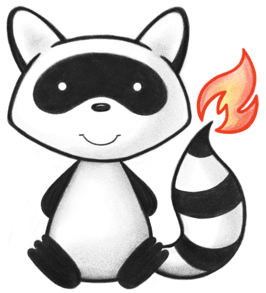
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A structured set of tests against a FHIR server or client implementation to 052 * determine compliance against the FHIR specification. 053 */ 054@ResourceDef(name = "TestScript", profile = "http://hl7.org/fhir/StructureDefinition/TestScript") 055@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", 056 "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "origin", "destination", "metadata", 057 "fixture", "profile", "variable", "setup", "test", "teardown" }) 058public class TestScript extends MetadataResource { 059 060 public enum TestScriptRequestMethodCode { 061 /** 062 * HTTP DELETE operation. 063 */ 064 DELETE, 065 /** 066 * HTTP GET operation. 067 */ 068 GET, 069 /** 070 * HTTP OPTIONS operation. 071 */ 072 OPTIONS, 073 /** 074 * HTTP PATCH operation. 075 */ 076 PATCH, 077 /** 078 * HTTP POST operation. 079 */ 080 POST, 081 /** 082 * HTTP PUT operation. 083 */ 084 PUT, 085 /** 086 * HTTP HEAD operation. 087 */ 088 HEAD, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 094 public static TestScriptRequestMethodCode fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("delete".equals(codeString)) 098 return DELETE; 099 if ("get".equals(codeString)) 100 return GET; 101 if ("options".equals(codeString)) 102 return OPTIONS; 103 if ("patch".equals(codeString)) 104 return PATCH; 105 if ("post".equals(codeString)) 106 return POST; 107 if ("put".equals(codeString)) 108 return PUT; 109 if ("head".equals(codeString)) 110 return HEAD; 111 if (Configuration.isAcceptInvalidEnums()) 112 return null; 113 else 114 throw new FHIRException("Unknown TestScriptRequestMethodCode code '" + codeString + "'"); 115 } 116 117 public String toCode() { 118 switch (this) { 119 case DELETE: 120 return "delete"; 121 case GET: 122 return "get"; 123 case OPTIONS: 124 return "options"; 125 case PATCH: 126 return "patch"; 127 case POST: 128 return "post"; 129 case PUT: 130 return "put"; 131 case HEAD: 132 return "head"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getSystem() { 141 switch (this) { 142 case DELETE: 143 return "http://hl7.org/fhir/http-operations"; 144 case GET: 145 return "http://hl7.org/fhir/http-operations"; 146 case OPTIONS: 147 return "http://hl7.org/fhir/http-operations"; 148 case PATCH: 149 return "http://hl7.org/fhir/http-operations"; 150 case POST: 151 return "http://hl7.org/fhir/http-operations"; 152 case PUT: 153 return "http://hl7.org/fhir/http-operations"; 154 case HEAD: 155 return "http://hl7.org/fhir/http-operations"; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 163 public String getDefinition() { 164 switch (this) { 165 case DELETE: 166 return "HTTP DELETE operation."; 167 case GET: 168 return "HTTP GET operation."; 169 case OPTIONS: 170 return "HTTP OPTIONS operation."; 171 case PATCH: 172 return "HTTP PATCH operation."; 173 case POST: 174 return "HTTP POST operation."; 175 case PUT: 176 return "HTTP PUT operation."; 177 case HEAD: 178 return "HTTP HEAD operation."; 179 case NULL: 180 return null; 181 default: 182 return "?"; 183 } 184 } 185 186 public String getDisplay() { 187 switch (this) { 188 case DELETE: 189 return "DELETE"; 190 case GET: 191 return "GET"; 192 case OPTIONS: 193 return "OPTIONS"; 194 case PATCH: 195 return "PATCH"; 196 case POST: 197 return "POST"; 198 case PUT: 199 return "PUT"; 200 case HEAD: 201 return "HEAD"; 202 case NULL: 203 return null; 204 default: 205 return "?"; 206 } 207 } 208 } 209 210 public static class TestScriptRequestMethodCodeEnumFactory implements EnumFactory<TestScriptRequestMethodCode> { 211 public TestScriptRequestMethodCode fromCode(String codeString) throws IllegalArgumentException { 212 if (codeString == null || "".equals(codeString)) 213 if (codeString == null || "".equals(codeString)) 214 return null; 215 if ("delete".equals(codeString)) 216 return TestScriptRequestMethodCode.DELETE; 217 if ("get".equals(codeString)) 218 return TestScriptRequestMethodCode.GET; 219 if ("options".equals(codeString)) 220 return TestScriptRequestMethodCode.OPTIONS; 221 if ("patch".equals(codeString)) 222 return TestScriptRequestMethodCode.PATCH; 223 if ("post".equals(codeString)) 224 return TestScriptRequestMethodCode.POST; 225 if ("put".equals(codeString)) 226 return TestScriptRequestMethodCode.PUT; 227 if ("head".equals(codeString)) 228 return TestScriptRequestMethodCode.HEAD; 229 throw new IllegalArgumentException("Unknown TestScriptRequestMethodCode code '" + codeString + "'"); 230 } 231 232 public Enumeration<TestScriptRequestMethodCode> fromType(PrimitiveType<?> code) throws FHIRException { 233 if (code == null) 234 return null; 235 if (code.isEmpty()) 236 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.NULL, code); 237 String codeString = code.asStringValue(); 238 if (codeString == null || "".equals(codeString)) 239 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.NULL, code); 240 if ("delete".equals(codeString)) 241 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.DELETE, code); 242 if ("get".equals(codeString)) 243 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.GET, code); 244 if ("options".equals(codeString)) 245 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.OPTIONS, code); 246 if ("patch".equals(codeString)) 247 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.PATCH, code); 248 if ("post".equals(codeString)) 249 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.POST, code); 250 if ("put".equals(codeString)) 251 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.PUT, code); 252 if ("head".equals(codeString)) 253 return new Enumeration<TestScriptRequestMethodCode>(this, TestScriptRequestMethodCode.HEAD, code); 254 throw new FHIRException("Unknown TestScriptRequestMethodCode code '" + codeString + "'"); 255 } 256 257 public String toCode(TestScriptRequestMethodCode code) { 258 if (code == TestScriptRequestMethodCode.NULL) 259 return null; 260 if (code == TestScriptRequestMethodCode.DELETE) 261 return "delete"; 262 if (code == TestScriptRequestMethodCode.GET) 263 return "get"; 264 if (code == TestScriptRequestMethodCode.OPTIONS) 265 return "options"; 266 if (code == TestScriptRequestMethodCode.PATCH) 267 return "patch"; 268 if (code == TestScriptRequestMethodCode.POST) 269 return "post"; 270 if (code == TestScriptRequestMethodCode.PUT) 271 return "put"; 272 if (code == TestScriptRequestMethodCode.HEAD) 273 return "head"; 274 return "?"; 275 } 276 277 public String toSystem(TestScriptRequestMethodCode code) { 278 return code.getSystem(); 279 } 280 } 281 282 public enum AssertionDirectionType { 283 /** 284 * The assertion is evaluated on the response. This is the default value. 285 */ 286 RESPONSE, 287 /** 288 * The assertion is evaluated on the request. 289 */ 290 REQUEST, 291 /** 292 * added to help the parsers with the generic types 293 */ 294 NULL; 295 296 public static AssertionDirectionType fromCode(String codeString) throws FHIRException { 297 if (codeString == null || "".equals(codeString)) 298 return null; 299 if ("response".equals(codeString)) 300 return RESPONSE; 301 if ("request".equals(codeString)) 302 return REQUEST; 303 if (Configuration.isAcceptInvalidEnums()) 304 return null; 305 else 306 throw new FHIRException("Unknown AssertionDirectionType code '" + codeString + "'"); 307 } 308 309 public String toCode() { 310 switch (this) { 311 case RESPONSE: 312 return "response"; 313 case REQUEST: 314 return "request"; 315 case NULL: 316 return null; 317 default: 318 return "?"; 319 } 320 } 321 322 public String getSystem() { 323 switch (this) { 324 case RESPONSE: 325 return "http://hl7.org/fhir/assert-direction-codes"; 326 case REQUEST: 327 return "http://hl7.org/fhir/assert-direction-codes"; 328 case NULL: 329 return null; 330 default: 331 return "?"; 332 } 333 } 334 335 public String getDefinition() { 336 switch (this) { 337 case RESPONSE: 338 return "The assertion is evaluated on the response. This is the default value."; 339 case REQUEST: 340 return "The assertion is evaluated on the request."; 341 case NULL: 342 return null; 343 default: 344 return "?"; 345 } 346 } 347 348 public String getDisplay() { 349 switch (this) { 350 case RESPONSE: 351 return "response"; 352 case REQUEST: 353 return "request"; 354 case NULL: 355 return null; 356 default: 357 return "?"; 358 } 359 } 360 } 361 362 public static class AssertionDirectionTypeEnumFactory implements EnumFactory<AssertionDirectionType> { 363 public AssertionDirectionType fromCode(String codeString) throws IllegalArgumentException { 364 if (codeString == null || "".equals(codeString)) 365 if (codeString == null || "".equals(codeString)) 366 return null; 367 if ("response".equals(codeString)) 368 return AssertionDirectionType.RESPONSE; 369 if ("request".equals(codeString)) 370 return AssertionDirectionType.REQUEST; 371 throw new IllegalArgumentException("Unknown AssertionDirectionType code '" + codeString + "'"); 372 } 373 374 public Enumeration<AssertionDirectionType> fromType(PrimitiveType<?> code) throws FHIRException { 375 if (code == null) 376 return null; 377 if (code.isEmpty()) 378 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.NULL, code); 379 String codeString = code.asStringValue(); 380 if (codeString == null || "".equals(codeString)) 381 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.NULL, code); 382 if ("response".equals(codeString)) 383 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.RESPONSE, code); 384 if ("request".equals(codeString)) 385 return new Enumeration<AssertionDirectionType>(this, AssertionDirectionType.REQUEST, code); 386 throw new FHIRException("Unknown AssertionDirectionType code '" + codeString + "'"); 387 } 388 389 public String toCode(AssertionDirectionType code) { 390 if (code == AssertionDirectionType.NULL) 391 return null; 392 if (code == AssertionDirectionType.RESPONSE) 393 return "response"; 394 if (code == AssertionDirectionType.REQUEST) 395 return "request"; 396 return "?"; 397 } 398 399 public String toSystem(AssertionDirectionType code) { 400 return code.getSystem(); 401 } 402 } 403 404 public enum AssertionOperatorType { 405 /** 406 * Default value. Equals comparison. 407 */ 408 EQUALS, 409 /** 410 * Not equals comparison. 411 */ 412 NOTEQUALS, 413 /** 414 * Compare value within a known set of values. 415 */ 416 IN, 417 /** 418 * Compare value not within a known set of values. 419 */ 420 NOTIN, 421 /** 422 * Compare value to be greater than a known value. 423 */ 424 GREATERTHAN, 425 /** 426 * Compare value to be less than a known value. 427 */ 428 LESSTHAN, 429 /** 430 * Compare value is empty. 431 */ 432 EMPTY, 433 /** 434 * Compare value is not empty. 435 */ 436 NOTEMPTY, 437 /** 438 * Compare value string contains a known value. 439 */ 440 CONTAINS, 441 /** 442 * Compare value string does not contain a known value. 443 */ 444 NOTCONTAINS, 445 /** 446 * Evaluate the FHIRPath expression as a boolean condition. 447 */ 448 EVAL, 449 /** 450 * added to help the parsers with the generic types 451 */ 452 NULL; 453 454 public static AssertionOperatorType fromCode(String codeString) throws FHIRException { 455 if (codeString == null || "".equals(codeString)) 456 return null; 457 if ("equals".equals(codeString)) 458 return EQUALS; 459 if ("notEquals".equals(codeString)) 460 return NOTEQUALS; 461 if ("in".equals(codeString)) 462 return IN; 463 if ("notIn".equals(codeString)) 464 return NOTIN; 465 if ("greaterThan".equals(codeString)) 466 return GREATERTHAN; 467 if ("lessThan".equals(codeString)) 468 return LESSTHAN; 469 if ("empty".equals(codeString)) 470 return EMPTY; 471 if ("notEmpty".equals(codeString)) 472 return NOTEMPTY; 473 if ("contains".equals(codeString)) 474 return CONTAINS; 475 if ("notContains".equals(codeString)) 476 return NOTCONTAINS; 477 if ("eval".equals(codeString)) 478 return EVAL; 479 if (Configuration.isAcceptInvalidEnums()) 480 return null; 481 else 482 throw new FHIRException("Unknown AssertionOperatorType code '" + codeString + "'"); 483 } 484 485 public String toCode() { 486 switch (this) { 487 case EQUALS: 488 return "equals"; 489 case NOTEQUALS: 490 return "notEquals"; 491 case IN: 492 return "in"; 493 case NOTIN: 494 return "notIn"; 495 case GREATERTHAN: 496 return "greaterThan"; 497 case LESSTHAN: 498 return "lessThan"; 499 case EMPTY: 500 return "empty"; 501 case NOTEMPTY: 502 return "notEmpty"; 503 case CONTAINS: 504 return "contains"; 505 case NOTCONTAINS: 506 return "notContains"; 507 case EVAL: 508 return "eval"; 509 case NULL: 510 return null; 511 default: 512 return "?"; 513 } 514 } 515 516 public String getSystem() { 517 switch (this) { 518 case EQUALS: 519 return "http://hl7.org/fhir/assert-operator-codes"; 520 case NOTEQUALS: 521 return "http://hl7.org/fhir/assert-operator-codes"; 522 case IN: 523 return "http://hl7.org/fhir/assert-operator-codes"; 524 case NOTIN: 525 return "http://hl7.org/fhir/assert-operator-codes"; 526 case GREATERTHAN: 527 return "http://hl7.org/fhir/assert-operator-codes"; 528 case LESSTHAN: 529 return "http://hl7.org/fhir/assert-operator-codes"; 530 case EMPTY: 531 return "http://hl7.org/fhir/assert-operator-codes"; 532 case NOTEMPTY: 533 return "http://hl7.org/fhir/assert-operator-codes"; 534 case CONTAINS: 535 return "http://hl7.org/fhir/assert-operator-codes"; 536 case NOTCONTAINS: 537 return "http://hl7.org/fhir/assert-operator-codes"; 538 case EVAL: 539 return "http://hl7.org/fhir/assert-operator-codes"; 540 case NULL: 541 return null; 542 default: 543 return "?"; 544 } 545 } 546 547 public String getDefinition() { 548 switch (this) { 549 case EQUALS: 550 return "Default value. Equals comparison."; 551 case NOTEQUALS: 552 return "Not equals comparison."; 553 case IN: 554 return "Compare value within a known set of values."; 555 case NOTIN: 556 return "Compare value not within a known set of values."; 557 case GREATERTHAN: 558 return "Compare value to be greater than a known value."; 559 case LESSTHAN: 560 return "Compare value to be less than a known value."; 561 case EMPTY: 562 return "Compare value is empty."; 563 case NOTEMPTY: 564 return "Compare value is not empty."; 565 case CONTAINS: 566 return "Compare value string contains a known value."; 567 case NOTCONTAINS: 568 return "Compare value string does not contain a known value."; 569 case EVAL: 570 return "Evaluate the FHIRPath expression as a boolean condition."; 571 case NULL: 572 return null; 573 default: 574 return "?"; 575 } 576 } 577 578 public String getDisplay() { 579 switch (this) { 580 case EQUALS: 581 return "equals"; 582 case NOTEQUALS: 583 return "notEquals"; 584 case IN: 585 return "in"; 586 case NOTIN: 587 return "notIn"; 588 case GREATERTHAN: 589 return "greaterThan"; 590 case LESSTHAN: 591 return "lessThan"; 592 case EMPTY: 593 return "empty"; 594 case NOTEMPTY: 595 return "notEmpty"; 596 case CONTAINS: 597 return "contains"; 598 case NOTCONTAINS: 599 return "notContains"; 600 case EVAL: 601 return "evaluate"; 602 case NULL: 603 return null; 604 default: 605 return "?"; 606 } 607 } 608 } 609 610 public static class AssertionOperatorTypeEnumFactory implements EnumFactory<AssertionOperatorType> { 611 public AssertionOperatorType fromCode(String codeString) throws IllegalArgumentException { 612 if (codeString == null || "".equals(codeString)) 613 if (codeString == null || "".equals(codeString)) 614 return null; 615 if ("equals".equals(codeString)) 616 return AssertionOperatorType.EQUALS; 617 if ("notEquals".equals(codeString)) 618 return AssertionOperatorType.NOTEQUALS; 619 if ("in".equals(codeString)) 620 return AssertionOperatorType.IN; 621 if ("notIn".equals(codeString)) 622 return AssertionOperatorType.NOTIN; 623 if ("greaterThan".equals(codeString)) 624 return AssertionOperatorType.GREATERTHAN; 625 if ("lessThan".equals(codeString)) 626 return AssertionOperatorType.LESSTHAN; 627 if ("empty".equals(codeString)) 628 return AssertionOperatorType.EMPTY; 629 if ("notEmpty".equals(codeString)) 630 return AssertionOperatorType.NOTEMPTY; 631 if ("contains".equals(codeString)) 632 return AssertionOperatorType.CONTAINS; 633 if ("notContains".equals(codeString)) 634 return AssertionOperatorType.NOTCONTAINS; 635 if ("eval".equals(codeString)) 636 return AssertionOperatorType.EVAL; 637 throw new IllegalArgumentException("Unknown AssertionOperatorType code '" + codeString + "'"); 638 } 639 640 public Enumeration<AssertionOperatorType> fromType(PrimitiveType<?> code) throws FHIRException { 641 if (code == null) 642 return null; 643 if (code.isEmpty()) 644 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NULL, code); 645 String codeString = code.asStringValue(); 646 if (codeString == null || "".equals(codeString)) 647 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NULL, code); 648 if ("equals".equals(codeString)) 649 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EQUALS, code); 650 if ("notEquals".equals(codeString)) 651 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTEQUALS, code); 652 if ("in".equals(codeString)) 653 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.IN, code); 654 if ("notIn".equals(codeString)) 655 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTIN, code); 656 if ("greaterThan".equals(codeString)) 657 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.GREATERTHAN, code); 658 if ("lessThan".equals(codeString)) 659 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.LESSTHAN, code); 660 if ("empty".equals(codeString)) 661 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EMPTY, code); 662 if ("notEmpty".equals(codeString)) 663 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTEMPTY, code); 664 if ("contains".equals(codeString)) 665 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.CONTAINS, code); 666 if ("notContains".equals(codeString)) 667 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.NOTCONTAINS, code); 668 if ("eval".equals(codeString)) 669 return new Enumeration<AssertionOperatorType>(this, AssertionOperatorType.EVAL, code); 670 throw new FHIRException("Unknown AssertionOperatorType code '" + codeString + "'"); 671 } 672 673 public String toCode(AssertionOperatorType code) { 674 if (code == AssertionOperatorType.NULL) 675 return null; 676 if (code == AssertionOperatorType.EQUALS) 677 return "equals"; 678 if (code == AssertionOperatorType.NOTEQUALS) 679 return "notEquals"; 680 if (code == AssertionOperatorType.IN) 681 return "in"; 682 if (code == AssertionOperatorType.NOTIN) 683 return "notIn"; 684 if (code == AssertionOperatorType.GREATERTHAN) 685 return "greaterThan"; 686 if (code == AssertionOperatorType.LESSTHAN) 687 return "lessThan"; 688 if (code == AssertionOperatorType.EMPTY) 689 return "empty"; 690 if (code == AssertionOperatorType.NOTEMPTY) 691 return "notEmpty"; 692 if (code == AssertionOperatorType.CONTAINS) 693 return "contains"; 694 if (code == AssertionOperatorType.NOTCONTAINS) 695 return "notContains"; 696 if (code == AssertionOperatorType.EVAL) 697 return "eval"; 698 return "?"; 699 } 700 701 public String toSystem(AssertionOperatorType code) { 702 return code.getSystem(); 703 } 704 } 705 706 public enum AssertionResponseTypes { 707 /** 708 * Response code is 200. 709 */ 710 OKAY, 711 /** 712 * Response code is 201. 713 */ 714 CREATED, 715 /** 716 * Response code is 204. 717 */ 718 NOCONTENT, 719 /** 720 * Response code is 304. 721 */ 722 NOTMODIFIED, 723 /** 724 * Response code is 400. 725 */ 726 BAD, 727 /** 728 * Response code is 403. 729 */ 730 FORBIDDEN, 731 /** 732 * Response code is 404. 733 */ 734 NOTFOUND, 735 /** 736 * Response code is 405. 737 */ 738 METHODNOTALLOWED, 739 /** 740 * Response code is 409. 741 */ 742 CONFLICT, 743 /** 744 * Response code is 410. 745 */ 746 GONE, 747 /** 748 * Response code is 412. 749 */ 750 PRECONDITIONFAILED, 751 /** 752 * Response code is 422. 753 */ 754 UNPROCESSABLE, 755 /** 756 * added to help the parsers with the generic types 757 */ 758 NULL; 759 760 public static AssertionResponseTypes fromCode(String codeString) throws FHIRException { 761 if (codeString == null || "".equals(codeString)) 762 return null; 763 if ("okay".equals(codeString)) 764 return OKAY; 765 if ("created".equals(codeString)) 766 return CREATED; 767 if ("noContent".equals(codeString)) 768 return NOCONTENT; 769 if ("notModified".equals(codeString)) 770 return NOTMODIFIED; 771 if ("bad".equals(codeString)) 772 return BAD; 773 if ("forbidden".equals(codeString)) 774 return FORBIDDEN; 775 if ("notFound".equals(codeString)) 776 return NOTFOUND; 777 if ("methodNotAllowed".equals(codeString)) 778 return METHODNOTALLOWED; 779 if ("conflict".equals(codeString)) 780 return CONFLICT; 781 if ("gone".equals(codeString)) 782 return GONE; 783 if ("preconditionFailed".equals(codeString)) 784 return PRECONDITIONFAILED; 785 if ("unprocessable".equals(codeString)) 786 return UNPROCESSABLE; 787 if (Configuration.isAcceptInvalidEnums()) 788 return null; 789 else 790 throw new FHIRException("Unknown AssertionResponseTypes code '" + codeString + "'"); 791 } 792 793 public String toCode() { 794 switch (this) { 795 case OKAY: 796 return "okay"; 797 case CREATED: 798 return "created"; 799 case NOCONTENT: 800 return "noContent"; 801 case NOTMODIFIED: 802 return "notModified"; 803 case BAD: 804 return "bad"; 805 case FORBIDDEN: 806 return "forbidden"; 807 case NOTFOUND: 808 return "notFound"; 809 case METHODNOTALLOWED: 810 return "methodNotAllowed"; 811 case CONFLICT: 812 return "conflict"; 813 case GONE: 814 return "gone"; 815 case PRECONDITIONFAILED: 816 return "preconditionFailed"; 817 case UNPROCESSABLE: 818 return "unprocessable"; 819 case NULL: 820 return null; 821 default: 822 return "?"; 823 } 824 } 825 826 public String getSystem() { 827 switch (this) { 828 case OKAY: 829 return "http://hl7.org/fhir/assert-response-code-types"; 830 case CREATED: 831 return "http://hl7.org/fhir/assert-response-code-types"; 832 case NOCONTENT: 833 return "http://hl7.org/fhir/assert-response-code-types"; 834 case NOTMODIFIED: 835 return "http://hl7.org/fhir/assert-response-code-types"; 836 case BAD: 837 return "http://hl7.org/fhir/assert-response-code-types"; 838 case FORBIDDEN: 839 return "http://hl7.org/fhir/assert-response-code-types"; 840 case NOTFOUND: 841 return "http://hl7.org/fhir/assert-response-code-types"; 842 case METHODNOTALLOWED: 843 return "http://hl7.org/fhir/assert-response-code-types"; 844 case CONFLICT: 845 return "http://hl7.org/fhir/assert-response-code-types"; 846 case GONE: 847 return "http://hl7.org/fhir/assert-response-code-types"; 848 case PRECONDITIONFAILED: 849 return "http://hl7.org/fhir/assert-response-code-types"; 850 case UNPROCESSABLE: 851 return "http://hl7.org/fhir/assert-response-code-types"; 852 case NULL: 853 return null; 854 default: 855 return "?"; 856 } 857 } 858 859 public String getDefinition() { 860 switch (this) { 861 case OKAY: 862 return "Response code is 200."; 863 case CREATED: 864 return "Response code is 201."; 865 case NOCONTENT: 866 return "Response code is 204."; 867 case NOTMODIFIED: 868 return "Response code is 304."; 869 case BAD: 870 return "Response code is 400."; 871 case FORBIDDEN: 872 return "Response code is 403."; 873 case NOTFOUND: 874 return "Response code is 404."; 875 case METHODNOTALLOWED: 876 return "Response code is 405."; 877 case CONFLICT: 878 return "Response code is 409."; 879 case GONE: 880 return "Response code is 410."; 881 case PRECONDITIONFAILED: 882 return "Response code is 412."; 883 case UNPROCESSABLE: 884 return "Response code is 422."; 885 case NULL: 886 return null; 887 default: 888 return "?"; 889 } 890 } 891 892 public String getDisplay() { 893 switch (this) { 894 case OKAY: 895 return "okay"; 896 case CREATED: 897 return "created"; 898 case NOCONTENT: 899 return "noContent"; 900 case NOTMODIFIED: 901 return "notModified"; 902 case BAD: 903 return "bad"; 904 case FORBIDDEN: 905 return "forbidden"; 906 case NOTFOUND: 907 return "notFound"; 908 case METHODNOTALLOWED: 909 return "methodNotAllowed"; 910 case CONFLICT: 911 return "conflict"; 912 case GONE: 913 return "gone"; 914 case PRECONDITIONFAILED: 915 return "preconditionFailed"; 916 case UNPROCESSABLE: 917 return "unprocessable"; 918 case NULL: 919 return null; 920 default: 921 return "?"; 922 } 923 } 924 } 925 926 public static class AssertionResponseTypesEnumFactory implements EnumFactory<AssertionResponseTypes> { 927 public AssertionResponseTypes fromCode(String codeString) throws IllegalArgumentException { 928 if (codeString == null || "".equals(codeString)) 929 if (codeString == null || "".equals(codeString)) 930 return null; 931 if ("okay".equals(codeString)) 932 return AssertionResponseTypes.OKAY; 933 if ("created".equals(codeString)) 934 return AssertionResponseTypes.CREATED; 935 if ("noContent".equals(codeString)) 936 return AssertionResponseTypes.NOCONTENT; 937 if ("notModified".equals(codeString)) 938 return AssertionResponseTypes.NOTMODIFIED; 939 if ("bad".equals(codeString)) 940 return AssertionResponseTypes.BAD; 941 if ("forbidden".equals(codeString)) 942 return AssertionResponseTypes.FORBIDDEN; 943 if ("notFound".equals(codeString)) 944 return AssertionResponseTypes.NOTFOUND; 945 if ("methodNotAllowed".equals(codeString)) 946 return AssertionResponseTypes.METHODNOTALLOWED; 947 if ("conflict".equals(codeString)) 948 return AssertionResponseTypes.CONFLICT; 949 if ("gone".equals(codeString)) 950 return AssertionResponseTypes.GONE; 951 if ("preconditionFailed".equals(codeString)) 952 return AssertionResponseTypes.PRECONDITIONFAILED; 953 if ("unprocessable".equals(codeString)) 954 return AssertionResponseTypes.UNPROCESSABLE; 955 throw new IllegalArgumentException("Unknown AssertionResponseTypes code '" + codeString + "'"); 956 } 957 958 public Enumeration<AssertionResponseTypes> fromType(PrimitiveType<?> code) throws FHIRException { 959 if (code == null) 960 return null; 961 if (code.isEmpty()) 962 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NULL, code); 963 String codeString = code.asStringValue(); 964 if (codeString == null || "".equals(codeString)) 965 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NULL, code); 966 if ("okay".equals(codeString)) 967 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.OKAY, code); 968 if ("created".equals(codeString)) 969 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CREATED, code); 970 if ("noContent".equals(codeString)) 971 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOCONTENT, code); 972 if ("notModified".equals(codeString)) 973 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTMODIFIED, code); 974 if ("bad".equals(codeString)) 975 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.BAD, code); 976 if ("forbidden".equals(codeString)) 977 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.FORBIDDEN, code); 978 if ("notFound".equals(codeString)) 979 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.NOTFOUND, code); 980 if ("methodNotAllowed".equals(codeString)) 981 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.METHODNOTALLOWED, code); 982 if ("conflict".equals(codeString)) 983 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.CONFLICT, code); 984 if ("gone".equals(codeString)) 985 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.GONE, code); 986 if ("preconditionFailed".equals(codeString)) 987 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.PRECONDITIONFAILED, code); 988 if ("unprocessable".equals(codeString)) 989 return new Enumeration<AssertionResponseTypes>(this, AssertionResponseTypes.UNPROCESSABLE, code); 990 throw new FHIRException("Unknown AssertionResponseTypes code '" + codeString + "'"); 991 } 992 993 public String toCode(AssertionResponseTypes code) { 994 if (code == AssertionResponseTypes.NULL) 995 return null; 996 if (code == AssertionResponseTypes.OKAY) 997 return "okay"; 998 if (code == AssertionResponseTypes.CREATED) 999 return "created"; 1000 if (code == AssertionResponseTypes.NOCONTENT) 1001 return "noContent"; 1002 if (code == AssertionResponseTypes.NOTMODIFIED) 1003 return "notModified"; 1004 if (code == AssertionResponseTypes.BAD) 1005 return "bad"; 1006 if (code == AssertionResponseTypes.FORBIDDEN) 1007 return "forbidden"; 1008 if (code == AssertionResponseTypes.NOTFOUND) 1009 return "notFound"; 1010 if (code == AssertionResponseTypes.METHODNOTALLOWED) 1011 return "methodNotAllowed"; 1012 if (code == AssertionResponseTypes.CONFLICT) 1013 return "conflict"; 1014 if (code == AssertionResponseTypes.GONE) 1015 return "gone"; 1016 if (code == AssertionResponseTypes.PRECONDITIONFAILED) 1017 return "preconditionFailed"; 1018 if (code == AssertionResponseTypes.UNPROCESSABLE) 1019 return "unprocessable"; 1020 return "?"; 1021 } 1022 1023 public String toSystem(AssertionResponseTypes code) { 1024 return code.getSystem(); 1025 } 1026 } 1027 1028 @Block() 1029 public static class TestScriptOriginComponent extends BackboneElement implements IBaseBackboneElement { 1030 /** 1031 * Abstract name given to an origin server in this test script. The name is 1032 * provided as a number starting at 1. 1033 */ 1034 @Child(name = "index", type = { IntegerType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1035 @Description(shortDefinition = "The index of the abstract origin server starting at 1", formalDefinition = "Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.") 1036 protected IntegerType index; 1037 1038 /** 1039 * The type of origin profile the test system supports. 1040 */ 1041 @Child(name = "profile", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1042 @Description(shortDefinition = "FHIR-Client | FHIR-SDC-FormFiller", formalDefinition = "The type of origin profile the test system supports.") 1043 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/testscript-profile-origin-types") 1044 protected Coding profile; 1045 1046 private static final long serialVersionUID = -1239935149L; 1047 1048 /** 1049 * Constructor 1050 */ 1051 public TestScriptOriginComponent() { 1052 super(); 1053 } 1054 1055 /** 1056 * Constructor 1057 */ 1058 public TestScriptOriginComponent(IntegerType index, Coding profile) { 1059 super(); 1060 this.index = index; 1061 this.profile = profile; 1062 } 1063 1064 /** 1065 * @return {@link #index} (Abstract name given to an origin server in this test 1066 * script. The name is provided as a number starting at 1.). This is the 1067 * underlying object with id, value and extensions. The accessor 1068 * "getIndex" gives direct access to the value 1069 */ 1070 public IntegerType getIndexElement() { 1071 if (this.index == null) 1072 if (Configuration.errorOnAutoCreate()) 1073 throw new Error("Attempt to auto-create TestScriptOriginComponent.index"); 1074 else if (Configuration.doAutoCreate()) 1075 this.index = new IntegerType(); // bb 1076 return this.index; 1077 } 1078 1079 public boolean hasIndexElement() { 1080 return this.index != null && !this.index.isEmpty(); 1081 } 1082 1083 public boolean hasIndex() { 1084 return this.index != null && !this.index.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #index} (Abstract name given to an origin server in this 1089 * test script. The name is provided as a number starting at 1.). 1090 * This is the underlying object with id, value and extensions. The 1091 * accessor "getIndex" gives direct access to the value 1092 */ 1093 public TestScriptOriginComponent setIndexElement(IntegerType value) { 1094 this.index = value; 1095 return this; 1096 } 1097 1098 /** 1099 * @return Abstract name given to an origin server in this test script. The name 1100 * is provided as a number starting at 1. 1101 */ 1102 public int getIndex() { 1103 return this.index == null || this.index.isEmpty() ? 0 : this.index.getValue(); 1104 } 1105 1106 /** 1107 * @param value Abstract name given to an origin server in this test script. The 1108 * name is provided as a number starting at 1. 1109 */ 1110 public TestScriptOriginComponent setIndex(int value) { 1111 if (this.index == null) 1112 this.index = new IntegerType(); 1113 this.index.setValue(value); 1114 return this; 1115 } 1116 1117 /** 1118 * @return {@link #profile} (The type of origin profile the test system 1119 * supports.) 1120 */ 1121 public Coding getProfile() { 1122 if (this.profile == null) 1123 if (Configuration.errorOnAutoCreate()) 1124 throw new Error("Attempt to auto-create TestScriptOriginComponent.profile"); 1125 else if (Configuration.doAutoCreate()) 1126 this.profile = new Coding(); // cc 1127 return this.profile; 1128 } 1129 1130 public boolean hasProfile() { 1131 return this.profile != null && !this.profile.isEmpty(); 1132 } 1133 1134 /** 1135 * @param value {@link #profile} (The type of origin profile the test system 1136 * supports.) 1137 */ 1138 public TestScriptOriginComponent setProfile(Coding value) { 1139 this.profile = value; 1140 return this; 1141 } 1142 1143 protected void listChildren(List<Property> children) { 1144 super.listChildren(children); 1145 children.add(new Property("index", "integer", 1146 "Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.", 1147 0, 1, index)); 1148 children.add( 1149 new Property("profile", "Coding", "The type of origin profile the test system supports.", 0, 1, profile)); 1150 } 1151 1152 @Override 1153 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1154 switch (_hash) { 1155 case 100346066: 1156 /* index */ return new Property("index", "integer", 1157 "Abstract name given to an origin server in this test script. The name is provided as a number starting at 1.", 1158 0, 1, index); 1159 case -309425751: 1160 /* profile */ return new Property("profile", "Coding", "The type of origin profile the test system supports.", 1161 0, 1, profile); 1162 default: 1163 return super.getNamedProperty(_hash, _name, _checkValid); 1164 } 1165 1166 } 1167 1168 @Override 1169 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1170 switch (hash) { 1171 case 100346066: 1172 /* index */ return this.index == null ? new Base[0] : new Base[] { this.index }; // IntegerType 1173 case -309425751: 1174 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // Coding 1175 default: 1176 return super.getProperty(hash, name, checkValid); 1177 } 1178 1179 } 1180 1181 @Override 1182 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1183 switch (hash) { 1184 case 100346066: // index 1185 this.index = castToInteger(value); // IntegerType 1186 return value; 1187 case -309425751: // profile 1188 this.profile = castToCoding(value); // Coding 1189 return value; 1190 default: 1191 return super.setProperty(hash, name, value); 1192 } 1193 1194 } 1195 1196 @Override 1197 public Base setProperty(String name, Base value) throws FHIRException { 1198 if (name.equals("index")) { 1199 this.index = castToInteger(value); // IntegerType 1200 } else if (name.equals("profile")) { 1201 this.profile = castToCoding(value); // Coding 1202 } else 1203 return super.setProperty(name, value); 1204 return value; 1205 } 1206 1207 @Override 1208 public void removeChild(String name, Base value) throws FHIRException { 1209 if (name.equals("index")) { 1210 this.index = null; 1211 } else if (name.equals("profile")) { 1212 this.profile = null; 1213 } else 1214 super.removeChild(name, value); 1215 1216 } 1217 1218 @Override 1219 public Base makeProperty(int hash, String name) throws FHIRException { 1220 switch (hash) { 1221 case 100346066: 1222 return getIndexElement(); 1223 case -309425751: 1224 return getProfile(); 1225 default: 1226 return super.makeProperty(hash, name); 1227 } 1228 1229 } 1230 1231 @Override 1232 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1233 switch (hash) { 1234 case 100346066: 1235 /* index */ return new String[] { "integer" }; 1236 case -309425751: 1237 /* profile */ return new String[] { "Coding" }; 1238 default: 1239 return super.getTypesForProperty(hash, name); 1240 } 1241 1242 } 1243 1244 @Override 1245 public Base addChild(String name) throws FHIRException { 1246 if (name.equals("index")) { 1247 throw new FHIRException("Cannot call addChild on a singleton property TestScript.index"); 1248 } else if (name.equals("profile")) { 1249 this.profile = new Coding(); 1250 return this.profile; 1251 } else 1252 return super.addChild(name); 1253 } 1254 1255 public TestScriptOriginComponent copy() { 1256 TestScriptOriginComponent dst = new TestScriptOriginComponent(); 1257 copyValues(dst); 1258 return dst; 1259 } 1260 1261 public void copyValues(TestScriptOriginComponent dst) { 1262 super.copyValues(dst); 1263 dst.index = index == null ? null : index.copy(); 1264 dst.profile = profile == null ? null : profile.copy(); 1265 } 1266 1267 @Override 1268 public boolean equalsDeep(Base other_) { 1269 if (!super.equalsDeep(other_)) 1270 return false; 1271 if (!(other_ instanceof TestScriptOriginComponent)) 1272 return false; 1273 TestScriptOriginComponent o = (TestScriptOriginComponent) other_; 1274 return compareDeep(index, o.index, true) && compareDeep(profile, o.profile, true); 1275 } 1276 1277 @Override 1278 public boolean equalsShallow(Base other_) { 1279 if (!super.equalsShallow(other_)) 1280 return false; 1281 if (!(other_ instanceof TestScriptOriginComponent)) 1282 return false; 1283 TestScriptOriginComponent o = (TestScriptOriginComponent) other_; 1284 return compareValues(index, o.index, true); 1285 } 1286 1287 public boolean isEmpty() { 1288 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(index, profile); 1289 } 1290 1291 public String fhirType() { 1292 return "TestScript.origin"; 1293 1294 } 1295 1296 } 1297 1298 @Block() 1299 public static class TestScriptDestinationComponent extends BackboneElement implements IBaseBackboneElement { 1300 /** 1301 * Abstract name given to a destination server in this test script. The name is 1302 * provided as a number starting at 1. 1303 */ 1304 @Child(name = "index", type = { IntegerType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1305 @Description(shortDefinition = "The index of the abstract destination server starting at 1", formalDefinition = "Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.") 1306 protected IntegerType index; 1307 1308 /** 1309 * The type of destination profile the test system supports. 1310 */ 1311 @Child(name = "profile", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1312 @Description(shortDefinition = "FHIR-Server | FHIR-SDC-FormManager | FHIR-SDC-FormReceiver | FHIR-SDC-FormProcessor", formalDefinition = "The type of destination profile the test system supports.") 1313 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/testscript-profile-destination-types") 1314 protected Coding profile; 1315 1316 private static final long serialVersionUID = -1239935149L; 1317 1318 /** 1319 * Constructor 1320 */ 1321 public TestScriptDestinationComponent() { 1322 super(); 1323 } 1324 1325 /** 1326 * Constructor 1327 */ 1328 public TestScriptDestinationComponent(IntegerType index, Coding profile) { 1329 super(); 1330 this.index = index; 1331 this.profile = profile; 1332 } 1333 1334 /** 1335 * @return {@link #index} (Abstract name given to a destination server in this 1336 * test script. The name is provided as a number starting at 1.). This 1337 * is the underlying object with id, value and extensions. The accessor 1338 * "getIndex" gives direct access to the value 1339 */ 1340 public IntegerType getIndexElement() { 1341 if (this.index == null) 1342 if (Configuration.errorOnAutoCreate()) 1343 throw new Error("Attempt to auto-create TestScriptDestinationComponent.index"); 1344 else if (Configuration.doAutoCreate()) 1345 this.index = new IntegerType(); // bb 1346 return this.index; 1347 } 1348 1349 public boolean hasIndexElement() { 1350 return this.index != null && !this.index.isEmpty(); 1351 } 1352 1353 public boolean hasIndex() { 1354 return this.index != null && !this.index.isEmpty(); 1355 } 1356 1357 /** 1358 * @param value {@link #index} (Abstract name given to a destination server in 1359 * this test script. The name is provided as a number starting at 1360 * 1.). This is the underlying object with id, value and 1361 * extensions. The accessor "getIndex" gives direct access to the 1362 * value 1363 */ 1364 public TestScriptDestinationComponent setIndexElement(IntegerType value) { 1365 this.index = value; 1366 return this; 1367 } 1368 1369 /** 1370 * @return Abstract name given to a destination server in this test script. The 1371 * name is provided as a number starting at 1. 1372 */ 1373 public int getIndex() { 1374 return this.index == null || this.index.isEmpty() ? 0 : this.index.getValue(); 1375 } 1376 1377 /** 1378 * @param value Abstract name given to a destination server in this test script. 1379 * The name is provided as a number starting at 1. 1380 */ 1381 public TestScriptDestinationComponent setIndex(int value) { 1382 if (this.index == null) 1383 this.index = new IntegerType(); 1384 this.index.setValue(value); 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #profile} (The type of destination profile the test system 1390 * supports.) 1391 */ 1392 public Coding getProfile() { 1393 if (this.profile == null) 1394 if (Configuration.errorOnAutoCreate()) 1395 throw new Error("Attempt to auto-create TestScriptDestinationComponent.profile"); 1396 else if (Configuration.doAutoCreate()) 1397 this.profile = new Coding(); // cc 1398 return this.profile; 1399 } 1400 1401 public boolean hasProfile() { 1402 return this.profile != null && !this.profile.isEmpty(); 1403 } 1404 1405 /** 1406 * @param value {@link #profile} (The type of destination profile the test 1407 * system supports.) 1408 */ 1409 public TestScriptDestinationComponent setProfile(Coding value) { 1410 this.profile = value; 1411 return this; 1412 } 1413 1414 protected void listChildren(List<Property> children) { 1415 super.listChildren(children); 1416 children.add(new Property("index", "integer", 1417 "Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.", 1418 0, 1, index)); 1419 children.add(new Property("profile", "Coding", "The type of destination profile the test system supports.", 0, 1, 1420 profile)); 1421 } 1422 1423 @Override 1424 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1425 switch (_hash) { 1426 case 100346066: 1427 /* index */ return new Property("index", "integer", 1428 "Abstract name given to a destination server in this test script. The name is provided as a number starting at 1.", 1429 0, 1, index); 1430 case -309425751: 1431 /* profile */ return new Property("profile", "Coding", 1432 "The type of destination profile the test system supports.", 0, 1, profile); 1433 default: 1434 return super.getNamedProperty(_hash, _name, _checkValid); 1435 } 1436 1437 } 1438 1439 @Override 1440 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1441 switch (hash) { 1442 case 100346066: 1443 /* index */ return this.index == null ? new Base[0] : new Base[] { this.index }; // IntegerType 1444 case -309425751: 1445 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // Coding 1446 default: 1447 return super.getProperty(hash, name, checkValid); 1448 } 1449 1450 } 1451 1452 @Override 1453 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1454 switch (hash) { 1455 case 100346066: // index 1456 this.index = castToInteger(value); // IntegerType 1457 return value; 1458 case -309425751: // profile 1459 this.profile = castToCoding(value); // Coding 1460 return value; 1461 default: 1462 return super.setProperty(hash, name, value); 1463 } 1464 1465 } 1466 1467 @Override 1468 public Base setProperty(String name, Base value) throws FHIRException { 1469 if (name.equals("index")) { 1470 this.index = castToInteger(value); // IntegerType 1471 } else if (name.equals("profile")) { 1472 this.profile = castToCoding(value); // Coding 1473 } else 1474 return super.setProperty(name, value); 1475 return value; 1476 } 1477 1478 @Override 1479 public void removeChild(String name, Base value) throws FHIRException { 1480 if (name.equals("index")) { 1481 this.index = null; 1482 } else if (name.equals("profile")) { 1483 this.profile = null; 1484 } else 1485 super.removeChild(name, value); 1486 1487 } 1488 1489 @Override 1490 public Base makeProperty(int hash, String name) throws FHIRException { 1491 switch (hash) { 1492 case 100346066: 1493 return getIndexElement(); 1494 case -309425751: 1495 return getProfile(); 1496 default: 1497 return super.makeProperty(hash, name); 1498 } 1499 1500 } 1501 1502 @Override 1503 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1504 switch (hash) { 1505 case 100346066: 1506 /* index */ return new String[] { "integer" }; 1507 case -309425751: 1508 /* profile */ return new String[] { "Coding" }; 1509 default: 1510 return super.getTypesForProperty(hash, name); 1511 } 1512 1513 } 1514 1515 @Override 1516 public Base addChild(String name) throws FHIRException { 1517 if (name.equals("index")) { 1518 throw new FHIRException("Cannot call addChild on a singleton property TestScript.index"); 1519 } else if (name.equals("profile")) { 1520 this.profile = new Coding(); 1521 return this.profile; 1522 } else 1523 return super.addChild(name); 1524 } 1525 1526 public TestScriptDestinationComponent copy() { 1527 TestScriptDestinationComponent dst = new TestScriptDestinationComponent(); 1528 copyValues(dst); 1529 return dst; 1530 } 1531 1532 public void copyValues(TestScriptDestinationComponent dst) { 1533 super.copyValues(dst); 1534 dst.index = index == null ? null : index.copy(); 1535 dst.profile = profile == null ? null : profile.copy(); 1536 } 1537 1538 @Override 1539 public boolean equalsDeep(Base other_) { 1540 if (!super.equalsDeep(other_)) 1541 return false; 1542 if (!(other_ instanceof TestScriptDestinationComponent)) 1543 return false; 1544 TestScriptDestinationComponent o = (TestScriptDestinationComponent) other_; 1545 return compareDeep(index, o.index, true) && compareDeep(profile, o.profile, true); 1546 } 1547 1548 @Override 1549 public boolean equalsShallow(Base other_) { 1550 if (!super.equalsShallow(other_)) 1551 return false; 1552 if (!(other_ instanceof TestScriptDestinationComponent)) 1553 return false; 1554 TestScriptDestinationComponent o = (TestScriptDestinationComponent) other_; 1555 return compareValues(index, o.index, true); 1556 } 1557 1558 public boolean isEmpty() { 1559 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(index, profile); 1560 } 1561 1562 public String fhirType() { 1563 return "TestScript.destination"; 1564 1565 } 1566 1567 } 1568 1569 @Block() 1570 public static class TestScriptMetadataComponent extends BackboneElement implements IBaseBackboneElement { 1571 /** 1572 * A link to the FHIR specification that this test is covering. 1573 */ 1574 @Child(name = "link", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1575 @Description(shortDefinition = "Links to the FHIR specification", formalDefinition = "A link to the FHIR specification that this test is covering.") 1576 protected List<TestScriptMetadataLinkComponent> link; 1577 1578 /** 1579 * Capabilities that must exist and are assumed to function correctly on the 1580 * FHIR server being tested. 1581 */ 1582 @Child(name = "capability", type = {}, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1583 @Description(shortDefinition = "Capabilities that are assumed to function correctly on the FHIR server being tested", formalDefinition = "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.") 1584 protected List<TestScriptMetadataCapabilityComponent> capability; 1585 1586 private static final long serialVersionUID = 745183328L; 1587 1588 /** 1589 * Constructor 1590 */ 1591 public TestScriptMetadataComponent() { 1592 super(); 1593 } 1594 1595 /** 1596 * @return {@link #link} (A link to the FHIR specification that this test is 1597 * covering.) 1598 */ 1599 public List<TestScriptMetadataLinkComponent> getLink() { 1600 if (this.link == null) 1601 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1602 return this.link; 1603 } 1604 1605 /** 1606 * @return Returns a reference to <code>this</code> for easy method chaining 1607 */ 1608 public TestScriptMetadataComponent setLink(List<TestScriptMetadataLinkComponent> theLink) { 1609 this.link = theLink; 1610 return this; 1611 } 1612 1613 public boolean hasLink() { 1614 if (this.link == null) 1615 return false; 1616 for (TestScriptMetadataLinkComponent item : this.link) 1617 if (!item.isEmpty()) 1618 return true; 1619 return false; 1620 } 1621 1622 public TestScriptMetadataLinkComponent addLink() { // 3 1623 TestScriptMetadataLinkComponent t = new TestScriptMetadataLinkComponent(); 1624 if (this.link == null) 1625 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1626 this.link.add(t); 1627 return t; 1628 } 1629 1630 public TestScriptMetadataComponent addLink(TestScriptMetadataLinkComponent t) { // 3 1631 if (t == null) 1632 return this; 1633 if (this.link == null) 1634 this.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1635 this.link.add(t); 1636 return this; 1637 } 1638 1639 /** 1640 * @return The first repetition of repeating field {@link #link}, creating it if 1641 * it does not already exist 1642 */ 1643 public TestScriptMetadataLinkComponent getLinkFirstRep() { 1644 if (getLink().isEmpty()) { 1645 addLink(); 1646 } 1647 return getLink().get(0); 1648 } 1649 1650 /** 1651 * @return {@link #capability} (Capabilities that must exist and are assumed to 1652 * function correctly on the FHIR server being tested.) 1653 */ 1654 public List<TestScriptMetadataCapabilityComponent> getCapability() { 1655 if (this.capability == null) 1656 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1657 return this.capability; 1658 } 1659 1660 /** 1661 * @return Returns a reference to <code>this</code> for easy method chaining 1662 */ 1663 public TestScriptMetadataComponent setCapability(List<TestScriptMetadataCapabilityComponent> theCapability) { 1664 this.capability = theCapability; 1665 return this; 1666 } 1667 1668 public boolean hasCapability() { 1669 if (this.capability == null) 1670 return false; 1671 for (TestScriptMetadataCapabilityComponent item : this.capability) 1672 if (!item.isEmpty()) 1673 return true; 1674 return false; 1675 } 1676 1677 public TestScriptMetadataCapabilityComponent addCapability() { // 3 1678 TestScriptMetadataCapabilityComponent t = new TestScriptMetadataCapabilityComponent(); 1679 if (this.capability == null) 1680 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1681 this.capability.add(t); 1682 return t; 1683 } 1684 1685 public TestScriptMetadataComponent addCapability(TestScriptMetadataCapabilityComponent t) { // 3 1686 if (t == null) 1687 return this; 1688 if (this.capability == null) 1689 this.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1690 this.capability.add(t); 1691 return this; 1692 } 1693 1694 /** 1695 * @return The first repetition of repeating field {@link #capability}, creating 1696 * it if it does not already exist 1697 */ 1698 public TestScriptMetadataCapabilityComponent getCapabilityFirstRep() { 1699 if (getCapability().isEmpty()) { 1700 addCapability(); 1701 } 1702 return getCapability().get(0); 1703 } 1704 1705 protected void listChildren(List<Property> children) { 1706 super.listChildren(children); 1707 children.add(new Property("link", "", "A link to the FHIR specification that this test is covering.", 0, 1708 java.lang.Integer.MAX_VALUE, link)); 1709 children.add(new Property("capability", "", 1710 "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, 1711 java.lang.Integer.MAX_VALUE, capability)); 1712 } 1713 1714 @Override 1715 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1716 switch (_hash) { 1717 case 3321850: 1718 /* link */ return new Property("link", "", "A link to the FHIR specification that this test is covering.", 0, 1719 java.lang.Integer.MAX_VALUE, link); 1720 case -783669992: 1721 /* capability */ return new Property("capability", "", 1722 "Capabilities that must exist and are assumed to function correctly on the FHIR server being tested.", 0, 1723 java.lang.Integer.MAX_VALUE, capability); 1724 default: 1725 return super.getNamedProperty(_hash, _name, _checkValid); 1726 } 1727 1728 } 1729 1730 @Override 1731 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1732 switch (hash) { 1733 case 3321850: 1734 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // TestScriptMetadataLinkComponent 1735 case -783669992: 1736 /* capability */ return this.capability == null ? new Base[0] 1737 : this.capability.toArray(new Base[this.capability.size()]); // TestScriptMetadataCapabilityComponent 1738 default: 1739 return super.getProperty(hash, name, checkValid); 1740 } 1741 1742 } 1743 1744 @Override 1745 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1746 switch (hash) { 1747 case 3321850: // link 1748 this.getLink().add((TestScriptMetadataLinkComponent) value); // TestScriptMetadataLinkComponent 1749 return value; 1750 case -783669992: // capability 1751 this.getCapability().add((TestScriptMetadataCapabilityComponent) value); // TestScriptMetadataCapabilityComponent 1752 return value; 1753 default: 1754 return super.setProperty(hash, name, value); 1755 } 1756 1757 } 1758 1759 @Override 1760 public Base setProperty(String name, Base value) throws FHIRException { 1761 if (name.equals("link")) { 1762 this.getLink().add((TestScriptMetadataLinkComponent) value); 1763 } else if (name.equals("capability")) { 1764 this.getCapability().add((TestScriptMetadataCapabilityComponent) value); 1765 } else 1766 return super.setProperty(name, value); 1767 return value; 1768 } 1769 1770 @Override 1771 public void removeChild(String name, Base value) throws FHIRException { 1772 if (name.equals("link")) { 1773 this.getLink().remove((TestScriptMetadataLinkComponent) value); 1774 } else if (name.equals("capability")) { 1775 this.getCapability().remove((TestScriptMetadataCapabilityComponent) value); 1776 } else 1777 super.removeChild(name, value); 1778 1779 } 1780 1781 @Override 1782 public Base makeProperty(int hash, String name) throws FHIRException { 1783 switch (hash) { 1784 case 3321850: 1785 return addLink(); 1786 case -783669992: 1787 return addCapability(); 1788 default: 1789 return super.makeProperty(hash, name); 1790 } 1791 1792 } 1793 1794 @Override 1795 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1796 switch (hash) { 1797 case 3321850: 1798 /* link */ return new String[] {}; 1799 case -783669992: 1800 /* capability */ return new String[] {}; 1801 default: 1802 return super.getTypesForProperty(hash, name); 1803 } 1804 1805 } 1806 1807 @Override 1808 public Base addChild(String name) throws FHIRException { 1809 if (name.equals("link")) { 1810 return addLink(); 1811 } else if (name.equals("capability")) { 1812 return addCapability(); 1813 } else 1814 return super.addChild(name); 1815 } 1816 1817 public TestScriptMetadataComponent copy() { 1818 TestScriptMetadataComponent dst = new TestScriptMetadataComponent(); 1819 copyValues(dst); 1820 return dst; 1821 } 1822 1823 public void copyValues(TestScriptMetadataComponent dst) { 1824 super.copyValues(dst); 1825 if (link != null) { 1826 dst.link = new ArrayList<TestScriptMetadataLinkComponent>(); 1827 for (TestScriptMetadataLinkComponent i : link) 1828 dst.link.add(i.copy()); 1829 } 1830 ; 1831 if (capability != null) { 1832 dst.capability = new ArrayList<TestScriptMetadataCapabilityComponent>(); 1833 for (TestScriptMetadataCapabilityComponent i : capability) 1834 dst.capability.add(i.copy()); 1835 } 1836 ; 1837 } 1838 1839 @Override 1840 public boolean equalsDeep(Base other_) { 1841 if (!super.equalsDeep(other_)) 1842 return false; 1843 if (!(other_ instanceof TestScriptMetadataComponent)) 1844 return false; 1845 TestScriptMetadataComponent o = (TestScriptMetadataComponent) other_; 1846 return compareDeep(link, o.link, true) && compareDeep(capability, o.capability, true); 1847 } 1848 1849 @Override 1850 public boolean equalsShallow(Base other_) { 1851 if (!super.equalsShallow(other_)) 1852 return false; 1853 if (!(other_ instanceof TestScriptMetadataComponent)) 1854 return false; 1855 TestScriptMetadataComponent o = (TestScriptMetadataComponent) other_; 1856 return true; 1857 } 1858 1859 public boolean isEmpty() { 1860 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(link, capability); 1861 } 1862 1863 public String fhirType() { 1864 return "TestScript.metadata"; 1865 1866 } 1867 1868 } 1869 1870 @Block() 1871 public static class TestScriptMetadataLinkComponent extends BackboneElement implements IBaseBackboneElement { 1872 /** 1873 * URL to a particular requirement or feature within the FHIR specification. 1874 */ 1875 @Child(name = "url", type = { UriType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1876 @Description(shortDefinition = "URL to the specification", formalDefinition = "URL to a particular requirement or feature within the FHIR specification.") 1877 protected UriType url; 1878 1879 /** 1880 * Short description of the link. 1881 */ 1882 @Child(name = "description", type = { 1883 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1884 @Description(shortDefinition = "Short description", formalDefinition = "Short description of the link.") 1885 protected StringType description; 1886 1887 private static final long serialVersionUID = 213372298L; 1888 1889 /** 1890 * Constructor 1891 */ 1892 public TestScriptMetadataLinkComponent() { 1893 super(); 1894 } 1895 1896 /** 1897 * Constructor 1898 */ 1899 public TestScriptMetadataLinkComponent(UriType url) { 1900 super(); 1901 this.url = url; 1902 } 1903 1904 /** 1905 * @return {@link #url} (URL to a particular requirement or feature within the 1906 * FHIR specification.). This is the underlying object with id, value 1907 * and extensions. The accessor "getUrl" gives direct access to the 1908 * value 1909 */ 1910 public UriType getUrlElement() { 1911 if (this.url == null) 1912 if (Configuration.errorOnAutoCreate()) 1913 throw new Error("Attempt to auto-create TestScriptMetadataLinkComponent.url"); 1914 else if (Configuration.doAutoCreate()) 1915 this.url = new UriType(); // bb 1916 return this.url; 1917 } 1918 1919 public boolean hasUrlElement() { 1920 return this.url != null && !this.url.isEmpty(); 1921 } 1922 1923 public boolean hasUrl() { 1924 return this.url != null && !this.url.isEmpty(); 1925 } 1926 1927 /** 1928 * @param value {@link #url} (URL to a particular requirement or feature within 1929 * the FHIR specification.). This is the underlying object with id, 1930 * value and extensions. The accessor "getUrl" gives direct access 1931 * to the value 1932 */ 1933 public TestScriptMetadataLinkComponent setUrlElement(UriType value) { 1934 this.url = value; 1935 return this; 1936 } 1937 1938 /** 1939 * @return URL to a particular requirement or feature within the FHIR 1940 * specification. 1941 */ 1942 public String getUrl() { 1943 return this.url == null ? null : this.url.getValue(); 1944 } 1945 1946 /** 1947 * @param value URL to a particular requirement or feature within the FHIR 1948 * specification. 1949 */ 1950 public TestScriptMetadataLinkComponent setUrl(String value) { 1951 if (this.url == null) 1952 this.url = new UriType(); 1953 this.url.setValue(value); 1954 return this; 1955 } 1956 1957 /** 1958 * @return {@link #description} (Short description of the link.). This is the 1959 * underlying object with id, value and extensions. The accessor 1960 * "getDescription" gives direct access to the value 1961 */ 1962 public StringType getDescriptionElement() { 1963 if (this.description == null) 1964 if (Configuration.errorOnAutoCreate()) 1965 throw new Error("Attempt to auto-create TestScriptMetadataLinkComponent.description"); 1966 else if (Configuration.doAutoCreate()) 1967 this.description = new StringType(); // bb 1968 return this.description; 1969 } 1970 1971 public boolean hasDescriptionElement() { 1972 return this.description != null && !this.description.isEmpty(); 1973 } 1974 1975 public boolean hasDescription() { 1976 return this.description != null && !this.description.isEmpty(); 1977 } 1978 1979 /** 1980 * @param value {@link #description} (Short description of the link.). This is 1981 * the underlying object with id, value and extensions. The 1982 * accessor "getDescription" gives direct access to the value 1983 */ 1984 public TestScriptMetadataLinkComponent setDescriptionElement(StringType value) { 1985 this.description = value; 1986 return this; 1987 } 1988 1989 /** 1990 * @return Short description of the link. 1991 */ 1992 public String getDescription() { 1993 return this.description == null ? null : this.description.getValue(); 1994 } 1995 1996 /** 1997 * @param value Short description of the link. 1998 */ 1999 public TestScriptMetadataLinkComponent setDescription(String value) { 2000 if (Utilities.noString(value)) 2001 this.description = null; 2002 else { 2003 if (this.description == null) 2004 this.description = new StringType(); 2005 this.description.setValue(value); 2006 } 2007 return this; 2008 } 2009 2010 protected void listChildren(List<Property> children) { 2011 super.listChildren(children); 2012 children.add(new Property("url", "uri", 2013 "URL to a particular requirement or feature within the FHIR specification.", 0, 1, url)); 2014 children.add(new Property("description", "string", "Short description of the link.", 0, 1, description)); 2015 } 2016 2017 @Override 2018 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2019 switch (_hash) { 2020 case 116079: 2021 /* url */ return new Property("url", "uri", 2022 "URL to a particular requirement or feature within the FHIR specification.", 0, 1, url); 2023 case -1724546052: 2024 /* description */ return new Property("description", "string", "Short description of the link.", 0, 1, 2025 description); 2026 default: 2027 return super.getNamedProperty(_hash, _name, _checkValid); 2028 } 2029 2030 } 2031 2032 @Override 2033 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2034 switch (hash) { 2035 case 116079: 2036 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 2037 case -1724546052: 2038 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2039 default: 2040 return super.getProperty(hash, name, checkValid); 2041 } 2042 2043 } 2044 2045 @Override 2046 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2047 switch (hash) { 2048 case 116079: // url 2049 this.url = castToUri(value); // UriType 2050 return value; 2051 case -1724546052: // description 2052 this.description = castToString(value); // StringType 2053 return value; 2054 default: 2055 return super.setProperty(hash, name, value); 2056 } 2057 2058 } 2059 2060 @Override 2061 public Base setProperty(String name, Base value) throws FHIRException { 2062 if (name.equals("url")) { 2063 this.url = castToUri(value); // UriType 2064 } else if (name.equals("description")) { 2065 this.description = castToString(value); // StringType 2066 } else 2067 return super.setProperty(name, value); 2068 return value; 2069 } 2070 2071 @Override 2072 public void removeChild(String name, Base value) throws FHIRException { 2073 if (name.equals("url")) { 2074 this.url = null; 2075 } else if (name.equals("description")) { 2076 this.description = null; 2077 } else 2078 super.removeChild(name, value); 2079 2080 } 2081 2082 @Override 2083 public Base makeProperty(int hash, String name) throws FHIRException { 2084 switch (hash) { 2085 case 116079: 2086 return getUrlElement(); 2087 case -1724546052: 2088 return getDescriptionElement(); 2089 default: 2090 return super.makeProperty(hash, name); 2091 } 2092 2093 } 2094 2095 @Override 2096 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2097 switch (hash) { 2098 case 116079: 2099 /* url */ return new String[] { "uri" }; 2100 case -1724546052: 2101 /* description */ return new String[] { "string" }; 2102 default: 2103 return super.getTypesForProperty(hash, name); 2104 } 2105 2106 } 2107 2108 @Override 2109 public Base addChild(String name) throws FHIRException { 2110 if (name.equals("url")) { 2111 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 2112 } else if (name.equals("description")) { 2113 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 2114 } else 2115 return super.addChild(name); 2116 } 2117 2118 public TestScriptMetadataLinkComponent copy() { 2119 TestScriptMetadataLinkComponent dst = new TestScriptMetadataLinkComponent(); 2120 copyValues(dst); 2121 return dst; 2122 } 2123 2124 public void copyValues(TestScriptMetadataLinkComponent dst) { 2125 super.copyValues(dst); 2126 dst.url = url == null ? null : url.copy(); 2127 dst.description = description == null ? null : description.copy(); 2128 } 2129 2130 @Override 2131 public boolean equalsDeep(Base other_) { 2132 if (!super.equalsDeep(other_)) 2133 return false; 2134 if (!(other_ instanceof TestScriptMetadataLinkComponent)) 2135 return false; 2136 TestScriptMetadataLinkComponent o = (TestScriptMetadataLinkComponent) other_; 2137 return compareDeep(url, o.url, true) && compareDeep(description, o.description, true); 2138 } 2139 2140 @Override 2141 public boolean equalsShallow(Base other_) { 2142 if (!super.equalsShallow(other_)) 2143 return false; 2144 if (!(other_ instanceof TestScriptMetadataLinkComponent)) 2145 return false; 2146 TestScriptMetadataLinkComponent o = (TestScriptMetadataLinkComponent) other_; 2147 return compareValues(url, o.url, true) && compareValues(description, o.description, true); 2148 } 2149 2150 public boolean isEmpty() { 2151 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, description); 2152 } 2153 2154 public String fhirType() { 2155 return "TestScript.metadata.link"; 2156 2157 } 2158 2159 } 2160 2161 @Block() 2162 public static class TestScriptMetadataCapabilityComponent extends BackboneElement implements IBaseBackboneElement { 2163 /** 2164 * Whether or not the test execution will require the given capabilities of the 2165 * server in order for this test script to execute. 2166 */ 2167 @Child(name = "required", type = { 2168 BooleanType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2169 @Description(shortDefinition = "Are the capabilities required?", formalDefinition = "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.") 2170 protected BooleanType required; 2171 2172 /** 2173 * Whether or not the test execution will validate the given capabilities of the 2174 * server in order for this test script to execute. 2175 */ 2176 @Child(name = "validated", type = { 2177 BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2178 @Description(shortDefinition = "Are the capabilities validated?", formalDefinition = "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.") 2179 protected BooleanType validated; 2180 2181 /** 2182 * Description of the capabilities that this test script is requiring the server 2183 * to support. 2184 */ 2185 @Child(name = "description", type = { 2186 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2187 @Description(shortDefinition = "The expected capabilities of the server", formalDefinition = "Description of the capabilities that this test script is requiring the server to support.") 2188 protected StringType description; 2189 2190 /** 2191 * Which origin server these requirements apply to. 2192 */ 2193 @Child(name = "origin", type = { 2194 IntegerType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2195 @Description(shortDefinition = "Which origin server these requirements apply to", formalDefinition = "Which origin server these requirements apply to.") 2196 protected List<IntegerType> origin; 2197 2198 /** 2199 * Which server these requirements apply to. 2200 */ 2201 @Child(name = "destination", type = { 2202 IntegerType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2203 @Description(shortDefinition = "Which server these requirements apply to", formalDefinition = "Which server these requirements apply to.") 2204 protected IntegerType destination; 2205 2206 /** 2207 * Links to the FHIR specification that describes this interaction and the 2208 * resources involved in more detail. 2209 */ 2210 @Child(name = "link", type = { 2211 UriType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2212 @Description(shortDefinition = "Links to the FHIR specification", formalDefinition = "Links to the FHIR specification that describes this interaction and the resources involved in more detail.") 2213 protected List<UriType> link; 2214 2215 /** 2216 * Minimum capabilities required of server for test script to execute 2217 * successfully. If server does not meet at a minimum the referenced capability 2218 * statement, then all tests in this script are skipped. 2219 */ 2220 @Child(name = "capabilities", type = { 2221 CanonicalType.class }, order = 7, min = 1, max = 1, modifier = false, summary = false) 2222 @Description(shortDefinition = "Required Capability Statement", formalDefinition = "Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.") 2223 protected CanonicalType capabilities; 2224 2225 private static final long serialVersionUID = -1368199288L; 2226 2227 /** 2228 * Constructor 2229 */ 2230 public TestScriptMetadataCapabilityComponent() { 2231 super(); 2232 } 2233 2234 /** 2235 * Constructor 2236 */ 2237 public TestScriptMetadataCapabilityComponent(BooleanType required, BooleanType validated, 2238 CanonicalType capabilities) { 2239 super(); 2240 this.required = required; 2241 this.validated = validated; 2242 this.capabilities = capabilities; 2243 } 2244 2245 /** 2246 * @return {@link #required} (Whether or not the test execution will require the 2247 * given capabilities of the server in order for this test script to 2248 * execute.). This is the underlying object with id, value and 2249 * extensions. The accessor "getRequired" gives direct access to the 2250 * value 2251 */ 2252 public BooleanType getRequiredElement() { 2253 if (this.required == null) 2254 if (Configuration.errorOnAutoCreate()) 2255 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.required"); 2256 else if (Configuration.doAutoCreate()) 2257 this.required = new BooleanType(); // bb 2258 return this.required; 2259 } 2260 2261 public boolean hasRequiredElement() { 2262 return this.required != null && !this.required.isEmpty(); 2263 } 2264 2265 public boolean hasRequired() { 2266 return this.required != null && !this.required.isEmpty(); 2267 } 2268 2269 /** 2270 * @param value {@link #required} (Whether or not the test execution will 2271 * require the given capabilities of the server in order for this 2272 * test script to execute.). This is the underlying object with id, 2273 * value and extensions. The accessor "getRequired" gives direct 2274 * access to the value 2275 */ 2276 public TestScriptMetadataCapabilityComponent setRequiredElement(BooleanType value) { 2277 this.required = value; 2278 return this; 2279 } 2280 2281 /** 2282 * @return Whether or not the test execution will require the given capabilities 2283 * of the server in order for this test script to execute. 2284 */ 2285 public boolean getRequired() { 2286 return this.required == null || this.required.isEmpty() ? false : this.required.getValue(); 2287 } 2288 2289 /** 2290 * @param value Whether or not the test execution will require the given 2291 * capabilities of the server in order for this test script to 2292 * execute. 2293 */ 2294 public TestScriptMetadataCapabilityComponent setRequired(boolean value) { 2295 if (this.required == null) 2296 this.required = new BooleanType(); 2297 this.required.setValue(value); 2298 return this; 2299 } 2300 2301 /** 2302 * @return {@link #validated} (Whether or not the test execution will validate 2303 * the given capabilities of the server in order for this test script to 2304 * execute.). This is the underlying object with id, value and 2305 * extensions. The accessor "getValidated" gives direct access to the 2306 * value 2307 */ 2308 public BooleanType getValidatedElement() { 2309 if (this.validated == null) 2310 if (Configuration.errorOnAutoCreate()) 2311 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.validated"); 2312 else if (Configuration.doAutoCreate()) 2313 this.validated = new BooleanType(); // bb 2314 return this.validated; 2315 } 2316 2317 public boolean hasValidatedElement() { 2318 return this.validated != null && !this.validated.isEmpty(); 2319 } 2320 2321 public boolean hasValidated() { 2322 return this.validated != null && !this.validated.isEmpty(); 2323 } 2324 2325 /** 2326 * @param value {@link #validated} (Whether or not the test execution will 2327 * validate the given capabilities of the server in order for this 2328 * test script to execute.). This is the underlying object with id, 2329 * value and extensions. The accessor "getValidated" gives direct 2330 * access to the value 2331 */ 2332 public TestScriptMetadataCapabilityComponent setValidatedElement(BooleanType value) { 2333 this.validated = value; 2334 return this; 2335 } 2336 2337 /** 2338 * @return Whether or not the test execution will validate the given 2339 * capabilities of the server in order for this test script to execute. 2340 */ 2341 public boolean getValidated() { 2342 return this.validated == null || this.validated.isEmpty() ? false : this.validated.getValue(); 2343 } 2344 2345 /** 2346 * @param value Whether or not the test execution will validate the given 2347 * capabilities of the server in order for this test script to 2348 * execute. 2349 */ 2350 public TestScriptMetadataCapabilityComponent setValidated(boolean value) { 2351 if (this.validated == null) 2352 this.validated = new BooleanType(); 2353 this.validated.setValue(value); 2354 return this; 2355 } 2356 2357 /** 2358 * @return {@link #description} (Description of the capabilities that this test 2359 * script is requiring the server to support.). This is the underlying 2360 * object with id, value and extensions. The accessor "getDescription" 2361 * gives direct access to the value 2362 */ 2363 public StringType getDescriptionElement() { 2364 if (this.description == null) 2365 if (Configuration.errorOnAutoCreate()) 2366 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.description"); 2367 else if (Configuration.doAutoCreate()) 2368 this.description = new StringType(); // bb 2369 return this.description; 2370 } 2371 2372 public boolean hasDescriptionElement() { 2373 return this.description != null && !this.description.isEmpty(); 2374 } 2375 2376 public boolean hasDescription() { 2377 return this.description != null && !this.description.isEmpty(); 2378 } 2379 2380 /** 2381 * @param value {@link #description} (Description of the capabilities that this 2382 * test script is requiring the server to support.). This is the 2383 * underlying object with id, value and extensions. The accessor 2384 * "getDescription" gives direct access to the value 2385 */ 2386 public TestScriptMetadataCapabilityComponent setDescriptionElement(StringType value) { 2387 this.description = value; 2388 return this; 2389 } 2390 2391 /** 2392 * @return Description of the capabilities that this test script is requiring 2393 * the server to support. 2394 */ 2395 public String getDescription() { 2396 return this.description == null ? null : this.description.getValue(); 2397 } 2398 2399 /** 2400 * @param value Description of the capabilities that this test script is 2401 * requiring the server to support. 2402 */ 2403 public TestScriptMetadataCapabilityComponent setDescription(String value) { 2404 if (Utilities.noString(value)) 2405 this.description = null; 2406 else { 2407 if (this.description == null) 2408 this.description = new StringType(); 2409 this.description.setValue(value); 2410 } 2411 return this; 2412 } 2413 2414 /** 2415 * @return {@link #origin} (Which origin server these requirements apply to.) 2416 */ 2417 public List<IntegerType> getOrigin() { 2418 if (this.origin == null) 2419 this.origin = new ArrayList<IntegerType>(); 2420 return this.origin; 2421 } 2422 2423 /** 2424 * @return Returns a reference to <code>this</code> for easy method chaining 2425 */ 2426 public TestScriptMetadataCapabilityComponent setOrigin(List<IntegerType> theOrigin) { 2427 this.origin = theOrigin; 2428 return this; 2429 } 2430 2431 public boolean hasOrigin() { 2432 if (this.origin == null) 2433 return false; 2434 for (IntegerType item : this.origin) 2435 if (!item.isEmpty()) 2436 return true; 2437 return false; 2438 } 2439 2440 /** 2441 * @return {@link #origin} (Which origin server these requirements apply to.) 2442 */ 2443 public IntegerType addOriginElement() {// 2 2444 IntegerType t = new IntegerType(); 2445 if (this.origin == null) 2446 this.origin = new ArrayList<IntegerType>(); 2447 this.origin.add(t); 2448 return t; 2449 } 2450 2451 /** 2452 * @param value {@link #origin} (Which origin server these requirements apply 2453 * to.) 2454 */ 2455 public TestScriptMetadataCapabilityComponent addOrigin(int value) { // 1 2456 IntegerType t = new IntegerType(); 2457 t.setValue(value); 2458 if (this.origin == null) 2459 this.origin = new ArrayList<IntegerType>(); 2460 this.origin.add(t); 2461 return this; 2462 } 2463 2464 /** 2465 * @param value {@link #origin} (Which origin server these requirements apply 2466 * to.) 2467 */ 2468 public boolean hasOrigin(int value) { 2469 if (this.origin == null) 2470 return false; 2471 for (IntegerType v : this.origin) 2472 if (v.getValue().equals(value)) // integer 2473 return true; 2474 return false; 2475 } 2476 2477 /** 2478 * @return {@link #destination} (Which server these requirements apply to.). 2479 * This is the underlying object with id, value and extensions. The 2480 * accessor "getDestination" gives direct access to the value 2481 */ 2482 public IntegerType getDestinationElement() { 2483 if (this.destination == null) 2484 if (Configuration.errorOnAutoCreate()) 2485 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.destination"); 2486 else if (Configuration.doAutoCreate()) 2487 this.destination = new IntegerType(); // bb 2488 return this.destination; 2489 } 2490 2491 public boolean hasDestinationElement() { 2492 return this.destination != null && !this.destination.isEmpty(); 2493 } 2494 2495 public boolean hasDestination() { 2496 return this.destination != null && !this.destination.isEmpty(); 2497 } 2498 2499 /** 2500 * @param value {@link #destination} (Which server these requirements apply 2501 * to.). This is the underlying object with id, value and 2502 * extensions. The accessor "getDestination" gives direct access to 2503 * the value 2504 */ 2505 public TestScriptMetadataCapabilityComponent setDestinationElement(IntegerType value) { 2506 this.destination = value; 2507 return this; 2508 } 2509 2510 /** 2511 * @return Which server these requirements apply to. 2512 */ 2513 public int getDestination() { 2514 return this.destination == null || this.destination.isEmpty() ? 0 : this.destination.getValue(); 2515 } 2516 2517 /** 2518 * @param value Which server these requirements apply to. 2519 */ 2520 public TestScriptMetadataCapabilityComponent setDestination(int value) { 2521 if (this.destination == null) 2522 this.destination = new IntegerType(); 2523 this.destination.setValue(value); 2524 return this; 2525 } 2526 2527 /** 2528 * @return {@link #link} (Links to the FHIR specification that describes this 2529 * interaction and the resources involved in more detail.) 2530 */ 2531 public List<UriType> getLink() { 2532 if (this.link == null) 2533 this.link = new ArrayList<UriType>(); 2534 return this.link; 2535 } 2536 2537 /** 2538 * @return Returns a reference to <code>this</code> for easy method chaining 2539 */ 2540 public TestScriptMetadataCapabilityComponent setLink(List<UriType> theLink) { 2541 this.link = theLink; 2542 return this; 2543 } 2544 2545 public boolean hasLink() { 2546 if (this.link == null) 2547 return false; 2548 for (UriType item : this.link) 2549 if (!item.isEmpty()) 2550 return true; 2551 return false; 2552 } 2553 2554 /** 2555 * @return {@link #link} (Links to the FHIR specification that describes this 2556 * interaction and the resources involved in more detail.) 2557 */ 2558 public UriType addLinkElement() {// 2 2559 UriType t = new UriType(); 2560 if (this.link == null) 2561 this.link = new ArrayList<UriType>(); 2562 this.link.add(t); 2563 return t; 2564 } 2565 2566 /** 2567 * @param value {@link #link} (Links to the FHIR specification that describes 2568 * this interaction and the resources involved in more detail.) 2569 */ 2570 public TestScriptMetadataCapabilityComponent addLink(String value) { // 1 2571 UriType t = new UriType(); 2572 t.setValue(value); 2573 if (this.link == null) 2574 this.link = new ArrayList<UriType>(); 2575 this.link.add(t); 2576 return this; 2577 } 2578 2579 /** 2580 * @param value {@link #link} (Links to the FHIR specification that describes 2581 * this interaction and the resources involved in more detail.) 2582 */ 2583 public boolean hasLink(String value) { 2584 if (this.link == null) 2585 return false; 2586 for (UriType v : this.link) 2587 if (v.getValue().equals(value)) // uri 2588 return true; 2589 return false; 2590 } 2591 2592 /** 2593 * @return {@link #capabilities} (Minimum capabilities required of server for 2594 * test script to execute successfully. If server does not meet at a 2595 * minimum the referenced capability statement, then all tests in this 2596 * script are skipped.). This is the underlying object with id, value 2597 * and extensions. The accessor "getCapabilities" gives direct access to 2598 * the value 2599 */ 2600 public CanonicalType getCapabilitiesElement() { 2601 if (this.capabilities == null) 2602 if (Configuration.errorOnAutoCreate()) 2603 throw new Error("Attempt to auto-create TestScriptMetadataCapabilityComponent.capabilities"); 2604 else if (Configuration.doAutoCreate()) 2605 this.capabilities = new CanonicalType(); // bb 2606 return this.capabilities; 2607 } 2608 2609 public boolean hasCapabilitiesElement() { 2610 return this.capabilities != null && !this.capabilities.isEmpty(); 2611 } 2612 2613 public boolean hasCapabilities() { 2614 return this.capabilities != null && !this.capabilities.isEmpty(); 2615 } 2616 2617 /** 2618 * @param value {@link #capabilities} (Minimum capabilities required of server 2619 * for test script to execute successfully. If server does not meet 2620 * at a minimum the referenced capability statement, then all tests 2621 * in this script are skipped.). This is the underlying object with 2622 * id, value and extensions. The accessor "getCapabilities" gives 2623 * direct access to the value 2624 */ 2625 public TestScriptMetadataCapabilityComponent setCapabilitiesElement(CanonicalType value) { 2626 this.capabilities = value; 2627 return this; 2628 } 2629 2630 /** 2631 * @return Minimum capabilities required of server for test script to execute 2632 * successfully. If server does not meet at a minimum the referenced 2633 * capability statement, then all tests in this script are skipped. 2634 */ 2635 public String getCapabilities() { 2636 return this.capabilities == null ? null : this.capabilities.getValue(); 2637 } 2638 2639 /** 2640 * @param value Minimum capabilities required of server for test script to 2641 * execute successfully. If server does not meet at a minimum the 2642 * referenced capability statement, then all tests in this script 2643 * are skipped. 2644 */ 2645 public TestScriptMetadataCapabilityComponent setCapabilities(String value) { 2646 if (this.capabilities == null) 2647 this.capabilities = new CanonicalType(); 2648 this.capabilities.setValue(value); 2649 return this; 2650 } 2651 2652 protected void listChildren(List<Property> children) { 2653 super.listChildren(children); 2654 children.add(new Property("required", "boolean", 2655 "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.", 2656 0, 1, required)); 2657 children.add(new Property("validated", "boolean", 2658 "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.", 2659 0, 1, validated)); 2660 children.add(new Property("description", "string", 2661 "Description of the capabilities that this test script is requiring the server to support.", 0, 1, 2662 description)); 2663 children.add(new Property("origin", "integer", "Which origin server these requirements apply to.", 0, 2664 java.lang.Integer.MAX_VALUE, origin)); 2665 children 2666 .add(new Property("destination", "integer", "Which server these requirements apply to.", 0, 1, destination)); 2667 children.add(new Property("link", "uri", 2668 "Links to the FHIR specification that describes this interaction and the resources involved in more detail.", 2669 0, java.lang.Integer.MAX_VALUE, link)); 2670 children.add(new Property("capabilities", "canonical(CapabilityStatement)", 2671 "Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.", 2672 0, 1, capabilities)); 2673 } 2674 2675 @Override 2676 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2677 switch (_hash) { 2678 case -393139297: 2679 /* required */ return new Property("required", "boolean", 2680 "Whether or not the test execution will require the given capabilities of the server in order for this test script to execute.", 2681 0, 1, required); 2682 case -1109784050: 2683 /* validated */ return new Property("validated", "boolean", 2684 "Whether or not the test execution will validate the given capabilities of the server in order for this test script to execute.", 2685 0, 1, validated); 2686 case -1724546052: 2687 /* description */ return new Property("description", "string", 2688 "Description of the capabilities that this test script is requiring the server to support.", 0, 1, 2689 description); 2690 case -1008619738: 2691 /* origin */ return new Property("origin", "integer", "Which origin server these requirements apply to.", 0, 2692 java.lang.Integer.MAX_VALUE, origin); 2693 case -1429847026: 2694 /* destination */ return new Property("destination", "integer", "Which server these requirements apply to.", 0, 2695 1, destination); 2696 case 3321850: 2697 /* link */ return new Property("link", "uri", 2698 "Links to the FHIR specification that describes this interaction and the resources involved in more detail.", 2699 0, java.lang.Integer.MAX_VALUE, link); 2700 case -1487597642: 2701 /* capabilities */ return new Property("capabilities", "canonical(CapabilityStatement)", 2702 "Minimum capabilities required of server for test script to execute successfully. If server does not meet at a minimum the referenced capability statement, then all tests in this script are skipped.", 2703 0, 1, capabilities); 2704 default: 2705 return super.getNamedProperty(_hash, _name, _checkValid); 2706 } 2707 2708 } 2709 2710 @Override 2711 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2712 switch (hash) { 2713 case -393139297: 2714 /* required */ return this.required == null ? new Base[0] : new Base[] { this.required }; // BooleanType 2715 case -1109784050: 2716 /* validated */ return this.validated == null ? new Base[0] : new Base[] { this.validated }; // BooleanType 2717 case -1724546052: 2718 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2719 case -1008619738: 2720 /* origin */ return this.origin == null ? new Base[0] : this.origin.toArray(new Base[this.origin.size()]); // IntegerType 2721 case -1429847026: 2722 /* destination */ return this.destination == null ? new Base[0] : new Base[] { this.destination }; // IntegerType 2723 case 3321850: 2724 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // UriType 2725 case -1487597642: 2726 /* capabilities */ return this.capabilities == null ? new Base[0] : new Base[] { this.capabilities }; // CanonicalType 2727 default: 2728 return super.getProperty(hash, name, checkValid); 2729 } 2730 2731 } 2732 2733 @Override 2734 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2735 switch (hash) { 2736 case -393139297: // required 2737 this.required = castToBoolean(value); // BooleanType 2738 return value; 2739 case -1109784050: // validated 2740 this.validated = castToBoolean(value); // BooleanType 2741 return value; 2742 case -1724546052: // description 2743 this.description = castToString(value); // StringType 2744 return value; 2745 case -1008619738: // origin 2746 this.getOrigin().add(castToInteger(value)); // IntegerType 2747 return value; 2748 case -1429847026: // destination 2749 this.destination = castToInteger(value); // IntegerType 2750 return value; 2751 case 3321850: // link 2752 this.getLink().add(castToUri(value)); // UriType 2753 return value; 2754 case -1487597642: // capabilities 2755 this.capabilities = castToCanonical(value); // CanonicalType 2756 return value; 2757 default: 2758 return super.setProperty(hash, name, value); 2759 } 2760 2761 } 2762 2763 @Override 2764 public Base setProperty(String name, Base value) throws FHIRException { 2765 if (name.equals("required")) { 2766 this.required = castToBoolean(value); // BooleanType 2767 } else if (name.equals("validated")) { 2768 this.validated = castToBoolean(value); // BooleanType 2769 } else if (name.equals("description")) { 2770 this.description = castToString(value); // StringType 2771 } else if (name.equals("origin")) { 2772 this.getOrigin().add(castToInteger(value)); 2773 } else if (name.equals("destination")) { 2774 this.destination = castToInteger(value); // IntegerType 2775 } else if (name.equals("link")) { 2776 this.getLink().add(castToUri(value)); 2777 } else if (name.equals("capabilities")) { 2778 this.capabilities = castToCanonical(value); // CanonicalType 2779 } else 2780 return super.setProperty(name, value); 2781 return value; 2782 } 2783 2784 @Override 2785 public void removeChild(String name, Base value) throws FHIRException { 2786 if (name.equals("required")) { 2787 this.required = null; 2788 } else if (name.equals("validated")) { 2789 this.validated = null; 2790 } else if (name.equals("description")) { 2791 this.description = null; 2792 } else if (name.equals("origin")) { 2793 this.getOrigin().remove(castToInteger(value)); 2794 } else if (name.equals("destination")) { 2795 this.destination = null; 2796 } else if (name.equals("link")) { 2797 this.getLink().remove(castToUri(value)); 2798 } else if (name.equals("capabilities")) { 2799 this.capabilities = null; 2800 } else 2801 super.removeChild(name, value); 2802 2803 } 2804 2805 @Override 2806 public Base makeProperty(int hash, String name) throws FHIRException { 2807 switch (hash) { 2808 case -393139297: 2809 return getRequiredElement(); 2810 case -1109784050: 2811 return getValidatedElement(); 2812 case -1724546052: 2813 return getDescriptionElement(); 2814 case -1008619738: 2815 return addOriginElement(); 2816 case -1429847026: 2817 return getDestinationElement(); 2818 case 3321850: 2819 return addLinkElement(); 2820 case -1487597642: 2821 return getCapabilitiesElement(); 2822 default: 2823 return super.makeProperty(hash, name); 2824 } 2825 2826 } 2827 2828 @Override 2829 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2830 switch (hash) { 2831 case -393139297: 2832 /* required */ return new String[] { "boolean" }; 2833 case -1109784050: 2834 /* validated */ return new String[] { "boolean" }; 2835 case -1724546052: 2836 /* description */ return new String[] { "string" }; 2837 case -1008619738: 2838 /* origin */ return new String[] { "integer" }; 2839 case -1429847026: 2840 /* destination */ return new String[] { "integer" }; 2841 case 3321850: 2842 /* link */ return new String[] { "uri" }; 2843 case -1487597642: 2844 /* capabilities */ return new String[] { "canonical" }; 2845 default: 2846 return super.getTypesForProperty(hash, name); 2847 } 2848 2849 } 2850 2851 @Override 2852 public Base addChild(String name) throws FHIRException { 2853 if (name.equals("required")) { 2854 throw new FHIRException("Cannot call addChild on a singleton property TestScript.required"); 2855 } else if (name.equals("validated")) { 2856 throw new FHIRException("Cannot call addChild on a singleton property TestScript.validated"); 2857 } else if (name.equals("description")) { 2858 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 2859 } else if (name.equals("origin")) { 2860 throw new FHIRException("Cannot call addChild on a singleton property TestScript.origin"); 2861 } else if (name.equals("destination")) { 2862 throw new FHIRException("Cannot call addChild on a singleton property TestScript.destination"); 2863 } else if (name.equals("link")) { 2864 throw new FHIRException("Cannot call addChild on a singleton property TestScript.link"); 2865 } else if (name.equals("capabilities")) { 2866 throw new FHIRException("Cannot call addChild on a singleton property TestScript.capabilities"); 2867 } else 2868 return super.addChild(name); 2869 } 2870 2871 public TestScriptMetadataCapabilityComponent copy() { 2872 TestScriptMetadataCapabilityComponent dst = new TestScriptMetadataCapabilityComponent(); 2873 copyValues(dst); 2874 return dst; 2875 } 2876 2877 public void copyValues(TestScriptMetadataCapabilityComponent dst) { 2878 super.copyValues(dst); 2879 dst.required = required == null ? null : required.copy(); 2880 dst.validated = validated == null ? null : validated.copy(); 2881 dst.description = description == null ? null : description.copy(); 2882 if (origin != null) { 2883 dst.origin = new ArrayList<IntegerType>(); 2884 for (IntegerType i : origin) 2885 dst.origin.add(i.copy()); 2886 } 2887 ; 2888 dst.destination = destination == null ? null : destination.copy(); 2889 if (link != null) { 2890 dst.link = new ArrayList<UriType>(); 2891 for (UriType i : link) 2892 dst.link.add(i.copy()); 2893 } 2894 ; 2895 dst.capabilities = capabilities == null ? null : capabilities.copy(); 2896 } 2897 2898 @Override 2899 public boolean equalsDeep(Base other_) { 2900 if (!super.equalsDeep(other_)) 2901 return false; 2902 if (!(other_ instanceof TestScriptMetadataCapabilityComponent)) 2903 return false; 2904 TestScriptMetadataCapabilityComponent o = (TestScriptMetadataCapabilityComponent) other_; 2905 return compareDeep(required, o.required, true) && compareDeep(validated, o.validated, true) 2906 && compareDeep(description, o.description, true) && compareDeep(origin, o.origin, true) 2907 && compareDeep(destination, o.destination, true) && compareDeep(link, o.link, true) 2908 && compareDeep(capabilities, o.capabilities, true); 2909 } 2910 2911 @Override 2912 public boolean equalsShallow(Base other_) { 2913 if (!super.equalsShallow(other_)) 2914 return false; 2915 if (!(other_ instanceof TestScriptMetadataCapabilityComponent)) 2916 return false; 2917 TestScriptMetadataCapabilityComponent o = (TestScriptMetadataCapabilityComponent) other_; 2918 return compareValues(required, o.required, true) && compareValues(validated, o.validated, true) 2919 && compareValues(description, o.description, true) && compareValues(origin, o.origin, true) 2920 && compareValues(destination, o.destination, true) && compareValues(link, o.link, true); 2921 } 2922 2923 public boolean isEmpty() { 2924 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(required, validated, description, origin, 2925 destination, link, capabilities); 2926 } 2927 2928 public String fhirType() { 2929 return "TestScript.metadata.capability"; 2930 2931 } 2932 2933 } 2934 2935 @Block() 2936 public static class TestScriptFixtureComponent extends BackboneElement implements IBaseBackboneElement { 2937 /** 2938 * Whether or not to implicitly create the fixture during setup. If true, the 2939 * fixture is automatically created on each server being tested during setup, 2940 * therefore no create operation is required for this fixture in the 2941 * TestScript.setup section. 2942 */ 2943 @Child(name = "autocreate", type = { 2944 BooleanType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2945 @Description(shortDefinition = "Whether or not to implicitly create the fixture during setup", formalDefinition = "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.") 2946 protected BooleanType autocreate; 2947 2948 /** 2949 * Whether or not to implicitly delete the fixture during teardown. If true, the 2950 * fixture is automatically deleted on each server being tested during teardown, 2951 * therefore no delete operation is required for this fixture in the 2952 * TestScript.teardown section. 2953 */ 2954 @Child(name = "autodelete", type = { 2955 BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2956 @Description(shortDefinition = "Whether or not to implicitly delete the fixture during teardown", formalDefinition = "Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.") 2957 protected BooleanType autodelete; 2958 2959 /** 2960 * Reference to the resource (containing the contents of the resource needed for 2961 * operations). 2962 */ 2963 @Child(name = "resource", type = { 2964 Reference.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2965 @Description(shortDefinition = "Reference of the resource", formalDefinition = "Reference to the resource (containing the contents of the resource needed for operations).") 2966 protected Reference resource; 2967 2968 /** 2969 * The actual object that is the target of the reference (Reference to the 2970 * resource (containing the contents of the resource needed for operations).) 2971 */ 2972 protected Resource resourceTarget; 2973 2974 private static final long serialVersionUID = 1110683307L; 2975 2976 /** 2977 * Constructor 2978 */ 2979 public TestScriptFixtureComponent() { 2980 super(); 2981 } 2982 2983 /** 2984 * Constructor 2985 */ 2986 public TestScriptFixtureComponent(BooleanType autocreate, BooleanType autodelete) { 2987 super(); 2988 this.autocreate = autocreate; 2989 this.autodelete = autodelete; 2990 } 2991 2992 /** 2993 * @return {@link #autocreate} (Whether or not to implicitly create the fixture 2994 * during setup. If true, the fixture is automatically created on each 2995 * server being tested during setup, therefore no create operation is 2996 * required for this fixture in the TestScript.setup section.). This is 2997 * the underlying object with id, value and extensions. The accessor 2998 * "getAutocreate" gives direct access to the value 2999 */ 3000 public BooleanType getAutocreateElement() { 3001 if (this.autocreate == null) 3002 if (Configuration.errorOnAutoCreate()) 3003 throw new Error("Attempt to auto-create TestScriptFixtureComponent.autocreate"); 3004 else if (Configuration.doAutoCreate()) 3005 this.autocreate = new BooleanType(); // bb 3006 return this.autocreate; 3007 } 3008 3009 public boolean hasAutocreateElement() { 3010 return this.autocreate != null && !this.autocreate.isEmpty(); 3011 } 3012 3013 public boolean hasAutocreate() { 3014 return this.autocreate != null && !this.autocreate.isEmpty(); 3015 } 3016 3017 /** 3018 * @param value {@link #autocreate} (Whether or not to implicitly create the 3019 * fixture during setup. If true, the fixture is automatically 3020 * created on each server being tested during setup, therefore no 3021 * create operation is required for this fixture in the 3022 * TestScript.setup section.). This is the underlying object with 3023 * id, value and extensions. The accessor "getAutocreate" gives 3024 * direct access to the value 3025 */ 3026 public TestScriptFixtureComponent setAutocreateElement(BooleanType value) { 3027 this.autocreate = value; 3028 return this; 3029 } 3030 3031 /** 3032 * @return Whether or not to implicitly create the fixture during setup. If 3033 * true, the fixture is automatically created on each server being 3034 * tested during setup, therefore no create operation is required for 3035 * this fixture in the TestScript.setup section. 3036 */ 3037 public boolean getAutocreate() { 3038 return this.autocreate == null || this.autocreate.isEmpty() ? false : this.autocreate.getValue(); 3039 } 3040 3041 /** 3042 * @param value Whether or not to implicitly create the fixture during setup. If 3043 * true, the fixture is automatically created on each server being 3044 * tested during setup, therefore no create operation is required 3045 * for this fixture in the TestScript.setup section. 3046 */ 3047 public TestScriptFixtureComponent setAutocreate(boolean value) { 3048 if (this.autocreate == null) 3049 this.autocreate = new BooleanType(); 3050 this.autocreate.setValue(value); 3051 return this; 3052 } 3053 3054 /** 3055 * @return {@link #autodelete} (Whether or not to implicitly delete the fixture 3056 * during teardown. If true, the fixture is automatically deleted on 3057 * each server being tested during teardown, therefore no delete 3058 * operation is required for this fixture in the TestScript.teardown 3059 * section.). This is the underlying object with id, value and 3060 * extensions. The accessor "getAutodelete" gives direct access to the 3061 * value 3062 */ 3063 public BooleanType getAutodeleteElement() { 3064 if (this.autodelete == null) 3065 if (Configuration.errorOnAutoCreate()) 3066 throw new Error("Attempt to auto-create TestScriptFixtureComponent.autodelete"); 3067 else if (Configuration.doAutoCreate()) 3068 this.autodelete = new BooleanType(); // bb 3069 return this.autodelete; 3070 } 3071 3072 public boolean hasAutodeleteElement() { 3073 return this.autodelete != null && !this.autodelete.isEmpty(); 3074 } 3075 3076 public boolean hasAutodelete() { 3077 return this.autodelete != null && !this.autodelete.isEmpty(); 3078 } 3079 3080 /** 3081 * @param value {@link #autodelete} (Whether or not to implicitly delete the 3082 * fixture during teardown. If true, the fixture is automatically 3083 * deleted on each server being tested during teardown, therefore 3084 * no delete operation is required for this fixture in the 3085 * TestScript.teardown section.). This is the underlying object 3086 * with id, value and extensions. The accessor "getAutodelete" 3087 * gives direct access to the value 3088 */ 3089 public TestScriptFixtureComponent setAutodeleteElement(BooleanType value) { 3090 this.autodelete = value; 3091 return this; 3092 } 3093 3094 /** 3095 * @return Whether or not to implicitly delete the fixture during teardown. If 3096 * true, the fixture is automatically deleted on each server being 3097 * tested during teardown, therefore no delete operation is required for 3098 * this fixture in the TestScript.teardown section. 3099 */ 3100 public boolean getAutodelete() { 3101 return this.autodelete == null || this.autodelete.isEmpty() ? false : this.autodelete.getValue(); 3102 } 3103 3104 /** 3105 * @param value Whether or not to implicitly delete the fixture during teardown. 3106 * If true, the fixture is automatically deleted on each server 3107 * being tested during teardown, therefore no delete operation is 3108 * required for this fixture in the TestScript.teardown section. 3109 */ 3110 public TestScriptFixtureComponent setAutodelete(boolean value) { 3111 if (this.autodelete == null) 3112 this.autodelete = new BooleanType(); 3113 this.autodelete.setValue(value); 3114 return this; 3115 } 3116 3117 /** 3118 * @return {@link #resource} (Reference to the resource (containing the contents 3119 * of the resource needed for operations).) 3120 */ 3121 public Reference getResource() { 3122 if (this.resource == null) 3123 if (Configuration.errorOnAutoCreate()) 3124 throw new Error("Attempt to auto-create TestScriptFixtureComponent.resource"); 3125 else if (Configuration.doAutoCreate()) 3126 this.resource = new Reference(); // cc 3127 return this.resource; 3128 } 3129 3130 public boolean hasResource() { 3131 return this.resource != null && !this.resource.isEmpty(); 3132 } 3133 3134 /** 3135 * @param value {@link #resource} (Reference to the resource (containing the 3136 * contents of the resource needed for operations).) 3137 */ 3138 public TestScriptFixtureComponent setResource(Reference value) { 3139 this.resource = value; 3140 return this; 3141 } 3142 3143 /** 3144 * @return {@link #resource} The actual object that is the target of the 3145 * reference. The reference library doesn't populate this, but you can 3146 * use it to hold the resource if you resolve it. (Reference to the 3147 * resource (containing the contents of the resource needed for 3148 * operations).) 3149 */ 3150 public Resource getResourceTarget() { 3151 return this.resourceTarget; 3152 } 3153 3154 /** 3155 * @param value {@link #resource} The actual object that is the target of the 3156 * reference. The reference library doesn't use these, but you can 3157 * use it to hold the resource if you resolve it. (Reference to the 3158 * resource (containing the contents of the resource needed for 3159 * operations).) 3160 */ 3161 public TestScriptFixtureComponent setResourceTarget(Resource value) { 3162 this.resourceTarget = value; 3163 return this; 3164 } 3165 3166 protected void listChildren(List<Property> children) { 3167 super.listChildren(children); 3168 children.add(new Property("autocreate", "boolean", 3169 "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.", 3170 0, 1, autocreate)); 3171 children.add(new Property("autodelete", "boolean", 3172 "Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.", 3173 0, 1, autodelete)); 3174 children.add(new Property("resource", "Reference(Any)", 3175 "Reference to the resource (containing the contents of the resource needed for operations).", 0, 1, 3176 resource)); 3177 } 3178 3179 @Override 3180 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3181 switch (_hash) { 3182 case 73154411: 3183 /* autocreate */ return new Property("autocreate", "boolean", 3184 "Whether or not to implicitly create the fixture during setup. If true, the fixture is automatically created on each server being tested during setup, therefore no create operation is required for this fixture in the TestScript.setup section.", 3185 0, 1, autocreate); 3186 case 89990170: 3187 /* autodelete */ return new Property("autodelete", "boolean", 3188 "Whether or not to implicitly delete the fixture during teardown. If true, the fixture is automatically deleted on each server being tested during teardown, therefore no delete operation is required for this fixture in the TestScript.teardown section.", 3189 0, 1, autodelete); 3190 case -341064690: 3191 /* resource */ return new Property("resource", "Reference(Any)", 3192 "Reference to the resource (containing the contents of the resource needed for operations).", 0, 1, 3193 resource); 3194 default: 3195 return super.getNamedProperty(_hash, _name, _checkValid); 3196 } 3197 3198 } 3199 3200 @Override 3201 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3202 switch (hash) { 3203 case 73154411: 3204 /* autocreate */ return this.autocreate == null ? new Base[0] : new Base[] { this.autocreate }; // BooleanType 3205 case 89990170: 3206 /* autodelete */ return this.autodelete == null ? new Base[0] : new Base[] { this.autodelete }; // BooleanType 3207 case -341064690: 3208 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // Reference 3209 default: 3210 return super.getProperty(hash, name, checkValid); 3211 } 3212 3213 } 3214 3215 @Override 3216 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3217 switch (hash) { 3218 case 73154411: // autocreate 3219 this.autocreate = castToBoolean(value); // BooleanType 3220 return value; 3221 case 89990170: // autodelete 3222 this.autodelete = castToBoolean(value); // BooleanType 3223 return value; 3224 case -341064690: // resource 3225 this.resource = castToReference(value); // Reference 3226 return value; 3227 default: 3228 return super.setProperty(hash, name, value); 3229 } 3230 3231 } 3232 3233 @Override 3234 public Base setProperty(String name, Base value) throws FHIRException { 3235 if (name.equals("autocreate")) { 3236 this.autocreate = castToBoolean(value); // BooleanType 3237 } else if (name.equals("autodelete")) { 3238 this.autodelete = castToBoolean(value); // BooleanType 3239 } else if (name.equals("resource")) { 3240 this.resource = castToReference(value); // Reference 3241 } else 3242 return super.setProperty(name, value); 3243 return value; 3244 } 3245 3246 @Override 3247 public void removeChild(String name, Base value) throws FHIRException { 3248 if (name.equals("autocreate")) { 3249 this.autocreate = null; 3250 } else if (name.equals("autodelete")) { 3251 this.autodelete = null; 3252 } else if (name.equals("resource")) { 3253 this.resource = null; 3254 } else 3255 super.removeChild(name, value); 3256 3257 } 3258 3259 @Override 3260 public Base makeProperty(int hash, String name) throws FHIRException { 3261 switch (hash) { 3262 case 73154411: 3263 return getAutocreateElement(); 3264 case 89990170: 3265 return getAutodeleteElement(); 3266 case -341064690: 3267 return getResource(); 3268 default: 3269 return super.makeProperty(hash, name); 3270 } 3271 3272 } 3273 3274 @Override 3275 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3276 switch (hash) { 3277 case 73154411: 3278 /* autocreate */ return new String[] { "boolean" }; 3279 case 89990170: 3280 /* autodelete */ return new String[] { "boolean" }; 3281 case -341064690: 3282 /* resource */ return new String[] { "Reference" }; 3283 default: 3284 return super.getTypesForProperty(hash, name); 3285 } 3286 3287 } 3288 3289 @Override 3290 public Base addChild(String name) throws FHIRException { 3291 if (name.equals("autocreate")) { 3292 throw new FHIRException("Cannot call addChild on a singleton property TestScript.autocreate"); 3293 } else if (name.equals("autodelete")) { 3294 throw new FHIRException("Cannot call addChild on a singleton property TestScript.autodelete"); 3295 } else if (name.equals("resource")) { 3296 this.resource = new Reference(); 3297 return this.resource; 3298 } else 3299 return super.addChild(name); 3300 } 3301 3302 public TestScriptFixtureComponent copy() { 3303 TestScriptFixtureComponent dst = new TestScriptFixtureComponent(); 3304 copyValues(dst); 3305 return dst; 3306 } 3307 3308 public void copyValues(TestScriptFixtureComponent dst) { 3309 super.copyValues(dst); 3310 dst.autocreate = autocreate == null ? null : autocreate.copy(); 3311 dst.autodelete = autodelete == null ? null : autodelete.copy(); 3312 dst.resource = resource == null ? null : resource.copy(); 3313 } 3314 3315 @Override 3316 public boolean equalsDeep(Base other_) { 3317 if (!super.equalsDeep(other_)) 3318 return false; 3319 if (!(other_ instanceof TestScriptFixtureComponent)) 3320 return false; 3321 TestScriptFixtureComponent o = (TestScriptFixtureComponent) other_; 3322 return compareDeep(autocreate, o.autocreate, true) && compareDeep(autodelete, o.autodelete, true) 3323 && compareDeep(resource, o.resource, true); 3324 } 3325 3326 @Override 3327 public boolean equalsShallow(Base other_) { 3328 if (!super.equalsShallow(other_)) 3329 return false; 3330 if (!(other_ instanceof TestScriptFixtureComponent)) 3331 return false; 3332 TestScriptFixtureComponent o = (TestScriptFixtureComponent) other_; 3333 return compareValues(autocreate, o.autocreate, true) && compareValues(autodelete, o.autodelete, true); 3334 } 3335 3336 public boolean isEmpty() { 3337 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(autocreate, autodelete, resource); 3338 } 3339 3340 public String fhirType() { 3341 return "TestScript.fixture"; 3342 3343 } 3344 3345 } 3346 3347 @Block() 3348 public static class TestScriptVariableComponent extends BackboneElement implements IBaseBackboneElement { 3349 /** 3350 * Descriptive name for this variable. 3351 */ 3352 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3353 @Description(shortDefinition = "Descriptive name for this variable", formalDefinition = "Descriptive name for this variable.") 3354 protected StringType name; 3355 3356 /** 3357 * A default, hard-coded, or user-defined value for this variable. 3358 */ 3359 @Child(name = "defaultValue", type = { 3360 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3361 @Description(shortDefinition = "Default, hard-coded, or user-defined value for this variable", formalDefinition = "A default, hard-coded, or user-defined value for this variable.") 3362 protected StringType defaultValue; 3363 3364 /** 3365 * A free text natural language description of the variable and its purpose. 3366 */ 3367 @Child(name = "description", type = { 3368 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3369 @Description(shortDefinition = "Natural language description of the variable", formalDefinition = "A free text natural language description of the variable and its purpose.") 3370 protected StringType description; 3371 3372 /** 3373 * The FHIRPath expression to evaluate against the fixture body. When variables 3374 * are defined, only one of either expression, headerField or path must be 3375 * specified. 3376 */ 3377 @Child(name = "expression", type = { 3378 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3379 @Description(shortDefinition = "The FHIRPath expression against the fixture body", formalDefinition = "The FHIRPath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.") 3380 protected StringType expression; 3381 3382 /** 3383 * Will be used to grab the HTTP header field value from the headers that 3384 * sourceId is pointing to. 3385 */ 3386 @Child(name = "headerField", type = { 3387 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3388 @Description(shortDefinition = "HTTP header field name for source", formalDefinition = "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.") 3389 protected StringType headerField; 3390 3391 /** 3392 * Displayable text string with hint help information to the user when entering 3393 * a default value. 3394 */ 3395 @Child(name = "hint", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 3396 @Description(shortDefinition = "Hint help text for default value to enter", formalDefinition = "Displayable text string with hint help information to the user when entering a default value.") 3397 protected StringType hint; 3398 3399 /** 3400 * XPath or JSONPath to evaluate against the fixture body. When variables are 3401 * defined, only one of either expression, headerField or path must be 3402 * specified. 3403 */ 3404 @Child(name = "path", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3405 @Description(shortDefinition = "XPath or JSONPath against the fixture body", formalDefinition = "XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.") 3406 protected StringType path; 3407 3408 /** 3409 * Fixture to evaluate the XPath/JSONPath expression or the headerField against 3410 * within this variable. 3411 */ 3412 @Child(name = "sourceId", type = { IdType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3413 @Description(shortDefinition = "Fixture Id of source expression or headerField within this variable", formalDefinition = "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.") 3414 protected IdType sourceId; 3415 3416 private static final long serialVersionUID = -1592325432L; 3417 3418 /** 3419 * Constructor 3420 */ 3421 public TestScriptVariableComponent() { 3422 super(); 3423 } 3424 3425 /** 3426 * Constructor 3427 */ 3428 public TestScriptVariableComponent(StringType name) { 3429 super(); 3430 this.name = name; 3431 } 3432 3433 /** 3434 * @return {@link #name} (Descriptive name for this variable.). This is the 3435 * underlying object with id, value and extensions. The accessor 3436 * "getName" gives direct access to the value 3437 */ 3438 public StringType getNameElement() { 3439 if (this.name == null) 3440 if (Configuration.errorOnAutoCreate()) 3441 throw new Error("Attempt to auto-create TestScriptVariableComponent.name"); 3442 else if (Configuration.doAutoCreate()) 3443 this.name = new StringType(); // bb 3444 return this.name; 3445 } 3446 3447 public boolean hasNameElement() { 3448 return this.name != null && !this.name.isEmpty(); 3449 } 3450 3451 public boolean hasName() { 3452 return this.name != null && !this.name.isEmpty(); 3453 } 3454 3455 /** 3456 * @param value {@link #name} (Descriptive name for this variable.). This is the 3457 * underlying object with id, value and extensions. The accessor 3458 * "getName" gives direct access to the value 3459 */ 3460 public TestScriptVariableComponent setNameElement(StringType value) { 3461 this.name = value; 3462 return this; 3463 } 3464 3465 /** 3466 * @return Descriptive name for this variable. 3467 */ 3468 public String getName() { 3469 return this.name == null ? null : this.name.getValue(); 3470 } 3471 3472 /** 3473 * @param value Descriptive name for this variable. 3474 */ 3475 public TestScriptVariableComponent setName(String value) { 3476 if (this.name == null) 3477 this.name = new StringType(); 3478 this.name.setValue(value); 3479 return this; 3480 } 3481 3482 /** 3483 * @return {@link #defaultValue} (A default, hard-coded, or user-defined value 3484 * for this variable.). This is the underlying object with id, value and 3485 * extensions. The accessor "getDefaultValue" gives direct access to the 3486 * value 3487 */ 3488 public StringType getDefaultValueElement() { 3489 if (this.defaultValue == null) 3490 if (Configuration.errorOnAutoCreate()) 3491 throw new Error("Attempt to auto-create TestScriptVariableComponent.defaultValue"); 3492 else if (Configuration.doAutoCreate()) 3493 this.defaultValue = new StringType(); // bb 3494 return this.defaultValue; 3495 } 3496 3497 public boolean hasDefaultValueElement() { 3498 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3499 } 3500 3501 public boolean hasDefaultValue() { 3502 return this.defaultValue != null && !this.defaultValue.isEmpty(); 3503 } 3504 3505 /** 3506 * @param value {@link #defaultValue} (A default, hard-coded, or user-defined 3507 * value for this variable.). This is the underlying object with 3508 * id, value and extensions. The accessor "getDefaultValue" gives 3509 * direct access to the value 3510 */ 3511 public TestScriptVariableComponent setDefaultValueElement(StringType value) { 3512 this.defaultValue = value; 3513 return this; 3514 } 3515 3516 /** 3517 * @return A default, hard-coded, or user-defined value for this variable. 3518 */ 3519 public String getDefaultValue() { 3520 return this.defaultValue == null ? null : this.defaultValue.getValue(); 3521 } 3522 3523 /** 3524 * @param value A default, hard-coded, or user-defined value for this variable. 3525 */ 3526 public TestScriptVariableComponent setDefaultValue(String value) { 3527 if (Utilities.noString(value)) 3528 this.defaultValue = null; 3529 else { 3530 if (this.defaultValue == null) 3531 this.defaultValue = new StringType(); 3532 this.defaultValue.setValue(value); 3533 } 3534 return this; 3535 } 3536 3537 /** 3538 * @return {@link #description} (A free text natural language description of the 3539 * variable and its purpose.). This is the underlying object with id, 3540 * value and extensions. The accessor "getDescription" gives direct 3541 * access to the value 3542 */ 3543 public StringType getDescriptionElement() { 3544 if (this.description == null) 3545 if (Configuration.errorOnAutoCreate()) 3546 throw new Error("Attempt to auto-create TestScriptVariableComponent.description"); 3547 else if (Configuration.doAutoCreate()) 3548 this.description = new StringType(); // bb 3549 return this.description; 3550 } 3551 3552 public boolean hasDescriptionElement() { 3553 return this.description != null && !this.description.isEmpty(); 3554 } 3555 3556 public boolean hasDescription() { 3557 return this.description != null && !this.description.isEmpty(); 3558 } 3559 3560 /** 3561 * @param value {@link #description} (A free text natural language description 3562 * of the variable and its purpose.). This is the underlying object 3563 * with id, value and extensions. The accessor "getDescription" 3564 * gives direct access to the value 3565 */ 3566 public TestScriptVariableComponent setDescriptionElement(StringType value) { 3567 this.description = value; 3568 return this; 3569 } 3570 3571 /** 3572 * @return A free text natural language description of the variable and its 3573 * purpose. 3574 */ 3575 public String getDescription() { 3576 return this.description == null ? null : this.description.getValue(); 3577 } 3578 3579 /** 3580 * @param value A free text natural language description of the variable and its 3581 * purpose. 3582 */ 3583 public TestScriptVariableComponent setDescription(String value) { 3584 if (Utilities.noString(value)) 3585 this.description = null; 3586 else { 3587 if (this.description == null) 3588 this.description = new StringType(); 3589 this.description.setValue(value); 3590 } 3591 return this; 3592 } 3593 3594 /** 3595 * @return {@link #expression} (The FHIRPath expression to evaluate against the 3596 * fixture body. When variables are defined, only one of either 3597 * expression, headerField or path must be specified.). This is the 3598 * underlying object with id, value and extensions. The accessor 3599 * "getExpression" gives direct access to the value 3600 */ 3601 public StringType getExpressionElement() { 3602 if (this.expression == null) 3603 if (Configuration.errorOnAutoCreate()) 3604 throw new Error("Attempt to auto-create TestScriptVariableComponent.expression"); 3605 else if (Configuration.doAutoCreate()) 3606 this.expression = new StringType(); // bb 3607 return this.expression; 3608 } 3609 3610 public boolean hasExpressionElement() { 3611 return this.expression != null && !this.expression.isEmpty(); 3612 } 3613 3614 public boolean hasExpression() { 3615 return this.expression != null && !this.expression.isEmpty(); 3616 } 3617 3618 /** 3619 * @param value {@link #expression} (The FHIRPath expression to evaluate against 3620 * the fixture body. When variables are defined, only one of either 3621 * expression, headerField or path must be specified.). This is the 3622 * underlying object with id, value and extensions. The accessor 3623 * "getExpression" gives direct access to the value 3624 */ 3625 public TestScriptVariableComponent setExpressionElement(StringType value) { 3626 this.expression = value; 3627 return this; 3628 } 3629 3630 /** 3631 * @return The FHIRPath expression to evaluate against the fixture body. When 3632 * variables are defined, only one of either expression, headerField or 3633 * path must be specified. 3634 */ 3635 public String getExpression() { 3636 return this.expression == null ? null : this.expression.getValue(); 3637 } 3638 3639 /** 3640 * @param value The FHIRPath expression to evaluate against the fixture body. 3641 * When variables are defined, only one of either expression, 3642 * headerField or path must be specified. 3643 */ 3644 public TestScriptVariableComponent setExpression(String value) { 3645 if (Utilities.noString(value)) 3646 this.expression = null; 3647 else { 3648 if (this.expression == null) 3649 this.expression = new StringType(); 3650 this.expression.setValue(value); 3651 } 3652 return this; 3653 } 3654 3655 /** 3656 * @return {@link #headerField} (Will be used to grab the HTTP header field 3657 * value from the headers that sourceId is pointing to.). This is the 3658 * underlying object with id, value and extensions. The accessor 3659 * "getHeaderField" gives direct access to the value 3660 */ 3661 public StringType getHeaderFieldElement() { 3662 if (this.headerField == null) 3663 if (Configuration.errorOnAutoCreate()) 3664 throw new Error("Attempt to auto-create TestScriptVariableComponent.headerField"); 3665 else if (Configuration.doAutoCreate()) 3666 this.headerField = new StringType(); // bb 3667 return this.headerField; 3668 } 3669 3670 public boolean hasHeaderFieldElement() { 3671 return this.headerField != null && !this.headerField.isEmpty(); 3672 } 3673 3674 public boolean hasHeaderField() { 3675 return this.headerField != null && !this.headerField.isEmpty(); 3676 } 3677 3678 /** 3679 * @param value {@link #headerField} (Will be used to grab the HTTP header field 3680 * value from the headers that sourceId is pointing to.). This is 3681 * the underlying object with id, value and extensions. The 3682 * accessor "getHeaderField" gives direct access to the value 3683 */ 3684 public TestScriptVariableComponent setHeaderFieldElement(StringType value) { 3685 this.headerField = value; 3686 return this; 3687 } 3688 3689 /** 3690 * @return Will be used to grab the HTTP header field value from the headers 3691 * that sourceId is pointing to. 3692 */ 3693 public String getHeaderField() { 3694 return this.headerField == null ? null : this.headerField.getValue(); 3695 } 3696 3697 /** 3698 * @param value Will be used to grab the HTTP header field value from the 3699 * headers that sourceId is pointing to. 3700 */ 3701 public TestScriptVariableComponent setHeaderField(String value) { 3702 if (Utilities.noString(value)) 3703 this.headerField = null; 3704 else { 3705 if (this.headerField == null) 3706 this.headerField = new StringType(); 3707 this.headerField.setValue(value); 3708 } 3709 return this; 3710 } 3711 3712 /** 3713 * @return {@link #hint} (Displayable text string with hint help information to 3714 * the user when entering a default value.). This is the underlying 3715 * object with id, value and extensions. The accessor "getHint" gives 3716 * direct access to the value 3717 */ 3718 public StringType getHintElement() { 3719 if (this.hint == null) 3720 if (Configuration.errorOnAutoCreate()) 3721 throw new Error("Attempt to auto-create TestScriptVariableComponent.hint"); 3722 else if (Configuration.doAutoCreate()) 3723 this.hint = new StringType(); // bb 3724 return this.hint; 3725 } 3726 3727 public boolean hasHintElement() { 3728 return this.hint != null && !this.hint.isEmpty(); 3729 } 3730 3731 public boolean hasHint() { 3732 return this.hint != null && !this.hint.isEmpty(); 3733 } 3734 3735 /** 3736 * @param value {@link #hint} (Displayable text string with hint help 3737 * information to the user when entering a default value.). This is 3738 * the underlying object with id, value and extensions. The 3739 * accessor "getHint" gives direct access to the value 3740 */ 3741 public TestScriptVariableComponent setHintElement(StringType value) { 3742 this.hint = value; 3743 return this; 3744 } 3745 3746 /** 3747 * @return Displayable text string with hint help information to the user when 3748 * entering a default value. 3749 */ 3750 public String getHint() { 3751 return this.hint == null ? null : this.hint.getValue(); 3752 } 3753 3754 /** 3755 * @param value Displayable text string with hint help information to the user 3756 * when entering a default value. 3757 */ 3758 public TestScriptVariableComponent setHint(String value) { 3759 if (Utilities.noString(value)) 3760 this.hint = null; 3761 else { 3762 if (this.hint == null) 3763 this.hint = new StringType(); 3764 this.hint.setValue(value); 3765 } 3766 return this; 3767 } 3768 3769 /** 3770 * @return {@link #path} (XPath or JSONPath to evaluate against the fixture 3771 * body. When variables are defined, only one of either expression, 3772 * headerField or path must be specified.). This is the underlying 3773 * object with id, value and extensions. The accessor "getPath" gives 3774 * direct access to the value 3775 */ 3776 public StringType getPathElement() { 3777 if (this.path == null) 3778 if (Configuration.errorOnAutoCreate()) 3779 throw new Error("Attempt to auto-create TestScriptVariableComponent.path"); 3780 else if (Configuration.doAutoCreate()) 3781 this.path = new StringType(); // bb 3782 return this.path; 3783 } 3784 3785 public boolean hasPathElement() { 3786 return this.path != null && !this.path.isEmpty(); 3787 } 3788 3789 public boolean hasPath() { 3790 return this.path != null && !this.path.isEmpty(); 3791 } 3792 3793 /** 3794 * @param value {@link #path} (XPath or JSONPath to evaluate against the fixture 3795 * body. When variables are defined, only one of either expression, 3796 * headerField or path must be specified.). This is the underlying 3797 * object with id, value and extensions. The accessor "getPath" 3798 * gives direct access to the value 3799 */ 3800 public TestScriptVariableComponent setPathElement(StringType value) { 3801 this.path = value; 3802 return this; 3803 } 3804 3805 /** 3806 * @return XPath or JSONPath to evaluate against the fixture body. When 3807 * variables are defined, only one of either expression, headerField or 3808 * path must be specified. 3809 */ 3810 public String getPath() { 3811 return this.path == null ? null : this.path.getValue(); 3812 } 3813 3814 /** 3815 * @param value XPath or JSONPath to evaluate against the fixture body. When 3816 * variables are defined, only one of either expression, 3817 * headerField or path must be specified. 3818 */ 3819 public TestScriptVariableComponent setPath(String value) { 3820 if (Utilities.noString(value)) 3821 this.path = null; 3822 else { 3823 if (this.path == null) 3824 this.path = new StringType(); 3825 this.path.setValue(value); 3826 } 3827 return this; 3828 } 3829 3830 /** 3831 * @return {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression 3832 * or the headerField against within this variable.). This is the 3833 * underlying object with id, value and extensions. The accessor 3834 * "getSourceId" gives direct access to the value 3835 */ 3836 public IdType getSourceIdElement() { 3837 if (this.sourceId == null) 3838 if (Configuration.errorOnAutoCreate()) 3839 throw new Error("Attempt to auto-create TestScriptVariableComponent.sourceId"); 3840 else if (Configuration.doAutoCreate()) 3841 this.sourceId = new IdType(); // bb 3842 return this.sourceId; 3843 } 3844 3845 public boolean hasSourceIdElement() { 3846 return this.sourceId != null && !this.sourceId.isEmpty(); 3847 } 3848 3849 public boolean hasSourceId() { 3850 return this.sourceId != null && !this.sourceId.isEmpty(); 3851 } 3852 3853 /** 3854 * @param value {@link #sourceId} (Fixture to evaluate the XPath/JSONPath 3855 * expression or the headerField against within this variable.). 3856 * This is the underlying object with id, value and extensions. The 3857 * accessor "getSourceId" gives direct access to the value 3858 */ 3859 public TestScriptVariableComponent setSourceIdElement(IdType value) { 3860 this.sourceId = value; 3861 return this; 3862 } 3863 3864 /** 3865 * @return Fixture to evaluate the XPath/JSONPath expression or the headerField 3866 * against within this variable. 3867 */ 3868 public String getSourceId() { 3869 return this.sourceId == null ? null : this.sourceId.getValue(); 3870 } 3871 3872 /** 3873 * @param value Fixture to evaluate the XPath/JSONPath expression or the 3874 * headerField against within this variable. 3875 */ 3876 public TestScriptVariableComponent setSourceId(String value) { 3877 if (Utilities.noString(value)) 3878 this.sourceId = null; 3879 else { 3880 if (this.sourceId == null) 3881 this.sourceId = new IdType(); 3882 this.sourceId.setValue(value); 3883 } 3884 return this; 3885 } 3886 3887 protected void listChildren(List<Property> children) { 3888 super.listChildren(children); 3889 children.add(new Property("name", "string", "Descriptive name for this variable.", 0, 1, name)); 3890 children.add(new Property("defaultValue", "string", 3891 "A default, hard-coded, or user-defined value for this variable.", 0, 1, defaultValue)); 3892 children.add(new Property("description", "string", 3893 "A free text natural language description of the variable and its purpose.", 0, 1, description)); 3894 children.add(new Property("expression", "string", 3895 "The FHIRPath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 3896 0, 1, expression)); 3897 children.add(new Property("headerField", "string", 3898 "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.", 0, 1, 3899 headerField)); 3900 children.add(new Property("hint", "string", 3901 "Displayable text string with hint help information to the user when entering a default value.", 0, 1, hint)); 3902 children.add(new Property("path", "string", 3903 "XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 3904 0, 1, path)); 3905 children.add(new Property("sourceId", "id", 3906 "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.", 0, 1, 3907 sourceId)); 3908 } 3909 3910 @Override 3911 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3912 switch (_hash) { 3913 case 3373707: 3914 /* name */ return new Property("name", "string", "Descriptive name for this variable.", 0, 1, name); 3915 case -659125328: 3916 /* defaultValue */ return new Property("defaultValue", "string", 3917 "A default, hard-coded, or user-defined value for this variable.", 0, 1, defaultValue); 3918 case -1724546052: 3919 /* description */ return new Property("description", "string", 3920 "A free text natural language description of the variable and its purpose.", 0, 1, description); 3921 case -1795452264: 3922 /* expression */ return new Property("expression", "string", 3923 "The FHIRPath expression to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 3924 0, 1, expression); 3925 case 1160732269: 3926 /* headerField */ return new Property("headerField", "string", 3927 "Will be used to grab the HTTP header field value from the headers that sourceId is pointing to.", 0, 1, 3928 headerField); 3929 case 3202695: 3930 /* hint */ return new Property("hint", "string", 3931 "Displayable text string with hint help information to the user when entering a default value.", 0, 1, 3932 hint); 3933 case 3433509: 3934 /* path */ return new Property("path", "string", 3935 "XPath or JSONPath to evaluate against the fixture body. When variables are defined, only one of either expression, headerField or path must be specified.", 3936 0, 1, path); 3937 case 1746327190: 3938 /* sourceId */ return new Property("sourceId", "id", 3939 "Fixture to evaluate the XPath/JSONPath expression or the headerField against within this variable.", 0, 1, 3940 sourceId); 3941 default: 3942 return super.getNamedProperty(_hash, _name, _checkValid); 3943 } 3944 3945 } 3946 3947 @Override 3948 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3949 switch (hash) { 3950 case 3373707: 3951 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3952 case -659125328: 3953 /* defaultValue */ return this.defaultValue == null ? new Base[0] : new Base[] { this.defaultValue }; // StringType 3954 case -1724546052: 3955 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 3956 case -1795452264: 3957 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 3958 case 1160732269: 3959 /* headerField */ return this.headerField == null ? new Base[0] : new Base[] { this.headerField }; // StringType 3960 case 3202695: 3961 /* hint */ return this.hint == null ? new Base[0] : new Base[] { this.hint }; // StringType 3962 case 3433509: 3963 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 3964 case 1746327190: 3965 /* sourceId */ return this.sourceId == null ? new Base[0] : new Base[] { this.sourceId }; // IdType 3966 default: 3967 return super.getProperty(hash, name, checkValid); 3968 } 3969 3970 } 3971 3972 @Override 3973 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3974 switch (hash) { 3975 case 3373707: // name 3976 this.name = castToString(value); // StringType 3977 return value; 3978 case -659125328: // defaultValue 3979 this.defaultValue = castToString(value); // StringType 3980 return value; 3981 case -1724546052: // description 3982 this.description = castToString(value); // StringType 3983 return value; 3984 case -1795452264: // expression 3985 this.expression = castToString(value); // StringType 3986 return value; 3987 case 1160732269: // headerField 3988 this.headerField = castToString(value); // StringType 3989 return value; 3990 case 3202695: // hint 3991 this.hint = castToString(value); // StringType 3992 return value; 3993 case 3433509: // path 3994 this.path = castToString(value); // StringType 3995 return value; 3996 case 1746327190: // sourceId 3997 this.sourceId = castToId(value); // IdType 3998 return value; 3999 default: 4000 return super.setProperty(hash, name, value); 4001 } 4002 4003 } 4004 4005 @Override 4006 public Base setProperty(String name, Base value) throws FHIRException { 4007 if (name.equals("name")) { 4008 this.name = castToString(value); // StringType 4009 } else if (name.equals("defaultValue")) { 4010 this.defaultValue = castToString(value); // StringType 4011 } else if (name.equals("description")) { 4012 this.description = castToString(value); // StringType 4013 } else if (name.equals("expression")) { 4014 this.expression = castToString(value); // StringType 4015 } else if (name.equals("headerField")) { 4016 this.headerField = castToString(value); // StringType 4017 } else if (name.equals("hint")) { 4018 this.hint = castToString(value); // StringType 4019 } else if (name.equals("path")) { 4020 this.path = castToString(value); // StringType 4021 } else if (name.equals("sourceId")) { 4022 this.sourceId = castToId(value); // IdType 4023 } else 4024 return super.setProperty(name, value); 4025 return value; 4026 } 4027 4028 @Override 4029 public void removeChild(String name, Base value) throws FHIRException { 4030 if (name.equals("name")) { 4031 this.name = null; 4032 } else if (name.equals("defaultValue")) { 4033 this.defaultValue = null; 4034 } else if (name.equals("description")) { 4035 this.description = null; 4036 } else if (name.equals("expression")) { 4037 this.expression = null; 4038 } else if (name.equals("headerField")) { 4039 this.headerField = null; 4040 } else if (name.equals("hint")) { 4041 this.hint = null; 4042 } else if (name.equals("path")) { 4043 this.path = null; 4044 } else if (name.equals("sourceId")) { 4045 this.sourceId = null; 4046 } else 4047 super.removeChild(name, value); 4048 4049 } 4050 4051 @Override 4052 public Base makeProperty(int hash, String name) throws FHIRException { 4053 switch (hash) { 4054 case 3373707: 4055 return getNameElement(); 4056 case -659125328: 4057 return getDefaultValueElement(); 4058 case -1724546052: 4059 return getDescriptionElement(); 4060 case -1795452264: 4061 return getExpressionElement(); 4062 case 1160732269: 4063 return getHeaderFieldElement(); 4064 case 3202695: 4065 return getHintElement(); 4066 case 3433509: 4067 return getPathElement(); 4068 case 1746327190: 4069 return getSourceIdElement(); 4070 default: 4071 return super.makeProperty(hash, name); 4072 } 4073 4074 } 4075 4076 @Override 4077 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4078 switch (hash) { 4079 case 3373707: 4080 /* name */ return new String[] { "string" }; 4081 case -659125328: 4082 /* defaultValue */ return new String[] { "string" }; 4083 case -1724546052: 4084 /* description */ return new String[] { "string" }; 4085 case -1795452264: 4086 /* expression */ return new String[] { "string" }; 4087 case 1160732269: 4088 /* headerField */ return new String[] { "string" }; 4089 case 3202695: 4090 /* hint */ return new String[] { "string" }; 4091 case 3433509: 4092 /* path */ return new String[] { "string" }; 4093 case 1746327190: 4094 /* sourceId */ return new String[] { "id" }; 4095 default: 4096 return super.getTypesForProperty(hash, name); 4097 } 4098 4099 } 4100 4101 @Override 4102 public Base addChild(String name) throws FHIRException { 4103 if (name.equals("name")) { 4104 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 4105 } else if (name.equals("defaultValue")) { 4106 throw new FHIRException("Cannot call addChild on a singleton property TestScript.defaultValue"); 4107 } else if (name.equals("description")) { 4108 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 4109 } else if (name.equals("expression")) { 4110 throw new FHIRException("Cannot call addChild on a singleton property TestScript.expression"); 4111 } else if (name.equals("headerField")) { 4112 throw new FHIRException("Cannot call addChild on a singleton property TestScript.headerField"); 4113 } else if (name.equals("hint")) { 4114 throw new FHIRException("Cannot call addChild on a singleton property TestScript.hint"); 4115 } else if (name.equals("path")) { 4116 throw new FHIRException("Cannot call addChild on a singleton property TestScript.path"); 4117 } else if (name.equals("sourceId")) { 4118 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 4119 } else 4120 return super.addChild(name); 4121 } 4122 4123 public TestScriptVariableComponent copy() { 4124 TestScriptVariableComponent dst = new TestScriptVariableComponent(); 4125 copyValues(dst); 4126 return dst; 4127 } 4128 4129 public void copyValues(TestScriptVariableComponent dst) { 4130 super.copyValues(dst); 4131 dst.name = name == null ? null : name.copy(); 4132 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 4133 dst.description = description == null ? null : description.copy(); 4134 dst.expression = expression == null ? null : expression.copy(); 4135 dst.headerField = headerField == null ? null : headerField.copy(); 4136 dst.hint = hint == null ? null : hint.copy(); 4137 dst.path = path == null ? null : path.copy(); 4138 dst.sourceId = sourceId == null ? null : sourceId.copy(); 4139 } 4140 4141 @Override 4142 public boolean equalsDeep(Base other_) { 4143 if (!super.equalsDeep(other_)) 4144 return false; 4145 if (!(other_ instanceof TestScriptVariableComponent)) 4146 return false; 4147 TestScriptVariableComponent o = (TestScriptVariableComponent) other_; 4148 return compareDeep(name, o.name, true) && compareDeep(defaultValue, o.defaultValue, true) 4149 && compareDeep(description, o.description, true) && compareDeep(expression, o.expression, true) 4150 && compareDeep(headerField, o.headerField, true) && compareDeep(hint, o.hint, true) 4151 && compareDeep(path, o.path, true) && compareDeep(sourceId, o.sourceId, true); 4152 } 4153 4154 @Override 4155 public boolean equalsShallow(Base other_) { 4156 if (!super.equalsShallow(other_)) 4157 return false; 4158 if (!(other_ instanceof TestScriptVariableComponent)) 4159 return false; 4160 TestScriptVariableComponent o = (TestScriptVariableComponent) other_; 4161 return compareValues(name, o.name, true) && compareValues(defaultValue, o.defaultValue, true) 4162 && compareValues(description, o.description, true) && compareValues(expression, o.expression, true) 4163 && compareValues(headerField, o.headerField, true) && compareValues(hint, o.hint, true) 4164 && compareValues(path, o.path, true) && compareValues(sourceId, o.sourceId, true); 4165 } 4166 4167 public boolean isEmpty() { 4168 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, defaultValue, description, expression, 4169 headerField, hint, path, sourceId); 4170 } 4171 4172 public String fhirType() { 4173 return "TestScript.variable"; 4174 4175 } 4176 4177 } 4178 4179 @Block() 4180 public static class TestScriptSetupComponent extends BackboneElement implements IBaseBackboneElement { 4181 /** 4182 * Action would contain either an operation or an assertion. 4183 */ 4184 @Child(name = "action", type = {}, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4185 @Description(shortDefinition = "A setup operation or assert to perform", formalDefinition = "Action would contain either an operation or an assertion.") 4186 protected List<SetupActionComponent> action; 4187 4188 private static final long serialVersionUID = -123374486L; 4189 4190 /** 4191 * Constructor 4192 */ 4193 public TestScriptSetupComponent() { 4194 super(); 4195 } 4196 4197 /** 4198 * @return {@link #action} (Action would contain either an operation or an 4199 * assertion.) 4200 */ 4201 public List<SetupActionComponent> getAction() { 4202 if (this.action == null) 4203 this.action = new ArrayList<SetupActionComponent>(); 4204 return this.action; 4205 } 4206 4207 /** 4208 * @return Returns a reference to <code>this</code> for easy method chaining 4209 */ 4210 public TestScriptSetupComponent setAction(List<SetupActionComponent> theAction) { 4211 this.action = theAction; 4212 return this; 4213 } 4214 4215 public boolean hasAction() { 4216 if (this.action == null) 4217 return false; 4218 for (SetupActionComponent item : this.action) 4219 if (!item.isEmpty()) 4220 return true; 4221 return false; 4222 } 4223 4224 public SetupActionComponent addAction() { // 3 4225 SetupActionComponent t = new SetupActionComponent(); 4226 if (this.action == null) 4227 this.action = new ArrayList<SetupActionComponent>(); 4228 this.action.add(t); 4229 return t; 4230 } 4231 4232 public TestScriptSetupComponent addAction(SetupActionComponent t) { // 3 4233 if (t == null) 4234 return this; 4235 if (this.action == null) 4236 this.action = new ArrayList<SetupActionComponent>(); 4237 this.action.add(t); 4238 return this; 4239 } 4240 4241 /** 4242 * @return The first repetition of repeating field {@link #action}, creating it 4243 * if it does not already exist 4244 */ 4245 public SetupActionComponent getActionFirstRep() { 4246 if (getAction().isEmpty()) { 4247 addAction(); 4248 } 4249 return getAction().get(0); 4250 } 4251 4252 protected void listChildren(List<Property> children) { 4253 super.listChildren(children); 4254 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, 4255 java.lang.Integer.MAX_VALUE, action)); 4256 } 4257 4258 @Override 4259 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4260 switch (_hash) { 4261 case -1422950858: 4262 /* action */ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, 4263 java.lang.Integer.MAX_VALUE, action); 4264 default: 4265 return super.getNamedProperty(_hash, _name, _checkValid); 4266 } 4267 4268 } 4269 4270 @Override 4271 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4272 switch (hash) { 4273 case -1422950858: 4274 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // SetupActionComponent 4275 default: 4276 return super.getProperty(hash, name, checkValid); 4277 } 4278 4279 } 4280 4281 @Override 4282 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4283 switch (hash) { 4284 case -1422950858: // action 4285 this.getAction().add((SetupActionComponent) value); // SetupActionComponent 4286 return value; 4287 default: 4288 return super.setProperty(hash, name, value); 4289 } 4290 4291 } 4292 4293 @Override 4294 public Base setProperty(String name, Base value) throws FHIRException { 4295 if (name.equals("action")) { 4296 this.getAction().add((SetupActionComponent) value); 4297 } else 4298 return super.setProperty(name, value); 4299 return value; 4300 } 4301 4302 @Override 4303 public void removeChild(String name, Base value) throws FHIRException { 4304 if (name.equals("action")) { 4305 this.getAction().remove((SetupActionComponent) value); 4306 } else 4307 super.removeChild(name, value); 4308 4309 } 4310 4311 @Override 4312 public Base makeProperty(int hash, String name) throws FHIRException { 4313 switch (hash) { 4314 case -1422950858: 4315 return addAction(); 4316 default: 4317 return super.makeProperty(hash, name); 4318 } 4319 4320 } 4321 4322 @Override 4323 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4324 switch (hash) { 4325 case -1422950858: 4326 /* action */ return new String[] {}; 4327 default: 4328 return super.getTypesForProperty(hash, name); 4329 } 4330 4331 } 4332 4333 @Override 4334 public Base addChild(String name) throws FHIRException { 4335 if (name.equals("action")) { 4336 return addAction(); 4337 } else 4338 return super.addChild(name); 4339 } 4340 4341 public TestScriptSetupComponent copy() { 4342 TestScriptSetupComponent dst = new TestScriptSetupComponent(); 4343 copyValues(dst); 4344 return dst; 4345 } 4346 4347 public void copyValues(TestScriptSetupComponent dst) { 4348 super.copyValues(dst); 4349 if (action != null) { 4350 dst.action = new ArrayList<SetupActionComponent>(); 4351 for (SetupActionComponent i : action) 4352 dst.action.add(i.copy()); 4353 } 4354 ; 4355 } 4356 4357 @Override 4358 public boolean equalsDeep(Base other_) { 4359 if (!super.equalsDeep(other_)) 4360 return false; 4361 if (!(other_ instanceof TestScriptSetupComponent)) 4362 return false; 4363 TestScriptSetupComponent o = (TestScriptSetupComponent) other_; 4364 return compareDeep(action, o.action, true); 4365 } 4366 4367 @Override 4368 public boolean equalsShallow(Base other_) { 4369 if (!super.equalsShallow(other_)) 4370 return false; 4371 if (!(other_ instanceof TestScriptSetupComponent)) 4372 return false; 4373 TestScriptSetupComponent o = (TestScriptSetupComponent) other_; 4374 return true; 4375 } 4376 4377 public boolean isEmpty() { 4378 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 4379 } 4380 4381 public String fhirType() { 4382 return "TestScript.setup"; 4383 4384 } 4385 4386 } 4387 4388 @Block() 4389 public static class SetupActionComponent extends BackboneElement implements IBaseBackboneElement { 4390 /** 4391 * The operation to perform. 4392 */ 4393 @Child(name = "operation", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = false) 4394 @Description(shortDefinition = "The setup operation to perform", formalDefinition = "The operation to perform.") 4395 protected SetupActionOperationComponent operation; 4396 4397 /** 4398 * Evaluates the results of previous operations to determine if the server under 4399 * test behaves appropriately. 4400 */ 4401 @Child(name = "assert", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = false) 4402 @Description(shortDefinition = "The assertion to perform", formalDefinition = "Evaluates the results of previous operations to determine if the server under test behaves appropriately.") 4403 protected SetupActionAssertComponent assert_; 4404 4405 private static final long serialVersionUID = -252088305L; 4406 4407 /** 4408 * Constructor 4409 */ 4410 public SetupActionComponent() { 4411 super(); 4412 } 4413 4414 /** 4415 * @return {@link #operation} (The operation to perform.) 4416 */ 4417 public SetupActionOperationComponent getOperation() { 4418 if (this.operation == null) 4419 if (Configuration.errorOnAutoCreate()) 4420 throw new Error("Attempt to auto-create SetupActionComponent.operation"); 4421 else if (Configuration.doAutoCreate()) 4422 this.operation = new SetupActionOperationComponent(); // cc 4423 return this.operation; 4424 } 4425 4426 public boolean hasOperation() { 4427 return this.operation != null && !this.operation.isEmpty(); 4428 } 4429 4430 /** 4431 * @param value {@link #operation} (The operation to perform.) 4432 */ 4433 public SetupActionComponent setOperation(SetupActionOperationComponent value) { 4434 this.operation = value; 4435 return this; 4436 } 4437 4438 /** 4439 * @return {@link #assert_} (Evaluates the results of previous operations to 4440 * determine if the server under test behaves appropriately.) 4441 */ 4442 public SetupActionAssertComponent getAssert() { 4443 if (this.assert_ == null) 4444 if (Configuration.errorOnAutoCreate()) 4445 throw new Error("Attempt to auto-create SetupActionComponent.assert_"); 4446 else if (Configuration.doAutoCreate()) 4447 this.assert_ = new SetupActionAssertComponent(); // cc 4448 return this.assert_; 4449 } 4450 4451 public boolean hasAssert() { 4452 return this.assert_ != null && !this.assert_.isEmpty(); 4453 } 4454 4455 /** 4456 * @param value {@link #assert_} (Evaluates the results of previous operations 4457 * to determine if the server under test behaves appropriately.) 4458 */ 4459 public SetupActionComponent setAssert(SetupActionAssertComponent value) { 4460 this.assert_ = value; 4461 return this; 4462 } 4463 4464 protected void listChildren(List<Property> children) { 4465 super.listChildren(children); 4466 children.add(new Property("operation", "", "The operation to perform.", 0, 1, operation)); 4467 children.add(new Property("assert", "", 4468 "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 4469 0, 1, assert_)); 4470 } 4471 4472 @Override 4473 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4474 switch (_hash) { 4475 case 1662702951: 4476 /* operation */ return new Property("operation", "", "The operation to perform.", 0, 1, operation); 4477 case -1408208058: 4478 /* assert */ return new Property("assert", "", 4479 "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 4480 0, 1, assert_); 4481 default: 4482 return super.getNamedProperty(_hash, _name, _checkValid); 4483 } 4484 4485 } 4486 4487 @Override 4488 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4489 switch (hash) { 4490 case 1662702951: 4491 /* operation */ return this.operation == null ? new Base[0] : new Base[] { this.operation }; // SetupActionOperationComponent 4492 case -1408208058: 4493 /* assert */ return this.assert_ == null ? new Base[0] : new Base[] { this.assert_ }; // SetupActionAssertComponent 4494 default: 4495 return super.getProperty(hash, name, checkValid); 4496 } 4497 4498 } 4499 4500 @Override 4501 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4502 switch (hash) { 4503 case 1662702951: // operation 4504 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 4505 return value; 4506 case -1408208058: // assert 4507 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 4508 return value; 4509 default: 4510 return super.setProperty(hash, name, value); 4511 } 4512 4513 } 4514 4515 @Override 4516 public Base setProperty(String name, Base value) throws FHIRException { 4517 if (name.equals("operation")) { 4518 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 4519 } else if (name.equals("assert")) { 4520 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 4521 } else 4522 return super.setProperty(name, value); 4523 return value; 4524 } 4525 4526 @Override 4527 public void removeChild(String name, Base value) throws FHIRException { 4528 if (name.equals("operation")) { 4529 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 4530 } else if (name.equals("assert")) { 4531 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 4532 } else 4533 super.removeChild(name, value); 4534 4535 } 4536 4537 @Override 4538 public Base makeProperty(int hash, String name) throws FHIRException { 4539 switch (hash) { 4540 case 1662702951: 4541 return getOperation(); 4542 case -1408208058: 4543 return getAssert(); 4544 default: 4545 return super.makeProperty(hash, name); 4546 } 4547 4548 } 4549 4550 @Override 4551 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4552 switch (hash) { 4553 case 1662702951: 4554 /* operation */ return new String[] {}; 4555 case -1408208058: 4556 /* assert */ return new String[] {}; 4557 default: 4558 return super.getTypesForProperty(hash, name); 4559 } 4560 4561 } 4562 4563 @Override 4564 public Base addChild(String name) throws FHIRException { 4565 if (name.equals("operation")) { 4566 this.operation = new SetupActionOperationComponent(); 4567 return this.operation; 4568 } else if (name.equals("assert")) { 4569 this.assert_ = new SetupActionAssertComponent(); 4570 return this.assert_; 4571 } else 4572 return super.addChild(name); 4573 } 4574 4575 public SetupActionComponent copy() { 4576 SetupActionComponent dst = new SetupActionComponent(); 4577 copyValues(dst); 4578 return dst; 4579 } 4580 4581 public void copyValues(SetupActionComponent dst) { 4582 super.copyValues(dst); 4583 dst.operation = operation == null ? null : operation.copy(); 4584 dst.assert_ = assert_ == null ? null : assert_.copy(); 4585 } 4586 4587 @Override 4588 public boolean equalsDeep(Base other_) { 4589 if (!super.equalsDeep(other_)) 4590 return false; 4591 if (!(other_ instanceof SetupActionComponent)) 4592 return false; 4593 SetupActionComponent o = (SetupActionComponent) other_; 4594 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 4595 } 4596 4597 @Override 4598 public boolean equalsShallow(Base other_) { 4599 if (!super.equalsShallow(other_)) 4600 return false; 4601 if (!(other_ instanceof SetupActionComponent)) 4602 return false; 4603 SetupActionComponent o = (SetupActionComponent) other_; 4604 return true; 4605 } 4606 4607 public boolean isEmpty() { 4608 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 4609 } 4610 4611 public String fhirType() { 4612 return "TestScript.setup.action"; 4613 4614 } 4615 4616 } 4617 4618 @Block() 4619 public static class SetupActionOperationComponent extends BackboneElement implements IBaseBackboneElement { 4620 /** 4621 * Server interaction or operation type. 4622 */ 4623 @Child(name = "type", type = { Coding.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 4624 @Description(shortDefinition = "The operation code type that will be executed", formalDefinition = "Server interaction or operation type.") 4625 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/testscript-operation-codes") 4626 protected Coding type; 4627 4628 /** 4629 * The type of the resource. See http://build.fhir.org/resourcelist.html. 4630 */ 4631 @Child(name = "resource", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4632 @Description(shortDefinition = "Resource type", formalDefinition = "The type of the resource. See http://build.fhir.org/resourcelist.html.") 4633 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/defined-types") 4634 protected CodeType resource; 4635 4636 /** 4637 * The label would be used for tracking/logging purposes by test engines. 4638 */ 4639 @Child(name = "label", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4640 @Description(shortDefinition = "Tracking/logging operation label", formalDefinition = "The label would be used for tracking/logging purposes by test engines.") 4641 protected StringType label; 4642 4643 /** 4644 * The description would be used by test engines for tracking and reporting 4645 * purposes. 4646 */ 4647 @Child(name = "description", type = { 4648 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 4649 @Description(shortDefinition = "Tracking/reporting operation description", formalDefinition = "The description would be used by test engines for tracking and reporting purposes.") 4650 protected StringType description; 4651 4652 /** 4653 * The mime-type to use for RESTful operation in the 'Accept' header. 4654 */ 4655 @Child(name = "accept", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 4656 @Description(shortDefinition = "Mime type to accept in the payload of the response, with charset etc.", formalDefinition = "The mime-type to use for RESTful operation in the 'Accept' header.") 4657 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 4658 protected CodeType accept; 4659 4660 /** 4661 * The mime-type to use for RESTful operation in the 'Content-Type' header. 4662 */ 4663 @Child(name = "contentType", type = { 4664 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 4665 @Description(shortDefinition = "Mime type of the request payload contents, with charset etc.", formalDefinition = "The mime-type to use for RESTful operation in the 'Content-Type' header.") 4666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 4667 protected CodeType contentType; 4668 4669 /** 4670 * The server where the request message is destined for. Must be one of the 4671 * server numbers listed in TestScript.destination section. 4672 */ 4673 @Child(name = "destination", type = { 4674 IntegerType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 4675 @Description(shortDefinition = "Server responding to the request", formalDefinition = "The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.") 4676 protected IntegerType destination; 4677 4678 /** 4679 * Whether or not to implicitly send the request url in encoded format. The 4680 * default is true to match the standard RESTful client behavior. Set to false 4681 * when communicating with a server that does not support encoded url paths. 4682 */ 4683 @Child(name = "encodeRequestUrl", type = { 4684 BooleanType.class }, order = 8, min = 1, max = 1, modifier = false, summary = false) 4685 @Description(shortDefinition = "Whether or not to send the request url in encoded format", formalDefinition = "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.") 4686 protected BooleanType encodeRequestUrl; 4687 4688 /** 4689 * The HTTP method the test engine MUST use for this operation regardless of any 4690 * other operation details. 4691 */ 4692 @Child(name = "method", type = { CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 4693 @Description(shortDefinition = "delete | get | options | patch | post | put | head", formalDefinition = "The HTTP method the test engine MUST use for this operation regardless of any other operation details.") 4694 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/http-operations") 4695 protected Enumeration<TestScriptRequestMethodCode> method; 4696 4697 /** 4698 * The server where the request message originates from. Must be one of the 4699 * server numbers listed in TestScript.origin section. 4700 */ 4701 @Child(name = "origin", type = { 4702 IntegerType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 4703 @Description(shortDefinition = "Server initiating the request", formalDefinition = "The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.") 4704 protected IntegerType origin; 4705 4706 /** 4707 * Path plus parameters after [type]. Used to set parts of the request URL 4708 * explicitly. 4709 */ 4710 @Child(name = "params", type = { 4711 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4712 @Description(shortDefinition = "Explicitly defined path parameters", formalDefinition = "Path plus parameters after [type]. Used to set parts of the request URL explicitly.") 4713 protected StringType params; 4714 4715 /** 4716 * Header elements would be used to set HTTP headers. 4717 */ 4718 @Child(name = "requestHeader", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4719 @Description(shortDefinition = "Each operation can have one or more header elements", formalDefinition = "Header elements would be used to set HTTP headers.") 4720 protected List<SetupActionOperationRequestHeaderComponent> requestHeader; 4721 4722 /** 4723 * The fixture id (maybe new) to map to the request. 4724 */ 4725 @Child(name = "requestId", type = { IdType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 4726 @Description(shortDefinition = "Fixture Id of mapped request", formalDefinition = "The fixture id (maybe new) to map to the request.") 4727 protected IdType requestId; 4728 4729 /** 4730 * The fixture id (maybe new) to map to the response. 4731 */ 4732 @Child(name = "responseId", type = { 4733 IdType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 4734 @Description(shortDefinition = "Fixture Id of mapped response", formalDefinition = "The fixture id (maybe new) to map to the response.") 4735 protected IdType responseId; 4736 4737 /** 4738 * The id of the fixture used as the body of a PUT or POST request. 4739 */ 4740 @Child(name = "sourceId", type = { IdType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 4741 @Description(shortDefinition = "Fixture Id of body for PUT and POST requests", formalDefinition = "The id of the fixture used as the body of a PUT or POST request.") 4742 protected IdType sourceId; 4743 4744 /** 4745 * Id of fixture used for extracting the [id], [type], and [vid] for GET 4746 * requests. 4747 */ 4748 @Child(name = "targetId", type = { IdType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 4749 @Description(shortDefinition = "Id of fixture used for extracting the [id], [type], and [vid] for GET requests", formalDefinition = "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.") 4750 protected IdType targetId; 4751 4752 /** 4753 * Complete request URL. 4754 */ 4755 @Child(name = "url", type = { StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 4756 @Description(shortDefinition = "Request URL", formalDefinition = "Complete request URL.") 4757 protected StringType url; 4758 4759 private static final long serialVersionUID = 300050202L; 4760 4761 /** 4762 * Constructor 4763 */ 4764 public SetupActionOperationComponent() { 4765 super(); 4766 } 4767 4768 /** 4769 * Constructor 4770 */ 4771 public SetupActionOperationComponent(BooleanType encodeRequestUrl) { 4772 super(); 4773 this.encodeRequestUrl = encodeRequestUrl; 4774 } 4775 4776 /** 4777 * @return {@link #type} (Server interaction or operation type.) 4778 */ 4779 public Coding getType() { 4780 if (this.type == null) 4781 if (Configuration.errorOnAutoCreate()) 4782 throw new Error("Attempt to auto-create SetupActionOperationComponent.type"); 4783 else if (Configuration.doAutoCreate()) 4784 this.type = new Coding(); // cc 4785 return this.type; 4786 } 4787 4788 public boolean hasType() { 4789 return this.type != null && !this.type.isEmpty(); 4790 } 4791 4792 /** 4793 * @param value {@link #type} (Server interaction or operation type.) 4794 */ 4795 public SetupActionOperationComponent setType(Coding value) { 4796 this.type = value; 4797 return this; 4798 } 4799 4800 /** 4801 * @return {@link #resource} (The type of the resource. See 4802 * http://build.fhir.org/resourcelist.html.). This is the underlying 4803 * object with id, value and extensions. The accessor "getResource" 4804 * gives direct access to the value 4805 */ 4806 public CodeType getResourceElement() { 4807 if (this.resource == null) 4808 if (Configuration.errorOnAutoCreate()) 4809 throw new Error("Attempt to auto-create SetupActionOperationComponent.resource"); 4810 else if (Configuration.doAutoCreate()) 4811 this.resource = new CodeType(); // bb 4812 return this.resource; 4813 } 4814 4815 public boolean hasResourceElement() { 4816 return this.resource != null && !this.resource.isEmpty(); 4817 } 4818 4819 public boolean hasResource() { 4820 return this.resource != null && !this.resource.isEmpty(); 4821 } 4822 4823 /** 4824 * @param value {@link #resource} (The type of the resource. See 4825 * http://build.fhir.org/resourcelist.html.). This is the 4826 * underlying object with id, value and extensions. The accessor 4827 * "getResource" gives direct access to the value 4828 */ 4829 public SetupActionOperationComponent setResourceElement(CodeType value) { 4830 this.resource = value; 4831 return this; 4832 } 4833 4834 /** 4835 * @return The type of the resource. See 4836 * http://build.fhir.org/resourcelist.html. 4837 */ 4838 public String getResource() { 4839 return this.resource == null ? null : this.resource.getValue(); 4840 } 4841 4842 /** 4843 * @param value The type of the resource. See 4844 * http://build.fhir.org/resourcelist.html. 4845 */ 4846 public SetupActionOperationComponent setResource(String value) { 4847 if (Utilities.noString(value)) 4848 this.resource = null; 4849 else { 4850 if (this.resource == null) 4851 this.resource = new CodeType(); 4852 this.resource.setValue(value); 4853 } 4854 return this; 4855 } 4856 4857 /** 4858 * @return {@link #label} (The label would be used for tracking/logging purposes 4859 * by test engines.). This is the underlying object with id, value and 4860 * extensions. The accessor "getLabel" gives direct access to the value 4861 */ 4862 public StringType getLabelElement() { 4863 if (this.label == null) 4864 if (Configuration.errorOnAutoCreate()) 4865 throw new Error("Attempt to auto-create SetupActionOperationComponent.label"); 4866 else if (Configuration.doAutoCreate()) 4867 this.label = new StringType(); // bb 4868 return this.label; 4869 } 4870 4871 public boolean hasLabelElement() { 4872 return this.label != null && !this.label.isEmpty(); 4873 } 4874 4875 public boolean hasLabel() { 4876 return this.label != null && !this.label.isEmpty(); 4877 } 4878 4879 /** 4880 * @param value {@link #label} (The label would be used for tracking/logging 4881 * purposes by test engines.). This is the underlying object with 4882 * id, value and extensions. The accessor "getLabel" gives direct 4883 * access to the value 4884 */ 4885 public SetupActionOperationComponent setLabelElement(StringType value) { 4886 this.label = value; 4887 return this; 4888 } 4889 4890 /** 4891 * @return The label would be used for tracking/logging purposes by test 4892 * engines. 4893 */ 4894 public String getLabel() { 4895 return this.label == null ? null : this.label.getValue(); 4896 } 4897 4898 /** 4899 * @param value The label would be used for tracking/logging purposes by test 4900 * engines. 4901 */ 4902 public SetupActionOperationComponent setLabel(String value) { 4903 if (Utilities.noString(value)) 4904 this.label = null; 4905 else { 4906 if (this.label == null) 4907 this.label = new StringType(); 4908 this.label.setValue(value); 4909 } 4910 return this; 4911 } 4912 4913 /** 4914 * @return {@link #description} (The description would be used by test engines 4915 * for tracking and reporting purposes.). This is the underlying object 4916 * with id, value and extensions. The accessor "getDescription" gives 4917 * direct access to the value 4918 */ 4919 public StringType getDescriptionElement() { 4920 if (this.description == null) 4921 if (Configuration.errorOnAutoCreate()) 4922 throw new Error("Attempt to auto-create SetupActionOperationComponent.description"); 4923 else if (Configuration.doAutoCreate()) 4924 this.description = new StringType(); // bb 4925 return this.description; 4926 } 4927 4928 public boolean hasDescriptionElement() { 4929 return this.description != null && !this.description.isEmpty(); 4930 } 4931 4932 public boolean hasDescription() { 4933 return this.description != null && !this.description.isEmpty(); 4934 } 4935 4936 /** 4937 * @param value {@link #description} (The description would be used by test 4938 * engines for tracking and reporting purposes.). This is the 4939 * underlying object with id, value and extensions. The accessor 4940 * "getDescription" gives direct access to the value 4941 */ 4942 public SetupActionOperationComponent setDescriptionElement(StringType value) { 4943 this.description = value; 4944 return this; 4945 } 4946 4947 /** 4948 * @return The description would be used by test engines for tracking and 4949 * reporting purposes. 4950 */ 4951 public String getDescription() { 4952 return this.description == null ? null : this.description.getValue(); 4953 } 4954 4955 /** 4956 * @param value The description would be used by test engines for tracking and 4957 * reporting purposes. 4958 */ 4959 public SetupActionOperationComponent setDescription(String value) { 4960 if (Utilities.noString(value)) 4961 this.description = null; 4962 else { 4963 if (this.description == null) 4964 this.description = new StringType(); 4965 this.description.setValue(value); 4966 } 4967 return this; 4968 } 4969 4970 /** 4971 * @return {@link #accept} (The mime-type to use for RESTful operation in the 4972 * 'Accept' header.). This is the underlying object with id, value and 4973 * extensions. The accessor "getAccept" gives direct access to the value 4974 */ 4975 public CodeType getAcceptElement() { 4976 if (this.accept == null) 4977 if (Configuration.errorOnAutoCreate()) 4978 throw new Error("Attempt to auto-create SetupActionOperationComponent.accept"); 4979 else if (Configuration.doAutoCreate()) 4980 this.accept = new CodeType(); // bb 4981 return this.accept; 4982 } 4983 4984 public boolean hasAcceptElement() { 4985 return this.accept != null && !this.accept.isEmpty(); 4986 } 4987 4988 public boolean hasAccept() { 4989 return this.accept != null && !this.accept.isEmpty(); 4990 } 4991 4992 /** 4993 * @param value {@link #accept} (The mime-type to use for RESTful operation in 4994 * the 'Accept' header.). This is the underlying object with id, 4995 * value and extensions. The accessor "getAccept" gives direct 4996 * access to the value 4997 */ 4998 public SetupActionOperationComponent setAcceptElement(CodeType value) { 4999 this.accept = value; 5000 return this; 5001 } 5002 5003 /** 5004 * @return The mime-type to use for RESTful operation in the 'Accept' header. 5005 */ 5006 public String getAccept() { 5007 return this.accept == null ? null : this.accept.getValue(); 5008 } 5009 5010 /** 5011 * @param value The mime-type to use for RESTful operation in the 'Accept' 5012 * header. 5013 */ 5014 public SetupActionOperationComponent setAccept(String value) { 5015 if (Utilities.noString(value)) 5016 this.accept = null; 5017 else { 5018 if (this.accept == null) 5019 this.accept = new CodeType(); 5020 this.accept.setValue(value); 5021 } 5022 return this; 5023 } 5024 5025 /** 5026 * @return {@link #contentType} (The mime-type to use for RESTful operation in 5027 * the 'Content-Type' header.). This is the underlying object with id, 5028 * value and extensions. The accessor "getContentType" gives direct 5029 * access to the value 5030 */ 5031 public CodeType getContentTypeElement() { 5032 if (this.contentType == null) 5033 if (Configuration.errorOnAutoCreate()) 5034 throw new Error("Attempt to auto-create SetupActionOperationComponent.contentType"); 5035 else if (Configuration.doAutoCreate()) 5036 this.contentType = new CodeType(); // bb 5037 return this.contentType; 5038 } 5039 5040 public boolean hasContentTypeElement() { 5041 return this.contentType != null && !this.contentType.isEmpty(); 5042 } 5043 5044 public boolean hasContentType() { 5045 return this.contentType != null && !this.contentType.isEmpty(); 5046 } 5047 5048 /** 5049 * @param value {@link #contentType} (The mime-type to use for RESTful operation 5050 * in the 'Content-Type' header.). This is the underlying object 5051 * with id, value and extensions. The accessor "getContentType" 5052 * gives direct access to the value 5053 */ 5054 public SetupActionOperationComponent setContentTypeElement(CodeType value) { 5055 this.contentType = value; 5056 return this; 5057 } 5058 5059 /** 5060 * @return The mime-type to use for RESTful operation in the 'Content-Type' 5061 * header. 5062 */ 5063 public String getContentType() { 5064 return this.contentType == null ? null : this.contentType.getValue(); 5065 } 5066 5067 /** 5068 * @param value The mime-type to use for RESTful operation in the 'Content-Type' 5069 * header. 5070 */ 5071 public SetupActionOperationComponent setContentType(String value) { 5072 if (Utilities.noString(value)) 5073 this.contentType = null; 5074 else { 5075 if (this.contentType == null) 5076 this.contentType = new CodeType(); 5077 this.contentType.setValue(value); 5078 } 5079 return this; 5080 } 5081 5082 /** 5083 * @return {@link #destination} (The server where the request message is 5084 * destined for. Must be one of the server numbers listed in 5085 * TestScript.destination section.). This is the underlying object with 5086 * id, value and extensions. The accessor "getDestination" gives direct 5087 * access to the value 5088 */ 5089 public IntegerType getDestinationElement() { 5090 if (this.destination == null) 5091 if (Configuration.errorOnAutoCreate()) 5092 throw new Error("Attempt to auto-create SetupActionOperationComponent.destination"); 5093 else if (Configuration.doAutoCreate()) 5094 this.destination = new IntegerType(); // bb 5095 return this.destination; 5096 } 5097 5098 public boolean hasDestinationElement() { 5099 return this.destination != null && !this.destination.isEmpty(); 5100 } 5101 5102 public boolean hasDestination() { 5103 return this.destination != null && !this.destination.isEmpty(); 5104 } 5105 5106 /** 5107 * @param value {@link #destination} (The server where the request message is 5108 * destined for. Must be one of the server numbers listed in 5109 * TestScript.destination section.). This is the underlying object 5110 * with id, value and extensions. The accessor "getDestination" 5111 * gives direct access to the value 5112 */ 5113 public SetupActionOperationComponent setDestinationElement(IntegerType value) { 5114 this.destination = value; 5115 return this; 5116 } 5117 5118 /** 5119 * @return The server where the request message is destined for. Must be one of 5120 * the server numbers listed in TestScript.destination section. 5121 */ 5122 public int getDestination() { 5123 return this.destination == null || this.destination.isEmpty() ? 0 : this.destination.getValue(); 5124 } 5125 5126 /** 5127 * @param value The server where the request message is destined for. Must be 5128 * one of the server numbers listed in TestScript.destination 5129 * section. 5130 */ 5131 public SetupActionOperationComponent setDestination(int value) { 5132 if (this.destination == null) 5133 this.destination = new IntegerType(); 5134 this.destination.setValue(value); 5135 return this; 5136 } 5137 5138 /** 5139 * @return {@link #encodeRequestUrl} (Whether or not to implicitly send the 5140 * request url in encoded format. The default is true to match the 5141 * standard RESTful client behavior. Set to false when communicating 5142 * with a server that does not support encoded url paths.). This is the 5143 * underlying object with id, value and extensions. The accessor 5144 * "getEncodeRequestUrl" gives direct access to the value 5145 */ 5146 public BooleanType getEncodeRequestUrlElement() { 5147 if (this.encodeRequestUrl == null) 5148 if (Configuration.errorOnAutoCreate()) 5149 throw new Error("Attempt to auto-create SetupActionOperationComponent.encodeRequestUrl"); 5150 else if (Configuration.doAutoCreate()) 5151 this.encodeRequestUrl = new BooleanType(); // bb 5152 return this.encodeRequestUrl; 5153 } 5154 5155 public boolean hasEncodeRequestUrlElement() { 5156 return this.encodeRequestUrl != null && !this.encodeRequestUrl.isEmpty(); 5157 } 5158 5159 public boolean hasEncodeRequestUrl() { 5160 return this.encodeRequestUrl != null && !this.encodeRequestUrl.isEmpty(); 5161 } 5162 5163 /** 5164 * @param value {@link #encodeRequestUrl} (Whether or not to implicitly send the 5165 * request url in encoded format. The default is true to match the 5166 * standard RESTful client behavior. Set to false when 5167 * communicating with a server that does not support encoded url 5168 * paths.). This is the underlying object with id, value and 5169 * extensions. The accessor "getEncodeRequestUrl" gives direct 5170 * access to the value 5171 */ 5172 public SetupActionOperationComponent setEncodeRequestUrlElement(BooleanType value) { 5173 this.encodeRequestUrl = value; 5174 return this; 5175 } 5176 5177 /** 5178 * @return Whether or not to implicitly send the request url in encoded format. 5179 * The default is true to match the standard RESTful client behavior. 5180 * Set to false when communicating with a server that does not support 5181 * encoded url paths. 5182 */ 5183 public boolean getEncodeRequestUrl() { 5184 return this.encodeRequestUrl == null || this.encodeRequestUrl.isEmpty() ? false 5185 : this.encodeRequestUrl.getValue(); 5186 } 5187 5188 /** 5189 * @param value Whether or not to implicitly send the request url in encoded 5190 * format. The default is true to match the standard RESTful client 5191 * behavior. Set to false when communicating with a server that 5192 * does not support encoded url paths. 5193 */ 5194 public SetupActionOperationComponent setEncodeRequestUrl(boolean value) { 5195 if (this.encodeRequestUrl == null) 5196 this.encodeRequestUrl = new BooleanType(); 5197 this.encodeRequestUrl.setValue(value); 5198 return this; 5199 } 5200 5201 /** 5202 * @return {@link #method} (The HTTP method the test engine MUST use for this 5203 * operation regardless of any other operation details.). This is the 5204 * underlying object with id, value and extensions. The accessor 5205 * "getMethod" gives direct access to the value 5206 */ 5207 public Enumeration<TestScriptRequestMethodCode> getMethodElement() { 5208 if (this.method == null) 5209 if (Configuration.errorOnAutoCreate()) 5210 throw new Error("Attempt to auto-create SetupActionOperationComponent.method"); 5211 else if (Configuration.doAutoCreate()) 5212 this.method = new Enumeration<TestScriptRequestMethodCode>(new TestScriptRequestMethodCodeEnumFactory()); // bb 5213 return this.method; 5214 } 5215 5216 public boolean hasMethodElement() { 5217 return this.method != null && !this.method.isEmpty(); 5218 } 5219 5220 public boolean hasMethod() { 5221 return this.method != null && !this.method.isEmpty(); 5222 } 5223 5224 /** 5225 * @param value {@link #method} (The HTTP method the test engine MUST use for 5226 * this operation regardless of any other operation details.). This 5227 * is the underlying object with id, value and extensions. The 5228 * accessor "getMethod" gives direct access to the value 5229 */ 5230 public SetupActionOperationComponent setMethodElement(Enumeration<TestScriptRequestMethodCode> value) { 5231 this.method = value; 5232 return this; 5233 } 5234 5235 /** 5236 * @return The HTTP method the test engine MUST use for this operation 5237 * regardless of any other operation details. 5238 */ 5239 public TestScriptRequestMethodCode getMethod() { 5240 return this.method == null ? null : this.method.getValue(); 5241 } 5242 5243 /** 5244 * @param value The HTTP method the test engine MUST use for this operation 5245 * regardless of any other operation details. 5246 */ 5247 public SetupActionOperationComponent setMethod(TestScriptRequestMethodCode value) { 5248 if (value == null) 5249 this.method = null; 5250 else { 5251 if (this.method == null) 5252 this.method = new Enumeration<TestScriptRequestMethodCode>(new TestScriptRequestMethodCodeEnumFactory()); 5253 this.method.setValue(value); 5254 } 5255 return this; 5256 } 5257 5258 /** 5259 * @return {@link #origin} (The server where the request message originates 5260 * from. Must be one of the server numbers listed in TestScript.origin 5261 * section.). This is the underlying object with id, value and 5262 * extensions. The accessor "getOrigin" gives direct access to the value 5263 */ 5264 public IntegerType getOriginElement() { 5265 if (this.origin == null) 5266 if (Configuration.errorOnAutoCreate()) 5267 throw new Error("Attempt to auto-create SetupActionOperationComponent.origin"); 5268 else if (Configuration.doAutoCreate()) 5269 this.origin = new IntegerType(); // bb 5270 return this.origin; 5271 } 5272 5273 public boolean hasOriginElement() { 5274 return this.origin != null && !this.origin.isEmpty(); 5275 } 5276 5277 public boolean hasOrigin() { 5278 return this.origin != null && !this.origin.isEmpty(); 5279 } 5280 5281 /** 5282 * @param value {@link #origin} (The server where the request message originates 5283 * from. Must be one of the server numbers listed in 5284 * TestScript.origin section.). This is the underlying object with 5285 * id, value and extensions. The accessor "getOrigin" gives direct 5286 * access to the value 5287 */ 5288 public SetupActionOperationComponent setOriginElement(IntegerType value) { 5289 this.origin = value; 5290 return this; 5291 } 5292 5293 /** 5294 * @return The server where the request message originates from. Must be one of 5295 * the server numbers listed in TestScript.origin section. 5296 */ 5297 public int getOrigin() { 5298 return this.origin == null || this.origin.isEmpty() ? 0 : this.origin.getValue(); 5299 } 5300 5301 /** 5302 * @param value The server where the request message originates from. Must be 5303 * one of the server numbers listed in TestScript.origin section. 5304 */ 5305 public SetupActionOperationComponent setOrigin(int value) { 5306 if (this.origin == null) 5307 this.origin = new IntegerType(); 5308 this.origin.setValue(value); 5309 return this; 5310 } 5311 5312 /** 5313 * @return {@link #params} (Path plus parameters after [type]. Used to set parts 5314 * of the request URL explicitly.). This is the underlying object with 5315 * id, value and extensions. The accessor "getParams" gives direct 5316 * access to the value 5317 */ 5318 public StringType getParamsElement() { 5319 if (this.params == null) 5320 if (Configuration.errorOnAutoCreate()) 5321 throw new Error("Attempt to auto-create SetupActionOperationComponent.params"); 5322 else if (Configuration.doAutoCreate()) 5323 this.params = new StringType(); // bb 5324 return this.params; 5325 } 5326 5327 public boolean hasParamsElement() { 5328 return this.params != null && !this.params.isEmpty(); 5329 } 5330 5331 public boolean hasParams() { 5332 return this.params != null && !this.params.isEmpty(); 5333 } 5334 5335 /** 5336 * @param value {@link #params} (Path plus parameters after [type]. Used to set 5337 * parts of the request URL explicitly.). This is the underlying 5338 * object with id, value and extensions. The accessor "getParams" 5339 * gives direct access to the value 5340 */ 5341 public SetupActionOperationComponent setParamsElement(StringType value) { 5342 this.params = value; 5343 return this; 5344 } 5345 5346 /** 5347 * @return Path plus parameters after [type]. Used to set parts of the request 5348 * URL explicitly. 5349 */ 5350 public String getParams() { 5351 return this.params == null ? null : this.params.getValue(); 5352 } 5353 5354 /** 5355 * @param value Path plus parameters after [type]. Used to set parts of the 5356 * request URL explicitly. 5357 */ 5358 public SetupActionOperationComponent setParams(String value) { 5359 if (Utilities.noString(value)) 5360 this.params = null; 5361 else { 5362 if (this.params == null) 5363 this.params = new StringType(); 5364 this.params.setValue(value); 5365 } 5366 return this; 5367 } 5368 5369 /** 5370 * @return {@link #requestHeader} (Header elements would be used to set HTTP 5371 * headers.) 5372 */ 5373 public List<SetupActionOperationRequestHeaderComponent> getRequestHeader() { 5374 if (this.requestHeader == null) 5375 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5376 return this.requestHeader; 5377 } 5378 5379 /** 5380 * @return Returns a reference to <code>this</code> for easy method chaining 5381 */ 5382 public SetupActionOperationComponent setRequestHeader( 5383 List<SetupActionOperationRequestHeaderComponent> theRequestHeader) { 5384 this.requestHeader = theRequestHeader; 5385 return this; 5386 } 5387 5388 public boolean hasRequestHeader() { 5389 if (this.requestHeader == null) 5390 return false; 5391 for (SetupActionOperationRequestHeaderComponent item : this.requestHeader) 5392 if (!item.isEmpty()) 5393 return true; 5394 return false; 5395 } 5396 5397 public SetupActionOperationRequestHeaderComponent addRequestHeader() { // 3 5398 SetupActionOperationRequestHeaderComponent t = new SetupActionOperationRequestHeaderComponent(); 5399 if (this.requestHeader == null) 5400 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5401 this.requestHeader.add(t); 5402 return t; 5403 } 5404 5405 public SetupActionOperationComponent addRequestHeader(SetupActionOperationRequestHeaderComponent t) { // 3 5406 if (t == null) 5407 return this; 5408 if (this.requestHeader == null) 5409 this.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 5410 this.requestHeader.add(t); 5411 return this; 5412 } 5413 5414 /** 5415 * @return The first repetition of repeating field {@link #requestHeader}, 5416 * creating it if it does not already exist 5417 */ 5418 public SetupActionOperationRequestHeaderComponent getRequestHeaderFirstRep() { 5419 if (getRequestHeader().isEmpty()) { 5420 addRequestHeader(); 5421 } 5422 return getRequestHeader().get(0); 5423 } 5424 5425 /** 5426 * @return {@link #requestId} (The fixture id (maybe new) to map to the 5427 * request.). This is the underlying object with id, value and 5428 * extensions. The accessor "getRequestId" gives direct access to the 5429 * value 5430 */ 5431 public IdType getRequestIdElement() { 5432 if (this.requestId == null) 5433 if (Configuration.errorOnAutoCreate()) 5434 throw new Error("Attempt to auto-create SetupActionOperationComponent.requestId"); 5435 else if (Configuration.doAutoCreate()) 5436 this.requestId = new IdType(); // bb 5437 return this.requestId; 5438 } 5439 5440 public boolean hasRequestIdElement() { 5441 return this.requestId != null && !this.requestId.isEmpty(); 5442 } 5443 5444 public boolean hasRequestId() { 5445 return this.requestId != null && !this.requestId.isEmpty(); 5446 } 5447 5448 /** 5449 * @param value {@link #requestId} (The fixture id (maybe new) to map to the 5450 * request.). This is the underlying object with id, value and 5451 * extensions. The accessor "getRequestId" gives direct access to 5452 * the value 5453 */ 5454 public SetupActionOperationComponent setRequestIdElement(IdType value) { 5455 this.requestId = value; 5456 return this; 5457 } 5458 5459 /** 5460 * @return The fixture id (maybe new) to map to the request. 5461 */ 5462 public String getRequestId() { 5463 return this.requestId == null ? null : this.requestId.getValue(); 5464 } 5465 5466 /** 5467 * @param value The fixture id (maybe new) to map to the request. 5468 */ 5469 public SetupActionOperationComponent setRequestId(String value) { 5470 if (Utilities.noString(value)) 5471 this.requestId = null; 5472 else { 5473 if (this.requestId == null) 5474 this.requestId = new IdType(); 5475 this.requestId.setValue(value); 5476 } 5477 return this; 5478 } 5479 5480 /** 5481 * @return {@link #responseId} (The fixture id (maybe new) to map to the 5482 * response.). This is the underlying object with id, value and 5483 * extensions. The accessor "getResponseId" gives direct access to the 5484 * value 5485 */ 5486 public IdType getResponseIdElement() { 5487 if (this.responseId == null) 5488 if (Configuration.errorOnAutoCreate()) 5489 throw new Error("Attempt to auto-create SetupActionOperationComponent.responseId"); 5490 else if (Configuration.doAutoCreate()) 5491 this.responseId = new IdType(); // bb 5492 return this.responseId; 5493 } 5494 5495 public boolean hasResponseIdElement() { 5496 return this.responseId != null && !this.responseId.isEmpty(); 5497 } 5498 5499 public boolean hasResponseId() { 5500 return this.responseId != null && !this.responseId.isEmpty(); 5501 } 5502 5503 /** 5504 * @param value {@link #responseId} (The fixture id (maybe new) to map to the 5505 * response.). This is the underlying object with id, value and 5506 * extensions. The accessor "getResponseId" gives direct access to 5507 * the value 5508 */ 5509 public SetupActionOperationComponent setResponseIdElement(IdType value) { 5510 this.responseId = value; 5511 return this; 5512 } 5513 5514 /** 5515 * @return The fixture id (maybe new) to map to the response. 5516 */ 5517 public String getResponseId() { 5518 return this.responseId == null ? null : this.responseId.getValue(); 5519 } 5520 5521 /** 5522 * @param value The fixture id (maybe new) to map to the response. 5523 */ 5524 public SetupActionOperationComponent setResponseId(String value) { 5525 if (Utilities.noString(value)) 5526 this.responseId = null; 5527 else { 5528 if (this.responseId == null) 5529 this.responseId = new IdType(); 5530 this.responseId.setValue(value); 5531 } 5532 return this; 5533 } 5534 5535 /** 5536 * @return {@link #sourceId} (The id of the fixture used as the body of a PUT or 5537 * POST request.). This is the underlying object with id, value and 5538 * extensions. The accessor "getSourceId" gives direct access to the 5539 * value 5540 */ 5541 public IdType getSourceIdElement() { 5542 if (this.sourceId == null) 5543 if (Configuration.errorOnAutoCreate()) 5544 throw new Error("Attempt to auto-create SetupActionOperationComponent.sourceId"); 5545 else if (Configuration.doAutoCreate()) 5546 this.sourceId = new IdType(); // bb 5547 return this.sourceId; 5548 } 5549 5550 public boolean hasSourceIdElement() { 5551 return this.sourceId != null && !this.sourceId.isEmpty(); 5552 } 5553 5554 public boolean hasSourceId() { 5555 return this.sourceId != null && !this.sourceId.isEmpty(); 5556 } 5557 5558 /** 5559 * @param value {@link #sourceId} (The id of the fixture used as the body of a 5560 * PUT or POST request.). This is the underlying object with id, 5561 * value and extensions. The accessor "getSourceId" gives direct 5562 * access to the value 5563 */ 5564 public SetupActionOperationComponent setSourceIdElement(IdType value) { 5565 this.sourceId = value; 5566 return this; 5567 } 5568 5569 /** 5570 * @return The id of the fixture used as the body of a PUT or POST request. 5571 */ 5572 public String getSourceId() { 5573 return this.sourceId == null ? null : this.sourceId.getValue(); 5574 } 5575 5576 /** 5577 * @param value The id of the fixture used as the body of a PUT or POST request. 5578 */ 5579 public SetupActionOperationComponent setSourceId(String value) { 5580 if (Utilities.noString(value)) 5581 this.sourceId = null; 5582 else { 5583 if (this.sourceId == null) 5584 this.sourceId = new IdType(); 5585 this.sourceId.setValue(value); 5586 } 5587 return this; 5588 } 5589 5590 /** 5591 * @return {@link #targetId} (Id of fixture used for extracting the [id], 5592 * [type], and [vid] for GET requests.). This is the underlying object 5593 * with id, value and extensions. The accessor "getTargetId" gives 5594 * direct access to the value 5595 */ 5596 public IdType getTargetIdElement() { 5597 if (this.targetId == null) 5598 if (Configuration.errorOnAutoCreate()) 5599 throw new Error("Attempt to auto-create SetupActionOperationComponent.targetId"); 5600 else if (Configuration.doAutoCreate()) 5601 this.targetId = new IdType(); // bb 5602 return this.targetId; 5603 } 5604 5605 public boolean hasTargetIdElement() { 5606 return this.targetId != null && !this.targetId.isEmpty(); 5607 } 5608 5609 public boolean hasTargetId() { 5610 return this.targetId != null && !this.targetId.isEmpty(); 5611 } 5612 5613 /** 5614 * @param value {@link #targetId} (Id of fixture used for extracting the [id], 5615 * [type], and [vid] for GET requests.). This is the underlying 5616 * object with id, value and extensions. The accessor "getTargetId" 5617 * gives direct access to the value 5618 */ 5619 public SetupActionOperationComponent setTargetIdElement(IdType value) { 5620 this.targetId = value; 5621 return this; 5622 } 5623 5624 /** 5625 * @return Id of fixture used for extracting the [id], [type], and [vid] for GET 5626 * requests. 5627 */ 5628 public String getTargetId() { 5629 return this.targetId == null ? null : this.targetId.getValue(); 5630 } 5631 5632 /** 5633 * @param value Id of fixture used for extracting the [id], [type], and [vid] 5634 * for GET requests. 5635 */ 5636 public SetupActionOperationComponent setTargetId(String value) { 5637 if (Utilities.noString(value)) 5638 this.targetId = null; 5639 else { 5640 if (this.targetId == null) 5641 this.targetId = new IdType(); 5642 this.targetId.setValue(value); 5643 } 5644 return this; 5645 } 5646 5647 /** 5648 * @return {@link #url} (Complete request URL.). This is the underlying object 5649 * with id, value and extensions. The accessor "getUrl" gives direct 5650 * access to the value 5651 */ 5652 public StringType getUrlElement() { 5653 if (this.url == null) 5654 if (Configuration.errorOnAutoCreate()) 5655 throw new Error("Attempt to auto-create SetupActionOperationComponent.url"); 5656 else if (Configuration.doAutoCreate()) 5657 this.url = new StringType(); // bb 5658 return this.url; 5659 } 5660 5661 public boolean hasUrlElement() { 5662 return this.url != null && !this.url.isEmpty(); 5663 } 5664 5665 public boolean hasUrl() { 5666 return this.url != null && !this.url.isEmpty(); 5667 } 5668 5669 /** 5670 * @param value {@link #url} (Complete request URL.). This is the underlying 5671 * object with id, value and extensions. The accessor "getUrl" 5672 * gives direct access to the value 5673 */ 5674 public SetupActionOperationComponent setUrlElement(StringType value) { 5675 this.url = value; 5676 return this; 5677 } 5678 5679 /** 5680 * @return Complete request URL. 5681 */ 5682 public String getUrl() { 5683 return this.url == null ? null : this.url.getValue(); 5684 } 5685 5686 /** 5687 * @param value Complete request URL. 5688 */ 5689 public SetupActionOperationComponent setUrl(String value) { 5690 if (Utilities.noString(value)) 5691 this.url = null; 5692 else { 5693 if (this.url == null) 5694 this.url = new StringType(); 5695 this.url.setValue(value); 5696 } 5697 return this; 5698 } 5699 5700 protected void listChildren(List<Property> children) { 5701 super.listChildren(children); 5702 children.add(new Property("type", "Coding", "Server interaction or operation type.", 0, 1, type)); 5703 children.add(new Property("resource", "code", 5704 "The type of the resource. See http://build.fhir.org/resourcelist.html.", 0, 1, resource)); 5705 children.add(new Property("label", "string", 5706 "The label would be used for tracking/logging purposes by test engines.", 0, 1, label)); 5707 children.add(new Property("description", "string", 5708 "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description)); 5709 children.add(new Property("accept", "code", "The mime-type to use for RESTful operation in the 'Accept' header.", 5710 0, 1, accept)); 5711 children.add(new Property("contentType", "code", 5712 "The mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 1, contentType)); 5713 children.add(new Property("destination", "integer", 5714 "The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.", 5715 0, 1, destination)); 5716 children.add(new Property("encodeRequestUrl", "boolean", 5717 "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.", 5718 0, 1, encodeRequestUrl)); 5719 children.add(new Property("method", "code", 5720 "The HTTP method the test engine MUST use for this operation regardless of any other operation details.", 0, 5721 1, method)); 5722 children.add(new Property("origin", "integer", 5723 "The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.", 5724 0, 1, origin)); 5725 children.add(new Property("params", "string", 5726 "Path plus parameters after [type]. Used to set parts of the request URL explicitly.", 0, 1, params)); 5727 children.add(new Property("requestHeader", "", "Header elements would be used to set HTTP headers.", 0, 5728 java.lang.Integer.MAX_VALUE, requestHeader)); 5729 children 5730 .add(new Property("requestId", "id", "The fixture id (maybe new) to map to the request.", 0, 1, requestId)); 5731 children.add( 5732 new Property("responseId", "id", "The fixture id (maybe new) to map to the response.", 0, 1, responseId)); 5733 children.add(new Property("sourceId", "id", "The id of the fixture used as the body of a PUT or POST request.", 0, 5734 1, sourceId)); 5735 children.add(new Property("targetId", "id", 5736 "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.", 0, 1, targetId)); 5737 children.add(new Property("url", "string", "Complete request URL.", 0, 1, url)); 5738 } 5739 5740 @Override 5741 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5742 switch (_hash) { 5743 case 3575610: 5744 /* type */ return new Property("type", "Coding", "Server interaction or operation type.", 0, 1, type); 5745 case -341064690: 5746 /* resource */ return new Property("resource", "code", 5747 "The type of the resource. See http://build.fhir.org/resourcelist.html.", 0, 1, resource); 5748 case 102727412: 5749 /* label */ return new Property("label", "string", 5750 "The label would be used for tracking/logging purposes by test engines.", 0, 1, label); 5751 case -1724546052: 5752 /* description */ return new Property("description", "string", 5753 "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description); 5754 case -1423461112: 5755 /* accept */ return new Property("accept", "code", 5756 "The mime-type to use for RESTful operation in the 'Accept' header.", 0, 1, accept); 5757 case -389131437: 5758 /* contentType */ return new Property("contentType", "code", 5759 "The mime-type to use for RESTful operation in the 'Content-Type' header.", 0, 1, contentType); 5760 case -1429847026: 5761 /* destination */ return new Property("destination", "integer", 5762 "The server where the request message is destined for. Must be one of the server numbers listed in TestScript.destination section.", 5763 0, 1, destination); 5764 case -1760554218: 5765 /* encodeRequestUrl */ return new Property("encodeRequestUrl", "boolean", 5766 "Whether or not to implicitly send the request url in encoded format. The default is true to match the standard RESTful client behavior. Set to false when communicating with a server that does not support encoded url paths.", 5767 0, 1, encodeRequestUrl); 5768 case -1077554975: 5769 /* method */ return new Property("method", "code", 5770 "The HTTP method the test engine MUST use for this operation regardless of any other operation details.", 0, 5771 1, method); 5772 case -1008619738: 5773 /* origin */ return new Property("origin", "integer", 5774 "The server where the request message originates from. Must be one of the server numbers listed in TestScript.origin section.", 5775 0, 1, origin); 5776 case -995427962: 5777 /* params */ return new Property("params", "string", 5778 "Path plus parameters after [type]. Used to set parts of the request URL explicitly.", 0, 1, params); 5779 case 1074158076: 5780 /* requestHeader */ return new Property("requestHeader", "", 5781 "Header elements would be used to set HTTP headers.", 0, java.lang.Integer.MAX_VALUE, requestHeader); 5782 case 693933066: 5783 /* requestId */ return new Property("requestId", "id", "The fixture id (maybe new) to map to the request.", 0, 5784 1, requestId); 5785 case -633138884: 5786 /* responseId */ return new Property("responseId", "id", "The fixture id (maybe new) to map to the response.", 5787 0, 1, responseId); 5788 case 1746327190: 5789 /* sourceId */ return new Property("sourceId", "id", 5790 "The id of the fixture used as the body of a PUT or POST request.", 0, 1, sourceId); 5791 case -441951604: 5792 /* targetId */ return new Property("targetId", "id", 5793 "Id of fixture used for extracting the [id], [type], and [vid] for GET requests.", 0, 1, targetId); 5794 case 116079: 5795 /* url */ return new Property("url", "string", "Complete request URL.", 0, 1, url); 5796 default: 5797 return super.getNamedProperty(_hash, _name, _checkValid); 5798 } 5799 5800 } 5801 5802 @Override 5803 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5804 switch (hash) { 5805 case 3575610: 5806 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Coding 5807 case -341064690: 5808 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // CodeType 5809 case 102727412: 5810 /* label */ return this.label == null ? new Base[0] : new Base[] { this.label }; // StringType 5811 case -1724546052: 5812 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 5813 case -1423461112: 5814 /* accept */ return this.accept == null ? new Base[0] : new Base[] { this.accept }; // CodeType 5815 case -389131437: 5816 /* contentType */ return this.contentType == null ? new Base[0] : new Base[] { this.contentType }; // CodeType 5817 case -1429847026: 5818 /* destination */ return this.destination == null ? new Base[0] : new Base[] { this.destination }; // IntegerType 5819 case -1760554218: 5820 /* encodeRequestUrl */ return this.encodeRequestUrl == null ? new Base[0] 5821 : new Base[] { this.encodeRequestUrl }; // BooleanType 5822 case -1077554975: 5823 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // Enumeration<TestScriptRequestMethodCode> 5824 case -1008619738: 5825 /* origin */ return this.origin == null ? new Base[0] : new Base[] { this.origin }; // IntegerType 5826 case -995427962: 5827 /* params */ return this.params == null ? new Base[0] : new Base[] { this.params }; // StringType 5828 case 1074158076: 5829 /* requestHeader */ return this.requestHeader == null ? new Base[0] 5830 : this.requestHeader.toArray(new Base[this.requestHeader.size()]); // SetupActionOperationRequestHeaderComponent 5831 case 693933066: 5832 /* requestId */ return this.requestId == null ? new Base[0] : new Base[] { this.requestId }; // IdType 5833 case -633138884: 5834 /* responseId */ return this.responseId == null ? new Base[0] : new Base[] { this.responseId }; // IdType 5835 case 1746327190: 5836 /* sourceId */ return this.sourceId == null ? new Base[0] : new Base[] { this.sourceId }; // IdType 5837 case -441951604: 5838 /* targetId */ return this.targetId == null ? new Base[0] : new Base[] { this.targetId }; // IdType 5839 case 116079: 5840 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // StringType 5841 default: 5842 return super.getProperty(hash, name, checkValid); 5843 } 5844 5845 } 5846 5847 @Override 5848 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5849 switch (hash) { 5850 case 3575610: // type 5851 this.type = castToCoding(value); // Coding 5852 return value; 5853 case -341064690: // resource 5854 this.resource = castToCode(value); // CodeType 5855 return value; 5856 case 102727412: // label 5857 this.label = castToString(value); // StringType 5858 return value; 5859 case -1724546052: // description 5860 this.description = castToString(value); // StringType 5861 return value; 5862 case -1423461112: // accept 5863 this.accept = castToCode(value); // CodeType 5864 return value; 5865 case -389131437: // contentType 5866 this.contentType = castToCode(value); // CodeType 5867 return value; 5868 case -1429847026: // destination 5869 this.destination = castToInteger(value); // IntegerType 5870 return value; 5871 case -1760554218: // encodeRequestUrl 5872 this.encodeRequestUrl = castToBoolean(value); // BooleanType 5873 return value; 5874 case -1077554975: // method 5875 value = new TestScriptRequestMethodCodeEnumFactory().fromType(castToCode(value)); 5876 this.method = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 5877 return value; 5878 case -1008619738: // origin 5879 this.origin = castToInteger(value); // IntegerType 5880 return value; 5881 case -995427962: // params 5882 this.params = castToString(value); // StringType 5883 return value; 5884 case 1074158076: // requestHeader 5885 this.getRequestHeader().add((SetupActionOperationRequestHeaderComponent) value); // SetupActionOperationRequestHeaderComponent 5886 return value; 5887 case 693933066: // requestId 5888 this.requestId = castToId(value); // IdType 5889 return value; 5890 case -633138884: // responseId 5891 this.responseId = castToId(value); // IdType 5892 return value; 5893 case 1746327190: // sourceId 5894 this.sourceId = castToId(value); // IdType 5895 return value; 5896 case -441951604: // targetId 5897 this.targetId = castToId(value); // IdType 5898 return value; 5899 case 116079: // url 5900 this.url = castToString(value); // StringType 5901 return value; 5902 default: 5903 return super.setProperty(hash, name, value); 5904 } 5905 5906 } 5907 5908 @Override 5909 public Base setProperty(String name, Base value) throws FHIRException { 5910 if (name.equals("type")) { 5911 this.type = castToCoding(value); // Coding 5912 } else if (name.equals("resource")) { 5913 this.resource = castToCode(value); // CodeType 5914 } else if (name.equals("label")) { 5915 this.label = castToString(value); // StringType 5916 } else if (name.equals("description")) { 5917 this.description = castToString(value); // StringType 5918 } else if (name.equals("accept")) { 5919 this.accept = castToCode(value); // CodeType 5920 } else if (name.equals("contentType")) { 5921 this.contentType = castToCode(value); // CodeType 5922 } else if (name.equals("destination")) { 5923 this.destination = castToInteger(value); // IntegerType 5924 } else if (name.equals("encodeRequestUrl")) { 5925 this.encodeRequestUrl = castToBoolean(value); // BooleanType 5926 } else if (name.equals("method")) { 5927 value = new TestScriptRequestMethodCodeEnumFactory().fromType(castToCode(value)); 5928 this.method = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 5929 } else if (name.equals("origin")) { 5930 this.origin = castToInteger(value); // IntegerType 5931 } else if (name.equals("params")) { 5932 this.params = castToString(value); // StringType 5933 } else if (name.equals("requestHeader")) { 5934 this.getRequestHeader().add((SetupActionOperationRequestHeaderComponent) value); 5935 } else if (name.equals("requestId")) { 5936 this.requestId = castToId(value); // IdType 5937 } else if (name.equals("responseId")) { 5938 this.responseId = castToId(value); // IdType 5939 } else if (name.equals("sourceId")) { 5940 this.sourceId = castToId(value); // IdType 5941 } else if (name.equals("targetId")) { 5942 this.targetId = castToId(value); // IdType 5943 } else if (name.equals("url")) { 5944 this.url = castToString(value); // StringType 5945 } else 5946 return super.setProperty(name, value); 5947 return value; 5948 } 5949 5950 @Override 5951 public void removeChild(String name, Base value) throws FHIRException { 5952 if (name.equals("type")) { 5953 this.type = null; 5954 } else if (name.equals("resource")) { 5955 this.resource = null; 5956 } else if (name.equals("label")) { 5957 this.label = null; 5958 } else if (name.equals("description")) { 5959 this.description = null; 5960 } else if (name.equals("accept")) { 5961 this.accept = null; 5962 } else if (name.equals("contentType")) { 5963 this.contentType = null; 5964 } else if (name.equals("destination")) { 5965 this.destination = null; 5966 } else if (name.equals("encodeRequestUrl")) { 5967 this.encodeRequestUrl = null; 5968 } else if (name.equals("method")) { 5969 this.method = null; 5970 } else if (name.equals("origin")) { 5971 this.origin = null; 5972 } else if (name.equals("params")) { 5973 this.params = null; 5974 } else if (name.equals("requestHeader")) { 5975 this.getRequestHeader().remove((SetupActionOperationRequestHeaderComponent) value); 5976 } else if (name.equals("requestId")) { 5977 this.requestId = null; 5978 } else if (name.equals("responseId")) { 5979 this.responseId = null; 5980 } else if (name.equals("sourceId")) { 5981 this.sourceId = null; 5982 } else if (name.equals("targetId")) { 5983 this.targetId = null; 5984 } else if (name.equals("url")) { 5985 this.url = null; 5986 } else 5987 super.removeChild(name, value); 5988 5989 } 5990 5991 @Override 5992 public Base makeProperty(int hash, String name) throws FHIRException { 5993 switch (hash) { 5994 case 3575610: 5995 return getType(); 5996 case -341064690: 5997 return getResourceElement(); 5998 case 102727412: 5999 return getLabelElement(); 6000 case -1724546052: 6001 return getDescriptionElement(); 6002 case -1423461112: 6003 return getAcceptElement(); 6004 case -389131437: 6005 return getContentTypeElement(); 6006 case -1429847026: 6007 return getDestinationElement(); 6008 case -1760554218: 6009 return getEncodeRequestUrlElement(); 6010 case -1077554975: 6011 return getMethodElement(); 6012 case -1008619738: 6013 return getOriginElement(); 6014 case -995427962: 6015 return getParamsElement(); 6016 case 1074158076: 6017 return addRequestHeader(); 6018 case 693933066: 6019 return getRequestIdElement(); 6020 case -633138884: 6021 return getResponseIdElement(); 6022 case 1746327190: 6023 return getSourceIdElement(); 6024 case -441951604: 6025 return getTargetIdElement(); 6026 case 116079: 6027 return getUrlElement(); 6028 default: 6029 return super.makeProperty(hash, name); 6030 } 6031 6032 } 6033 6034 @Override 6035 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6036 switch (hash) { 6037 case 3575610: 6038 /* type */ return new String[] { "Coding" }; 6039 case -341064690: 6040 /* resource */ return new String[] { "code" }; 6041 case 102727412: 6042 /* label */ return new String[] { "string" }; 6043 case -1724546052: 6044 /* description */ return new String[] { "string" }; 6045 case -1423461112: 6046 /* accept */ return new String[] { "code" }; 6047 case -389131437: 6048 /* contentType */ return new String[] { "code" }; 6049 case -1429847026: 6050 /* destination */ return new String[] { "integer" }; 6051 case -1760554218: 6052 /* encodeRequestUrl */ return new String[] { "boolean" }; 6053 case -1077554975: 6054 /* method */ return new String[] { "code" }; 6055 case -1008619738: 6056 /* origin */ return new String[] { "integer" }; 6057 case -995427962: 6058 /* params */ return new String[] { "string" }; 6059 case 1074158076: 6060 /* requestHeader */ return new String[] {}; 6061 case 693933066: 6062 /* requestId */ return new String[] { "id" }; 6063 case -633138884: 6064 /* responseId */ return new String[] { "id" }; 6065 case 1746327190: 6066 /* sourceId */ return new String[] { "id" }; 6067 case -441951604: 6068 /* targetId */ return new String[] { "id" }; 6069 case 116079: 6070 /* url */ return new String[] { "string" }; 6071 default: 6072 return super.getTypesForProperty(hash, name); 6073 } 6074 6075 } 6076 6077 @Override 6078 public Base addChild(String name) throws FHIRException { 6079 if (name.equals("type")) { 6080 this.type = new Coding(); 6081 return this.type; 6082 } else if (name.equals("resource")) { 6083 throw new FHIRException("Cannot call addChild on a singleton property TestScript.resource"); 6084 } else if (name.equals("label")) { 6085 throw new FHIRException("Cannot call addChild on a singleton property TestScript.label"); 6086 } else if (name.equals("description")) { 6087 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 6088 } else if (name.equals("accept")) { 6089 throw new FHIRException("Cannot call addChild on a singleton property TestScript.accept"); 6090 } else if (name.equals("contentType")) { 6091 throw new FHIRException("Cannot call addChild on a singleton property TestScript.contentType"); 6092 } else if (name.equals("destination")) { 6093 throw new FHIRException("Cannot call addChild on a singleton property TestScript.destination"); 6094 } else if (name.equals("encodeRequestUrl")) { 6095 throw new FHIRException("Cannot call addChild on a singleton property TestScript.encodeRequestUrl"); 6096 } else if (name.equals("method")) { 6097 throw new FHIRException("Cannot call addChild on a singleton property TestScript.method"); 6098 } else if (name.equals("origin")) { 6099 throw new FHIRException("Cannot call addChild on a singleton property TestScript.origin"); 6100 } else if (name.equals("params")) { 6101 throw new FHIRException("Cannot call addChild on a singleton property TestScript.params"); 6102 } else if (name.equals("requestHeader")) { 6103 return addRequestHeader(); 6104 } else if (name.equals("requestId")) { 6105 throw new FHIRException("Cannot call addChild on a singleton property TestScript.requestId"); 6106 } else if (name.equals("responseId")) { 6107 throw new FHIRException("Cannot call addChild on a singleton property TestScript.responseId"); 6108 } else if (name.equals("sourceId")) { 6109 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 6110 } else if (name.equals("targetId")) { 6111 throw new FHIRException("Cannot call addChild on a singleton property TestScript.targetId"); 6112 } else if (name.equals("url")) { 6113 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 6114 } else 6115 return super.addChild(name); 6116 } 6117 6118 public SetupActionOperationComponent copy() { 6119 SetupActionOperationComponent dst = new SetupActionOperationComponent(); 6120 copyValues(dst); 6121 return dst; 6122 } 6123 6124 public void copyValues(SetupActionOperationComponent dst) { 6125 super.copyValues(dst); 6126 dst.type = type == null ? null : type.copy(); 6127 dst.resource = resource == null ? null : resource.copy(); 6128 dst.label = label == null ? null : label.copy(); 6129 dst.description = description == null ? null : description.copy(); 6130 dst.accept = accept == null ? null : accept.copy(); 6131 dst.contentType = contentType == null ? null : contentType.copy(); 6132 dst.destination = destination == null ? null : destination.copy(); 6133 dst.encodeRequestUrl = encodeRequestUrl == null ? null : encodeRequestUrl.copy(); 6134 dst.method = method == null ? null : method.copy(); 6135 dst.origin = origin == null ? null : origin.copy(); 6136 dst.params = params == null ? null : params.copy(); 6137 if (requestHeader != null) { 6138 dst.requestHeader = new ArrayList<SetupActionOperationRequestHeaderComponent>(); 6139 for (SetupActionOperationRequestHeaderComponent i : requestHeader) 6140 dst.requestHeader.add(i.copy()); 6141 } 6142 ; 6143 dst.requestId = requestId == null ? null : requestId.copy(); 6144 dst.responseId = responseId == null ? null : responseId.copy(); 6145 dst.sourceId = sourceId == null ? null : sourceId.copy(); 6146 dst.targetId = targetId == null ? null : targetId.copy(); 6147 dst.url = url == null ? null : url.copy(); 6148 } 6149 6150 @Override 6151 public boolean equalsDeep(Base other_) { 6152 if (!super.equalsDeep(other_)) 6153 return false; 6154 if (!(other_ instanceof SetupActionOperationComponent)) 6155 return false; 6156 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 6157 return compareDeep(type, o.type, true) && compareDeep(resource, o.resource, true) 6158 && compareDeep(label, o.label, true) && compareDeep(description, o.description, true) 6159 && compareDeep(accept, o.accept, true) && compareDeep(contentType, o.contentType, true) 6160 && compareDeep(destination, o.destination, true) && compareDeep(encodeRequestUrl, o.encodeRequestUrl, true) 6161 && compareDeep(method, o.method, true) && compareDeep(origin, o.origin, true) 6162 && compareDeep(params, o.params, true) && compareDeep(requestHeader, o.requestHeader, true) 6163 && compareDeep(requestId, o.requestId, true) && compareDeep(responseId, o.responseId, true) 6164 && compareDeep(sourceId, o.sourceId, true) && compareDeep(targetId, o.targetId, true) 6165 && compareDeep(url, o.url, true); 6166 } 6167 6168 @Override 6169 public boolean equalsShallow(Base other_) { 6170 if (!super.equalsShallow(other_)) 6171 return false; 6172 if (!(other_ instanceof SetupActionOperationComponent)) 6173 return false; 6174 SetupActionOperationComponent o = (SetupActionOperationComponent) other_; 6175 return compareValues(resource, o.resource, true) && compareValues(label, o.label, true) 6176 && compareValues(description, o.description, true) && compareValues(accept, o.accept, true) 6177 && compareValues(contentType, o.contentType, true) && compareValues(destination, o.destination, true) 6178 && compareValues(encodeRequestUrl, o.encodeRequestUrl, true) && compareValues(method, o.method, true) 6179 && compareValues(origin, o.origin, true) && compareValues(params, o.params, true) 6180 && compareValues(requestId, o.requestId, true) && compareValues(responseId, o.responseId, true) 6181 && compareValues(sourceId, o.sourceId, true) && compareValues(targetId, o.targetId, true) 6182 && compareValues(url, o.url, true); 6183 } 6184 6185 public boolean isEmpty() { 6186 return super.isEmpty() 6187 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resource, label, description, accept, contentType, destination, 6188 encodeRequestUrl, method, origin, params, requestHeader, requestId, responseId, sourceId, targetId, url); 6189 } 6190 6191 public String fhirType() { 6192 return "TestScript.setup.action.operation"; 6193 6194 } 6195 6196 } 6197 6198 @Block() 6199 public static class SetupActionOperationRequestHeaderComponent extends BackboneElement 6200 implements IBaseBackboneElement { 6201 /** 6202 * The HTTP header field e.g. "Accept". 6203 */ 6204 @Child(name = "field", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6205 @Description(shortDefinition = "HTTP header field name", formalDefinition = "The HTTP header field e.g. \"Accept\".") 6206 protected StringType field; 6207 6208 /** 6209 * The value of the header e.g. "application/fhir+xml". 6210 */ 6211 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 6212 @Description(shortDefinition = "HTTP headerfield value", formalDefinition = "The value of the header e.g. \"application/fhir+xml\".") 6213 protected StringType value; 6214 6215 private static final long serialVersionUID = 274395337L; 6216 6217 /** 6218 * Constructor 6219 */ 6220 public SetupActionOperationRequestHeaderComponent() { 6221 super(); 6222 } 6223 6224 /** 6225 * Constructor 6226 */ 6227 public SetupActionOperationRequestHeaderComponent(StringType field, StringType value) { 6228 super(); 6229 this.field = field; 6230 this.value = value; 6231 } 6232 6233 /** 6234 * @return {@link #field} (The HTTP header field e.g. "Accept".). This is the 6235 * underlying object with id, value and extensions. The accessor 6236 * "getField" gives direct access to the value 6237 */ 6238 public StringType getFieldElement() { 6239 if (this.field == null) 6240 if (Configuration.errorOnAutoCreate()) 6241 throw new Error("Attempt to auto-create SetupActionOperationRequestHeaderComponent.field"); 6242 else if (Configuration.doAutoCreate()) 6243 this.field = new StringType(); // bb 6244 return this.field; 6245 } 6246 6247 public boolean hasFieldElement() { 6248 return this.field != null && !this.field.isEmpty(); 6249 } 6250 6251 public boolean hasField() { 6252 return this.field != null && !this.field.isEmpty(); 6253 } 6254 6255 /** 6256 * @param value {@link #field} (The HTTP header field e.g. "Accept".). This is 6257 * the underlying object with id, value and extensions. The 6258 * accessor "getField" gives direct access to the value 6259 */ 6260 public SetupActionOperationRequestHeaderComponent setFieldElement(StringType value) { 6261 this.field = value; 6262 return this; 6263 } 6264 6265 /** 6266 * @return The HTTP header field e.g. "Accept". 6267 */ 6268 public String getField() { 6269 return this.field == null ? null : this.field.getValue(); 6270 } 6271 6272 /** 6273 * @param value The HTTP header field e.g. "Accept". 6274 */ 6275 public SetupActionOperationRequestHeaderComponent setField(String value) { 6276 if (this.field == null) 6277 this.field = new StringType(); 6278 this.field.setValue(value); 6279 return this; 6280 } 6281 6282 /** 6283 * @return {@link #value} (The value of the header e.g. 6284 * "application/fhir+xml".). This is the underlying object with id, 6285 * value and extensions. The accessor "getValue" gives direct access to 6286 * the value 6287 */ 6288 public StringType getValueElement() { 6289 if (this.value == null) 6290 if (Configuration.errorOnAutoCreate()) 6291 throw new Error("Attempt to auto-create SetupActionOperationRequestHeaderComponent.value"); 6292 else if (Configuration.doAutoCreate()) 6293 this.value = new StringType(); // bb 6294 return this.value; 6295 } 6296 6297 public boolean hasValueElement() { 6298 return this.value != null && !this.value.isEmpty(); 6299 } 6300 6301 public boolean hasValue() { 6302 return this.value != null && !this.value.isEmpty(); 6303 } 6304 6305 /** 6306 * @param value {@link #value} (The value of the header e.g. 6307 * "application/fhir+xml".). This is the underlying object with id, 6308 * value and extensions. The accessor "getValue" gives direct 6309 * access to the value 6310 */ 6311 public SetupActionOperationRequestHeaderComponent setValueElement(StringType value) { 6312 this.value = value; 6313 return this; 6314 } 6315 6316 /** 6317 * @return The value of the header e.g. "application/fhir+xml". 6318 */ 6319 public String getValue() { 6320 return this.value == null ? null : this.value.getValue(); 6321 } 6322 6323 /** 6324 * @param value The value of the header e.g. "application/fhir+xml". 6325 */ 6326 public SetupActionOperationRequestHeaderComponent setValue(String value) { 6327 if (this.value == null) 6328 this.value = new StringType(); 6329 this.value.setValue(value); 6330 return this; 6331 } 6332 6333 protected void listChildren(List<Property> children) { 6334 super.listChildren(children); 6335 children.add(new Property("field", "string", "The HTTP header field e.g. \"Accept\".", 0, 1, field)); 6336 children 6337 .add(new Property("value", "string", "The value of the header e.g. \"application/fhir+xml\".", 0, 1, value)); 6338 } 6339 6340 @Override 6341 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6342 switch (_hash) { 6343 case 97427706: 6344 /* field */ return new Property("field", "string", "The HTTP header field e.g. \"Accept\".", 0, 1, field); 6345 case 111972721: 6346 /* value */ return new Property("value", "string", "The value of the header e.g. \"application/fhir+xml\".", 0, 6347 1, value); 6348 default: 6349 return super.getNamedProperty(_hash, _name, _checkValid); 6350 } 6351 6352 } 6353 6354 @Override 6355 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6356 switch (hash) { 6357 case 97427706: 6358 /* field */ return this.field == null ? new Base[0] : new Base[] { this.field }; // StringType 6359 case 111972721: 6360 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 6361 default: 6362 return super.getProperty(hash, name, checkValid); 6363 } 6364 6365 } 6366 6367 @Override 6368 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6369 switch (hash) { 6370 case 97427706: // field 6371 this.field = castToString(value); // StringType 6372 return value; 6373 case 111972721: // value 6374 this.value = castToString(value); // StringType 6375 return value; 6376 default: 6377 return super.setProperty(hash, name, value); 6378 } 6379 6380 } 6381 6382 @Override 6383 public Base setProperty(String name, Base value) throws FHIRException { 6384 if (name.equals("field")) { 6385 this.field = castToString(value); // StringType 6386 } else if (name.equals("value")) { 6387 this.value = castToString(value); // StringType 6388 } else 6389 return super.setProperty(name, value); 6390 return value; 6391 } 6392 6393 @Override 6394 public void removeChild(String name, Base value) throws FHIRException { 6395 if (name.equals("field")) { 6396 this.field = null; 6397 } else if (name.equals("value")) { 6398 this.value = null; 6399 } else 6400 super.removeChild(name, value); 6401 6402 } 6403 6404 @Override 6405 public Base makeProperty(int hash, String name) throws FHIRException { 6406 switch (hash) { 6407 case 97427706: 6408 return getFieldElement(); 6409 case 111972721: 6410 return getValueElement(); 6411 default: 6412 return super.makeProperty(hash, name); 6413 } 6414 6415 } 6416 6417 @Override 6418 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6419 switch (hash) { 6420 case 97427706: 6421 /* field */ return new String[] { "string" }; 6422 case 111972721: 6423 /* value */ return new String[] { "string" }; 6424 default: 6425 return super.getTypesForProperty(hash, name); 6426 } 6427 6428 } 6429 6430 @Override 6431 public Base addChild(String name) throws FHIRException { 6432 if (name.equals("field")) { 6433 throw new FHIRException("Cannot call addChild on a singleton property TestScript.field"); 6434 } else if (name.equals("value")) { 6435 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 6436 } else 6437 return super.addChild(name); 6438 } 6439 6440 public SetupActionOperationRequestHeaderComponent copy() { 6441 SetupActionOperationRequestHeaderComponent dst = new SetupActionOperationRequestHeaderComponent(); 6442 copyValues(dst); 6443 return dst; 6444 } 6445 6446 public void copyValues(SetupActionOperationRequestHeaderComponent dst) { 6447 super.copyValues(dst); 6448 dst.field = field == null ? null : field.copy(); 6449 dst.value = value == null ? null : value.copy(); 6450 } 6451 6452 @Override 6453 public boolean equalsDeep(Base other_) { 6454 if (!super.equalsDeep(other_)) 6455 return false; 6456 if (!(other_ instanceof SetupActionOperationRequestHeaderComponent)) 6457 return false; 6458 SetupActionOperationRequestHeaderComponent o = (SetupActionOperationRequestHeaderComponent) other_; 6459 return compareDeep(field, o.field, true) && compareDeep(value, o.value, true); 6460 } 6461 6462 @Override 6463 public boolean equalsShallow(Base other_) { 6464 if (!super.equalsShallow(other_)) 6465 return false; 6466 if (!(other_ instanceof SetupActionOperationRequestHeaderComponent)) 6467 return false; 6468 SetupActionOperationRequestHeaderComponent o = (SetupActionOperationRequestHeaderComponent) other_; 6469 return compareValues(field, o.field, true) && compareValues(value, o.value, true); 6470 } 6471 6472 public boolean isEmpty() { 6473 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(field, value); 6474 } 6475 6476 public String fhirType() { 6477 return "TestScript.setup.action.operation.requestHeader"; 6478 6479 } 6480 6481 } 6482 6483 @Block() 6484 public static class SetupActionAssertComponent extends BackboneElement implements IBaseBackboneElement { 6485 /** 6486 * The label would be used for tracking/logging purposes by test engines. 6487 */ 6488 @Child(name = "label", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6489 @Description(shortDefinition = "Tracking/logging assertion label", formalDefinition = "The label would be used for tracking/logging purposes by test engines.") 6490 protected StringType label; 6491 6492 /** 6493 * The description would be used by test engines for tracking and reporting 6494 * purposes. 6495 */ 6496 @Child(name = "description", type = { 6497 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6498 @Description(shortDefinition = "Tracking/reporting assertion description", formalDefinition = "The description would be used by test engines for tracking and reporting purposes.") 6499 protected StringType description; 6500 6501 /** 6502 * The direction to use for the assertion. 6503 */ 6504 @Child(name = "direction", type = { 6505 CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6506 @Description(shortDefinition = "response | request", formalDefinition = "The direction to use for the assertion.") 6507 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/assert-direction-codes") 6508 protected Enumeration<AssertionDirectionType> direction; 6509 6510 /** 6511 * Id of the source fixture used as the contents to be evaluated by either the 6512 * "source/expression" or "sourceId/path" definition. 6513 */ 6514 @Child(name = "compareToSourceId", type = { 6515 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6516 @Description(shortDefinition = "Id of the source fixture to be evaluated", formalDefinition = "Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition.") 6517 protected StringType compareToSourceId; 6518 6519 /** 6520 * The FHIRPath expression to evaluate against the source fixture. When 6521 * compareToSourceId is defined, either compareToSourceExpression or 6522 * compareToSourcePath must be defined, but not both. 6523 */ 6524 @Child(name = "compareToSourceExpression", type = { 6525 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6526 @Description(shortDefinition = "The FHIRPath expression to evaluate against the source fixture", formalDefinition = "The FHIRPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.") 6527 protected StringType compareToSourceExpression; 6528 6529 /** 6530 * XPath or JSONPath expression to evaluate against the source fixture. When 6531 * compareToSourceId is defined, either compareToSourceExpression or 6532 * compareToSourcePath must be defined, but not both. 6533 */ 6534 @Child(name = "compareToSourcePath", type = { 6535 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6536 @Description(shortDefinition = "XPath or JSONPath expression to evaluate against the source fixture", formalDefinition = "XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.") 6537 protected StringType compareToSourcePath; 6538 6539 /** 6540 * The mime-type contents to compare against the request or response message 6541 * 'Content-Type' header. 6542 */ 6543 @Child(name = "contentType", type = { 6544 CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6545 @Description(shortDefinition = "Mime type to compare against the 'Content-Type' header", formalDefinition = "The mime-type contents to compare against the request or response message 'Content-Type' header.") 6546 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 6547 protected CodeType contentType; 6548 6549 /** 6550 * The FHIRPath expression to be evaluated against the request or response 6551 * message contents - HTTP headers and payload. 6552 */ 6553 @Child(name = "expression", type = { 6554 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6555 @Description(shortDefinition = "The FHIRPath expression to be evaluated", formalDefinition = "The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload.") 6556 protected StringType expression; 6557 6558 /** 6559 * The HTTP header field name e.g. 'Location'. 6560 */ 6561 @Child(name = "headerField", type = { 6562 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 6563 @Description(shortDefinition = "HTTP header field name", formalDefinition = "The HTTP header field name e.g. 'Location'.") 6564 protected StringType headerField; 6565 6566 /** 6567 * The ID of a fixture. Asserts that the response contains at a minimum the 6568 * fixture specified by minimumId. 6569 */ 6570 @Child(name = "minimumId", type = { 6571 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 6572 @Description(shortDefinition = "Fixture Id of minimum content resource", formalDefinition = "The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.") 6573 protected StringType minimumId; 6574 6575 /** 6576 * Whether or not the test execution performs validation on the bundle 6577 * navigation links. 6578 */ 6579 @Child(name = "navigationLinks", type = { 6580 BooleanType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 6581 @Description(shortDefinition = "Perform validation on navigation links?", formalDefinition = "Whether or not the test execution performs validation on the bundle navigation links.") 6582 protected BooleanType navigationLinks; 6583 6584 /** 6585 * The operator type defines the conditional behavior of the assert. If not 6586 * defined, the default is equals. 6587 */ 6588 @Child(name = "operator", type = { 6589 CodeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 6590 @Description(shortDefinition = "equals | notEquals | in | notIn | greaterThan | lessThan | empty | notEmpty | contains | notContains | eval", formalDefinition = "The operator type defines the conditional behavior of the assert. If not defined, the default is equals.") 6591 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/assert-operator-codes") 6592 protected Enumeration<AssertionOperatorType> operator; 6593 6594 /** 6595 * The XPath or JSONPath expression to be evaluated against the fixture 6596 * representing the response received from server. 6597 */ 6598 @Child(name = "path", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 6599 @Description(shortDefinition = "XPath or JSONPath expression", formalDefinition = "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.") 6600 protected StringType path; 6601 6602 /** 6603 * The request method or HTTP operation code to compare against that used by the 6604 * client system under test. 6605 */ 6606 @Child(name = "requestMethod", type = { 6607 CodeType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 6608 @Description(shortDefinition = "delete | get | options | patch | post | put | head", formalDefinition = "The request method or HTTP operation code to compare against that used by the client system under test.") 6609 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/http-operations") 6610 protected Enumeration<TestScriptRequestMethodCode> requestMethod; 6611 6612 /** 6613 * The value to use in a comparison against the request URL path string. 6614 */ 6615 @Child(name = "requestURL", type = { 6616 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 6617 @Description(shortDefinition = "Request URL comparison value", formalDefinition = "The value to use in a comparison against the request URL path string.") 6618 protected StringType requestURL; 6619 6620 /** 6621 * The type of the resource. See http://build.fhir.org/resourcelist.html. 6622 */ 6623 @Child(name = "resource", type = { 6624 CodeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 6625 @Description(shortDefinition = "Resource type", formalDefinition = "The type of the resource. See http://build.fhir.org/resourcelist.html.") 6626 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/defined-types") 6627 protected CodeType resource; 6628 6629 /** 6630 * okay | created | noContent | notModified | bad | forbidden | notFound | 6631 * methodNotAllowed | conflict | gone | preconditionFailed | unprocessable. 6632 */ 6633 @Child(name = "response", type = { 6634 CodeType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 6635 @Description(shortDefinition = "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable", formalDefinition = "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.") 6636 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/assert-response-code-types") 6637 protected Enumeration<AssertionResponseTypes> response; 6638 6639 /** 6640 * The value of the HTTP response code to be tested. 6641 */ 6642 @Child(name = "responseCode", type = { 6643 StringType.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 6644 @Description(shortDefinition = "HTTP response code to test", formalDefinition = "The value of the HTTP response code to be tested.") 6645 protected StringType responseCode; 6646 6647 /** 6648 * Fixture to evaluate the XPath/JSONPath expression or the headerField against. 6649 */ 6650 @Child(name = "sourceId", type = { IdType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 6651 @Description(shortDefinition = "Fixture Id of source expression or headerField", formalDefinition = "Fixture to evaluate the XPath/JSONPath expression or the headerField against.") 6652 protected IdType sourceId; 6653 6654 /** 6655 * The ID of the Profile to validate against. 6656 */ 6657 @Child(name = "validateProfileId", type = { 6658 IdType.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 6659 @Description(shortDefinition = "Profile Id of validation profile reference", formalDefinition = "The ID of the Profile to validate against.") 6660 protected IdType validateProfileId; 6661 6662 /** 6663 * The value to compare to. 6664 */ 6665 @Child(name = "value", type = { StringType.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 6666 @Description(shortDefinition = "The value to compare to", formalDefinition = "The value to compare to.") 6667 protected StringType value; 6668 6669 /** 6670 * Whether or not the test execution will produce a warning only on error for 6671 * this assert. 6672 */ 6673 @Child(name = "warningOnly", type = { 6674 BooleanType.class }, order = 22, min = 1, max = 1, modifier = false, summary = false) 6675 @Description(shortDefinition = "Will this assert produce a warning only on error?", formalDefinition = "Whether or not the test execution will produce a warning only on error for this assert.") 6676 protected BooleanType warningOnly; 6677 6678 private static final long serialVersionUID = -1086518778L; 6679 6680 /** 6681 * Constructor 6682 */ 6683 public SetupActionAssertComponent() { 6684 super(); 6685 } 6686 6687 /** 6688 * Constructor 6689 */ 6690 public SetupActionAssertComponent(BooleanType warningOnly) { 6691 super(); 6692 this.warningOnly = warningOnly; 6693 } 6694 6695 /** 6696 * @return {@link #label} (The label would be used for tracking/logging purposes 6697 * by test engines.). This is the underlying object with id, value and 6698 * extensions. The accessor "getLabel" gives direct access to the value 6699 */ 6700 public StringType getLabelElement() { 6701 if (this.label == null) 6702 if (Configuration.errorOnAutoCreate()) 6703 throw new Error("Attempt to auto-create SetupActionAssertComponent.label"); 6704 else if (Configuration.doAutoCreate()) 6705 this.label = new StringType(); // bb 6706 return this.label; 6707 } 6708 6709 public boolean hasLabelElement() { 6710 return this.label != null && !this.label.isEmpty(); 6711 } 6712 6713 public boolean hasLabel() { 6714 return this.label != null && !this.label.isEmpty(); 6715 } 6716 6717 /** 6718 * @param value {@link #label} (The label would be used for tracking/logging 6719 * purposes by test engines.). This is the underlying object with 6720 * id, value and extensions. The accessor "getLabel" gives direct 6721 * access to the value 6722 */ 6723 public SetupActionAssertComponent setLabelElement(StringType value) { 6724 this.label = value; 6725 return this; 6726 } 6727 6728 /** 6729 * @return The label would be used for tracking/logging purposes by test 6730 * engines. 6731 */ 6732 public String getLabel() { 6733 return this.label == null ? null : this.label.getValue(); 6734 } 6735 6736 /** 6737 * @param value The label would be used for tracking/logging purposes by test 6738 * engines. 6739 */ 6740 public SetupActionAssertComponent setLabel(String value) { 6741 if (Utilities.noString(value)) 6742 this.label = null; 6743 else { 6744 if (this.label == null) 6745 this.label = new StringType(); 6746 this.label.setValue(value); 6747 } 6748 return this; 6749 } 6750 6751 /** 6752 * @return {@link #description} (The description would be used by test engines 6753 * for tracking and reporting purposes.). This is the underlying object 6754 * with id, value and extensions. The accessor "getDescription" gives 6755 * direct access to the value 6756 */ 6757 public StringType getDescriptionElement() { 6758 if (this.description == null) 6759 if (Configuration.errorOnAutoCreate()) 6760 throw new Error("Attempt to auto-create SetupActionAssertComponent.description"); 6761 else if (Configuration.doAutoCreate()) 6762 this.description = new StringType(); // bb 6763 return this.description; 6764 } 6765 6766 public boolean hasDescriptionElement() { 6767 return this.description != null && !this.description.isEmpty(); 6768 } 6769 6770 public boolean hasDescription() { 6771 return this.description != null && !this.description.isEmpty(); 6772 } 6773 6774 /** 6775 * @param value {@link #description} (The description would be used by test 6776 * engines for tracking and reporting purposes.). This is the 6777 * underlying object with id, value and extensions. The accessor 6778 * "getDescription" gives direct access to the value 6779 */ 6780 public SetupActionAssertComponent setDescriptionElement(StringType value) { 6781 this.description = value; 6782 return this; 6783 } 6784 6785 /** 6786 * @return The description would be used by test engines for tracking and 6787 * reporting purposes. 6788 */ 6789 public String getDescription() { 6790 return this.description == null ? null : this.description.getValue(); 6791 } 6792 6793 /** 6794 * @param value The description would be used by test engines for tracking and 6795 * reporting purposes. 6796 */ 6797 public SetupActionAssertComponent setDescription(String value) { 6798 if (Utilities.noString(value)) 6799 this.description = null; 6800 else { 6801 if (this.description == null) 6802 this.description = new StringType(); 6803 this.description.setValue(value); 6804 } 6805 return this; 6806 } 6807 6808 /** 6809 * @return {@link #direction} (The direction to use for the assertion.). This is 6810 * the underlying object with id, value and extensions. The accessor 6811 * "getDirection" gives direct access to the value 6812 */ 6813 public Enumeration<AssertionDirectionType> getDirectionElement() { 6814 if (this.direction == null) 6815 if (Configuration.errorOnAutoCreate()) 6816 throw new Error("Attempt to auto-create SetupActionAssertComponent.direction"); 6817 else if (Configuration.doAutoCreate()) 6818 this.direction = new Enumeration<AssertionDirectionType>(new AssertionDirectionTypeEnumFactory()); // bb 6819 return this.direction; 6820 } 6821 6822 public boolean hasDirectionElement() { 6823 return this.direction != null && !this.direction.isEmpty(); 6824 } 6825 6826 public boolean hasDirection() { 6827 return this.direction != null && !this.direction.isEmpty(); 6828 } 6829 6830 /** 6831 * @param value {@link #direction} (The direction to use for the assertion.). 6832 * This is the underlying object with id, value and extensions. The 6833 * accessor "getDirection" gives direct access to the value 6834 */ 6835 public SetupActionAssertComponent setDirectionElement(Enumeration<AssertionDirectionType> value) { 6836 this.direction = value; 6837 return this; 6838 } 6839 6840 /** 6841 * @return The direction to use for the assertion. 6842 */ 6843 public AssertionDirectionType getDirection() { 6844 return this.direction == null ? null : this.direction.getValue(); 6845 } 6846 6847 /** 6848 * @param value The direction to use for the assertion. 6849 */ 6850 public SetupActionAssertComponent setDirection(AssertionDirectionType value) { 6851 if (value == null) 6852 this.direction = null; 6853 else { 6854 if (this.direction == null) 6855 this.direction = new Enumeration<AssertionDirectionType>(new AssertionDirectionTypeEnumFactory()); 6856 this.direction.setValue(value); 6857 } 6858 return this; 6859 } 6860 6861 /** 6862 * @return {@link #compareToSourceId} (Id of the source fixture used as the 6863 * contents to be evaluated by either the "source/expression" or 6864 * "sourceId/path" definition.). This is the underlying object with id, 6865 * value and extensions. The accessor "getCompareToSourceId" gives 6866 * direct access to the value 6867 */ 6868 public StringType getCompareToSourceIdElement() { 6869 if (this.compareToSourceId == null) 6870 if (Configuration.errorOnAutoCreate()) 6871 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourceId"); 6872 else if (Configuration.doAutoCreate()) 6873 this.compareToSourceId = new StringType(); // bb 6874 return this.compareToSourceId; 6875 } 6876 6877 public boolean hasCompareToSourceIdElement() { 6878 return this.compareToSourceId != null && !this.compareToSourceId.isEmpty(); 6879 } 6880 6881 public boolean hasCompareToSourceId() { 6882 return this.compareToSourceId != null && !this.compareToSourceId.isEmpty(); 6883 } 6884 6885 /** 6886 * @param value {@link #compareToSourceId} (Id of the source fixture used as the 6887 * contents to be evaluated by either the "source/expression" or 6888 * "sourceId/path" definition.). This is the underlying object with 6889 * id, value and extensions. The accessor "getCompareToSourceId" 6890 * gives direct access to the value 6891 */ 6892 public SetupActionAssertComponent setCompareToSourceIdElement(StringType value) { 6893 this.compareToSourceId = value; 6894 return this; 6895 } 6896 6897 /** 6898 * @return Id of the source fixture used as the contents to be evaluated by 6899 * either the "source/expression" or "sourceId/path" definition. 6900 */ 6901 public String getCompareToSourceId() { 6902 return this.compareToSourceId == null ? null : this.compareToSourceId.getValue(); 6903 } 6904 6905 /** 6906 * @param value Id of the source fixture used as the contents to be evaluated by 6907 * either the "source/expression" or "sourceId/path" definition. 6908 */ 6909 public SetupActionAssertComponent setCompareToSourceId(String value) { 6910 if (Utilities.noString(value)) 6911 this.compareToSourceId = null; 6912 else { 6913 if (this.compareToSourceId == null) 6914 this.compareToSourceId = new StringType(); 6915 this.compareToSourceId.setValue(value); 6916 } 6917 return this; 6918 } 6919 6920 /** 6921 * @return {@link #compareToSourceExpression} (The FHIRPath expression to 6922 * evaluate against the source fixture. When compareToSourceId is 6923 * defined, either compareToSourceExpression or compareToSourcePath must 6924 * be defined, but not both.). This is the underlying object with id, 6925 * value and extensions. The accessor "getCompareToSourceExpression" 6926 * gives direct access to the value 6927 */ 6928 public StringType getCompareToSourceExpressionElement() { 6929 if (this.compareToSourceExpression == null) 6930 if (Configuration.errorOnAutoCreate()) 6931 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourceExpression"); 6932 else if (Configuration.doAutoCreate()) 6933 this.compareToSourceExpression = new StringType(); // bb 6934 return this.compareToSourceExpression; 6935 } 6936 6937 public boolean hasCompareToSourceExpressionElement() { 6938 return this.compareToSourceExpression != null && !this.compareToSourceExpression.isEmpty(); 6939 } 6940 6941 public boolean hasCompareToSourceExpression() { 6942 return this.compareToSourceExpression != null && !this.compareToSourceExpression.isEmpty(); 6943 } 6944 6945 /** 6946 * @param value {@link #compareToSourceExpression} (The FHIRPath expression to 6947 * evaluate against the source fixture. When compareToSourceId is 6948 * defined, either compareToSourceExpression or compareToSourcePath 6949 * must be defined, but not both.). This is the underlying object 6950 * with id, value and extensions. The accessor 6951 * "getCompareToSourceExpression" gives direct access to the value 6952 */ 6953 public SetupActionAssertComponent setCompareToSourceExpressionElement(StringType value) { 6954 this.compareToSourceExpression = value; 6955 return this; 6956 } 6957 6958 /** 6959 * @return The FHIRPath expression to evaluate against the source fixture. When 6960 * compareToSourceId is defined, either compareToSourceExpression or 6961 * compareToSourcePath must be defined, but not both. 6962 */ 6963 public String getCompareToSourceExpression() { 6964 return this.compareToSourceExpression == null ? null : this.compareToSourceExpression.getValue(); 6965 } 6966 6967 /** 6968 * @param value The FHIRPath expression to evaluate against the source fixture. 6969 * When compareToSourceId is defined, either 6970 * compareToSourceExpression or compareToSourcePath must be 6971 * defined, but not both. 6972 */ 6973 public SetupActionAssertComponent setCompareToSourceExpression(String value) { 6974 if (Utilities.noString(value)) 6975 this.compareToSourceExpression = null; 6976 else { 6977 if (this.compareToSourceExpression == null) 6978 this.compareToSourceExpression = new StringType(); 6979 this.compareToSourceExpression.setValue(value); 6980 } 6981 return this; 6982 } 6983 6984 /** 6985 * @return {@link #compareToSourcePath} (XPath or JSONPath expression to 6986 * evaluate against the source fixture. When compareToSourceId is 6987 * defined, either compareToSourceExpression or compareToSourcePath must 6988 * be defined, but not both.). This is the underlying object with id, 6989 * value and extensions. The accessor "getCompareToSourcePath" gives 6990 * direct access to the value 6991 */ 6992 public StringType getCompareToSourcePathElement() { 6993 if (this.compareToSourcePath == null) 6994 if (Configuration.errorOnAutoCreate()) 6995 throw new Error("Attempt to auto-create SetupActionAssertComponent.compareToSourcePath"); 6996 else if (Configuration.doAutoCreate()) 6997 this.compareToSourcePath = new StringType(); // bb 6998 return this.compareToSourcePath; 6999 } 7000 7001 public boolean hasCompareToSourcePathElement() { 7002 return this.compareToSourcePath != null && !this.compareToSourcePath.isEmpty(); 7003 } 7004 7005 public boolean hasCompareToSourcePath() { 7006 return this.compareToSourcePath != null && !this.compareToSourcePath.isEmpty(); 7007 } 7008 7009 /** 7010 * @param value {@link #compareToSourcePath} (XPath or JSONPath expression to 7011 * evaluate against the source fixture. When compareToSourceId is 7012 * defined, either compareToSourceExpression or compareToSourcePath 7013 * must be defined, but not both.). This is the underlying object 7014 * with id, value and extensions. The accessor 7015 * "getCompareToSourcePath" gives direct access to the value 7016 */ 7017 public SetupActionAssertComponent setCompareToSourcePathElement(StringType value) { 7018 this.compareToSourcePath = value; 7019 return this; 7020 } 7021 7022 /** 7023 * @return XPath or JSONPath expression to evaluate against the source fixture. 7024 * When compareToSourceId is defined, either compareToSourceExpression 7025 * or compareToSourcePath must be defined, but not both. 7026 */ 7027 public String getCompareToSourcePath() { 7028 return this.compareToSourcePath == null ? null : this.compareToSourcePath.getValue(); 7029 } 7030 7031 /** 7032 * @param value XPath or JSONPath expression to evaluate against the source 7033 * fixture. When compareToSourceId is defined, either 7034 * compareToSourceExpression or compareToSourcePath must be 7035 * defined, but not both. 7036 */ 7037 public SetupActionAssertComponent setCompareToSourcePath(String value) { 7038 if (Utilities.noString(value)) 7039 this.compareToSourcePath = null; 7040 else { 7041 if (this.compareToSourcePath == null) 7042 this.compareToSourcePath = new StringType(); 7043 this.compareToSourcePath.setValue(value); 7044 } 7045 return this; 7046 } 7047 7048 /** 7049 * @return {@link #contentType} (The mime-type contents to compare against the 7050 * request or response message 'Content-Type' header.). This is the 7051 * underlying object with id, value and extensions. The accessor 7052 * "getContentType" gives direct access to the value 7053 */ 7054 public CodeType getContentTypeElement() { 7055 if (this.contentType == null) 7056 if (Configuration.errorOnAutoCreate()) 7057 throw new Error("Attempt to auto-create SetupActionAssertComponent.contentType"); 7058 else if (Configuration.doAutoCreate()) 7059 this.contentType = new CodeType(); // bb 7060 return this.contentType; 7061 } 7062 7063 public boolean hasContentTypeElement() { 7064 return this.contentType != null && !this.contentType.isEmpty(); 7065 } 7066 7067 public boolean hasContentType() { 7068 return this.contentType != null && !this.contentType.isEmpty(); 7069 } 7070 7071 /** 7072 * @param value {@link #contentType} (The mime-type contents to compare against 7073 * the request or response message 'Content-Type' header.). This is 7074 * the underlying object with id, value and extensions. The 7075 * accessor "getContentType" gives direct access to the value 7076 */ 7077 public SetupActionAssertComponent setContentTypeElement(CodeType value) { 7078 this.contentType = value; 7079 return this; 7080 } 7081 7082 /** 7083 * @return The mime-type contents to compare against the request or response 7084 * message 'Content-Type' header. 7085 */ 7086 public String getContentType() { 7087 return this.contentType == null ? null : this.contentType.getValue(); 7088 } 7089 7090 /** 7091 * @param value The mime-type contents to compare against the request or 7092 * response message 'Content-Type' header. 7093 */ 7094 public SetupActionAssertComponent setContentType(String value) { 7095 if (Utilities.noString(value)) 7096 this.contentType = null; 7097 else { 7098 if (this.contentType == null) 7099 this.contentType = new CodeType(); 7100 this.contentType.setValue(value); 7101 } 7102 return this; 7103 } 7104 7105 /** 7106 * @return {@link #expression} (The FHIRPath expression to be evaluated against 7107 * the request or response message contents - HTTP headers and 7108 * payload.). This is the underlying object with id, value and 7109 * extensions. The accessor "getExpression" gives direct access to the 7110 * value 7111 */ 7112 public StringType getExpressionElement() { 7113 if (this.expression == null) 7114 if (Configuration.errorOnAutoCreate()) 7115 throw new Error("Attempt to auto-create SetupActionAssertComponent.expression"); 7116 else if (Configuration.doAutoCreate()) 7117 this.expression = new StringType(); // bb 7118 return this.expression; 7119 } 7120 7121 public boolean hasExpressionElement() { 7122 return this.expression != null && !this.expression.isEmpty(); 7123 } 7124 7125 public boolean hasExpression() { 7126 return this.expression != null && !this.expression.isEmpty(); 7127 } 7128 7129 /** 7130 * @param value {@link #expression} (The FHIRPath expression to be evaluated 7131 * against the request or response message contents - HTTP headers 7132 * and payload.). This is the underlying object with id, value and 7133 * extensions. The accessor "getExpression" gives direct access to 7134 * the value 7135 */ 7136 public SetupActionAssertComponent setExpressionElement(StringType value) { 7137 this.expression = value; 7138 return this; 7139 } 7140 7141 /** 7142 * @return The FHIRPath expression to be evaluated against the request or 7143 * response message contents - HTTP headers and payload. 7144 */ 7145 public String getExpression() { 7146 return this.expression == null ? null : this.expression.getValue(); 7147 } 7148 7149 /** 7150 * @param value The FHIRPath expression to be evaluated against the request or 7151 * response message contents - HTTP headers and payload. 7152 */ 7153 public SetupActionAssertComponent setExpression(String value) { 7154 if (Utilities.noString(value)) 7155 this.expression = null; 7156 else { 7157 if (this.expression == null) 7158 this.expression = new StringType(); 7159 this.expression.setValue(value); 7160 } 7161 return this; 7162 } 7163 7164 /** 7165 * @return {@link #headerField} (The HTTP header field name e.g. 'Location'.). 7166 * This is the underlying object with id, value and extensions. The 7167 * accessor "getHeaderField" gives direct access to the value 7168 */ 7169 public StringType getHeaderFieldElement() { 7170 if (this.headerField == null) 7171 if (Configuration.errorOnAutoCreate()) 7172 throw new Error("Attempt to auto-create SetupActionAssertComponent.headerField"); 7173 else if (Configuration.doAutoCreate()) 7174 this.headerField = new StringType(); // bb 7175 return this.headerField; 7176 } 7177 7178 public boolean hasHeaderFieldElement() { 7179 return this.headerField != null && !this.headerField.isEmpty(); 7180 } 7181 7182 public boolean hasHeaderField() { 7183 return this.headerField != null && !this.headerField.isEmpty(); 7184 } 7185 7186 /** 7187 * @param value {@link #headerField} (The HTTP header field name e.g. 7188 * 'Location'.). This is the underlying object with id, value and 7189 * extensions. The accessor "getHeaderField" gives direct access to 7190 * the value 7191 */ 7192 public SetupActionAssertComponent setHeaderFieldElement(StringType value) { 7193 this.headerField = value; 7194 return this; 7195 } 7196 7197 /** 7198 * @return The HTTP header field name e.g. 'Location'. 7199 */ 7200 public String getHeaderField() { 7201 return this.headerField == null ? null : this.headerField.getValue(); 7202 } 7203 7204 /** 7205 * @param value The HTTP header field name e.g. 'Location'. 7206 */ 7207 public SetupActionAssertComponent setHeaderField(String value) { 7208 if (Utilities.noString(value)) 7209 this.headerField = null; 7210 else { 7211 if (this.headerField == null) 7212 this.headerField = new StringType(); 7213 this.headerField.setValue(value); 7214 } 7215 return this; 7216 } 7217 7218 /** 7219 * @return {@link #minimumId} (The ID of a fixture. Asserts that the response 7220 * contains at a minimum the fixture specified by minimumId.). This is 7221 * the underlying object with id, value and extensions. The accessor 7222 * "getMinimumId" gives direct access to the value 7223 */ 7224 public StringType getMinimumIdElement() { 7225 if (this.minimumId == null) 7226 if (Configuration.errorOnAutoCreate()) 7227 throw new Error("Attempt to auto-create SetupActionAssertComponent.minimumId"); 7228 else if (Configuration.doAutoCreate()) 7229 this.minimumId = new StringType(); // bb 7230 return this.minimumId; 7231 } 7232 7233 public boolean hasMinimumIdElement() { 7234 return this.minimumId != null && !this.minimumId.isEmpty(); 7235 } 7236 7237 public boolean hasMinimumId() { 7238 return this.minimumId != null && !this.minimumId.isEmpty(); 7239 } 7240 7241 /** 7242 * @param value {@link #minimumId} (The ID of a fixture. Asserts that the 7243 * response contains at a minimum the fixture specified by 7244 * minimumId.). This is the underlying object with id, value and 7245 * extensions. The accessor "getMinimumId" gives direct access to 7246 * the value 7247 */ 7248 public SetupActionAssertComponent setMinimumIdElement(StringType value) { 7249 this.minimumId = value; 7250 return this; 7251 } 7252 7253 /** 7254 * @return The ID of a fixture. Asserts that the response contains at a minimum 7255 * the fixture specified by minimumId. 7256 */ 7257 public String getMinimumId() { 7258 return this.minimumId == null ? null : this.minimumId.getValue(); 7259 } 7260 7261 /** 7262 * @param value The ID of a fixture. Asserts that the response contains at a 7263 * minimum the fixture specified by minimumId. 7264 */ 7265 public SetupActionAssertComponent setMinimumId(String value) { 7266 if (Utilities.noString(value)) 7267 this.minimumId = null; 7268 else { 7269 if (this.minimumId == null) 7270 this.minimumId = new StringType(); 7271 this.minimumId.setValue(value); 7272 } 7273 return this; 7274 } 7275 7276 /** 7277 * @return {@link #navigationLinks} (Whether or not the test execution performs 7278 * validation on the bundle navigation links.). This is the underlying 7279 * object with id, value and extensions. The accessor 7280 * "getNavigationLinks" gives direct access to the value 7281 */ 7282 public BooleanType getNavigationLinksElement() { 7283 if (this.navigationLinks == null) 7284 if (Configuration.errorOnAutoCreate()) 7285 throw new Error("Attempt to auto-create SetupActionAssertComponent.navigationLinks"); 7286 else if (Configuration.doAutoCreate()) 7287 this.navigationLinks = new BooleanType(); // bb 7288 return this.navigationLinks; 7289 } 7290 7291 public boolean hasNavigationLinksElement() { 7292 return this.navigationLinks != null && !this.navigationLinks.isEmpty(); 7293 } 7294 7295 public boolean hasNavigationLinks() { 7296 return this.navigationLinks != null && !this.navigationLinks.isEmpty(); 7297 } 7298 7299 /** 7300 * @param value {@link #navigationLinks} (Whether or not the test execution 7301 * performs validation on the bundle navigation links.). This is 7302 * the underlying object with id, value and extensions. The 7303 * accessor "getNavigationLinks" gives direct access to the value 7304 */ 7305 public SetupActionAssertComponent setNavigationLinksElement(BooleanType value) { 7306 this.navigationLinks = value; 7307 return this; 7308 } 7309 7310 /** 7311 * @return Whether or not the test execution performs validation on the bundle 7312 * navigation links. 7313 */ 7314 public boolean getNavigationLinks() { 7315 return this.navigationLinks == null || this.navigationLinks.isEmpty() ? false : this.navigationLinks.getValue(); 7316 } 7317 7318 /** 7319 * @param value Whether or not the test execution performs validation on the 7320 * bundle navigation links. 7321 */ 7322 public SetupActionAssertComponent setNavigationLinks(boolean value) { 7323 if (this.navigationLinks == null) 7324 this.navigationLinks = new BooleanType(); 7325 this.navigationLinks.setValue(value); 7326 return this; 7327 } 7328 7329 /** 7330 * @return {@link #operator} (The operator type defines the conditional behavior 7331 * of the assert. If not defined, the default is equals.). This is the 7332 * underlying object with id, value and extensions. The accessor 7333 * "getOperator" gives direct access to the value 7334 */ 7335 public Enumeration<AssertionOperatorType> getOperatorElement() { 7336 if (this.operator == null) 7337 if (Configuration.errorOnAutoCreate()) 7338 throw new Error("Attempt to auto-create SetupActionAssertComponent.operator"); 7339 else if (Configuration.doAutoCreate()) 7340 this.operator = new Enumeration<AssertionOperatorType>(new AssertionOperatorTypeEnumFactory()); // bb 7341 return this.operator; 7342 } 7343 7344 public boolean hasOperatorElement() { 7345 return this.operator != null && !this.operator.isEmpty(); 7346 } 7347 7348 public boolean hasOperator() { 7349 return this.operator != null && !this.operator.isEmpty(); 7350 } 7351 7352 /** 7353 * @param value {@link #operator} (The operator type defines the conditional 7354 * behavior of the assert. If not defined, the default is equals.). 7355 * This is the underlying object with id, value and extensions. The 7356 * accessor "getOperator" gives direct access to the value 7357 */ 7358 public SetupActionAssertComponent setOperatorElement(Enumeration<AssertionOperatorType> value) { 7359 this.operator = value; 7360 return this; 7361 } 7362 7363 /** 7364 * @return The operator type defines the conditional behavior of the assert. If 7365 * not defined, the default is equals. 7366 */ 7367 public AssertionOperatorType getOperator() { 7368 return this.operator == null ? null : this.operator.getValue(); 7369 } 7370 7371 /** 7372 * @param value The operator type defines the conditional behavior of the 7373 * assert. If not defined, the default is equals. 7374 */ 7375 public SetupActionAssertComponent setOperator(AssertionOperatorType value) { 7376 if (value == null) 7377 this.operator = null; 7378 else { 7379 if (this.operator == null) 7380 this.operator = new Enumeration<AssertionOperatorType>(new AssertionOperatorTypeEnumFactory()); 7381 this.operator.setValue(value); 7382 } 7383 return this; 7384 } 7385 7386 /** 7387 * @return {@link #path} (The XPath or JSONPath expression to be evaluated 7388 * against the fixture representing the response received from server.). 7389 * This is the underlying object with id, value and extensions. The 7390 * accessor "getPath" gives direct access to the value 7391 */ 7392 public StringType getPathElement() { 7393 if (this.path == null) 7394 if (Configuration.errorOnAutoCreate()) 7395 throw new Error("Attempt to auto-create SetupActionAssertComponent.path"); 7396 else if (Configuration.doAutoCreate()) 7397 this.path = new StringType(); // bb 7398 return this.path; 7399 } 7400 7401 public boolean hasPathElement() { 7402 return this.path != null && !this.path.isEmpty(); 7403 } 7404 7405 public boolean hasPath() { 7406 return this.path != null && !this.path.isEmpty(); 7407 } 7408 7409 /** 7410 * @param value {@link #path} (The XPath or JSONPath expression to be evaluated 7411 * against the fixture representing the response received from 7412 * server.). This is the underlying object with id, value and 7413 * extensions. The accessor "getPath" gives direct access to the 7414 * value 7415 */ 7416 public SetupActionAssertComponent setPathElement(StringType value) { 7417 this.path = value; 7418 return this; 7419 } 7420 7421 /** 7422 * @return The XPath or JSONPath expression to be evaluated against the fixture 7423 * representing the response received from server. 7424 */ 7425 public String getPath() { 7426 return this.path == null ? null : this.path.getValue(); 7427 } 7428 7429 /** 7430 * @param value The XPath or JSONPath expression to be evaluated against the 7431 * fixture representing the response received from server. 7432 */ 7433 public SetupActionAssertComponent setPath(String value) { 7434 if (Utilities.noString(value)) 7435 this.path = null; 7436 else { 7437 if (this.path == null) 7438 this.path = new StringType(); 7439 this.path.setValue(value); 7440 } 7441 return this; 7442 } 7443 7444 /** 7445 * @return {@link #requestMethod} (The request method or HTTP operation code to 7446 * compare against that used by the client system under test.). This is 7447 * the underlying object with id, value and extensions. The accessor 7448 * "getRequestMethod" gives direct access to the value 7449 */ 7450 public Enumeration<TestScriptRequestMethodCode> getRequestMethodElement() { 7451 if (this.requestMethod == null) 7452 if (Configuration.errorOnAutoCreate()) 7453 throw new Error("Attempt to auto-create SetupActionAssertComponent.requestMethod"); 7454 else if (Configuration.doAutoCreate()) 7455 this.requestMethod = new Enumeration<TestScriptRequestMethodCode>( 7456 new TestScriptRequestMethodCodeEnumFactory()); // bb 7457 return this.requestMethod; 7458 } 7459 7460 public boolean hasRequestMethodElement() { 7461 return this.requestMethod != null && !this.requestMethod.isEmpty(); 7462 } 7463 7464 public boolean hasRequestMethod() { 7465 return this.requestMethod != null && !this.requestMethod.isEmpty(); 7466 } 7467 7468 /** 7469 * @param value {@link #requestMethod} (The request method or HTTP operation 7470 * code to compare against that used by the client system under 7471 * test.). This is the underlying object with id, value and 7472 * extensions. The accessor "getRequestMethod" gives direct access 7473 * to the value 7474 */ 7475 public SetupActionAssertComponent setRequestMethodElement(Enumeration<TestScriptRequestMethodCode> value) { 7476 this.requestMethod = value; 7477 return this; 7478 } 7479 7480 /** 7481 * @return The request method or HTTP operation code to compare against that 7482 * used by the client system under test. 7483 */ 7484 public TestScriptRequestMethodCode getRequestMethod() { 7485 return this.requestMethod == null ? null : this.requestMethod.getValue(); 7486 } 7487 7488 /** 7489 * @param value The request method or HTTP operation code to compare against 7490 * that used by the client system under test. 7491 */ 7492 public SetupActionAssertComponent setRequestMethod(TestScriptRequestMethodCode value) { 7493 if (value == null) 7494 this.requestMethod = null; 7495 else { 7496 if (this.requestMethod == null) 7497 this.requestMethod = new Enumeration<TestScriptRequestMethodCode>( 7498 new TestScriptRequestMethodCodeEnumFactory()); 7499 this.requestMethod.setValue(value); 7500 } 7501 return this; 7502 } 7503 7504 /** 7505 * @return {@link #requestURL} (The value to use in a comparison against the 7506 * request URL path string.). This is the underlying object with id, 7507 * value and extensions. The accessor "getRequestURL" gives direct 7508 * access to the value 7509 */ 7510 public StringType getRequestURLElement() { 7511 if (this.requestURL == null) 7512 if (Configuration.errorOnAutoCreate()) 7513 throw new Error("Attempt to auto-create SetupActionAssertComponent.requestURL"); 7514 else if (Configuration.doAutoCreate()) 7515 this.requestURL = new StringType(); // bb 7516 return this.requestURL; 7517 } 7518 7519 public boolean hasRequestURLElement() { 7520 return this.requestURL != null && !this.requestURL.isEmpty(); 7521 } 7522 7523 public boolean hasRequestURL() { 7524 return this.requestURL != null && !this.requestURL.isEmpty(); 7525 } 7526 7527 /** 7528 * @param value {@link #requestURL} (The value to use in a comparison against 7529 * the request URL path string.). This is the underlying object 7530 * with id, value and extensions. The accessor "getRequestURL" 7531 * gives direct access to the value 7532 */ 7533 public SetupActionAssertComponent setRequestURLElement(StringType value) { 7534 this.requestURL = value; 7535 return this; 7536 } 7537 7538 /** 7539 * @return The value to use in a comparison against the request URL path string. 7540 */ 7541 public String getRequestURL() { 7542 return this.requestURL == null ? null : this.requestURL.getValue(); 7543 } 7544 7545 /** 7546 * @param value The value to use in a comparison against the request URL path 7547 * string. 7548 */ 7549 public SetupActionAssertComponent setRequestURL(String value) { 7550 if (Utilities.noString(value)) 7551 this.requestURL = null; 7552 else { 7553 if (this.requestURL == null) 7554 this.requestURL = new StringType(); 7555 this.requestURL.setValue(value); 7556 } 7557 return this; 7558 } 7559 7560 /** 7561 * @return {@link #resource} (The type of the resource. See 7562 * http://build.fhir.org/resourcelist.html.). This is the underlying 7563 * object with id, value and extensions. The accessor "getResource" 7564 * gives direct access to the value 7565 */ 7566 public CodeType getResourceElement() { 7567 if (this.resource == null) 7568 if (Configuration.errorOnAutoCreate()) 7569 throw new Error("Attempt to auto-create SetupActionAssertComponent.resource"); 7570 else if (Configuration.doAutoCreate()) 7571 this.resource = new CodeType(); // bb 7572 return this.resource; 7573 } 7574 7575 public boolean hasResourceElement() { 7576 return this.resource != null && !this.resource.isEmpty(); 7577 } 7578 7579 public boolean hasResource() { 7580 return this.resource != null && !this.resource.isEmpty(); 7581 } 7582 7583 /** 7584 * @param value {@link #resource} (The type of the resource. See 7585 * http://build.fhir.org/resourcelist.html.). This is the 7586 * underlying object with id, value and extensions. The accessor 7587 * "getResource" gives direct access to the value 7588 */ 7589 public SetupActionAssertComponent setResourceElement(CodeType value) { 7590 this.resource = value; 7591 return this; 7592 } 7593 7594 /** 7595 * @return The type of the resource. See 7596 * http://build.fhir.org/resourcelist.html. 7597 */ 7598 public String getResource() { 7599 return this.resource == null ? null : this.resource.getValue(); 7600 } 7601 7602 /** 7603 * @param value The type of the resource. See 7604 * http://build.fhir.org/resourcelist.html. 7605 */ 7606 public SetupActionAssertComponent setResource(String value) { 7607 if (Utilities.noString(value)) 7608 this.resource = null; 7609 else { 7610 if (this.resource == null) 7611 this.resource = new CodeType(); 7612 this.resource.setValue(value); 7613 } 7614 return this; 7615 } 7616 7617 /** 7618 * @return {@link #response} (okay | created | noContent | notModified | bad | 7619 * forbidden | notFound | methodNotAllowed | conflict | gone | 7620 * preconditionFailed | unprocessable.). This is the underlying object 7621 * with id, value and extensions. The accessor "getResponse" gives 7622 * direct access to the value 7623 */ 7624 public Enumeration<AssertionResponseTypes> getResponseElement() { 7625 if (this.response == null) 7626 if (Configuration.errorOnAutoCreate()) 7627 throw new Error("Attempt to auto-create SetupActionAssertComponent.response"); 7628 else if (Configuration.doAutoCreate()) 7629 this.response = new Enumeration<AssertionResponseTypes>(new AssertionResponseTypesEnumFactory()); // bb 7630 return this.response; 7631 } 7632 7633 public boolean hasResponseElement() { 7634 return this.response != null && !this.response.isEmpty(); 7635 } 7636 7637 public boolean hasResponse() { 7638 return this.response != null && !this.response.isEmpty(); 7639 } 7640 7641 /** 7642 * @param value {@link #response} (okay | created | noContent | notModified | 7643 * bad | forbidden | notFound | methodNotAllowed | conflict | gone 7644 * | preconditionFailed | unprocessable.). This is the underlying 7645 * object with id, value and extensions. The accessor "getResponse" 7646 * gives direct access to the value 7647 */ 7648 public SetupActionAssertComponent setResponseElement(Enumeration<AssertionResponseTypes> value) { 7649 this.response = value; 7650 return this; 7651 } 7652 7653 /** 7654 * @return okay | created | noContent | notModified | bad | forbidden | notFound 7655 * | methodNotAllowed | conflict | gone | preconditionFailed | 7656 * unprocessable. 7657 */ 7658 public AssertionResponseTypes getResponse() { 7659 return this.response == null ? null : this.response.getValue(); 7660 } 7661 7662 /** 7663 * @param value okay | created | noContent | notModified | bad | forbidden | 7664 * notFound | methodNotAllowed | conflict | gone | 7665 * preconditionFailed | unprocessable. 7666 */ 7667 public SetupActionAssertComponent setResponse(AssertionResponseTypes value) { 7668 if (value == null) 7669 this.response = null; 7670 else { 7671 if (this.response == null) 7672 this.response = new Enumeration<AssertionResponseTypes>(new AssertionResponseTypesEnumFactory()); 7673 this.response.setValue(value); 7674 } 7675 return this; 7676 } 7677 7678 /** 7679 * @return {@link #responseCode} (The value of the HTTP response code to be 7680 * tested.). This is the underlying object with id, value and 7681 * extensions. The accessor "getResponseCode" gives direct access to the 7682 * value 7683 */ 7684 public StringType getResponseCodeElement() { 7685 if (this.responseCode == null) 7686 if (Configuration.errorOnAutoCreate()) 7687 throw new Error("Attempt to auto-create SetupActionAssertComponent.responseCode"); 7688 else if (Configuration.doAutoCreate()) 7689 this.responseCode = new StringType(); // bb 7690 return this.responseCode; 7691 } 7692 7693 public boolean hasResponseCodeElement() { 7694 return this.responseCode != null && !this.responseCode.isEmpty(); 7695 } 7696 7697 public boolean hasResponseCode() { 7698 return this.responseCode != null && !this.responseCode.isEmpty(); 7699 } 7700 7701 /** 7702 * @param value {@link #responseCode} (The value of the HTTP response code to be 7703 * tested.). This is the underlying object with id, value and 7704 * extensions. The accessor "getResponseCode" gives direct access 7705 * to the value 7706 */ 7707 public SetupActionAssertComponent setResponseCodeElement(StringType value) { 7708 this.responseCode = value; 7709 return this; 7710 } 7711 7712 /** 7713 * @return The value of the HTTP response code to be tested. 7714 */ 7715 public String getResponseCode() { 7716 return this.responseCode == null ? null : this.responseCode.getValue(); 7717 } 7718 7719 /** 7720 * @param value The value of the HTTP response code to be tested. 7721 */ 7722 public SetupActionAssertComponent setResponseCode(String value) { 7723 if (Utilities.noString(value)) 7724 this.responseCode = null; 7725 else { 7726 if (this.responseCode == null) 7727 this.responseCode = new StringType(); 7728 this.responseCode.setValue(value); 7729 } 7730 return this; 7731 } 7732 7733 /** 7734 * @return {@link #sourceId} (Fixture to evaluate the XPath/JSONPath expression 7735 * or the headerField against.). This is the underlying object with id, 7736 * value and extensions. The accessor "getSourceId" gives direct access 7737 * to the value 7738 */ 7739 public IdType getSourceIdElement() { 7740 if (this.sourceId == null) 7741 if (Configuration.errorOnAutoCreate()) 7742 throw new Error("Attempt to auto-create SetupActionAssertComponent.sourceId"); 7743 else if (Configuration.doAutoCreate()) 7744 this.sourceId = new IdType(); // bb 7745 return this.sourceId; 7746 } 7747 7748 public boolean hasSourceIdElement() { 7749 return this.sourceId != null && !this.sourceId.isEmpty(); 7750 } 7751 7752 public boolean hasSourceId() { 7753 return this.sourceId != null && !this.sourceId.isEmpty(); 7754 } 7755 7756 /** 7757 * @param value {@link #sourceId} (Fixture to evaluate the XPath/JSONPath 7758 * expression or the headerField against.). This is the underlying 7759 * object with id, value and extensions. The accessor "getSourceId" 7760 * gives direct access to the value 7761 */ 7762 public SetupActionAssertComponent setSourceIdElement(IdType value) { 7763 this.sourceId = value; 7764 return this; 7765 } 7766 7767 /** 7768 * @return Fixture to evaluate the XPath/JSONPath expression or the headerField 7769 * against. 7770 */ 7771 public String getSourceId() { 7772 return this.sourceId == null ? null : this.sourceId.getValue(); 7773 } 7774 7775 /** 7776 * @param value Fixture to evaluate the XPath/JSONPath expression or the 7777 * headerField against. 7778 */ 7779 public SetupActionAssertComponent setSourceId(String value) { 7780 if (Utilities.noString(value)) 7781 this.sourceId = null; 7782 else { 7783 if (this.sourceId == null) 7784 this.sourceId = new IdType(); 7785 this.sourceId.setValue(value); 7786 } 7787 return this; 7788 } 7789 7790 /** 7791 * @return {@link #validateProfileId} (The ID of the Profile to validate 7792 * against.). This is the underlying object with id, value and 7793 * extensions. The accessor "getValidateProfileId" gives direct access 7794 * to the value 7795 */ 7796 public IdType getValidateProfileIdElement() { 7797 if (this.validateProfileId == null) 7798 if (Configuration.errorOnAutoCreate()) 7799 throw new Error("Attempt to auto-create SetupActionAssertComponent.validateProfileId"); 7800 else if (Configuration.doAutoCreate()) 7801 this.validateProfileId = new IdType(); // bb 7802 return this.validateProfileId; 7803 } 7804 7805 public boolean hasValidateProfileIdElement() { 7806 return this.validateProfileId != null && !this.validateProfileId.isEmpty(); 7807 } 7808 7809 public boolean hasValidateProfileId() { 7810 return this.validateProfileId != null && !this.validateProfileId.isEmpty(); 7811 } 7812 7813 /** 7814 * @param value {@link #validateProfileId} (The ID of the Profile to validate 7815 * against.). This is the underlying object with id, value and 7816 * extensions. The accessor "getValidateProfileId" gives direct 7817 * access to the value 7818 */ 7819 public SetupActionAssertComponent setValidateProfileIdElement(IdType value) { 7820 this.validateProfileId = value; 7821 return this; 7822 } 7823 7824 /** 7825 * @return The ID of the Profile to validate against. 7826 */ 7827 public String getValidateProfileId() { 7828 return this.validateProfileId == null ? null : this.validateProfileId.getValue(); 7829 } 7830 7831 /** 7832 * @param value The ID of the Profile to validate against. 7833 */ 7834 public SetupActionAssertComponent setValidateProfileId(String value) { 7835 if (Utilities.noString(value)) 7836 this.validateProfileId = null; 7837 else { 7838 if (this.validateProfileId == null) 7839 this.validateProfileId = new IdType(); 7840 this.validateProfileId.setValue(value); 7841 } 7842 return this; 7843 } 7844 7845 /** 7846 * @return {@link #value} (The value to compare to.). This is the underlying 7847 * object with id, value and extensions. The accessor "getValue" gives 7848 * direct access to the value 7849 */ 7850 public StringType getValueElement() { 7851 if (this.value == null) 7852 if (Configuration.errorOnAutoCreate()) 7853 throw new Error("Attempt to auto-create SetupActionAssertComponent.value"); 7854 else if (Configuration.doAutoCreate()) 7855 this.value = new StringType(); // bb 7856 return this.value; 7857 } 7858 7859 public boolean hasValueElement() { 7860 return this.value != null && !this.value.isEmpty(); 7861 } 7862 7863 public boolean hasValue() { 7864 return this.value != null && !this.value.isEmpty(); 7865 } 7866 7867 /** 7868 * @param value {@link #value} (The value to compare to.). This is the 7869 * underlying object with id, value and extensions. The accessor 7870 * "getValue" gives direct access to the value 7871 */ 7872 public SetupActionAssertComponent setValueElement(StringType value) { 7873 this.value = value; 7874 return this; 7875 } 7876 7877 /** 7878 * @return The value to compare to. 7879 */ 7880 public String getValue() { 7881 return this.value == null ? null : this.value.getValue(); 7882 } 7883 7884 /** 7885 * @param value The value to compare to. 7886 */ 7887 public SetupActionAssertComponent setValue(String value) { 7888 if (Utilities.noString(value)) 7889 this.value = null; 7890 else { 7891 if (this.value == null) 7892 this.value = new StringType(); 7893 this.value.setValue(value); 7894 } 7895 return this; 7896 } 7897 7898 /** 7899 * @return {@link #warningOnly} (Whether or not the test execution will produce 7900 * a warning only on error for this assert.). This is the underlying 7901 * object with id, value and extensions. The accessor "getWarningOnly" 7902 * gives direct access to the value 7903 */ 7904 public BooleanType getWarningOnlyElement() { 7905 if (this.warningOnly == null) 7906 if (Configuration.errorOnAutoCreate()) 7907 throw new Error("Attempt to auto-create SetupActionAssertComponent.warningOnly"); 7908 else if (Configuration.doAutoCreate()) 7909 this.warningOnly = new BooleanType(); // bb 7910 return this.warningOnly; 7911 } 7912 7913 public boolean hasWarningOnlyElement() { 7914 return this.warningOnly != null && !this.warningOnly.isEmpty(); 7915 } 7916 7917 public boolean hasWarningOnly() { 7918 return this.warningOnly != null && !this.warningOnly.isEmpty(); 7919 } 7920 7921 /** 7922 * @param value {@link #warningOnly} (Whether or not the test execution will 7923 * produce a warning only on error for this assert.). This is the 7924 * underlying object with id, value and extensions. The accessor 7925 * "getWarningOnly" gives direct access to the value 7926 */ 7927 public SetupActionAssertComponent setWarningOnlyElement(BooleanType value) { 7928 this.warningOnly = value; 7929 return this; 7930 } 7931 7932 /** 7933 * @return Whether or not the test execution will produce a warning only on 7934 * error for this assert. 7935 */ 7936 public boolean getWarningOnly() { 7937 return this.warningOnly == null || this.warningOnly.isEmpty() ? false : this.warningOnly.getValue(); 7938 } 7939 7940 /** 7941 * @param value Whether or not the test execution will produce a warning only on 7942 * error for this assert. 7943 */ 7944 public SetupActionAssertComponent setWarningOnly(boolean value) { 7945 if (this.warningOnly == null) 7946 this.warningOnly = new BooleanType(); 7947 this.warningOnly.setValue(value); 7948 return this; 7949 } 7950 7951 protected void listChildren(List<Property> children) { 7952 super.listChildren(children); 7953 children.add(new Property("label", "string", 7954 "The label would be used for tracking/logging purposes by test engines.", 0, 1, label)); 7955 children.add(new Property("description", "string", 7956 "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description)); 7957 children.add(new Property("direction", "code", "The direction to use for the assertion.", 0, 1, direction)); 7958 children.add(new Property("compareToSourceId", "string", 7959 "Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition.", 7960 0, 1, compareToSourceId)); 7961 children.add(new Property("compareToSourceExpression", "string", 7962 "The FHIRPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 7963 0, 1, compareToSourceExpression)); 7964 children.add(new Property("compareToSourcePath", "string", 7965 "XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 7966 0, 1, compareToSourcePath)); 7967 children.add(new Property("contentType", "code", 7968 "The mime-type contents to compare against the request or response message 'Content-Type' header.", 0, 1, 7969 contentType)); 7970 children.add(new Property("expression", "string", 7971 "The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload.", 7972 0, 1, expression)); 7973 children 7974 .add(new Property("headerField", "string", "The HTTP header field name e.g. 'Location'.", 0, 1, headerField)); 7975 children.add(new Property("minimumId", "string", 7976 "The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.", 7977 0, 1, minimumId)); 7978 children.add(new Property("navigationLinks", "boolean", 7979 "Whether or not the test execution performs validation on the bundle navigation links.", 0, 1, 7980 navigationLinks)); 7981 children.add(new Property("operator", "code", 7982 "The operator type defines the conditional behavior of the assert. If not defined, the default is equals.", 0, 7983 1, operator)); 7984 children.add(new Property("path", "string", 7985 "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.", 7986 0, 1, path)); 7987 children.add(new Property("requestMethod", "code", 7988 "The request method or HTTP operation code to compare against that used by the client system under test.", 0, 7989 1, requestMethod)); 7990 children.add(new Property("requestURL", "string", 7991 "The value to use in a comparison against the request URL path string.", 0, 1, requestURL)); 7992 children.add(new Property("resource", "code", 7993 "The type of the resource. See http://build.fhir.org/resourcelist.html.", 0, 1, resource)); 7994 children.add(new Property("response", "code", 7995 "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.", 7996 0, 1, response)); 7997 children.add(new Property("responseCode", "string", "The value of the HTTP response code to be tested.", 0, 1, 7998 responseCode)); 7999 children.add(new Property("sourceId", "id", 8000 "Fixture to evaluate the XPath/JSONPath expression or the headerField against.", 0, 1, sourceId)); 8001 children.add(new Property("validateProfileId", "id", "The ID of the Profile to validate against.", 0, 1, 8002 validateProfileId)); 8003 children.add(new Property("value", "string", "The value to compare to.", 0, 1, value)); 8004 children.add(new Property("warningOnly", "boolean", 8005 "Whether or not the test execution will produce a warning only on error for this assert.", 0, 1, 8006 warningOnly)); 8007 } 8008 8009 @Override 8010 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8011 switch (_hash) { 8012 case 102727412: 8013 /* label */ return new Property("label", "string", 8014 "The label would be used for tracking/logging purposes by test engines.", 0, 1, label); 8015 case -1724546052: 8016 /* description */ return new Property("description", "string", 8017 "The description would be used by test engines for tracking and reporting purposes.", 0, 1, description); 8018 case -962590849: 8019 /* direction */ return new Property("direction", "code", "The direction to use for the assertion.", 0, 1, 8020 direction); 8021 case 2081856758: 8022 /* compareToSourceId */ return new Property("compareToSourceId", "string", 8023 "Id of the source fixture used as the contents to be evaluated by either the \"source/expression\" or \"sourceId/path\" definition.", 8024 0, 1, compareToSourceId); 8025 case -1415702669: 8026 /* compareToSourceExpression */ return new Property("compareToSourceExpression", "string", 8027 "The FHIRPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 8028 0, 1, compareToSourceExpression); 8029 case -790206144: 8030 /* compareToSourcePath */ return new Property("compareToSourcePath", "string", 8031 "XPath or JSONPath expression to evaluate against the source fixture. When compareToSourceId is defined, either compareToSourceExpression or compareToSourcePath must be defined, but not both.", 8032 0, 1, compareToSourcePath); 8033 case -389131437: 8034 /* contentType */ return new Property("contentType", "code", 8035 "The mime-type contents to compare against the request or response message 'Content-Type' header.", 0, 1, 8036 contentType); 8037 case -1795452264: 8038 /* expression */ return new Property("expression", "string", 8039 "The FHIRPath expression to be evaluated against the request or response message contents - HTTP headers and payload.", 8040 0, 1, expression); 8041 case 1160732269: 8042 /* headerField */ return new Property("headerField", "string", "The HTTP header field name e.g. 'Location'.", 0, 8043 1, headerField); 8044 case 818925001: 8045 /* minimumId */ return new Property("minimumId", "string", 8046 "The ID of a fixture. Asserts that the response contains at a minimum the fixture specified by minimumId.", 8047 0, 1, minimumId); 8048 case 1001488901: 8049 /* navigationLinks */ return new Property("navigationLinks", "boolean", 8050 "Whether or not the test execution performs validation on the bundle navigation links.", 0, 1, 8051 navigationLinks); 8052 case -500553564: 8053 /* operator */ return new Property("operator", "code", 8054 "The operator type defines the conditional behavior of the assert. If not defined, the default is equals.", 8055 0, 1, operator); 8056 case 3433509: 8057 /* path */ return new Property("path", "string", 8058 "The XPath or JSONPath expression to be evaluated against the fixture representing the response received from server.", 8059 0, 1, path); 8060 case 1217874000: 8061 /* requestMethod */ return new Property("requestMethod", "code", 8062 "The request method or HTTP operation code to compare against that used by the client system under test.", 8063 0, 1, requestMethod); 8064 case 37099616: 8065 /* requestURL */ return new Property("requestURL", "string", 8066 "The value to use in a comparison against the request URL path string.", 0, 1, requestURL); 8067 case -341064690: 8068 /* resource */ return new Property("resource", "code", 8069 "The type of the resource. See http://build.fhir.org/resourcelist.html.", 0, 1, resource); 8070 case -340323263: 8071 /* response */ return new Property("response", "code", 8072 "okay | created | noContent | notModified | bad | forbidden | notFound | methodNotAllowed | conflict | gone | preconditionFailed | unprocessable.", 8073 0, 1, response); 8074 case 1438723534: 8075 /* responseCode */ return new Property("responseCode", "string", 8076 "The value of the HTTP response code to be tested.", 0, 1, responseCode); 8077 case 1746327190: 8078 /* sourceId */ return new Property("sourceId", "id", 8079 "Fixture to evaluate the XPath/JSONPath expression or the headerField against.", 0, 1, sourceId); 8080 case 1555541038: 8081 /* validateProfileId */ return new Property("validateProfileId", "id", 8082 "The ID of the Profile to validate against.", 0, 1, validateProfileId); 8083 case 111972721: 8084 /* value */ return new Property("value", "string", "The value to compare to.", 0, 1, value); 8085 case -481159832: 8086 /* warningOnly */ return new Property("warningOnly", "boolean", 8087 "Whether or not the test execution will produce a warning only on error for this assert.", 0, 1, 8088 warningOnly); 8089 default: 8090 return super.getNamedProperty(_hash, _name, _checkValid); 8091 } 8092 8093 } 8094 8095 @Override 8096 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8097 switch (hash) { 8098 case 102727412: 8099 /* label */ return this.label == null ? new Base[0] : new Base[] { this.label }; // StringType 8100 case -1724546052: 8101 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 8102 case -962590849: 8103 /* direction */ return this.direction == null ? new Base[0] : new Base[] { this.direction }; // Enumeration<AssertionDirectionType> 8104 case 2081856758: 8105 /* compareToSourceId */ return this.compareToSourceId == null ? new Base[0] 8106 : new Base[] { this.compareToSourceId }; // StringType 8107 case -1415702669: 8108 /* compareToSourceExpression */ return this.compareToSourceExpression == null ? new Base[0] 8109 : new Base[] { this.compareToSourceExpression }; // StringType 8110 case -790206144: 8111 /* compareToSourcePath */ return this.compareToSourcePath == null ? new Base[0] 8112 : new Base[] { this.compareToSourcePath }; // StringType 8113 case -389131437: 8114 /* contentType */ return this.contentType == null ? new Base[0] : new Base[] { this.contentType }; // CodeType 8115 case -1795452264: 8116 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // StringType 8117 case 1160732269: 8118 /* headerField */ return this.headerField == null ? new Base[0] : new Base[] { this.headerField }; // StringType 8119 case 818925001: 8120 /* minimumId */ return this.minimumId == null ? new Base[0] : new Base[] { this.minimumId }; // StringType 8121 case 1001488901: 8122 /* navigationLinks */ return this.navigationLinks == null ? new Base[0] : new Base[] { this.navigationLinks }; // BooleanType 8123 case -500553564: 8124 /* operator */ return this.operator == null ? new Base[0] : new Base[] { this.operator }; // Enumeration<AssertionOperatorType> 8125 case 3433509: 8126 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 8127 case 1217874000: 8128 /* requestMethod */ return this.requestMethod == null ? new Base[0] : new Base[] { this.requestMethod }; // Enumeration<TestScriptRequestMethodCode> 8129 case 37099616: 8130 /* requestURL */ return this.requestURL == null ? new Base[0] : new Base[] { this.requestURL }; // StringType 8131 case -341064690: 8132 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // CodeType 8133 case -340323263: 8134 /* response */ return this.response == null ? new Base[0] : new Base[] { this.response }; // Enumeration<AssertionResponseTypes> 8135 case 1438723534: 8136 /* responseCode */ return this.responseCode == null ? new Base[0] : new Base[] { this.responseCode }; // StringType 8137 case 1746327190: 8138 /* sourceId */ return this.sourceId == null ? new Base[0] : new Base[] { this.sourceId }; // IdType 8139 case 1555541038: 8140 /* validateProfileId */ return this.validateProfileId == null ? new Base[0] 8141 : new Base[] { this.validateProfileId }; // IdType 8142 case 111972721: 8143 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 8144 case -481159832: 8145 /* warningOnly */ return this.warningOnly == null ? new Base[0] : new Base[] { this.warningOnly }; // BooleanType 8146 default: 8147 return super.getProperty(hash, name, checkValid); 8148 } 8149 8150 } 8151 8152 @Override 8153 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8154 switch (hash) { 8155 case 102727412: // label 8156 this.label = castToString(value); // StringType 8157 return value; 8158 case -1724546052: // description 8159 this.description = castToString(value); // StringType 8160 return value; 8161 case -962590849: // direction 8162 value = new AssertionDirectionTypeEnumFactory().fromType(castToCode(value)); 8163 this.direction = (Enumeration) value; // Enumeration<AssertionDirectionType> 8164 return value; 8165 case 2081856758: // compareToSourceId 8166 this.compareToSourceId = castToString(value); // StringType 8167 return value; 8168 case -1415702669: // compareToSourceExpression 8169 this.compareToSourceExpression = castToString(value); // StringType 8170 return value; 8171 case -790206144: // compareToSourcePath 8172 this.compareToSourcePath = castToString(value); // StringType 8173 return value; 8174 case -389131437: // contentType 8175 this.contentType = castToCode(value); // CodeType 8176 return value; 8177 case -1795452264: // expression 8178 this.expression = castToString(value); // StringType 8179 return value; 8180 case 1160732269: // headerField 8181 this.headerField = castToString(value); // StringType 8182 return value; 8183 case 818925001: // minimumId 8184 this.minimumId = castToString(value); // StringType 8185 return value; 8186 case 1001488901: // navigationLinks 8187 this.navigationLinks = castToBoolean(value); // BooleanType 8188 return value; 8189 case -500553564: // operator 8190 value = new AssertionOperatorTypeEnumFactory().fromType(castToCode(value)); 8191 this.operator = (Enumeration) value; // Enumeration<AssertionOperatorType> 8192 return value; 8193 case 3433509: // path 8194 this.path = castToString(value); // StringType 8195 return value; 8196 case 1217874000: // requestMethod 8197 value = new TestScriptRequestMethodCodeEnumFactory().fromType(castToCode(value)); 8198 this.requestMethod = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 8199 return value; 8200 case 37099616: // requestURL 8201 this.requestURL = castToString(value); // StringType 8202 return value; 8203 case -341064690: // resource 8204 this.resource = castToCode(value); // CodeType 8205 return value; 8206 case -340323263: // response 8207 value = new AssertionResponseTypesEnumFactory().fromType(castToCode(value)); 8208 this.response = (Enumeration) value; // Enumeration<AssertionResponseTypes> 8209 return value; 8210 case 1438723534: // responseCode 8211 this.responseCode = castToString(value); // StringType 8212 return value; 8213 case 1746327190: // sourceId 8214 this.sourceId = castToId(value); // IdType 8215 return value; 8216 case 1555541038: // validateProfileId 8217 this.validateProfileId = castToId(value); // IdType 8218 return value; 8219 case 111972721: // value 8220 this.value = castToString(value); // StringType 8221 return value; 8222 case -481159832: // warningOnly 8223 this.warningOnly = castToBoolean(value); // BooleanType 8224 return value; 8225 default: 8226 return super.setProperty(hash, name, value); 8227 } 8228 8229 } 8230 8231 @Override 8232 public Base setProperty(String name, Base value) throws FHIRException { 8233 if (name.equals("label")) { 8234 this.label = castToString(value); // StringType 8235 } else if (name.equals("description")) { 8236 this.description = castToString(value); // StringType 8237 } else if (name.equals("direction")) { 8238 value = new AssertionDirectionTypeEnumFactory().fromType(castToCode(value)); 8239 this.direction = (Enumeration) value; // Enumeration<AssertionDirectionType> 8240 } else if (name.equals("compareToSourceId")) { 8241 this.compareToSourceId = castToString(value); // StringType 8242 } else if (name.equals("compareToSourceExpression")) { 8243 this.compareToSourceExpression = castToString(value); // StringType 8244 } else if (name.equals("compareToSourcePath")) { 8245 this.compareToSourcePath = castToString(value); // StringType 8246 } else if (name.equals("contentType")) { 8247 this.contentType = castToCode(value); // CodeType 8248 } else if (name.equals("expression")) { 8249 this.expression = castToString(value); // StringType 8250 } else if (name.equals("headerField")) { 8251 this.headerField = castToString(value); // StringType 8252 } else if (name.equals("minimumId")) { 8253 this.minimumId = castToString(value); // StringType 8254 } else if (name.equals("navigationLinks")) { 8255 this.navigationLinks = castToBoolean(value); // BooleanType 8256 } else if (name.equals("operator")) { 8257 value = new AssertionOperatorTypeEnumFactory().fromType(castToCode(value)); 8258 this.operator = (Enumeration) value; // Enumeration<AssertionOperatorType> 8259 } else if (name.equals("path")) { 8260 this.path = castToString(value); // StringType 8261 } else if (name.equals("requestMethod")) { 8262 value = new TestScriptRequestMethodCodeEnumFactory().fromType(castToCode(value)); 8263 this.requestMethod = (Enumeration) value; // Enumeration<TestScriptRequestMethodCode> 8264 } else if (name.equals("requestURL")) { 8265 this.requestURL = castToString(value); // StringType 8266 } else if (name.equals("resource")) { 8267 this.resource = castToCode(value); // CodeType 8268 } else if (name.equals("response")) { 8269 value = new AssertionResponseTypesEnumFactory().fromType(castToCode(value)); 8270 this.response = (Enumeration) value; // Enumeration<AssertionResponseTypes> 8271 } else if (name.equals("responseCode")) { 8272 this.responseCode = castToString(value); // StringType 8273 } else if (name.equals("sourceId")) { 8274 this.sourceId = castToId(value); // IdType 8275 } else if (name.equals("validateProfileId")) { 8276 this.validateProfileId = castToId(value); // IdType 8277 } else if (name.equals("value")) { 8278 this.value = castToString(value); // StringType 8279 } else if (name.equals("warningOnly")) { 8280 this.warningOnly = castToBoolean(value); // BooleanType 8281 } else 8282 return super.setProperty(name, value); 8283 return value; 8284 } 8285 8286 @Override 8287 public void removeChild(String name, Base value) throws FHIRException { 8288 if (name.equals("label")) { 8289 this.label = null; 8290 } else if (name.equals("description")) { 8291 this.description = null; 8292 } else if (name.equals("direction")) { 8293 this.direction = null; 8294 } else if (name.equals("compareToSourceId")) { 8295 this.compareToSourceId = null; 8296 } else if (name.equals("compareToSourceExpression")) { 8297 this.compareToSourceExpression = null; 8298 } else if (name.equals("compareToSourcePath")) { 8299 this.compareToSourcePath = null; 8300 } else if (name.equals("contentType")) { 8301 this.contentType = null; 8302 } else if (name.equals("expression")) { 8303 this.expression = null; 8304 } else if (name.equals("headerField")) { 8305 this.headerField = null; 8306 } else if (name.equals("minimumId")) { 8307 this.minimumId = null; 8308 } else if (name.equals("navigationLinks")) { 8309 this.navigationLinks = null; 8310 } else if (name.equals("operator")) { 8311 this.operator = null; 8312 } else if (name.equals("path")) { 8313 this.path = null; 8314 } else if (name.equals("requestMethod")) { 8315 this.requestMethod = null; 8316 } else if (name.equals("requestURL")) { 8317 this.requestURL = null; 8318 } else if (name.equals("resource")) { 8319 this.resource = null; 8320 } else if (name.equals("response")) { 8321 this.response = null; 8322 } else if (name.equals("responseCode")) { 8323 this.responseCode = null; 8324 } else if (name.equals("sourceId")) { 8325 this.sourceId = null; 8326 } else if (name.equals("validateProfileId")) { 8327 this.validateProfileId = null; 8328 } else if (name.equals("value")) { 8329 this.value = null; 8330 } else if (name.equals("warningOnly")) { 8331 this.warningOnly = null; 8332 } else 8333 super.removeChild(name, value); 8334 8335 } 8336 8337 @Override 8338 public Base makeProperty(int hash, String name) throws FHIRException { 8339 switch (hash) { 8340 case 102727412: 8341 return getLabelElement(); 8342 case -1724546052: 8343 return getDescriptionElement(); 8344 case -962590849: 8345 return getDirectionElement(); 8346 case 2081856758: 8347 return getCompareToSourceIdElement(); 8348 case -1415702669: 8349 return getCompareToSourceExpressionElement(); 8350 case -790206144: 8351 return getCompareToSourcePathElement(); 8352 case -389131437: 8353 return getContentTypeElement(); 8354 case -1795452264: 8355 return getExpressionElement(); 8356 case 1160732269: 8357 return getHeaderFieldElement(); 8358 case 818925001: 8359 return getMinimumIdElement(); 8360 case 1001488901: 8361 return getNavigationLinksElement(); 8362 case -500553564: 8363 return getOperatorElement(); 8364 case 3433509: 8365 return getPathElement(); 8366 case 1217874000: 8367 return getRequestMethodElement(); 8368 case 37099616: 8369 return getRequestURLElement(); 8370 case -341064690: 8371 return getResourceElement(); 8372 case -340323263: 8373 return getResponseElement(); 8374 case 1438723534: 8375 return getResponseCodeElement(); 8376 case 1746327190: 8377 return getSourceIdElement(); 8378 case 1555541038: 8379 return getValidateProfileIdElement(); 8380 case 111972721: 8381 return getValueElement(); 8382 case -481159832: 8383 return getWarningOnlyElement(); 8384 default: 8385 return super.makeProperty(hash, name); 8386 } 8387 8388 } 8389 8390 @Override 8391 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8392 switch (hash) { 8393 case 102727412: 8394 /* label */ return new String[] { "string" }; 8395 case -1724546052: 8396 /* description */ return new String[] { "string" }; 8397 case -962590849: 8398 /* direction */ return new String[] { "code" }; 8399 case 2081856758: 8400 /* compareToSourceId */ return new String[] { "string" }; 8401 case -1415702669: 8402 /* compareToSourceExpression */ return new String[] { "string" }; 8403 case -790206144: 8404 /* compareToSourcePath */ return new String[] { "string" }; 8405 case -389131437: 8406 /* contentType */ return new String[] { "code" }; 8407 case -1795452264: 8408 /* expression */ return new String[] { "string" }; 8409 case 1160732269: 8410 /* headerField */ return new String[] { "string" }; 8411 case 818925001: 8412 /* minimumId */ return new String[] { "string" }; 8413 case 1001488901: 8414 /* navigationLinks */ return new String[] { "boolean" }; 8415 case -500553564: 8416 /* operator */ return new String[] { "code" }; 8417 case 3433509: 8418 /* path */ return new String[] { "string" }; 8419 case 1217874000: 8420 /* requestMethod */ return new String[] { "code" }; 8421 case 37099616: 8422 /* requestURL */ return new String[] { "string" }; 8423 case -341064690: 8424 /* resource */ return new String[] { "code" }; 8425 case -340323263: 8426 /* response */ return new String[] { "code" }; 8427 case 1438723534: 8428 /* responseCode */ return new String[] { "string" }; 8429 case 1746327190: 8430 /* sourceId */ return new String[] { "id" }; 8431 case 1555541038: 8432 /* validateProfileId */ return new String[] { "id" }; 8433 case 111972721: 8434 /* value */ return new String[] { "string" }; 8435 case -481159832: 8436 /* warningOnly */ return new String[] { "boolean" }; 8437 default: 8438 return super.getTypesForProperty(hash, name); 8439 } 8440 8441 } 8442 8443 @Override 8444 public Base addChild(String name) throws FHIRException { 8445 if (name.equals("label")) { 8446 throw new FHIRException("Cannot call addChild on a singleton property TestScript.label"); 8447 } else if (name.equals("description")) { 8448 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 8449 } else if (name.equals("direction")) { 8450 throw new FHIRException("Cannot call addChild on a singleton property TestScript.direction"); 8451 } else if (name.equals("compareToSourceId")) { 8452 throw new FHIRException("Cannot call addChild on a singleton property TestScript.compareToSourceId"); 8453 } else if (name.equals("compareToSourceExpression")) { 8454 throw new FHIRException("Cannot call addChild on a singleton property TestScript.compareToSourceExpression"); 8455 } else if (name.equals("compareToSourcePath")) { 8456 throw new FHIRException("Cannot call addChild on a singleton property TestScript.compareToSourcePath"); 8457 } else if (name.equals("contentType")) { 8458 throw new FHIRException("Cannot call addChild on a singleton property TestScript.contentType"); 8459 } else if (name.equals("expression")) { 8460 throw new FHIRException("Cannot call addChild on a singleton property TestScript.expression"); 8461 } else if (name.equals("headerField")) { 8462 throw new FHIRException("Cannot call addChild on a singleton property TestScript.headerField"); 8463 } else if (name.equals("minimumId")) { 8464 throw new FHIRException("Cannot call addChild on a singleton property TestScript.minimumId"); 8465 } else if (name.equals("navigationLinks")) { 8466 throw new FHIRException("Cannot call addChild on a singleton property TestScript.navigationLinks"); 8467 } else if (name.equals("operator")) { 8468 throw new FHIRException("Cannot call addChild on a singleton property TestScript.operator"); 8469 } else if (name.equals("path")) { 8470 throw new FHIRException("Cannot call addChild on a singleton property TestScript.path"); 8471 } else if (name.equals("requestMethod")) { 8472 throw new FHIRException("Cannot call addChild on a singleton property TestScript.requestMethod"); 8473 } else if (name.equals("requestURL")) { 8474 throw new FHIRException("Cannot call addChild on a singleton property TestScript.requestURL"); 8475 } else if (name.equals("resource")) { 8476 throw new FHIRException("Cannot call addChild on a singleton property TestScript.resource"); 8477 } else if (name.equals("response")) { 8478 throw new FHIRException("Cannot call addChild on a singleton property TestScript.response"); 8479 } else if (name.equals("responseCode")) { 8480 throw new FHIRException("Cannot call addChild on a singleton property TestScript.responseCode"); 8481 } else if (name.equals("sourceId")) { 8482 throw new FHIRException("Cannot call addChild on a singleton property TestScript.sourceId"); 8483 } else if (name.equals("validateProfileId")) { 8484 throw new FHIRException("Cannot call addChild on a singleton property TestScript.validateProfileId"); 8485 } else if (name.equals("value")) { 8486 throw new FHIRException("Cannot call addChild on a singleton property TestScript.value"); 8487 } else if (name.equals("warningOnly")) { 8488 throw new FHIRException("Cannot call addChild on a singleton property TestScript.warningOnly"); 8489 } else 8490 return super.addChild(name); 8491 } 8492 8493 public SetupActionAssertComponent copy() { 8494 SetupActionAssertComponent dst = new SetupActionAssertComponent(); 8495 copyValues(dst); 8496 return dst; 8497 } 8498 8499 public void copyValues(SetupActionAssertComponent dst) { 8500 super.copyValues(dst); 8501 dst.label = label == null ? null : label.copy(); 8502 dst.description = description == null ? null : description.copy(); 8503 dst.direction = direction == null ? null : direction.copy(); 8504 dst.compareToSourceId = compareToSourceId == null ? null : compareToSourceId.copy(); 8505 dst.compareToSourceExpression = compareToSourceExpression == null ? null : compareToSourceExpression.copy(); 8506 dst.compareToSourcePath = compareToSourcePath == null ? null : compareToSourcePath.copy(); 8507 dst.contentType = contentType == null ? null : contentType.copy(); 8508 dst.expression = expression == null ? null : expression.copy(); 8509 dst.headerField = headerField == null ? null : headerField.copy(); 8510 dst.minimumId = minimumId == null ? null : minimumId.copy(); 8511 dst.navigationLinks = navigationLinks == null ? null : navigationLinks.copy(); 8512 dst.operator = operator == null ? null : operator.copy(); 8513 dst.path = path == null ? null : path.copy(); 8514 dst.requestMethod = requestMethod == null ? null : requestMethod.copy(); 8515 dst.requestURL = requestURL == null ? null : requestURL.copy(); 8516 dst.resource = resource == null ? null : resource.copy(); 8517 dst.response = response == null ? null : response.copy(); 8518 dst.responseCode = responseCode == null ? null : responseCode.copy(); 8519 dst.sourceId = sourceId == null ? null : sourceId.copy(); 8520 dst.validateProfileId = validateProfileId == null ? null : validateProfileId.copy(); 8521 dst.value = value == null ? null : value.copy(); 8522 dst.warningOnly = warningOnly == null ? null : warningOnly.copy(); 8523 } 8524 8525 @Override 8526 public boolean equalsDeep(Base other_) { 8527 if (!super.equalsDeep(other_)) 8528 return false; 8529 if (!(other_ instanceof SetupActionAssertComponent)) 8530 return false; 8531 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 8532 return compareDeep(label, o.label, true) && compareDeep(description, o.description, true) 8533 && compareDeep(direction, o.direction, true) && compareDeep(compareToSourceId, o.compareToSourceId, true) 8534 && compareDeep(compareToSourceExpression, o.compareToSourceExpression, true) 8535 && compareDeep(compareToSourcePath, o.compareToSourcePath, true) 8536 && compareDeep(contentType, o.contentType, true) && compareDeep(expression, o.expression, true) 8537 && compareDeep(headerField, o.headerField, true) && compareDeep(minimumId, o.minimumId, true) 8538 && compareDeep(navigationLinks, o.navigationLinks, true) && compareDeep(operator, o.operator, true) 8539 && compareDeep(path, o.path, true) && compareDeep(requestMethod, o.requestMethod, true) 8540 && compareDeep(requestURL, o.requestURL, true) && compareDeep(resource, o.resource, true) 8541 && compareDeep(response, o.response, true) && compareDeep(responseCode, o.responseCode, true) 8542 && compareDeep(sourceId, o.sourceId, true) && compareDeep(validateProfileId, o.validateProfileId, true) 8543 && compareDeep(value, o.value, true) && compareDeep(warningOnly, o.warningOnly, true); 8544 } 8545 8546 @Override 8547 public boolean equalsShallow(Base other_) { 8548 if (!super.equalsShallow(other_)) 8549 return false; 8550 if (!(other_ instanceof SetupActionAssertComponent)) 8551 return false; 8552 SetupActionAssertComponent o = (SetupActionAssertComponent) other_; 8553 return compareValues(label, o.label, true) && compareValues(description, o.description, true) 8554 && compareValues(direction, o.direction, true) && compareValues(compareToSourceId, o.compareToSourceId, true) 8555 && compareValues(compareToSourceExpression, o.compareToSourceExpression, true) 8556 && compareValues(compareToSourcePath, o.compareToSourcePath, true) 8557 && compareValues(contentType, o.contentType, true) && compareValues(expression, o.expression, true) 8558 && compareValues(headerField, o.headerField, true) && compareValues(minimumId, o.minimumId, true) 8559 && compareValues(navigationLinks, o.navigationLinks, true) && compareValues(operator, o.operator, true) 8560 && compareValues(path, o.path, true) && compareValues(requestMethod, o.requestMethod, true) 8561 && compareValues(requestURL, o.requestURL, true) && compareValues(resource, o.resource, true) 8562 && compareValues(response, o.response, true) && compareValues(responseCode, o.responseCode, true) 8563 && compareValues(sourceId, o.sourceId, true) && compareValues(validateProfileId, o.validateProfileId, true) 8564 && compareValues(value, o.value, true) && compareValues(warningOnly, o.warningOnly, true); 8565 } 8566 8567 public boolean isEmpty() { 8568 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, description, direction, compareToSourceId, 8569 compareToSourceExpression, compareToSourcePath, contentType, expression, headerField, minimumId, 8570 navigationLinks, operator, path, requestMethod, requestURL, resource, response, responseCode, sourceId, 8571 validateProfileId, value, warningOnly); 8572 } 8573 8574 public String fhirType() { 8575 return "TestScript.setup.action.assert"; 8576 8577 } 8578 8579 } 8580 8581 @Block() 8582 public static class TestScriptTestComponent extends BackboneElement implements IBaseBackboneElement { 8583 /** 8584 * The name of this test used for tracking/logging purposes by test engines. 8585 */ 8586 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 8587 @Description(shortDefinition = "Tracking/logging name of this test", formalDefinition = "The name of this test used for tracking/logging purposes by test engines.") 8588 protected StringType name; 8589 8590 /** 8591 * A short description of the test used by test engines for tracking and 8592 * reporting purposes. 8593 */ 8594 @Child(name = "description", type = { 8595 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 8596 @Description(shortDefinition = "Tracking/reporting short description of the test", formalDefinition = "A short description of the test used by test engines for tracking and reporting purposes.") 8597 protected StringType description; 8598 8599 /** 8600 * Action would contain either an operation or an assertion. 8601 */ 8602 @Child(name = "action", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8603 @Description(shortDefinition = "A test operation or assert to perform", formalDefinition = "Action would contain either an operation or an assertion.") 8604 protected List<TestActionComponent> action; 8605 8606 private static final long serialVersionUID = -865006110L; 8607 8608 /** 8609 * Constructor 8610 */ 8611 public TestScriptTestComponent() { 8612 super(); 8613 } 8614 8615 /** 8616 * @return {@link #name} (The name of this test used for tracking/logging 8617 * purposes by test engines.). This is the underlying object with id, 8618 * value and extensions. The accessor "getName" gives direct access to 8619 * the value 8620 */ 8621 public StringType getNameElement() { 8622 if (this.name == null) 8623 if (Configuration.errorOnAutoCreate()) 8624 throw new Error("Attempt to auto-create TestScriptTestComponent.name"); 8625 else if (Configuration.doAutoCreate()) 8626 this.name = new StringType(); // bb 8627 return this.name; 8628 } 8629 8630 public boolean hasNameElement() { 8631 return this.name != null && !this.name.isEmpty(); 8632 } 8633 8634 public boolean hasName() { 8635 return this.name != null && !this.name.isEmpty(); 8636 } 8637 8638 /** 8639 * @param value {@link #name} (The name of this test used for tracking/logging 8640 * purposes by test engines.). This is the underlying object with 8641 * id, value and extensions. The accessor "getName" gives direct 8642 * access to the value 8643 */ 8644 public TestScriptTestComponent setNameElement(StringType value) { 8645 this.name = value; 8646 return this; 8647 } 8648 8649 /** 8650 * @return The name of this test used for tracking/logging purposes by test 8651 * engines. 8652 */ 8653 public String getName() { 8654 return this.name == null ? null : this.name.getValue(); 8655 } 8656 8657 /** 8658 * @param value The name of this test used for tracking/logging purposes by test 8659 * engines. 8660 */ 8661 public TestScriptTestComponent setName(String value) { 8662 if (Utilities.noString(value)) 8663 this.name = null; 8664 else { 8665 if (this.name == null) 8666 this.name = new StringType(); 8667 this.name.setValue(value); 8668 } 8669 return this; 8670 } 8671 8672 /** 8673 * @return {@link #description} (A short description of the test used by test 8674 * engines for tracking and reporting purposes.). This is the underlying 8675 * object with id, value and extensions. The accessor "getDescription" 8676 * gives direct access to the value 8677 */ 8678 public StringType getDescriptionElement() { 8679 if (this.description == null) 8680 if (Configuration.errorOnAutoCreate()) 8681 throw new Error("Attempt to auto-create TestScriptTestComponent.description"); 8682 else if (Configuration.doAutoCreate()) 8683 this.description = new StringType(); // bb 8684 return this.description; 8685 } 8686 8687 public boolean hasDescriptionElement() { 8688 return this.description != null && !this.description.isEmpty(); 8689 } 8690 8691 public boolean hasDescription() { 8692 return this.description != null && !this.description.isEmpty(); 8693 } 8694 8695 /** 8696 * @param value {@link #description} (A short description of the test used by 8697 * test engines for tracking and reporting purposes.). This is the 8698 * underlying object with id, value and extensions. The accessor 8699 * "getDescription" gives direct access to the value 8700 */ 8701 public TestScriptTestComponent setDescriptionElement(StringType value) { 8702 this.description = value; 8703 return this; 8704 } 8705 8706 /** 8707 * @return A short description of the test used by test engines for tracking and 8708 * reporting purposes. 8709 */ 8710 public String getDescription() { 8711 return this.description == null ? null : this.description.getValue(); 8712 } 8713 8714 /** 8715 * @param value A short description of the test used by test engines for 8716 * tracking and reporting purposes. 8717 */ 8718 public TestScriptTestComponent setDescription(String value) { 8719 if (Utilities.noString(value)) 8720 this.description = null; 8721 else { 8722 if (this.description == null) 8723 this.description = new StringType(); 8724 this.description.setValue(value); 8725 } 8726 return this; 8727 } 8728 8729 /** 8730 * @return {@link #action} (Action would contain either an operation or an 8731 * assertion.) 8732 */ 8733 public List<TestActionComponent> getAction() { 8734 if (this.action == null) 8735 this.action = new ArrayList<TestActionComponent>(); 8736 return this.action; 8737 } 8738 8739 /** 8740 * @return Returns a reference to <code>this</code> for easy method chaining 8741 */ 8742 public TestScriptTestComponent setAction(List<TestActionComponent> theAction) { 8743 this.action = theAction; 8744 return this; 8745 } 8746 8747 public boolean hasAction() { 8748 if (this.action == null) 8749 return false; 8750 for (TestActionComponent item : this.action) 8751 if (!item.isEmpty()) 8752 return true; 8753 return false; 8754 } 8755 8756 public TestActionComponent addAction() { // 3 8757 TestActionComponent t = new TestActionComponent(); 8758 if (this.action == null) 8759 this.action = new ArrayList<TestActionComponent>(); 8760 this.action.add(t); 8761 return t; 8762 } 8763 8764 public TestScriptTestComponent addAction(TestActionComponent t) { // 3 8765 if (t == null) 8766 return this; 8767 if (this.action == null) 8768 this.action = new ArrayList<TestActionComponent>(); 8769 this.action.add(t); 8770 return this; 8771 } 8772 8773 /** 8774 * @return The first repetition of repeating field {@link #action}, creating it 8775 * if it does not already exist 8776 */ 8777 public TestActionComponent getActionFirstRep() { 8778 if (getAction().isEmpty()) { 8779 addAction(); 8780 } 8781 return getAction().get(0); 8782 } 8783 8784 protected void listChildren(List<Property> children) { 8785 super.listChildren(children); 8786 children.add(new Property("name", "string", 8787 "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name)); 8788 children.add(new Property("description", "string", 8789 "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, 8790 description)); 8791 children.add(new Property("action", "", "Action would contain either an operation or an assertion.", 0, 8792 java.lang.Integer.MAX_VALUE, action)); 8793 } 8794 8795 @Override 8796 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8797 switch (_hash) { 8798 case 3373707: 8799 /* name */ return new Property("name", "string", 8800 "The name of this test used for tracking/logging purposes by test engines.", 0, 1, name); 8801 case -1724546052: 8802 /* description */ return new Property("description", "string", 8803 "A short description of the test used by test engines for tracking and reporting purposes.", 0, 1, 8804 description); 8805 case -1422950858: 8806 /* action */ return new Property("action", "", "Action would contain either an operation or an assertion.", 0, 8807 java.lang.Integer.MAX_VALUE, action); 8808 default: 8809 return super.getNamedProperty(_hash, _name, _checkValid); 8810 } 8811 8812 } 8813 8814 @Override 8815 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8816 switch (hash) { 8817 case 3373707: 8818 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 8819 case -1724546052: 8820 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 8821 case -1422950858: 8822 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TestActionComponent 8823 default: 8824 return super.getProperty(hash, name, checkValid); 8825 } 8826 8827 } 8828 8829 @Override 8830 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8831 switch (hash) { 8832 case 3373707: // name 8833 this.name = castToString(value); // StringType 8834 return value; 8835 case -1724546052: // description 8836 this.description = castToString(value); // StringType 8837 return value; 8838 case -1422950858: // action 8839 this.getAction().add((TestActionComponent) value); // TestActionComponent 8840 return value; 8841 default: 8842 return super.setProperty(hash, name, value); 8843 } 8844 8845 } 8846 8847 @Override 8848 public Base setProperty(String name, Base value) throws FHIRException { 8849 if (name.equals("name")) { 8850 this.name = castToString(value); // StringType 8851 } else if (name.equals("description")) { 8852 this.description = castToString(value); // StringType 8853 } else if (name.equals("action")) { 8854 this.getAction().add((TestActionComponent) value); 8855 } else 8856 return super.setProperty(name, value); 8857 return value; 8858 } 8859 8860 @Override 8861 public void removeChild(String name, Base value) throws FHIRException { 8862 if (name.equals("name")) { 8863 this.name = null; 8864 } else if (name.equals("description")) { 8865 this.description = null; 8866 } else if (name.equals("action")) { 8867 this.getAction().remove((TestActionComponent) value); 8868 } else 8869 super.removeChild(name, value); 8870 8871 } 8872 8873 @Override 8874 public Base makeProperty(int hash, String name) throws FHIRException { 8875 switch (hash) { 8876 case 3373707: 8877 return getNameElement(); 8878 case -1724546052: 8879 return getDescriptionElement(); 8880 case -1422950858: 8881 return addAction(); 8882 default: 8883 return super.makeProperty(hash, name); 8884 } 8885 8886 } 8887 8888 @Override 8889 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8890 switch (hash) { 8891 case 3373707: 8892 /* name */ return new String[] { "string" }; 8893 case -1724546052: 8894 /* description */ return new String[] { "string" }; 8895 case -1422950858: 8896 /* action */ return new String[] {}; 8897 default: 8898 return super.getTypesForProperty(hash, name); 8899 } 8900 8901 } 8902 8903 @Override 8904 public Base addChild(String name) throws FHIRException { 8905 if (name.equals("name")) { 8906 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 8907 } else if (name.equals("description")) { 8908 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 8909 } else if (name.equals("action")) { 8910 return addAction(); 8911 } else 8912 return super.addChild(name); 8913 } 8914 8915 public TestScriptTestComponent copy() { 8916 TestScriptTestComponent dst = new TestScriptTestComponent(); 8917 copyValues(dst); 8918 return dst; 8919 } 8920 8921 public void copyValues(TestScriptTestComponent dst) { 8922 super.copyValues(dst); 8923 dst.name = name == null ? null : name.copy(); 8924 dst.description = description == null ? null : description.copy(); 8925 if (action != null) { 8926 dst.action = new ArrayList<TestActionComponent>(); 8927 for (TestActionComponent i : action) 8928 dst.action.add(i.copy()); 8929 } 8930 ; 8931 } 8932 8933 @Override 8934 public boolean equalsDeep(Base other_) { 8935 if (!super.equalsDeep(other_)) 8936 return false; 8937 if (!(other_ instanceof TestScriptTestComponent)) 8938 return false; 8939 TestScriptTestComponent o = (TestScriptTestComponent) other_; 8940 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 8941 && compareDeep(action, o.action, true); 8942 } 8943 8944 @Override 8945 public boolean equalsShallow(Base other_) { 8946 if (!super.equalsShallow(other_)) 8947 return false; 8948 if (!(other_ instanceof TestScriptTestComponent)) 8949 return false; 8950 TestScriptTestComponent o = (TestScriptTestComponent) other_; 8951 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 8952 } 8953 8954 public boolean isEmpty() { 8955 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, action); 8956 } 8957 8958 public String fhirType() { 8959 return "TestScript.test"; 8960 8961 } 8962 8963 } 8964 8965 @Block() 8966 public static class TestActionComponent extends BackboneElement implements IBaseBackboneElement { 8967 /** 8968 * An operation would involve a REST request to a server. 8969 */ 8970 @Child(name = "operation", type = { 8971 SetupActionOperationComponent.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 8972 @Description(shortDefinition = "The setup operation to perform", formalDefinition = "An operation would involve a REST request to a server.") 8973 protected SetupActionOperationComponent operation; 8974 8975 /** 8976 * Evaluates the results of previous operations to determine if the server under 8977 * test behaves appropriately. 8978 */ 8979 @Child(name = "assert", type = { 8980 SetupActionAssertComponent.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 8981 @Description(shortDefinition = "The setup assertion to perform", formalDefinition = "Evaluates the results of previous operations to determine if the server under test behaves appropriately.") 8982 protected SetupActionAssertComponent assert_; 8983 8984 private static final long serialVersionUID = -252088305L; 8985 8986 /** 8987 * Constructor 8988 */ 8989 public TestActionComponent() { 8990 super(); 8991 } 8992 8993 /** 8994 * @return {@link #operation} (An operation would involve a REST request to a 8995 * server.) 8996 */ 8997 public SetupActionOperationComponent getOperation() { 8998 if (this.operation == null) 8999 if (Configuration.errorOnAutoCreate()) 9000 throw new Error("Attempt to auto-create TestActionComponent.operation"); 9001 else if (Configuration.doAutoCreate()) 9002 this.operation = new SetupActionOperationComponent(); // cc 9003 return this.operation; 9004 } 9005 9006 public boolean hasOperation() { 9007 return this.operation != null && !this.operation.isEmpty(); 9008 } 9009 9010 /** 9011 * @param value {@link #operation} (An operation would involve a REST request to 9012 * a server.) 9013 */ 9014 public TestActionComponent setOperation(SetupActionOperationComponent value) { 9015 this.operation = value; 9016 return this; 9017 } 9018 9019 /** 9020 * @return {@link #assert_} (Evaluates the results of previous operations to 9021 * determine if the server under test behaves appropriately.) 9022 */ 9023 public SetupActionAssertComponent getAssert() { 9024 if (this.assert_ == null) 9025 if (Configuration.errorOnAutoCreate()) 9026 throw new Error("Attempt to auto-create TestActionComponent.assert_"); 9027 else if (Configuration.doAutoCreate()) 9028 this.assert_ = new SetupActionAssertComponent(); // cc 9029 return this.assert_; 9030 } 9031 9032 public boolean hasAssert() { 9033 return this.assert_ != null && !this.assert_.isEmpty(); 9034 } 9035 9036 /** 9037 * @param value {@link #assert_} (Evaluates the results of previous operations 9038 * to determine if the server under test behaves appropriately.) 9039 */ 9040 public TestActionComponent setAssert(SetupActionAssertComponent value) { 9041 this.assert_ = value; 9042 return this; 9043 } 9044 9045 protected void listChildren(List<Property> children) { 9046 super.listChildren(children); 9047 children.add(new Property("operation", "@TestScript.setup.action.operation", 9048 "An operation would involve a REST request to a server.", 0, 1, operation)); 9049 children.add(new Property("assert", "@TestScript.setup.action.assert", 9050 "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 9051 0, 1, assert_)); 9052 } 9053 9054 @Override 9055 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9056 switch (_hash) { 9057 case 1662702951: 9058 /* operation */ return new Property("operation", "@TestScript.setup.action.operation", 9059 "An operation would involve a REST request to a server.", 0, 1, operation); 9060 case -1408208058: 9061 /* assert */ return new Property("assert", "@TestScript.setup.action.assert", 9062 "Evaluates the results of previous operations to determine if the server under test behaves appropriately.", 9063 0, 1, assert_); 9064 default: 9065 return super.getNamedProperty(_hash, _name, _checkValid); 9066 } 9067 9068 } 9069 9070 @Override 9071 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9072 switch (hash) { 9073 case 1662702951: 9074 /* operation */ return this.operation == null ? new Base[0] : new Base[] { this.operation }; // SetupActionOperationComponent 9075 case -1408208058: 9076 /* assert */ return this.assert_ == null ? new Base[0] : new Base[] { this.assert_ }; // SetupActionAssertComponent 9077 default: 9078 return super.getProperty(hash, name, checkValid); 9079 } 9080 9081 } 9082 9083 @Override 9084 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9085 switch (hash) { 9086 case 1662702951: // operation 9087 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9088 return value; 9089 case -1408208058: // assert 9090 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 9091 return value; 9092 default: 9093 return super.setProperty(hash, name, value); 9094 } 9095 9096 } 9097 9098 @Override 9099 public Base setProperty(String name, Base value) throws FHIRException { 9100 if (name.equals("operation")) { 9101 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9102 } else if (name.equals("assert")) { 9103 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 9104 } else 9105 return super.setProperty(name, value); 9106 return value; 9107 } 9108 9109 @Override 9110 public void removeChild(String name, Base value) throws FHIRException { 9111 if (name.equals("operation")) { 9112 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9113 } else if (name.equals("assert")) { 9114 this.assert_ = (SetupActionAssertComponent) value; // SetupActionAssertComponent 9115 } else 9116 super.removeChild(name, value); 9117 9118 } 9119 9120 @Override 9121 public Base makeProperty(int hash, String name) throws FHIRException { 9122 switch (hash) { 9123 case 1662702951: 9124 return getOperation(); 9125 case -1408208058: 9126 return getAssert(); 9127 default: 9128 return super.makeProperty(hash, name); 9129 } 9130 9131 } 9132 9133 @Override 9134 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9135 switch (hash) { 9136 case 1662702951: 9137 /* operation */ return new String[] { "@TestScript.setup.action.operation" }; 9138 case -1408208058: 9139 /* assert */ return new String[] { "@TestScript.setup.action.assert" }; 9140 default: 9141 return super.getTypesForProperty(hash, name); 9142 } 9143 9144 } 9145 9146 @Override 9147 public Base addChild(String name) throws FHIRException { 9148 if (name.equals("operation")) { 9149 this.operation = new SetupActionOperationComponent(); 9150 return this.operation; 9151 } else if (name.equals("assert")) { 9152 this.assert_ = new SetupActionAssertComponent(); 9153 return this.assert_; 9154 } else 9155 return super.addChild(name); 9156 } 9157 9158 public TestActionComponent copy() { 9159 TestActionComponent dst = new TestActionComponent(); 9160 copyValues(dst); 9161 return dst; 9162 } 9163 9164 public void copyValues(TestActionComponent dst) { 9165 super.copyValues(dst); 9166 dst.operation = operation == null ? null : operation.copy(); 9167 dst.assert_ = assert_ == null ? null : assert_.copy(); 9168 } 9169 9170 @Override 9171 public boolean equalsDeep(Base other_) { 9172 if (!super.equalsDeep(other_)) 9173 return false; 9174 if (!(other_ instanceof TestActionComponent)) 9175 return false; 9176 TestActionComponent o = (TestActionComponent) other_; 9177 return compareDeep(operation, o.operation, true) && compareDeep(assert_, o.assert_, true); 9178 } 9179 9180 @Override 9181 public boolean equalsShallow(Base other_) { 9182 if (!super.equalsShallow(other_)) 9183 return false; 9184 if (!(other_ instanceof TestActionComponent)) 9185 return false; 9186 TestActionComponent o = (TestActionComponent) other_; 9187 return true; 9188 } 9189 9190 public boolean isEmpty() { 9191 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation, assert_); 9192 } 9193 9194 public String fhirType() { 9195 return "TestScript.test.action"; 9196 9197 } 9198 9199 } 9200 9201 @Block() 9202 public static class TestScriptTeardownComponent extends BackboneElement implements IBaseBackboneElement { 9203 /** 9204 * The teardown action will only contain an operation. 9205 */ 9206 @Child(name = "action", type = {}, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9207 @Description(shortDefinition = "One or more teardown operations to perform", formalDefinition = "The teardown action will only contain an operation.") 9208 protected List<TeardownActionComponent> action; 9209 9210 private static final long serialVersionUID = 1168638089L; 9211 9212 /** 9213 * Constructor 9214 */ 9215 public TestScriptTeardownComponent() { 9216 super(); 9217 } 9218 9219 /** 9220 * @return {@link #action} (The teardown action will only contain an operation.) 9221 */ 9222 public List<TeardownActionComponent> getAction() { 9223 if (this.action == null) 9224 this.action = new ArrayList<TeardownActionComponent>(); 9225 return this.action; 9226 } 9227 9228 /** 9229 * @return Returns a reference to <code>this</code> for easy method chaining 9230 */ 9231 public TestScriptTeardownComponent setAction(List<TeardownActionComponent> theAction) { 9232 this.action = theAction; 9233 return this; 9234 } 9235 9236 public boolean hasAction() { 9237 if (this.action == null) 9238 return false; 9239 for (TeardownActionComponent item : this.action) 9240 if (!item.isEmpty()) 9241 return true; 9242 return false; 9243 } 9244 9245 public TeardownActionComponent addAction() { // 3 9246 TeardownActionComponent t = new TeardownActionComponent(); 9247 if (this.action == null) 9248 this.action = new ArrayList<TeardownActionComponent>(); 9249 this.action.add(t); 9250 return t; 9251 } 9252 9253 public TestScriptTeardownComponent addAction(TeardownActionComponent t) { // 3 9254 if (t == null) 9255 return this; 9256 if (this.action == null) 9257 this.action = new ArrayList<TeardownActionComponent>(); 9258 this.action.add(t); 9259 return this; 9260 } 9261 9262 /** 9263 * @return The first repetition of repeating field {@link #action}, creating it 9264 * if it does not already exist 9265 */ 9266 public TeardownActionComponent getActionFirstRep() { 9267 if (getAction().isEmpty()) { 9268 addAction(); 9269 } 9270 return getAction().get(0); 9271 } 9272 9273 protected void listChildren(List<Property> children) { 9274 super.listChildren(children); 9275 children.add(new Property("action", "", "The teardown action will only contain an operation.", 0, 9276 java.lang.Integer.MAX_VALUE, action)); 9277 } 9278 9279 @Override 9280 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9281 switch (_hash) { 9282 case -1422950858: 9283 /* action */ return new Property("action", "", "The teardown action will only contain an operation.", 0, 9284 java.lang.Integer.MAX_VALUE, action); 9285 default: 9286 return super.getNamedProperty(_hash, _name, _checkValid); 9287 } 9288 9289 } 9290 9291 @Override 9292 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9293 switch (hash) { 9294 case -1422950858: 9295 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // TeardownActionComponent 9296 default: 9297 return super.getProperty(hash, name, checkValid); 9298 } 9299 9300 } 9301 9302 @Override 9303 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9304 switch (hash) { 9305 case -1422950858: // action 9306 this.getAction().add((TeardownActionComponent) value); // TeardownActionComponent 9307 return value; 9308 default: 9309 return super.setProperty(hash, name, value); 9310 } 9311 9312 } 9313 9314 @Override 9315 public Base setProperty(String name, Base value) throws FHIRException { 9316 if (name.equals("action")) { 9317 this.getAction().add((TeardownActionComponent) value); 9318 } else 9319 return super.setProperty(name, value); 9320 return value; 9321 } 9322 9323 @Override 9324 public void removeChild(String name, Base value) throws FHIRException { 9325 if (name.equals("action")) { 9326 this.getAction().remove((TeardownActionComponent) value); 9327 } else 9328 super.removeChild(name, value); 9329 9330 } 9331 9332 @Override 9333 public Base makeProperty(int hash, String name) throws FHIRException { 9334 switch (hash) { 9335 case -1422950858: 9336 return addAction(); 9337 default: 9338 return super.makeProperty(hash, name); 9339 } 9340 9341 } 9342 9343 @Override 9344 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9345 switch (hash) { 9346 case -1422950858: 9347 /* action */ return new String[] {}; 9348 default: 9349 return super.getTypesForProperty(hash, name); 9350 } 9351 9352 } 9353 9354 @Override 9355 public Base addChild(String name) throws FHIRException { 9356 if (name.equals("action")) { 9357 return addAction(); 9358 } else 9359 return super.addChild(name); 9360 } 9361 9362 public TestScriptTeardownComponent copy() { 9363 TestScriptTeardownComponent dst = new TestScriptTeardownComponent(); 9364 copyValues(dst); 9365 return dst; 9366 } 9367 9368 public void copyValues(TestScriptTeardownComponent dst) { 9369 super.copyValues(dst); 9370 if (action != null) { 9371 dst.action = new ArrayList<TeardownActionComponent>(); 9372 for (TeardownActionComponent i : action) 9373 dst.action.add(i.copy()); 9374 } 9375 ; 9376 } 9377 9378 @Override 9379 public boolean equalsDeep(Base other_) { 9380 if (!super.equalsDeep(other_)) 9381 return false; 9382 if (!(other_ instanceof TestScriptTeardownComponent)) 9383 return false; 9384 TestScriptTeardownComponent o = (TestScriptTeardownComponent) other_; 9385 return compareDeep(action, o.action, true); 9386 } 9387 9388 @Override 9389 public boolean equalsShallow(Base other_) { 9390 if (!super.equalsShallow(other_)) 9391 return false; 9392 if (!(other_ instanceof TestScriptTeardownComponent)) 9393 return false; 9394 TestScriptTeardownComponent o = (TestScriptTeardownComponent) other_; 9395 return true; 9396 } 9397 9398 public boolean isEmpty() { 9399 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action); 9400 } 9401 9402 public String fhirType() { 9403 return "TestScript.teardown"; 9404 9405 } 9406 9407 } 9408 9409 @Block() 9410 public static class TeardownActionComponent extends BackboneElement implements IBaseBackboneElement { 9411 /** 9412 * An operation would involve a REST request to a server. 9413 */ 9414 @Child(name = "operation", type = { 9415 SetupActionOperationComponent.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 9416 @Description(shortDefinition = "The teardown operation to perform", formalDefinition = "An operation would involve a REST request to a server.") 9417 protected SetupActionOperationComponent operation; 9418 9419 private static final long serialVersionUID = -1099598054L; 9420 9421 /** 9422 * Constructor 9423 */ 9424 public TeardownActionComponent() { 9425 super(); 9426 } 9427 9428 /** 9429 * Constructor 9430 */ 9431 public TeardownActionComponent(SetupActionOperationComponent operation) { 9432 super(); 9433 this.operation = operation; 9434 } 9435 9436 /** 9437 * @return {@link #operation} (An operation would involve a REST request to a 9438 * server.) 9439 */ 9440 public SetupActionOperationComponent getOperation() { 9441 if (this.operation == null) 9442 if (Configuration.errorOnAutoCreate()) 9443 throw new Error("Attempt to auto-create TeardownActionComponent.operation"); 9444 else if (Configuration.doAutoCreate()) 9445 this.operation = new SetupActionOperationComponent(); // cc 9446 return this.operation; 9447 } 9448 9449 public boolean hasOperation() { 9450 return this.operation != null && !this.operation.isEmpty(); 9451 } 9452 9453 /** 9454 * @param value {@link #operation} (An operation would involve a REST request to 9455 * a server.) 9456 */ 9457 public TeardownActionComponent setOperation(SetupActionOperationComponent value) { 9458 this.operation = value; 9459 return this; 9460 } 9461 9462 protected void listChildren(List<Property> children) { 9463 super.listChildren(children); 9464 children.add(new Property("operation", "@TestScript.setup.action.operation", 9465 "An operation would involve a REST request to a server.", 0, 1, operation)); 9466 } 9467 9468 @Override 9469 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9470 switch (_hash) { 9471 case 1662702951: 9472 /* operation */ return new Property("operation", "@TestScript.setup.action.operation", 9473 "An operation would involve a REST request to a server.", 0, 1, operation); 9474 default: 9475 return super.getNamedProperty(_hash, _name, _checkValid); 9476 } 9477 9478 } 9479 9480 @Override 9481 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9482 switch (hash) { 9483 case 1662702951: 9484 /* operation */ return this.operation == null ? new Base[0] : new Base[] { this.operation }; // SetupActionOperationComponent 9485 default: 9486 return super.getProperty(hash, name, checkValid); 9487 } 9488 9489 } 9490 9491 @Override 9492 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9493 switch (hash) { 9494 case 1662702951: // operation 9495 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9496 return value; 9497 default: 9498 return super.setProperty(hash, name, value); 9499 } 9500 9501 } 9502 9503 @Override 9504 public Base setProperty(String name, Base value) throws FHIRException { 9505 if (name.equals("operation")) { 9506 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9507 } else 9508 return super.setProperty(name, value); 9509 return value; 9510 } 9511 9512 @Override 9513 public void removeChild(String name, Base value) throws FHIRException { 9514 if (name.equals("operation")) { 9515 this.operation = (SetupActionOperationComponent) value; // SetupActionOperationComponent 9516 } else 9517 super.removeChild(name, value); 9518 9519 } 9520 9521 @Override 9522 public Base makeProperty(int hash, String name) throws FHIRException { 9523 switch (hash) { 9524 case 1662702951: 9525 return getOperation(); 9526 default: 9527 return super.makeProperty(hash, name); 9528 } 9529 9530 } 9531 9532 @Override 9533 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9534 switch (hash) { 9535 case 1662702951: 9536 /* operation */ return new String[] { "@TestScript.setup.action.operation" }; 9537 default: 9538 return super.getTypesForProperty(hash, name); 9539 } 9540 9541 } 9542 9543 @Override 9544 public Base addChild(String name) throws FHIRException { 9545 if (name.equals("operation")) { 9546 this.operation = new SetupActionOperationComponent(); 9547 return this.operation; 9548 } else 9549 return super.addChild(name); 9550 } 9551 9552 public TeardownActionComponent copy() { 9553 TeardownActionComponent dst = new TeardownActionComponent(); 9554 copyValues(dst); 9555 return dst; 9556 } 9557 9558 public void copyValues(TeardownActionComponent dst) { 9559 super.copyValues(dst); 9560 dst.operation = operation == null ? null : operation.copy(); 9561 } 9562 9563 @Override 9564 public boolean equalsDeep(Base other_) { 9565 if (!super.equalsDeep(other_)) 9566 return false; 9567 if (!(other_ instanceof TeardownActionComponent)) 9568 return false; 9569 TeardownActionComponent o = (TeardownActionComponent) other_; 9570 return compareDeep(operation, o.operation, true); 9571 } 9572 9573 @Override 9574 public boolean equalsShallow(Base other_) { 9575 if (!super.equalsShallow(other_)) 9576 return false; 9577 if (!(other_ instanceof TeardownActionComponent)) 9578 return false; 9579 TeardownActionComponent o = (TeardownActionComponent) other_; 9580 return true; 9581 } 9582 9583 public boolean isEmpty() { 9584 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operation); 9585 } 9586 9587 public String fhirType() { 9588 return "TestScript.teardown.action"; 9589 9590 } 9591 9592 } 9593 9594 /** 9595 * A formal identifier that is used to identify this test script when it is 9596 * represented in other formats, or referenced in a specification, model, design 9597 * or an instance. 9598 */ 9599 @Child(name = "identifier", type = { 9600 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 9601 @Description(shortDefinition = "Additional identifier for the test script", formalDefinition = "A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.") 9602 protected Identifier identifier; 9603 9604 /** 9605 * Explanation of why this test script is needed and why it has been designed as 9606 * it has. 9607 */ 9608 @Child(name = "purpose", type = { 9609 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 9610 @Description(shortDefinition = "Why this test script is defined", formalDefinition = "Explanation of why this test script is needed and why it has been designed as it has.") 9611 protected MarkdownType purpose; 9612 9613 /** 9614 * A copyright statement relating to the test script and/or its contents. 9615 * Copyright statements are generally legal restrictions on the use and 9616 * publishing of the test script. 9617 */ 9618 @Child(name = "copyright", type = { 9619 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 9620 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.") 9621 protected MarkdownType copyright; 9622 9623 /** 9624 * An abstract server used in operations within this test script in the origin 9625 * element. 9626 */ 9627 @Child(name = "origin", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9628 @Description(shortDefinition = "An abstract server representing a client or sender in a message exchange", formalDefinition = "An abstract server used in operations within this test script in the origin element.") 9629 protected List<TestScriptOriginComponent> origin; 9630 9631 /** 9632 * An abstract server used in operations within this test script in the 9633 * destination element. 9634 */ 9635 @Child(name = "destination", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9636 @Description(shortDefinition = "An abstract server representing a destination or receiver in a message exchange", formalDefinition = "An abstract server used in operations within this test script in the destination element.") 9637 protected List<TestScriptDestinationComponent> destination; 9638 9639 /** 9640 * The required capability must exist and are assumed to function correctly on 9641 * the FHIR server being tested. 9642 */ 9643 @Child(name = "metadata", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = false) 9644 @Description(shortDefinition = "Required capability that is assumed to function correctly on the FHIR server being tested", formalDefinition = "The required capability must exist and are assumed to function correctly on the FHIR server being tested.") 9645 protected TestScriptMetadataComponent metadata; 9646 9647 /** 9648 * Fixture in the test script - by reference (uri). All fixtures are required 9649 * for the test script to execute. 9650 */ 9651 @Child(name = "fixture", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9652 @Description(shortDefinition = "Fixture in the test script - by reference (uri)", formalDefinition = "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.") 9653 protected List<TestScriptFixtureComponent> fixture; 9654 9655 /** 9656 * Reference to the profile to be used for validation. 9657 */ 9658 @Child(name = "profile", type = { 9659 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9660 @Description(shortDefinition = "Reference of the validation profile", formalDefinition = "Reference to the profile to be used for validation.") 9661 protected List<Reference> profile; 9662 /** 9663 * The actual objects that are the target of the reference (Reference to the 9664 * profile to be used for validation.) 9665 */ 9666 protected List<Resource> profileTarget; 9667 9668 /** 9669 * Variable is set based either on element value in response body or on header 9670 * field value in the response headers. 9671 */ 9672 @Child(name = "variable", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9673 @Description(shortDefinition = "Placeholder for evaluated elements", formalDefinition = "Variable is set based either on element value in response body or on header field value in the response headers.") 9674 protected List<TestScriptVariableComponent> variable; 9675 9676 /** 9677 * A series of required setup operations before tests are executed. 9678 */ 9679 @Child(name = "setup", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 9680 @Description(shortDefinition = "A series of required setup operations before tests are executed", formalDefinition = "A series of required setup operations before tests are executed.") 9681 protected TestScriptSetupComponent setup; 9682 9683 /** 9684 * A test in this script. 9685 */ 9686 @Child(name = "test", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 9687 @Description(shortDefinition = "A test in this script", formalDefinition = "A test in this script.") 9688 protected List<TestScriptTestComponent> test; 9689 9690 /** 9691 * A series of operations required to clean up after all the tests are executed 9692 * (successfully or otherwise). 9693 */ 9694 @Child(name = "teardown", type = {}, order = 11, min = 0, max = 1, modifier = false, summary = false) 9695 @Description(shortDefinition = "A series of required clean up steps", formalDefinition = "A series of operations required to clean up after all the tests are executed (successfully or otherwise).") 9696 protected TestScriptTeardownComponent teardown; 9697 9698 private static final long serialVersionUID = -1433230137L; 9699 9700 /** 9701 * Constructor 9702 */ 9703 public TestScript() { 9704 super(); 9705 } 9706 9707 /** 9708 * Constructor 9709 */ 9710 public TestScript(UriType url, StringType name, Enumeration<PublicationStatus> status) { 9711 super(); 9712 this.url = url; 9713 this.name = name; 9714 this.status = status; 9715 } 9716 9717 /** 9718 * @return {@link #url} (An absolute URI that is used to identify this test 9719 * script when it is referenced in a specification, model, design or an 9720 * instance; also called its canonical identifier. This SHOULD be 9721 * globally unique and SHOULD be a literal address at which at which an 9722 * authoritative instance of this test script is (or will be) published. 9723 * This URL can be the target of a canonical reference. It SHALL remain 9724 * the same when the test script is stored on different servers.). This 9725 * is the underlying object with id, value and extensions. The accessor 9726 * "getUrl" gives direct access to the value 9727 */ 9728 public UriType getUrlElement() { 9729 if (this.url == null) 9730 if (Configuration.errorOnAutoCreate()) 9731 throw new Error("Attempt to auto-create TestScript.url"); 9732 else if (Configuration.doAutoCreate()) 9733 this.url = new UriType(); // bb 9734 return this.url; 9735 } 9736 9737 public boolean hasUrlElement() { 9738 return this.url != null && !this.url.isEmpty(); 9739 } 9740 9741 public boolean hasUrl() { 9742 return this.url != null && !this.url.isEmpty(); 9743 } 9744 9745 /** 9746 * @param value {@link #url} (An absolute URI that is used to identify this test 9747 * script when it is referenced in a specification, model, design 9748 * or an instance; also called its canonical identifier. This 9749 * SHOULD be globally unique and SHOULD be a literal address at 9750 * which at which an authoritative instance of this test script is 9751 * (or will be) published. This URL can be the target of a 9752 * canonical reference. It SHALL remain the same when the test 9753 * script is stored on different servers.). This is the underlying 9754 * object with id, value and extensions. The accessor "getUrl" 9755 * gives direct access to the value 9756 */ 9757 public TestScript setUrlElement(UriType value) { 9758 this.url = value; 9759 return this; 9760 } 9761 9762 /** 9763 * @return An absolute URI that is used to identify this test script when it is 9764 * referenced in a specification, model, design or an instance; also 9765 * called its canonical identifier. This SHOULD be globally unique and 9766 * SHOULD be a literal address at which at which an authoritative 9767 * instance of this test script is (or will be) published. This URL can 9768 * be the target of a canonical reference. It SHALL remain the same when 9769 * the test script is stored on different servers. 9770 */ 9771 public String getUrl() { 9772 return this.url == null ? null : this.url.getValue(); 9773 } 9774 9775 /** 9776 * @param value An absolute URI that is used to identify this test script when 9777 * it is referenced in a specification, model, design or an 9778 * instance; also called its canonical identifier. This SHOULD be 9779 * globally unique and SHOULD be a literal address at which at 9780 * which an authoritative instance of this test script is (or will 9781 * be) published. This URL can be the target of a canonical 9782 * reference. It SHALL remain the same when the test script is 9783 * stored on different servers. 9784 */ 9785 public TestScript setUrl(String value) { 9786 if (this.url == null) 9787 this.url = new UriType(); 9788 this.url.setValue(value); 9789 return this; 9790 } 9791 9792 /** 9793 * @return {@link #identifier} (A formal identifier that is used to identify 9794 * this test script when it is represented in other formats, or 9795 * referenced in a specification, model, design or an instance.) 9796 */ 9797 public Identifier getIdentifier() { 9798 if (this.identifier == null) 9799 if (Configuration.errorOnAutoCreate()) 9800 throw new Error("Attempt to auto-create TestScript.identifier"); 9801 else if (Configuration.doAutoCreate()) 9802 this.identifier = new Identifier(); // cc 9803 return this.identifier; 9804 } 9805 9806 public boolean hasIdentifier() { 9807 return this.identifier != null && !this.identifier.isEmpty(); 9808 } 9809 9810 /** 9811 * @param value {@link #identifier} (A formal identifier that is used to 9812 * identify this test script when it is represented in other 9813 * formats, or referenced in a specification, model, design or an 9814 * instance.) 9815 */ 9816 public TestScript setIdentifier(Identifier value) { 9817 this.identifier = value; 9818 return this; 9819 } 9820 9821 /** 9822 * @return {@link #version} (The identifier that is used to identify this 9823 * version of the test script when it is referenced in a specification, 9824 * model, design or instance. This is an arbitrary value managed by the 9825 * test script author and is not expected to be globally unique. For 9826 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 9827 * is not available. There is also no expectation that versions can be 9828 * placed in a lexicographical sequence.). This is the underlying object 9829 * with id, value and extensions. The accessor "getVersion" gives direct 9830 * access to the value 9831 */ 9832 public StringType getVersionElement() { 9833 if (this.version == null) 9834 if (Configuration.errorOnAutoCreate()) 9835 throw new Error("Attempt to auto-create TestScript.version"); 9836 else if (Configuration.doAutoCreate()) 9837 this.version = new StringType(); // bb 9838 return this.version; 9839 } 9840 9841 public boolean hasVersionElement() { 9842 return this.version != null && !this.version.isEmpty(); 9843 } 9844 9845 public boolean hasVersion() { 9846 return this.version != null && !this.version.isEmpty(); 9847 } 9848 9849 /** 9850 * @param value {@link #version} (The identifier that is used to identify this 9851 * version of the test script when it is referenced in a 9852 * specification, model, design or instance. This is an arbitrary 9853 * value managed by the test script author and is not expected to 9854 * be globally unique. For example, it might be a timestamp (e.g. 9855 * yyyymmdd) if a managed version is not available. There is also 9856 * no expectation that versions can be placed in a lexicographical 9857 * sequence.). This is the underlying object with id, value and 9858 * extensions. The accessor "getVersion" gives direct access to the 9859 * value 9860 */ 9861 public TestScript setVersionElement(StringType value) { 9862 this.version = value; 9863 return this; 9864 } 9865 9866 /** 9867 * @return The identifier that is used to identify this version of the test 9868 * script when it is referenced in a specification, model, design or 9869 * instance. This is an arbitrary value managed by the test script 9870 * author and is not expected to be globally unique. For example, it 9871 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 9872 * available. There is also no expectation that versions can be placed 9873 * in a lexicographical sequence. 9874 */ 9875 public String getVersion() { 9876 return this.version == null ? null : this.version.getValue(); 9877 } 9878 9879 /** 9880 * @param value The identifier that is used to identify this version of the test 9881 * script when it is referenced in a specification, model, design 9882 * or instance. This is an arbitrary value managed by the test 9883 * script author and is not expected to be globally unique. For 9884 * example, it might be a timestamp (e.g. yyyymmdd) if a managed 9885 * version is not available. There is also no expectation that 9886 * versions can be placed in a lexicographical sequence. 9887 */ 9888 public TestScript setVersion(String value) { 9889 if (Utilities.noString(value)) 9890 this.version = null; 9891 else { 9892 if (this.version == null) 9893 this.version = new StringType(); 9894 this.version.setValue(value); 9895 } 9896 return this; 9897 } 9898 9899 /** 9900 * @return {@link #name} (A natural language name identifying the test script. 9901 * This name should be usable as an identifier for the module by machine 9902 * processing applications such as code generation.). This is the 9903 * underlying object with id, value and extensions. The accessor 9904 * "getName" gives direct access to the value 9905 */ 9906 public StringType getNameElement() { 9907 if (this.name == null) 9908 if (Configuration.errorOnAutoCreate()) 9909 throw new Error("Attempt to auto-create TestScript.name"); 9910 else if (Configuration.doAutoCreate()) 9911 this.name = new StringType(); // bb 9912 return this.name; 9913 } 9914 9915 public boolean hasNameElement() { 9916 return this.name != null && !this.name.isEmpty(); 9917 } 9918 9919 public boolean hasName() { 9920 return this.name != null && !this.name.isEmpty(); 9921 } 9922 9923 /** 9924 * @param value {@link #name} (A natural language name identifying the test 9925 * script. This name should be usable as an identifier for the 9926 * module by machine processing applications such as code 9927 * generation.). This is the underlying object with id, value and 9928 * extensions. The accessor "getName" gives direct access to the 9929 * value 9930 */ 9931 public TestScript setNameElement(StringType value) { 9932 this.name = value; 9933 return this; 9934 } 9935 9936 /** 9937 * @return A natural language name identifying the test script. This name should 9938 * be usable as an identifier for the module by machine processing 9939 * applications such as code generation. 9940 */ 9941 public String getName() { 9942 return this.name == null ? null : this.name.getValue(); 9943 } 9944 9945 /** 9946 * @param value A natural language name identifying the test script. This name 9947 * should be usable as an identifier for the module by machine 9948 * processing applications such as code generation. 9949 */ 9950 public TestScript setName(String value) { 9951 if (this.name == null) 9952 this.name = new StringType(); 9953 this.name.setValue(value); 9954 return this; 9955 } 9956 9957 /** 9958 * @return {@link #title} (A short, descriptive, user-friendly title for the 9959 * test script.). This is the underlying object with id, value and 9960 * extensions. The accessor "getTitle" gives direct access to the value 9961 */ 9962 public StringType getTitleElement() { 9963 if (this.title == null) 9964 if (Configuration.errorOnAutoCreate()) 9965 throw new Error("Attempt to auto-create TestScript.title"); 9966 else if (Configuration.doAutoCreate()) 9967 this.title = new StringType(); // bb 9968 return this.title; 9969 } 9970 9971 public boolean hasTitleElement() { 9972 return this.title != null && !this.title.isEmpty(); 9973 } 9974 9975 public boolean hasTitle() { 9976 return this.title != null && !this.title.isEmpty(); 9977 } 9978 9979 /** 9980 * @param value {@link #title} (A short, descriptive, user-friendly title for 9981 * the test script.). This is the underlying object with id, value 9982 * and extensions. The accessor "getTitle" gives direct access to 9983 * the value 9984 */ 9985 public TestScript setTitleElement(StringType value) { 9986 this.title = value; 9987 return this; 9988 } 9989 9990 /** 9991 * @return A short, descriptive, user-friendly title for the test script. 9992 */ 9993 public String getTitle() { 9994 return this.title == null ? null : this.title.getValue(); 9995 } 9996 9997 /** 9998 * @param value A short, descriptive, user-friendly title for the test script. 9999 */ 10000 public TestScript setTitle(String value) { 10001 if (Utilities.noString(value)) 10002 this.title = null; 10003 else { 10004 if (this.title == null) 10005 this.title = new StringType(); 10006 this.title.setValue(value); 10007 } 10008 return this; 10009 } 10010 10011 /** 10012 * @return {@link #status} (The status of this test script. Enables tracking the 10013 * life-cycle of the content.). This is the underlying object with id, 10014 * value and extensions. The accessor "getStatus" gives direct access to 10015 * the value 10016 */ 10017 public Enumeration<PublicationStatus> getStatusElement() { 10018 if (this.status == null) 10019 if (Configuration.errorOnAutoCreate()) 10020 throw new Error("Attempt to auto-create TestScript.status"); 10021 else if (Configuration.doAutoCreate()) 10022 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 10023 return this.status; 10024 } 10025 10026 public boolean hasStatusElement() { 10027 return this.status != null && !this.status.isEmpty(); 10028 } 10029 10030 public boolean hasStatus() { 10031 return this.status != null && !this.status.isEmpty(); 10032 } 10033 10034 /** 10035 * @param value {@link #status} (The status of this test script. Enables 10036 * tracking the life-cycle of the content.). This is the underlying 10037 * object with id, value and extensions. The accessor "getStatus" 10038 * gives direct access to the value 10039 */ 10040 public TestScript setStatusElement(Enumeration<PublicationStatus> value) { 10041 this.status = value; 10042 return this; 10043 } 10044 10045 /** 10046 * @return The status of this test script. Enables tracking the life-cycle of 10047 * the content. 10048 */ 10049 public PublicationStatus getStatus() { 10050 return this.status == null ? null : this.status.getValue(); 10051 } 10052 10053 /** 10054 * @param value The status of this test script. Enables tracking the life-cycle 10055 * of the content. 10056 */ 10057 public TestScript setStatus(PublicationStatus value) { 10058 if (this.status == null) 10059 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 10060 this.status.setValue(value); 10061 return this; 10062 } 10063 10064 /** 10065 * @return {@link #experimental} (A Boolean value to indicate that this test 10066 * script is authored for testing purposes (or 10067 * education/evaluation/marketing) and is not intended to be used for 10068 * genuine usage.). This is the underlying object with id, value and 10069 * extensions. The accessor "getExperimental" gives direct access to the 10070 * value 10071 */ 10072 public BooleanType getExperimentalElement() { 10073 if (this.experimental == null) 10074 if (Configuration.errorOnAutoCreate()) 10075 throw new Error("Attempt to auto-create TestScript.experimental"); 10076 else if (Configuration.doAutoCreate()) 10077 this.experimental = new BooleanType(); // bb 10078 return this.experimental; 10079 } 10080 10081 public boolean hasExperimentalElement() { 10082 return this.experimental != null && !this.experimental.isEmpty(); 10083 } 10084 10085 public boolean hasExperimental() { 10086 return this.experimental != null && !this.experimental.isEmpty(); 10087 } 10088 10089 /** 10090 * @param value {@link #experimental} (A Boolean value to indicate that this 10091 * test script is authored for testing purposes (or 10092 * education/evaluation/marketing) and is not intended to be used 10093 * for genuine usage.). This is the underlying object with id, 10094 * value and extensions. The accessor "getExperimental" gives 10095 * direct access to the value 10096 */ 10097 public TestScript setExperimentalElement(BooleanType value) { 10098 this.experimental = value; 10099 return this; 10100 } 10101 10102 /** 10103 * @return A Boolean value to indicate that this test script is authored for 10104 * testing purposes (or education/evaluation/marketing) and is not 10105 * intended to be used for genuine usage. 10106 */ 10107 public boolean getExperimental() { 10108 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 10109 } 10110 10111 /** 10112 * @param value A Boolean value to indicate that this test script is authored 10113 * for testing purposes (or education/evaluation/marketing) and is 10114 * not intended to be used for genuine usage. 10115 */ 10116 public TestScript setExperimental(boolean value) { 10117 if (this.experimental == null) 10118 this.experimental = new BooleanType(); 10119 this.experimental.setValue(value); 10120 return this; 10121 } 10122 10123 /** 10124 * @return {@link #date} (The date (and optionally time) when the test script 10125 * was published. The date must change when the business version changes 10126 * and it must change if the status code changes. In addition, it should 10127 * change when the substantive content of the test script changes.). 10128 * This is the underlying object with id, value and extensions. The 10129 * accessor "getDate" gives direct access to the value 10130 */ 10131 public DateTimeType getDateElement() { 10132 if (this.date == null) 10133 if (Configuration.errorOnAutoCreate()) 10134 throw new Error("Attempt to auto-create TestScript.date"); 10135 else if (Configuration.doAutoCreate()) 10136 this.date = new DateTimeType(); // bb 10137 return this.date; 10138 } 10139 10140 public boolean hasDateElement() { 10141 return this.date != null && !this.date.isEmpty(); 10142 } 10143 10144 public boolean hasDate() { 10145 return this.date != null && !this.date.isEmpty(); 10146 } 10147 10148 /** 10149 * @param value {@link #date} (The date (and optionally time) when the test 10150 * script was published. The date must change when the business 10151 * version changes and it must change if the status code changes. 10152 * In addition, it should change when the substantive content of 10153 * the test script changes.). This is the underlying object with 10154 * id, value and extensions. The accessor "getDate" gives direct 10155 * access to the value 10156 */ 10157 public TestScript setDateElement(DateTimeType value) { 10158 this.date = value; 10159 return this; 10160 } 10161 10162 /** 10163 * @return The date (and optionally time) when the test script was published. 10164 * The date must change when the business version changes and it must 10165 * change if the status code changes. In addition, it should change when 10166 * the substantive content of the test script changes. 10167 */ 10168 public Date getDate() { 10169 return this.date == null ? null : this.date.getValue(); 10170 } 10171 10172 /** 10173 * @param value The date (and optionally time) when the test script was 10174 * published. The date must change when the business version 10175 * changes and it must change if the status code changes. In 10176 * addition, it should change when the substantive content of the 10177 * test script changes. 10178 */ 10179 public TestScript setDate(Date value) { 10180 if (value == null) 10181 this.date = null; 10182 else { 10183 if (this.date == null) 10184 this.date = new DateTimeType(); 10185 this.date.setValue(value); 10186 } 10187 return this; 10188 } 10189 10190 /** 10191 * @return {@link #publisher} (The name of the organization or individual that 10192 * published the test script.). This is the underlying object with id, 10193 * value and extensions. The accessor "getPublisher" gives direct access 10194 * to the value 10195 */ 10196 public StringType getPublisherElement() { 10197 if (this.publisher == null) 10198 if (Configuration.errorOnAutoCreate()) 10199 throw new Error("Attempt to auto-create TestScript.publisher"); 10200 else if (Configuration.doAutoCreate()) 10201 this.publisher = new StringType(); // bb 10202 return this.publisher; 10203 } 10204 10205 public boolean hasPublisherElement() { 10206 return this.publisher != null && !this.publisher.isEmpty(); 10207 } 10208 10209 public boolean hasPublisher() { 10210 return this.publisher != null && !this.publisher.isEmpty(); 10211 } 10212 10213 /** 10214 * @param value {@link #publisher} (The name of the organization or individual 10215 * that published the test script.). This is the underlying object 10216 * with id, value and extensions. The accessor "getPublisher" gives 10217 * direct access to the value 10218 */ 10219 public TestScript setPublisherElement(StringType value) { 10220 this.publisher = value; 10221 return this; 10222 } 10223 10224 /** 10225 * @return The name of the organization or individual that published the test 10226 * script. 10227 */ 10228 public String getPublisher() { 10229 return this.publisher == null ? null : this.publisher.getValue(); 10230 } 10231 10232 /** 10233 * @param value The name of the organization or individual that published the 10234 * test script. 10235 */ 10236 public TestScript setPublisher(String value) { 10237 if (Utilities.noString(value)) 10238 this.publisher = null; 10239 else { 10240 if (this.publisher == null) 10241 this.publisher = new StringType(); 10242 this.publisher.setValue(value); 10243 } 10244 return this; 10245 } 10246 10247 /** 10248 * @return {@link #contact} (Contact details to assist a user in finding and 10249 * communicating with the publisher.) 10250 */ 10251 public List<ContactDetail> getContact() { 10252 if (this.contact == null) 10253 this.contact = new ArrayList<ContactDetail>(); 10254 return this.contact; 10255 } 10256 10257 /** 10258 * @return Returns a reference to <code>this</code> for easy method chaining 10259 */ 10260 public TestScript setContact(List<ContactDetail> theContact) { 10261 this.contact = theContact; 10262 return this; 10263 } 10264 10265 public boolean hasContact() { 10266 if (this.contact == null) 10267 return false; 10268 for (ContactDetail item : this.contact) 10269 if (!item.isEmpty()) 10270 return true; 10271 return false; 10272 } 10273 10274 public ContactDetail addContact() { // 3 10275 ContactDetail t = new ContactDetail(); 10276 if (this.contact == null) 10277 this.contact = new ArrayList<ContactDetail>(); 10278 this.contact.add(t); 10279 return t; 10280 } 10281 10282 public TestScript addContact(ContactDetail t) { // 3 10283 if (t == null) 10284 return this; 10285 if (this.contact == null) 10286 this.contact = new ArrayList<ContactDetail>(); 10287 this.contact.add(t); 10288 return this; 10289 } 10290 10291 /** 10292 * @return The first repetition of repeating field {@link #contact}, creating it 10293 * if it does not already exist 10294 */ 10295 public ContactDetail getContactFirstRep() { 10296 if (getContact().isEmpty()) { 10297 addContact(); 10298 } 10299 return getContact().get(0); 10300 } 10301 10302 /** 10303 * @return {@link #description} (A free text natural language description of the 10304 * test script from a consumer's perspective.). This is the underlying 10305 * object with id, value and extensions. The accessor "getDescription" 10306 * gives direct access to the value 10307 */ 10308 public MarkdownType getDescriptionElement() { 10309 if (this.description == null) 10310 if (Configuration.errorOnAutoCreate()) 10311 throw new Error("Attempt to auto-create TestScript.description"); 10312 else if (Configuration.doAutoCreate()) 10313 this.description = new MarkdownType(); // bb 10314 return this.description; 10315 } 10316 10317 public boolean hasDescriptionElement() { 10318 return this.description != null && !this.description.isEmpty(); 10319 } 10320 10321 public boolean hasDescription() { 10322 return this.description != null && !this.description.isEmpty(); 10323 } 10324 10325 /** 10326 * @param value {@link #description} (A free text natural language description 10327 * of the test script from a consumer's perspective.). This is the 10328 * underlying object with id, value and extensions. The accessor 10329 * "getDescription" gives direct access to the value 10330 */ 10331 public TestScript setDescriptionElement(MarkdownType value) { 10332 this.description = value; 10333 return this; 10334 } 10335 10336 /** 10337 * @return A free text natural language description of the test script from a 10338 * consumer's perspective. 10339 */ 10340 public String getDescription() { 10341 return this.description == null ? null : this.description.getValue(); 10342 } 10343 10344 /** 10345 * @param value A free text natural language description of the test script from 10346 * a consumer's perspective. 10347 */ 10348 public TestScript setDescription(String value) { 10349 if (value == null) 10350 this.description = null; 10351 else { 10352 if (this.description == null) 10353 this.description = new MarkdownType(); 10354 this.description.setValue(value); 10355 } 10356 return this; 10357 } 10358 10359 /** 10360 * @return {@link #useContext} (The content was developed with a focus and 10361 * intent of supporting the contexts that are listed. These contexts may 10362 * be general categories (gender, age, ...) or may be references to 10363 * specific programs (insurance plans, studies, ...) and may be used to 10364 * assist with indexing and searching for appropriate test script 10365 * instances.) 10366 */ 10367 public List<UsageContext> getUseContext() { 10368 if (this.useContext == null) 10369 this.useContext = new ArrayList<UsageContext>(); 10370 return this.useContext; 10371 } 10372 10373 /** 10374 * @return Returns a reference to <code>this</code> for easy method chaining 10375 */ 10376 public TestScript setUseContext(List<UsageContext> theUseContext) { 10377 this.useContext = theUseContext; 10378 return this; 10379 } 10380 10381 public boolean hasUseContext() { 10382 if (this.useContext == null) 10383 return false; 10384 for (UsageContext item : this.useContext) 10385 if (!item.isEmpty()) 10386 return true; 10387 return false; 10388 } 10389 10390 public UsageContext addUseContext() { // 3 10391 UsageContext t = new UsageContext(); 10392 if (this.useContext == null) 10393 this.useContext = new ArrayList<UsageContext>(); 10394 this.useContext.add(t); 10395 return t; 10396 } 10397 10398 public TestScript addUseContext(UsageContext t) { // 3 10399 if (t == null) 10400 return this; 10401 if (this.useContext == null) 10402 this.useContext = new ArrayList<UsageContext>(); 10403 this.useContext.add(t); 10404 return this; 10405 } 10406 10407 /** 10408 * @return The first repetition of repeating field {@link #useContext}, creating 10409 * it if it does not already exist 10410 */ 10411 public UsageContext getUseContextFirstRep() { 10412 if (getUseContext().isEmpty()) { 10413 addUseContext(); 10414 } 10415 return getUseContext().get(0); 10416 } 10417 10418 /** 10419 * @return {@link #jurisdiction} (A legal or geographic region in which the test 10420 * script is intended to be used.) 10421 */ 10422 public List<CodeableConcept> getJurisdiction() { 10423 if (this.jurisdiction == null) 10424 this.jurisdiction = new ArrayList<CodeableConcept>(); 10425 return this.jurisdiction; 10426 } 10427 10428 /** 10429 * @return Returns a reference to <code>this</code> for easy method chaining 10430 */ 10431 public TestScript setJurisdiction(List<CodeableConcept> theJurisdiction) { 10432 this.jurisdiction = theJurisdiction; 10433 return this; 10434 } 10435 10436 public boolean hasJurisdiction() { 10437 if (this.jurisdiction == null) 10438 return false; 10439 for (CodeableConcept item : this.jurisdiction) 10440 if (!item.isEmpty()) 10441 return true; 10442 return false; 10443 } 10444 10445 public CodeableConcept addJurisdiction() { // 3 10446 CodeableConcept t = new CodeableConcept(); 10447 if (this.jurisdiction == null) 10448 this.jurisdiction = new ArrayList<CodeableConcept>(); 10449 this.jurisdiction.add(t); 10450 return t; 10451 } 10452 10453 public TestScript addJurisdiction(CodeableConcept t) { // 3 10454 if (t == null) 10455 return this; 10456 if (this.jurisdiction == null) 10457 this.jurisdiction = new ArrayList<CodeableConcept>(); 10458 this.jurisdiction.add(t); 10459 return this; 10460 } 10461 10462 /** 10463 * @return The first repetition of repeating field {@link #jurisdiction}, 10464 * creating it if it does not already exist 10465 */ 10466 public CodeableConcept getJurisdictionFirstRep() { 10467 if (getJurisdiction().isEmpty()) { 10468 addJurisdiction(); 10469 } 10470 return getJurisdiction().get(0); 10471 } 10472 10473 /** 10474 * @return {@link #purpose} (Explanation of why this test script is needed and 10475 * why it has been designed as it has.). This is the underlying object 10476 * with id, value and extensions. The accessor "getPurpose" gives direct 10477 * access to the value 10478 */ 10479 public MarkdownType getPurposeElement() { 10480 if (this.purpose == null) 10481 if (Configuration.errorOnAutoCreate()) 10482 throw new Error("Attempt to auto-create TestScript.purpose"); 10483 else if (Configuration.doAutoCreate()) 10484 this.purpose = new MarkdownType(); // bb 10485 return this.purpose; 10486 } 10487 10488 public boolean hasPurposeElement() { 10489 return this.purpose != null && !this.purpose.isEmpty(); 10490 } 10491 10492 public boolean hasPurpose() { 10493 return this.purpose != null && !this.purpose.isEmpty(); 10494 } 10495 10496 /** 10497 * @param value {@link #purpose} (Explanation of why this test script is needed 10498 * and why it has been designed as it has.). This is the underlying 10499 * object with id, value and extensions. The accessor "getPurpose" 10500 * gives direct access to the value 10501 */ 10502 public TestScript setPurposeElement(MarkdownType value) { 10503 this.purpose = value; 10504 return this; 10505 } 10506 10507 /** 10508 * @return Explanation of why this test script is needed and why it has been 10509 * designed as it has. 10510 */ 10511 public String getPurpose() { 10512 return this.purpose == null ? null : this.purpose.getValue(); 10513 } 10514 10515 /** 10516 * @param value Explanation of why this test script is needed and why it has 10517 * been designed as it has. 10518 */ 10519 public TestScript setPurpose(String value) { 10520 if (value == null) 10521 this.purpose = null; 10522 else { 10523 if (this.purpose == null) 10524 this.purpose = new MarkdownType(); 10525 this.purpose.setValue(value); 10526 } 10527 return this; 10528 } 10529 10530 /** 10531 * @return {@link #copyright} (A copyright statement relating to the test script 10532 * and/or its contents. Copyright statements are generally legal 10533 * restrictions on the use and publishing of the test script.). This is 10534 * the underlying object with id, value and extensions. The accessor 10535 * "getCopyright" gives direct access to the value 10536 */ 10537 public MarkdownType getCopyrightElement() { 10538 if (this.copyright == null) 10539 if (Configuration.errorOnAutoCreate()) 10540 throw new Error("Attempt to auto-create TestScript.copyright"); 10541 else if (Configuration.doAutoCreate()) 10542 this.copyright = new MarkdownType(); // bb 10543 return this.copyright; 10544 } 10545 10546 public boolean hasCopyrightElement() { 10547 return this.copyright != null && !this.copyright.isEmpty(); 10548 } 10549 10550 public boolean hasCopyright() { 10551 return this.copyright != null && !this.copyright.isEmpty(); 10552 } 10553 10554 /** 10555 * @param value {@link #copyright} (A copyright statement relating to the test 10556 * script and/or its contents. Copyright statements are generally 10557 * legal restrictions on the use and publishing of the test 10558 * script.). This is the underlying object with id, value and 10559 * extensions. The accessor "getCopyright" gives direct access to 10560 * the value 10561 */ 10562 public TestScript setCopyrightElement(MarkdownType value) { 10563 this.copyright = value; 10564 return this; 10565 } 10566 10567 /** 10568 * @return A copyright statement relating to the test script and/or its 10569 * contents. Copyright statements are generally legal restrictions on 10570 * the use and publishing of the test script. 10571 */ 10572 public String getCopyright() { 10573 return this.copyright == null ? null : this.copyright.getValue(); 10574 } 10575 10576 /** 10577 * @param value A copyright statement relating to the test script and/or its 10578 * contents. Copyright statements are generally legal restrictions 10579 * on the use and publishing of the test script. 10580 */ 10581 public TestScript setCopyright(String value) { 10582 if (value == null) 10583 this.copyright = null; 10584 else { 10585 if (this.copyright == null) 10586 this.copyright = new MarkdownType(); 10587 this.copyright.setValue(value); 10588 } 10589 return this; 10590 } 10591 10592 /** 10593 * @return {@link #origin} (An abstract server used in operations within this 10594 * test script in the origin element.) 10595 */ 10596 public List<TestScriptOriginComponent> getOrigin() { 10597 if (this.origin == null) 10598 this.origin = new ArrayList<TestScriptOriginComponent>(); 10599 return this.origin; 10600 } 10601 10602 /** 10603 * @return Returns a reference to <code>this</code> for easy method chaining 10604 */ 10605 public TestScript setOrigin(List<TestScriptOriginComponent> theOrigin) { 10606 this.origin = theOrigin; 10607 return this; 10608 } 10609 10610 public boolean hasOrigin() { 10611 if (this.origin == null) 10612 return false; 10613 for (TestScriptOriginComponent item : this.origin) 10614 if (!item.isEmpty()) 10615 return true; 10616 return false; 10617 } 10618 10619 public TestScriptOriginComponent addOrigin() { // 3 10620 TestScriptOriginComponent t = new TestScriptOriginComponent(); 10621 if (this.origin == null) 10622 this.origin = new ArrayList<TestScriptOriginComponent>(); 10623 this.origin.add(t); 10624 return t; 10625 } 10626 10627 public TestScript addOrigin(TestScriptOriginComponent t) { // 3 10628 if (t == null) 10629 return this; 10630 if (this.origin == null) 10631 this.origin = new ArrayList<TestScriptOriginComponent>(); 10632 this.origin.add(t); 10633 return this; 10634 } 10635 10636 /** 10637 * @return The first repetition of repeating field {@link #origin}, creating it 10638 * if it does not already exist 10639 */ 10640 public TestScriptOriginComponent getOriginFirstRep() { 10641 if (getOrigin().isEmpty()) { 10642 addOrigin(); 10643 } 10644 return getOrigin().get(0); 10645 } 10646 10647 /** 10648 * @return {@link #destination} (An abstract server used in operations within 10649 * this test script in the destination element.) 10650 */ 10651 public List<TestScriptDestinationComponent> getDestination() { 10652 if (this.destination == null) 10653 this.destination = new ArrayList<TestScriptDestinationComponent>(); 10654 return this.destination; 10655 } 10656 10657 /** 10658 * @return Returns a reference to <code>this</code> for easy method chaining 10659 */ 10660 public TestScript setDestination(List<TestScriptDestinationComponent> theDestination) { 10661 this.destination = theDestination; 10662 return this; 10663 } 10664 10665 public boolean hasDestination() { 10666 if (this.destination == null) 10667 return false; 10668 for (TestScriptDestinationComponent item : this.destination) 10669 if (!item.isEmpty()) 10670 return true; 10671 return false; 10672 } 10673 10674 public TestScriptDestinationComponent addDestination() { // 3 10675 TestScriptDestinationComponent t = new TestScriptDestinationComponent(); 10676 if (this.destination == null) 10677 this.destination = new ArrayList<TestScriptDestinationComponent>(); 10678 this.destination.add(t); 10679 return t; 10680 } 10681 10682 public TestScript addDestination(TestScriptDestinationComponent t) { // 3 10683 if (t == null) 10684 return this; 10685 if (this.destination == null) 10686 this.destination = new ArrayList<TestScriptDestinationComponent>(); 10687 this.destination.add(t); 10688 return this; 10689 } 10690 10691 /** 10692 * @return The first repetition of repeating field {@link #destination}, 10693 * creating it if it does not already exist 10694 */ 10695 public TestScriptDestinationComponent getDestinationFirstRep() { 10696 if (getDestination().isEmpty()) { 10697 addDestination(); 10698 } 10699 return getDestination().get(0); 10700 } 10701 10702 /** 10703 * @return {@link #metadata} (The required capability must exist and are assumed 10704 * to function correctly on the FHIR server being tested.) 10705 */ 10706 public TestScriptMetadataComponent getMetadata() { 10707 if (this.metadata == null) 10708 if (Configuration.errorOnAutoCreate()) 10709 throw new Error("Attempt to auto-create TestScript.metadata"); 10710 else if (Configuration.doAutoCreate()) 10711 this.metadata = new TestScriptMetadataComponent(); // cc 10712 return this.metadata; 10713 } 10714 10715 public boolean hasMetadata() { 10716 return this.metadata != null && !this.metadata.isEmpty(); 10717 } 10718 10719 /** 10720 * @param value {@link #metadata} (The required capability must exist and are 10721 * assumed to function correctly on the FHIR server being tested.) 10722 */ 10723 public TestScript setMetadata(TestScriptMetadataComponent value) { 10724 this.metadata = value; 10725 return this; 10726 } 10727 10728 /** 10729 * @return {@link #fixture} (Fixture in the test script - by reference (uri). 10730 * All fixtures are required for the test script to execute.) 10731 */ 10732 public List<TestScriptFixtureComponent> getFixture() { 10733 if (this.fixture == null) 10734 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 10735 return this.fixture; 10736 } 10737 10738 /** 10739 * @return Returns a reference to <code>this</code> for easy method chaining 10740 */ 10741 public TestScript setFixture(List<TestScriptFixtureComponent> theFixture) { 10742 this.fixture = theFixture; 10743 return this; 10744 } 10745 10746 public boolean hasFixture() { 10747 if (this.fixture == null) 10748 return false; 10749 for (TestScriptFixtureComponent item : this.fixture) 10750 if (!item.isEmpty()) 10751 return true; 10752 return false; 10753 } 10754 10755 public TestScriptFixtureComponent addFixture() { // 3 10756 TestScriptFixtureComponent t = new TestScriptFixtureComponent(); 10757 if (this.fixture == null) 10758 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 10759 this.fixture.add(t); 10760 return t; 10761 } 10762 10763 public TestScript addFixture(TestScriptFixtureComponent t) { // 3 10764 if (t == null) 10765 return this; 10766 if (this.fixture == null) 10767 this.fixture = new ArrayList<TestScriptFixtureComponent>(); 10768 this.fixture.add(t); 10769 return this; 10770 } 10771 10772 /** 10773 * @return The first repetition of repeating field {@link #fixture}, creating it 10774 * if it does not already exist 10775 */ 10776 public TestScriptFixtureComponent getFixtureFirstRep() { 10777 if (getFixture().isEmpty()) { 10778 addFixture(); 10779 } 10780 return getFixture().get(0); 10781 } 10782 10783 /** 10784 * @return {@link #profile} (Reference to the profile to be used for 10785 * validation.) 10786 */ 10787 public List<Reference> getProfile() { 10788 if (this.profile == null) 10789 this.profile = new ArrayList<Reference>(); 10790 return this.profile; 10791 } 10792 10793 /** 10794 * @return Returns a reference to <code>this</code> for easy method chaining 10795 */ 10796 public TestScript setProfile(List<Reference> theProfile) { 10797 this.profile = theProfile; 10798 return this; 10799 } 10800 10801 public boolean hasProfile() { 10802 if (this.profile == null) 10803 return false; 10804 for (Reference item : this.profile) 10805 if (!item.isEmpty()) 10806 return true; 10807 return false; 10808 } 10809 10810 public Reference addProfile() { // 3 10811 Reference t = new Reference(); 10812 if (this.profile == null) 10813 this.profile = new ArrayList<Reference>(); 10814 this.profile.add(t); 10815 return t; 10816 } 10817 10818 public TestScript addProfile(Reference t) { // 3 10819 if (t == null) 10820 return this; 10821 if (this.profile == null) 10822 this.profile = new ArrayList<Reference>(); 10823 this.profile.add(t); 10824 return this; 10825 } 10826 10827 /** 10828 * @return The first repetition of repeating field {@link #profile}, creating it 10829 * if it does not already exist 10830 */ 10831 public Reference getProfileFirstRep() { 10832 if (getProfile().isEmpty()) { 10833 addProfile(); 10834 } 10835 return getProfile().get(0); 10836 } 10837 10838 /** 10839 * @deprecated Use Reference#setResource(IBaseResource) instead 10840 */ 10841 @Deprecated 10842 public List<Resource> getProfileTarget() { 10843 if (this.profileTarget == null) 10844 this.profileTarget = new ArrayList<Resource>(); 10845 return this.profileTarget; 10846 } 10847 10848 /** 10849 * @return {@link #variable} (Variable is set based either on element value in 10850 * response body or on header field value in the response headers.) 10851 */ 10852 public List<TestScriptVariableComponent> getVariable() { 10853 if (this.variable == null) 10854 this.variable = new ArrayList<TestScriptVariableComponent>(); 10855 return this.variable; 10856 } 10857 10858 /** 10859 * @return Returns a reference to <code>this</code> for easy method chaining 10860 */ 10861 public TestScript setVariable(List<TestScriptVariableComponent> theVariable) { 10862 this.variable = theVariable; 10863 return this; 10864 } 10865 10866 public boolean hasVariable() { 10867 if (this.variable == null) 10868 return false; 10869 for (TestScriptVariableComponent item : this.variable) 10870 if (!item.isEmpty()) 10871 return true; 10872 return false; 10873 } 10874 10875 public TestScriptVariableComponent addVariable() { // 3 10876 TestScriptVariableComponent t = new TestScriptVariableComponent(); 10877 if (this.variable == null) 10878 this.variable = new ArrayList<TestScriptVariableComponent>(); 10879 this.variable.add(t); 10880 return t; 10881 } 10882 10883 public TestScript addVariable(TestScriptVariableComponent t) { // 3 10884 if (t == null) 10885 return this; 10886 if (this.variable == null) 10887 this.variable = new ArrayList<TestScriptVariableComponent>(); 10888 this.variable.add(t); 10889 return this; 10890 } 10891 10892 /** 10893 * @return The first repetition of repeating field {@link #variable}, creating 10894 * it if it does not already exist 10895 */ 10896 public TestScriptVariableComponent getVariableFirstRep() { 10897 if (getVariable().isEmpty()) { 10898 addVariable(); 10899 } 10900 return getVariable().get(0); 10901 } 10902 10903 /** 10904 * @return {@link #setup} (A series of required setup operations before tests 10905 * are executed.) 10906 */ 10907 public TestScriptSetupComponent getSetup() { 10908 if (this.setup == null) 10909 if (Configuration.errorOnAutoCreate()) 10910 throw new Error("Attempt to auto-create TestScript.setup"); 10911 else if (Configuration.doAutoCreate()) 10912 this.setup = new TestScriptSetupComponent(); // cc 10913 return this.setup; 10914 } 10915 10916 public boolean hasSetup() { 10917 return this.setup != null && !this.setup.isEmpty(); 10918 } 10919 10920 /** 10921 * @param value {@link #setup} (A series of required setup operations before 10922 * tests are executed.) 10923 */ 10924 public TestScript setSetup(TestScriptSetupComponent value) { 10925 this.setup = value; 10926 return this; 10927 } 10928 10929 /** 10930 * @return {@link #test} (A test in this script.) 10931 */ 10932 public List<TestScriptTestComponent> getTest() { 10933 if (this.test == null) 10934 this.test = new ArrayList<TestScriptTestComponent>(); 10935 return this.test; 10936 } 10937 10938 /** 10939 * @return Returns a reference to <code>this</code> for easy method chaining 10940 */ 10941 public TestScript setTest(List<TestScriptTestComponent> theTest) { 10942 this.test = theTest; 10943 return this; 10944 } 10945 10946 public boolean hasTest() { 10947 if (this.test == null) 10948 return false; 10949 for (TestScriptTestComponent item : this.test) 10950 if (!item.isEmpty()) 10951 return true; 10952 return false; 10953 } 10954 10955 public TestScriptTestComponent addTest() { // 3 10956 TestScriptTestComponent t = new TestScriptTestComponent(); 10957 if (this.test == null) 10958 this.test = new ArrayList<TestScriptTestComponent>(); 10959 this.test.add(t); 10960 return t; 10961 } 10962 10963 public TestScript addTest(TestScriptTestComponent t) { // 3 10964 if (t == null) 10965 return this; 10966 if (this.test == null) 10967 this.test = new ArrayList<TestScriptTestComponent>(); 10968 this.test.add(t); 10969 return this; 10970 } 10971 10972 /** 10973 * @return The first repetition of repeating field {@link #test}, creating it if 10974 * it does not already exist 10975 */ 10976 public TestScriptTestComponent getTestFirstRep() { 10977 if (getTest().isEmpty()) { 10978 addTest(); 10979 } 10980 return getTest().get(0); 10981 } 10982 10983 /** 10984 * @return {@link #teardown} (A series of operations required to clean up after 10985 * all the tests are executed (successfully or otherwise).) 10986 */ 10987 public TestScriptTeardownComponent getTeardown() { 10988 if (this.teardown == null) 10989 if (Configuration.errorOnAutoCreate()) 10990 throw new Error("Attempt to auto-create TestScript.teardown"); 10991 else if (Configuration.doAutoCreate()) 10992 this.teardown = new TestScriptTeardownComponent(); // cc 10993 return this.teardown; 10994 } 10995 10996 public boolean hasTeardown() { 10997 return this.teardown != null && !this.teardown.isEmpty(); 10998 } 10999 11000 /** 11001 * @param value {@link #teardown} (A series of operations required to clean up 11002 * after all the tests are executed (successfully or otherwise).) 11003 */ 11004 public TestScript setTeardown(TestScriptTeardownComponent value) { 11005 this.teardown = value; 11006 return this; 11007 } 11008 11009 protected void listChildren(List<Property> children) { 11010 super.listChildren(children); 11011 children.add(new Property("url", "uri", 11012 "An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers.", 11013 0, 1, url)); 11014 children.add(new Property("identifier", "Identifier", 11015 "A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.", 11016 0, 1, identifier)); 11017 children.add(new Property("version", "string", 11018 "The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 11019 0, 1, version)); 11020 children.add(new Property("name", "string", 11021 "A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 11022 0, 1, name)); 11023 children.add( 11024 new Property("title", "string", "A short, descriptive, user-friendly title for the test script.", 0, 1, title)); 11025 children.add(new Property("status", "code", 11026 "The status of this test script. Enables tracking the life-cycle of the content.", 0, 1, status)); 11027 children.add(new Property("experimental", "boolean", 11028 "A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 11029 0, 1, experimental)); 11030 children.add(new Property("date", "dateTime", 11031 "The date (and optionally time) when the test script was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.", 11032 0, 1, date)); 11033 children.add(new Property("publisher", "string", 11034 "The name of the organization or individual that published the test script.", 0, 1, publisher)); 11035 children.add(new Property("contact", "ContactDetail", 11036 "Contact details to assist a user in finding and communicating with the publisher.", 0, 11037 java.lang.Integer.MAX_VALUE, contact)); 11038 children.add(new Property("description", "markdown", 11039 "A free text natural language description of the test script from a consumer's perspective.", 0, 1, 11040 description)); 11041 children.add(new Property("useContext", "UsageContext", 11042 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test script instances.", 11043 0, java.lang.Integer.MAX_VALUE, useContext)); 11044 children.add(new Property("jurisdiction", "CodeableConcept", 11045 "A legal or geographic region in which the test script is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 11046 jurisdiction)); 11047 children.add(new Property("purpose", "markdown", 11048 "Explanation of why this test script is needed and why it has been designed as it has.", 0, 1, purpose)); 11049 children.add(new Property("copyright", "markdown", 11050 "A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.", 11051 0, 1, copyright)); 11052 children.add(new Property("origin", "", 11053 "An abstract server used in operations within this test script in the origin element.", 0, 11054 java.lang.Integer.MAX_VALUE, origin)); 11055 children.add(new Property("destination", "", 11056 "An abstract server used in operations within this test script in the destination element.", 0, 11057 java.lang.Integer.MAX_VALUE, destination)); 11058 children.add(new Property("metadata", "", 11059 "The required capability must exist and are assumed to function correctly on the FHIR server being tested.", 0, 11060 1, metadata)); 11061 children.add(new Property("fixture", "", 11062 "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.", 0, 11063 java.lang.Integer.MAX_VALUE, fixture)); 11064 children.add(new Property("profile", "Reference(Any)", "Reference to the profile to be used for validation.", 0, 11065 java.lang.Integer.MAX_VALUE, profile)); 11066 children.add(new Property("variable", "", 11067 "Variable is set based either on element value in response body or on header field value in the response headers.", 11068 0, java.lang.Integer.MAX_VALUE, variable)); 11069 children.add( 11070 new Property("setup", "", "A series of required setup operations before tests are executed.", 0, 1, setup)); 11071 children.add(new Property("test", "", "A test in this script.", 0, java.lang.Integer.MAX_VALUE, test)); 11072 children.add(new Property("teardown", "", 11073 "A series of operations required to clean up after all the tests are executed (successfully or otherwise).", 0, 11074 1, teardown)); 11075 } 11076 11077 @Override 11078 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11079 switch (_hash) { 11080 case 116079: 11081 /* url */ return new Property("url", "uri", 11082 "An absolute URI that is used to identify this test script when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this test script is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the test script is stored on different servers.", 11083 0, 1, url); 11084 case -1618432855: 11085 /* identifier */ return new Property("identifier", "Identifier", 11086 "A formal identifier that is used to identify this test script when it is represented in other formats, or referenced in a specification, model, design or an instance.", 11087 0, 1, identifier); 11088 case 351608024: 11089 /* version */ return new Property("version", "string", 11090 "The identifier that is used to identify this version of the test script when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the test script author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 11091 0, 1, version); 11092 case 3373707: 11093 /* name */ return new Property("name", "string", 11094 "A natural language name identifying the test script. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 11095 0, 1, name); 11096 case 110371416: 11097 /* title */ return new Property("title", "string", 11098 "A short, descriptive, user-friendly title for the test script.", 0, 1, title); 11099 case -892481550: 11100 /* status */ return new Property("status", "code", 11101 "The status of this test script. Enables tracking the life-cycle of the content.", 0, 1, status); 11102 case -404562712: 11103 /* experimental */ return new Property("experimental", "boolean", 11104 "A Boolean value to indicate that this test script is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 11105 0, 1, experimental); 11106 case 3076014: 11107 /* date */ return new Property("date", "dateTime", 11108 "The date (and optionally time) when the test script was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the test script changes.", 11109 0, 1, date); 11110 case 1447404028: 11111 /* publisher */ return new Property("publisher", "string", 11112 "The name of the organization or individual that published the test script.", 0, 1, publisher); 11113 case 951526432: 11114 /* contact */ return new Property("contact", "ContactDetail", 11115 "Contact details to assist a user in finding and communicating with the publisher.", 0, 11116 java.lang.Integer.MAX_VALUE, contact); 11117 case -1724546052: 11118 /* description */ return new Property("description", "markdown", 11119 "A free text natural language description of the test script from a consumer's perspective.", 0, 1, 11120 description); 11121 case -669707736: 11122 /* useContext */ return new Property("useContext", "UsageContext", 11123 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate test script instances.", 11124 0, java.lang.Integer.MAX_VALUE, useContext); 11125 case -507075711: 11126 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 11127 "A legal or geographic region in which the test script is intended to be used.", 0, 11128 java.lang.Integer.MAX_VALUE, jurisdiction); 11129 case -220463842: 11130 /* purpose */ return new Property("purpose", "markdown", 11131 "Explanation of why this test script is needed and why it has been designed as it has.", 0, 1, purpose); 11132 case 1522889671: 11133 /* copyright */ return new Property("copyright", "markdown", 11134 "A copyright statement relating to the test script and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the test script.", 11135 0, 1, copyright); 11136 case -1008619738: 11137 /* origin */ return new Property("origin", "", 11138 "An abstract server used in operations within this test script in the origin element.", 0, 11139 java.lang.Integer.MAX_VALUE, origin); 11140 case -1429847026: 11141 /* destination */ return new Property("destination", "", 11142 "An abstract server used in operations within this test script in the destination element.", 0, 11143 java.lang.Integer.MAX_VALUE, destination); 11144 case -450004177: 11145 /* metadata */ return new Property("metadata", "", 11146 "The required capability must exist and are assumed to function correctly on the FHIR server being tested.", 11147 0, 1, metadata); 11148 case -843449847: 11149 /* fixture */ return new Property("fixture", "", 11150 "Fixture in the test script - by reference (uri). All fixtures are required for the test script to execute.", 11151 0, java.lang.Integer.MAX_VALUE, fixture); 11152 case -309425751: 11153 /* profile */ return new Property("profile", "Reference(Any)", 11154 "Reference to the profile to be used for validation.", 0, java.lang.Integer.MAX_VALUE, profile); 11155 case -1249586564: 11156 /* variable */ return new Property("variable", "", 11157 "Variable is set based either on element value in response body or on header field value in the response headers.", 11158 0, java.lang.Integer.MAX_VALUE, variable); 11159 case 109329021: 11160 /* setup */ return new Property("setup", "", "A series of required setup operations before tests are executed.", 11161 0, 1, setup); 11162 case 3556498: 11163 /* test */ return new Property("test", "", "A test in this script.", 0, java.lang.Integer.MAX_VALUE, test); 11164 case -1663474172: 11165 /* teardown */ return new Property("teardown", "", 11166 "A series of operations required to clean up after all the tests are executed (successfully or otherwise).", 11167 0, 1, teardown); 11168 default: 11169 return super.getNamedProperty(_hash, _name, _checkValid); 11170 } 11171 11172 } 11173 11174 @Override 11175 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11176 switch (hash) { 11177 case 116079: 11178 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 11179 case -1618432855: 11180 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 11181 case 351608024: 11182 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 11183 case 3373707: 11184 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 11185 case 110371416: 11186 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 11187 case -892481550: 11188 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 11189 case -404562712: 11190 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 11191 case 3076014: 11192 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 11193 case 1447404028: 11194 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 11195 case 951526432: 11196 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 11197 case -1724546052: 11198 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 11199 case -669707736: 11200 /* useContext */ return this.useContext == null ? new Base[0] 11201 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 11202 case -507075711: 11203 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 11204 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 11205 case -220463842: 11206 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 11207 case 1522889671: 11208 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 11209 case -1008619738: 11210 /* origin */ return this.origin == null ? new Base[0] : this.origin.toArray(new Base[this.origin.size()]); // TestScriptOriginComponent 11211 case -1429847026: 11212 /* destination */ return this.destination == null ? new Base[0] 11213 : this.destination.toArray(new Base[this.destination.size()]); // TestScriptDestinationComponent 11214 case -450004177: 11215 /* metadata */ return this.metadata == null ? new Base[0] : new Base[] { this.metadata }; // TestScriptMetadataComponent 11216 case -843449847: 11217 /* fixture */ return this.fixture == null ? new Base[0] : this.fixture.toArray(new Base[this.fixture.size()]); // TestScriptFixtureComponent 11218 case -309425751: 11219 /* profile */ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // Reference 11220 case -1249586564: 11221 /* variable */ return this.variable == null ? new Base[0] : this.variable.toArray(new Base[this.variable.size()]); // TestScriptVariableComponent 11222 case 109329021: 11223 /* setup */ return this.setup == null ? new Base[0] : new Base[] { this.setup }; // TestScriptSetupComponent 11224 case 3556498: 11225 /* test */ return this.test == null ? new Base[0] : this.test.toArray(new Base[this.test.size()]); // TestScriptTestComponent 11226 case -1663474172: 11227 /* teardown */ return this.teardown == null ? new Base[0] : new Base[] { this.teardown }; // TestScriptTeardownComponent 11228 default: 11229 return super.getProperty(hash, name, checkValid); 11230 } 11231 11232 } 11233 11234 @Override 11235 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11236 switch (hash) { 11237 case 116079: // url 11238 this.url = castToUri(value); // UriType 11239 return value; 11240 case -1618432855: // identifier 11241 this.identifier = castToIdentifier(value); // Identifier 11242 return value; 11243 case 351608024: // version 11244 this.version = castToString(value); // StringType 11245 return value; 11246 case 3373707: // name 11247 this.name = castToString(value); // StringType 11248 return value; 11249 case 110371416: // title 11250 this.title = castToString(value); // StringType 11251 return value; 11252 case -892481550: // status 11253 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 11254 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 11255 return value; 11256 case -404562712: // experimental 11257 this.experimental = castToBoolean(value); // BooleanType 11258 return value; 11259 case 3076014: // date 11260 this.date = castToDateTime(value); // DateTimeType 11261 return value; 11262 case 1447404028: // publisher 11263 this.publisher = castToString(value); // StringType 11264 return value; 11265 case 951526432: // contact 11266 this.getContact().add(castToContactDetail(value)); // ContactDetail 11267 return value; 11268 case -1724546052: // description 11269 this.description = castToMarkdown(value); // MarkdownType 11270 return value; 11271 case -669707736: // useContext 11272 this.getUseContext().add(castToUsageContext(value)); // UsageContext 11273 return value; 11274 case -507075711: // jurisdiction 11275 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 11276 return value; 11277 case -220463842: // purpose 11278 this.purpose = castToMarkdown(value); // MarkdownType 11279 return value; 11280 case 1522889671: // copyright 11281 this.copyright = castToMarkdown(value); // MarkdownType 11282 return value; 11283 case -1008619738: // origin 11284 this.getOrigin().add((TestScriptOriginComponent) value); // TestScriptOriginComponent 11285 return value; 11286 case -1429847026: // destination 11287 this.getDestination().add((TestScriptDestinationComponent) value); // TestScriptDestinationComponent 11288 return value; 11289 case -450004177: // metadata 11290 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 11291 return value; 11292 case -843449847: // fixture 11293 this.getFixture().add((TestScriptFixtureComponent) value); // TestScriptFixtureComponent 11294 return value; 11295 case -309425751: // profile 11296 this.getProfile().add(castToReference(value)); // Reference 11297 return value; 11298 case -1249586564: // variable 11299 this.getVariable().add((TestScriptVariableComponent) value); // TestScriptVariableComponent 11300 return value; 11301 case 109329021: // setup 11302 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 11303 return value; 11304 case 3556498: // test 11305 this.getTest().add((TestScriptTestComponent) value); // TestScriptTestComponent 11306 return value; 11307 case -1663474172: // teardown 11308 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 11309 return value; 11310 default: 11311 return super.setProperty(hash, name, value); 11312 } 11313 11314 } 11315 11316 @Override 11317 public Base setProperty(String name, Base value) throws FHIRException { 11318 if (name.equals("url")) { 11319 this.url = castToUri(value); // UriType 11320 } else if (name.equals("identifier")) { 11321 this.identifier = castToIdentifier(value); // Identifier 11322 } else if (name.equals("version")) { 11323 this.version = castToString(value); // StringType 11324 } else if (name.equals("name")) { 11325 this.name = castToString(value); // StringType 11326 } else if (name.equals("title")) { 11327 this.title = castToString(value); // StringType 11328 } else if (name.equals("status")) { 11329 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 11330 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 11331 } else if (name.equals("experimental")) { 11332 this.experimental = castToBoolean(value); // BooleanType 11333 } else if (name.equals("date")) { 11334 this.date = castToDateTime(value); // DateTimeType 11335 } else if (name.equals("publisher")) { 11336 this.publisher = castToString(value); // StringType 11337 } else if (name.equals("contact")) { 11338 this.getContact().add(castToContactDetail(value)); 11339 } else if (name.equals("description")) { 11340 this.description = castToMarkdown(value); // MarkdownType 11341 } else if (name.equals("useContext")) { 11342 this.getUseContext().add(castToUsageContext(value)); 11343 } else if (name.equals("jurisdiction")) { 11344 this.getJurisdiction().add(castToCodeableConcept(value)); 11345 } else if (name.equals("purpose")) { 11346 this.purpose = castToMarkdown(value); // MarkdownType 11347 } else if (name.equals("copyright")) { 11348 this.copyright = castToMarkdown(value); // MarkdownType 11349 } else if (name.equals("origin")) { 11350 this.getOrigin().add((TestScriptOriginComponent) value); 11351 } else if (name.equals("destination")) { 11352 this.getDestination().add((TestScriptDestinationComponent) value); 11353 } else if (name.equals("metadata")) { 11354 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 11355 } else if (name.equals("fixture")) { 11356 this.getFixture().add((TestScriptFixtureComponent) value); 11357 } else if (name.equals("profile")) { 11358 this.getProfile().add(castToReference(value)); 11359 } else if (name.equals("variable")) { 11360 this.getVariable().add((TestScriptVariableComponent) value); 11361 } else if (name.equals("setup")) { 11362 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 11363 } else if (name.equals("test")) { 11364 this.getTest().add((TestScriptTestComponent) value); 11365 } else if (name.equals("teardown")) { 11366 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 11367 } else 11368 return super.setProperty(name, value); 11369 return value; 11370 } 11371 11372 @Override 11373 public void removeChild(String name, Base value) throws FHIRException { 11374 if (name.equals("url")) { 11375 this.url = null; 11376 } else if (name.equals("identifier")) { 11377 this.identifier = null; 11378 } else if (name.equals("version")) { 11379 this.version = null; 11380 } else if (name.equals("name")) { 11381 this.name = null; 11382 } else if (name.equals("title")) { 11383 this.title = null; 11384 } else if (name.equals("status")) { 11385 this.status = null; 11386 } else if (name.equals("experimental")) { 11387 this.experimental = null; 11388 } else if (name.equals("date")) { 11389 this.date = null; 11390 } else if (name.equals("publisher")) { 11391 this.publisher = null; 11392 } else if (name.equals("contact")) { 11393 this.getContact().remove(castToContactDetail(value)); 11394 } else if (name.equals("description")) { 11395 this.description = null; 11396 } else if (name.equals("useContext")) { 11397 this.getUseContext().remove(castToUsageContext(value)); 11398 } else if (name.equals("jurisdiction")) { 11399 this.getJurisdiction().remove(castToCodeableConcept(value)); 11400 } else if (name.equals("purpose")) { 11401 this.purpose = null; 11402 } else if (name.equals("copyright")) { 11403 this.copyright = null; 11404 } else if (name.equals("origin")) { 11405 this.getOrigin().remove((TestScriptOriginComponent) value); 11406 } else if (name.equals("destination")) { 11407 this.getDestination().remove((TestScriptDestinationComponent) value); 11408 } else if (name.equals("metadata")) { 11409 this.metadata = (TestScriptMetadataComponent) value; // TestScriptMetadataComponent 11410 } else if (name.equals("fixture")) { 11411 this.getFixture().remove((TestScriptFixtureComponent) value); 11412 } else if (name.equals("profile")) { 11413 this.getProfile().remove(castToReference(value)); 11414 } else if (name.equals("variable")) { 11415 this.getVariable().remove((TestScriptVariableComponent) value); 11416 } else if (name.equals("setup")) { 11417 this.setup = (TestScriptSetupComponent) value; // TestScriptSetupComponent 11418 } else if (name.equals("test")) { 11419 this.getTest().remove((TestScriptTestComponent) value); 11420 } else if (name.equals("teardown")) { 11421 this.teardown = (TestScriptTeardownComponent) value; // TestScriptTeardownComponent 11422 } else 11423 super.removeChild(name, value); 11424 11425 } 11426 11427 @Override 11428 public Base makeProperty(int hash, String name) throws FHIRException { 11429 switch (hash) { 11430 case 116079: 11431 return getUrlElement(); 11432 case -1618432855: 11433 return getIdentifier(); 11434 case 351608024: 11435 return getVersionElement(); 11436 case 3373707: 11437 return getNameElement(); 11438 case 110371416: 11439 return getTitleElement(); 11440 case -892481550: 11441 return getStatusElement(); 11442 case -404562712: 11443 return getExperimentalElement(); 11444 case 3076014: 11445 return getDateElement(); 11446 case 1447404028: 11447 return getPublisherElement(); 11448 case 951526432: 11449 return addContact(); 11450 case -1724546052: 11451 return getDescriptionElement(); 11452 case -669707736: 11453 return addUseContext(); 11454 case -507075711: 11455 return addJurisdiction(); 11456 case -220463842: 11457 return getPurposeElement(); 11458 case 1522889671: 11459 return getCopyrightElement(); 11460 case -1008619738: 11461 return addOrigin(); 11462 case -1429847026: 11463 return addDestination(); 11464 case -450004177: 11465 return getMetadata(); 11466 case -843449847: 11467 return addFixture(); 11468 case -309425751: 11469 return addProfile(); 11470 case -1249586564: 11471 return addVariable(); 11472 case 109329021: 11473 return getSetup(); 11474 case 3556498: 11475 return addTest(); 11476 case -1663474172: 11477 return getTeardown(); 11478 default: 11479 return super.makeProperty(hash, name); 11480 } 11481 11482 } 11483 11484 @Override 11485 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11486 switch (hash) { 11487 case 116079: 11488 /* url */ return new String[] { "uri" }; 11489 case -1618432855: 11490 /* identifier */ return new String[] { "Identifier" }; 11491 case 351608024: 11492 /* version */ return new String[] { "string" }; 11493 case 3373707: 11494 /* name */ return new String[] { "string" }; 11495 case 110371416: 11496 /* title */ return new String[] { "string" }; 11497 case -892481550: 11498 /* status */ return new String[] { "code" }; 11499 case -404562712: 11500 /* experimental */ return new String[] { "boolean" }; 11501 case 3076014: 11502 /* date */ return new String[] { "dateTime" }; 11503 case 1447404028: 11504 /* publisher */ return new String[] { "string" }; 11505 case 951526432: 11506 /* contact */ return new String[] { "ContactDetail" }; 11507 case -1724546052: 11508 /* description */ return new String[] { "markdown" }; 11509 case -669707736: 11510 /* useContext */ return new String[] { "UsageContext" }; 11511 case -507075711: 11512 /* jurisdiction */ return new String[] { "CodeableConcept" }; 11513 case -220463842: 11514 /* purpose */ return new String[] { "markdown" }; 11515 case 1522889671: 11516 /* copyright */ return new String[] { "markdown" }; 11517 case -1008619738: 11518 /* origin */ return new String[] {}; 11519 case -1429847026: 11520 /* destination */ return new String[] {}; 11521 case -450004177: 11522 /* metadata */ return new String[] {}; 11523 case -843449847: 11524 /* fixture */ return new String[] {}; 11525 case -309425751: 11526 /* profile */ return new String[] { "Reference" }; 11527 case -1249586564: 11528 /* variable */ return new String[] {}; 11529 case 109329021: 11530 /* setup */ return new String[] {}; 11531 case 3556498: 11532 /* test */ return new String[] {}; 11533 case -1663474172: 11534 /* teardown */ return new String[] {}; 11535 default: 11536 return super.getTypesForProperty(hash, name); 11537 } 11538 11539 } 11540 11541 @Override 11542 public Base addChild(String name) throws FHIRException { 11543 if (name.equals("url")) { 11544 throw new FHIRException("Cannot call addChild on a singleton property TestScript.url"); 11545 } else if (name.equals("identifier")) { 11546 this.identifier = new Identifier(); 11547 return this.identifier; 11548 } else if (name.equals("version")) { 11549 throw new FHIRException("Cannot call addChild on a singleton property TestScript.version"); 11550 } else if (name.equals("name")) { 11551 throw new FHIRException("Cannot call addChild on a singleton property TestScript.name"); 11552 } else if (name.equals("title")) { 11553 throw new FHIRException("Cannot call addChild on a singleton property TestScript.title"); 11554 } else if (name.equals("status")) { 11555 throw new FHIRException("Cannot call addChild on a singleton property TestScript.status"); 11556 } else if (name.equals("experimental")) { 11557 throw new FHIRException("Cannot call addChild on a singleton property TestScript.experimental"); 11558 } else if (name.equals("date")) { 11559 throw new FHIRException("Cannot call addChild on a singleton property TestScript.date"); 11560 } else if (name.equals("publisher")) { 11561 throw new FHIRException("Cannot call addChild on a singleton property TestScript.publisher"); 11562 } else if (name.equals("contact")) { 11563 return addContact(); 11564 } else if (name.equals("description")) { 11565 throw new FHIRException("Cannot call addChild on a singleton property TestScript.description"); 11566 } else if (name.equals("useContext")) { 11567 return addUseContext(); 11568 } else if (name.equals("jurisdiction")) { 11569 return addJurisdiction(); 11570 } else if (name.equals("purpose")) { 11571 throw new FHIRException("Cannot call addChild on a singleton property TestScript.purpose"); 11572 } else if (name.equals("copyright")) { 11573 throw new FHIRException("Cannot call addChild on a singleton property TestScript.copyright"); 11574 } else if (name.equals("origin")) { 11575 return addOrigin(); 11576 } else if (name.equals("destination")) { 11577 return addDestination(); 11578 } else if (name.equals("metadata")) { 11579 this.metadata = new TestScriptMetadataComponent(); 11580 return this.metadata; 11581 } else if (name.equals("fixture")) { 11582 return addFixture(); 11583 } else if (name.equals("profile")) { 11584 return addProfile(); 11585 } else if (name.equals("variable")) { 11586 return addVariable(); 11587 } else if (name.equals("setup")) { 11588 this.setup = new TestScriptSetupComponent(); 11589 return this.setup; 11590 } else if (name.equals("test")) { 11591 return addTest(); 11592 } else if (name.equals("teardown")) { 11593 this.teardown = new TestScriptTeardownComponent(); 11594 return this.teardown; 11595 } else 11596 return super.addChild(name); 11597 } 11598 11599 public String fhirType() { 11600 return "TestScript"; 11601 11602 } 11603 11604 public TestScript copy() { 11605 TestScript dst = new TestScript(); 11606 copyValues(dst); 11607 return dst; 11608 } 11609 11610 public void copyValues(TestScript dst) { 11611 super.copyValues(dst); 11612 dst.url = url == null ? null : url.copy(); 11613 dst.identifier = identifier == null ? null : identifier.copy(); 11614 dst.version = version == null ? null : version.copy(); 11615 dst.name = name == null ? null : name.copy(); 11616 dst.title = title == null ? null : title.copy(); 11617 dst.status = status == null ? null : status.copy(); 11618 dst.experimental = experimental == null ? null : experimental.copy(); 11619 dst.date = date == null ? null : date.copy(); 11620 dst.publisher = publisher == null ? null : publisher.copy(); 11621 if (contact != null) { 11622 dst.contact = new ArrayList<ContactDetail>(); 11623 for (ContactDetail i : contact) 11624 dst.contact.add(i.copy()); 11625 } 11626 ; 11627 dst.description = description == null ? null : description.copy(); 11628 if (useContext != null) { 11629 dst.useContext = new ArrayList<UsageContext>(); 11630 for (UsageContext i : useContext) 11631 dst.useContext.add(i.copy()); 11632 } 11633 ; 11634 if (jurisdiction != null) { 11635 dst.jurisdiction = new ArrayList<CodeableConcept>(); 11636 for (CodeableConcept i : jurisdiction) 11637 dst.jurisdiction.add(i.copy()); 11638 } 11639 ; 11640 dst.purpose = purpose == null ? null : purpose.copy(); 11641 dst.copyright = copyright == null ? null : copyright.copy(); 11642 if (origin != null) { 11643 dst.origin = new ArrayList<TestScriptOriginComponent>(); 11644 for (TestScriptOriginComponent i : origin) 11645 dst.origin.add(i.copy()); 11646 } 11647 ; 11648 if (destination != null) { 11649 dst.destination = new ArrayList<TestScriptDestinationComponent>(); 11650 for (TestScriptDestinationComponent i : destination) 11651 dst.destination.add(i.copy()); 11652 } 11653 ; 11654 dst.metadata = metadata == null ? null : metadata.copy(); 11655 if (fixture != null) { 11656 dst.fixture = new ArrayList<TestScriptFixtureComponent>(); 11657 for (TestScriptFixtureComponent i : fixture) 11658 dst.fixture.add(i.copy()); 11659 } 11660 ; 11661 if (profile != null) { 11662 dst.profile = new ArrayList<Reference>(); 11663 for (Reference i : profile) 11664 dst.profile.add(i.copy()); 11665 } 11666 ; 11667 if (variable != null) { 11668 dst.variable = new ArrayList<TestScriptVariableComponent>(); 11669 for (TestScriptVariableComponent i : variable) 11670 dst.variable.add(i.copy()); 11671 } 11672 ; 11673 dst.setup = setup == null ? null : setup.copy(); 11674 if (test != null) { 11675 dst.test = new ArrayList<TestScriptTestComponent>(); 11676 for (TestScriptTestComponent i : test) 11677 dst.test.add(i.copy()); 11678 } 11679 ; 11680 dst.teardown = teardown == null ? null : teardown.copy(); 11681 } 11682 11683 protected TestScript typedCopy() { 11684 return copy(); 11685 } 11686 11687 @Override 11688 public boolean equalsDeep(Base other_) { 11689 if (!super.equalsDeep(other_)) 11690 return false; 11691 if (!(other_ instanceof TestScript)) 11692 return false; 11693 TestScript o = (TestScript) other_; 11694 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) 11695 && compareDeep(copyright, o.copyright, true) && compareDeep(origin, o.origin, true) 11696 && compareDeep(destination, o.destination, true) && compareDeep(metadata, o.metadata, true) 11697 && compareDeep(fixture, o.fixture, true) && compareDeep(profile, o.profile, true) 11698 && compareDeep(variable, o.variable, true) && compareDeep(setup, o.setup, true) 11699 && compareDeep(test, o.test, true) && compareDeep(teardown, o.teardown, true); 11700 } 11701 11702 @Override 11703 public boolean equalsShallow(Base other_) { 11704 if (!super.equalsShallow(other_)) 11705 return false; 11706 if (!(other_ instanceof TestScript)) 11707 return false; 11708 TestScript o = (TestScript) other_; 11709 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true); 11710 } 11711 11712 public boolean isEmpty() { 11713 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright, origin, destination, 11714 metadata, fixture, profile, variable, setup, test, teardown); 11715 } 11716 11717 @Override 11718 public ResourceType getResourceType() { 11719 return ResourceType.TestScript; 11720 } 11721 11722 /** 11723 * Search parameter: <b>date</b> 11724 * <p> 11725 * Description: <b>The test script publication date</b><br> 11726 * Type: <b>date</b><br> 11727 * Path: <b>TestScript.date</b><br> 11728 * </p> 11729 */ 11730 @SearchParamDefinition(name = "date", path = "TestScript.date", description = "The test script publication date", type = "date") 11731 public static final String SP_DATE = "date"; 11732 /** 11733 * <b>Fluent Client</b> search parameter constant for <b>date</b> 11734 * <p> 11735 * Description: <b>The test script publication date</b><br> 11736 * Type: <b>date</b><br> 11737 * Path: <b>TestScript.date</b><br> 11738 * </p> 11739 */ 11740 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 11741 SP_DATE); 11742 11743 /** 11744 * Search parameter: <b>identifier</b> 11745 * <p> 11746 * Description: <b>External identifier for the test script</b><br> 11747 * Type: <b>token</b><br> 11748 * Path: <b>TestScript.identifier</b><br> 11749 * </p> 11750 */ 11751 @SearchParamDefinition(name = "identifier", path = "TestScript.identifier", description = "External identifier for the test script", type = "token") 11752 public static final String SP_IDENTIFIER = "identifier"; 11753 /** 11754 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 11755 * <p> 11756 * Description: <b>External identifier for the test script</b><br> 11757 * Type: <b>token</b><br> 11758 * Path: <b>TestScript.identifier</b><br> 11759 * </p> 11760 */ 11761 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11762 SP_IDENTIFIER); 11763 11764 /** 11765 * Search parameter: <b>context-type-value</b> 11766 * <p> 11767 * Description: <b>A use context type and value assigned to the test 11768 * script</b><br> 11769 * Type: <b>composite</b><br> 11770 * Path: <b></b><br> 11771 * </p> 11772 */ 11773 @SearchParamDefinition(name = "context-type-value", path = "TestScript.useContext", description = "A use context type and value assigned to the test script", type = "composite", compositeOf = { 11774 "context-type", "context" }) 11775 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 11776 /** 11777 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 11778 * <p> 11779 * Description: <b>A use context type and value assigned to the test 11780 * script</b><br> 11781 * Type: <b>composite</b><br> 11782 * Path: <b></b><br> 11783 * </p> 11784 */ 11785 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 11786 SP_CONTEXT_TYPE_VALUE); 11787 11788 /** 11789 * Search parameter: <b>jurisdiction</b> 11790 * <p> 11791 * Description: <b>Intended jurisdiction for the test script</b><br> 11792 * Type: <b>token</b><br> 11793 * Path: <b>TestScript.jurisdiction</b><br> 11794 * </p> 11795 */ 11796 @SearchParamDefinition(name = "jurisdiction", path = "TestScript.jurisdiction", description = "Intended jurisdiction for the test script", type = "token") 11797 public static final String SP_JURISDICTION = "jurisdiction"; 11798 /** 11799 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 11800 * <p> 11801 * Description: <b>Intended jurisdiction for the test script</b><br> 11802 * Type: <b>token</b><br> 11803 * Path: <b>TestScript.jurisdiction</b><br> 11804 * </p> 11805 */ 11806 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11807 SP_JURISDICTION); 11808 11809 /** 11810 * Search parameter: <b>description</b> 11811 * <p> 11812 * Description: <b>The description of the test script</b><br> 11813 * Type: <b>string</b><br> 11814 * Path: <b>TestScript.description</b><br> 11815 * </p> 11816 */ 11817 @SearchParamDefinition(name = "description", path = "TestScript.description", description = "The description of the test script", type = "string") 11818 public static final String SP_DESCRIPTION = "description"; 11819 /** 11820 * <b>Fluent Client</b> search parameter constant for <b>description</b> 11821 * <p> 11822 * Description: <b>The description of the test script</b><br> 11823 * Type: <b>string</b><br> 11824 * Path: <b>TestScript.description</b><br> 11825 * </p> 11826 */ 11827 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 11828 SP_DESCRIPTION); 11829 11830 /** 11831 * Search parameter: <b>testscript-capability</b> 11832 * <p> 11833 * Description: <b>TestScript required and validated capability</b><br> 11834 * Type: <b>string</b><br> 11835 * Path: <b>TestScript.metadata.capability.description</b><br> 11836 * </p> 11837 */ 11838 @SearchParamDefinition(name = "testscript-capability", path = "TestScript.metadata.capability.description", description = "TestScript required and validated capability", type = "string") 11839 public static final String SP_TESTSCRIPT_CAPABILITY = "testscript-capability"; 11840 /** 11841 * <b>Fluent Client</b> search parameter constant for 11842 * <b>testscript-capability</b> 11843 * <p> 11844 * Description: <b>TestScript required and validated capability</b><br> 11845 * Type: <b>string</b><br> 11846 * Path: <b>TestScript.metadata.capability.description</b><br> 11847 * </p> 11848 */ 11849 public static final ca.uhn.fhir.rest.gclient.StringClientParam TESTSCRIPT_CAPABILITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 11850 SP_TESTSCRIPT_CAPABILITY); 11851 11852 /** 11853 * Search parameter: <b>context-type</b> 11854 * <p> 11855 * Description: <b>A type of use context assigned to the test script</b><br> 11856 * Type: <b>token</b><br> 11857 * Path: <b>TestScript.useContext.code</b><br> 11858 * </p> 11859 */ 11860 @SearchParamDefinition(name = "context-type", path = "TestScript.useContext.code", description = "A type of use context assigned to the test script", type = "token") 11861 public static final String SP_CONTEXT_TYPE = "context-type"; 11862 /** 11863 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 11864 * <p> 11865 * Description: <b>A type of use context assigned to the test script</b><br> 11866 * Type: <b>token</b><br> 11867 * Path: <b>TestScript.useContext.code</b><br> 11868 * </p> 11869 */ 11870 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11871 SP_CONTEXT_TYPE); 11872 11873 /** 11874 * Search parameter: <b>title</b> 11875 * <p> 11876 * Description: <b>The human-friendly name of the test script</b><br> 11877 * Type: <b>string</b><br> 11878 * Path: <b>TestScript.title</b><br> 11879 * </p> 11880 */ 11881 @SearchParamDefinition(name = "title", path = "TestScript.title", description = "The human-friendly name of the test script", type = "string") 11882 public static final String SP_TITLE = "title"; 11883 /** 11884 * <b>Fluent Client</b> search parameter constant for <b>title</b> 11885 * <p> 11886 * Description: <b>The human-friendly name of the test script</b><br> 11887 * Type: <b>string</b><br> 11888 * Path: <b>TestScript.title</b><br> 11889 * </p> 11890 */ 11891 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 11892 SP_TITLE); 11893 11894 /** 11895 * Search parameter: <b>version</b> 11896 * <p> 11897 * Description: <b>The business version of the test script</b><br> 11898 * Type: <b>token</b><br> 11899 * Path: <b>TestScript.version</b><br> 11900 * </p> 11901 */ 11902 @SearchParamDefinition(name = "version", path = "TestScript.version", description = "The business version of the test script", type = "token") 11903 public static final String SP_VERSION = "version"; 11904 /** 11905 * <b>Fluent Client</b> search parameter constant for <b>version</b> 11906 * <p> 11907 * Description: <b>The business version of the test script</b><br> 11908 * Type: <b>token</b><br> 11909 * Path: <b>TestScript.version</b><br> 11910 * </p> 11911 */ 11912 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 11913 SP_VERSION); 11914 11915 /** 11916 * Search parameter: <b>url</b> 11917 * <p> 11918 * Description: <b>The uri that identifies the test script</b><br> 11919 * Type: <b>uri</b><br> 11920 * Path: <b>TestScript.url</b><br> 11921 * </p> 11922 */ 11923 @SearchParamDefinition(name = "url", path = "TestScript.url", description = "The uri that identifies the test script", type = "uri") 11924 public static final String SP_URL = "url"; 11925 /** 11926 * <b>Fluent Client</b> search parameter constant for <b>url</b> 11927 * <p> 11928 * Description: <b>The uri that identifies the test script</b><br> 11929 * Type: <b>uri</b><br> 11930 * Path: <b>TestScript.url</b><br> 11931 * </p> 11932 */ 11933 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 11934 11935 /** 11936 * Search parameter: <b>context-quantity</b> 11937 * <p> 11938 * Description: <b>A quantity- or range-valued use context assigned to the test 11939 * script</b><br> 11940 * Type: <b>quantity</b><br> 11941 * Path: <b>TestScript.useContext.valueQuantity, 11942 * TestScript.useContext.valueRange</b><br> 11943 * </p> 11944 */ 11945 @SearchParamDefinition(name = "context-quantity", path = "(TestScript.useContext.value as Quantity) | (TestScript.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the test script", type = "quantity") 11946 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 11947 /** 11948 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 11949 * <p> 11950 * Description: <b>A quantity- or range-valued use context assigned to the test 11951 * script</b><br> 11952 * Type: <b>quantity</b><br> 11953 * Path: <b>TestScript.useContext.valueQuantity, 11954 * TestScript.useContext.valueRange</b><br> 11955 * </p> 11956 */ 11957 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 11958 SP_CONTEXT_QUANTITY); 11959 11960 /** 11961 * Search parameter: <b>name</b> 11962 * <p> 11963 * Description: <b>Computationally friendly name of the test script</b><br> 11964 * Type: <b>string</b><br> 11965 * Path: <b>TestScript.name</b><br> 11966 * </p> 11967 */ 11968 @SearchParamDefinition(name = "name", path = "TestScript.name", description = "Computationally friendly name of the test script", type = "string") 11969 public static final String SP_NAME = "name"; 11970 /** 11971 * <b>Fluent Client</b> search parameter constant for <b>name</b> 11972 * <p> 11973 * Description: <b>Computationally friendly name of the test script</b><br> 11974 * Type: <b>string</b><br> 11975 * Path: <b>TestScript.name</b><br> 11976 * </p> 11977 */ 11978 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 11979 SP_NAME); 11980 11981 /** 11982 * Search parameter: <b>context</b> 11983 * <p> 11984 * Description: <b>A use context assigned to the test script</b><br> 11985 * Type: <b>token</b><br> 11986 * Path: <b>TestScript.useContext.valueCodeableConcept</b><br> 11987 * </p> 11988 */ 11989 @SearchParamDefinition(name = "context", path = "(TestScript.useContext.value as CodeableConcept)", description = "A use context assigned to the test script", type = "token") 11990 public static final String SP_CONTEXT = "context"; 11991 /** 11992 * <b>Fluent Client</b> search parameter constant for <b>context</b> 11993 * <p> 11994 * Description: <b>A use context assigned to the test script</b><br> 11995 * Type: <b>token</b><br> 11996 * Path: <b>TestScript.useContext.valueCodeableConcept</b><br> 11997 * </p> 11998 */ 11999 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 12000 SP_CONTEXT); 12001 12002 /** 12003 * Search parameter: <b>publisher</b> 12004 * <p> 12005 * Description: <b>Name of the publisher of the test script</b><br> 12006 * Type: <b>string</b><br> 12007 * Path: <b>TestScript.publisher</b><br> 12008 * </p> 12009 */ 12010 @SearchParamDefinition(name = "publisher", path = "TestScript.publisher", description = "Name of the publisher of the test script", type = "string") 12011 public static final String SP_PUBLISHER = "publisher"; 12012 /** 12013 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 12014 * <p> 12015 * Description: <b>Name of the publisher of the test script</b><br> 12016 * Type: <b>string</b><br> 12017 * Path: <b>TestScript.publisher</b><br> 12018 * </p> 12019 */ 12020 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 12021 SP_PUBLISHER); 12022 12023 /** 12024 * Search parameter: <b>context-type-quantity</b> 12025 * <p> 12026 * Description: <b>A use context type and quantity- or range-based value 12027 * assigned to the test script</b><br> 12028 * Type: <b>composite</b><br> 12029 * Path: <b></b><br> 12030 * </p> 12031 */ 12032 @SearchParamDefinition(name = "context-type-quantity", path = "TestScript.useContext", description = "A use context type and quantity- or range-based value assigned to the test script", type = "composite", compositeOf = { 12033 "context-type", "context-quantity" }) 12034 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 12035 /** 12036 * <b>Fluent Client</b> search parameter constant for 12037 * <b>context-type-quantity</b> 12038 * <p> 12039 * Description: <b>A use context type and quantity- or range-based value 12040 * assigned to the test script</b><br> 12041 * Type: <b>composite</b><br> 12042 * Path: <b></b><br> 12043 * </p> 12044 */ 12045 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 12046 SP_CONTEXT_TYPE_QUANTITY); 12047 12048 /** 12049 * Search parameter: <b>status</b> 12050 * <p> 12051 * Description: <b>The current status of the test script</b><br> 12052 * Type: <b>token</b><br> 12053 * Path: <b>TestScript.status</b><br> 12054 * </p> 12055 */ 12056 @SearchParamDefinition(name = "status", path = "TestScript.status", description = "The current status of the test script", type = "token") 12057 public static final String SP_STATUS = "status"; 12058 /** 12059 * <b>Fluent Client</b> search parameter constant for <b>status</b> 12060 * <p> 12061 * Description: <b>The current status of the test script</b><br> 12062 * Type: <b>token</b><br> 12063 * Path: <b>TestScript.status</b><br> 12064 * </p> 12065 */ 12066 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 12067 SP_STATUS); 12068 12069}