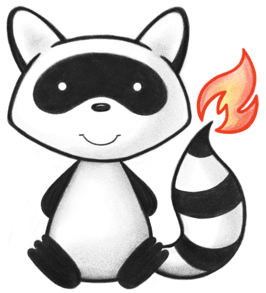
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A task to be performed. 049 */ 050@ResourceDef(name = "Task", profile = "http://hl7.org/fhir/StructureDefinition/Task") 051public class Task extends DomainResource { 052 053 public enum TaskStatus { 054 /** 055 * The task is not yet ready to be acted upon. 056 */ 057 DRAFT, 058 /** 059 * The task is ready to be acted upon and action is sought. 060 */ 061 REQUESTED, 062 /** 063 * A potential performer has claimed ownership of the task and is evaluating 064 * whether to perform it. 065 */ 066 RECEIVED, 067 /** 068 * The potential performer has agreed to execute the task but has not yet 069 * started work. 070 */ 071 ACCEPTED, 072 /** 073 * The potential performer who claimed ownership of the task has decided not to 074 * execute it prior to performing any action. 075 */ 076 REJECTED, 077 /** 078 * The task is ready to be performed, but no action has yet been taken. Used in 079 * place of requested/received/accepted/rejected when request assignment and 080 * acceptance is a given. 081 */ 082 READY, 083 /** 084 * The task was not completed. 085 */ 086 CANCELLED, 087 /** 088 * The task has been started but is not yet complete. 089 */ 090 INPROGRESS, 091 /** 092 * The task has been started but work has been paused. 093 */ 094 ONHOLD, 095 /** 096 * The task was attempted but could not be completed due to some error. 097 */ 098 FAILED, 099 /** 100 * The task has been completed. 101 */ 102 COMPLETED, 103 /** 104 * The task should never have existed and is retained only because of the 105 * possibility it may have used. 106 */ 107 ENTEREDINERROR, 108 /** 109 * added to help the parsers with the generic types 110 */ 111 NULL; 112 113 public static TaskStatus fromCode(String codeString) throws FHIRException { 114 if (codeString == null || "".equals(codeString)) 115 return null; 116 if ("draft".equals(codeString)) 117 return DRAFT; 118 if ("requested".equals(codeString)) 119 return REQUESTED; 120 if ("received".equals(codeString)) 121 return RECEIVED; 122 if ("accepted".equals(codeString)) 123 return ACCEPTED; 124 if ("rejected".equals(codeString)) 125 return REJECTED; 126 if ("ready".equals(codeString)) 127 return READY; 128 if ("cancelled".equals(codeString)) 129 return CANCELLED; 130 if ("in-progress".equals(codeString)) 131 return INPROGRESS; 132 if ("on-hold".equals(codeString)) 133 return ONHOLD; 134 if ("failed".equals(codeString)) 135 return FAILED; 136 if ("completed".equals(codeString)) 137 return COMPLETED; 138 if ("entered-in-error".equals(codeString)) 139 return ENTEREDINERROR; 140 if (Configuration.isAcceptInvalidEnums()) 141 return null; 142 else 143 throw new FHIRException("Unknown TaskStatus code '" + codeString + "'"); 144 } 145 146 public String toCode() { 147 switch (this) { 148 case DRAFT: 149 return "draft"; 150 case REQUESTED: 151 return "requested"; 152 case RECEIVED: 153 return "received"; 154 case ACCEPTED: 155 return "accepted"; 156 case REJECTED: 157 return "rejected"; 158 case READY: 159 return "ready"; 160 case CANCELLED: 161 return "cancelled"; 162 case INPROGRESS: 163 return "in-progress"; 164 case ONHOLD: 165 return "on-hold"; 166 case FAILED: 167 return "failed"; 168 case COMPLETED: 169 return "completed"; 170 case ENTEREDINERROR: 171 return "entered-in-error"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getSystem() { 180 switch (this) { 181 case DRAFT: 182 return "http://hl7.org/fhir/task-status"; 183 case REQUESTED: 184 return "http://hl7.org/fhir/task-status"; 185 case RECEIVED: 186 return "http://hl7.org/fhir/task-status"; 187 case ACCEPTED: 188 return "http://hl7.org/fhir/task-status"; 189 case REJECTED: 190 return "http://hl7.org/fhir/task-status"; 191 case READY: 192 return "http://hl7.org/fhir/task-status"; 193 case CANCELLED: 194 return "http://hl7.org/fhir/task-status"; 195 case INPROGRESS: 196 return "http://hl7.org/fhir/task-status"; 197 case ONHOLD: 198 return "http://hl7.org/fhir/task-status"; 199 case FAILED: 200 return "http://hl7.org/fhir/task-status"; 201 case COMPLETED: 202 return "http://hl7.org/fhir/task-status"; 203 case ENTEREDINERROR: 204 return "http://hl7.org/fhir/task-status"; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212 public String getDefinition() { 213 switch (this) { 214 case DRAFT: 215 return "The task is not yet ready to be acted upon."; 216 case REQUESTED: 217 return "The task is ready to be acted upon and action is sought."; 218 case RECEIVED: 219 return "A potential performer has claimed ownership of the task and is evaluating whether to perform it."; 220 case ACCEPTED: 221 return "The potential performer has agreed to execute the task but has not yet started work."; 222 case REJECTED: 223 return "The potential performer who claimed ownership of the task has decided not to execute it prior to performing any action."; 224 case READY: 225 return "The task is ready to be performed, but no action has yet been taken. Used in place of requested/received/accepted/rejected when request assignment and acceptance is a given."; 226 case CANCELLED: 227 return "The task was not completed."; 228 case INPROGRESS: 229 return "The task has been started but is not yet complete."; 230 case ONHOLD: 231 return "The task has been started but work has been paused."; 232 case FAILED: 233 return "The task was attempted but could not be completed due to some error."; 234 case COMPLETED: 235 return "The task has been completed."; 236 case ENTEREDINERROR: 237 return "The task should never have existed and is retained only because of the possibility it may have used."; 238 case NULL: 239 return null; 240 default: 241 return "?"; 242 } 243 } 244 245 public String getDisplay() { 246 switch (this) { 247 case DRAFT: 248 return "Draft"; 249 case REQUESTED: 250 return "Requested"; 251 case RECEIVED: 252 return "Received"; 253 case ACCEPTED: 254 return "Accepted"; 255 case REJECTED: 256 return "Rejected"; 257 case READY: 258 return "Ready"; 259 case CANCELLED: 260 return "Cancelled"; 261 case INPROGRESS: 262 return "In Progress"; 263 case ONHOLD: 264 return "On Hold"; 265 case FAILED: 266 return "Failed"; 267 case COMPLETED: 268 return "Completed"; 269 case ENTEREDINERROR: 270 return "Entered in Error"; 271 case NULL: 272 return null; 273 default: 274 return "?"; 275 } 276 } 277 } 278 279 public static class TaskStatusEnumFactory implements EnumFactory<TaskStatus> { 280 public TaskStatus fromCode(String codeString) throws IllegalArgumentException { 281 if (codeString == null || "".equals(codeString)) 282 if (codeString == null || "".equals(codeString)) 283 return null; 284 if ("draft".equals(codeString)) 285 return TaskStatus.DRAFT; 286 if ("requested".equals(codeString)) 287 return TaskStatus.REQUESTED; 288 if ("received".equals(codeString)) 289 return TaskStatus.RECEIVED; 290 if ("accepted".equals(codeString)) 291 return TaskStatus.ACCEPTED; 292 if ("rejected".equals(codeString)) 293 return TaskStatus.REJECTED; 294 if ("ready".equals(codeString)) 295 return TaskStatus.READY; 296 if ("cancelled".equals(codeString)) 297 return TaskStatus.CANCELLED; 298 if ("in-progress".equals(codeString)) 299 return TaskStatus.INPROGRESS; 300 if ("on-hold".equals(codeString)) 301 return TaskStatus.ONHOLD; 302 if ("failed".equals(codeString)) 303 return TaskStatus.FAILED; 304 if ("completed".equals(codeString)) 305 return TaskStatus.COMPLETED; 306 if ("entered-in-error".equals(codeString)) 307 return TaskStatus.ENTEREDINERROR; 308 throw new IllegalArgumentException("Unknown TaskStatus code '" + codeString + "'"); 309 } 310 311 public Enumeration<TaskStatus> fromType(PrimitiveType<?> code) throws FHIRException { 312 if (code == null) 313 return null; 314 if (code.isEmpty()) 315 return new Enumeration<TaskStatus>(this, TaskStatus.NULL, code); 316 String codeString = code.asStringValue(); 317 if (codeString == null || "".equals(codeString)) 318 return new Enumeration<TaskStatus>(this, TaskStatus.NULL, code); 319 if ("draft".equals(codeString)) 320 return new Enumeration<TaskStatus>(this, TaskStatus.DRAFT, code); 321 if ("requested".equals(codeString)) 322 return new Enumeration<TaskStatus>(this, TaskStatus.REQUESTED, code); 323 if ("received".equals(codeString)) 324 return new Enumeration<TaskStatus>(this, TaskStatus.RECEIVED, code); 325 if ("accepted".equals(codeString)) 326 return new Enumeration<TaskStatus>(this, TaskStatus.ACCEPTED, code); 327 if ("rejected".equals(codeString)) 328 return new Enumeration<TaskStatus>(this, TaskStatus.REJECTED, code); 329 if ("ready".equals(codeString)) 330 return new Enumeration<TaskStatus>(this, TaskStatus.READY, code); 331 if ("cancelled".equals(codeString)) 332 return new Enumeration<TaskStatus>(this, TaskStatus.CANCELLED, code); 333 if ("in-progress".equals(codeString)) 334 return new Enumeration<TaskStatus>(this, TaskStatus.INPROGRESS, code); 335 if ("on-hold".equals(codeString)) 336 return new Enumeration<TaskStatus>(this, TaskStatus.ONHOLD, code); 337 if ("failed".equals(codeString)) 338 return new Enumeration<TaskStatus>(this, TaskStatus.FAILED, code); 339 if ("completed".equals(codeString)) 340 return new Enumeration<TaskStatus>(this, TaskStatus.COMPLETED, code); 341 if ("entered-in-error".equals(codeString)) 342 return new Enumeration<TaskStatus>(this, TaskStatus.ENTEREDINERROR, code); 343 throw new FHIRException("Unknown TaskStatus code '" + codeString + "'"); 344 } 345 346 public String toCode(TaskStatus code) { 347 if (code == TaskStatus.NULL) 348 return null; 349 if (code == TaskStatus.DRAFT) 350 return "draft"; 351 if (code == TaskStatus.REQUESTED) 352 return "requested"; 353 if (code == TaskStatus.RECEIVED) 354 return "received"; 355 if (code == TaskStatus.ACCEPTED) 356 return "accepted"; 357 if (code == TaskStatus.REJECTED) 358 return "rejected"; 359 if (code == TaskStatus.READY) 360 return "ready"; 361 if (code == TaskStatus.CANCELLED) 362 return "cancelled"; 363 if (code == TaskStatus.INPROGRESS) 364 return "in-progress"; 365 if (code == TaskStatus.ONHOLD) 366 return "on-hold"; 367 if (code == TaskStatus.FAILED) 368 return "failed"; 369 if (code == TaskStatus.COMPLETED) 370 return "completed"; 371 if (code == TaskStatus.ENTEREDINERROR) 372 return "entered-in-error"; 373 return "?"; 374 } 375 376 public String toSystem(TaskStatus code) { 377 return code.getSystem(); 378 } 379 } 380 381 public enum TaskIntent { 382 /** 383 * The intent is not known. When dealing with Task, it's not always known (or 384 * relevant) how the task was initiated - i.e. whether it was proposed, planned, 385 * ordered or just done spontaneously. 386 */ 387 UNKNOWN, 388 /** 389 * null 390 */ 391 PROPOSAL, 392 /** 393 * null 394 */ 395 PLAN, 396 /** 397 * null 398 */ 399 ORDER, 400 /** 401 * null 402 */ 403 ORIGINALORDER, 404 /** 405 * null 406 */ 407 REFLEXORDER, 408 /** 409 * null 410 */ 411 FILLERORDER, 412 /** 413 * null 414 */ 415 INSTANCEORDER, 416 /** 417 * null 418 */ 419 OPTION, 420 /** 421 * added to help the parsers with the generic types 422 */ 423 NULL; 424 425 public static TaskIntent fromCode(String codeString) throws FHIRException { 426 if (codeString == null || "".equals(codeString)) 427 return null; 428 if ("unknown".equals(codeString)) 429 return UNKNOWN; 430 if ("proposal".equals(codeString)) 431 return PROPOSAL; 432 if ("plan".equals(codeString)) 433 return PLAN; 434 if ("order".equals(codeString)) 435 return ORDER; 436 if ("original-order".equals(codeString)) 437 return ORIGINALORDER; 438 if ("reflex-order".equals(codeString)) 439 return REFLEXORDER; 440 if ("filler-order".equals(codeString)) 441 return FILLERORDER; 442 if ("instance-order".equals(codeString)) 443 return INSTANCEORDER; 444 if ("option".equals(codeString)) 445 return OPTION; 446 if (Configuration.isAcceptInvalidEnums()) 447 return null; 448 else 449 throw new FHIRException("Unknown TaskIntent code '" + codeString + "'"); 450 } 451 452 public String toCode() { 453 switch (this) { 454 case UNKNOWN: 455 return "unknown"; 456 case PROPOSAL: 457 return "proposal"; 458 case PLAN: 459 return "plan"; 460 case ORDER: 461 return "order"; 462 case ORIGINALORDER: 463 return "original-order"; 464 case REFLEXORDER: 465 return "reflex-order"; 466 case FILLERORDER: 467 return "filler-order"; 468 case INSTANCEORDER: 469 return "instance-order"; 470 case OPTION: 471 return "option"; 472 case NULL: 473 return null; 474 default: 475 return "?"; 476 } 477 } 478 479 public String getSystem() { 480 switch (this) { 481 case UNKNOWN: 482 return "http://hl7.org/fhir/task-intent"; 483 case PROPOSAL: 484 return "http://hl7.org/fhir/request-intent"; 485 case PLAN: 486 return "http://hl7.org/fhir/request-intent"; 487 case ORDER: 488 return "http://hl7.org/fhir/request-intent"; 489 case ORIGINALORDER: 490 return "http://hl7.org/fhir/request-intent"; 491 case REFLEXORDER: 492 return "http://hl7.org/fhir/request-intent"; 493 case FILLERORDER: 494 return "http://hl7.org/fhir/request-intent"; 495 case INSTANCEORDER: 496 return "http://hl7.org/fhir/request-intent"; 497 case OPTION: 498 return "http://hl7.org/fhir/request-intent"; 499 case NULL: 500 return null; 501 default: 502 return "?"; 503 } 504 } 505 506 public String getDefinition() { 507 switch (this) { 508 case UNKNOWN: 509 return "The intent is not known. When dealing with Task, it's not always known (or relevant) how the task was initiated - i.e. whether it was proposed, planned, ordered or just done spontaneously."; 510 case PROPOSAL: 511 return ""; 512 case PLAN: 513 return ""; 514 case ORDER: 515 return ""; 516 case ORIGINALORDER: 517 return ""; 518 case REFLEXORDER: 519 return ""; 520 case FILLERORDER: 521 return ""; 522 case INSTANCEORDER: 523 return ""; 524 case OPTION: 525 return ""; 526 case NULL: 527 return null; 528 default: 529 return "?"; 530 } 531 } 532 533 public String getDisplay() { 534 switch (this) { 535 case UNKNOWN: 536 return "Unknown"; 537 case PROPOSAL: 538 return "proposal"; 539 case PLAN: 540 return "plan"; 541 case ORDER: 542 return "order"; 543 case ORIGINALORDER: 544 return "original-order"; 545 case REFLEXORDER: 546 return "reflex-order"; 547 case FILLERORDER: 548 return "filler-order"; 549 case INSTANCEORDER: 550 return "instance-order"; 551 case OPTION: 552 return "option"; 553 case NULL: 554 return null; 555 default: 556 return "?"; 557 } 558 } 559 } 560 561 public static class TaskIntentEnumFactory implements EnumFactory<TaskIntent> { 562 public TaskIntent fromCode(String codeString) throws IllegalArgumentException { 563 if (codeString == null || "".equals(codeString)) 564 if (codeString == null || "".equals(codeString)) 565 return null; 566 if ("unknown".equals(codeString)) 567 return TaskIntent.UNKNOWN; 568 if ("proposal".equals(codeString)) 569 return TaskIntent.PROPOSAL; 570 if ("plan".equals(codeString)) 571 return TaskIntent.PLAN; 572 if ("order".equals(codeString)) 573 return TaskIntent.ORDER; 574 if ("original-order".equals(codeString)) 575 return TaskIntent.ORIGINALORDER; 576 if ("reflex-order".equals(codeString)) 577 return TaskIntent.REFLEXORDER; 578 if ("filler-order".equals(codeString)) 579 return TaskIntent.FILLERORDER; 580 if ("instance-order".equals(codeString)) 581 return TaskIntent.INSTANCEORDER; 582 if ("option".equals(codeString)) 583 return TaskIntent.OPTION; 584 throw new IllegalArgumentException("Unknown TaskIntent code '" + codeString + "'"); 585 } 586 587 public Enumeration<TaskIntent> fromType(PrimitiveType<?> code) throws FHIRException { 588 if (code == null) 589 return null; 590 if (code.isEmpty()) 591 return new Enumeration<TaskIntent>(this, TaskIntent.NULL, code); 592 String codeString = code.asStringValue(); 593 if (codeString == null || "".equals(codeString)) 594 return new Enumeration<TaskIntent>(this, TaskIntent.NULL, code); 595 if ("unknown".equals(codeString)) 596 return new Enumeration<TaskIntent>(this, TaskIntent.UNKNOWN, code); 597 if ("proposal".equals(codeString)) 598 return new Enumeration<TaskIntent>(this, TaskIntent.PROPOSAL, code); 599 if ("plan".equals(codeString)) 600 return new Enumeration<TaskIntent>(this, TaskIntent.PLAN, code); 601 if ("order".equals(codeString)) 602 return new Enumeration<TaskIntent>(this, TaskIntent.ORDER, code); 603 if ("original-order".equals(codeString)) 604 return new Enumeration<TaskIntent>(this, TaskIntent.ORIGINALORDER, code); 605 if ("reflex-order".equals(codeString)) 606 return new Enumeration<TaskIntent>(this, TaskIntent.REFLEXORDER, code); 607 if ("filler-order".equals(codeString)) 608 return new Enumeration<TaskIntent>(this, TaskIntent.FILLERORDER, code); 609 if ("instance-order".equals(codeString)) 610 return new Enumeration<TaskIntent>(this, TaskIntent.INSTANCEORDER, code); 611 if ("option".equals(codeString)) 612 return new Enumeration<TaskIntent>(this, TaskIntent.OPTION, code); 613 throw new FHIRException("Unknown TaskIntent code '" + codeString + "'"); 614 } 615 616 public String toCode(TaskIntent code) { 617 if (code == TaskIntent.NULL) 618 return null; 619 if (code == TaskIntent.UNKNOWN) 620 return "unknown"; 621 if (code == TaskIntent.PROPOSAL) 622 return "proposal"; 623 if (code == TaskIntent.PLAN) 624 return "plan"; 625 if (code == TaskIntent.ORDER) 626 return "order"; 627 if (code == TaskIntent.ORIGINALORDER) 628 return "original-order"; 629 if (code == TaskIntent.REFLEXORDER) 630 return "reflex-order"; 631 if (code == TaskIntent.FILLERORDER) 632 return "filler-order"; 633 if (code == TaskIntent.INSTANCEORDER) 634 return "instance-order"; 635 if (code == TaskIntent.OPTION) 636 return "option"; 637 return "?"; 638 } 639 640 public String toSystem(TaskIntent code) { 641 return code.getSystem(); 642 } 643 } 644 645 public enum TaskPriority { 646 /** 647 * The request has normal priority. 648 */ 649 ROUTINE, 650 /** 651 * The request should be actioned promptly - higher priority than routine. 652 */ 653 URGENT, 654 /** 655 * The request should be actioned as soon as possible - higher priority than 656 * urgent. 657 */ 658 ASAP, 659 /** 660 * The request should be actioned immediately - highest possible priority. E.g. 661 * an emergency. 662 */ 663 STAT, 664 /** 665 * added to help the parsers with the generic types 666 */ 667 NULL; 668 669 public static TaskPriority fromCode(String codeString) throws FHIRException { 670 if (codeString == null || "".equals(codeString)) 671 return null; 672 if ("routine".equals(codeString)) 673 return ROUTINE; 674 if ("urgent".equals(codeString)) 675 return URGENT; 676 if ("asap".equals(codeString)) 677 return ASAP; 678 if ("stat".equals(codeString)) 679 return STAT; 680 if (Configuration.isAcceptInvalidEnums()) 681 return null; 682 else 683 throw new FHIRException("Unknown TaskPriority code '" + codeString + "'"); 684 } 685 686 public String toCode() { 687 switch (this) { 688 case ROUTINE: 689 return "routine"; 690 case URGENT: 691 return "urgent"; 692 case ASAP: 693 return "asap"; 694 case STAT: 695 return "stat"; 696 case NULL: 697 return null; 698 default: 699 return "?"; 700 } 701 } 702 703 public String getSystem() { 704 switch (this) { 705 case ROUTINE: 706 return "http://hl7.org/fhir/request-priority"; 707 case URGENT: 708 return "http://hl7.org/fhir/request-priority"; 709 case ASAP: 710 return "http://hl7.org/fhir/request-priority"; 711 case STAT: 712 return "http://hl7.org/fhir/request-priority"; 713 case NULL: 714 return null; 715 default: 716 return "?"; 717 } 718 } 719 720 public String getDefinition() { 721 switch (this) { 722 case ROUTINE: 723 return "The request has normal priority."; 724 case URGENT: 725 return "The request should be actioned promptly - higher priority than routine."; 726 case ASAP: 727 return "The request should be actioned as soon as possible - higher priority than urgent."; 728 case STAT: 729 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 730 case NULL: 731 return null; 732 default: 733 return "?"; 734 } 735 } 736 737 public String getDisplay() { 738 switch (this) { 739 case ROUTINE: 740 return "Routine"; 741 case URGENT: 742 return "Urgent"; 743 case ASAP: 744 return "ASAP"; 745 case STAT: 746 return "STAT"; 747 case NULL: 748 return null; 749 default: 750 return "?"; 751 } 752 } 753 } 754 755 public static class TaskPriorityEnumFactory implements EnumFactory<TaskPriority> { 756 public TaskPriority fromCode(String codeString) throws IllegalArgumentException { 757 if (codeString == null || "".equals(codeString)) 758 if (codeString == null || "".equals(codeString)) 759 return null; 760 if ("routine".equals(codeString)) 761 return TaskPriority.ROUTINE; 762 if ("urgent".equals(codeString)) 763 return TaskPriority.URGENT; 764 if ("asap".equals(codeString)) 765 return TaskPriority.ASAP; 766 if ("stat".equals(codeString)) 767 return TaskPriority.STAT; 768 throw new IllegalArgumentException("Unknown TaskPriority code '" + codeString + "'"); 769 } 770 771 public Enumeration<TaskPriority> fromType(PrimitiveType<?> code) throws FHIRException { 772 if (code == null) 773 return null; 774 if (code.isEmpty()) 775 return new Enumeration<TaskPriority>(this, TaskPriority.NULL, code); 776 String codeString = code.asStringValue(); 777 if (codeString == null || "".equals(codeString)) 778 return new Enumeration<TaskPriority>(this, TaskPriority.NULL, code); 779 if ("routine".equals(codeString)) 780 return new Enumeration<TaskPriority>(this, TaskPriority.ROUTINE, code); 781 if ("urgent".equals(codeString)) 782 return new Enumeration<TaskPriority>(this, TaskPriority.URGENT, code); 783 if ("asap".equals(codeString)) 784 return new Enumeration<TaskPriority>(this, TaskPriority.ASAP, code); 785 if ("stat".equals(codeString)) 786 return new Enumeration<TaskPriority>(this, TaskPriority.STAT, code); 787 throw new FHIRException("Unknown TaskPriority code '" + codeString + "'"); 788 } 789 790 public String toCode(TaskPriority code) { 791 if (code == TaskPriority.NULL) 792 return null; 793 if (code == TaskPriority.ROUTINE) 794 return "routine"; 795 if (code == TaskPriority.URGENT) 796 return "urgent"; 797 if (code == TaskPriority.ASAP) 798 return "asap"; 799 if (code == TaskPriority.STAT) 800 return "stat"; 801 return "?"; 802 } 803 804 public String toSystem(TaskPriority code) { 805 return code.getSystem(); 806 } 807 } 808 809 @Block() 810 public static class TaskRestrictionComponent extends BackboneElement implements IBaseBackboneElement { 811 /** 812 * Indicates the number of times the requested action should occur. 813 */ 814 @Child(name = "repetitions", type = { 815 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 816 @Description(shortDefinition = "How many times to repeat", formalDefinition = "Indicates the number of times the requested action should occur.") 817 protected PositiveIntType repetitions; 818 819 /** 820 * Over what time-period is fulfillment sought. 821 */ 822 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 823 @Description(shortDefinition = "When fulfillment sought", formalDefinition = "Over what time-period is fulfillment sought.") 824 protected Period period; 825 826 /** 827 * For requests that are targeted to more than on potential recipient/target, 828 * for whom is fulfillment sought? 829 */ 830 @Child(name = "recipient", type = { Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, 831 Group.class, 832 Organization.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 833 @Description(shortDefinition = "For whom is fulfillment sought?", formalDefinition = "For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?") 834 protected List<Reference> recipient; 835 /** 836 * The actual objects that are the target of the reference (For requests that 837 * are targeted to more than on potential recipient/target, for whom is 838 * fulfillment sought?) 839 */ 840 protected List<Resource> recipientTarget; 841 842 private static final long serialVersionUID = 1503908360L; 843 844 /** 845 * Constructor 846 */ 847 public TaskRestrictionComponent() { 848 super(); 849 } 850 851 /** 852 * @return {@link #repetitions} (Indicates the number of times the requested 853 * action should occur.). This is the underlying object with id, value 854 * and extensions. The accessor "getRepetitions" gives direct access to 855 * the value 856 */ 857 public PositiveIntType getRepetitionsElement() { 858 if (this.repetitions == null) 859 if (Configuration.errorOnAutoCreate()) 860 throw new Error("Attempt to auto-create TaskRestrictionComponent.repetitions"); 861 else if (Configuration.doAutoCreate()) 862 this.repetitions = new PositiveIntType(); // bb 863 return this.repetitions; 864 } 865 866 public boolean hasRepetitionsElement() { 867 return this.repetitions != null && !this.repetitions.isEmpty(); 868 } 869 870 public boolean hasRepetitions() { 871 return this.repetitions != null && !this.repetitions.isEmpty(); 872 } 873 874 /** 875 * @param value {@link #repetitions} (Indicates the number of times the 876 * requested action should occur.). This is the underlying object 877 * with id, value and extensions. The accessor "getRepetitions" 878 * gives direct access to the value 879 */ 880 public TaskRestrictionComponent setRepetitionsElement(PositiveIntType value) { 881 this.repetitions = value; 882 return this; 883 } 884 885 /** 886 * @return Indicates the number of times the requested action should occur. 887 */ 888 public int getRepetitions() { 889 return this.repetitions == null || this.repetitions.isEmpty() ? 0 : this.repetitions.getValue(); 890 } 891 892 /** 893 * @param value Indicates the number of times the requested action should occur. 894 */ 895 public TaskRestrictionComponent setRepetitions(int value) { 896 if (this.repetitions == null) 897 this.repetitions = new PositiveIntType(); 898 this.repetitions.setValue(value); 899 return this; 900 } 901 902 /** 903 * @return {@link #period} (Over what time-period is fulfillment sought.) 904 */ 905 public Period getPeriod() { 906 if (this.period == null) 907 if (Configuration.errorOnAutoCreate()) 908 throw new Error("Attempt to auto-create TaskRestrictionComponent.period"); 909 else if (Configuration.doAutoCreate()) 910 this.period = new Period(); // cc 911 return this.period; 912 } 913 914 public boolean hasPeriod() { 915 return this.period != null && !this.period.isEmpty(); 916 } 917 918 /** 919 * @param value {@link #period} (Over what time-period is fulfillment sought.) 920 */ 921 public TaskRestrictionComponent setPeriod(Period value) { 922 this.period = value; 923 return this; 924 } 925 926 /** 927 * @return {@link #recipient} (For requests that are targeted to more than on 928 * potential recipient/target, for whom is fulfillment sought?) 929 */ 930 public List<Reference> getRecipient() { 931 if (this.recipient == null) 932 this.recipient = new ArrayList<Reference>(); 933 return this.recipient; 934 } 935 936 /** 937 * @return Returns a reference to <code>this</code> for easy method chaining 938 */ 939 public TaskRestrictionComponent setRecipient(List<Reference> theRecipient) { 940 this.recipient = theRecipient; 941 return this; 942 } 943 944 public boolean hasRecipient() { 945 if (this.recipient == null) 946 return false; 947 for (Reference item : this.recipient) 948 if (!item.isEmpty()) 949 return true; 950 return false; 951 } 952 953 public Reference addRecipient() { // 3 954 Reference t = new Reference(); 955 if (this.recipient == null) 956 this.recipient = new ArrayList<Reference>(); 957 this.recipient.add(t); 958 return t; 959 } 960 961 public TaskRestrictionComponent addRecipient(Reference t) { // 3 962 if (t == null) 963 return this; 964 if (this.recipient == null) 965 this.recipient = new ArrayList<Reference>(); 966 this.recipient.add(t); 967 return this; 968 } 969 970 /** 971 * @return The first repetition of repeating field {@link #recipient}, creating 972 * it if it does not already exist 973 */ 974 public Reference getRecipientFirstRep() { 975 if (getRecipient().isEmpty()) { 976 addRecipient(); 977 } 978 return getRecipient().get(0); 979 } 980 981 /** 982 * @deprecated Use Reference#setResource(IBaseResource) instead 983 */ 984 @Deprecated 985 public List<Resource> getRecipientTarget() { 986 if (this.recipientTarget == null) 987 this.recipientTarget = new ArrayList<Resource>(); 988 return this.recipientTarget; 989 } 990 991 protected void listChildren(List<Property> children) { 992 super.listChildren(children); 993 children.add(new Property("repetitions", "positiveInt", 994 "Indicates the number of times the requested action should occur.", 0, 1, repetitions)); 995 children.add(new Property("period", "Period", "Over what time-period is fulfillment sought.", 0, 1, period)); 996 children.add(new Property("recipient", 997 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Group|Organization)", 998 "For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?", 999 0, java.lang.Integer.MAX_VALUE, recipient)); 1000 } 1001 1002 @Override 1003 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1004 switch (_hash) { 1005 case 984367650: 1006 /* repetitions */ return new Property("repetitions", "positiveInt", 1007 "Indicates the number of times the requested action should occur.", 0, 1, repetitions); 1008 case -991726143: 1009 /* period */ return new Property("period", "Period", "Over what time-period is fulfillment sought.", 0, 1, 1010 period); 1011 case 820081177: 1012 /* recipient */ return new Property("recipient", 1013 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Group|Organization)", 1014 "For requests that are targeted to more than on potential recipient/target, for whom is fulfillment sought?", 1015 0, java.lang.Integer.MAX_VALUE, recipient); 1016 default: 1017 return super.getNamedProperty(_hash, _name, _checkValid); 1018 } 1019 1020 } 1021 1022 @Override 1023 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1024 switch (hash) { 1025 case 984367650: 1026 /* repetitions */ return this.repetitions == null ? new Base[0] : new Base[] { this.repetitions }; // PositiveIntType 1027 case -991726143: 1028 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1029 case 820081177: 1030 /* recipient */ return this.recipient == null ? new Base[0] 1031 : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1032 default: 1033 return super.getProperty(hash, name, checkValid); 1034 } 1035 1036 } 1037 1038 @Override 1039 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1040 switch (hash) { 1041 case 984367650: // repetitions 1042 this.repetitions = castToPositiveInt(value); // PositiveIntType 1043 return value; 1044 case -991726143: // period 1045 this.period = castToPeriod(value); // Period 1046 return value; 1047 case 820081177: // recipient 1048 this.getRecipient().add(castToReference(value)); // Reference 1049 return value; 1050 default: 1051 return super.setProperty(hash, name, value); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base setProperty(String name, Base value) throws FHIRException { 1058 if (name.equals("repetitions")) { 1059 this.repetitions = castToPositiveInt(value); // PositiveIntType 1060 } else if (name.equals("period")) { 1061 this.period = castToPeriod(value); // Period 1062 } else if (name.equals("recipient")) { 1063 this.getRecipient().add(castToReference(value)); 1064 } else 1065 return super.setProperty(name, value); 1066 return value; 1067 } 1068 1069 @Override 1070 public void removeChild(String name, Base value) throws FHIRException { 1071 if (name.equals("repetitions")) { 1072 this.repetitions = null; 1073 } else if (name.equals("period")) { 1074 this.period = null; 1075 } else if (name.equals("recipient")) { 1076 this.getRecipient().remove(castToReference(value)); 1077 } else 1078 super.removeChild(name, value); 1079 1080 } 1081 1082 @Override 1083 public Base makeProperty(int hash, String name) throws FHIRException { 1084 switch (hash) { 1085 case 984367650: 1086 return getRepetitionsElement(); 1087 case -991726143: 1088 return getPeriod(); 1089 case 820081177: 1090 return addRecipient(); 1091 default: 1092 return super.makeProperty(hash, name); 1093 } 1094 1095 } 1096 1097 @Override 1098 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1099 switch (hash) { 1100 case 984367650: 1101 /* repetitions */ return new String[] { "positiveInt" }; 1102 case -991726143: 1103 /* period */ return new String[] { "Period" }; 1104 case 820081177: 1105 /* recipient */ return new String[] { "Reference" }; 1106 default: 1107 return super.getTypesForProperty(hash, name); 1108 } 1109 1110 } 1111 1112 @Override 1113 public Base addChild(String name) throws FHIRException { 1114 if (name.equals("repetitions")) { 1115 throw new FHIRException("Cannot call addChild on a singleton property Task.repetitions"); 1116 } else if (name.equals("period")) { 1117 this.period = new Period(); 1118 return this.period; 1119 } else if (name.equals("recipient")) { 1120 return addRecipient(); 1121 } else 1122 return super.addChild(name); 1123 } 1124 1125 public TaskRestrictionComponent copy() { 1126 TaskRestrictionComponent dst = new TaskRestrictionComponent(); 1127 copyValues(dst); 1128 return dst; 1129 } 1130 1131 public void copyValues(TaskRestrictionComponent dst) { 1132 super.copyValues(dst); 1133 dst.repetitions = repetitions == null ? null : repetitions.copy(); 1134 dst.period = period == null ? null : period.copy(); 1135 if (recipient != null) { 1136 dst.recipient = new ArrayList<Reference>(); 1137 for (Reference i : recipient) 1138 dst.recipient.add(i.copy()); 1139 } 1140 ; 1141 } 1142 1143 @Override 1144 public boolean equalsDeep(Base other_) { 1145 if (!super.equalsDeep(other_)) 1146 return false; 1147 if (!(other_ instanceof TaskRestrictionComponent)) 1148 return false; 1149 TaskRestrictionComponent o = (TaskRestrictionComponent) other_; 1150 return compareDeep(repetitions, o.repetitions, true) && compareDeep(period, o.period, true) 1151 && compareDeep(recipient, o.recipient, true); 1152 } 1153 1154 @Override 1155 public boolean equalsShallow(Base other_) { 1156 if (!super.equalsShallow(other_)) 1157 return false; 1158 if (!(other_ instanceof TaskRestrictionComponent)) 1159 return false; 1160 TaskRestrictionComponent o = (TaskRestrictionComponent) other_; 1161 return compareValues(repetitions, o.repetitions, true); 1162 } 1163 1164 public boolean isEmpty() { 1165 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(repetitions, period, recipient); 1166 } 1167 1168 public String fhirType() { 1169 return "Task.restriction"; 1170 1171 } 1172 1173 } 1174 1175 @Block() 1176 public static class ParameterComponent extends BackboneElement implements IBaseBackboneElement { 1177 /** 1178 * A code or description indicating how the input is intended to be used as part 1179 * of the task execution. 1180 */ 1181 @Child(name = "type", type = { 1182 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1183 @Description(shortDefinition = "Label for the input", formalDefinition = "A code or description indicating how the input is intended to be used as part of the task execution.") 1184 protected CodeableConcept type; 1185 1186 /** 1187 * The value of the input parameter as a basic type. 1188 */ 1189 @Child(name = "value", type = {}, order = 2, min = 1, max = 1, modifier = false, summary = false) 1190 @Description(shortDefinition = "Content to use in performing the task", formalDefinition = "The value of the input parameter as a basic type.") 1191 protected org.hl7.fhir.r4.model.Type value; 1192 1193 private static final long serialVersionUID = -850267045L; 1194 1195 /** 1196 * Constructor 1197 */ 1198 public ParameterComponent() { 1199 super(); 1200 } 1201 1202 /** 1203 * Constructor 1204 */ 1205 public ParameterComponent(CodeableConcept type, org.hl7.fhir.r4.model.Type value) { 1206 super(); 1207 this.type = type; 1208 this.value = value; 1209 } 1210 1211 /** 1212 * @return {@link #type} (A code or description indicating how the input is 1213 * intended to be used as part of the task execution.) 1214 */ 1215 public CodeableConcept getType() { 1216 if (this.type == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create ParameterComponent.type"); 1219 else if (Configuration.doAutoCreate()) 1220 this.type = new CodeableConcept(); // cc 1221 return this.type; 1222 } 1223 1224 public boolean hasType() { 1225 return this.type != null && !this.type.isEmpty(); 1226 } 1227 1228 /** 1229 * @param value {@link #type} (A code or description indicating how the input is 1230 * intended to be used as part of the task execution.) 1231 */ 1232 public ParameterComponent setType(CodeableConcept value) { 1233 this.type = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return {@link #value} (The value of the input parameter as a basic type.) 1239 */ 1240 public org.hl7.fhir.r4.model.Type getValue() { 1241 return this.value; 1242 } 1243 1244 public boolean hasValue() { 1245 return this.value != null && !this.value.isEmpty(); 1246 } 1247 1248 /** 1249 * @param value {@link #value} (The value of the input parameter as a basic 1250 * type.) 1251 */ 1252 public ParameterComponent setValue(org.hl7.fhir.r4.model.Type value) { 1253 this.value = value; 1254 return this; 1255 } 1256 1257 protected void listChildren(List<Property> children) { 1258 super.listChildren(children); 1259 children.add(new Property("type", "CodeableConcept", 1260 "A code or description indicating how the input is intended to be used as part of the task execution.", 0, 1, 1261 type)); 1262 children.add(new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, value)); 1263 } 1264 1265 @Override 1266 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1267 switch (_hash) { 1268 case 3575610: 1269 /* type */ return new Property("type", "CodeableConcept", 1270 "A code or description indicating how the input is intended to be used as part of the task execution.", 0, 1271 1, type); 1272 case -1410166417: 1273 /* value[x] */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1274 value); 1275 case 111972721: 1276 /* value */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1277 value); 1278 case -1535024575: 1279 /* valueBase64Binary */ return new Property("value[x]", "*", 1280 "The value of the input parameter as a basic type.", 0, 1, value); 1281 case 733421943: 1282 /* valueBoolean */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1283 1, value); 1284 case -786218365: 1285 /* valueCanonical */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1286 0, 1, value); 1287 case -766209282: 1288 /* valueCode */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1289 value); 1290 case -766192449: 1291 /* valueDate */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1292 value); 1293 case 1047929900: 1294 /* valueDateTime */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1295 1, value); 1296 case -2083993440: 1297 /* valueDecimal */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1298 1, value); 1299 case 231604844: 1300 /* valueId */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1301 value); 1302 case -1668687056: 1303 /* valueInstant */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1304 1, value); 1305 case -1668204915: 1306 /* valueInteger */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1307 1, value); 1308 case -497880704: 1309 /* valueMarkdown */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1310 1, value); 1311 case -1410178407: 1312 /* valueOid */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1313 value); 1314 case -1249932027: 1315 /* valuePositiveInt */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1316 0, 1, value); 1317 case -1424603934: 1318 /* valueString */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1319 1, value); 1320 case -765708322: 1321 /* valueTime */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1322 value); 1323 case 26529417: 1324 /* valueUnsignedInt */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1325 0, 1, value); 1326 case -1410172357: 1327 /* valueUri */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1328 value); 1329 case -1410172354: 1330 /* valueUrl */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1331 value); 1332 case -765667124: 1333 /* valueUuid */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1334 value); 1335 case -478981821: 1336 /* valueAddress */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1337 1, value); 1338 case -67108992: 1339 /* valueAnnotation */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1340 0, 1, value); 1341 case -475566732: 1342 /* valueAttachment */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1343 0, 1, value); 1344 case 924902896: 1345 /* valueCodeableConcept */ return new Property("value[x]", "*", 1346 "The value of the input parameter as a basic type.", 0, 1, value); 1347 case -1887705029: 1348 /* valueCoding */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1349 1, value); 1350 case 944904545: 1351 /* valueContactPoint */ return new Property("value[x]", "*", 1352 "The value of the input parameter as a basic type.", 0, 1, value); 1353 case -2026205465: 1354 /* valueHumanName */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1355 0, 1, value); 1356 case -130498310: 1357 /* valueIdentifier */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1358 0, 1, value); 1359 case -1524344174: 1360 /* valuePeriod */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1361 1, value); 1362 case -2029823716: 1363 /* valueQuantity */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1364 1, value); 1365 case 2030761548: 1366 /* valueRange */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1367 value); 1368 case 2030767386: 1369 /* valueRatio */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1, 1370 value); 1371 case 1755241690: 1372 /* valueReference */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1373 0, 1, value); 1374 case -962229101: 1375 /* valueSampledData */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1376 0, 1, value); 1377 case -540985785: 1378 /* valueSignature */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 1379 0, 1, value); 1380 case -1406282469: 1381 /* valueTiming */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1382 1, value); 1383 case -1858636920: 1384 /* valueDosage */ return new Property("value[x]", "*", "The value of the input parameter as a basic type.", 0, 1385 1, value); 1386 default: 1387 return super.getNamedProperty(_hash, _name, _checkValid); 1388 } 1389 1390 } 1391 1392 @Override 1393 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1394 switch (hash) { 1395 case 3575610: 1396 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1397 case 111972721: 1398 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // org.hl7.fhir.r4.model.Type 1399 default: 1400 return super.getProperty(hash, name, checkValid); 1401 } 1402 1403 } 1404 1405 @Override 1406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1407 switch (hash) { 1408 case 3575610: // type 1409 this.type = castToCodeableConcept(value); // CodeableConcept 1410 return value; 1411 case 111972721: // value 1412 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 1413 return value; 1414 default: 1415 return super.setProperty(hash, name, value); 1416 } 1417 1418 } 1419 1420 @Override 1421 public Base setProperty(String name, Base value) throws FHIRException { 1422 if (name.equals("type")) { 1423 this.type = castToCodeableConcept(value); // CodeableConcept 1424 } else if (name.equals("value[x]")) { 1425 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 1426 } else 1427 return super.setProperty(name, value); 1428 return value; 1429 } 1430 1431 @Override 1432 public void removeChild(String name, Base value) throws FHIRException { 1433 if (name.equals("type")) { 1434 this.type = null; 1435 } else if (name.equals("value[x]")) { 1436 this.value = null; 1437 } else 1438 super.removeChild(name, value); 1439 1440 } 1441 1442 @Override 1443 public Base makeProperty(int hash, String name) throws FHIRException { 1444 switch (hash) { 1445 case 3575610: 1446 return getType(); 1447 case -1410166417: 1448 return getValue(); 1449 case 111972721: 1450 return getValue(); 1451 default: 1452 return super.makeProperty(hash, name); 1453 } 1454 1455 } 1456 1457 @Override 1458 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1459 switch (hash) { 1460 case 3575610: 1461 /* type */ return new String[] { "CodeableConcept" }; 1462 case 111972721: 1463 /* value */ return new String[] { "*" }; 1464 default: 1465 return super.getTypesForProperty(hash, name); 1466 } 1467 1468 } 1469 1470 @Override 1471 public Base addChild(String name) throws FHIRException { 1472 if (name.equals("type")) { 1473 this.type = new CodeableConcept(); 1474 return this.type; 1475 } else if (name.equals("valueBase64Binary")) { 1476 this.value = new Base64BinaryType(); 1477 return this.value; 1478 } else if (name.equals("valueBoolean")) { 1479 this.value = new BooleanType(); 1480 return this.value; 1481 } else if (name.equals("valueCanonical")) { 1482 this.value = new CanonicalType(); 1483 return this.value; 1484 } else if (name.equals("valueCode")) { 1485 this.value = new CodeType(); 1486 return this.value; 1487 } else if (name.equals("valueDate")) { 1488 this.value = new DateType(); 1489 return this.value; 1490 } else if (name.equals("valueDateTime")) { 1491 this.value = new DateTimeType(); 1492 return this.value; 1493 } else if (name.equals("valueDecimal")) { 1494 this.value = new DecimalType(); 1495 return this.value; 1496 } else if (name.equals("valueId")) { 1497 this.value = new IdType(); 1498 return this.value; 1499 } else if (name.equals("valueInstant")) { 1500 this.value = new InstantType(); 1501 return this.value; 1502 } else if (name.equals("valueInteger")) { 1503 this.value = new IntegerType(); 1504 return this.value; 1505 } else if (name.equals("valueMarkdown")) { 1506 this.value = new MarkdownType(); 1507 return this.value; 1508 } else if (name.equals("valueOid")) { 1509 this.value = new OidType(); 1510 return this.value; 1511 } else if (name.equals("valuePositiveInt")) { 1512 this.value = new PositiveIntType(); 1513 return this.value; 1514 } else if (name.equals("valueString")) { 1515 this.value = new StringType(); 1516 return this.value; 1517 } else if (name.equals("valueTime")) { 1518 this.value = new TimeType(); 1519 return this.value; 1520 } else if (name.equals("valueUnsignedInt")) { 1521 this.value = new UnsignedIntType(); 1522 return this.value; 1523 } else if (name.equals("valueUri")) { 1524 this.value = new UriType(); 1525 return this.value; 1526 } else if (name.equals("valueUrl")) { 1527 this.value = new UrlType(); 1528 return this.value; 1529 } else if (name.equals("valueUuid")) { 1530 this.value = new UuidType(); 1531 return this.value; 1532 } else if (name.equals("valueAddress")) { 1533 this.value = new Address(); 1534 return this.value; 1535 } else if (name.equals("valueAge")) { 1536 this.value = new Age(); 1537 return this.value; 1538 } else if (name.equals("valueAnnotation")) { 1539 this.value = new Annotation(); 1540 return this.value; 1541 } else if (name.equals("valueAttachment")) { 1542 this.value = new Attachment(); 1543 return this.value; 1544 } else if (name.equals("valueCodeableConcept")) { 1545 this.value = new CodeableConcept(); 1546 return this.value; 1547 } else if (name.equals("valueCoding")) { 1548 this.value = new Coding(); 1549 return this.value; 1550 } else if (name.equals("valueContactPoint")) { 1551 this.value = new ContactPoint(); 1552 return this.value; 1553 } else if (name.equals("valueCount")) { 1554 this.value = new Count(); 1555 return this.value; 1556 } else if (name.equals("valueDistance")) { 1557 this.value = new Distance(); 1558 return this.value; 1559 } else if (name.equals("valueDuration")) { 1560 this.value = new Duration(); 1561 return this.value; 1562 } else if (name.equals("valueHumanName")) { 1563 this.value = new HumanName(); 1564 return this.value; 1565 } else if (name.equals("valueIdentifier")) { 1566 this.value = new Identifier(); 1567 return this.value; 1568 } else if (name.equals("valueMoney")) { 1569 this.value = new Money(); 1570 return this.value; 1571 } else if (name.equals("valuePeriod")) { 1572 this.value = new Period(); 1573 return this.value; 1574 } else if (name.equals("valueQuantity")) { 1575 this.value = new Quantity(); 1576 return this.value; 1577 } else if (name.equals("valueRange")) { 1578 this.value = new Range(); 1579 return this.value; 1580 } else if (name.equals("valueRatio")) { 1581 this.value = new Ratio(); 1582 return this.value; 1583 } else if (name.equals("valueReference")) { 1584 this.value = new Reference(); 1585 return this.value; 1586 } else if (name.equals("valueSampledData")) { 1587 this.value = new SampledData(); 1588 return this.value; 1589 } else if (name.equals("valueSignature")) { 1590 this.value = new Signature(); 1591 return this.value; 1592 } else if (name.equals("valueTiming")) { 1593 this.value = new Timing(); 1594 return this.value; 1595 } else if (name.equals("valueContactDetail")) { 1596 this.value = new ContactDetail(); 1597 return this.value; 1598 } else if (name.equals("valueContributor")) { 1599 this.value = new Contributor(); 1600 return this.value; 1601 } else if (name.equals("valueDataRequirement")) { 1602 this.value = new DataRequirement(); 1603 return this.value; 1604 } else if (name.equals("valueExpression")) { 1605 this.value = new Expression(); 1606 return this.value; 1607 } else if (name.equals("valueParameterDefinition")) { 1608 this.value = new ParameterDefinition(); 1609 return this.value; 1610 } else if (name.equals("valueRelatedArtifact")) { 1611 this.value = new RelatedArtifact(); 1612 return this.value; 1613 } else if (name.equals("valueTriggerDefinition")) { 1614 this.value = new TriggerDefinition(); 1615 return this.value; 1616 } else if (name.equals("valueUsageContext")) { 1617 this.value = new UsageContext(); 1618 return this.value; 1619 } else if (name.equals("valueDosage")) { 1620 this.value = new Dosage(); 1621 return this.value; 1622 } else if (name.equals("valueMeta")) { 1623 this.value = new Meta(); 1624 return this.value; 1625 } else 1626 return super.addChild(name); 1627 } 1628 1629 public ParameterComponent copy() { 1630 ParameterComponent dst = new ParameterComponent(); 1631 copyValues(dst); 1632 return dst; 1633 } 1634 1635 public void copyValues(ParameterComponent dst) { 1636 super.copyValues(dst); 1637 dst.type = type == null ? null : type.copy(); 1638 dst.value = value == null ? null : value.copy(); 1639 } 1640 1641 @Override 1642 public boolean equalsDeep(Base other_) { 1643 if (!super.equalsDeep(other_)) 1644 return false; 1645 if (!(other_ instanceof ParameterComponent)) 1646 return false; 1647 ParameterComponent o = (ParameterComponent) other_; 1648 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 1649 } 1650 1651 @Override 1652 public boolean equalsShallow(Base other_) { 1653 if (!super.equalsShallow(other_)) 1654 return false; 1655 if (!(other_ instanceof ParameterComponent)) 1656 return false; 1657 ParameterComponent o = (ParameterComponent) other_; 1658 return true; 1659 } 1660 1661 public boolean isEmpty() { 1662 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 1663 } 1664 1665 public String fhirType() { 1666 return "Task.input"; 1667 1668 } 1669 1670 } 1671 1672 @Block() 1673 public static class TaskOutputComponent extends BackboneElement implements IBaseBackboneElement { 1674 /** 1675 * The name of the Output parameter. 1676 */ 1677 @Child(name = "type", type = { 1678 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1679 @Description(shortDefinition = "Label for output", formalDefinition = "The name of the Output parameter.") 1680 protected CodeableConcept type; 1681 1682 /** 1683 * The value of the Output parameter as a basic type. 1684 */ 1685 @Child(name = "value", type = {}, order = 2, min = 1, max = 1, modifier = false, summary = false) 1686 @Description(shortDefinition = "Result of output", formalDefinition = "The value of the Output parameter as a basic type.") 1687 protected org.hl7.fhir.r4.model.Type value; 1688 1689 private static final long serialVersionUID = -850267045L; 1690 1691 /** 1692 * Constructor 1693 */ 1694 public TaskOutputComponent() { 1695 super(); 1696 } 1697 1698 /** 1699 * Constructor 1700 */ 1701 public TaskOutputComponent(CodeableConcept type, org.hl7.fhir.r4.model.Type value) { 1702 super(); 1703 this.type = type; 1704 this.value = value; 1705 } 1706 1707 /** 1708 * @return {@link #type} (The name of the Output parameter.) 1709 */ 1710 public CodeableConcept getType() { 1711 if (this.type == null) 1712 if (Configuration.errorOnAutoCreate()) 1713 throw new Error("Attempt to auto-create TaskOutputComponent.type"); 1714 else if (Configuration.doAutoCreate()) 1715 this.type = new CodeableConcept(); // cc 1716 return this.type; 1717 } 1718 1719 public boolean hasType() { 1720 return this.type != null && !this.type.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #type} (The name of the Output parameter.) 1725 */ 1726 public TaskOutputComponent setType(CodeableConcept value) { 1727 this.type = value; 1728 return this; 1729 } 1730 1731 /** 1732 * @return {@link #value} (The value of the Output parameter as a basic type.) 1733 */ 1734 public org.hl7.fhir.r4.model.Type getValue() { 1735 return this.value; 1736 } 1737 1738 public boolean hasValue() { 1739 return this.value != null && !this.value.isEmpty(); 1740 } 1741 1742 /** 1743 * @param value {@link #value} (The value of the Output parameter as a basic 1744 * type.) 1745 */ 1746 public TaskOutputComponent setValue(org.hl7.fhir.r4.model.Type value) { 1747 this.value = value; 1748 return this; 1749 } 1750 1751 protected void listChildren(List<Property> children) { 1752 super.listChildren(children); 1753 children.add(new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type)); 1754 children.add(new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, value)); 1755 } 1756 1757 @Override 1758 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1759 switch (_hash) { 1760 case 3575610: 1761 /* type */ return new Property("type", "CodeableConcept", "The name of the Output parameter.", 0, 1, type); 1762 case -1410166417: 1763 /* value[x] */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1764 value); 1765 case 111972721: 1766 /* value */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1767 value); 1768 case -1535024575: 1769 /* valueBase64Binary */ return new Property("value[x]", "*", 1770 "The value of the Output parameter as a basic type.", 0, 1, value); 1771 case 733421943: 1772 /* valueBoolean */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1773 1, value); 1774 case -786218365: 1775 /* valueCanonical */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1776 0, 1, value); 1777 case -766209282: 1778 /* valueCode */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1779 value); 1780 case -766192449: 1781 /* valueDate */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1782 value); 1783 case 1047929900: 1784 /* valueDateTime */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1785 0, 1, value); 1786 case -2083993440: 1787 /* valueDecimal */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1788 1, value); 1789 case 231604844: 1790 /* valueId */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1791 value); 1792 case -1668687056: 1793 /* valueInstant */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1794 1, value); 1795 case -1668204915: 1796 /* valueInteger */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1797 1, value); 1798 case -497880704: 1799 /* valueMarkdown */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1800 0, 1, value); 1801 case -1410178407: 1802 /* valueOid */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1803 value); 1804 case -1249932027: 1805 /* valuePositiveInt */ return new Property("value[x]", "*", 1806 "The value of the Output parameter as a basic type.", 0, 1, value); 1807 case -1424603934: 1808 /* valueString */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1809 1, value); 1810 case -765708322: 1811 /* valueTime */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1812 value); 1813 case 26529417: 1814 /* valueUnsignedInt */ return new Property("value[x]", "*", 1815 "The value of the Output parameter as a basic type.", 0, 1, value); 1816 case -1410172357: 1817 /* valueUri */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1818 value); 1819 case -1410172354: 1820 /* valueUrl */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1821 value); 1822 case -765667124: 1823 /* valueUuid */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1, 1824 value); 1825 case -478981821: 1826 /* valueAddress */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1827 1, value); 1828 case -67108992: 1829 /* valueAnnotation */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1830 0, 1, value); 1831 case -475566732: 1832 /* valueAttachment */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1833 0, 1, value); 1834 case 924902896: 1835 /* valueCodeableConcept */ return new Property("value[x]", "*", 1836 "The value of the Output parameter as a basic type.", 0, 1, value); 1837 case -1887705029: 1838 /* valueCoding */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1839 1, value); 1840 case 944904545: 1841 /* valueContactPoint */ return new Property("value[x]", "*", 1842 "The value of the Output parameter as a basic type.", 0, 1, value); 1843 case -2026205465: 1844 /* valueHumanName */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1845 0, 1, value); 1846 case -130498310: 1847 /* valueIdentifier */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1848 0, 1, value); 1849 case -1524344174: 1850 /* valuePeriod */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1851 1, value); 1852 case -2029823716: 1853 /* valueQuantity */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1854 0, 1, value); 1855 case 2030761548: 1856 /* valueRange */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1857 1, value); 1858 case 2030767386: 1859 /* valueRatio */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1860 1, value); 1861 case 1755241690: 1862 /* valueReference */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1863 0, 1, value); 1864 case -962229101: 1865 /* valueSampledData */ return new Property("value[x]", "*", 1866 "The value of the Output parameter as a basic type.", 0, 1, value); 1867 case -540985785: 1868 /* valueSignature */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 1869 0, 1, value); 1870 case -1406282469: 1871 /* valueTiming */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1872 1, value); 1873 case -1858636920: 1874 /* valueDosage */ return new Property("value[x]", "*", "The value of the Output parameter as a basic type.", 0, 1875 1, value); 1876 default: 1877 return super.getNamedProperty(_hash, _name, _checkValid); 1878 } 1879 1880 } 1881 1882 @Override 1883 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1884 switch (hash) { 1885 case 3575610: 1886 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1887 case 111972721: 1888 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // org.hl7.fhir.r4.model.Type 1889 default: 1890 return super.getProperty(hash, name, checkValid); 1891 } 1892 1893 } 1894 1895 @Override 1896 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1897 switch (hash) { 1898 case 3575610: // type 1899 this.type = castToCodeableConcept(value); // CodeableConcept 1900 return value; 1901 case 111972721: // value 1902 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 1903 return value; 1904 default: 1905 return super.setProperty(hash, name, value); 1906 } 1907 1908 } 1909 1910 @Override 1911 public Base setProperty(String name, Base value) throws FHIRException { 1912 if (name.equals("type")) { 1913 this.type = castToCodeableConcept(value); // CodeableConcept 1914 } else if (name.equals("value[x]")) { 1915 this.value = castToType(value); // org.hl7.fhir.r4.model.Type 1916 } else 1917 return super.setProperty(name, value); 1918 return value; 1919 } 1920 1921 @Override 1922 public void removeChild(String name, Base value) throws FHIRException { 1923 if (name.equals("type")) { 1924 this.type = null; 1925 } else if (name.equals("value[x]")) { 1926 this.value = null; 1927 } else 1928 super.removeChild(name, value); 1929 1930 } 1931 1932 @Override 1933 public Base makeProperty(int hash, String name) throws FHIRException { 1934 switch (hash) { 1935 case 3575610: 1936 return getType(); 1937 case -1410166417: 1938 return getValue(); 1939 case 111972721: 1940 return getValue(); 1941 default: 1942 return super.makeProperty(hash, name); 1943 } 1944 1945 } 1946 1947 @Override 1948 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1949 switch (hash) { 1950 case 3575610: 1951 /* type */ return new String[] { "CodeableConcept" }; 1952 case 111972721: 1953 /* value */ return new String[] { "*" }; 1954 default: 1955 return super.getTypesForProperty(hash, name); 1956 } 1957 1958 } 1959 1960 @Override 1961 public Base addChild(String name) throws FHIRException { 1962 if (name.equals("type")) { 1963 this.type = new CodeableConcept(); 1964 return this.type; 1965 } else if (name.equals("valueBase64Binary")) { 1966 this.value = new Base64BinaryType(); 1967 return this.value; 1968 } else if (name.equals("valueBoolean")) { 1969 this.value = new BooleanType(); 1970 return this.value; 1971 } else if (name.equals("valueCanonical")) { 1972 this.value = new CanonicalType(); 1973 return this.value; 1974 } else if (name.equals("valueCode")) { 1975 this.value = new CodeType(); 1976 return this.value; 1977 } else if (name.equals("valueDate")) { 1978 this.value = new DateType(); 1979 return this.value; 1980 } else if (name.equals("valueDateTime")) { 1981 this.value = new DateTimeType(); 1982 return this.value; 1983 } else if (name.equals("valueDecimal")) { 1984 this.value = new DecimalType(); 1985 return this.value; 1986 } else if (name.equals("valueId")) { 1987 this.value = new IdType(); 1988 return this.value; 1989 } else if (name.equals("valueInstant")) { 1990 this.value = new InstantType(); 1991 return this.value; 1992 } else if (name.equals("valueInteger")) { 1993 this.value = new IntegerType(); 1994 return this.value; 1995 } else if (name.equals("valueMarkdown")) { 1996 this.value = new MarkdownType(); 1997 return this.value; 1998 } else if (name.equals("valueOid")) { 1999 this.value = new OidType(); 2000 return this.value; 2001 } else if (name.equals("valuePositiveInt")) { 2002 this.value = new PositiveIntType(); 2003 return this.value; 2004 } else if (name.equals("valueString")) { 2005 this.value = new StringType(); 2006 return this.value; 2007 } else if (name.equals("valueTime")) { 2008 this.value = new TimeType(); 2009 return this.value; 2010 } else if (name.equals("valueUnsignedInt")) { 2011 this.value = new UnsignedIntType(); 2012 return this.value; 2013 } else if (name.equals("valueUri")) { 2014 this.value = new UriType(); 2015 return this.value; 2016 } else if (name.equals("valueUrl")) { 2017 this.value = new UrlType(); 2018 return this.value; 2019 } else if (name.equals("valueUuid")) { 2020 this.value = new UuidType(); 2021 return this.value; 2022 } else if (name.equals("valueAddress")) { 2023 this.value = new Address(); 2024 return this.value; 2025 } else if (name.equals("valueAge")) { 2026 this.value = new Age(); 2027 return this.value; 2028 } else if (name.equals("valueAnnotation")) { 2029 this.value = new Annotation(); 2030 return this.value; 2031 } else if (name.equals("valueAttachment")) { 2032 this.value = new Attachment(); 2033 return this.value; 2034 } else if (name.equals("valueCodeableConcept")) { 2035 this.value = new CodeableConcept(); 2036 return this.value; 2037 } else if (name.equals("valueCoding")) { 2038 this.value = new Coding(); 2039 return this.value; 2040 } else if (name.equals("valueContactPoint")) { 2041 this.value = new ContactPoint(); 2042 return this.value; 2043 } else if (name.equals("valueCount")) { 2044 this.value = new Count(); 2045 return this.value; 2046 } else if (name.equals("valueDistance")) { 2047 this.value = new Distance(); 2048 return this.value; 2049 } else if (name.equals("valueDuration")) { 2050 this.value = new Duration(); 2051 return this.value; 2052 } else if (name.equals("valueHumanName")) { 2053 this.value = new HumanName(); 2054 return this.value; 2055 } else if (name.equals("valueIdentifier")) { 2056 this.value = new Identifier(); 2057 return this.value; 2058 } else if (name.equals("valueMoney")) { 2059 this.value = new Money(); 2060 return this.value; 2061 } else if (name.equals("valuePeriod")) { 2062 this.value = new Period(); 2063 return this.value; 2064 } else if (name.equals("valueQuantity")) { 2065 this.value = new Quantity(); 2066 return this.value; 2067 } else if (name.equals("valueRange")) { 2068 this.value = new Range(); 2069 return this.value; 2070 } else if (name.equals("valueRatio")) { 2071 this.value = new Ratio(); 2072 return this.value; 2073 } else if (name.equals("valueReference")) { 2074 this.value = new Reference(); 2075 return this.value; 2076 } else if (name.equals("valueSampledData")) { 2077 this.value = new SampledData(); 2078 return this.value; 2079 } else if (name.equals("valueSignature")) { 2080 this.value = new Signature(); 2081 return this.value; 2082 } else if (name.equals("valueTiming")) { 2083 this.value = new Timing(); 2084 return this.value; 2085 } else if (name.equals("valueContactDetail")) { 2086 this.value = new ContactDetail(); 2087 return this.value; 2088 } else if (name.equals("valueContributor")) { 2089 this.value = new Contributor(); 2090 return this.value; 2091 } else if (name.equals("valueDataRequirement")) { 2092 this.value = new DataRequirement(); 2093 return this.value; 2094 } else if (name.equals("valueExpression")) { 2095 this.value = new Expression(); 2096 return this.value; 2097 } else if (name.equals("valueParameterDefinition")) { 2098 this.value = new ParameterDefinition(); 2099 return this.value; 2100 } else if (name.equals("valueRelatedArtifact")) { 2101 this.value = new RelatedArtifact(); 2102 return this.value; 2103 } else if (name.equals("valueTriggerDefinition")) { 2104 this.value = new TriggerDefinition(); 2105 return this.value; 2106 } else if (name.equals("valueUsageContext")) { 2107 this.value = new UsageContext(); 2108 return this.value; 2109 } else if (name.equals("valueDosage")) { 2110 this.value = new Dosage(); 2111 return this.value; 2112 } else if (name.equals("valueMeta")) { 2113 this.value = new Meta(); 2114 return this.value; 2115 } else 2116 return super.addChild(name); 2117 } 2118 2119 public TaskOutputComponent copy() { 2120 TaskOutputComponent dst = new TaskOutputComponent(); 2121 copyValues(dst); 2122 return dst; 2123 } 2124 2125 public void copyValues(TaskOutputComponent dst) { 2126 super.copyValues(dst); 2127 dst.type = type == null ? null : type.copy(); 2128 dst.value = value == null ? null : value.copy(); 2129 } 2130 2131 @Override 2132 public boolean equalsDeep(Base other_) { 2133 if (!super.equalsDeep(other_)) 2134 return false; 2135 if (!(other_ instanceof TaskOutputComponent)) 2136 return false; 2137 TaskOutputComponent o = (TaskOutputComponent) other_; 2138 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2139 } 2140 2141 @Override 2142 public boolean equalsShallow(Base other_) { 2143 if (!super.equalsShallow(other_)) 2144 return false; 2145 if (!(other_ instanceof TaskOutputComponent)) 2146 return false; 2147 TaskOutputComponent o = (TaskOutputComponent) other_; 2148 return true; 2149 } 2150 2151 public boolean isEmpty() { 2152 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2153 } 2154 2155 public String fhirType() { 2156 return "Task.output"; 2157 2158 } 2159 2160 } 2161 2162 /** 2163 * The business identifier for this task. 2164 */ 2165 @Child(name = "identifier", type = { 2166 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2167 @Description(shortDefinition = "Task Instance Identifier", formalDefinition = "The business identifier for this task.") 2168 protected List<Identifier> identifier; 2169 2170 /** 2171 * The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other 2172 * definition that is adhered to in whole or in part by this Task. 2173 */ 2174 @Child(name = "instantiatesCanonical", type = { 2175 CanonicalType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2176 @Description(shortDefinition = "Formal definition of task", formalDefinition = "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.") 2177 protected CanonicalType instantiatesCanonical; 2178 2179 /** 2180 * The URL pointing to an *externally* maintained protocol, guideline, orderset 2181 * or other definition that is adhered to in whole or in part by this Task. 2182 */ 2183 @Child(name = "instantiatesUri", type = { 2184 UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2185 @Description(shortDefinition = "Formal definition of task", formalDefinition = "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.") 2186 protected UriType instantiatesUri; 2187 2188 /** 2189 * BasedOn refers to a higher-level authorization that triggered the creation of 2190 * the task. It references a "request" resource such as a ServiceRequest, 2191 * MedicationRequest, ServiceRequest, CarePlan, etc. which is distinct from the 2192 * "request" resource the task is seeking to fulfill. This latter resource is 2193 * referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a 2194 * task is created to fulfill a procedureRequest ( = FocusOn ) to collect a 2195 * specimen from a patient. 2196 */ 2197 @Child(name = "basedOn", type = { 2198 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2199 @Description(shortDefinition = "Request fulfilled by this task", formalDefinition = "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, ServiceRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a task is created to fulfill a procedureRequest ( = FocusOn ) to collect a specimen from a patient.") 2200 protected List<Reference> basedOn; 2201 /** 2202 * The actual objects that are the target of the reference (BasedOn refers to a 2203 * higher-level authorization that triggered the creation of the task. It 2204 * references a "request" resource such as a ServiceRequest, MedicationRequest, 2205 * ServiceRequest, CarePlan, etc. which is distinct from the "request" resource 2206 * the task is seeking to fulfill. This latter resource is referenced by 2207 * FocusOn. For example, based on a ServiceRequest (= BasedOn), a task is 2208 * created to fulfill a procedureRequest ( = FocusOn ) to collect a specimen 2209 * from a patient.) 2210 */ 2211 protected List<Resource> basedOnTarget; 2212 2213 /** 2214 * An identifier that links together multiple tasks and other requests that were 2215 * created in the same context. 2216 */ 2217 @Child(name = "groupIdentifier", type = { 2218 Identifier.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2219 @Description(shortDefinition = "Requisition or grouper id", formalDefinition = "An identifier that links together multiple tasks and other requests that were created in the same context.") 2220 protected Identifier groupIdentifier; 2221 2222 /** 2223 * Task that this particular task is part of. 2224 */ 2225 @Child(name = "partOf", type = { 2226 Task.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2227 @Description(shortDefinition = "Composite task", formalDefinition = "Task that this particular task is part of.") 2228 protected List<Reference> partOf; 2229 /** 2230 * The actual objects that are the target of the reference (Task that this 2231 * particular task is part of.) 2232 */ 2233 protected List<Task> partOfTarget; 2234 2235 /** 2236 * The current status of the task. 2237 */ 2238 @Child(name = "status", type = { CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 2239 @Description(shortDefinition = "draft | requested | received | accepted | +", formalDefinition = "The current status of the task.") 2240 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/task-status") 2241 protected Enumeration<TaskStatus> status; 2242 2243 /** 2244 * An explanation as to why this task is held, failed, was refused, etc. 2245 */ 2246 @Child(name = "statusReason", type = { 2247 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2248 @Description(shortDefinition = "Reason for current status", formalDefinition = "An explanation as to why this task is held, failed, was refused, etc.") 2249 protected CodeableConcept statusReason; 2250 2251 /** 2252 * Contains business-specific nuances of the business state. 2253 */ 2254 @Child(name = "businessStatus", type = { 2255 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2256 @Description(shortDefinition = "E.g. \"Specimen collected\", \"IV prepped\"", formalDefinition = "Contains business-specific nuances of the business state.") 2257 protected CodeableConcept businessStatus; 2258 2259 /** 2260 * Indicates the "level" of actionability associated with the Task, i.e. 2261 * i+R[9]Cs this a proposed task, a planned task, an actionable task, etc. 2262 */ 2263 @Child(name = "intent", type = { CodeType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 2264 @Description(shortDefinition = "unknown | proposal | plan | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.") 2265 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/task-intent") 2266 protected Enumeration<TaskIntent> intent; 2267 2268 /** 2269 * Indicates how quickly the Task should be addressed with respect to other 2270 * requests. 2271 */ 2272 @Child(name = "priority", type = { CodeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2273 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the Task should be addressed with respect to other requests.") 2274 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 2275 protected Enumeration<TaskPriority> priority; 2276 2277 /** 2278 * A name or code (or both) briefly describing what the task involves. 2279 */ 2280 @Child(name = "code", type = { 2281 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 2282 @Description(shortDefinition = "Task Type", formalDefinition = "A name or code (or both) briefly describing what the task involves.") 2283 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/task-code") 2284 protected CodeableConcept code; 2285 2286 /** 2287 * A free-text description of what is to be performed. 2288 */ 2289 @Child(name = "description", type = { 2290 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 2291 @Description(shortDefinition = "Human-readable explanation of task", formalDefinition = "A free-text description of what is to be performed.") 2292 protected StringType description; 2293 2294 /** 2295 * The request being actioned or the resource being manipulated by this task. 2296 */ 2297 @Child(name = "focus", type = { Reference.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 2298 @Description(shortDefinition = "What task is acting on", formalDefinition = "The request being actioned or the resource being manipulated by this task.") 2299 protected Reference focus; 2300 2301 /** 2302 * The actual object that is the target of the reference (The request being 2303 * actioned or the resource being manipulated by this task.) 2304 */ 2305 protected Resource focusTarget; 2306 2307 /** 2308 * The entity who benefits from the performance of the service specified in the 2309 * task (e.g., the patient). 2310 */ 2311 @Child(name = "for", type = { Reference.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 2312 @Description(shortDefinition = "Beneficiary of the Task", formalDefinition = "The entity who benefits from the performance of the service specified in the task (e.g., the patient).") 2313 protected Reference for_; 2314 2315 /** 2316 * The actual object that is the target of the reference (The entity who 2317 * benefits from the performance of the service specified in the task (e.g., the 2318 * patient).) 2319 */ 2320 protected Resource for_Target; 2321 2322 /** 2323 * The healthcare event (e.g. a patient and healthcare provider interaction) 2324 * during which this task was created. 2325 */ 2326 @Child(name = "encounter", type = { Encounter.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 2327 @Description(shortDefinition = "Healthcare event during which this task originated", formalDefinition = "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.") 2328 protected Reference encounter; 2329 2330 /** 2331 * The actual object that is the target of the reference (The healthcare event 2332 * (e.g. a patient and healthcare provider interaction) during which this task 2333 * was created.) 2334 */ 2335 protected Encounter encounterTarget; 2336 2337 /** 2338 * Identifies the time action was first taken against the task (start) and/or 2339 * the time final action was taken against the task prior to marking it as 2340 * completed (end). 2341 */ 2342 @Child(name = "executionPeriod", type = { 2343 Period.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 2344 @Description(shortDefinition = "Start and end time of execution", formalDefinition = "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).") 2345 protected Period executionPeriod; 2346 2347 /** 2348 * The date and time this task was created. 2349 */ 2350 @Child(name = "authoredOn", type = { 2351 DateTimeType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 2352 @Description(shortDefinition = "Task Creation Date", formalDefinition = "The date and time this task was created.") 2353 protected DateTimeType authoredOn; 2354 2355 /** 2356 * The date and time of last modification to this task. 2357 */ 2358 @Child(name = "lastModified", type = { 2359 DateTimeType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 2360 @Description(shortDefinition = "Task Last Modified Date", formalDefinition = "The date and time of last modification to this task.") 2361 protected DateTimeType lastModified; 2362 2363 /** 2364 * The creator of the task. 2365 */ 2366 @Child(name = "requester", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 2367 PractitionerRole.class, RelatedPerson.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 2368 @Description(shortDefinition = "Who is asking for task to be done", formalDefinition = "The creator of the task.") 2369 protected Reference requester; 2370 2371 /** 2372 * The actual object that is the target of the reference (The creator of the 2373 * task.) 2374 */ 2375 protected Resource requesterTarget; 2376 2377 /** 2378 * The kind of participant that should perform the task. 2379 */ 2380 @Child(name = "performerType", type = { 2381 CodeableConcept.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2382 @Description(shortDefinition = "Requested performer", formalDefinition = "The kind of participant that should perform the task.") 2383 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/performer-role") 2384 protected List<CodeableConcept> performerType; 2385 2386 /** 2387 * Individual organization or Device currently responsible for task execution. 2388 */ 2389 @Child(name = "owner", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 2390 HealthcareService.class, Patient.class, Device.class, 2391 RelatedPerson.class }, order = 21, min = 0, max = 1, modifier = false, summary = true) 2392 @Description(shortDefinition = "Responsible individual", formalDefinition = "Individual organization or Device currently responsible for task execution.") 2393 protected Reference owner; 2394 2395 /** 2396 * The actual object that is the target of the reference (Individual 2397 * organization or Device currently responsible for task execution.) 2398 */ 2399 protected Resource ownerTarget; 2400 2401 /** 2402 * Principal physical location where the this task is performed. 2403 */ 2404 @Child(name = "location", type = { Location.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 2405 @Description(shortDefinition = "Where task occurs", formalDefinition = "Principal physical location where the this task is performed.") 2406 protected Reference location; 2407 2408 /** 2409 * The actual object that is the target of the reference (Principal physical 2410 * location where the this task is performed.) 2411 */ 2412 protected Location locationTarget; 2413 2414 /** 2415 * A description or code indicating why this task needs to be performed. 2416 */ 2417 @Child(name = "reasonCode", type = { 2418 CodeableConcept.class }, order = 23, min = 0, max = 1, modifier = false, summary = false) 2419 @Description(shortDefinition = "Why task is needed", formalDefinition = "A description or code indicating why this task needs to be performed.") 2420 protected CodeableConcept reasonCode; 2421 2422 /** 2423 * A resource reference indicating why this task needs to be performed. 2424 */ 2425 @Child(name = "reasonReference", type = { 2426 Reference.class }, order = 24, min = 0, max = 1, modifier = false, summary = false) 2427 @Description(shortDefinition = "Why task is needed", formalDefinition = "A resource reference indicating why this task needs to be performed.") 2428 protected Reference reasonReference; 2429 2430 /** 2431 * The actual object that is the target of the reference (A resource reference 2432 * indicating why this task needs to be performed.) 2433 */ 2434 protected Resource reasonReferenceTarget; 2435 2436 /** 2437 * Insurance plans, coverage extensions, pre-authorizations and/or 2438 * pre-determinations that may be relevant to the Task. 2439 */ 2440 @Child(name = "insurance", type = { Coverage.class, 2441 ClaimResponse.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2442 @Description(shortDefinition = "Associated insurance coverage", formalDefinition = "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.") 2443 protected List<Reference> insurance; 2444 /** 2445 * The actual objects that are the target of the reference (Insurance plans, 2446 * coverage extensions, pre-authorizations and/or pre-determinations that may be 2447 * relevant to the Task.) 2448 */ 2449 protected List<Resource> insuranceTarget; 2450 2451 /** 2452 * Free-text information captured about the task as it progresses. 2453 */ 2454 @Child(name = "note", type = { 2455 Annotation.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2456 @Description(shortDefinition = "Comments made about the task", formalDefinition = "Free-text information captured about the task as it progresses.") 2457 protected List<Annotation> note; 2458 2459 /** 2460 * Links to Provenance records for past versions of this Task that identify key 2461 * state transitions or updates that are likely to be relevant to a user looking 2462 * at the current version of the task. 2463 */ 2464 @Child(name = "relevantHistory", type = { 2465 Provenance.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2466 @Description(shortDefinition = "Key events in history of the Task", formalDefinition = "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.") 2467 protected List<Reference> relevantHistory; 2468 /** 2469 * The actual objects that are the target of the reference (Links to Provenance 2470 * records for past versions of this Task that identify key state transitions or 2471 * updates that are likely to be relevant to a user looking at the current 2472 * version of the task.) 2473 */ 2474 protected List<Provenance> relevantHistoryTarget; 2475 2476 /** 2477 * If the Task.focus is a request resource and the task is seeking fulfillment 2478 * (i.e. is asking for the request to be actioned), this element identifies any 2479 * limitations on what parts of the referenced request should be actioned. 2480 */ 2481 @Child(name = "restriction", type = {}, order = 28, min = 0, max = 1, modifier = false, summary = false) 2482 @Description(shortDefinition = "Constraints on fulfillment tasks", formalDefinition = "If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.") 2483 protected TaskRestrictionComponent restriction; 2484 2485 /** 2486 * Additional information that may be needed in the execution of the task. 2487 */ 2488 @Child(name = "input", type = {}, order = 29, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2489 @Description(shortDefinition = "Information used to perform task", formalDefinition = "Additional information that may be needed in the execution of the task.") 2490 protected List<ParameterComponent> input; 2491 2492 /** 2493 * Outputs produced by the Task. 2494 */ 2495 @Child(name = "output", type = {}, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2496 @Description(shortDefinition = "Information produced as part of task", formalDefinition = "Outputs produced by the Task.") 2497 protected List<TaskOutputComponent> output; 2498 2499 private static final long serialVersionUID = -765029272L; 2500 2501 /** 2502 * Constructor 2503 */ 2504 public Task() { 2505 super(); 2506 } 2507 2508 /** 2509 * Constructor 2510 */ 2511 public Task(Enumeration<TaskStatus> status, Enumeration<TaskIntent> intent) { 2512 super(); 2513 this.status = status; 2514 this.intent = intent; 2515 } 2516 2517 /** 2518 * @return {@link #identifier} (The business identifier for this task.) 2519 */ 2520 public List<Identifier> getIdentifier() { 2521 if (this.identifier == null) 2522 this.identifier = new ArrayList<Identifier>(); 2523 return this.identifier; 2524 } 2525 2526 /** 2527 * @return Returns a reference to <code>this</code> for easy method chaining 2528 */ 2529 public Task setIdentifier(List<Identifier> theIdentifier) { 2530 this.identifier = theIdentifier; 2531 return this; 2532 } 2533 2534 public boolean hasIdentifier() { 2535 if (this.identifier == null) 2536 return false; 2537 for (Identifier item : this.identifier) 2538 if (!item.isEmpty()) 2539 return true; 2540 return false; 2541 } 2542 2543 public Identifier addIdentifier() { // 3 2544 Identifier t = new Identifier(); 2545 if (this.identifier == null) 2546 this.identifier = new ArrayList<Identifier>(); 2547 this.identifier.add(t); 2548 return t; 2549 } 2550 2551 public Task addIdentifier(Identifier t) { // 3 2552 if (t == null) 2553 return this; 2554 if (this.identifier == null) 2555 this.identifier = new ArrayList<Identifier>(); 2556 this.identifier.add(t); 2557 return this; 2558 } 2559 2560 /** 2561 * @return The first repetition of repeating field {@link #identifier}, creating 2562 * it if it does not already exist 2563 */ 2564 public Identifier getIdentifierFirstRep() { 2565 if (getIdentifier().isEmpty()) { 2566 addIdentifier(); 2567 } 2568 return getIdentifier().get(0); 2569 } 2570 2571 /** 2572 * @return {@link #instantiatesCanonical} (The URL pointing to a *FHIR*-defined 2573 * protocol, guideline, orderset or other definition that is adhered to 2574 * in whole or in part by this Task.). This is the underlying object 2575 * with id, value and extensions. The accessor 2576 * "getInstantiatesCanonical" gives direct access to the value 2577 */ 2578 public CanonicalType getInstantiatesCanonicalElement() { 2579 if (this.instantiatesCanonical == null) 2580 if (Configuration.errorOnAutoCreate()) 2581 throw new Error("Attempt to auto-create Task.instantiatesCanonical"); 2582 else if (Configuration.doAutoCreate()) 2583 this.instantiatesCanonical = new CanonicalType(); // bb 2584 return this.instantiatesCanonical; 2585 } 2586 2587 public boolean hasInstantiatesCanonicalElement() { 2588 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 2589 } 2590 2591 public boolean hasInstantiatesCanonical() { 2592 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 2593 } 2594 2595 /** 2596 * @param value {@link #instantiatesCanonical} (The URL pointing to a 2597 * *FHIR*-defined protocol, guideline, orderset or other definition 2598 * that is adhered to in whole or in part by this Task.). This is 2599 * the underlying object with id, value and extensions. The 2600 * accessor "getInstantiatesCanonical" gives direct access to the 2601 * value 2602 */ 2603 public Task setInstantiatesCanonicalElement(CanonicalType value) { 2604 this.instantiatesCanonical = value; 2605 return this; 2606 } 2607 2608 /** 2609 * @return The URL pointing to a *FHIR*-defined protocol, guideline, orderset or 2610 * other definition that is adhered to in whole or in part by this Task. 2611 */ 2612 public String getInstantiatesCanonical() { 2613 return this.instantiatesCanonical == null ? null : this.instantiatesCanonical.getValue(); 2614 } 2615 2616 /** 2617 * @param value The URL pointing to a *FHIR*-defined protocol, guideline, 2618 * orderset or other definition that is adhered to in whole or in 2619 * part by this Task. 2620 */ 2621 public Task setInstantiatesCanonical(String value) { 2622 if (Utilities.noString(value)) 2623 this.instantiatesCanonical = null; 2624 else { 2625 if (this.instantiatesCanonical == null) 2626 this.instantiatesCanonical = new CanonicalType(); 2627 this.instantiatesCanonical.setValue(value); 2628 } 2629 return this; 2630 } 2631 2632 /** 2633 * @return {@link #instantiatesUri} (The URL pointing to an *externally* 2634 * maintained protocol, guideline, orderset or other definition that is 2635 * adhered to in whole or in part by this Task.). This is the underlying 2636 * object with id, value and extensions. The accessor 2637 * "getInstantiatesUri" gives direct access to the value 2638 */ 2639 public UriType getInstantiatesUriElement() { 2640 if (this.instantiatesUri == null) 2641 if (Configuration.errorOnAutoCreate()) 2642 throw new Error("Attempt to auto-create Task.instantiatesUri"); 2643 else if (Configuration.doAutoCreate()) 2644 this.instantiatesUri = new UriType(); // bb 2645 return this.instantiatesUri; 2646 } 2647 2648 public boolean hasInstantiatesUriElement() { 2649 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 2650 } 2651 2652 public boolean hasInstantiatesUri() { 2653 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 2654 } 2655 2656 /** 2657 * @param value {@link #instantiatesUri} (The URL pointing to an *externally* 2658 * maintained protocol, guideline, orderset or other definition 2659 * that is adhered to in whole or in part by this Task.). This is 2660 * the underlying object with id, value and extensions. The 2661 * accessor "getInstantiatesUri" gives direct access to the value 2662 */ 2663 public Task setInstantiatesUriElement(UriType value) { 2664 this.instantiatesUri = value; 2665 return this; 2666 } 2667 2668 /** 2669 * @return The URL pointing to an *externally* maintained protocol, guideline, 2670 * orderset or other definition that is adhered to in whole or in part 2671 * by this Task. 2672 */ 2673 public String getInstantiatesUri() { 2674 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 2675 } 2676 2677 /** 2678 * @param value The URL pointing to an *externally* maintained protocol, 2679 * guideline, orderset or other definition that is adhered to in 2680 * whole or in part by this Task. 2681 */ 2682 public Task setInstantiatesUri(String value) { 2683 if (Utilities.noString(value)) 2684 this.instantiatesUri = null; 2685 else { 2686 if (this.instantiatesUri == null) 2687 this.instantiatesUri = new UriType(); 2688 this.instantiatesUri.setValue(value); 2689 } 2690 return this; 2691 } 2692 2693 /** 2694 * @return {@link #basedOn} (BasedOn refers to a higher-level authorization that 2695 * triggered the creation of the task. It references a "request" 2696 * resource such as a ServiceRequest, MedicationRequest, ServiceRequest, 2697 * CarePlan, etc. which is distinct from the "request" resource the task 2698 * is seeking to fulfill. This latter resource is referenced by FocusOn. 2699 * For example, based on a ServiceRequest (= BasedOn), a task is created 2700 * to fulfill a procedureRequest ( = FocusOn ) to collect a specimen 2701 * from a patient.) 2702 */ 2703 public List<Reference> getBasedOn() { 2704 if (this.basedOn == null) 2705 this.basedOn = new ArrayList<Reference>(); 2706 return this.basedOn; 2707 } 2708 2709 /** 2710 * @return Returns a reference to <code>this</code> for easy method chaining 2711 */ 2712 public Task setBasedOn(List<Reference> theBasedOn) { 2713 this.basedOn = theBasedOn; 2714 return this; 2715 } 2716 2717 public boolean hasBasedOn() { 2718 if (this.basedOn == null) 2719 return false; 2720 for (Reference item : this.basedOn) 2721 if (!item.isEmpty()) 2722 return true; 2723 return false; 2724 } 2725 2726 public Reference addBasedOn() { // 3 2727 Reference t = new Reference(); 2728 if (this.basedOn == null) 2729 this.basedOn = new ArrayList<Reference>(); 2730 this.basedOn.add(t); 2731 return t; 2732 } 2733 2734 public Task addBasedOn(Reference t) { // 3 2735 if (t == null) 2736 return this; 2737 if (this.basedOn == null) 2738 this.basedOn = new ArrayList<Reference>(); 2739 this.basedOn.add(t); 2740 return this; 2741 } 2742 2743 /** 2744 * @return The first repetition of repeating field {@link #basedOn}, creating it 2745 * if it does not already exist 2746 */ 2747 public Reference getBasedOnFirstRep() { 2748 if (getBasedOn().isEmpty()) { 2749 addBasedOn(); 2750 } 2751 return getBasedOn().get(0); 2752 } 2753 2754 /** 2755 * @deprecated Use Reference#setResource(IBaseResource) instead 2756 */ 2757 @Deprecated 2758 public List<Resource> getBasedOnTarget() { 2759 if (this.basedOnTarget == null) 2760 this.basedOnTarget = new ArrayList<Resource>(); 2761 return this.basedOnTarget; 2762 } 2763 2764 /** 2765 * @return {@link #groupIdentifier} (An identifier that links together multiple 2766 * tasks and other requests that were created in the same context.) 2767 */ 2768 public Identifier getGroupIdentifier() { 2769 if (this.groupIdentifier == null) 2770 if (Configuration.errorOnAutoCreate()) 2771 throw new Error("Attempt to auto-create Task.groupIdentifier"); 2772 else if (Configuration.doAutoCreate()) 2773 this.groupIdentifier = new Identifier(); // cc 2774 return this.groupIdentifier; 2775 } 2776 2777 public boolean hasGroupIdentifier() { 2778 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 2779 } 2780 2781 /** 2782 * @param value {@link #groupIdentifier} (An identifier that links together 2783 * multiple tasks and other requests that were created in the same 2784 * context.) 2785 */ 2786 public Task setGroupIdentifier(Identifier value) { 2787 this.groupIdentifier = value; 2788 return this; 2789 } 2790 2791 /** 2792 * @return {@link #partOf} (Task that this particular task is part of.) 2793 */ 2794 public List<Reference> getPartOf() { 2795 if (this.partOf == null) 2796 this.partOf = new ArrayList<Reference>(); 2797 return this.partOf; 2798 } 2799 2800 /** 2801 * @return Returns a reference to <code>this</code> for easy method chaining 2802 */ 2803 public Task setPartOf(List<Reference> thePartOf) { 2804 this.partOf = thePartOf; 2805 return this; 2806 } 2807 2808 public boolean hasPartOf() { 2809 if (this.partOf == null) 2810 return false; 2811 for (Reference item : this.partOf) 2812 if (!item.isEmpty()) 2813 return true; 2814 return false; 2815 } 2816 2817 public Reference addPartOf() { // 3 2818 Reference t = new Reference(); 2819 if (this.partOf == null) 2820 this.partOf = new ArrayList<Reference>(); 2821 this.partOf.add(t); 2822 return t; 2823 } 2824 2825 public Task addPartOf(Reference t) { // 3 2826 if (t == null) 2827 return this; 2828 if (this.partOf == null) 2829 this.partOf = new ArrayList<Reference>(); 2830 this.partOf.add(t); 2831 return this; 2832 } 2833 2834 /** 2835 * @return The first repetition of repeating field {@link #partOf}, creating it 2836 * if it does not already exist 2837 */ 2838 public Reference getPartOfFirstRep() { 2839 if (getPartOf().isEmpty()) { 2840 addPartOf(); 2841 } 2842 return getPartOf().get(0); 2843 } 2844 2845 /** 2846 * @deprecated Use Reference#setResource(IBaseResource) instead 2847 */ 2848 @Deprecated 2849 public List<Task> getPartOfTarget() { 2850 if (this.partOfTarget == null) 2851 this.partOfTarget = new ArrayList<Task>(); 2852 return this.partOfTarget; 2853 } 2854 2855 /** 2856 * @deprecated Use Reference#setResource(IBaseResource) instead 2857 */ 2858 @Deprecated 2859 public Task addPartOfTarget() { 2860 Task r = new Task(); 2861 if (this.partOfTarget == null) 2862 this.partOfTarget = new ArrayList<Task>(); 2863 this.partOfTarget.add(r); 2864 return r; 2865 } 2866 2867 /** 2868 * @return {@link #status} (The current status of the task.). This is the 2869 * underlying object with id, value and extensions. The accessor 2870 * "getStatus" gives direct access to the value 2871 */ 2872 public Enumeration<TaskStatus> getStatusElement() { 2873 if (this.status == null) 2874 if (Configuration.errorOnAutoCreate()) 2875 throw new Error("Attempt to auto-create Task.status"); 2876 else if (Configuration.doAutoCreate()) 2877 this.status = new Enumeration<TaskStatus>(new TaskStatusEnumFactory()); // bb 2878 return this.status; 2879 } 2880 2881 public boolean hasStatusElement() { 2882 return this.status != null && !this.status.isEmpty(); 2883 } 2884 2885 public boolean hasStatus() { 2886 return this.status != null && !this.status.isEmpty(); 2887 } 2888 2889 /** 2890 * @param value {@link #status} (The current status of the task.). This is the 2891 * underlying object with id, value and extensions. The accessor 2892 * "getStatus" gives direct access to the value 2893 */ 2894 public Task setStatusElement(Enumeration<TaskStatus> value) { 2895 this.status = value; 2896 return this; 2897 } 2898 2899 /** 2900 * @return The current status of the task. 2901 */ 2902 public TaskStatus getStatus() { 2903 return this.status == null ? null : this.status.getValue(); 2904 } 2905 2906 /** 2907 * @param value The current status of the task. 2908 */ 2909 public Task setStatus(TaskStatus value) { 2910 if (this.status == null) 2911 this.status = new Enumeration<TaskStatus>(new TaskStatusEnumFactory()); 2912 this.status.setValue(value); 2913 return this; 2914 } 2915 2916 /** 2917 * @return {@link #statusReason} (An explanation as to why this task is held, 2918 * failed, was refused, etc.) 2919 */ 2920 public CodeableConcept getStatusReason() { 2921 if (this.statusReason == null) 2922 if (Configuration.errorOnAutoCreate()) 2923 throw new Error("Attempt to auto-create Task.statusReason"); 2924 else if (Configuration.doAutoCreate()) 2925 this.statusReason = new CodeableConcept(); // cc 2926 return this.statusReason; 2927 } 2928 2929 public boolean hasStatusReason() { 2930 return this.statusReason != null && !this.statusReason.isEmpty(); 2931 } 2932 2933 /** 2934 * @param value {@link #statusReason} (An explanation as to why this task is 2935 * held, failed, was refused, etc.) 2936 */ 2937 public Task setStatusReason(CodeableConcept value) { 2938 this.statusReason = value; 2939 return this; 2940 } 2941 2942 /** 2943 * @return {@link #businessStatus} (Contains business-specific nuances of the 2944 * business state.) 2945 */ 2946 public CodeableConcept getBusinessStatus() { 2947 if (this.businessStatus == null) 2948 if (Configuration.errorOnAutoCreate()) 2949 throw new Error("Attempt to auto-create Task.businessStatus"); 2950 else if (Configuration.doAutoCreate()) 2951 this.businessStatus = new CodeableConcept(); // cc 2952 return this.businessStatus; 2953 } 2954 2955 public boolean hasBusinessStatus() { 2956 return this.businessStatus != null && !this.businessStatus.isEmpty(); 2957 } 2958 2959 /** 2960 * @param value {@link #businessStatus} (Contains business-specific nuances of 2961 * the business state.) 2962 */ 2963 public Task setBusinessStatus(CodeableConcept value) { 2964 this.businessStatus = value; 2965 return this; 2966 } 2967 2968 /** 2969 * @return {@link #intent} (Indicates the "level" of actionability associated 2970 * with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an 2971 * actionable task, etc.). This is the underlying object with id, value 2972 * and extensions. The accessor "getIntent" gives direct access to the 2973 * value 2974 */ 2975 public Enumeration<TaskIntent> getIntentElement() { 2976 if (this.intent == null) 2977 if (Configuration.errorOnAutoCreate()) 2978 throw new Error("Attempt to auto-create Task.intent"); 2979 else if (Configuration.doAutoCreate()) 2980 this.intent = new Enumeration<TaskIntent>(new TaskIntentEnumFactory()); // bb 2981 return this.intent; 2982 } 2983 2984 public boolean hasIntentElement() { 2985 return this.intent != null && !this.intent.isEmpty(); 2986 } 2987 2988 public boolean hasIntent() { 2989 return this.intent != null && !this.intent.isEmpty(); 2990 } 2991 2992 /** 2993 * @param value {@link #intent} (Indicates the "level" of actionability 2994 * associated with the Task, i.e. i+R[9]Cs this a proposed task, a 2995 * planned task, an actionable task, etc.). This is the underlying 2996 * object with id, value and extensions. The accessor "getIntent" 2997 * gives direct access to the value 2998 */ 2999 public Task setIntentElement(Enumeration<TaskIntent> value) { 3000 this.intent = value; 3001 return this; 3002 } 3003 3004 /** 3005 * @return Indicates the "level" of actionability associated with the Task, i.e. 3006 * i+R[9]Cs this a proposed task, a planned task, an actionable task, 3007 * etc. 3008 */ 3009 public TaskIntent getIntent() { 3010 return this.intent == null ? null : this.intent.getValue(); 3011 } 3012 3013 /** 3014 * @param value Indicates the "level" of actionability associated with the Task, 3015 * i.e. i+R[9]Cs this a proposed task, a planned task, an 3016 * actionable task, etc. 3017 */ 3018 public Task setIntent(TaskIntent value) { 3019 if (this.intent == null) 3020 this.intent = new Enumeration<TaskIntent>(new TaskIntentEnumFactory()); 3021 this.intent.setValue(value); 3022 return this; 3023 } 3024 3025 /** 3026 * @return {@link #priority} (Indicates how quickly the Task should be addressed 3027 * with respect to other requests.). This is the underlying object with 3028 * id, value and extensions. The accessor "getPriority" gives direct 3029 * access to the value 3030 */ 3031 public Enumeration<TaskPriority> getPriorityElement() { 3032 if (this.priority == null) 3033 if (Configuration.errorOnAutoCreate()) 3034 throw new Error("Attempt to auto-create Task.priority"); 3035 else if (Configuration.doAutoCreate()) 3036 this.priority = new Enumeration<TaskPriority>(new TaskPriorityEnumFactory()); // bb 3037 return this.priority; 3038 } 3039 3040 public boolean hasPriorityElement() { 3041 return this.priority != null && !this.priority.isEmpty(); 3042 } 3043 3044 public boolean hasPriority() { 3045 return this.priority != null && !this.priority.isEmpty(); 3046 } 3047 3048 /** 3049 * @param value {@link #priority} (Indicates how quickly the Task should be 3050 * addressed with respect to other requests.). This is the 3051 * underlying object with id, value and extensions. The accessor 3052 * "getPriority" gives direct access to the value 3053 */ 3054 public Task setPriorityElement(Enumeration<TaskPriority> value) { 3055 this.priority = value; 3056 return this; 3057 } 3058 3059 /** 3060 * @return Indicates how quickly the Task should be addressed with respect to 3061 * other requests. 3062 */ 3063 public TaskPriority getPriority() { 3064 return this.priority == null ? null : this.priority.getValue(); 3065 } 3066 3067 /** 3068 * @param value Indicates how quickly the Task should be addressed with respect 3069 * to other requests. 3070 */ 3071 public Task setPriority(TaskPriority value) { 3072 if (value == null) 3073 this.priority = null; 3074 else { 3075 if (this.priority == null) 3076 this.priority = new Enumeration<TaskPriority>(new TaskPriorityEnumFactory()); 3077 this.priority.setValue(value); 3078 } 3079 return this; 3080 } 3081 3082 /** 3083 * @return {@link #code} (A name or code (or both) briefly describing what the 3084 * task involves.) 3085 */ 3086 public CodeableConcept getCode() { 3087 if (this.code == null) 3088 if (Configuration.errorOnAutoCreate()) 3089 throw new Error("Attempt to auto-create Task.code"); 3090 else if (Configuration.doAutoCreate()) 3091 this.code = new CodeableConcept(); // cc 3092 return this.code; 3093 } 3094 3095 public boolean hasCode() { 3096 return this.code != null && !this.code.isEmpty(); 3097 } 3098 3099 /** 3100 * @param value {@link #code} (A name or code (or both) briefly describing what 3101 * the task involves.) 3102 */ 3103 public Task setCode(CodeableConcept value) { 3104 this.code = value; 3105 return this; 3106 } 3107 3108 /** 3109 * @return {@link #description} (A free-text description of what is to be 3110 * performed.). This is the underlying object with id, value and 3111 * extensions. The accessor "getDescription" gives direct access to the 3112 * value 3113 */ 3114 public StringType getDescriptionElement() { 3115 if (this.description == null) 3116 if (Configuration.errorOnAutoCreate()) 3117 throw new Error("Attempt to auto-create Task.description"); 3118 else if (Configuration.doAutoCreate()) 3119 this.description = new StringType(); // bb 3120 return this.description; 3121 } 3122 3123 public boolean hasDescriptionElement() { 3124 return this.description != null && !this.description.isEmpty(); 3125 } 3126 3127 public boolean hasDescription() { 3128 return this.description != null && !this.description.isEmpty(); 3129 } 3130 3131 /** 3132 * @param value {@link #description} (A free-text description of what is to be 3133 * performed.). This is the underlying object with id, value and 3134 * extensions. The accessor "getDescription" gives direct access to 3135 * the value 3136 */ 3137 public Task setDescriptionElement(StringType value) { 3138 this.description = value; 3139 return this; 3140 } 3141 3142 /** 3143 * @return A free-text description of what is to be performed. 3144 */ 3145 public String getDescription() { 3146 return this.description == null ? null : this.description.getValue(); 3147 } 3148 3149 /** 3150 * @param value A free-text description of what is to be performed. 3151 */ 3152 public Task setDescription(String value) { 3153 if (Utilities.noString(value)) 3154 this.description = null; 3155 else { 3156 if (this.description == null) 3157 this.description = new StringType(); 3158 this.description.setValue(value); 3159 } 3160 return this; 3161 } 3162 3163 /** 3164 * @return {@link #focus} (The request being actioned or the resource being 3165 * manipulated by this task.) 3166 */ 3167 public Reference getFocus() { 3168 if (this.focus == null) 3169 if (Configuration.errorOnAutoCreate()) 3170 throw new Error("Attempt to auto-create Task.focus"); 3171 else if (Configuration.doAutoCreate()) 3172 this.focus = new Reference(); // cc 3173 return this.focus; 3174 } 3175 3176 public boolean hasFocus() { 3177 return this.focus != null && !this.focus.isEmpty(); 3178 } 3179 3180 /** 3181 * @param value {@link #focus} (The request being actioned or the resource being 3182 * manipulated by this task.) 3183 */ 3184 public Task setFocus(Reference value) { 3185 this.focus = value; 3186 return this; 3187 } 3188 3189 /** 3190 * @return {@link #focus} The actual object that is the target of the reference. 3191 * The reference library doesn't populate this, but you can use it to 3192 * hold the resource if you resolve it. (The request being actioned or 3193 * the resource being manipulated by this task.) 3194 */ 3195 public Resource getFocusTarget() { 3196 return this.focusTarget; 3197 } 3198 3199 /** 3200 * @param value {@link #focus} The actual object that is the target of the 3201 * reference. The reference library doesn't use these, but you can 3202 * use it to hold the resource if you resolve it. (The request 3203 * being actioned or the resource being manipulated by this task.) 3204 */ 3205 public Task setFocusTarget(Resource value) { 3206 this.focusTarget = value; 3207 return this; 3208 } 3209 3210 /** 3211 * @return {@link #for_} (The entity who benefits from the performance of the 3212 * service specified in the task (e.g., the patient).) 3213 */ 3214 public Reference getFor() { 3215 if (this.for_ == null) 3216 if (Configuration.errorOnAutoCreate()) 3217 throw new Error("Attempt to auto-create Task.for_"); 3218 else if (Configuration.doAutoCreate()) 3219 this.for_ = new Reference(); // cc 3220 return this.for_; 3221 } 3222 3223 public boolean hasFor() { 3224 return this.for_ != null && !this.for_.isEmpty(); 3225 } 3226 3227 /** 3228 * @param value {@link #for_} (The entity who benefits from the performance of 3229 * the service specified in the task (e.g., the patient).) 3230 */ 3231 public Task setFor(Reference value) { 3232 this.for_ = value; 3233 return this; 3234 } 3235 3236 /** 3237 * @return {@link #for_} The actual object that is the target of the reference. 3238 * The reference library doesn't populate this, but you can use it to 3239 * hold the resource if you resolve it. (The entity who benefits from 3240 * the performance of the service specified in the task (e.g., the 3241 * patient).) 3242 */ 3243 public Resource getForTarget() { 3244 return this.for_Target; 3245 } 3246 3247 /** 3248 * @param value {@link #for_} The actual object that is the target of the 3249 * reference. The reference library doesn't use these, but you can 3250 * use it to hold the resource if you resolve it. (The entity who 3251 * benefits from the performance of the service specified in the 3252 * task (e.g., the patient).) 3253 */ 3254 public Task setForTarget(Resource value) { 3255 this.for_Target = value; 3256 return this; 3257 } 3258 3259 /** 3260 * @return {@link #encounter} (The healthcare event (e.g. a patient and 3261 * healthcare provider interaction) during which this task was created.) 3262 */ 3263 public Reference getEncounter() { 3264 if (this.encounter == null) 3265 if (Configuration.errorOnAutoCreate()) 3266 throw new Error("Attempt to auto-create Task.encounter"); 3267 else if (Configuration.doAutoCreate()) 3268 this.encounter = new Reference(); // cc 3269 return this.encounter; 3270 } 3271 3272 public boolean hasEncounter() { 3273 return this.encounter != null && !this.encounter.isEmpty(); 3274 } 3275 3276 /** 3277 * @param value {@link #encounter} (The healthcare event (e.g. a patient and 3278 * healthcare provider interaction) during which this task was 3279 * created.) 3280 */ 3281 public Task setEncounter(Reference value) { 3282 this.encounter = value; 3283 return this; 3284 } 3285 3286 /** 3287 * @return {@link #encounter} The actual object that is the target of the 3288 * reference. The reference library doesn't populate this, but you can 3289 * use it to hold the resource if you resolve it. (The healthcare event 3290 * (e.g. a patient and healthcare provider interaction) during which 3291 * this task was created.) 3292 */ 3293 public Encounter getEncounterTarget() { 3294 if (this.encounterTarget == null) 3295 if (Configuration.errorOnAutoCreate()) 3296 throw new Error("Attempt to auto-create Task.encounter"); 3297 else if (Configuration.doAutoCreate()) 3298 this.encounterTarget = new Encounter(); // aa 3299 return this.encounterTarget; 3300 } 3301 3302 /** 3303 * @param value {@link #encounter} The actual object that is the target of the 3304 * reference. The reference library doesn't use these, but you can 3305 * use it to hold the resource if you resolve it. (The healthcare 3306 * event (e.g. a patient and healthcare provider interaction) 3307 * during which this task was created.) 3308 */ 3309 public Task setEncounterTarget(Encounter value) { 3310 this.encounterTarget = value; 3311 return this; 3312 } 3313 3314 /** 3315 * @return {@link #executionPeriod} (Identifies the time action was first taken 3316 * against the task (start) and/or the time final action was taken 3317 * against the task prior to marking it as completed (end).) 3318 */ 3319 public Period getExecutionPeriod() { 3320 if (this.executionPeriod == null) 3321 if (Configuration.errorOnAutoCreate()) 3322 throw new Error("Attempt to auto-create Task.executionPeriod"); 3323 else if (Configuration.doAutoCreate()) 3324 this.executionPeriod = new Period(); // cc 3325 return this.executionPeriod; 3326 } 3327 3328 public boolean hasExecutionPeriod() { 3329 return this.executionPeriod != null && !this.executionPeriod.isEmpty(); 3330 } 3331 3332 /** 3333 * @param value {@link #executionPeriod} (Identifies the time action was first 3334 * taken against the task (start) and/or the time final action was 3335 * taken against the task prior to marking it as completed (end).) 3336 */ 3337 public Task setExecutionPeriod(Period value) { 3338 this.executionPeriod = value; 3339 return this; 3340 } 3341 3342 /** 3343 * @return {@link #authoredOn} (The date and time this task was created.). This 3344 * is the underlying object with id, value and extensions. The accessor 3345 * "getAuthoredOn" gives direct access to the value 3346 */ 3347 public DateTimeType getAuthoredOnElement() { 3348 if (this.authoredOn == null) 3349 if (Configuration.errorOnAutoCreate()) 3350 throw new Error("Attempt to auto-create Task.authoredOn"); 3351 else if (Configuration.doAutoCreate()) 3352 this.authoredOn = new DateTimeType(); // bb 3353 return this.authoredOn; 3354 } 3355 3356 public boolean hasAuthoredOnElement() { 3357 return this.authoredOn != null && !this.authoredOn.isEmpty(); 3358 } 3359 3360 public boolean hasAuthoredOn() { 3361 return this.authoredOn != null && !this.authoredOn.isEmpty(); 3362 } 3363 3364 /** 3365 * @param value {@link #authoredOn} (The date and time this task was created.). 3366 * This is the underlying object with id, value and extensions. The 3367 * accessor "getAuthoredOn" gives direct access to the value 3368 */ 3369 public Task setAuthoredOnElement(DateTimeType value) { 3370 this.authoredOn = value; 3371 return this; 3372 } 3373 3374 /** 3375 * @return The date and time this task was created. 3376 */ 3377 public Date getAuthoredOn() { 3378 return this.authoredOn == null ? null : this.authoredOn.getValue(); 3379 } 3380 3381 /** 3382 * @param value The date and time this task was created. 3383 */ 3384 public Task setAuthoredOn(Date value) { 3385 if (value == null) 3386 this.authoredOn = null; 3387 else { 3388 if (this.authoredOn == null) 3389 this.authoredOn = new DateTimeType(); 3390 this.authoredOn.setValue(value); 3391 } 3392 return this; 3393 } 3394 3395 /** 3396 * @return {@link #lastModified} (The date and time of last modification to this 3397 * task.). This is the underlying object with id, value and extensions. 3398 * The accessor "getLastModified" gives direct access to the value 3399 */ 3400 public DateTimeType getLastModifiedElement() { 3401 if (this.lastModified == null) 3402 if (Configuration.errorOnAutoCreate()) 3403 throw new Error("Attempt to auto-create Task.lastModified"); 3404 else if (Configuration.doAutoCreate()) 3405 this.lastModified = new DateTimeType(); // bb 3406 return this.lastModified; 3407 } 3408 3409 public boolean hasLastModifiedElement() { 3410 return this.lastModified != null && !this.lastModified.isEmpty(); 3411 } 3412 3413 public boolean hasLastModified() { 3414 return this.lastModified != null && !this.lastModified.isEmpty(); 3415 } 3416 3417 /** 3418 * @param value {@link #lastModified} (The date and time of last modification to 3419 * this task.). This is the underlying object with id, value and 3420 * extensions. The accessor "getLastModified" gives direct access 3421 * to the value 3422 */ 3423 public Task setLastModifiedElement(DateTimeType value) { 3424 this.lastModified = value; 3425 return this; 3426 } 3427 3428 /** 3429 * @return The date and time of last modification to this task. 3430 */ 3431 public Date getLastModified() { 3432 return this.lastModified == null ? null : this.lastModified.getValue(); 3433 } 3434 3435 /** 3436 * @param value The date and time of last modification to this task. 3437 */ 3438 public Task setLastModified(Date value) { 3439 if (value == null) 3440 this.lastModified = null; 3441 else { 3442 if (this.lastModified == null) 3443 this.lastModified = new DateTimeType(); 3444 this.lastModified.setValue(value); 3445 } 3446 return this; 3447 } 3448 3449 /** 3450 * @return {@link #requester} (The creator of the task.) 3451 */ 3452 public Reference getRequester() { 3453 if (this.requester == null) 3454 if (Configuration.errorOnAutoCreate()) 3455 throw new Error("Attempt to auto-create Task.requester"); 3456 else if (Configuration.doAutoCreate()) 3457 this.requester = new Reference(); // cc 3458 return this.requester; 3459 } 3460 3461 public boolean hasRequester() { 3462 return this.requester != null && !this.requester.isEmpty(); 3463 } 3464 3465 /** 3466 * @param value {@link #requester} (The creator of the task.) 3467 */ 3468 public Task setRequester(Reference value) { 3469 this.requester = value; 3470 return this; 3471 } 3472 3473 /** 3474 * @return {@link #requester} The actual object that is the target of the 3475 * reference. The reference library doesn't populate this, but you can 3476 * use it to hold the resource if you resolve it. (The creator of the 3477 * task.) 3478 */ 3479 public Resource getRequesterTarget() { 3480 return this.requesterTarget; 3481 } 3482 3483 /** 3484 * @param value {@link #requester} The actual object that is the target of the 3485 * reference. The reference library doesn't use these, but you can 3486 * use it to hold the resource if you resolve it. (The creator of 3487 * the task.) 3488 */ 3489 public Task setRequesterTarget(Resource value) { 3490 this.requesterTarget = value; 3491 return this; 3492 } 3493 3494 /** 3495 * @return {@link #performerType} (The kind of participant that should perform 3496 * the task.) 3497 */ 3498 public List<CodeableConcept> getPerformerType() { 3499 if (this.performerType == null) 3500 this.performerType = new ArrayList<CodeableConcept>(); 3501 return this.performerType; 3502 } 3503 3504 /** 3505 * @return Returns a reference to <code>this</code> for easy method chaining 3506 */ 3507 public Task setPerformerType(List<CodeableConcept> thePerformerType) { 3508 this.performerType = thePerformerType; 3509 return this; 3510 } 3511 3512 public boolean hasPerformerType() { 3513 if (this.performerType == null) 3514 return false; 3515 for (CodeableConcept item : this.performerType) 3516 if (!item.isEmpty()) 3517 return true; 3518 return false; 3519 } 3520 3521 public CodeableConcept addPerformerType() { // 3 3522 CodeableConcept t = new CodeableConcept(); 3523 if (this.performerType == null) 3524 this.performerType = new ArrayList<CodeableConcept>(); 3525 this.performerType.add(t); 3526 return t; 3527 } 3528 3529 public Task addPerformerType(CodeableConcept t) { // 3 3530 if (t == null) 3531 return this; 3532 if (this.performerType == null) 3533 this.performerType = new ArrayList<CodeableConcept>(); 3534 this.performerType.add(t); 3535 return this; 3536 } 3537 3538 /** 3539 * @return The first repetition of repeating field {@link #performerType}, 3540 * creating it if it does not already exist 3541 */ 3542 public CodeableConcept getPerformerTypeFirstRep() { 3543 if (getPerformerType().isEmpty()) { 3544 addPerformerType(); 3545 } 3546 return getPerformerType().get(0); 3547 } 3548 3549 /** 3550 * @return {@link #owner} (Individual organization or Device currently 3551 * responsible for task execution.) 3552 */ 3553 public Reference getOwner() { 3554 if (this.owner == null) 3555 if (Configuration.errorOnAutoCreate()) 3556 throw new Error("Attempt to auto-create Task.owner"); 3557 else if (Configuration.doAutoCreate()) 3558 this.owner = new Reference(); // cc 3559 return this.owner; 3560 } 3561 3562 public boolean hasOwner() { 3563 return this.owner != null && !this.owner.isEmpty(); 3564 } 3565 3566 /** 3567 * @param value {@link #owner} (Individual organization or Device currently 3568 * responsible for task execution.) 3569 */ 3570 public Task setOwner(Reference value) { 3571 this.owner = value; 3572 return this; 3573 } 3574 3575 /** 3576 * @return {@link #owner} The actual object that is the target of the reference. 3577 * The reference library doesn't populate this, but you can use it to 3578 * hold the resource if you resolve it. (Individual organization or 3579 * Device currently responsible for task execution.) 3580 */ 3581 public Resource getOwnerTarget() { 3582 return this.ownerTarget; 3583 } 3584 3585 /** 3586 * @param value {@link #owner} The actual object that is the target of the 3587 * reference. The reference library doesn't use these, but you can 3588 * use it to hold the resource if you resolve it. (Individual 3589 * organization or Device currently responsible for task 3590 * execution.) 3591 */ 3592 public Task setOwnerTarget(Resource value) { 3593 this.ownerTarget = value; 3594 return this; 3595 } 3596 3597 /** 3598 * @return {@link #location} (Principal physical location where the this task is 3599 * performed.) 3600 */ 3601 public Reference getLocation() { 3602 if (this.location == null) 3603 if (Configuration.errorOnAutoCreate()) 3604 throw new Error("Attempt to auto-create Task.location"); 3605 else if (Configuration.doAutoCreate()) 3606 this.location = new Reference(); // cc 3607 return this.location; 3608 } 3609 3610 public boolean hasLocation() { 3611 return this.location != null && !this.location.isEmpty(); 3612 } 3613 3614 /** 3615 * @param value {@link #location} (Principal physical location where the this 3616 * task is performed.) 3617 */ 3618 public Task setLocation(Reference value) { 3619 this.location = value; 3620 return this; 3621 } 3622 3623 /** 3624 * @return {@link #location} The actual object that is the target of the 3625 * reference. The reference library doesn't populate this, but you can 3626 * use it to hold the resource if you resolve it. (Principal physical 3627 * location where the this task is performed.) 3628 */ 3629 public Location getLocationTarget() { 3630 if (this.locationTarget == null) 3631 if (Configuration.errorOnAutoCreate()) 3632 throw new Error("Attempt to auto-create Task.location"); 3633 else if (Configuration.doAutoCreate()) 3634 this.locationTarget = new Location(); // aa 3635 return this.locationTarget; 3636 } 3637 3638 /** 3639 * @param value {@link #location} The actual object that is the target of the 3640 * reference. The reference library doesn't use these, but you can 3641 * use it to hold the resource if you resolve it. (Principal 3642 * physical location where the this task is performed.) 3643 */ 3644 public Task setLocationTarget(Location value) { 3645 this.locationTarget = value; 3646 return this; 3647 } 3648 3649 /** 3650 * @return {@link #reasonCode} (A description or code indicating why this task 3651 * needs to be performed.) 3652 */ 3653 public CodeableConcept getReasonCode() { 3654 if (this.reasonCode == null) 3655 if (Configuration.errorOnAutoCreate()) 3656 throw new Error("Attempt to auto-create Task.reasonCode"); 3657 else if (Configuration.doAutoCreate()) 3658 this.reasonCode = new CodeableConcept(); // cc 3659 return this.reasonCode; 3660 } 3661 3662 public boolean hasReasonCode() { 3663 return this.reasonCode != null && !this.reasonCode.isEmpty(); 3664 } 3665 3666 /** 3667 * @param value {@link #reasonCode} (A description or code indicating why this 3668 * task needs to be performed.) 3669 */ 3670 public Task setReasonCode(CodeableConcept value) { 3671 this.reasonCode = value; 3672 return this; 3673 } 3674 3675 /** 3676 * @return {@link #reasonReference} (A resource reference indicating why this 3677 * task needs to be performed.) 3678 */ 3679 public Reference getReasonReference() { 3680 if (this.reasonReference == null) 3681 if (Configuration.errorOnAutoCreate()) 3682 throw new Error("Attempt to auto-create Task.reasonReference"); 3683 else if (Configuration.doAutoCreate()) 3684 this.reasonReference = new Reference(); // cc 3685 return this.reasonReference; 3686 } 3687 3688 public boolean hasReasonReference() { 3689 return this.reasonReference != null && !this.reasonReference.isEmpty(); 3690 } 3691 3692 /** 3693 * @param value {@link #reasonReference} (A resource reference indicating why 3694 * this task needs to be performed.) 3695 */ 3696 public Task setReasonReference(Reference value) { 3697 this.reasonReference = value; 3698 return this; 3699 } 3700 3701 /** 3702 * @return {@link #reasonReference} The actual object that is the target of the 3703 * reference. The reference library doesn't populate this, but you can 3704 * use it to hold the resource if you resolve it. (A resource reference 3705 * indicating why this task needs to be performed.) 3706 */ 3707 public Resource getReasonReferenceTarget() { 3708 return this.reasonReferenceTarget; 3709 } 3710 3711 /** 3712 * @param value {@link #reasonReference} The actual object that is the target of 3713 * the reference. The reference library doesn't use these, but you 3714 * can use it to hold the resource if you resolve it. (A resource 3715 * reference indicating why this task needs to be performed.) 3716 */ 3717 public Task setReasonReferenceTarget(Resource value) { 3718 this.reasonReferenceTarget = value; 3719 return this; 3720 } 3721 3722 /** 3723 * @return {@link #insurance} (Insurance plans, coverage extensions, 3724 * pre-authorizations and/or pre-determinations that may be relevant to 3725 * the Task.) 3726 */ 3727 public List<Reference> getInsurance() { 3728 if (this.insurance == null) 3729 this.insurance = new ArrayList<Reference>(); 3730 return this.insurance; 3731 } 3732 3733 /** 3734 * @return Returns a reference to <code>this</code> for easy method chaining 3735 */ 3736 public Task setInsurance(List<Reference> theInsurance) { 3737 this.insurance = theInsurance; 3738 return this; 3739 } 3740 3741 public boolean hasInsurance() { 3742 if (this.insurance == null) 3743 return false; 3744 for (Reference item : this.insurance) 3745 if (!item.isEmpty()) 3746 return true; 3747 return false; 3748 } 3749 3750 public Reference addInsurance() { // 3 3751 Reference t = new Reference(); 3752 if (this.insurance == null) 3753 this.insurance = new ArrayList<Reference>(); 3754 this.insurance.add(t); 3755 return t; 3756 } 3757 3758 public Task addInsurance(Reference t) { // 3 3759 if (t == null) 3760 return this; 3761 if (this.insurance == null) 3762 this.insurance = new ArrayList<Reference>(); 3763 this.insurance.add(t); 3764 return this; 3765 } 3766 3767 /** 3768 * @return The first repetition of repeating field {@link #insurance}, creating 3769 * it if it does not already exist 3770 */ 3771 public Reference getInsuranceFirstRep() { 3772 if (getInsurance().isEmpty()) { 3773 addInsurance(); 3774 } 3775 return getInsurance().get(0); 3776 } 3777 3778 /** 3779 * @deprecated Use Reference#setResource(IBaseResource) instead 3780 */ 3781 @Deprecated 3782 public List<Resource> getInsuranceTarget() { 3783 if (this.insuranceTarget == null) 3784 this.insuranceTarget = new ArrayList<Resource>(); 3785 return this.insuranceTarget; 3786 } 3787 3788 /** 3789 * @return {@link #note} (Free-text information captured about the task as it 3790 * progresses.) 3791 */ 3792 public List<Annotation> getNote() { 3793 if (this.note == null) 3794 this.note = new ArrayList<Annotation>(); 3795 return this.note; 3796 } 3797 3798 /** 3799 * @return Returns a reference to <code>this</code> for easy method chaining 3800 */ 3801 public Task setNote(List<Annotation> theNote) { 3802 this.note = theNote; 3803 return this; 3804 } 3805 3806 public boolean hasNote() { 3807 if (this.note == null) 3808 return false; 3809 for (Annotation item : this.note) 3810 if (!item.isEmpty()) 3811 return true; 3812 return false; 3813 } 3814 3815 public Annotation addNote() { // 3 3816 Annotation t = new Annotation(); 3817 if (this.note == null) 3818 this.note = new ArrayList<Annotation>(); 3819 this.note.add(t); 3820 return t; 3821 } 3822 3823 public Task addNote(Annotation t) { // 3 3824 if (t == null) 3825 return this; 3826 if (this.note == null) 3827 this.note = new ArrayList<Annotation>(); 3828 this.note.add(t); 3829 return this; 3830 } 3831 3832 /** 3833 * @return The first repetition of repeating field {@link #note}, creating it if 3834 * it does not already exist 3835 */ 3836 public Annotation getNoteFirstRep() { 3837 if (getNote().isEmpty()) { 3838 addNote(); 3839 } 3840 return getNote().get(0); 3841 } 3842 3843 /** 3844 * @return {@link #relevantHistory} (Links to Provenance records for past 3845 * versions of this Task that identify key state transitions or updates 3846 * that are likely to be relevant to a user looking at the current 3847 * version of the task.) 3848 */ 3849 public List<Reference> getRelevantHistory() { 3850 if (this.relevantHistory == null) 3851 this.relevantHistory = new ArrayList<Reference>(); 3852 return this.relevantHistory; 3853 } 3854 3855 /** 3856 * @return Returns a reference to <code>this</code> for easy method chaining 3857 */ 3858 public Task setRelevantHistory(List<Reference> theRelevantHistory) { 3859 this.relevantHistory = theRelevantHistory; 3860 return this; 3861 } 3862 3863 public boolean hasRelevantHistory() { 3864 if (this.relevantHistory == null) 3865 return false; 3866 for (Reference item : this.relevantHistory) 3867 if (!item.isEmpty()) 3868 return true; 3869 return false; 3870 } 3871 3872 public Reference addRelevantHistory() { // 3 3873 Reference t = new Reference(); 3874 if (this.relevantHistory == null) 3875 this.relevantHistory = new ArrayList<Reference>(); 3876 this.relevantHistory.add(t); 3877 return t; 3878 } 3879 3880 public Task addRelevantHistory(Reference t) { // 3 3881 if (t == null) 3882 return this; 3883 if (this.relevantHistory == null) 3884 this.relevantHistory = new ArrayList<Reference>(); 3885 this.relevantHistory.add(t); 3886 return this; 3887 } 3888 3889 /** 3890 * @return The first repetition of repeating field {@link #relevantHistory}, 3891 * creating it if it does not already exist 3892 */ 3893 public Reference getRelevantHistoryFirstRep() { 3894 if (getRelevantHistory().isEmpty()) { 3895 addRelevantHistory(); 3896 } 3897 return getRelevantHistory().get(0); 3898 } 3899 3900 /** 3901 * @deprecated Use Reference#setResource(IBaseResource) instead 3902 */ 3903 @Deprecated 3904 public List<Provenance> getRelevantHistoryTarget() { 3905 if (this.relevantHistoryTarget == null) 3906 this.relevantHistoryTarget = new ArrayList<Provenance>(); 3907 return this.relevantHistoryTarget; 3908 } 3909 3910 /** 3911 * @deprecated Use Reference#setResource(IBaseResource) instead 3912 */ 3913 @Deprecated 3914 public Provenance addRelevantHistoryTarget() { 3915 Provenance r = new Provenance(); 3916 if (this.relevantHistoryTarget == null) 3917 this.relevantHistoryTarget = new ArrayList<Provenance>(); 3918 this.relevantHistoryTarget.add(r); 3919 return r; 3920 } 3921 3922 /** 3923 * @return {@link #restriction} (If the Task.focus is a request resource and the 3924 * task is seeking fulfillment (i.e. is asking for the request to be 3925 * actioned), this element identifies any limitations on what parts of 3926 * the referenced request should be actioned.) 3927 */ 3928 public TaskRestrictionComponent getRestriction() { 3929 if (this.restriction == null) 3930 if (Configuration.errorOnAutoCreate()) 3931 throw new Error("Attempt to auto-create Task.restriction"); 3932 else if (Configuration.doAutoCreate()) 3933 this.restriction = new TaskRestrictionComponent(); // cc 3934 return this.restriction; 3935 } 3936 3937 public boolean hasRestriction() { 3938 return this.restriction != null && !this.restriction.isEmpty(); 3939 } 3940 3941 /** 3942 * @param value {@link #restriction} (If the Task.focus is a request resource 3943 * and the task is seeking fulfillment (i.e. is asking for the 3944 * request to be actioned), this element identifies any limitations 3945 * on what parts of the referenced request should be actioned.) 3946 */ 3947 public Task setRestriction(TaskRestrictionComponent value) { 3948 this.restriction = value; 3949 return this; 3950 } 3951 3952 /** 3953 * @return {@link #input} (Additional information that may be needed in the 3954 * execution of the task.) 3955 */ 3956 public List<ParameterComponent> getInput() { 3957 if (this.input == null) 3958 this.input = new ArrayList<ParameterComponent>(); 3959 return this.input; 3960 } 3961 3962 /** 3963 * @return Returns a reference to <code>this</code> for easy method chaining 3964 */ 3965 public Task setInput(List<ParameterComponent> theInput) { 3966 this.input = theInput; 3967 return this; 3968 } 3969 3970 public boolean hasInput() { 3971 if (this.input == null) 3972 return false; 3973 for (ParameterComponent item : this.input) 3974 if (!item.isEmpty()) 3975 return true; 3976 return false; 3977 } 3978 3979 public ParameterComponent addInput() { // 3 3980 ParameterComponent t = new ParameterComponent(); 3981 if (this.input == null) 3982 this.input = new ArrayList<ParameterComponent>(); 3983 this.input.add(t); 3984 return t; 3985 } 3986 3987 public Task addInput(ParameterComponent t) { // 3 3988 if (t == null) 3989 return this; 3990 if (this.input == null) 3991 this.input = new ArrayList<ParameterComponent>(); 3992 this.input.add(t); 3993 return this; 3994 } 3995 3996 /** 3997 * @return The first repetition of repeating field {@link #input}, creating it 3998 * if it does not already exist 3999 */ 4000 public ParameterComponent getInputFirstRep() { 4001 if (getInput().isEmpty()) { 4002 addInput(); 4003 } 4004 return getInput().get(0); 4005 } 4006 4007 /** 4008 * @return {@link #output} (Outputs produced by the Task.) 4009 */ 4010 public List<TaskOutputComponent> getOutput() { 4011 if (this.output == null) 4012 this.output = new ArrayList<TaskOutputComponent>(); 4013 return this.output; 4014 } 4015 4016 /** 4017 * @return Returns a reference to <code>this</code> for easy method chaining 4018 */ 4019 public Task setOutput(List<TaskOutputComponent> theOutput) { 4020 this.output = theOutput; 4021 return this; 4022 } 4023 4024 public boolean hasOutput() { 4025 if (this.output == null) 4026 return false; 4027 for (TaskOutputComponent item : this.output) 4028 if (!item.isEmpty()) 4029 return true; 4030 return false; 4031 } 4032 4033 public TaskOutputComponent addOutput() { // 3 4034 TaskOutputComponent t = new TaskOutputComponent(); 4035 if (this.output == null) 4036 this.output = new ArrayList<TaskOutputComponent>(); 4037 this.output.add(t); 4038 return t; 4039 } 4040 4041 public Task addOutput(TaskOutputComponent t) { // 3 4042 if (t == null) 4043 return this; 4044 if (this.output == null) 4045 this.output = new ArrayList<TaskOutputComponent>(); 4046 this.output.add(t); 4047 return this; 4048 } 4049 4050 /** 4051 * @return The first repetition of repeating field {@link #output}, creating it 4052 * if it does not already exist 4053 */ 4054 public TaskOutputComponent getOutputFirstRep() { 4055 if (getOutput().isEmpty()) { 4056 addOutput(); 4057 } 4058 return getOutput().get(0); 4059 } 4060 4061 protected void listChildren(List<Property> children) { 4062 super.listChildren(children); 4063 children.add(new Property("identifier", "Identifier", "The business identifier for this task.", 0, 4064 java.lang.Integer.MAX_VALUE, identifier)); 4065 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition)", 4066 "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 4067 0, 1, instantiatesCanonical)); 4068 children.add(new Property("instantiatesUri", "uri", 4069 "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 4070 0, 1, instantiatesUri)); 4071 children.add(new Property("basedOn", "Reference(Any)", 4072 "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, ServiceRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a task is created to fulfill a procedureRequest ( = FocusOn ) to collect a specimen from a patient.", 4073 0, java.lang.Integer.MAX_VALUE, basedOn)); 4074 children.add(new Property("groupIdentifier", "Identifier", 4075 "An identifier that links together multiple tasks and other requests that were created in the same context.", 0, 4076 1, groupIdentifier)); 4077 children.add(new Property("partOf", "Reference(Task)", "Task that this particular task is part of.", 0, 4078 java.lang.Integer.MAX_VALUE, partOf)); 4079 children.add(new Property("status", "code", "The current status of the task.", 0, 1, status)); 4080 children.add(new Property("statusReason", "CodeableConcept", 4081 "An explanation as to why this task is held, failed, was refused, etc.", 0, 1, statusReason)); 4082 children.add(new Property("businessStatus", "CodeableConcept", 4083 "Contains business-specific nuances of the business state.", 0, 1, businessStatus)); 4084 children.add(new Property("intent", "code", 4085 "Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.", 4086 0, 1, intent)); 4087 children.add(new Property("priority", "code", 4088 "Indicates how quickly the Task should be addressed with respect to other requests.", 0, 1, priority)); 4089 children.add(new Property("code", "CodeableConcept", 4090 "A name or code (or both) briefly describing what the task involves.", 0, 1, code)); 4091 children.add(new Property("description", "string", "A free-text description of what is to be performed.", 0, 1, 4092 description)); 4093 children.add(new Property("focus", "Reference(Any)", 4094 "The request being actioned or the resource being manipulated by this task.", 0, 1, focus)); 4095 children.add(new Property("for", "Reference(Any)", 4096 "The entity who benefits from the performance of the service specified in the task (e.g., the patient).", 0, 1, 4097 for_)); 4098 children.add(new Property("encounter", "Reference(Encounter)", 4099 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.", 4100 0, 1, encounter)); 4101 children.add(new Property("executionPeriod", "Period", 4102 "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).", 4103 0, 1, executionPeriod)); 4104 children.add(new Property("authoredOn", "dateTime", "The date and time this task was created.", 0, 1, authoredOn)); 4105 children.add(new Property("lastModified", "dateTime", "The date and time of last modification to this task.", 0, 1, 4106 lastModified)); 4107 children.add( 4108 new Property("requester", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 4109 "The creator of the task.", 0, 1, requester)); 4110 children.add(new Property("performerType", "CodeableConcept", 4111 "The kind of participant that should perform the task.", 0, java.lang.Integer.MAX_VALUE, performerType)); 4112 children.add(new Property("owner", 4113 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", 4114 "Individual organization or Device currently responsible for task execution.", 0, 1, owner)); 4115 children.add(new Property("location", "Reference(Location)", 4116 "Principal physical location where the this task is performed.", 0, 1, location)); 4117 children.add(new Property("reasonCode", "CodeableConcept", 4118 "A description or code indicating why this task needs to be performed.", 0, 1, reasonCode)); 4119 children.add(new Property("reasonReference", "Reference(Any)", 4120 "A resource reference indicating why this task needs to be performed.", 0, 1, reasonReference)); 4121 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", 4122 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.", 4123 0, java.lang.Integer.MAX_VALUE, insurance)); 4124 children.add(new Property("note", "Annotation", "Free-text information captured about the task as it progresses.", 4125 0, java.lang.Integer.MAX_VALUE, note)); 4126 children.add(new Property("relevantHistory", "Reference(Provenance)", 4127 "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.", 4128 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 4129 children.add(new Property("restriction", "", 4130 "If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 4131 0, 1, restriction)); 4132 children.add(new Property("input", "", "Additional information that may be needed in the execution of the task.", 0, 4133 java.lang.Integer.MAX_VALUE, input)); 4134 children.add(new Property("output", "", "Outputs produced by the Task.", 0, java.lang.Integer.MAX_VALUE, output)); 4135 } 4136 4137 @Override 4138 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4139 switch (_hash) { 4140 case -1618432855: 4141 /* identifier */ return new Property("identifier", "Identifier", "The business identifier for this task.", 0, 4142 java.lang.Integer.MAX_VALUE, identifier); 4143 case 8911915: 4144 /* instantiatesCanonical */ return new Property("instantiatesCanonical", "canonical(ActivityDefinition)", 4145 "The URL pointing to a *FHIR*-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 4146 0, 1, instantiatesCanonical); 4147 case -1926393373: 4148 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 4149 "The URL pointing to an *externally* maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this Task.", 4150 0, 1, instantiatesUri); 4151 case -332612366: 4152 /* basedOn */ return new Property("basedOn", "Reference(Any)", 4153 "BasedOn refers to a higher-level authorization that triggered the creation of the task. It references a \"request\" resource such as a ServiceRequest, MedicationRequest, ServiceRequest, CarePlan, etc. which is distinct from the \"request\" resource the task is seeking to fulfill. This latter resource is referenced by FocusOn. For example, based on a ServiceRequest (= BasedOn), a task is created to fulfill a procedureRequest ( = FocusOn ) to collect a specimen from a patient.", 4154 0, java.lang.Integer.MAX_VALUE, basedOn); 4155 case -445338488: 4156 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", 4157 "An identifier that links together multiple tasks and other requests that were created in the same context.", 4158 0, 1, groupIdentifier); 4159 case -995410646: 4160 /* partOf */ return new Property("partOf", "Reference(Task)", "Task that this particular task is part of.", 0, 4161 java.lang.Integer.MAX_VALUE, partOf); 4162 case -892481550: 4163 /* status */ return new Property("status", "code", "The current status of the task.", 0, 1, status); 4164 case 2051346646: 4165 /* statusReason */ return new Property("statusReason", "CodeableConcept", 4166 "An explanation as to why this task is held, failed, was refused, etc.", 0, 1, statusReason); 4167 case 2008591314: 4168 /* businessStatus */ return new Property("businessStatus", "CodeableConcept", 4169 "Contains business-specific nuances of the business state.", 0, 1, businessStatus); 4170 case -1183762788: 4171 /* intent */ return new Property("intent", "code", 4172 "Indicates the \"level\" of actionability associated with the Task, i.e. i+R[9]Cs this a proposed task, a planned task, an actionable task, etc.", 4173 0, 1, intent); 4174 case -1165461084: 4175 /* priority */ return new Property("priority", "code", 4176 "Indicates how quickly the Task should be addressed with respect to other requests.", 0, 1, priority); 4177 case 3059181: 4178 /* code */ return new Property("code", "CodeableConcept", 4179 "A name or code (or both) briefly describing what the task involves.", 0, 1, code); 4180 case -1724546052: 4181 /* description */ return new Property("description", "string", 4182 "A free-text description of what is to be performed.", 0, 1, description); 4183 case 97604824: 4184 /* focus */ return new Property("focus", "Reference(Any)", 4185 "The request being actioned or the resource being manipulated by this task.", 0, 1, focus); 4186 case 101577: 4187 /* for */ return new Property("for", "Reference(Any)", 4188 "The entity who benefits from the performance of the service specified in the task (e.g., the patient).", 0, 4189 1, for_); 4190 case 1524132147: 4191 /* encounter */ return new Property("encounter", "Reference(Encounter)", 4192 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this task was created.", 4193 0, 1, encounter); 4194 case 1218624249: 4195 /* executionPeriod */ return new Property("executionPeriod", "Period", 4196 "Identifies the time action was first taken against the task (start) and/or the time final action was taken against the task prior to marking it as completed (end).", 4197 0, 1, executionPeriod); 4198 case -1500852503: 4199 /* authoredOn */ return new Property("authoredOn", "dateTime", "The date and time this task was created.", 0, 1, 4200 authoredOn); 4201 case 1959003007: 4202 /* lastModified */ return new Property("lastModified", "dateTime", 4203 "The date and time of last modification to this task.", 0, 1, lastModified); 4204 case 693933948: 4205 /* requester */ return new Property("requester", 4206 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 4207 "The creator of the task.", 0, 1, requester); 4208 case -901444568: 4209 /* performerType */ return new Property("performerType", "CodeableConcept", 4210 "The kind of participant that should perform the task.", 0, java.lang.Integer.MAX_VALUE, performerType); 4211 case 106164915: 4212 /* owner */ return new Property("owner", 4213 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|HealthcareService|Patient|Device|RelatedPerson)", 4214 "Individual organization or Device currently responsible for task execution.", 0, 1, owner); 4215 case 1901043637: 4216 /* location */ return new Property("location", "Reference(Location)", 4217 "Principal physical location where the this task is performed.", 0, 1, location); 4218 case 722137681: 4219 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 4220 "A description or code indicating why this task needs to be performed.", 0, 1, reasonCode); 4221 case -1146218137: 4222 /* reasonReference */ return new Property("reasonReference", "Reference(Any)", 4223 "A resource reference indicating why this task needs to be performed.", 0, 1, reasonReference); 4224 case 73049818: 4225 /* insurance */ return new Property("insurance", "Reference(Coverage|ClaimResponse)", 4226 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be relevant to the Task.", 4227 0, java.lang.Integer.MAX_VALUE, insurance); 4228 case 3387378: 4229 /* note */ return new Property("note", "Annotation", 4230 "Free-text information captured about the task as it progresses.", 0, java.lang.Integer.MAX_VALUE, note); 4231 case 1538891575: 4232 /* relevantHistory */ return new Property("relevantHistory", "Reference(Provenance)", 4233 "Links to Provenance records for past versions of this Task that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the task.", 4234 0, java.lang.Integer.MAX_VALUE, relevantHistory); 4235 case -1561062452: 4236 /* restriction */ return new Property("restriction", "", 4237 "If the Task.focus is a request resource and the task is seeking fulfillment (i.e. is asking for the request to be actioned), this element identifies any limitations on what parts of the referenced request should be actioned.", 4238 0, 1, restriction); 4239 case 100358090: 4240 /* input */ return new Property("input", "", 4241 "Additional information that may be needed in the execution of the task.", 0, java.lang.Integer.MAX_VALUE, 4242 input); 4243 case -1005512447: 4244 /* output */ return new Property("output", "", "Outputs produced by the Task.", 0, java.lang.Integer.MAX_VALUE, 4245 output); 4246 default: 4247 return super.getNamedProperty(_hash, _name, _checkValid); 4248 } 4249 4250 } 4251 4252 @Override 4253 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4254 switch (hash) { 4255 case -1618432855: 4256 /* identifier */ return this.identifier == null ? new Base[0] 4257 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4258 case 8911915: 4259 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 4260 : new Base[] { this.instantiatesCanonical }; // CanonicalType 4261 case -1926393373: 4262 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] : new Base[] { this.instantiatesUri }; // UriType 4263 case -332612366: 4264 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4265 case -445338488: 4266 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 4267 case -995410646: 4268 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 4269 case -892481550: 4270 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<TaskStatus> 4271 case 2051346646: 4272 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 4273 case 2008591314: 4274 /* businessStatus */ return this.businessStatus == null ? new Base[0] : new Base[] { this.businessStatus }; // CodeableConcept 4275 case -1183762788: 4276 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<TaskIntent> 4277 case -1165461084: 4278 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<TaskPriority> 4279 case 3059181: 4280 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 4281 case -1724546052: 4282 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 4283 case 97604824: 4284 /* focus */ return this.focus == null ? new Base[0] : new Base[] { this.focus }; // Reference 4285 case 101577: 4286 /* for */ return this.for_ == null ? new Base[0] : new Base[] { this.for_ }; // Reference 4287 case 1524132147: 4288 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 4289 case 1218624249: 4290 /* executionPeriod */ return this.executionPeriod == null ? new Base[0] : new Base[] { this.executionPeriod }; // Period 4291 case -1500852503: 4292 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 4293 case 1959003007: 4294 /* lastModified */ return this.lastModified == null ? new Base[0] : new Base[] { this.lastModified }; // DateTimeType 4295 case 693933948: 4296 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 4297 case -901444568: 4298 /* performerType */ return this.performerType == null ? new Base[0] 4299 : this.performerType.toArray(new Base[this.performerType.size()]); // CodeableConcept 4300 case 106164915: 4301 /* owner */ return this.owner == null ? new Base[0] : new Base[] { this.owner }; // Reference 4302 case 1901043637: 4303 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 4304 case 722137681: 4305 /* reasonCode */ return this.reasonCode == null ? new Base[0] : new Base[] { this.reasonCode }; // CodeableConcept 4306 case -1146218137: 4307 /* reasonReference */ return this.reasonReference == null ? new Base[0] : new Base[] { this.reasonReference }; // Reference 4308 case 73049818: 4309 /* insurance */ return this.insurance == null ? new Base[0] 4310 : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 4311 case 3387378: 4312 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4313 case 1538891575: 4314 /* relevantHistory */ return this.relevantHistory == null ? new Base[0] 4315 : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 4316 case -1561062452: 4317 /* restriction */ return this.restriction == null ? new Base[0] : new Base[] { this.restriction }; // TaskRestrictionComponent 4318 case 100358090: 4319 /* input */ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // ParameterComponent 4320 case -1005512447: 4321 /* output */ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // TaskOutputComponent 4322 default: 4323 return super.getProperty(hash, name, checkValid); 4324 } 4325 4326 } 4327 4328 @Override 4329 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4330 switch (hash) { 4331 case -1618432855: // identifier 4332 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4333 return value; 4334 case 8911915: // instantiatesCanonical 4335 this.instantiatesCanonical = castToCanonical(value); // CanonicalType 4336 return value; 4337 case -1926393373: // instantiatesUri 4338 this.instantiatesUri = castToUri(value); // UriType 4339 return value; 4340 case -332612366: // basedOn 4341 this.getBasedOn().add(castToReference(value)); // Reference 4342 return value; 4343 case -445338488: // groupIdentifier 4344 this.groupIdentifier = castToIdentifier(value); // Identifier 4345 return value; 4346 case -995410646: // partOf 4347 this.getPartOf().add(castToReference(value)); // Reference 4348 return value; 4349 case -892481550: // status 4350 value = new TaskStatusEnumFactory().fromType(castToCode(value)); 4351 this.status = (Enumeration) value; // Enumeration<TaskStatus> 4352 return value; 4353 case 2051346646: // statusReason 4354 this.statusReason = castToCodeableConcept(value); // CodeableConcept 4355 return value; 4356 case 2008591314: // businessStatus 4357 this.businessStatus = castToCodeableConcept(value); // CodeableConcept 4358 return value; 4359 case -1183762788: // intent 4360 value = new TaskIntentEnumFactory().fromType(castToCode(value)); 4361 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 4362 return value; 4363 case -1165461084: // priority 4364 value = new TaskPriorityEnumFactory().fromType(castToCode(value)); 4365 this.priority = (Enumeration) value; // Enumeration<TaskPriority> 4366 return value; 4367 case 3059181: // code 4368 this.code = castToCodeableConcept(value); // CodeableConcept 4369 return value; 4370 case -1724546052: // description 4371 this.description = castToString(value); // StringType 4372 return value; 4373 case 97604824: // focus 4374 this.focus = castToReference(value); // Reference 4375 return value; 4376 case 101577: // for 4377 this.for_ = castToReference(value); // Reference 4378 return value; 4379 case 1524132147: // encounter 4380 this.encounter = castToReference(value); // Reference 4381 return value; 4382 case 1218624249: // executionPeriod 4383 this.executionPeriod = castToPeriod(value); // Period 4384 return value; 4385 case -1500852503: // authoredOn 4386 this.authoredOn = castToDateTime(value); // DateTimeType 4387 return value; 4388 case 1959003007: // lastModified 4389 this.lastModified = castToDateTime(value); // DateTimeType 4390 return value; 4391 case 693933948: // requester 4392 this.requester = castToReference(value); // Reference 4393 return value; 4394 case -901444568: // performerType 4395 this.getPerformerType().add(castToCodeableConcept(value)); // CodeableConcept 4396 return value; 4397 case 106164915: // owner 4398 this.owner = castToReference(value); // Reference 4399 return value; 4400 case 1901043637: // location 4401 this.location = castToReference(value); // Reference 4402 return value; 4403 case 722137681: // reasonCode 4404 this.reasonCode = castToCodeableConcept(value); // CodeableConcept 4405 return value; 4406 case -1146218137: // reasonReference 4407 this.reasonReference = castToReference(value); // Reference 4408 return value; 4409 case 73049818: // insurance 4410 this.getInsurance().add(castToReference(value)); // Reference 4411 return value; 4412 case 3387378: // note 4413 this.getNote().add(castToAnnotation(value)); // Annotation 4414 return value; 4415 case 1538891575: // relevantHistory 4416 this.getRelevantHistory().add(castToReference(value)); // Reference 4417 return value; 4418 case -1561062452: // restriction 4419 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 4420 return value; 4421 case 100358090: // input 4422 this.getInput().add((ParameterComponent) value); // ParameterComponent 4423 return value; 4424 case -1005512447: // output 4425 this.getOutput().add((TaskOutputComponent) value); // TaskOutputComponent 4426 return value; 4427 default: 4428 return super.setProperty(hash, name, value); 4429 } 4430 4431 } 4432 4433 @Override 4434 public Base setProperty(String name, Base value) throws FHIRException { 4435 if (name.equals("identifier")) { 4436 this.getIdentifier().add(castToIdentifier(value)); 4437 } else if (name.equals("instantiatesCanonical")) { 4438 this.instantiatesCanonical = castToCanonical(value); // CanonicalType 4439 } else if (name.equals("instantiatesUri")) { 4440 this.instantiatesUri = castToUri(value); // UriType 4441 } else if (name.equals("basedOn")) { 4442 this.getBasedOn().add(castToReference(value)); 4443 } else if (name.equals("groupIdentifier")) { 4444 this.groupIdentifier = castToIdentifier(value); // Identifier 4445 } else if (name.equals("partOf")) { 4446 this.getPartOf().add(castToReference(value)); 4447 } else if (name.equals("status")) { 4448 value = new TaskStatusEnumFactory().fromType(castToCode(value)); 4449 this.status = (Enumeration) value; // Enumeration<TaskStatus> 4450 } else if (name.equals("statusReason")) { 4451 this.statusReason = castToCodeableConcept(value); // CodeableConcept 4452 } else if (name.equals("businessStatus")) { 4453 this.businessStatus = castToCodeableConcept(value); // CodeableConcept 4454 } else if (name.equals("intent")) { 4455 value = new TaskIntentEnumFactory().fromType(castToCode(value)); 4456 this.intent = (Enumeration) value; // Enumeration<TaskIntent> 4457 } else if (name.equals("priority")) { 4458 value = new TaskPriorityEnumFactory().fromType(castToCode(value)); 4459 this.priority = (Enumeration) value; // Enumeration<TaskPriority> 4460 } else if (name.equals("code")) { 4461 this.code = castToCodeableConcept(value); // CodeableConcept 4462 } else if (name.equals("description")) { 4463 this.description = castToString(value); // StringType 4464 } else if (name.equals("focus")) { 4465 this.focus = castToReference(value); // Reference 4466 } else if (name.equals("for")) { 4467 this.for_ = castToReference(value); // Reference 4468 } else if (name.equals("encounter")) { 4469 this.encounter = castToReference(value); // Reference 4470 } else if (name.equals("executionPeriod")) { 4471 this.executionPeriod = castToPeriod(value); // Period 4472 } else if (name.equals("authoredOn")) { 4473 this.authoredOn = castToDateTime(value); // DateTimeType 4474 } else if (name.equals("lastModified")) { 4475 this.lastModified = castToDateTime(value); // DateTimeType 4476 } else if (name.equals("requester")) { 4477 this.requester = castToReference(value); // Reference 4478 } else if (name.equals("performerType")) { 4479 this.getPerformerType().add(castToCodeableConcept(value)); 4480 } else if (name.equals("owner")) { 4481 this.owner = castToReference(value); // Reference 4482 } else if (name.equals("location")) { 4483 this.location = castToReference(value); // Reference 4484 } else if (name.equals("reasonCode")) { 4485 this.reasonCode = castToCodeableConcept(value); // CodeableConcept 4486 } else if (name.equals("reasonReference")) { 4487 this.reasonReference = castToReference(value); // Reference 4488 } else if (name.equals("insurance")) { 4489 this.getInsurance().add(castToReference(value)); 4490 } else if (name.equals("note")) { 4491 this.getNote().add(castToAnnotation(value)); 4492 } else if (name.equals("relevantHistory")) { 4493 this.getRelevantHistory().add(castToReference(value)); 4494 } else if (name.equals("restriction")) { 4495 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 4496 } else if (name.equals("input")) { 4497 this.getInput().add((ParameterComponent) value); 4498 } else if (name.equals("output")) { 4499 this.getOutput().add((TaskOutputComponent) value); 4500 } else 4501 return super.setProperty(name, value); 4502 return value; 4503 } 4504 4505 @Override 4506 public void removeChild(String name, Base value) throws FHIRException { 4507 if (name.equals("identifier")) { 4508 this.getIdentifier().remove(castToIdentifier(value)); 4509 } else if (name.equals("instantiatesCanonical")) { 4510 this.instantiatesCanonical = null; 4511 } else if (name.equals("instantiatesUri")) { 4512 this.instantiatesUri = null; 4513 } else if (name.equals("basedOn")) { 4514 this.getBasedOn().remove(castToReference(value)); 4515 } else if (name.equals("groupIdentifier")) { 4516 this.groupIdentifier = null; 4517 } else if (name.equals("partOf")) { 4518 this.getPartOf().remove(castToReference(value)); 4519 } else if (name.equals("status")) { 4520 this.status = null; 4521 } else if (name.equals("statusReason")) { 4522 this.statusReason = null; 4523 } else if (name.equals("businessStatus")) { 4524 this.businessStatus = null; 4525 } else if (name.equals("intent")) { 4526 this.intent = null; 4527 } else if (name.equals("priority")) { 4528 this.priority = null; 4529 } else if (name.equals("code")) { 4530 this.code = null; 4531 } else if (name.equals("description")) { 4532 this.description = null; 4533 } else if (name.equals("focus")) { 4534 this.focus = null; 4535 } else if (name.equals("for")) { 4536 this.for_ = null; 4537 } else if (name.equals("encounter")) { 4538 this.encounter = null; 4539 } else if (name.equals("executionPeriod")) { 4540 this.executionPeriod = null; 4541 } else if (name.equals("authoredOn")) { 4542 this.authoredOn = null; 4543 } else if (name.equals("lastModified")) { 4544 this.lastModified = null; 4545 } else if (name.equals("requester")) { 4546 this.requester = null; 4547 } else if (name.equals("performerType")) { 4548 this.getPerformerType().remove(castToCodeableConcept(value)); 4549 } else if (name.equals("owner")) { 4550 this.owner = null; 4551 } else if (name.equals("location")) { 4552 this.location = null; 4553 } else if (name.equals("reasonCode")) { 4554 this.reasonCode = null; 4555 } else if (name.equals("reasonReference")) { 4556 this.reasonReference = null; 4557 } else if (name.equals("insurance")) { 4558 this.getInsurance().remove(castToReference(value)); 4559 } else if (name.equals("note")) { 4560 this.getNote().remove(castToAnnotation(value)); 4561 } else if (name.equals("relevantHistory")) { 4562 this.getRelevantHistory().remove(castToReference(value)); 4563 } else if (name.equals("restriction")) { 4564 this.restriction = (TaskRestrictionComponent) value; // TaskRestrictionComponent 4565 } else if (name.equals("input")) { 4566 this.getInput().remove((ParameterComponent) value); 4567 } else if (name.equals("output")) { 4568 this.getOutput().remove((TaskOutputComponent) value); 4569 } else 4570 super.removeChild(name, value); 4571 4572 } 4573 4574 @Override 4575 public Base makeProperty(int hash, String name) throws FHIRException { 4576 switch (hash) { 4577 case -1618432855: 4578 return addIdentifier(); 4579 case 8911915: 4580 return getInstantiatesCanonicalElement(); 4581 case -1926393373: 4582 return getInstantiatesUriElement(); 4583 case -332612366: 4584 return addBasedOn(); 4585 case -445338488: 4586 return getGroupIdentifier(); 4587 case -995410646: 4588 return addPartOf(); 4589 case -892481550: 4590 return getStatusElement(); 4591 case 2051346646: 4592 return getStatusReason(); 4593 case 2008591314: 4594 return getBusinessStatus(); 4595 case -1183762788: 4596 return getIntentElement(); 4597 case -1165461084: 4598 return getPriorityElement(); 4599 case 3059181: 4600 return getCode(); 4601 case -1724546052: 4602 return getDescriptionElement(); 4603 case 97604824: 4604 return getFocus(); 4605 case 101577: 4606 return getFor(); 4607 case 1524132147: 4608 return getEncounter(); 4609 case 1218624249: 4610 return getExecutionPeriod(); 4611 case -1500852503: 4612 return getAuthoredOnElement(); 4613 case 1959003007: 4614 return getLastModifiedElement(); 4615 case 693933948: 4616 return getRequester(); 4617 case -901444568: 4618 return addPerformerType(); 4619 case 106164915: 4620 return getOwner(); 4621 case 1901043637: 4622 return getLocation(); 4623 case 722137681: 4624 return getReasonCode(); 4625 case -1146218137: 4626 return getReasonReference(); 4627 case 73049818: 4628 return addInsurance(); 4629 case 3387378: 4630 return addNote(); 4631 case 1538891575: 4632 return addRelevantHistory(); 4633 case -1561062452: 4634 return getRestriction(); 4635 case 100358090: 4636 return addInput(); 4637 case -1005512447: 4638 return addOutput(); 4639 default: 4640 return super.makeProperty(hash, name); 4641 } 4642 4643 } 4644 4645 @Override 4646 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4647 switch (hash) { 4648 case -1618432855: 4649 /* identifier */ return new String[] { "Identifier" }; 4650 case 8911915: 4651 /* instantiatesCanonical */ return new String[] { "canonical" }; 4652 case -1926393373: 4653 /* instantiatesUri */ return new String[] { "uri" }; 4654 case -332612366: 4655 /* basedOn */ return new String[] { "Reference" }; 4656 case -445338488: 4657 /* groupIdentifier */ return new String[] { "Identifier" }; 4658 case -995410646: 4659 /* partOf */ return new String[] { "Reference" }; 4660 case -892481550: 4661 /* status */ return new String[] { "code" }; 4662 case 2051346646: 4663 /* statusReason */ return new String[] { "CodeableConcept" }; 4664 case 2008591314: 4665 /* businessStatus */ return new String[] { "CodeableConcept" }; 4666 case -1183762788: 4667 /* intent */ return new String[] { "code" }; 4668 case -1165461084: 4669 /* priority */ return new String[] { "code" }; 4670 case 3059181: 4671 /* code */ return new String[] { "CodeableConcept" }; 4672 case -1724546052: 4673 /* description */ return new String[] { "string" }; 4674 case 97604824: 4675 /* focus */ return new String[] { "Reference" }; 4676 case 101577: 4677 /* for */ return new String[] { "Reference" }; 4678 case 1524132147: 4679 /* encounter */ return new String[] { "Reference" }; 4680 case 1218624249: 4681 /* executionPeriod */ return new String[] { "Period" }; 4682 case -1500852503: 4683 /* authoredOn */ return new String[] { "dateTime" }; 4684 case 1959003007: 4685 /* lastModified */ return new String[] { "dateTime" }; 4686 case 693933948: 4687 /* requester */ return new String[] { "Reference" }; 4688 case -901444568: 4689 /* performerType */ return new String[] { "CodeableConcept" }; 4690 case 106164915: 4691 /* owner */ return new String[] { "Reference" }; 4692 case 1901043637: 4693 /* location */ return new String[] { "Reference" }; 4694 case 722137681: 4695 /* reasonCode */ return new String[] { "CodeableConcept" }; 4696 case -1146218137: 4697 /* reasonReference */ return new String[] { "Reference" }; 4698 case 73049818: 4699 /* insurance */ return new String[] { "Reference" }; 4700 case 3387378: 4701 /* note */ return new String[] { "Annotation" }; 4702 case 1538891575: 4703 /* relevantHistory */ return new String[] { "Reference" }; 4704 case -1561062452: 4705 /* restriction */ return new String[] {}; 4706 case 100358090: 4707 /* input */ return new String[] {}; 4708 case -1005512447: 4709 /* output */ return new String[] {}; 4710 default: 4711 return super.getTypesForProperty(hash, name); 4712 } 4713 4714 } 4715 4716 @Override 4717 public Base addChild(String name) throws FHIRException { 4718 if (name.equals("identifier")) { 4719 return addIdentifier(); 4720 } else if (name.equals("instantiatesCanonical")) { 4721 throw new FHIRException("Cannot call addChild on a singleton property Task.instantiatesCanonical"); 4722 } else if (name.equals("instantiatesUri")) { 4723 throw new FHIRException("Cannot call addChild on a singleton property Task.instantiatesUri"); 4724 } else if (name.equals("basedOn")) { 4725 return addBasedOn(); 4726 } else if (name.equals("groupIdentifier")) { 4727 this.groupIdentifier = new Identifier(); 4728 return this.groupIdentifier; 4729 } else if (name.equals("partOf")) { 4730 return addPartOf(); 4731 } else if (name.equals("status")) { 4732 throw new FHIRException("Cannot call addChild on a singleton property Task.status"); 4733 } else if (name.equals("statusReason")) { 4734 this.statusReason = new CodeableConcept(); 4735 return this.statusReason; 4736 } else if (name.equals("businessStatus")) { 4737 this.businessStatus = new CodeableConcept(); 4738 return this.businessStatus; 4739 } else if (name.equals("intent")) { 4740 throw new FHIRException("Cannot call addChild on a singleton property Task.intent"); 4741 } else if (name.equals("priority")) { 4742 throw new FHIRException("Cannot call addChild on a singleton property Task.priority"); 4743 } else if (name.equals("code")) { 4744 this.code = new CodeableConcept(); 4745 return this.code; 4746 } else if (name.equals("description")) { 4747 throw new FHIRException("Cannot call addChild on a singleton property Task.description"); 4748 } else if (name.equals("focus")) { 4749 this.focus = new Reference(); 4750 return this.focus; 4751 } else if (name.equals("for")) { 4752 this.for_ = new Reference(); 4753 return this.for_; 4754 } else if (name.equals("encounter")) { 4755 this.encounter = new Reference(); 4756 return this.encounter; 4757 } else if (name.equals("executionPeriod")) { 4758 this.executionPeriod = new Period(); 4759 return this.executionPeriod; 4760 } else if (name.equals("authoredOn")) { 4761 throw new FHIRException("Cannot call addChild on a singleton property Task.authoredOn"); 4762 } else if (name.equals("lastModified")) { 4763 throw new FHIRException("Cannot call addChild on a singleton property Task.lastModified"); 4764 } else if (name.equals("requester")) { 4765 this.requester = new Reference(); 4766 return this.requester; 4767 } else if (name.equals("performerType")) { 4768 return addPerformerType(); 4769 } else if (name.equals("owner")) { 4770 this.owner = new Reference(); 4771 return this.owner; 4772 } else if (name.equals("location")) { 4773 this.location = new Reference(); 4774 return this.location; 4775 } else if (name.equals("reasonCode")) { 4776 this.reasonCode = new CodeableConcept(); 4777 return this.reasonCode; 4778 } else if (name.equals("reasonReference")) { 4779 this.reasonReference = new Reference(); 4780 return this.reasonReference; 4781 } else if (name.equals("insurance")) { 4782 return addInsurance(); 4783 } else if (name.equals("note")) { 4784 return addNote(); 4785 } else if (name.equals("relevantHistory")) { 4786 return addRelevantHistory(); 4787 } else if (name.equals("restriction")) { 4788 this.restriction = new TaskRestrictionComponent(); 4789 return this.restriction; 4790 } else if (name.equals("input")) { 4791 return addInput(); 4792 } else if (name.equals("output")) { 4793 return addOutput(); 4794 } else 4795 return super.addChild(name); 4796 } 4797 4798 public String fhirType() { 4799 return "Task"; 4800 4801 } 4802 4803 public Task copy() { 4804 Task dst = new Task(); 4805 copyValues(dst); 4806 return dst; 4807 } 4808 4809 public void copyValues(Task dst) { 4810 super.copyValues(dst); 4811 if (identifier != null) { 4812 dst.identifier = new ArrayList<Identifier>(); 4813 for (Identifier i : identifier) 4814 dst.identifier.add(i.copy()); 4815 } 4816 ; 4817 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 4818 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 4819 if (basedOn != null) { 4820 dst.basedOn = new ArrayList<Reference>(); 4821 for (Reference i : basedOn) 4822 dst.basedOn.add(i.copy()); 4823 } 4824 ; 4825 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 4826 if (partOf != null) { 4827 dst.partOf = new ArrayList<Reference>(); 4828 for (Reference i : partOf) 4829 dst.partOf.add(i.copy()); 4830 } 4831 ; 4832 dst.status = status == null ? null : status.copy(); 4833 dst.statusReason = statusReason == null ? null : statusReason.copy(); 4834 dst.businessStatus = businessStatus == null ? null : businessStatus.copy(); 4835 dst.intent = intent == null ? null : intent.copy(); 4836 dst.priority = priority == null ? null : priority.copy(); 4837 dst.code = code == null ? null : code.copy(); 4838 dst.description = description == null ? null : description.copy(); 4839 dst.focus = focus == null ? null : focus.copy(); 4840 dst.for_ = for_ == null ? null : for_.copy(); 4841 dst.encounter = encounter == null ? null : encounter.copy(); 4842 dst.executionPeriod = executionPeriod == null ? null : executionPeriod.copy(); 4843 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 4844 dst.lastModified = lastModified == null ? null : lastModified.copy(); 4845 dst.requester = requester == null ? null : requester.copy(); 4846 if (performerType != null) { 4847 dst.performerType = new ArrayList<CodeableConcept>(); 4848 for (CodeableConcept i : performerType) 4849 dst.performerType.add(i.copy()); 4850 } 4851 ; 4852 dst.owner = owner == null ? null : owner.copy(); 4853 dst.location = location == null ? null : location.copy(); 4854 dst.reasonCode = reasonCode == null ? null : reasonCode.copy(); 4855 dst.reasonReference = reasonReference == null ? null : reasonReference.copy(); 4856 if (insurance != null) { 4857 dst.insurance = new ArrayList<Reference>(); 4858 for (Reference i : insurance) 4859 dst.insurance.add(i.copy()); 4860 } 4861 ; 4862 if (note != null) { 4863 dst.note = new ArrayList<Annotation>(); 4864 for (Annotation i : note) 4865 dst.note.add(i.copy()); 4866 } 4867 ; 4868 if (relevantHistory != null) { 4869 dst.relevantHistory = new ArrayList<Reference>(); 4870 for (Reference i : relevantHistory) 4871 dst.relevantHistory.add(i.copy()); 4872 } 4873 ; 4874 dst.restriction = restriction == null ? null : restriction.copy(); 4875 if (input != null) { 4876 dst.input = new ArrayList<ParameterComponent>(); 4877 for (ParameterComponent i : input) 4878 dst.input.add(i.copy()); 4879 } 4880 ; 4881 if (output != null) { 4882 dst.output = new ArrayList<TaskOutputComponent>(); 4883 for (TaskOutputComponent i : output) 4884 dst.output.add(i.copy()); 4885 } 4886 ; 4887 } 4888 4889 protected Task typedCopy() { 4890 return copy(); 4891 } 4892 4893 @Override 4894 public boolean equalsDeep(Base other_) { 4895 if (!super.equalsDeep(other_)) 4896 return false; 4897 if (!(other_ instanceof Task)) 4898 return false; 4899 Task o = (Task) other_; 4900 return compareDeep(identifier, o.identifier, true) 4901 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 4902 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 4903 && compareDeep(groupIdentifier, o.groupIdentifier, true) && compareDeep(partOf, o.partOf, true) 4904 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 4905 && compareDeep(businessStatus, o.businessStatus, true) && compareDeep(intent, o.intent, true) 4906 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 4907 && compareDeep(description, o.description, true) && compareDeep(focus, o.focus, true) 4908 && compareDeep(for_, o.for_, true) && compareDeep(encounter, o.encounter, true) 4909 && compareDeep(executionPeriod, o.executionPeriod, true) && compareDeep(authoredOn, o.authoredOn, true) 4910 && compareDeep(lastModified, o.lastModified, true) && compareDeep(requester, o.requester, true) 4911 && compareDeep(performerType, o.performerType, true) && compareDeep(owner, o.owner, true) 4912 && compareDeep(location, o.location, true) && compareDeep(reasonCode, o.reasonCode, true) 4913 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(insurance, o.insurance, true) 4914 && compareDeep(note, o.note, true) && compareDeep(relevantHistory, o.relevantHistory, true) 4915 && compareDeep(restriction, o.restriction, true) && compareDeep(input, o.input, true) 4916 && compareDeep(output, o.output, true); 4917 } 4918 4919 @Override 4920 public boolean equalsShallow(Base other_) { 4921 if (!super.equalsShallow(other_)) 4922 return false; 4923 if (!(other_ instanceof Task)) 4924 return false; 4925 Task o = (Task) other_; 4926 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 4927 && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 4928 && compareValues(description, o.description, true) && compareValues(authoredOn, o.authoredOn, true) 4929 && compareValues(lastModified, o.lastModified, true); 4930 } 4931 4932 public boolean isEmpty() { 4933 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 4934 basedOn, groupIdentifier, partOf, status, statusReason, businessStatus, intent, priority, code, description, 4935 focus, for_, encounter, executionPeriod, authoredOn, lastModified, requester, performerType, owner, location, 4936 reasonCode, reasonReference, insurance, note, relevantHistory, restriction, input, output); 4937 } 4938 4939 @Override 4940 public ResourceType getResourceType() { 4941 return ResourceType.Task; 4942 } 4943 4944 /** 4945 * Search parameter: <b>owner</b> 4946 * <p> 4947 * Description: <b>Search by task owner</b><br> 4948 * Type: <b>reference</b><br> 4949 * Path: <b>Task.owner</b><br> 4950 * </p> 4951 */ 4952 @SearchParamDefinition(name = "owner", path = "Task.owner", description = "Search by task owner", type = "reference", target = { 4953 CareTeam.class, Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, 4954 PractitionerRole.class, RelatedPerson.class }) 4955 public static final String SP_OWNER = "owner"; 4956 /** 4957 * <b>Fluent Client</b> search parameter constant for <b>owner</b> 4958 * <p> 4959 * Description: <b>Search by task owner</b><br> 4960 * Type: <b>reference</b><br> 4961 * Path: <b>Task.owner</b><br> 4962 * </p> 4963 */ 4964 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4965 SP_OWNER); 4966 4967 /** 4968 * Constant for fluent queries to be used to add include statements. Specifies 4969 * the path value of "<b>Task:owner</b>". 4970 */ 4971 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNER = new ca.uhn.fhir.model.api.Include("Task:owner") 4972 .toLocked(); 4973 4974 /** 4975 * Search parameter: <b>requester</b> 4976 * <p> 4977 * Description: <b>Search by task requester</b><br> 4978 * Type: <b>reference</b><br> 4979 * Path: <b>Task.requester</b><br> 4980 * </p> 4981 */ 4982 @SearchParamDefinition(name = "requester", path = "Task.requester", description = "Search by task requester", type = "reference", target = { 4983 Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 4984 RelatedPerson.class }) 4985 public static final String SP_REQUESTER = "requester"; 4986 /** 4987 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 4988 * <p> 4989 * Description: <b>Search by task requester</b><br> 4990 * Type: <b>reference</b><br> 4991 * Path: <b>Task.requester</b><br> 4992 * </p> 4993 */ 4994 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4995 SP_REQUESTER); 4996 4997 /** 4998 * Constant for fluent queries to be used to add include statements. Specifies 4999 * the path value of "<b>Task:requester</b>". 5000 */ 5001 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 5002 "Task:requester").toLocked(); 5003 5004 /** 5005 * Search parameter: <b>identifier</b> 5006 * <p> 5007 * Description: <b>Search for a task instance by its business identifier</b><br> 5008 * Type: <b>token</b><br> 5009 * Path: <b>Task.identifier</b><br> 5010 * </p> 5011 */ 5012 @SearchParamDefinition(name = "identifier", path = "Task.identifier", description = "Search for a task instance by its business identifier", type = "token") 5013 public static final String SP_IDENTIFIER = "identifier"; 5014 /** 5015 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5016 * <p> 5017 * Description: <b>Search for a task instance by its business identifier</b><br> 5018 * Type: <b>token</b><br> 5019 * Path: <b>Task.identifier</b><br> 5020 * </p> 5021 */ 5022 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5023 SP_IDENTIFIER); 5024 5025 /** 5026 * Search parameter: <b>business-status</b> 5027 * <p> 5028 * Description: <b>Search by business status</b><br> 5029 * Type: <b>token</b><br> 5030 * Path: <b>Task.businessStatus</b><br> 5031 * </p> 5032 */ 5033 @SearchParamDefinition(name = "business-status", path = "Task.businessStatus", description = "Search by business status", type = "token") 5034 public static final String SP_BUSINESS_STATUS = "business-status"; 5035 /** 5036 * <b>Fluent Client</b> search parameter constant for <b>business-status</b> 5037 * <p> 5038 * Description: <b>Search by business status</b><br> 5039 * Type: <b>token</b><br> 5040 * Path: <b>Task.businessStatus</b><br> 5041 * </p> 5042 */ 5043 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BUSINESS_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5044 SP_BUSINESS_STATUS); 5045 5046 /** 5047 * Search parameter: <b>period</b> 5048 * <p> 5049 * Description: <b>Search by period Task is/was underway</b><br> 5050 * Type: <b>date</b><br> 5051 * Path: <b>Task.executionPeriod</b><br> 5052 * </p> 5053 */ 5054 @SearchParamDefinition(name = "period", path = "Task.executionPeriod", description = "Search by period Task is/was underway", type = "date") 5055 public static final String SP_PERIOD = "period"; 5056 /** 5057 * <b>Fluent Client</b> search parameter constant for <b>period</b> 5058 * <p> 5059 * Description: <b>Search by period Task is/was underway</b><br> 5060 * Type: <b>date</b><br> 5061 * Path: <b>Task.executionPeriod</b><br> 5062 * </p> 5063 */ 5064 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 5065 SP_PERIOD); 5066 5067 /** 5068 * Search parameter: <b>code</b> 5069 * <p> 5070 * Description: <b>Search by task code</b><br> 5071 * Type: <b>token</b><br> 5072 * Path: <b>Task.code</b><br> 5073 * </p> 5074 */ 5075 @SearchParamDefinition(name = "code", path = "Task.code", description = "Search by task code", type = "token") 5076 public static final String SP_CODE = "code"; 5077 /** 5078 * <b>Fluent Client</b> search parameter constant for <b>code</b> 5079 * <p> 5080 * Description: <b>Search by task code</b><br> 5081 * Type: <b>token</b><br> 5082 * Path: <b>Task.code</b><br> 5083 * </p> 5084 */ 5085 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5086 SP_CODE); 5087 5088 /** 5089 * Search parameter: <b>performer</b> 5090 * <p> 5091 * Description: <b>Search by recommended type of performer (e.g., Requester, 5092 * Performer, Scheduler).</b><br> 5093 * Type: <b>token</b><br> 5094 * Path: <b>Task.performerType</b><br> 5095 * </p> 5096 */ 5097 @SearchParamDefinition(name = "performer", path = "Task.performerType", description = "Search by recommended type of performer (e.g., Requester, Performer, Scheduler).", type = "token") 5098 public static final String SP_PERFORMER = "performer"; 5099 /** 5100 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 5101 * <p> 5102 * Description: <b>Search by recommended type of performer (e.g., Requester, 5103 * Performer, Scheduler).</b><br> 5104 * Type: <b>token</b><br> 5105 * Path: <b>Task.performerType</b><br> 5106 * </p> 5107 */ 5108 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5109 SP_PERFORMER); 5110 5111 /** 5112 * Search parameter: <b>subject</b> 5113 * <p> 5114 * Description: <b>Search by subject</b><br> 5115 * Type: <b>reference</b><br> 5116 * Path: <b>Task.for</b><br> 5117 * </p> 5118 */ 5119 @SearchParamDefinition(name = "subject", path = "Task.for", description = "Search by subject", type = "reference") 5120 public static final String SP_SUBJECT = "subject"; 5121 /** 5122 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5123 * <p> 5124 * Description: <b>Search by subject</b><br> 5125 * Type: <b>reference</b><br> 5126 * Path: <b>Task.for</b><br> 5127 * </p> 5128 */ 5129 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5130 SP_SUBJECT); 5131 5132 /** 5133 * Constant for fluent queries to be used to add include statements. Specifies 5134 * the path value of "<b>Task:subject</b>". 5135 */ 5136 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Task:subject") 5137 .toLocked(); 5138 5139 /** 5140 * Search parameter: <b>focus</b> 5141 * <p> 5142 * Description: <b>Search by task focus</b><br> 5143 * Type: <b>reference</b><br> 5144 * Path: <b>Task.focus</b><br> 5145 * </p> 5146 */ 5147 @SearchParamDefinition(name = "focus", path = "Task.focus", description = "Search by task focus", type = "reference") 5148 public static final String SP_FOCUS = "focus"; 5149 /** 5150 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 5151 * <p> 5152 * Description: <b>Search by task focus</b><br> 5153 * Type: <b>reference</b><br> 5154 * Path: <b>Task.focus</b><br> 5155 * </p> 5156 */ 5157 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5158 SP_FOCUS); 5159 5160 /** 5161 * Constant for fluent queries to be used to add include statements. Specifies 5162 * the path value of "<b>Task:focus</b>". 5163 */ 5164 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("Task:focus") 5165 .toLocked(); 5166 5167 /** 5168 * Search parameter: <b>part-of</b> 5169 * <p> 5170 * Description: <b>Search by task this task is part of</b><br> 5171 * Type: <b>reference</b><br> 5172 * Path: <b>Task.partOf</b><br> 5173 * </p> 5174 */ 5175 @SearchParamDefinition(name = "part-of", path = "Task.partOf", description = "Search by task this task is part of", type = "reference", target = { 5176 Task.class }) 5177 public static final String SP_PART_OF = "part-of"; 5178 /** 5179 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 5180 * <p> 5181 * Description: <b>Search by task this task is part of</b><br> 5182 * Type: <b>reference</b><br> 5183 * Path: <b>Task.partOf</b><br> 5184 * </p> 5185 */ 5186 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5187 SP_PART_OF); 5188 5189 /** 5190 * Constant for fluent queries to be used to add include statements. Specifies 5191 * the path value of "<b>Task:part-of</b>". 5192 */ 5193 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Task:part-of") 5194 .toLocked(); 5195 5196 /** 5197 * Search parameter: <b>encounter</b> 5198 * <p> 5199 * Description: <b>Search by encounter</b><br> 5200 * Type: <b>reference</b><br> 5201 * Path: <b>Task.encounter</b><br> 5202 * </p> 5203 */ 5204 @SearchParamDefinition(name = "encounter", path = "Task.encounter", description = "Search by encounter", type = "reference", target = { 5205 Encounter.class }) 5206 public static final String SP_ENCOUNTER = "encounter"; 5207 /** 5208 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5209 * <p> 5210 * Description: <b>Search by encounter</b><br> 5211 * Type: <b>reference</b><br> 5212 * Path: <b>Task.encounter</b><br> 5213 * </p> 5214 */ 5215 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5216 SP_ENCOUNTER); 5217 5218 /** 5219 * Constant for fluent queries to be used to add include statements. Specifies 5220 * the path value of "<b>Task:encounter</b>". 5221 */ 5222 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 5223 "Task:encounter").toLocked(); 5224 5225 /** 5226 * Search parameter: <b>priority</b> 5227 * <p> 5228 * Description: <b>Search by task priority</b><br> 5229 * Type: <b>token</b><br> 5230 * Path: <b>Task.priority</b><br> 5231 * </p> 5232 */ 5233 @SearchParamDefinition(name = "priority", path = "Task.priority", description = "Search by task priority", type = "token") 5234 public static final String SP_PRIORITY = "priority"; 5235 /** 5236 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 5237 * <p> 5238 * Description: <b>Search by task priority</b><br> 5239 * Type: <b>token</b><br> 5240 * Path: <b>Task.priority</b><br> 5241 * </p> 5242 */ 5243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5244 SP_PRIORITY); 5245 5246 /** 5247 * Search parameter: <b>authored-on</b> 5248 * <p> 5249 * Description: <b>Search by creation date</b><br> 5250 * Type: <b>date</b><br> 5251 * Path: <b>Task.authoredOn</b><br> 5252 * </p> 5253 */ 5254 @SearchParamDefinition(name = "authored-on", path = "Task.authoredOn", description = "Search by creation date", type = "date") 5255 public static final String SP_AUTHORED_ON = "authored-on"; 5256 /** 5257 * <b>Fluent Client</b> search parameter constant for <b>authored-on</b> 5258 * <p> 5259 * Description: <b>Search by creation date</b><br> 5260 * Type: <b>date</b><br> 5261 * Path: <b>Task.authoredOn</b><br> 5262 * </p> 5263 */ 5264 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED_ON = new ca.uhn.fhir.rest.gclient.DateClientParam( 5265 SP_AUTHORED_ON); 5266 5267 /** 5268 * Search parameter: <b>intent</b> 5269 * <p> 5270 * Description: <b>Search by task intent</b><br> 5271 * Type: <b>token</b><br> 5272 * Path: <b>Task.intent</b><br> 5273 * </p> 5274 */ 5275 @SearchParamDefinition(name = "intent", path = "Task.intent", description = "Search by task intent", type = "token") 5276 public static final String SP_INTENT = "intent"; 5277 /** 5278 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 5279 * <p> 5280 * Description: <b>Search by task intent</b><br> 5281 * Type: <b>token</b><br> 5282 * Path: <b>Task.intent</b><br> 5283 * </p> 5284 */ 5285 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5286 SP_INTENT); 5287 5288 /** 5289 * Search parameter: <b>group-identifier</b> 5290 * <p> 5291 * Description: <b>Search by group identifier</b><br> 5292 * Type: <b>token</b><br> 5293 * Path: <b>Task.groupIdentifier</b><br> 5294 * </p> 5295 */ 5296 @SearchParamDefinition(name = "group-identifier", path = "Task.groupIdentifier", description = "Search by group identifier", type = "token") 5297 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 5298 /** 5299 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 5300 * <p> 5301 * Description: <b>Search by group identifier</b><br> 5302 * Type: <b>token</b><br> 5303 * Path: <b>Task.groupIdentifier</b><br> 5304 * </p> 5305 */ 5306 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5307 SP_GROUP_IDENTIFIER); 5308 5309 /** 5310 * Search parameter: <b>based-on</b> 5311 * <p> 5312 * Description: <b>Search by requests this task is based on</b><br> 5313 * Type: <b>reference</b><br> 5314 * Path: <b>Task.basedOn</b><br> 5315 * </p> 5316 */ 5317 @SearchParamDefinition(name = "based-on", path = "Task.basedOn", description = "Search by requests this task is based on", type = "reference") 5318 public static final String SP_BASED_ON = "based-on"; 5319 /** 5320 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 5321 * <p> 5322 * Description: <b>Search by requests this task is based on</b><br> 5323 * Type: <b>reference</b><br> 5324 * Path: <b>Task.basedOn</b><br> 5325 * </p> 5326 */ 5327 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5328 SP_BASED_ON); 5329 5330 /** 5331 * Constant for fluent queries to be used to add include statements. Specifies 5332 * the path value of "<b>Task:based-on</b>". 5333 */ 5334 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 5335 "Task:based-on").toLocked(); 5336 5337 /** 5338 * Search parameter: <b>patient</b> 5339 * <p> 5340 * Description: <b>Search by patient</b><br> 5341 * Type: <b>reference</b><br> 5342 * Path: <b>Task.for</b><br> 5343 * </p> 5344 */ 5345 @SearchParamDefinition(name = "patient", path = "Task.for.where(resolve() is Patient)", description = "Search by patient", type = "reference", target = { 5346 Patient.class }) 5347 public static final String SP_PATIENT = "patient"; 5348 /** 5349 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5350 * <p> 5351 * Description: <b>Search by patient</b><br> 5352 * Type: <b>reference</b><br> 5353 * Path: <b>Task.for</b><br> 5354 * </p> 5355 */ 5356 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5357 SP_PATIENT); 5358 5359 /** 5360 * Constant for fluent queries to be used to add include statements. Specifies 5361 * the path value of "<b>Task:patient</b>". 5362 */ 5363 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Task:patient") 5364 .toLocked(); 5365 5366 /** 5367 * Search parameter: <b>modified</b> 5368 * <p> 5369 * Description: <b>Search by last modification date</b><br> 5370 * Type: <b>date</b><br> 5371 * Path: <b>Task.lastModified</b><br> 5372 * </p> 5373 */ 5374 @SearchParamDefinition(name = "modified", path = "Task.lastModified", description = "Search by last modification date", type = "date") 5375 public static final String SP_MODIFIED = "modified"; 5376 /** 5377 * <b>Fluent Client</b> search parameter constant for <b>modified</b> 5378 * <p> 5379 * Description: <b>Search by last modification date</b><br> 5380 * Type: <b>date</b><br> 5381 * Path: <b>Task.lastModified</b><br> 5382 * </p> 5383 */ 5384 public static final ca.uhn.fhir.rest.gclient.DateClientParam MODIFIED = new ca.uhn.fhir.rest.gclient.DateClientParam( 5385 SP_MODIFIED); 5386 5387 /** 5388 * Search parameter: <b>status</b> 5389 * <p> 5390 * Description: <b>Search by task status</b><br> 5391 * Type: <b>token</b><br> 5392 * Path: <b>Task.status</b><br> 5393 * </p> 5394 */ 5395 @SearchParamDefinition(name = "status", path = "Task.status", description = "Search by task status", type = "token") 5396 public static final String SP_STATUS = "status"; 5397 /** 5398 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5399 * <p> 5400 * Description: <b>Search by task status</b><br> 5401 * Type: <b>token</b><br> 5402 * Path: <b>Task.status</b><br> 5403 * </p> 5404 */ 5405 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5406 SP_STATUS); 5407 5408}