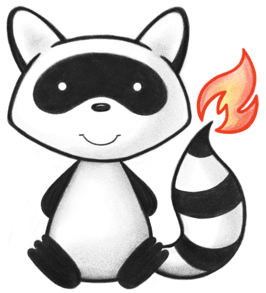
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.DatatypeDef; 040import ca.uhn.fhir.model.api.annotation.Description; 041 042/** 043 * Specifies clinical/business/etc. metadata that can be used to retrieve, index 044 * and/or categorize an artifact. This metadata can either be specific to the 045 * applicable population (e.g., age category, DRG) or the specific context of 046 * care (e.g., venue, care setting, provider of care). 047 */ 048@DatatypeDef(name = "UsageContext") 049public class UsageContext extends Type implements ICompositeType { 050 051 /** 052 * A code that identifies the type of context being specified by this usage 053 * context. 054 */ 055 @Child(name = "code", type = { Coding.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 056 @Description(shortDefinition = "Type of context being specified", formalDefinition = "A code that identifies the type of context being specified by this usage context.") 057 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/usage-context-type") 058 protected Coding code; 059 060 /** 061 * A value that defines the context specified in this context of use. The 062 * interpretation of the value is defined by the code. 063 */ 064 @Child(name = "value", type = { CodeableConcept.class, Quantity.class, Range.class, PlanDefinition.class, 065 ResearchStudy.class, InsurancePlan.class, HealthcareService.class, Group.class, Location.class, 066 Organization.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 067 @Description(shortDefinition = "Value that defines the context", formalDefinition = "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.") 068 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/use-context") 069 protected Type value; 070 071 private static final long serialVersionUID = -1092486508L; 072 073 /** 074 * Constructor 075 */ 076 public UsageContext() { 077 super(); 078 } 079 080 /** 081 * Constructor 082 */ 083 public UsageContext(Coding code, Type value) { 084 super(); 085 this.code = code; 086 this.value = value; 087 } 088 089 /** 090 * @return {@link #code} (A code that identifies the type of context being 091 * specified by this usage context.) 092 */ 093 public Coding getCode() { 094 if (this.code == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create UsageContext.code"); 097 else if (Configuration.doAutoCreate()) 098 this.code = new Coding(); // cc 099 return this.code; 100 } 101 102 public boolean hasCode() { 103 return this.code != null && !this.code.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #code} (A code that identifies the type of context being 108 * specified by this usage context.) 109 */ 110 public UsageContext setCode(Coding value) { 111 this.code = value; 112 return this; 113 } 114 115 /** 116 * @return {@link #value} (A value that defines the context specified in this 117 * context of use. The interpretation of the value is defined by the 118 * code.) 119 */ 120 public Type getValue() { 121 return this.value; 122 } 123 124 /** 125 * @return {@link #value} (A value that defines the context specified in this 126 * context of use. The interpretation of the value is defined by the 127 * code.) 128 */ 129 public CodeableConcept getValueCodeableConcept() throws FHIRException { 130 if (this.value == null) 131 this.value = new CodeableConcept(); 132 if (!(this.value instanceof CodeableConcept)) 133 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 134 + this.value.getClass().getName() + " was encountered"); 135 return (CodeableConcept) this.value; 136 } 137 138 public boolean hasValueCodeableConcept() { 139 return this.value instanceof CodeableConcept; 140 } 141 142 /** 143 * @return {@link #value} (A value that defines the context specified in this 144 * context of use. The interpretation of the value is defined by the 145 * code.) 146 */ 147 public Quantity getValueQuantity() throws FHIRException { 148 if (this.value == null) 149 this.value = new Quantity(); 150 if (!(this.value instanceof Quantity)) 151 throw new FHIRException( 152 "Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() + " was encountered"); 153 return (Quantity) this.value; 154 } 155 156 public boolean hasValueQuantity() { 157 return this.value instanceof Quantity; 158 } 159 160 /** 161 * @return {@link #value} (A value that defines the context specified in this 162 * context of use. The interpretation of the value is defined by the 163 * code.) 164 */ 165 public Range getValueRange() throws FHIRException { 166 if (this.value == null) 167 this.value = new Range(); 168 if (!(this.value instanceof Range)) 169 throw new FHIRException( 170 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 171 return (Range) this.value; 172 } 173 174 public boolean hasValueRange() { 175 return this.value instanceof Range; 176 } 177 178 /** 179 * @return {@link #value} (A value that defines the context specified in this 180 * context of use. The interpretation of the value is defined by the 181 * code.) 182 */ 183 public Reference getValueReference() throws FHIRException { 184 if (this.value == null) 185 this.value = new Reference(); 186 if (!(this.value instanceof Reference)) 187 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 188 + " was encountered"); 189 return (Reference) this.value; 190 } 191 192 public boolean hasValueReference() { 193 return this.value instanceof Reference; 194 } 195 196 public boolean hasValue() { 197 return this.value != null && !this.value.isEmpty(); 198 } 199 200 /** 201 * @param value {@link #value} (A value that defines the context specified in 202 * this context of use. The interpretation of the value is defined 203 * by the code.) 204 */ 205 public UsageContext setValue(Type value) { 206 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range 207 || value instanceof Reference)) 208 throw new Error("Not the right type for UsageContext.value[x]: " + value.fhirType()); 209 this.value = value; 210 return this; 211 } 212 213 protected void listChildren(List<Property> children) { 214 super.listChildren(children); 215 children.add(new Property("code", "Coding", 216 "A code that identifies the type of context being specified by this usage context.", 0, 1, code)); 217 children.add(new Property("value[x]", 218 "CodeableConcept|Quantity|Range|Reference(PlanDefinition|ResearchStudy|InsurancePlan|HealthcareService|Group|Location|Organization)", 219 "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 220 0, 1, value)); 221 } 222 223 @Override 224 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 225 switch (_hash) { 226 case 3059181: 227 /* code */ return new Property("code", "Coding", 228 "A code that identifies the type of context being specified by this usage context.", 0, 1, code); 229 case -1410166417: 230 /* value[x] */ return new Property("value[x]", 231 "CodeableConcept|Quantity|Range|Reference(PlanDefinition|ResearchStudy|InsurancePlan|HealthcareService|Group|Location|Organization)", 232 "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 233 0, 1, value); 234 case 111972721: 235 /* value */ return new Property("value[x]", 236 "CodeableConcept|Quantity|Range|Reference(PlanDefinition|ResearchStudy|InsurancePlan|HealthcareService|Group|Location|Organization)", 237 "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 238 0, 1, value); 239 case 924902896: 240 /* valueCodeableConcept */ return new Property("value[x]", 241 "CodeableConcept|Quantity|Range|Reference(PlanDefinition|ResearchStudy|InsurancePlan|HealthcareService|Group|Location|Organization)", 242 "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 243 0, 1, value); 244 case -2029823716: 245 /* valueQuantity */ return new Property("value[x]", 246 "CodeableConcept|Quantity|Range|Reference(PlanDefinition|ResearchStudy|InsurancePlan|HealthcareService|Group|Location|Organization)", 247 "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 248 0, 1, value); 249 case 2030761548: 250 /* valueRange */ return new Property("value[x]", 251 "CodeableConcept|Quantity|Range|Reference(PlanDefinition|ResearchStudy|InsurancePlan|HealthcareService|Group|Location|Organization)", 252 "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 253 0, 1, value); 254 case 1755241690: 255 /* valueReference */ return new Property("value[x]", 256 "CodeableConcept|Quantity|Range|Reference(PlanDefinition|ResearchStudy|InsurancePlan|HealthcareService|Group|Location|Organization)", 257 "A value that defines the context specified in this context of use. The interpretation of the value is defined by the code.", 258 0, 1, value); 259 default: 260 return super.getNamedProperty(_hash, _name, _checkValid); 261 } 262 263 } 264 265 @Override 266 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 267 switch (hash) { 268 case 3059181: 269 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Coding 270 case 111972721: 271 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 272 default: 273 return super.getProperty(hash, name, checkValid); 274 } 275 276 } 277 278 @Override 279 public Base setProperty(int hash, String name, Base value) throws FHIRException { 280 switch (hash) { 281 case 3059181: // code 282 this.code = castToCoding(value); // Coding 283 return value; 284 case 111972721: // value 285 this.value = castToType(value); // Type 286 return value; 287 default: 288 return super.setProperty(hash, name, value); 289 } 290 291 } 292 293 @Override 294 public Base setProperty(String name, Base value) throws FHIRException { 295 if (name.equals("code")) { 296 this.code = castToCoding(value); // Coding 297 } else if (name.equals("value[x]")) { 298 this.value = castToType(value); // Type 299 } else 300 return super.setProperty(name, value); 301 return value; 302 } 303 304 @Override 305 public void removeChild(String name, Base value) throws FHIRException { 306 if (name.equals("code")) { 307 this.code = null; 308 } else if (name.equals("value[x]")) { 309 this.value = null; 310 } else 311 super.removeChild(name, value); 312 313 } 314 315 @Override 316 public Base makeProperty(int hash, String name) throws FHIRException { 317 switch (hash) { 318 case 3059181: 319 return getCode(); 320 case -1410166417: 321 return getValue(); 322 case 111972721: 323 return getValue(); 324 default: 325 return super.makeProperty(hash, name); 326 } 327 328 } 329 330 @Override 331 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 332 switch (hash) { 333 case 3059181: 334 /* code */ return new String[] { "Coding" }; 335 case 111972721: 336 /* value */ return new String[] { "CodeableConcept", "Quantity", "Range", "Reference" }; 337 default: 338 return super.getTypesForProperty(hash, name); 339 } 340 341 } 342 343 @Override 344 public Base addChild(String name) throws FHIRException { 345 if (name.equals("code")) { 346 this.code = new Coding(); 347 return this.code; 348 } else if (name.equals("valueCodeableConcept")) { 349 this.value = new CodeableConcept(); 350 return this.value; 351 } else if (name.equals("valueQuantity")) { 352 this.value = new Quantity(); 353 return this.value; 354 } else if (name.equals("valueRange")) { 355 this.value = new Range(); 356 return this.value; 357 } else if (name.equals("valueReference")) { 358 this.value = new Reference(); 359 return this.value; 360 } else 361 return super.addChild(name); 362 } 363 364 public String fhirType() { 365 return "UsageContext"; 366 367 } 368 369 public UsageContext copy() { 370 UsageContext dst = new UsageContext(); 371 copyValues(dst); 372 return dst; 373 } 374 375 public void copyValues(UsageContext dst) { 376 super.copyValues(dst); 377 dst.code = code == null ? null : code.copy(); 378 dst.value = value == null ? null : value.copy(); 379 } 380 381 protected UsageContext typedCopy() { 382 return copy(); 383 } 384 385 @Override 386 public boolean equalsDeep(Base other_) { 387 if (!super.equalsDeep(other_)) 388 return false; 389 if (!(other_ instanceof UsageContext)) 390 return false; 391 UsageContext o = (UsageContext) other_; 392 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 393 } 394 395 @Override 396 public boolean equalsShallow(Base other_) { 397 if (!super.equalsShallow(other_)) 398 return false; 399 if (!(other_ instanceof UsageContext)) 400 return false; 401 UsageContext o = (UsageContext) other_; 402 return true; 403 } 404 405 public boolean isEmpty() { 406 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 407 } 408 409}