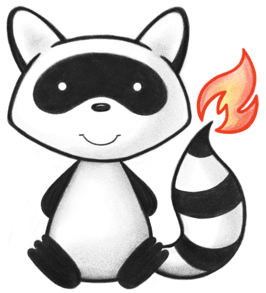
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A TerminologyCapabilities resource documents a set of capabilities 052 * (behaviors) of a FHIR Terminology Server that may be used as a statement of 053 * actual server functionality or a statement of required or desired server 054 * implementation. 055 */ 056@ResourceDef(name = "TerminologyCapabilities", profile = "http://hl7.org/fhir/StructureDefinition/TerminologyCapabilities") 057@ChildOrder(names = { "url", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", 058 "description", "useContext", "jurisdiction", "purpose", "copyright", "kind", "software", "implementation", 059 "lockedDate", "codeSystem", "expansion", "codeSearch", "validateCode", "translation", "closure" }) 060public class TerminologyCapabilities extends MetadataResource { 061 062 public enum CapabilityStatementKind { 063 /** 064 * The CapabilityStatement instance represents the present capabilities of a 065 * specific system instance. This is the kind returned by /metadata for a FHIR 066 * server end-point. 067 */ 068 INSTANCE, 069 /** 070 * The CapabilityStatement instance represents the capabilities of a system or 071 * piece of software, independent of a particular installation. 072 */ 073 CAPABILITY, 074 /** 075 * The CapabilityStatement instance represents a set of requirements for other 076 * systems to meet; e.g. as part of an implementation guide or 'request for 077 * proposal'. 078 */ 079 REQUIREMENTS, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 085 public static CapabilityStatementKind fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("instance".equals(codeString)) 089 return INSTANCE; 090 if ("capability".equals(codeString)) 091 return CAPABILITY; 092 if ("requirements".equals(codeString)) 093 return REQUIREMENTS; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown CapabilityStatementKind code '" + codeString + "'"); 098 } 099 100 public String toCode() { 101 switch (this) { 102 case INSTANCE: 103 return "instance"; 104 case CAPABILITY: 105 return "capability"; 106 case REQUIREMENTS: 107 return "requirements"; 108 case NULL: 109 return null; 110 default: 111 return "?"; 112 } 113 } 114 115 public String getSystem() { 116 switch (this) { 117 case INSTANCE: 118 return "http://hl7.org/fhir/capability-statement-kind"; 119 case CAPABILITY: 120 return "http://hl7.org/fhir/capability-statement-kind"; 121 case REQUIREMENTS: 122 return "http://hl7.org/fhir/capability-statement-kind"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDefinition() { 131 switch (this) { 132 case INSTANCE: 133 return "The CapabilityStatement instance represents the present capabilities of a specific system instance. This is the kind returned by /metadata for a FHIR server end-point."; 134 case CAPABILITY: 135 return "The CapabilityStatement instance represents the capabilities of a system or piece of software, independent of a particular installation."; 136 case REQUIREMENTS: 137 return "The CapabilityStatement instance represents a set of requirements for other systems to meet; e.g. as part of an implementation guide or 'request for proposal'."; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 145 public String getDisplay() { 146 switch (this) { 147 case INSTANCE: 148 return "Instance"; 149 case CAPABILITY: 150 return "Capability"; 151 case REQUIREMENTS: 152 return "Requirements"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class CapabilityStatementKindEnumFactory implements EnumFactory<CapabilityStatementKind> { 162 public CapabilityStatementKind fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("instance".equals(codeString)) 167 return CapabilityStatementKind.INSTANCE; 168 if ("capability".equals(codeString)) 169 return CapabilityStatementKind.CAPABILITY; 170 if ("requirements".equals(codeString)) 171 return CapabilityStatementKind.REQUIREMENTS; 172 throw new IllegalArgumentException("Unknown CapabilityStatementKind code '" + codeString + "'"); 173 } 174 175 public Enumeration<CapabilityStatementKind> fromType(PrimitiveType<?> code) throws FHIRException { 176 if (code == null) 177 return null; 178 if (code.isEmpty()) 179 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.NULL, code); 180 String codeString = code.asStringValue(); 181 if (codeString == null || "".equals(codeString)) 182 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.NULL, code); 183 if ("instance".equals(codeString)) 184 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.INSTANCE, code); 185 if ("capability".equals(codeString)) 186 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.CAPABILITY, code); 187 if ("requirements".equals(codeString)) 188 return new Enumeration<CapabilityStatementKind>(this, CapabilityStatementKind.REQUIREMENTS, code); 189 throw new FHIRException("Unknown CapabilityStatementKind code '" + codeString + "'"); 190 } 191 192 public String toCode(CapabilityStatementKind code) { 193 if (code == CapabilityStatementKind.NULL) 194 return null; 195 if (code == CapabilityStatementKind.INSTANCE) 196 return "instance"; 197 if (code == CapabilityStatementKind.CAPABILITY) 198 return "capability"; 199 if (code == CapabilityStatementKind.REQUIREMENTS) 200 return "requirements"; 201 return "?"; 202 } 203 204 public String toSystem(CapabilityStatementKind code) { 205 return code.getSystem(); 206 } 207 } 208 209 public enum CodeSearchSupport { 210 /** 211 * The search for code on ValueSet only includes codes explicitly detailed on 212 * includes or expansions. 213 */ 214 EXPLICIT, 215 /** 216 * The search for code on ValueSet only includes all codes based on the 217 * expansion of the value set. 218 */ 219 ALL, 220 /** 221 * added to help the parsers with the generic types 222 */ 223 NULL; 224 225 public static CodeSearchSupport fromCode(String codeString) throws FHIRException { 226 if (codeString == null || "".equals(codeString)) 227 return null; 228 if ("explicit".equals(codeString)) 229 return EXPLICIT; 230 if ("all".equals(codeString)) 231 return ALL; 232 if (Configuration.isAcceptInvalidEnums()) 233 return null; 234 else 235 throw new FHIRException("Unknown CodeSearchSupport code '" + codeString + "'"); 236 } 237 238 public String toCode() { 239 switch (this) { 240 case EXPLICIT: 241 return "explicit"; 242 case ALL: 243 return "all"; 244 case NULL: 245 return null; 246 default: 247 return "?"; 248 } 249 } 250 251 public String getSystem() { 252 switch (this) { 253 case EXPLICIT: 254 return "http://hl7.org/fhir/code-search-support"; 255 case ALL: 256 return "http://hl7.org/fhir/code-search-support"; 257 case NULL: 258 return null; 259 default: 260 return "?"; 261 } 262 } 263 264 public String getDefinition() { 265 switch (this) { 266 case EXPLICIT: 267 return "The search for code on ValueSet only includes codes explicitly detailed on includes or expansions."; 268 case ALL: 269 return "The search for code on ValueSet only includes all codes based on the expansion of the value set."; 270 case NULL: 271 return null; 272 default: 273 return "?"; 274 } 275 } 276 277 public String getDisplay() { 278 switch (this) { 279 case EXPLICIT: 280 return "Explicit Codes"; 281 case ALL: 282 return "Implicit Codes"; 283 case NULL: 284 return null; 285 default: 286 return "?"; 287 } 288 } 289 } 290 291 public static class CodeSearchSupportEnumFactory implements EnumFactory<CodeSearchSupport> { 292 public CodeSearchSupport fromCode(String codeString) throws IllegalArgumentException { 293 if (codeString == null || "".equals(codeString)) 294 if (codeString == null || "".equals(codeString)) 295 return null; 296 if ("explicit".equals(codeString)) 297 return CodeSearchSupport.EXPLICIT; 298 if ("all".equals(codeString)) 299 return CodeSearchSupport.ALL; 300 throw new IllegalArgumentException("Unknown CodeSearchSupport code '" + codeString + "'"); 301 } 302 303 public Enumeration<CodeSearchSupport> fromType(PrimitiveType<?> code) throws FHIRException { 304 if (code == null) 305 return null; 306 if (code.isEmpty()) 307 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.NULL, code); 308 String codeString = code.asStringValue(); 309 if (codeString == null || "".equals(codeString)) 310 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.NULL, code); 311 if ("explicit".equals(codeString)) 312 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.EXPLICIT, code); 313 if ("all".equals(codeString)) 314 return new Enumeration<CodeSearchSupport>(this, CodeSearchSupport.ALL, code); 315 throw new FHIRException("Unknown CodeSearchSupport code '" + codeString + "'"); 316 } 317 318 public String toCode(CodeSearchSupport code) { 319 if (code == CodeSearchSupport.NULL) 320 return null; 321 if (code == CodeSearchSupport.EXPLICIT) 322 return "explicit"; 323 if (code == CodeSearchSupport.ALL) 324 return "all"; 325 return "?"; 326 } 327 328 public String toSystem(CodeSearchSupport code) { 329 return code.getSystem(); 330 } 331 } 332 333 @Block() 334 public static class TerminologyCapabilitiesSoftwareComponent extends BackboneElement implements IBaseBackboneElement { 335 /** 336 * Name the software is known by. 337 */ 338 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 339 @Description(shortDefinition = "A name the software is known by", formalDefinition = "Name the software is known by.") 340 protected StringType name; 341 342 /** 343 * The version identifier for the software covered by this statement. 344 */ 345 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 346 @Description(shortDefinition = "Version covered by this statement", formalDefinition = "The version identifier for the software covered by this statement.") 347 protected StringType version; 348 349 private static final long serialVersionUID = -790299911L; 350 351 /** 352 * Constructor 353 */ 354 public TerminologyCapabilitiesSoftwareComponent() { 355 super(); 356 } 357 358 /** 359 * Constructor 360 */ 361 public TerminologyCapabilitiesSoftwareComponent(StringType name) { 362 super(); 363 this.name = name; 364 } 365 366 /** 367 * @return {@link #name} (Name the software is known by.). This is the 368 * underlying object with id, value and extensions. The accessor 369 * "getName" gives direct access to the value 370 */ 371 public StringType getNameElement() { 372 if (this.name == null) 373 if (Configuration.errorOnAutoCreate()) 374 throw new Error("Attempt to auto-create TerminologyCapabilitiesSoftwareComponent.name"); 375 else if (Configuration.doAutoCreate()) 376 this.name = new StringType(); // bb 377 return this.name; 378 } 379 380 public boolean hasNameElement() { 381 return this.name != null && !this.name.isEmpty(); 382 } 383 384 public boolean hasName() { 385 return this.name != null && !this.name.isEmpty(); 386 } 387 388 /** 389 * @param value {@link #name} (Name the software is known by.). This is the 390 * underlying object with id, value and extensions. The accessor 391 * "getName" gives direct access to the value 392 */ 393 public TerminologyCapabilitiesSoftwareComponent setNameElement(StringType value) { 394 this.name = value; 395 return this; 396 } 397 398 /** 399 * @return Name the software is known by. 400 */ 401 public String getName() { 402 return this.name == null ? null : this.name.getValue(); 403 } 404 405 /** 406 * @param value Name the software is known by. 407 */ 408 public TerminologyCapabilitiesSoftwareComponent setName(String value) { 409 if (this.name == null) 410 this.name = new StringType(); 411 this.name.setValue(value); 412 return this; 413 } 414 415 /** 416 * @return {@link #version} (The version identifier for the software covered by 417 * this statement.). This is the underlying object with id, value and 418 * extensions. The accessor "getVersion" gives direct access to the 419 * value 420 */ 421 public StringType getVersionElement() { 422 if (this.version == null) 423 if (Configuration.errorOnAutoCreate()) 424 throw new Error("Attempt to auto-create TerminologyCapabilitiesSoftwareComponent.version"); 425 else if (Configuration.doAutoCreate()) 426 this.version = new StringType(); // bb 427 return this.version; 428 } 429 430 public boolean hasVersionElement() { 431 return this.version != null && !this.version.isEmpty(); 432 } 433 434 public boolean hasVersion() { 435 return this.version != null && !this.version.isEmpty(); 436 } 437 438 /** 439 * @param value {@link #version} (The version identifier for the software 440 * covered by this statement.). This is the underlying object with 441 * id, value and extensions. The accessor "getVersion" gives direct 442 * access to the value 443 */ 444 public TerminologyCapabilitiesSoftwareComponent setVersionElement(StringType value) { 445 this.version = value; 446 return this; 447 } 448 449 /** 450 * @return The version identifier for the software covered by this statement. 451 */ 452 public String getVersion() { 453 return this.version == null ? null : this.version.getValue(); 454 } 455 456 /** 457 * @param value The version identifier for the software covered by this 458 * statement. 459 */ 460 public TerminologyCapabilitiesSoftwareComponent setVersion(String value) { 461 if (Utilities.noString(value)) 462 this.version = null; 463 else { 464 if (this.version == null) 465 this.version = new StringType(); 466 this.version.setValue(value); 467 } 468 return this; 469 } 470 471 protected void listChildren(List<Property> children) { 472 super.listChildren(children); 473 children.add(new Property("name", "string", "Name the software is known by.", 0, 1, name)); 474 children.add(new Property("version", "string", 475 "The version identifier for the software covered by this statement.", 0, 1, version)); 476 } 477 478 @Override 479 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 480 switch (_hash) { 481 case 3373707: 482 /* name */ return new Property("name", "string", "Name the software is known by.", 0, 1, name); 483 case 351608024: 484 /* version */ return new Property("version", "string", 485 "The version identifier for the software covered by this statement.", 0, 1, version); 486 default: 487 return super.getNamedProperty(_hash, _name, _checkValid); 488 } 489 490 } 491 492 @Override 493 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 494 switch (hash) { 495 case 3373707: 496 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 497 case 351608024: 498 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 499 default: 500 return super.getProperty(hash, name, checkValid); 501 } 502 503 } 504 505 @Override 506 public Base setProperty(int hash, String name, Base value) throws FHIRException { 507 switch (hash) { 508 case 3373707: // name 509 this.name = castToString(value); // StringType 510 return value; 511 case 351608024: // version 512 this.version = castToString(value); // StringType 513 return value; 514 default: 515 return super.setProperty(hash, name, value); 516 } 517 518 } 519 520 @Override 521 public Base setProperty(String name, Base value) throws FHIRException { 522 if (name.equals("name")) { 523 this.name = castToString(value); // StringType 524 } else if (name.equals("version")) { 525 this.version = castToString(value); // StringType 526 } else 527 return super.setProperty(name, value); 528 return value; 529 } 530 531 @Override 532 public void removeChild(String name, Base value) throws FHIRException { 533 if (name.equals("name")) { 534 this.name = null; 535 } else if (name.equals("version")) { 536 this.version = null; 537 } else 538 super.removeChild(name, value); 539 540 } 541 542 @Override 543 public Base makeProperty(int hash, String name) throws FHIRException { 544 switch (hash) { 545 case 3373707: 546 return getNameElement(); 547 case 351608024: 548 return getVersionElement(); 549 default: 550 return super.makeProperty(hash, name); 551 } 552 553 } 554 555 @Override 556 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 557 switch (hash) { 558 case 3373707: 559 /* name */ return new String[] { "string" }; 560 case 351608024: 561 /* version */ return new String[] { "string" }; 562 default: 563 return super.getTypesForProperty(hash, name); 564 } 565 566 } 567 568 @Override 569 public Base addChild(String name) throws FHIRException { 570 if (name.equals("name")) { 571 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.name"); 572 } else if (name.equals("version")) { 573 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.version"); 574 } else 575 return super.addChild(name); 576 } 577 578 public TerminologyCapabilitiesSoftwareComponent copy() { 579 TerminologyCapabilitiesSoftwareComponent dst = new TerminologyCapabilitiesSoftwareComponent(); 580 copyValues(dst); 581 return dst; 582 } 583 584 public void copyValues(TerminologyCapabilitiesSoftwareComponent dst) { 585 super.copyValues(dst); 586 dst.name = name == null ? null : name.copy(); 587 dst.version = version == null ? null : version.copy(); 588 } 589 590 @Override 591 public boolean equalsDeep(Base other_) { 592 if (!super.equalsDeep(other_)) 593 return false; 594 if (!(other_ instanceof TerminologyCapabilitiesSoftwareComponent)) 595 return false; 596 TerminologyCapabilitiesSoftwareComponent o = (TerminologyCapabilitiesSoftwareComponent) other_; 597 return compareDeep(name, o.name, true) && compareDeep(version, o.version, true); 598 } 599 600 @Override 601 public boolean equalsShallow(Base other_) { 602 if (!super.equalsShallow(other_)) 603 return false; 604 if (!(other_ instanceof TerminologyCapabilitiesSoftwareComponent)) 605 return false; 606 TerminologyCapabilitiesSoftwareComponent o = (TerminologyCapabilitiesSoftwareComponent) other_; 607 return compareValues(name, o.name, true) && compareValues(version, o.version, true); 608 } 609 610 public boolean isEmpty() { 611 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, version); 612 } 613 614 public String fhirType() { 615 return "TerminologyCapabilities.software"; 616 617 } 618 619 } 620 621 @Block() 622 public static class TerminologyCapabilitiesImplementationComponent extends BackboneElement 623 implements IBaseBackboneElement { 624 /** 625 * Information about the specific installation that this terminology capability 626 * statement relates to. 627 */ 628 @Child(name = "description", type = { 629 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 630 @Description(shortDefinition = "Describes this specific instance", formalDefinition = "Information about the specific installation that this terminology capability statement relates to.") 631 protected StringType description; 632 633 /** 634 * An absolute base URL for the implementation. 635 */ 636 @Child(name = "url", type = { UrlType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 637 @Description(shortDefinition = "Base URL for the implementation", formalDefinition = "An absolute base URL for the implementation.") 638 protected UrlType url; 639 640 private static final long serialVersionUID = 98009649L; 641 642 /** 643 * Constructor 644 */ 645 public TerminologyCapabilitiesImplementationComponent() { 646 super(); 647 } 648 649 /** 650 * Constructor 651 */ 652 public TerminologyCapabilitiesImplementationComponent(StringType description) { 653 super(); 654 this.description = description; 655 } 656 657 /** 658 * @return {@link #description} (Information about the specific installation 659 * that this terminology capability statement relates to.). This is the 660 * underlying object with id, value and extensions. The accessor 661 * "getDescription" gives direct access to the value 662 */ 663 public StringType getDescriptionElement() { 664 if (this.description == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create TerminologyCapabilitiesImplementationComponent.description"); 667 else if (Configuration.doAutoCreate()) 668 this.description = new StringType(); // bb 669 return this.description; 670 } 671 672 public boolean hasDescriptionElement() { 673 return this.description != null && !this.description.isEmpty(); 674 } 675 676 public boolean hasDescription() { 677 return this.description != null && !this.description.isEmpty(); 678 } 679 680 /** 681 * @param value {@link #description} (Information about the specific 682 * installation that this terminology capability statement relates 683 * to.). This is the underlying object with id, value and 684 * extensions. The accessor "getDescription" gives direct access to 685 * the value 686 */ 687 public TerminologyCapabilitiesImplementationComponent setDescriptionElement(StringType value) { 688 this.description = value; 689 return this; 690 } 691 692 /** 693 * @return Information about the specific installation that this terminology 694 * capability statement relates to. 695 */ 696 public String getDescription() { 697 return this.description == null ? null : this.description.getValue(); 698 } 699 700 /** 701 * @param value Information about the specific installation that this 702 * terminology capability statement relates to. 703 */ 704 public TerminologyCapabilitiesImplementationComponent setDescription(String value) { 705 if (this.description == null) 706 this.description = new StringType(); 707 this.description.setValue(value); 708 return this; 709 } 710 711 /** 712 * @return {@link #url} (An absolute base URL for the implementation.). This is 713 * the underlying object with id, value and extensions. The accessor 714 * "getUrl" gives direct access to the value 715 */ 716 public UrlType getUrlElement() { 717 if (this.url == null) 718 if (Configuration.errorOnAutoCreate()) 719 throw new Error("Attempt to auto-create TerminologyCapabilitiesImplementationComponent.url"); 720 else if (Configuration.doAutoCreate()) 721 this.url = new UrlType(); // bb 722 return this.url; 723 } 724 725 public boolean hasUrlElement() { 726 return this.url != null && !this.url.isEmpty(); 727 } 728 729 public boolean hasUrl() { 730 return this.url != null && !this.url.isEmpty(); 731 } 732 733 /** 734 * @param value {@link #url} (An absolute base URL for the implementation.). 735 * This is the underlying object with id, value and extensions. The 736 * accessor "getUrl" gives direct access to the value 737 */ 738 public TerminologyCapabilitiesImplementationComponent setUrlElement(UrlType value) { 739 this.url = value; 740 return this; 741 } 742 743 /** 744 * @return An absolute base URL for the implementation. 745 */ 746 public String getUrl() { 747 return this.url == null ? null : this.url.getValue(); 748 } 749 750 /** 751 * @param value An absolute base URL for the implementation. 752 */ 753 public TerminologyCapabilitiesImplementationComponent setUrl(String value) { 754 if (Utilities.noString(value)) 755 this.url = null; 756 else { 757 if (this.url == null) 758 this.url = new UrlType(); 759 this.url.setValue(value); 760 } 761 return this; 762 } 763 764 protected void listChildren(List<Property> children) { 765 super.listChildren(children); 766 children.add(new Property("description", "string", 767 "Information about the specific installation that this terminology capability statement relates to.", 0, 1, 768 description)); 769 children.add(new Property("url", "url", "An absolute base URL for the implementation.", 0, 1, url)); 770 } 771 772 @Override 773 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 774 switch (_hash) { 775 case -1724546052: 776 /* description */ return new Property("description", "string", 777 "Information about the specific installation that this terminology capability statement relates to.", 0, 1, 778 description); 779 case 116079: 780 /* url */ return new Property("url", "url", "An absolute base URL for the implementation.", 0, 1, url); 781 default: 782 return super.getNamedProperty(_hash, _name, _checkValid); 783 } 784 785 } 786 787 @Override 788 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 789 switch (hash) { 790 case -1724546052: 791 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 792 case 116079: 793 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UrlType 794 default: 795 return super.getProperty(hash, name, checkValid); 796 } 797 798 } 799 800 @Override 801 public Base setProperty(int hash, String name, Base value) throws FHIRException { 802 switch (hash) { 803 case -1724546052: // description 804 this.description = castToString(value); // StringType 805 return value; 806 case 116079: // url 807 this.url = castToUrl(value); // UrlType 808 return value; 809 default: 810 return super.setProperty(hash, name, value); 811 } 812 813 } 814 815 @Override 816 public Base setProperty(String name, Base value) throws FHIRException { 817 if (name.equals("description")) { 818 this.description = castToString(value); // StringType 819 } else if (name.equals("url")) { 820 this.url = castToUrl(value); // UrlType 821 } else 822 return super.setProperty(name, value); 823 return value; 824 } 825 826 @Override 827 public void removeChild(String name, Base value) throws FHIRException { 828 if (name.equals("description")) { 829 this.description = null; 830 } else if (name.equals("url")) { 831 this.url = null; 832 } else 833 super.removeChild(name, value); 834 835 } 836 837 @Override 838 public Base makeProperty(int hash, String name) throws FHIRException { 839 switch (hash) { 840 case -1724546052: 841 return getDescriptionElement(); 842 case 116079: 843 return getUrlElement(); 844 default: 845 return super.makeProperty(hash, name); 846 } 847 848 } 849 850 @Override 851 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 852 switch (hash) { 853 case -1724546052: 854 /* description */ return new String[] { "string" }; 855 case 116079: 856 /* url */ return new String[] { "url" }; 857 default: 858 return super.getTypesForProperty(hash, name); 859 } 860 861 } 862 863 @Override 864 public Base addChild(String name) throws FHIRException { 865 if (name.equals("description")) { 866 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.description"); 867 } else if (name.equals("url")) { 868 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.url"); 869 } else 870 return super.addChild(name); 871 } 872 873 public TerminologyCapabilitiesImplementationComponent copy() { 874 TerminologyCapabilitiesImplementationComponent dst = new TerminologyCapabilitiesImplementationComponent(); 875 copyValues(dst); 876 return dst; 877 } 878 879 public void copyValues(TerminologyCapabilitiesImplementationComponent dst) { 880 super.copyValues(dst); 881 dst.description = description == null ? null : description.copy(); 882 dst.url = url == null ? null : url.copy(); 883 } 884 885 @Override 886 public boolean equalsDeep(Base other_) { 887 if (!super.equalsDeep(other_)) 888 return false; 889 if (!(other_ instanceof TerminologyCapabilitiesImplementationComponent)) 890 return false; 891 TerminologyCapabilitiesImplementationComponent o = (TerminologyCapabilitiesImplementationComponent) other_; 892 return compareDeep(description, o.description, true) && compareDeep(url, o.url, true); 893 } 894 895 @Override 896 public boolean equalsShallow(Base other_) { 897 if (!super.equalsShallow(other_)) 898 return false; 899 if (!(other_ instanceof TerminologyCapabilitiesImplementationComponent)) 900 return false; 901 TerminologyCapabilitiesImplementationComponent o = (TerminologyCapabilitiesImplementationComponent) other_; 902 return compareValues(description, o.description, true) && compareValues(url, o.url, true); 903 } 904 905 public boolean isEmpty() { 906 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, url); 907 } 908 909 public String fhirType() { 910 return "TerminologyCapabilities.implementation"; 911 912 } 913 914 } 915 916 @Block() 917 public static class TerminologyCapabilitiesCodeSystemComponent extends BackboneElement 918 implements IBaseBackboneElement { 919 /** 920 * URI for the Code System. 921 */ 922 @Child(name = "uri", type = { CanonicalType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 923 @Description(shortDefinition = "URI for the Code System", formalDefinition = "URI for the Code System.") 924 protected CanonicalType uri; 925 926 /** 927 * For the code system, a list of versions that are supported by the server. 928 */ 929 @Child(name = "version", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 930 @Description(shortDefinition = "Version of Code System supported", formalDefinition = "For the code system, a list of versions that are supported by the server.") 931 protected List<TerminologyCapabilitiesCodeSystemVersionComponent> version; 932 933 /** 934 * True if subsumption is supported for this version of the code system. 935 */ 936 @Child(name = "subsumption", type = { 937 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 938 @Description(shortDefinition = "Whether subsumption is supported", formalDefinition = "True if subsumption is supported for this version of the code system.") 939 protected BooleanType subsumption; 940 941 private static final long serialVersionUID = -1593622817L; 942 943 /** 944 * Constructor 945 */ 946 public TerminologyCapabilitiesCodeSystemComponent() { 947 super(); 948 } 949 950 /** 951 * @return {@link #uri} (URI for the Code System.). This is the underlying 952 * object with id, value and extensions. The accessor "getUri" gives 953 * direct access to the value 954 */ 955 public CanonicalType getUriElement() { 956 if (this.uri == null) 957 if (Configuration.errorOnAutoCreate()) 958 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemComponent.uri"); 959 else if (Configuration.doAutoCreate()) 960 this.uri = new CanonicalType(); // bb 961 return this.uri; 962 } 963 964 public boolean hasUriElement() { 965 return this.uri != null && !this.uri.isEmpty(); 966 } 967 968 public boolean hasUri() { 969 return this.uri != null && !this.uri.isEmpty(); 970 } 971 972 /** 973 * @param value {@link #uri} (URI for the Code System.). This is the underlying 974 * object with id, value and extensions. The accessor "getUri" 975 * gives direct access to the value 976 */ 977 public TerminologyCapabilitiesCodeSystemComponent setUriElement(CanonicalType value) { 978 this.uri = value; 979 return this; 980 } 981 982 /** 983 * @return URI for the Code System. 984 */ 985 public String getUri() { 986 return this.uri == null ? null : this.uri.getValue(); 987 } 988 989 /** 990 * @param value URI for the Code System. 991 */ 992 public TerminologyCapabilitiesCodeSystemComponent setUri(String value) { 993 if (Utilities.noString(value)) 994 this.uri = null; 995 else { 996 if (this.uri == null) 997 this.uri = new CanonicalType(); 998 this.uri.setValue(value); 999 } 1000 return this; 1001 } 1002 1003 /** 1004 * @return {@link #version} (For the code system, a list of versions that are 1005 * supported by the server.) 1006 */ 1007 public List<TerminologyCapabilitiesCodeSystemVersionComponent> getVersion() { 1008 if (this.version == null) 1009 this.version = new ArrayList<TerminologyCapabilitiesCodeSystemVersionComponent>(); 1010 return this.version; 1011 } 1012 1013 /** 1014 * @return Returns a reference to <code>this</code> for easy method chaining 1015 */ 1016 public TerminologyCapabilitiesCodeSystemComponent setVersion( 1017 List<TerminologyCapabilitiesCodeSystemVersionComponent> theVersion) { 1018 this.version = theVersion; 1019 return this; 1020 } 1021 1022 public boolean hasVersion() { 1023 if (this.version == null) 1024 return false; 1025 for (TerminologyCapabilitiesCodeSystemVersionComponent item : this.version) 1026 if (!item.isEmpty()) 1027 return true; 1028 return false; 1029 } 1030 1031 public TerminologyCapabilitiesCodeSystemVersionComponent addVersion() { // 3 1032 TerminologyCapabilitiesCodeSystemVersionComponent t = new TerminologyCapabilitiesCodeSystemVersionComponent(); 1033 if (this.version == null) 1034 this.version = new ArrayList<TerminologyCapabilitiesCodeSystemVersionComponent>(); 1035 this.version.add(t); 1036 return t; 1037 } 1038 1039 public TerminologyCapabilitiesCodeSystemComponent addVersion(TerminologyCapabilitiesCodeSystemVersionComponent t) { // 3 1040 if (t == null) 1041 return this; 1042 if (this.version == null) 1043 this.version = new ArrayList<TerminologyCapabilitiesCodeSystemVersionComponent>(); 1044 this.version.add(t); 1045 return this; 1046 } 1047 1048 /** 1049 * @return The first repetition of repeating field {@link #version}, creating it 1050 * if it does not already exist 1051 */ 1052 public TerminologyCapabilitiesCodeSystemVersionComponent getVersionFirstRep() { 1053 if (getVersion().isEmpty()) { 1054 addVersion(); 1055 } 1056 return getVersion().get(0); 1057 } 1058 1059 /** 1060 * @return {@link #subsumption} (True if subsumption is supported for this 1061 * version of the code system.). This is the underlying object with id, 1062 * value and extensions. The accessor "getSubsumption" gives direct 1063 * access to the value 1064 */ 1065 public BooleanType getSubsumptionElement() { 1066 if (this.subsumption == null) 1067 if (Configuration.errorOnAutoCreate()) 1068 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemComponent.subsumption"); 1069 else if (Configuration.doAutoCreate()) 1070 this.subsumption = new BooleanType(); // bb 1071 return this.subsumption; 1072 } 1073 1074 public boolean hasSubsumptionElement() { 1075 return this.subsumption != null && !this.subsumption.isEmpty(); 1076 } 1077 1078 public boolean hasSubsumption() { 1079 return this.subsumption != null && !this.subsumption.isEmpty(); 1080 } 1081 1082 /** 1083 * @param value {@link #subsumption} (True if subsumption is supported for this 1084 * version of the code system.). This is the underlying object with 1085 * id, value and extensions. The accessor "getSubsumption" gives 1086 * direct access to the value 1087 */ 1088 public TerminologyCapabilitiesCodeSystemComponent setSubsumptionElement(BooleanType value) { 1089 this.subsumption = value; 1090 return this; 1091 } 1092 1093 /** 1094 * @return True if subsumption is supported for this version of the code system. 1095 */ 1096 public boolean getSubsumption() { 1097 return this.subsumption == null || this.subsumption.isEmpty() ? false : this.subsumption.getValue(); 1098 } 1099 1100 /** 1101 * @param value True if subsumption is supported for this version of the code 1102 * system. 1103 */ 1104 public TerminologyCapabilitiesCodeSystemComponent setSubsumption(boolean value) { 1105 if (this.subsumption == null) 1106 this.subsumption = new BooleanType(); 1107 this.subsumption.setValue(value); 1108 return this; 1109 } 1110 1111 protected void listChildren(List<Property> children) { 1112 super.listChildren(children); 1113 children.add(new Property("uri", "canonical(CodeSystem)", "URI for the Code System.", 0, 1, uri)); 1114 children 1115 .add(new Property("version", "", "For the code system, a list of versions that are supported by the server.", 1116 0, java.lang.Integer.MAX_VALUE, version)); 1117 children.add(new Property("subsumption", "boolean", 1118 "True if subsumption is supported for this version of the code system.", 0, 1, subsumption)); 1119 } 1120 1121 @Override 1122 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1123 switch (_hash) { 1124 case 116076: 1125 /* uri */ return new Property("uri", "canonical(CodeSystem)", "URI for the Code System.", 0, 1, uri); 1126 case 351608024: 1127 /* version */ return new Property("version", "", 1128 "For the code system, a list of versions that are supported by the server.", 0, java.lang.Integer.MAX_VALUE, 1129 version); 1130 case -499084711: 1131 /* subsumption */ return new Property("subsumption", "boolean", 1132 "True if subsumption is supported for this version of the code system.", 0, 1, subsumption); 1133 default: 1134 return super.getNamedProperty(_hash, _name, _checkValid); 1135 } 1136 1137 } 1138 1139 @Override 1140 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1141 switch (hash) { 1142 case 116076: 1143 /* uri */ return this.uri == null ? new Base[0] : new Base[] { this.uri }; // CanonicalType 1144 case 351608024: 1145 /* version */ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // TerminologyCapabilitiesCodeSystemVersionComponent 1146 case -499084711: 1147 /* subsumption */ return this.subsumption == null ? new Base[0] : new Base[] { this.subsumption }; // BooleanType 1148 default: 1149 return super.getProperty(hash, name, checkValid); 1150 } 1151 1152 } 1153 1154 @Override 1155 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1156 switch (hash) { 1157 case 116076: // uri 1158 this.uri = castToCanonical(value); // CanonicalType 1159 return value; 1160 case 351608024: // version 1161 this.getVersion().add((TerminologyCapabilitiesCodeSystemVersionComponent) value); // TerminologyCapabilitiesCodeSystemVersionComponent 1162 return value; 1163 case -499084711: // subsumption 1164 this.subsumption = castToBoolean(value); // BooleanType 1165 return value; 1166 default: 1167 return super.setProperty(hash, name, value); 1168 } 1169 1170 } 1171 1172 @Override 1173 public Base setProperty(String name, Base value) throws FHIRException { 1174 if (name.equals("uri")) { 1175 this.uri = castToCanonical(value); // CanonicalType 1176 } else if (name.equals("version")) { 1177 this.getVersion().add((TerminologyCapabilitiesCodeSystemVersionComponent) value); 1178 } else if (name.equals("subsumption")) { 1179 this.subsumption = castToBoolean(value); // BooleanType 1180 } else 1181 return super.setProperty(name, value); 1182 return value; 1183 } 1184 1185 @Override 1186 public void removeChild(String name, Base value) throws FHIRException { 1187 if (name.equals("uri")) { 1188 this.uri = null; 1189 } else if (name.equals("version")) { 1190 this.getVersion().remove((TerminologyCapabilitiesCodeSystemVersionComponent) value); 1191 } else if (name.equals("subsumption")) { 1192 this.subsumption = null; 1193 } else 1194 super.removeChild(name, value); 1195 1196 } 1197 1198 @Override 1199 public Base makeProperty(int hash, String name) throws FHIRException { 1200 switch (hash) { 1201 case 116076: 1202 return getUriElement(); 1203 case 351608024: 1204 return addVersion(); 1205 case -499084711: 1206 return getSubsumptionElement(); 1207 default: 1208 return super.makeProperty(hash, name); 1209 } 1210 1211 } 1212 1213 @Override 1214 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1215 switch (hash) { 1216 case 116076: 1217 /* uri */ return new String[] { "canonical" }; 1218 case 351608024: 1219 /* version */ return new String[] {}; 1220 case -499084711: 1221 /* subsumption */ return new String[] { "boolean" }; 1222 default: 1223 return super.getTypesForProperty(hash, name); 1224 } 1225 1226 } 1227 1228 @Override 1229 public Base addChild(String name) throws FHIRException { 1230 if (name.equals("uri")) { 1231 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.uri"); 1232 } else if (name.equals("version")) { 1233 return addVersion(); 1234 } else if (name.equals("subsumption")) { 1235 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.subsumption"); 1236 } else 1237 return super.addChild(name); 1238 } 1239 1240 public TerminologyCapabilitiesCodeSystemComponent copy() { 1241 TerminologyCapabilitiesCodeSystemComponent dst = new TerminologyCapabilitiesCodeSystemComponent(); 1242 copyValues(dst); 1243 return dst; 1244 } 1245 1246 public void copyValues(TerminologyCapabilitiesCodeSystemComponent dst) { 1247 super.copyValues(dst); 1248 dst.uri = uri == null ? null : uri.copy(); 1249 if (version != null) { 1250 dst.version = new ArrayList<TerminologyCapabilitiesCodeSystemVersionComponent>(); 1251 for (TerminologyCapabilitiesCodeSystemVersionComponent i : version) 1252 dst.version.add(i.copy()); 1253 } 1254 ; 1255 dst.subsumption = subsumption == null ? null : subsumption.copy(); 1256 } 1257 1258 @Override 1259 public boolean equalsDeep(Base other_) { 1260 if (!super.equalsDeep(other_)) 1261 return false; 1262 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemComponent)) 1263 return false; 1264 TerminologyCapabilitiesCodeSystemComponent o = (TerminologyCapabilitiesCodeSystemComponent) other_; 1265 return compareDeep(uri, o.uri, true) && compareDeep(version, o.version, true) 1266 && compareDeep(subsumption, o.subsumption, true); 1267 } 1268 1269 @Override 1270 public boolean equalsShallow(Base other_) { 1271 if (!super.equalsShallow(other_)) 1272 return false; 1273 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemComponent)) 1274 return false; 1275 TerminologyCapabilitiesCodeSystemComponent o = (TerminologyCapabilitiesCodeSystemComponent) other_; 1276 return compareValues(subsumption, o.subsumption, true); 1277 } 1278 1279 public boolean isEmpty() { 1280 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uri, version, subsumption); 1281 } 1282 1283 public String fhirType() { 1284 return "TerminologyCapabilities.codeSystem"; 1285 1286 } 1287 1288 } 1289 1290 @Block() 1291 public static class TerminologyCapabilitiesCodeSystemVersionComponent extends BackboneElement 1292 implements IBaseBackboneElement { 1293 /** 1294 * For version-less code systems, there should be a single version with no 1295 * identifier. 1296 */ 1297 @Child(name = "code", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1298 @Description(shortDefinition = "Version identifier for this version", formalDefinition = "For version-less code systems, there should be a single version with no identifier.") 1299 protected StringType code; 1300 1301 /** 1302 * If this is the default version for this code system. 1303 */ 1304 @Child(name = "isDefault", type = { 1305 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1306 @Description(shortDefinition = "If this is the default version for this code system", formalDefinition = "If this is the default version for this code system.") 1307 protected BooleanType isDefault; 1308 1309 /** 1310 * If the compositional grammar defined by the code system is supported. 1311 */ 1312 @Child(name = "compositional", type = { 1313 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1314 @Description(shortDefinition = "If compositional grammar is supported", formalDefinition = "If the compositional grammar defined by the code system is supported.") 1315 protected BooleanType compositional; 1316 1317 /** 1318 * Language Displays supported. 1319 */ 1320 @Child(name = "language", type = { 1321 CodeType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1322 @Description(shortDefinition = "Language Displays supported", formalDefinition = "Language Displays supported.") 1323 protected List<CodeType> language; 1324 1325 /** 1326 * Filter Properties supported. 1327 */ 1328 @Child(name = "filter", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1329 @Description(shortDefinition = "Filter Properties supported", formalDefinition = "Filter Properties supported.") 1330 protected List<TerminologyCapabilitiesCodeSystemVersionFilterComponent> filter; 1331 1332 /** 1333 * Properties supported for $lookup. 1334 */ 1335 @Child(name = "property", type = { 1336 CodeType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1337 @Description(shortDefinition = "Properties supported for $lookup", formalDefinition = "Properties supported for $lookup.") 1338 protected List<CodeType> property; 1339 1340 private static final long serialVersionUID = 1857571343L; 1341 1342 /** 1343 * Constructor 1344 */ 1345 public TerminologyCapabilitiesCodeSystemVersionComponent() { 1346 super(); 1347 } 1348 1349 /** 1350 * @return {@link #code} (For version-less code systems, there should be a 1351 * single version with no identifier.). This is the underlying object 1352 * with id, value and extensions. The accessor "getCode" gives direct 1353 * access to the value 1354 */ 1355 public StringType getCodeElement() { 1356 if (this.code == null) 1357 if (Configuration.errorOnAutoCreate()) 1358 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemVersionComponent.code"); 1359 else if (Configuration.doAutoCreate()) 1360 this.code = new StringType(); // bb 1361 return this.code; 1362 } 1363 1364 public boolean hasCodeElement() { 1365 return this.code != null && !this.code.isEmpty(); 1366 } 1367 1368 public boolean hasCode() { 1369 return this.code != null && !this.code.isEmpty(); 1370 } 1371 1372 /** 1373 * @param value {@link #code} (For version-less code systems, there should be a 1374 * single version with no identifier.). This is the underlying 1375 * object with id, value and extensions. The accessor "getCode" 1376 * gives direct access to the value 1377 */ 1378 public TerminologyCapabilitiesCodeSystemVersionComponent setCodeElement(StringType value) { 1379 this.code = value; 1380 return this; 1381 } 1382 1383 /** 1384 * @return For version-less code systems, there should be a single version with 1385 * no identifier. 1386 */ 1387 public String getCode() { 1388 return this.code == null ? null : this.code.getValue(); 1389 } 1390 1391 /** 1392 * @param value For version-less code systems, there should be a single version 1393 * with no identifier. 1394 */ 1395 public TerminologyCapabilitiesCodeSystemVersionComponent setCode(String value) { 1396 if (Utilities.noString(value)) 1397 this.code = null; 1398 else { 1399 if (this.code == null) 1400 this.code = new StringType(); 1401 this.code.setValue(value); 1402 } 1403 return this; 1404 } 1405 1406 /** 1407 * @return {@link #isDefault} (If this is the default version for this code 1408 * system.). This is the underlying object with id, value and 1409 * extensions. The accessor "getIsDefault" gives direct access to the 1410 * value 1411 */ 1412 public BooleanType getIsDefaultElement() { 1413 if (this.isDefault == null) 1414 if (Configuration.errorOnAutoCreate()) 1415 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemVersionComponent.isDefault"); 1416 else if (Configuration.doAutoCreate()) 1417 this.isDefault = new BooleanType(); // bb 1418 return this.isDefault; 1419 } 1420 1421 public boolean hasIsDefaultElement() { 1422 return this.isDefault != null && !this.isDefault.isEmpty(); 1423 } 1424 1425 public boolean hasIsDefault() { 1426 return this.isDefault != null && !this.isDefault.isEmpty(); 1427 } 1428 1429 /** 1430 * @param value {@link #isDefault} (If this is the default version for this code 1431 * system.). This is the underlying object with id, value and 1432 * extensions. The accessor "getIsDefault" gives direct access to 1433 * the value 1434 */ 1435 public TerminologyCapabilitiesCodeSystemVersionComponent setIsDefaultElement(BooleanType value) { 1436 this.isDefault = value; 1437 return this; 1438 } 1439 1440 /** 1441 * @return If this is the default version for this code system. 1442 */ 1443 public boolean getIsDefault() { 1444 return this.isDefault == null || this.isDefault.isEmpty() ? false : this.isDefault.getValue(); 1445 } 1446 1447 /** 1448 * @param value If this is the default version for this code system. 1449 */ 1450 public TerminologyCapabilitiesCodeSystemVersionComponent setIsDefault(boolean value) { 1451 if (this.isDefault == null) 1452 this.isDefault = new BooleanType(); 1453 this.isDefault.setValue(value); 1454 return this; 1455 } 1456 1457 /** 1458 * @return {@link #compositional} (If the compositional grammar defined by the 1459 * code system is supported.). This is the underlying object with id, 1460 * value and extensions. The accessor "getCompositional" gives direct 1461 * access to the value 1462 */ 1463 public BooleanType getCompositionalElement() { 1464 if (this.compositional == null) 1465 if (Configuration.errorOnAutoCreate()) 1466 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemVersionComponent.compositional"); 1467 else if (Configuration.doAutoCreate()) 1468 this.compositional = new BooleanType(); // bb 1469 return this.compositional; 1470 } 1471 1472 public boolean hasCompositionalElement() { 1473 return this.compositional != null && !this.compositional.isEmpty(); 1474 } 1475 1476 public boolean hasCompositional() { 1477 return this.compositional != null && !this.compositional.isEmpty(); 1478 } 1479 1480 /** 1481 * @param value {@link #compositional} (If the compositional grammar defined by 1482 * the code system is supported.). This is the underlying object 1483 * with id, value and extensions. The accessor "getCompositional" 1484 * gives direct access to the value 1485 */ 1486 public TerminologyCapabilitiesCodeSystemVersionComponent setCompositionalElement(BooleanType value) { 1487 this.compositional = value; 1488 return this; 1489 } 1490 1491 /** 1492 * @return If the compositional grammar defined by the code system is supported. 1493 */ 1494 public boolean getCompositional() { 1495 return this.compositional == null || this.compositional.isEmpty() ? false : this.compositional.getValue(); 1496 } 1497 1498 /** 1499 * @param value If the compositional grammar defined by the code system is 1500 * supported. 1501 */ 1502 public TerminologyCapabilitiesCodeSystemVersionComponent setCompositional(boolean value) { 1503 if (this.compositional == null) 1504 this.compositional = new BooleanType(); 1505 this.compositional.setValue(value); 1506 return this; 1507 } 1508 1509 /** 1510 * @return {@link #language} (Language Displays supported.) 1511 */ 1512 public List<CodeType> getLanguage() { 1513 if (this.language == null) 1514 this.language = new ArrayList<CodeType>(); 1515 return this.language; 1516 } 1517 1518 /** 1519 * @return Returns a reference to <code>this</code> for easy method chaining 1520 */ 1521 public TerminologyCapabilitiesCodeSystemVersionComponent setLanguage(List<CodeType> theLanguage) { 1522 this.language = theLanguage; 1523 return this; 1524 } 1525 1526 public boolean hasLanguage() { 1527 if (this.language == null) 1528 return false; 1529 for (CodeType item : this.language) 1530 if (!item.isEmpty()) 1531 return true; 1532 return false; 1533 } 1534 1535 /** 1536 * @return {@link #language} (Language Displays supported.) 1537 */ 1538 public CodeType addLanguageElement() {// 2 1539 CodeType t = new CodeType(); 1540 if (this.language == null) 1541 this.language = new ArrayList<CodeType>(); 1542 this.language.add(t); 1543 return t; 1544 } 1545 1546 /** 1547 * @param value {@link #language} (Language Displays supported.) 1548 */ 1549 public TerminologyCapabilitiesCodeSystemVersionComponent addLanguage(String value) { // 1 1550 CodeType t = new CodeType(); 1551 t.setValue(value); 1552 if (this.language == null) 1553 this.language = new ArrayList<CodeType>(); 1554 this.language.add(t); 1555 return this; 1556 } 1557 1558 /** 1559 * @param value {@link #language} (Language Displays supported.) 1560 */ 1561 public boolean hasLanguage(String value) { 1562 if (this.language == null) 1563 return false; 1564 for (CodeType v : this.language) 1565 if (v.getValue().equals(value)) // code 1566 return true; 1567 return false; 1568 } 1569 1570 /** 1571 * @return {@link #filter} (Filter Properties supported.) 1572 */ 1573 public List<TerminologyCapabilitiesCodeSystemVersionFilterComponent> getFilter() { 1574 if (this.filter == null) 1575 this.filter = new ArrayList<TerminologyCapabilitiesCodeSystemVersionFilterComponent>(); 1576 return this.filter; 1577 } 1578 1579 /** 1580 * @return Returns a reference to <code>this</code> for easy method chaining 1581 */ 1582 public TerminologyCapabilitiesCodeSystemVersionComponent setFilter( 1583 List<TerminologyCapabilitiesCodeSystemVersionFilterComponent> theFilter) { 1584 this.filter = theFilter; 1585 return this; 1586 } 1587 1588 public boolean hasFilter() { 1589 if (this.filter == null) 1590 return false; 1591 for (TerminologyCapabilitiesCodeSystemVersionFilterComponent item : this.filter) 1592 if (!item.isEmpty()) 1593 return true; 1594 return false; 1595 } 1596 1597 public TerminologyCapabilitiesCodeSystemVersionFilterComponent addFilter() { // 3 1598 TerminologyCapabilitiesCodeSystemVersionFilterComponent t = new TerminologyCapabilitiesCodeSystemVersionFilterComponent(); 1599 if (this.filter == null) 1600 this.filter = new ArrayList<TerminologyCapabilitiesCodeSystemVersionFilterComponent>(); 1601 this.filter.add(t); 1602 return t; 1603 } 1604 1605 public TerminologyCapabilitiesCodeSystemVersionComponent addFilter( 1606 TerminologyCapabilitiesCodeSystemVersionFilterComponent t) { // 3 1607 if (t == null) 1608 return this; 1609 if (this.filter == null) 1610 this.filter = new ArrayList<TerminologyCapabilitiesCodeSystemVersionFilterComponent>(); 1611 this.filter.add(t); 1612 return this; 1613 } 1614 1615 /** 1616 * @return The first repetition of repeating field {@link #filter}, creating it 1617 * if it does not already exist 1618 */ 1619 public TerminologyCapabilitiesCodeSystemVersionFilterComponent getFilterFirstRep() { 1620 if (getFilter().isEmpty()) { 1621 addFilter(); 1622 } 1623 return getFilter().get(0); 1624 } 1625 1626 /** 1627 * @return {@link #property} (Properties supported for $lookup.) 1628 */ 1629 public List<CodeType> getProperty() { 1630 if (this.property == null) 1631 this.property = new ArrayList<CodeType>(); 1632 return this.property; 1633 } 1634 1635 /** 1636 * @return Returns a reference to <code>this</code> for easy method chaining 1637 */ 1638 public TerminologyCapabilitiesCodeSystemVersionComponent setProperty(List<CodeType> theProperty) { 1639 this.property = theProperty; 1640 return this; 1641 } 1642 1643 public boolean hasProperty() { 1644 if (this.property == null) 1645 return false; 1646 for (CodeType item : this.property) 1647 if (!item.isEmpty()) 1648 return true; 1649 return false; 1650 } 1651 1652 /** 1653 * @return {@link #property} (Properties supported for $lookup.) 1654 */ 1655 public CodeType addPropertyElement() {// 2 1656 CodeType t = new CodeType(); 1657 if (this.property == null) 1658 this.property = new ArrayList<CodeType>(); 1659 this.property.add(t); 1660 return t; 1661 } 1662 1663 /** 1664 * @param value {@link #property} (Properties supported for $lookup.) 1665 */ 1666 public TerminologyCapabilitiesCodeSystemVersionComponent addProperty(String value) { // 1 1667 CodeType t = new CodeType(); 1668 t.setValue(value); 1669 if (this.property == null) 1670 this.property = new ArrayList<CodeType>(); 1671 this.property.add(t); 1672 return this; 1673 } 1674 1675 /** 1676 * @param value {@link #property} (Properties supported for $lookup.) 1677 */ 1678 public boolean hasProperty(String value) { 1679 if (this.property == null) 1680 return false; 1681 for (CodeType v : this.property) 1682 if (v.getValue().equals(value)) // code 1683 return true; 1684 return false; 1685 } 1686 1687 protected void listChildren(List<Property> children) { 1688 super.listChildren(children); 1689 children.add(new Property("code", "string", 1690 "For version-less code systems, there should be a single version with no identifier.", 0, 1, code)); 1691 children.add(new Property("isDefault", "boolean", "If this is the default version for this code system.", 0, 1, 1692 isDefault)); 1693 children.add(new Property("compositional", "boolean", 1694 "If the compositional grammar defined by the code system is supported.", 0, 1, compositional)); 1695 children.add( 1696 new Property("language", "code", "Language Displays supported.", 0, java.lang.Integer.MAX_VALUE, language)); 1697 children.add(new Property("filter", "", "Filter Properties supported.", 0, java.lang.Integer.MAX_VALUE, filter)); 1698 children.add(new Property("property", "code", "Properties supported for $lookup.", 0, java.lang.Integer.MAX_VALUE, 1699 property)); 1700 } 1701 1702 @Override 1703 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1704 switch (_hash) { 1705 case 3059181: 1706 /* code */ return new Property("code", "string", 1707 "For version-less code systems, there should be a single version with no identifier.", 0, 1, code); 1708 case 965025207: 1709 /* isDefault */ return new Property("isDefault", "boolean", 1710 "If this is the default version for this code system.", 0, 1, isDefault); 1711 case 1248023381: 1712 /* compositional */ return new Property("compositional", "boolean", 1713 "If the compositional grammar defined by the code system is supported.", 0, 1, compositional); 1714 case -1613589672: 1715 /* language */ return new Property("language", "code", "Language Displays supported.", 0, 1716 java.lang.Integer.MAX_VALUE, language); 1717 case -1274492040: 1718 /* filter */ return new Property("filter", "", "Filter Properties supported.", 0, java.lang.Integer.MAX_VALUE, 1719 filter); 1720 case -993141291: 1721 /* property */ return new Property("property", "code", "Properties supported for $lookup.", 0, 1722 java.lang.Integer.MAX_VALUE, property); 1723 default: 1724 return super.getNamedProperty(_hash, _name, _checkValid); 1725 } 1726 1727 } 1728 1729 @Override 1730 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1731 switch (hash) { 1732 case 3059181: 1733 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // StringType 1734 case 965025207: 1735 /* isDefault */ return this.isDefault == null ? new Base[0] : new Base[] { this.isDefault }; // BooleanType 1736 case 1248023381: 1737 /* compositional */ return this.compositional == null ? new Base[0] : new Base[] { this.compositional }; // BooleanType 1738 case -1613589672: 1739 /* language */ return this.language == null ? new Base[0] 1740 : this.language.toArray(new Base[this.language.size()]); // CodeType 1741 case -1274492040: 1742 /* filter */ return this.filter == null ? new Base[0] : this.filter.toArray(new Base[this.filter.size()]); // TerminologyCapabilitiesCodeSystemVersionFilterComponent 1743 case -993141291: 1744 /* property */ return this.property == null ? new Base[0] 1745 : this.property.toArray(new Base[this.property.size()]); // CodeType 1746 default: 1747 return super.getProperty(hash, name, checkValid); 1748 } 1749 1750 } 1751 1752 @Override 1753 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1754 switch (hash) { 1755 case 3059181: // code 1756 this.code = castToString(value); // StringType 1757 return value; 1758 case 965025207: // isDefault 1759 this.isDefault = castToBoolean(value); // BooleanType 1760 return value; 1761 case 1248023381: // compositional 1762 this.compositional = castToBoolean(value); // BooleanType 1763 return value; 1764 case -1613589672: // language 1765 this.getLanguage().add(castToCode(value)); // CodeType 1766 return value; 1767 case -1274492040: // filter 1768 this.getFilter().add((TerminologyCapabilitiesCodeSystemVersionFilterComponent) value); // TerminologyCapabilitiesCodeSystemVersionFilterComponent 1769 return value; 1770 case -993141291: // property 1771 this.getProperty().add(castToCode(value)); // CodeType 1772 return value; 1773 default: 1774 return super.setProperty(hash, name, value); 1775 } 1776 1777 } 1778 1779 @Override 1780 public Base setProperty(String name, Base value) throws FHIRException { 1781 if (name.equals("code")) { 1782 this.code = castToString(value); // StringType 1783 } else if (name.equals("isDefault")) { 1784 this.isDefault = castToBoolean(value); // BooleanType 1785 } else if (name.equals("compositional")) { 1786 this.compositional = castToBoolean(value); // BooleanType 1787 } else if (name.equals("language")) { 1788 this.getLanguage().add(castToCode(value)); 1789 } else if (name.equals("filter")) { 1790 this.getFilter().add((TerminologyCapabilitiesCodeSystemVersionFilterComponent) value); 1791 } else if (name.equals("property")) { 1792 this.getProperty().add(castToCode(value)); 1793 } else 1794 return super.setProperty(name, value); 1795 return value; 1796 } 1797 1798 @Override 1799 public void removeChild(String name, Base value) throws FHIRException { 1800 if (name.equals("code")) { 1801 this.code = null; 1802 } else if (name.equals("isDefault")) { 1803 this.isDefault = null; 1804 } else if (name.equals("compositional")) { 1805 this.compositional = null; 1806 } else if (name.equals("language")) { 1807 this.getLanguage().remove(castToCode(value)); 1808 } else if (name.equals("filter")) { 1809 this.getFilter().remove((TerminologyCapabilitiesCodeSystemVersionFilterComponent) value); 1810 } else if (name.equals("property")) { 1811 this.getProperty().remove(castToCode(value)); 1812 } else 1813 super.removeChild(name, value); 1814 1815 } 1816 1817 @Override 1818 public Base makeProperty(int hash, String name) throws FHIRException { 1819 switch (hash) { 1820 case 3059181: 1821 return getCodeElement(); 1822 case 965025207: 1823 return getIsDefaultElement(); 1824 case 1248023381: 1825 return getCompositionalElement(); 1826 case -1613589672: 1827 return addLanguageElement(); 1828 case -1274492040: 1829 return addFilter(); 1830 case -993141291: 1831 return addPropertyElement(); 1832 default: 1833 return super.makeProperty(hash, name); 1834 } 1835 1836 } 1837 1838 @Override 1839 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1840 switch (hash) { 1841 case 3059181: 1842 /* code */ return new String[] { "string" }; 1843 case 965025207: 1844 /* isDefault */ return new String[] { "boolean" }; 1845 case 1248023381: 1846 /* compositional */ return new String[] { "boolean" }; 1847 case -1613589672: 1848 /* language */ return new String[] { "code" }; 1849 case -1274492040: 1850 /* filter */ return new String[] {}; 1851 case -993141291: 1852 /* property */ return new String[] { "code" }; 1853 default: 1854 return super.getTypesForProperty(hash, name); 1855 } 1856 1857 } 1858 1859 @Override 1860 public Base addChild(String name) throws FHIRException { 1861 if (name.equals("code")) { 1862 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.code"); 1863 } else if (name.equals("isDefault")) { 1864 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.isDefault"); 1865 } else if (name.equals("compositional")) { 1866 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.compositional"); 1867 } else if (name.equals("language")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.language"); 1869 } else if (name.equals("filter")) { 1870 return addFilter(); 1871 } else if (name.equals("property")) { 1872 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.property"); 1873 } else 1874 return super.addChild(name); 1875 } 1876 1877 public TerminologyCapabilitiesCodeSystemVersionComponent copy() { 1878 TerminologyCapabilitiesCodeSystemVersionComponent dst = new TerminologyCapabilitiesCodeSystemVersionComponent(); 1879 copyValues(dst); 1880 return dst; 1881 } 1882 1883 public void copyValues(TerminologyCapabilitiesCodeSystemVersionComponent dst) { 1884 super.copyValues(dst); 1885 dst.code = code == null ? null : code.copy(); 1886 dst.isDefault = isDefault == null ? null : isDefault.copy(); 1887 dst.compositional = compositional == null ? null : compositional.copy(); 1888 if (language != null) { 1889 dst.language = new ArrayList<CodeType>(); 1890 for (CodeType i : language) 1891 dst.language.add(i.copy()); 1892 } 1893 ; 1894 if (filter != null) { 1895 dst.filter = new ArrayList<TerminologyCapabilitiesCodeSystemVersionFilterComponent>(); 1896 for (TerminologyCapabilitiesCodeSystemVersionFilterComponent i : filter) 1897 dst.filter.add(i.copy()); 1898 } 1899 ; 1900 if (property != null) { 1901 dst.property = new ArrayList<CodeType>(); 1902 for (CodeType i : property) 1903 dst.property.add(i.copy()); 1904 } 1905 ; 1906 } 1907 1908 @Override 1909 public boolean equalsDeep(Base other_) { 1910 if (!super.equalsDeep(other_)) 1911 return false; 1912 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemVersionComponent)) 1913 return false; 1914 TerminologyCapabilitiesCodeSystemVersionComponent o = (TerminologyCapabilitiesCodeSystemVersionComponent) other_; 1915 return compareDeep(code, o.code, true) && compareDeep(isDefault, o.isDefault, true) 1916 && compareDeep(compositional, o.compositional, true) && compareDeep(language, o.language, true) 1917 && compareDeep(filter, o.filter, true) && compareDeep(property, o.property, true); 1918 } 1919 1920 @Override 1921 public boolean equalsShallow(Base other_) { 1922 if (!super.equalsShallow(other_)) 1923 return false; 1924 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemVersionComponent)) 1925 return false; 1926 TerminologyCapabilitiesCodeSystemVersionComponent o = (TerminologyCapabilitiesCodeSystemVersionComponent) other_; 1927 return compareValues(code, o.code, true) && compareValues(isDefault, o.isDefault, true) 1928 && compareValues(compositional, o.compositional, true) && compareValues(language, o.language, true) 1929 && compareValues(property, o.property, true); 1930 } 1931 1932 public boolean isEmpty() { 1933 return super.isEmpty() 1934 && ca.uhn.fhir.util.ElementUtil.isEmpty(code, isDefault, compositional, language, filter, property); 1935 } 1936 1937 public String fhirType() { 1938 return "TerminologyCapabilities.codeSystem.version"; 1939 1940 } 1941 1942 } 1943 1944 @Block() 1945 public static class TerminologyCapabilitiesCodeSystemVersionFilterComponent extends BackboneElement 1946 implements IBaseBackboneElement { 1947 /** 1948 * Code of the property supported. 1949 */ 1950 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1951 @Description(shortDefinition = "Code of the property supported", formalDefinition = "Code of the property supported.") 1952 protected CodeType code; 1953 1954 /** 1955 * Operations supported for the property. 1956 */ 1957 @Child(name = "op", type = { 1958 CodeType.class }, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1959 @Description(shortDefinition = "Operations supported for the property", formalDefinition = "Operations supported for the property.") 1960 protected List<CodeType> op; 1961 1962 private static final long serialVersionUID = -489160282L; 1963 1964 /** 1965 * Constructor 1966 */ 1967 public TerminologyCapabilitiesCodeSystemVersionFilterComponent() { 1968 super(); 1969 } 1970 1971 /** 1972 * Constructor 1973 */ 1974 public TerminologyCapabilitiesCodeSystemVersionFilterComponent(CodeType code) { 1975 super(); 1976 this.code = code; 1977 } 1978 1979 /** 1980 * @return {@link #code} (Code of the property supported.). This is the 1981 * underlying object with id, value and extensions. The accessor 1982 * "getCode" gives direct access to the value 1983 */ 1984 public CodeType getCodeElement() { 1985 if (this.code == null) 1986 if (Configuration.errorOnAutoCreate()) 1987 throw new Error("Attempt to auto-create TerminologyCapabilitiesCodeSystemVersionFilterComponent.code"); 1988 else if (Configuration.doAutoCreate()) 1989 this.code = new CodeType(); // bb 1990 return this.code; 1991 } 1992 1993 public boolean hasCodeElement() { 1994 return this.code != null && !this.code.isEmpty(); 1995 } 1996 1997 public boolean hasCode() { 1998 return this.code != null && !this.code.isEmpty(); 1999 } 2000 2001 /** 2002 * @param value {@link #code} (Code of the property supported.). This is the 2003 * underlying object with id, value and extensions. The accessor 2004 * "getCode" gives direct access to the value 2005 */ 2006 public TerminologyCapabilitiesCodeSystemVersionFilterComponent setCodeElement(CodeType value) { 2007 this.code = value; 2008 return this; 2009 } 2010 2011 /** 2012 * @return Code of the property supported. 2013 */ 2014 public String getCode() { 2015 return this.code == null ? null : this.code.getValue(); 2016 } 2017 2018 /** 2019 * @param value Code of the property supported. 2020 */ 2021 public TerminologyCapabilitiesCodeSystemVersionFilterComponent setCode(String value) { 2022 if (this.code == null) 2023 this.code = new CodeType(); 2024 this.code.setValue(value); 2025 return this; 2026 } 2027 2028 /** 2029 * @return {@link #op} (Operations supported for the property.) 2030 */ 2031 public List<CodeType> getOp() { 2032 if (this.op == null) 2033 this.op = new ArrayList<CodeType>(); 2034 return this.op; 2035 } 2036 2037 /** 2038 * @return Returns a reference to <code>this</code> for easy method chaining 2039 */ 2040 public TerminologyCapabilitiesCodeSystemVersionFilterComponent setOp(List<CodeType> theOp) { 2041 this.op = theOp; 2042 return this; 2043 } 2044 2045 public boolean hasOp() { 2046 if (this.op == null) 2047 return false; 2048 for (CodeType item : this.op) 2049 if (!item.isEmpty()) 2050 return true; 2051 return false; 2052 } 2053 2054 /** 2055 * @return {@link #op} (Operations supported for the property.) 2056 */ 2057 public CodeType addOpElement() {// 2 2058 CodeType t = new CodeType(); 2059 if (this.op == null) 2060 this.op = new ArrayList<CodeType>(); 2061 this.op.add(t); 2062 return t; 2063 } 2064 2065 /** 2066 * @param value {@link #op} (Operations supported for the property.) 2067 */ 2068 public TerminologyCapabilitiesCodeSystemVersionFilterComponent addOp(String value) { // 1 2069 CodeType t = new CodeType(); 2070 t.setValue(value); 2071 if (this.op == null) 2072 this.op = new ArrayList<CodeType>(); 2073 this.op.add(t); 2074 return this; 2075 } 2076 2077 /** 2078 * @param value {@link #op} (Operations supported for the property.) 2079 */ 2080 public boolean hasOp(String value) { 2081 if (this.op == null) 2082 return false; 2083 for (CodeType v : this.op) 2084 if (v.getValue().equals(value)) // code 2085 return true; 2086 return false; 2087 } 2088 2089 protected void listChildren(List<Property> children) { 2090 super.listChildren(children); 2091 children.add(new Property("code", "code", "Code of the property supported.", 0, 1, code)); 2092 children.add( 2093 new Property("op", "code", "Operations supported for the property.", 0, java.lang.Integer.MAX_VALUE, op)); 2094 } 2095 2096 @Override 2097 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2098 switch (_hash) { 2099 case 3059181: 2100 /* code */ return new Property("code", "code", "Code of the property supported.", 0, 1, code); 2101 case 3553: 2102 /* op */ return new Property("op", "code", "Operations supported for the property.", 0, 2103 java.lang.Integer.MAX_VALUE, op); 2104 default: 2105 return super.getNamedProperty(_hash, _name, _checkValid); 2106 } 2107 2108 } 2109 2110 @Override 2111 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2112 switch (hash) { 2113 case 3059181: 2114 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 2115 case 3553: 2116 /* op */ return this.op == null ? new Base[0] : this.op.toArray(new Base[this.op.size()]); // CodeType 2117 default: 2118 return super.getProperty(hash, name, checkValid); 2119 } 2120 2121 } 2122 2123 @Override 2124 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2125 switch (hash) { 2126 case 3059181: // code 2127 this.code = castToCode(value); // CodeType 2128 return value; 2129 case 3553: // op 2130 this.getOp().add(castToCode(value)); // CodeType 2131 return value; 2132 default: 2133 return super.setProperty(hash, name, value); 2134 } 2135 2136 } 2137 2138 @Override 2139 public Base setProperty(String name, Base value) throws FHIRException { 2140 if (name.equals("code")) { 2141 this.code = castToCode(value); // CodeType 2142 } else if (name.equals("op")) { 2143 this.getOp().add(castToCode(value)); 2144 } else 2145 return super.setProperty(name, value); 2146 return value; 2147 } 2148 2149 @Override 2150 public void removeChild(String name, Base value) throws FHIRException { 2151 if (name.equals("code")) { 2152 this.code = null; 2153 } else if (name.equals("op")) { 2154 this.getOp().remove(castToCode(value)); 2155 } else 2156 super.removeChild(name, value); 2157 2158 } 2159 2160 @Override 2161 public Base makeProperty(int hash, String name) throws FHIRException { 2162 switch (hash) { 2163 case 3059181: 2164 return getCodeElement(); 2165 case 3553: 2166 return addOpElement(); 2167 default: 2168 return super.makeProperty(hash, name); 2169 } 2170 2171 } 2172 2173 @Override 2174 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2175 switch (hash) { 2176 case 3059181: 2177 /* code */ return new String[] { "code" }; 2178 case 3553: 2179 /* op */ return new String[] { "code" }; 2180 default: 2181 return super.getTypesForProperty(hash, name); 2182 } 2183 2184 } 2185 2186 @Override 2187 public Base addChild(String name) throws FHIRException { 2188 if (name.equals("code")) { 2189 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.code"); 2190 } else if (name.equals("op")) { 2191 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.op"); 2192 } else 2193 return super.addChild(name); 2194 } 2195 2196 public TerminologyCapabilitiesCodeSystemVersionFilterComponent copy() { 2197 TerminologyCapabilitiesCodeSystemVersionFilterComponent dst = new TerminologyCapabilitiesCodeSystemVersionFilterComponent(); 2198 copyValues(dst); 2199 return dst; 2200 } 2201 2202 public void copyValues(TerminologyCapabilitiesCodeSystemVersionFilterComponent dst) { 2203 super.copyValues(dst); 2204 dst.code = code == null ? null : code.copy(); 2205 if (op != null) { 2206 dst.op = new ArrayList<CodeType>(); 2207 for (CodeType i : op) 2208 dst.op.add(i.copy()); 2209 } 2210 ; 2211 } 2212 2213 @Override 2214 public boolean equalsDeep(Base other_) { 2215 if (!super.equalsDeep(other_)) 2216 return false; 2217 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemVersionFilterComponent)) 2218 return false; 2219 TerminologyCapabilitiesCodeSystemVersionFilterComponent o = (TerminologyCapabilitiesCodeSystemVersionFilterComponent) other_; 2220 return compareDeep(code, o.code, true) && compareDeep(op, o.op, true); 2221 } 2222 2223 @Override 2224 public boolean equalsShallow(Base other_) { 2225 if (!super.equalsShallow(other_)) 2226 return false; 2227 if (!(other_ instanceof TerminologyCapabilitiesCodeSystemVersionFilterComponent)) 2228 return false; 2229 TerminologyCapabilitiesCodeSystemVersionFilterComponent o = (TerminologyCapabilitiesCodeSystemVersionFilterComponent) other_; 2230 return compareValues(code, o.code, true) && compareValues(op, o.op, true); 2231 } 2232 2233 public boolean isEmpty() { 2234 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, op); 2235 } 2236 2237 public String fhirType() { 2238 return "TerminologyCapabilities.codeSystem.version.filter"; 2239 2240 } 2241 2242 } 2243 2244 @Block() 2245 public static class TerminologyCapabilitiesExpansionComponent extends BackboneElement 2246 implements IBaseBackboneElement { 2247 /** 2248 * Whether the server can return nested value sets. 2249 */ 2250 @Child(name = "hierarchical", type = { 2251 BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2252 @Description(shortDefinition = "Whether the server can return nested value sets", formalDefinition = "Whether the server can return nested value sets.") 2253 protected BooleanType hierarchical; 2254 2255 /** 2256 * Whether the server supports paging on expansion. 2257 */ 2258 @Child(name = "paging", type = { 2259 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2260 @Description(shortDefinition = "Whether the server supports paging on expansion", formalDefinition = "Whether the server supports paging on expansion.") 2261 protected BooleanType paging; 2262 2263 /** 2264 * Allow request for incomplete expansions? 2265 */ 2266 @Child(name = "incomplete", type = { 2267 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2268 @Description(shortDefinition = "Allow request for incomplete expansions?", formalDefinition = "Allow request for incomplete expansions?") 2269 protected BooleanType incomplete; 2270 2271 /** 2272 * Supported expansion parameter. 2273 */ 2274 @Child(name = "parameter", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2275 @Description(shortDefinition = "Supported expansion parameter", formalDefinition = "Supported expansion parameter.") 2276 protected List<TerminologyCapabilitiesExpansionParameterComponent> parameter; 2277 2278 /** 2279 * Documentation about text searching works. 2280 */ 2281 @Child(name = "textFilter", type = { 2282 MarkdownType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2283 @Description(shortDefinition = "Documentation about text searching works", formalDefinition = "Documentation about text searching works.") 2284 protected MarkdownType textFilter; 2285 2286 private static final long serialVersionUID = -1011350616L; 2287 2288 /** 2289 * Constructor 2290 */ 2291 public TerminologyCapabilitiesExpansionComponent() { 2292 super(); 2293 } 2294 2295 /** 2296 * @return {@link #hierarchical} (Whether the server can return nested value 2297 * sets.). This is the underlying object with id, value and extensions. 2298 * The accessor "getHierarchical" gives direct access to the value 2299 */ 2300 public BooleanType getHierarchicalElement() { 2301 if (this.hierarchical == null) 2302 if (Configuration.errorOnAutoCreate()) 2303 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionComponent.hierarchical"); 2304 else if (Configuration.doAutoCreate()) 2305 this.hierarchical = new BooleanType(); // bb 2306 return this.hierarchical; 2307 } 2308 2309 public boolean hasHierarchicalElement() { 2310 return this.hierarchical != null && !this.hierarchical.isEmpty(); 2311 } 2312 2313 public boolean hasHierarchical() { 2314 return this.hierarchical != null && !this.hierarchical.isEmpty(); 2315 } 2316 2317 /** 2318 * @param value {@link #hierarchical} (Whether the server can return nested 2319 * value sets.). This is the underlying object with id, value and 2320 * extensions. The accessor "getHierarchical" gives direct access 2321 * to the value 2322 */ 2323 public TerminologyCapabilitiesExpansionComponent setHierarchicalElement(BooleanType value) { 2324 this.hierarchical = value; 2325 return this; 2326 } 2327 2328 /** 2329 * @return Whether the server can return nested value sets. 2330 */ 2331 public boolean getHierarchical() { 2332 return this.hierarchical == null || this.hierarchical.isEmpty() ? false : this.hierarchical.getValue(); 2333 } 2334 2335 /** 2336 * @param value Whether the server can return nested value sets. 2337 */ 2338 public TerminologyCapabilitiesExpansionComponent setHierarchical(boolean value) { 2339 if (this.hierarchical == null) 2340 this.hierarchical = new BooleanType(); 2341 this.hierarchical.setValue(value); 2342 return this; 2343 } 2344 2345 /** 2346 * @return {@link #paging} (Whether the server supports paging on expansion.). 2347 * This is the underlying object with id, value and extensions. The 2348 * accessor "getPaging" gives direct access to the value 2349 */ 2350 public BooleanType getPagingElement() { 2351 if (this.paging == null) 2352 if (Configuration.errorOnAutoCreate()) 2353 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionComponent.paging"); 2354 else if (Configuration.doAutoCreate()) 2355 this.paging = new BooleanType(); // bb 2356 return this.paging; 2357 } 2358 2359 public boolean hasPagingElement() { 2360 return this.paging != null && !this.paging.isEmpty(); 2361 } 2362 2363 public boolean hasPaging() { 2364 return this.paging != null && !this.paging.isEmpty(); 2365 } 2366 2367 /** 2368 * @param value {@link #paging} (Whether the server supports paging on 2369 * expansion.). This is the underlying object with id, value and 2370 * extensions. The accessor "getPaging" gives direct access to the 2371 * value 2372 */ 2373 public TerminologyCapabilitiesExpansionComponent setPagingElement(BooleanType value) { 2374 this.paging = value; 2375 return this; 2376 } 2377 2378 /** 2379 * @return Whether the server supports paging on expansion. 2380 */ 2381 public boolean getPaging() { 2382 return this.paging == null || this.paging.isEmpty() ? false : this.paging.getValue(); 2383 } 2384 2385 /** 2386 * @param value Whether the server supports paging on expansion. 2387 */ 2388 public TerminologyCapabilitiesExpansionComponent setPaging(boolean value) { 2389 if (this.paging == null) 2390 this.paging = new BooleanType(); 2391 this.paging.setValue(value); 2392 return this; 2393 } 2394 2395 /** 2396 * @return {@link #incomplete} (Allow request for incomplete expansions?). This 2397 * is the underlying object with id, value and extensions. The accessor 2398 * "getIncomplete" gives direct access to the value 2399 */ 2400 public BooleanType getIncompleteElement() { 2401 if (this.incomplete == null) 2402 if (Configuration.errorOnAutoCreate()) 2403 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionComponent.incomplete"); 2404 else if (Configuration.doAutoCreate()) 2405 this.incomplete = new BooleanType(); // bb 2406 return this.incomplete; 2407 } 2408 2409 public boolean hasIncompleteElement() { 2410 return this.incomplete != null && !this.incomplete.isEmpty(); 2411 } 2412 2413 public boolean hasIncomplete() { 2414 return this.incomplete != null && !this.incomplete.isEmpty(); 2415 } 2416 2417 /** 2418 * @param value {@link #incomplete} (Allow request for incomplete expansions?). 2419 * This is the underlying object with id, value and extensions. The 2420 * accessor "getIncomplete" gives direct access to the value 2421 */ 2422 public TerminologyCapabilitiesExpansionComponent setIncompleteElement(BooleanType value) { 2423 this.incomplete = value; 2424 return this; 2425 } 2426 2427 /** 2428 * @return Allow request for incomplete expansions? 2429 */ 2430 public boolean getIncomplete() { 2431 return this.incomplete == null || this.incomplete.isEmpty() ? false : this.incomplete.getValue(); 2432 } 2433 2434 /** 2435 * @param value Allow request for incomplete expansions? 2436 */ 2437 public TerminologyCapabilitiesExpansionComponent setIncomplete(boolean value) { 2438 if (this.incomplete == null) 2439 this.incomplete = new BooleanType(); 2440 this.incomplete.setValue(value); 2441 return this; 2442 } 2443 2444 /** 2445 * @return {@link #parameter} (Supported expansion parameter.) 2446 */ 2447 public List<TerminologyCapabilitiesExpansionParameterComponent> getParameter() { 2448 if (this.parameter == null) 2449 this.parameter = new ArrayList<TerminologyCapabilitiesExpansionParameterComponent>(); 2450 return this.parameter; 2451 } 2452 2453 /** 2454 * @return Returns a reference to <code>this</code> for easy method chaining 2455 */ 2456 public TerminologyCapabilitiesExpansionComponent setParameter( 2457 List<TerminologyCapabilitiesExpansionParameterComponent> theParameter) { 2458 this.parameter = theParameter; 2459 return this; 2460 } 2461 2462 public boolean hasParameter() { 2463 if (this.parameter == null) 2464 return false; 2465 for (TerminologyCapabilitiesExpansionParameterComponent item : this.parameter) 2466 if (!item.isEmpty()) 2467 return true; 2468 return false; 2469 } 2470 2471 public TerminologyCapabilitiesExpansionParameterComponent addParameter() { // 3 2472 TerminologyCapabilitiesExpansionParameterComponent t = new TerminologyCapabilitiesExpansionParameterComponent(); 2473 if (this.parameter == null) 2474 this.parameter = new ArrayList<TerminologyCapabilitiesExpansionParameterComponent>(); 2475 this.parameter.add(t); 2476 return t; 2477 } 2478 2479 public TerminologyCapabilitiesExpansionComponent addParameter( 2480 TerminologyCapabilitiesExpansionParameterComponent t) { // 3 2481 if (t == null) 2482 return this; 2483 if (this.parameter == null) 2484 this.parameter = new ArrayList<TerminologyCapabilitiesExpansionParameterComponent>(); 2485 this.parameter.add(t); 2486 return this; 2487 } 2488 2489 /** 2490 * @return The first repetition of repeating field {@link #parameter}, creating 2491 * it if it does not already exist 2492 */ 2493 public TerminologyCapabilitiesExpansionParameterComponent getParameterFirstRep() { 2494 if (getParameter().isEmpty()) { 2495 addParameter(); 2496 } 2497 return getParameter().get(0); 2498 } 2499 2500 /** 2501 * @return {@link #textFilter} (Documentation about text searching works.). This 2502 * is the underlying object with id, value and extensions. The accessor 2503 * "getTextFilter" gives direct access to the value 2504 */ 2505 public MarkdownType getTextFilterElement() { 2506 if (this.textFilter == null) 2507 if (Configuration.errorOnAutoCreate()) 2508 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionComponent.textFilter"); 2509 else if (Configuration.doAutoCreate()) 2510 this.textFilter = new MarkdownType(); // bb 2511 return this.textFilter; 2512 } 2513 2514 public boolean hasTextFilterElement() { 2515 return this.textFilter != null && !this.textFilter.isEmpty(); 2516 } 2517 2518 public boolean hasTextFilter() { 2519 return this.textFilter != null && !this.textFilter.isEmpty(); 2520 } 2521 2522 /** 2523 * @param value {@link #textFilter} (Documentation about text searching works.). 2524 * This is the underlying object with id, value and extensions. The 2525 * accessor "getTextFilter" gives direct access to the value 2526 */ 2527 public TerminologyCapabilitiesExpansionComponent setTextFilterElement(MarkdownType value) { 2528 this.textFilter = value; 2529 return this; 2530 } 2531 2532 /** 2533 * @return Documentation about text searching works. 2534 */ 2535 public String getTextFilter() { 2536 return this.textFilter == null ? null : this.textFilter.getValue(); 2537 } 2538 2539 /** 2540 * @param value Documentation about text searching works. 2541 */ 2542 public TerminologyCapabilitiesExpansionComponent setTextFilter(String value) { 2543 if (value == null) 2544 this.textFilter = null; 2545 else { 2546 if (this.textFilter == null) 2547 this.textFilter = new MarkdownType(); 2548 this.textFilter.setValue(value); 2549 } 2550 return this; 2551 } 2552 2553 protected void listChildren(List<Property> children) { 2554 super.listChildren(children); 2555 children.add(new Property("hierarchical", "boolean", "Whether the server can return nested value sets.", 0, 1, 2556 hierarchical)); 2557 children.add(new Property("paging", "boolean", "Whether the server supports paging on expansion.", 0, 1, paging)); 2558 children.add(new Property("incomplete", "boolean", "Allow request for incomplete expansions?", 0, 1, incomplete)); 2559 children.add( 2560 new Property("parameter", "", "Supported expansion parameter.", 0, java.lang.Integer.MAX_VALUE, parameter)); 2561 children 2562 .add(new Property("textFilter", "markdown", "Documentation about text searching works.", 0, 1, textFilter)); 2563 } 2564 2565 @Override 2566 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2567 switch (_hash) { 2568 case 857636745: 2569 /* hierarchical */ return new Property("hierarchical", "boolean", 2570 "Whether the server can return nested value sets.", 0, 1, hierarchical); 2571 case -995747956: 2572 /* paging */ return new Property("paging", "boolean", "Whether the server supports paging on expansion.", 0, 1, 2573 paging); 2574 case -1010022050: 2575 /* incomplete */ return new Property("incomplete", "boolean", "Allow request for incomplete expansions?", 0, 1, 2576 incomplete); 2577 case 1954460585: 2578 /* parameter */ return new Property("parameter", "", "Supported expansion parameter.", 0, 2579 java.lang.Integer.MAX_VALUE, parameter); 2580 case 1469359877: 2581 /* textFilter */ return new Property("textFilter", "markdown", "Documentation about text searching works.", 0, 2582 1, textFilter); 2583 default: 2584 return super.getNamedProperty(_hash, _name, _checkValid); 2585 } 2586 2587 } 2588 2589 @Override 2590 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2591 switch (hash) { 2592 case 857636745: 2593 /* hierarchical */ return this.hierarchical == null ? new Base[0] : new Base[] { this.hierarchical }; // BooleanType 2594 case -995747956: 2595 /* paging */ return this.paging == null ? new Base[0] : new Base[] { this.paging }; // BooleanType 2596 case -1010022050: 2597 /* incomplete */ return this.incomplete == null ? new Base[0] : new Base[] { this.incomplete }; // BooleanType 2598 case 1954460585: 2599 /* parameter */ return this.parameter == null ? new Base[0] 2600 : this.parameter.toArray(new Base[this.parameter.size()]); // TerminologyCapabilitiesExpansionParameterComponent 2601 case 1469359877: 2602 /* textFilter */ return this.textFilter == null ? new Base[0] : new Base[] { this.textFilter }; // MarkdownType 2603 default: 2604 return super.getProperty(hash, name, checkValid); 2605 } 2606 2607 } 2608 2609 @Override 2610 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2611 switch (hash) { 2612 case 857636745: // hierarchical 2613 this.hierarchical = castToBoolean(value); // BooleanType 2614 return value; 2615 case -995747956: // paging 2616 this.paging = castToBoolean(value); // BooleanType 2617 return value; 2618 case -1010022050: // incomplete 2619 this.incomplete = castToBoolean(value); // BooleanType 2620 return value; 2621 case 1954460585: // parameter 2622 this.getParameter().add((TerminologyCapabilitiesExpansionParameterComponent) value); // TerminologyCapabilitiesExpansionParameterComponent 2623 return value; 2624 case 1469359877: // textFilter 2625 this.textFilter = castToMarkdown(value); // MarkdownType 2626 return value; 2627 default: 2628 return super.setProperty(hash, name, value); 2629 } 2630 2631 } 2632 2633 @Override 2634 public Base setProperty(String name, Base value) throws FHIRException { 2635 if (name.equals("hierarchical")) { 2636 this.hierarchical = castToBoolean(value); // BooleanType 2637 } else if (name.equals("paging")) { 2638 this.paging = castToBoolean(value); // BooleanType 2639 } else if (name.equals("incomplete")) { 2640 this.incomplete = castToBoolean(value); // BooleanType 2641 } else if (name.equals("parameter")) { 2642 this.getParameter().add((TerminologyCapabilitiesExpansionParameterComponent) value); 2643 } else if (name.equals("textFilter")) { 2644 this.textFilter = castToMarkdown(value); // MarkdownType 2645 } else 2646 return super.setProperty(name, value); 2647 return value; 2648 } 2649 2650 @Override 2651 public void removeChild(String name, Base value) throws FHIRException { 2652 if (name.equals("hierarchical")) { 2653 this.hierarchical = null; 2654 } else if (name.equals("paging")) { 2655 this.paging = null; 2656 } else if (name.equals("incomplete")) { 2657 this.incomplete = null; 2658 } else if (name.equals("parameter")) { 2659 this.getParameter().remove((TerminologyCapabilitiesExpansionParameterComponent) value); 2660 } else if (name.equals("textFilter")) { 2661 this.textFilter = null; 2662 } else 2663 super.removeChild(name, value); 2664 2665 } 2666 2667 @Override 2668 public Base makeProperty(int hash, String name) throws FHIRException { 2669 switch (hash) { 2670 case 857636745: 2671 return getHierarchicalElement(); 2672 case -995747956: 2673 return getPagingElement(); 2674 case -1010022050: 2675 return getIncompleteElement(); 2676 case 1954460585: 2677 return addParameter(); 2678 case 1469359877: 2679 return getTextFilterElement(); 2680 default: 2681 return super.makeProperty(hash, name); 2682 } 2683 2684 } 2685 2686 @Override 2687 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2688 switch (hash) { 2689 case 857636745: 2690 /* hierarchical */ return new String[] { "boolean" }; 2691 case -995747956: 2692 /* paging */ return new String[] { "boolean" }; 2693 case -1010022050: 2694 /* incomplete */ return new String[] { "boolean" }; 2695 case 1954460585: 2696 /* parameter */ return new String[] {}; 2697 case 1469359877: 2698 /* textFilter */ return new String[] { "markdown" }; 2699 default: 2700 return super.getTypesForProperty(hash, name); 2701 } 2702 2703 } 2704 2705 @Override 2706 public Base addChild(String name) throws FHIRException { 2707 if (name.equals("hierarchical")) { 2708 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.hierarchical"); 2709 } else if (name.equals("paging")) { 2710 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.paging"); 2711 } else if (name.equals("incomplete")) { 2712 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.incomplete"); 2713 } else if (name.equals("parameter")) { 2714 return addParameter(); 2715 } else if (name.equals("textFilter")) { 2716 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.textFilter"); 2717 } else 2718 return super.addChild(name); 2719 } 2720 2721 public TerminologyCapabilitiesExpansionComponent copy() { 2722 TerminologyCapabilitiesExpansionComponent dst = new TerminologyCapabilitiesExpansionComponent(); 2723 copyValues(dst); 2724 return dst; 2725 } 2726 2727 public void copyValues(TerminologyCapabilitiesExpansionComponent dst) { 2728 super.copyValues(dst); 2729 dst.hierarchical = hierarchical == null ? null : hierarchical.copy(); 2730 dst.paging = paging == null ? null : paging.copy(); 2731 dst.incomplete = incomplete == null ? null : incomplete.copy(); 2732 if (parameter != null) { 2733 dst.parameter = new ArrayList<TerminologyCapabilitiesExpansionParameterComponent>(); 2734 for (TerminologyCapabilitiesExpansionParameterComponent i : parameter) 2735 dst.parameter.add(i.copy()); 2736 } 2737 ; 2738 dst.textFilter = textFilter == null ? null : textFilter.copy(); 2739 } 2740 2741 @Override 2742 public boolean equalsDeep(Base other_) { 2743 if (!super.equalsDeep(other_)) 2744 return false; 2745 if (!(other_ instanceof TerminologyCapabilitiesExpansionComponent)) 2746 return false; 2747 TerminologyCapabilitiesExpansionComponent o = (TerminologyCapabilitiesExpansionComponent) other_; 2748 return compareDeep(hierarchical, o.hierarchical, true) && compareDeep(paging, o.paging, true) 2749 && compareDeep(incomplete, o.incomplete, true) && compareDeep(parameter, o.parameter, true) 2750 && compareDeep(textFilter, o.textFilter, true); 2751 } 2752 2753 @Override 2754 public boolean equalsShallow(Base other_) { 2755 if (!super.equalsShallow(other_)) 2756 return false; 2757 if (!(other_ instanceof TerminologyCapabilitiesExpansionComponent)) 2758 return false; 2759 TerminologyCapabilitiesExpansionComponent o = (TerminologyCapabilitiesExpansionComponent) other_; 2760 return compareValues(hierarchical, o.hierarchical, true) && compareValues(paging, o.paging, true) 2761 && compareValues(incomplete, o.incomplete, true) && compareValues(textFilter, o.textFilter, true); 2762 } 2763 2764 public boolean isEmpty() { 2765 return super.isEmpty() 2766 && ca.uhn.fhir.util.ElementUtil.isEmpty(hierarchical, paging, incomplete, parameter, textFilter); 2767 } 2768 2769 public String fhirType() { 2770 return "TerminologyCapabilities.expansion"; 2771 2772 } 2773 2774 } 2775 2776 @Block() 2777 public static class TerminologyCapabilitiesExpansionParameterComponent extends BackboneElement 2778 implements IBaseBackboneElement { 2779 /** 2780 * Expansion Parameter name. 2781 */ 2782 @Child(name = "name", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2783 @Description(shortDefinition = "Expansion Parameter name", formalDefinition = "Expansion Parameter name.") 2784 protected CodeType name; 2785 2786 /** 2787 * Description of support for parameter. 2788 */ 2789 @Child(name = "documentation", type = { 2790 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2791 @Description(shortDefinition = "Description of support for parameter", formalDefinition = "Description of support for parameter.") 2792 protected StringType documentation; 2793 2794 private static final long serialVersionUID = -1703372741L; 2795 2796 /** 2797 * Constructor 2798 */ 2799 public TerminologyCapabilitiesExpansionParameterComponent() { 2800 super(); 2801 } 2802 2803 /** 2804 * Constructor 2805 */ 2806 public TerminologyCapabilitiesExpansionParameterComponent(CodeType name) { 2807 super(); 2808 this.name = name; 2809 } 2810 2811 /** 2812 * @return {@link #name} (Expansion Parameter name.). This is the underlying 2813 * object with id, value and extensions. The accessor "getName" gives 2814 * direct access to the value 2815 */ 2816 public CodeType getNameElement() { 2817 if (this.name == null) 2818 if (Configuration.errorOnAutoCreate()) 2819 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionParameterComponent.name"); 2820 else if (Configuration.doAutoCreate()) 2821 this.name = new CodeType(); // bb 2822 return this.name; 2823 } 2824 2825 public boolean hasNameElement() { 2826 return this.name != null && !this.name.isEmpty(); 2827 } 2828 2829 public boolean hasName() { 2830 return this.name != null && !this.name.isEmpty(); 2831 } 2832 2833 /** 2834 * @param value {@link #name} (Expansion Parameter name.). This is the 2835 * underlying object with id, value and extensions. The accessor 2836 * "getName" gives direct access to the value 2837 */ 2838 public TerminologyCapabilitiesExpansionParameterComponent setNameElement(CodeType value) { 2839 this.name = value; 2840 return this; 2841 } 2842 2843 /** 2844 * @return Expansion Parameter name. 2845 */ 2846 public String getName() { 2847 return this.name == null ? null : this.name.getValue(); 2848 } 2849 2850 /** 2851 * @param value Expansion Parameter name. 2852 */ 2853 public TerminologyCapabilitiesExpansionParameterComponent setName(String value) { 2854 if (this.name == null) 2855 this.name = new CodeType(); 2856 this.name.setValue(value); 2857 return this; 2858 } 2859 2860 /** 2861 * @return {@link #documentation} (Description of support for parameter.). This 2862 * is the underlying object with id, value and extensions. The accessor 2863 * "getDocumentation" gives direct access to the value 2864 */ 2865 public StringType getDocumentationElement() { 2866 if (this.documentation == null) 2867 if (Configuration.errorOnAutoCreate()) 2868 throw new Error("Attempt to auto-create TerminologyCapabilitiesExpansionParameterComponent.documentation"); 2869 else if (Configuration.doAutoCreate()) 2870 this.documentation = new StringType(); // bb 2871 return this.documentation; 2872 } 2873 2874 public boolean hasDocumentationElement() { 2875 return this.documentation != null && !this.documentation.isEmpty(); 2876 } 2877 2878 public boolean hasDocumentation() { 2879 return this.documentation != null && !this.documentation.isEmpty(); 2880 } 2881 2882 /** 2883 * @param value {@link #documentation} (Description of support for parameter.). 2884 * This is the underlying object with id, value and extensions. The 2885 * accessor "getDocumentation" gives direct access to the value 2886 */ 2887 public TerminologyCapabilitiesExpansionParameterComponent setDocumentationElement(StringType value) { 2888 this.documentation = value; 2889 return this; 2890 } 2891 2892 /** 2893 * @return Description of support for parameter. 2894 */ 2895 public String getDocumentation() { 2896 return this.documentation == null ? null : this.documentation.getValue(); 2897 } 2898 2899 /** 2900 * @param value Description of support for parameter. 2901 */ 2902 public TerminologyCapabilitiesExpansionParameterComponent setDocumentation(String value) { 2903 if (Utilities.noString(value)) 2904 this.documentation = null; 2905 else { 2906 if (this.documentation == null) 2907 this.documentation = new StringType(); 2908 this.documentation.setValue(value); 2909 } 2910 return this; 2911 } 2912 2913 protected void listChildren(List<Property> children) { 2914 super.listChildren(children); 2915 children.add(new Property("name", "code", "Expansion Parameter name.", 0, 1, name)); 2916 children 2917 .add(new Property("documentation", "string", "Description of support for parameter.", 0, 1, documentation)); 2918 } 2919 2920 @Override 2921 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2922 switch (_hash) { 2923 case 3373707: 2924 /* name */ return new Property("name", "code", "Expansion Parameter name.", 0, 1, name); 2925 case 1587405498: 2926 /* documentation */ return new Property("documentation", "string", "Description of support for parameter.", 0, 2927 1, documentation); 2928 default: 2929 return super.getNamedProperty(_hash, _name, _checkValid); 2930 } 2931 2932 } 2933 2934 @Override 2935 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2936 switch (hash) { 2937 case 3373707: 2938 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // CodeType 2939 case 1587405498: 2940 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 2941 default: 2942 return super.getProperty(hash, name, checkValid); 2943 } 2944 2945 } 2946 2947 @Override 2948 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2949 switch (hash) { 2950 case 3373707: // name 2951 this.name = castToCode(value); // CodeType 2952 return value; 2953 case 1587405498: // documentation 2954 this.documentation = castToString(value); // StringType 2955 return value; 2956 default: 2957 return super.setProperty(hash, name, value); 2958 } 2959 2960 } 2961 2962 @Override 2963 public Base setProperty(String name, Base value) throws FHIRException { 2964 if (name.equals("name")) { 2965 this.name = castToCode(value); // CodeType 2966 } else if (name.equals("documentation")) { 2967 this.documentation = castToString(value); // StringType 2968 } else 2969 return super.setProperty(name, value); 2970 return value; 2971 } 2972 2973 @Override 2974 public void removeChild(String name, Base value) throws FHIRException { 2975 if (name.equals("name")) { 2976 this.name = null; 2977 } else if (name.equals("documentation")) { 2978 this.documentation = null; 2979 } else 2980 super.removeChild(name, value); 2981 2982 } 2983 2984 @Override 2985 public Base makeProperty(int hash, String name) throws FHIRException { 2986 switch (hash) { 2987 case 3373707: 2988 return getNameElement(); 2989 case 1587405498: 2990 return getDocumentationElement(); 2991 default: 2992 return super.makeProperty(hash, name); 2993 } 2994 2995 } 2996 2997 @Override 2998 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2999 switch (hash) { 3000 case 3373707: 3001 /* name */ return new String[] { "code" }; 3002 case 1587405498: 3003 /* documentation */ return new String[] { "string" }; 3004 default: 3005 return super.getTypesForProperty(hash, name); 3006 } 3007 3008 } 3009 3010 @Override 3011 public Base addChild(String name) throws FHIRException { 3012 if (name.equals("name")) { 3013 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.name"); 3014 } else if (name.equals("documentation")) { 3015 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.documentation"); 3016 } else 3017 return super.addChild(name); 3018 } 3019 3020 public TerminologyCapabilitiesExpansionParameterComponent copy() { 3021 TerminologyCapabilitiesExpansionParameterComponent dst = new TerminologyCapabilitiesExpansionParameterComponent(); 3022 copyValues(dst); 3023 return dst; 3024 } 3025 3026 public void copyValues(TerminologyCapabilitiesExpansionParameterComponent dst) { 3027 super.copyValues(dst); 3028 dst.name = name == null ? null : name.copy(); 3029 dst.documentation = documentation == null ? null : documentation.copy(); 3030 } 3031 3032 @Override 3033 public boolean equalsDeep(Base other_) { 3034 if (!super.equalsDeep(other_)) 3035 return false; 3036 if (!(other_ instanceof TerminologyCapabilitiesExpansionParameterComponent)) 3037 return false; 3038 TerminologyCapabilitiesExpansionParameterComponent o = (TerminologyCapabilitiesExpansionParameterComponent) other_; 3039 return compareDeep(name, o.name, true) && compareDeep(documentation, o.documentation, true); 3040 } 3041 3042 @Override 3043 public boolean equalsShallow(Base other_) { 3044 if (!super.equalsShallow(other_)) 3045 return false; 3046 if (!(other_ instanceof TerminologyCapabilitiesExpansionParameterComponent)) 3047 return false; 3048 TerminologyCapabilitiesExpansionParameterComponent o = (TerminologyCapabilitiesExpansionParameterComponent) other_; 3049 return compareValues(name, o.name, true) && compareValues(documentation, o.documentation, true); 3050 } 3051 3052 public boolean isEmpty() { 3053 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, documentation); 3054 } 3055 3056 public String fhirType() { 3057 return "TerminologyCapabilities.expansion.parameter"; 3058 3059 } 3060 3061 } 3062 3063 @Block() 3064 public static class TerminologyCapabilitiesValidateCodeComponent extends BackboneElement 3065 implements IBaseBackboneElement { 3066 /** 3067 * Whether translations are validated. 3068 */ 3069 @Child(name = "translations", type = { 3070 BooleanType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3071 @Description(shortDefinition = "Whether translations are validated", formalDefinition = "Whether translations are validated.") 3072 protected BooleanType translations; 3073 3074 private static final long serialVersionUID = -1212814906L; 3075 3076 /** 3077 * Constructor 3078 */ 3079 public TerminologyCapabilitiesValidateCodeComponent() { 3080 super(); 3081 } 3082 3083 /** 3084 * Constructor 3085 */ 3086 public TerminologyCapabilitiesValidateCodeComponent(BooleanType translations) { 3087 super(); 3088 this.translations = translations; 3089 } 3090 3091 /** 3092 * @return {@link #translations} (Whether translations are validated.). This is 3093 * the underlying object with id, value and extensions. The accessor 3094 * "getTranslations" gives direct access to the value 3095 */ 3096 public BooleanType getTranslationsElement() { 3097 if (this.translations == null) 3098 if (Configuration.errorOnAutoCreate()) 3099 throw new Error("Attempt to auto-create TerminologyCapabilitiesValidateCodeComponent.translations"); 3100 else if (Configuration.doAutoCreate()) 3101 this.translations = new BooleanType(); // bb 3102 return this.translations; 3103 } 3104 3105 public boolean hasTranslationsElement() { 3106 return this.translations != null && !this.translations.isEmpty(); 3107 } 3108 3109 public boolean hasTranslations() { 3110 return this.translations != null && !this.translations.isEmpty(); 3111 } 3112 3113 /** 3114 * @param value {@link #translations} (Whether translations are validated.). 3115 * This is the underlying object with id, value and extensions. The 3116 * accessor "getTranslations" gives direct access to the value 3117 */ 3118 public TerminologyCapabilitiesValidateCodeComponent setTranslationsElement(BooleanType value) { 3119 this.translations = value; 3120 return this; 3121 } 3122 3123 /** 3124 * @return Whether translations are validated. 3125 */ 3126 public boolean getTranslations() { 3127 return this.translations == null || this.translations.isEmpty() ? false : this.translations.getValue(); 3128 } 3129 3130 /** 3131 * @param value Whether translations are validated. 3132 */ 3133 public TerminologyCapabilitiesValidateCodeComponent setTranslations(boolean value) { 3134 if (this.translations == null) 3135 this.translations = new BooleanType(); 3136 this.translations.setValue(value); 3137 return this; 3138 } 3139 3140 protected void listChildren(List<Property> children) { 3141 super.listChildren(children); 3142 children.add(new Property("translations", "boolean", "Whether translations are validated.", 0, 1, translations)); 3143 } 3144 3145 @Override 3146 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3147 switch (_hash) { 3148 case -1225497630: 3149 /* translations */ return new Property("translations", "boolean", "Whether translations are validated.", 0, 1, 3150 translations); 3151 default: 3152 return super.getNamedProperty(_hash, _name, _checkValid); 3153 } 3154 3155 } 3156 3157 @Override 3158 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3159 switch (hash) { 3160 case -1225497630: 3161 /* translations */ return this.translations == null ? new Base[0] : new Base[] { this.translations }; // BooleanType 3162 default: 3163 return super.getProperty(hash, name, checkValid); 3164 } 3165 3166 } 3167 3168 @Override 3169 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3170 switch (hash) { 3171 case -1225497630: // translations 3172 this.translations = castToBoolean(value); // BooleanType 3173 return value; 3174 default: 3175 return super.setProperty(hash, name, value); 3176 } 3177 3178 } 3179 3180 @Override 3181 public Base setProperty(String name, Base value) throws FHIRException { 3182 if (name.equals("translations")) { 3183 this.translations = castToBoolean(value); // BooleanType 3184 } else 3185 return super.setProperty(name, value); 3186 return value; 3187 } 3188 3189 @Override 3190 public void removeChild(String name, Base value) throws FHIRException { 3191 if (name.equals("translations")) { 3192 this.translations = null; 3193 } else 3194 super.removeChild(name, value); 3195 3196 } 3197 3198 @Override 3199 public Base makeProperty(int hash, String name) throws FHIRException { 3200 switch (hash) { 3201 case -1225497630: 3202 return getTranslationsElement(); 3203 default: 3204 return super.makeProperty(hash, name); 3205 } 3206 3207 } 3208 3209 @Override 3210 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3211 switch (hash) { 3212 case -1225497630: 3213 /* translations */ return new String[] { "boolean" }; 3214 default: 3215 return super.getTypesForProperty(hash, name); 3216 } 3217 3218 } 3219 3220 @Override 3221 public Base addChild(String name) throws FHIRException { 3222 if (name.equals("translations")) { 3223 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.translations"); 3224 } else 3225 return super.addChild(name); 3226 } 3227 3228 public TerminologyCapabilitiesValidateCodeComponent copy() { 3229 TerminologyCapabilitiesValidateCodeComponent dst = new TerminologyCapabilitiesValidateCodeComponent(); 3230 copyValues(dst); 3231 return dst; 3232 } 3233 3234 public void copyValues(TerminologyCapabilitiesValidateCodeComponent dst) { 3235 super.copyValues(dst); 3236 dst.translations = translations == null ? null : translations.copy(); 3237 } 3238 3239 @Override 3240 public boolean equalsDeep(Base other_) { 3241 if (!super.equalsDeep(other_)) 3242 return false; 3243 if (!(other_ instanceof TerminologyCapabilitiesValidateCodeComponent)) 3244 return false; 3245 TerminologyCapabilitiesValidateCodeComponent o = (TerminologyCapabilitiesValidateCodeComponent) other_; 3246 return compareDeep(translations, o.translations, true); 3247 } 3248 3249 @Override 3250 public boolean equalsShallow(Base other_) { 3251 if (!super.equalsShallow(other_)) 3252 return false; 3253 if (!(other_ instanceof TerminologyCapabilitiesValidateCodeComponent)) 3254 return false; 3255 TerminologyCapabilitiesValidateCodeComponent o = (TerminologyCapabilitiesValidateCodeComponent) other_; 3256 return compareValues(translations, o.translations, true); 3257 } 3258 3259 public boolean isEmpty() { 3260 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(translations); 3261 } 3262 3263 public String fhirType() { 3264 return "TerminologyCapabilities.validateCode"; 3265 3266 } 3267 3268 } 3269 3270 @Block() 3271 public static class TerminologyCapabilitiesTranslationComponent extends BackboneElement 3272 implements IBaseBackboneElement { 3273 /** 3274 * Whether the client must identify the map. 3275 */ 3276 @Child(name = "needsMap", type = { 3277 BooleanType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3278 @Description(shortDefinition = "Whether the client must identify the map", formalDefinition = "Whether the client must identify the map.") 3279 protected BooleanType needsMap; 3280 3281 private static final long serialVersionUID = -1727843575L; 3282 3283 /** 3284 * Constructor 3285 */ 3286 public TerminologyCapabilitiesTranslationComponent() { 3287 super(); 3288 } 3289 3290 /** 3291 * Constructor 3292 */ 3293 public TerminologyCapabilitiesTranslationComponent(BooleanType needsMap) { 3294 super(); 3295 this.needsMap = needsMap; 3296 } 3297 3298 /** 3299 * @return {@link #needsMap} (Whether the client must identify the map.). This 3300 * is the underlying object with id, value and extensions. The accessor 3301 * "getNeedsMap" gives direct access to the value 3302 */ 3303 public BooleanType getNeedsMapElement() { 3304 if (this.needsMap == null) 3305 if (Configuration.errorOnAutoCreate()) 3306 throw new Error("Attempt to auto-create TerminologyCapabilitiesTranslationComponent.needsMap"); 3307 else if (Configuration.doAutoCreate()) 3308 this.needsMap = new BooleanType(); // bb 3309 return this.needsMap; 3310 } 3311 3312 public boolean hasNeedsMapElement() { 3313 return this.needsMap != null && !this.needsMap.isEmpty(); 3314 } 3315 3316 public boolean hasNeedsMap() { 3317 return this.needsMap != null && !this.needsMap.isEmpty(); 3318 } 3319 3320 /** 3321 * @param value {@link #needsMap} (Whether the client must identify the map.). 3322 * This is the underlying object with id, value and extensions. The 3323 * accessor "getNeedsMap" gives direct access to the value 3324 */ 3325 public TerminologyCapabilitiesTranslationComponent setNeedsMapElement(BooleanType value) { 3326 this.needsMap = value; 3327 return this; 3328 } 3329 3330 /** 3331 * @return Whether the client must identify the map. 3332 */ 3333 public boolean getNeedsMap() { 3334 return this.needsMap == null || this.needsMap.isEmpty() ? false : this.needsMap.getValue(); 3335 } 3336 3337 /** 3338 * @param value Whether the client must identify the map. 3339 */ 3340 public TerminologyCapabilitiesTranslationComponent setNeedsMap(boolean value) { 3341 if (this.needsMap == null) 3342 this.needsMap = new BooleanType(); 3343 this.needsMap.setValue(value); 3344 return this; 3345 } 3346 3347 protected void listChildren(List<Property> children) { 3348 super.listChildren(children); 3349 children.add(new Property("needsMap", "boolean", "Whether the client must identify the map.", 0, 1, needsMap)); 3350 } 3351 3352 @Override 3353 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3354 switch (_hash) { 3355 case 866566527: 3356 /* needsMap */ return new Property("needsMap", "boolean", "Whether the client must identify the map.", 0, 1, 3357 needsMap); 3358 default: 3359 return super.getNamedProperty(_hash, _name, _checkValid); 3360 } 3361 3362 } 3363 3364 @Override 3365 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3366 switch (hash) { 3367 case 866566527: 3368 /* needsMap */ return this.needsMap == null ? new Base[0] : new Base[] { this.needsMap }; // BooleanType 3369 default: 3370 return super.getProperty(hash, name, checkValid); 3371 } 3372 3373 } 3374 3375 @Override 3376 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3377 switch (hash) { 3378 case 866566527: // needsMap 3379 this.needsMap = castToBoolean(value); // BooleanType 3380 return value; 3381 default: 3382 return super.setProperty(hash, name, value); 3383 } 3384 3385 } 3386 3387 @Override 3388 public Base setProperty(String name, Base value) throws FHIRException { 3389 if (name.equals("needsMap")) { 3390 this.needsMap = castToBoolean(value); // BooleanType 3391 } else 3392 return super.setProperty(name, value); 3393 return value; 3394 } 3395 3396 @Override 3397 public void removeChild(String name, Base value) throws FHIRException { 3398 if (name.equals("needsMap")) { 3399 this.needsMap = null; 3400 } else 3401 super.removeChild(name, value); 3402 3403 } 3404 3405 @Override 3406 public Base makeProperty(int hash, String name) throws FHIRException { 3407 switch (hash) { 3408 case 866566527: 3409 return getNeedsMapElement(); 3410 default: 3411 return super.makeProperty(hash, name); 3412 } 3413 3414 } 3415 3416 @Override 3417 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3418 switch (hash) { 3419 case 866566527: 3420 /* needsMap */ return new String[] { "boolean" }; 3421 default: 3422 return super.getTypesForProperty(hash, name); 3423 } 3424 3425 } 3426 3427 @Override 3428 public Base addChild(String name) throws FHIRException { 3429 if (name.equals("needsMap")) { 3430 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.needsMap"); 3431 } else 3432 return super.addChild(name); 3433 } 3434 3435 public TerminologyCapabilitiesTranslationComponent copy() { 3436 TerminologyCapabilitiesTranslationComponent dst = new TerminologyCapabilitiesTranslationComponent(); 3437 copyValues(dst); 3438 return dst; 3439 } 3440 3441 public void copyValues(TerminologyCapabilitiesTranslationComponent dst) { 3442 super.copyValues(dst); 3443 dst.needsMap = needsMap == null ? null : needsMap.copy(); 3444 } 3445 3446 @Override 3447 public boolean equalsDeep(Base other_) { 3448 if (!super.equalsDeep(other_)) 3449 return false; 3450 if (!(other_ instanceof TerminologyCapabilitiesTranslationComponent)) 3451 return false; 3452 TerminologyCapabilitiesTranslationComponent o = (TerminologyCapabilitiesTranslationComponent) other_; 3453 return compareDeep(needsMap, o.needsMap, true); 3454 } 3455 3456 @Override 3457 public boolean equalsShallow(Base other_) { 3458 if (!super.equalsShallow(other_)) 3459 return false; 3460 if (!(other_ instanceof TerminologyCapabilitiesTranslationComponent)) 3461 return false; 3462 TerminologyCapabilitiesTranslationComponent o = (TerminologyCapabilitiesTranslationComponent) other_; 3463 return compareValues(needsMap, o.needsMap, true); 3464 } 3465 3466 public boolean isEmpty() { 3467 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(needsMap); 3468 } 3469 3470 public String fhirType() { 3471 return "TerminologyCapabilities.translation"; 3472 3473 } 3474 3475 } 3476 3477 @Block() 3478 public static class TerminologyCapabilitiesClosureComponent extends BackboneElement implements IBaseBackboneElement { 3479 /** 3480 * If cross-system closure is supported. 3481 */ 3482 @Child(name = "translation", type = { 3483 BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3484 @Description(shortDefinition = "If cross-system closure is supported", formalDefinition = "If cross-system closure is supported.") 3485 protected BooleanType translation; 3486 3487 private static final long serialVersionUID = 1900484343L; 3488 3489 /** 3490 * Constructor 3491 */ 3492 public TerminologyCapabilitiesClosureComponent() { 3493 super(); 3494 } 3495 3496 /** 3497 * @return {@link #translation} (If cross-system closure is supported.). This is 3498 * the underlying object with id, value and extensions. The accessor 3499 * "getTranslation" gives direct access to the value 3500 */ 3501 public BooleanType getTranslationElement() { 3502 if (this.translation == null) 3503 if (Configuration.errorOnAutoCreate()) 3504 throw new Error("Attempt to auto-create TerminologyCapabilitiesClosureComponent.translation"); 3505 else if (Configuration.doAutoCreate()) 3506 this.translation = new BooleanType(); // bb 3507 return this.translation; 3508 } 3509 3510 public boolean hasTranslationElement() { 3511 return this.translation != null && !this.translation.isEmpty(); 3512 } 3513 3514 public boolean hasTranslation() { 3515 return this.translation != null && !this.translation.isEmpty(); 3516 } 3517 3518 /** 3519 * @param value {@link #translation} (If cross-system closure is supported.). 3520 * This is the underlying object with id, value and extensions. The 3521 * accessor "getTranslation" gives direct access to the value 3522 */ 3523 public TerminologyCapabilitiesClosureComponent setTranslationElement(BooleanType value) { 3524 this.translation = value; 3525 return this; 3526 } 3527 3528 /** 3529 * @return If cross-system closure is supported. 3530 */ 3531 public boolean getTranslation() { 3532 return this.translation == null || this.translation.isEmpty() ? false : this.translation.getValue(); 3533 } 3534 3535 /** 3536 * @param value If cross-system closure is supported. 3537 */ 3538 public TerminologyCapabilitiesClosureComponent setTranslation(boolean value) { 3539 if (this.translation == null) 3540 this.translation = new BooleanType(); 3541 this.translation.setValue(value); 3542 return this; 3543 } 3544 3545 protected void listChildren(List<Property> children) { 3546 super.listChildren(children); 3547 children.add(new Property("translation", "boolean", "If cross-system closure is supported.", 0, 1, translation)); 3548 } 3549 3550 @Override 3551 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3552 switch (_hash) { 3553 case -1840647503: 3554 /* translation */ return new Property("translation", "boolean", "If cross-system closure is supported.", 0, 1, 3555 translation); 3556 default: 3557 return super.getNamedProperty(_hash, _name, _checkValid); 3558 } 3559 3560 } 3561 3562 @Override 3563 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3564 switch (hash) { 3565 case -1840647503: 3566 /* translation */ return this.translation == null ? new Base[0] : new Base[] { this.translation }; // BooleanType 3567 default: 3568 return super.getProperty(hash, name, checkValid); 3569 } 3570 3571 } 3572 3573 @Override 3574 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3575 switch (hash) { 3576 case -1840647503: // translation 3577 this.translation = castToBoolean(value); // BooleanType 3578 return value; 3579 default: 3580 return super.setProperty(hash, name, value); 3581 } 3582 3583 } 3584 3585 @Override 3586 public Base setProperty(String name, Base value) throws FHIRException { 3587 if (name.equals("translation")) { 3588 this.translation = castToBoolean(value); // BooleanType 3589 } else 3590 return super.setProperty(name, value); 3591 return value; 3592 } 3593 3594 @Override 3595 public void removeChild(String name, Base value) throws FHIRException { 3596 if (name.equals("translation")) { 3597 this.translation = null; 3598 } else 3599 super.removeChild(name, value); 3600 3601 } 3602 3603 @Override 3604 public Base makeProperty(int hash, String name) throws FHIRException { 3605 switch (hash) { 3606 case -1840647503: 3607 return getTranslationElement(); 3608 default: 3609 return super.makeProperty(hash, name); 3610 } 3611 3612 } 3613 3614 @Override 3615 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3616 switch (hash) { 3617 case -1840647503: 3618 /* translation */ return new String[] { "boolean" }; 3619 default: 3620 return super.getTypesForProperty(hash, name); 3621 } 3622 3623 } 3624 3625 @Override 3626 public Base addChild(String name) throws FHIRException { 3627 if (name.equals("translation")) { 3628 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.translation"); 3629 } else 3630 return super.addChild(name); 3631 } 3632 3633 public TerminologyCapabilitiesClosureComponent copy() { 3634 TerminologyCapabilitiesClosureComponent dst = new TerminologyCapabilitiesClosureComponent(); 3635 copyValues(dst); 3636 return dst; 3637 } 3638 3639 public void copyValues(TerminologyCapabilitiesClosureComponent dst) { 3640 super.copyValues(dst); 3641 dst.translation = translation == null ? null : translation.copy(); 3642 } 3643 3644 @Override 3645 public boolean equalsDeep(Base other_) { 3646 if (!super.equalsDeep(other_)) 3647 return false; 3648 if (!(other_ instanceof TerminologyCapabilitiesClosureComponent)) 3649 return false; 3650 TerminologyCapabilitiesClosureComponent o = (TerminologyCapabilitiesClosureComponent) other_; 3651 return compareDeep(translation, o.translation, true); 3652 } 3653 3654 @Override 3655 public boolean equalsShallow(Base other_) { 3656 if (!super.equalsShallow(other_)) 3657 return false; 3658 if (!(other_ instanceof TerminologyCapabilitiesClosureComponent)) 3659 return false; 3660 TerminologyCapabilitiesClosureComponent o = (TerminologyCapabilitiesClosureComponent) other_; 3661 return compareValues(translation, o.translation, true); 3662 } 3663 3664 public boolean isEmpty() { 3665 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(translation); 3666 } 3667 3668 public String fhirType() { 3669 return "TerminologyCapabilities.closure"; 3670 3671 } 3672 3673 } 3674 3675 /** 3676 * Explanation of why this terminology capabilities is needed and why it has 3677 * been designed as it has. 3678 */ 3679 @Child(name = "purpose", type = { 3680 MarkdownType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 3681 @Description(shortDefinition = "Why this terminology capabilities is defined", formalDefinition = "Explanation of why this terminology capabilities is needed and why it has been designed as it has.") 3682 protected MarkdownType purpose; 3683 3684 /** 3685 * A copyright statement relating to the terminology capabilities and/or its 3686 * contents. Copyright statements are generally legal restrictions on the use 3687 * and publishing of the terminology capabilities. 3688 */ 3689 @Child(name = "copyright", type = { 3690 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 3691 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities.") 3692 protected MarkdownType copyright; 3693 3694 /** 3695 * The way that this statement is intended to be used, to describe an actual 3696 * running instance of software, a particular product (kind, not instance of 3697 * software) or a class of implementation (e.g. a desired purchase). 3698 */ 3699 @Child(name = "kind", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 3700 @Description(shortDefinition = "instance | capability | requirements", formalDefinition = "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).") 3701 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/capability-statement-kind") 3702 protected Enumeration<CapabilityStatementKind> kind; 3703 3704 /** 3705 * Software that is covered by this terminology capability statement. It is used 3706 * when the statement describes the capabilities of a particular software 3707 * version, independent of an installation. 3708 */ 3709 @Child(name = "software", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = true) 3710 @Description(shortDefinition = "Software that is covered by this terminology capability statement", formalDefinition = "Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation.") 3711 protected TerminologyCapabilitiesSoftwareComponent software; 3712 3713 /** 3714 * Identifies a specific implementation instance that is described by the 3715 * terminology capability statement - i.e. a particular installation, rather 3716 * than the capabilities of a software program. 3717 */ 3718 @Child(name = "implementation", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = true) 3719 @Description(shortDefinition = "If this describes a specific instance", formalDefinition = "Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program.") 3720 protected TerminologyCapabilitiesImplementationComponent implementation; 3721 3722 /** 3723 * Whether the server supports lockedDate. 3724 */ 3725 @Child(name = "lockedDate", type = { 3726 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3727 @Description(shortDefinition = "Whether lockedDate is supported", formalDefinition = "Whether the server supports lockedDate.") 3728 protected BooleanType lockedDate; 3729 3730 /** 3731 * Identifies a code system that is supported by the server. If there is a no 3732 * code system URL, then this declares the general assumptions a client can make 3733 * about support for any CodeSystem resource. 3734 */ 3735 @Child(name = "codeSystem", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3736 @Description(shortDefinition = "A code system supported by the server", formalDefinition = "Identifies a code system that is supported by the server. If there is a no code system URL, then this declares the general assumptions a client can make about support for any CodeSystem resource.") 3737 protected List<TerminologyCapabilitiesCodeSystemComponent> codeSystem; 3738 3739 /** 3740 * Information about the [ValueSet/$expand](valueset-operation-expand.html) 3741 * operation. 3742 */ 3743 @Child(name = "expansion", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = false) 3744 @Description(shortDefinition = "Information about the [ValueSet/$expand](valueset-operation-expand.html) operation", formalDefinition = "Information about the [ValueSet/$expand](valueset-operation-expand.html) operation.") 3745 protected TerminologyCapabilitiesExpansionComponent expansion; 3746 3747 /** 3748 * The degree to which the server supports the code search parameter on 3749 * ValueSet, if it is supported. 3750 */ 3751 @Child(name = "codeSearch", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3752 @Description(shortDefinition = "explicit | all", formalDefinition = "The degree to which the server supports the code search parameter on ValueSet, if it is supported.") 3753 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/code-search-support") 3754 protected Enumeration<CodeSearchSupport> codeSearch; 3755 3756 /** 3757 * Information about the 3758 * [ValueSet/$validate-code](valueset-operation-validate-code.html) operation. 3759 */ 3760 @Child(name = "validateCode", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 3761 @Description(shortDefinition = "Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation", formalDefinition = "Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation.") 3762 protected TerminologyCapabilitiesValidateCodeComponent validateCode; 3763 3764 /** 3765 * Information about the 3766 * [ConceptMap/$translate](conceptmap-operation-translate.html) operation. 3767 */ 3768 @Child(name = "translation", type = {}, order = 10, min = 0, max = 1, modifier = false, summary = false) 3769 @Description(shortDefinition = "Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation", formalDefinition = "Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation.") 3770 protected TerminologyCapabilitiesTranslationComponent translation; 3771 3772 /** 3773 * Whether the $closure operation is supported. 3774 */ 3775 @Child(name = "closure", type = {}, order = 11, min = 0, max = 1, modifier = false, summary = false) 3776 @Description(shortDefinition = "Information about the [ConceptMap/$closure](conceptmap-operation-closure.html) operation", formalDefinition = "Whether the $closure operation is supported.") 3777 protected TerminologyCapabilitiesClosureComponent closure; 3778 3779 private static final long serialVersionUID = -1899106119L; 3780 3781 /** 3782 * Constructor 3783 */ 3784 public TerminologyCapabilities() { 3785 super(); 3786 } 3787 3788 /** 3789 * Constructor 3790 */ 3791 public TerminologyCapabilities(Enumeration<PublicationStatus> status, DateTimeType date, 3792 Enumeration<CapabilityStatementKind> kind) { 3793 super(); 3794 this.status = status; 3795 this.date = date; 3796 this.kind = kind; 3797 } 3798 3799 /** 3800 * @return {@link #url} (An absolute URI that is used to identify this 3801 * terminology capabilities when it is referenced in a specification, 3802 * model, design or an instance; also called its canonical identifier. 3803 * This SHOULD be globally unique and SHOULD be a literal address at 3804 * which at which an authoritative instance of this terminology 3805 * capabilities is (or will be) published. This URL can be the target of 3806 * a canonical reference. It SHALL remain the same when the terminology 3807 * capabilities is stored on different servers.). This is the underlying 3808 * object with id, value and extensions. The accessor "getUrl" gives 3809 * direct access to the value 3810 */ 3811 public UriType getUrlElement() { 3812 if (this.url == null) 3813 if (Configuration.errorOnAutoCreate()) 3814 throw new Error("Attempt to auto-create TerminologyCapabilities.url"); 3815 else if (Configuration.doAutoCreate()) 3816 this.url = new UriType(); // bb 3817 return this.url; 3818 } 3819 3820 public boolean hasUrlElement() { 3821 return this.url != null && !this.url.isEmpty(); 3822 } 3823 3824 public boolean hasUrl() { 3825 return this.url != null && !this.url.isEmpty(); 3826 } 3827 3828 /** 3829 * @param value {@link #url} (An absolute URI that is used to identify this 3830 * terminology capabilities when it is referenced in a 3831 * specification, model, design or an instance; also called its 3832 * canonical identifier. This SHOULD be globally unique and SHOULD 3833 * be a literal address at which at which an authoritative instance 3834 * of this terminology capabilities is (or will be) published. This 3835 * URL can be the target of a canonical reference. It SHALL remain 3836 * the same when the terminology capabilities is stored on 3837 * different servers.). This is the underlying object with id, 3838 * value and extensions. The accessor "getUrl" gives direct access 3839 * to the value 3840 */ 3841 public TerminologyCapabilities setUrlElement(UriType value) { 3842 this.url = value; 3843 return this; 3844 } 3845 3846 /** 3847 * @return An absolute URI that is used to identify this terminology 3848 * capabilities when it is referenced in a specification, model, design 3849 * or an instance; also called its canonical identifier. This SHOULD be 3850 * globally unique and SHOULD be a literal address at which at which an 3851 * authoritative instance of this terminology capabilities is (or will 3852 * be) published. This URL can be the target of a canonical reference. 3853 * It SHALL remain the same when the terminology capabilities is stored 3854 * on different servers. 3855 */ 3856 public String getUrl() { 3857 return this.url == null ? null : this.url.getValue(); 3858 } 3859 3860 /** 3861 * @param value An absolute URI that is used to identify this terminology 3862 * capabilities when it is referenced in a specification, model, 3863 * design or an instance; also called its canonical identifier. 3864 * This SHOULD be globally unique and SHOULD be a literal address 3865 * at which at which an authoritative instance of this terminology 3866 * capabilities is (or will be) published. This URL can be the 3867 * target of a canonical reference. It SHALL remain the same when 3868 * the terminology capabilities is stored on different servers. 3869 */ 3870 public TerminologyCapabilities setUrl(String value) { 3871 if (Utilities.noString(value)) 3872 this.url = null; 3873 else { 3874 if (this.url == null) 3875 this.url = new UriType(); 3876 this.url.setValue(value); 3877 } 3878 return this; 3879 } 3880 3881 /** 3882 * @return {@link #version} (The identifier that is used to identify this 3883 * version of the terminology capabilities when it is referenced in a 3884 * specification, model, design or instance. This is an arbitrary value 3885 * managed by the terminology capabilities author and is not expected to 3886 * be globally unique. For example, it might be a timestamp (e.g. 3887 * yyyymmdd) if a managed version is not available. There is also no 3888 * expectation that versions can be placed in a lexicographical 3889 * sequence.). This is the underlying object with id, value and 3890 * extensions. The accessor "getVersion" gives direct access to the 3891 * value 3892 */ 3893 public StringType getVersionElement() { 3894 if (this.version == null) 3895 if (Configuration.errorOnAutoCreate()) 3896 throw new Error("Attempt to auto-create TerminologyCapabilities.version"); 3897 else if (Configuration.doAutoCreate()) 3898 this.version = new StringType(); // bb 3899 return this.version; 3900 } 3901 3902 public boolean hasVersionElement() { 3903 return this.version != null && !this.version.isEmpty(); 3904 } 3905 3906 public boolean hasVersion() { 3907 return this.version != null && !this.version.isEmpty(); 3908 } 3909 3910 /** 3911 * @param value {@link #version} (The identifier that is used to identify this 3912 * version of the terminology capabilities when it is referenced in 3913 * a specification, model, design or instance. This is an arbitrary 3914 * value managed by the terminology capabilities author and is not 3915 * expected to be globally unique. For example, it might be a 3916 * timestamp (e.g. yyyymmdd) if a managed version is not available. 3917 * There is also no expectation that versions can be placed in a 3918 * lexicographical sequence.). This is the underlying object with 3919 * id, value and extensions. The accessor "getVersion" gives direct 3920 * access to the value 3921 */ 3922 public TerminologyCapabilities setVersionElement(StringType value) { 3923 this.version = value; 3924 return this; 3925 } 3926 3927 /** 3928 * @return The identifier that is used to identify this version of the 3929 * terminology capabilities when it is referenced in a specification, 3930 * model, design or instance. This is an arbitrary value managed by the 3931 * terminology capabilities author and is not expected to be globally 3932 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if a 3933 * managed version is not available. There is also no expectation that 3934 * versions can be placed in a lexicographical sequence. 3935 */ 3936 public String getVersion() { 3937 return this.version == null ? null : this.version.getValue(); 3938 } 3939 3940 /** 3941 * @param value The identifier that is used to identify this version of the 3942 * terminology capabilities when it is referenced in a 3943 * specification, model, design or instance. This is an arbitrary 3944 * value managed by the terminology capabilities author and is not 3945 * expected to be globally unique. For example, it might be a 3946 * timestamp (e.g. yyyymmdd) if a managed version is not available. 3947 * There is also no expectation that versions can be placed in a 3948 * lexicographical sequence. 3949 */ 3950 public TerminologyCapabilities setVersion(String value) { 3951 if (Utilities.noString(value)) 3952 this.version = null; 3953 else { 3954 if (this.version == null) 3955 this.version = new StringType(); 3956 this.version.setValue(value); 3957 } 3958 return this; 3959 } 3960 3961 /** 3962 * @return {@link #name} (A natural language name identifying the terminology 3963 * capabilities. This name should be usable as an identifier for the 3964 * module by machine processing applications such as code generation.). 3965 * This is the underlying object with id, value and extensions. The 3966 * accessor "getName" gives direct access to the value 3967 */ 3968 public StringType getNameElement() { 3969 if (this.name == null) 3970 if (Configuration.errorOnAutoCreate()) 3971 throw new Error("Attempt to auto-create TerminologyCapabilities.name"); 3972 else if (Configuration.doAutoCreate()) 3973 this.name = new StringType(); // bb 3974 return this.name; 3975 } 3976 3977 public boolean hasNameElement() { 3978 return this.name != null && !this.name.isEmpty(); 3979 } 3980 3981 public boolean hasName() { 3982 return this.name != null && !this.name.isEmpty(); 3983 } 3984 3985 /** 3986 * @param value {@link #name} (A natural language name identifying the 3987 * terminology capabilities. This name should be usable as an 3988 * identifier for the module by machine processing applications 3989 * such as code generation.). This is the underlying object with 3990 * id, value and extensions. The accessor "getName" gives direct 3991 * access to the value 3992 */ 3993 public TerminologyCapabilities setNameElement(StringType value) { 3994 this.name = value; 3995 return this; 3996 } 3997 3998 /** 3999 * @return A natural language name identifying the terminology capabilities. 4000 * This name should be usable as an identifier for the module by machine 4001 * processing applications such as code generation. 4002 */ 4003 public String getName() { 4004 return this.name == null ? null : this.name.getValue(); 4005 } 4006 4007 /** 4008 * @param value A natural language name identifying the terminology 4009 * capabilities. This name should be usable as an identifier for 4010 * the module by machine processing applications such as code 4011 * generation. 4012 */ 4013 public TerminologyCapabilities setName(String value) { 4014 if (Utilities.noString(value)) 4015 this.name = null; 4016 else { 4017 if (this.name == null) 4018 this.name = new StringType(); 4019 this.name.setValue(value); 4020 } 4021 return this; 4022 } 4023 4024 /** 4025 * @return {@link #title} (A short, descriptive, user-friendly title for the 4026 * terminology capabilities.). This is the underlying object with id, 4027 * value and extensions. The accessor "getTitle" gives direct access to 4028 * the value 4029 */ 4030 public StringType getTitleElement() { 4031 if (this.title == null) 4032 if (Configuration.errorOnAutoCreate()) 4033 throw new Error("Attempt to auto-create TerminologyCapabilities.title"); 4034 else if (Configuration.doAutoCreate()) 4035 this.title = new StringType(); // bb 4036 return this.title; 4037 } 4038 4039 public boolean hasTitleElement() { 4040 return this.title != null && !this.title.isEmpty(); 4041 } 4042 4043 public boolean hasTitle() { 4044 return this.title != null && !this.title.isEmpty(); 4045 } 4046 4047 /** 4048 * @param value {@link #title} (A short, descriptive, user-friendly title for 4049 * the terminology capabilities.). This is the underlying object 4050 * with id, value and extensions. The accessor "getTitle" gives 4051 * direct access to the value 4052 */ 4053 public TerminologyCapabilities setTitleElement(StringType value) { 4054 this.title = value; 4055 return this; 4056 } 4057 4058 /** 4059 * @return A short, descriptive, user-friendly title for the terminology 4060 * capabilities. 4061 */ 4062 public String getTitle() { 4063 return this.title == null ? null : this.title.getValue(); 4064 } 4065 4066 /** 4067 * @param value A short, descriptive, user-friendly title for the terminology 4068 * capabilities. 4069 */ 4070 public TerminologyCapabilities setTitle(String value) { 4071 if (Utilities.noString(value)) 4072 this.title = null; 4073 else { 4074 if (this.title == null) 4075 this.title = new StringType(); 4076 this.title.setValue(value); 4077 } 4078 return this; 4079 } 4080 4081 /** 4082 * @return {@link #status} (The status of this terminology capabilities. Enables 4083 * tracking the life-cycle of the content.). This is the underlying 4084 * object with id, value and extensions. The accessor "getStatus" gives 4085 * direct access to the value 4086 */ 4087 public Enumeration<PublicationStatus> getStatusElement() { 4088 if (this.status == null) 4089 if (Configuration.errorOnAutoCreate()) 4090 throw new Error("Attempt to auto-create TerminologyCapabilities.status"); 4091 else if (Configuration.doAutoCreate()) 4092 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 4093 return this.status; 4094 } 4095 4096 public boolean hasStatusElement() { 4097 return this.status != null && !this.status.isEmpty(); 4098 } 4099 4100 public boolean hasStatus() { 4101 return this.status != null && !this.status.isEmpty(); 4102 } 4103 4104 /** 4105 * @param value {@link #status} (The status of this terminology capabilities. 4106 * Enables tracking the life-cycle of the content.). This is the 4107 * underlying object with id, value and extensions. The accessor 4108 * "getStatus" gives direct access to the value 4109 */ 4110 public TerminologyCapabilities setStatusElement(Enumeration<PublicationStatus> value) { 4111 this.status = value; 4112 return this; 4113 } 4114 4115 /** 4116 * @return The status of this terminology capabilities. Enables tracking the 4117 * life-cycle of the content. 4118 */ 4119 public PublicationStatus getStatus() { 4120 return this.status == null ? null : this.status.getValue(); 4121 } 4122 4123 /** 4124 * @param value The status of this terminology capabilities. Enables tracking 4125 * the life-cycle of the content. 4126 */ 4127 public TerminologyCapabilities setStatus(PublicationStatus value) { 4128 if (this.status == null) 4129 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 4130 this.status.setValue(value); 4131 return this; 4132 } 4133 4134 /** 4135 * @return {@link #experimental} (A Boolean value to indicate that this 4136 * terminology capabilities is authored for testing purposes (or 4137 * education/evaluation/marketing) and is not intended to be used for 4138 * genuine usage.). This is the underlying object with id, value and 4139 * extensions. The accessor "getExperimental" gives direct access to the 4140 * value 4141 */ 4142 public BooleanType getExperimentalElement() { 4143 if (this.experimental == null) 4144 if (Configuration.errorOnAutoCreate()) 4145 throw new Error("Attempt to auto-create TerminologyCapabilities.experimental"); 4146 else if (Configuration.doAutoCreate()) 4147 this.experimental = new BooleanType(); // bb 4148 return this.experimental; 4149 } 4150 4151 public boolean hasExperimentalElement() { 4152 return this.experimental != null && !this.experimental.isEmpty(); 4153 } 4154 4155 public boolean hasExperimental() { 4156 return this.experimental != null && !this.experimental.isEmpty(); 4157 } 4158 4159 /** 4160 * @param value {@link #experimental} (A Boolean value to indicate that this 4161 * terminology capabilities is authored for testing purposes (or 4162 * education/evaluation/marketing) and is not intended to be used 4163 * for genuine usage.). This is the underlying object with id, 4164 * value and extensions. The accessor "getExperimental" gives 4165 * direct access to the value 4166 */ 4167 public TerminologyCapabilities setExperimentalElement(BooleanType value) { 4168 this.experimental = value; 4169 return this; 4170 } 4171 4172 /** 4173 * @return A Boolean value to indicate that this terminology capabilities is 4174 * authored for testing purposes (or education/evaluation/marketing) and 4175 * is not intended to be used for genuine usage. 4176 */ 4177 public boolean getExperimental() { 4178 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 4179 } 4180 4181 /** 4182 * @param value A Boolean value to indicate that this terminology capabilities 4183 * is authored for testing purposes (or 4184 * education/evaluation/marketing) and is not intended to be used 4185 * for genuine usage. 4186 */ 4187 public TerminologyCapabilities setExperimental(boolean value) { 4188 if (this.experimental == null) 4189 this.experimental = new BooleanType(); 4190 this.experimental.setValue(value); 4191 return this; 4192 } 4193 4194 /** 4195 * @return {@link #date} (The date (and optionally time) when the terminology 4196 * capabilities was published. The date must change when the business 4197 * version changes and it must change if the status code changes. In 4198 * addition, it should change when the substantive content of the 4199 * terminology capabilities changes.). This is the underlying object 4200 * with id, value and extensions. The accessor "getDate" gives direct 4201 * access to the value 4202 */ 4203 public DateTimeType getDateElement() { 4204 if (this.date == null) 4205 if (Configuration.errorOnAutoCreate()) 4206 throw new Error("Attempt to auto-create TerminologyCapabilities.date"); 4207 else if (Configuration.doAutoCreate()) 4208 this.date = new DateTimeType(); // bb 4209 return this.date; 4210 } 4211 4212 public boolean hasDateElement() { 4213 return this.date != null && !this.date.isEmpty(); 4214 } 4215 4216 public boolean hasDate() { 4217 return this.date != null && !this.date.isEmpty(); 4218 } 4219 4220 /** 4221 * @param value {@link #date} (The date (and optionally time) when the 4222 * terminology capabilities was published. The date must change 4223 * when the business version changes and it must change if the 4224 * status code changes. In addition, it should change when the 4225 * substantive content of the terminology capabilities changes.). 4226 * This is the underlying object with id, value and extensions. The 4227 * accessor "getDate" gives direct access to the value 4228 */ 4229 public TerminologyCapabilities setDateElement(DateTimeType value) { 4230 this.date = value; 4231 return this; 4232 } 4233 4234 /** 4235 * @return The date (and optionally time) when the terminology capabilities was 4236 * published. The date must change when the business version changes and 4237 * it must change if the status code changes. In addition, it should 4238 * change when the substantive content of the terminology capabilities 4239 * changes. 4240 */ 4241 public Date getDate() { 4242 return this.date == null ? null : this.date.getValue(); 4243 } 4244 4245 /** 4246 * @param value The date (and optionally time) when the terminology capabilities 4247 * was published. The date must change when the business version 4248 * changes and it must change if the status code changes. In 4249 * addition, it should change when the substantive content of the 4250 * terminology capabilities changes. 4251 */ 4252 public TerminologyCapabilities setDate(Date value) { 4253 if (this.date == null) 4254 this.date = new DateTimeType(); 4255 this.date.setValue(value); 4256 return this; 4257 } 4258 4259 /** 4260 * @return {@link #publisher} (The name of the organization or individual that 4261 * published the terminology capabilities.). This is the underlying 4262 * object with id, value and extensions. The accessor "getPublisher" 4263 * gives direct access to the value 4264 */ 4265 public StringType getPublisherElement() { 4266 if (this.publisher == null) 4267 if (Configuration.errorOnAutoCreate()) 4268 throw new Error("Attempt to auto-create TerminologyCapabilities.publisher"); 4269 else if (Configuration.doAutoCreate()) 4270 this.publisher = new StringType(); // bb 4271 return this.publisher; 4272 } 4273 4274 public boolean hasPublisherElement() { 4275 return this.publisher != null && !this.publisher.isEmpty(); 4276 } 4277 4278 public boolean hasPublisher() { 4279 return this.publisher != null && !this.publisher.isEmpty(); 4280 } 4281 4282 /** 4283 * @param value {@link #publisher} (The name of the organization or individual 4284 * that published the terminology capabilities.). This is the 4285 * underlying object with id, value and extensions. The accessor 4286 * "getPublisher" gives direct access to the value 4287 */ 4288 public TerminologyCapabilities setPublisherElement(StringType value) { 4289 this.publisher = value; 4290 return this; 4291 } 4292 4293 /** 4294 * @return The name of the organization or individual that published the 4295 * terminology capabilities. 4296 */ 4297 public String getPublisher() { 4298 return this.publisher == null ? null : this.publisher.getValue(); 4299 } 4300 4301 /** 4302 * @param value The name of the organization or individual that published the 4303 * terminology capabilities. 4304 */ 4305 public TerminologyCapabilities setPublisher(String value) { 4306 if (Utilities.noString(value)) 4307 this.publisher = null; 4308 else { 4309 if (this.publisher == null) 4310 this.publisher = new StringType(); 4311 this.publisher.setValue(value); 4312 } 4313 return this; 4314 } 4315 4316 /** 4317 * @return {@link #contact} (Contact details to assist a user in finding and 4318 * communicating with the publisher.) 4319 */ 4320 public List<ContactDetail> getContact() { 4321 if (this.contact == null) 4322 this.contact = new ArrayList<ContactDetail>(); 4323 return this.contact; 4324 } 4325 4326 /** 4327 * @return Returns a reference to <code>this</code> for easy method chaining 4328 */ 4329 public TerminologyCapabilities setContact(List<ContactDetail> theContact) { 4330 this.contact = theContact; 4331 return this; 4332 } 4333 4334 public boolean hasContact() { 4335 if (this.contact == null) 4336 return false; 4337 for (ContactDetail item : this.contact) 4338 if (!item.isEmpty()) 4339 return true; 4340 return false; 4341 } 4342 4343 public ContactDetail addContact() { // 3 4344 ContactDetail t = new ContactDetail(); 4345 if (this.contact == null) 4346 this.contact = new ArrayList<ContactDetail>(); 4347 this.contact.add(t); 4348 return t; 4349 } 4350 4351 public TerminologyCapabilities addContact(ContactDetail t) { // 3 4352 if (t == null) 4353 return this; 4354 if (this.contact == null) 4355 this.contact = new ArrayList<ContactDetail>(); 4356 this.contact.add(t); 4357 return this; 4358 } 4359 4360 /** 4361 * @return The first repetition of repeating field {@link #contact}, creating it 4362 * if it does not already exist 4363 */ 4364 public ContactDetail getContactFirstRep() { 4365 if (getContact().isEmpty()) { 4366 addContact(); 4367 } 4368 return getContact().get(0); 4369 } 4370 4371 /** 4372 * @return {@link #description} (A free text natural language description of the 4373 * terminology capabilities from a consumer's perspective. Typically, 4374 * this is used when the capability statement describes a desired rather 4375 * than an actual solution, for example as a formal expression of 4376 * requirements as part of an RFP.). This is the underlying object with 4377 * id, value and extensions. The accessor "getDescription" gives direct 4378 * access to the value 4379 */ 4380 public MarkdownType getDescriptionElement() { 4381 if (this.description == null) 4382 if (Configuration.errorOnAutoCreate()) 4383 throw new Error("Attempt to auto-create TerminologyCapabilities.description"); 4384 else if (Configuration.doAutoCreate()) 4385 this.description = new MarkdownType(); // bb 4386 return this.description; 4387 } 4388 4389 public boolean hasDescriptionElement() { 4390 return this.description != null && !this.description.isEmpty(); 4391 } 4392 4393 public boolean hasDescription() { 4394 return this.description != null && !this.description.isEmpty(); 4395 } 4396 4397 /** 4398 * @param value {@link #description} (A free text natural language description 4399 * of the terminology capabilities from a consumer's perspective. 4400 * Typically, this is used when the capability statement describes 4401 * a desired rather than an actual solution, for example as a 4402 * formal expression of requirements as part of an RFP.). This is 4403 * the underlying object with id, value and extensions. The 4404 * accessor "getDescription" gives direct access to the value 4405 */ 4406 public TerminologyCapabilities setDescriptionElement(MarkdownType value) { 4407 this.description = value; 4408 return this; 4409 } 4410 4411 /** 4412 * @return A free text natural language description of the terminology 4413 * capabilities from a consumer's perspective. Typically, this is used 4414 * when the capability statement describes a desired rather than an 4415 * actual solution, for example as a formal expression of requirements 4416 * as part of an RFP. 4417 */ 4418 public String getDescription() { 4419 return this.description == null ? null : this.description.getValue(); 4420 } 4421 4422 /** 4423 * @param value A free text natural language description of the terminology 4424 * capabilities from a consumer's perspective. Typically, this is 4425 * used when the capability statement describes a desired rather 4426 * than an actual solution, for example as a formal expression of 4427 * requirements as part of an RFP. 4428 */ 4429 public TerminologyCapabilities setDescription(String value) { 4430 if (value == null) 4431 this.description = null; 4432 else { 4433 if (this.description == null) 4434 this.description = new MarkdownType(); 4435 this.description.setValue(value); 4436 } 4437 return this; 4438 } 4439 4440 /** 4441 * @return {@link #useContext} (The content was developed with a focus and 4442 * intent of supporting the contexts that are listed. These contexts may 4443 * be general categories (gender, age, ...) or may be references to 4444 * specific programs (insurance plans, studies, ...) and may be used to 4445 * assist with indexing and searching for appropriate terminology 4446 * capabilities instances.) 4447 */ 4448 public List<UsageContext> getUseContext() { 4449 if (this.useContext == null) 4450 this.useContext = new ArrayList<UsageContext>(); 4451 return this.useContext; 4452 } 4453 4454 /** 4455 * @return Returns a reference to <code>this</code> for easy method chaining 4456 */ 4457 public TerminologyCapabilities setUseContext(List<UsageContext> theUseContext) { 4458 this.useContext = theUseContext; 4459 return this; 4460 } 4461 4462 public boolean hasUseContext() { 4463 if (this.useContext == null) 4464 return false; 4465 for (UsageContext item : this.useContext) 4466 if (!item.isEmpty()) 4467 return true; 4468 return false; 4469 } 4470 4471 public UsageContext addUseContext() { // 3 4472 UsageContext t = new UsageContext(); 4473 if (this.useContext == null) 4474 this.useContext = new ArrayList<UsageContext>(); 4475 this.useContext.add(t); 4476 return t; 4477 } 4478 4479 public TerminologyCapabilities addUseContext(UsageContext t) { // 3 4480 if (t == null) 4481 return this; 4482 if (this.useContext == null) 4483 this.useContext = new ArrayList<UsageContext>(); 4484 this.useContext.add(t); 4485 return this; 4486 } 4487 4488 /** 4489 * @return The first repetition of repeating field {@link #useContext}, creating 4490 * it if it does not already exist 4491 */ 4492 public UsageContext getUseContextFirstRep() { 4493 if (getUseContext().isEmpty()) { 4494 addUseContext(); 4495 } 4496 return getUseContext().get(0); 4497 } 4498 4499 /** 4500 * @return {@link #jurisdiction} (A legal or geographic region in which the 4501 * terminology capabilities is intended to be used.) 4502 */ 4503 public List<CodeableConcept> getJurisdiction() { 4504 if (this.jurisdiction == null) 4505 this.jurisdiction = new ArrayList<CodeableConcept>(); 4506 return this.jurisdiction; 4507 } 4508 4509 /** 4510 * @return Returns a reference to <code>this</code> for easy method chaining 4511 */ 4512 public TerminologyCapabilities setJurisdiction(List<CodeableConcept> theJurisdiction) { 4513 this.jurisdiction = theJurisdiction; 4514 return this; 4515 } 4516 4517 public boolean hasJurisdiction() { 4518 if (this.jurisdiction == null) 4519 return false; 4520 for (CodeableConcept item : this.jurisdiction) 4521 if (!item.isEmpty()) 4522 return true; 4523 return false; 4524 } 4525 4526 public CodeableConcept addJurisdiction() { // 3 4527 CodeableConcept t = new CodeableConcept(); 4528 if (this.jurisdiction == null) 4529 this.jurisdiction = new ArrayList<CodeableConcept>(); 4530 this.jurisdiction.add(t); 4531 return t; 4532 } 4533 4534 public TerminologyCapabilities addJurisdiction(CodeableConcept t) { // 3 4535 if (t == null) 4536 return this; 4537 if (this.jurisdiction == null) 4538 this.jurisdiction = new ArrayList<CodeableConcept>(); 4539 this.jurisdiction.add(t); 4540 return this; 4541 } 4542 4543 /** 4544 * @return The first repetition of repeating field {@link #jurisdiction}, 4545 * creating it if it does not already exist 4546 */ 4547 public CodeableConcept getJurisdictionFirstRep() { 4548 if (getJurisdiction().isEmpty()) { 4549 addJurisdiction(); 4550 } 4551 return getJurisdiction().get(0); 4552 } 4553 4554 /** 4555 * @return {@link #purpose} (Explanation of why this terminology capabilities is 4556 * needed and why it has been designed as it has.). This is the 4557 * underlying object with id, value and extensions. The accessor 4558 * "getPurpose" gives direct access to the value 4559 */ 4560 public MarkdownType getPurposeElement() { 4561 if (this.purpose == null) 4562 if (Configuration.errorOnAutoCreate()) 4563 throw new Error("Attempt to auto-create TerminologyCapabilities.purpose"); 4564 else if (Configuration.doAutoCreate()) 4565 this.purpose = new MarkdownType(); // bb 4566 return this.purpose; 4567 } 4568 4569 public boolean hasPurposeElement() { 4570 return this.purpose != null && !this.purpose.isEmpty(); 4571 } 4572 4573 public boolean hasPurpose() { 4574 return this.purpose != null && !this.purpose.isEmpty(); 4575 } 4576 4577 /** 4578 * @param value {@link #purpose} (Explanation of why this terminology 4579 * capabilities is needed and why it has been designed as it has.). 4580 * This is the underlying object with id, value and extensions. The 4581 * accessor "getPurpose" gives direct access to the value 4582 */ 4583 public TerminologyCapabilities setPurposeElement(MarkdownType value) { 4584 this.purpose = value; 4585 return this; 4586 } 4587 4588 /** 4589 * @return Explanation of why this terminology capabilities is needed and why it 4590 * has been designed as it has. 4591 */ 4592 public String getPurpose() { 4593 return this.purpose == null ? null : this.purpose.getValue(); 4594 } 4595 4596 /** 4597 * @param value Explanation of why this terminology capabilities is needed and 4598 * why it has been designed as it has. 4599 */ 4600 public TerminologyCapabilities setPurpose(String value) { 4601 if (value == null) 4602 this.purpose = null; 4603 else { 4604 if (this.purpose == null) 4605 this.purpose = new MarkdownType(); 4606 this.purpose.setValue(value); 4607 } 4608 return this; 4609 } 4610 4611 /** 4612 * @return {@link #copyright} (A copyright statement relating to the terminology 4613 * capabilities and/or its contents. Copyright statements are generally 4614 * legal restrictions on the use and publishing of the terminology 4615 * capabilities.). This is the underlying object with id, value and 4616 * extensions. The accessor "getCopyright" gives direct access to the 4617 * value 4618 */ 4619 public MarkdownType getCopyrightElement() { 4620 if (this.copyright == null) 4621 if (Configuration.errorOnAutoCreate()) 4622 throw new Error("Attempt to auto-create TerminologyCapabilities.copyright"); 4623 else if (Configuration.doAutoCreate()) 4624 this.copyright = new MarkdownType(); // bb 4625 return this.copyright; 4626 } 4627 4628 public boolean hasCopyrightElement() { 4629 return this.copyright != null && !this.copyright.isEmpty(); 4630 } 4631 4632 public boolean hasCopyright() { 4633 return this.copyright != null && !this.copyright.isEmpty(); 4634 } 4635 4636 /** 4637 * @param value {@link #copyright} (A copyright statement relating to the 4638 * terminology capabilities and/or its contents. Copyright 4639 * statements are generally legal restrictions on the use and 4640 * publishing of the terminology capabilities.). This is the 4641 * underlying object with id, value and extensions. The accessor 4642 * "getCopyright" gives direct access to the value 4643 */ 4644 public TerminologyCapabilities setCopyrightElement(MarkdownType value) { 4645 this.copyright = value; 4646 return this; 4647 } 4648 4649 /** 4650 * @return A copyright statement relating to the terminology capabilities and/or 4651 * its contents. Copyright statements are generally legal restrictions 4652 * on the use and publishing of the terminology capabilities. 4653 */ 4654 public String getCopyright() { 4655 return this.copyright == null ? null : this.copyright.getValue(); 4656 } 4657 4658 /** 4659 * @param value A copyright statement relating to the terminology capabilities 4660 * and/or its contents. Copyright statements are generally legal 4661 * restrictions on the use and publishing of the terminology 4662 * capabilities. 4663 */ 4664 public TerminologyCapabilities setCopyright(String value) { 4665 if (value == null) 4666 this.copyright = null; 4667 else { 4668 if (this.copyright == null) 4669 this.copyright = new MarkdownType(); 4670 this.copyright.setValue(value); 4671 } 4672 return this; 4673 } 4674 4675 /** 4676 * @return {@link #kind} (The way that this statement is intended to be used, to 4677 * describe an actual running instance of software, a particular product 4678 * (kind, not instance of software) or a class of implementation (e.g. a 4679 * desired purchase).). This is the underlying object with id, value and 4680 * extensions. The accessor "getKind" gives direct access to the value 4681 */ 4682 public Enumeration<CapabilityStatementKind> getKindElement() { 4683 if (this.kind == null) 4684 if (Configuration.errorOnAutoCreate()) 4685 throw new Error("Attempt to auto-create TerminologyCapabilities.kind"); 4686 else if (Configuration.doAutoCreate()) 4687 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); // bb 4688 return this.kind; 4689 } 4690 4691 public boolean hasKindElement() { 4692 return this.kind != null && !this.kind.isEmpty(); 4693 } 4694 4695 public boolean hasKind() { 4696 return this.kind != null && !this.kind.isEmpty(); 4697 } 4698 4699 /** 4700 * @param value {@link #kind} (The way that this statement is intended to be 4701 * used, to describe an actual running instance of software, a 4702 * particular product (kind, not instance of software) or a class 4703 * of implementation (e.g. a desired purchase).). This is the 4704 * underlying object with id, value and extensions. The accessor 4705 * "getKind" gives direct access to the value 4706 */ 4707 public TerminologyCapabilities setKindElement(Enumeration<CapabilityStatementKind> value) { 4708 this.kind = value; 4709 return this; 4710 } 4711 4712 /** 4713 * @return The way that this statement is intended to be used, to describe an 4714 * actual running instance of software, a particular product (kind, not 4715 * instance of software) or a class of implementation (e.g. a desired 4716 * purchase). 4717 */ 4718 public CapabilityStatementKind getKind() { 4719 return this.kind == null ? null : this.kind.getValue(); 4720 } 4721 4722 /** 4723 * @param value The way that this statement is intended to be used, to describe 4724 * an actual running instance of software, a particular product 4725 * (kind, not instance of software) or a class of implementation 4726 * (e.g. a desired purchase). 4727 */ 4728 public TerminologyCapabilities setKind(CapabilityStatementKind value) { 4729 if (this.kind == null) 4730 this.kind = new Enumeration<CapabilityStatementKind>(new CapabilityStatementKindEnumFactory()); 4731 this.kind.setValue(value); 4732 return this; 4733 } 4734 4735 /** 4736 * @return {@link #software} (Software that is covered by this terminology 4737 * capability statement. It is used when the statement describes the 4738 * capabilities of a particular software version, independent of an 4739 * installation.) 4740 */ 4741 public TerminologyCapabilitiesSoftwareComponent getSoftware() { 4742 if (this.software == null) 4743 if (Configuration.errorOnAutoCreate()) 4744 throw new Error("Attempt to auto-create TerminologyCapabilities.software"); 4745 else if (Configuration.doAutoCreate()) 4746 this.software = new TerminologyCapabilitiesSoftwareComponent(); // cc 4747 return this.software; 4748 } 4749 4750 public boolean hasSoftware() { 4751 return this.software != null && !this.software.isEmpty(); 4752 } 4753 4754 /** 4755 * @param value {@link #software} (Software that is covered by this terminology 4756 * capability statement. It is used when the statement describes 4757 * the capabilities of a particular software version, independent 4758 * of an installation.) 4759 */ 4760 public TerminologyCapabilities setSoftware(TerminologyCapabilitiesSoftwareComponent value) { 4761 this.software = value; 4762 return this; 4763 } 4764 4765 /** 4766 * @return {@link #implementation} (Identifies a specific implementation 4767 * instance that is described by the terminology capability statement - 4768 * i.e. a particular installation, rather than the capabilities of a 4769 * software program.) 4770 */ 4771 public TerminologyCapabilitiesImplementationComponent getImplementation() { 4772 if (this.implementation == null) 4773 if (Configuration.errorOnAutoCreate()) 4774 throw new Error("Attempt to auto-create TerminologyCapabilities.implementation"); 4775 else if (Configuration.doAutoCreate()) 4776 this.implementation = new TerminologyCapabilitiesImplementationComponent(); // cc 4777 return this.implementation; 4778 } 4779 4780 public boolean hasImplementation() { 4781 return this.implementation != null && !this.implementation.isEmpty(); 4782 } 4783 4784 /** 4785 * @param value {@link #implementation} (Identifies a specific implementation 4786 * instance that is described by the terminology capability 4787 * statement - i.e. a particular installation, rather than the 4788 * capabilities of a software program.) 4789 */ 4790 public TerminologyCapabilities setImplementation(TerminologyCapabilitiesImplementationComponent value) { 4791 this.implementation = value; 4792 return this; 4793 } 4794 4795 /** 4796 * @return {@link #lockedDate} (Whether the server supports lockedDate.). This 4797 * is the underlying object with id, value and extensions. The accessor 4798 * "getLockedDate" gives direct access to the value 4799 */ 4800 public BooleanType getLockedDateElement() { 4801 if (this.lockedDate == null) 4802 if (Configuration.errorOnAutoCreate()) 4803 throw new Error("Attempt to auto-create TerminologyCapabilities.lockedDate"); 4804 else if (Configuration.doAutoCreate()) 4805 this.lockedDate = new BooleanType(); // bb 4806 return this.lockedDate; 4807 } 4808 4809 public boolean hasLockedDateElement() { 4810 return this.lockedDate != null && !this.lockedDate.isEmpty(); 4811 } 4812 4813 public boolean hasLockedDate() { 4814 return this.lockedDate != null && !this.lockedDate.isEmpty(); 4815 } 4816 4817 /** 4818 * @param value {@link #lockedDate} (Whether the server supports lockedDate.). 4819 * This is the underlying object with id, value and extensions. The 4820 * accessor "getLockedDate" gives direct access to the value 4821 */ 4822 public TerminologyCapabilities setLockedDateElement(BooleanType value) { 4823 this.lockedDate = value; 4824 return this; 4825 } 4826 4827 /** 4828 * @return Whether the server supports lockedDate. 4829 */ 4830 public boolean getLockedDate() { 4831 return this.lockedDate == null || this.lockedDate.isEmpty() ? false : this.lockedDate.getValue(); 4832 } 4833 4834 /** 4835 * @param value Whether the server supports lockedDate. 4836 */ 4837 public TerminologyCapabilities setLockedDate(boolean value) { 4838 if (this.lockedDate == null) 4839 this.lockedDate = new BooleanType(); 4840 this.lockedDate.setValue(value); 4841 return this; 4842 } 4843 4844 /** 4845 * @return {@link #codeSystem} (Identifies a code system that is supported by 4846 * the server. If there is a no code system URL, then this declares the 4847 * general assumptions a client can make about support for any 4848 * CodeSystem resource.) 4849 */ 4850 public List<TerminologyCapabilitiesCodeSystemComponent> getCodeSystem() { 4851 if (this.codeSystem == null) 4852 this.codeSystem = new ArrayList<TerminologyCapabilitiesCodeSystemComponent>(); 4853 return this.codeSystem; 4854 } 4855 4856 /** 4857 * @return Returns a reference to <code>this</code> for easy method chaining 4858 */ 4859 public TerminologyCapabilities setCodeSystem(List<TerminologyCapabilitiesCodeSystemComponent> theCodeSystem) { 4860 this.codeSystem = theCodeSystem; 4861 return this; 4862 } 4863 4864 public boolean hasCodeSystem() { 4865 if (this.codeSystem == null) 4866 return false; 4867 for (TerminologyCapabilitiesCodeSystemComponent item : this.codeSystem) 4868 if (!item.isEmpty()) 4869 return true; 4870 return false; 4871 } 4872 4873 public TerminologyCapabilitiesCodeSystemComponent addCodeSystem() { // 3 4874 TerminologyCapabilitiesCodeSystemComponent t = new TerminologyCapabilitiesCodeSystemComponent(); 4875 if (this.codeSystem == null) 4876 this.codeSystem = new ArrayList<TerminologyCapabilitiesCodeSystemComponent>(); 4877 this.codeSystem.add(t); 4878 return t; 4879 } 4880 4881 public TerminologyCapabilities addCodeSystem(TerminologyCapabilitiesCodeSystemComponent t) { // 3 4882 if (t == null) 4883 return this; 4884 if (this.codeSystem == null) 4885 this.codeSystem = new ArrayList<TerminologyCapabilitiesCodeSystemComponent>(); 4886 this.codeSystem.add(t); 4887 return this; 4888 } 4889 4890 /** 4891 * @return The first repetition of repeating field {@link #codeSystem}, creating 4892 * it if it does not already exist 4893 */ 4894 public TerminologyCapabilitiesCodeSystemComponent getCodeSystemFirstRep() { 4895 if (getCodeSystem().isEmpty()) { 4896 addCodeSystem(); 4897 } 4898 return getCodeSystem().get(0); 4899 } 4900 4901 /** 4902 * @return {@link #expansion} (Information about the 4903 * [ValueSet/$expand](valueset-operation-expand.html) operation.) 4904 */ 4905 public TerminologyCapabilitiesExpansionComponent getExpansion() { 4906 if (this.expansion == null) 4907 if (Configuration.errorOnAutoCreate()) 4908 throw new Error("Attempt to auto-create TerminologyCapabilities.expansion"); 4909 else if (Configuration.doAutoCreate()) 4910 this.expansion = new TerminologyCapabilitiesExpansionComponent(); // cc 4911 return this.expansion; 4912 } 4913 4914 public boolean hasExpansion() { 4915 return this.expansion != null && !this.expansion.isEmpty(); 4916 } 4917 4918 /** 4919 * @param value {@link #expansion} (Information about the 4920 * [ValueSet/$expand](valueset-operation-expand.html) operation.) 4921 */ 4922 public TerminologyCapabilities setExpansion(TerminologyCapabilitiesExpansionComponent value) { 4923 this.expansion = value; 4924 return this; 4925 } 4926 4927 /** 4928 * @return {@link #codeSearch} (The degree to which the server supports the code 4929 * search parameter on ValueSet, if it is supported.). This is the 4930 * underlying object with id, value and extensions. The accessor 4931 * "getCodeSearch" gives direct access to the value 4932 */ 4933 public Enumeration<CodeSearchSupport> getCodeSearchElement() { 4934 if (this.codeSearch == null) 4935 if (Configuration.errorOnAutoCreate()) 4936 throw new Error("Attempt to auto-create TerminologyCapabilities.codeSearch"); 4937 else if (Configuration.doAutoCreate()) 4938 this.codeSearch = new Enumeration<CodeSearchSupport>(new CodeSearchSupportEnumFactory()); // bb 4939 return this.codeSearch; 4940 } 4941 4942 public boolean hasCodeSearchElement() { 4943 return this.codeSearch != null && !this.codeSearch.isEmpty(); 4944 } 4945 4946 public boolean hasCodeSearch() { 4947 return this.codeSearch != null && !this.codeSearch.isEmpty(); 4948 } 4949 4950 /** 4951 * @param value {@link #codeSearch} (The degree to which the server supports the 4952 * code search parameter on ValueSet, if it is supported.). This is 4953 * the underlying object with id, value and extensions. The 4954 * accessor "getCodeSearch" gives direct access to the value 4955 */ 4956 public TerminologyCapabilities setCodeSearchElement(Enumeration<CodeSearchSupport> value) { 4957 this.codeSearch = value; 4958 return this; 4959 } 4960 4961 /** 4962 * @return The degree to which the server supports the code search parameter on 4963 * ValueSet, if it is supported. 4964 */ 4965 public CodeSearchSupport getCodeSearch() { 4966 return this.codeSearch == null ? null : this.codeSearch.getValue(); 4967 } 4968 4969 /** 4970 * @param value The degree to which the server supports the code search 4971 * parameter on ValueSet, if it is supported. 4972 */ 4973 public TerminologyCapabilities setCodeSearch(CodeSearchSupport value) { 4974 if (value == null) 4975 this.codeSearch = null; 4976 else { 4977 if (this.codeSearch == null) 4978 this.codeSearch = new Enumeration<CodeSearchSupport>(new CodeSearchSupportEnumFactory()); 4979 this.codeSearch.setValue(value); 4980 } 4981 return this; 4982 } 4983 4984 /** 4985 * @return {@link #validateCode} (Information about the 4986 * [ValueSet/$validate-code](valueset-operation-validate-code.html) 4987 * operation.) 4988 */ 4989 public TerminologyCapabilitiesValidateCodeComponent getValidateCode() { 4990 if (this.validateCode == null) 4991 if (Configuration.errorOnAutoCreate()) 4992 throw new Error("Attempt to auto-create TerminologyCapabilities.validateCode"); 4993 else if (Configuration.doAutoCreate()) 4994 this.validateCode = new TerminologyCapabilitiesValidateCodeComponent(); // cc 4995 return this.validateCode; 4996 } 4997 4998 public boolean hasValidateCode() { 4999 return this.validateCode != null && !this.validateCode.isEmpty(); 5000 } 5001 5002 /** 5003 * @param value {@link #validateCode} (Information about the 5004 * [ValueSet/$validate-code](valueset-operation-validate-code.html) 5005 * operation.) 5006 */ 5007 public TerminologyCapabilities setValidateCode(TerminologyCapabilitiesValidateCodeComponent value) { 5008 this.validateCode = value; 5009 return this; 5010 } 5011 5012 /** 5013 * @return {@link #translation} (Information about the 5014 * [ConceptMap/$translate](conceptmap-operation-translate.html) 5015 * operation.) 5016 */ 5017 public TerminologyCapabilitiesTranslationComponent getTranslation() { 5018 if (this.translation == null) 5019 if (Configuration.errorOnAutoCreate()) 5020 throw new Error("Attempt to auto-create TerminologyCapabilities.translation"); 5021 else if (Configuration.doAutoCreate()) 5022 this.translation = new TerminologyCapabilitiesTranslationComponent(); // cc 5023 return this.translation; 5024 } 5025 5026 public boolean hasTranslation() { 5027 return this.translation != null && !this.translation.isEmpty(); 5028 } 5029 5030 /** 5031 * @param value {@link #translation} (Information about the 5032 * [ConceptMap/$translate](conceptmap-operation-translate.html) 5033 * operation.) 5034 */ 5035 public TerminologyCapabilities setTranslation(TerminologyCapabilitiesTranslationComponent value) { 5036 this.translation = value; 5037 return this; 5038 } 5039 5040 /** 5041 * @return {@link #closure} (Whether the $closure operation is supported.) 5042 */ 5043 public TerminologyCapabilitiesClosureComponent getClosure() { 5044 if (this.closure == null) 5045 if (Configuration.errorOnAutoCreate()) 5046 throw new Error("Attempt to auto-create TerminologyCapabilities.closure"); 5047 else if (Configuration.doAutoCreate()) 5048 this.closure = new TerminologyCapabilitiesClosureComponent(); // cc 5049 return this.closure; 5050 } 5051 5052 public boolean hasClosure() { 5053 return this.closure != null && !this.closure.isEmpty(); 5054 } 5055 5056 /** 5057 * @param value {@link #closure} (Whether the $closure operation is supported.) 5058 */ 5059 public TerminologyCapabilities setClosure(TerminologyCapabilitiesClosureComponent value) { 5060 this.closure = value; 5061 return this; 5062 } 5063 5064 protected void listChildren(List<Property> children) { 5065 super.listChildren(children); 5066 children.add(new Property("url", "uri", 5067 "An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers.", 5068 0, 1, url)); 5069 children.add(new Property("version", "string", 5070 "The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 5071 0, 1, version)); 5072 children.add(new Property("name", "string", 5073 "A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 5074 0, 1, name)); 5075 children.add(new Property("title", "string", 5076 "A short, descriptive, user-friendly title for the terminology capabilities.", 0, 1, title)); 5077 children.add(new Property("status", "code", 5078 "The status of this terminology capabilities. Enables tracking the life-cycle of the content.", 0, 1, status)); 5079 children.add(new Property("experimental", "boolean", 5080 "A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 5081 0, 1, experimental)); 5082 children.add(new Property("date", "dateTime", 5083 "The date (and optionally time) when the terminology capabilities was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes.", 5084 0, 1, date)); 5085 children.add(new Property("publisher", "string", 5086 "The name of the organization or individual that published the terminology capabilities.", 0, 1, publisher)); 5087 children.add(new Property("contact", "ContactDetail", 5088 "Contact details to assist a user in finding and communicating with the publisher.", 0, 5089 java.lang.Integer.MAX_VALUE, contact)); 5090 children.add(new Property("description", "markdown", 5091 "A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 5092 0, 1, description)); 5093 children.add(new Property("useContext", "UsageContext", 5094 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate terminology capabilities instances.", 5095 0, java.lang.Integer.MAX_VALUE, useContext)); 5096 children.add(new Property("jurisdiction", "CodeableConcept", 5097 "A legal or geographic region in which the terminology capabilities is intended to be used.", 0, 5098 java.lang.Integer.MAX_VALUE, jurisdiction)); 5099 children.add(new Property("purpose", "markdown", 5100 "Explanation of why this terminology capabilities is needed and why it has been designed as it has.", 0, 1, 5101 purpose)); 5102 children.add(new Property("copyright", "markdown", 5103 "A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities.", 5104 0, 1, copyright)); 5105 children.add(new Property("kind", "code", 5106 "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 5107 0, 1, kind)); 5108 children.add(new Property("software", "", 5109 "Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation.", 5110 0, 1, software)); 5111 children.add(new Property("implementation", "", 5112 "Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 5113 0, 1, implementation)); 5114 children.add(new Property("lockedDate", "boolean", "Whether the server supports lockedDate.", 0, 1, lockedDate)); 5115 children.add(new Property("codeSystem", "", 5116 "Identifies a code system that is supported by the server. If there is a no code system URL, then this declares the general assumptions a client can make about support for any CodeSystem resource.", 5117 0, java.lang.Integer.MAX_VALUE, codeSystem)); 5118 children.add(new Property("expansion", "", 5119 "Information about the [ValueSet/$expand](valueset-operation-expand.html) operation.", 0, 1, expansion)); 5120 children.add(new Property("codeSearch", "code", 5121 "The degree to which the server supports the code search parameter on ValueSet, if it is supported.", 0, 1, 5122 codeSearch)); 5123 children.add(new Property("validateCode", "", 5124 "Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation.", 0, 1, 5125 validateCode)); 5126 children.add(new Property("translation", "", 5127 "Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation.", 0, 1, 5128 translation)); 5129 children.add(new Property("closure", "", "Whether the $closure operation is supported.", 0, 1, closure)); 5130 } 5131 5132 @Override 5133 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5134 switch (_hash) { 5135 case 116079: 5136 /* url */ return new Property("url", "uri", 5137 "An absolute URI that is used to identify this terminology capabilities when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this terminology capabilities is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the terminology capabilities is stored on different servers.", 5138 0, 1, url); 5139 case 351608024: 5140 /* version */ return new Property("version", "string", 5141 "The identifier that is used to identify this version of the terminology capabilities when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the terminology capabilities author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 5142 0, 1, version); 5143 case 3373707: 5144 /* name */ return new Property("name", "string", 5145 "A natural language name identifying the terminology capabilities. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 5146 0, 1, name); 5147 case 110371416: 5148 /* title */ return new Property("title", "string", 5149 "A short, descriptive, user-friendly title for the terminology capabilities.", 0, 1, title); 5150 case -892481550: 5151 /* status */ return new Property("status", "code", 5152 "The status of this terminology capabilities. Enables tracking the life-cycle of the content.", 0, 1, status); 5153 case -404562712: 5154 /* experimental */ return new Property("experimental", "boolean", 5155 "A Boolean value to indicate that this terminology capabilities is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 5156 0, 1, experimental); 5157 case 3076014: 5158 /* date */ return new Property("date", "dateTime", 5159 "The date (and optionally time) when the terminology capabilities was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the terminology capabilities changes.", 5160 0, 1, date); 5161 case 1447404028: 5162 /* publisher */ return new Property("publisher", "string", 5163 "The name of the organization or individual that published the terminology capabilities.", 0, 1, publisher); 5164 case 951526432: 5165 /* contact */ return new Property("contact", "ContactDetail", 5166 "Contact details to assist a user in finding and communicating with the publisher.", 0, 5167 java.lang.Integer.MAX_VALUE, contact); 5168 case -1724546052: 5169 /* description */ return new Property("description", "markdown", 5170 "A free text natural language description of the terminology capabilities from a consumer's perspective. Typically, this is used when the capability statement describes a desired rather than an actual solution, for example as a formal expression of requirements as part of an RFP.", 5171 0, 1, description); 5172 case -669707736: 5173 /* useContext */ return new Property("useContext", "UsageContext", 5174 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate terminology capabilities instances.", 5175 0, java.lang.Integer.MAX_VALUE, useContext); 5176 case -507075711: 5177 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 5178 "A legal or geographic region in which the terminology capabilities is intended to be used.", 0, 5179 java.lang.Integer.MAX_VALUE, jurisdiction); 5180 case -220463842: 5181 /* purpose */ return new Property("purpose", "markdown", 5182 "Explanation of why this terminology capabilities is needed and why it has been designed as it has.", 0, 1, 5183 purpose); 5184 case 1522889671: 5185 /* copyright */ return new Property("copyright", "markdown", 5186 "A copyright statement relating to the terminology capabilities and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the terminology capabilities.", 5187 0, 1, copyright); 5188 case 3292052: 5189 /* kind */ return new Property("kind", "code", 5190 "The way that this statement is intended to be used, to describe an actual running instance of software, a particular product (kind, not instance of software) or a class of implementation (e.g. a desired purchase).", 5191 0, 1, kind); 5192 case 1319330215: 5193 /* software */ return new Property("software", "", 5194 "Software that is covered by this terminology capability statement. It is used when the statement describes the capabilities of a particular software version, independent of an installation.", 5195 0, 1, software); 5196 case 1683336114: 5197 /* implementation */ return new Property("implementation", "", 5198 "Identifies a specific implementation instance that is described by the terminology capability statement - i.e. a particular installation, rather than the capabilities of a software program.", 5199 0, 1, implementation); 5200 case 1391591896: 5201 /* lockedDate */ return new Property("lockedDate", "boolean", "Whether the server supports lockedDate.", 0, 1, 5202 lockedDate); 5203 case -916511108: 5204 /* codeSystem */ return new Property("codeSystem", "", 5205 "Identifies a code system that is supported by the server. If there is a no code system URL, then this declares the general assumptions a client can make about support for any CodeSystem resource.", 5206 0, java.lang.Integer.MAX_VALUE, codeSystem); 5207 case 17878207: 5208 /* expansion */ return new Property("expansion", "", 5209 "Information about the [ValueSet/$expand](valueset-operation-expand.html) operation.", 0, 1, expansion); 5210 case -935519755: 5211 /* codeSearch */ return new Property("codeSearch", "code", 5212 "The degree to which the server supports the code search parameter on ValueSet, if it is supported.", 0, 1, 5213 codeSearch); 5214 case 1080737827: 5215 /* validateCode */ return new Property("validateCode", "", 5216 "Information about the [ValueSet/$validate-code](valueset-operation-validate-code.html) operation.", 0, 1, 5217 validateCode); 5218 case -1840647503: 5219 /* translation */ return new Property("translation", "", 5220 "Information about the [ConceptMap/$translate](conceptmap-operation-translate.html) operation.", 0, 1, 5221 translation); 5222 case 866552379: 5223 /* closure */ return new Property("closure", "", "Whether the $closure operation is supported.", 0, 1, closure); 5224 default: 5225 return super.getNamedProperty(_hash, _name, _checkValid); 5226 } 5227 5228 } 5229 5230 @Override 5231 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5232 switch (hash) { 5233 case 116079: 5234 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5235 case 351608024: 5236 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 5237 case 3373707: 5238 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5239 case 110371416: 5240 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5241 case -892481550: 5242 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 5243 case -404562712: 5244 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 5245 case 3076014: 5246 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 5247 case 1447404028: 5248 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 5249 case 951526432: 5250 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5251 case -1724546052: 5252 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5253 case -669707736: 5254 /* useContext */ return this.useContext == null ? new Base[0] 5255 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5256 case -507075711: 5257 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5258 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5259 case -220463842: 5260 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 5261 case 1522889671: 5262 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5263 case 3292052: 5264 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<CapabilityStatementKind> 5265 case 1319330215: 5266 /* software */ return this.software == null ? new Base[0] : new Base[] { this.software }; // TerminologyCapabilitiesSoftwareComponent 5267 case 1683336114: 5268 /* implementation */ return this.implementation == null ? new Base[0] : new Base[] { this.implementation }; // TerminologyCapabilitiesImplementationComponent 5269 case 1391591896: 5270 /* lockedDate */ return this.lockedDate == null ? new Base[0] : new Base[] { this.lockedDate }; // BooleanType 5271 case -916511108: 5272 /* codeSystem */ return this.codeSystem == null ? new Base[0] 5273 : this.codeSystem.toArray(new Base[this.codeSystem.size()]); // TerminologyCapabilitiesCodeSystemComponent 5274 case 17878207: 5275 /* expansion */ return this.expansion == null ? new Base[0] : new Base[] { this.expansion }; // TerminologyCapabilitiesExpansionComponent 5276 case -935519755: 5277 /* codeSearch */ return this.codeSearch == null ? new Base[0] : new Base[] { this.codeSearch }; // Enumeration<CodeSearchSupport> 5278 case 1080737827: 5279 /* validateCode */ return this.validateCode == null ? new Base[0] : new Base[] { this.validateCode }; // TerminologyCapabilitiesValidateCodeComponent 5280 case -1840647503: 5281 /* translation */ return this.translation == null ? new Base[0] : new Base[] { this.translation }; // TerminologyCapabilitiesTranslationComponent 5282 case 866552379: 5283 /* closure */ return this.closure == null ? new Base[0] : new Base[] { this.closure }; // TerminologyCapabilitiesClosureComponent 5284 default: 5285 return super.getProperty(hash, name, checkValid); 5286 } 5287 5288 } 5289 5290 @Override 5291 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5292 switch (hash) { 5293 case 116079: // url 5294 this.url = castToUri(value); // UriType 5295 return value; 5296 case 351608024: // version 5297 this.version = castToString(value); // StringType 5298 return value; 5299 case 3373707: // name 5300 this.name = castToString(value); // StringType 5301 return value; 5302 case 110371416: // title 5303 this.title = castToString(value); // StringType 5304 return value; 5305 case -892481550: // status 5306 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5307 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5308 return value; 5309 case -404562712: // experimental 5310 this.experimental = castToBoolean(value); // BooleanType 5311 return value; 5312 case 3076014: // date 5313 this.date = castToDateTime(value); // DateTimeType 5314 return value; 5315 case 1447404028: // publisher 5316 this.publisher = castToString(value); // StringType 5317 return value; 5318 case 951526432: // contact 5319 this.getContact().add(castToContactDetail(value)); // ContactDetail 5320 return value; 5321 case -1724546052: // description 5322 this.description = castToMarkdown(value); // MarkdownType 5323 return value; 5324 case -669707736: // useContext 5325 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5326 return value; 5327 case -507075711: // jurisdiction 5328 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5329 return value; 5330 case -220463842: // purpose 5331 this.purpose = castToMarkdown(value); // MarkdownType 5332 return value; 5333 case 1522889671: // copyright 5334 this.copyright = castToMarkdown(value); // MarkdownType 5335 return value; 5336 case 3292052: // kind 5337 value = new CapabilityStatementKindEnumFactory().fromType(castToCode(value)); 5338 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 5339 return value; 5340 case 1319330215: // software 5341 this.software = (TerminologyCapabilitiesSoftwareComponent) value; // TerminologyCapabilitiesSoftwareComponent 5342 return value; 5343 case 1683336114: // implementation 5344 this.implementation = (TerminologyCapabilitiesImplementationComponent) value; // TerminologyCapabilitiesImplementationComponent 5345 return value; 5346 case 1391591896: // lockedDate 5347 this.lockedDate = castToBoolean(value); // BooleanType 5348 return value; 5349 case -916511108: // codeSystem 5350 this.getCodeSystem().add((TerminologyCapabilitiesCodeSystemComponent) value); // TerminologyCapabilitiesCodeSystemComponent 5351 return value; 5352 case 17878207: // expansion 5353 this.expansion = (TerminologyCapabilitiesExpansionComponent) value; // TerminologyCapabilitiesExpansionComponent 5354 return value; 5355 case -935519755: // codeSearch 5356 value = new CodeSearchSupportEnumFactory().fromType(castToCode(value)); 5357 this.codeSearch = (Enumeration) value; // Enumeration<CodeSearchSupport> 5358 return value; 5359 case 1080737827: // validateCode 5360 this.validateCode = (TerminologyCapabilitiesValidateCodeComponent) value; // TerminologyCapabilitiesValidateCodeComponent 5361 return value; 5362 case -1840647503: // translation 5363 this.translation = (TerminologyCapabilitiesTranslationComponent) value; // TerminologyCapabilitiesTranslationComponent 5364 return value; 5365 case 866552379: // closure 5366 this.closure = (TerminologyCapabilitiesClosureComponent) value; // TerminologyCapabilitiesClosureComponent 5367 return value; 5368 default: 5369 return super.setProperty(hash, name, value); 5370 } 5371 5372 } 5373 5374 @Override 5375 public Base setProperty(String name, Base value) throws FHIRException { 5376 if (name.equals("url")) { 5377 this.url = castToUri(value); // UriType 5378 } else if (name.equals("version")) { 5379 this.version = castToString(value); // StringType 5380 } else if (name.equals("name")) { 5381 this.name = castToString(value); // StringType 5382 } else if (name.equals("title")) { 5383 this.title = castToString(value); // StringType 5384 } else if (name.equals("status")) { 5385 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5386 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5387 } else if (name.equals("experimental")) { 5388 this.experimental = castToBoolean(value); // BooleanType 5389 } else if (name.equals("date")) { 5390 this.date = castToDateTime(value); // DateTimeType 5391 } else if (name.equals("publisher")) { 5392 this.publisher = castToString(value); // StringType 5393 } else if (name.equals("contact")) { 5394 this.getContact().add(castToContactDetail(value)); 5395 } else if (name.equals("description")) { 5396 this.description = castToMarkdown(value); // MarkdownType 5397 } else if (name.equals("useContext")) { 5398 this.getUseContext().add(castToUsageContext(value)); 5399 } else if (name.equals("jurisdiction")) { 5400 this.getJurisdiction().add(castToCodeableConcept(value)); 5401 } else if (name.equals("purpose")) { 5402 this.purpose = castToMarkdown(value); // MarkdownType 5403 } else if (name.equals("copyright")) { 5404 this.copyright = castToMarkdown(value); // MarkdownType 5405 } else if (name.equals("kind")) { 5406 value = new CapabilityStatementKindEnumFactory().fromType(castToCode(value)); 5407 this.kind = (Enumeration) value; // Enumeration<CapabilityStatementKind> 5408 } else if (name.equals("software")) { 5409 this.software = (TerminologyCapabilitiesSoftwareComponent) value; // TerminologyCapabilitiesSoftwareComponent 5410 } else if (name.equals("implementation")) { 5411 this.implementation = (TerminologyCapabilitiesImplementationComponent) value; // TerminologyCapabilitiesImplementationComponent 5412 } else if (name.equals("lockedDate")) { 5413 this.lockedDate = castToBoolean(value); // BooleanType 5414 } else if (name.equals("codeSystem")) { 5415 this.getCodeSystem().add((TerminologyCapabilitiesCodeSystemComponent) value); 5416 } else if (name.equals("expansion")) { 5417 this.expansion = (TerminologyCapabilitiesExpansionComponent) value; // TerminologyCapabilitiesExpansionComponent 5418 } else if (name.equals("codeSearch")) { 5419 value = new CodeSearchSupportEnumFactory().fromType(castToCode(value)); 5420 this.codeSearch = (Enumeration) value; // Enumeration<CodeSearchSupport> 5421 } else if (name.equals("validateCode")) { 5422 this.validateCode = (TerminologyCapabilitiesValidateCodeComponent) value; // TerminologyCapabilitiesValidateCodeComponent 5423 } else if (name.equals("translation")) { 5424 this.translation = (TerminologyCapabilitiesTranslationComponent) value; // TerminologyCapabilitiesTranslationComponent 5425 } else if (name.equals("closure")) { 5426 this.closure = (TerminologyCapabilitiesClosureComponent) value; // TerminologyCapabilitiesClosureComponent 5427 } else 5428 return super.setProperty(name, value); 5429 return value; 5430 } 5431 5432 @Override 5433 public void removeChild(String name, Base value) throws FHIRException { 5434 if (name.equals("url")) { 5435 this.url = null; 5436 } else if (name.equals("version")) { 5437 this.version = null; 5438 } else if (name.equals("name")) { 5439 this.name = null; 5440 } else if (name.equals("title")) { 5441 this.title = null; 5442 } else if (name.equals("status")) { 5443 this.status = null; 5444 } else if (name.equals("experimental")) { 5445 this.experimental = null; 5446 } else if (name.equals("date")) { 5447 this.date = null; 5448 } else if (name.equals("publisher")) { 5449 this.publisher = null; 5450 } else if (name.equals("contact")) { 5451 this.getContact().remove(castToContactDetail(value)); 5452 } else if (name.equals("description")) { 5453 this.description = null; 5454 } else if (name.equals("useContext")) { 5455 this.getUseContext().remove(castToUsageContext(value)); 5456 } else if (name.equals("jurisdiction")) { 5457 this.getJurisdiction().remove(castToCodeableConcept(value)); 5458 } else if (name.equals("purpose")) { 5459 this.purpose = null; 5460 } else if (name.equals("copyright")) { 5461 this.copyright = null; 5462 } else if (name.equals("kind")) { 5463 this.kind = null; 5464 } else if (name.equals("software")) { 5465 this.software = (TerminologyCapabilitiesSoftwareComponent) value; // TerminologyCapabilitiesSoftwareComponent 5466 } else if (name.equals("implementation")) { 5467 this.implementation = (TerminologyCapabilitiesImplementationComponent) value; // TerminologyCapabilitiesImplementationComponent 5468 } else if (name.equals("lockedDate")) { 5469 this.lockedDate = null; 5470 } else if (name.equals("codeSystem")) { 5471 this.getCodeSystem().remove((TerminologyCapabilitiesCodeSystemComponent) value); 5472 } else if (name.equals("expansion")) { 5473 this.expansion = (TerminologyCapabilitiesExpansionComponent) value; // TerminologyCapabilitiesExpansionComponent 5474 } else if (name.equals("codeSearch")) { 5475 this.codeSearch = null; 5476 } else if (name.equals("validateCode")) { 5477 this.validateCode = (TerminologyCapabilitiesValidateCodeComponent) value; // TerminologyCapabilitiesValidateCodeComponent 5478 } else if (name.equals("translation")) { 5479 this.translation = (TerminologyCapabilitiesTranslationComponent) value; // TerminologyCapabilitiesTranslationComponent 5480 } else if (name.equals("closure")) { 5481 this.closure = (TerminologyCapabilitiesClosureComponent) value; // TerminologyCapabilitiesClosureComponent 5482 } else 5483 super.removeChild(name, value); 5484 5485 } 5486 5487 @Override 5488 public Base makeProperty(int hash, String name) throws FHIRException { 5489 switch (hash) { 5490 case 116079: 5491 return getUrlElement(); 5492 case 351608024: 5493 return getVersionElement(); 5494 case 3373707: 5495 return getNameElement(); 5496 case 110371416: 5497 return getTitleElement(); 5498 case -892481550: 5499 return getStatusElement(); 5500 case -404562712: 5501 return getExperimentalElement(); 5502 case 3076014: 5503 return getDateElement(); 5504 case 1447404028: 5505 return getPublisherElement(); 5506 case 951526432: 5507 return addContact(); 5508 case -1724546052: 5509 return getDescriptionElement(); 5510 case -669707736: 5511 return addUseContext(); 5512 case -507075711: 5513 return addJurisdiction(); 5514 case -220463842: 5515 return getPurposeElement(); 5516 case 1522889671: 5517 return getCopyrightElement(); 5518 case 3292052: 5519 return getKindElement(); 5520 case 1319330215: 5521 return getSoftware(); 5522 case 1683336114: 5523 return getImplementation(); 5524 case 1391591896: 5525 return getLockedDateElement(); 5526 case -916511108: 5527 return addCodeSystem(); 5528 case 17878207: 5529 return getExpansion(); 5530 case -935519755: 5531 return getCodeSearchElement(); 5532 case 1080737827: 5533 return getValidateCode(); 5534 case -1840647503: 5535 return getTranslation(); 5536 case 866552379: 5537 return getClosure(); 5538 default: 5539 return super.makeProperty(hash, name); 5540 } 5541 5542 } 5543 5544 @Override 5545 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5546 switch (hash) { 5547 case 116079: 5548 /* url */ return new String[] { "uri" }; 5549 case 351608024: 5550 /* version */ return new String[] { "string" }; 5551 case 3373707: 5552 /* name */ return new String[] { "string" }; 5553 case 110371416: 5554 /* title */ return new String[] { "string" }; 5555 case -892481550: 5556 /* status */ return new String[] { "code" }; 5557 case -404562712: 5558 /* experimental */ return new String[] { "boolean" }; 5559 case 3076014: 5560 /* date */ return new String[] { "dateTime" }; 5561 case 1447404028: 5562 /* publisher */ return new String[] { "string" }; 5563 case 951526432: 5564 /* contact */ return new String[] { "ContactDetail" }; 5565 case -1724546052: 5566 /* description */ return new String[] { "markdown" }; 5567 case -669707736: 5568 /* useContext */ return new String[] { "UsageContext" }; 5569 case -507075711: 5570 /* jurisdiction */ return new String[] { "CodeableConcept" }; 5571 case -220463842: 5572 /* purpose */ return new String[] { "markdown" }; 5573 case 1522889671: 5574 /* copyright */ return new String[] { "markdown" }; 5575 case 3292052: 5576 /* kind */ return new String[] { "code" }; 5577 case 1319330215: 5578 /* software */ return new String[] {}; 5579 case 1683336114: 5580 /* implementation */ return new String[] {}; 5581 case 1391591896: 5582 /* lockedDate */ return new String[] { "boolean" }; 5583 case -916511108: 5584 /* codeSystem */ return new String[] {}; 5585 case 17878207: 5586 /* expansion */ return new String[] {}; 5587 case -935519755: 5588 /* codeSearch */ return new String[] { "code" }; 5589 case 1080737827: 5590 /* validateCode */ return new String[] {}; 5591 case -1840647503: 5592 /* translation */ return new String[] {}; 5593 case 866552379: 5594 /* closure */ return new String[] {}; 5595 default: 5596 return super.getTypesForProperty(hash, name); 5597 } 5598 5599 } 5600 5601 @Override 5602 public Base addChild(String name) throws FHIRException { 5603 if (name.equals("url")) { 5604 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.url"); 5605 } else if (name.equals("version")) { 5606 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.version"); 5607 } else if (name.equals("name")) { 5608 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.name"); 5609 } else if (name.equals("title")) { 5610 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.title"); 5611 } else if (name.equals("status")) { 5612 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.status"); 5613 } else if (name.equals("experimental")) { 5614 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.experimental"); 5615 } else if (name.equals("date")) { 5616 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.date"); 5617 } else if (name.equals("publisher")) { 5618 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.publisher"); 5619 } else if (name.equals("contact")) { 5620 return addContact(); 5621 } else if (name.equals("description")) { 5622 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.description"); 5623 } else if (name.equals("useContext")) { 5624 return addUseContext(); 5625 } else if (name.equals("jurisdiction")) { 5626 return addJurisdiction(); 5627 } else if (name.equals("purpose")) { 5628 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.purpose"); 5629 } else if (name.equals("copyright")) { 5630 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.copyright"); 5631 } else if (name.equals("kind")) { 5632 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.kind"); 5633 } else if (name.equals("software")) { 5634 this.software = new TerminologyCapabilitiesSoftwareComponent(); 5635 return this.software; 5636 } else if (name.equals("implementation")) { 5637 this.implementation = new TerminologyCapabilitiesImplementationComponent(); 5638 return this.implementation; 5639 } else if (name.equals("lockedDate")) { 5640 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.lockedDate"); 5641 } else if (name.equals("codeSystem")) { 5642 return addCodeSystem(); 5643 } else if (name.equals("expansion")) { 5644 this.expansion = new TerminologyCapabilitiesExpansionComponent(); 5645 return this.expansion; 5646 } else if (name.equals("codeSearch")) { 5647 throw new FHIRException("Cannot call addChild on a singleton property TerminologyCapabilities.codeSearch"); 5648 } else if (name.equals("validateCode")) { 5649 this.validateCode = new TerminologyCapabilitiesValidateCodeComponent(); 5650 return this.validateCode; 5651 } else if (name.equals("translation")) { 5652 this.translation = new TerminologyCapabilitiesTranslationComponent(); 5653 return this.translation; 5654 } else if (name.equals("closure")) { 5655 this.closure = new TerminologyCapabilitiesClosureComponent(); 5656 return this.closure; 5657 } else 5658 return super.addChild(name); 5659 } 5660 5661 public String fhirType() { 5662 return "TerminologyCapabilities"; 5663 5664 } 5665 5666 public TerminologyCapabilities copy() { 5667 TerminologyCapabilities dst = new TerminologyCapabilities(); 5668 copyValues(dst); 5669 return dst; 5670 } 5671 5672 public void copyValues(TerminologyCapabilities dst) { 5673 super.copyValues(dst); 5674 dst.url = url == null ? null : url.copy(); 5675 dst.version = version == null ? null : version.copy(); 5676 dst.name = name == null ? null : name.copy(); 5677 dst.title = title == null ? null : title.copy(); 5678 dst.status = status == null ? null : status.copy(); 5679 dst.experimental = experimental == null ? null : experimental.copy(); 5680 dst.date = date == null ? null : date.copy(); 5681 dst.publisher = publisher == null ? null : publisher.copy(); 5682 if (contact != null) { 5683 dst.contact = new ArrayList<ContactDetail>(); 5684 for (ContactDetail i : contact) 5685 dst.contact.add(i.copy()); 5686 } 5687 ; 5688 dst.description = description == null ? null : description.copy(); 5689 if (useContext != null) { 5690 dst.useContext = new ArrayList<UsageContext>(); 5691 for (UsageContext i : useContext) 5692 dst.useContext.add(i.copy()); 5693 } 5694 ; 5695 if (jurisdiction != null) { 5696 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5697 for (CodeableConcept i : jurisdiction) 5698 dst.jurisdiction.add(i.copy()); 5699 } 5700 ; 5701 dst.purpose = purpose == null ? null : purpose.copy(); 5702 dst.copyright = copyright == null ? null : copyright.copy(); 5703 dst.kind = kind == null ? null : kind.copy(); 5704 dst.software = software == null ? null : software.copy(); 5705 dst.implementation = implementation == null ? null : implementation.copy(); 5706 dst.lockedDate = lockedDate == null ? null : lockedDate.copy(); 5707 if (codeSystem != null) { 5708 dst.codeSystem = new ArrayList<TerminologyCapabilitiesCodeSystemComponent>(); 5709 for (TerminologyCapabilitiesCodeSystemComponent i : codeSystem) 5710 dst.codeSystem.add(i.copy()); 5711 } 5712 ; 5713 dst.expansion = expansion == null ? null : expansion.copy(); 5714 dst.codeSearch = codeSearch == null ? null : codeSearch.copy(); 5715 dst.validateCode = validateCode == null ? null : validateCode.copy(); 5716 dst.translation = translation == null ? null : translation.copy(); 5717 dst.closure = closure == null ? null : closure.copy(); 5718 } 5719 5720 protected TerminologyCapabilities typedCopy() { 5721 return copy(); 5722 } 5723 5724 @Override 5725 public boolean equalsDeep(Base other_) { 5726 if (!super.equalsDeep(other_)) 5727 return false; 5728 if (!(other_ instanceof TerminologyCapabilities)) 5729 return false; 5730 TerminologyCapabilities o = (TerminologyCapabilities) other_; 5731 return compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 5732 && compareDeep(kind, o.kind, true) && compareDeep(software, o.software, true) 5733 && compareDeep(implementation, o.implementation, true) && compareDeep(lockedDate, o.lockedDate, true) 5734 && compareDeep(codeSystem, o.codeSystem, true) && compareDeep(expansion, o.expansion, true) 5735 && compareDeep(codeSearch, o.codeSearch, true) && compareDeep(validateCode, o.validateCode, true) 5736 && compareDeep(translation, o.translation, true) && compareDeep(closure, o.closure, true); 5737 } 5738 5739 @Override 5740 public boolean equalsShallow(Base other_) { 5741 if (!super.equalsShallow(other_)) 5742 return false; 5743 if (!(other_ instanceof TerminologyCapabilities)) 5744 return false; 5745 TerminologyCapabilities o = (TerminologyCapabilities) other_; 5746 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 5747 && compareValues(kind, o.kind, true) && compareValues(lockedDate, o.lockedDate, true) 5748 && compareValues(codeSearch, o.codeSearch, true); 5749 } 5750 5751 public boolean isEmpty() { 5752 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, copyright, kind, software, implementation, 5753 lockedDate, codeSystem, expansion, codeSearch, validateCode, translation, closure); 5754 } 5755 5756 @Override 5757 public ResourceType getResourceType() { 5758 return ResourceType.TerminologyCapabilities; 5759 } 5760 5761 /** 5762 * Search parameter: <b>date</b> 5763 * <p> 5764 * Description: <b>The terminology capabilities publication date</b><br> 5765 * Type: <b>date</b><br> 5766 * Path: <b>TerminologyCapabilities.date</b><br> 5767 * </p> 5768 */ 5769 @SearchParamDefinition(name = "date", path = "TerminologyCapabilities.date", description = "The terminology capabilities publication date", type = "date") 5770 public static final String SP_DATE = "date"; 5771 /** 5772 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5773 * <p> 5774 * Description: <b>The terminology capabilities publication date</b><br> 5775 * Type: <b>date</b><br> 5776 * Path: <b>TerminologyCapabilities.date</b><br> 5777 * </p> 5778 */ 5779 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5780 SP_DATE); 5781 5782 /** 5783 * Search parameter: <b>context-type-value</b> 5784 * <p> 5785 * Description: <b>A use context type and value assigned to the terminology 5786 * capabilities</b><br> 5787 * Type: <b>composite</b><br> 5788 * Path: <b></b><br> 5789 * </p> 5790 */ 5791 @SearchParamDefinition(name = "context-type-value", path = "TerminologyCapabilities.useContext", description = "A use context type and value assigned to the terminology capabilities", type = "composite", compositeOf = { 5792 "context-type", "context" }) 5793 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5794 /** 5795 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5796 * <p> 5797 * Description: <b>A use context type and value assigned to the terminology 5798 * capabilities</b><br> 5799 * Type: <b>composite</b><br> 5800 * Path: <b></b><br> 5801 * </p> 5802 */ 5803 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5804 SP_CONTEXT_TYPE_VALUE); 5805 5806 /** 5807 * Search parameter: <b>jurisdiction</b> 5808 * <p> 5809 * Description: <b>Intended jurisdiction for the terminology 5810 * capabilities</b><br> 5811 * Type: <b>token</b><br> 5812 * Path: <b>TerminologyCapabilities.jurisdiction</b><br> 5813 * </p> 5814 */ 5815 @SearchParamDefinition(name = "jurisdiction", path = "TerminologyCapabilities.jurisdiction", description = "Intended jurisdiction for the terminology capabilities", type = "token") 5816 public static final String SP_JURISDICTION = "jurisdiction"; 5817 /** 5818 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5819 * <p> 5820 * Description: <b>Intended jurisdiction for the terminology 5821 * capabilities</b><br> 5822 * Type: <b>token</b><br> 5823 * Path: <b>TerminologyCapabilities.jurisdiction</b><br> 5824 * </p> 5825 */ 5826 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5827 SP_JURISDICTION); 5828 5829 /** 5830 * Search parameter: <b>description</b> 5831 * <p> 5832 * Description: <b>The description of the terminology capabilities</b><br> 5833 * Type: <b>string</b><br> 5834 * Path: <b>TerminologyCapabilities.description</b><br> 5835 * </p> 5836 */ 5837 @SearchParamDefinition(name = "description", path = "TerminologyCapabilities.description", description = "The description of the terminology capabilities", type = "string") 5838 public static final String SP_DESCRIPTION = "description"; 5839 /** 5840 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5841 * <p> 5842 * Description: <b>The description of the terminology capabilities</b><br> 5843 * Type: <b>string</b><br> 5844 * Path: <b>TerminologyCapabilities.description</b><br> 5845 * </p> 5846 */ 5847 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 5848 SP_DESCRIPTION); 5849 5850 /** 5851 * Search parameter: <b>context-type</b> 5852 * <p> 5853 * Description: <b>A type of use context assigned to the terminology 5854 * capabilities</b><br> 5855 * Type: <b>token</b><br> 5856 * Path: <b>TerminologyCapabilities.useContext.code</b><br> 5857 * </p> 5858 */ 5859 @SearchParamDefinition(name = "context-type", path = "TerminologyCapabilities.useContext.code", description = "A type of use context assigned to the terminology capabilities", type = "token") 5860 public static final String SP_CONTEXT_TYPE = "context-type"; 5861 /** 5862 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5863 * <p> 5864 * Description: <b>A type of use context assigned to the terminology 5865 * capabilities</b><br> 5866 * Type: <b>token</b><br> 5867 * Path: <b>TerminologyCapabilities.useContext.code</b><br> 5868 * </p> 5869 */ 5870 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5871 SP_CONTEXT_TYPE); 5872 5873 /** 5874 * Search parameter: <b>title</b> 5875 * <p> 5876 * Description: <b>The human-friendly name of the terminology 5877 * capabilities</b><br> 5878 * Type: <b>string</b><br> 5879 * Path: <b>TerminologyCapabilities.title</b><br> 5880 * </p> 5881 */ 5882 @SearchParamDefinition(name = "title", path = "TerminologyCapabilities.title", description = "The human-friendly name of the terminology capabilities", type = "string") 5883 public static final String SP_TITLE = "title"; 5884 /** 5885 * <b>Fluent Client</b> search parameter constant for <b>title</b> 5886 * <p> 5887 * Description: <b>The human-friendly name of the terminology 5888 * capabilities</b><br> 5889 * Type: <b>string</b><br> 5890 * Path: <b>TerminologyCapabilities.title</b><br> 5891 * </p> 5892 */ 5893 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 5894 SP_TITLE); 5895 5896 /** 5897 * Search parameter: <b>version</b> 5898 * <p> 5899 * Description: <b>The business version of the terminology capabilities</b><br> 5900 * Type: <b>token</b><br> 5901 * Path: <b>TerminologyCapabilities.version</b><br> 5902 * </p> 5903 */ 5904 @SearchParamDefinition(name = "version", path = "TerminologyCapabilities.version", description = "The business version of the terminology capabilities", type = "token") 5905 public static final String SP_VERSION = "version"; 5906 /** 5907 * <b>Fluent Client</b> search parameter constant for <b>version</b> 5908 * <p> 5909 * Description: <b>The business version of the terminology capabilities</b><br> 5910 * Type: <b>token</b><br> 5911 * Path: <b>TerminologyCapabilities.version</b><br> 5912 * </p> 5913 */ 5914 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5915 SP_VERSION); 5916 5917 /** 5918 * Search parameter: <b>url</b> 5919 * <p> 5920 * Description: <b>The uri that identifies the terminology capabilities</b><br> 5921 * Type: <b>uri</b><br> 5922 * Path: <b>TerminologyCapabilities.url</b><br> 5923 * </p> 5924 */ 5925 @SearchParamDefinition(name = "url", path = "TerminologyCapabilities.url", description = "The uri that identifies the terminology capabilities", type = "uri") 5926 public static final String SP_URL = "url"; 5927 /** 5928 * <b>Fluent Client</b> search parameter constant for <b>url</b> 5929 * <p> 5930 * Description: <b>The uri that identifies the terminology capabilities</b><br> 5931 * Type: <b>uri</b><br> 5932 * Path: <b>TerminologyCapabilities.url</b><br> 5933 * </p> 5934 */ 5935 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 5936 5937 /** 5938 * Search parameter: <b>context-quantity</b> 5939 * <p> 5940 * Description: <b>A quantity- or range-valued use context assigned to the 5941 * terminology capabilities</b><br> 5942 * Type: <b>quantity</b><br> 5943 * Path: <b>TerminologyCapabilities.useContext.valueQuantity, 5944 * TerminologyCapabilities.useContext.valueRange</b><br> 5945 * </p> 5946 */ 5947 @SearchParamDefinition(name = "context-quantity", path = "(TerminologyCapabilities.useContext.value as Quantity) | (TerminologyCapabilities.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the terminology capabilities", type = "quantity") 5948 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5949 /** 5950 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5951 * <p> 5952 * Description: <b>A quantity- or range-valued use context assigned to the 5953 * terminology capabilities</b><br> 5954 * Type: <b>quantity</b><br> 5955 * Path: <b>TerminologyCapabilities.useContext.valueQuantity, 5956 * TerminologyCapabilities.useContext.valueRange</b><br> 5957 * </p> 5958 */ 5959 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5960 SP_CONTEXT_QUANTITY); 5961 5962 /** 5963 * Search parameter: <b>name</b> 5964 * <p> 5965 * Description: <b>Computationally friendly name of the terminology 5966 * capabilities</b><br> 5967 * Type: <b>string</b><br> 5968 * Path: <b>TerminologyCapabilities.name</b><br> 5969 * </p> 5970 */ 5971 @SearchParamDefinition(name = "name", path = "TerminologyCapabilities.name", description = "Computationally friendly name of the terminology capabilities", type = "string") 5972 public static final String SP_NAME = "name"; 5973 /** 5974 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5975 * <p> 5976 * Description: <b>Computationally friendly name of the terminology 5977 * capabilities</b><br> 5978 * Type: <b>string</b><br> 5979 * Path: <b>TerminologyCapabilities.name</b><br> 5980 * </p> 5981 */ 5982 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5983 SP_NAME); 5984 5985 /** 5986 * Search parameter: <b>context</b> 5987 * <p> 5988 * Description: <b>A use context assigned to the terminology 5989 * capabilities</b><br> 5990 * Type: <b>token</b><br> 5991 * Path: <b>TerminologyCapabilities.useContext.valueCodeableConcept</b><br> 5992 * </p> 5993 */ 5994 @SearchParamDefinition(name = "context", path = "(TerminologyCapabilities.useContext.value as CodeableConcept)", description = "A use context assigned to the terminology capabilities", type = "token") 5995 public static final String SP_CONTEXT = "context"; 5996 /** 5997 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5998 * <p> 5999 * Description: <b>A use context assigned to the terminology 6000 * capabilities</b><br> 6001 * Type: <b>token</b><br> 6002 * Path: <b>TerminologyCapabilities.useContext.valueCodeableConcept</b><br> 6003 * </p> 6004 */ 6005 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6006 SP_CONTEXT); 6007 6008 /** 6009 * Search parameter: <b>publisher</b> 6010 * <p> 6011 * Description: <b>Name of the publisher of the terminology capabilities</b><br> 6012 * Type: <b>string</b><br> 6013 * Path: <b>TerminologyCapabilities.publisher</b><br> 6014 * </p> 6015 */ 6016 @SearchParamDefinition(name = "publisher", path = "TerminologyCapabilities.publisher", description = "Name of the publisher of the terminology capabilities", type = "string") 6017 public static final String SP_PUBLISHER = "publisher"; 6018 /** 6019 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6020 * <p> 6021 * Description: <b>Name of the publisher of the terminology capabilities</b><br> 6022 * Type: <b>string</b><br> 6023 * Path: <b>TerminologyCapabilities.publisher</b><br> 6024 * </p> 6025 */ 6026 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 6027 SP_PUBLISHER); 6028 6029 /** 6030 * Search parameter: <b>context-type-quantity</b> 6031 * <p> 6032 * Description: <b>A use context type and quantity- or range-based value 6033 * assigned to the terminology capabilities</b><br> 6034 * Type: <b>composite</b><br> 6035 * Path: <b></b><br> 6036 * </p> 6037 */ 6038 @SearchParamDefinition(name = "context-type-quantity", path = "TerminologyCapabilities.useContext", description = "A use context type and quantity- or range-based value assigned to the terminology capabilities", type = "composite", compositeOf = { 6039 "context-type", "context-quantity" }) 6040 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6041 /** 6042 * <b>Fluent Client</b> search parameter constant for 6043 * <b>context-type-quantity</b> 6044 * <p> 6045 * Description: <b>A use context type and quantity- or range-based value 6046 * assigned to the terminology capabilities</b><br> 6047 * Type: <b>composite</b><br> 6048 * Path: <b></b><br> 6049 * </p> 6050 */ 6051 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 6052 SP_CONTEXT_TYPE_QUANTITY); 6053 6054 /** 6055 * Search parameter: <b>status</b> 6056 * <p> 6057 * Description: <b>The current status of the terminology capabilities</b><br> 6058 * Type: <b>token</b><br> 6059 * Path: <b>TerminologyCapabilities.status</b><br> 6060 * </p> 6061 */ 6062 @SearchParamDefinition(name = "status", path = "TerminologyCapabilities.status", description = "The current status of the terminology capabilities", type = "token") 6063 public static final String SP_STATUS = "status"; 6064 /** 6065 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6066 * <p> 6067 * Description: <b>The current status of the terminology capabilities</b><br> 6068 * Type: <b>token</b><br> 6069 * Path: <b>TerminologyCapabilities.status</b><br> 6070 * </p> 6071 */ 6072 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6073 SP_STATUS); 6074 6075}