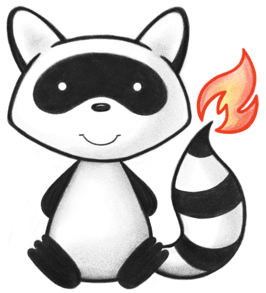
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * A summary of information based on the results of executing a TestScript. 051 */ 052@ResourceDef(name = "TestReport", profile = "http://hl7.org/fhir/StructureDefinition/TestReport") 053public class TestReport extends DomainResource { 054 055 public enum TestReportStatus { 056 /** 057 * All test operations have completed. 058 */ 059 COMPLETED, 060 /** 061 * A test operations is currently executing. 062 */ 063 INPROGRESS, 064 /** 065 * A test operation is waiting for an external client request. 066 */ 067 WAITING, 068 /** 069 * The test script execution was manually stopped. 070 */ 071 STOPPED, 072 /** 073 * This test report was entered or created in error. 074 */ 075 ENTEREDINERROR, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 081 public static TestReportStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("completed".equals(codeString)) 085 return COMPLETED; 086 if ("in-progress".equals(codeString)) 087 return INPROGRESS; 088 if ("waiting".equals(codeString)) 089 return WAITING; 090 if ("stopped".equals(codeString)) 091 return STOPPED; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown TestReportStatus code '" + codeString + "'"); 098 } 099 100 public String toCode() { 101 switch (this) { 102 case COMPLETED: 103 return "completed"; 104 case INPROGRESS: 105 return "in-progress"; 106 case WAITING: 107 return "waiting"; 108 case STOPPED: 109 return "stopped"; 110 case ENTEREDINERROR: 111 return "entered-in-error"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getSystem() { 120 switch (this) { 121 case COMPLETED: 122 return "http://hl7.org/fhir/report-status-codes"; 123 case INPROGRESS: 124 return "http://hl7.org/fhir/report-status-codes"; 125 case WAITING: 126 return "http://hl7.org/fhir/report-status-codes"; 127 case STOPPED: 128 return "http://hl7.org/fhir/report-status-codes"; 129 case ENTEREDINERROR: 130 return "http://hl7.org/fhir/report-status-codes"; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case COMPLETED: 141 return "All test operations have completed."; 142 case INPROGRESS: 143 return "A test operations is currently executing."; 144 case WAITING: 145 return "A test operation is waiting for an external client request."; 146 case STOPPED: 147 return "The test script execution was manually stopped."; 148 case ENTEREDINERROR: 149 return "This test report was entered or created in error."; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDisplay() { 158 switch (this) { 159 case COMPLETED: 160 return "Completed"; 161 case INPROGRESS: 162 return "In Progress"; 163 case WAITING: 164 return "Waiting"; 165 case STOPPED: 166 return "Stopped"; 167 case ENTEREDINERROR: 168 return "Entered In Error"; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 } 176 177 public static class TestReportStatusEnumFactory implements EnumFactory<TestReportStatus> { 178 public TestReportStatus fromCode(String codeString) throws IllegalArgumentException { 179 if (codeString == null || "".equals(codeString)) 180 if (codeString == null || "".equals(codeString)) 181 return null; 182 if ("completed".equals(codeString)) 183 return TestReportStatus.COMPLETED; 184 if ("in-progress".equals(codeString)) 185 return TestReportStatus.INPROGRESS; 186 if ("waiting".equals(codeString)) 187 return TestReportStatus.WAITING; 188 if ("stopped".equals(codeString)) 189 return TestReportStatus.STOPPED; 190 if ("entered-in-error".equals(codeString)) 191 return TestReportStatus.ENTEREDINERROR; 192 throw new IllegalArgumentException("Unknown TestReportStatus code '" + codeString + "'"); 193 } 194 195 public Enumeration<TestReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 196 if (code == null) 197 return null; 198 if (code.isEmpty()) 199 return new Enumeration<TestReportStatus>(this, TestReportStatus.NULL, code); 200 String codeString = code.asStringValue(); 201 if (codeString == null || "".equals(codeString)) 202 return new Enumeration<TestReportStatus>(this, TestReportStatus.NULL, code); 203 if ("completed".equals(codeString)) 204 return new Enumeration<TestReportStatus>(this, TestReportStatus.COMPLETED, code); 205 if ("in-progress".equals(codeString)) 206 return new Enumeration<TestReportStatus>(this, TestReportStatus.INPROGRESS, code); 207 if ("waiting".equals(codeString)) 208 return new Enumeration<TestReportStatus>(this, TestReportStatus.WAITING, code); 209 if ("stopped".equals(codeString)) 210 return new Enumeration<TestReportStatus>(this, TestReportStatus.STOPPED, code); 211 if ("entered-in-error".equals(codeString)) 212 return new Enumeration<TestReportStatus>(this, TestReportStatus.ENTEREDINERROR, code); 213 throw new FHIRException("Unknown TestReportStatus code '" + codeString + "'"); 214 } 215 216 public String toCode(TestReportStatus code) { 217 if (code == TestReportStatus.NULL) 218 return null; 219 if (code == TestReportStatus.COMPLETED) 220 return "completed"; 221 if (code == TestReportStatus.INPROGRESS) 222 return "in-progress"; 223 if (code == TestReportStatus.WAITING) 224 return "waiting"; 225 if (code == TestReportStatus.STOPPED) 226 return "stopped"; 227 if (code == TestReportStatus.ENTEREDINERROR) 228 return "entered-in-error"; 229 return "?"; 230 } 231 232 public String toSystem(TestReportStatus code) { 233 return code.getSystem(); 234 } 235 } 236 237 public enum TestReportResult { 238 /** 239 * All test operations successfully passed all asserts. 240 */ 241 PASS, 242 /** 243 * One or more test operations failed one or more asserts. 244 */ 245 FAIL, 246 /** 247 * One or more test operations is pending execution completion. 248 */ 249 PENDING, 250 /** 251 * added to help the parsers with the generic types 252 */ 253 NULL; 254 255 public static TestReportResult fromCode(String codeString) throws FHIRException { 256 if (codeString == null || "".equals(codeString)) 257 return null; 258 if ("pass".equals(codeString)) 259 return PASS; 260 if ("fail".equals(codeString)) 261 return FAIL; 262 if ("pending".equals(codeString)) 263 return PENDING; 264 if (Configuration.isAcceptInvalidEnums()) 265 return null; 266 else 267 throw new FHIRException("Unknown TestReportResult code '" + codeString + "'"); 268 } 269 270 public String toCode() { 271 switch (this) { 272 case PASS: 273 return "pass"; 274 case FAIL: 275 return "fail"; 276 case PENDING: 277 return "pending"; 278 case NULL: 279 return null; 280 default: 281 return "?"; 282 } 283 } 284 285 public String getSystem() { 286 switch (this) { 287 case PASS: 288 return "http://hl7.org/fhir/report-result-codes"; 289 case FAIL: 290 return "http://hl7.org/fhir/report-result-codes"; 291 case PENDING: 292 return "http://hl7.org/fhir/report-result-codes"; 293 case NULL: 294 return null; 295 default: 296 return "?"; 297 } 298 } 299 300 public String getDefinition() { 301 switch (this) { 302 case PASS: 303 return "All test operations successfully passed all asserts."; 304 case FAIL: 305 return "One or more test operations failed one or more asserts."; 306 case PENDING: 307 return "One or more test operations is pending execution completion."; 308 case NULL: 309 return null; 310 default: 311 return "?"; 312 } 313 } 314 315 public String getDisplay() { 316 switch (this) { 317 case PASS: 318 return "Pass"; 319 case FAIL: 320 return "Fail"; 321 case PENDING: 322 return "Pending"; 323 case NULL: 324 return null; 325 default: 326 return "?"; 327 } 328 } 329 } 330 331 public static class TestReportResultEnumFactory implements EnumFactory<TestReportResult> { 332 public TestReportResult fromCode(String codeString) throws IllegalArgumentException { 333 if (codeString == null || "".equals(codeString)) 334 if (codeString == null || "".equals(codeString)) 335 return null; 336 if ("pass".equals(codeString)) 337 return TestReportResult.PASS; 338 if ("fail".equals(codeString)) 339 return TestReportResult.FAIL; 340 if ("pending".equals(codeString)) 341 return TestReportResult.PENDING; 342 throw new IllegalArgumentException("Unknown TestReportResult code '" + codeString + "'"); 343 } 344 345 public Enumeration<TestReportResult> fromType(PrimitiveType<?> code) throws FHIRException { 346 if (code == null) 347 return null; 348 if (code.isEmpty()) 349 return new Enumeration<TestReportResult>(this, TestReportResult.NULL, code); 350 String codeString = code.asStringValue(); 351 if (codeString == null || "".equals(codeString)) 352 return new Enumeration<TestReportResult>(this, TestReportResult.NULL, code); 353 if ("pass".equals(codeString)) 354 return new Enumeration<TestReportResult>(this, TestReportResult.PASS, code); 355 if ("fail".equals(codeString)) 356 return new Enumeration<TestReportResult>(this, TestReportResult.FAIL, code); 357 if ("pending".equals(codeString)) 358 return new Enumeration<TestReportResult>(this, TestReportResult.PENDING, code); 359 throw new FHIRException("Unknown TestReportResult code '" + codeString + "'"); 360 } 361 362 public String toCode(TestReportResult code) { 363 if (code == TestReportResult.NULL) 364 return null; 365 if (code == TestReportResult.PASS) 366 return "pass"; 367 if (code == TestReportResult.FAIL) 368 return "fail"; 369 if (code == TestReportResult.PENDING) 370 return "pending"; 371 return "?"; 372 } 373 374 public String toSystem(TestReportResult code) { 375 return code.getSystem(); 376 } 377 } 378 379 public enum TestReportParticipantType { 380 /** 381 * The test execution engine. 382 */ 383 TESTENGINE, 384 /** 385 * A FHIR Client. 386 */ 387 CLIENT, 388 /** 389 * A FHIR Server. 390 */ 391 SERVER, 392 /** 393 * added to help the parsers with the generic types 394 */ 395 NULL; 396 397 public static TestReportParticipantType fromCode(String codeString) throws FHIRException { 398 if (codeString == null || "".equals(codeString)) 399 return null; 400 if ("test-engine".equals(codeString)) 401 return TESTENGINE; 402 if ("client".equals(codeString)) 403 return CLIENT; 404 if ("server".equals(codeString)) 405 return SERVER; 406 if (Configuration.isAcceptInvalidEnums()) 407 return null; 408 else 409 throw new FHIRException("Unknown TestReportParticipantType code '" + codeString + "'"); 410 } 411 412 public String toCode() { 413 switch (this) { 414 case TESTENGINE: 415 return "test-engine"; 416 case CLIENT: 417 return "client"; 418 case SERVER: 419 return "server"; 420 case NULL: 421 return null; 422 default: 423 return "?"; 424 } 425 } 426 427 public String getSystem() { 428 switch (this) { 429 case TESTENGINE: 430 return "http://hl7.org/fhir/report-participant-type"; 431 case CLIENT: 432 return "http://hl7.org/fhir/report-participant-type"; 433 case SERVER: 434 return "http://hl7.org/fhir/report-participant-type"; 435 case NULL: 436 return null; 437 default: 438 return "?"; 439 } 440 } 441 442 public String getDefinition() { 443 switch (this) { 444 case TESTENGINE: 445 return "The test execution engine."; 446 case CLIENT: 447 return "A FHIR Client."; 448 case SERVER: 449 return "A FHIR Server."; 450 case NULL: 451 return null; 452 default: 453 return "?"; 454 } 455 } 456 457 public String getDisplay() { 458 switch (this) { 459 case TESTENGINE: 460 return "Test Engine"; 461 case CLIENT: 462 return "Client"; 463 case SERVER: 464 return "Server"; 465 case NULL: 466 return null; 467 default: 468 return "?"; 469 } 470 } 471 } 472 473 public static class TestReportParticipantTypeEnumFactory implements EnumFactory<TestReportParticipantType> { 474 public TestReportParticipantType fromCode(String codeString) throws IllegalArgumentException { 475 if (codeString == null || "".equals(codeString)) 476 if (codeString == null || "".equals(codeString)) 477 return null; 478 if ("test-engine".equals(codeString)) 479 return TestReportParticipantType.TESTENGINE; 480 if ("client".equals(codeString)) 481 return TestReportParticipantType.CLIENT; 482 if ("server".equals(codeString)) 483 return TestReportParticipantType.SERVER; 484 throw new IllegalArgumentException("Unknown TestReportParticipantType code '" + codeString + "'"); 485 } 486 487 public Enumeration<TestReportParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 488 if (code == null) 489 return null; 490 if (code.isEmpty()) 491 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.NULL, code); 492 String codeString = code.asStringValue(); 493 if (codeString == null || "".equals(codeString)) 494 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.NULL, code); 495 if ("test-engine".equals(codeString)) 496 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.TESTENGINE, code); 497 if ("client".equals(codeString)) 498 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.CLIENT, code); 499 if ("server".equals(codeString)) 500 return new Enumeration<TestReportParticipantType>(this, TestReportParticipantType.SERVER, code); 501 throw new FHIRException("Unknown TestReportParticipantType code '" + codeString + "'"); 502 } 503 504 public String toCode(TestReportParticipantType code) { 505 if (code == TestReportParticipantType.NULL) 506 return null; 507 if (code == TestReportParticipantType.TESTENGINE) 508 return "test-engine"; 509 if (code == TestReportParticipantType.CLIENT) 510 return "client"; 511 if (code == TestReportParticipantType.SERVER) 512 return "server"; 513 return "?"; 514 } 515 516 public String toSystem(TestReportParticipantType code) { 517 return code.getSystem(); 518 } 519 } 520 521 public enum TestReportActionResult { 522 /** 523 * The action was successful. 524 */ 525 PASS, 526 /** 527 * The action was skipped. 528 */ 529 SKIP, 530 /** 531 * The action failed. 532 */ 533 FAIL, 534 /** 535 * The action passed but with warnings. 536 */ 537 WARNING, 538 /** 539 * The action encountered a fatal error and the engine was unable to process. 540 */ 541 ERROR, 542 /** 543 * added to help the parsers with the generic types 544 */ 545 NULL; 546 547 public static TestReportActionResult fromCode(String codeString) throws FHIRException { 548 if (codeString == null || "".equals(codeString)) 549 return null; 550 if ("pass".equals(codeString)) 551 return PASS; 552 if ("skip".equals(codeString)) 553 return SKIP; 554 if ("fail".equals(codeString)) 555 return FAIL; 556 if ("warning".equals(codeString)) 557 return WARNING; 558 if ("error".equals(codeString)) 559 return ERROR; 560 if (Configuration.isAcceptInvalidEnums()) 561 return null; 562 else 563 throw new FHIRException("Unknown TestReportActionResult code '" + codeString + "'"); 564 } 565 566 public String toCode() { 567 switch (this) { 568 case PASS: 569 return "pass"; 570 case SKIP: 571 return "skip"; 572 case FAIL: 573 return "fail"; 574 case WARNING: 575 return "warning"; 576 case ERROR: 577 return "error"; 578 case NULL: 579 return null; 580 default: 581 return "?"; 582 } 583 } 584 585 public String getSystem() { 586 switch (this) { 587 case PASS: 588 return "http://hl7.org/fhir/report-action-result-codes"; 589 case SKIP: 590 return "http://hl7.org/fhir/report-action-result-codes"; 591 case FAIL: 592 return "http://hl7.org/fhir/report-action-result-codes"; 593 case WARNING: 594 return "http://hl7.org/fhir/report-action-result-codes"; 595 case ERROR: 596 return "http://hl7.org/fhir/report-action-result-codes"; 597 case NULL: 598 return null; 599 default: 600 return "?"; 601 } 602 } 603 604 public String getDefinition() { 605 switch (this) { 606 case PASS: 607 return "The action was successful."; 608 case SKIP: 609 return "The action was skipped."; 610 case FAIL: 611 return "The action failed."; 612 case WARNING: 613 return "The action passed but with warnings."; 614 case ERROR: 615 return "The action encountered a fatal error and the engine was unable to process."; 616 case NULL: 617 return null; 618 default: 619 return "?"; 620 } 621 } 622 623 public String getDisplay() { 624 switch (this) { 625 case PASS: 626 return "Pass"; 627 case SKIP: 628 return "Skip"; 629 case FAIL: 630 return "Fail"; 631 case WARNING: 632 return "Warning"; 633 case ERROR: 634 return "Error"; 635 case NULL: 636 return null; 637 default: 638 return "?"; 639 } 640 } 641 } 642 643 public static class TestReportActionResultEnumFactory implements EnumFactory<TestReportActionResult> { 644 public TestReportActionResult fromCode(String codeString) throws IllegalArgumentException { 645 if (codeString == null || "".equals(codeString)) 646 if (codeString == null || "".equals(codeString)) 647 return null; 648 if ("pass".equals(codeString)) 649 return TestReportActionResult.PASS; 650 if ("skip".equals(codeString)) 651 return TestReportActionResult.SKIP; 652 if ("fail".equals(codeString)) 653 return TestReportActionResult.FAIL; 654 if ("warning".equals(codeString)) 655 return TestReportActionResult.WARNING; 656 if ("error".equals(codeString)) 657 return TestReportActionResult.ERROR; 658 throw new IllegalArgumentException("Unknown TestReportActionResult code '" + codeString + "'"); 659 } 660 661 public Enumeration<TestReportActionResult> fromType(PrimitiveType<?> code) throws FHIRException { 662 if (code == null) 663 return null; 664 if (code.isEmpty()) 665 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.NULL, code); 666 String codeString = code.asStringValue(); 667 if (codeString == null || "".equals(codeString)) 668 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.NULL, code); 669 if ("pass".equals(codeString)) 670 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.PASS, code); 671 if ("skip".equals(codeString)) 672 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.SKIP, code); 673 if ("fail".equals(codeString)) 674 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.FAIL, code); 675 if ("warning".equals(codeString)) 676 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.WARNING, code); 677 if ("error".equals(codeString)) 678 return new Enumeration<TestReportActionResult>(this, TestReportActionResult.ERROR, code); 679 throw new FHIRException("Unknown TestReportActionResult code '" + codeString + "'"); 680 } 681 682 public String toCode(TestReportActionResult code) { 683 if (code == TestReportActionResult.NULL) 684 return null; 685 if (code == TestReportActionResult.PASS) 686 return "pass"; 687 if (code == TestReportActionResult.SKIP) 688 return "skip"; 689 if (code == TestReportActionResult.FAIL) 690 return "fail"; 691 if (code == TestReportActionResult.WARNING) 692 return "warning"; 693 if (code == TestReportActionResult.ERROR) 694 return "error"; 695 return "?"; 696 } 697 698 public String toSystem(TestReportActionResult code) { 699 return code.getSystem(); 700 } 701 } 702 703 @Block() 704 public static class TestReportParticipantComponent extends BackboneElement implements IBaseBackboneElement { 705 /** 706 * The type of participant. 707 */ 708 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 709 @Description(shortDefinition = "test-engine | client | server", formalDefinition = "The type of participant.") 710 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/report-participant-type") 711 protected Enumeration<TestReportParticipantType> type; 712 713 /** 714 * The uri of the participant. An absolute URL is preferred. 715 */ 716 @Child(name = "uri", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 717 @Description(shortDefinition = "The uri of the participant. An absolute URL is preferred", formalDefinition = "The uri of the participant. An absolute URL is preferred.") 718 protected UriType uri; 719 720 /** 721 * The display name of the participant. 722 */ 723 @Child(name = "display", type = { 724 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 725 @Description(shortDefinition = "The display name of the participant", formalDefinition = "The display name of the participant.") 726 protected StringType display; 727 728 private static final long serialVersionUID = 577488357L; 729 730 /** 731 * Constructor 732 */ 733 public TestReportParticipantComponent() { 734 super(); 735 } 736 737 /** 738 * Constructor 739 */ 740 public TestReportParticipantComponent(Enumeration<TestReportParticipantType> type, UriType uri) { 741 super(); 742 this.type = type; 743 this.uri = uri; 744 } 745 746 /** 747 * @return {@link #type} (The type of participant.). This is the underlying 748 * object with id, value and extensions. The accessor "getType" gives 749 * direct access to the value 750 */ 751 public Enumeration<TestReportParticipantType> getTypeElement() { 752 if (this.type == null) 753 if (Configuration.errorOnAutoCreate()) 754 throw new Error("Attempt to auto-create TestReportParticipantComponent.type"); 755 else if (Configuration.doAutoCreate()) 756 this.type = new Enumeration<TestReportParticipantType>(new TestReportParticipantTypeEnumFactory()); // bb 757 return this.type; 758 } 759 760 public boolean hasTypeElement() { 761 return this.type != null && !this.type.isEmpty(); 762 } 763 764 public boolean hasType() { 765 return this.type != null && !this.type.isEmpty(); 766 } 767 768 /** 769 * @param value {@link #type} (The type of participant.). This is the underlying 770 * object with id, value and extensions. The accessor "getType" 771 * gives direct access to the value 772 */ 773 public TestReportParticipantComponent setTypeElement(Enumeration<TestReportParticipantType> value) { 774 this.type = value; 775 return this; 776 } 777 778 /** 779 * @return The type of participant. 780 */ 781 public TestReportParticipantType getType() { 782 return this.type == null ? null : this.type.getValue(); 783 } 784 785 /** 786 * @param value The type of participant. 787 */ 788 public TestReportParticipantComponent setType(TestReportParticipantType value) { 789 if (this.type == null) 790 this.type = new Enumeration<TestReportParticipantType>(new TestReportParticipantTypeEnumFactory()); 791 this.type.setValue(value); 792 return this; 793 } 794 795 /** 796 * @return {@link #uri} (The uri of the participant. An absolute URL is 797 * preferred.). This is the underlying object with id, value and 798 * extensions. The accessor "getUri" gives direct access to the value 799 */ 800 public UriType getUriElement() { 801 if (this.uri == null) 802 if (Configuration.errorOnAutoCreate()) 803 throw new Error("Attempt to auto-create TestReportParticipantComponent.uri"); 804 else if (Configuration.doAutoCreate()) 805 this.uri = new UriType(); // bb 806 return this.uri; 807 } 808 809 public boolean hasUriElement() { 810 return this.uri != null && !this.uri.isEmpty(); 811 } 812 813 public boolean hasUri() { 814 return this.uri != null && !this.uri.isEmpty(); 815 } 816 817 /** 818 * @param value {@link #uri} (The uri of the participant. An absolute URL is 819 * preferred.). This is the underlying object with id, value and 820 * extensions. The accessor "getUri" gives direct access to the 821 * value 822 */ 823 public TestReportParticipantComponent setUriElement(UriType value) { 824 this.uri = value; 825 return this; 826 } 827 828 /** 829 * @return The uri of the participant. An absolute URL is preferred. 830 */ 831 public String getUri() { 832 return this.uri == null ? null : this.uri.getValue(); 833 } 834 835 /** 836 * @param value The uri of the participant. An absolute URL is preferred. 837 */ 838 public TestReportParticipantComponent setUri(String value) { 839 if (this.uri == null) 840 this.uri = new UriType(); 841 this.uri.setValue(value); 842 return this; 843 } 844 845 /** 846 * @return {@link #display} (The display name of the participant.). This is the 847 * underlying object with id, value and extensions. The accessor 848 * "getDisplay" gives direct access to the value 849 */ 850 public StringType getDisplayElement() { 851 if (this.display == null) 852 if (Configuration.errorOnAutoCreate()) 853 throw new Error("Attempt to auto-create TestReportParticipantComponent.display"); 854 else if (Configuration.doAutoCreate()) 855 this.display = new StringType(); // bb 856 return this.display; 857 } 858 859 public boolean hasDisplayElement() { 860 return this.display != null && !this.display.isEmpty(); 861 } 862 863 public boolean hasDisplay() { 864 return this.display != null && !this.display.isEmpty(); 865 } 866 867 /** 868 * @param value {@link #display} (The display name of the participant.). This is 869 * the underlying object with id, value and extensions. The 870 * accessor "getDisplay" gives direct access to the value 871 */ 872 public TestReportParticipantComponent setDisplayElement(StringType value) { 873 this.display = value; 874 return this; 875 } 876 877 /** 878 * @return The display name of the participant. 879 */ 880 public String getDisplay() { 881 return this.display == null ? null : this.display.getValue(); 882 } 883 884 /** 885 * @param value The display name of the participant. 886 */ 887 public TestReportParticipantComponent setDisplay(String value) { 888 if (Utilities.noString(value)) 889 this.display = null; 890 else { 891 if (this.display == null) 892 this.display = new StringType(); 893 this.display.setValue(value); 894 } 895 return this; 896 } 897 898 protected void listChildren(List<Property> children) { 899 super.listChildren(children); 900 children.add(new Property("type", "code", "The type of participant.", 0, 1, type)); 901 children.add(new Property("uri", "uri", "The uri of the participant. An absolute URL is preferred.", 0, 1, uri)); 902 children.add(new Property("display", "string", "The display name of the participant.", 0, 1, display)); 903 } 904 905 @Override 906 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 907 switch (_hash) { 908 case 3575610: 909 /* type */ return new Property("type", "code", "The type of participant.", 0, 1, type); 910 case 116076: 911 /* uri */ return new Property("uri", "uri", "The uri of the participant. An absolute URL is preferred.", 0, 1, 912 uri); 913 case 1671764162: 914 /* display */ return new Property("display", "string", "The display name of the participant.", 0, 1, display); 915 default: 916 return super.getNamedProperty(_hash, _name, _checkValid); 917 } 918 919 } 920 921 @Override 922 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 923 switch (hash) { 924 case 3575610: 925 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<TestReportParticipantType> 926 case 116076: 927 /* uri */ return this.uri == null ? new Base[0] : new Base[] { this.uri }; // UriType 928 case 1671764162: 929 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 930 default: 931 return super.getProperty(hash, name, checkValid); 932 } 933 934 } 935 936 @Override 937 public Base setProperty(int hash, String name, Base value) throws FHIRException { 938 switch (hash) { 939 case 3575610: // type 940 value = new TestReportParticipantTypeEnumFactory().fromType(castToCode(value)); 941 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 942 return value; 943 case 116076: // uri 944 this.uri = castToUri(value); // UriType 945 return value; 946 case 1671764162: // display 947 this.display = castToString(value); // StringType 948 return value; 949 default: 950 return super.setProperty(hash, name, value); 951 } 952 953 } 954 955 @Override 956 public Base setProperty(String name, Base value) throws FHIRException { 957 if (name.equals("type")) { 958 value = new TestReportParticipantTypeEnumFactory().fromType(castToCode(value)); 959 this.type = (Enumeration) value; // Enumeration<TestReportParticipantType> 960 } else if (name.equals("uri")) { 961 this.uri = castToUri(value); // UriType 962 } else if (name.equals("display")) { 963 this.display = castToString(value); // StringType 964 } else 965 return super.setProperty(name, value); 966 return value; 967 } 968 969 @Override 970 public void removeChild(String name, Base value) throws FHIRException { 971 if (name.equals("type")) { 972 this.type = null; 973 } else if (name.equals("uri")) { 974 this.uri = null; 975 } else if (name.equals("display")) { 976 this.display = null; 977 } else 978 super.removeChild(name, value); 979 980 } 981 982 @Override 983 public Base makeProperty(int hash, String name) throws FHIRException { 984 switch (hash) { 985 case 3575610: 986 return getTypeElement(); 987 case 116076: 988 return getUriElement(); 989 case 1671764162: 990 return getDisplayElement(); 991 default: 992 return super.makeProperty(hash, name); 993 } 994 995 } 996 997 @Override 998 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 999 switch (hash) { 1000 case 3575610: 1001 /* type */ return new String[] { "code" }; 1002 case 116076: 1003 /* uri */ return new String[] { "uri" }; 1004 case 1671764162: 1005 /* display */ return new String[] { "string" }; 1006 default: 1007 return super.getTypesForProperty(hash, name); 1008 } 1009 1010 } 1011 1012 @Override 1013 public Base addChild(String name) throws FHIRException { 1014 if (name.equals("type")) { 1015 throw new FHIRException("Cannot call addChild on a singleton property TestReport.type"); 1016 } else if (name.equals("uri")) { 1017 throw new FHIRException("Cannot call addChild on a singleton property TestReport.uri"); 1018 } else if (name.equals("display")) { 1019 throw new FHIRException("Cannot call addChild on a singleton property TestReport.display"); 1020 } else 1021 return super.addChild(name); 1022 } 1023 1024 public TestReportParticipantComponent copy() { 1025 TestReportParticipantComponent dst = new TestReportParticipantComponent(); 1026 copyValues(dst); 1027 return dst; 1028 } 1029 1030 public void copyValues(TestReportParticipantComponent dst) { 1031 super.copyValues(dst); 1032 dst.type = type == null ? null : type.copy(); 1033 dst.uri = uri == null ? null : uri.copy(); 1034 dst.display = display == null ? null : display.copy(); 1035 } 1036 1037 @Override 1038 public boolean equalsDeep(Base other_) { 1039 if (!super.equalsDeep(other_)) 1040 return false; 1041 if (!(other_ instanceof TestReportParticipantComponent)) 1042 return false; 1043 TestReportParticipantComponent o = (TestReportParticipantComponent) other_; 1044 return compareDeep(type, o.type, true) && compareDeep(uri, o.uri, true) && compareDeep(display, o.display, true); 1045 } 1046 1047 @Override 1048 public boolean equalsShallow(Base other_) { 1049 if (!super.equalsShallow(other_)) 1050 return false; 1051 if (!(other_ instanceof TestReportParticipantComponent)) 1052 return false; 1053 TestReportParticipantComponent o = (TestReportParticipantComponent) other_; 1054 return compareValues(type, o.type, true) && compareValues(uri, o.uri, true) 1055 && compareValues(display, o.display, true); 1056 } 1057 1058 public boolean isEmpty() { 1059 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, uri, display); 1060 } 1061 1062 public String fhirType() { 1063 return "TestReport.participant"; 1064 1065 } 1066 1067 } 1068 1069 @Block() 1070 public static class TestReportSetupComponent extends BackboneElement implements IBaseBackboneElement { 1071 /** 1072 * Action would contain either an operation or an assertion. 1073 */ 1074 @Child(name = "action", type = {}, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1075 @Description(shortDefinition = "A setup operation or assert that was executed", formalDefinition = "Action would contain either an operation or an assertion.") 1076 protected List<SetupActionComponent> action; 1077 1078 private static final long serialVersionUID = -123374486L; 1079 1080 /** 1081 * Constructor 1082 */ 1083 public TestReportSetupComponent() { 1084 super(); 1085 } 1086 1087 /** 1088 * @return {@link #action} (Action would contain either an operation or an 1089 * assertion.) 1090 */ 1091 public List<SetupActionComponent> getAction() { 1092 if (this.action == null) 1093 this.action = new ArrayList<SetupActionComponent>(); 1094 return this.action; 1095 } 1096 1097 /** 1098 * @return Returns a reference to <code>this</code> for easy method chaining 1099 */ 1100 public TestReportSetupComponent setAction(List<SetupActionComponent> theAction) { 1101 this.action = theAction; 1102 return this; 1103 } 1104 1105 public boolean hasAction() { 1106 if (this.action == null) 1107 return false; 1108 for (SetupActionComponent item : this.action) 1109 if (!item.isEmpty()) 1110 return true; 1111 return false; 1112 } 1113 1114 public SetupActionComponent addAction() { // 3 1115 SetupActionComponent t = new SetupActionComponent(); 1116 if (this.action == null) 1117 this.action = new ArrayList<SetupActionComponent>(); 1118 this.action.add(t); 1119 return t; 1120 } 1121 1122 public TestReportSetupComponent addAction(SetupActionComponent t) { // 3 1123 if (t == null) 1124 return this; 1125 if (this.action == null) 1126 this.action = new ArrayList<SetupActionComponent>(); 1127 this.action.add(t); 1128 return this; 1129 } 1130 1131 /** 1132 * @return The first repetition of repeating field {@link #action}, creating it 1133 * if it does not already exist 1134 */ 1135 public SetupActionComponent getActionFirstRep() { 1136 if (getAction().isEmpty()) { 1137 addAction(); 1138 } 1139 return getAction().get(0); 1140 } 1141 1142 protected void listChildren(List<Property> children) { 1143 super.listChildren(children); 1144 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, 1145 java.lang.Integer.MAX_VALUE, action)); 1146 } 1147 1148 @Override 1149 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1150 switch (_hash) { 1151 case -1422950858: 1152 /* action */ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, 1153 java.lang.Integer.MAX_VALUE, action); 1154 default: 1155 return super.getNamedProperty(_hash, _name, _checkValid); 1156 } 1157 1158 } 1159 1160 @Override 1161 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1162 switch (hash) { 1163 case -1422950858: 1164 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // SetupActionComponent 1165 default: 1166 return super.getProperty(hash, name, checkValid); 1167 } 1168 1169 } 1170 1171 @Override 1172 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1173 switch (hash) { 1174 case -1422950858: // action 1175 this.getAction().add((SetupActionComponent) value); // SetupActionComponent 1176 return value; 1177 default: 1178 return super.setProperty(hash, name, value); 1179 } 1180 1181 } 1182 1183 @Override 1184 public Base setProperty(String name, Base value) throws FHIRException { 1185 if (name.equals("action")) { 1186 this.getAction().add((SetupActionComponent) value); 1187 } else 1188 return super.setProperty(name, value); 1189 return value; 1190 } 1191 1192 @Override 1193 public void removeChild(String name, Base value) throws FHIRException { 1194 if (name.equals("action")) { 1195 this.getAction().remove((SetupActionComponent) value); 1196 } else 1197 super.removeChild(name, value); 1198 1199 } 1200 1201 @Override 1202 public Base makeProperty(int hash, String name) throws FHIRException { 1203 switch (hash) { 1204 case -1422950858: 1205 return addAction(); 1206 default: 1207 return super.makeProperty(hash, name); 1208 } 1209 1210 } 1211 1212 @Override 1213 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1214 switch (hash) { 1215 case -1422950858: 1216 /* action */ return new String[] {}; 1217 default: 1218 return super.getTypesForProperty(hash, name); 1219 } 1220 1221 } 1222 1223 @Override 1224 public Base addChild(String name) throws FHIRException { 1225 if (name.equals("action")) { 1226 return addAction(); 1227 } else 1228 return super.addChild(name); 1229 } 1230 1231 public TestReportSetupComponent copy() { 1232 TestReportSetupComponent dst = new TestReportSetupComponent(); 1233 copyValues(dst); 1234 return dst; 1235 } 1236 1237 public void copyValues(TestReportSetupComponent dst) { 1238 super.copyValues(dst); 1239 if (action != null) { 1240 dst.action = new ArrayList<SetupActionComponent>(); 1241 for (SetupActionComponent i : action) 1242 dst.action.add(i.copy()); 1243 } 1244 ; 1245 } 1246 1247 @Override 1248 public boolean equalsDeep(Base other_) { 1249 if (!super.equalsDeep(other_)) 1250 return false; 1251 if (!(other_ instanceof TestReportSetupComponent)) 1252 return false; 1253 TestReportSetupComponent o = (TestReportSetupComponent) other_; 1254 return compareDeep(action, o.action, true); 1255 } 1256 1257 @Override 1258 public boolean equalsShallow(Base other_) { 1259 if (!super.equalsShallow(other_)) 1260 return false; 1261 if (!(other_ instanceof TestReportSetupComponent)) 1262 return false; 1263 TestReportSetupComponent o = (TestReportSetupComponent) other_; 1264 return true; 1265 } 1266 1267 public boolean isEmpty() { 1268 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 1269 } 1270 1271 public String fhirType() { 1272 return "TestReport.setup"; 1273 1274 } 1275 1276 } 1277 1278 @Block() 1279 public static class SetupActionComponent extends BackboneElement implements IBaseBackboneElement { 1280 /** 1281 * The operation performed. 1282 */ 1283 @Child(name = "operation", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = false) 1284 @Description(shortDefinition = "The operation to perform", formalDefinition = "The operation performed.") 1285 protected SetupActionOperationComponent operation; 1286 1287 /** 1288 * The results of the assertion performed on the previous operations. 1289 */ 1290 @Child(name = "assert", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = false) 1291 @Description(shortDefinition = "The assertion to perform", formalDefinition = "The results of the assertion performed on the previous operations.") 1292 protected SetupActionAssertComponent assert_; 1293 1294 private static final long serialVersionUID = -252088305L; 1295 1296 /** 1297 * Constructor 1298 */ 1299 public SetupActionComponent() { 1300 super(); 1301 } 1302 1303 /** 1304 * @return {@link #operation} (The operation performed.) 1305 */ 1306 public SetupActionOperationComponent getOperation() { 1307 if (this.operation == null) 1308 if (Configuration.errorOnAutoCreate()) 1309 throw new Error("Attempt to auto-create SetupActionComponent.operation"); 1310 else if (Configuration.doAutoCreate()) 1311 this.operation = new SetupActionOperationComponent(); // cc 1312 return this.operation; 1313 } 1314 1315 public boolean hasOperation() { 1316 return this.operation != null && !this.operation.isEmpty(); 1317 } 1318 1319 /** 1320 * @param value {@link #operation} (The operation performed.) 1321 */ 1322 public SetupActionComponent setOperation(SetupActionOperationComponent value) { 1323 this.operation = value; 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #assert_} (The results of the assertion performed on the 1329 * previous operations.) 1330 */ 1331 public SetupActionAssertComponent getAssert() { 1332 if (this.assert_ == null) 1333 if (Configuration.errorOnAutoCreate()) 1334 throw new Error("Attempt to auto-create SetupActionComponent.assert_"); 1335 else if (Configuration.doAutoCreate()) 1336 this.assert_ = new SetupActionAssertComponent(); // cc 1337 return this.assert_; 1338 } 1339 1340 public boolean hasAssert() { 1341 return this.assert_ != null && !this.assert_.isEmpty(); 1342 } 1343 1344 /** 1345 * @param value {@link #assert_} (The results of the assertion performed on the 1346 * previous operations.) 1347 */ 1348 public SetupActionComponent setAssert(SetupActionAssertComponent value) { 1349 this.assert_ = value; 1350 return this; 1351 } 1352 1353 protected void listChildren(List<Property> children) { 1354 super.listChildren(children); 1355 children.add(new Property("operation", "", "The operation performed.", 0, 1, operation)); 1356 children.add(new Property("assert", "", "The results of the assertion performed on the previous operations.", 0, 1357 1, assert_)); 1358 } 1359 1360 @Override 1361 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1362 switch (_hash) { 1363 case 1662702951: 1364 /* operation */ return new Property("operation", "", "The operation performed.", 0, 1, operation); 1365 case -1408208058: 1366 /* assert */ return new Property("assert", "", 1367 "The results of the assertion performed on the previous operations.", 0, 1, assert_); 1368 default: 1369 return super.getNamedProperty(_hash, _name, _checkValid); 1370 } 1371 1372 } 1373 1374 @Override 1375 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1376 switch (hash) { 1377 case 1662702951: 1378 /* operation */ return this.operation == null ? new Base[0] : new Base[] { this.operation }; // SetupActionOperationComponent 1379 case -1408208058: 1380 /* assert */ return this.assert_ == null ? new Base[0] : new Base[] { this.assert_ }; // SetupActionAssertComponent 1381 default: 1382 return super.getProperty(hash, name, checkValid); 1383 } 1384 1385 } 1386 1387 @Override 1388 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1389 switch (hash) { 1390 case 1662702951: // operation 1391 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1392 return value; 1393 case -1408208058: // assert 1394 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1395 return value; 1396 default: 1397 return super.setProperty(hash, name, value); 1398 } 1399 1400 } 1401 1402 @Override 1403 public Base setProperty(String name, Base value) throws FHIRException { 1404 if (name.equals("operation")) { 1405 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1406 } else if (name.equals("assert")) { 1407 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1408 } else 1409 return super.setProperty(name, value); 1410 return value; 1411 } 1412 1413 @Override 1414 public void removeChild(String name, Base value) throws FHIRException { 1415 if (name.equals("operation")) { 1416 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 1417 } else if (name.equals("assert")) { 1418 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 1419 } else 1420 super.removeChild(name, value); 1421 1422 } 1423 1424 @Override 1425 public Base makeProperty(int hash, String name) throws FHIRException { 1426 switch (hash) { 1427 case 1662702951: 1428 return getOperation(); 1429 case -1408208058: 1430 return getAssert(); 1431 default: 1432 return super.makeProperty(hash, name); 1433 } 1434 1435 } 1436 1437 @Override 1438 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1439 switch (hash) { 1440 case 1662702951: 1441 /* operation */ return new String[] {}; 1442 case -1408208058: 1443 /* assert */ return new String[] {}; 1444 default: 1445 return super.getTypesForProperty(hash, name); 1446 } 1447 1448 } 1449 1450 @Override 1451 public Base addChild(String name) throws FHIRException { 1452 if (name.equals("operation")) { 1453 this.operation = new SetupActionOperationComponent(); 1454 return this.operation; 1455 } else if (name.equals("assert")) { 1456 this.assert_ = new SetupActionAssertComponent(); 1457 return this.assert_; 1458 } else 1459 return super.addChild(name); 1460 } 1461 1462 public SetupActionComponent copy() { 1463 SetupActionComponent dst = new SetupActionComponent(); 1464 copyValues(dst); 1465 return dst; 1466 } 1467 1468 public void copyValues(SetupActionComponent dst) { 1469 super.copyValues(dst); 1470 dst.operation = operation == null ? null : operation.copy(); 1471 dst.assert_ = assert_ == null ? null : assert_.copy(); 1472 } 1473 1474 @Override 1475 public boolean equalsDeep(Base other_) { 1476 if (!super.equalsDeep(other_)) 1477 return false; 1478 if (!(other_ instanceof SetupActionComponent)) 1479 return false; 1480 SetupActionComponent o = (SetupActionComponent) other_; 1481 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 1482 } 1483 1484 @Override 1485 public boolean equalsShallow(Base other_) { 1486 if (!super.equalsShallow(other_)) 1487 return false; 1488 if (!(other_ instanceof SetupActionComponent)) 1489 return false; 1490 SetupActionComponent o = (SetupActionComponent) other_; 1491 return true; 1492 } 1493 1494 public boolean isEmpty() { 1495 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 1496 } 1497 1498 public String fhirType() { 1499 return "TestReport.setup.action"; 1500 1501 } 1502 1503 } 1504 1505 @Block() 1506 public static class SetupActionOperationComponent extends BackboneElement implements IBaseBackboneElement { 1507 /** 1508 * The result of this operation. 1509 */ 1510 @Child(name = "result", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1511 @Description(shortDefinition = "pass | skip | fail | warning | error", formalDefinition = "The result of this operation.") 1512 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/report-action-result-codes") 1513 protected Enumeration<TestReportActionResult> result; 1514 1515 /** 1516 * An explanatory message associated with the result. 1517 */ 1518 @Child(name = "message", type = { 1519 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1520 @Description(shortDefinition = "A message associated with the result", formalDefinition = "An explanatory message associated with the result.") 1521 protected MarkdownType message; 1522 1523 /** 1524 * A link to further details on the result. 1525 */ 1526 @Child(name = "detail", type = { UriType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1527 @Description(shortDefinition = "A link to further details on the result", formalDefinition = "A link to further details on the result.") 1528 protected UriType detail; 1529 1530 private static final long serialVersionUID = 269088798L; 1531 1532 /** 1533 * Constructor 1534 */ 1535 public SetupActionOperationComponent() { 1536 super(); 1537 } 1538 1539 /** 1540 * Constructor 1541 */ 1542 public SetupActionOperationComponent(Enumeration<TestReportActionResult> result) { 1543 super(); 1544 this.result = result; 1545 } 1546 1547 /** 1548 * @return {@link #result} (The result of this operation.). This is the 1549 * underlying object with id, value and extensions. The accessor 1550 * "getResult" gives direct access to the value 1551 */ 1552 public Enumeration<TestReportActionResult> getResultElement() { 1553 if (this.result == null) 1554 if (Configuration.errorOnAutoCreate()) 1555 throw new Error("Attempt to auto-create SetupActionOperationComponent.result"); 1556 else if (Configuration.doAutoCreate()) 1557 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); // bb 1558 return this.result; 1559 } 1560 1561 public boolean hasResultElement() { 1562 return this.result != null && !this.result.isEmpty(); 1563 } 1564 1565 public boolean hasResult() { 1566 return this.result != null && !this.result.isEmpty(); 1567 } 1568 1569 /** 1570 * @param value {@link #result} (The result of this operation.). This is the 1571 * underlying object with id, value and extensions. The accessor 1572 * "getResult" gives direct access to the value 1573 */ 1574 public SetupActionOperationComponent setResultElement(Enumeration<TestReportActionResult> value) { 1575 this.result = value; 1576 return this; 1577 } 1578 1579 /** 1580 * @return The result of this operation. 1581 */ 1582 public TestReportActionResult getResult() { 1583 return this.result == null ? null : this.result.getValue(); 1584 } 1585 1586 /** 1587 * @param value The result of this operation. 1588 */ 1589 public SetupActionOperationComponent setResult(TestReportActionResult value) { 1590 if (this.result == null) 1591 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); 1592 this.result.setValue(value); 1593 return this; 1594 } 1595 1596 /** 1597 * @return {@link #message} (An explanatory message associated with the 1598 * result.). This is the underlying object with id, value and 1599 * extensions. The accessor "getMessage" gives direct access to the 1600 * value 1601 */ 1602 public MarkdownType getMessageElement() { 1603 if (this.message == null) 1604 if (Configuration.errorOnAutoCreate()) 1605 throw new Error("Attempt to auto-create SetupActionOperationComponent.message"); 1606 else if (Configuration.doAutoCreate()) 1607 this.message = new MarkdownType(); // bb 1608 return this.message; 1609 } 1610 1611 public boolean hasMessageElement() { 1612 return this.message != null && !this.message.isEmpty(); 1613 } 1614 1615 public boolean hasMessage() { 1616 return this.message != null && !this.message.isEmpty(); 1617 } 1618 1619 /** 1620 * @param value {@link #message} (An explanatory message associated with the 1621 * result.). This is the underlying object with id, value and 1622 * extensions. The accessor "getMessage" gives direct access to the 1623 * value 1624 */ 1625 public SetupActionOperationComponent setMessageElement(MarkdownType value) { 1626 this.message = value; 1627 return this; 1628 } 1629 1630 /** 1631 * @return An explanatory message associated with the result. 1632 */ 1633 public String getMessage() { 1634 return this.message == null ? null : this.message.getValue(); 1635 } 1636 1637 /** 1638 * @param value An explanatory message associated with the result. 1639 */ 1640 public SetupActionOperationComponent setMessage(String value) { 1641 if (value == null) 1642 this.message = null; 1643 else { 1644 if (this.message == null) 1645 this.message = new MarkdownType(); 1646 this.message.setValue(value); 1647 } 1648 return this; 1649 } 1650 1651 /** 1652 * @return {@link #detail} (A link to further details on the result.). This is 1653 * the underlying object with id, value and extensions. The accessor 1654 * "getDetail" gives direct access to the value 1655 */ 1656 public UriType getDetailElement() { 1657 if (this.detail == null) 1658 if (Configuration.errorOnAutoCreate()) 1659 throw new Error("Attempt to auto-create SetupActionOperationComponent.detail"); 1660 else if (Configuration.doAutoCreate()) 1661 this.detail = new UriType(); // bb 1662 return this.detail; 1663 } 1664 1665 public boolean hasDetailElement() { 1666 return this.detail != null && !this.detail.isEmpty(); 1667 } 1668 1669 public boolean hasDetail() { 1670 return this.detail != null && !this.detail.isEmpty(); 1671 } 1672 1673 /** 1674 * @param value {@link #detail} (A link to further details on the result.). This 1675 * is the underlying object with id, value and extensions. The 1676 * accessor "getDetail" gives direct access to the value 1677 */ 1678 public SetupActionOperationComponent setDetailElement(UriType value) { 1679 this.detail = value; 1680 return this; 1681 } 1682 1683 /** 1684 * @return A link to further details on the result. 1685 */ 1686 public String getDetail() { 1687 return this.detail == null ? null : this.detail.getValue(); 1688 } 1689 1690 /** 1691 * @param value A link to further details on the result. 1692 */ 1693 public SetupActionOperationComponent setDetail(String value) { 1694 if (Utilities.noString(value)) 1695 this.detail = null; 1696 else { 1697 if (this.detail == null) 1698 this.detail = new UriType(); 1699 this.detail.setValue(value); 1700 } 1701 return this; 1702 } 1703 1704 protected void listChildren(List<Property> children) { 1705 super.listChildren(children); 1706 children.add(new Property("result", "code", "The result of this operation.", 0, 1, result)); 1707 children.add( 1708 new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message)); 1709 children.add(new Property("detail", "uri", "A link to further details on the result.", 0, 1, detail)); 1710 } 1711 1712 @Override 1713 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1714 switch (_hash) { 1715 case -934426595: 1716 /* result */ return new Property("result", "code", "The result of this operation.", 0, 1, result); 1717 case 954925063: 1718 /* message */ return new Property("message", "markdown", "An explanatory message associated with the result.", 1719 0, 1, message); 1720 case -1335224239: 1721 /* detail */ return new Property("detail", "uri", "A link to further details on the result.", 0, 1, detail); 1722 default: 1723 return super.getNamedProperty(_hash, _name, _checkValid); 1724 } 1725 1726 } 1727 1728 @Override 1729 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1730 switch (hash) { 1731 case -934426595: 1732 /* result */ return this.result == null ? new Base[0] : new Base[] { this.result }; // Enumeration<TestReportActionResult> 1733 case 954925063: 1734 /* message */ return this.message == null ? new Base[0] : new Base[] { this.message }; // MarkdownType 1735 case -1335224239: 1736 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // UriType 1737 default: 1738 return super.getProperty(hash, name, checkValid); 1739 } 1740 1741 } 1742 1743 @Override 1744 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1745 switch (hash) { 1746 case -934426595: // result 1747 value = new TestReportActionResultEnumFactory().fromType(castToCode(value)); 1748 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1749 return value; 1750 case 954925063: // message 1751 this.message = castToMarkdown(value); // MarkdownType 1752 return value; 1753 case -1335224239: // detail 1754 this.detail = castToUri(value); // UriType 1755 return value; 1756 default: 1757 return super.setProperty(hash, name, value); 1758 } 1759 1760 } 1761 1762 @Override 1763 public Base setProperty(String name, Base value) throws FHIRException { 1764 if (name.equals("result")) { 1765 value = new TestReportActionResultEnumFactory().fromType(castToCode(value)); 1766 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 1767 } else if (name.equals("message")) { 1768 this.message = castToMarkdown(value); // MarkdownType 1769 } else if (name.equals("detail")) { 1770 this.detail = castToUri(value); // UriType 1771 } else 1772 return super.setProperty(name, value); 1773 return value; 1774 } 1775 1776 @Override 1777 public void removeChild(String name, Base value) throws FHIRException { 1778 if (name.equals("result")) { 1779 this.result = null; 1780 } else if (name.equals("message")) { 1781 this.message = null; 1782 } else if (name.equals("detail")) { 1783 this.detail = null; 1784 } else 1785 super.removeChild(name, value); 1786 1787 } 1788 1789 @Override 1790 public Base makeProperty(int hash, String name) throws FHIRException { 1791 switch (hash) { 1792 case -934426595: 1793 return getResultElement(); 1794 case 954925063: 1795 return getMessageElement(); 1796 case -1335224239: 1797 return getDetailElement(); 1798 default: 1799 return super.makeProperty(hash, name); 1800 } 1801 1802 } 1803 1804 @Override 1805 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1806 switch (hash) { 1807 case -934426595: 1808 /* result */ return new String[] { "code" }; 1809 case 954925063: 1810 /* message */ return new String[] { "markdown" }; 1811 case -1335224239: 1812 /* detail */ return new String[] { "uri" }; 1813 default: 1814 return super.getTypesForProperty(hash, name); 1815 } 1816 1817 } 1818 1819 @Override 1820 public Base addChild(String name) throws FHIRException { 1821 if (name.equals("result")) { 1822 throw new FHIRException("Cannot call addChild on a singleton property TestReport.result"); 1823 } else if (name.equals("message")) { 1824 throw new FHIRException("Cannot call addChild on a singleton property TestReport.message"); 1825 } else if (name.equals("detail")) { 1826 throw new FHIRException("Cannot call addChild on a singleton property TestReport.detail"); 1827 } else 1828 return super.addChild(name); 1829 } 1830 1831 public SetupActionOperationComponent copy() { 1832 SetupActionOperationComponent dst = new SetupActionOperationComponent(); 1833 copyValues(dst); 1834 return dst; 1835 } 1836 1837 public void copyValues(SetupActionOperationComponent dst) { 1838 super.copyValues(dst); 1839 dst.result = result == null ? null : result.copy(); 1840 dst.message = message == null ? null : message.copy(); 1841 dst.detail = detail == null ? null : detail.copy(); 1842 } 1843 1844 @Override 1845 public boolean equalsDeep(Base other_) { 1846 if (!super.equalsDeep(other_)) 1847 return false; 1848 if (!(other_ instanceof SetupActionOperationComponent)) 1849 return false; 1850 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 1851 return compareDeep(result, o.result, true) && compareDeep(message, o.message, true) 1852 && compareDeep(detail, o.detail, true); 1853 } 1854 1855 @Override 1856 public boolean equalsShallow(Base other_) { 1857 if (!super.equalsShallow(other_)) 1858 return false; 1859 if (!(other_ instanceof SetupActionOperationComponent)) 1860 return false; 1861 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 1862 return compareValues(result, o.result, true) && compareValues(message, o.message, true) 1863 && compareValues(detail, o.detail, true); 1864 } 1865 1866 public boolean isEmpty() { 1867 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(result, message, detail); 1868 } 1869 1870 public String fhirType() { 1871 return "TestReport.setup.action.operation"; 1872 1873 } 1874 1875 } 1876 1877 @Block() 1878 public static class SetupActionAssertComponent extends BackboneElement implements IBaseBackboneElement { 1879 /** 1880 * The result of this assertion. 1881 */ 1882 @Child(name = "result", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1883 @Description(shortDefinition = "pass | skip | fail | warning | error", formalDefinition = "The result of this assertion.") 1884 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/report-action-result-codes") 1885 protected Enumeration<TestReportActionResult> result; 1886 1887 /** 1888 * An explanatory message associated with the result. 1889 */ 1890 @Child(name = "message", type = { 1891 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1892 @Description(shortDefinition = "A message associated with the result", formalDefinition = "An explanatory message associated with the result.") 1893 protected MarkdownType message; 1894 1895 /** 1896 * A link to further details on the result. 1897 */ 1898 @Child(name = "detail", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1899 @Description(shortDefinition = "A link to further details on the result", formalDefinition = "A link to further details on the result.") 1900 protected StringType detail; 1901 1902 private static final long serialVersionUID = 467968193L; 1903 1904 /** 1905 * Constructor 1906 */ 1907 public SetupActionAssertComponent() { 1908 super(); 1909 } 1910 1911 /** 1912 * Constructor 1913 */ 1914 public SetupActionAssertComponent(Enumeration<TestReportActionResult> result) { 1915 super(); 1916 this.result = result; 1917 } 1918 1919 /** 1920 * @return {@link #result} (The result of this assertion.). This is the 1921 * underlying object with id, value and extensions. The accessor 1922 * "getResult" gives direct access to the value 1923 */ 1924 public Enumeration<TestReportActionResult> getResultElement() { 1925 if (this.result == null) 1926 if (Configuration.errorOnAutoCreate()) 1927 throw new Error("Attempt to auto-create SetupActionAssertComponent.result"); 1928 else if (Configuration.doAutoCreate()) 1929 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); // bb 1930 return this.result; 1931 } 1932 1933 public boolean hasResultElement() { 1934 return this.result != null && !this.result.isEmpty(); 1935 } 1936 1937 public boolean hasResult() { 1938 return this.result != null && !this.result.isEmpty(); 1939 } 1940 1941 /** 1942 * @param value {@link #result} (The result of this assertion.). This is the 1943 * underlying object with id, value and extensions. The accessor 1944 * "getResult" gives direct access to the value 1945 */ 1946 public SetupActionAssertComponent setResultElement(Enumeration<TestReportActionResult> value) { 1947 this.result = value; 1948 return this; 1949 } 1950 1951 /** 1952 * @return The result of this assertion. 1953 */ 1954 public TestReportActionResult getResult() { 1955 return this.result == null ? null : this.result.getValue(); 1956 } 1957 1958 /** 1959 * @param value The result of this assertion. 1960 */ 1961 public SetupActionAssertComponent setResult(TestReportActionResult value) { 1962 if (this.result == null) 1963 this.result = new Enumeration<TestReportActionResult>(new TestReportActionResultEnumFactory()); 1964 this.result.setValue(value); 1965 return this; 1966 } 1967 1968 /** 1969 * @return {@link #message} (An explanatory message associated with the 1970 * result.). This is the underlying object with id, value and 1971 * extensions. The accessor "getMessage" gives direct access to the 1972 * value 1973 */ 1974 public MarkdownType getMessageElement() { 1975 if (this.message == null) 1976 if (Configuration.errorOnAutoCreate()) 1977 throw new Error("Attempt to auto-create SetupActionAssertComponent.message"); 1978 else if (Configuration.doAutoCreate()) 1979 this.message = new MarkdownType(); // bb 1980 return this.message; 1981 } 1982 1983 public boolean hasMessageElement() { 1984 return this.message != null && !this.message.isEmpty(); 1985 } 1986 1987 public boolean hasMessage() { 1988 return this.message != null && !this.message.isEmpty(); 1989 } 1990 1991 /** 1992 * @param value {@link #message} (An explanatory message associated with the 1993 * result.). This is the underlying object with id, value and 1994 * extensions. The accessor "getMessage" gives direct access to the 1995 * value 1996 */ 1997 public SetupActionAssertComponent setMessageElement(MarkdownType value) { 1998 this.message = value; 1999 return this; 2000 } 2001 2002 /** 2003 * @return An explanatory message associated with the result. 2004 */ 2005 public String getMessage() { 2006 return this.message == null ? null : this.message.getValue(); 2007 } 2008 2009 /** 2010 * @param value An explanatory message associated with the result. 2011 */ 2012 public SetupActionAssertComponent setMessage(String value) { 2013 if (value == null) 2014 this.message = null; 2015 else { 2016 if (this.message == null) 2017 this.message = new MarkdownType(); 2018 this.message.setValue(value); 2019 } 2020 return this; 2021 } 2022 2023 /** 2024 * @return {@link #detail} (A link to further details on the result.). This is 2025 * the underlying object with id, value and extensions. The accessor 2026 * "getDetail" gives direct access to the value 2027 */ 2028 public StringType getDetailElement() { 2029 if (this.detail == null) 2030 if (Configuration.errorOnAutoCreate()) 2031 throw new Error("Attempt to auto-create SetupActionAssertComponent.detail"); 2032 else if (Configuration.doAutoCreate()) 2033 this.detail = new StringType(); // bb 2034 return this.detail; 2035 } 2036 2037 public boolean hasDetailElement() { 2038 return this.detail != null && !this.detail.isEmpty(); 2039 } 2040 2041 public boolean hasDetail() { 2042 return this.detail != null && !this.detail.isEmpty(); 2043 } 2044 2045 /** 2046 * @param value {@link #detail} (A link to further details on the result.). This 2047 * is the underlying object with id, value and extensions. The 2048 * accessor "getDetail" gives direct access to the value 2049 */ 2050 public SetupActionAssertComponent setDetailElement(StringType value) { 2051 this.detail = value; 2052 return this; 2053 } 2054 2055 /** 2056 * @return A link to further details on the result. 2057 */ 2058 public String getDetail() { 2059 return this.detail == null ? null : this.detail.getValue(); 2060 } 2061 2062 /** 2063 * @param value A link to further details on the result. 2064 */ 2065 public SetupActionAssertComponent setDetail(String value) { 2066 if (Utilities.noString(value)) 2067 this.detail = null; 2068 else { 2069 if (this.detail == null) 2070 this.detail = new StringType(); 2071 this.detail.setValue(value); 2072 } 2073 return this; 2074 } 2075 2076 protected void listChildren(List<Property> children) { 2077 super.listChildren(children); 2078 children.add(new Property("result", "code", "The result of this assertion.", 0, 1, result)); 2079 children.add( 2080 new Property("message", "markdown", "An explanatory message associated with the result.", 0, 1, message)); 2081 children.add(new Property("detail", "string", "A link to further details on the result.", 0, 1, detail)); 2082 } 2083 2084 @Override 2085 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2086 switch (_hash) { 2087 case -934426595: 2088 /* result */ return new Property("result", "code", "The result of this assertion.", 0, 1, result); 2089 case 954925063: 2090 /* message */ return new Property("message", "markdown", "An explanatory message associated with the result.", 2091 0, 1, message); 2092 case -1335224239: 2093 /* detail */ return new Property("detail", "string", "A link to further details on the result.", 0, 1, detail); 2094 default: 2095 return super.getNamedProperty(_hash, _name, _checkValid); 2096 } 2097 2098 } 2099 2100 @Override 2101 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2102 switch (hash) { 2103 case -934426595: 2104 /* result */ return this.result == null ? new Base[0] : new Base[] { this.result }; // Enumeration<TestReportActionResult> 2105 case 954925063: 2106 /* message */ return this.message == null ? new Base[0] : new Base[] { this.message }; // MarkdownType 2107 case -1335224239: 2108 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // StringType 2109 default: 2110 return super.getProperty(hash, name, checkValid); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2117 switch (hash) { 2118 case -934426595: // result 2119 value = new TestReportActionResultEnumFactory().fromType(castToCode(value)); 2120 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 2121 return value; 2122 case 954925063: // message 2123 this.message = castToMarkdown(value); // MarkdownType 2124 return value; 2125 case -1335224239: // detail 2126 this.detail = castToString(value); // StringType 2127 return value; 2128 default: 2129 return super.setProperty(hash, name, value); 2130 } 2131 2132 } 2133 2134 @Override 2135 public Base setProperty(String name, Base value) throws FHIRException { 2136 if (name.equals("result")) { 2137 value = new TestReportActionResultEnumFactory().fromType(castToCode(value)); 2138 this.result = (Enumeration) value; // Enumeration<TestReportActionResult> 2139 } else if (name.equals("message")) { 2140 this.message = castToMarkdown(value); // MarkdownType 2141 } else if (name.equals("detail")) { 2142 this.detail = castToString(value); // StringType 2143 } else 2144 return super.setProperty(name, value); 2145 return value; 2146 } 2147 2148 @Override 2149 public void removeChild(String name, Base value) throws FHIRException { 2150 if (name.equals("result")) { 2151 this.result = null; 2152 } else if (name.equals("message")) { 2153 this.message = null; 2154 } else if (name.equals("detail")) { 2155 this.detail = null; 2156 } else 2157 super.removeChild(name, value); 2158 2159 } 2160 2161 @Override 2162 public Base makeProperty(int hash, String name) throws FHIRException { 2163 switch (hash) { 2164 case -934426595: 2165 return getResultElement(); 2166 case 954925063: 2167 return getMessageElement(); 2168 case -1335224239: 2169 return getDetailElement(); 2170 default: 2171 return super.makeProperty(hash, name); 2172 } 2173 2174 } 2175 2176 @Override 2177 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2178 switch (hash) { 2179 case -934426595: 2180 /* result */ return new String[] { "code" }; 2181 case 954925063: 2182 /* message */ return new String[] { "markdown" }; 2183 case -1335224239: 2184 /* detail */ return new String[] { "string" }; 2185 default: 2186 return super.getTypesForProperty(hash, name); 2187 } 2188 2189 } 2190 2191 @Override 2192 public Base addChild(String name) throws FHIRException { 2193 if (name.equals("result")) { 2194 throw new FHIRException("Cannot call addChild on a singleton property TestReport.result"); 2195 } else if (name.equals("message")) { 2196 throw new FHIRException("Cannot call addChild on a singleton property TestReport.message"); 2197 } else if (name.equals("detail")) { 2198 throw new FHIRException("Cannot call addChild on a singleton property TestReport.detail"); 2199 } else 2200 return super.addChild(name); 2201 } 2202 2203 public SetupActionAssertComponent copy() { 2204 SetupActionAssertComponent dst = new SetupActionAssertComponent(); 2205 copyValues(dst); 2206 return dst; 2207 } 2208 2209 public void copyValues(SetupActionAssertComponent dst) { 2210 super.copyValues(dst); 2211 dst.result = result == null ? null : result.copy(); 2212 dst.message = message == null ? null : message.copy(); 2213 dst.detail = detail == null ? null : detail.copy(); 2214 } 2215 2216 @Override 2217 public boolean equalsDeep(Base other_) { 2218 if (!super.equalsDeep(other_)) 2219 return false; 2220 if (!(other_ instanceof SetupActionAssertComponent)) 2221 return false; 2222 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 2223 return compareDeep(result, o.result, true) && compareDeep(message, o.message, true) 2224 && compareDeep(detail, o.detail, true); 2225 } 2226 2227 @Override 2228 public boolean equalsShallow(Base other_) { 2229 if (!super.equalsShallow(other_)) 2230 return false; 2231 if (!(other_ instanceof SetupActionAssertComponent)) 2232 return false; 2233 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 2234 return compareValues(result, o.result, true) && compareValues(message, o.message, true) 2235 && compareValues(detail, o.detail, true); 2236 } 2237 2238 public boolean isEmpty() { 2239 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(result, message, detail); 2240 } 2241 2242 public String fhirType() { 2243 return "TestReport.setup.action.assert"; 2244 2245 } 2246 2247 } 2248 2249 @Block() 2250 public static class TestReportTestComponent extends BackboneElement implements IBaseBackboneElement { 2251 /** 2252 * The name of this test used for tracking/logging purposes by test engines. 2253 */ 2254 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2255 @Description(shortDefinition = "Tracking/logging name of this test", formalDefinition = "The name of this test used for tracking/logging purposes by test engines.") 2256 protected StringType name; 2257 2258 /** 2259 * A short description of the test used by test engines for tracking and 2260 * reporting purposes. 2261 */ 2262 @Child(name = "description", type = { 2263 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2264 @Description(shortDefinition = "Tracking/reporting short description of the test", formalDefinition = "A short description of the test used by test engines for tracking and reporting purposes.") 2265 protected StringType description; 2266 2267 /** 2268 * Action would contain either an operation or an assertion. 2269 */ 2270 @Child(name = "action", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2271 @Description(shortDefinition = "A test operation or assert that was performed", formalDefinition = "Action would contain either an operation or an assertion.") 2272 protected List<TestActionComponent> action; 2273 2274 private static final long serialVersionUID = -865006110L; 2275 2276 /** 2277 * Constructor 2278 */ 2279 public TestReportTestComponent() { 2280 super(); 2281 } 2282 2283 /** 2284 * @return {@link #name} (The name of this test used for tracking/logging 2285 * purposes by test engines.). This is the underlying object with id, 2286 * value and extensions. The accessor "getName" gives direct access to 2287 * the value 2288 */ 2289 public StringType getNameElement() { 2290 if (this.name == null) 2291 if (Configuration.errorOnAutoCreate()) 2292 throw new Error("Attempt to auto-create TestReportTestComponent.name"); 2293 else if (Configuration.doAutoCreate()) 2294 this.name = new StringType(); // bb 2295 return this.name; 2296 } 2297 2298 public boolean hasNameElement() { 2299 return this.name != null && !this.name.isEmpty(); 2300 } 2301 2302 public boolean hasName() { 2303 return this.name != null && !this.name.isEmpty(); 2304 } 2305 2306 /** 2307 * @param value {@link #name} (The name of this test used for tracking/logging 2308 * purposes by test engines.). This is the underlying object with 2309 * id, value and extensions. The accessor "getName" gives direct 2310 * access to the value 2311 */ 2312 public TestReportTestComponent setNameElement(StringType value) { 2313 this.name = value; 2314 return this; 2315 } 2316 2317 /** 2318 * @return The name of this test used for tracking/logging purposes by test 2319 * engines. 2320 */ 2321 public String getName() { 2322 return this.name == null ? null : this.name.getValue(); 2323 } 2324 2325 /** 2326 * @param value The name of this test used for tracking/logging purposes by test 2327 * engines. 2328 */ 2329 public TestReportTestComponent setName(String value) { 2330 if (Utilities.noString(value)) 2331 this.name = null; 2332 else { 2333 if (this.name == null) 2334 this.name = new StringType(); 2335 this.name.setValue(value); 2336 } 2337 return this; 2338 } 2339 2340 /** 2341 * @return {@link #description} (A short description of the test used by test 2342 * engines for tracking and reporting purposes.). This is the underlying 2343 * object with id, value and extensions. The accessor "getDescription" 2344 * gives direct access to the value 2345 */ 2346 public StringType getDescriptionElement() { 2347 if (this.description == null) 2348 if (Configuration.errorOnAutoCreate()) 2349 throw new Error("Attempt to auto-create TestReportTestComponent.description"); 2350 else if (Configuration.doAutoCreate()) 2351 this.description = new StringType(); // bb 2352 return this.description; 2353 } 2354 2355 public boolean hasDescriptionElement() { 2356 return this.description != null && !this.description.isEmpty(); 2357 } 2358 2359 public boolean hasDescription() { 2360 return this.description != null && !this.description.isEmpty(); 2361 } 2362 2363 /** 2364 * @param value {@link #description} (A short description of the test used by 2365 * test engines for tracking and reporting purposes.). This is the 2366 * underlying object with id, value and extensions. The accessor 2367 * "getDescription" gives direct access to the value 2368 */ 2369 public TestReportTestComponent setDescriptionElement(StringType value) { 2370 this.description = value; 2371 return this; 2372 } 2373 2374 /** 2375 * @return A short description of the test used by test engines for tracking and 2376 * reporting purposes. 2377 */ 2378 public String getDescription() { 2379 return this.description == null ? null : this.description.getValue(); 2380 } 2381 2382 /** 2383 * @param value A short description of the test used by test engines for 2384 * tracking and reporting purposes. 2385 */ 2386 public TestReportTestComponent setDescription(String value) { 2387 if (Utilities.noString(value)) 2388 this.description = null; 2389 else { 2390 if (this.description == null) 2391 this.description = new StringType(); 2392 this.description.setValue(value); 2393 } 2394 return this; 2395 } 2396 2397 /** 2398 * @return {@link #action} (Action would contain either an operation or an 2399 * assertion.) 2400 */ 2401 public List<TestActionComponent> getAction() { 2402 if (this.action == null) 2403 this.action = new ArrayList<TestActionComponent>(); 2404 return this.action; 2405 } 2406 2407 /** 2408 * @return Returns a reference to <code>this</code> for easy method chaining 2409 */ 2410 public TestReportTestComponent setAction(List<TestActionComponent> theAction) { 2411 this.action = theAction; 2412 return this; 2413 } 2414 2415 public boolean hasAction() { 2416 if (this.action == null) 2417 return false; 2418 for (TestActionComponent item : this.action) 2419 if (!item.isEmpty()) 2420 return true; 2421 return false; 2422 } 2423 2424 public TestActionComponent addAction() { // 3 2425 TestActionComponent t = new TestActionComponent(); 2426 if (this.action == null) 2427 this.action = new ArrayList<TestActionComponent>(); 2428 this.action.add(t); 2429 return t; 2430 } 2431 2432 public TestReportTestComponent addAction(TestActionComponent t) { // 3 2433 if (t == null) 2434 return this; 2435 if (this.action == null) 2436 this.action = new ArrayList<TestActionComponent>(); 2437 this.action.add(t); 2438 return this; 2439 } 2440 2441 /** 2442 * @return The first repetition of repeating field {@link #action}, creating it 2443 * if it does not already exist 2444 */ 2445 public TestActionComponent getActionFirstRep() { 2446 if (getAction().isEmpty()) { 2447 addAction(); 2448 } 2449 return getAction().get(0); 2450 } 2451 2452 protected void listChildren(List<Property> children) { 2453 super.listChildren(children); 2454 children.add(new Property("name", "string", 2455 "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name)); 2456 children.add(new Property("description", "string", 2457 "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, 2458 description)); 2459 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, 2460 java.lang.Integer.MAX_VALUE, action)); 2461 } 2462 2463 @Override 2464 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2465 switch (_hash) { 2466 case 3373707: 2467 /* name */ return new Property("name", "string", 2468 "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name); 2469 case -1724546052: 2470 /* description */ return new Property("description", "string", 2471 "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, 2472 description); 2473 case -1422950858: 2474 /* action */ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, 2475 java.lang.Integer.MAX_VALUE, action); 2476 default: 2477 return super.getNamedProperty(_hash, _name, _checkValid); 2478 } 2479 2480 } 2481 2482 @Override 2483 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2484 switch (hash) { 2485 case 3373707: 2486 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2487 case -1724546052: 2488 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2489 case -1422950858: 2490 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TestActionComponent 2491 default: 2492 return super.getProperty(hash, name, checkValid); 2493 } 2494 2495 } 2496 2497 @Override 2498 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2499 switch (hash) { 2500 case 3373707: // name 2501 this.name = castToString(value); // StringType 2502 return value; 2503 case -1724546052: // description 2504 this.description = castToString(value); // StringType 2505 return value; 2506 case -1422950858: // action 2507 this.getAction().add((TestActionComponent) value); // TestActionComponent 2508 return value; 2509 default: 2510 return super.setProperty(hash, name, value); 2511 } 2512 2513 } 2514 2515 @Override 2516 public Base setProperty(String name, Base value) throws FHIRException { 2517 if (name.equals("name")) { 2518 this.name = castToString(value); // StringType 2519 } else if (name.equals("description")) { 2520 this.description = castToString(value); // StringType 2521 } else if (name.equals("action")) { 2522 this.getAction().add((TestActionComponent) value); 2523 } else 2524 return super.setProperty(name, value); 2525 return value; 2526 } 2527 2528 @Override 2529 public void removeChild(String name, Base value) throws FHIRException { 2530 if (name.equals("name")) { 2531 this.name = null; 2532 } else if (name.equals("description")) { 2533 this.description = null; 2534 } else if (name.equals("action")) { 2535 this.getAction().remove((TestActionComponent) value); 2536 } else 2537 super.removeChild(name, value); 2538 2539 } 2540 2541 @Override 2542 public Base makeProperty(int hash, String name) throws FHIRException { 2543 switch (hash) { 2544 case 3373707: 2545 return getNameElement(); 2546 case -1724546052: 2547 return getDescriptionElement(); 2548 case -1422950858: 2549 return addAction(); 2550 default: 2551 return super.makeProperty(hash, name); 2552 } 2553 2554 } 2555 2556 @Override 2557 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2558 switch (hash) { 2559 case 3373707: 2560 /* name */ return new String[] { "string" }; 2561 case -1724546052: 2562 /* description */ return new String[] { "string" }; 2563 case -1422950858: 2564 /* action */ return new String[] {}; 2565 default: 2566 return super.getTypesForProperty(hash, name); 2567 } 2568 2569 } 2570 2571 @Override 2572 public Base addChild(String name) throws FHIRException { 2573 if (name.equals("name")) { 2574 throw new FHIRException("Cannot call addChild on a singleton property TestReport.name"); 2575 } else if (name.equals("description")) { 2576 throw new FHIRException("Cannot call addChild on a singleton property TestReport.description"); 2577 } else if (name.equals("action")) { 2578 return addAction(); 2579 } else 2580 return super.addChild(name); 2581 } 2582 2583 public TestReportTestComponent copy() { 2584 TestReportTestComponent dst = new TestReportTestComponent(); 2585 copyValues(dst); 2586 return dst; 2587 } 2588 2589 public void copyValues(TestReportTestComponent dst) { 2590 super.copyValues(dst); 2591 dst.name = name == null ? null : name.copy(); 2592 dst.description = description == null ? null : description.copy(); 2593 if (action != null) { 2594 dst.action = new ArrayList<TestActionComponent>(); 2595 for (TestActionComponent i : action) 2596 dst.action.add(i.copy()); 2597 } 2598 ; 2599 } 2600 2601 @Override 2602 public boolean equalsDeep(Base other_) { 2603 if (!super.equalsDeep(other_)) 2604 return false; 2605 if (!(other_ instanceof TestReportTestComponent)) 2606 return false; 2607 TestReportTestComponent o = (TestReportTestComponent) other_; 2608 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 2609 && compareDeep(action, o.action, true); 2610 } 2611 2612 @Override 2613 public boolean equalsShallow(Base other_) { 2614 if (!super.equalsShallow(other_)) 2615 return false; 2616 if (!(other_ instanceof TestReportTestComponent)) 2617 return false; 2618 TestReportTestComponent o = (TestReportTestComponent) other_; 2619 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 2620 } 2621 2622 public boolean isEmpty() { 2623 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, action); 2624 } 2625 2626 public String fhirType() { 2627 return "TestReport.test"; 2628 2629 } 2630 2631 } 2632 2633 @Block() 2634 public static class TestActionComponent extends BackboneElement implements IBaseBackboneElement { 2635 /** 2636 * An operation would involve a REST request to a server. 2637 */ 2638 @Child(name = "operation", type = { 2639 SetupActionOperationComponent.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2640 @Description(shortDefinition = "The operation performed", formalDefinition = "An operation would involve a REST request to a server.") 2641 protected SetupActionOperationComponent operation; 2642 2643 /** 2644 * The results of the assertion performed on the previous operations. 2645 */ 2646 @Child(name = "assert", type = { 2647 SetupActionAssertComponent.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2648 @Description(shortDefinition = "The assertion performed", formalDefinition = "The results of the assertion performed on the previous operations.") 2649 protected SetupActionAssertComponent assert_; 2650 2651 private static final long serialVersionUID = -252088305L; 2652 2653 /** 2654 * Constructor 2655 */ 2656 public TestActionComponent() { 2657 super(); 2658 } 2659 2660 /** 2661 * @return {@link #operation} (An operation would involve a REST request to a 2662 * server.) 2663 */ 2664 public SetupActionOperationComponent getOperation() { 2665 if (this.operation == null) 2666 if (Configuration.errorOnAutoCreate()) 2667 throw new Error("Attempt to auto-create TestActionComponent.operation"); 2668 else if (Configuration.doAutoCreate()) 2669 this.operation = new SetupActionOperationComponent(); // cc 2670 return this.operation; 2671 } 2672 2673 public boolean hasOperation() { 2674 return this.operation != null && !this.operation.isEmpty(); 2675 } 2676 2677 /** 2678 * @param value {@link #operation} (An operation would involve a REST request to 2679 * a server.) 2680 */ 2681 public TestActionComponent setOperation(SetupActionOperationComponent value) { 2682 this.operation = value; 2683 return this; 2684 } 2685 2686 /** 2687 * @return {@link #assert_} (The results of the assertion performed on the 2688 * previous operations.) 2689 */ 2690 public SetupActionAssertComponent getAssert() { 2691 if (this.assert_ == null) 2692 if (Configuration.errorOnAutoCreate()) 2693 throw new Error("Attempt to auto-create TestActionComponent.assert_"); 2694 else if (Configuration.doAutoCreate()) 2695 this.assert_ = new SetupActionAssertComponent(); // cc 2696 return this.assert_; 2697 } 2698 2699 public boolean hasAssert() { 2700 return this.assert_ != null && !this.assert_.isEmpty(); 2701 } 2702 2703 /** 2704 * @param value {@link #assert_} (The results of the assertion performed on the 2705 * previous operations.) 2706 */ 2707 public TestActionComponent setAssert(SetupActionAssertComponent value) { 2708 this.assert_ = value; 2709 return this; 2710 } 2711 2712 protected void listChildren(List<Property> children) { 2713 super.listChildren(children); 2714 children.add(new Property("operation", "@TestReport.setup.action.operation", 2715 "An operation would involve a REST request to a server.", 0, 1, operation)); 2716 children.add(new Property("assert", "@TestReport.setup.action.assert", 2717 "The results of the assertion performed on the previous operations.", 0, 1, assert_)); 2718 } 2719 2720 @Override 2721 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2722 switch (_hash) { 2723 case 1662702951: 2724 /* operation */ return new Property("operation", "@TestReport.setup.action.operation", 2725 "An operation would involve a REST request to a server.", 0, 1, operation); 2726 case -1408208058: 2727 /* assert */ return new Property("assert", "@TestReport.setup.action.assert", 2728 "The results of the assertion performed on the previous operations.", 0, 1, assert_); 2729 default: 2730 return super.getNamedProperty(_hash, _name, _checkValid); 2731 } 2732 2733 } 2734 2735 @Override 2736 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2737 switch (hash) { 2738 case 1662702951: 2739 /* operation */ return this.operation == null ? new Base[0] : new Base[] { this.operation }; // SetupActionOperationComponent 2740 case -1408208058: 2741 /* assert */ return this.assert_ == null ? new Base[0] : new Base[] { this.assert_ }; // SetupActionAssertComponent 2742 default: 2743 return super.getProperty(hash, name, checkValid); 2744 } 2745 2746 } 2747 2748 @Override 2749 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2750 switch (hash) { 2751 case 1662702951: // operation 2752 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2753 return value; 2754 case -1408208058: // assert 2755 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2756 return value; 2757 default: 2758 return super.setProperty(hash, name, value); 2759 } 2760 2761 } 2762 2763 @Override 2764 public Base setProperty(String name, Base value) throws FHIRException { 2765 if (name.equals("operation")) { 2766 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2767 } else if (name.equals("assert")) { 2768 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2769 } else 2770 return super.setProperty(name, value); 2771 return value; 2772 } 2773 2774 @Override 2775 public void removeChild(String name, Base value) throws FHIRException { 2776 if (name.equals("operation")) { 2777 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 2778 } else if (name.equals("assert")) { 2779 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 2780 } else 2781 super.removeChild(name, value); 2782 2783 } 2784 2785 @Override 2786 public Base makeProperty(int hash, String name) throws FHIRException { 2787 switch (hash) { 2788 case 1662702951: 2789 return getOperation(); 2790 case -1408208058: 2791 return getAssert(); 2792 default: 2793 return super.makeProperty(hash, name); 2794 } 2795 2796 } 2797 2798 @Override 2799 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2800 switch (hash) { 2801 case 1662702951: 2802 /* operation */ return new String[] { "@TestReport.setup.action.operation" }; 2803 case -1408208058: 2804 /* assert */ return new String[] { "@TestReport.setup.action.assert" }; 2805 default: 2806 return super.getTypesForProperty(hash, name); 2807 } 2808 2809 } 2810 2811 @Override 2812 public Base addChild(String name) throws FHIRException { 2813 if (name.equals("operation")) { 2814 this.operation = new SetupActionOperationComponent(); 2815 return this.operation; 2816 } else if (name.equals("assert")) { 2817 this.assert_ = new SetupActionAssertComponent(); 2818 return this.assert_; 2819 } else 2820 return super.addChild(name); 2821 } 2822 2823 public TestActionComponent copy() { 2824 TestActionComponent dst = new TestActionComponent(); 2825 copyValues(dst); 2826 return dst; 2827 } 2828 2829 public void copyValues(TestActionComponent dst) { 2830 super.copyValues(dst); 2831 dst.operation = operation == null ? null : operation.copy(); 2832 dst.assert_ = assert_ == null ? null : assert_.copy(); 2833 } 2834 2835 @Override 2836 public boolean equalsDeep(Base other_) { 2837 if (!super.equalsDeep(other_)) 2838 return false; 2839 if (!(other_ instanceof TestActionComponent)) 2840 return false; 2841 TestActionComponent o = (TestActionComponent) other_; 2842 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 2843 } 2844 2845 @Override 2846 public boolean equalsShallow(Base other_) { 2847 if (!super.equalsShallow(other_)) 2848 return false; 2849 if (!(other_ instanceof TestActionComponent)) 2850 return false; 2851 TestActionComponent o = (TestActionComponent) other_; 2852 return true; 2853 } 2854 2855 public boolean isEmpty() { 2856 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 2857 } 2858 2859 public String fhirType() { 2860 return "TestReport.test.action"; 2861 2862 } 2863 2864 } 2865 2866 @Block() 2867 public static class TestReportTeardownComponent extends BackboneElement implements IBaseBackboneElement { 2868 /** 2869 * The teardown action will only contain an operation. 2870 */ 2871 @Child(name = "action", type = {}, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2872 @Description(shortDefinition = "One or more teardown operations performed", formalDefinition = "The teardown action will only contain an operation.") 2873 protected List<TeardownActionComponent> action; 2874 2875 private static final long serialVersionUID = 1168638089L; 2876 2877 /** 2878 * Constructor 2879 */ 2880 public TestReportTeardownComponent() { 2881 super(); 2882 } 2883 2884 /** 2885 * @return {@link #action} (The teardown action will only contain an operation.) 2886 */ 2887 public List<TeardownActionComponent> getAction() { 2888 if (this.action == null) 2889 this.action = new ArrayList<TeardownActionComponent>(); 2890 return this.action; 2891 } 2892 2893 /** 2894 * @return Returns a reference to <code>this</code> for easy method chaining 2895 */ 2896 public TestReportTeardownComponent setAction(List<TeardownActionComponent> theAction) { 2897 this.action = theAction; 2898 return this; 2899 } 2900 2901 public boolean hasAction() { 2902 if (this.action == null) 2903 return false; 2904 for (TeardownActionComponent item : this.action) 2905 if (!item.isEmpty()) 2906 return true; 2907 return false; 2908 } 2909 2910 public TeardownActionComponent addAction() { // 3 2911 TeardownActionComponent t = new TeardownActionComponent(); 2912 if (this.action == null) 2913 this.action = new ArrayList<TeardownActionComponent>(); 2914 this.action.add(t); 2915 return t; 2916 } 2917 2918 public TestReportTeardownComponent addAction(TeardownActionComponent t) { // 3 2919 if (t == null) 2920 return this; 2921 if (this.action == null) 2922 this.action = new ArrayList<TeardownActionComponent>(); 2923 this.action.add(t); 2924 return this; 2925 } 2926 2927 /** 2928 * @return The first repetition of repeating field {@link #action}, creating it 2929 * if it does not already exist 2930 */ 2931 public TeardownActionComponent getActionFirstRep() { 2932 if (getAction().isEmpty()) { 2933 addAction(); 2934 } 2935 return getAction().get(0); 2936 } 2937 2938 protected void listChildren(List<Property> children) { 2939 super.listChildren(children); 2940 children.add(new Property("action", "", "The teardown action will only contain an operation.", 0, 2941 java.lang.Integer.MAX_VALUE, action)); 2942 } 2943 2944 @Override 2945 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2946 switch (_hash) { 2947 case -1422950858: 2948 /* action */ return new Property("action", "", "The teardown action will only contain an operation.", 0, 2949 java.lang.Integer.MAX_VALUE, action); 2950 default: 2951 return super.getNamedProperty(_hash, _name, _checkValid); 2952 } 2953 2954 } 2955 2956 @Override 2957 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2958 switch (hash) { 2959 case -1422950858: 2960 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TeardownActionComponent 2961 default: 2962 return super.getProperty(hash, name, checkValid); 2963 } 2964 2965 } 2966 2967 @Override 2968 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2969 switch (hash) { 2970 case -1422950858: // action 2971 this.getAction().add((TeardownActionComponent) value); // TeardownActionComponent 2972 return value; 2973 default: 2974 return super.setProperty(hash, name, value); 2975 } 2976 2977 } 2978 2979 @Override 2980 public Base setProperty(String name, Base value) throws FHIRException { 2981 if (name.equals("action")) { 2982 this.getAction().add((TeardownActionComponent) value); 2983 } else 2984 return super.setProperty(name, value); 2985 return value; 2986 } 2987 2988 @Override 2989 public void removeChild(String name, Base value) throws FHIRException { 2990 if (name.equals("action")) { 2991 this.getAction().remove((TeardownActionComponent) value); 2992 } else 2993 super.removeChild(name, value); 2994 2995 } 2996 2997 @Override 2998 public Base makeProperty(int hash, String name) throws FHIRException { 2999 switch (hash) { 3000 case -1422950858: 3001 return addAction(); 3002 default: 3003 return super.makeProperty(hash, name); 3004 } 3005 3006 } 3007 3008 @Override 3009 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3010 switch (hash) { 3011 case -1422950858: 3012 /* action */ return new String[] {}; 3013 default: 3014 return super.getTypesForProperty(hash, name); 3015 } 3016 3017 } 3018 3019 @Override 3020 public Base addChild(String name) throws FHIRException { 3021 if (name.equals("action")) { 3022 return addAction(); 3023 } else 3024 return super.addChild(name); 3025 } 3026 3027 public TestReportTeardownComponent copy() { 3028 TestReportTeardownComponent dst = new TestReportTeardownComponent(); 3029 copyValues(dst); 3030 return dst; 3031 } 3032 3033 public void copyValues(TestReportTeardownComponent dst) { 3034 super.copyValues(dst); 3035 if (action != null) { 3036 dst.action = new ArrayList<TeardownActionComponent>(); 3037 for (TeardownActionComponent i : action) 3038 dst.action.add(i.copy()); 3039 } 3040 ; 3041 } 3042 3043 @Override 3044 public boolean equalsDeep(Base other_) { 3045 if (!super.equalsDeep(other_)) 3046 return false; 3047 if (!(other_ instanceof TestReportTeardownComponent)) 3048 return false; 3049 TestReportTeardownComponent o = (TestReportTeardownComponent) other_; 3050 return compareDeep(action, o.action, true); 3051 } 3052 3053 @Override 3054 public boolean equalsShallow(Base other_) { 3055 if (!super.equalsShallow(other_)) 3056 return false; 3057 if (!(other_ instanceof TestReportTeardownComponent)) 3058 return false; 3059 TestReportTeardownComponent o = (TestReportTeardownComponent) other_; 3060 return true; 3061 } 3062 3063 public boolean isEmpty() { 3064 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 3065 } 3066 3067 public String fhirType() { 3068 return "TestReport.teardown"; 3069 3070 } 3071 3072 } 3073 3074 @Block() 3075 public static class TeardownActionComponent extends BackboneElement implements IBaseBackboneElement { 3076 /** 3077 * An operation would involve a REST request to a server. 3078 */ 3079 @Child(name = "operation", type = { 3080 SetupActionOperationComponent.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3081 @Description(shortDefinition = "The teardown operation performed", formalDefinition = "An operation would involve a REST request to a server.") 3082 protected SetupActionOperationComponent operation; 3083 3084 private static final long serialVersionUID = -1099598054L; 3085 3086 /** 3087 * Constructor 3088 */ 3089 public TeardownActionComponent() { 3090 super(); 3091 } 3092 3093 /** 3094 * Constructor 3095 */ 3096 public TeardownActionComponent(SetupActionOperationComponent operation) { 3097 super(); 3098 this.operation = operation; 3099 } 3100 3101 /** 3102 * @return {@link #operation} (An operation would involve a REST request to a 3103 * server.) 3104 */ 3105 public SetupActionOperationComponent getOperation() { 3106 if (this.operation == null) 3107 if (Configuration.errorOnAutoCreate()) 3108 throw new Error("Attempt to auto-create TeardownActionComponent.operation"); 3109 else if (Configuration.doAutoCreate()) 3110 this.operation = new SetupActionOperationComponent(); // cc 3111 return this.operation; 3112 } 3113 3114 public boolean hasOperation() { 3115 return this.operation != null && !this.operation.isEmpty(); 3116 } 3117 3118 /** 3119 * @param value {@link #operation} (An operation would involve a REST request to 3120 * a server.) 3121 */ 3122 public TeardownActionComponent setOperation(SetupActionOperationComponent value) { 3123 this.operation = value; 3124 return this; 3125 } 3126 3127 protected void listChildren(List<Property> children) { 3128 super.listChildren(children); 3129 children.add(new Property("operation", "@TestReport.setup.action.operation", 3130 "An operation would involve a REST request to a server.", 0, 1, operation)); 3131 } 3132 3133 @Override 3134 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3135 switch (_hash) { 3136 case 1662702951: 3137 /* operation */ return new Property("operation", "@TestReport.setup.action.operation", 3138 "An operation would involve a REST request to a server.", 0, 1, operation); 3139 default: 3140 return super.getNamedProperty(_hash, _name, _checkValid); 3141 } 3142 3143 } 3144 3145 @Override 3146 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3147 switch (hash) { 3148 case 1662702951: 3149 /* operation */ return this.operation == null ? new Base[0] : new Base[] { this.operation }; // SetupActionOperationComponent 3150 default: 3151 return super.getProperty(hash, name, checkValid); 3152 } 3153 3154 } 3155 3156 @Override 3157 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3158 switch (hash) { 3159 case 1662702951: // operation 3160 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3161 return value; 3162 default: 3163 return super.setProperty(hash, name, value); 3164 } 3165 3166 } 3167 3168 @Override 3169 public Base setProperty(String name, Base value) throws FHIRException { 3170 if (name.equals("operation")) { 3171 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3172 } else 3173 return super.setProperty(name, value); 3174 return value; 3175 } 3176 3177 @Override 3178 public void removeChild(String name, Base value) throws FHIRException { 3179 if (name.equals("operation")) { 3180 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 3181 } else 3182 super.removeChild(name, value); 3183 3184 } 3185 3186 @Override 3187 public Base makeProperty(int hash, String name) throws FHIRException { 3188 switch (hash) { 3189 case 1662702951: 3190 return getOperation(); 3191 default: 3192 return super.makeProperty(hash, name); 3193 } 3194 3195 } 3196 3197 @Override 3198 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3199 switch (hash) { 3200 case 1662702951: 3201 /* operation */ return new String[] { "@TestReport.setup.action.operation" }; 3202 default: 3203 return super.getTypesForProperty(hash, name); 3204 } 3205 3206 } 3207 3208 @Override 3209 public Base addChild(String name) throws FHIRException { 3210 if (name.equals("operation")) { 3211 this.operation = new SetupActionOperationComponent(); 3212 return this.operation; 3213 } else 3214 return super.addChild(name); 3215 } 3216 3217 public TeardownActionComponent copy() { 3218 TeardownActionComponent dst = new TeardownActionComponent(); 3219 copyValues(dst); 3220 return dst; 3221 } 3222 3223 public void copyValues(TeardownActionComponent dst) { 3224 super.copyValues(dst); 3225 dst.operation = operation == null ? null : operation.copy(); 3226 } 3227 3228 @Override 3229 public boolean equalsDeep(Base other_) { 3230 if (!super.equalsDeep(other_)) 3231 return false; 3232 if (!(other_ instanceof TeardownActionComponent)) 3233 return false; 3234 TeardownActionComponent o = (TeardownActionComponent) other_; 3235 return compareDeep(operation, o.operation, true); 3236 } 3237 3238 @Override 3239 public boolean equalsShallow(Base other_) { 3240 if (!super.equalsShallow(other_)) 3241 return false; 3242 if (!(other_ instanceof TeardownActionComponent)) 3243 return false; 3244 TeardownActionComponent o = (TeardownActionComponent) other_; 3245 return true; 3246 } 3247 3248 public boolean isEmpty() { 3249 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation); 3250 } 3251 3252 public String fhirType() { 3253 return "TestReport.teardown.action"; 3254 3255 } 3256 3257 } 3258 3259 /** 3260 * Identifier for the TestScript assigned for external purposes outside the 3261 * context of FHIR. 3262 */ 3263 @Child(name = "identifier", type = { 3264 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 3265 @Description(shortDefinition = "External identifier", formalDefinition = "Identifier for the TestScript assigned for external purposes outside the context of FHIR.") 3266 protected Identifier identifier; 3267 3268 /** 3269 * A free text natural language name identifying the executed TestScript. 3270 */ 3271 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 3272 @Description(shortDefinition = "Informal name of the executed TestScript", formalDefinition = "A free text natural language name identifying the executed TestScript.") 3273 protected StringType name; 3274 3275 /** 3276 * The current state of this test report. 3277 */ 3278 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 3279 @Description(shortDefinition = "completed | in-progress | waiting | stopped | entered-in-error", formalDefinition = "The current state of this test report.") 3280 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/report-status-codes") 3281 protected Enumeration<TestReportStatus> status; 3282 3283 /** 3284 * Ideally this is an absolute URL that is used to identify the version-specific 3285 * TestScript that was executed, matching the `TestScript.url`. 3286 */ 3287 @Child(name = "testScript", type = { 3288 TestScript.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 3289 @Description(shortDefinition = "Reference to the version-specific TestScript that was executed to produce this TestReport", formalDefinition = "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.") 3290 protected Reference testScript; 3291 3292 /** 3293 * The actual object that is the target of the reference (Ideally this is an 3294 * absolute URL that is used to identify the version-specific TestScript that 3295 * was executed, matching the `TestScript.url`.) 3296 */ 3297 protected TestScript testScriptTarget; 3298 3299 /** 3300 * The overall result from the execution of the TestScript. 3301 */ 3302 @Child(name = "result", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 3303 @Description(shortDefinition = "pass | fail | pending", formalDefinition = "The overall result from the execution of the TestScript.") 3304 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/report-result-codes") 3305 protected Enumeration<TestReportResult> result; 3306 3307 /** 3308 * The final score (percentage of tests passed) resulting from the execution of 3309 * the TestScript. 3310 */ 3311 @Child(name = "score", type = { DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3312 @Description(shortDefinition = "The final score (percentage of tests passed) resulting from the execution of the TestScript", formalDefinition = "The final score (percentage of tests passed) resulting from the execution of the TestScript.") 3313 protected DecimalType score; 3314 3315 /** 3316 * Name of the tester producing this report (Organization or individual). 3317 */ 3318 @Child(name = "tester", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3319 @Description(shortDefinition = "Name of the tester producing this report (Organization or individual)", formalDefinition = "Name of the tester producing this report (Organization or individual).") 3320 protected StringType tester; 3321 3322 /** 3323 * When the TestScript was executed and this TestReport was generated. 3324 */ 3325 @Child(name = "issued", type = { DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3326 @Description(shortDefinition = "When the TestScript was executed and this TestReport was generated", formalDefinition = "When the TestScript was executed and this TestReport was generated.") 3327 protected DateTimeType issued; 3328 3329 /** 3330 * A participant in the test execution, either the execution engine, a client, 3331 * or a server. 3332 */ 3333 @Child(name = "participant", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3334 @Description(shortDefinition = "A participant in the test execution, either the execution engine, a client, or a server", formalDefinition = "A participant in the test execution, either the execution engine, a client, or a server.") 3335 protected List<TestReportParticipantComponent> participant; 3336 3337 /** 3338 * The results of the series of required setup operations before the tests were 3339 * executed. 3340 */ 3341 @Child(name = "setup", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 3342 @Description(shortDefinition = "The results of the series of required setup operations before the tests were executed", formalDefinition = "The results of the series of required setup operations before the tests were executed.") 3343 protected TestReportSetupComponent setup; 3344 3345 /** 3346 * A test executed from the test script. 3347 */ 3348 @Child(name = "test", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3349 @Description(shortDefinition = "A test executed from the test script", formalDefinition = "A test executed from the test script.") 3350 protected List<TestReportTestComponent> test; 3351 3352 /** 3353 * The results of the series of operations required to clean up after all the 3354 * tests were executed (successfully or otherwise). 3355 */ 3356 @Child(name = "teardown", type = {}, order = 11, min = 0, max = 1, modifier = false, summary = false) 3357 @Description(shortDefinition = "The results of running the series of required clean up steps", formalDefinition = "The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).") 3358 protected TestReportTeardownComponent teardown; 3359 3360 private static final long serialVersionUID = 79474516L; 3361 3362 /** 3363 * Constructor 3364 */ 3365 public TestReport() { 3366 super(); 3367 } 3368 3369 /** 3370 * Constructor 3371 */ 3372 public TestReport(Enumeration<TestReportStatus> status, Reference testScript, Enumeration<TestReportResult> result) { 3373 super(); 3374 this.status = status; 3375 this.testScript = testScript; 3376 this.result = result; 3377 } 3378 3379 /** 3380 * @return {@link #identifier} (Identifier for the TestScript assigned for 3381 * external purposes outside the context of FHIR.) 3382 */ 3383 public Identifier getIdentifier() { 3384 if (this.identifier == null) 3385 if (Configuration.errorOnAutoCreate()) 3386 throw new Error("Attempt to auto-create TestReport.identifier"); 3387 else if (Configuration.doAutoCreate()) 3388 this.identifier = new Identifier(); // cc 3389 return this.identifier; 3390 } 3391 3392 public boolean hasIdentifier() { 3393 return this.identifier != null && !this.identifier.isEmpty(); 3394 } 3395 3396 /** 3397 * @param value {@link #identifier} (Identifier for the TestScript assigned for 3398 * external purposes outside the context of FHIR.) 3399 */ 3400 public TestReport setIdentifier(Identifier value) { 3401 this.identifier = value; 3402 return this; 3403 } 3404 3405 /** 3406 * @return {@link #name} (A free text natural language name identifying the 3407 * executed TestScript.). This is the underlying object with id, value 3408 * and extensions. The accessor "getName" gives direct access to the 3409 * value 3410 */ 3411 public StringType getNameElement() { 3412 if (this.name == null) 3413 if (Configuration.errorOnAutoCreate()) 3414 throw new Error("Attempt to auto-create TestReport.name"); 3415 else if (Configuration.doAutoCreate()) 3416 this.name = new StringType(); // bb 3417 return this.name; 3418 } 3419 3420 public boolean hasNameElement() { 3421 return this.name != null && !this.name.isEmpty(); 3422 } 3423 3424 public boolean hasName() { 3425 return this.name != null && !this.name.isEmpty(); 3426 } 3427 3428 /** 3429 * @param value {@link #name} (A free text natural language name identifying the 3430 * executed TestScript.). This is the underlying object with id, 3431 * value and extensions. The accessor "getName" gives direct access 3432 * to the value 3433 */ 3434 public TestReport setNameElement(StringType value) { 3435 this.name = value; 3436 return this; 3437 } 3438 3439 /** 3440 * @return A free text natural language name identifying the executed 3441 * TestScript. 3442 */ 3443 public String getName() { 3444 return this.name == null ? null : this.name.getValue(); 3445 } 3446 3447 /** 3448 * @param value A free text natural language name identifying the executed 3449 * TestScript. 3450 */ 3451 public TestReport setName(String value) { 3452 if (Utilities.noString(value)) 3453 this.name = null; 3454 else { 3455 if (this.name == null) 3456 this.name = new StringType(); 3457 this.name.setValue(value); 3458 } 3459 return this; 3460 } 3461 3462 /** 3463 * @return {@link #status} (The current state of this test report.). This is the 3464 * underlying object with id, value and extensions. The accessor 3465 * "getStatus" gives direct access to the value 3466 */ 3467 public Enumeration<TestReportStatus> getStatusElement() { 3468 if (this.status == null) 3469 if (Configuration.errorOnAutoCreate()) 3470 throw new Error("Attempt to auto-create TestReport.status"); 3471 else if (Configuration.doAutoCreate()) 3472 this.status = new Enumeration<TestReportStatus>(new TestReportStatusEnumFactory()); // bb 3473 return this.status; 3474 } 3475 3476 public boolean hasStatusElement() { 3477 return this.status != null && !this.status.isEmpty(); 3478 } 3479 3480 public boolean hasStatus() { 3481 return this.status != null && !this.status.isEmpty(); 3482 } 3483 3484 /** 3485 * @param value {@link #status} (The current state of this test report.). This 3486 * is the underlying object with id, value and extensions. The 3487 * accessor "getStatus" gives direct access to the value 3488 */ 3489 public TestReport setStatusElement(Enumeration<TestReportStatus> value) { 3490 this.status = value; 3491 return this; 3492 } 3493 3494 /** 3495 * @return The current state of this test report. 3496 */ 3497 public TestReportStatus getStatus() { 3498 return this.status == null ? null : this.status.getValue(); 3499 } 3500 3501 /** 3502 * @param value The current state of this test report. 3503 */ 3504 public TestReport setStatus(TestReportStatus value) { 3505 if (this.status == null) 3506 this.status = new Enumeration<TestReportStatus>(new TestReportStatusEnumFactory()); 3507 this.status.setValue(value); 3508 return this; 3509 } 3510 3511 /** 3512 * @return {@link #testScript} (Ideally this is an absolute URL that is used to 3513 * identify the version-specific TestScript that was executed, matching 3514 * the `TestScript.url`.) 3515 */ 3516 public Reference getTestScript() { 3517 if (this.testScript == null) 3518 if (Configuration.errorOnAutoCreate()) 3519 throw new Error("Attempt to auto-create TestReport.testScript"); 3520 else if (Configuration.doAutoCreate()) 3521 this.testScript = new Reference(); // cc 3522 return this.testScript; 3523 } 3524 3525 public boolean hasTestScript() { 3526 return this.testScript != null && !this.testScript.isEmpty(); 3527 } 3528 3529 /** 3530 * @param value {@link #testScript} (Ideally this is an absolute URL that is 3531 * used to identify the version-specific TestScript that was 3532 * executed, matching the `TestScript.url`.) 3533 */ 3534 public TestReport setTestScript(Reference value) { 3535 this.testScript = value; 3536 return this; 3537 } 3538 3539 /** 3540 * @return {@link #testScript} The actual object that is the target of the 3541 * reference. The reference library doesn't populate this, but you can 3542 * use it to hold the resource if you resolve it. (Ideally this is an 3543 * absolute URL that is used to identify the version-specific TestScript 3544 * that was executed, matching the `TestScript.url`.) 3545 */ 3546 public TestScript getTestScriptTarget() { 3547 if (this.testScriptTarget == null) 3548 if (Configuration.errorOnAutoCreate()) 3549 throw new Error("Attempt to auto-create TestReport.testScript"); 3550 else if (Configuration.doAutoCreate()) 3551 this.testScriptTarget = new TestScript(); // aa 3552 return this.testScriptTarget; 3553 } 3554 3555 /** 3556 * @param value {@link #testScript} The actual object that is the target of the 3557 * reference. The reference library doesn't use these, but you can 3558 * use it to hold the resource if you resolve it. (Ideally this is 3559 * an absolute URL that is used to identify the version-specific 3560 * TestScript that was executed, matching the `TestScript.url`.) 3561 */ 3562 public TestReport setTestScriptTarget(TestScript value) { 3563 this.testScriptTarget = value; 3564 return this; 3565 } 3566 3567 /** 3568 * @return {@link #result} (The overall result from the execution of the 3569 * TestScript.). This is the underlying object with id, value and 3570 * extensions. The accessor "getResult" gives direct access to the value 3571 */ 3572 public Enumeration<TestReportResult> getResultElement() { 3573 if (this.result == null) 3574 if (Configuration.errorOnAutoCreate()) 3575 throw new Error("Attempt to auto-create TestReport.result"); 3576 else if (Configuration.doAutoCreate()) 3577 this.result = new Enumeration<TestReportResult>(new TestReportResultEnumFactory()); // bb 3578 return this.result; 3579 } 3580 3581 public boolean hasResultElement() { 3582 return this.result != null && !this.result.isEmpty(); 3583 } 3584 3585 public boolean hasResult() { 3586 return this.result != null && !this.result.isEmpty(); 3587 } 3588 3589 /** 3590 * @param value {@link #result} (The overall result from the execution of the 3591 * TestScript.). This is the underlying object with id, value and 3592 * extensions. The accessor "getResult" gives direct access to the 3593 * value 3594 */ 3595 public TestReport setResultElement(Enumeration<TestReportResult> value) { 3596 this.result = value; 3597 return this; 3598 } 3599 3600 /** 3601 * @return The overall result from the execution of the TestScript. 3602 */ 3603 public TestReportResult getResult() { 3604 return this.result == null ? null : this.result.getValue(); 3605 } 3606 3607 /** 3608 * @param value The overall result from the execution of the TestScript. 3609 */ 3610 public TestReport setResult(TestReportResult value) { 3611 if (this.result == null) 3612 this.result = new Enumeration<TestReportResult>(new TestReportResultEnumFactory()); 3613 this.result.setValue(value); 3614 return this; 3615 } 3616 3617 /** 3618 * @return {@link #score} (The final score (percentage of tests passed) 3619 * resulting from the execution of the TestScript.). This is the 3620 * underlying object with id, value and extensions. The accessor 3621 * "getScore" gives direct access to the value 3622 */ 3623 public DecimalType getScoreElement() { 3624 if (this.score == null) 3625 if (Configuration.errorOnAutoCreate()) 3626 throw new Error("Attempt to auto-create TestReport.score"); 3627 else if (Configuration.doAutoCreate()) 3628 this.score = new DecimalType(); // bb 3629 return this.score; 3630 } 3631 3632 public boolean hasScoreElement() { 3633 return this.score != null && !this.score.isEmpty(); 3634 } 3635 3636 public boolean hasScore() { 3637 return this.score != null && !this.score.isEmpty(); 3638 } 3639 3640 /** 3641 * @param value {@link #score} (The final score (percentage of tests passed) 3642 * resulting from the execution of the TestScript.). This is the 3643 * underlying object with id, value and extensions. The accessor 3644 * "getScore" gives direct access to the value 3645 */ 3646 public TestReport setScoreElement(DecimalType value) { 3647 this.score = value; 3648 return this; 3649 } 3650 3651 /** 3652 * @return The final score (percentage of tests passed) resulting from the 3653 * execution of the TestScript. 3654 */ 3655 public BigDecimal getScore() { 3656 return this.score == null ? null : this.score.getValue(); 3657 } 3658 3659 /** 3660 * @param value The final score (percentage of tests passed) resulting from the 3661 * execution of the TestScript. 3662 */ 3663 public TestReport setScore(BigDecimal value) { 3664 if (value == null) 3665 this.score = null; 3666 else { 3667 if (this.score == null) 3668 this.score = new DecimalType(); 3669 this.score.setValue(value); 3670 } 3671 return this; 3672 } 3673 3674 /** 3675 * @param value The final score (percentage of tests passed) resulting from the 3676 * execution of the TestScript. 3677 */ 3678 public TestReport setScore(long value) { 3679 this.score = new DecimalType(); 3680 this.score.setValue(value); 3681 return this; 3682 } 3683 3684 /** 3685 * @param value The final score (percentage of tests passed) resulting from the 3686 * execution of the TestScript. 3687 */ 3688 public TestReport setScore(double value) { 3689 this.score = new DecimalType(); 3690 this.score.setValue(value); 3691 return this; 3692 } 3693 3694 /** 3695 * @return {@link #tester} (Name of the tester producing this report 3696 * (Organization or individual).). This is the underlying object with 3697 * id, value and extensions. The accessor "getTester" gives direct 3698 * access to the value 3699 */ 3700 public StringType getTesterElement() { 3701 if (this.tester == null) 3702 if (Configuration.errorOnAutoCreate()) 3703 throw new Error("Attempt to auto-create TestReport.tester"); 3704 else if (Configuration.doAutoCreate()) 3705 this.tester = new StringType(); // bb 3706 return this.tester; 3707 } 3708 3709 public boolean hasTesterElement() { 3710 return this.tester != null && !this.tester.isEmpty(); 3711 } 3712 3713 public boolean hasTester() { 3714 return this.tester != null && !this.tester.isEmpty(); 3715 } 3716 3717 /** 3718 * @param value {@link #tester} (Name of the tester producing this report 3719 * (Organization or individual).). This is the underlying object 3720 * with id, value and extensions. The accessor "getTester" gives 3721 * direct access to the value 3722 */ 3723 public TestReport setTesterElement(StringType value) { 3724 this.tester = value; 3725 return this; 3726 } 3727 3728 /** 3729 * @return Name of the tester producing this report (Organization or 3730 * individual). 3731 */ 3732 public String getTester() { 3733 return this.tester == null ? null : this.tester.getValue(); 3734 } 3735 3736 /** 3737 * @param value Name of the tester producing this report (Organization or 3738 * individual). 3739 */ 3740 public TestReport setTester(String value) { 3741 if (Utilities.noString(value)) 3742 this.tester = null; 3743 else { 3744 if (this.tester == null) 3745 this.tester = new StringType(); 3746 this.tester.setValue(value); 3747 } 3748 return this; 3749 } 3750 3751 /** 3752 * @return {@link #issued} (When the TestScript was executed and this TestReport 3753 * was generated.). This is the underlying object with id, value and 3754 * extensions. The accessor "getIssued" gives direct access to the value 3755 */ 3756 public DateTimeType getIssuedElement() { 3757 if (this.issued == null) 3758 if (Configuration.errorOnAutoCreate()) 3759 throw new Error("Attempt to auto-create TestReport.issued"); 3760 else if (Configuration.doAutoCreate()) 3761 this.issued = new DateTimeType(); // bb 3762 return this.issued; 3763 } 3764 3765 public boolean hasIssuedElement() { 3766 return this.issued != null && !this.issued.isEmpty(); 3767 } 3768 3769 public boolean hasIssued() { 3770 return this.issued != null && !this.issued.isEmpty(); 3771 } 3772 3773 /** 3774 * @param value {@link #issued} (When the TestScript was executed and this 3775 * TestReport was generated.). This is the underlying object with 3776 * id, value and extensions. The accessor "getIssued" gives direct 3777 * access to the value 3778 */ 3779 public TestReport setIssuedElement(DateTimeType value) { 3780 this.issued = value; 3781 return this; 3782 } 3783 3784 /** 3785 * @return When the TestScript was executed and this TestReport was generated. 3786 */ 3787 public Date getIssued() { 3788 return this.issued == null ? null : this.issued.getValue(); 3789 } 3790 3791 /** 3792 * @param value When the TestScript was executed and this TestReport was 3793 * generated. 3794 */ 3795 public TestReport setIssued(Date value) { 3796 if (value == null) 3797 this.issued = null; 3798 else { 3799 if (this.issued == null) 3800 this.issued = new DateTimeType(); 3801 this.issued.setValue(value); 3802 } 3803 return this; 3804 } 3805 3806 /** 3807 * @return {@link #participant} (A participant in the test execution, either the 3808 * execution engine, a client, or a server.) 3809 */ 3810 public List<TestReportParticipantComponent> getParticipant() { 3811 if (this.participant == null) 3812 this.participant = new ArrayList<TestReportParticipantComponent>(); 3813 return this.participant; 3814 } 3815 3816 /** 3817 * @return Returns a reference to <code>this</code> for easy method chaining 3818 */ 3819 public TestReport setParticipant(List<TestReportParticipantComponent> theParticipant) { 3820 this.participant = theParticipant; 3821 return this; 3822 } 3823 3824 public boolean hasParticipant() { 3825 if (this.participant == null) 3826 return false; 3827 for (TestReportParticipantComponent item : this.participant) 3828 if (!item.isEmpty()) 3829 return true; 3830 return false; 3831 } 3832 3833 public TestReportParticipantComponent addParticipant() { // 3 3834 TestReportParticipantComponent t = new TestReportParticipantComponent(); 3835 if (this.participant == null) 3836 this.participant = new ArrayList<TestReportParticipantComponent>(); 3837 this.participant.add(t); 3838 return t; 3839 } 3840 3841 public TestReport addParticipant(TestReportParticipantComponent t) { // 3 3842 if (t == null) 3843 return this; 3844 if (this.participant == null) 3845 this.participant = new ArrayList<TestReportParticipantComponent>(); 3846 this.participant.add(t); 3847 return this; 3848 } 3849 3850 /** 3851 * @return The first repetition of repeating field {@link #participant}, 3852 * creating it if it does not already exist 3853 */ 3854 public TestReportParticipantComponent getParticipantFirstRep() { 3855 if (getParticipant().isEmpty()) { 3856 addParticipant(); 3857 } 3858 return getParticipant().get(0); 3859 } 3860 3861 /** 3862 * @return {@link #setup} (The results of the series of required setup 3863 * operations before the tests were executed.) 3864 */ 3865 public TestReportSetupComponent getSetup() { 3866 if (this.setup == null) 3867 if (Configuration.errorOnAutoCreate()) 3868 throw new Error("Attempt to auto-create TestReport.setup"); 3869 else if (Configuration.doAutoCreate()) 3870 this.setup = new TestReportSetupComponent(); // cc 3871 return this.setup; 3872 } 3873 3874 public boolean hasSetup() { 3875 return this.setup != null && !this.setup.isEmpty(); 3876 } 3877 3878 /** 3879 * @param value {@link #setup} (The results of the series of required setup 3880 * operations before the tests were executed.) 3881 */ 3882 public TestReport setSetup(TestReportSetupComponent value) { 3883 this.setup = value; 3884 return this; 3885 } 3886 3887 /** 3888 * @return {@link #test} (A test executed from the test script.) 3889 */ 3890 public List<TestReportTestComponent> getTest() { 3891 if (this.test == null) 3892 this.test = new ArrayList<TestReportTestComponent>(); 3893 return this.test; 3894 } 3895 3896 /** 3897 * @return Returns a reference to <code>this</code> for easy method chaining 3898 */ 3899 public TestReport setTest(List<TestReportTestComponent> theTest) { 3900 this.test = theTest; 3901 return this; 3902 } 3903 3904 public boolean hasTest() { 3905 if (this.test == null) 3906 return false; 3907 for (TestReportTestComponent item : this.test) 3908 if (!item.isEmpty()) 3909 return true; 3910 return false; 3911 } 3912 3913 public TestReportTestComponent addTest() { // 3 3914 TestReportTestComponent t = new TestReportTestComponent(); 3915 if (this.test == null) 3916 this.test = new ArrayList<TestReportTestComponent>(); 3917 this.test.add(t); 3918 return t; 3919 } 3920 3921 public TestReport addTest(TestReportTestComponent t) { // 3 3922 if (t == null) 3923 return this; 3924 if (this.test == null) 3925 this.test = new ArrayList<TestReportTestComponent>(); 3926 this.test.add(t); 3927 return this; 3928 } 3929 3930 /** 3931 * @return The first repetition of repeating field {@link #test}, creating it if 3932 * it does not already exist 3933 */ 3934 public TestReportTestComponent getTestFirstRep() { 3935 if (getTest().isEmpty()) { 3936 addTest(); 3937 } 3938 return getTest().get(0); 3939 } 3940 3941 /** 3942 * @return {@link #teardown} (The results of the series of operations required 3943 * to clean up after all the tests were executed (successfully or 3944 * otherwise).) 3945 */ 3946 public TestReportTeardownComponent getTeardown() { 3947 if (this.teardown == null) 3948 if (Configuration.errorOnAutoCreate()) 3949 throw new Error("Attempt to auto-create TestReport.teardown"); 3950 else if (Configuration.doAutoCreate()) 3951 this.teardown = new TestReportTeardownComponent(); // cc 3952 return this.teardown; 3953 } 3954 3955 public boolean hasTeardown() { 3956 return this.teardown != null && !this.teardown.isEmpty(); 3957 } 3958 3959 /** 3960 * @param value {@link #teardown} (The results of the series of operations 3961 * required to clean up after all the tests were executed 3962 * (successfully or otherwise).) 3963 */ 3964 public TestReport setTeardown(TestReportTeardownComponent value) { 3965 this.teardown = value; 3966 return this; 3967 } 3968 3969 protected void listChildren(List<Property> children) { 3970 super.listChildren(children); 3971 children.add(new Property("identifier", "Identifier", 3972 "Identifier for the TestScript assigned for external purposes outside the context of FHIR.", 0, 1, identifier)); 3973 children.add(new Property("name", "string", 3974 "A free text natural language name identifying the executed TestScript.", 0, 1, name)); 3975 children.add(new Property("status", "code", "The current state of this test report.", 0, 1, status)); 3976 children.add(new Property("testScript", "Reference(TestScript)", 3977 "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.", 3978 0, 1, testScript)); 3979 children 3980 .add(new Property("result", "code", "The overall result from the execution of the TestScript.", 0, 1, result)); 3981 children.add(new Property("score", "decimal", 3982 "The final score (percentage of tests passed) resulting from the execution of the TestScript.", 0, 1, score)); 3983 children.add(new Property("tester", "string", 3984 "Name of the tester producing this report (Organization or individual).", 0, 1, tester)); 3985 children.add(new Property("issued", "dateTime", 3986 "When the TestScript was executed and this TestReport was generated.", 0, 1, issued)); 3987 children.add(new Property("participant", "", 3988 "A participant in the test execution, either the execution engine, a client, or a server.", 0, 3989 java.lang.Integer.MAX_VALUE, participant)); 3990 children.add(new Property("setup", "", 3991 "The results of the series of required setup operations before the tests were executed.", 0, 1, setup)); 3992 children 3993 .add(new Property("test", "", "A test executed from the test script.", 0, java.lang.Integer.MAX_VALUE, test)); 3994 children.add(new Property("teardown", "", 3995 "The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).", 3996 0, 1, teardown)); 3997 } 3998 3999 @Override 4000 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4001 switch (_hash) { 4002 case -1618432855: 4003 /* identifier */ return new Property("identifier", "Identifier", 4004 "Identifier for the TestScript assigned for external purposes outside the context of FHIR.", 0, 1, 4005 identifier); 4006 case 3373707: 4007 /* name */ return new Property("name", "string", 4008 "A free text natural language name identifying the executed TestScript.", 0, 1, name); 4009 case -892481550: 4010 /* status */ return new Property("status", "code", "The current state of this test report.", 0, 1, status); 4011 case 1712049149: 4012 /* testScript */ return new Property("testScript", "Reference(TestScript)", 4013 "Ideally this is an absolute URL that is used to identify the version-specific TestScript that was executed, matching the `TestScript.url`.", 4014 0, 1, testScript); 4015 case -934426595: 4016 /* result */ return new Property("result", "code", "The overall result from the execution of the TestScript.", 0, 4017 1, result); 4018 case 109264530: 4019 /* score */ return new Property("score", "decimal", 4020 "The final score (percentage of tests passed) resulting from the execution of the TestScript.", 0, 1, score); 4021 case -877169473: 4022 /* tester */ return new Property("tester", "string", 4023 "Name of the tester producing this report (Organization or individual).", 0, 1, tester); 4024 case -1179159893: 4025 /* issued */ return new Property("issued", "dateTime", 4026 "When the TestScript was executed and this TestReport was generated.", 0, 1, issued); 4027 case 767422259: 4028 /* participant */ return new Property("participant", "", 4029 "A participant in the test execution, either the execution engine, a client, or a server.", 0, 4030 java.lang.Integer.MAX_VALUE, participant); 4031 case 109329021: 4032 /* setup */ return new Property("setup", "", 4033 "The results of the series of required setup operations before the tests were executed.", 0, 1, setup); 4034 case 3556498: 4035 /* test */ return new Property("test", "", "A test executed from the test script.", 0, 4036 java.lang.Integer.MAX_VALUE, test); 4037 case -1663474172: 4038 /* teardown */ return new Property("teardown", "", 4039 "The results of the series of operations required to clean up after all the tests were executed (successfully or otherwise).", 4040 0, 1, teardown); 4041 default: 4042 return super.getNamedProperty(_hash, _name, _checkValid); 4043 } 4044 4045 } 4046 4047 @Override 4048 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4049 switch (hash) { 4050 case -1618432855: 4051 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 4052 case 3373707: 4053 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4054 case -892481550: 4055 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<TestReportStatus> 4056 case 1712049149: 4057 /* testScript */ return this.testScript == null ? new Base[0] : new Base[] { this.testScript }; // Reference 4058 case -934426595: 4059 /* result */ return this.result == null ? new Base[0] : new Base[] { this.result }; // Enumeration<TestReportResult> 4060 case 109264530: 4061 /* score */ return this.score == null ? new Base[0] : new Base[] { this.score }; // DecimalType 4062 case -877169473: 4063 /* tester */ return this.tester == null ? new Base[0] : new Base[] { this.tester }; // StringType 4064 case -1179159893: 4065 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // DateTimeType 4066 case 767422259: 4067 /* participant */ return this.participant == null ? new Base[0] 4068 : this.participant.toArray(new Base[this.participant.size()]); // TestReportParticipantComponent 4069 case 109329021: 4070 /* setup */ return this.setup == null ? new Base[0] : new Base[] { this.setup }; // TestReportSetupComponent 4071 case 3556498: 4072 /* test */ return this.test == null ? new Base[0] : this.test.toArray(new Base[this.test.size()]); // TestReportTestComponent 4073 case -1663474172: 4074 /* teardown */ return this.teardown == null ? new Base[0] : new Base[] { this.teardown }; // TestReportTeardownComponent 4075 default: 4076 return super.getProperty(hash, name, checkValid); 4077 } 4078 4079 } 4080 4081 @Override 4082 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4083 switch (hash) { 4084 case -1618432855: // identifier 4085 this.identifier = castToIdentifier(value); // Identifier 4086 return value; 4087 case 3373707: // name 4088 this.name = castToString(value); // StringType 4089 return value; 4090 case -892481550: // status 4091 value = new TestReportStatusEnumFactory().fromType(castToCode(value)); 4092 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 4093 return value; 4094 case 1712049149: // testScript 4095 this.testScript = castToReference(value); // Reference 4096 return value; 4097 case -934426595: // result 4098 value = new TestReportResultEnumFactory().fromType(castToCode(value)); 4099 this.result = (Enumeration) value; // Enumeration<TestReportResult> 4100 return value; 4101 case 109264530: // score 4102 this.score = castToDecimal(value); // DecimalType 4103 return value; 4104 case -877169473: // tester 4105 this.tester = castToString(value); // StringType 4106 return value; 4107 case -1179159893: // issued 4108 this.issued = castToDateTime(value); // DateTimeType 4109 return value; 4110 case 767422259: // participant 4111 this.getParticipant().add((TestReportParticipantComponent) value); // TestReportParticipantComponent 4112 return value; 4113 case 109329021: // setup 4114 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 4115 return value; 4116 case 3556498: // test 4117 this.getTest().add((TestReportTestComponent) value); // TestReportTestComponent 4118 return value; 4119 case -1663474172: // teardown 4120 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 4121 return value; 4122 default: 4123 return super.setProperty(hash, name, value); 4124 } 4125 4126 } 4127 4128 @Override 4129 public Base setProperty(String name, Base value) throws FHIRException { 4130 if (name.equals("identifier")) { 4131 this.identifier = castToIdentifier(value); // Identifier 4132 } else if (name.equals("name")) { 4133 this.name = castToString(value); // StringType 4134 } else if (name.equals("status")) { 4135 value = new TestReportStatusEnumFactory().fromType(castToCode(value)); 4136 this.status = (Enumeration) value; // Enumeration<TestReportStatus> 4137 } else if (name.equals("testScript")) { 4138 this.testScript = castToReference(value); // Reference 4139 } else if (name.equals("result")) { 4140 value = new TestReportResultEnumFactory().fromType(castToCode(value)); 4141 this.result = (Enumeration) value; // Enumeration<TestReportResult> 4142 } else if (name.equals("score")) { 4143 this.score = castToDecimal(value); // DecimalType 4144 } else if (name.equals("tester")) { 4145 this.tester = castToString(value); // StringType 4146 } else if (name.equals("issued")) { 4147 this.issued = castToDateTime(value); // DateTimeType 4148 } else if (name.equals("participant")) { 4149 this.getParticipant().add((TestReportParticipantComponent) value); 4150 } else if (name.equals("setup")) { 4151 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 4152 } else if (name.equals("test")) { 4153 this.getTest().add((TestReportTestComponent) value); 4154 } else if (name.equals("teardown")) { 4155 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 4156 } else 4157 return super.setProperty(name, value); 4158 return value; 4159 } 4160 4161 @Override 4162 public void removeChild(String name, Base value) throws FHIRException { 4163 if (name.equals("identifier")) { 4164 this.identifier = null; 4165 } else if (name.equals("name")) { 4166 this.name = null; 4167 } else if (name.equals("status")) { 4168 this.status = null; 4169 } else if (name.equals("testScript")) { 4170 this.testScript = null; 4171 } else if (name.equals("result")) { 4172 this.result = null; 4173 } else if (name.equals("score")) { 4174 this.score = null; 4175 } else if (name.equals("tester")) { 4176 this.tester = null; 4177 } else if (name.equals("issued")) { 4178 this.issued = null; 4179 } else if (name.equals("participant")) { 4180 this.getParticipant().remove((TestReportParticipantComponent) value); 4181 } else if (name.equals("setup")) { 4182 this.setup = (TestReportSetupComponent) value; // TestReportSetupComponent 4183 } else if (name.equals("test")) { 4184 this.getTest().remove((TestReportTestComponent) value); 4185 } else if (name.equals("teardown")) { 4186 this.teardown = (TestReportTeardownComponent) value; // TestReportTeardownComponent 4187 } else 4188 super.removeChild(name, value); 4189 4190 } 4191 4192 @Override 4193 public Base makeProperty(int hash, String name) throws FHIRException { 4194 switch (hash) { 4195 case -1618432855: 4196 return getIdentifier(); 4197 case 3373707: 4198 return getNameElement(); 4199 case -892481550: 4200 return getStatusElement(); 4201 case 1712049149: 4202 return getTestScript(); 4203 case -934426595: 4204 return getResultElement(); 4205 case 109264530: 4206 return getScoreElement(); 4207 case -877169473: 4208 return getTesterElement(); 4209 case -1179159893: 4210 return getIssuedElement(); 4211 case 767422259: 4212 return addParticipant(); 4213 case 109329021: 4214 return getSetup(); 4215 case 3556498: 4216 return addTest(); 4217 case -1663474172: 4218 return getTeardown(); 4219 default: 4220 return super.makeProperty(hash, name); 4221 } 4222 4223 } 4224 4225 @Override 4226 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4227 switch (hash) { 4228 case -1618432855: 4229 /* identifier */ return new String[] { "Identifier" }; 4230 case 3373707: 4231 /* name */ return new String[] { "string" }; 4232 case -892481550: 4233 /* status */ return new String[] { "code" }; 4234 case 1712049149: 4235 /* testScript */ return new String[] { "Reference" }; 4236 case -934426595: 4237 /* result */ return new String[] { "code" }; 4238 case 109264530: 4239 /* score */ return new String[] { "decimal" }; 4240 case -877169473: 4241 /* tester */ return new String[] { "string" }; 4242 case -1179159893: 4243 /* issued */ return new String[] { "dateTime" }; 4244 case 767422259: 4245 /* participant */ return new String[] {}; 4246 case 109329021: 4247 /* setup */ return new String[] {}; 4248 case 3556498: 4249 /* test */ return new String[] {}; 4250 case -1663474172: 4251 /* teardown */ return new String[] {}; 4252 default: 4253 return super.getTypesForProperty(hash, name); 4254 } 4255 4256 } 4257 4258 @Override 4259 public Base addChild(String name) throws FHIRException { 4260 if (name.equals("identifier")) { 4261 this.identifier = new Identifier(); 4262 return this.identifier; 4263 } else if (name.equals("name")) { 4264 throw new FHIRException("Cannot call addChild on a singleton property TestReport.name"); 4265 } else if (name.equals("status")) { 4266 throw new FHIRException("Cannot call addChild on a singleton property TestReport.status"); 4267 } else if (name.equals("testScript")) { 4268 this.testScript = new Reference(); 4269 return this.testScript; 4270 } else if (name.equals("result")) { 4271 throw new FHIRException("Cannot call addChild on a singleton property TestReport.result"); 4272 } else if (name.equals("score")) { 4273 throw new FHIRException("Cannot call addChild on a singleton property TestReport.score"); 4274 } else if (name.equals("tester")) { 4275 throw new FHIRException("Cannot call addChild on a singleton property TestReport.tester"); 4276 } else if (name.equals("issued")) { 4277 throw new FHIRException("Cannot call addChild on a singleton property TestReport.issued"); 4278 } else if (name.equals("participant")) { 4279 return addParticipant(); 4280 } else if (name.equals("setup")) { 4281 this.setup = new TestReportSetupComponent(); 4282 return this.setup; 4283 } else if (name.equals("test")) { 4284 return addTest(); 4285 } else if (name.equals("teardown")) { 4286 this.teardown = new TestReportTeardownComponent(); 4287 return this.teardown; 4288 } else 4289 return super.addChild(name); 4290 } 4291 4292 public String fhirType() { 4293 return "TestReport"; 4294 4295 } 4296 4297 public TestReport copy() { 4298 TestReport dst = new TestReport(); 4299 copyValues(dst); 4300 return dst; 4301 } 4302 4303 public void copyValues(TestReport dst) { 4304 super.copyValues(dst); 4305 dst.identifier = identifier == null ? null : identifier.copy(); 4306 dst.name = name == null ? null : name.copy(); 4307 dst.status = status == null ? null : status.copy(); 4308 dst.testScript = testScript == null ? null : testScript.copy(); 4309 dst.result = result == null ? null : result.copy(); 4310 dst.score = score == null ? null : score.copy(); 4311 dst.tester = tester == null ? null : tester.copy(); 4312 dst.issued = issued == null ? null : issued.copy(); 4313 if (participant != null) { 4314 dst.participant = new ArrayList<TestReportParticipantComponent>(); 4315 for (TestReportParticipantComponent i : participant) 4316 dst.participant.add(i.copy()); 4317 } 4318 ; 4319 dst.setup = setup == null ? null : setup.copy(); 4320 if (test != null) { 4321 dst.test = new ArrayList<TestReportTestComponent>(); 4322 for (TestReportTestComponent i : test) 4323 dst.test.add(i.copy()); 4324 } 4325 ; 4326 dst.teardown = teardown == null ? null : teardown.copy(); 4327 } 4328 4329 protected TestReport typedCopy() { 4330 return copy(); 4331 } 4332 4333 @Override 4334 public boolean equalsDeep(Base other_) { 4335 if (!super.equalsDeep(other_)) 4336 return false; 4337 if (!(other_ instanceof TestReport)) 4338 return false; 4339 TestReport o = (TestReport) other_; 4340 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 4341 && compareDeep(status, o.status, true) && compareDeep(testScript, o.testScript, true) 4342 && compareDeep(result, o.result, true) && compareDeep(score, o.score, true) 4343 && compareDeep(tester, o.tester, true) && compareDeep(issued, o.issued, true) 4344 && compareDeep(participant, o.participant, true) && compareDeep(setup, o.setup, true) 4345 && compareDeep(test, o.test, true) && compareDeep(teardown, o.teardown, true); 4346 } 4347 4348 @Override 4349 public boolean equalsShallow(Base other_) { 4350 if (!super.equalsShallow(other_)) 4351 return false; 4352 if (!(other_ instanceof TestReport)) 4353 return false; 4354 TestReport o = (TestReport) other_; 4355 return compareValues(name, o.name, true) && compareValues(status, o.status, true) 4356 && compareValues(result, o.result, true) && compareValues(score, o.score, true) 4357 && compareValues(tester, o.tester, true) && compareValues(issued, o.issued, true); 4358 } 4359 4360 public boolean isEmpty() { 4361 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, status, testScript, result, score, 4362 tester, issued, participant, setup, test, teardown); 4363 } 4364 4365 @Override 4366 public ResourceType getResourceType() { 4367 return ResourceType.TestReport; 4368 } 4369 4370 /** 4371 * Search parameter: <b>result</b> 4372 * <p> 4373 * Description: <b>The result disposition of the test execution</b><br> 4374 * Type: <b>token</b><br> 4375 * Path: <b>TestReport.result</b><br> 4376 * </p> 4377 */ 4378 @SearchParamDefinition(name = "result", path = "TestReport.result", description = "The result disposition of the test execution", type = "token") 4379 public static final String SP_RESULT = "result"; 4380 /** 4381 * <b>Fluent Client</b> search parameter constant for <b>result</b> 4382 * <p> 4383 * Description: <b>The result disposition of the test execution</b><br> 4384 * Type: <b>token</b><br> 4385 * Path: <b>TestReport.result</b><br> 4386 * </p> 4387 */ 4388 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESULT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4389 SP_RESULT); 4390 4391 /** 4392 * Search parameter: <b>identifier</b> 4393 * <p> 4394 * Description: <b>An external identifier for the test report</b><br> 4395 * Type: <b>token</b><br> 4396 * Path: <b>TestReport.identifier</b><br> 4397 * </p> 4398 */ 4399 @SearchParamDefinition(name = "identifier", path = "TestReport.identifier", description = "An external identifier for the test report", type = "token") 4400 public static final String SP_IDENTIFIER = "identifier"; 4401 /** 4402 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4403 * <p> 4404 * Description: <b>An external identifier for the test report</b><br> 4405 * Type: <b>token</b><br> 4406 * Path: <b>TestReport.identifier</b><br> 4407 * </p> 4408 */ 4409 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4410 SP_IDENTIFIER); 4411 4412 /** 4413 * Search parameter: <b>tester</b> 4414 * <p> 4415 * Description: <b>The name of the testing organization</b><br> 4416 * Type: <b>string</b><br> 4417 * Path: <b>TestReport.tester</b><br> 4418 * </p> 4419 */ 4420 @SearchParamDefinition(name = "tester", path = "TestReport.tester", description = "The name of the testing organization", type = "string") 4421 public static final String SP_TESTER = "tester"; 4422 /** 4423 * <b>Fluent Client</b> search parameter constant for <b>tester</b> 4424 * <p> 4425 * Description: <b>The name of the testing organization</b><br> 4426 * Type: <b>string</b><br> 4427 * Path: <b>TestReport.tester</b><br> 4428 * </p> 4429 */ 4430 public static final ca.uhn.fhir.rest.gclient.StringClientParam TESTER = new ca.uhn.fhir.rest.gclient.StringClientParam( 4431 SP_TESTER); 4432 4433 /** 4434 * Search parameter: <b>testscript</b> 4435 * <p> 4436 * Description: <b>The test script executed to produce this report</b><br> 4437 * Type: <b>reference</b><br> 4438 * Path: <b>TestReport.testScript</b><br> 4439 * </p> 4440 */ 4441 @SearchParamDefinition(name = "testscript", path = "TestReport.testScript", description = "The test script executed to produce this report", type = "reference", target = { 4442 TestScript.class }) 4443 public static final String SP_TESTSCRIPT = "testscript"; 4444 /** 4445 * <b>Fluent Client</b> search parameter constant for <b>testscript</b> 4446 * <p> 4447 * Description: <b>The test script executed to produce this report</b><br> 4448 * Type: <b>reference</b><br> 4449 * Path: <b>TestReport.testScript</b><br> 4450 * </p> 4451 */ 4452 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TESTSCRIPT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4453 SP_TESTSCRIPT); 4454 4455 /** 4456 * Constant for fluent queries to be used to add include statements. Specifies 4457 * the path value of "<b>TestReport:testscript</b>". 4458 */ 4459 public static final ca.uhn.fhir.model.api.Include INCLUDE_TESTSCRIPT = new ca.uhn.fhir.model.api.Include( 4460 "TestReport:testscript").toLocked(); 4461 4462 /** 4463 * Search parameter: <b>issued</b> 4464 * <p> 4465 * Description: <b>The test report generation date</b><br> 4466 * Type: <b>date</b><br> 4467 * Path: <b>TestReport.issued</b><br> 4468 * </p> 4469 */ 4470 @SearchParamDefinition(name = "issued", path = "TestReport.issued", description = "The test report generation date", type = "date") 4471 public static final String SP_ISSUED = "issued"; 4472 /** 4473 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 4474 * <p> 4475 * Description: <b>The test report generation date</b><br> 4476 * Type: <b>date</b><br> 4477 * Path: <b>TestReport.issued</b><br> 4478 * </p> 4479 */ 4480 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam( 4481 SP_ISSUED); 4482 4483 /** 4484 * Search parameter: <b>participant</b> 4485 * <p> 4486 * Description: <b>The reference to a participant in the test execution</b><br> 4487 * Type: <b>uri</b><br> 4488 * Path: <b>TestReport.participant.uri</b><br> 4489 * </p> 4490 */ 4491 @SearchParamDefinition(name = "participant", path = "TestReport.participant.uri", description = "The reference to a participant in the test execution", type = "uri") 4492 public static final String SP_PARTICIPANT = "participant"; 4493 /** 4494 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 4495 * <p> 4496 * Description: <b>The reference to a participant in the test execution</b><br> 4497 * Type: <b>uri</b><br> 4498 * Path: <b>TestReport.participant.uri</b><br> 4499 * </p> 4500 */ 4501 public static final ca.uhn.fhir.rest.gclient.UriClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.UriClientParam( 4502 SP_PARTICIPANT); 4503 4504}