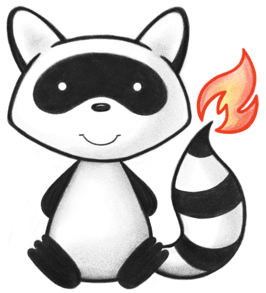
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * A description of a triggering event. Triggering events can be named events, 046 * data events, or periodic, as determined by the type element. 047 */ 048@DatatypeDef(name = "TriggerDefinition") 049public class TriggerDefinition extends Type implements ICompositeType { 050 051 public enum TriggerType { 052 /** 053 * The trigger occurs in response to a specific named event, and no other 054 * information about the trigger is specified. Named events are completely 055 * pre-coordinated, and the formal semantics of the trigger are not provided. 056 */ 057 NAMEDEVENT, 058 /** 059 * The trigger occurs at a specific time or periodically as described by a 060 * timing or schedule. A periodic event cannot have any data elements, but may 061 * have a name assigned as a shorthand for the event. 062 */ 063 PERIODIC, 064 /** 065 * The trigger occurs whenever data of a particular type is changed in any way, 066 * either added, modified, or removed. 067 */ 068 DATACHANGED, 069 /** 070 * The trigger occurs whenever data of a particular type is added. 071 */ 072 DATAADDED, 073 /** 074 * The trigger occurs whenever data of a particular type is modified. 075 */ 076 DATAMODIFIED, 077 /** 078 * The trigger occurs whenever data of a particular type is removed. 079 */ 080 DATAREMOVED, 081 /** 082 * The trigger occurs whenever data of a particular type is accessed. 083 */ 084 DATAACCESSED, 085 /** 086 * The trigger occurs whenever access to data of a particular type is completed. 087 */ 088 DATAACCESSENDED, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 094 public static TriggerType fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("named-event".equals(codeString)) 098 return NAMEDEVENT; 099 if ("periodic".equals(codeString)) 100 return PERIODIC; 101 if ("data-changed".equals(codeString)) 102 return DATACHANGED; 103 if ("data-added".equals(codeString)) 104 return DATAADDED; 105 if ("data-modified".equals(codeString)) 106 return DATAMODIFIED; 107 if ("data-removed".equals(codeString)) 108 return DATAREMOVED; 109 if ("data-accessed".equals(codeString)) 110 return DATAACCESSED; 111 if ("data-access-ended".equals(codeString)) 112 return DATAACCESSENDED; 113 if (Configuration.isAcceptInvalidEnums()) 114 return null; 115 else 116 throw new FHIRException("Unknown TriggerType code '" + codeString + "'"); 117 } 118 119 public String toCode() { 120 switch (this) { 121 case NAMEDEVENT: 122 return "named-event"; 123 case PERIODIC: 124 return "periodic"; 125 case DATACHANGED: 126 return "data-changed"; 127 case DATAADDED: 128 return "data-added"; 129 case DATAMODIFIED: 130 return "data-modified"; 131 case DATAREMOVED: 132 return "data-removed"; 133 case DATAACCESSED: 134 return "data-accessed"; 135 case DATAACCESSENDED: 136 return "data-access-ended"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case NAMEDEVENT: 147 return "http://hl7.org/fhir/trigger-type"; 148 case PERIODIC: 149 return "http://hl7.org/fhir/trigger-type"; 150 case DATACHANGED: 151 return "http://hl7.org/fhir/trigger-type"; 152 case DATAADDED: 153 return "http://hl7.org/fhir/trigger-type"; 154 case DATAMODIFIED: 155 return "http://hl7.org/fhir/trigger-type"; 156 case DATAREMOVED: 157 return "http://hl7.org/fhir/trigger-type"; 158 case DATAACCESSED: 159 return "http://hl7.org/fhir/trigger-type"; 160 case DATAACCESSENDED: 161 return "http://hl7.org/fhir/trigger-type"; 162 case NULL: 163 return null; 164 default: 165 return "?"; 166 } 167 } 168 169 public String getDefinition() { 170 switch (this) { 171 case NAMEDEVENT: 172 return "The trigger occurs in response to a specific named event, and no other information about the trigger is specified. Named events are completely pre-coordinated, and the formal semantics of the trigger are not provided."; 173 case PERIODIC: 174 return "The trigger occurs at a specific time or periodically as described by a timing or schedule. A periodic event cannot have any data elements, but may have a name assigned as a shorthand for the event."; 175 case DATACHANGED: 176 return "The trigger occurs whenever data of a particular type is changed in any way, either added, modified, or removed."; 177 case DATAADDED: 178 return "The trigger occurs whenever data of a particular type is added."; 179 case DATAMODIFIED: 180 return "The trigger occurs whenever data of a particular type is modified."; 181 case DATAREMOVED: 182 return "The trigger occurs whenever data of a particular type is removed."; 183 case DATAACCESSED: 184 return "The trigger occurs whenever data of a particular type is accessed."; 185 case DATAACCESSENDED: 186 return "The trigger occurs whenever access to data of a particular type is completed."; 187 case NULL: 188 return null; 189 default: 190 return "?"; 191 } 192 } 193 194 public String getDisplay() { 195 switch (this) { 196 case NAMEDEVENT: 197 return "Named Event"; 198 case PERIODIC: 199 return "Periodic"; 200 case DATACHANGED: 201 return "Data Changed"; 202 case DATAADDED: 203 return "Data Added"; 204 case DATAMODIFIED: 205 return "Data Updated"; 206 case DATAREMOVED: 207 return "Data Removed"; 208 case DATAACCESSED: 209 return "Data Accessed"; 210 case DATAACCESSENDED: 211 return "Data Access Ended"; 212 case NULL: 213 return null; 214 default: 215 return "?"; 216 } 217 } 218 } 219 220 public static class TriggerTypeEnumFactory implements EnumFactory<TriggerType> { 221 public TriggerType fromCode(String codeString) throws IllegalArgumentException { 222 if (codeString == null || "".equals(codeString)) 223 if (codeString == null || "".equals(codeString)) 224 return null; 225 if ("named-event".equals(codeString)) 226 return TriggerType.NAMEDEVENT; 227 if ("periodic".equals(codeString)) 228 return TriggerType.PERIODIC; 229 if ("data-changed".equals(codeString)) 230 return TriggerType.DATACHANGED; 231 if ("data-added".equals(codeString)) 232 return TriggerType.DATAADDED; 233 if ("data-modified".equals(codeString)) 234 return TriggerType.DATAMODIFIED; 235 if ("data-removed".equals(codeString)) 236 return TriggerType.DATAREMOVED; 237 if ("data-accessed".equals(codeString)) 238 return TriggerType.DATAACCESSED; 239 if ("data-access-ended".equals(codeString)) 240 return TriggerType.DATAACCESSENDED; 241 throw new IllegalArgumentException("Unknown TriggerType code '" + codeString + "'"); 242 } 243 244 public Enumeration<TriggerType> fromType(PrimitiveType<?> code) throws FHIRException { 245 if (code == null) 246 return null; 247 if (code.isEmpty()) 248 return new Enumeration<TriggerType>(this, TriggerType.NULL, code); 249 String codeString = code.asStringValue(); 250 if (codeString == null || "".equals(codeString)) 251 return new Enumeration<TriggerType>(this, TriggerType.NULL, code); 252 if ("named-event".equals(codeString)) 253 return new Enumeration<TriggerType>(this, TriggerType.NAMEDEVENT, code); 254 if ("periodic".equals(codeString)) 255 return new Enumeration<TriggerType>(this, TriggerType.PERIODIC, code); 256 if ("data-changed".equals(codeString)) 257 return new Enumeration<TriggerType>(this, TriggerType.DATACHANGED, code); 258 if ("data-added".equals(codeString)) 259 return new Enumeration<TriggerType>(this, TriggerType.DATAADDED, code); 260 if ("data-modified".equals(codeString)) 261 return new Enumeration<TriggerType>(this, TriggerType.DATAMODIFIED, code); 262 if ("data-removed".equals(codeString)) 263 return new Enumeration<TriggerType>(this, TriggerType.DATAREMOVED, code); 264 if ("data-accessed".equals(codeString)) 265 return new Enumeration<TriggerType>(this, TriggerType.DATAACCESSED, code); 266 if ("data-access-ended".equals(codeString)) 267 return new Enumeration<TriggerType>(this, TriggerType.DATAACCESSENDED, code); 268 throw new FHIRException("Unknown TriggerType code '" + codeString + "'"); 269 } 270 271 public String toCode(TriggerType code) { 272 if (code == TriggerType.NULL) 273 return null; 274 if (code == TriggerType.NAMEDEVENT) 275 return "named-event"; 276 if (code == TriggerType.PERIODIC) 277 return "periodic"; 278 if (code == TriggerType.DATACHANGED) 279 return "data-changed"; 280 if (code == TriggerType.DATAADDED) 281 return "data-added"; 282 if (code == TriggerType.DATAMODIFIED) 283 return "data-modified"; 284 if (code == TriggerType.DATAREMOVED) 285 return "data-removed"; 286 if (code == TriggerType.DATAACCESSED) 287 return "data-accessed"; 288 if (code == TriggerType.DATAACCESSENDED) 289 return "data-access-ended"; 290 return "?"; 291 } 292 293 public String toSystem(TriggerType code) { 294 return code.getSystem(); 295 } 296 } 297 298 /** 299 * The type of triggering event. 300 */ 301 @Child(name = "type", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 302 @Description(shortDefinition = "named-event | periodic | data-changed | data-added | data-modified | data-removed | data-accessed | data-access-ended", formalDefinition = "The type of triggering event.") 303 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/trigger-type") 304 protected Enumeration<TriggerType> type; 305 306 /** 307 * A formal name for the event. This may be an absolute URI that identifies the 308 * event formally (e.g. from a trigger registry), or a simple relative URI that 309 * identifies the event in a local context. 310 */ 311 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 312 @Description(shortDefinition = "Name or URI that identifies the event", formalDefinition = "A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.") 313 protected StringType name; 314 315 /** 316 * The timing of the event (if this is a periodic trigger). 317 */ 318 @Child(name = "timing", type = { Timing.class, Schedule.class, DateType.class, 319 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 320 @Description(shortDefinition = "Timing of the event", formalDefinition = "The timing of the event (if this is a periodic trigger).") 321 protected Type timing; 322 323 /** 324 * The triggering data of the event (if this is a data trigger). If more than 325 * one data is requirement is specified, then all the data requirements must be 326 * true. 327 */ 328 @Child(name = "data", type = { 329 DataRequirement.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 330 @Description(shortDefinition = "Triggering data of the event (multiple = 'and')", formalDefinition = "The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.") 331 protected List<DataRequirement> data; 332 333 /** 334 * A boolean-valued expression that is evaluated in the context of the container 335 * of the trigger definition and returns whether or not the trigger fires. 336 */ 337 @Child(name = "condition", type = { Expression.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 338 @Description(shortDefinition = "Whether the event triggers (boolean expression)", formalDefinition = "A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.") 339 protected Expression condition; 340 341 private static final long serialVersionUID = -1706033335L; 342 343 /** 344 * Constructor 345 */ 346 public TriggerDefinition() { 347 super(); 348 } 349 350 /** 351 * Constructor 352 */ 353 public TriggerDefinition(Enumeration<TriggerType> type) { 354 super(); 355 this.type = type; 356 } 357 358 /** 359 * @return {@link #type} (The type of triggering event.). This is the underlying 360 * object with id, value and extensions. The accessor "getType" gives 361 * direct access to the value 362 */ 363 public Enumeration<TriggerType> getTypeElement() { 364 if (this.type == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create TriggerDefinition.type"); 367 else if (Configuration.doAutoCreate()) 368 this.type = new Enumeration<TriggerType>(new TriggerTypeEnumFactory()); // bb 369 return this.type; 370 } 371 372 public boolean hasTypeElement() { 373 return this.type != null && !this.type.isEmpty(); 374 } 375 376 public boolean hasType() { 377 return this.type != null && !this.type.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #type} (The type of triggering event.). This is the 382 * underlying object with id, value and extensions. The accessor 383 * "getType" gives direct access to the value 384 */ 385 public TriggerDefinition setTypeElement(Enumeration<TriggerType> value) { 386 this.type = value; 387 return this; 388 } 389 390 /** 391 * @return The type of triggering event. 392 */ 393 public TriggerType getType() { 394 return this.type == null ? null : this.type.getValue(); 395 } 396 397 /** 398 * @param value The type of triggering event. 399 */ 400 public TriggerDefinition setType(TriggerType value) { 401 if (this.type == null) 402 this.type = new Enumeration<TriggerType>(new TriggerTypeEnumFactory()); 403 this.type.setValue(value); 404 return this; 405 } 406 407 /** 408 * @return {@link #name} (A formal name for the event. This may be an absolute 409 * URI that identifies the event formally (e.g. from a trigger 410 * registry), or a simple relative URI that identifies the event in a 411 * local context.). This is the underlying object with id, value and 412 * extensions. The accessor "getName" gives direct access to the value 413 */ 414 public StringType getNameElement() { 415 if (this.name == null) 416 if (Configuration.errorOnAutoCreate()) 417 throw new Error("Attempt to auto-create TriggerDefinition.name"); 418 else if (Configuration.doAutoCreate()) 419 this.name = new StringType(); // bb 420 return this.name; 421 } 422 423 public boolean hasNameElement() { 424 return this.name != null && !this.name.isEmpty(); 425 } 426 427 public boolean hasName() { 428 return this.name != null && !this.name.isEmpty(); 429 } 430 431 /** 432 * @param value {@link #name} (A formal name for the event. This may be an 433 * absolute URI that identifies the event formally (e.g. from a 434 * trigger registry), or a simple relative URI that identifies the 435 * event in a local context.). This is the underlying object with 436 * id, value and extensions. The accessor "getName" gives direct 437 * access to the value 438 */ 439 public TriggerDefinition setNameElement(StringType value) { 440 this.name = value; 441 return this; 442 } 443 444 /** 445 * @return A formal name for the event. This may be an absolute URI that 446 * identifies the event formally (e.g. from a trigger registry), or a 447 * simple relative URI that identifies the event in a local context. 448 */ 449 public String getName() { 450 return this.name == null ? null : this.name.getValue(); 451 } 452 453 /** 454 * @param value A formal name for the event. This may be an absolute URI that 455 * identifies the event formally (e.g. from a trigger registry), or 456 * a simple relative URI that identifies the event in a local 457 * context. 458 */ 459 public TriggerDefinition setName(String value) { 460 if (Utilities.noString(value)) 461 this.name = null; 462 else { 463 if (this.name == null) 464 this.name = new StringType(); 465 this.name.setValue(value); 466 } 467 return this; 468 } 469 470 /** 471 * @return {@link #timing} (The timing of the event (if this is a periodic 472 * trigger).) 473 */ 474 public Type getTiming() { 475 return this.timing; 476 } 477 478 /** 479 * @return {@link #timing} (The timing of the event (if this is a periodic 480 * trigger).) 481 */ 482 public Timing getTimingTiming() throws FHIRException { 483 if (this.timing == null) 484 this.timing = new Timing(); 485 if (!(this.timing instanceof Timing)) 486 throw new FHIRException( 487 "Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() + " was encountered"); 488 return (Timing) this.timing; 489 } 490 491 public boolean hasTimingTiming() { 492 return this.timing instanceof Timing; 493 } 494 495 /** 496 * @return {@link #timing} (The timing of the event (if this is a periodic 497 * trigger).) 498 */ 499 public Reference getTimingReference() throws FHIRException { 500 if (this.timing == null) 501 this.timing = new Reference(); 502 if (!(this.timing instanceof Reference)) 503 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.timing.getClass().getName() 504 + " was encountered"); 505 return (Reference) this.timing; 506 } 507 508 public boolean hasTimingReference() { 509 return this.timing instanceof Reference; 510 } 511 512 /** 513 * @return {@link #timing} (The timing of the event (if this is a periodic 514 * trigger).) 515 */ 516 public DateType getTimingDateType() throws FHIRException { 517 if (this.timing == null) 518 this.timing = new DateType(); 519 if (!(this.timing instanceof DateType)) 520 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.timing.getClass().getName() 521 + " was encountered"); 522 return (DateType) this.timing; 523 } 524 525 public boolean hasTimingDateType() { 526 return this.timing instanceof DateType; 527 } 528 529 /** 530 * @return {@link #timing} (The timing of the event (if this is a periodic 531 * trigger).) 532 */ 533 public DateTimeType getTimingDateTimeType() throws FHIRException { 534 if (this.timing == null) 535 this.timing = new DateTimeType(); 536 if (!(this.timing instanceof DateTimeType)) 537 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 538 + this.timing.getClass().getName() + " was encountered"); 539 return (DateTimeType) this.timing; 540 } 541 542 public boolean hasTimingDateTimeType() { 543 return this.timing instanceof DateTimeType; 544 } 545 546 public boolean hasTiming() { 547 return this.timing != null && !this.timing.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #timing} (The timing of the event (if this is a periodic 552 * trigger).) 553 */ 554 public TriggerDefinition setTiming(Type value) { 555 if (value != null && !(value instanceof Timing || value instanceof Reference || value instanceof DateType 556 || value instanceof DateTimeType)) 557 throw new Error("Not the right type for TriggerDefinition.timing[x]: " + value.fhirType()); 558 this.timing = value; 559 return this; 560 } 561 562 /** 563 * @return {@link #data} (The triggering data of the event (if this is a data 564 * trigger). If more than one data is requirement is specified, then all 565 * the data requirements must be true.) 566 */ 567 public List<DataRequirement> getData() { 568 if (this.data == null) 569 this.data = new ArrayList<DataRequirement>(); 570 return this.data; 571 } 572 573 /** 574 * @return Returns a reference to <code>this</code> for easy method chaining 575 */ 576 public TriggerDefinition setData(List<DataRequirement> theData) { 577 this.data = theData; 578 return this; 579 } 580 581 public boolean hasData() { 582 if (this.data == null) 583 return false; 584 for (DataRequirement item : this.data) 585 if (!item.isEmpty()) 586 return true; 587 return false; 588 } 589 590 public DataRequirement addData() { // 3 591 DataRequirement t = new DataRequirement(); 592 if (this.data == null) 593 this.data = new ArrayList<DataRequirement>(); 594 this.data.add(t); 595 return t; 596 } 597 598 public TriggerDefinition addData(DataRequirement t) { // 3 599 if (t == null) 600 return this; 601 if (this.data == null) 602 this.data = new ArrayList<DataRequirement>(); 603 this.data.add(t); 604 return this; 605 } 606 607 /** 608 * @return The first repetition of repeating field {@link #data}, creating it if 609 * it does not already exist 610 */ 611 public DataRequirement getDataFirstRep() { 612 if (getData().isEmpty()) { 613 addData(); 614 } 615 return getData().get(0); 616 } 617 618 /** 619 * @return {@link #condition} (A boolean-valued expression that is evaluated in 620 * the context of the container of the trigger definition and returns 621 * whether or not the trigger fires.) 622 */ 623 public Expression getCondition() { 624 if (this.condition == null) 625 if (Configuration.errorOnAutoCreate()) 626 throw new Error("Attempt to auto-create TriggerDefinition.condition"); 627 else if (Configuration.doAutoCreate()) 628 this.condition = new Expression(); // cc 629 return this.condition; 630 } 631 632 public boolean hasCondition() { 633 return this.condition != null && !this.condition.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #condition} (A boolean-valued expression that is 638 * evaluated in the context of the container of the trigger 639 * definition and returns whether or not the trigger fires.) 640 */ 641 public TriggerDefinition setCondition(Expression value) { 642 this.condition = value; 643 return this; 644 } 645 646 protected void listChildren(List<Property> children) { 647 super.listChildren(children); 648 children.add(new Property("type", "code", "The type of triggering event.", 0, 1, type)); 649 children.add(new Property("name", "string", 650 "A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.", 651 0, 1, name)); 652 children.add(new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", 653 "The timing of the event (if this is a periodic trigger).", 0, 1, timing)); 654 children.add(new Property("data", "DataRequirement", 655 "The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.", 656 0, java.lang.Integer.MAX_VALUE, data)); 657 children.add(new Property("condition", "Expression", 658 "A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.", 659 0, 1, condition)); 660 } 661 662 @Override 663 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 664 switch (_hash) { 665 case 3575610: 666 /* type */ return new Property("type", "code", "The type of triggering event.", 0, 1, type); 667 case 3373707: 668 /* name */ return new Property("name", "string", 669 "A formal name for the event. This may be an absolute URI that identifies the event formally (e.g. from a trigger registry), or a simple relative URI that identifies the event in a local context.", 670 0, 1, name); 671 case 164632566: 672 /* timing[x] */ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", 673 "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 674 case -873664438: 675 /* timing */ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", 676 "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 677 case -497554124: 678 /* timingTiming */ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", 679 "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 680 case -1792466399: 681 /* timingReference */ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", 682 "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 683 case 807935768: 684 /* timingDate */ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", 685 "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 686 case -1837458939: 687 /* timingDateTime */ return new Property("timing[x]", "Timing|Reference(Schedule)|date|dateTime", 688 "The timing of the event (if this is a periodic trigger).", 0, 1, timing); 689 case 3076010: 690 /* data */ return new Property("data", "DataRequirement", 691 "The triggering data of the event (if this is a data trigger). If more than one data is requirement is specified, then all the data requirements must be true.", 692 0, java.lang.Integer.MAX_VALUE, data); 693 case -861311717: 694 /* condition */ return new Property("condition", "Expression", 695 "A boolean-valued expression that is evaluated in the context of the container of the trigger definition and returns whether or not the trigger fires.", 696 0, 1, condition); 697 default: 698 return super.getNamedProperty(_hash, _name, _checkValid); 699 } 700 701 } 702 703 @Override 704 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 705 switch (hash) { 706 case 3575610: 707 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<TriggerType> 708 case 3373707: 709 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 710 case -873664438: 711 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 712 case 3076010: 713 /* data */ return this.data == null ? new Base[0] : this.data.toArray(new Base[this.data.size()]); // DataRequirement 714 case -861311717: 715 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // Expression 716 default: 717 return super.getProperty(hash, name, checkValid); 718 } 719 720 } 721 722 @Override 723 public Base setProperty(int hash, String name, Base value) throws FHIRException { 724 switch (hash) { 725 case 3575610: // type 726 value = new TriggerTypeEnumFactory().fromType(castToCode(value)); 727 this.type = (Enumeration) value; // Enumeration<TriggerType> 728 return value; 729 case 3373707: // name 730 this.name = castToString(value); // StringType 731 return value; 732 case -873664438: // timing 733 this.timing = castToType(value); // Type 734 return value; 735 case 3076010: // data 736 this.getData().add(castToDataRequirement(value)); // DataRequirement 737 return value; 738 case -861311717: // condition 739 this.condition = castToExpression(value); // Expression 740 return value; 741 default: 742 return super.setProperty(hash, name, value); 743 } 744 745 } 746 747 @Override 748 public Base setProperty(String name, Base value) throws FHIRException { 749 if (name.equals("type")) { 750 value = new TriggerTypeEnumFactory().fromType(castToCode(value)); 751 this.type = (Enumeration) value; // Enumeration<TriggerType> 752 } else if (name.equals("name")) { 753 this.name = castToString(value); // StringType 754 } else if (name.equals("timing[x]")) { 755 this.timing = castToType(value); // Type 756 } else if (name.equals("data")) { 757 this.getData().add(castToDataRequirement(value)); 758 } else if (name.equals("condition")) { 759 this.condition = castToExpression(value); // Expression 760 } else 761 return super.setProperty(name, value); 762 return value; 763 } 764 765 @Override 766 public void removeChild(String name, Base value) throws FHIRException { 767 if (name.equals("type")) { 768 this.type = null; 769 } else if (name.equals("name")) { 770 this.name = null; 771 } else if (name.equals("timing[x]")) { 772 this.timing = null; 773 } else if (name.equals("data")) { 774 this.getData().remove(castToDataRequirement(value)); 775 } else if (name.equals("condition")) { 776 this.condition = null; 777 } else 778 super.removeChild(name, value); 779 780 } 781 782 @Override 783 public Base makeProperty(int hash, String name) throws FHIRException { 784 switch (hash) { 785 case 3575610: 786 return getTypeElement(); 787 case 3373707: 788 return getNameElement(); 789 case 164632566: 790 return getTiming(); 791 case -873664438: 792 return getTiming(); 793 case 3076010: 794 return addData(); 795 case -861311717: 796 return getCondition(); 797 default: 798 return super.makeProperty(hash, name); 799 } 800 801 } 802 803 @Override 804 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 805 switch (hash) { 806 case 3575610: 807 /* type */ return new String[] { "code" }; 808 case 3373707: 809 /* name */ return new String[] { "string" }; 810 case -873664438: 811 /* timing */ return new String[] { "Timing", "Reference", "date", "dateTime" }; 812 case 3076010: 813 /* data */ return new String[] { "DataRequirement" }; 814 case -861311717: 815 /* condition */ return new String[] { "Expression" }; 816 default: 817 return super.getTypesForProperty(hash, name); 818 } 819 820 } 821 822 @Override 823 public Base addChild(String name) throws FHIRException { 824 if (name.equals("type")) { 825 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.type"); 826 } else if (name.equals("name")) { 827 throw new FHIRException("Cannot call addChild on a singleton property TriggerDefinition.name"); 828 } else if (name.equals("timingTiming")) { 829 this.timing = new Timing(); 830 return this.timing; 831 } else if (name.equals("timingReference")) { 832 this.timing = new Reference(); 833 return this.timing; 834 } else if (name.equals("timingDate")) { 835 this.timing = new DateType(); 836 return this.timing; 837 } else if (name.equals("timingDateTime")) { 838 this.timing = new DateTimeType(); 839 return this.timing; 840 } else if (name.equals("data")) { 841 return addData(); 842 } else if (name.equals("condition")) { 843 this.condition = new Expression(); 844 return this.condition; 845 } else 846 return super.addChild(name); 847 } 848 849 public String fhirType() { 850 return "TriggerDefinition"; 851 852 } 853 854 public TriggerDefinition copy() { 855 TriggerDefinition dst = new TriggerDefinition(); 856 copyValues(dst); 857 return dst; 858 } 859 860 public void copyValues(TriggerDefinition dst) { 861 super.copyValues(dst); 862 dst.type = type == null ? null : type.copy(); 863 dst.name = name == null ? null : name.copy(); 864 dst.timing = timing == null ? null : timing.copy(); 865 if (data != null) { 866 dst.data = new ArrayList<DataRequirement>(); 867 for (DataRequirement i : data) 868 dst.data.add(i.copy()); 869 } 870 ; 871 dst.condition = condition == null ? null : condition.copy(); 872 } 873 874 protected TriggerDefinition typedCopy() { 875 return copy(); 876 } 877 878 @Override 879 public boolean equalsDeep(Base other_) { 880 if (!super.equalsDeep(other_)) 881 return false; 882 if (!(other_ instanceof TriggerDefinition)) 883 return false; 884 TriggerDefinition o = (TriggerDefinition) other_; 885 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(timing, o.timing, true) 886 && compareDeep(data, o.data, true) && compareDeep(condition, o.condition, true); 887 } 888 889 @Override 890 public boolean equalsShallow(Base other_) { 891 if (!super.equalsShallow(other_)) 892 return false; 893 if (!(other_ instanceof TriggerDefinition)) 894 return false; 895 TriggerDefinition o = (TriggerDefinition) other_; 896 return compareValues(type, o.type, true) && compareValues(name, o.name, true); 897 } 898 899 public boolean isEmpty() { 900 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name, timing, data, condition); 901 } 902 903}