; 1680 1681 /** 1682 * Constructor 1683 */ 1684 public TaskOutputComponent() { 1685 super(); 1686 } 1687 1688 /** 1689 * Constructor 1690 */ 1691 public TaskOutputComponent(CodeableConcept type, org.hl7.fhir.r4.model.Type value) { 1692 super(); 1693 this.type = type; 1694 this.value = value; 1695 } 1696 1697 /** 1698 * @return {@link #type} (The name of the Output parameter.) 1699 */ 1700 public CodeableConcept getType() { 1701 if (this.type == null) 1702 if (Configuration.errorOnAutoCreate()) 1703 throw new Error("Attempt to auto-create TaskOutputComponent.type"); 1704 else if (Configuration.doAutoCreate()) 1705 this.type = new CodeableConcept(); // cc 1706 return this.type; 1707 } 1708 1709 public boolean hasType() { 1710 return this.type != null && !this.type.isEmpty(); 1711 } 1712 1713 /** 1714 * @param value {@link #type} (The name of the Output parameter.) 1715 */ 1716 public TaskOutputComponent setType(CodeableConcept value) { 1717 this.type = value; 1718 return this; 1719 } 1720 1721 /** 1722 * @return {@link #value} (The value of the Output parameter as a basic type.) 1723 */ 1724 public org.hl7.fhir.r4.model.Type getValue() { 1725 return this.value; 1726 } 1727 1728 public boolean hasValue() { 1729 return this.value != null && !this.value.isEmpty(); 1730 } 1731 1732 /** 1733 * @param value {@link #value} (The value of the Output parameter as a basic 1734 * type.) 1735 */ 1736 public TaskOutputComponent setValue(org.hl7.fhir.r4.model.Type value) { 1737 this.value = value; 1738 return this; 1739 } 1740 1741 protected void listChildren(List<Property> children) { 1742 super.listChildren(children); 1743 children.add(new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type)); 1744 children.add(new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value)); 1745 } 1746 1747 @Override 1748 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1749 switch (_hash) { 1750 case 3575610: 1751 /* type */ return new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type); 1752 case -1410166417: 1753 /* value[x] */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1754 value); 1755 case 111972721: 1756 /* value */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1757 value); 1758 case -1535024575: 1759 /* valueBase64Binary */ return new Property("value[x]", "*", 1760 "The value of the Output parameter as a basic type.", 0, 1, value); 1761 case 733421943: 1762 /* valueBoolean */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1763 1, value); 1764 case -786218365: 1765 /* valueCanonical */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1766 0, 1, value); 1767 case -766209282: 1768 /* valueCode */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1769 value); 1770 case -766192449: 1771 /* valueDate */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1772 value); 1773 case 1047929900: 1774 /* valueDateTime */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1775 0, 1, value); 1776 case -2083993440: 1777 /* valueDecimal */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1778 1, value); 1779 case 231604844: 1780 /* valueId */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1781 value); 1782 case -1668687056: 1783 /* valueInstant */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1784 1, value); 1785 case -1668204915: 1786 /* valueInteger */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1787 1, value); 1788 case -497880704: 1789 /* valueMarkdown */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1790 0, 1, value); 1791 case -1410178407: 1792 /* valueOid */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1793 value); 1794 case -1249932027: 1795 /* valuePositiveInt */ return new Property("value[x]", "*", 1796 "The value of the Output parameter as a basic type.", 0, 1, value); 1797 case -1424603934: 1798 /* valueString */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1799 1, value); 1800 case -765708322: 1801 /* valueTime */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1802 value); 1803 case 26529417: 1804 /* valueUnsignedInt */ return new Property("value[x]", "*", 1805 "The value of the Output parameter as a basic type.", 0, 1, value); 1806 case -1410172357: 1807 /* valueUri */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1808 value); 1809 case -1410172354: 1810 /* valueUrl */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1811 value); 1812 case -765667124: 1813 /* valueUuid */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1814 value); 1815 case -478981821: 1816 /* valueAddress */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1817 1, value); 1818 case -67108992: 1819 /* valueAnnotation */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1820 0, 1, value); 1821 case -475566732: 1822 /* valueAttachment */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1823 0, 1, value); 1824 case 924902896: 1825 /* valueCodeableConcept */ return new Property("value[x]", "*", 1826 "The value of the Output parameter as a basic type.", 0, 1, value); 1827 case -1887705029: 1828 /* valueCoding */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1829 1, value); 1830 case 944904545: 1831 /* valueContactPoint */ return new Property("value[x]", "*", 1832 "The value of the Output parameter as a basic type.", 0, 1, value); 1833 case -2026205465: 1834 /* valueHumanName */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1835 0, 1, value); 1836 case -130498310: 1837 /* valueIdentifier */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1838 0, 1, value); 1839 case -1524344174: 1840 /* valuePeriod */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1841 1, value); 1842 case -2029823716: 1843 /* valueQuantity */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1844 0, 1, value); 1845 case 2030761548: 1846 /* valueRange */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1847 1, value); 1848 case 2030767386: 1849 /* valueRatio */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1850 1, value); 1851 case 1755241690: 1852 /* valueReference */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1853 0, 1, value); 1854 case -962229101: 1855 /* valueSampledData */ return new Property("value[x]", "*", 1856 "The value of the Output parameter as a basic type.", 0, 1, value); 1857 case -540985785: 1858 /* valueSignature */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1859 0, 1, value); 1860 case -1406282469: 1861 /* valueTiming */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1862 1, value); 1863 case -1858636920: 1864 /* valueDosage */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1865 1, value); 1866 default: 1867 return super.getNamedProperty(_hash, _name, _checkValid); 1868 } 1869 1870 } 1871 1872 @Override 1873 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1874 switch (hash) { 1875 case 3575610: 1876 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1877 case 111972721: 1878 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // org.hl7.fhir.r4.model.Type 1879 default: 1880 return super.getProperty(hash, name, checkValid); 1881 } 1882 1883 } 1884 1885 @Override 1886 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1887 switch (hash) { 1888 case 3575610: // type 1889 this.type = castToCodeableConcept(value); // CodeableConcept 1890 return value; 1891 case 111972721: // value 1892 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 1893 return value; 1894 default: 1895 return super.setProperty(hash, name, value); 1896 } 1897 1898 } 1899 1900 @Override 1901 public Base setProperty(String name, Base value) throws FHIRException { 1902 if (name.equals("type")) { 1903 this.type = castToCodeableConcept(value); // CodeableConcept 1904 } else if (name.equals("value[x]")) { 1905 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 1906 } else 1907 return super.setProperty(name, value); 1908 return value; 1909 } 1910 1911 @Override 1912 public void removeChild(String name, Base value) throws FHIRException { 1913 if (name.equals("type")) { 1914 this.type = null; 1915 } else if (name.equals("value[x]")) { 1916 this.value = null; 1917 } else 1918 super.removeChild(name, value); 1919 1920 } 1921 1922 @Override 1923 public Base makeProperty(int hash, String name) throws FHIRException { 1924 switch (hash) { 1925 case 3575610: 1926 return getType(); 1927 case -1410166417: 1928 return getValue(); 1929 case 111972721: 1930 return getValue(); 1931 default: 1932 return super.makeProperty(hash, name); 1933 } 1934 1935 } 1936 1937 @Override 1938 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1939 switch (hash) { 1940 case 3575610: 1941 /* type */ return new String[] { "CodeableConcept" }; 1942 case 111972721: 1943 /* value */ return new String[] { "*" }; 1944 default: 1945 return super.getTypesForProperty(hash, name); 1946 } 1947 1948 } 1949 1950 @Override 1951 public Base addChild(String name) throws FHIRException { 1952 if (name.equals("type")) { 1953 this.type = new CodeableConcept(); 1954 return this.type; 1955 } else if (name.equals("valueBase64Binary")) { 1956 this.value = new Base64BinaryType(); 1957 return this.value; 1958 } else if (name.equals("valueBoolean")) { 1959 this.value = new BooleanType(); 1960 return this.value; 1961 } else if (name.equals("valueCanonical")) { 1962 this.value = new CanonicalType(); 1963 return this.value; 1964 } else if (name.equals("valueCode")) { 1965 this.value = new CodeType(); 1966 return this.value; 1967 } else if (name.equals("valueDate")) { 1968 this.value = new DateType(); 1969 return this.value; 1970 } else if (name.equals("valueDateTime")) { 1971 this.value = new DateTimeType(); 1972 return this.value; 1973 } else if (name.equals("valueDecimal")) { 1974 this.value = new DecimalType(); 1975 return this.value; 1976 } else if (name.equals("valueId")) { 1977 this.value = new IdType(); 1978 return this.value; 1979 } else if (name.equals("valueInstant")) { 1980 this.value = new InstantType(); 1981 return this.value; 1982 } else if (name.equals("valueInteger")) { 1983 this.value = new IntegerType(); 1984 return this.value; 1985 } else if (name.equals("valueMarkdown")) { 1986 this.value = new MarkdownType(); 1987 return this.value; 1988 } else if (name.equals("valueOid")) { 1989 this.value = new OidType(); 1990 return this.value; 1991 } else if (name.equals("valuePositiveInt")) { 1992 this.value = new PositiveIntType(); 1993 return this.value; 1994 } else if (name.equals("valueString")) { 1995 this.value = new StringType(); 1996 return this.value; 1997 } else if (name.equals("valueTime")) { 1998 this.value = new TimeType(); 1999 return this.value; 2000 } else if (name.equals("valueUnsignedInt")) { 2001 this.value = new UnsignedIntType(); 2002 return this.value; 2003 } else if (name.equals("valueUri")) { 2004 this.value = new UriType(); 2005 return this.value; 2006 } else if (name.equals("valueUrl")) { 2007 this.value = new UrlType(); 2008 return this.value; 2009 } else if (name.equals("valueUuid")) { 2010 this.value = new UuidType(); 2011 return this.value; 2012 } else if (name.equals("valueAddress")) { 2013 this.value = new Address(); 2014 return this.value; 2015 } else if (name.equals("valueAge")) { 2016 this.value = new Age(); 2017 return this.value; 2018 } else if (name.equals("valueAnnotation")) { 2019 this.value = new Annotation(); 2020 return this.value; 2021 } else if (name.equals("valueAttachment")) { 2022 this.value = new Attachment(); 2023 return this.value; 2024 } else if (name.equals("valueCodeableConcept")) { 2025 this.value = new CodeableConcept(); 2026 return this.value; 2027 } else if (name.equals("valueCoding")) { 2028 this.value = new Coding(); 2029 return this.value; 2030 } else if (name.equals("valueContactPoint")) { 2031 this.value = new ContactPoint(); 2032 return this.value; 2033 } else if (name.equals("valueCount")) { 2034 this.value = new Count(); 2035 return this.value; 2036 } else if (name.equals("valueDistance")) { 2037 this.value = new Distance(); 2038 return this.value; 2039 } else if (name.equals("valueDuration")) { 2040 this.value = new Duration(); 2041 return this.value; 2042 } else if (name.equals("valueHumanName")) { 2043 this.value = new HumanName(); 2044 return this.value; 2045 } else if (name.equals("valueIdentifier")) { 2046 this.value = new Identifier(); 2047 return this.value; 2048 } else if (name.equals("valueMoney")) { 2049 this.value = new Money(); 2050 return this.value; 2051 } else if (name.equals("valuePeriod")) { 2052 this.value = new Period(); 2053 return this.value; 2054 } else if (name.equals("valueQuantity")) { 2055 this.value = new Quantity(); 2056 return this.value; 2057 } else if (name.equals("valueRange")) { 2058 this.value = new Range(); 2059 return this.value; 2060 } else if (name.equals("valueRatio")) { 2061 this.value = new Ratio(); 2062 return this.value; 2063 } else if (name.equals("valueReference")) { 2064 this.value = new Reference(); 2065 return this.value; 2066 } else if (name.equals("valueSampledData")) { 2067 this.value = new SampledData(); 2068 return this.value; 2069 } else if (name.equals("valueSignature")) { 2070 this.value = new Signature(); 2071 return this.value; 2072 } else if (name.equals("valueTiming")) { 2073 this.value = new Timing(); 2074 return this.value; 2075 } else if (name.equals("valueContactDetail")) { 2076 this.value = new ContactDetail(); 2077 return this.value; 2078 } else if (name.equals("valueContributor")) { 2079 this.value = new Contributor(); 2080 return this.value; 2081 } else if (name.equals("valueDataRequirement")) { 2082 this.value = new DataRequirement(); 2083 return this.value; 2084 } else if (name.equals("valueExpression")) { 2085 this.value = new Expression(); 2086 return this.value; 2087 } else if (name.equals("valueParameterDefinition")) { 2088 this.value = new ParameterDefinition(); 2089 return this.value; 2090 } else if (name.equals("valueRelatedArtifact")) { 2091 this.value = new RelatedArtifact(); 2092 return this.value; 2093 } else if (name.equals("valueTriggerDefinition")) { 2094 this.value = new TriggerDefinition(); 2095 return this.value; 2096 } else if (name.equals("valueUsageContext")) { 2097 this.value = new UsageContext(); 2098 return this.value; 2099 } else if (name.equals("valueDosage")) { 2100 this.value = new Dosage(); 2101 return this.value; 2102 } else if (name.equals("valueMeta")) { 2103 this.value = new Meta(); 2104 return this.value; 2105 } else 2106 return super.addChild(name); 2107 } 2108 2109 public TaskOutputComponent copy() { 2110 TaskOutputComponent dst = new TaskOutputComponent(); 2111 copyValues(dst); 2112 return dst; 2113 } 2114 2115 public void copyValues(TaskOutputComponent dst) { 2116 super.copyValues(dst); 2117 dst.type = type == null ? null : type.copy(); 2118 dst.value = value == null ? null : value.copy(); 2119 } 2120 2121 @Override 2122 public boolean equalsDeep(Base other_) { 2123 if (!super.equalsDeep(other_)) 2124 return false; 2125 if (!(other_ instanceof TaskOutputComponent)) 2126 return false; 2127 TaskOutputComponent o = (TaskOutputComponent) other_; 2128 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2129 } 2130 2131 @Override 2132 public boolean equalsShallow(Base other_) { 2133 if (!super.equalsShallow(other_)) 2134 return false; 2135 if (!(other_ instanceof TaskOutputComponent)) 2136 return false; 2137 TaskOutputComponent o = (TaskOutputComponent) other_; 2138 return true; 2139 } 2140 2141 public boolean isEmpty() { 2142 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2143 } 2144 2145 public String fhirType() { 2146 return "Task.output"; 2147 2148 } 2149 2150 } 2151 2152 /** 2153 * The business identifier for this task. 2154 */ 2155 @Child(name = "identifier", type = { 2156 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2157 @Description(shortDefinition = "Task Instance Identifier", formalDefinition = "The business identifier for this task.") 2158 protected List<Identifier> identifier; 2159 2160 /** 2161 * The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other 2162 * definition that is adhered to in whole or in part by this Task. 2163 */ 2164 @Child(name = "instantiatesCanonical", type = { 2165 CanonicalType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2166 @Description(shortDefinition = "Formal definition of task", formalDefinition = "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.") 2167 protected CanonicalType instantiatesCanonical; 2168 2169 /** 2170 * The URL pointing to an *externally* maintained protocol, guideline, orderset 2171 * or other definition that is adhered to in whole or in part by this Task. 2172 */ 2173 @Child(name = "instantiatesUri", type = { 2174 UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2175 @Description(shortDefinition = "Formal definition of task", formalDefinition = "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.") 2176 protected UriType instantiatesUri; 2177 2178 /** 2179 * BasedOn refers to a higher-level authorization that triggered the creation of 2180 * the task. It references a "request" resource such as a ServiceRequest, 2181 * MedicationRequest, ServiceRequest, CarePlan, etc. which is distinct from the 2182 * "request" resource the task is seeking to fulfill. This latter resource is 2183 * referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a 2184 * task is created to fulfill a procedureRequest ( = FocusOn ) to collect a 2185 * specimen from a patient. 2186 */ 2187 @Child(name = "basedOn", type = { 2188 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2189 @Description(shortDefinition = "Request fulfilled by this task", formalDefinition = "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, ServiceRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a task is created to fulfill a procedureRequest ( = FocusOn ) to collect a specimen from a patient.") 2190 protected List<Reference> basedOn; 2191 /** 2192 * The actual objects that are the target of the reference (BasedOn refers to a 2193 * higher-level authorization that triggered the creation of the task. It 2194 * references a "request" resource such as a ServiceRequest, MedicationRequest, 2195 * ServiceRequest, CarePlan, etc. which is distinct from the "request" resource 2196 * the task is seeking to fulfill. This latter resource is referenced by 2197 * FocusOn. For example, based on a ServiceRequest (= BasedOn), a task is 2198 * created to fulfill a procedureRequest ( = FocusOn ) to collect a specimen 2199 * from a patient.) 2200 */ 2201 protected List<Resource> basedOnTarget; 2202 2203 /** 2204 * An identifier that links together multiple tasks and other requests that were 2205 * created in the same context. 2206 */ 2207 @Child(name = "groupIdentifier", type = { 2208 Identifier.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2209 @Description(shortDefinition = "Requisition or grouper id", formalDefinition = "An identifier that links together multiple tasks and other requests that were created in the same context.") 2210 protected Identifier groupIdentifier; 2211 2212 /** 2213 * Task that this particular task is part of. 2214 */ 2215 @Child(name = "partOf", type = { 2216 Task.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2217 @Description(shortDefinition = "Composite task", formalDefinition = "Task that this particular task is part of.") 2218 protected List<Reference> partOf; 2219 /** 2220 * The actual objects that are the target of the reference (Task that this 2221 * particular task is part of.) 2222 */ 2223 protected List<Task> partOfTarget; 2224 2225 /** 2226 * The current status of the task. 2227 */ 2228 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 2229 @Description(shortDefinition = "draft | requested | received | accepted | +", formalDefinition = "The current status of the task.") 2230 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/task-status") 2231 protected Enumeration<TaskStatus> status; 2232 2233 /** 2234 * An explanation as to why this task is held, failed, was refused, etc. 2235 */ 2236 @Child(name = "statusReason", type = { 2237 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2238 @Description(shortDefinition = "Reason for current status", formalDefinition = "An explanation as to why this task is held, failed, was refused, etc.") 2239 protected CodeableConcept statusReason; 2240 2241 /** 2242 * Contains business-specific nuances of the business state. 2243 */ 2244 @Child(name = "businessStatus", type = { 2245 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2246 @Description(shortDefinition = "E.g. \"Specimen collected\", \"IV prepped\"", formalDefinition = "Contains business-specific nuances of the business state.") 2247 protected CodeableConcept businessStatus; 2248 2249 /** 2250 * Indicates the "level" of actionability associated with the Task, i.e. 2251 * i+R[9]Cs this a proposed task, a planned task, an actionable task, etc. 2252 */ 2253 @Child(name = "intent", type = { CodeType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 2254 @Description(shortDefinition = "unknown | proposal | plan | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.") 2255 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/task-intent") 2256 protected Enumeration<TaskIntent> intent; 2257 2258 /** 2259 * Indicates how quickly the Task should be addressed with respect to other 2260 * requests. 2261 */ 2262 @Child(name = "priority", type = { CodeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2263 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the Task should be addressed with respect to other requests.") 2264 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 2265 protected Enumeration<TaskPriority> priority; 2266 2267 /** 2268 * A name or code (or both) briefly describing what the task involves. 2269 */ 2270 @Child(name = "code", type = { 2271 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 2272 @Description(shortDefinition = "Task Type", formalDefinition = "A name or code (or both) briefly describing what the task involves.") 2273 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/task-code") 2274 protected CodeableConcept code; 2275 2276 /** 2277 * A free-text description of what is to be performed. 2278 */ 2279 @Child(name = "description", type = { 2280 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 2281 @Description(shortDefinition = "Human-readable explanation of task", formalDefinition = "A free-text description of what is to be performed.") 2282 protected StringType description; 2283 2284 /** 2285 * The request being actioned or the resource being manipulated by this task. 2286 */ 2287 @Child(name = "focus", type = { Reference.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 2288 @Description(shortDefinition = "What task is acting on", formalDefinition = "The request being actioned or the resource being manipulated by this task.") 2289 protected Reference focus; 2290 2291 /** 2292 * The actual object that is the target of the reference (The request being 2293 * actioned or the resource being manipulated by this task.) 2294 */ 2295 protected Resource focusTarget; 2296 2297 /** 2298 * The entity who benefits from the performance of the service specified in the 2299 * task (e.g., the patient). 2300 */ 2301 @Child(name = "for", type = { Reference.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 2302 @Description(shortDefinition = "Beneficiary of the Task", formalDefinition = "The entity who benefits from the performance of the service specified in the task (e.g., the patient).") 2303 protected Reference for_; 2304 2305 /** 2306 * The actual object that is the target of the reference (The entity who 2307 * benefits from the performance of the service specified in the task (e.g., the 2308 * patient).) 2309 */ 2310 protected Resource for_Target; 2311 2312 /** 2313 * The healthcare event (e.g. a patient and healthcare provider interaction) 2314 * during which this task was created. 2315 */ 2316 @Child(name = "encounter", type = { Encounter.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 2317 @Description(shortDefinition = "Healthcare event during which this task originated", formalDefinition = "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.") 2318 protected Reference encounter; 2319 2320 /** 2321 * The actual object that is the target of the reference (The healthcare event 2322 * (e.g. a patient and healthcare provider interaction) during which this task 2323 * was created.) 2324 */ 2325 protected Encounter encounterTarget; 2326 2327 /** 2328 * Identifies the time action was first taken against the task (start) and/or 2329 * the time final action was taken against the task prior to marking it as 2330 * completed (end). 2331 */ 2332 @Child(name = "executionPeriod", type = { 2333 Period.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 2334 @Description(shortDefinition = "Start and end time of execution", formalDefinition = "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).") 2335 protected Period executionPeriod; 2336 2337 /** 2338 * The date and time this task was created. 2339 */ 2340 @Child(name = "authoredOn", type = { 2341 DateTimeType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 2342 @Description(shortDefinition = "Task Creation Date", formalDefinition = "The date and time this task was created.") 2343 protected DateTimeType authoredOn; 2344 2345 /** 2346 * The date and time of last modification to this task. 2347 */ 2348 @Child(name = "lastModified", type = { 2349 DateTimeType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 2350 @Description(shortDefinition = "Task Last Modified Date", formalDefinition = "The date and time of last modification to this task.") 2351 protected DateTimeType lastModified; 2352 2353 /** 2354 * The creator of the task. 2355 */ 2356 @Child(name = "requester", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 2357 PractitionerRole.class, RelatedPerson.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 2358 @Description(shortDefinition = "Who is asking for task to be done", formalDefinition = "The creator of the task.") 2359 protected Reference requester; 2360 2361 /** 2362 * The actual object that is the target of the reference (The creator of the 2363 * task.) 2364 */ 2365 protected Resource requesterTarget; 2366 2367 /** 2368 * The kind of participant that should perform the task. 2369 */ 2370 @Child(name = "performerType", type = { 2371 CodeableConcept.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2372 @Description(shortDefinition = "Requested performer", formalDefinition = "The kind of participant that should perform the task.") 2373 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/performer-role") 2374 protected List<CodeableConcept> performerType; 2375 2376 /** 2377 * Individual organization or Device currently responsible for task execution. 2378 */ 2379 @Child(name = "owner", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 2380 HealthcareService.class, Patient.class, Device.class, 2381 RelatedPerson.class }, order = 21, min = 0, max = 1, modifier = false, summary = true) 2382 @Description(shortDefinition = "Responsible individual", formalDefinition = "Individual organization or Device currently responsible for task execution.") 2383 protected Reference owner; 2384 2385 /** 2386 * The actual object that is the target of the reference (Individual 2387 * organization or Device currently responsible for task execution.) 2388 */ 2389 protected Resource ownerTarget; 2390 2391 /** 2392 * Principal physical location where the this task is performed. 2393 */ 2394 @Child(name = "location", type = { Location.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 2395 @Description(shortDefinition = "Where task occurs", formalDefinition = "Principal physical location where the this task is performed.") 2396 protected Reference location; 2397 2398 /** 2399 * The actual object that is the target of the reference (Principal physical 2400 * location where the this task is performed.) 2401 */ 2402 protected Location locationTarget; 2403 2404 /** 2405 * A description or code indicating why this task needs to be performed. 2406 */ 2407 @Child(name = "reasonCode", type = { 2408 CodeableConcept.class }, order = 23, min = 0, max = 1, modifier = false, summary = false) 2409 @Description(shortDefinition = "Why task is needed", formalDefinition = "A description or code indicating why this task needs to be performed.") 2410 protected CodeableConcept reasonCode; 2411 2412 /** 2413 * A resource reference indicating why this task needs to be performed. 2414 */ 2415 @Child(name = "reasonReference", type = { 2416 Reference.class }, order = 24, min = 0, max = 1, modifier = false, summary = false) 2417 @Description(shortDefinition = "Why task is needed", formalDefinition = "A resource reference indicating why this task needs to be performed.") 2418 protected Reference reasonReference; 2419 2420 /** 2421 * The actual object that is the target of the reference (A resource reference 2422 * indicating why this task needs to be performed.) 2423 */ 2424 protected Resource reasonReferenceTarget; 2425 2426 /** 2427 * Insurance plans, coverage extensions, pre-authorizations and/or 2428 * pre-determinations that may be relevant to the Task. 2429 */ 2430 @Child(name = "insurance", type = { Coverage.class, 2431 ClaimResponse.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2432 @Description(shortDefinition = "Associated insurance coverage", formalDefinition = "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.") 2433 protected List<Reference> insurance; 2434 /** 2435 * The actual objects that are the target of the reference (Insurance plans, 2436 * coverage extensions, pre-authorizations and/or pre-determinations that may be 2437 * relevant to the Task.) 2438 */ 2439 protected List<Resource> insuranceTarget; 2440 2441 /** 2442 * Free-text information captured about the task as it progresses. 2443 */ 2444 @Child(name = "note", type = { 2445 Annotation.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2446 @Description(shortDefinition = "Comments made about the task", formalDefinition = "Free-text information captured about the task as it progresses.") 2447 protected List<Annotation> note; 2448 2449 /** 2450 * Links to Provenance records for past versions of this Task that identify key 2451 * state transitions or updates that are likely to be relevant to a user looking 2452 * at the current version of the task. 2453 */ 2454 @Child(name = "relevantHistory", type = { 2455 Provenance.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2456 @Description(shortDefinition = "Key events in history of the Task", formalDefinition = "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.") 2457 protected List<Reference> relevantHistory; 2458 /** 2459 * The actual objects that are the target of the reference (Links to Provenance 2460 * records for past versions of this Task that identify key state transitions or 2461 * updates that are likely to be relevant to a user looking at the current 2462 * version of the task.) 2463 */ 2464 protected List<Provenance> relevantHistoryTarget; 2465 2466 /** 2467 * If the Task.focus is a request resource and the task is seeking fulfillment 2468 * (i.e. is asking for the request to be actioned), this element identifies any 2469 * limitations on what parts of the referenced request should be actioned. 2470 */ 2471 @Child(name = "restriction", type = {}, order = 28, min = 0, max = 1, modifier = false, summary = false) 2472 @Description(shortDefinition = "Constraints on fulfillment tasks", formalDefinition = "If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.") 2473 protected TaskRestrictionComponent restriction; 2474 2475 /** 2476 * Additional information that may be needed in the execution of the task. 2477 */ 2478 @Child(name = "input", type = {}, order = 29, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2479 @Description(shortDefinition = "Information used to perform task", formalDefinition = "Additional information that may be needed in the execution of the task.") 2480 protected List<ParameterComponent> input; 2481 2482 /** 2483 * Outputs produced by the Task. 2484 */ 2485 @Child(name = "output", type = {}, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2486 @Description(shortDefinition = "Information produced as part of task", formalDefinition = "Outputs produced by the Task.") 2487 protected List<TaskOutputComponent> output; 2488 2489 private static final long serialVersionUID = -765029272L; 2490 2491 /** 2492 * Constructor 2493 */ 2494 public Task() { 2495 super(); 2496 } 2497 2498 /** 2499 * Constructor 2500 */ 2501 public Task(Enumeration<TaskStatus> status, Enumeration<TaskIntent> intent) { 2502 super(); 2503 this.status = status; 2504 this.intent = intent; 2505 } 2506 2507 /** 2508 * @return {@link #identifier} (The business identifier for this task.) 2509 */ 2510 public List<Identifier> getIdentifier() { 2511 if (this.identifier == null) 2512 this.identifier = new ArrayList<Identifier>(); 2513 return this.identifier; 2514 } 2515 2516 /** 2517 * @return Returns a reference to <code>this</code> for easy method chaining 2518 */ 2519 public Task setIdentifier(List<Identifier> theIdentifier) { 2520 this.identifier = theIdentifier; 2521 return this; 2522 } 2523 2524 public boolean hasIdentifier() { 2525 if (this.identifier == null) 2526 return false; 2527 for (Identifier item : this.identifier) 2528 if (!item.isEmpty()) 2529 return true; 2530 return false; 2531 } 2532 2533 public Identifier addIdentifier() { // 3 2534 Identifier t = new Identifier(); 2535 if (this.identifier == null) 2536 this.identifier = new ArrayList<Identifier>(); 2537 this.identifier.add(t); 2538 return t; 2539 } 2540 2541 public Task addIdentifier(Identifier t) { // 3 2542 if (t == null) 2543 return this; 2544 if (this.identifier == null) 2545 this.identifier = new ArrayList<Identifier>(); 2546 this.identifier.add(t); 2547 return this; 2548 } 2549 2550 /** 2551 * @return The first repetition of repeating field {@link #identifier}, creating 2552 * it if it does not already exist 2553 */ 2554 public Identifier getIdentifierFirstRep() { 2555 if (getIdentifier().isEmpty()) { 2556 addIdentifier(); 2557 } 2558 return getIdentifier().get(0); 2559 } 2560 2561 /** 2562 * @return {@link #instantiatesCanonical} (The URL pointing to a *FHIR*-defined 2563 * protocol, guideline, orderset or other definition that is adhered to 2564 * in whole or in part by this Task.). This is the underlying object 2565 * with id, value and extensions. The accessor 2566 * "getInstantiatesCanonical" gives direct access to the value 2567 */ 2568 public CanonicalType getInstantiatesCanonicalElement() { 2569 if (this.instantiatesCanonical == null) 2570 if (Configuration.errorOnAutoCreate()) 2571 throw new Error("Attempt to auto-create Task.instantiatesCanonical"); 2572 else if (Configuration.doAutoCreate()) 2573 this.instantiatesCanonical = new CanonicalType(); // bb 2574 return this.instantiatesCanonical; 2575 } 2576 2577 public boolean hasInstantiatesCanonicalElement() { 2578 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 2579 } 2580 2581 public boolean hasInstantiatesCanonical() { 2582 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 2583 } 2584 2585 /** 2586 * @param value {@link #instantiatesCanonical} (The URL pointing to a 2587 * *FHIR*-defined protocol, guideline, orderset or other definition 2588 * that is adhered to in whole or in part by this Task.). This is 2589 * the underlying object with id, value and extensions. The 2590 * accessor "getInstantiatesCanonical" gives direct access to the 2591 * value 2592 */ 2593 public Task setInstantiatesCanonicalElement(CanonicalType value) { 2594 this.instantiatesCanonical = value; 2595 return this; 2596 } 2597 2598 /** 2599 * @return The URL pointing to a *FHIR*-defined protocol, guideline, orderset or 2600 * other definition that is adhered to in whole or in part by this Task. 2601 */ 2602 public String getInstantiatesCanonical() { 2603 return this.instantiatesCanonical == null ? null : this.instantiatesCanonical.getValue(); 2604 } 2605 2606 /** 2607 * @param value The URL pointing to a *FHIR*-defined protocol, guideline, 2608 * orderset or other definition that is adhered to in whole or in 2609 * part by this Task. 2610 */ 2611 public Task setInstantiatesCanonical(String value) { 2612 if (Utilities.noString(value)) 2613 this.instantiatesCanonical = null; 2614 else { 2615 if (this.instantiatesCanonical == null) 2616 this.instantiatesCanonical = new CanonicalType(); 2617 this.instantiatesCanonical.setValue(value); 2618 } 2619 return this; 2620 } 2621 2622 /** 2623 * @return {@link #instantiatesUri} (The URL pointing to an *externally* 2624 * maintained protocol, guideline, orderset or other definition that is 2625 * adhered to in whole or in part by this Task.). This is the underlying 2626 * object with id, value and extensions. The accessor 2627 * "getInstantiatesUri" gives direct access to the value 2628 */ 2629 public UriType getInstantiatesUriElement() { 2630 if (this.instantiatesUri == null) 2631 if (Configuration.errorOnAutoCreate()) 2632 throw new Error("Attempt to auto-create Task.instantiatesUri"); 2633 else if (Configuration.doAutoCreate()) 2634 this.instantiatesUri = new UriType(); // bb 2635 return this.instantiatesUri; 2636 } 2637 2638 public boolean hasInstantiatesUriElement() { 2639 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 2640 } 2641 2642 public boolean hasInstantiatesUri() { 2643 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 2644 } 2645 2646 /** 2647 * @param value {@link #instantiatesUri} (The URL pointing to an *externally* 2648 * maintained protocol, guideline, orderset or other definition 2649 * that is adhered to in whole or in part by this Task.). This is 2650 * the underlying object with id, value and extensions. The 2651 * accessor "getInstantiatesUri" gives direct access to the value 2652 */ 2653 public Task setInstantiatesUriElement(UriType value) { 2654 this.instantiatesUri = value; 2655 return this; 2656 } 2657 2658 /** 2659 * @return The URL pointing to an *externally* maintained protocol, guideline, 2660 * orderset or other definition that is adhered to in whole or in part 2661 * by this Task. 2662 */ 2663 public String getInstantiatesUri() { 2664 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 2665 } 2666 2667 /** 2668 * @param value The URL pointing to an *externally* maintained protocol, 2669 * guideline, orderset or other definition that is adhered to in 2670 * whole or in part by this Task. 2671 */ 2672 public Task setInstantiatesUri(String value) { 2673 if (Utilities.noString(value)) 2674 this.instantiatesUri = null; 2675 else { 2676 if (this.instantiatesUri == null) 2677 this.instantiatesUri = new UriType(); 2678 this.instantiatesUri.setValue(value); 2679 } 2680 return this; 2681 } 2682 2683 /** 2684 * @return {@link #basedOn} (BasedOn refers to a higher-level authorization that 2685 * triggered the creation of the task. It references a "request" 2686 * resource such as a ServiceRequest, MedicationRequest, ServiceRequest, 2687 * CarePlan, etc. which is distinct from the "request" resource the task 2688 * is seeking to fulfill. This latter resource is referenced by FocusOn. 2689 * For example, based on a ServiceRequest (= BasedOn), a task is created 2690 * to fulfill a procedureRequest ( = FocusOn ) to collect a specimen 2691 * from a patient.) 2692 */ 2693 public List<Reference> getBasedOn() { 2694 if (this.basedOn == null) 2695 this.basedOn = new ArrayList<Reference>(); 2696 return this.basedOn; 2697 } 2698 2699 /** 2700 * @return Returns a reference to <code>this</code> for easy method chaining 2701 */ 2702 public Task setBasedOn(List<Reference> theBasedOn) { 2703 this.basedOn = theBasedOn; 2704 return this; 2705 } 2706 2707 public boolean hasBasedOn() { 2708 if (this.basedOn == null) 2709 return false; 2710 for (Reference item : this.basedOn) 2711 if (!item.isEmpty()) 2712 return true; 2713 return false; 2714 } 2715 2716 public Reference addBasedOn() { // 3 2717 Reference t = new Reference(); 2718 if (this.basedOn == null) 2719 this.basedOn = new ArrayList<Reference>(); 2720 this.basedOn.add(t); 2721 return t; 2722 } 2723 2724 public Task addBasedOn(Reference t) { // 3 2725 if (t == null) 2726 return this; 2727 if (this.basedOn == null) 2728 this.basedOn = new ArrayList<Reference>(); 2729 this.basedOn.add(t); 2730 return this; 2731 } 2732 2733 /** 2734 * @return The first repetition of repeating field {@link #basedOn}, creating it 2735 * if it does not already exist 2736 */ 2737 public Reference getBasedOnFirstRep() { 2738 if (getBasedOn().isEmpty()) { 2739 addBasedOn(); 2740 } 2741 return getBasedOn().get(0); 2742 } 2743 2744 /** 2745 * @return {@link #groupIdentifier} (An identifier that links together multiple 2746 * tasks and other requests that were created in the same context.) 2747 */ 2748 public Identifier getGroupIdentifier() { 2749 if (this.groupIdentifier == null) 2750 if (Configuration.errorOnAutoCreate()) 2751 throw new Error("Attempt to auto-create Task.groupIdentifier"); 2752 else if (Configuration.doAutoCreate()) 2753 this.groupIdentifier = new Identifier(); // cc 2754 return this.groupIdentifier; 2755 } 2756 2757 public boolean hasGroupIdentifier() { 2758 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 2759 } 2760 2761 /** 2762 * @param value {@link #groupIdentifier} (An identifier that links together 2763 * multiple tasks and other requests that were created in the same 2764 * context.) 2765 */ 2766 public Task setGroupIdentifier(Identifier value) { 2767 this.groupIdentifier = value; 2768 return this; 2769 } 2770 2771 /** 2772 * @return {@link #partOf} (Task that this particular task is part of.) 2773 */ 2774 public List<Reference> getPartOf() { 2775 if (this.partOf == null) 2776 this.partOf = new ArrayList<Reference>(); 2777 return this.partOf; 2778 } 2779 2780 /** 2781 * @return Returns a reference to <code>this</code> for easy method chaining 2782 */ 2783 public Task setPartOf(List<Reference> thePartOf) { 2784 this.partOf = thePartOf; 2785 return this; 2786 } 2787 2788 public boolean hasPartOf() { 2789 if (this.partOf == null) 2790 return false; 2791 for (Reference item : this.partOf) 2792 if (!item.isEmpty()) 2793 return true; 2794 return false; 2795 } 2796 2797 public Reference addPartOf() { // 3 2798 Reference t = new Reference(); 2799 if (this.partOf == null) 2800 this.partOf = new ArrayList<Reference>(); 2801 this.partOf.add(t); 2802 return t; 2803 } 2804 2805 public Task addPartOf(Reference t) { // 3 2806 if (t == null) 2807 return this; 2808 if (this.partOf == null) 2809 this.partOf = new ArrayList<Reference>(); 2810 this.partOf.add(t); 2811 return this; 2812 } 2813 2814 /** 2815 * @return The first repetition of repeating field {@link #partOf}, creating it 2816 * if it does not already exist 2817 */ 2818 public Reference getPartOfFirstRep() { 2819 if (getPartOf().isEmpty()) { 2820 addPartOf(); 2821 } 2822 return getPartOf().get(0); 2823 } 2824 2825 /** 2826 * @return {@link #status} (The current status of the task.). This is the 2827 * underlying object with id, value and extensions. The accessor 2828 * "getStatus" gives direct access to the value 2829 */ 2830 public Enumeration<TaskStatus> getStatusElement() { 2831 if (this.status == null) 2832 if (Configuration.errorOnAutoCreate()) 2833 throw new Error("Attempt to auto-create Task.status"); 2834 else if (Configuration.doAutoCreate()) 2835 this.status = new Enumeration<TaskStatus>(new TaskStatusEnumFactory()); // bb 2836 return this.status; 2837 } 2838 2839 public boolean hasStatusElement() { 2840 return this.status != null && !this.status.isEmpty(); 2841 } 2842 2843 public boolean hasStatus() { 2844 return this.status != null && !this.status.isEmpty(); 2845 } 2846 2847 /** 2848 * @param value {@link #status} (The current status of the task.). This is the 2849 * underlying object with id, value and extensions. The accessor 2850 * "getStatus" gives direct access to the value 2851 */ 2852 public Task setStatusElement(Enumeration<TaskStatus> value) { 2853 this.status = value; 2854 return this; 2855 } 2856 2857 /** 2858 * @return The current status of the task. 2859 */ 2860 public TaskStatus getStatus() { 2861 return this.status == null ? null : this.status.getValue(); 2862 } 2863 2864 /** 2865 * @param value The current status of the task. 2866 */ 2867 public Task setStatus(TaskStatus value) { 2868 if (this.status == null) 2869 this.status = new Enumeration<TaskStatus>(new TaskStatusEnumFactory()); 2870 this.status.setValue(value); 2871 return this; 2872 } 2873 2874 /** 2875 * @return {@link #statusReason} (An explanation as to why this task is held, 2876 * failed, was refused, etc.) 2877 */ 2878 public CodeableConcept getStatusReason() { 2879 if (this.statusReason == null) 2880 if (Configuration.errorOnAutoCreate()) 2881 throw new Error("Attempt to auto-create Task.statusReason"); 2882 else if (Configuration.doAutoCreate()) 2883 this.statusReason = new CodeableConcept(); // cc 2884 return this.statusReason; 2885 } 2886 2887 public boolean hasStatusReason() { 2888 return this.statusReason != null && !this.statusReason.isEmpty(); 2889 } 2890 2891 /** 2892 * @param value {@link #statusReason} (An explanation as to why this task is 2893 * held, failed, was refused, etc.) 2894 */ 2895 public Task setStatusReason(CodeableConcept value) { 2896 this.statusReason = value; 2897 return this; 2898 } 2899 2900 /** 2901 * @return {@link #businessStatus} (Contains business-specific nuances of the 2902 * business state.) 2903 */ 2904 public CodeableConcept getBusinessStatus() { 2905 if (this.businessStatus == null) 2906 if (Configuration.errorOnAutoCreate()) 2907 throw new Error("Attempt to auto-create Task.businessStatus"); 2908 else if (Configuration.doAutoCreate()) 2909 this.businessStatus = new CodeableConcept(); // cc 2910 return this.businessStatus; 2911 } 2912 2913 public boolean hasBusinessStatus() { 2914 return this.businessStatus != null && !this.businessStatus.isEmpty(); 2915 } 2916 2917 /** 2918 * @param value {@link #businessStatus} (Contains business-specific nuances of 2919 * the business state.) 2920 */ 2921 public Task setBusinessStatus(CodeableConcept value) { 2922 this.businessStatus = value; 2923 return this; 2924 } 2925 2926 /** 2927 * @return {@link #intent} (Indicates the "level" of actionability associated 2928 * with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an 2929 * actionable task, etc.). This is the underlying object with id, value 2930 * and extensions. The accessor "getIntent" gives direct access to the 2931 * value 2932 */ 2933 public Enumeration<TaskIntent> getIntentElement() { 2934 if (this.intent == null) 2935 if (Configuration.errorOnAutoCreate()) 2936 throw new Error("Attempt to auto-create Task.intent"); 2937 else if (Configuration.doAutoCreate()) 2938 this.intent = new Enumeration<TaskIntent>(new TaskIntentEnumFactory()); // bb 2939 return this.intent; 2940 } 2941 2942 public boolean hasIntentElement() { 2943 return this.intent != null && !this.intent.isEmpty(); 2944 } 2945 2946 public boolean hasIntent() { 2947 return this.intent != null && !this.intent.isEmpty(); 2948 } 2949 2950 /** 2951 * @param value {@link #intent} (Indicates the "level" of actionability 2952 * associated with the Task, i.e. i+R[9]Cs this a proposed task, a 2953 * planned task, an actionable task, etc.). This is the underlying 2954 * object with id, value and extensions. The accessor "getIntent" 2955 * gives direct access to the value 2956 */ 2957 public Task setIntentElement(Enumeration<TaskIntent> value) { 2958 this.intent = value; 2959 return this; 2960 } 2961 2962 /** 2963 * @return Indicates the "level" of actionability associated with the Task, i.e. 2964 * i+R[9]Cs this a proposed task, a planned task, an actionable task, 2965 * etc. 2966 */ 2967 public TaskIntent getIntent() { 2968 return this.intent == null ? null : this.intent.getValue(); 2969 } 2970 2971 /** 2972 * @param value Indicates the "level" of actionability associated with the Task, 2973 * i.e. i+R[9]Cs this a proposed task, a planned task, an 2974 * actionable task, etc. 2975 */ 2976 public Task setIntent(TaskIntent value) { 2977 if (this.intent == null) 2978 this.intent = new Enumeration<TaskIntent>(new TaskIntentEnumFactory()); 2979 this.intent.setValue(value); 2980 return this; 2981 } 2982 2983 /** 2984 * @return {@link #priority} (Indicates how quickly the Task should be addressed 2985 * with respect to other requests.). This is the underlying object with 2986 * id, value and extensions. The accessor "getPriority" gives direct 2987 * access to the value 2988 */ 2989 public Enumeration<TaskPriority> getPriorityElement() { 2990 if (this.priority == null) 2991 if (Configuration.errorOnAutoCreate()) 2992 throw new Error("Attempt to auto-create Task.priority"); 2993 else if (Configuration.doAutoCreate()) 2994 this.priority = new Enumeration<TaskPriority>(new TaskPriorityEnumFactory()); // bb 2995 return this.priority; 2996 } 2997 2998 public boolean hasPriorityElement() { 2999 return this.priority != null && !this.priority.isEmpty(); 3000 } 3001 3002 public boolean hasPriority() { 3003 return this.priority != null && !this.priority.isEmpty(); 3004 } 3005 3006 /** 3007 * @param value {@link #priority} (Indicates how quickly the Task should be 3008 * addressed with respect to other requests.). This is the 3009 * underlying object with id, value and extensions. The accessor 3010 * "getPriority" gives direct access to the value 3011 */ 3012 public Task setPriorityElement(Enumeration<TaskPriority> value) { 3013 this.priority = value; 3014 return this; 3015 } 3016 3017 /** 3018 * @return Indicates how quickly the Task should be addressed with respect to 3019 * other requests. 3020 */ 3021 public TaskPriority getPriority() { 3022 return this.priority == null ? null : this.priority.getValue(); 3023 } 3024 3025 /** 3026 * @param value Indicates how quickly the Task should be addressed with respect 3027 * to other requests. 3028 */ 3029 public Task setPriority(TaskPriority value) { 3030 if (value == null) 3031 this.priority = null; 3032 else { 3033 if (this.priority == null) 3034 this.priority = new Enumeration<TaskPriority>(new TaskPriorityEnumFactory()); 3035 this.priority.setValue(value); 3036 } 3037 return this; 3038 } 3039 3040 /** 3041 * @return {@link #code} (A name or code (or both) briefly describing what the 3042 * task involves.) 3043 */ 3044 public CodeableConcept getCode() { 3045 if (this.code == null) 3046 if (Configuration.errorOnAutoCreate()) 3047 throw new Error("Attempt to auto-create Task.code"); 3048 else if (Configuration.doAutoCreate()) 3049 this.code = new CodeableConcept(); // cc 3050 return this.code; 3051 } 3052 3053 public boolean hasCode() { 3054 return this.code != null && !this.code.isEmpty(); 3055 } 3056 3057 /** 3058 * @param value {@link #code} (A name or code (or both) briefly describing what 3059 * the task involves.) 3060 */ 3061 public Task setCode(CodeableConcept value) { 3062 this.code = value; 3063 return this; 3064 } 3065 3066 /** 3067 * @return {@link #description} (A free-text description of what is to be 3068 * performed.). This is the underlying object with id, value and 3069 * extensions. The accessor "getDescription" gives direct access to the 3070 * value 3071 */ 3072 public StringType getDescriptionElement() { 3073 if (this.description == null) 3074 if (Configuration.errorOnAutoCreate()) 3075 throw new Error("Attempt to auto-create Task.description"); 3076 else if (Configuration.doAutoCreate()) 3077 this.description = new StringType(); // bb 3078 return this.description; 3079 } 3080 3081 public boolean hasDescriptionElement() { 3082 return this.description != null && !this.description.isEmpty(); 3083 } 3084 3085 public boolean hasDescription() { 3086 return this.description != null && !this.description.isEmpty(); 3087 } 3088 3089 /** 3090 * @param value {@link #description} (A free-text description of what is to be 3091 * performed.). This is the underlying object with id, value and 3092 * extensions. The accessor "getDescription" gives direct access to 3093 * the value 3094 */ 3095 public Task setDescriptionElement(StringType value) { 3096 this.description = value; 3097 return this; 3098 } 3099 3100 /** 3101 * @return A free-text description of what is to be performed. 3102 */ 3103 public String getDescription() { 3104 return this.description == null ? null : this.description.getValue(); 3105 } 3106 3107 /** 3108 * @param value A free-text description of what is to be performed. 3109 */ 3110 public Task setDescription(String value) { 3111 if (Utilities.noString(value)) 3112 this.description = null; 3113 else { 3114 if (this.description == null) 3115 this.description = new StringType(); 3116 this.description.setValue(value); 3117 } 3118 return this; 3119 } 3120 3121 /** 3122 * @return {@link #focus} (The request being actioned or the resource being 3123 * manipulated by this task.) 3124 */ 3125 public Reference getFocus() { 3126 if (this.focus == null) 3127 if (Configuration.errorOnAutoCreate()) 3128 throw new Error("Attempt to auto-create Task.focus"); 3129 else if (Configuration.doAutoCreate()) 3130 this.focus = new Reference(); // cc 3131 return this.focus; 3132 } 3133 3134 public boolean hasFocus() { 3135 return this.focus != null && !this.focus.isEmpty(); 3136 } 3137 3138 /** 3139 * @param value {@link #focus} (The request being actioned or the resource being 3140 * manipulated by this task.) 3141 */ 3142 public Task setFocus(Reference value) { 3143 this.focus = value; 3144 return this; 3145 } 3146 3147 /** 3148 * @return {@link #focus} The actual object that is the target of the reference. 3149 * The reference library doesn't populate this, but you can use it to 3150 * hold the resource if you resolve it. (The request being actioned or 3151 * the resource being manipulated by this task.) 3152 */ 3153 public Resource getFocusTarget() { 3154 return this.focusTarget; 3155 } 3156 3157 /** 3158 * @param value {@link #focus} The actual object that is the target of the 3159 * reference. The reference library doesn't use these, but you can 3160 * use it to hold the resource if you resolve it. (The request 3161 * being actioned or the resource being manipulated by this task.) 3162 */ 3163 public Task setFocusTarget(Resource value) { 3164 this.focusTarget = value; 3165 return this; 3166 } 3167 3168 /** 3169 * @return {@link #for_} (The entity who benefits from the performance of the 3170 * service specified in the task (e.g., the patient).) 3171 */ 3172 public Reference getFor() { 3173 if (this.for_ == null) 3174 if (Configuration.errorOnAutoCreate()) 3175 throw new Error("Attempt to auto-create Task.for_"); 3176 else if (Configuration.doAutoCreate()) 3177 this.for_ = new Reference(); // cc 3178 return this.for_; 3179 } 3180 3181 public boolean hasFor() { 3182 return this.for_ != null && !this.for_.isEmpty(); 3183 } 3184 3185 /** 3186 * @param value {@link #for_} (The entity who benefits from the performance of 3187 * the service specified in the task (e.g., the patient).) 3188 */ 3189 public Task setFor(Reference value) { 3190 this.for_ = value; 3191 return this; 3192 } 3193 3194 /** 3195 * @return {@link #for_} The actual object that is the target of the reference. 3196 * The reference library doesn't populate this, but you can use it to 3197 * hold the resource if you resolve it. (The entity who benefits from 3198 * the performance of the service specified in the task (e.g., the 3199 * patient).) 3200 */ 3201 public Resource getForTarget() { 3202 return this.for_Target; 3203 } 3204 3205 /** 3206 * @param value {@link #for_} The actual object that is the target of the 3207 * reference. The reference library doesn't use these, but you can 3208 * use it to hold the resource if you resolve it. (The entity who 3209 * benefits from the performance of the service specified in the 3210 * task (e.g., the patient).) 3211 */ 3212 public Task setForTarget(Resource value) { 3213 this.for_Target = value; 3214 return this; 3215 } 3216 3217 /** 3218 * @return {@link #encounter} (The healthcare event (e.g. a patient and 3219 * healthcare provider interaction) during which this task was created.) 3220 */ 3221 public Reference getEncounter() { 3222 if (this.encounter == null) 3223 if (Configuration.errorOnAutoCreate()) 3224 throw new Error("Attempt to auto-create Task.encounter"); 3225 else if (Configuration.doAutoCreate()) 3226 this.encounter = new Reference(); // cc 3227 return this.encounter; 3228 } 3229 3230 public boolean hasEncounter() { 3231 return this.encounter != null && !this.encounter.isEmpty(); 3232 } 3233 3234 /** 3235 * @param value {@link #encounter} (The healthcare event (e.g. a patient and 3236 * healthcare provider interaction) during which this task was 3237 * created.) 3238 */ 3239 public Task setEncounter(Reference value) { 3240 this.encounter = value; 3241 return this; 3242 } 3243 3244 /** 3245 * @return {@link #encounter} The actual object that is the target of the 3246 * reference. The reference library doesn't populate this, but you can 3247 * use it to hold the resource if you resolve it. (The healthcare event 3248 * (e.g. a patient and healthcare provider interaction) during which 3249 * this task was created.) 3250 */ 3251 public Encounter getEncounterTarget() { 3252 if (this.encounterTarget == null) 3253 if (Configuration.errorOnAutoCreate()) 3254 throw new Error("Attempt to auto-create Task.encounter"); 3255 else if (Configuration.doAutoCreate()) 3256 this.encounterTarget = new Encounter(); // aa 3257 return this.encounterTarget; 3258 } 3259 3260 /** 3261 * @param value {@link #encounter} The actual object that is the target of the 3262 * reference. The reference library doesn't use these, but you can 3263 * use it to hold the resource if you resolve it. (The healthcare 3264 * event (e.g. a patient and healthcare provider interaction) 3265 * during which this task was created.) 3266 */ 3267 public Task setEncounterTarget(Encounter value) { 3268 this.encounterTarget = value; 3269 return this; 3270 } 3271 3272 /** 3273 * @return {@link #executionPeriod} (Identifies the time action was first taken 3274 * against the task (start) and/or the time final action was taken 3275 * against the task prior to marking it as completed (end).) 3276 */ 3277 public Period getExecutionPeriod() { 3278 if (this.executionPeriod == null) 3279 if (Configuration.errorOnAutoCreate()) 3280 throw new Error("Attempt to auto-create Task.executionPeriod"); 3281 else if (Configuration.doAutoCreate()) 3282 this.executionPeriod = new Period(); // cc 3283 return this.executionPeriod; 3284 } 3285 3286 public boolean hasExecutionPeriod() { 3287 return this.executionPeriod != null && !this.executionPeriod.isEmpty(); 3288 } 3289 3290 /** 3291 * @param value {@link #executionPeriod} (Identifies the time action was first 3292 * taken against the task (start) and/or the time final action was 3293 * taken against the task prior to marking it as completed (end).) 3294 */ 3295 public Task setExecutionPeriod(Period value) { 3296 this.executionPeriod = value; 3297 return this; 3298 } 3299 3300 /** 3301 * @return {@link #authoredOn} (The date and time this task was created.). This 3302 * is the underlying object with id, value and extensions. The accessor 3303 * "getAuthoredOn" gives direct access to the value 3304 */ 3305 public DateTimeType getAuthoredOnElement() { 3306 if (this.authoredOn == null) 3307 if (Configuration.errorOnAutoCreate()) 3308 throw new Error("Attempt to auto-create Task.authoredOn"); 3309 else if (Configuration.doAutoCreate()) 3310 this.authoredOn = new DateTimeType(); // bb 3311 return this.authoredOn; 3312 } 3313 3314 public boolean hasAuthoredOnElement() { 3315 return this.authoredOn != null && !this.authoredOn.isEmpty(); 3316 } 3317 3318 public boolean hasAuthoredOn() { 3319 return this.authoredOn != null && !this.authoredOn.isEmpty(); 3320 } 3321 3322 /** 3323 * @param value {@link #authoredOn} (The date and time this task was created.). 3324 * This is the underlying object with id, value and extensions. The 3325 * accessor "getAuthoredOn" gives direct access to the value 3326 */ 3327 public Task setAuthoredOnElement(DateTimeType value) { 3328 this.authoredOn = value; 3329 return this; 3330 } 3331 3332 /** 3333 * @return The date and time this task was created. 3334 */ 3335 public Date getAuthoredOn() { 3336 return this.authoredOn == null ? null : this.authoredOn.getValue(); 3337 } 3338 3339 /** 3340 * @param value The date and time this task was created. 3341 */ 3342 public Task setAuthoredOn(Date value) { 3343 if (value == null) 3344 this.authoredOn = null; 3345 else { 3346 if (this.authoredOn == null) 3347 this.authoredOn = new DateTimeType(); 3348 this.authoredOn.setValue(value); 3349 } 3350 return this; 3351 } 3352 3353 /** 3354 * @return {@link #lastModified} (The date and time of last modification to this 3355 * task.). This is the underlying object with id, value and extensions. 3356 * The accessor "getLastModified" gives direct access to the value 3357 */ 3358 public DateTimeType getLastModifiedElement() { 3359 if (this.lastModified == null) 3360 if (Configuration.errorOnAutoCreate()) 3361 throw new Error("Attempt to auto-create Task.lastModified"); 3362 else if (Configuration.doAutoCreate()) 3363 this.lastModified = new DateTimeType(); // bb 3364 return this.lastModified; 3365 } 3366 3367 public boolean hasLastModifiedElement() { 3368 return this.lastModified != null && !this.lastModified.isEmpty(); 3369 } 3370 3371 public boolean hasLastModified() { 3372 return this.lastModified != null && !this.lastModified.isEmpty(); 3373 } 3374 3375 /** 3376 * @param value {@link #lastModified} (The date and time of last modification to 3377 * this task.). This is the underlying object with id, value and 3378 * extensions. The accessor "getLastModified" gives direct access 3379 * to the value 3380 */ 3381 public Task setLastModifiedElement(DateTimeType value) { 3382 this.lastModified = value; 3383 return this; 3384 } 3385 3386 /** 3387 * @return The date and time of last modification to this task. 3388 */ 3389 public Date getLastModified() { 3390 return this.lastModified == null ? null : this.lastModified.getValue(); 3391 } 3392 3393 /** 3394 * @param value The date and time of last modification to this task. 3395 */ 3396 public Task setLastModified(Date value) { 3397 if (value == null) 3398 this.lastModified = null; 3399 else { 3400 if (this.lastModified == null) 3401 this.lastModified = new DateTimeType(); 3402 this.lastModified.setValue(value); 3403 } 3404 return this; 3405 } 3406 3407 /** 3408 * @return {@link #requester} (The creator of the task.) 3409 */ 3410 public Reference getRequester() { 3411 if (this.requester == null) 3412 if (Configuration.errorOnAutoCreate()) 3413 throw new Error("Attempt to auto-create Task.requester"); 3414 else if (Configuration.doAutoCreate()) 3415 this.requester = new Reference(); // cc 3416 return this.requester; 3417 } 3418 3419 public boolean hasRequester() { 3420 return this.requester != null && !this.requester.isEmpty(); 3421 } 3422 3423 /** 3424 * @param value {@link #requester} (The creator of the task.) 3425 */ 3426 public Task setRequester(Reference value) { 3427 this.requester = value; 3428 return this; 3429 } 3430 3431 /** 3432 * @return {@link #requester} The actual object that is the target of the 3433 * reference. The reference library doesn't populate this, but you can 3434 * use it to hold the resource if you resolve it. (The creator of the 3435 * task.) 3436 */ 3437 public Resource getRequesterTarget() { 3438 return this.requesterTarget; 3439 } 3440 3441 /** 3442 * @param value {@link #requester} The actual object that is the target of the 3443 * reference. The reference library doesn't use these, but you can 3444 * use it to hold the resource if you resolve it. (The creator of 3445 * the task.) 3446 */ 3447 public Task setRequesterTarget(Resource value) { 3448 this.requesterTarget = value; 3449 return this; 3450 } 3451 3452 /** 3453 * @return {@link #performerType} (The kind of participant that should perform 3454 * the task.) 3455 */ 3456 public List<CodeableConcept> getPerformerType() { 3457 if (this.performerType == null) 3458 this.performerType = new ArrayList<CodeableConcept>(); 3459 return this.performerType; 3460 } 3461 3462 /** 3463 * @return Returns a reference to <code>this</code> for easy method chaining 3464 */ 3465 public Task setPerformerType(List<CodeableConcept> thePerformerType) { 3466 this.performerType = thePerformerType; 3467 return this; 3468 } 3469 3470 public boolean hasPerformerType() { 3471 if (this.performerType == null) 3472 return false; 3473 for (CodeableConcept item : this.performerType) 3474 if (!item.isEmpty()) 3475 return true; 3476 return false; 3477 } 3478 3479 public CodeableConcept addPerformerType() { // 3 3480 CodeableConcept t = new CodeableConcept(); 3481 if (this.performerType == null) 3482 this.performerType = new ArrayList<CodeableConcept>(); 3483 this.performerType.add(t); 3484 return t; 3485 } 3486 3487 public Task addPerformerType(CodeableConcept t) { // 3 3488 if (t == null) 3489 return this; 3490 if (this.performerType == null) 3491 this.performerType = new ArrayList<CodeableConcept>(); 3492 this.performerType.add(t); 3493 return this; 3494 } 3495 3496 /** 3497 * @return The first repetition of repeating field {@link #performerType}, 3498 * creating it if it does not already exist 3499 */ 3500 public CodeableConcept getPerformerTypeFirstRep() { 3501 if (getPerformerType().isEmpty()) { 3502 addPerformerType(); 3503 } 3504 return getPerformerType().get(0); 3505 } 3506 3507 /** 3508 * @return {@link #owner} (Individual organization or Device currently 3509 * responsible for task execution.) 3510 */ 3511 public Reference getOwner() { 3512 if (this.owner == null) 3513 if (Configuration.errorOnAutoCreate()) 3514 throw new Error("Attempt to auto-create Task.owner"); 3515 else if (Configuration.doAutoCreate()) 3516 this.owner = new Reference(); // cc 3517 return this.owner; 3518 } 3519 3520 public boolean hasOwner() { 3521 return this.owner != null && !this.owner.isEmpty(); 3522 } 3523 3524 /** 3525 * @param value {@link #owner} (Individual organization or Device currently 3526 * responsible for task execution.) 3527 */ 3528 public Task setOwner(Reference value) { 3529 this.owner = value; 3530 return this; 3531 } 3532 3533 /** 3534 * @return {@link #owner} The actual object that is the target of the reference. 3535 * The reference library doesn't populate this, but you can use it to 3536 * hold the resource if you resolve it. (Individual organization or 3537 * Device currently responsible for task execution.) 3538 */ 3539 public Resource getOwnerTarget() { 3540 return this.ownerTarget; 3541 } 3542 3543 /** 3544 * @param value {@link #owner} The actual object that is the target of the 3545 * reference. The reference library doesn't use these, but you can 3546 * use it to hold the resource if you resolve it. (Individual 3547 * organization or Device currently responsible for task 3548 * execution.) 3549 */ 3550 public Task setOwnerTarget(Resource value) { 3551 this.ownerTarget = value; 3552 return this; 3553 } 3554 3555 /** 3556 * @return {@link #location} (Principal physical location where the this task is 3557 * performed.) 3558 */ 3559 public Reference getLocation() { 3560 if (this.location == null) 3561 if (Configuration.errorOnAutoCreate()) 3562 throw new Error("Attempt to auto-create Task.location"); 3563 else if (Configuration.doAutoCreate()) 3564 this.location = new Reference(); // cc 3565 return this.location; 3566 } 3567 3568 public boolean hasLocation() { 3569 return this.location != null && !this.location.isEmpty(); 3570 } 3571 3572 /** 3573 * @param value {@link #location} (Principal physical location where the this 3574 * task is performed.) 3575 */ 3576 public Task setLocation(Reference value) { 3577 this.location = value; 3578 return this; 3579 } 3580 3581 /** 3582 * @return {@link #location} The actual object that is the target of the 3583 * reference. The reference library doesn't populate this, but you can 3584 * use it to hold the resource if you resolve it. (Principal physical 3585 * location where the this task is performed.) 3586 */ 3587 public Location getLocationTarget() { 3588 if (this.locationTarget == null) 3589 if (Configuration.errorOnAutoCreate()) 3590 throw new Error("Attempt to auto-create Task.location"); 3591 else if (Configuration.doAutoCreate()) 3592 this.locationTarget = new Location(); // aa 3593 return this.locationTarget; 3594 } 3595 3596 /** 3597 * @param value {@link #location} The actual object that is the target of the 3598 * reference. The reference library doesn't use these, but you can 3599 * use it to hold the resource if you resolve it. (Principal 3600 * physical location where the this task is performed.) 3601 */ 3602 public Task setLocationTarget(Location value) { 3603 this.locationTarget = value; 3604 return this; 3605 } 3606 3607 /** 3608 * @return {@link #reasonCode} (A description or code indicating why this task 3609 * needs to be performed.) 3610 */ 3611 public CodeableConcept getReasonCode() { 3612 if (this.reasonCode == null) 3613 if (Configuration.errorOnAutoCreate()) 3614 throw new Error("Attempt to auto-create Task.reasonCode"); 3615 else if (Configuration.doAutoCreate()) 3616 this.reasonCode = new CodeableConcept(); // cc 3617 return this.reasonCode; 3618 } 3619 3620 public boolean hasReasonCode() { 3621 return this.reasonCode != null && !this.reasonCode.isEmpty(); 3622 } 3623 3624 /** 3625 * @param value {@link #reasonCode} (A description or code indicating why this 3626 * task needs to be performed.) 3627 */ 3628 public Task setReasonCode(CodeableConcept value) { 3629 this.reasonCode = value; 3630 return this; 3631 } 3632 3633 /** 3634 * @return {@link #reasonReference} (A resource reference indicating why this 3635 * task needs to be performed.) 3636 */ 3637 public Reference getReasonReference() { 3638 if (this.reasonReference == null) 3639 if (Configuration.errorOnAutoCreate()) 3640 throw new Error("Attempt to auto-create Task.reasonReference"); 3641 else if (Configuration.doAutoCreate()) 3642 this.reasonReference = new Reference(); // cc 3643 return this.reasonReference; 3644 } 3645 3646 public boolean hasReasonReference() { 3647 return this.reasonReference != null && !this.reasonReference.isEmpty(); 3648 } 3649 3650 /** 3651 * @param value {@link #reasonReference} (A resource reference indicating why 3652 * this task needs to be performed.) 3653 */ 3654 public Task setReasonReference(Reference value) { 3655 this.reasonReference = value; 3656 return this; 3657 } 3658 3659 /** 3660 * @return {@link #reasonReference} The actual object that is the target of the 3661 * reference. The reference library doesn't populate this, but you can 3662 * use it to hold the resource if you resolve it. (A resource reference 3663 * indicating why this task needs to be performed.) 3664 */ 3665 public Resource getReasonReferenceTarget() { 3666 return this.reasonReferenceTarget; 3667 } 3668 3669 /** 3670 * @param value {@link #reasonReference} The actual object that is the target of 3671 * the reference. The reference library doesn't use these, but you 3672 * can use it to hold the resource if you resolve it. (A resource 3673 * reference indicating why this task needs to be performed.) 3674 */ 3675 public Task setReasonReferenceTarget(Resource value) { 3676 this.reasonReferenceTarget = value; 3677 return this; 3678 } 3679 3680 /** 3681 * @return {@link #insurance} (Insurance plans, coverage extensions, 3682 * pre-authorizations and/or pre-determinations that may be relevant to 3683 * the Task.) 3684 */ 3685 public List<Reference> getInsurance() { 3686 if (this.insurance == null) 3687 this.insurance = new ArrayList<Reference>(); 3688 return this.insurance; 3689 } 3690 3691 /** 3692 * @return Returns a reference to <code>this</code> for easy method chaining 3693 */ 3694 public Task setInsurance(List<Reference> theInsurance) { 3695 this.insurance = theInsurance; 3696 return this; 3697 } 3698 3699 public boolean hasInsurance() { 3700 if (this.insurance == null) 3701 return false; 3702 for (Reference item : this.insurance) 3703 if (!item.isEmpty()) 3704 return true; 3705 return false; 3706 } 3707 3708 public Reference addInsurance() { // 3 3709 Reference t = new Reference(); 3710 if (this.insurance == null) 3711 this.insurance = new ArrayList<Reference>(); 3712 this.insurance.add(t); 3713 return t; 3714 } 3715 3716 public Task addInsurance(Reference t) { // 3 3717 if (t == null) 3718 return this; 3719 if (this.insurance == null) 3720 this.insurance = new ArrayList<Reference>(); 3721 this.insurance.add(t); 3722 return this; 3723 } 3724 3725 /** 3726 * @return The first repetition of repeating field {@link #insurance}, creating 3727 * it if it does not already exist 3728 */ 3729 public Reference getInsuranceFirstRep() { 3730 if (getInsurance().isEmpty()) { 3731 addInsurance(); 3732 } 3733 return getInsurance().get(0); 3734 } 3735 3736 /** 3737 * @return {@link #note} (Free-text information captured about the task as it 3738 * progresses.) 3739 */ 3740 public List<Annotation> getNote() { 3741 if (this.note == null) 3742 this.note = new ArrayList<Annotation>(); 3743 return this.note; 3744 } 3745 3746 /** 3747 * @return Returns a reference to <code>this</code> for easy method chaining 3748 */ 3749 public Task setNote(List<Annotation> theNote) { 3750 this.note = theNote; 3751 return this; 3752 } 3753 3754 public boolean hasNote() { 3755 if (this.note == null) 3756 return false; 3757 for (Annotation item : this.note) 3758 if (!item.isEmpty()) 3759 return true; 3760 return false; 3761 } 3762 3763 public Annotation addNote() { // 3 3764 Annotation t = new Annotation(); 3765 if (this.note == null) 3766 this.note = new ArrayList<Annotation>(); 3767 this.note.add(t); 3768 return t; 3769 } 3770 3771 public Task addNote(Annotation t) { // 3 3772 if (t == null) 3773 return this; 3774 if (this.note == null) 3775 this.note = new ArrayList<Annotation>(); 3776 this.note.add(t); 3777 return this; 3778 } 3779 3780 /** 3781 * @return The first repetition of repeating field {@link #note}, creating it if 3782 * it does not already exist 3783 */ 3784 public Annotation getNoteFirstRep() { 3785 if (getNote().isEmpty()) { 3786 addNote(); 3787 } 3788 return getNote().get(0); 3789 } 3790 3791 /** 3792 * @return {@link #relevantHistory} (Links to Provenance records for past 3793 * versions of this Task that identify key state transitions or updates 3794 * that are likely to be relevant to a user looking at the current 3795 * version of the task.) 3796 */ 3797 public List<Reference> getRelevantHistory() { 3798 if (this.relevantHistory == null) 3799 this.relevantHistory = new ArrayList<Reference>(); 3800 return this.relevantHistory; 3801 } 3802 3803 /** 3804 * @return Returns a reference to <code>this</code> for easy method chaining 3805 */ 3806 public Task setRelevantHistory(List<Reference> theRelevantHistory) { 3807 this.relevantHistory = theRelevantHistory; 3808 return this; 3809 } 3810 3811 public boolean hasRelevantHistory() { 3812 if (this.relevantHistory == null) 3813 return false; 3814 for (Reference item : this.relevantHistory) 3815 if (!item.isEmpty()) 3816 return true; 3817 return false; 3818 } 3819 3820 public Reference addRelevantHistory() { // 3 3821 Reference t = new Reference(); 3822 if (this.relevantHistory == null) 3823 this.relevantHistory = new ArrayList<Reference>(); 3824 this.relevantHistory.add(t); 3825 return t; 3826 } 3827 3828 public Task addRelevantHistory(Reference t) { // 3 3829 if (t == null) 3830 return this; 3831 if (this.relevantHistory == null) 3832 this.relevantHistory = new ArrayList<Reference>(); 3833 this.relevantHistory.add(t); 3834 return this; 3835 } 3836 3837 /** 3838 * @return The first repetition of repeating field {@link #relevantHistory}, 3839 * creating it if it does not already exist 3840 */ 3841 public Reference getRelevantHistoryFirstRep() { 3842 if (getRelevantHistory().isEmpty()) { 3843 addRelevantHistory(); 3844 } 3845 return getRelevantHistory().get(0); 3846 } 3847 3848 /** 3849 * @return {@link #restriction} (If the Task.focus is a request resource and the 3850 * task is seeking fulfillment (i.e. is asking for the request to be 3851 * actioned), this element identifies any limitations on what parts of 3852 * the referenced request should be actioned.) 3853 */ 3854 public TaskRestrictionComponent getRestriction() { 3855 if (this.restriction == null) 3856 if (Configuration.errorOnAutoCreate()) 3857 throw new Error("Attempt to auto-create Task.restriction"); 3858 else if (Configuration.doAutoCreate()) 3859 this.restriction = new TaskRestrictionComponent(); // cc 3860 return this.restriction; 3861 } 3862 3863 public boolean hasRestriction() { 3864 return this.restriction != null && !this.restriction.isEmpty(); 3865 } 3866 3867 /** 3868 * @param value {@link #restriction} (If the Task.focus is a request resource 3869 * and the task is seeking fulfillment (i.e. is asking for the 3870 * request to be actioned), this element identifies any limitations 3871 * on what parts of the referenced request should be actioned.) 3872 */ 3873 public Task setRestriction(TaskRestrictionComponent value) { 3874 this.restriction = value; 3875 return this; 3876 } 3877 3878 /** 3879 * @return {@link #input} (Additional information that may be needed in the 3880 * execution of the task.) 3881 */ 3882 public List<ParameterComponent> getInput() { 3883 if (this.input == null) 3884 this.input = new ArrayList<ParameterComponent>(); 3885 return this.input; 3886 } 3887 3888 /** 3889 * @return Returns a reference to <code>this</code> for easy method chaining 3890 */ 3891 public Task setInput(List<ParameterComponent> theInput) { 3892 this.input = theInput; 3893 return this; 3894 } 3895 3896 public boolean hasInput() { 3897 if (this.input == null) 3898 return false; 3899 for (ParameterComponent item : this.input) 3900 if (!item.isEmpty()) 3901 return true; 3902 return false; 3903 } 3904 3905 public ParameterComponent addInput() { // 3 3906 ParameterComponent t = new ParameterComponent(); 3907 if (this.input == null) 3908 this.input = new ArrayList<ParameterComponent>(); 3909 this.input.add(t); 3910 return t; 3911 } 3912 3913 public Task addInput(ParameterComponent t) { // 3 3914 if (t == null) 3915 return this; 3916 if (this.input == null) 3917 this.input = new ArrayList<ParameterComponent>(); 3918 this.input.add(t); 3919 return this; 3920 } 3921 3922 /** 3923 * @return The first repetition of repeating field {@link #input}, creating it 3924 * if it does not already exist 3925 */ 3926 public ParameterComponent getInputFirstRep() { 3927 if (getInput().isEmpty()) { 3928 addInput(); 3929 } 3930 return getInput().get(0); 3931 } 3932 3933 /** 3934 * @return {@link #output} (Outputs produced by the Task.) 3935 */ 3936 public List<TaskOutputComponent> getOutput() { 3937 if (this.output == null) 3938 this.output = new ArrayList<TaskOutputComponent>(); 3939 return this.output; 3940 } 3941 3942 /** 3943 * @return Returns a reference to <code>this</code> for easy method chaining 3944 */ 3945 public Task setOutput(List<TaskOutputComponent> theOutput) { 3946 this.output = theOutput; 3947 return this; 3948 } 3949 3950 public boolean hasOutput() { 3951 if (this.output == null) 3952 return false; 3953 for (TaskOutputComponent item : this.output) 3954 if (!item.isEmpty()) 3955 return true; 3956 return false; 3957 } 3958 3959 public TaskOutputComponent addOutput() { // 3 3960 TaskOutputComponent t = new TaskOutputComponent(); 3961 if (this.output == null) 3962 this.output = new ArrayList<TaskOutputComponent>(); 3963 this.output.add(t); 3964 return t; 3965 } 3966 3967 public Task addOutput(TaskOutputComponent t) { // 3 3968 if (t == null) 3969 return this; 3970 if (this.output == null) 3971 this.output = new ArrayList<TaskOutputComponent>(); 3972 this.output.add(t); 3973 return this; 3974 } 3975 3976 /** 3977 * @return The first repetition of repeating field {@link #output}, creating it 3978 * if it does not already exist 3979 */ 3980 public TaskOutputComponent getOutputFirstRep() { 3981 if (getOutput().isEmpty()) { 3982 addOutput(); 3983 } 3984 return getOutput().get(0); 3985 } 3986 3987 protected void listChildren(List<Property> children) { 3988 super.listChildren(children); 3989 children.add(new Property("identifier", "Identifier", "The business identifier for this task.", 0, 3990 java.lang.Integer.MAX_VALUE, identifier)); 3991 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition)", 3992 "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 3993 0, 1, instantiatesCanonical)); 3994 children.add(new Property("instantiatesUri", "uri", 3995 "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 3996 0, 1, instantiatesUri)); 3997 children.add(new Property("basedOn", "Reference(Any)", 3998 "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, ServiceRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a task is created to fulfill a procedureRequest ( = FocusOn ) to collect a specimen from a patient.", 3999 0, java.lang.Integer.MAX_VALUE, basedOn)); 4000 children.add(new Property("groupIdentifier", "Identifier", 4001 "An identifier that links together multiple tasks and other requests that were created in the same context.", 0, 4002 1, groupIdentifier)); 4003 children.add(new Property("partOf", "Reference(Task)", "Task that this particular task is part of.", 0, 4004 java.lang.Integer.MAX_VALUE, partOf)); 4005 children.add(new Property("status", "code", "The current status of the task.", 0, 1, status)); 4006 children.add(new Property("statusReason", "CodeableConcept", 4007 "An explanation as to why this task is held, failed, was refused, etc.", 0, 1, statusReason)); 4008 children.add(new Property("businessStatus", "CodeableConcept", 4009 "Contains business-specific nuances of the business state.", 0, 1, businessStatus)); 4010 children.add(new Property("intent", "code", 4011 "Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.", 4012 0, 1, intent)); 4013 children.add(new Property("priority", "code", 4014 "Indicates how quickly the Task should be addressed with respect to other requests.", 0, 1, priority)); 4015 children.add(new Property("code", "CodeableConcept", 4016 "A name or code (or both) briefly describing what the task involves.", 0, 1, code)); 4017 children.add(new Property("description", "string", "A free-text description of what is to be performed.", 0, 1, 4018 description)); 4019 children.add(new Property("focus", "Reference(Any)", 4020 "The request being actioned or the resource being manipulated by this task.", 0, 1, focus)); 4021 children.add(new Property("for", "Reference(Any)", 4022 "The entity who benefits from the performance of the service specified in the task (e.g., the patient).", 0, 1, 4023 for_)); 4024 children.add(new Property("encounter", "Reference(Encounter)", 4025 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.", 4026 0, 1, encounter)); 4027 children.add(new Property("executionPeriod", "Period", 4028 "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).", 4029 0, 1, executionPeriod)); 4030 children.add(new Property("authoredOn", "dateTime", "The date and time this task was created.", 0, 1, authoredOn)); 4031 children.add(new Property("lastModified", "dateTime", "The date and time of last modification to this task.", 0, 1, 4032 lastModified)); 4033 children.add( 4034 new Property("requester", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 4035 "The creator of the task.", 0, 1, requester)); 4036 children.add(new Property("performerType", "CodeableConcept", 4037 "The kind of participant that should perform the task.", 0, java.lang.Integer.MAX_VALUE, performerType)); 4038 children.add(new Property("owner", 4039 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", 4040 "Individual organization or Device currently responsible for task execution.", 0, 1, owner)); 4041 children.add(new Property("location", "Reference(Location)", 4042 "Principal physical location where the this task is performed.", 0, 1, location)); 4043 children.add(new Property("reasonCode", "CodeableConcept", 4044 "A description or code indicating why this task needs to be performed.", 0, 1, reasonCode)); 4045 children.add(new Property("reasonReference", "Reference(Any)", 4046 "A resource reference indicating why this task needs to be performed.", 0, 1, reasonReference)); 4047 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", 4048 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.", 4049 0, java.lang.Integer.MAX_VALUE, insurance)); 4050 children.add(new Property("note", "Annotation", "Free-text information captured about the task as it progresses.", 4051 0, java.lang.Integer.MAX_VALUE, note)); 4052 children.add(new Property("relevantHistory", "Reference(Provenance)", 4053 "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.", 4054 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 4055 children.add(new Property("restriction", "", 4056 "If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 4057 0, 1, restriction)); 4058 children.add(new Property("input", "", "Additional information that may be needed in the execution of the task.", 0, 4059 java.lang.Integer.MAX_VALUE, input)); 4060 children.add(new Property("output", "", "Outputs produced by the Task.", 0, java.lang.Integer.MAX_VALUE, output)); 4061 } 4062 4063 @Override 4064 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4065 switch (_hash) { 4066 case -1618432855: 4067 /* identifier */ return new Property("identifier", "Identifier", "The business identifier for this task.", 0, 4068 java.lang.Integer.MAX_VALUE, identifier); 4069 case 8911915: 4070 /* instantiatesCanonical */ return new Property("instantiatesCanonical", "canonical(ActivityDefinition)", 4071 "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 4072 0, 1, instantiatesCanonical); 4073 case -1926393373: 4074 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 4075 "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 4076 0, 1, instantiatesUri); 4077 case -332612366: 4078 /* basedOn */ return new Property("basedOn", "Reference(Any)", 4079 "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, ServiceRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a task is created to fulfill a procedureRequest ( = FocusOn ) to collect a specimen from a patient.", 4080 0, java.lang.Integer.MAX_VALUE, basedOn); 4081 case -445338488: 4082 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", 4083 "An identifier that links together multiple tasks and other requests that were created in the same context.", 4084 0, 1, groupIdentifier); 4085 case -995410646: 4086 /* partOf */ return new Property("partOf", "Reference(Task)", "Task that this particular task is part of.", 0, 4087 java.lang.Integer.MAX_VALUE, partOf); 4088 case -892481550: 4089 /* status */ return new Property("status", "code", "The current status of the task.", 0, 1, status); 4090 case 2051346646: 4091 /* statusReason */ return new Property("statusReason", "CodeableConcept", 4092 "An explanation as to why this task is held, failed, was refused, etc.", 0, 1, statusReason); 4093 case 2008591314: 4094 /* businessStatus */ return new Property("businessStatus", "CodeableConcept", 4095 "Contains business-specific nuances of the business state.", 0, 1, businessStatus); 4096 case -1183762788: 4097 /* intent */ return new Property("intent", "code", 4098 "Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.", 4099 0, 1, intent); 4100 case -1165461084: 4101 /* priority */ return new Property("priority", "code", 4102 "Indicates how quickly the Task should be addressed with respect to other requests.", 0, 1, priority); 4103 case 3059181: 4104 /* code */ return new Property("code", "CodeableConcept", 4105 "A name or code (or both) briefly describing what the task involves.", 0, 1, code); 4106 case -1724546052: 4107 /* description */ return new Property("description", "string", 4108 "A free-text description of what is to be performed.", 0, 1, description); 4109 case 97604824: 4110 /* focus */ return new Property("focus", "Reference(Any)", 4111 "The request being actioned or the resource being manipulated by this task.", 0, 1, focus); 4112 case 101577: 4113 /* for */ return new Property("for", "Reference(Any)", 4114 "The entity who benefits from the performance of the service specified in the task (e.g., the patient).", 0, 4115 1, for_); 4116 case 1524132147: 4117 /* encounter */ return new Property("encounter", "Reference(Encounter)", 4118 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.", 4119 0, 1, encounter); 4120 case 1218624249: 4121 /* executionPeriod */ return new Property("executionPeriod", "Period", 4122 "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).", 4123 0, 1, executionPeriod); 4124 case -1500852503: 4125 /* authoredOn */ return new Property("authoredOn", "dateTime", "The date and time this task was created.", 0, 1, 4126 authoredOn); 4127 case 1959003007: 4128 /* lastModified */ return new Property("lastModified", "dateTime", 4129 "The date and time of last modification to this task.", 0, 1, lastModified); 4130 case 693933948: 4131 /* requester */ return new Property("requester", 4132 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 4133 "The creator of the task.", 0, 1, requester); 4134 case -901444568: 4135 /* performerType */ return new Property("performerType", "CodeableConcept", 4136 "The kind of participant that should perform the task.", 0, java.lang.Integer.MAX_VALUE, performerType); 4137 case 106164915: 4138 /* owner */ return new Property("owner", 4139 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", 4140 "Individual organization or Device currently responsible for task execution.", 0, 1, owner); 4141 case 1901043637: 4142 /* location */ return new Property("location", "Reference(Location)", 4143 "Principal physical location where the this task is performed.", 0, 1, location); 4144 case 722137681: 4145 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 4146 "A description or code indicating why this task needs to be performed.", 0, 1, reasonCode); 4147 case -1146218137: 4148 /* reasonReference */ return new Property("reasonReference", "Reference(Any)", 4149 "A resource reference indicating why this task needs to be performed.", 0, 1, reasonReference); 4150 case 73049818: 4151 /* insurance */ return new Property("insurance", "Reference(Coverage|ClaimResponse)", 4152 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.", 4153 0, java.lang.Integer.MAX_VALUE, insurance); 4154 case 3387378: 4155 /* note */ return new Property("note", "Annotation", 4156 "Free-text information captured about the task as it progresses.", 0, java.lang.Integer.MAX_VALUE, note); 4157 case 1538891575: 4158 /* relevantHistory */ return new Property("relevantHistory", "Reference(Provenance)", 4159 "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.", 4160 0, java.lang.Integer.MAX_VALUE, relevantHistory); 4161 case -1561062452: 4162 /* restriction */ return new Property("restriction", "", 4163 "If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 4164 0, 1, restriction); 4165 case 100358090: 4166 /* input */ return new Property("input", "", 4167 "Additional information that may be needed in the execution of the task.", 0, java.lang.Integer.MAX_VALUE, 4168 input); 4169 case -1005512447: 4170 /* output */ return new Property("output", "", "Outputs produced by the Task.", 0, java.lang.Integer.MAX_VALUE, 4171 output); 4172 default: 4173 return super.getNamedProperty(_hash, _name, _checkValid); 4174 } 4175 4176 } 4177 4178 @Override 4179 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4180 switch (hash) { 4181 case -1618432855: 4182 /* identifier */ return this.identifier == null ? new Base[0] 4183 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4184 case 8911915: 4185 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 4186 : new Base[] { this.instantiatesCanonical }; // CanonicalType 4187 case -1926393373: 4188 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] : new Base[] { this.instantiatesUri }; // UriType 4189 case -332612366: 4190 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4191 case -445338488: 4192 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 4193 case -995410646: 4194 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 4195 case -892481550: 4196 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<TaskStatus> 4197 case 2051346646: 4198 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 4199 case 2008591314: 4200 /* businessStatus */ return this.businessStatus == null ? new Base[0] : new Base[] { this.businessStatus }; // CodeableConcept 4201 case -1183762788: 4202 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<TaskIntent> 4203 case -1165461084: 4204 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<TaskPriority> 4205 case 3059181: 4206 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 4207 case -1724546052: 4208 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 4209 case 97604824: 4210 /* focus */ return this.focus == null ? new Base[0] : new Base[] { this.focus }; // Reference 4211 case 101577: 4212 /* for */ return this.for_ == null ? new Base[0] : new Base[] { this.for_ }; // Reference 4213 case 1524132147: 4214 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 4215 case 1218624249: 4216 /* executionPeriod */ return this.executionPeriod == null ? new Base[0] : new Base[] { this.executionPeriod }; // Period 4217 case -1500852503: 4218 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 4219 case 1959003007: 4220 /* lastModified */ return this.lastModified == null ? new Base[0] : new Base[] { this.lastModified }; // DateTimeType 4221 case 693933948: 4222 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 4223 case -901444568: 4224 /* performerType */ return this.performerType == null ? new Base[0] 4225 : this.performerType.toArray(new Base[this.performerType.size()]); // CodeableConcept 4226 case 106164915: 4227 /* owner */ return this.owner == null ? new Base[0] : new Base[] { this.owner }; // Reference 4228 case 1901043637: 4229 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 4230 case 722137681: 4231 /* reasonCode */ return this.reasonCode == null ? new Base[0] : new Base[] { this.reasonCode }; // CodeableConcept 4232 case -1146218137: 4233 /* reasonReference */ return this.reasonReference == null ? new Base[0] : new Base[] { this.reasonReference }; // Reference 4234 case 73049818: 4235 /* insurance */ return this.insurance == null ? new Base[0] 4236 : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 4237 case 3387378: 4238 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4239 case 1538891575: 4240 /* relevantHistory */ return this.relevantHistory == null ? new Base[0] 4241 : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 4242 case -1561062452: 4243 /* restriction */ return this.restriction == null ? new Base[0] : new Base[] { this.restriction }; // TaskRestrictionComponent 4244 case 100358090: 4245 /* input */ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // ParameterComponent 4246 case -1005512447: 4247 /* output */ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // TaskOutputComponent 4248 default: 4249 return super.getProperty(hash, name, checkValid); 4250 } 4251 4252 } 4253 4254 @Override 4255 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4256 switch (hash) { 4257 case -1618432855: // identifier 4258 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4259 return value; 4260 case 8911915: // instantiatesCanonical 4261 this.instantiatesCanonical = castToCanonical(value); // CanonicalType 4262 return value; 4263 case -1926393373: // instantiatesUri 4264 this.instantiatesUri = castToUri(value); // UriType 4265 return value; 4266 case -332612366: // basedOn 4267 this.getBasedOn().add(castToReference(value)); // Reference 4268 return value; 4269 case -445338488: // groupIdentifier 4270 this.groupIdentifier = castToIdentifier(value); // Identifier 4271 return value; 4272 case -995410646: // partOf 4273 this.getPartOf().add(castToReference(value)); // Reference 4274 return value; 4275 case -892481550: // status 4276 value = new TaskStatusEnumFactory().fromType(castToCode(value)); 4277 this.status = (Enumeration) value; // Enumeration<TaskStatus> 4278 return value; 4279 case 2051346646: // statusReason 4280 this.statusReason = castToCodeableConcept(value); // CodeableConcept 4281 return value; 4282 case 2008591314: // businessStatus 4283 this.businessStatus = castToCodeableConcept(value); // CodeableConcept 4284 return value; 4285 case -1183762788: // intent 4286 value = new TaskIntentEnumFactory().fromType(castToCode(value)); 4287 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 4288 return value; 4289 case -1165461084: // priority 4290 value = new TaskPriorityEnumFactory().fromType(castToCode(value)); 4291 this.priority = (Enumeration) value; // Enumeration<TaskPriority> 4292 return value; 4293 case 3059181: // code 4294 this.code = castToCodeableConcept(value); // CodeableConcept 4295 return value; 4296 case -1724546052: // description 4297 this.description = castToString(value); // StringType 4298 return value; 4299 case 97604824: // focus 4300 this.focus = castToReference(value); // Reference 4301 return value; 4302 case 101577: // for 4303 this.for_ = castToReference(value); // Reference 4304 return value; 4305 case 1524132147: // encounter 4306 this.encounter = castToReference(value); // Reference 4307 return value; 4308 case 1218624249: // executionPeriod 4309 this.executionPeriod = castToPeriod(value); // Period 4310 return value; 4311 case -1500852503: // authoredOn 4312 this.authoredOn = castToDateTime(value); // DateTimeType 4313 return value; 4314 case 1959003007: // lastModified 4315 this.lastModified = castToDateTime(value); // DateTimeType 4316 return value; 4317 case 693933948: // requester 4318 this.requester = castToReference(value); // Reference 4319 return value; 4320 case -901444568: // performerType 4321 this.getPerformerType().add(castToCodeableConcept(value)); // CodeableConcept 4322 return value; 4323 case 106164915: // owner 4324 this.owner = castToReference(value); // Reference 4325 return value; 4326 case 1901043637: // location 4327 this.location = castToReference(value); // Reference 4328 return value; 4329 case 722137681: // reasonCode 4330 this.reasonCode = castToCodeableConcept(value); // CodeableConcept 4331 return value; 4332 case -1146218137: // reasonReference 4333 this.reasonReference = castToReference(value); // Reference 4334 return value; 4335 case 73049818: // insurance 4336 this.getInsurance().add(castToReference(value)); // Reference 4337 return value; 4338 case 3387378: // note 4339 this.getNote().add(castToAnnotation(value)); // Annotation 4340 return value; 4341 case 1538891575: // relevantHistory 4342 this.getRelevantHistory().add(castToReference(value)); // Reference 4343 return value; 4344 case -1561062452: // restriction 4345 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 4346 return value; 4347 case 100358090: // input 4348 this.getInput().add((ParameterComponent) value); // ParameterComponent 4349 return value; 4350 case -1005512447: // output 4351 this.getOutput().add((TaskOutputComponent) value); // TaskOutputComponent 4352 return value; 4353 default: 4354 return super.setProperty(hash, name, value); 4355 } 4356 4357 } 4358 4359 @Override 4360 public Base setProperty(String name, Base value) throws FHIRException { 4361 if (name.equals("identifier")) { 4362 this.getIdentifier().add(castToIdentifier(value)); 4363 } else if (name.equals("instantiatesCanonical")) { 4364 this.instantiatesCanonical = castToCanonical(value); // CanonicalType 4365 } else if (name.equals("instantiatesUri")) { 4366 this.instantiatesUri = castToUri(value); // UriType 4367 } else if (name.equals("basedOn")) { 4368 this.getBasedOn().add(castToReference(value)); 4369 } else if (name.equals("groupIdentifier")) { 4370 this.groupIdentifier = castToIdentifier(value); // Identifier 4371 } else if (name.equals("partOf")) { 4372 this.getPartOf().add(castToReference(value)); 4373 } else if (name.equals("status")) { 4374 value = new TaskStatusEnumFactory().fromType(castToCode(value)); 4375 this.status = (Enumeration) value; // Enumeration<TaskStatus> 4376 } else if (name.equals("statusReason")) { 4377 this.statusReason = castToCodeableConcept(value); // CodeableConcept 4378 } else if (name.equals("businessStatus")) { 4379 this.businessStatus = castToCodeableConcept(value); // CodeableConcept 4380 } else if (name.equals("intent")) { 4381 value = new TaskIntentEnumFactory().fromType(castToCode(value)); 4382 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 4383 } else if (name.equals("priority")) { 4384 value = new TaskPriorityEnumFactory().fromType(castToCode(value)); 4385 this.priority = (Enumeration) value; // Enumeration<TaskPriority> 4386 } else if (name.equals("code")) { 4387 this.code = castToCodeableConcept(value); // CodeableConcept 4388 } else if (name.equals("description")) { 4389 this.description = castToString(value); // StringType 4390 } else if (name.equals("focus")) { 4391 this.focus = castToReference(value); // Reference 4392 } else if (name.equals("for")) { 4393 this.for_ = castToReference(value); // Reference 4394 } else if (name.equals("encounter")) { 4395 this.encounter = castToReference(value); // Reference 4396 } else if (name.equals("executionPeriod")) { 4397 this.executionPeriod = castToPeriod(value); // Period 4398 } else if (name.equals("authoredOn")) { 4399 this.authoredOn = castToDateTime(value); // DateTimeType 4400 } else if (name.equals("lastModified")) { 4401 this.lastModified = castToDateTime(value); // DateTimeType 4402 } else if (name.equals("requester")) { 4403 this.requester = castToReference(value); // Reference 4404 } else if (name.equals("performerType")) { 4405 this.getPerformerType().add(castToCodeableConcept(value)); 4406 } else if (name.equals("owner")) { 4407 this.owner = castToReference(value); // Reference 4408 } else if (name.equals("location")) { 4409 this.location = castToReference(value); // Reference 4410 } else if (name.equals("reasonCode")) { 4411 this.reasonCode = castToCodeableConcept(value); // CodeableConcept 4412 } else if (name.equals("reasonReference")) { 4413 this.reasonReference = castToReference(value); // Reference 4414 } else if (name.equals("insurance")) { 4415 this.getInsurance().add(castToReference(value)); 4416 } else if (name.equals("note")) { 4417 this.getNote().add(castToAnnotation(value)); 4418 } else if (name.equals("relevantHistory")) { 4419 this.getRelevantHistory().add(castToReference(value)); 4420 } else if (name.equals("restriction")) { 4421 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 4422 } else if (name.equals("input")) { 4423 this.getInput().add((ParameterComponent) value); 4424 } else if (name.equals("output")) { 4425 this.getOutput().add((TaskOutputComponent) value); 4426 } else 4427 return super.setProperty(name, value); 4428 return value; 4429 } 4430 4431 @Override 4432 public void removeChild(String name, Base value) throws FHIRException { 4433 if (name.equals("identifier")) { 4434 this.getIdentifier().remove(castToIdentifier(value)); 4435 } else if (name.equals("instantiatesCanonical")) { 4436 this.instantiatesCanonical = null; 4437 } else if (name.equals("instantiatesUri")) { 4438 this.instantiatesUri = null; 4439 } else if (name.equals("basedOn")) { 4440 this.getBasedOn().remove(castToReference(value)); 4441 } else if (name.equals("groupIdentifier")) { 4442 this.groupIdentifier = null; 4443 } else if (name.equals("partOf")) { 4444 this.getPartOf().remove(castToReference(value)); 4445 } else if (name.equals("status")) { 4446 this.status = null; 4447 } else if (name.equals("statusReason")) { 4448 this.statusReason = null; 4449 } else if (name.equals("businessStatus")) { 4450 this.businessStatus = null; 4451 } else if (name.equals("intent")) { 4452 this.intent = null; 4453 } else if (name.equals("priority")) { 4454 this.priority = null; 4455 } else if (name.equals("code")) { 4456 this.code = null; 4457 } else if (name.equals("description")) { 4458 this.description = null; 4459 } else if (name.equals("focus")) { 4460 this.focus = null; 4461 } else if (name.equals("for")) { 4462 this.for_ = null; 4463 } else if (name.equals("encounter")) { 4464 this.encounter = null; 4465 } else if (name.equals("executionPeriod")) { 4466 this.executionPeriod = null; 4467 } else if (name.equals("authoredOn")) { 4468 this.authoredOn = null; 4469 } else if (name.equals("lastModified")) { 4470 this.lastModified = null; 4471 } else if (name.equals("requester")) { 4472 this.requester = null; 4473 } else if (name.equals("performerType")) { 4474 this.getPerformerType().remove(castToCodeableConcept(value)); 4475 } else if (name.equals("owner")) { 4476 this.owner = null; 4477 } else if (name.equals("location")) { 4478 this.location = null; 4479 } else if (name.equals("reasonCode")) { 4480 this.reasonCode = null; 4481 } else if (name.equals("reasonReference")) { 4482 this.reasonReference = null; 4483 } else if (name.equals("insurance")) { 4484 this.getInsurance().remove(castToReference(value)); 4485 } else if (name.equals("note")) { 4486 this.getNote().remove(castToAnnotation(value)); 4487 } else if (name.equals("relevantHistory")) { 4488 this.getRelevantHistory().remove(castToReference(value)); 4489 } else if (name.equals("restriction")) { 4490 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 4491 } else if (name.equals("input")) { 4492 this.getInput().remove((ParameterComponent) value); 4493 } else if (name.equals("output")) { 4494 this.getOutput().remove((TaskOutputComponent) value); 4495 } else 4496 super.removeChild(name, value); 4497 4498 } 4499 4500 @Override 4501 public Base makeProperty(int hash, String name) throws FHIRException { 4502 switch (hash) { 4503 case -1618432855: 4504 return addIdentifier(); 4505 case 8911915: 4506 return getInstantiatesCanonicalElement(); 4507 case -1926393373: 4508 return getInstantiatesUriElement(); 4509 case -332612366: 4510 return addBasedOn(); 4511 case -445338488: 4512 return getGroupIdentifier(); 4513 case -995410646: 4514 return addPartOf(); 4515 case -892481550: 4516 return getStatusElement(); 4517 case 2051346646: 4518 return getStatusReason(); 4519 case 2008591314: 4520 return getBusinessStatus(); 4521 case -1183762788: 4522 return getIntentElement(); 4523 case -1165461084: 4524 return getPriorityElement(); 4525 case 3059181: 4526 return getCode(); 4527 case -1724546052: 4528 return getDescriptionElement(); 4529 case 97604824: 4530 return getFocus(); 4531 case 101577: 4532 return getFor(); 4533 case 1524132147: 4534 return getEncounter(); 4535 case 1218624249: 4536 return getExecutionPeriod(); 4537 case -1500852503: 4538 return getAuthoredOnElement(); 4539 case 1959003007: 4540 return getLastModifiedElement(); 4541 case 693933948: 4542 return getRequester(); 4543 case -901444568: 4544 return addPerformerType(); 4545 case 106164915: 4546 return getOwner(); 4547 case 1901043637: 4548 return getLocation(); 4549 case 722137681: 4550 return getReasonCode(); 4551 case -1146218137: 4552 return getReasonReference(); 4553 case 73049818: 4554 return addInsurance(); 4555 case 3387378: 4556 return addNote(); 4557 case 1538891575: 4558 return addRelevantHistory(); 4559 case -1561062452: 4560 return getRestriction(); 4561 case 100358090: 4562 return addInput(); 4563 case -1005512447: 4564 return addOutput(); 4565 default: 4566 return super.makeProperty(hash, name); 4567 } 4568 4569 } 4570 4571 @Override 4572 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4573 switch (hash) { 4574 case -1618432855: 4575 /* identifier */ return new String[] { "Identifier" }; 4576 case 8911915: 4577 /* instantiatesCanonical */ return new String[] { "canonical" }; 4578 case -1926393373: 4579 /* instantiatesUri */ return new String[] { "uri" }; 4580 case -332612366: 4581 /* basedOn */ return new String[] { "Reference" }; 4582 case -445338488: 4583 /* groupIdentifier */ return new String[] { "Identifier" }; 4584 case -995410646: 4585 /* partOf */ return new String[] { "Reference" }; 4586 case -892481550: 4587 /* status */ return new String[] { "code" }; 4588 case 2051346646: 4589 /* statusReason */ return new String[] { "CodeableConcept" }; 4590 case 2008591314: 4591 /* businessStatus */ return new String[] { "CodeableConcept" }; 4592 case -1183762788: 4593 /* intent */ return new String[] { "code" }; 4594 case -1165461084: 4595 /* priority */ return new String[] { "code" }; 4596 case 3059181: 4597 /* code */ return new String[] { "CodeableConcept" }; 4598 case -1724546052: 4599 /* description */ return new String[] { "string" }; 4600 case 97604824: 4601 /* focus */ return new String[] { "Reference" }; 4602 case 101577: 4603 /* for */ return new String[] { "Reference" }; 4604 case 1524132147: 4605 /* encounter */ return new String[] { "Reference" }; 4606 case 1218624249: 4607 /* executionPeriod */ return new String[] { "Period" }; 4608 case -1500852503: 4609 /* authoredOn */ return new String[] { "dateTime" }; 4610 case 1959003007: 4611 /* lastModified */ return new String[] { "dateTime" }; 4612 case 693933948: 4613 /* requester */ return new String[] { "Reference" }; 4614 case -901444568: 4615 /* performerType */ return new String[] { "CodeableConcept" }; 4616 case 106164915: 4617 /* owner */ return new String[] { "Reference" }; 4618 case 1901043637: 4619 /* location */ return new String[] { "Reference" }; 4620 case 722137681: 4621 /* reasonCode */ return new String[] { "CodeableConcept" }; 4622 case -1146218137: 4623 /* reasonReference */ return new String[] { "Reference" }; 4624 case 73049818: 4625 /* insurance */ return new String[] { "Reference" }; 4626 case 3387378: 4627 /* note */ return new String[] { "Annotation" }; 4628 case 1538891575: 4629 /* relevantHistory */ return new String[] { "Reference" }; 4630 case -1561062452: 4631 /* restriction */ return new String[] {}; 4632 case 100358090: 4633 /* input */ return new String[] {}; 4634 case -1005512447: 4635 /* output */ return new String[] {}; 4636 default: 4637 return super.getTypesForProperty(hash, name); 4638 } 4639 4640 } 4641 4642 @Override 4643 public Base addChild(String name) throws FHIRException { 4644 if (name.equals("identifier")) { 4645 return addIdentifier(); 4646 } else if (name.equals("instantiatesCanonical")) { 4647 throw new FHIRException("Cannot call addChild on a singleton property Task.instantiatesCanonical"); 4648 } else if (name.equals("instantiatesUri")) { 4649 throw new FHIRException("Cannot call addChild on a singleton property Task.instantiatesUri"); 4650 } else if (name.equals("basedOn")) { 4651 return addBasedOn(); 4652 } else if (name.equals("groupIdentifier")) { 4653 this.groupIdentifier = new Identifier(); 4654 return this.groupIdentifier; 4655 } else if (name.equals("partOf")) { 4656 return addPartOf(); 4657 } else if (name.equals("status")) { 4658 throw new FHIRException("Cannot call addChild on a singleton property Task.status"); 4659 } else if (name.equals("statusReason")) { 4660 this.statusReason = new CodeableConcept(); 4661 return this.statusReason; 4662 } else if (name.equals("businessStatus")) { 4663 this.businessStatus = new CodeableConcept(); 4664 return this.businessStatus; 4665 } else if (name.equals("intent")) { 4666 throw new FHIRException("Cannot call addChild on a singleton property Task.intent"); 4667 } else if (name.equals("priority")) { 4668 throw new FHIRException("Cannot call addChild on a singleton property Task.priority"); 4669 } else if (name.equals("code")) { 4670 this.code = new CodeableConcept(); 4671 return this.code; 4672 } else if (name.equals("description")) { 4673 throw new FHIRException("Cannot call addChild on a singleton property Task.description"); 4674 } else if (name.equals("focus")) { 4675 this.focus = new Reference(); 4676 return this.focus; 4677 } else if (name.equals("for")) { 4678 this.for_ = new Reference(); 4679 return this.for_; 4680 } else if (name.equals("encounter")) { 4681 this.encounter = new Reference(); 4682 return this.encounter; 4683 } else if (name.equals("executionPeriod")) { 4684 this.executionPeriod = new Period(); 4685 return this.executionPeriod; 4686 } else if (name.equals("authoredOn")) { 4687 throw new FHIRException("Cannot call addChild on a singleton property Task.authoredOn"); 4688 } else if (name.equals("lastModified")) { 4689 throw new FHIRException("Cannot call addChild on a singleton property Task.lastModified"); 4690 } else if (name.equals("requester")) { 4691 this.requester = new Reference(); 4692 return this.requester; 4693 } else if (name.equals("performerType")) { 4694 return addPerformerType(); 4695 } else if (name.equals("owner")) { 4696 this.owner = new Reference(); 4697 return this.owner; 4698 } else if (name.equals("location")) { 4699 this.location = new Reference(); 4700 return this.location; 4701 } else if (name.equals("reasonCode")) { 4702 this.reasonCode = new CodeableConcept(); 4703 return this.reasonCode; 4704 } else if (name.equals("reasonReference")) { 4705 this.reasonReference = new Reference(); 4706 return this.reasonReference; 4707 } else if (name.equals("insurance")) { 4708 return addInsurance(); 4709 } else if (name.equals("note")) { 4710 return addNote(); 4711 } else if (name.equals("relevantHistory")) { 4712 return addRelevantHistory(); 4713 } else if (name.equals("restriction")) { 4714 this.restriction = new TaskRestrictionComponent(); 4715 return this.restriction; 4716 } else if (name.equals("input")) { 4717 return addInput(); 4718 } else if (name.equals("output")) { 4719 return addOutput(); 4720 } else 4721 return super.addChild(name); 4722 } 4723 4724 public String fhirType() { 4725 return "Task"; 4726 4727 } 4728 4729 public Task copy() { 4730 Task dst = new Task(); 4731 copyValues(dst); 4732 return dst; 4733 } 4734 4735 public void copyValues(Task dst) { 4736 super.copyValues(dst); 4737 if (identifier != null) { 4738 dst.identifier = new ArrayList<Identifier>(); 4739 for (Identifier i : identifier) 4740 dst.identifier.add(i.copy()); 4741 } 4742 ; 4743 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 4744 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 4745 if (basedOn != null) { 4746 dst.basedOn = new ArrayList<Reference>(); 4747 for (Reference i : basedOn) 4748 dst.basedOn.add(i.copy()); 4749 } 4750 ; 4751 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 4752 if (partOf != null) { 4753 dst.partOf = new ArrayList<Reference>(); 4754 for (Reference i : partOf) 4755 dst.partOf.add(i.copy()); 4756 } 4757 ; 4758 dst.status = status == null ? null : status.copy(); 4759 dst.statusReason = statusReason == null ? null : statusReason.copy(); 4760 dst.businessStatus = businessStatus == null ? null : businessStatus.copy(); 4761 dst.intent = intent == null ? null : intent.copy(); 4762 dst.priority = priority == null ? null : priority.copy(); 4763 dst.code = code == null ? null : code.copy(); 4764 dst.description = description == null ? null : description.copy(); 4765 dst.focus = focus == null ? null : focus.copy(); 4766 dst.for_ = for_ == null ? null : for_.copy(); 4767 dst.encounter = encounter == null ? null : encounter.copy(); 4768 dst.executionPeriod = executionPeriod == null ? null : executionPeriod.copy(); 4769 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 4770 dst.lastModified = lastModified == null ? null : lastModified.copy(); 4771 dst.requester = requester == null ? null : requester.copy(); 4772 if (performerType != null) { 4773 dst.performerType = new ArrayList<CodeableConcept>(); 4774 for (CodeableConcept i : performerType) 4775 dst.performerType.add(i.copy()); 4776 } 4777 ; 4778 dst.owner = owner == null ? null : owner.copy(); 4779 dst.location = location == null ? null : location.copy(); 4780 dst.reasonCode = reasonCode == null ? null : reasonCode.copy(); 4781 dst.reasonReference = reasonReference == null ? null : reasonReference.copy(); 4782 if (insurance != null) { 4783 dst.insurance = new ArrayList<Reference>(); 4784 for (Reference i : insurance) 4785 dst.insurance.add(i.copy()); 4786 } 4787 ; 4788 if (note != null) { 4789 dst.note = new ArrayList<Annotation>(); 4790 for (Annotation i : note) 4791 dst.note.add(i.copy()); 4792 } 4793 ; 4794 if (relevantHistory != null) { 4795 dst.relevantHistory = new ArrayList<Reference>(); 4796 for (Reference i : relevantHistory) 4797 dst.relevantHistory.add(i.copy()); 4798 } 4799 ; 4800 dst.restriction = restriction == null ? null : restriction.copy(); 4801 if (input != null) { 4802 dst.input = new ArrayList<ParameterComponent>(); 4803 for (ParameterComponent i : input) 4804 dst.input.add(i.copy()); 4805 } 4806 ; 4807 if (output != null) { 4808 dst.output = new ArrayList<TaskOutputComponent>(); 4809 for (TaskOutputComponent i : output) 4810 dst.output.add(i.copy()); 4811 } 4812 ; 4813 } 4814 4815 protected Task typedCopy() { 4816 return copy(); 4817 } 4818 4819 @Override 4820 public boolean equalsDeep(Base other_) { 4821 if (!super.equalsDeep(other_)) 4822 return false; 4823 if (!(other_ instanceof Task)) 4824 return false; 4825 Task o = (Task) other_; 4826 return compareDeep(identifier, o.identifier, true) 4827 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 4828 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 4829 && compareDeep(groupIdentifier, o.groupIdentifier, true) && compareDeep(partOf, o.partOf, true) 4830 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 4831 && compareDeep(businessStatus, o.businessStatus, true) && compareDeep(intent, o.intent, true) 4832 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 4833 && compareDeep(description, o.description, true) && compareDeep(focus, o.focus, true) 4834 && compareDeep(for_, o.for_, true) && compareDeep(encounter, o.encounter, true) 4835 && compareDeep(executionPeriod, o.executionPeriod, true) && compareDeep(authoredOn, o.authoredOn, true) 4836 && compareDeep(lastModified, o.lastModified, true) && compareDeep(requester, o.requester, true) 4837 && compareDeep(performerType, o.performerType, true) && compareDeep(owner, o.owner, true) 4838 && compareDeep(location, o.location, true) && compareDeep(reasonCode, o.reasonCode, true) 4839 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(insurance, o.insurance, true) 4840 && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true) 4841 && compareDeep(restriction, o.restriction, true) && compareDeep(input, o.input, true) 4842 && compareDeep(output, o.output, true); 4843 } 4844 4845 @Override 4846 public boolean equalsShallow(Base other_) { 4847 if (!super.equalsShallow(other_)) 4848 return false; 4849 if (!(other_ instanceof Task)) 4850 return false; 4851 Task o = (Task) other_; 4852 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 4853 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 4854 && compareValues(description, o.description, true) && compareValues(authoredOn, o.authoredOn, true) 4855 && compareValues(lastModified, o.lastModified, true); 4856 } 4857 4858 public boolean isEmpty() { 4859 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 4860 basedOn, groupIdentifier, partOf, status, statusReason, businessStatus, intent, priority, code, description, 4861 focus, for_, encounter, executionPeriod, authoredOn, lastModified, requester, performerType, owner, location, 4862 reasonCode, reasonReference, insurance, note, relevantHistory, restriction, input, output); 4863 } 4864 4865 @Override 4866 public ResourceType getResourceType() { 4867 return ResourceType.Task; 4868 } 4869 4870 /** 4871 * Search parameter: <b>owner</b> 4872 * <p> 4873 * Description: <b>Search by task owner</b><br> 4874 * Type: <b>reference</b><br> 4875 * Path: <b>Task.owner</b><br> 4876 * </p> 4877 */ 4878 @SearchParamDefinition(name = "owner", path = "Task.owner", description = "Search by task owner", type = "reference", target = { 4879 CareTeam.class, Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, 4880 PractitionerRole.class, RelatedPerson.class }) 4881 public static final String SP_OWNER = "owner"; 4882 /** 4883 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 4884 * <p> 4885 * Description: <b>Search by task owner</b><br> 4886 * Type: <b>reference</b><br> 4887 * Path: <b>Task.owner</b><br> 4888 * </p> 4889 */ 4890 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4891 SP_OWNER); 4892 4893 /** 4894 * Constant for fluent queries to be used to add include statements. Specifies 4895 * the path value of "<b>Task:owner</b>". 4896 */ 4897 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Task:owner") 4898 .toLocked(); 4899 4900 /** 4901 * Search parameter: <b>requester</b> 4902 * <p> 4903 * Description: <b>Search by task requester</b><br> 4904 * Type: <b>reference</b><br> 4905 * Path: <b>Task.requester</b><br> 4906 * </p> 4907 */ 4908 @SearchParamDefinition(name = "requester", path = "Task.requester", description = "Search by task requester", type = "reference", target = { 4909 Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 4910 RelatedPerson.class }) 4911 public static final String SP_REQUESTER = "requester"; 4912 /** 4913 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 4914 * <p> 4915 * Description: <b>Search by task requester</b><br> 4916 * Type: <b>reference</b><br> 4917 * Path: <b>Task.requester</b><br> 4918 * </p> 4919 */ 4920 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4921 SP_REQUESTER); 4922 4923 /** 4924 * Constant for fluent queries to be used to add include statements. Specifies 4925 * the path value of "<b>Task:requester</b>". 4926 */ 4927 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 4928 "Task:requester").toLocked(); 4929 4930 /** 4931 * Search parameter: <b>identifier</b> 4932 * <p> 4933 * Description: <b>Search for a task instance by its business identifier</b><br> 4934 * Type: <b>token</b><br> 4935 * Path: <b>Task.identifier</b><br> 4936 * </p> 4937 */ 4938 @SearchParamDefinition(name = "identifier", path = "Task.identifier", description = "Search for a task instance by its business identifier", type = "token") 4939 public static final String SP_IDENTIFIER = "identifier"; 4940 /** 4941 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4942 * <p> 4943 * Description: <b>Search for a task instance by its business identifier</b><br> 4944 * Type: <b>token</b><br> 4945 * Path: <b>Task.identifier</b><br> 4946 * </p> 4947 */ 4948 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4949 SP_IDENTIFIER); 4950 4951 /** 4952 * Search parameter: <b>business-status</b> 4953 * <p> 4954 * Description: <b>Search by business status</b><br> 4955 * Type: <b>token</b><br> 4956 * Path: <b>Task.businessStatus</b><br> 4957 * </p> 4958 */ 4959 @SearchParamDefinition(name = "business-status", path = "Task.businessStatus", description = "Search by business status", type = "token") 4960 public static final String SP_BUSINESS_STATUS = "business-status"; 4961 /** 4962 * <b>Fluent Client</b> search parameter constant for <b>business-status</b> 4963 * <p> 4964 * Description: <b>Search by business status</b><br> 4965 * Type: <b>token</b><br> 4966 * Path: <b>Task.businessStatus</b><br> 4967 * </p> 4968 */ 4969 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BUSINESS_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4970 SP_BUSINESS_STATUS); 4971 4972 /** 4973 * Search parameter: <b>period</b> 4974 * <p> 4975 * Description: <b>Search by period Task is/was underway</b><br> 4976 * Type: <b>date</b><br> 4977 * Path: <b>Task.executionPeriod</b><br> 4978 * </p> 4979 */ 4980 @SearchParamDefinition(name = "period", path = "Task.executionPeriod", description = "Search by period Task is/was underway", type = "date") 4981 public static final String SP_PERIOD = "period"; 4982 /** 4983 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4984 * <p> 4985 * Description: <b>Search by period Task is/was underway</b><br> 4986 * Type: <b>date</b><br> 4987 * Path: <b>Task.executionPeriod</b><br> 4988 * </p> 4989 */ 4990 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 4991 SP_PERIOD); 4992 4993 /** 4994 * Search parameter: <b>code</b> 4995 * <p> 4996 * Description: <b>Search by task code</b><br> 4997 * Type: <b>token</b><br> 4998 * Path: <b>Task.code</b><br> 4999 * </p> 5000 */ 5001 @SearchParamDefinition(name = "code", path = "Task.code", description = "Search by task code", type = "token") 5002 public static final String SP_CODE = "code"; 5003 /** 5004 * <b>Fluent Client</b> search parameter constant for <b>code</b> 5005 * <p> 5006 * Description: <b>Search by task code</b><br> 5007 * Type: <b>token</b><br> 5008 * Path: <b>Task.code</b><br> 5009 * </p> 5010 */ 5011 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5012 SP_CODE); 5013 5014 /** 5015 * Search parameter: <b>performer</b> 5016 * <p> 5017 * Description: <b>Search by recommended type of performer (e.g., Requester, 5018 * Performer, Scheduler).</b><br> 5019 * Type: <b>token</b><br> 5020 * Path: <b>Task.performerType</b><br> 5021 * </p> 5022 */ 5023 @SearchParamDefinition(name = "performer", path = "Task.performerType", description = "Search by recommended type of performer (e.g., Requester, Performer, Scheduler).", type = "token") 5024 public static final String SP_PERFORMER = "performer"; 5025 /** 5026 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 5027 * <p> 5028 * Description: <b>Search by recommended type of performer (e.g., Requester, 5029 * Performer, Scheduler).</b><br> 5030 * Type: <b>token</b><br> 5031 * Path: <b>Task.performerType</b><br> 5032 * </p> 5033 */ 5034 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5035 SP_PERFORMER); 5036 5037 /** 5038 * Search parameter: <b>subject</b> 5039 * <p> 5040 * Description: <b>Search by subject</b><br> 5041 * Type: <b>reference</b><br> 5042 * Path: <b>Task.for</b><br> 5043 * </p> 5044 */ 5045 @SearchParamDefinition(name = "subject", path = "Task.for", description = "Search by subject", type = "reference") 5046 public static final String SP_SUBJECT = "subject"; 5047 /** 5048 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5049 * <p> 5050 * Description: <b>Search by subject</b><br> 5051 * Type: <b>reference</b><br> 5052 * Path: <b>Task.for</b><br> 5053 * </p> 5054 */ 5055 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5056 SP_SUBJECT); 5057 5058 /** 5059 * Constant for fluent queries to be used to add include statements. Specifies 5060 * the path value of "<b>Task:subject</b>". 5061 */ 5062 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Task:subject") 5063 .toLocked(); 5064 5065 /** 5066 * Search parameter: <b>focus</b> 5067 * <p> 5068 * Description: <b>Search by task focus</b><br> 5069 * Type: <b>reference</b><br> 5070 * Path: <b>Task.focus</b><br> 5071 * </p> 5072 */ 5073 @SearchParamDefinition(name = "focus", path = "Task.focus", description = "Search by task focus", type = "reference") 5074 public static final String SP_FOCUS = "focus"; 5075 /** 5076 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 5077 * <p> 5078 * Description: <b>Search by task focus</b><br> 5079 * Type: <b>reference</b><br> 5080 * Path: <b>Task.focus</b><br> 5081 * </p> 5082 */ 5083 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5084 SP_FOCUS); 5085 5086 /** 5087 * Constant for fluent queries to be used to add include statements. Specifies 5088 * the path value of "<b>Task:focus</b>". 5089 */ 5090 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("Task:focus") 5091 .toLocked(); 5092 5093 /** 5094 * Search parameter: <b>part-of</b> 5095 * <p> 5096 * Description: <b>Search by task this task is part of</b><br> 5097 * Type: <b>reference</b><br> 5098 * Path: <b>Task.partOf</b><br> 5099 * </p> 5100 */ 5101 @SearchParamDefinition(name = "part-of", path = "Task.partOf", description = "Search by task this task is part of", type = "reference", target = { 5102 Task.class }) 5103 public static final String SP_PART_OF = "part-of"; 5104 /** 5105 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 5106 * <p> 5107 * Description: <b>Search by task this task is part of</b><br> 5108 * Type: <b>reference</b><br> 5109 * Path: <b>Task.partOf</b><br> 5110 * </p> 5111 */ 5112 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5113 SP_PART_OF); 5114 5115 /** 5116 * Constant for fluent queries to be used to add include statements. Specifies 5117 * the path value of "<b>Task:part-of</b>". 5118 */ 5119 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Task:part-of") 5120 .toLocked(); 5121 5122 /** 5123 * Search parameter: <b>encounter</b> 5124 * <p> 5125 * Description: <b>Search by encounter</b><br> 5126 * Type: <b>reference</b><br> 5127 * Path: <b>Task.encounter</b><br> 5128 * </p> 5129 */ 5130 @SearchParamDefinition(name = "encounter", path = "Task.encounter", description = "Search by encounter", type = "reference", target = { 5131 Encounter.class }) 5132 public static final String SP_ENCOUNTER = "encounter"; 5133 /** 5134 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5135 * <p> 5136 * Description: <b>Search by encounter</b><br> 5137 * Type: <b>reference</b><br> 5138 * Path: <b>Task.encounter</b><br> 5139 * </p> 5140 */ 5141 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5142 SP_ENCOUNTER); 5143 5144 /** 5145 * Constant for fluent queries to be used to add include statements. Specifies 5146 * the path value of "<b>Task:encounter</b>". 5147 */ 5148 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 5149 "Task:encounter").toLocked(); 5150 5151 /** 5152 * Search parameter: <b>priority</b> 5153 * <p> 5154 * Description: <b>Search by task priority</b><br> 5155 * Type: <b>token</b><br> 5156 * Path: <b>Task.priority</b><br> 5157 * </p> 5158 */ 5159 @SearchParamDefinition(name = "priority", path = "Task.priority", description = "Search by task priority", type = "token") 5160 public static final String SP_PRIORITY = "priority"; 5161 /** 5162 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 5163 * <p> 5164 * Description: <b>Search by task priority</b><br> 5165 * Type: <b>token</b><br> 5166 * Path: <b>Task.priority</b><br> 5167 * </p> 5168 */ 5169 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5170 SP_PRIORITY); 5171 5172 /** 5173 * Search parameter: <b>authored-on</b> 5174 * <p> 5175 * Description: <b>Search by creation date</b><br> 5176 * Type: <b>date</b><br> 5177 * Path: <b>Task.authoredOn</b><br> 5178 * </p> 5179 */ 5180 @SearchParamDefinition(name = "authored-on", path = "Task.authoredOn", description = "Search by creation date", type = "date") 5181 public static final String SP_AUTHORED_ON = "authored-on"; 5182 /** 5183 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 5184 * <p> 5185 * Description: <b>Search by creation date</b><br> 5186 * Type: <b>date</b><br> 5187 * Path: <b>Task.authoredOn</b><br> 5188 * </p> 5189 */ 5190 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam( 5191 SP_AUTHORED_ON); 5192 5193 /** 5194 * Search parameter: <b>intent</b> 5195 * <p> 5196 * Description: <b>Search by task intent</b><br> 5197 * Type: <b>token</b><br> 5198 * Path: <b>Task.intent</b><br> 5199 * </p> 5200 */ 5201 @SearchParamDefinition(name = "intent", path = "Task.intent", description = "Search by task intent", type = "token") 5202 public static final String SP_INTENT = "intent"; 5203 /** 5204 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 5205 * <p> 5206 * Description: <b>Search by task intent</b><br> 5207 * Type: <b>token</b><br> 5208 * Path: <b>Task.intent</b><br> 5209 * </p> 5210 */ 5211 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5212 SP_INTENT); 5213 5214 /** 5215 * Search parameter: <b>group-identifier</b> 5216 * <p> 5217 * Description: <b>Search by group identifier</b><br> 5218 * Type: <b>token</b><br> 5219 * Path: <b>Task.groupIdentifier</b><br> 5220 * </p> 5221 */ 5222 @SearchParamDefinition(name = "group-identifier", path = "Task.groupIdentifier", description = "Search by group identifier", type = "token") 5223 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 5224 /** 5225 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 5226 * <p> 5227 * Description: <b>Search by group identifier</b><br> 5228 * Type: <b>token</b><br> 5229 * Path: <b>Task.groupIdentifier</b><br> 5230 * </p> 5231 */ 5232 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5233 SP_GROUP_IDENTIFIER); 5234 5235 /** 5236 * Search parameter: <b>based-on</b> 5237 * <p> 5238 * Description: <b>Search by requests this task is based on</b><br> 5239 * Type: <b>reference</b><br> 5240 * Path: <b>Task.basedOn</b><br> 5241 * </p> 5242 */ 5243 @SearchParamDefinition(name = "based-on", path = "Task.basedOn", description = "Search by requests this task is based on", type = "reference") 5244 public static final String SP_BASED_ON = "based-on"; 5245 /** 5246 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 5247 * <p> 5248 * Description: <b>Search by requests this task is based on</b><br> 5249 * Type: <b>reference</b><br> 5250 * Path: <b>Task.basedOn</b><br> 5251 * </p> 5252 */ 5253 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5254 SP_BASED_ON); 5255 5256 /** 5257 * Constant for fluent queries to be used to add include statements. Specifies 5258 * the path value of "<b>Task:based-on</b>". 5259 */ 5260 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 5261 "Task:based-on").toLocked(); 5262 5263 /** 5264 * Search parameter: <b>patient</b> 5265 * <p> 5266 * Description: <b>Search by patient</b><br> 5267 * Type: <b>reference</b><br> 5268 * Path: <b>Task.for</b><br> 5269 * </p> 5270 */ 5271 @SearchParamDefinition(name = "patient", path = "Task.for.where(resolve() is Patient)", description = "Search by patient", type = "reference", target = { 5272 Patient.class }) 5273 public static final String SP_PATIENT = "patient"; 5274 /** 5275 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5276 * <p> 5277 * Description: <b>Search by patient</b><br> 5278 * Type: <b>reference</b><br> 5279 * Path: <b>Task.for</b><br> 5280 * </p> 5281 */ 5282 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5283 SP_PATIENT); 5284 5285 /** 5286 * Constant for fluent queries to be used to add include statements. Specifies 5287 * the path value of "<b>Task:patient</b>". 5288 */ 5289 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Task:patient") 5290 .toLocked(); 5291 5292 /** 5293 * Search parameter: <b>modified</b> 5294 * <p> 5295 * Description: <b>Search by last modification date</b><br> 5296 * Type: <b>date</b><br> 5297 * Path: <b>Task.lastModified</b><br> 5298 * </p> 5299 */ 5300 @SearchParamDefinition(name = "modified", path = "Task.lastModified", description = "Search by last modification date", type = "date") 5301 public static final String SP_MODIFIED = "modified"; 5302 /** 5303 * <b>Fluent Client</b> search parameter constant for <b>modified</b> 5304 * <p> 5305 * Description: <b>Search by last modification date</b><br> 5306 * Type: <b>date</b><br> 5307 * Path: <b>Task.lastModified</b><br> 5308 * </p> 5309 */ 5310 public static final ca.uhn.fhir.rest.gclient.DateClientParam MODIFIED = new ca.uhn.fhir.rest.gclient.DateClientParam( 5311 SP_MODIFIED); 5312 5313 /** 5314 * Search parameter: <b>status</b> 5315 * <p> 5316 * Description: <b>Search by task status</b><br> 5317 * Type: <b>token</b><br> 5318 * Path: <b>Task.status</b><br> 5319 * </p> 5320 */ 5321 @SearchParamDefinition(name = "status", path = "Task.status", description = "Search by task status", type = "token") 5322 public static final String SP_STATUS = "status"; 5323 /** 5324 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5325 * <p> 5326 * Description: <b>Search by task status</b><br> 5327 * Type: <b>token</b><br> 5328 * Path: <b>Task.status</b><br> 5329 * </p> 5330 */ 5331 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5332 SP_STATUS); 5333 5334}