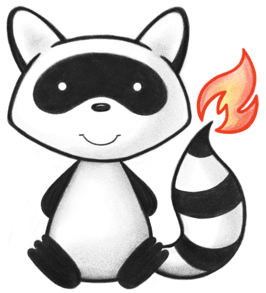
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.DatatypeDef; 046import ca.uhn.fhir.model.api.annotation.Description; 047 048/** 049 * Specifies an event that may occur multiple times. Timing schedules are used 050 * to record when things are planned, expected or requested to occur. The most 051 * common usage is in dosage instructions for medications. They are also used 052 * when planning care of various kinds, and may be used for reporting the 053 * schedule to which past regular activities were carried out. 054 */ 055@DatatypeDef(name = "Timing") 056public class Timing extends BackboneType implements ICompositeType { 057 058 public enum UnitsOfTime { 059 /** 060 * null 061 */ 062 S, 063 /** 064 * null 065 */ 066 MIN, 067 /** 068 * null 069 */ 070 H, 071 /** 072 * null 073 */ 074 D, 075 /** 076 * null 077 */ 078 WK, 079 /** 080 * null 081 */ 082 MO, 083 /** 084 * null 085 */ 086 A, 087 /** 088 * added to help the parsers with the generic types 089 */ 090 NULL; 091 092 public static UnitsOfTime fromCode(String codeString) throws FHIRException { 093 if (codeString == null || "".equals(codeString)) 094 return null; 095 if ("s".equals(codeString)) 096 return S; 097 if ("min".equals(codeString)) 098 return MIN; 099 if ("h".equals(codeString)) 100 return H; 101 if ("d".equals(codeString)) 102 return D; 103 if ("wk".equals(codeString)) 104 return WK; 105 if ("mo".equals(codeString)) 106 return MO; 107 if ("a".equals(codeString)) 108 return A; 109 if (Configuration.isAcceptInvalidEnums()) 110 return null; 111 else 112 throw new FHIRException("Unknown UnitsOfTime code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case S: 118 return "s"; 119 case MIN: 120 return "min"; 121 case H: 122 return "h"; 123 case D: 124 return "d"; 125 case WK: 126 return "wk"; 127 case MO: 128 return "mo"; 129 case A: 130 return "a"; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getSystem() { 139 switch (this) { 140 case S: 141 return "http://unitsofmeasure.org"; 142 case MIN: 143 return "http://unitsofmeasure.org"; 144 case H: 145 return "http://unitsofmeasure.org"; 146 case D: 147 return "http://unitsofmeasure.org"; 148 case WK: 149 return "http://unitsofmeasure.org"; 150 case MO: 151 return "http://unitsofmeasure.org"; 152 case A: 153 return "http://unitsofmeasure.org"; 154 case NULL: 155 return null; 156 default: 157 return "?"; 158 } 159 } 160 161 public String getDefinition() { 162 switch (this) { 163 case S: 164 return ""; 165 case MIN: 166 return ""; 167 case H: 168 return ""; 169 case D: 170 return ""; 171 case WK: 172 return ""; 173 case MO: 174 return ""; 175 case A: 176 return ""; 177 case NULL: 178 return null; 179 default: 180 return "?"; 181 } 182 } 183 184 public String getDisplay() { 185 switch (this) { 186 case S: 187 return "second"; 188 case MIN: 189 return "minute"; 190 case H: 191 return "hour"; 192 case D: 193 return "day"; 194 case WK: 195 return "week"; 196 case MO: 197 return "month"; 198 case A: 199 return "year"; 200 case NULL: 201 return null; 202 default: 203 return "?"; 204 } 205 } 206 } 207 208 public static class UnitsOfTimeEnumFactory implements EnumFactory<UnitsOfTime> { 209 public UnitsOfTime fromCode(String codeString) throws IllegalArgumentException { 210 if (codeString == null || "".equals(codeString)) 211 if (codeString == null || "".equals(codeString)) 212 return null; 213 if ("s".equals(codeString)) 214 return UnitsOfTime.S; 215 if ("min".equals(codeString)) 216 return UnitsOfTime.MIN; 217 if ("h".equals(codeString)) 218 return UnitsOfTime.H; 219 if ("d".equals(codeString)) 220 return UnitsOfTime.D; 221 if ("wk".equals(codeString)) 222 return UnitsOfTime.WK; 223 if ("mo".equals(codeString)) 224 return UnitsOfTime.MO; 225 if ("a".equals(codeString)) 226 return UnitsOfTime.A; 227 throw new IllegalArgumentException("Unknown UnitsOfTime code '" + codeString + "'"); 228 } 229 230 public Enumeration<UnitsOfTime> fromType(PrimitiveType<?> code) throws FHIRException { 231 if (code == null) 232 return null; 233 if (code.isEmpty()) 234 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.NULL, code); 235 String codeString = code.asStringValue(); 236 if (codeString == null || "".equals(codeString)) 237 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.NULL, code); 238 if ("s".equals(codeString)) 239 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.S, code); 240 if ("min".equals(codeString)) 241 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MIN, code); 242 if ("h".equals(codeString)) 243 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.H, code); 244 if ("d".equals(codeString)) 245 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.D, code); 246 if ("wk".equals(codeString)) 247 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.WK, code); 248 if ("mo".equals(codeString)) 249 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.MO, code); 250 if ("a".equals(codeString)) 251 return new Enumeration<UnitsOfTime>(this, UnitsOfTime.A, code); 252 throw new FHIRException("Unknown UnitsOfTime code '" + codeString + "'"); 253 } 254 255 public String toCode(UnitsOfTime code) { 256 if (code == UnitsOfTime.NULL) 257 return null; 258 if (code == UnitsOfTime.S) 259 return "s"; 260 if (code == UnitsOfTime.MIN) 261 return "min"; 262 if (code == UnitsOfTime.H) 263 return "h"; 264 if (code == UnitsOfTime.D) 265 return "d"; 266 if (code == UnitsOfTime.WK) 267 return "wk"; 268 if (code == UnitsOfTime.MO) 269 return "mo"; 270 if (code == UnitsOfTime.A) 271 return "a"; 272 return "?"; 273 } 274 275 public String toSystem(UnitsOfTime code) { 276 return code.getSystem(); 277 } 278 } 279 280 public enum DayOfWeek { 281 /** 282 * Monday. 283 */ 284 MON, 285 /** 286 * Tuesday. 287 */ 288 TUE, 289 /** 290 * Wednesday. 291 */ 292 WED, 293 /** 294 * Thursday. 295 */ 296 THU, 297 /** 298 * Friday. 299 */ 300 FRI, 301 /** 302 * Saturday. 303 */ 304 SAT, 305 /** 306 * Sunday. 307 */ 308 SUN, 309 /** 310 * added to help the parsers with the generic types 311 */ 312 NULL; 313 314 public static DayOfWeek fromCode(String codeString) throws FHIRException { 315 if (codeString == null || "".equals(codeString)) 316 return null; 317 if ("mon".equals(codeString)) 318 return MON; 319 if ("tue".equals(codeString)) 320 return TUE; 321 if ("wed".equals(codeString)) 322 return WED; 323 if ("thu".equals(codeString)) 324 return THU; 325 if ("fri".equals(codeString)) 326 return FRI; 327 if ("sat".equals(codeString)) 328 return SAT; 329 if ("sun".equals(codeString)) 330 return SUN; 331 if (Configuration.isAcceptInvalidEnums()) 332 return null; 333 else 334 throw new FHIRException("Unknown DayOfWeek code '" + codeString + "'"); 335 } 336 337 public String toCode() { 338 switch (this) { 339 case MON: 340 return "mon"; 341 case TUE: 342 return "tue"; 343 case WED: 344 return "wed"; 345 case THU: 346 return "thu"; 347 case FRI: 348 return "fri"; 349 case SAT: 350 return "sat"; 351 case SUN: 352 return "sun"; 353 case NULL: 354 return null; 355 default: 356 return "?"; 357 } 358 } 359 360 public String getSystem() { 361 switch (this) { 362 case MON: 363 return "http://hl7.org/fhir/days-of-week"; 364 case TUE: 365 return "http://hl7.org/fhir/days-of-week"; 366 case WED: 367 return "http://hl7.org/fhir/days-of-week"; 368 case THU: 369 return "http://hl7.org/fhir/days-of-week"; 370 case FRI: 371 return "http://hl7.org/fhir/days-of-week"; 372 case SAT: 373 return "http://hl7.org/fhir/days-of-week"; 374 case SUN: 375 return "http://hl7.org/fhir/days-of-week"; 376 case NULL: 377 return null; 378 default: 379 return "?"; 380 } 381 } 382 383 public String getDefinition() { 384 switch (this) { 385 case MON: 386 return "Monday."; 387 case TUE: 388 return "Tuesday."; 389 case WED: 390 return "Wednesday."; 391 case THU: 392 return "Thursday."; 393 case FRI: 394 return "Friday."; 395 case SAT: 396 return "Saturday."; 397 case SUN: 398 return "Sunday."; 399 case NULL: 400 return null; 401 default: 402 return "?"; 403 } 404 } 405 406 public String getDisplay() { 407 switch (this) { 408 case MON: 409 return "Monday"; 410 case TUE: 411 return "Tuesday"; 412 case WED: 413 return "Wednesday"; 414 case THU: 415 return "Thursday"; 416 case FRI: 417 return "Friday"; 418 case SAT: 419 return "Saturday"; 420 case SUN: 421 return "Sunday"; 422 case NULL: 423 return null; 424 default: 425 return "?"; 426 } 427 } 428 } 429 430 public static class DayOfWeekEnumFactory implements EnumFactory<DayOfWeek> { 431 public DayOfWeek fromCode(String codeString) throws IllegalArgumentException { 432 if (codeString == null || "".equals(codeString)) 433 if (codeString == null || "".equals(codeString)) 434 return null; 435 if ("mon".equals(codeString)) 436 return DayOfWeek.MON; 437 if ("tue".equals(codeString)) 438 return DayOfWeek.TUE; 439 if ("wed".equals(codeString)) 440 return DayOfWeek.WED; 441 if ("thu".equals(codeString)) 442 return DayOfWeek.THU; 443 if ("fri".equals(codeString)) 444 return DayOfWeek.FRI; 445 if ("sat".equals(codeString)) 446 return DayOfWeek.SAT; 447 if ("sun".equals(codeString)) 448 return DayOfWeek.SUN; 449 throw new IllegalArgumentException("Unknown DayOfWeek code '" + codeString + "'"); 450 } 451 452 public Enumeration<DayOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 453 if (code == null) 454 return null; 455 if (code.isEmpty()) 456 return new Enumeration<DayOfWeek>(this, DayOfWeek.NULL, code); 457 String codeString = code.asStringValue(); 458 if (codeString == null || "".equals(codeString)) 459 return new Enumeration<DayOfWeek>(this, DayOfWeek.NULL, code); 460 if ("mon".equals(codeString)) 461 return new Enumeration<DayOfWeek>(this, DayOfWeek.MON, code); 462 if ("tue".equals(codeString)) 463 return new Enumeration<DayOfWeek>(this, DayOfWeek.TUE, code); 464 if ("wed".equals(codeString)) 465 return new Enumeration<DayOfWeek>(this, DayOfWeek.WED, code); 466 if ("thu".equals(codeString)) 467 return new Enumeration<DayOfWeek>(this, DayOfWeek.THU, code); 468 if ("fri".equals(codeString)) 469 return new Enumeration<DayOfWeek>(this, DayOfWeek.FRI, code); 470 if ("sat".equals(codeString)) 471 return new Enumeration<DayOfWeek>(this, DayOfWeek.SAT, code); 472 if ("sun".equals(codeString)) 473 return new Enumeration<DayOfWeek>(this, DayOfWeek.SUN, code); 474 throw new FHIRException("Unknown DayOfWeek code '" + codeString + "'"); 475 } 476 477 public String toCode(DayOfWeek code) { 478 if (code == DayOfWeek.NULL) 479 return null; 480 if (code == DayOfWeek.MON) 481 return "mon"; 482 if (code == DayOfWeek.TUE) 483 return "tue"; 484 if (code == DayOfWeek.WED) 485 return "wed"; 486 if (code == DayOfWeek.THU) 487 return "thu"; 488 if (code == DayOfWeek.FRI) 489 return "fri"; 490 if (code == DayOfWeek.SAT) 491 return "sat"; 492 if (code == DayOfWeek.SUN) 493 return "sun"; 494 return "?"; 495 } 496 497 public String toSystem(DayOfWeek code) { 498 return code.getSystem(); 499 } 500 } 501 502 public enum EventTiming { 503 /** 504 * Event occurs during the morning. The exact time is unspecified and 505 * established by institution convention or patient interpretation. 506 */ 507 MORN, 508 /** 509 * Event occurs during the early morning. The exact time is unspecified and 510 * established by institution convention or patient interpretation. 511 */ 512 MORN_EARLY, 513 /** 514 * Event occurs during the late morning. The exact time is unspecified and 515 * established by institution convention or patient interpretation. 516 */ 517 MORN_LATE, 518 /** 519 * Event occurs around 12:00pm. The exact time is unspecified and established by 520 * institution convention or patient interpretation. 521 */ 522 NOON, 523 /** 524 * Event occurs during the afternoon. The exact time is unspecified and 525 * established by institution convention or patient interpretation. 526 */ 527 AFT, 528 /** 529 * Event occurs during the early afternoon. The exact time is unspecified and 530 * established by institution convention or patient interpretation. 531 */ 532 AFT_EARLY, 533 /** 534 * Event occurs during the late afternoon. The exact time is unspecified and 535 * established by institution convention or patient interpretation. 536 */ 537 AFT_LATE, 538 /** 539 * Event occurs during the evening. The exact time is unspecified and 540 * established by institution convention or patient interpretation. 541 */ 542 EVE, 543 /** 544 * Event occurs during the early evening. The exact time is unspecified and 545 * established by institution convention or patient interpretation. 546 */ 547 EVE_EARLY, 548 /** 549 * Event occurs during the late evening. The exact time is unspecified and 550 * established by institution convention or patient interpretation. 551 */ 552 EVE_LATE, 553 /** 554 * Event occurs during the night. The exact time is unspecified and established 555 * by institution convention or patient interpretation. 556 */ 557 NIGHT, 558 /** 559 * Event occurs [offset] after subject goes to sleep. The exact time is 560 * unspecified and established by institution convention or patient 561 * interpretation. 562 */ 563 PHS, 564 /** 565 * null 566 */ 567 HS, 568 /** 569 * null 570 */ 571 WAKE, 572 /** 573 * null 574 */ 575 C, 576 /** 577 * null 578 */ 579 CM, 580 /** 581 * null 582 */ 583 CD, 584 /** 585 * null 586 */ 587 CV, 588 /** 589 * null 590 */ 591 AC, 592 /** 593 * null 594 */ 595 ACM, 596 /** 597 * null 598 */ 599 ACD, 600 /** 601 * null 602 */ 603 ACV, 604 /** 605 * null 606 */ 607 PC, 608 /** 609 * null 610 */ 611 PCM, 612 /** 613 * null 614 */ 615 PCD, 616 /** 617 * null 618 */ 619 PCV, 620 /** 621 * added to help the parsers with the generic types 622 */ 623 NULL; 624 625 public static EventTiming fromCode(String codeString) throws FHIRException { 626 if (codeString == null || "".equals(codeString)) 627 return null; 628 if ("MORN".equals(codeString)) 629 return MORN; 630 if ("MORN.early".equals(codeString)) 631 return MORN_EARLY; 632 if ("MORN.late".equals(codeString)) 633 return MORN_LATE; 634 if ("NOON".equals(codeString)) 635 return NOON; 636 if ("AFT".equals(codeString)) 637 return AFT; 638 if ("AFT.early".equals(codeString)) 639 return AFT_EARLY; 640 if ("AFT.late".equals(codeString)) 641 return AFT_LATE; 642 if ("EVE".equals(codeString)) 643 return EVE; 644 if ("EVE.early".equals(codeString)) 645 return EVE_EARLY; 646 if ("EVE.late".equals(codeString)) 647 return EVE_LATE; 648 if ("NIGHT".equals(codeString)) 649 return NIGHT; 650 if ("PHS".equals(codeString)) 651 return PHS; 652 if ("HS".equals(codeString)) 653 return HS; 654 if ("WAKE".equals(codeString)) 655 return WAKE; 656 if ("C".equals(codeString)) 657 return C; 658 if ("CM".equals(codeString)) 659 return CM; 660 if ("CD".equals(codeString)) 661 return CD; 662 if ("CV".equals(codeString)) 663 return CV; 664 if ("AC".equals(codeString)) 665 return AC; 666 if ("ACM".equals(codeString)) 667 return ACM; 668 if ("ACD".equals(codeString)) 669 return ACD; 670 if ("ACV".equals(codeString)) 671 return ACV; 672 if ("PC".equals(codeString)) 673 return PC; 674 if ("PCM".equals(codeString)) 675 return PCM; 676 if ("PCD".equals(codeString)) 677 return PCD; 678 if ("PCV".equals(codeString)) 679 return PCV; 680 if (Configuration.isAcceptInvalidEnums()) 681 return null; 682 else 683 throw new FHIRException("Unknown EventTiming code '" + codeString + "'"); 684 } 685 686 public String toCode() { 687 switch (this) { 688 case MORN: 689 return "MORN"; 690 case MORN_EARLY: 691 return "MORN.early"; 692 case MORN_LATE: 693 return "MORN.late"; 694 case NOON: 695 return "NOON"; 696 case AFT: 697 return "AFT"; 698 case AFT_EARLY: 699 return "AFT.early"; 700 case AFT_LATE: 701 return "AFT.late"; 702 case EVE: 703 return "EVE"; 704 case EVE_EARLY: 705 return "EVE.early"; 706 case EVE_LATE: 707 return "EVE.late"; 708 case NIGHT: 709 return "NIGHT"; 710 case PHS: 711 return "PHS"; 712 case HS: 713 return "HS"; 714 case WAKE: 715 return "WAKE"; 716 case C: 717 return "C"; 718 case CM: 719 return "CM"; 720 case CD: 721 return "CD"; 722 case CV: 723 return "CV"; 724 case AC: 725 return "AC"; 726 case ACM: 727 return "ACM"; 728 case ACD: 729 return "ACD"; 730 case ACV: 731 return "ACV"; 732 case PC: 733 return "PC"; 734 case PCM: 735 return "PCM"; 736 case PCD: 737 return "PCD"; 738 case PCV: 739 return "PCV"; 740 case NULL: 741 return null; 742 default: 743 return "?"; 744 } 745 } 746 747 public String getSystem() { 748 switch (this) { 749 case MORN: 750 return "http://hl7.org/fhir/event-timing"; 751 case MORN_EARLY: 752 return "http://hl7.org/fhir/event-timing"; 753 case MORN_LATE: 754 return "http://hl7.org/fhir/event-timing"; 755 case NOON: 756 return "http://hl7.org/fhir/event-timing"; 757 case AFT: 758 return "http://hl7.org/fhir/event-timing"; 759 case AFT_EARLY: 760 return "http://hl7.org/fhir/event-timing"; 761 case AFT_LATE: 762 return "http://hl7.org/fhir/event-timing"; 763 case EVE: 764 return "http://hl7.org/fhir/event-timing"; 765 case EVE_EARLY: 766 return "http://hl7.org/fhir/event-timing"; 767 case EVE_LATE: 768 return "http://hl7.org/fhir/event-timing"; 769 case NIGHT: 770 return "http://hl7.org/fhir/event-timing"; 771 case PHS: 772 return "http://hl7.org/fhir/event-timing"; 773 case HS: 774 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 775 case WAKE: 776 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 777 case C: 778 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 779 case CM: 780 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 781 case CD: 782 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 783 case CV: 784 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 785 case AC: 786 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 787 case ACM: 788 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 789 case ACD: 790 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 791 case ACV: 792 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 793 case PC: 794 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 795 case PCM: 796 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 797 case PCD: 798 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 799 case PCV: 800 return "http://terminology.hl7.org/CodeSystem/v3-TimingEvent"; 801 case NULL: 802 return null; 803 default: 804 return "?"; 805 } 806 } 807 808 public String getDefinition() { 809 switch (this) { 810 case MORN: 811 return "Event occurs during the morning. The exact time is unspecified and established by institution convention or patient interpretation."; 812 case MORN_EARLY: 813 return "Event occurs during the early morning. The exact time is unspecified and established by institution convention or patient interpretation."; 814 case MORN_LATE: 815 return "Event occurs during the late morning. The exact time is unspecified and established by institution convention or patient interpretation."; 816 case NOON: 817 return "Event occurs around 12:00pm. The exact time is unspecified and established by institution convention or patient interpretation."; 818 case AFT: 819 return "Event occurs during the afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 820 case AFT_EARLY: 821 return "Event occurs during the early afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 822 case AFT_LATE: 823 return "Event occurs during the late afternoon. The exact time is unspecified and established by institution convention or patient interpretation."; 824 case EVE: 825 return "Event occurs during the evening. The exact time is unspecified and established by institution convention or patient interpretation."; 826 case EVE_EARLY: 827 return "Event occurs during the early evening. The exact time is unspecified and established by institution convention or patient interpretation."; 828 case EVE_LATE: 829 return "Event occurs during the late evening. The exact time is unspecified and established by institution convention or patient interpretation."; 830 case NIGHT: 831 return "Event occurs during the night. The exact time is unspecified and established by institution convention or patient interpretation."; 832 case PHS: 833 return "Event occurs [offset] after subject goes to sleep. The exact time is unspecified and established by institution convention or patient interpretation."; 834 case HS: 835 return ""; 836 case WAKE: 837 return ""; 838 case C: 839 return ""; 840 case CM: 841 return ""; 842 case CD: 843 return ""; 844 case CV: 845 return ""; 846 case AC: 847 return ""; 848 case ACM: 849 return ""; 850 case ACD: 851 return ""; 852 case ACV: 853 return ""; 854 case PC: 855 return ""; 856 case PCM: 857 return ""; 858 case PCD: 859 return ""; 860 case PCV: 861 return ""; 862 case NULL: 863 return null; 864 default: 865 return "?"; 866 } 867 } 868 869 public String getDisplay() { 870 switch (this) { 871 case MORN: 872 return "Morning"; 873 case MORN_EARLY: 874 return "Early Morning"; 875 case MORN_LATE: 876 return "Late Morning"; 877 case NOON: 878 return "Noon"; 879 case AFT: 880 return "Afternoon"; 881 case AFT_EARLY: 882 return "Early Afternoon"; 883 case AFT_LATE: 884 return "Late Afternoon"; 885 case EVE: 886 return "Evening"; 887 case EVE_EARLY: 888 return "Early Evening"; 889 case EVE_LATE: 890 return "Late Evening"; 891 case NIGHT: 892 return "Night"; 893 case PHS: 894 return "After Sleep"; 895 case HS: 896 return "HS"; 897 case WAKE: 898 return "WAKE"; 899 case C: 900 return "C"; 901 case CM: 902 return "CM"; 903 case CD: 904 return "CD"; 905 case CV: 906 return "CV"; 907 case AC: 908 return "AC"; 909 case ACM: 910 return "ACM"; 911 case ACD: 912 return "ACD"; 913 case ACV: 914 return "ACV"; 915 case PC: 916 return "PC"; 917 case PCM: 918 return "PCM"; 919 case PCD: 920 return "PCD"; 921 case PCV: 922 return "PCV"; 923 case NULL: 924 return null; 925 default: 926 return "?"; 927 } 928 } 929 } 930 931 public static class EventTimingEnumFactory implements EnumFactory<EventTiming> { 932 public EventTiming fromCode(String codeString) throws IllegalArgumentException { 933 if (codeString == null || "".equals(codeString)) 934 if (codeString == null || "".equals(codeString)) 935 return null; 936 if ("MORN".equals(codeString)) 937 return EventTiming.MORN; 938 if ("MORN.early".equals(codeString)) 939 return EventTiming.MORN_EARLY; 940 if ("MORN.late".equals(codeString)) 941 return EventTiming.MORN_LATE; 942 if ("NOON".equals(codeString)) 943 return EventTiming.NOON; 944 if ("AFT".equals(codeString)) 945 return EventTiming.AFT; 946 if ("AFT.early".equals(codeString)) 947 return EventTiming.AFT_EARLY; 948 if ("AFT.late".equals(codeString)) 949 return EventTiming.AFT_LATE; 950 if ("EVE".equals(codeString)) 951 return EventTiming.EVE; 952 if ("EVE.early".equals(codeString)) 953 return EventTiming.EVE_EARLY; 954 if ("EVE.late".equals(codeString)) 955 return EventTiming.EVE_LATE; 956 if ("NIGHT".equals(codeString)) 957 return EventTiming.NIGHT; 958 if ("PHS".equals(codeString)) 959 return EventTiming.PHS; 960 if ("HS".equals(codeString)) 961 return EventTiming.HS; 962 if ("WAKE".equals(codeString)) 963 return EventTiming.WAKE; 964 if ("C".equals(codeString)) 965 return EventTiming.C; 966 if ("CM".equals(codeString)) 967 return EventTiming.CM; 968 if ("CD".equals(codeString)) 969 return EventTiming.CD; 970 if ("CV".equals(codeString)) 971 return EventTiming.CV; 972 if ("AC".equals(codeString)) 973 return EventTiming.AC; 974 if ("ACM".equals(codeString)) 975 return EventTiming.ACM; 976 if ("ACD".equals(codeString)) 977 return EventTiming.ACD; 978 if ("ACV".equals(codeString)) 979 return EventTiming.ACV; 980 if ("PC".equals(codeString)) 981 return EventTiming.PC; 982 if ("PCM".equals(codeString)) 983 return EventTiming.PCM; 984 if ("PCD".equals(codeString)) 985 return EventTiming.PCD; 986 if ("PCV".equals(codeString)) 987 return EventTiming.PCV; 988 throw new IllegalArgumentException("Unknown EventTiming code '" + codeString + "'"); 989 } 990 991 public Enumeration<EventTiming> fromType(PrimitiveType<?> code) throws FHIRException { 992 if (code == null) 993 return null; 994 if (code.isEmpty()) 995 return new Enumeration<EventTiming>(this, EventTiming.NULL, code); 996 String codeString = code.asStringValue(); 997 if (codeString == null || "".equals(codeString)) 998 return new Enumeration<EventTiming>(this, EventTiming.NULL, code); 999 if ("MORN".equals(codeString)) 1000 return new Enumeration<EventTiming>(this, EventTiming.MORN, code); 1001 if ("MORN.early".equals(codeString)) 1002 return new Enumeration<EventTiming>(this, EventTiming.MORN_EARLY, code); 1003 if ("MORN.late".equals(codeString)) 1004 return new Enumeration<EventTiming>(this, EventTiming.MORN_LATE, code); 1005 if ("NOON".equals(codeString)) 1006 return new Enumeration<EventTiming>(this, EventTiming.NOON, code); 1007 if ("AFT".equals(codeString)) 1008 return new Enumeration<EventTiming>(this, EventTiming.AFT, code); 1009 if ("AFT.early".equals(codeString)) 1010 return new Enumeration<EventTiming>(this, EventTiming.AFT_EARLY, code); 1011 if ("AFT.late".equals(codeString)) 1012 return new Enumeration<EventTiming>(this, EventTiming.AFT_LATE, code); 1013 if ("EVE".equals(codeString)) 1014 return new Enumeration<EventTiming>(this, EventTiming.EVE, code); 1015 if ("EVE.early".equals(codeString)) 1016 return new Enumeration<EventTiming>(this, EventTiming.EVE_EARLY, code); 1017 if ("EVE.late".equals(codeString)) 1018 return new Enumeration<EventTiming>(this, EventTiming.EVE_LATE, code); 1019 if ("NIGHT".equals(codeString)) 1020 return new Enumeration<EventTiming>(this, EventTiming.NIGHT, code); 1021 if ("PHS".equals(codeString)) 1022 return new Enumeration<EventTiming>(this, EventTiming.PHS, code); 1023 if ("HS".equals(codeString)) 1024 return new Enumeration<EventTiming>(this, EventTiming.HS, code); 1025 if ("WAKE".equals(codeString)) 1026 return new Enumeration<EventTiming>(this, EventTiming.WAKE, code); 1027 if ("C".equals(codeString)) 1028 return new Enumeration<EventTiming>(this, EventTiming.C, code); 1029 if ("CM".equals(codeString)) 1030 return new Enumeration<EventTiming>(this, EventTiming.CM, code); 1031 if ("CD".equals(codeString)) 1032 return new Enumeration<EventTiming>(this, EventTiming.CD, code); 1033 if ("CV".equals(codeString)) 1034 return new Enumeration<EventTiming>(this, EventTiming.CV, code); 1035 if ("AC".equals(codeString)) 1036 return new Enumeration<EventTiming>(this, EventTiming.AC, code); 1037 if ("ACM".equals(codeString)) 1038 return new Enumeration<EventTiming>(this, EventTiming.ACM, code); 1039 if ("ACD".equals(codeString)) 1040 return new Enumeration<EventTiming>(this, EventTiming.ACD, code); 1041 if ("ACV".equals(codeString)) 1042 return new Enumeration<EventTiming>(this, EventTiming.ACV, code); 1043 if ("PC".equals(codeString)) 1044 return new Enumeration<EventTiming>(this, EventTiming.PC, code); 1045 if ("PCM".equals(codeString)) 1046 return new Enumeration<EventTiming>(this, EventTiming.PCM, code); 1047 if ("PCD".equals(codeString)) 1048 return new Enumeration<EventTiming>(this, EventTiming.PCD, code); 1049 if ("PCV".equals(codeString)) 1050 return new Enumeration<EventTiming>(this, EventTiming.PCV, code); 1051 throw new FHIRException("Unknown EventTiming code '" + codeString + "'"); 1052 } 1053 1054 public String toCode(EventTiming code) { 1055 if (code == EventTiming.NULL) 1056 return null; 1057 if (code == EventTiming.MORN) 1058 return "MORN"; 1059 if (code == EventTiming.MORN_EARLY) 1060 return "MORN.early"; 1061 if (code == EventTiming.MORN_LATE) 1062 return "MORN.late"; 1063 if (code == EventTiming.NOON) 1064 return "NOON"; 1065 if (code == EventTiming.AFT) 1066 return "AFT"; 1067 if (code == EventTiming.AFT_EARLY) 1068 return "AFT.early"; 1069 if (code == EventTiming.AFT_LATE) 1070 return "AFT.late"; 1071 if (code == EventTiming.EVE) 1072 return "EVE"; 1073 if (code == EventTiming.EVE_EARLY) 1074 return "EVE.early"; 1075 if (code == EventTiming.EVE_LATE) 1076 return "EVE.late"; 1077 if (code == EventTiming.NIGHT) 1078 return "NIGHT"; 1079 if (code == EventTiming.PHS) 1080 return "PHS"; 1081 if (code == EventTiming.HS) 1082 return "HS"; 1083 if (code == EventTiming.WAKE) 1084 return "WAKE"; 1085 if (code == EventTiming.C) 1086 return "C"; 1087 if (code == EventTiming.CM) 1088 return "CM"; 1089 if (code == EventTiming.CD) 1090 return "CD"; 1091 if (code == EventTiming.CV) 1092 return "CV"; 1093 if (code == EventTiming.AC) 1094 return "AC"; 1095 if (code == EventTiming.ACM) 1096 return "ACM"; 1097 if (code == EventTiming.ACD) 1098 return "ACD"; 1099 if (code == EventTiming.ACV) 1100 return "ACV"; 1101 if (code == EventTiming.PC) 1102 return "PC"; 1103 if (code == EventTiming.PCM) 1104 return "PCM"; 1105 if (code == EventTiming.PCD) 1106 return "PCD"; 1107 if (code == EventTiming.PCV) 1108 return "PCV"; 1109 return "?"; 1110 } 1111 1112 public String toSystem(EventTiming code) { 1113 return code.getSystem(); 1114 } 1115 } 1116 1117 @Block() 1118 public static class TimingRepeatComponent extends Element implements IBaseDatatypeElement { 1119 /** 1120 * Either a duration for the length of the timing schedule, a range of possible 1121 * length, or outer bounds for start and/or end limits of the timing schedule. 1122 */ 1123 @Child(name = "bounds", type = { Duration.class, Range.class, 1124 Period.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1125 @Description(shortDefinition = "Length/Range of lengths, or (Start and/or end) limits", formalDefinition = "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.") 1126 protected Type bounds; 1127 1128 /** 1129 * A total count of the desired number of repetitions across the duration of the 1130 * entire timing specification. If countMax is present, this element indicates 1131 * the lower bound of the allowed range of count values. 1132 */ 1133 @Child(name = "count", type = { 1134 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1135 @Description(shortDefinition = "Number of times to repeat", formalDefinition = "A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.") 1136 protected PositiveIntType count; 1137 1138 /** 1139 * If present, indicates that the count is a range - so to perform the action 1140 * between [count] and [countMax] times. 1141 */ 1142 @Child(name = "countMax", type = { 1143 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1144 @Description(shortDefinition = "Maximum number of times to repeat", formalDefinition = "If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.") 1145 protected PositiveIntType countMax; 1146 1147 /** 1148 * How long this thing happens for when it happens. If durationMax is present, 1149 * this element indicates the lower bound of the allowed range of the duration. 1150 */ 1151 @Child(name = "duration", type = { 1152 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1153 @Description(shortDefinition = "How long when it happens", formalDefinition = "How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.") 1154 protected DecimalType duration; 1155 1156 /** 1157 * If present, indicates that the duration is a range - so to perform the action 1158 * between [duration] and [durationMax] time length. 1159 */ 1160 @Child(name = "durationMax", type = { 1161 DecimalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1162 @Description(shortDefinition = "How long when it happens (Max)", formalDefinition = "If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.") 1163 protected DecimalType durationMax; 1164 1165 /** 1166 * The units of time for the duration, in UCUM units. 1167 */ 1168 @Child(name = "durationUnit", type = { 1169 CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1170 @Description(shortDefinition = "s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition = "The units of time for the duration, in UCUM units.") 1171 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/units-of-time") 1172 protected Enumeration<UnitsOfTime> durationUnit; 1173 1174 /** 1175 * The number of times to repeat the action within the specified period. If 1176 * frequencyMax is present, this element indicates the lower bound of the 1177 * allowed range of the frequency. 1178 */ 1179 @Child(name = "frequency", type = { 1180 PositiveIntType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1181 @Description(shortDefinition = "Event occurs frequency times per period", formalDefinition = "The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.") 1182 protected PositiveIntType frequency; 1183 1184 /** 1185 * If present, indicates that the frequency is a range - so to repeat between 1186 * [frequency] and [frequencyMax] times within the period or period range. 1187 */ 1188 @Child(name = "frequencyMax", type = { 1189 PositiveIntType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1190 @Description(shortDefinition = "Event occurs up to frequencyMax times per period", formalDefinition = "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.") 1191 protected PositiveIntType frequencyMax; 1192 1193 /** 1194 * Indicates the duration of time over which repetitions are to occur; e.g. to 1195 * express "3 times per day", 3 would be the frequency and "1 day" would be the 1196 * period. If periodMax is present, this element indicates the lower bound of 1197 * the allowed range of the period length. 1198 */ 1199 @Child(name = "period", type = { DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1200 @Description(shortDefinition = "Event occurs frequency times per period", formalDefinition = "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.") 1201 protected DecimalType period; 1202 1203 /** 1204 * If present, indicates that the period is a range from [period] to 1205 * [periodMax], allowing expressing concepts such as "do this once every 3-5 1206 * days. 1207 */ 1208 @Child(name = "periodMax", type = { 1209 DecimalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1210 @Description(shortDefinition = "Upper limit of period (3-4 hours)", formalDefinition = "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.") 1211 protected DecimalType periodMax; 1212 1213 /** 1214 * The units of time for the period in UCUM units. 1215 */ 1216 @Child(name = "periodUnit", type = { 1217 CodeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1218 @Description(shortDefinition = "s | min | h | d | wk | mo | a - unit of time (UCUM)", formalDefinition = "The units of time for the period in UCUM units.") 1219 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/units-of-time") 1220 protected Enumeration<UnitsOfTime> periodUnit; 1221 1222 /** 1223 * If one or more days of week is provided, then the action happens only on the 1224 * specified day(s). 1225 */ 1226 @Child(name = "dayOfWeek", type = { 1227 CodeType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1228 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "If one or more days of week is provided, then the action happens only on the specified day(s).") 1229 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/days-of-week") 1230 protected List<Enumeration<DayOfWeek>> dayOfWeek; 1231 1232 /** 1233 * Specified time of day for action to take place. 1234 */ 1235 @Child(name = "timeOfDay", type = { 1236 TimeType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1237 @Description(shortDefinition = "Time of day for action", formalDefinition = "Specified time of day for action to take place.") 1238 protected List<TimeType> timeOfDay; 1239 1240 /** 1241 * An approximate time period during the day, potentially linked to an event of 1242 * daily living that indicates when the action should occur. 1243 */ 1244 @Child(name = "when", type = { 1245 CodeType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1246 @Description(shortDefinition = "Code for time period of occurrence", formalDefinition = "An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.") 1247 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-timing") 1248 protected List<Enumeration<EventTiming>> when; 1249 1250 /** 1251 * The number of minutes from the event. If the event code does not indicate 1252 * whether the minutes is before or after the event, then the offset is assumed 1253 * to be after the event. 1254 */ 1255 @Child(name = "offset", type = { 1256 UnsignedIntType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 1257 @Description(shortDefinition = "Minutes from event (before or after)", formalDefinition = "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.") 1258 protected UnsignedIntType offset; 1259 1260 private static final long serialVersionUID = -900253756L; 1261 1262 /** 1263 * Constructor 1264 */ 1265 public TimingRepeatComponent() { 1266 super(); 1267 } 1268 1269 /** 1270 * @return {@link #bounds} (Either a duration for the length of the timing 1271 * schedule, a range of possible length, or outer bounds for start 1272 * and/or end limits of the timing schedule.) 1273 */ 1274 public Type getBounds() { 1275 return this.bounds; 1276 } 1277 1278 /** 1279 * @return {@link #bounds} (Either a duration for the length of the timing 1280 * schedule, a range of possible length, or outer bounds for start 1281 * and/or end limits of the timing schedule.) 1282 */ 1283 public Duration getBoundsDuration() throws FHIRException { 1284 if (this.bounds == null) 1285 this.bounds = new Duration(); 1286 if (!(this.bounds instanceof Duration)) 1287 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.bounds.getClass().getName() 1288 + " was encountered"); 1289 return (Duration) this.bounds; 1290 } 1291 1292 public boolean hasBoundsDuration() { 1293 return this != null && this.bounds instanceof Duration; 1294 } 1295 1296 /** 1297 * @return {@link #bounds} (Either a duration for the length of the timing 1298 * schedule, a range of possible length, or outer bounds for start 1299 * and/or end limits of the timing schedule.) 1300 */ 1301 public Range getBoundsRange() throws FHIRException { 1302 if (this.bounds == null) 1303 this.bounds = new Range(); 1304 if (!(this.bounds instanceof Range)) 1305 throw new FHIRException( 1306 "Type mismatch: the type Range was expected, but " + this.bounds.getClass().getName() + " was encountered"); 1307 return (Range) this.bounds; 1308 } 1309 1310 public boolean hasBoundsRange() { 1311 return this != null && this.bounds instanceof Range; 1312 } 1313 1314 /** 1315 * @return {@link #bounds} (Either a duration for the length of the timing 1316 * schedule, a range of possible length, or outer bounds for start 1317 * and/or end limits of the timing schedule.) 1318 */ 1319 public Period getBoundsPeriod() throws FHIRException { 1320 if (this.bounds == null) 1321 this.bounds = new Period(); 1322 if (!(this.bounds instanceof Period)) 1323 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.bounds.getClass().getName() 1324 + " was encountered"); 1325 return (Period) this.bounds; 1326 } 1327 1328 public boolean hasBoundsPeriod() { 1329 return this != null && this.bounds instanceof Period; 1330 } 1331 1332 public boolean hasBounds() { 1333 return this.bounds != null && !this.bounds.isEmpty(); 1334 } 1335 1336 /** 1337 * @param value {@link #bounds} (Either a duration for the length of the timing 1338 * schedule, a range of possible length, or outer bounds for start 1339 * and/or end limits of the timing schedule.) 1340 */ 1341 public TimingRepeatComponent setBounds(Type value) { 1342 if (value != null && !(value instanceof Duration || value instanceof Range || value instanceof Period)) 1343 throw new Error("Not the right type for Timing.repeat.bounds[x]: " + value.fhirType()); 1344 this.bounds = value; 1345 return this; 1346 } 1347 1348 /** 1349 * @return {@link #count} (A total count of the desired number of repetitions 1350 * across the duration of the entire timing specification. If countMax 1351 * is present, this element indicates the lower bound of the allowed 1352 * range of count values.). This is the underlying object with id, value 1353 * and extensions. The accessor "getCount" gives direct access to the 1354 * value 1355 */ 1356 public PositiveIntType getCountElement() { 1357 if (this.count == null) 1358 if (Configuration.errorOnAutoCreate()) 1359 throw new Error("Attempt to auto-create TimingRepeatComponent.count"); 1360 else if (Configuration.doAutoCreate()) 1361 this.count = new PositiveIntType(); // bb 1362 return this.count; 1363 } 1364 1365 public boolean hasCountElement() { 1366 return this.count != null && !this.count.isEmpty(); 1367 } 1368 1369 public boolean hasCount() { 1370 return this.count != null && !this.count.isEmpty(); 1371 } 1372 1373 /** 1374 * @param value {@link #count} (A total count of the desired number of 1375 * repetitions across the duration of the entire timing 1376 * specification. If countMax is present, this element indicates 1377 * the lower bound of the allowed range of count values.). This is 1378 * the underlying object with id, value and extensions. The 1379 * accessor "getCount" gives direct access to the value 1380 */ 1381 public TimingRepeatComponent setCountElement(PositiveIntType value) { 1382 this.count = value; 1383 return this; 1384 } 1385 1386 /** 1387 * @return A total count of the desired number of repetitions across the 1388 * duration of the entire timing specification. If countMax is present, 1389 * this element indicates the lower bound of the allowed range of count 1390 * values. 1391 */ 1392 public int getCount() { 1393 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 1394 } 1395 1396 /** 1397 * @param value A total count of the desired number of repetitions across the 1398 * duration of the entire timing specification. If countMax is 1399 * present, this element indicates the lower bound of the allowed 1400 * range of count values. 1401 */ 1402 public TimingRepeatComponent setCount(int value) { 1403 if (this.count == null) 1404 this.count = new PositiveIntType(); 1405 this.count.setValue(value); 1406 return this; 1407 } 1408 1409 /** 1410 * @return {@link #countMax} (If present, indicates that the count is a range - 1411 * so to perform the action between [count] and [countMax] times.). This 1412 * is the underlying object with id, value and extensions. The accessor 1413 * "getCountMax" gives direct access to the value 1414 */ 1415 public PositiveIntType getCountMaxElement() { 1416 if (this.countMax == null) 1417 if (Configuration.errorOnAutoCreate()) 1418 throw new Error("Attempt to auto-create TimingRepeatComponent.countMax"); 1419 else if (Configuration.doAutoCreate()) 1420 this.countMax = new PositiveIntType(); // bb 1421 return this.countMax; 1422 } 1423 1424 public boolean hasCountMaxElement() { 1425 return this.countMax != null && !this.countMax.isEmpty(); 1426 } 1427 1428 public boolean hasCountMax() { 1429 return this.countMax != null && !this.countMax.isEmpty(); 1430 } 1431 1432 /** 1433 * @param value {@link #countMax} (If present, indicates that the count is a 1434 * range - so to perform the action between [count] and [countMax] 1435 * times.). This is the underlying object with id, value and 1436 * extensions. The accessor "getCountMax" gives direct access to 1437 * the value 1438 */ 1439 public TimingRepeatComponent setCountMaxElement(PositiveIntType value) { 1440 this.countMax = value; 1441 return this; 1442 } 1443 1444 /** 1445 * @return If present, indicates that the count is a range - so to perform the 1446 * action between [count] and [countMax] times. 1447 */ 1448 public int getCountMax() { 1449 return this.countMax == null || this.countMax.isEmpty() ? 0 : this.countMax.getValue(); 1450 } 1451 1452 /** 1453 * @param value If present, indicates that the count is a range - so to perform 1454 * the action between [count] and [countMax] times. 1455 */ 1456 public TimingRepeatComponent setCountMax(int value) { 1457 if (this.countMax == null) 1458 this.countMax = new PositiveIntType(); 1459 this.countMax.setValue(value); 1460 return this; 1461 } 1462 1463 /** 1464 * @return {@link #duration} (How long this thing happens for when it happens. 1465 * If durationMax is present, this element indicates the lower bound of 1466 * the allowed range of the duration.). This is the underlying object 1467 * with id, value and extensions. The accessor "getDuration" gives 1468 * direct access to the value 1469 */ 1470 public DecimalType getDurationElement() { 1471 if (this.duration == null) 1472 if (Configuration.errorOnAutoCreate()) 1473 throw new Error("Attempt to auto-create TimingRepeatComponent.duration"); 1474 else if (Configuration.doAutoCreate()) 1475 this.duration = new DecimalType(); // bb 1476 return this.duration; 1477 } 1478 1479 public boolean hasDurationElement() { 1480 return this.duration != null && !this.duration.isEmpty(); 1481 } 1482 1483 public boolean hasDuration() { 1484 return this.duration != null && !this.duration.isEmpty(); 1485 } 1486 1487 /** 1488 * @param value {@link #duration} (How long this thing happens for when it 1489 * happens. If durationMax is present, this element indicates the 1490 * lower bound of the allowed range of the duration.). This is the 1491 * underlying object with id, value and extensions. The accessor 1492 * "getDuration" gives direct access to the value 1493 */ 1494 public TimingRepeatComponent setDurationElement(DecimalType value) { 1495 this.duration = value; 1496 return this; 1497 } 1498 1499 /** 1500 * @return How long this thing happens for when it happens. If durationMax is 1501 * present, this element indicates the lower bound of the allowed range 1502 * of the duration. 1503 */ 1504 public BigDecimal getDuration() { 1505 return this.duration == null ? null : this.duration.getValue(); 1506 } 1507 1508 /** 1509 * @param value How long this thing happens for when it happens. If durationMax 1510 * is present, this element indicates the lower bound of the 1511 * allowed range of the duration. 1512 */ 1513 public TimingRepeatComponent setDuration(BigDecimal value) { 1514 if (value == null) 1515 this.duration = null; 1516 else { 1517 if (this.duration == null) 1518 this.duration = new DecimalType(); 1519 this.duration.setValue(value); 1520 } 1521 return this; 1522 } 1523 1524 /** 1525 * @param value How long this thing happens for when it happens. If durationMax 1526 * is present, this element indicates the lower bound of the 1527 * allowed range of the duration. 1528 */ 1529 public TimingRepeatComponent setDuration(long value) { 1530 this.duration = new DecimalType(); 1531 this.duration.setValue(value); 1532 return this; 1533 } 1534 1535 /** 1536 * @param value How long this thing happens for when it happens. If durationMax 1537 * is present, this element indicates the lower bound of the 1538 * allowed range of the duration. 1539 */ 1540 public TimingRepeatComponent setDuration(double value) { 1541 this.duration = new DecimalType(); 1542 this.duration.setValue(value); 1543 return this; 1544 } 1545 1546 /** 1547 * @return {@link #durationMax} (If present, indicates that the duration is a 1548 * range - so to perform the action between [duration] and [durationMax] 1549 * time length.). This is the underlying object with id, value and 1550 * extensions. The accessor "getDurationMax" gives direct access to the 1551 * value 1552 */ 1553 public DecimalType getDurationMaxElement() { 1554 if (this.durationMax == null) 1555 if (Configuration.errorOnAutoCreate()) 1556 throw new Error("Attempt to auto-create TimingRepeatComponent.durationMax"); 1557 else if (Configuration.doAutoCreate()) 1558 this.durationMax = new DecimalType(); // bb 1559 return this.durationMax; 1560 } 1561 1562 public boolean hasDurationMaxElement() { 1563 return this.durationMax != null && !this.durationMax.isEmpty(); 1564 } 1565 1566 public boolean hasDurationMax() { 1567 return this.durationMax != null && !this.durationMax.isEmpty(); 1568 } 1569 1570 /** 1571 * @param value {@link #durationMax} (If present, indicates that the duration is 1572 * a range - so to perform the action between [duration] and 1573 * [durationMax] time length.). This is the underlying object with 1574 * id, value and extensions. The accessor "getDurationMax" gives 1575 * direct access to the value 1576 */ 1577 public TimingRepeatComponent setDurationMaxElement(DecimalType value) { 1578 this.durationMax = value; 1579 return this; 1580 } 1581 1582 /** 1583 * @return If present, indicates that the duration is a range - so to perform 1584 * the action between [duration] and [durationMax] time length. 1585 */ 1586 public BigDecimal getDurationMax() { 1587 return this.durationMax == null ? null : this.durationMax.getValue(); 1588 } 1589 1590 /** 1591 * @param value If present, indicates that the duration is a range - so to 1592 * perform the action between [duration] and [durationMax] time 1593 * length. 1594 */ 1595 public TimingRepeatComponent setDurationMax(BigDecimal value) { 1596 if (value == null) 1597 this.durationMax = null; 1598 else { 1599 if (this.durationMax == null) 1600 this.durationMax = new DecimalType(); 1601 this.durationMax.setValue(value); 1602 } 1603 return this; 1604 } 1605 1606 /** 1607 * @param value If present, indicates that the duration is a range - so to 1608 * perform the action between [duration] and [durationMax] time 1609 * length. 1610 */ 1611 public TimingRepeatComponent setDurationMax(long value) { 1612 this.durationMax = new DecimalType(); 1613 this.durationMax.setValue(value); 1614 return this; 1615 } 1616 1617 /** 1618 * @param value If present, indicates that the duration is a range - so to 1619 * perform the action between [duration] and [durationMax] time 1620 * length. 1621 */ 1622 public TimingRepeatComponent setDurationMax(double value) { 1623 this.durationMax = new DecimalType(); 1624 this.durationMax.setValue(value); 1625 return this; 1626 } 1627 1628 /** 1629 * @return {@link #durationUnit} (The units of time for the duration, in UCUM 1630 * units.). This is the underlying object with id, value and extensions. 1631 * The accessor "getDurationUnit" gives direct access to the value 1632 */ 1633 public Enumeration<UnitsOfTime> getDurationUnitElement() { 1634 if (this.durationUnit == null) 1635 if (Configuration.errorOnAutoCreate()) 1636 throw new Error("Attempt to auto-create TimingRepeatComponent.durationUnit"); 1637 else if (Configuration.doAutoCreate()) 1638 this.durationUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1639 return this.durationUnit; 1640 } 1641 1642 public boolean hasDurationUnitElement() { 1643 return this.durationUnit != null && !this.durationUnit.isEmpty(); 1644 } 1645 1646 public boolean hasDurationUnit() { 1647 return this.durationUnit != null && !this.durationUnit.isEmpty(); 1648 } 1649 1650 /** 1651 * @param value {@link #durationUnit} (The units of time for the duration, in 1652 * UCUM units.). This is the underlying object with id, value and 1653 * extensions. The accessor "getDurationUnit" gives direct access 1654 * to the value 1655 */ 1656 public TimingRepeatComponent setDurationUnitElement(Enumeration<UnitsOfTime> value) { 1657 this.durationUnit = value; 1658 return this; 1659 } 1660 1661 /** 1662 * @return The units of time for the duration, in UCUM units. 1663 */ 1664 public UnitsOfTime getDurationUnit() { 1665 return this.durationUnit == null ? null : this.durationUnit.getValue(); 1666 } 1667 1668 /** 1669 * @param value The units of time for the duration, in UCUM units. 1670 */ 1671 public TimingRepeatComponent setDurationUnit(UnitsOfTime value) { 1672 if (value == null) 1673 this.durationUnit = null; 1674 else { 1675 if (this.durationUnit == null) 1676 this.durationUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 1677 this.durationUnit.setValue(value); 1678 } 1679 return this; 1680 } 1681 1682 /** 1683 * @return {@link #frequency} (The number of times to repeat the action within 1684 * the specified period. If frequencyMax is present, this element 1685 * indicates the lower bound of the allowed range of the frequency.). 1686 * This is the underlying object with id, value and extensions. The 1687 * accessor "getFrequency" gives direct access to the value 1688 */ 1689 public PositiveIntType getFrequencyElement() { 1690 if (this.frequency == null) 1691 if (Configuration.errorOnAutoCreate()) 1692 throw new Error("Attempt to auto-create TimingRepeatComponent.frequency"); 1693 else if (Configuration.doAutoCreate()) 1694 this.frequency = new PositiveIntType(); // bb 1695 return this.frequency; 1696 } 1697 1698 public boolean hasFrequencyElement() { 1699 return this.frequency != null && !this.frequency.isEmpty(); 1700 } 1701 1702 public boolean hasFrequency() { 1703 return this.frequency != null && !this.frequency.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #frequency} (The number of times to repeat the action 1708 * within the specified period. If frequencyMax is present, this 1709 * element indicates the lower bound of the allowed range of the 1710 * frequency.). This is the underlying object with id, value and 1711 * extensions. The accessor "getFrequency" gives direct access to 1712 * the value 1713 */ 1714 public TimingRepeatComponent setFrequencyElement(PositiveIntType value) { 1715 this.frequency = value; 1716 return this; 1717 } 1718 1719 /** 1720 * @return The number of times to repeat the action within the specified period. 1721 * If frequencyMax is present, this element indicates the lower bound of 1722 * the allowed range of the frequency. 1723 */ 1724 public int getFrequency() { 1725 return this.frequency == null || this.frequency.isEmpty() ? 0 : this.frequency.getValue(); 1726 } 1727 1728 /** 1729 * @param value The number of times to repeat the action within the specified 1730 * period. If frequencyMax is present, this element indicates the 1731 * lower bound of the allowed range of the frequency. 1732 */ 1733 public TimingRepeatComponent setFrequency(int value) { 1734 if (this.frequency == null) 1735 this.frequency = new PositiveIntType(); 1736 this.frequency.setValue(value); 1737 return this; 1738 } 1739 1740 /** 1741 * @return {@link #frequencyMax} (If present, indicates that the frequency is a 1742 * range - so to repeat between [frequency] and [frequencyMax] times 1743 * within the period or period range.). This is the underlying object 1744 * with id, value and extensions. The accessor "getFrequencyMax" gives 1745 * direct access to the value 1746 */ 1747 public PositiveIntType getFrequencyMaxElement() { 1748 if (this.frequencyMax == null) 1749 if (Configuration.errorOnAutoCreate()) 1750 throw new Error("Attempt to auto-create TimingRepeatComponent.frequencyMax"); 1751 else if (Configuration.doAutoCreate()) 1752 this.frequencyMax = new PositiveIntType(); // bb 1753 return this.frequencyMax; 1754 } 1755 1756 public boolean hasFrequencyMaxElement() { 1757 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1758 } 1759 1760 public boolean hasFrequencyMax() { 1761 return this.frequencyMax != null && !this.frequencyMax.isEmpty(); 1762 } 1763 1764 /** 1765 * @param value {@link #frequencyMax} (If present, indicates that the frequency 1766 * is a range - so to repeat between [frequency] and [frequencyMax] 1767 * times within the period or period range.). This is the 1768 * underlying object with id, value and extensions. The accessor 1769 * "getFrequencyMax" gives direct access to the value 1770 */ 1771 public TimingRepeatComponent setFrequencyMaxElement(PositiveIntType value) { 1772 this.frequencyMax = value; 1773 return this; 1774 } 1775 1776 /** 1777 * @return If present, indicates that the frequency is a range - so to repeat 1778 * between [frequency] and [frequencyMax] times within the period or 1779 * period range. 1780 */ 1781 public int getFrequencyMax() { 1782 return this.frequencyMax == null || this.frequencyMax.isEmpty() ? 0 : this.frequencyMax.getValue(); 1783 } 1784 1785 /** 1786 * @param value If present, indicates that the frequency is a range - so to 1787 * repeat between [frequency] and [frequencyMax] times within the 1788 * period or period range. 1789 */ 1790 public TimingRepeatComponent setFrequencyMax(int value) { 1791 if (this.frequencyMax == null) 1792 this.frequencyMax = new PositiveIntType(); 1793 this.frequencyMax.setValue(value); 1794 return this; 1795 } 1796 1797 /** 1798 * @return {@link #period} (Indicates the duration of time over which 1799 * repetitions are to occur; e.g. to express "3 times per day", 3 would 1800 * be the frequency and "1 day" would be the period. If periodMax is 1801 * present, this element indicates the lower bound of the allowed range 1802 * of the period length.). This is the underlying object with id, value 1803 * and extensions. The accessor "getPeriod" gives direct access to the 1804 * value 1805 */ 1806 public DecimalType getPeriodElement() { 1807 if (this.period == null) 1808 if (Configuration.errorOnAutoCreate()) 1809 throw new Error("Attempt to auto-create TimingRepeatComponent.period"); 1810 else if (Configuration.doAutoCreate()) 1811 this.period = new DecimalType(); // bb 1812 return this.period; 1813 } 1814 1815 public boolean hasPeriodElement() { 1816 return this.period != null && !this.period.isEmpty(); 1817 } 1818 1819 public boolean hasPeriod() { 1820 return this.period != null && !this.period.isEmpty(); 1821 } 1822 1823 /** 1824 * @param value {@link #period} (Indicates the duration of time over which 1825 * repetitions are to occur; e.g. to express "3 times per day", 3 1826 * would be the frequency and "1 day" would be the period. If 1827 * periodMax is present, this element indicates the lower bound of 1828 * the allowed range of the period length.). This is the underlying 1829 * object with id, value and extensions. The accessor "getPeriod" 1830 * gives direct access to the value 1831 */ 1832 public TimingRepeatComponent setPeriodElement(DecimalType value) { 1833 this.period = value; 1834 return this; 1835 } 1836 1837 /** 1838 * @return Indicates the duration of time over which repetitions are to occur; 1839 * e.g. to express "3 times per day", 3 would be the frequency and "1 1840 * day" would be the period. If periodMax is present, this element 1841 * indicates the lower bound of the allowed range of the period length. 1842 */ 1843 public BigDecimal getPeriod() { 1844 return this.period == null ? null : this.period.getValue(); 1845 } 1846 1847 /** 1848 * @param value Indicates the duration of time over which repetitions are to 1849 * occur; e.g. to express "3 times per day", 3 would be the 1850 * frequency and "1 day" would be the period. If periodMax is 1851 * present, this element indicates the lower bound of the allowed 1852 * range of the period length. 1853 */ 1854 public TimingRepeatComponent setPeriod(BigDecimal value) { 1855 if (value == null) 1856 this.period = null; 1857 else { 1858 if (this.period == null) 1859 this.period = new DecimalType(); 1860 this.period.setValue(value); 1861 } 1862 return this; 1863 } 1864 1865 /** 1866 * @param value Indicates the duration of time over which repetitions are to 1867 * occur; e.g. to express "3 times per day", 3 would be the 1868 * frequency and "1 day" would be the period. If periodMax is 1869 * present, this element indicates the lower bound of the allowed 1870 * range of the period length. 1871 */ 1872 public TimingRepeatComponent setPeriod(long value) { 1873 this.period = new DecimalType(); 1874 this.period.setValue(value); 1875 return this; 1876 } 1877 1878 /** 1879 * @param value Indicates the duration of time over which repetitions are to 1880 * occur; e.g. to express "3 times per day", 3 would be the 1881 * frequency and "1 day" would be the period. If periodMax is 1882 * present, this element indicates the lower bound of the allowed 1883 * range of the period length. 1884 */ 1885 public TimingRepeatComponent setPeriod(double value) { 1886 this.period = new DecimalType(); 1887 this.period.setValue(value); 1888 return this; 1889 } 1890 1891 /** 1892 * @return {@link #periodMax} (If present, indicates that the period is a range 1893 * from [period] to [periodMax], allowing expressing concepts such as 1894 * "do this once every 3-5 days.). This is the underlying object with 1895 * id, value and extensions. The accessor "getPeriodMax" gives direct 1896 * access to the value 1897 */ 1898 public DecimalType getPeriodMaxElement() { 1899 if (this.periodMax == null) 1900 if (Configuration.errorOnAutoCreate()) 1901 throw new Error("Attempt to auto-create TimingRepeatComponent.periodMax"); 1902 else if (Configuration.doAutoCreate()) 1903 this.periodMax = new DecimalType(); // bb 1904 return this.periodMax; 1905 } 1906 1907 public boolean hasPeriodMaxElement() { 1908 return this.periodMax != null && !this.periodMax.isEmpty(); 1909 } 1910 1911 public boolean hasPeriodMax() { 1912 return this.periodMax != null && !this.periodMax.isEmpty(); 1913 } 1914 1915 /** 1916 * @param value {@link #periodMax} (If present, indicates that the period is a 1917 * range from [period] to [periodMax], allowing expressing concepts 1918 * such as "do this once every 3-5 days.). This is the underlying 1919 * object with id, value and extensions. The accessor 1920 * "getPeriodMax" gives direct access to the value 1921 */ 1922 public TimingRepeatComponent setPeriodMaxElement(DecimalType value) { 1923 this.periodMax = value; 1924 return this; 1925 } 1926 1927 /** 1928 * @return If present, indicates that the period is a range from [period] to 1929 * [periodMax], allowing expressing concepts such as "do this once every 1930 * 3-5 days. 1931 */ 1932 public BigDecimal getPeriodMax() { 1933 return this.periodMax == null ? null : this.periodMax.getValue(); 1934 } 1935 1936 /** 1937 * @param value If present, indicates that the period is a range from [period] 1938 * to [periodMax], allowing expressing concepts such as "do this 1939 * once every 3-5 days. 1940 */ 1941 public TimingRepeatComponent setPeriodMax(BigDecimal value) { 1942 if (value == null) 1943 this.periodMax = null; 1944 else { 1945 if (this.periodMax == null) 1946 this.periodMax = new DecimalType(); 1947 this.periodMax.setValue(value); 1948 } 1949 return this; 1950 } 1951 1952 /** 1953 * @param value If present, indicates that the period is a range from [period] 1954 * to [periodMax], allowing expressing concepts such as "do this 1955 * once every 3-5 days. 1956 */ 1957 public TimingRepeatComponent setPeriodMax(long value) { 1958 this.periodMax = new DecimalType(); 1959 this.periodMax.setValue(value); 1960 return this; 1961 } 1962 1963 /** 1964 * @param value If present, indicates that the period is a range from [period] 1965 * to [periodMax], allowing expressing concepts such as "do this 1966 * once every 3-5 days. 1967 */ 1968 public TimingRepeatComponent setPeriodMax(double value) { 1969 this.periodMax = new DecimalType(); 1970 this.periodMax.setValue(value); 1971 return this; 1972 } 1973 1974 /** 1975 * @return {@link #periodUnit} (The units of time for the period in UCUM 1976 * units.). This is the underlying object with id, value and extensions. 1977 * The accessor "getPeriodUnit" gives direct access to the value 1978 */ 1979 public Enumeration<UnitsOfTime> getPeriodUnitElement() { 1980 if (this.periodUnit == null) 1981 if (Configuration.errorOnAutoCreate()) 1982 throw new Error("Attempt to auto-create TimingRepeatComponent.periodUnit"); 1983 else if (Configuration.doAutoCreate()) 1984 this.periodUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); // bb 1985 return this.periodUnit; 1986 } 1987 1988 public boolean hasPeriodUnitElement() { 1989 return this.periodUnit != null && !this.periodUnit.isEmpty(); 1990 } 1991 1992 public boolean hasPeriodUnit() { 1993 return this.periodUnit != null && !this.periodUnit.isEmpty(); 1994 } 1995 1996 /** 1997 * @param value {@link #periodUnit} (The units of time for the period in UCUM 1998 * units.). This is the underlying object with id, value and 1999 * extensions. The accessor "getPeriodUnit" gives direct access to 2000 * the value 2001 */ 2002 public TimingRepeatComponent setPeriodUnitElement(Enumeration<UnitsOfTime> value) { 2003 this.periodUnit = value; 2004 return this; 2005 } 2006 2007 /** 2008 * @return The units of time for the period in UCUM units. 2009 */ 2010 public UnitsOfTime getPeriodUnit() { 2011 return this.periodUnit == null ? null : this.periodUnit.getValue(); 2012 } 2013 2014 /** 2015 * @param value The units of time for the period in UCUM units. 2016 */ 2017 public TimingRepeatComponent setPeriodUnit(UnitsOfTime value) { 2018 if (value == null) 2019 this.periodUnit = null; 2020 else { 2021 if (this.periodUnit == null) 2022 this.periodUnit = new Enumeration<UnitsOfTime>(new UnitsOfTimeEnumFactory()); 2023 this.periodUnit.setValue(value); 2024 } 2025 return this; 2026 } 2027 2028 /** 2029 * @return {@link #dayOfWeek} (If one or more days of week is provided, then the 2030 * action happens only on the specified day(s).) 2031 */ 2032 public List<Enumeration<DayOfWeek>> getDayOfWeek() { 2033 if (this.dayOfWeek == null) 2034 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2035 return this.dayOfWeek; 2036 } 2037 2038 /** 2039 * @return Returns a reference to <code>this</code> for easy method chaining 2040 */ 2041 public TimingRepeatComponent setDayOfWeek(List<Enumeration<DayOfWeek>> theDayOfWeek) { 2042 this.dayOfWeek = theDayOfWeek; 2043 return this; 2044 } 2045 2046 public boolean hasDayOfWeek() { 2047 if (this.dayOfWeek == null) 2048 return false; 2049 for (Enumeration<DayOfWeek> item : this.dayOfWeek) 2050 if (!item.isEmpty()) 2051 return true; 2052 return false; 2053 } 2054 2055 /** 2056 * @return {@link #dayOfWeek} (If one or more days of week is provided, then the 2057 * action happens only on the specified day(s).) 2058 */ 2059 public Enumeration<DayOfWeek> addDayOfWeekElement() {// 2 2060 Enumeration<DayOfWeek> t = new Enumeration<DayOfWeek>(new DayOfWeekEnumFactory()); 2061 if (this.dayOfWeek == null) 2062 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2063 this.dayOfWeek.add(t); 2064 return t; 2065 } 2066 2067 /** 2068 * @param value {@link #dayOfWeek} (If one or more days of week is provided, 2069 * then the action happens only on the specified day(s).) 2070 */ 2071 public TimingRepeatComponent addDayOfWeek(DayOfWeek value) { // 1 2072 Enumeration<DayOfWeek> t = new Enumeration<DayOfWeek>(new DayOfWeekEnumFactory()); 2073 t.setValue(value); 2074 if (this.dayOfWeek == null) 2075 this.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2076 this.dayOfWeek.add(t); 2077 return this; 2078 } 2079 2080 /** 2081 * @param value {@link #dayOfWeek} (If one or more days of week is provided, 2082 * then the action happens only on the specified day(s).) 2083 */ 2084 public boolean hasDayOfWeek(DayOfWeek value) { 2085 if (this.dayOfWeek == null) 2086 return false; 2087 for (Enumeration<DayOfWeek> v : this.dayOfWeek) 2088 if (v.getValue().equals(value)) // code 2089 return true; 2090 return false; 2091 } 2092 2093 /** 2094 * @return {@link #timeOfDay} (Specified time of day for action to take place.) 2095 */ 2096 public List<TimeType> getTimeOfDay() { 2097 if (this.timeOfDay == null) 2098 this.timeOfDay = new ArrayList<TimeType>(); 2099 return this.timeOfDay; 2100 } 2101 2102 /** 2103 * @return Returns a reference to <code>this</code> for easy method chaining 2104 */ 2105 public TimingRepeatComponent setTimeOfDay(List<TimeType> theTimeOfDay) { 2106 this.timeOfDay = theTimeOfDay; 2107 return this; 2108 } 2109 2110 public boolean hasTimeOfDay() { 2111 if (this.timeOfDay == null) 2112 return false; 2113 for (TimeType item : this.timeOfDay) 2114 if (!item.isEmpty()) 2115 return true; 2116 return false; 2117 } 2118 2119 /** 2120 * @return {@link #timeOfDay} (Specified time of day for action to take place.) 2121 */ 2122 public TimeType addTimeOfDayElement() {// 2 2123 TimeType t = new TimeType(); 2124 if (this.timeOfDay == null) 2125 this.timeOfDay = new ArrayList<TimeType>(); 2126 this.timeOfDay.add(t); 2127 return t; 2128 } 2129 2130 /** 2131 * @param value {@link #timeOfDay} (Specified time of day for action to take 2132 * place.) 2133 */ 2134 public TimingRepeatComponent addTimeOfDay(String value) { // 1 2135 TimeType t = new TimeType(); 2136 t.setValue(value); 2137 if (this.timeOfDay == null) 2138 this.timeOfDay = new ArrayList<TimeType>(); 2139 this.timeOfDay.add(t); 2140 return this; 2141 } 2142 2143 /** 2144 * @param value {@link #timeOfDay} (Specified time of day for action to take 2145 * place.) 2146 */ 2147 public boolean hasTimeOfDay(String value) { 2148 if (this.timeOfDay == null) 2149 return false; 2150 for (TimeType v : this.timeOfDay) 2151 if (v.getValue().equals(value)) // time 2152 return true; 2153 return false; 2154 } 2155 2156 /** 2157 * @return {@link #when} (An approximate time period during the day, potentially 2158 * linked to an event of daily living that indicates when the action 2159 * should occur.) 2160 */ 2161 public List<Enumeration<EventTiming>> getWhen() { 2162 if (this.when == null) 2163 this.when = new ArrayList<Enumeration<EventTiming>>(); 2164 return this.when; 2165 } 2166 2167 /** 2168 * @return Returns a reference to <code>this</code> for easy method chaining 2169 */ 2170 public TimingRepeatComponent setWhen(List<Enumeration<EventTiming>> theWhen) { 2171 this.when = theWhen; 2172 return this; 2173 } 2174 2175 public boolean hasWhen() { 2176 if (this.when == null) 2177 return false; 2178 for (Enumeration<EventTiming> item : this.when) 2179 if (!item.isEmpty()) 2180 return true; 2181 return false; 2182 } 2183 2184 /** 2185 * @return {@link #when} (An approximate time period during the day, potentially 2186 * linked to an event of daily living that indicates when the action 2187 * should occur.) 2188 */ 2189 public Enumeration<EventTiming> addWhenElement() {// 2 2190 Enumeration<EventTiming> t = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 2191 if (this.when == null) 2192 this.when = new ArrayList<Enumeration<EventTiming>>(); 2193 this.when.add(t); 2194 return t; 2195 } 2196 2197 /** 2198 * @param value {@link #when} (An approximate time period during the day, 2199 * potentially linked to an event of daily living that indicates 2200 * when the action should occur.) 2201 */ 2202 public TimingRepeatComponent addWhen(EventTiming value) { // 1 2203 Enumeration<EventTiming> t = new Enumeration<EventTiming>(new EventTimingEnumFactory()); 2204 t.setValue(value); 2205 if (this.when == null) 2206 this.when = new ArrayList<Enumeration<EventTiming>>(); 2207 this.when.add(t); 2208 return this; 2209 } 2210 2211 /** 2212 * @param value {@link #when} (An approximate time period during the day, 2213 * potentially linked to an event of daily living that indicates 2214 * when the action should occur.) 2215 */ 2216 public boolean hasWhen(EventTiming value) { 2217 if (this.when == null) 2218 return false; 2219 for (Enumeration<EventTiming> v : this.when) 2220 if (v.getValue().equals(value)) // code 2221 return true; 2222 return false; 2223 } 2224 2225 /** 2226 * @return {@link #offset} (The number of minutes from the event. If the event 2227 * code does not indicate whether the minutes is before or after the 2228 * event, then the offset is assumed to be after the event.). This is 2229 * the underlying object with id, value and extensions. The accessor 2230 * "getOffset" gives direct access to the value 2231 */ 2232 public UnsignedIntType getOffsetElement() { 2233 if (this.offset == null) 2234 if (Configuration.errorOnAutoCreate()) 2235 throw new Error("Attempt to auto-create TimingRepeatComponent.offset"); 2236 else if (Configuration.doAutoCreate()) 2237 this.offset = new UnsignedIntType(); // bb 2238 return this.offset; 2239 } 2240 2241 public boolean hasOffsetElement() { 2242 return this.offset != null && !this.offset.isEmpty(); 2243 } 2244 2245 public boolean hasOffset() { 2246 return this.offset != null && !this.offset.isEmpty(); 2247 } 2248 2249 /** 2250 * @param value {@link #offset} (The number of minutes from the event. If the 2251 * event code does not indicate whether the minutes is before or 2252 * after the event, then the offset is assumed to be after the 2253 * event.). This is the underlying object with id, value and 2254 * extensions. The accessor "getOffset" gives direct access to the 2255 * value 2256 */ 2257 public TimingRepeatComponent setOffsetElement(UnsignedIntType value) { 2258 this.offset = value; 2259 return this; 2260 } 2261 2262 /** 2263 * @return The number of minutes from the event. If the event code does not 2264 * indicate whether the minutes is before or after the event, then the 2265 * offset is assumed to be after the event. 2266 */ 2267 public int getOffset() { 2268 return this.offset == null || this.offset.isEmpty() ? 0 : this.offset.getValue(); 2269 } 2270 2271 /** 2272 * @param value The number of minutes from the event. If the event code does not 2273 * indicate whether the minutes is before or after the event, then 2274 * the offset is assumed to be after the event. 2275 */ 2276 public TimingRepeatComponent setOffset(int value) { 2277 if (this.offset == null) 2278 this.offset = new UnsignedIntType(); 2279 this.offset.setValue(value); 2280 return this; 2281 } 2282 2283 protected void listChildren(List<Property> children) { 2284 super.listChildren(children); 2285 children.add(new Property("bounds[x]", "Duration|Range|Period", 2286 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2287 0, 1, bounds)); 2288 children.add(new Property("count", "positiveInt", 2289 "A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.", 2290 0, 1, count)); 2291 children.add(new Property("countMax", "positiveInt", 2292 "If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.", 2293 0, 1, countMax)); 2294 children.add(new Property("duration", "decimal", 2295 "How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.", 2296 0, 1, duration)); 2297 children.add(new Property("durationMax", "decimal", 2298 "If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.", 2299 0, 1, durationMax)); 2300 children.add(new Property("durationUnit", "code", "The units of time for the duration, in UCUM units.", 0, 1, 2301 durationUnit)); 2302 children.add(new Property("frequency", "positiveInt", 2303 "The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.", 2304 0, 1, frequency)); 2305 children.add(new Property("frequencyMax", "positiveInt", 2306 "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.", 2307 0, 1, frequencyMax)); 2308 children.add(new Property("period", "decimal", 2309 "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.", 2310 0, 1, period)); 2311 children.add(new Property("periodMax", "decimal", 2312 "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 2313 0, 1, periodMax)); 2314 children 2315 .add(new Property("periodUnit", "code", "The units of time for the period in UCUM units.", 0, 1, periodUnit)); 2316 children.add(new Property("dayOfWeek", "code", 2317 "If one or more days of week is provided, then the action happens only on the specified day(s).", 0, 2318 java.lang.Integer.MAX_VALUE, dayOfWeek)); 2319 children.add(new Property("timeOfDay", "time", "Specified time of day for action to take place.", 0, 2320 java.lang.Integer.MAX_VALUE, timeOfDay)); 2321 children.add(new Property("when", "code", 2322 "An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.", 2323 0, java.lang.Integer.MAX_VALUE, when)); 2324 children.add(new Property("offset", "unsignedInt", 2325 "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.", 2326 0, 1, offset)); 2327 } 2328 2329 @Override 2330 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2331 switch (_hash) { 2332 case -1149635157: 2333 /* bounds[x] */ return new Property("bounds[x]", "Duration|Range|Period", 2334 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2335 0, 1, bounds); 2336 case -1383205195: 2337 /* bounds */ return new Property("bounds[x]", "Duration|Range|Period", 2338 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2339 0, 1, bounds); 2340 case -189193367: 2341 /* boundsDuration */ return new Property("bounds[x]", "Duration|Range|Period", 2342 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2343 0, 1, bounds); 2344 case -1001768056: 2345 /* boundsRange */ return new Property("bounds[x]", "Duration|Range|Period", 2346 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2347 0, 1, bounds); 2348 case -1043481386: 2349 /* boundsPeriod */ return new Property("bounds[x]", "Duration|Range|Period", 2350 "Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule.", 2351 0, 1, bounds); 2352 case 94851343: 2353 /* count */ return new Property("count", "positiveInt", 2354 "A total count of the desired number of repetitions across the duration of the entire timing specification. If countMax is present, this element indicates the lower bound of the allowed range of count values.", 2355 0, 1, count); 2356 case -372044331: 2357 /* countMax */ return new Property("countMax", "positiveInt", 2358 "If present, indicates that the count is a range - so to perform the action between [count] and [countMax] times.", 2359 0, 1, countMax); 2360 case -1992012396: 2361 /* duration */ return new Property("duration", "decimal", 2362 "How long this thing happens for when it happens. If durationMax is present, this element indicates the lower bound of the allowed range of the duration.", 2363 0, 1, duration); 2364 case -478083280: 2365 /* durationMax */ return new Property("durationMax", "decimal", 2366 "If present, indicates that the duration is a range - so to perform the action between [duration] and [durationMax] time length.", 2367 0, 1, durationMax); 2368 case -1935429320: 2369 /* durationUnit */ return new Property("durationUnit", "code", 2370 "The units of time for the duration, in UCUM units.", 0, 1, durationUnit); 2371 case -70023844: 2372 /* frequency */ return new Property("frequency", "positiveInt", 2373 "The number of times to repeat the action within the specified period. If frequencyMax is present, this element indicates the lower bound of the allowed range of the frequency.", 2374 0, 1, frequency); 2375 case 1273846376: 2376 /* frequencyMax */ return new Property("frequencyMax", "positiveInt", 2377 "If present, indicates that the frequency is a range - so to repeat between [frequency] and [frequencyMax] times within the period or period range.", 2378 0, 1, frequencyMax); 2379 case -991726143: 2380 /* period */ return new Property("period", "decimal", 2381 "Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period. If periodMax is present, this element indicates the lower bound of the allowed range of the period length.", 2382 0, 1, period); 2383 case 566580195: 2384 /* periodMax */ return new Property("periodMax", "decimal", 2385 "If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days.", 2386 0, 1, periodMax); 2387 case 384367333: 2388 /* periodUnit */ return new Property("periodUnit", "code", "The units of time for the period in UCUM units.", 0, 2389 1, periodUnit); 2390 case -730552025: 2391 /* dayOfWeek */ return new Property("dayOfWeek", "code", 2392 "If one or more days of week is provided, then the action happens only on the specified day(s).", 0, 2393 java.lang.Integer.MAX_VALUE, dayOfWeek); 2394 case 21434232: 2395 /* timeOfDay */ return new Property("timeOfDay", "time", "Specified time of day for action to take place.", 0, 2396 java.lang.Integer.MAX_VALUE, timeOfDay); 2397 case 3648314: 2398 /* when */ return new Property("when", "code", 2399 "An approximate time period during the day, potentially linked to an event of daily living that indicates when the action should occur.", 2400 0, java.lang.Integer.MAX_VALUE, when); 2401 case -1019779949: 2402 /* offset */ return new Property("offset", "unsignedInt", 2403 "The number of minutes from the event. If the event code does not indicate whether the minutes is before or after the event, then the offset is assumed to be after the event.", 2404 0, 1, offset); 2405 default: 2406 return super.getNamedProperty(_hash, _name, _checkValid); 2407 } 2408 2409 } 2410 2411 @Override 2412 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2413 switch (hash) { 2414 case -1383205195: 2415 /* bounds */ return this.bounds == null ? new Base[0] : new Base[] { this.bounds }; // Type 2416 case 94851343: 2417 /* count */ return this.count == null ? new Base[0] : new Base[] { this.count }; // PositiveIntType 2418 case -372044331: 2419 /* countMax */ return this.countMax == null ? new Base[0] : new Base[] { this.countMax }; // PositiveIntType 2420 case -1992012396: 2421 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // DecimalType 2422 case -478083280: 2423 /* durationMax */ return this.durationMax == null ? new Base[0] : new Base[] { this.durationMax }; // DecimalType 2424 case -1935429320: 2425 /* durationUnit */ return this.durationUnit == null ? new Base[0] : new Base[] { this.durationUnit }; // Enumeration<UnitsOfTime> 2426 case -70023844: 2427 /* frequency */ return this.frequency == null ? new Base[0] : new Base[] { this.frequency }; // PositiveIntType 2428 case 1273846376: 2429 /* frequencyMax */ return this.frequencyMax == null ? new Base[0] : new Base[] { this.frequencyMax }; // PositiveIntType 2430 case -991726143: 2431 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // DecimalType 2432 case 566580195: 2433 /* periodMax */ return this.periodMax == null ? new Base[0] : new Base[] { this.periodMax }; // DecimalType 2434 case 384367333: 2435 /* periodUnit */ return this.periodUnit == null ? new Base[0] : new Base[] { this.periodUnit }; // Enumeration<UnitsOfTime> 2436 case -730552025: 2437 /* dayOfWeek */ return this.dayOfWeek == null ? new Base[0] 2438 : this.dayOfWeek.toArray(new Base[this.dayOfWeek.size()]); // Enumeration<DayOfWeek> 2439 case 21434232: 2440 /* timeOfDay */ return this.timeOfDay == null ? new Base[0] 2441 : this.timeOfDay.toArray(new Base[this.timeOfDay.size()]); // TimeType 2442 case 3648314: 2443 /* when */ return this.when == null ? new Base[0] : this.when.toArray(new Base[this.when.size()]); // Enumeration<EventTiming> 2444 case -1019779949: 2445 /* offset */ return this.offset == null ? new Base[0] : new Base[] { this.offset }; // UnsignedIntType 2446 default: 2447 return super.getProperty(hash, name, checkValid); 2448 } 2449 2450 } 2451 2452 @Override 2453 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2454 switch (hash) { 2455 case -1383205195: // bounds 2456 this.bounds = castToType(value); // Type 2457 return value; 2458 case 94851343: // count 2459 this.count = castToPositiveInt(value); // PositiveIntType 2460 return value; 2461 case -372044331: // countMax 2462 this.countMax = castToPositiveInt(value); // PositiveIntType 2463 return value; 2464 case -1992012396: // duration 2465 this.duration = castToDecimal(value); // DecimalType 2466 return value; 2467 case -478083280: // durationMax 2468 this.durationMax = castToDecimal(value); // DecimalType 2469 return value; 2470 case -1935429320: // durationUnit 2471 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 2472 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 2473 return value; 2474 case -70023844: // frequency 2475 this.frequency = castToPositiveInt(value); // PositiveIntType 2476 return value; 2477 case 1273846376: // frequencyMax 2478 this.frequencyMax = castToPositiveInt(value); // PositiveIntType 2479 return value; 2480 case -991726143: // period 2481 this.period = castToDecimal(value); // DecimalType 2482 return value; 2483 case 566580195: // periodMax 2484 this.periodMax = castToDecimal(value); // DecimalType 2485 return value; 2486 case 384367333: // periodUnit 2487 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 2488 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 2489 return value; 2490 case -730552025: // dayOfWeek 2491 value = new DayOfWeekEnumFactory().fromType(castToCode(value)); 2492 this.getDayOfWeek().add((Enumeration) value); // Enumeration<DayOfWeek> 2493 return value; 2494 case 21434232: // timeOfDay 2495 this.getTimeOfDay().add(castToTime(value)); // TimeType 2496 return value; 2497 case 3648314: // when 2498 value = new EventTimingEnumFactory().fromType(castToCode(value)); 2499 this.getWhen().add((Enumeration) value); // Enumeration<EventTiming> 2500 return value; 2501 case -1019779949: // offset 2502 this.offset = castToUnsignedInt(value); // UnsignedIntType 2503 return value; 2504 default: 2505 return super.setProperty(hash, name, value); 2506 } 2507 2508 } 2509 2510 @Override 2511 public Base setProperty(String name, Base value) throws FHIRException { 2512 if (name.equals("bounds[x]")) { 2513 this.bounds = castToType(value); // Type 2514 } else if (name.equals("count")) { 2515 this.count = castToPositiveInt(value); // PositiveIntType 2516 } else if (name.equals("countMax")) { 2517 this.countMax = castToPositiveInt(value); // PositiveIntType 2518 } else if (name.equals("duration")) { 2519 this.duration = castToDecimal(value); // DecimalType 2520 } else if (name.equals("durationMax")) { 2521 this.durationMax = castToDecimal(value); // DecimalType 2522 } else if (name.equals("durationUnit")) { 2523 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 2524 this.durationUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 2525 } else if (name.equals("frequency")) { 2526 this.frequency = castToPositiveInt(value); // PositiveIntType 2527 } else if (name.equals("frequencyMax")) { 2528 this.frequencyMax = castToPositiveInt(value); // PositiveIntType 2529 } else if (name.equals("period")) { 2530 this.period = castToDecimal(value); // DecimalType 2531 } else if (name.equals("periodMax")) { 2532 this.periodMax = castToDecimal(value); // DecimalType 2533 } else if (name.equals("periodUnit")) { 2534 value = new UnitsOfTimeEnumFactory().fromType(castToCode(value)); 2535 this.periodUnit = (Enumeration) value; // Enumeration<UnitsOfTime> 2536 } else if (name.equals("dayOfWeek")) { 2537 value = new DayOfWeekEnumFactory().fromType(castToCode(value)); 2538 this.getDayOfWeek().add((Enumeration) value); 2539 } else if (name.equals("timeOfDay")) { 2540 this.getTimeOfDay().add(castToTime(value)); 2541 } else if (name.equals("when")) { 2542 value = new EventTimingEnumFactory().fromType(castToCode(value)); 2543 this.getWhen().add((Enumeration) value); 2544 } else if (name.equals("offset")) { 2545 this.offset = castToUnsignedInt(value); // UnsignedIntType 2546 } else 2547 return super.setProperty(name, value); 2548 return value; 2549 } 2550 2551 @Override 2552 public void removeChild(String name, Base value) throws FHIRException { 2553 if (name.equals("bounds[x]")) { 2554 this.bounds = null; 2555 } else if (name.equals("count")) { 2556 this.count = null; 2557 } else if (name.equals("countMax")) { 2558 this.countMax = null; 2559 } else if (name.equals("duration")) { 2560 this.duration = null; 2561 } else if (name.equals("durationMax")) { 2562 this.durationMax = null; 2563 } else if (name.equals("durationUnit")) { 2564 this.durationUnit = null; 2565 } else if (name.equals("frequency")) { 2566 this.frequency = null; 2567 } else if (name.equals("frequencyMax")) { 2568 this.frequencyMax = null; 2569 } else if (name.equals("period")) { 2570 this.period = null; 2571 } else if (name.equals("periodMax")) { 2572 this.periodMax = null; 2573 } else if (name.equals("periodUnit")) { 2574 this.periodUnit = null; 2575 } else if (name.equals("dayOfWeek")) { 2576 this.getDayOfWeek().remove((Enumeration) value); 2577 } else if (name.equals("timeOfDay")) { 2578 this.getTimeOfDay().remove(castToTime(value)); 2579 } else if (name.equals("when")) { 2580 value = null; 2581 this.getWhen().remove((Enumeration) value); 2582 } else if (name.equals("offset")) { 2583 this.offset = null; 2584 } else 2585 super.removeChild(name, value); 2586 2587 } 2588 2589 @Override 2590 public Base makeProperty(int hash, String name) throws FHIRException { 2591 switch (hash) { 2592 case -1149635157: 2593 return getBounds(); 2594 case -1383205195: 2595 return getBounds(); 2596 case 94851343: 2597 return getCountElement(); 2598 case -372044331: 2599 return getCountMaxElement(); 2600 case -1992012396: 2601 return getDurationElement(); 2602 case -478083280: 2603 return getDurationMaxElement(); 2604 case -1935429320: 2605 return getDurationUnitElement(); 2606 case -70023844: 2607 return getFrequencyElement(); 2608 case 1273846376: 2609 return getFrequencyMaxElement(); 2610 case -991726143: 2611 return getPeriodElement(); 2612 case 566580195: 2613 return getPeriodMaxElement(); 2614 case 384367333: 2615 return getPeriodUnitElement(); 2616 case -730552025: 2617 return addDayOfWeekElement(); 2618 case 21434232: 2619 return addTimeOfDayElement(); 2620 case 3648314: 2621 return addWhenElement(); 2622 case -1019779949: 2623 return getOffsetElement(); 2624 default: 2625 return super.makeProperty(hash, name); 2626 } 2627 2628 } 2629 2630 @Override 2631 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2632 switch (hash) { 2633 case -1383205195: 2634 /* bounds */ return new String[] { "Duration", "Range", "Period" }; 2635 case 94851343: 2636 /* count */ return new String[] { "positiveInt" }; 2637 case -372044331: 2638 /* countMax */ return new String[] { "positiveInt" }; 2639 case -1992012396: 2640 /* duration */ return new String[] { "decimal" }; 2641 case -478083280: 2642 /* durationMax */ return new String[] { "decimal" }; 2643 case -1935429320: 2644 /* durationUnit */ return new String[] { "code" }; 2645 case -70023844: 2646 /* frequency */ return new String[] { "positiveInt" }; 2647 case 1273846376: 2648 /* frequencyMax */ return new String[] { "positiveInt" }; 2649 case -991726143: 2650 /* period */ return new String[] { "decimal" }; 2651 case 566580195: 2652 /* periodMax */ return new String[] { "decimal" }; 2653 case 384367333: 2654 /* periodUnit */ return new String[] { "code" }; 2655 case -730552025: 2656 /* dayOfWeek */ return new String[] { "code" }; 2657 case 21434232: 2658 /* timeOfDay */ return new String[] { "time" }; 2659 case 3648314: 2660 /* when */ return new String[] { "code" }; 2661 case -1019779949: 2662 /* offset */ return new String[] { "unsignedInt" }; 2663 default: 2664 return super.getTypesForProperty(hash, name); 2665 } 2666 2667 } 2668 2669 @Override 2670 public Base addChild(String name) throws FHIRException { 2671 if (name.equals("boundsDuration")) { 2672 this.bounds = new Duration(); 2673 return this.bounds; 2674 } else if (name.equals("boundsRange")) { 2675 this.bounds = new Range(); 2676 return this.bounds; 2677 } else if (name.equals("boundsPeriod")) { 2678 this.bounds = new Period(); 2679 return this.bounds; 2680 } else if (name.equals("count")) { 2681 throw new FHIRException("Cannot call addChild on a singleton property Timing.count"); 2682 } else if (name.equals("countMax")) { 2683 throw new FHIRException("Cannot call addChild on a singleton property Timing.countMax"); 2684 } else if (name.equals("duration")) { 2685 throw new FHIRException("Cannot call addChild on a singleton property Timing.duration"); 2686 } else if (name.equals("durationMax")) { 2687 throw new FHIRException("Cannot call addChild on a singleton property Timing.durationMax"); 2688 } else if (name.equals("durationUnit")) { 2689 throw new FHIRException("Cannot call addChild on a singleton property Timing.durationUnit"); 2690 } else if (name.equals("frequency")) { 2691 throw new FHIRException("Cannot call addChild on a singleton property Timing.frequency"); 2692 } else if (name.equals("frequencyMax")) { 2693 throw new FHIRException("Cannot call addChild on a singleton property Timing.frequencyMax"); 2694 } else if (name.equals("period")) { 2695 throw new FHIRException("Cannot call addChild on a singleton property Timing.period"); 2696 } else if (name.equals("periodMax")) { 2697 throw new FHIRException("Cannot call addChild on a singleton property Timing.periodMax"); 2698 } else if (name.equals("periodUnit")) { 2699 throw new FHIRException("Cannot call addChild on a singleton property Timing.periodUnit"); 2700 } else if (name.equals("dayOfWeek")) { 2701 throw new FHIRException("Cannot call addChild on a singleton property Timing.dayOfWeek"); 2702 } else if (name.equals("timeOfDay")) { 2703 throw new FHIRException("Cannot call addChild on a singleton property Timing.timeOfDay"); 2704 } else if (name.equals("when")) { 2705 throw new FHIRException("Cannot call addChild on a singleton property Timing.when"); 2706 } else if (name.equals("offset")) { 2707 throw new FHIRException("Cannot call addChild on a singleton property Timing.offset"); 2708 } else 2709 return super.addChild(name); 2710 } 2711 2712 public TimingRepeatComponent copy() { 2713 TimingRepeatComponent dst = new TimingRepeatComponent(); 2714 copyValues(dst); 2715 return dst; 2716 } 2717 2718 public void copyValues(TimingRepeatComponent dst) { 2719 super.copyValues(dst); 2720 dst.bounds = bounds == null ? null : bounds.copy(); 2721 dst.count = count == null ? null : count.copy(); 2722 dst.countMax = countMax == null ? null : countMax.copy(); 2723 dst.duration = duration == null ? null : duration.copy(); 2724 dst.durationMax = durationMax == null ? null : durationMax.copy(); 2725 dst.durationUnit = durationUnit == null ? null : durationUnit.copy(); 2726 dst.frequency = frequency == null ? null : frequency.copy(); 2727 dst.frequencyMax = frequencyMax == null ? null : frequencyMax.copy(); 2728 dst.period = period == null ? null : period.copy(); 2729 dst.periodMax = periodMax == null ? null : periodMax.copy(); 2730 dst.periodUnit = periodUnit == null ? null : periodUnit.copy(); 2731 if (dayOfWeek != null) { 2732 dst.dayOfWeek = new ArrayList<Enumeration<DayOfWeek>>(); 2733 for (Enumeration<DayOfWeek> i : dayOfWeek) 2734 dst.dayOfWeek.add(i.copy()); 2735 } 2736 ; 2737 if (timeOfDay != null) { 2738 dst.timeOfDay = new ArrayList<TimeType>(); 2739 for (TimeType i : timeOfDay) 2740 dst.timeOfDay.add(i.copy()); 2741 } 2742 ; 2743 if (when != null) { 2744 dst.when = new ArrayList<Enumeration<EventTiming>>(); 2745 for (Enumeration<EventTiming> i : when) 2746 dst.when.add(i.copy()); 2747 } 2748 ; 2749 dst.offset = offset == null ? null : offset.copy(); 2750 } 2751 2752 @Override 2753 public boolean equalsDeep(Base other_) { 2754 if (!super.equalsDeep(other_)) 2755 return false; 2756 if (!(other_ instanceof TimingRepeatComponent)) 2757 return false; 2758 TimingRepeatComponent o = (TimingRepeatComponent) other_; 2759 return compareDeep(bounds, o.bounds, true) && compareDeep(count, o.count, true) 2760 && compareDeep(countMax, o.countMax, true) && compareDeep(duration, o.duration, true) 2761 && compareDeep(durationMax, o.durationMax, true) && compareDeep(durationUnit, o.durationUnit, true) 2762 && compareDeep(frequency, o.frequency, true) && compareDeep(frequencyMax, o.frequencyMax, true) 2763 && compareDeep(period, o.period, true) && compareDeep(periodMax, o.periodMax, true) 2764 && compareDeep(periodUnit, o.periodUnit, true) && compareDeep(dayOfWeek, o.dayOfWeek, true) 2765 && compareDeep(timeOfDay, o.timeOfDay, true) && compareDeep(when, o.when, true) 2766 && compareDeep(offset, o.offset, true); 2767 } 2768 2769 @Override 2770 public boolean equalsShallow(Base other_) { 2771 if (!super.equalsShallow(other_)) 2772 return false; 2773 if (!(other_ instanceof TimingRepeatComponent)) 2774 return false; 2775 TimingRepeatComponent o = (TimingRepeatComponent) other_; 2776 return compareValues(count, o.count, true) && compareValues(countMax, o.countMax, true) 2777 && compareValues(duration, o.duration, true) && compareValues(durationMax, o.durationMax, true) 2778 && compareValues(durationUnit, o.durationUnit, true) && compareValues(frequency, o.frequency, true) 2779 && compareValues(frequencyMax, o.frequencyMax, true) && compareValues(period, o.period, true) 2780 && compareValues(periodMax, o.periodMax, true) && compareValues(periodUnit, o.periodUnit, true) 2781 && compareValues(dayOfWeek, o.dayOfWeek, true) && compareValues(timeOfDay, o.timeOfDay, true) 2782 && compareValues(when, o.when, true) && compareValues(offset, o.offset, true); 2783 } 2784 2785 public boolean isEmpty() { 2786 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(bounds, count, countMax, duration, durationMax, 2787 durationUnit, frequency, frequencyMax, period, periodMax, periodUnit, dayOfWeek, timeOfDay, when, offset); 2788 } 2789 2790 public String fhirType() { 2791 return "Timing.repeat"; 2792 2793 } 2794 2795 } 2796 2797 /** 2798 * Identifies specific times when the event occurs. 2799 */ 2800 @Child(name = "event", type = { 2801 DateTimeType.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2802 @Description(shortDefinition = "When the event occurs", formalDefinition = "Identifies specific times when the event occurs.") 2803 protected List<DateTimeType> event; 2804 2805 /** 2806 * A set of rules that describe when the event is scheduled. 2807 */ 2808 @Child(name = "repeat", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = true) 2809 @Description(shortDefinition = "When the event is to occur", formalDefinition = "A set of rules that describe when the event is scheduled.") 2810 protected TimingRepeatComponent repeat; 2811 2812 /** 2813 * A code for the timing schedule (or just text in code.text). Some codes such 2814 * as BID are ubiquitous, but many institutions define their own additional 2815 * codes. If a code is provided, the code is understood to be a complete 2816 * statement of whatever is specified in the structured timing data, and either 2817 * the code or the data may be used to interpret the Timing, with the exception 2818 * that .repeat.bounds still applies over the code (and is not contained in the 2819 * code). 2820 */ 2821 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2822 @Description(shortDefinition = "BID | TID | QID | AM | PM | QD | QOD | +", formalDefinition = "A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).") 2823 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/timing-abbreviation") 2824 protected CodeableConcept code; 2825 2826 private static final long serialVersionUID = 791565112L; 2827 2828 /** 2829 * Constructor 2830 */ 2831 public Timing() { 2832 super(); 2833 } 2834 2835 /** 2836 * @return {@link #event} (Identifies specific times when the event occurs.) 2837 */ 2838 public List<DateTimeType> getEvent() { 2839 if (this.event == null) 2840 this.event = new ArrayList<DateTimeType>(); 2841 return this.event; 2842 } 2843 2844 /** 2845 * @return Returns a reference to <code>this</code> for easy method chaining 2846 */ 2847 public Timing setEvent(List<DateTimeType> theEvent) { 2848 this.event = theEvent; 2849 return this; 2850 } 2851 2852 public boolean hasEvent() { 2853 if (this.event == null) 2854 return false; 2855 for (DateTimeType item : this.event) 2856 if (!item.isEmpty()) 2857 return true; 2858 return false; 2859 } 2860 2861 /** 2862 * @return {@link #event} (Identifies specific times when the event occurs.) 2863 */ 2864 public DateTimeType addEventElement() {// 2 2865 DateTimeType t = new DateTimeType(); 2866 if (this.event == null) 2867 this.event = new ArrayList<DateTimeType>(); 2868 this.event.add(t); 2869 return t; 2870 } 2871 2872 /** 2873 * @param value {@link #event} (Identifies specific times when the event 2874 * occurs.) 2875 */ 2876 public Timing addEvent(Date value) { // 1 2877 DateTimeType t = new DateTimeType(); 2878 t.setValue(value); 2879 if (this.event == null) 2880 this.event = new ArrayList<DateTimeType>(); 2881 this.event.add(t); 2882 return this; 2883 } 2884 2885 /** 2886 * @param value {@link #event} (Identifies specific times when the event 2887 * occurs.) 2888 */ 2889 public boolean hasEvent(Date value) { 2890 if (this.event == null) 2891 return false; 2892 for (DateTimeType v : this.event) 2893 if (v.getValue().equals(value)) // dateTime 2894 return true; 2895 return false; 2896 } 2897 2898 /** 2899 * @return {@link #repeat} (A set of rules that describe when the event is 2900 * scheduled.) 2901 */ 2902 public TimingRepeatComponent getRepeat() { 2903 if (this.repeat == null) 2904 if (Configuration.errorOnAutoCreate()) 2905 throw new Error("Attempt to auto-create Timing.repeat"); 2906 else if (Configuration.doAutoCreate()) 2907 this.repeat = new TimingRepeatComponent(); // cc 2908 return this.repeat; 2909 } 2910 2911 public boolean hasRepeat() { 2912 return this.repeat != null && !this.repeat.isEmpty(); 2913 } 2914 2915 /** 2916 * @param value {@link #repeat} (A set of rules that describe when the event is 2917 * scheduled.) 2918 */ 2919 public Timing setRepeat(TimingRepeatComponent value) { 2920 this.repeat = value; 2921 return this; 2922 } 2923 2924 /** 2925 * @return {@link #code} (A code for the timing schedule (or just text in 2926 * code.text). Some codes such as BID are ubiquitous, but many 2927 * institutions define their own additional codes. If a code is 2928 * provided, the code is understood to be a complete statement of 2929 * whatever is specified in the structured timing data, and either the 2930 * code or the data may be used to interpret the Timing, with the 2931 * exception that .repeat.bounds still applies over the code (and is not 2932 * contained in the code).) 2933 */ 2934 public CodeableConcept getCode() { 2935 if (this.code == null) 2936 if (Configuration.errorOnAutoCreate()) 2937 throw new Error("Attempt to auto-create Timing.code"); 2938 else if (Configuration.doAutoCreate()) 2939 this.code = new CodeableConcept(); // cc 2940 return this.code; 2941 } 2942 2943 public boolean hasCode() { 2944 return this.code != null && !this.code.isEmpty(); 2945 } 2946 2947 /** 2948 * @param value {@link #code} (A code for the timing schedule (or just text in 2949 * code.text). Some codes such as BID are ubiquitous, but many 2950 * institutions define their own additional codes. If a code is 2951 * provided, the code is understood to be a complete statement of 2952 * whatever is specified in the structured timing data, and either 2953 * the code or the data may be used to interpret the Timing, with 2954 * the exception that .repeat.bounds still applies over the code 2955 * (and is not contained in the code).) 2956 */ 2957 public Timing setCode(CodeableConcept value) { 2958 this.code = value; 2959 return this; 2960 } 2961 2962 protected void listChildren(List<Property> children) { 2963 super.listChildren(children); 2964 children.add(new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, 2965 java.lang.Integer.MAX_VALUE, event)); 2966 children.add(new Property("repeat", "", "A set of rules that describe when the event is scheduled.", 0, 1, repeat)); 2967 children.add(new Property("code", "CodeableConcept", 2968 "A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).", 2969 0, 1, code)); 2970 } 2971 2972 @Override 2973 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2974 switch (_hash) { 2975 case 96891546: 2976 /* event */ return new Property("event", "dateTime", "Identifies specific times when the event occurs.", 0, 2977 java.lang.Integer.MAX_VALUE, event); 2978 case -934531685: 2979 /* repeat */ return new Property("repeat", "", "A set of rules that describe when the event is scheduled.", 0, 1, 2980 repeat); 2981 case 3059181: 2982 /* code */ return new Property("code", "CodeableConcept", 2983 "A code for the timing schedule (or just text in code.text). Some codes such as BID are ubiquitous, but many institutions define their own additional codes. If a code is provided, the code is understood to be a complete statement of whatever is specified in the structured timing data, and either the code or the data may be used to interpret the Timing, with the exception that .repeat.bounds still applies over the code (and is not contained in the code).", 2984 0, 1, code); 2985 default: 2986 return super.getNamedProperty(_hash, _name, _checkValid); 2987 } 2988 2989 } 2990 2991 @Override 2992 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2993 switch (hash) { 2994 case 96891546: 2995 /* event */ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // DateTimeType 2996 case -934531685: 2997 /* repeat */ return this.repeat == null ? new Base[0] : new Base[] { this.repeat }; // TimingRepeatComponent 2998 case 3059181: 2999 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 3000 default: 3001 return super.getProperty(hash, name, checkValid); 3002 } 3003 3004 } 3005 3006 @Override 3007 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3008 switch (hash) { 3009 case 96891546: // event 3010 this.getEvent().add(castToDateTime(value)); // DateTimeType 3011 return value; 3012 case -934531685: // repeat 3013 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 3014 return value; 3015 case 3059181: // code 3016 this.code = castToCodeableConcept(value); // CodeableConcept 3017 return value; 3018 default: 3019 return super.setProperty(hash, name, value); 3020 } 3021 3022 } 3023 3024 @Override 3025 public Base setProperty(String name, Base value) throws FHIRException { 3026 if (name.equals("event")) { 3027 this.getEvent().add(castToDateTime(value)); 3028 } else if (name.equals("repeat")) { 3029 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 3030 } else if (name.equals("code")) { 3031 this.code = castToCodeableConcept(value); // CodeableConcept 3032 } else 3033 return super.setProperty(name, value); 3034 return value; 3035 } 3036 3037 @Override 3038 public void removeChild(String name, Base value) throws FHIRException { 3039 if (name.equals("event")) { 3040 this.getEvent().remove(castToDateTime(value)); 3041 } else if (name.equals("repeat")) { 3042 this.repeat = (TimingRepeatComponent) value; // TimingRepeatComponent 3043 } else if (name.equals("code")) { 3044 this.code = null; 3045 } else 3046 super.removeChild(name, value); 3047 3048 } 3049 3050 @Override 3051 public Base makeProperty(int hash, String name) throws FHIRException { 3052 switch (hash) { 3053 case 96891546: 3054 return addEventElement(); 3055 case -934531685: 3056 return getRepeat(); 3057 case 3059181: 3058 return getCode(); 3059 default: 3060 return super.makeProperty(hash, name); 3061 } 3062 3063 } 3064 3065 @Override 3066 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3067 switch (hash) { 3068 case 96891546: 3069 /* event */ return new String[] { "dateTime" }; 3070 case -934531685: 3071 /* repeat */ return new String[] {}; 3072 case 3059181: 3073 /* code */ return new String[] { "CodeableConcept" }; 3074 default: 3075 return super.getTypesForProperty(hash, name); 3076 } 3077 3078 } 3079 3080 @Override 3081 public Base addChild(String name) throws FHIRException { 3082 if (name.equals("event")) { 3083 throw new FHIRException("Cannot call addChild on a singleton property Timing.event"); 3084 } else if (name.equals("repeat")) { 3085 this.repeat = new TimingRepeatComponent(); 3086 return this.repeat; 3087 } else if (name.equals("code")) { 3088 this.code = new CodeableConcept(); 3089 return this.code; 3090 } else 3091 return super.addChild(name); 3092 } 3093 3094 public String fhirType() { 3095 return "Timing"; 3096 3097 } 3098 3099 public Timing copy() { 3100 Timing dst = new Timing(); 3101 copyValues(dst); 3102 return dst; 3103 } 3104 3105 public void copyValues(Timing dst) { 3106 super.copyValues(dst); 3107 if (event != null) { 3108 dst.event = new ArrayList<DateTimeType>(); 3109 for (DateTimeType i : event) 3110 dst.event.add(i.copy()); 3111 } 3112 ; 3113 dst.repeat = repeat == null ? null : repeat.copy(); 3114 dst.code = code == null ? null : code.copy(); 3115 } 3116 3117 protected Timing typedCopy() { 3118 return copy(); 3119 } 3120 3121 @Override 3122 public boolean equalsDeep(Base other_) { 3123 if (!super.equalsDeep(other_)) 3124 return false; 3125 if (!(other_ instanceof Timing)) 3126 return false; 3127 Timing o = (Timing) other_; 3128 return compareDeep(event, o.event, true) && compareDeep(repeat, o.repeat, true) && compareDeep(code, o.code, true); 3129 } 3130 3131 @Override 3132 public boolean equalsShallow(Base other_) { 3133 if (!super.equalsShallow(other_)) 3134 return false; 3135 if (!(other_ instanceof Timing)) 3136 return false; 3137 Timing o = (Timing) other_; 3138 return compareValues(event, o.event, true); 3139 } 3140 3141 public boolean isEmpty() { 3142 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(event, repeat, code); 3143 } 3144 3145}