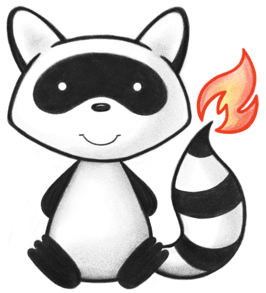
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A ValueSet resource instance specifies a set of codes drawn from one or more 052 * code systems, intended for use in a particular context. Value sets link 053 * between [[[CodeSystem]]] definitions and their use in [coded 054 * elements](terminologies.html). 055 */ 056@ResourceDef(name = "ValueSet", profile = "http://hl7.org/fhir/StructureDefinition/ValueSet") 057@ChildOrder(names = { "url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", 058 "contact", "description", "useContext", "jurisdiction", "immutable", "purpose", "copyright", "compose", 059 "expansion" }) 060public class ValueSet extends MetadataResource { 061 062 public enum FilterOperator { 063 /** 064 * The specified property of the code equals the provided value. 065 */ 066 EQUAL, 067 /** 068 * Includes all concept ids that have a transitive is-a relationship with the 069 * concept Id provided as the value, including the provided concept itself 070 * (include descendant codes and self). 071 */ 072 ISA, 073 /** 074 * Includes all concept ids that have a transitive is-a relationship with the 075 * concept Id provided as the value, excluding the provided concept itself i.e. 076 * include descendant codes only). 077 */ 078 DESCENDENTOF, 079 /** 080 * The specified property of the code does not have an is-a relationship with 081 * the provided value. 082 */ 083 ISNOTA, 084 /** 085 * The specified property of the code matches the regex specified in the 086 * provided value. 087 */ 088 REGEX, 089 /** 090 * The specified property of the code is in the set of codes or concepts 091 * specified in the provided value (comma separated list). 092 */ 093 IN, 094 /** 095 * The specified property of the code is not in the set of codes or concepts 096 * specified in the provided value (comma separated list). 097 */ 098 NOTIN, 099 /** 100 * Includes all concept ids that have a transitive is-a relationship from the 101 * concept Id provided as the value, including the provided concept itself (i.e. 102 * include ancestor codes and self). 103 */ 104 GENERALIZES, 105 /** 106 * The specified property of the code has at least one value (if the specified 107 * value is true; if the specified value is false, then matches when the 108 * specified property of the code has no values). 109 */ 110 EXISTS, 111 /** 112 * added to help the parsers with the generic types 113 */ 114 NULL; 115 116 public static FilterOperator fromCode(String codeString) throws FHIRException { 117 if (codeString == null || "".equals(codeString)) 118 return null; 119 if ("=".equals(codeString)) 120 return EQUAL; 121 if ("is-a".equals(codeString)) 122 return ISA; 123 if ("descendent-of".equals(codeString)) 124 return DESCENDENTOF; 125 if ("is-not-a".equals(codeString)) 126 return ISNOTA; 127 if ("regex".equals(codeString)) 128 return REGEX; 129 if ("in".equals(codeString)) 130 return IN; 131 if ("not-in".equals(codeString)) 132 return NOTIN; 133 if ("generalizes".equals(codeString)) 134 return GENERALIZES; 135 if ("exists".equals(codeString)) 136 return EXISTS; 137 if (Configuration.isAcceptInvalidEnums()) 138 return null; 139 else 140 throw new FHIRException("Unknown FilterOperator code '" + codeString + "'"); 141 } 142 143 public String toCode() { 144 switch (this) { 145 case EQUAL: 146 return "="; 147 case ISA: 148 return "is-a"; 149 case DESCENDENTOF: 150 return "descendent-of"; 151 case ISNOTA: 152 return "is-not-a"; 153 case REGEX: 154 return "regex"; 155 case IN: 156 return "in"; 157 case NOTIN: 158 return "not-in"; 159 case GENERALIZES: 160 return "generalizes"; 161 case EXISTS: 162 return "exists"; 163 case NULL: 164 return null; 165 default: 166 return "?"; 167 } 168 } 169 170 public String getSystem() { 171 switch (this) { 172 case EQUAL: 173 return "http://hl7.org/fhir/filter-operator"; 174 case ISA: 175 return "http://hl7.org/fhir/filter-operator"; 176 case DESCENDENTOF: 177 return "http://hl7.org/fhir/filter-operator"; 178 case ISNOTA: 179 return "http://hl7.org/fhir/filter-operator"; 180 case REGEX: 181 return "http://hl7.org/fhir/filter-operator"; 182 case IN: 183 return "http://hl7.org/fhir/filter-operator"; 184 case NOTIN: 185 return "http://hl7.org/fhir/filter-operator"; 186 case GENERALIZES: 187 return "http://hl7.org/fhir/filter-operator"; 188 case EXISTS: 189 return "http://hl7.org/fhir/filter-operator"; 190 case NULL: 191 return null; 192 default: 193 return "?"; 194 } 195 } 196 197 public String getDefinition() { 198 switch (this) { 199 case EQUAL: 200 return "The specified property of the code equals the provided value."; 201 case ISA: 202 return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, including the provided concept itself (include descendant codes and self)."; 203 case DESCENDENTOF: 204 return "Includes all concept ids that have a transitive is-a relationship with the concept Id provided as the value, excluding the provided concept itself i.e. include descendant codes only)."; 205 case ISNOTA: 206 return "The specified property of the code does not have an is-a relationship with the provided value."; 207 case REGEX: 208 return "The specified property of the code matches the regex specified in the provided value."; 209 case IN: 210 return "The specified property of the code is in the set of codes or concepts specified in the provided value (comma separated list)."; 211 case NOTIN: 212 return "The specified property of the code is not in the set of codes or concepts specified in the provided value (comma separated list)."; 213 case GENERALIZES: 214 return "Includes all concept ids that have a transitive is-a relationship from the concept Id provided as the value, including the provided concept itself (i.e. include ancestor codes and self)."; 215 case EXISTS: 216 return "The specified property of the code has at least one value (if the specified value is true; if the specified value is false, then matches when the specified property of the code has no values)."; 217 case NULL: 218 return null; 219 default: 220 return "?"; 221 } 222 } 223 224 public String getDisplay() { 225 switch (this) { 226 case EQUAL: 227 return "Equals"; 228 case ISA: 229 return "Is A (by subsumption)"; 230 case DESCENDENTOF: 231 return "Descendent Of (by subsumption)"; 232 case ISNOTA: 233 return "Not (Is A) (by subsumption)"; 234 case REGEX: 235 return "Regular Expression"; 236 case IN: 237 return "In Set"; 238 case NOTIN: 239 return "Not in Set"; 240 case GENERALIZES: 241 return "Generalizes (by Subsumption)"; 242 case EXISTS: 243 return "Exists"; 244 case NULL: 245 return null; 246 default: 247 return "?"; 248 } 249 } 250 } 251 252 public static class FilterOperatorEnumFactory implements EnumFactory<FilterOperator> { 253 public FilterOperator fromCode(String codeString) throws IllegalArgumentException { 254 if (codeString == null || "".equals(codeString)) 255 if (codeString == null || "".equals(codeString)) 256 return null; 257 if ("=".equals(codeString)) 258 return FilterOperator.EQUAL; 259 if ("is-a".equals(codeString)) 260 return FilterOperator.ISA; 261 if ("descendent-of".equals(codeString)) 262 return FilterOperator.DESCENDENTOF; 263 if ("is-not-a".equals(codeString)) 264 return FilterOperator.ISNOTA; 265 if ("regex".equals(codeString)) 266 return FilterOperator.REGEX; 267 if ("in".equals(codeString)) 268 return FilterOperator.IN; 269 if ("not-in".equals(codeString)) 270 return FilterOperator.NOTIN; 271 if ("generalizes".equals(codeString)) 272 return FilterOperator.GENERALIZES; 273 if ("exists".equals(codeString)) 274 return FilterOperator.EXISTS; 275 throw new IllegalArgumentException("Unknown FilterOperator code '" + codeString + "'"); 276 } 277 278 public Enumeration<FilterOperator> fromType(PrimitiveType<?> code) throws FHIRException { 279 if (code == null) 280 return null; 281 if (code.isEmpty()) 282 return new Enumeration<FilterOperator>(this, FilterOperator.NULL, code); 283 String codeString = code.asStringValue(); 284 if (codeString == null || "".equals(codeString)) 285 return new Enumeration<FilterOperator>(this, FilterOperator.NULL, code); 286 if ("=".equals(codeString)) 287 return new Enumeration<FilterOperator>(this, FilterOperator.EQUAL, code); 288 if ("is-a".equals(codeString)) 289 return new Enumeration<FilterOperator>(this, FilterOperator.ISA, code); 290 if ("descendent-of".equals(codeString)) 291 return new Enumeration<FilterOperator>(this, FilterOperator.DESCENDENTOF, code); 292 if ("is-not-a".equals(codeString)) 293 return new Enumeration<FilterOperator>(this, FilterOperator.ISNOTA, code); 294 if ("regex".equals(codeString)) 295 return new Enumeration<FilterOperator>(this, FilterOperator.REGEX, code); 296 if ("in".equals(codeString)) 297 return new Enumeration<FilterOperator>(this, FilterOperator.IN, code); 298 if ("not-in".equals(codeString)) 299 return new Enumeration<FilterOperator>(this, FilterOperator.NOTIN, code); 300 if ("generalizes".equals(codeString)) 301 return new Enumeration<FilterOperator>(this, FilterOperator.GENERALIZES, code); 302 if ("exists".equals(codeString)) 303 return new Enumeration<FilterOperator>(this, FilterOperator.EXISTS, code); 304 throw new FHIRException("Unknown FilterOperator code '" + codeString + "'"); 305 } 306 307 public String toCode(FilterOperator code) { 308 if (code == FilterOperator.NULL) 309 return null; 310 if (code == FilterOperator.EQUAL) 311 return "="; 312 if (code == FilterOperator.ISA) 313 return "is-a"; 314 if (code == FilterOperator.DESCENDENTOF) 315 return "descendent-of"; 316 if (code == FilterOperator.ISNOTA) 317 return "is-not-a"; 318 if (code == FilterOperator.REGEX) 319 return "regex"; 320 if (code == FilterOperator.IN) 321 return "in"; 322 if (code == FilterOperator.NOTIN) 323 return "not-in"; 324 if (code == FilterOperator.GENERALIZES) 325 return "generalizes"; 326 if (code == FilterOperator.EXISTS) 327 return "exists"; 328 return "?"; 329 } 330 331 public String toSystem(FilterOperator code) { 332 return code.getSystem(); 333 } 334 } 335 336 @Block() 337 public static class ValueSetComposeComponent extends BackboneElement implements IBaseBackboneElement { 338 /** 339 * The Locked Date is the effective date that is used to determine the version 340 * of all referenced Code Systems and Value Set Definitions included in the 341 * compose that are not already tied to a specific version. 342 */ 343 @Child(name = "lockedDate", type = { 344 DateType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 345 @Description(shortDefinition = "Fixed date for references with no specified version (transitive)", formalDefinition = "The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version.") 346 protected DateType lockedDate; 347 348 /** 349 * Whether inactive codes - codes that are not approved for current use - are in 350 * the value set. If inactive = true, inactive codes are to be included in the 351 * expansion, if inactive = false, the inactive codes will not be included in 352 * the expansion. If absent, the behavior is determined by the implementation, 353 * or by the applicable $expand parameters (but generally, inactive codes would 354 * be expected to be included). 355 */ 356 @Child(name = "inactive", type = { 357 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 358 @Description(shortDefinition = "Whether inactive codes are in the value set", formalDefinition = "Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included).") 359 protected BooleanType inactive; 360 361 /** 362 * Include one or more codes from a code system or other value set(s). 363 */ 364 @Child(name = "include", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 365 @Description(shortDefinition = "Include one or more codes from a code system or other value set(s)", formalDefinition = "Include one or more codes from a code system or other value set(s).") 366 protected List<ConceptSetComponent> include; 367 368 /** 369 * Exclude one or more codes from the value set based on code system filters 370 * and/or other value sets. 371 */ 372 @Child(name = "exclude", type = { 373 ConceptSetComponent.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 374 @Description(shortDefinition = "Explicitly exclude codes from a code system or other value sets", formalDefinition = "Exclude one or more codes from the value set based on code system filters and/or other value sets.") 375 protected List<ConceptSetComponent> exclude; 376 377 private static final long serialVersionUID = -765941757L; 378 379 /** 380 * Constructor 381 */ 382 public ValueSetComposeComponent() { 383 super(); 384 } 385 386 /** 387 * @return {@link #lockedDate} (The Locked Date is the effective date that is 388 * used to determine the version of all referenced Code Systems and 389 * Value Set Definitions included in the compose that are not already 390 * tied to a specific version.). This is the underlying object with id, 391 * value and extensions. The accessor "getLockedDate" gives direct 392 * access to the value 393 */ 394 public DateType getLockedDateElement() { 395 if (this.lockedDate == null) 396 if (Configuration.errorOnAutoCreate()) 397 throw new Error("Attempt to auto-create ValueSetComposeComponent.lockedDate"); 398 else if (Configuration.doAutoCreate()) 399 this.lockedDate = new DateType(); // bb 400 return this.lockedDate; 401 } 402 403 public boolean hasLockedDateElement() { 404 return this.lockedDate != null && !this.lockedDate.isEmpty(); 405 } 406 407 public boolean hasLockedDate() { 408 return this.lockedDate != null && !this.lockedDate.isEmpty(); 409 } 410 411 /** 412 * @param value {@link #lockedDate} (The Locked Date is the effective date that 413 * is used to determine the version of all referenced Code Systems 414 * and Value Set Definitions included in the compose that are not 415 * already tied to a specific version.). This is the underlying 416 * object with id, value and extensions. The accessor 417 * "getLockedDate" gives direct access to the value 418 */ 419 public ValueSetComposeComponent setLockedDateElement(DateType value) { 420 this.lockedDate = value; 421 return this; 422 } 423 424 /** 425 * @return The Locked Date is the effective date that is used to determine the 426 * version of all referenced Code Systems and Value Set Definitions 427 * included in the compose that are not already tied to a specific 428 * version. 429 */ 430 public Date getLockedDate() { 431 return this.lockedDate == null ? null : this.lockedDate.getValue(); 432 } 433 434 /** 435 * @param value The Locked Date is the effective date that is used to determine 436 * the version of all referenced Code Systems and Value Set 437 * Definitions included in the compose that are not already tied to 438 * a specific version. 439 */ 440 public ValueSetComposeComponent setLockedDate(Date value) { 441 if (value == null) 442 this.lockedDate = null; 443 else { 444 if (this.lockedDate == null) 445 this.lockedDate = new DateType(); 446 this.lockedDate.setValue(value); 447 } 448 return this; 449 } 450 451 /** 452 * @return {@link #inactive} (Whether inactive codes - codes that are not 453 * approved for current use - are in the value set. If inactive = true, 454 * inactive codes are to be included in the expansion, if inactive = 455 * false, the inactive codes will not be included in the expansion. If 456 * absent, the behavior is determined by the implementation, or by the 457 * applicable $expand parameters (but generally, inactive codes would be 458 * expected to be included).). This is the underlying object with id, 459 * value and extensions. The accessor "getInactive" gives direct access 460 * to the value 461 */ 462 public BooleanType getInactiveElement() { 463 if (this.inactive == null) 464 if (Configuration.errorOnAutoCreate()) 465 throw new Error("Attempt to auto-create ValueSetComposeComponent.inactive"); 466 else if (Configuration.doAutoCreate()) 467 this.inactive = new BooleanType(); // bb 468 return this.inactive; 469 } 470 471 public boolean hasInactiveElement() { 472 return this.inactive != null && !this.inactive.isEmpty(); 473 } 474 475 public boolean hasInactive() { 476 return this.inactive != null && !this.inactive.isEmpty(); 477 } 478 479 /** 480 * @param value {@link #inactive} (Whether inactive codes - codes that are not 481 * approved for current use - are in the value set. If inactive = 482 * true, inactive codes are to be included in the expansion, if 483 * inactive = false, the inactive codes will not be included in the 484 * expansion. If absent, the behavior is determined by the 485 * implementation, or by the applicable $expand parameters (but 486 * generally, inactive codes would be expected to be included).). 487 * This is the underlying object with id, value and extensions. The 488 * accessor "getInactive" gives direct access to the value 489 */ 490 public ValueSetComposeComponent setInactiveElement(BooleanType value) { 491 this.inactive = value; 492 return this; 493 } 494 495 /** 496 * @return Whether inactive codes - codes that are not approved for current use 497 * - are in the value set. If inactive = true, inactive codes are to be 498 * included in the expansion, if inactive = false, the inactive codes 499 * will not be included in the expansion. If absent, the behavior is 500 * determined by the implementation, or by the applicable $expand 501 * parameters (but generally, inactive codes would be expected to be 502 * included). 503 */ 504 public boolean getInactive() { 505 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 506 } 507 508 /** 509 * @param value Whether inactive codes - codes that are not approved for current 510 * use - are in the value set. If inactive = true, inactive codes 511 * are to be included in the expansion, if inactive = false, the 512 * inactive codes will not be included in the expansion. If absent, 513 * the behavior is determined by the implementation, or by the 514 * applicable $expand parameters (but generally, inactive codes 515 * would be expected to be included). 516 */ 517 public ValueSetComposeComponent setInactive(boolean value) { 518 if (this.inactive == null) 519 this.inactive = new BooleanType(); 520 this.inactive.setValue(value); 521 return this; 522 } 523 524 /** 525 * @return {@link #include} (Include one or more codes from a code system or 526 * other value set(s).) 527 */ 528 public List<ConceptSetComponent> getInclude() { 529 if (this.include == null) 530 this.include = new ArrayList<ConceptSetComponent>(); 531 return this.include; 532 } 533 534 /** 535 * @return Returns a reference to <code>this</code> for easy method chaining 536 */ 537 public ValueSetComposeComponent setInclude(List<ConceptSetComponent> theInclude) { 538 this.include = theInclude; 539 return this; 540 } 541 542 public boolean hasInclude() { 543 if (this.include == null) 544 return false; 545 for (ConceptSetComponent item : this.include) 546 if (!item.isEmpty()) 547 return true; 548 return false; 549 } 550 551 public ConceptSetComponent addInclude() { // 3 552 ConceptSetComponent t = new ConceptSetComponent(); 553 if (this.include == null) 554 this.include = new ArrayList<ConceptSetComponent>(); 555 this.include.add(t); 556 return t; 557 } 558 559 public ValueSetComposeComponent addInclude(ConceptSetComponent t) { // 3 560 if (t == null) 561 return this; 562 if (this.include == null) 563 this.include = new ArrayList<ConceptSetComponent>(); 564 this.include.add(t); 565 return this; 566 } 567 568 /** 569 * @return The first repetition of repeating field {@link #include}, creating it 570 * if it does not already exist 571 */ 572 public ConceptSetComponent getIncludeFirstRep() { 573 if (getInclude().isEmpty()) { 574 addInclude(); 575 } 576 return getInclude().get(0); 577 } 578 579 /** 580 * @return {@link #exclude} (Exclude one or more codes from the value set based 581 * on code system filters and/or other value sets.) 582 */ 583 public List<ConceptSetComponent> getExclude() { 584 if (this.exclude == null) 585 this.exclude = new ArrayList<ConceptSetComponent>(); 586 return this.exclude; 587 } 588 589 /** 590 * @return Returns a reference to <code>this</code> for easy method chaining 591 */ 592 public ValueSetComposeComponent setExclude(List<ConceptSetComponent> theExclude) { 593 this.exclude = theExclude; 594 return this; 595 } 596 597 public boolean hasExclude() { 598 if (this.exclude == null) 599 return false; 600 for (ConceptSetComponent item : this.exclude) 601 if (!item.isEmpty()) 602 return true; 603 return false; 604 } 605 606 public ConceptSetComponent addExclude() { // 3 607 ConceptSetComponent t = new ConceptSetComponent(); 608 if (this.exclude == null) 609 this.exclude = new ArrayList<ConceptSetComponent>(); 610 this.exclude.add(t); 611 return t; 612 } 613 614 public ValueSetComposeComponent addExclude(ConceptSetComponent t) { // 3 615 if (t == null) 616 return this; 617 if (this.exclude == null) 618 this.exclude = new ArrayList<ConceptSetComponent>(); 619 this.exclude.add(t); 620 return this; 621 } 622 623 /** 624 * @return The first repetition of repeating field {@link #exclude}, creating it 625 * if it does not already exist 626 */ 627 public ConceptSetComponent getExcludeFirstRep() { 628 if (getExclude().isEmpty()) { 629 addExclude(); 630 } 631 return getExclude().get(0); 632 } 633 634 protected void listChildren(List<Property> children) { 635 super.listChildren(children); 636 children.add(new Property("lockedDate", "date", 637 "The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version.", 638 0, 1, lockedDate)); 639 children.add(new Property("inactive", "boolean", 640 "Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included).", 641 0, 1, inactive)); 642 children.add(new Property("include", "", "Include one or more codes from a code system or other value set(s).", 0, 643 java.lang.Integer.MAX_VALUE, include)); 644 children.add(new Property("exclude", "@ValueSet.compose.include", 645 "Exclude one or more codes from the value set based on code system filters and/or other value sets.", 0, 646 java.lang.Integer.MAX_VALUE, exclude)); 647 } 648 649 @Override 650 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 651 switch (_hash) { 652 case 1391591896: 653 /* lockedDate */ return new Property("lockedDate", "date", 654 "The Locked Date is the effective date that is used to determine the version of all referenced Code Systems and Value Set Definitions included in the compose that are not already tied to a specific version.", 655 0, 1, lockedDate); 656 case 24665195: 657 /* inactive */ return new Property("inactive", "boolean", 658 "Whether inactive codes - codes that are not approved for current use - are in the value set. If inactive = true, inactive codes are to be included in the expansion, if inactive = false, the inactive codes will not be included in the expansion. If absent, the behavior is determined by the implementation, or by the applicable $expand parameters (but generally, inactive codes would be expected to be included).", 659 0, 1, inactive); 660 case 1942574248: 661 /* include */ return new Property("include", "", 662 "Include one or more codes from a code system or other value set(s).", 0, java.lang.Integer.MAX_VALUE, 663 include); 664 case -1321148966: 665 /* exclude */ return new Property("exclude", "@ValueSet.compose.include", 666 "Exclude one or more codes from the value set based on code system filters and/or other value sets.", 0, 667 java.lang.Integer.MAX_VALUE, exclude); 668 default: 669 return super.getNamedProperty(_hash, _name, _checkValid); 670 } 671 672 } 673 674 @Override 675 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 676 switch (hash) { 677 case 1391591896: 678 /* lockedDate */ return this.lockedDate == null ? new Base[0] : new Base[] { this.lockedDate }; // DateType 679 case 24665195: 680 /* inactive */ return this.inactive == null ? new Base[0] : new Base[] { this.inactive }; // BooleanType 681 case 1942574248: 682 /* include */ return this.include == null ? new Base[0] : this.include.toArray(new Base[this.include.size()]); // ConceptSetComponent 683 case -1321148966: 684 /* exclude */ return this.exclude == null ? new Base[0] : this.exclude.toArray(new Base[this.exclude.size()]); // ConceptSetComponent 685 default: 686 return super.getProperty(hash, name, checkValid); 687 } 688 689 } 690 691 @Override 692 public Base setProperty(int hash, String name, Base value) throws FHIRException { 693 switch (hash) { 694 case 1391591896: // lockedDate 695 this.lockedDate = castToDate(value); // DateType 696 return value; 697 case 24665195: // inactive 698 this.inactive = castToBoolean(value); // BooleanType 699 return value; 700 case 1942574248: // include 701 this.getInclude().add((ConceptSetComponent) value); // ConceptSetComponent 702 return value; 703 case -1321148966: // exclude 704 this.getExclude().add((ConceptSetComponent) value); // ConceptSetComponent 705 return value; 706 default: 707 return super.setProperty(hash, name, value); 708 } 709 710 } 711 712 @Override 713 public Base setProperty(String name, Base value) throws FHIRException { 714 if (name.equals("lockedDate")) { 715 this.lockedDate = castToDate(value); // DateType 716 } else if (name.equals("inactive")) { 717 this.inactive = castToBoolean(value); // BooleanType 718 } else if (name.equals("include")) { 719 this.getInclude().add((ConceptSetComponent) value); 720 } else if (name.equals("exclude")) { 721 this.getExclude().add((ConceptSetComponent) value); 722 } else 723 return super.setProperty(name, value); 724 return value; 725 } 726 727 @Override 728 public void removeChild(String name, Base value) throws FHIRException { 729 if (name.equals("lockedDate")) { 730 this.lockedDate = null; 731 } else if (name.equals("inactive")) { 732 this.inactive = null; 733 } else if (name.equals("include")) { 734 this.getInclude().remove((ConceptSetComponent) value); 735 } else if (name.equals("exclude")) { 736 this.getExclude().remove((ConceptSetComponent) value); 737 } else 738 super.removeChild(name, value); 739 740 } 741 742 @Override 743 public Base makeProperty(int hash, String name) throws FHIRException { 744 switch (hash) { 745 case 1391591896: 746 return getLockedDateElement(); 747 case 24665195: 748 return getInactiveElement(); 749 case 1942574248: 750 return addInclude(); 751 case -1321148966: 752 return addExclude(); 753 default: 754 return super.makeProperty(hash, name); 755 } 756 757 } 758 759 @Override 760 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 761 switch (hash) { 762 case 1391591896: 763 /* lockedDate */ return new String[] { "date" }; 764 case 24665195: 765 /* inactive */ return new String[] { "boolean" }; 766 case 1942574248: 767 /* include */ return new String[] {}; 768 case -1321148966: 769 /* exclude */ return new String[] { "@ValueSet.compose.include" }; 770 default: 771 return super.getTypesForProperty(hash, name); 772 } 773 774 } 775 776 @Override 777 public Base addChild(String name) throws FHIRException { 778 if (name.equals("lockedDate")) { 779 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.lockedDate"); 780 } else if (name.equals("inactive")) { 781 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.inactive"); 782 } else if (name.equals("include")) { 783 return addInclude(); 784 } else if (name.equals("exclude")) { 785 return addExclude(); 786 } else 787 return super.addChild(name); 788 } 789 790 public ValueSetComposeComponent copy() { 791 ValueSetComposeComponent dst = new ValueSetComposeComponent(); 792 copyValues(dst); 793 return dst; 794 } 795 796 public void copyValues(ValueSetComposeComponent dst) { 797 super.copyValues(dst); 798 dst.lockedDate = lockedDate == null ? null : lockedDate.copy(); 799 dst.inactive = inactive == null ? null : inactive.copy(); 800 if (include != null) { 801 dst.include = new ArrayList<ConceptSetComponent>(); 802 for (ConceptSetComponent i : include) 803 dst.include.add(i.copy()); 804 } 805 ; 806 if (exclude != null) { 807 dst.exclude = new ArrayList<ConceptSetComponent>(); 808 for (ConceptSetComponent i : exclude) 809 dst.exclude.add(i.copy()); 810 } 811 ; 812 } 813 814 @Override 815 public boolean equalsDeep(Base other_) { 816 if (!super.equalsDeep(other_)) 817 return false; 818 if (!(other_ instanceof ValueSetComposeComponent)) 819 return false; 820 ValueSetComposeComponent o = (ValueSetComposeComponent) other_; 821 return compareDeep(lockedDate, o.lockedDate, true) && compareDeep(inactive, o.inactive, true) 822 && compareDeep(include, o.include, true) && compareDeep(exclude, o.exclude, true); 823 } 824 825 @Override 826 public boolean equalsShallow(Base other_) { 827 if (!super.equalsShallow(other_)) 828 return false; 829 if (!(other_ instanceof ValueSetComposeComponent)) 830 return false; 831 ValueSetComposeComponent o = (ValueSetComposeComponent) other_; 832 return compareValues(lockedDate, o.lockedDate, true) && compareValues(inactive, o.inactive, true); 833 } 834 835 public boolean isEmpty() { 836 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lockedDate, inactive, include, exclude); 837 } 838 839 public String fhirType() { 840 return "ValueSet.compose"; 841 842 } 843 844 } 845 846 @Block() 847 public static class ConceptSetComponent extends BackboneElement implements IBaseBackboneElement { 848 /** 849 * An absolute URI which is the code system from which the selected codes come 850 * from. 851 */ 852 @Child(name = "system", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 853 @Description(shortDefinition = "The system the codes come from", formalDefinition = "An absolute URI which is the code system from which the selected codes come from.") 854 protected UriType system; 855 856 /** 857 * The version of the code system that the codes are selected from, or the 858 * special version '*' for all versions. 859 */ 860 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 861 @Description(shortDefinition = "Specific version of the code system referred to", formalDefinition = "The version of the code system that the codes are selected from, or the special version '*' for all versions.") 862 protected StringType version; 863 864 /** 865 * Specifies a concept to be included or excluded. 866 */ 867 @Child(name = "concept", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 868 @Description(shortDefinition = "A concept defined in the system", formalDefinition = "Specifies a concept to be included or excluded.") 869 protected List<ConceptReferenceComponent> concept; 870 871 /** 872 * Select concepts by specify a matching criterion based on the properties 873 * (including relationships) defined by the system, or on filters defined by the 874 * system. If multiple filters are specified, they SHALL all be true. 875 */ 876 @Child(name = "filter", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 877 @Description(shortDefinition = "Select codes/concepts by their properties (including relationships)", formalDefinition = "Select concepts by specify a matching criterion based on the properties (including relationships) defined by the system, or on filters defined by the system. If multiple filters are specified, they SHALL all be true.") 878 protected List<ConceptSetFilterComponent> filter; 879 880 /** 881 * Selects the concepts found in this value set (based on its value set 882 * definition). This is an absolute URI that is a reference to ValueSet.url. If 883 * multiple value sets are specified this includes the union of the contents of 884 * all of the referenced value sets. 885 */ 886 @Child(name = "valueSet", type = { 887 CanonicalType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 888 @Description(shortDefinition = "Select the contents included in this value set", formalDefinition = "Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the union of the contents of all of the referenced value sets.") 889 protected List<CanonicalType> valueSet; 890 891 private static final long serialVersionUID = 969391146L; 892 893 /** 894 * Constructor 895 */ 896 public ConceptSetComponent() { 897 super(); 898 } 899 900 /** 901 * @return {@link #system} (An absolute URI which is the code system from which 902 * the selected codes come from.). This is the underlying object with 903 * id, value and extensions. The accessor "getSystem" gives direct 904 * access to the value 905 */ 906 public UriType getSystemElement() { 907 if (this.system == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create ConceptSetComponent.system"); 910 else if (Configuration.doAutoCreate()) 911 this.system = new UriType(); // bb 912 return this.system; 913 } 914 915 public boolean hasSystemElement() { 916 return this.system != null && !this.system.isEmpty(); 917 } 918 919 public boolean hasSystem() { 920 return this.system != null && !this.system.isEmpty(); 921 } 922 923 /** 924 * @param value {@link #system} (An absolute URI which is the code system from 925 * which the selected codes come from.). This is the underlying 926 * object with id, value and extensions. The accessor "getSystem" 927 * gives direct access to the value 928 */ 929 public ConceptSetComponent setSystemElement(UriType value) { 930 this.system = value; 931 return this; 932 } 933 934 /** 935 * @return An absolute URI which is the code system from which the selected 936 * codes come from. 937 */ 938 public String getSystem() { 939 return this.system == null ? null : this.system.getValue(); 940 } 941 942 /** 943 * @param value An absolute URI which is the code system from which the selected 944 * codes come from. 945 */ 946 public ConceptSetComponent setSystem(String value) { 947 if (Utilities.noString(value)) 948 this.system = null; 949 else { 950 if (this.system == null) 951 this.system = new UriType(); 952 this.system.setValue(value); 953 } 954 return this; 955 } 956 957 /** 958 * @return {@link #version} (The version of the code system that the codes are 959 * selected from, or the special version '*' for all versions.). This is 960 * the underlying object with id, value and extensions. The accessor 961 * "getVersion" gives direct access to the value 962 */ 963 public StringType getVersionElement() { 964 if (this.version == null) 965 if (Configuration.errorOnAutoCreate()) 966 throw new Error("Attempt to auto-create ConceptSetComponent.version"); 967 else if (Configuration.doAutoCreate()) 968 this.version = new StringType(); // bb 969 return this.version; 970 } 971 972 public boolean hasVersionElement() { 973 return this.version != null && !this.version.isEmpty(); 974 } 975 976 public boolean hasVersion() { 977 return this.version != null && !this.version.isEmpty(); 978 } 979 980 /** 981 * @param value {@link #version} (The version of the code system that the codes 982 * are selected from, or the special version '*' for all 983 * versions.). This is the underlying object with id, value and 984 * extensions. The accessor "getVersion" gives direct access to the 985 * value 986 */ 987 public ConceptSetComponent setVersionElement(StringType value) { 988 this.version = value; 989 return this; 990 } 991 992 /** 993 * @return The version of the code system that the codes are selected from, or 994 * the special version '*' for all versions. 995 */ 996 public String getVersion() { 997 return this.version == null ? null : this.version.getValue(); 998 } 999 1000 /** 1001 * @param value The version of the code system that the codes are selected from, 1002 * or the special version '*' for all versions. 1003 */ 1004 public ConceptSetComponent setVersion(String value) { 1005 if (Utilities.noString(value)) 1006 this.version = null; 1007 else { 1008 if (this.version == null) 1009 this.version = new StringType(); 1010 this.version.setValue(value); 1011 } 1012 return this; 1013 } 1014 1015 /** 1016 * @return {@link #concept} (Specifies a concept to be included or excluded.) 1017 */ 1018 public List<ConceptReferenceComponent> getConcept() { 1019 if (this.concept == null) 1020 this.concept = new ArrayList<ConceptReferenceComponent>(); 1021 return this.concept; 1022 } 1023 1024 /** 1025 * @return Returns a reference to <code>this</code> for easy method chaining 1026 */ 1027 public ConceptSetComponent setConcept(List<ConceptReferenceComponent> theConcept) { 1028 this.concept = theConcept; 1029 return this; 1030 } 1031 1032 public boolean hasConcept() { 1033 if (this.concept == null) 1034 return false; 1035 for (ConceptReferenceComponent item : this.concept) 1036 if (!item.isEmpty()) 1037 return true; 1038 return false; 1039 } 1040 1041 public ConceptReferenceComponent addConcept() { // 3 1042 ConceptReferenceComponent t = new ConceptReferenceComponent(); 1043 if (this.concept == null) 1044 this.concept = new ArrayList<ConceptReferenceComponent>(); 1045 this.concept.add(t); 1046 return t; 1047 } 1048 1049 public ConceptSetComponent addConcept(ConceptReferenceComponent t) { // 3 1050 if (t == null) 1051 return this; 1052 if (this.concept == null) 1053 this.concept = new ArrayList<ConceptReferenceComponent>(); 1054 this.concept.add(t); 1055 return this; 1056 } 1057 1058 /** 1059 * @return The first repetition of repeating field {@link #concept}, creating it 1060 * if it does not already exist 1061 */ 1062 public ConceptReferenceComponent getConceptFirstRep() { 1063 if (getConcept().isEmpty()) { 1064 addConcept(); 1065 } 1066 return getConcept().get(0); 1067 } 1068 1069 /** 1070 * @return {@link #filter} (Select concepts by specify a matching criterion 1071 * based on the properties (including relationships) defined by the 1072 * system, or on filters defined by the system. If multiple filters are 1073 * specified, they SHALL all be true.) 1074 */ 1075 public List<ConceptSetFilterComponent> getFilter() { 1076 if (this.filter == null) 1077 this.filter = new ArrayList<ConceptSetFilterComponent>(); 1078 return this.filter; 1079 } 1080 1081 /** 1082 * @return Returns a reference to <code>this</code> for easy method chaining 1083 */ 1084 public ConceptSetComponent setFilter(List<ConceptSetFilterComponent> theFilter) { 1085 this.filter = theFilter; 1086 return this; 1087 } 1088 1089 public boolean hasFilter() { 1090 if (this.filter == null) 1091 return false; 1092 for (ConceptSetFilterComponent item : this.filter) 1093 if (!item.isEmpty()) 1094 return true; 1095 return false; 1096 } 1097 1098 public ConceptSetFilterComponent addFilter() { // 3 1099 ConceptSetFilterComponent t = new ConceptSetFilterComponent(); 1100 if (this.filter == null) 1101 this.filter = new ArrayList<ConceptSetFilterComponent>(); 1102 this.filter.add(t); 1103 return t; 1104 } 1105 1106 public ConceptSetComponent addFilter(ConceptSetFilterComponent t) { // 3 1107 if (t == null) 1108 return this; 1109 if (this.filter == null) 1110 this.filter = new ArrayList<ConceptSetFilterComponent>(); 1111 this.filter.add(t); 1112 return this; 1113 } 1114 1115 /** 1116 * @return The first repetition of repeating field {@link #filter}, creating it 1117 * if it does not already exist 1118 */ 1119 public ConceptSetFilterComponent getFilterFirstRep() { 1120 if (getFilter().isEmpty()) { 1121 addFilter(); 1122 } 1123 return getFilter().get(0); 1124 } 1125 1126 /** 1127 * @return {@link #valueSet} (Selects the concepts found in this value set 1128 * (based on its value set definition). This is an absolute URI that is 1129 * a reference to ValueSet.url. If multiple value sets are specified 1130 * this includes the union of the contents of all of the referenced 1131 * value sets.) 1132 */ 1133 public List<CanonicalType> getValueSet() { 1134 if (this.valueSet == null) 1135 this.valueSet = new ArrayList<CanonicalType>(); 1136 return this.valueSet; 1137 } 1138 1139 /** 1140 * @return Returns a reference to <code>this</code> for easy method chaining 1141 */ 1142 public ConceptSetComponent setValueSet(List<CanonicalType> theValueSet) { 1143 this.valueSet = theValueSet; 1144 return this; 1145 } 1146 1147 public boolean hasValueSet() { 1148 if (this.valueSet == null) 1149 return false; 1150 for (CanonicalType item : this.valueSet) 1151 if (!item.isEmpty()) 1152 return true; 1153 return false; 1154 } 1155 1156 /** 1157 * @return {@link #valueSet} (Selects the concepts found in this value set 1158 * (based on its value set definition). This is an absolute URI that is 1159 * a reference to ValueSet.url. If multiple value sets are specified 1160 * this includes the union of the contents of all of the referenced 1161 * value sets.) 1162 */ 1163 public CanonicalType addValueSetElement() {// 2 1164 CanonicalType t = new CanonicalType(); 1165 if (this.valueSet == null) 1166 this.valueSet = new ArrayList<CanonicalType>(); 1167 this.valueSet.add(t); 1168 return t; 1169 } 1170 1171 /** 1172 * @param value {@link #valueSet} (Selects the concepts found in this value set 1173 * (based on its value set definition). This is an absolute URI 1174 * that is a reference to ValueSet.url. If multiple value sets are 1175 * specified this includes the union of the contents of all of the 1176 * referenced value sets.) 1177 */ 1178 public ConceptSetComponent addValueSet(String value) { // 1 1179 CanonicalType t = new CanonicalType(); 1180 t.setValue(value); 1181 if (this.valueSet == null) 1182 this.valueSet = new ArrayList<CanonicalType>(); 1183 this.valueSet.add(t); 1184 return this; 1185 } 1186 1187 /** 1188 * @param value {@link #valueSet} (Selects the concepts found in this value set 1189 * (based on its value set definition). This is an absolute URI 1190 * that is a reference to ValueSet.url. If multiple value sets are 1191 * specified this includes the union of the contents of all of the 1192 * referenced value sets.) 1193 */ 1194 public boolean hasValueSet(String value) { 1195 if (this.valueSet == null) 1196 return false; 1197 for (CanonicalType v : this.valueSet) 1198 if (v.getValue().equals(value)) // canonical(ValueSet) 1199 return true; 1200 return false; 1201 } 1202 1203 protected void listChildren(List<Property> children) { 1204 super.listChildren(children); 1205 children.add(new Property("system", "uri", 1206 "An absolute URI which is the code system from which the selected codes come from.", 0, 1, system)); 1207 children.add(new Property("version", "string", 1208 "The version of the code system that the codes are selected from, or the special version '*' for all versions.", 1209 0, 1, version)); 1210 children.add(new Property("concept", "", "Specifies a concept to be included or excluded.", 0, 1211 java.lang.Integer.MAX_VALUE, concept)); 1212 children.add(new Property("filter", "", 1213 "Select concepts by specify a matching criterion based on the properties (including relationships) defined by the system, or on filters defined by the system. If multiple filters are specified, they SHALL all be true.", 1214 0, java.lang.Integer.MAX_VALUE, filter)); 1215 children.add(new Property("valueSet", "canonical(ValueSet)", 1216 "Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the union of the contents of all of the referenced value sets.", 1217 0, java.lang.Integer.MAX_VALUE, valueSet)); 1218 } 1219 1220 @Override 1221 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1222 switch (_hash) { 1223 case -887328209: 1224 /* system */ return new Property("system", "uri", 1225 "An absolute URI which is the code system from which the selected codes come from.", 0, 1, system); 1226 case 351608024: 1227 /* version */ return new Property("version", "string", 1228 "The version of the code system that the codes are selected from, or the special version '*' for all versions.", 1229 0, 1, version); 1230 case 951024232: 1231 /* concept */ return new Property("concept", "", "Specifies a concept to be included or excluded.", 0, 1232 java.lang.Integer.MAX_VALUE, concept); 1233 case -1274492040: 1234 /* filter */ return new Property("filter", "", 1235 "Select concepts by specify a matching criterion based on the properties (including relationships) defined by the system, or on filters defined by the system. If multiple filters are specified, they SHALL all be true.", 1236 0, java.lang.Integer.MAX_VALUE, filter); 1237 case -1410174671: 1238 /* valueSet */ return new Property("valueSet", "canonical(ValueSet)", 1239 "Selects the concepts found in this value set (based on its value set definition). This is an absolute URI that is a reference to ValueSet.url. If multiple value sets are specified this includes the union of the contents of all of the referenced value sets.", 1240 0, java.lang.Integer.MAX_VALUE, valueSet); 1241 default: 1242 return super.getNamedProperty(_hash, _name, _checkValid); 1243 } 1244 1245 } 1246 1247 @Override 1248 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1249 switch (hash) { 1250 case -887328209: 1251 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // UriType 1252 case 351608024: 1253 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 1254 case 951024232: 1255 /* concept */ return this.concept == null ? new Base[0] : this.concept.toArray(new Base[this.concept.size()]); // ConceptReferenceComponent 1256 case -1274492040: 1257 /* filter */ return this.filter == null ? new Base[0] : this.filter.toArray(new Base[this.filter.size()]); // ConceptSetFilterComponent 1258 case -1410174671: 1259 /* valueSet */ return this.valueSet == null ? new Base[0] 1260 : this.valueSet.toArray(new Base[this.valueSet.size()]); // CanonicalType 1261 default: 1262 return super.getProperty(hash, name, checkValid); 1263 } 1264 1265 } 1266 1267 @Override 1268 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1269 switch (hash) { 1270 case -887328209: // system 1271 this.system = castToUri(value); // UriType 1272 return value; 1273 case 351608024: // version 1274 this.version = castToString(value); // StringType 1275 return value; 1276 case 951024232: // concept 1277 this.getConcept().add((ConceptReferenceComponent) value); // ConceptReferenceComponent 1278 return value; 1279 case -1274492040: // filter 1280 this.getFilter().add((ConceptSetFilterComponent) value); // ConceptSetFilterComponent 1281 return value; 1282 case -1410174671: // valueSet 1283 this.getValueSet().add(castToCanonical(value)); // CanonicalType 1284 return value; 1285 default: 1286 return super.setProperty(hash, name, value); 1287 } 1288 1289 } 1290 1291 @Override 1292 public Base setProperty(String name, Base value) throws FHIRException { 1293 if (name.equals("system")) { 1294 this.system = castToUri(value); // UriType 1295 } else if (name.equals("version")) { 1296 this.version = castToString(value); // StringType 1297 } else if (name.equals("concept")) { 1298 this.getConcept().add((ConceptReferenceComponent) value); 1299 } else if (name.equals("filter")) { 1300 this.getFilter().add((ConceptSetFilterComponent) value); 1301 } else if (name.equals("valueSet")) { 1302 this.getValueSet().add(castToCanonical(value)); 1303 } else 1304 return super.setProperty(name, value); 1305 return value; 1306 } 1307 1308 @Override 1309 public void removeChild(String name, Base value) throws FHIRException { 1310 if (name.equals("system")) { 1311 this.system = null; 1312 } else if (name.equals("version")) { 1313 this.version = null; 1314 } else if (name.equals("concept")) { 1315 this.getConcept().remove((ConceptReferenceComponent) value); 1316 } else if (name.equals("filter")) { 1317 this.getFilter().remove((ConceptSetFilterComponent) value); 1318 } else if (name.equals("valueSet")) { 1319 this.getValueSet().remove(castToCanonical(value)); 1320 } else 1321 super.removeChild(name, value); 1322 1323 } 1324 1325 @Override 1326 public Base makeProperty(int hash, String name) throws FHIRException { 1327 switch (hash) { 1328 case -887328209: 1329 return getSystemElement(); 1330 case 351608024: 1331 return getVersionElement(); 1332 case 951024232: 1333 return addConcept(); 1334 case -1274492040: 1335 return addFilter(); 1336 case -1410174671: 1337 return addValueSetElement(); 1338 default: 1339 return super.makeProperty(hash, name); 1340 } 1341 1342 } 1343 1344 @Override 1345 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1346 switch (hash) { 1347 case -887328209: 1348 /* system */ return new String[] { "uri" }; 1349 case 351608024: 1350 /* version */ return new String[] { "string" }; 1351 case 951024232: 1352 /* concept */ return new String[] {}; 1353 case -1274492040: 1354 /* filter */ return new String[] {}; 1355 case -1410174671: 1356 /* valueSet */ return new String[] { "canonical" }; 1357 default: 1358 return super.getTypesForProperty(hash, name); 1359 } 1360 1361 } 1362 1363 @Override 1364 public Base addChild(String name) throws FHIRException { 1365 if (name.equals("system")) { 1366 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.system"); 1367 } else if (name.equals("version")) { 1368 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 1369 } else if (name.equals("concept")) { 1370 return addConcept(); 1371 } else if (name.equals("filter")) { 1372 return addFilter(); 1373 } else if (name.equals("valueSet")) { 1374 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.valueSet"); 1375 } else 1376 return super.addChild(name); 1377 } 1378 1379 public ConceptSetComponent copy() { 1380 ConceptSetComponent dst = new ConceptSetComponent(); 1381 copyValues(dst); 1382 return dst; 1383 } 1384 1385 public void copyValues(ConceptSetComponent dst) { 1386 super.copyValues(dst); 1387 dst.system = system == null ? null : system.copy(); 1388 dst.version = version == null ? null : version.copy(); 1389 if (concept != null) { 1390 dst.concept = new ArrayList<ConceptReferenceComponent>(); 1391 for (ConceptReferenceComponent i : concept) 1392 dst.concept.add(i.copy()); 1393 } 1394 ; 1395 if (filter != null) { 1396 dst.filter = new ArrayList<ConceptSetFilterComponent>(); 1397 for (ConceptSetFilterComponent i : filter) 1398 dst.filter.add(i.copy()); 1399 } 1400 ; 1401 if (valueSet != null) { 1402 dst.valueSet = new ArrayList<CanonicalType>(); 1403 for (CanonicalType i : valueSet) 1404 dst.valueSet.add(i.copy()); 1405 } 1406 ; 1407 } 1408 1409 @Override 1410 public boolean equalsDeep(Base other_) { 1411 if (!super.equalsDeep(other_)) 1412 return false; 1413 if (!(other_ instanceof ConceptSetComponent)) 1414 return false; 1415 ConceptSetComponent o = (ConceptSetComponent) other_; 1416 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) 1417 && compareDeep(concept, o.concept, true) && compareDeep(filter, o.filter, true) 1418 && compareDeep(valueSet, o.valueSet, true); 1419 } 1420 1421 @Override 1422 public boolean equalsShallow(Base other_) { 1423 if (!super.equalsShallow(other_)) 1424 return false; 1425 if (!(other_ instanceof ConceptSetComponent)) 1426 return false; 1427 ConceptSetComponent o = (ConceptSetComponent) other_; 1428 return compareValues(system, o.system, true) && compareValues(version, o.version, true); 1429 } 1430 1431 public boolean isEmpty() { 1432 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version, concept, filter, valueSet); 1433 } 1434 1435 public String fhirType() { 1436 return "ValueSet.compose.include"; 1437 1438 } 1439 1440 } 1441 1442 @Block() 1443 public static class ConceptReferenceComponent extends BackboneElement implements IBaseBackboneElement { 1444 /** 1445 * Specifies a code for the concept to be included or excluded. 1446 */ 1447 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1448 @Description(shortDefinition = "Code or expression from system", formalDefinition = "Specifies a code for the concept to be included or excluded.") 1449 protected CodeType code; 1450 1451 /** 1452 * The text to display to the user for this concept in the context of this 1453 * valueset. If no display is provided, then applications using the value set 1454 * use the display specified for the code by the system. 1455 */ 1456 @Child(name = "display", type = { 1457 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1458 @Description(shortDefinition = "Text to display for this code for this value set in this valueset", formalDefinition = "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.") 1459 protected StringType display; 1460 1461 /** 1462 * Additional representations for this concept when used in this value set - 1463 * other languages, aliases, specialized purposes, used for particular purposes, 1464 * etc. 1465 */ 1466 @Child(name = "designation", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1467 @Description(shortDefinition = "Additional representations for this concept", formalDefinition = "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.") 1468 protected List<ConceptReferenceDesignationComponent> designation; 1469 1470 private static final long serialVersionUID = 260579971L; 1471 1472 /** 1473 * Constructor 1474 */ 1475 public ConceptReferenceComponent() { 1476 super(); 1477 } 1478 1479 /** 1480 * Constructor 1481 */ 1482 public ConceptReferenceComponent(CodeType code) { 1483 super(); 1484 this.code = code; 1485 } 1486 1487 /** 1488 * @return {@link #code} (Specifies a code for the concept to be included or 1489 * excluded.). This is the underlying object with id, value and 1490 * extensions. The accessor "getCode" gives direct access to the value 1491 */ 1492 public CodeType getCodeElement() { 1493 if (this.code == null) 1494 if (Configuration.errorOnAutoCreate()) 1495 throw new Error("Attempt to auto-create ConceptReferenceComponent.code"); 1496 else if (Configuration.doAutoCreate()) 1497 this.code = new CodeType(); // bb 1498 return this.code; 1499 } 1500 1501 public boolean hasCodeElement() { 1502 return this.code != null && !this.code.isEmpty(); 1503 } 1504 1505 public boolean hasCode() { 1506 return this.code != null && !this.code.isEmpty(); 1507 } 1508 1509 /** 1510 * @param value {@link #code} (Specifies a code for the concept to be included 1511 * or excluded.). This is the underlying object with id, value and 1512 * extensions. The accessor "getCode" gives direct access to the 1513 * value 1514 */ 1515 public ConceptReferenceComponent setCodeElement(CodeType value) { 1516 this.code = value; 1517 return this; 1518 } 1519 1520 /** 1521 * @return Specifies a code for the concept to be included or excluded. 1522 */ 1523 public String getCode() { 1524 return this.code == null ? null : this.code.getValue(); 1525 } 1526 1527 /** 1528 * @param value Specifies a code for the concept to be included or excluded. 1529 */ 1530 public ConceptReferenceComponent setCode(String value) { 1531 if (this.code == null) 1532 this.code = new CodeType(); 1533 this.code.setValue(value); 1534 return this; 1535 } 1536 1537 /** 1538 * @return {@link #display} (The text to display to the user for this concept in 1539 * the context of this valueset. If no display is provided, then 1540 * applications using the value set use the display specified for the 1541 * code by the system.). This is the underlying object with id, value 1542 * and extensions. The accessor "getDisplay" gives direct access to the 1543 * value 1544 */ 1545 public StringType getDisplayElement() { 1546 if (this.display == null) 1547 if (Configuration.errorOnAutoCreate()) 1548 throw new Error("Attempt to auto-create ConceptReferenceComponent.display"); 1549 else if (Configuration.doAutoCreate()) 1550 this.display = new StringType(); // bb 1551 return this.display; 1552 } 1553 1554 public boolean hasDisplayElement() { 1555 return this.display != null && !this.display.isEmpty(); 1556 } 1557 1558 public boolean hasDisplay() { 1559 return this.display != null && !this.display.isEmpty(); 1560 } 1561 1562 /** 1563 * @param value {@link #display} (The text to display to the user for this 1564 * concept in the context of this valueset. If no display is 1565 * provided, then applications using the value set use the display 1566 * specified for the code by the system.). This is the underlying 1567 * object with id, value and extensions. The accessor "getDisplay" 1568 * gives direct access to the value 1569 */ 1570 public ConceptReferenceComponent setDisplayElement(StringType value) { 1571 this.display = value; 1572 return this; 1573 } 1574 1575 /** 1576 * @return The text to display to the user for this concept in the context of 1577 * this valueset. If no display is provided, then applications using the 1578 * value set use the display specified for the code by the system. 1579 */ 1580 public String getDisplay() { 1581 return this.display == null ? null : this.display.getValue(); 1582 } 1583 1584 /** 1585 * @param value The text to display to the user for this concept in the context 1586 * of this valueset. If no display is provided, then applications 1587 * using the value set use the display specified for the code by 1588 * the system. 1589 */ 1590 public ConceptReferenceComponent setDisplay(String value) { 1591 if (Utilities.noString(value)) 1592 this.display = null; 1593 else { 1594 if (this.display == null) 1595 this.display = new StringType(); 1596 this.display.setValue(value); 1597 } 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #designation} (Additional representations for this concept 1603 * when used in this value set - other languages, aliases, specialized 1604 * purposes, used for particular purposes, etc.) 1605 */ 1606 public List<ConceptReferenceDesignationComponent> getDesignation() { 1607 if (this.designation == null) 1608 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1609 return this.designation; 1610 } 1611 1612 /** 1613 * @return Returns a reference to <code>this</code> for easy method chaining 1614 */ 1615 public ConceptReferenceComponent setDesignation(List<ConceptReferenceDesignationComponent> theDesignation) { 1616 this.designation = theDesignation; 1617 return this; 1618 } 1619 1620 public boolean hasDesignation() { 1621 if (this.designation == null) 1622 return false; 1623 for (ConceptReferenceDesignationComponent item : this.designation) 1624 if (!item.isEmpty()) 1625 return true; 1626 return false; 1627 } 1628 1629 public ConceptReferenceDesignationComponent addDesignation() { // 3 1630 ConceptReferenceDesignationComponent t = new ConceptReferenceDesignationComponent(); 1631 if (this.designation == null) 1632 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1633 this.designation.add(t); 1634 return t; 1635 } 1636 1637 public ConceptReferenceComponent addDesignation(ConceptReferenceDesignationComponent t) { // 3 1638 if (t == null) 1639 return this; 1640 if (this.designation == null) 1641 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1642 this.designation.add(t); 1643 return this; 1644 } 1645 1646 /** 1647 * @return The first repetition of repeating field {@link #designation}, 1648 * creating it if it does not already exist 1649 */ 1650 public ConceptReferenceDesignationComponent getDesignationFirstRep() { 1651 if (getDesignation().isEmpty()) { 1652 addDesignation(); 1653 } 1654 return getDesignation().get(0); 1655 } 1656 1657 protected void listChildren(List<Property> children) { 1658 super.listChildren(children); 1659 children.add( 1660 new Property("code", "code", "Specifies a code for the concept to be included or excluded.", 0, 1, code)); 1661 children.add(new Property("display", "string", 1662 "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.", 1663 0, 1, display)); 1664 children.add(new Property("designation", "", 1665 "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.", 1666 0, java.lang.Integer.MAX_VALUE, designation)); 1667 } 1668 1669 @Override 1670 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1671 switch (_hash) { 1672 case 3059181: 1673 /* code */ return new Property("code", "code", "Specifies a code for the concept to be included or excluded.", 1674 0, 1, code); 1675 case 1671764162: 1676 /* display */ return new Property("display", "string", 1677 "The text to display to the user for this concept in the context of this valueset. If no display is provided, then applications using the value set use the display specified for the code by the system.", 1678 0, 1, display); 1679 case -900931593: 1680 /* designation */ return new Property("designation", "", 1681 "Additional representations for this concept when used in this value set - other languages, aliases, specialized purposes, used for particular purposes, etc.", 1682 0, java.lang.Integer.MAX_VALUE, designation); 1683 default: 1684 return super.getNamedProperty(_hash, _name, _checkValid); 1685 } 1686 1687 } 1688 1689 @Override 1690 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1691 switch (hash) { 1692 case 3059181: 1693 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 1694 case 1671764162: 1695 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 1696 case -900931593: 1697 /* designation */ return this.designation == null ? new Base[0] 1698 : this.designation.toArray(new Base[this.designation.size()]); // ConceptReferenceDesignationComponent 1699 default: 1700 return super.getProperty(hash, name, checkValid); 1701 } 1702 1703 } 1704 1705 @Override 1706 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1707 switch (hash) { 1708 case 3059181: // code 1709 this.code = castToCode(value); // CodeType 1710 return value; 1711 case 1671764162: // display 1712 this.display = castToString(value); // StringType 1713 return value; 1714 case -900931593: // designation 1715 this.getDesignation().add((ConceptReferenceDesignationComponent) value); // ConceptReferenceDesignationComponent 1716 return value; 1717 default: 1718 return super.setProperty(hash, name, value); 1719 } 1720 1721 } 1722 1723 @Override 1724 public Base setProperty(String name, Base value) throws FHIRException { 1725 if (name.equals("code")) { 1726 this.code = castToCode(value); // CodeType 1727 } else if (name.equals("display")) { 1728 this.display = castToString(value); // StringType 1729 } else if (name.equals("designation")) { 1730 this.getDesignation().add((ConceptReferenceDesignationComponent) value); 1731 } else 1732 return super.setProperty(name, value); 1733 return value; 1734 } 1735 1736 @Override 1737 public void removeChild(String name, Base value) throws FHIRException { 1738 if (name.equals("code")) { 1739 this.code = null; 1740 } else if (name.equals("display")) { 1741 this.display = null; 1742 } else if (name.equals("designation")) { 1743 this.getDesignation().remove((ConceptReferenceDesignationComponent) value); 1744 } else 1745 super.removeChild(name, value); 1746 1747 } 1748 1749 @Override 1750 public Base makeProperty(int hash, String name) throws FHIRException { 1751 switch (hash) { 1752 case 3059181: 1753 return getCodeElement(); 1754 case 1671764162: 1755 return getDisplayElement(); 1756 case -900931593: 1757 return addDesignation(); 1758 default: 1759 return super.makeProperty(hash, name); 1760 } 1761 1762 } 1763 1764 @Override 1765 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1766 switch (hash) { 1767 case 3059181: 1768 /* code */ return new String[] { "code" }; 1769 case 1671764162: 1770 /* display */ return new String[] { "string" }; 1771 case -900931593: 1772 /* designation */ return new String[] {}; 1773 default: 1774 return super.getTypesForProperty(hash, name); 1775 } 1776 1777 } 1778 1779 @Override 1780 public Base addChild(String name) throws FHIRException { 1781 if (name.equals("code")) { 1782 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.code"); 1783 } else if (name.equals("display")) { 1784 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.display"); 1785 } else if (name.equals("designation")) { 1786 return addDesignation(); 1787 } else 1788 return super.addChild(name); 1789 } 1790 1791 public ConceptReferenceComponent copy() { 1792 ConceptReferenceComponent dst = new ConceptReferenceComponent(); 1793 copyValues(dst); 1794 return dst; 1795 } 1796 1797 public void copyValues(ConceptReferenceComponent dst) { 1798 super.copyValues(dst); 1799 dst.code = code == null ? null : code.copy(); 1800 dst.display = display == null ? null : display.copy(); 1801 if (designation != null) { 1802 dst.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 1803 for (ConceptReferenceDesignationComponent i : designation) 1804 dst.designation.add(i.copy()); 1805 } 1806 ; 1807 } 1808 1809 @Override 1810 public boolean equalsDeep(Base other_) { 1811 if (!super.equalsDeep(other_)) 1812 return false; 1813 if (!(other_ instanceof ConceptReferenceComponent)) 1814 return false; 1815 ConceptReferenceComponent o = (ConceptReferenceComponent) other_; 1816 return compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 1817 && compareDeep(designation, o.designation, true); 1818 } 1819 1820 @Override 1821 public boolean equalsShallow(Base other_) { 1822 if (!super.equalsShallow(other_)) 1823 return false; 1824 if (!(other_ instanceof ConceptReferenceComponent)) 1825 return false; 1826 ConceptReferenceComponent o = (ConceptReferenceComponent) other_; 1827 return compareValues(code, o.code, true) && compareValues(display, o.display, true); 1828 } 1829 1830 public boolean isEmpty() { 1831 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, display, designation); 1832 } 1833 1834 public String fhirType() { 1835 return "ValueSet.compose.include.concept"; 1836 1837 } 1838 1839 } 1840 1841 @Block() 1842 public static class ConceptReferenceDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1843 /** 1844 * The language this designation is defined for. 1845 */ 1846 @Child(name = "language", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1847 @Description(shortDefinition = "Human language of the designation", formalDefinition = "The language this designation is defined for.") 1848 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 1849 protected CodeType language; 1850 1851 /** 1852 * A code that represents types of uses of designations. 1853 */ 1854 @Child(name = "use", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1855 @Description(shortDefinition = "Types of uses of designations", formalDefinition = "A code that represents types of uses of designations.") 1856 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/designation-use") 1857 protected Coding use; 1858 1859 /** 1860 * The text value for this designation. 1861 */ 1862 @Child(name = "value", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1863 @Description(shortDefinition = "The text value for this designation", formalDefinition = "The text value for this designation.") 1864 protected StringType value; 1865 1866 private static final long serialVersionUID = 1515662414L; 1867 1868 /** 1869 * Constructor 1870 */ 1871 public ConceptReferenceDesignationComponent() { 1872 super(); 1873 } 1874 1875 /** 1876 * Constructor 1877 */ 1878 public ConceptReferenceDesignationComponent(StringType value) { 1879 super(); 1880 this.value = value; 1881 } 1882 1883 /** 1884 * @return {@link #language} (The language this designation is defined for.). 1885 * This is the underlying object with id, value and extensions. The 1886 * accessor "getLanguage" gives direct access to the value 1887 */ 1888 public CodeType getLanguageElement() { 1889 if (this.language == null) 1890 if (Configuration.errorOnAutoCreate()) 1891 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.language"); 1892 else if (Configuration.doAutoCreate()) 1893 this.language = new CodeType(); // bb 1894 return this.language; 1895 } 1896 1897 public boolean hasLanguageElement() { 1898 return this.language != null && !this.language.isEmpty(); 1899 } 1900 1901 public boolean hasLanguage() { 1902 return this.language != null && !this.language.isEmpty(); 1903 } 1904 1905 /** 1906 * @param value {@link #language} (The language this designation is defined 1907 * for.). This is the underlying object with id, value and 1908 * extensions. The accessor "getLanguage" gives direct access to 1909 * the value 1910 */ 1911 public ConceptReferenceDesignationComponent setLanguageElement(CodeType value) { 1912 this.language = value; 1913 return this; 1914 } 1915 1916 /** 1917 * @return The language this designation is defined for. 1918 */ 1919 public String getLanguage() { 1920 return this.language == null ? null : this.language.getValue(); 1921 } 1922 1923 /** 1924 * @param value The language this designation is defined for. 1925 */ 1926 public ConceptReferenceDesignationComponent setLanguage(String value) { 1927 if (Utilities.noString(value)) 1928 this.language = null; 1929 else { 1930 if (this.language == null) 1931 this.language = new CodeType(); 1932 this.language.setValue(value); 1933 } 1934 return this; 1935 } 1936 1937 /** 1938 * @return {@link #use} (A code that represents types of uses of designations.) 1939 */ 1940 public Coding getUse() { 1941 if (this.use == null) 1942 if (Configuration.errorOnAutoCreate()) 1943 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.use"); 1944 else if (Configuration.doAutoCreate()) 1945 this.use = new Coding(); // cc 1946 return this.use; 1947 } 1948 1949 public boolean hasUse() { 1950 return this.use != null && !this.use.isEmpty(); 1951 } 1952 1953 /** 1954 * @param value {@link #use} (A code that represents types of uses of 1955 * designations.) 1956 */ 1957 public ConceptReferenceDesignationComponent setUse(Coding value) { 1958 this.use = value; 1959 return this; 1960 } 1961 1962 /** 1963 * @return {@link #value} (The text value for this designation.). This is the 1964 * underlying object with id, value and extensions. The accessor 1965 * "getValue" gives direct access to the value 1966 */ 1967 public StringType getValueElement() { 1968 if (this.value == null) 1969 if (Configuration.errorOnAutoCreate()) 1970 throw new Error("Attempt to auto-create ConceptReferenceDesignationComponent.value"); 1971 else if (Configuration.doAutoCreate()) 1972 this.value = new StringType(); // bb 1973 return this.value; 1974 } 1975 1976 public boolean hasValueElement() { 1977 return this.value != null && !this.value.isEmpty(); 1978 } 1979 1980 public boolean hasValue() { 1981 return this.value != null && !this.value.isEmpty(); 1982 } 1983 1984 /** 1985 * @param value {@link #value} (The text value for this designation.). This is 1986 * the underlying object with id, value and extensions. The 1987 * accessor "getValue" gives direct access to the value 1988 */ 1989 public ConceptReferenceDesignationComponent setValueElement(StringType value) { 1990 this.value = value; 1991 return this; 1992 } 1993 1994 /** 1995 * @return The text value for this designation. 1996 */ 1997 public String getValue() { 1998 return this.value == null ? null : this.value.getValue(); 1999 } 2000 2001 /** 2002 * @param value The text value for this designation. 2003 */ 2004 public ConceptReferenceDesignationComponent setValue(String value) { 2005 if (this.value == null) 2006 this.value = new StringType(); 2007 this.value.setValue(value); 2008 return this; 2009 } 2010 2011 protected void listChildren(List<Property> children) { 2012 super.listChildren(children); 2013 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 2014 children.add(new Property("use", "Coding", "A code that represents types of uses of designations.", 0, 1, use)); 2015 children.add(new Property("value", "string", "The text value for this designation.", 0, 1, value)); 2016 } 2017 2018 @Override 2019 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2020 switch (_hash) { 2021 case -1613589672: 2022 /* language */ return new Property("language", "code", "The language this designation is defined for.", 0, 1, 2023 language); 2024 case 116103: 2025 /* use */ return new Property("use", "Coding", "A code that represents types of uses of designations.", 0, 1, 2026 use); 2027 case 111972721: 2028 /* value */ return new Property("value", "string", "The text value for this designation.", 0, 1, value); 2029 default: 2030 return super.getNamedProperty(_hash, _name, _checkValid); 2031 } 2032 2033 } 2034 2035 @Override 2036 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2037 switch (hash) { 2038 case -1613589672: 2039 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeType 2040 case 116103: 2041 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Coding 2042 case 111972721: 2043 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 2044 default: 2045 return super.getProperty(hash, name, checkValid); 2046 } 2047 2048 } 2049 2050 @Override 2051 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2052 switch (hash) { 2053 case -1613589672: // language 2054 this.language = castToCode(value); // CodeType 2055 return value; 2056 case 116103: // use 2057 this.use = castToCoding(value); // Coding 2058 return value; 2059 case 111972721: // value 2060 this.value = castToString(value); // StringType 2061 return value; 2062 default: 2063 return super.setProperty(hash, name, value); 2064 } 2065 2066 } 2067 2068 @Override 2069 public Base setProperty(String name, Base value) throws FHIRException { 2070 if (name.equals("language")) { 2071 this.language = castToCode(value); // CodeType 2072 } else if (name.equals("use")) { 2073 this.use = castToCoding(value); // Coding 2074 } else if (name.equals("value")) { 2075 this.value = castToString(value); // StringType 2076 } else 2077 return super.setProperty(name, value); 2078 return value; 2079 } 2080 2081 @Override 2082 public void removeChild(String name, Base value) throws FHIRException { 2083 if (name.equals("language")) { 2084 this.language = null; 2085 } else if (name.equals("use")) { 2086 this.use = null; 2087 } else if (name.equals("value")) { 2088 this.value = null; 2089 } else 2090 super.removeChild(name, value); 2091 2092 } 2093 2094 @Override 2095 public Base makeProperty(int hash, String name) throws FHIRException { 2096 switch (hash) { 2097 case -1613589672: 2098 return getLanguageElement(); 2099 case 116103: 2100 return getUse(); 2101 case 111972721: 2102 return getValueElement(); 2103 default: 2104 return super.makeProperty(hash, name); 2105 } 2106 2107 } 2108 2109 @Override 2110 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2111 switch (hash) { 2112 case -1613589672: 2113 /* language */ return new String[] { "code" }; 2114 case 116103: 2115 /* use */ return new String[] { "Coding" }; 2116 case 111972721: 2117 /* value */ return new String[] { "string" }; 2118 default: 2119 return super.getTypesForProperty(hash, name); 2120 } 2121 2122 } 2123 2124 @Override 2125 public Base addChild(String name) throws FHIRException { 2126 if (name.equals("language")) { 2127 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.language"); 2128 } else if (name.equals("use")) { 2129 this.use = new Coding(); 2130 return this.use; 2131 } else if (name.equals("value")) { 2132 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.value"); 2133 } else 2134 return super.addChild(name); 2135 } 2136 2137 public ConceptReferenceDesignationComponent copy() { 2138 ConceptReferenceDesignationComponent dst = new ConceptReferenceDesignationComponent(); 2139 copyValues(dst); 2140 return dst; 2141 } 2142 2143 public void copyValues(ConceptReferenceDesignationComponent dst) { 2144 super.copyValues(dst); 2145 dst.language = language == null ? null : language.copy(); 2146 dst.use = use == null ? null : use.copy(); 2147 dst.value = value == null ? null : value.copy(); 2148 } 2149 2150 @Override 2151 public boolean equalsDeep(Base other_) { 2152 if (!super.equalsDeep(other_)) 2153 return false; 2154 if (!(other_ instanceof ConceptReferenceDesignationComponent)) 2155 return false; 2156 ConceptReferenceDesignationComponent o = (ConceptReferenceDesignationComponent) other_; 2157 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true) 2158 && compareDeep(value, o.value, true); 2159 } 2160 2161 @Override 2162 public boolean equalsShallow(Base other_) { 2163 if (!super.equalsShallow(other_)) 2164 return false; 2165 if (!(other_ instanceof ConceptReferenceDesignationComponent)) 2166 return false; 2167 ConceptReferenceDesignationComponent o = (ConceptReferenceDesignationComponent) other_; 2168 return compareValues(language, o.language, true) && compareValues(value, o.value, true); 2169 } 2170 2171 public boolean isEmpty() { 2172 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use, value); 2173 } 2174 2175 public String fhirType() { 2176 return "ValueSet.compose.include.concept.designation"; 2177 2178 } 2179 2180 } 2181 2182 @Block() 2183 public static class ConceptSetFilterComponent extends BackboneElement implements IBaseBackboneElement { 2184 /** 2185 * A code that identifies a property or a filter defined in the code system. 2186 */ 2187 @Child(name = "property", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2188 @Description(shortDefinition = "A property/filter defined by the code system", formalDefinition = "A code that identifies a property or a filter defined in the code system.") 2189 protected CodeType property; 2190 2191 /** 2192 * The kind of operation to perform as a part of the filter criteria. 2193 */ 2194 @Child(name = "op", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2195 @Description(shortDefinition = "= | is-a | descendent-of | is-not-a | regex | in | not-in | generalizes | exists", formalDefinition = "The kind of operation to perform as a part of the filter criteria.") 2196 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/filter-operator") 2197 protected Enumeration<FilterOperator> op; 2198 2199 /** 2200 * The match value may be either a code defined by the system, or a string 2201 * value, which is a regex match on the literal string of the property value (if 2202 * the filter represents a property defined in CodeSystem) or of the system 2203 * filter value (if the filter represents a filter defined in CodeSystem) when 2204 * the operation is 'regex', or one of the values (true and false), when the 2205 * operation is 'exists'. 2206 */ 2207 @Child(name = "value", type = { StringType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2208 @Description(shortDefinition = "Code from the system, or regex criteria, or boolean value for exists", formalDefinition = "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.") 2209 protected StringType value; 2210 2211 private static final long serialVersionUID = 1259153492L; 2212 2213 /** 2214 * Constructor 2215 */ 2216 public ConceptSetFilterComponent() { 2217 super(); 2218 } 2219 2220 /** 2221 * Constructor 2222 */ 2223 public ConceptSetFilterComponent(CodeType property, Enumeration<FilterOperator> op, StringType value) { 2224 super(); 2225 this.property = property; 2226 this.op = op; 2227 this.value = value; 2228 } 2229 2230 /** 2231 * @return {@link #property} (A code that identifies a property or a filter 2232 * defined in the code system.). This is the underlying object with id, 2233 * value and extensions. The accessor "getProperty" gives direct access 2234 * to the value 2235 */ 2236 public CodeType getPropertyElement() { 2237 if (this.property == null) 2238 if (Configuration.errorOnAutoCreate()) 2239 throw new Error("Attempt to auto-create ConceptSetFilterComponent.property"); 2240 else if (Configuration.doAutoCreate()) 2241 this.property = new CodeType(); // bb 2242 return this.property; 2243 } 2244 2245 public boolean hasPropertyElement() { 2246 return this.property != null && !this.property.isEmpty(); 2247 } 2248 2249 public boolean hasProperty() { 2250 return this.property != null && !this.property.isEmpty(); 2251 } 2252 2253 /** 2254 * @param value {@link #property} (A code that identifies a property or a filter 2255 * defined in the code system.). This is the underlying object with 2256 * id, value and extensions. The accessor "getProperty" gives 2257 * direct access to the value 2258 */ 2259 public ConceptSetFilterComponent setPropertyElement(CodeType value) { 2260 this.property = value; 2261 return this; 2262 } 2263 2264 /** 2265 * @return A code that identifies a property or a filter defined in the code 2266 * system. 2267 */ 2268 public String getProperty() { 2269 return this.property == null ? null : this.property.getValue(); 2270 } 2271 2272 /** 2273 * @param value A code that identifies a property or a filter defined in the 2274 * code system. 2275 */ 2276 public ConceptSetFilterComponent setProperty(String value) { 2277 if (this.property == null) 2278 this.property = new CodeType(); 2279 this.property.setValue(value); 2280 return this; 2281 } 2282 2283 /** 2284 * @return {@link #op} (The kind of operation to perform as a part of the filter 2285 * criteria.). This is the underlying object with id, value and 2286 * extensions. The accessor "getOp" gives direct access to the value 2287 */ 2288 public Enumeration<FilterOperator> getOpElement() { 2289 if (this.op == null) 2290 if (Configuration.errorOnAutoCreate()) 2291 throw new Error("Attempt to auto-create ConceptSetFilterComponent.op"); 2292 else if (Configuration.doAutoCreate()) 2293 this.op = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); // bb 2294 return this.op; 2295 } 2296 2297 public boolean hasOpElement() { 2298 return this.op != null && !this.op.isEmpty(); 2299 } 2300 2301 public boolean hasOp() { 2302 return this.op != null && !this.op.isEmpty(); 2303 } 2304 2305 /** 2306 * @param value {@link #op} (The kind of operation to perform as a part of the 2307 * filter criteria.). This is the underlying object with id, value 2308 * and extensions. The accessor "getOp" gives direct access to the 2309 * value 2310 */ 2311 public ConceptSetFilterComponent setOpElement(Enumeration<FilterOperator> value) { 2312 this.op = value; 2313 return this; 2314 } 2315 2316 /** 2317 * @return The kind of operation to perform as a part of the filter criteria. 2318 */ 2319 public FilterOperator getOp() { 2320 return this.op == null ? null : this.op.getValue(); 2321 } 2322 2323 /** 2324 * @param value The kind of operation to perform as a part of the filter 2325 * criteria. 2326 */ 2327 public ConceptSetFilterComponent setOp(FilterOperator value) { 2328 if (this.op == null) 2329 this.op = new Enumeration<FilterOperator>(new FilterOperatorEnumFactory()); 2330 this.op.setValue(value); 2331 return this; 2332 } 2333 2334 /** 2335 * @return {@link #value} (The match value may be either a code defined by the 2336 * system, or a string value, which is a regex match on the literal 2337 * string of the property value (if the filter represents a property 2338 * defined in CodeSystem) or of the system filter value (if the filter 2339 * represents a filter defined in CodeSystem) when the operation is 2340 * 'regex', or one of the values (true and false), when the operation is 2341 * 'exists'.). This is the underlying object with id, value and 2342 * extensions. The accessor "getValue" gives direct access to the value 2343 */ 2344 public StringType getValueElement() { 2345 if (this.value == null) 2346 if (Configuration.errorOnAutoCreate()) 2347 throw new Error("Attempt to auto-create ConceptSetFilterComponent.value"); 2348 else if (Configuration.doAutoCreate()) 2349 this.value = new StringType(); // bb 2350 return this.value; 2351 } 2352 2353 public boolean hasValueElement() { 2354 return this.value != null && !this.value.isEmpty(); 2355 } 2356 2357 public boolean hasValue() { 2358 return this.value != null && !this.value.isEmpty(); 2359 } 2360 2361 /** 2362 * @param value {@link #value} (The match value may be either a code defined by 2363 * the system, or a string value, which is a regex match on the 2364 * literal string of the property value (if the filter represents a 2365 * property defined in CodeSystem) or of the system filter value 2366 * (if the filter represents a filter defined in CodeSystem) when 2367 * the operation is 'regex', or one of the values (true and false), 2368 * when the operation is 'exists'.). This is the underlying object 2369 * with id, value and extensions. The accessor "getValue" gives 2370 * direct access to the value 2371 */ 2372 public ConceptSetFilterComponent setValueElement(StringType value) { 2373 this.value = value; 2374 return this; 2375 } 2376 2377 /** 2378 * @return The match value may be either a code defined by the system, or a 2379 * string value, which is a regex match on the literal string of the 2380 * property value (if the filter represents a property defined in 2381 * CodeSystem) or of the system filter value (if the filter represents a 2382 * filter defined in CodeSystem) when the operation is 'regex', or one 2383 * of the values (true and false), when the operation is 'exists'. 2384 */ 2385 public String getValue() { 2386 return this.value == null ? null : this.value.getValue(); 2387 } 2388 2389 /** 2390 * @param value The match value may be either a code defined by the system, or a 2391 * string value, which is a regex match on the literal string of 2392 * the property value (if the filter represents a property defined 2393 * in CodeSystem) or of the system filter value (if the filter 2394 * represents a filter defined in CodeSystem) when the operation is 2395 * 'regex', or one of the values (true and false), when the 2396 * operation is 'exists'. 2397 */ 2398 public ConceptSetFilterComponent setValue(String value) { 2399 if (this.value == null) 2400 this.value = new StringType(); 2401 this.value.setValue(value); 2402 return this; 2403 } 2404 2405 protected void listChildren(List<Property> children) { 2406 super.listChildren(children); 2407 children.add(new Property("property", "code", 2408 "A code that identifies a property or a filter defined in the code system.", 0, 1, property)); 2409 children.add( 2410 new Property("op", "code", "The kind of operation to perform as a part of the filter criteria.", 0, 1, op)); 2411 children.add(new Property("value", "string", 2412 "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.", 2413 0, 1, value)); 2414 } 2415 2416 @Override 2417 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2418 switch (_hash) { 2419 case -993141291: 2420 /* property */ return new Property("property", "code", 2421 "A code that identifies a property or a filter defined in the code system.", 0, 1, property); 2422 case 3553: 2423 /* op */ return new Property("op", "code", "The kind of operation to perform as a part of the filter criteria.", 2424 0, 1, op); 2425 case 111972721: 2426 /* value */ return new Property("value", "string", 2427 "The match value may be either a code defined by the system, or a string value, which is a regex match on the literal string of the property value (if the filter represents a property defined in CodeSystem) or of the system filter value (if the filter represents a filter defined in CodeSystem) when the operation is 'regex', or one of the values (true and false), when the operation is 'exists'.", 2428 0, 1, value); 2429 default: 2430 return super.getNamedProperty(_hash, _name, _checkValid); 2431 } 2432 2433 } 2434 2435 @Override 2436 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2437 switch (hash) { 2438 case -993141291: 2439 /* property */ return this.property == null ? new Base[0] : new Base[] { this.property }; // CodeType 2440 case 3553: 2441 /* op */ return this.op == null ? new Base[0] : new Base[] { this.op }; // Enumeration<FilterOperator> 2442 case 111972721: 2443 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 2444 default: 2445 return super.getProperty(hash, name, checkValid); 2446 } 2447 2448 } 2449 2450 @Override 2451 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2452 switch (hash) { 2453 case -993141291: // property 2454 this.property = castToCode(value); // CodeType 2455 return value; 2456 case 3553: // op 2457 value = new FilterOperatorEnumFactory().fromType(castToCode(value)); 2458 this.op = (Enumeration) value; // Enumeration<FilterOperator> 2459 return value; 2460 case 111972721: // value 2461 this.value = castToString(value); // StringType 2462 return value; 2463 default: 2464 return super.setProperty(hash, name, value); 2465 } 2466 2467 } 2468 2469 @Override 2470 public Base setProperty(String name, Base value) throws FHIRException { 2471 if (name.equals("property")) { 2472 this.property = castToCode(value); // CodeType 2473 } else if (name.equals("op")) { 2474 value = new FilterOperatorEnumFactory().fromType(castToCode(value)); 2475 this.op = (Enumeration) value; // Enumeration<FilterOperator> 2476 } else if (name.equals("value")) { 2477 this.value = castToString(value); // StringType 2478 } else 2479 return super.setProperty(name, value); 2480 return value; 2481 } 2482 2483 @Override 2484 public void removeChild(String name, Base value) throws FHIRException { 2485 if (name.equals("property")) { 2486 this.property = null; 2487 } else if (name.equals("op")) { 2488 this.op = null; 2489 } else if (name.equals("value")) { 2490 this.value = null; 2491 } else 2492 super.removeChild(name, value); 2493 2494 } 2495 2496 @Override 2497 public Base makeProperty(int hash, String name) throws FHIRException { 2498 switch (hash) { 2499 case -993141291: 2500 return getPropertyElement(); 2501 case 3553: 2502 return getOpElement(); 2503 case 111972721: 2504 return getValueElement(); 2505 default: 2506 return super.makeProperty(hash, name); 2507 } 2508 2509 } 2510 2511 @Override 2512 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2513 switch (hash) { 2514 case -993141291: 2515 /* property */ return new String[] { "code" }; 2516 case 3553: 2517 /* op */ return new String[] { "code" }; 2518 case 111972721: 2519 /* value */ return new String[] { "string" }; 2520 default: 2521 return super.getTypesForProperty(hash, name); 2522 } 2523 2524 } 2525 2526 @Override 2527 public Base addChild(String name) throws FHIRException { 2528 if (name.equals("property")) { 2529 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.property"); 2530 } else if (name.equals("op")) { 2531 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.op"); 2532 } else if (name.equals("value")) { 2533 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.value"); 2534 } else 2535 return super.addChild(name); 2536 } 2537 2538 public ConceptSetFilterComponent copy() { 2539 ConceptSetFilterComponent dst = new ConceptSetFilterComponent(); 2540 copyValues(dst); 2541 return dst; 2542 } 2543 2544 public void copyValues(ConceptSetFilterComponent dst) { 2545 super.copyValues(dst); 2546 dst.property = property == null ? null : property.copy(); 2547 dst.op = op == null ? null : op.copy(); 2548 dst.value = value == null ? null : value.copy(); 2549 } 2550 2551 @Override 2552 public boolean equalsDeep(Base other_) { 2553 if (!super.equalsDeep(other_)) 2554 return false; 2555 if (!(other_ instanceof ConceptSetFilterComponent)) 2556 return false; 2557 ConceptSetFilterComponent o = (ConceptSetFilterComponent) other_; 2558 return compareDeep(property, o.property, true) && compareDeep(op, o.op, true) 2559 && compareDeep(value, o.value, true); 2560 } 2561 2562 @Override 2563 public boolean equalsShallow(Base other_) { 2564 if (!super.equalsShallow(other_)) 2565 return false; 2566 if (!(other_ instanceof ConceptSetFilterComponent)) 2567 return false; 2568 ConceptSetFilterComponent o = (ConceptSetFilterComponent) other_; 2569 return compareValues(property, o.property, true) && compareValues(op, o.op, true) 2570 && compareValues(value, o.value, true); 2571 } 2572 2573 public boolean isEmpty() { 2574 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(property, op, value); 2575 } 2576 2577 public String fhirType() { 2578 return "ValueSet.compose.include.filter"; 2579 2580 } 2581 2582 } 2583 2584 @Block() 2585 public static class ValueSetExpansionComponent extends BackboneElement implements IBaseBackboneElement { 2586 /** 2587 * An identifier that uniquely identifies this expansion of the valueset, based 2588 * on a unique combination of the provided parameters, the system default 2589 * parameters, and the underlying system code system versions etc. Systems may 2590 * re-use the same identifier as long as those factors remain the same, and the 2591 * expansion is the same, but are not required to do so. This is a business 2592 * identifier. 2593 */ 2594 @Child(name = "identifier", type = { 2595 UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2596 @Description(shortDefinition = "Identifies the value set expansion (business identifier)", formalDefinition = "An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier.") 2597 protected UriType identifier; 2598 2599 /** 2600 * The time at which the expansion was produced by the expanding system. 2601 */ 2602 @Child(name = "timestamp", type = { 2603 DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2604 @Description(shortDefinition = "Time ValueSet expansion happened", formalDefinition = "The time at which the expansion was produced by the expanding system.") 2605 protected DateTimeType timestamp; 2606 2607 /** 2608 * The total number of concepts in the expansion. If the number of concept nodes 2609 * in this resource is less than the stated number, then the server can return 2610 * more using the offset parameter. 2611 */ 2612 @Child(name = "total", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2613 @Description(shortDefinition = "Total number of codes in the expansion", formalDefinition = "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.") 2614 protected IntegerType total; 2615 2616 /** 2617 * If paging is being used, the offset at which this resource starts. I.e. this 2618 * resource is a partial view into the expansion. If paging is not being used, 2619 * this element SHALL NOT be present. 2620 */ 2621 @Child(name = "offset", type = { 2622 IntegerType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2623 @Description(shortDefinition = "Offset at which this resource starts", formalDefinition = "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present.") 2624 protected IntegerType offset; 2625 2626 /** 2627 * A parameter that controlled the expansion process. These parameters may be 2628 * used by users of expanded value sets to check whether the expansion is 2629 * suitable for a particular purpose, or to pick the correct expansion. 2630 */ 2631 @Child(name = "parameter", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2632 @Description(shortDefinition = "Parameter that controlled the expansion process", formalDefinition = "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.") 2633 protected List<ValueSetExpansionParameterComponent> parameter; 2634 2635 /** 2636 * The codes that are contained in the value set expansion. 2637 */ 2638 @Child(name = "contains", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2639 @Description(shortDefinition = "Codes in the value set", formalDefinition = "The codes that are contained in the value set expansion.") 2640 protected List<ValueSetExpansionContainsComponent> contains; 2641 2642 private static final long serialVersionUID = -43471993L; 2643 2644 /** 2645 * Constructor 2646 */ 2647 public ValueSetExpansionComponent() { 2648 super(); 2649 } 2650 2651 /** 2652 * Constructor 2653 */ 2654 public ValueSetExpansionComponent(DateTimeType timestamp) { 2655 super(); 2656 this.timestamp = timestamp; 2657 } 2658 2659 /** 2660 * @return {@link #identifier} (An identifier that uniquely identifies this 2661 * expansion of the valueset, based on a unique combination of the 2662 * provided parameters, the system default parameters, and the 2663 * underlying system code system versions etc. Systems may re-use the 2664 * same identifier as long as those factors remain the same, and the 2665 * expansion is the same, but are not required to do so. This is a 2666 * business identifier.). This is the underlying object with id, value 2667 * and extensions. The accessor "getIdentifier" gives direct access to 2668 * the value 2669 */ 2670 public UriType getIdentifierElement() { 2671 if (this.identifier == null) 2672 if (Configuration.errorOnAutoCreate()) 2673 throw new Error("Attempt to auto-create ValueSetExpansionComponent.identifier"); 2674 else if (Configuration.doAutoCreate()) 2675 this.identifier = new UriType(); // bb 2676 return this.identifier; 2677 } 2678 2679 public boolean hasIdentifierElement() { 2680 return this.identifier != null && !this.identifier.isEmpty(); 2681 } 2682 2683 public boolean hasIdentifier() { 2684 return this.identifier != null && !this.identifier.isEmpty(); 2685 } 2686 2687 /** 2688 * @param value {@link #identifier} (An identifier that uniquely identifies this 2689 * expansion of the valueset, based on a unique combination of the 2690 * provided parameters, the system default parameters, and the 2691 * underlying system code system versions etc. Systems may re-use 2692 * the same identifier as long as those factors remain the same, 2693 * and the expansion is the same, but are not required to do so. 2694 * This is a business identifier.). This is the underlying object 2695 * with id, value and extensions. The accessor "getIdentifier" 2696 * gives direct access to the value 2697 */ 2698 public ValueSetExpansionComponent setIdentifierElement(UriType value) { 2699 this.identifier = value; 2700 return this; 2701 } 2702 2703 /** 2704 * @return An identifier that uniquely identifies this expansion of the 2705 * valueset, based on a unique combination of the provided parameters, 2706 * the system default parameters, and the underlying system code system 2707 * versions etc. Systems may re-use the same identifier as long as those 2708 * factors remain the same, and the expansion is the same, but are not 2709 * required to do so. This is a business identifier. 2710 */ 2711 public String getIdentifier() { 2712 return this.identifier == null ? null : this.identifier.getValue(); 2713 } 2714 2715 /** 2716 * @param value An identifier that uniquely identifies this expansion of the 2717 * valueset, based on a unique combination of the provided 2718 * parameters, the system default parameters, and the underlying 2719 * system code system versions etc. Systems may re-use the same 2720 * identifier as long as those factors remain the same, and the 2721 * expansion is the same, but are not required to do so. This is a 2722 * business identifier. 2723 */ 2724 public ValueSetExpansionComponent setIdentifier(String value) { 2725 if (Utilities.noString(value)) 2726 this.identifier = null; 2727 else { 2728 if (this.identifier == null) 2729 this.identifier = new UriType(); 2730 this.identifier.setValue(value); 2731 } 2732 return this; 2733 } 2734 2735 /** 2736 * @return {@link #timestamp} (The time at which the expansion was produced by 2737 * the expanding system.). This is the underlying object with id, value 2738 * and extensions. The accessor "getTimestamp" gives direct access to 2739 * the value 2740 */ 2741 public DateTimeType getTimestampElement() { 2742 if (this.timestamp == null) 2743 if (Configuration.errorOnAutoCreate()) 2744 throw new Error("Attempt to auto-create ValueSetExpansionComponent.timestamp"); 2745 else if (Configuration.doAutoCreate()) 2746 this.timestamp = new DateTimeType(); // bb 2747 return this.timestamp; 2748 } 2749 2750 public boolean hasTimestampElement() { 2751 return this.timestamp != null && !this.timestamp.isEmpty(); 2752 } 2753 2754 public boolean hasTimestamp() { 2755 return this.timestamp != null && !this.timestamp.isEmpty(); 2756 } 2757 2758 /** 2759 * @param value {@link #timestamp} (The time at which the expansion was produced 2760 * by the expanding system.). This is the underlying object with 2761 * id, value and extensions. The accessor "getTimestamp" gives 2762 * direct access to the value 2763 */ 2764 public ValueSetExpansionComponent setTimestampElement(DateTimeType value) { 2765 this.timestamp = value; 2766 return this; 2767 } 2768 2769 /** 2770 * @return The time at which the expansion was produced by the expanding system. 2771 */ 2772 public Date getTimestamp() { 2773 return this.timestamp == null ? null : this.timestamp.getValue(); 2774 } 2775 2776 /** 2777 * @param value The time at which the expansion was produced by the expanding 2778 * system. 2779 */ 2780 public ValueSetExpansionComponent setTimestamp(Date value) { 2781 if (this.timestamp == null) 2782 this.timestamp = new DateTimeType(); 2783 this.timestamp.setValue(value); 2784 return this; 2785 } 2786 2787 /** 2788 * @return {@link #total} (The total number of concepts in the expansion. If the 2789 * number of concept nodes in this resource is less than the stated 2790 * number, then the server can return more using the offset parameter.). 2791 * This is the underlying object with id, value and extensions. The 2792 * accessor "getTotal" gives direct access to the value 2793 */ 2794 public IntegerType getTotalElement() { 2795 if (this.total == null) 2796 if (Configuration.errorOnAutoCreate()) 2797 throw new Error("Attempt to auto-create ValueSetExpansionComponent.total"); 2798 else if (Configuration.doAutoCreate()) 2799 this.total = new IntegerType(); // bb 2800 return this.total; 2801 } 2802 2803 public boolean hasTotalElement() { 2804 return this.total != null && !this.total.isEmpty(); 2805 } 2806 2807 public boolean hasTotal() { 2808 return this.total != null && !this.total.isEmpty(); 2809 } 2810 2811 /** 2812 * @param value {@link #total} (The total number of concepts in the expansion. 2813 * If the number of concept nodes in this resource is less than the 2814 * stated number, then the server can return more using the offset 2815 * parameter.). This is the underlying object with id, value and 2816 * extensions. The accessor "getTotal" gives direct access to the 2817 * value 2818 */ 2819 public ValueSetExpansionComponent setTotalElement(IntegerType value) { 2820 this.total = value; 2821 return this; 2822 } 2823 2824 /** 2825 * @return The total number of concepts in the expansion. If the number of 2826 * concept nodes in this resource is less than the stated number, then 2827 * the server can return more using the offset parameter. 2828 */ 2829 public int getTotal() { 2830 return this.total == null || this.total.isEmpty() ? 0 : this.total.getValue(); 2831 } 2832 2833 /** 2834 * @param value The total number of concepts in the expansion. If the number of 2835 * concept nodes in this resource is less than the stated number, 2836 * then the server can return more using the offset parameter. 2837 */ 2838 public ValueSetExpansionComponent setTotal(int value) { 2839 if (this.total == null) 2840 this.total = new IntegerType(); 2841 this.total.setValue(value); 2842 return this; 2843 } 2844 2845 /** 2846 * @return {@link #offset} (If paging is being used, the offset at which this 2847 * resource starts. I.e. this resource is a partial view into the 2848 * expansion. If paging is not being used, this element SHALL NOT be 2849 * present.). This is the underlying object with id, value and 2850 * extensions. The accessor "getOffset" gives direct access to the value 2851 */ 2852 public IntegerType getOffsetElement() { 2853 if (this.offset == null) 2854 if (Configuration.errorOnAutoCreate()) 2855 throw new Error("Attempt to auto-create ValueSetExpansionComponent.offset"); 2856 else if (Configuration.doAutoCreate()) 2857 this.offset = new IntegerType(); // bb 2858 return this.offset; 2859 } 2860 2861 public boolean hasOffsetElement() { 2862 return this.offset != null && !this.offset.isEmpty(); 2863 } 2864 2865 public boolean hasOffset() { 2866 return this.offset != null && !this.offset.isEmpty(); 2867 } 2868 2869 /** 2870 * @param value {@link #offset} (If paging is being used, the offset at which 2871 * this resource starts. I.e. this resource is a partial view into 2872 * the expansion. If paging is not being used, this element SHALL 2873 * NOT be present.). This is the underlying object with id, value 2874 * and extensions. The accessor "getOffset" gives direct access to 2875 * the value 2876 */ 2877 public ValueSetExpansionComponent setOffsetElement(IntegerType value) { 2878 this.offset = value; 2879 return this; 2880 } 2881 2882 /** 2883 * @return If paging is being used, the offset at which this resource starts. 2884 * I.e. this resource is a partial view into the expansion. If paging is 2885 * not being used, this element SHALL NOT be present. 2886 */ 2887 public int getOffset() { 2888 return this.offset == null || this.offset.isEmpty() ? 0 : this.offset.getValue(); 2889 } 2890 2891 /** 2892 * @param value If paging is being used, the offset at which this resource 2893 * starts. I.e. this resource is a partial view into the expansion. 2894 * If paging is not being used, this element SHALL NOT be present. 2895 */ 2896 public ValueSetExpansionComponent setOffset(int value) { 2897 if (this.offset == null) 2898 this.offset = new IntegerType(); 2899 this.offset.setValue(value); 2900 return this; 2901 } 2902 2903 /** 2904 * @return {@link #parameter} (A parameter that controlled the expansion 2905 * process. These parameters may be used by users of expanded value sets 2906 * to check whether the expansion is suitable for a particular purpose, 2907 * or to pick the correct expansion.) 2908 */ 2909 public List<ValueSetExpansionParameterComponent> getParameter() { 2910 if (this.parameter == null) 2911 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2912 return this.parameter; 2913 } 2914 2915 /** 2916 * @return Returns a reference to <code>this</code> for easy method chaining 2917 */ 2918 public ValueSetExpansionComponent setParameter(List<ValueSetExpansionParameterComponent> theParameter) { 2919 this.parameter = theParameter; 2920 return this; 2921 } 2922 2923 public boolean hasParameter() { 2924 if (this.parameter == null) 2925 return false; 2926 for (ValueSetExpansionParameterComponent item : this.parameter) 2927 if (!item.isEmpty()) 2928 return true; 2929 return false; 2930 } 2931 2932 public ValueSetExpansionParameterComponent addParameter() { // 3 2933 ValueSetExpansionParameterComponent t = new ValueSetExpansionParameterComponent(); 2934 if (this.parameter == null) 2935 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2936 this.parameter.add(t); 2937 return t; 2938 } 2939 2940 public ValueSetExpansionComponent addParameter(ValueSetExpansionParameterComponent t) { // 3 2941 if (t == null) 2942 return this; 2943 if (this.parameter == null) 2944 this.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 2945 this.parameter.add(t); 2946 return this; 2947 } 2948 2949 /** 2950 * @return The first repetition of repeating field {@link #parameter}, creating 2951 * it if it does not already exist 2952 */ 2953 public ValueSetExpansionParameterComponent getParameterFirstRep() { 2954 if (getParameter().isEmpty()) { 2955 addParameter(); 2956 } 2957 return getParameter().get(0); 2958 } 2959 2960 /** 2961 * @return {@link #contains} (The codes that are contained in the value set 2962 * expansion.) 2963 */ 2964 public List<ValueSetExpansionContainsComponent> getContains() { 2965 if (this.contains == null) 2966 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2967 return this.contains; 2968 } 2969 2970 /** 2971 * @return Returns a reference to <code>this</code> for easy method chaining 2972 */ 2973 public ValueSetExpansionComponent setContains(List<ValueSetExpansionContainsComponent> theContains) { 2974 this.contains = theContains; 2975 return this; 2976 } 2977 2978 public boolean hasContains() { 2979 if (this.contains == null) 2980 return false; 2981 for (ValueSetExpansionContainsComponent item : this.contains) 2982 if (!item.isEmpty()) 2983 return true; 2984 return false; 2985 } 2986 2987 public ValueSetExpansionContainsComponent addContains() { // 3 2988 ValueSetExpansionContainsComponent t = new ValueSetExpansionContainsComponent(); 2989 if (this.contains == null) 2990 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 2991 this.contains.add(t); 2992 return t; 2993 } 2994 2995 public ValueSetExpansionComponent addContains(ValueSetExpansionContainsComponent t) { // 3 2996 if (t == null) 2997 return this; 2998 if (this.contains == null) 2999 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3000 this.contains.add(t); 3001 return this; 3002 } 3003 3004 /** 3005 * @return The first repetition of repeating field {@link #contains}, creating 3006 * it if it does not already exist 3007 */ 3008 public ValueSetExpansionContainsComponent getContainsFirstRep() { 3009 if (getContains().isEmpty()) { 3010 addContains(); 3011 } 3012 return getContains().get(0); 3013 } 3014 3015 protected void listChildren(List<Property> children) { 3016 super.listChildren(children); 3017 children.add(new Property("identifier", "uri", 3018 "An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier.", 3019 0, 1, identifier)); 3020 children.add(new Property("timestamp", "dateTime", 3021 "The time at which the expansion was produced by the expanding system.", 0, 1, timestamp)); 3022 children.add(new Property("total", "integer", 3023 "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.", 3024 0, 1, total)); 3025 children.add(new Property("offset", "integer", 3026 "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present.", 3027 0, 1, offset)); 3028 children.add(new Property("parameter", "", 3029 "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.", 3030 0, java.lang.Integer.MAX_VALUE, parameter)); 3031 children.add(new Property("contains", "", "The codes that are contained in the value set expansion.", 0, 3032 java.lang.Integer.MAX_VALUE, contains)); 3033 } 3034 3035 @Override 3036 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3037 switch (_hash) { 3038 case -1618432855: 3039 /* identifier */ return new Property("identifier", "uri", 3040 "An identifier that uniquely identifies this expansion of the valueset, based on a unique combination of the provided parameters, the system default parameters, and the underlying system code system versions etc. Systems may re-use the same identifier as long as those factors remain the same, and the expansion is the same, but are not required to do so. This is a business identifier.", 3041 0, 1, identifier); 3042 case 55126294: 3043 /* timestamp */ return new Property("timestamp", "dateTime", 3044 "The time at which the expansion was produced by the expanding system.", 0, 1, timestamp); 3045 case 110549828: 3046 /* total */ return new Property("total", "integer", 3047 "The total number of concepts in the expansion. If the number of concept nodes in this resource is less than the stated number, then the server can return more using the offset parameter.", 3048 0, 1, total); 3049 case -1019779949: 3050 /* offset */ return new Property("offset", "integer", 3051 "If paging is being used, the offset at which this resource starts. I.e. this resource is a partial view into the expansion. If paging is not being used, this element SHALL NOT be present.", 3052 0, 1, offset); 3053 case 1954460585: 3054 /* parameter */ return new Property("parameter", "", 3055 "A parameter that controlled the expansion process. These parameters may be used by users of expanded value sets to check whether the expansion is suitable for a particular purpose, or to pick the correct expansion.", 3056 0, java.lang.Integer.MAX_VALUE, parameter); 3057 case -567445985: 3058 /* contains */ return new Property("contains", "", "The codes that are contained in the value set expansion.", 3059 0, java.lang.Integer.MAX_VALUE, contains); 3060 default: 3061 return super.getNamedProperty(_hash, _name, _checkValid); 3062 } 3063 3064 } 3065 3066 @Override 3067 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3068 switch (hash) { 3069 case -1618432855: 3070 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // UriType 3071 case 55126294: 3072 /* timestamp */ return this.timestamp == null ? new Base[0] : new Base[] { this.timestamp }; // DateTimeType 3073 case 110549828: 3074 /* total */ return this.total == null ? new Base[0] : new Base[] { this.total }; // IntegerType 3075 case -1019779949: 3076 /* offset */ return this.offset == null ? new Base[0] : new Base[] { this.offset }; // IntegerType 3077 case 1954460585: 3078 /* parameter */ return this.parameter == null ? new Base[0] 3079 : this.parameter.toArray(new Base[this.parameter.size()]); // ValueSetExpansionParameterComponent 3080 case -567445985: 3081 /* contains */ return this.contains == null ? new Base[0] 3082 : this.contains.toArray(new Base[this.contains.size()]); // ValueSetExpansionContainsComponent 3083 default: 3084 return super.getProperty(hash, name, checkValid); 3085 } 3086 3087 } 3088 3089 @Override 3090 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3091 switch (hash) { 3092 case -1618432855: // identifier 3093 this.identifier = castToUri(value); // UriType 3094 return value; 3095 case 55126294: // timestamp 3096 this.timestamp = castToDateTime(value); // DateTimeType 3097 return value; 3098 case 110549828: // total 3099 this.total = castToInteger(value); // IntegerType 3100 return value; 3101 case -1019779949: // offset 3102 this.offset = castToInteger(value); // IntegerType 3103 return value; 3104 case 1954460585: // parameter 3105 this.getParameter().add((ValueSetExpansionParameterComponent) value); // ValueSetExpansionParameterComponent 3106 return value; 3107 case -567445985: // contains 3108 this.getContains().add((ValueSetExpansionContainsComponent) value); // ValueSetExpansionContainsComponent 3109 return value; 3110 default: 3111 return super.setProperty(hash, name, value); 3112 } 3113 3114 } 3115 3116 @Override 3117 public Base setProperty(String name, Base value) throws FHIRException { 3118 if (name.equals("identifier")) { 3119 this.identifier = castToUri(value); // UriType 3120 } else if (name.equals("timestamp")) { 3121 this.timestamp = castToDateTime(value); // DateTimeType 3122 } else if (name.equals("total")) { 3123 this.total = castToInteger(value); // IntegerType 3124 } else if (name.equals("offset")) { 3125 this.offset = castToInteger(value); // IntegerType 3126 } else if (name.equals("parameter")) { 3127 this.getParameter().add((ValueSetExpansionParameterComponent) value); 3128 } else if (name.equals("contains")) { 3129 this.getContains().add((ValueSetExpansionContainsComponent) value); 3130 } else 3131 return super.setProperty(name, value); 3132 return value; 3133 } 3134 3135 @Override 3136 public void removeChild(String name, Base value) throws FHIRException { 3137 if (name.equals("identifier")) { 3138 this.identifier = null; 3139 } else if (name.equals("timestamp")) { 3140 this.timestamp = null; 3141 } else if (name.equals("total")) { 3142 this.total = null; 3143 } else if (name.equals("offset")) { 3144 this.offset = null; 3145 } else if (name.equals("parameter")) { 3146 this.getParameter().remove((ValueSetExpansionParameterComponent) value); 3147 } else if (name.equals("contains")) { 3148 this.getContains().remove((ValueSetExpansionContainsComponent) value); 3149 } else 3150 super.removeChild(name, value); 3151 3152 } 3153 3154 @Override 3155 public Base makeProperty(int hash, String name) throws FHIRException { 3156 switch (hash) { 3157 case -1618432855: 3158 return getIdentifierElement(); 3159 case 55126294: 3160 return getTimestampElement(); 3161 case 110549828: 3162 return getTotalElement(); 3163 case -1019779949: 3164 return getOffsetElement(); 3165 case 1954460585: 3166 return addParameter(); 3167 case -567445985: 3168 return addContains(); 3169 default: 3170 return super.makeProperty(hash, name); 3171 } 3172 3173 } 3174 3175 @Override 3176 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3177 switch (hash) { 3178 case -1618432855: 3179 /* identifier */ return new String[] { "uri" }; 3180 case 55126294: 3181 /* timestamp */ return new String[] { "dateTime" }; 3182 case 110549828: 3183 /* total */ return new String[] { "integer" }; 3184 case -1019779949: 3185 /* offset */ return new String[] { "integer" }; 3186 case 1954460585: 3187 /* parameter */ return new String[] {}; 3188 case -567445985: 3189 /* contains */ return new String[] {}; 3190 default: 3191 return super.getTypesForProperty(hash, name); 3192 } 3193 3194 } 3195 3196 @Override 3197 public Base addChild(String name) throws FHIRException { 3198 if (name.equals("identifier")) { 3199 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.identifier"); 3200 } else if (name.equals("timestamp")) { 3201 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.timestamp"); 3202 } else if (name.equals("total")) { 3203 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.total"); 3204 } else if (name.equals("offset")) { 3205 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.offset"); 3206 } else if (name.equals("parameter")) { 3207 return addParameter(); 3208 } else if (name.equals("contains")) { 3209 return addContains(); 3210 } else 3211 return super.addChild(name); 3212 } 3213 3214 public ValueSetExpansionComponent copy() { 3215 ValueSetExpansionComponent dst = new ValueSetExpansionComponent(); 3216 copyValues(dst); 3217 return dst; 3218 } 3219 3220 public void copyValues(ValueSetExpansionComponent dst) { 3221 super.copyValues(dst); 3222 dst.identifier = identifier == null ? null : identifier.copy(); 3223 dst.timestamp = timestamp == null ? null : timestamp.copy(); 3224 dst.total = total == null ? null : total.copy(); 3225 dst.offset = offset == null ? null : offset.copy(); 3226 if (parameter != null) { 3227 dst.parameter = new ArrayList<ValueSetExpansionParameterComponent>(); 3228 for (ValueSetExpansionParameterComponent i : parameter) 3229 dst.parameter.add(i.copy()); 3230 } 3231 ; 3232 if (contains != null) { 3233 dst.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 3234 for (ValueSetExpansionContainsComponent i : contains) 3235 dst.contains.add(i.copy()); 3236 } 3237 ; 3238 } 3239 3240 @Override 3241 public boolean equalsDeep(Base other_) { 3242 if (!super.equalsDeep(other_)) 3243 return false; 3244 if (!(other_ instanceof ValueSetExpansionComponent)) 3245 return false; 3246 ValueSetExpansionComponent o = (ValueSetExpansionComponent) other_; 3247 return compareDeep(identifier, o.identifier, true) && compareDeep(timestamp, o.timestamp, true) 3248 && compareDeep(total, o.total, true) && compareDeep(offset, o.offset, true) 3249 && compareDeep(parameter, o.parameter, true) && compareDeep(contains, o.contains, true); 3250 } 3251 3252 @Override 3253 public boolean equalsShallow(Base other_) { 3254 if (!super.equalsShallow(other_)) 3255 return false; 3256 if (!(other_ instanceof ValueSetExpansionComponent)) 3257 return false; 3258 ValueSetExpansionComponent o = (ValueSetExpansionComponent) other_; 3259 return compareValues(identifier, o.identifier, true) && compareValues(timestamp, o.timestamp, true) 3260 && compareValues(total, o.total, true) && compareValues(offset, o.offset, true); 3261 } 3262 3263 public boolean isEmpty() { 3264 return super.isEmpty() 3265 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, timestamp, total, offset, parameter, contains); 3266 } 3267 3268 public String fhirType() { 3269 return "ValueSet.expansion"; 3270 3271 } 3272 3273 public ValueSetExpansionParameterComponent getParameter(String name) { 3274 for (ValueSetExpansionParameterComponent t : getParameter()) { 3275 if (name.equals(t.getName())) { 3276 return t; 3277 } 3278 } 3279 return null; 3280 } 3281 } 3282 3283 @Block() 3284 public static class ValueSetExpansionParameterComponent extends BackboneElement implements IBaseBackboneElement { 3285 /** 3286 * Name of the input parameter to the $expand operation; may be a 3287 * server-assigned name for additional default or other server-supplied 3288 * parameters used to control the expansion process. 3289 */ 3290 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3291 @Description(shortDefinition = "Name as assigned by the client or server", formalDefinition = "Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process.") 3292 protected StringType name; 3293 3294 /** 3295 * The value of the parameter. 3296 */ 3297 @Child(name = "value", type = { StringType.class, BooleanType.class, IntegerType.class, DecimalType.class, 3298 UriType.class, CodeType.class, 3299 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3300 @Description(shortDefinition = "Value of the named parameter", formalDefinition = "The value of the parameter.") 3301 protected Type value; 3302 3303 private static final long serialVersionUID = 1172641169L; 3304 3305 /** 3306 * Constructor 3307 */ 3308 public ValueSetExpansionParameterComponent() { 3309 super(); 3310 } 3311 3312 /** 3313 * Constructor 3314 */ 3315 public ValueSetExpansionParameterComponent(StringType name) { 3316 super(); 3317 this.name = name; 3318 } 3319 3320 /** 3321 * @return {@link #name} (Name of the input parameter to the $expand operation; 3322 * may be a server-assigned name for additional default or other 3323 * server-supplied parameters used to control the expansion process.). 3324 * This is the underlying object with id, value and extensions. The 3325 * accessor "getName" gives direct access to the value 3326 */ 3327 public StringType getNameElement() { 3328 if (this.name == null) 3329 if (Configuration.errorOnAutoCreate()) 3330 throw new Error("Attempt to auto-create ValueSetExpansionParameterComponent.name"); 3331 else if (Configuration.doAutoCreate()) 3332 this.name = new StringType(); // bb 3333 return this.name; 3334 } 3335 3336 public boolean hasNameElement() { 3337 return this.name != null && !this.name.isEmpty(); 3338 } 3339 3340 public boolean hasName() { 3341 return this.name != null && !this.name.isEmpty(); 3342 } 3343 3344 /** 3345 * @param value {@link #name} (Name of the input parameter to the $expand 3346 * operation; may be a server-assigned name for additional default 3347 * or other server-supplied parameters used to control the 3348 * expansion process.). This is the underlying object with id, 3349 * value and extensions. The accessor "getName" gives direct access 3350 * to the value 3351 */ 3352 public ValueSetExpansionParameterComponent setNameElement(StringType value) { 3353 this.name = value; 3354 return this; 3355 } 3356 3357 /** 3358 * @return Name of the input parameter to the $expand operation; may be a 3359 * server-assigned name for additional default or other server-supplied 3360 * parameters used to control the expansion process. 3361 */ 3362 public String getName() { 3363 return this.name == null ? null : this.name.getValue(); 3364 } 3365 3366 /** 3367 * @param value Name of the input parameter to the $expand operation; may be a 3368 * server-assigned name for additional default or other 3369 * server-supplied parameters used to control the expansion 3370 * process. 3371 */ 3372 public ValueSetExpansionParameterComponent setName(String value) { 3373 if (this.name == null) 3374 this.name = new StringType(); 3375 this.name.setValue(value); 3376 return this; 3377 } 3378 3379 /** 3380 * @return {@link #value} (The value of the parameter.) 3381 */ 3382 public Type getValue() { 3383 return this.value; 3384 } 3385 3386 /** 3387 * @return {@link #value} (The value of the parameter.) 3388 */ 3389 public StringType getValueStringType() throws FHIRException { 3390 if (this.value == null) 3391 this.value = new StringType(); 3392 if (!(this.value instanceof StringType)) 3393 throw new FHIRException("Type mismatch: the type StringType was expected, but " 3394 + this.value.getClass().getName() + " was encountered"); 3395 return (StringType) this.value; 3396 } 3397 3398 public boolean hasValueStringType() { 3399 return this != null && this.value instanceof StringType; 3400 } 3401 3402 /** 3403 * @return {@link #value} (The value of the parameter.) 3404 */ 3405 public BooleanType getValueBooleanType() throws FHIRException { 3406 if (this.value == null) 3407 this.value = new BooleanType(); 3408 if (!(this.value instanceof BooleanType)) 3409 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 3410 + this.value.getClass().getName() + " was encountered"); 3411 return (BooleanType) this.value; 3412 } 3413 3414 public boolean hasValueBooleanType() { 3415 return this != null && this.value instanceof BooleanType; 3416 } 3417 3418 /** 3419 * @return {@link #value} (The value of the parameter.) 3420 */ 3421 public IntegerType getValueIntegerType() throws FHIRException { 3422 if (this.value == null) 3423 this.value = new IntegerType(); 3424 if (!(this.value instanceof IntegerType)) 3425 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 3426 + this.value.getClass().getName() + " was encountered"); 3427 return (IntegerType) this.value; 3428 } 3429 3430 public boolean hasValueIntegerType() { 3431 return this != null && this.value instanceof IntegerType; 3432 } 3433 3434 /** 3435 * @return {@link #value} (The value of the parameter.) 3436 */ 3437 public DecimalType getValueDecimalType() throws FHIRException { 3438 if (this.value == null) 3439 this.value = new DecimalType(); 3440 if (!(this.value instanceof DecimalType)) 3441 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 3442 + this.value.getClass().getName() + " was encountered"); 3443 return (DecimalType) this.value; 3444 } 3445 3446 public boolean hasValueDecimalType() { 3447 return this != null && this.value instanceof DecimalType; 3448 } 3449 3450 /** 3451 * @return {@link #value} (The value of the parameter.) 3452 */ 3453 public UriType getValueUriType() throws FHIRException { 3454 if (this.value == null) 3455 this.value = new UriType(); 3456 if (!(this.value instanceof UriType)) 3457 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName() 3458 + " was encountered"); 3459 return (UriType) this.value; 3460 } 3461 3462 public boolean hasValueUriType() { 3463 return this != null && this.value instanceof UriType; 3464 } 3465 3466 /** 3467 * @return {@link #value} (The value of the parameter.) 3468 */ 3469 public CodeType getValueCodeType() throws FHIRException { 3470 if (this.value == null) 3471 this.value = new CodeType(); 3472 if (!(this.value instanceof CodeType)) 3473 throw new FHIRException("Type mismatch: the type CodeType was expected, but " + this.value.getClass().getName() 3474 + " was encountered"); 3475 return (CodeType) this.value; 3476 } 3477 3478 public boolean hasValueCodeType() { 3479 return this != null && this.value instanceof CodeType; 3480 } 3481 3482 /** 3483 * @return {@link #value} (The value of the parameter.) 3484 */ 3485 public DateTimeType getValueDateTimeType() throws FHIRException { 3486 if (this.value == null) 3487 this.value = new DateTimeType(); 3488 if (!(this.value instanceof DateTimeType)) 3489 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 3490 + this.value.getClass().getName() + " was encountered"); 3491 return (DateTimeType) this.value; 3492 } 3493 3494 public boolean hasValueDateTimeType() { 3495 return this != null && this.value instanceof DateTimeType; 3496 } 3497 3498 public boolean hasValue() { 3499 return this.value != null && !this.value.isEmpty(); 3500 } 3501 3502 /** 3503 * @param value {@link #value} (The value of the parameter.) 3504 */ 3505 public ValueSetExpansionParameterComponent setValue(Type value) { 3506 if (value != null && !(value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType 3507 || value instanceof DecimalType || value instanceof UriType || value instanceof CodeType 3508 || value instanceof DateTimeType)) 3509 throw new Error("Not the right type for ValueSet.expansion.parameter.value[x]: " + value.fhirType()); 3510 this.value = value; 3511 return this; 3512 } 3513 3514 protected void listChildren(List<Property> children) { 3515 super.listChildren(children); 3516 children.add(new Property("name", "string", 3517 "Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process.", 3518 0, 1, name)); 3519 children.add(new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3520 "The value of the parameter.", 0, 1, value)); 3521 } 3522 3523 @Override 3524 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3525 switch (_hash) { 3526 case 3373707: 3527 /* name */ return new Property("name", "string", 3528 "Name of the input parameter to the $expand operation; may be a server-assigned name for additional default or other server-supplied parameters used to control the expansion process.", 3529 0, 1, name); 3530 case -1410166417: 3531 /* value[x] */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3532 "The value of the parameter.", 0, 1, value); 3533 case 111972721: 3534 /* value */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3535 "The value of the parameter.", 0, 1, value); 3536 case -1424603934: 3537 /* valueString */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3538 "The value of the parameter.", 0, 1, value); 3539 case 733421943: 3540 /* valueBoolean */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3541 "The value of the parameter.", 0, 1, value); 3542 case -1668204915: 3543 /* valueInteger */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3544 "The value of the parameter.", 0, 1, value); 3545 case -2083993440: 3546 /* valueDecimal */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3547 "The value of the parameter.", 0, 1, value); 3548 case -1410172357: 3549 /* valueUri */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3550 "The value of the parameter.", 0, 1, value); 3551 case -766209282: 3552 /* valueCode */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3553 "The value of the parameter.", 0, 1, value); 3554 case 1047929900: 3555 /* valueDateTime */ return new Property("value[x]", "string|boolean|integer|decimal|uri|code|dateTime", 3556 "The value of the parameter.", 0, 1, value); 3557 default: 3558 return super.getNamedProperty(_hash, _name, _checkValid); 3559 } 3560 3561 } 3562 3563 @Override 3564 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3565 switch (hash) { 3566 case 3373707: 3567 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3568 case 111972721: 3569 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3570 default: 3571 return super.getProperty(hash, name, checkValid); 3572 } 3573 3574 } 3575 3576 @Override 3577 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3578 switch (hash) { 3579 case 3373707: // name 3580 this.name = castToString(value); // StringType 3581 return value; 3582 case 111972721: // value 3583 this.value = castToType(value); // Type 3584 return value; 3585 default: 3586 return super.setProperty(hash, name, value); 3587 } 3588 3589 } 3590 3591 @Override 3592 public Base setProperty(String name, Base value) throws FHIRException { 3593 if (name.equals("name")) { 3594 this.name = castToString(value); // StringType 3595 } else if (name.equals("value[x]")) { 3596 this.value = castToType(value); // Type 3597 } else 3598 return super.setProperty(name, value); 3599 return value; 3600 } 3601 3602 @Override 3603 public void removeChild(String name, Base value) throws FHIRException { 3604 if (name.equals("name")) { 3605 this.name = null; 3606 } else if (name.equals("value[x]")) { 3607 this.value = null; 3608 } else 3609 super.removeChild(name, value); 3610 3611 } 3612 3613 @Override 3614 public Base makeProperty(int hash, String name) throws FHIRException { 3615 switch (hash) { 3616 case 3373707: 3617 return getNameElement(); 3618 case -1410166417: 3619 return getValue(); 3620 case 111972721: 3621 return getValue(); 3622 default: 3623 return super.makeProperty(hash, name); 3624 } 3625 3626 } 3627 3628 @Override 3629 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3630 switch (hash) { 3631 case 3373707: 3632 /* name */ return new String[] { "string" }; 3633 case 111972721: 3634 /* value */ return new String[] { "string", "boolean", "integer", "decimal", "uri", "code", "dateTime" }; 3635 default: 3636 return super.getTypesForProperty(hash, name); 3637 } 3638 3639 } 3640 3641 @Override 3642 public Base addChild(String name) throws FHIRException { 3643 if (name.equals("name")) { 3644 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.name"); 3645 } else if (name.equals("valueString")) { 3646 this.value = new StringType(); 3647 return this.value; 3648 } else if (name.equals("valueBoolean")) { 3649 this.value = new BooleanType(); 3650 return this.value; 3651 } else if (name.equals("valueInteger")) { 3652 this.value = new IntegerType(); 3653 return this.value; 3654 } else if (name.equals("valueDecimal")) { 3655 this.value = new DecimalType(); 3656 return this.value; 3657 } else if (name.equals("valueUri")) { 3658 this.value = new UriType(); 3659 return this.value; 3660 } else if (name.equals("valueCode")) { 3661 this.value = new CodeType(); 3662 return this.value; 3663 } else if (name.equals("valueDateTime")) { 3664 this.value = new DateTimeType(); 3665 return this.value; 3666 } else 3667 return super.addChild(name); 3668 } 3669 3670 public ValueSetExpansionParameterComponent copy() { 3671 ValueSetExpansionParameterComponent dst = new ValueSetExpansionParameterComponent(); 3672 copyValues(dst); 3673 return dst; 3674 } 3675 3676 public void copyValues(ValueSetExpansionParameterComponent dst) { 3677 super.copyValues(dst); 3678 dst.name = name == null ? null : name.copy(); 3679 dst.value = value == null ? null : value.copy(); 3680 } 3681 3682 @Override 3683 public boolean equalsDeep(Base other_) { 3684 if (!super.equalsDeep(other_)) 3685 return false; 3686 if (!(other_ instanceof ValueSetExpansionParameterComponent)) 3687 return false; 3688 ValueSetExpansionParameterComponent o = (ValueSetExpansionParameterComponent) other_; 3689 return compareDeep(name, o.name, true) && compareDeep(value, o.value, true); 3690 } 3691 3692 @Override 3693 public boolean equalsShallow(Base other_) { 3694 if (!super.equalsShallow(other_)) 3695 return false; 3696 if (!(other_ instanceof ValueSetExpansionParameterComponent)) 3697 return false; 3698 ValueSetExpansionParameterComponent o = (ValueSetExpansionParameterComponent) other_; 3699 return compareValues(name, o.name, true); 3700 } 3701 3702 public boolean isEmpty() { 3703 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, value); 3704 } 3705 3706 public String fhirType() { 3707 return "ValueSet.expansion.parameter"; 3708 3709 } 3710 3711 } 3712 3713 @Block() 3714 public static class ValueSetExpansionContainsComponent extends BackboneElement implements IBaseBackboneElement { 3715 /** 3716 * An absolute URI which is the code system in which the code for this item in 3717 * the expansion is defined. 3718 */ 3719 @Child(name = "system", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3720 @Description(shortDefinition = "System value for the code", formalDefinition = "An absolute URI which is the code system in which the code for this item in the expansion is defined.") 3721 protected UriType system; 3722 3723 /** 3724 * If true, this entry is included in the expansion for navigational purposes, 3725 * and the user cannot select the code directly as a proper value. 3726 */ 3727 @Child(name = "abstract", type = { 3728 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3729 @Description(shortDefinition = "If user cannot select this entry", formalDefinition = "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.") 3730 protected BooleanType abstract_; 3731 3732 /** 3733 * If the concept is inactive in the code system that defines it. Inactive codes 3734 * are those that are no longer to be used, but are maintained by the code 3735 * system for understanding legacy data. It might not be known or specified 3736 * whether an concept is inactive (and it may depend on the context of use). 3737 */ 3738 @Child(name = "inactive", type = { 3739 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3740 @Description(shortDefinition = "If concept is inactive in the code system", formalDefinition = "If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether an concept is inactive (and it may depend on the context of use).") 3741 protected BooleanType inactive; 3742 3743 /** 3744 * The version of the code system from this code was taken. Note that a 3745 * well-maintained code system does not need the version reported, because the 3746 * meaning of codes is consistent across versions. However this cannot 3747 * consistently be assured, and when the meaning is not guaranteed to be 3748 * consistent, the version SHOULD be exchanged. 3749 */ 3750 @Child(name = "version", type = { 3751 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3752 @Description(shortDefinition = "Version in which this code/display is defined", formalDefinition = "The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.") 3753 protected StringType version; 3754 3755 /** 3756 * The code for this item in the expansion hierarchy. If this code is missing 3757 * the entry in the hierarchy is a place holder (abstract) and does not 3758 * represent a valid code in the value set. 3759 */ 3760 @Child(name = "code", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3761 @Description(shortDefinition = "Code - if blank, this is not a selectable code", formalDefinition = "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.") 3762 protected CodeType code; 3763 3764 /** 3765 * The recommended display for this item in the expansion. 3766 */ 3767 @Child(name = "display", type = { 3768 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 3769 @Description(shortDefinition = "User display for the concept", formalDefinition = "The recommended display for this item in the expansion.") 3770 protected StringType display; 3771 3772 /** 3773 * Additional representations for this item - other languages, aliases, 3774 * specialized purposes, used for particular purposes, etc. These are relevant 3775 * when the conditions of the expansion do not fix to a single correct 3776 * representation. 3777 */ 3778 @Child(name = "designation", type = { 3779 ConceptReferenceDesignationComponent.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3780 @Description(shortDefinition = "Additional representations for this item", formalDefinition = "Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.") 3781 protected List<ConceptReferenceDesignationComponent> designation; 3782 3783 /** 3784 * Other codes and entries contained under this entry in the hierarchy. 3785 */ 3786 @Child(name = "contains", type = { 3787 ValueSetExpansionContainsComponent.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3788 @Description(shortDefinition = "Codes contained under this entry", formalDefinition = "Other codes and entries contained under this entry in the hierarchy.") 3789 protected List<ValueSetExpansionContainsComponent> contains; 3790 3791 private static final long serialVersionUID = 719458860L; 3792 3793 /** 3794 * Constructor 3795 */ 3796 public ValueSetExpansionContainsComponent() { 3797 super(); 3798 } 3799 3800 /** 3801 * @return {@link #system} (An absolute URI which is the code system in which 3802 * the code for this item in the expansion is defined.). This is the 3803 * underlying object with id, value and extensions. The accessor 3804 * "getSystem" gives direct access to the value 3805 */ 3806 public UriType getSystemElement() { 3807 if (this.system == null) 3808 if (Configuration.errorOnAutoCreate()) 3809 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.system"); 3810 else if (Configuration.doAutoCreate()) 3811 this.system = new UriType(); // bb 3812 return this.system; 3813 } 3814 3815 public boolean hasSystemElement() { 3816 return this.system != null && !this.system.isEmpty(); 3817 } 3818 3819 public boolean hasSystem() { 3820 return this.system != null && !this.system.isEmpty(); 3821 } 3822 3823 /** 3824 * @param value {@link #system} (An absolute URI which is the code system in 3825 * which the code for this item in the expansion is defined.). This 3826 * is the underlying object with id, value and extensions. The 3827 * accessor "getSystem" gives direct access to the value 3828 */ 3829 public ValueSetExpansionContainsComponent setSystemElement(UriType value) { 3830 this.system = value; 3831 return this; 3832 } 3833 3834 /** 3835 * @return An absolute URI which is the code system in which the code for this 3836 * item in the expansion is defined. 3837 */ 3838 public String getSystem() { 3839 return this.system == null ? null : this.system.getValue(); 3840 } 3841 3842 /** 3843 * @param value An absolute URI which is the code system in which the code for 3844 * this item in the expansion is defined. 3845 */ 3846 public ValueSetExpansionContainsComponent setSystem(String value) { 3847 if (Utilities.noString(value)) 3848 this.system = null; 3849 else { 3850 if (this.system == null) 3851 this.system = new UriType(); 3852 this.system.setValue(value); 3853 } 3854 return this; 3855 } 3856 3857 /** 3858 * @return {@link #abstract_} (If true, this entry is included in the expansion 3859 * for navigational purposes, and the user cannot select the code 3860 * directly as a proper value.). This is the underlying object with id, 3861 * value and extensions. The accessor "getAbstract" gives direct access 3862 * to the value 3863 */ 3864 public BooleanType getAbstractElement() { 3865 if (this.abstract_ == null) 3866 if (Configuration.errorOnAutoCreate()) 3867 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.abstract_"); 3868 else if (Configuration.doAutoCreate()) 3869 this.abstract_ = new BooleanType(); // bb 3870 return this.abstract_; 3871 } 3872 3873 public boolean hasAbstractElement() { 3874 return this.abstract_ != null && !this.abstract_.isEmpty(); 3875 } 3876 3877 public boolean hasAbstract() { 3878 return this.abstract_ != null && !this.abstract_.isEmpty(); 3879 } 3880 3881 /** 3882 * @param value {@link #abstract_} (If true, this entry is included in the 3883 * expansion for navigational purposes, and the user cannot select 3884 * the code directly as a proper value.). This is the underlying 3885 * object with id, value and extensions. The accessor "getAbstract" 3886 * gives direct access to the value 3887 */ 3888 public ValueSetExpansionContainsComponent setAbstractElement(BooleanType value) { 3889 this.abstract_ = value; 3890 return this; 3891 } 3892 3893 /** 3894 * @return If true, this entry is included in the expansion for navigational 3895 * purposes, and the user cannot select the code directly as a proper 3896 * value. 3897 */ 3898 public boolean getAbstract() { 3899 return this.abstract_ == null || this.abstract_.isEmpty() ? false : this.abstract_.getValue(); 3900 } 3901 3902 /** 3903 * @param value If true, this entry is included in the expansion for 3904 * navigational purposes, and the user cannot select the code 3905 * directly as a proper value. 3906 */ 3907 public ValueSetExpansionContainsComponent setAbstract(boolean value) { 3908 if (this.abstract_ == null) 3909 this.abstract_ = new BooleanType(); 3910 this.abstract_.setValue(value); 3911 return this; 3912 } 3913 3914 /** 3915 * @return {@link #inactive} (If the concept is inactive in the code system that 3916 * defines it. Inactive codes are those that are no longer to be used, 3917 * but are maintained by the code system for understanding legacy data. 3918 * It might not be known or specified whether an concept is inactive 3919 * (and it may depend on the context of use).). This is the underlying 3920 * object with id, value and extensions. The accessor "getInactive" 3921 * gives direct access to the value 3922 */ 3923 public BooleanType getInactiveElement() { 3924 if (this.inactive == null) 3925 if (Configuration.errorOnAutoCreate()) 3926 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.inactive"); 3927 else if (Configuration.doAutoCreate()) 3928 this.inactive = new BooleanType(); // bb 3929 return this.inactive; 3930 } 3931 3932 public boolean hasInactiveElement() { 3933 return this.inactive != null && !this.inactive.isEmpty(); 3934 } 3935 3936 public boolean hasInactive() { 3937 return this.inactive != null && !this.inactive.isEmpty(); 3938 } 3939 3940 /** 3941 * @param value {@link #inactive} (If the concept is inactive in the code system 3942 * that defines it. Inactive codes are those that are no longer to 3943 * be used, but are maintained by the code system for understanding 3944 * legacy data. It might not be known or specified whether an 3945 * concept is inactive (and it may depend on the context of use).). 3946 * This is the underlying object with id, value and extensions. The 3947 * accessor "getInactive" gives direct access to the value 3948 */ 3949 public ValueSetExpansionContainsComponent setInactiveElement(BooleanType value) { 3950 this.inactive = value; 3951 return this; 3952 } 3953 3954 /** 3955 * @return If the concept is inactive in the code system that defines it. 3956 * Inactive codes are those that are no longer to be used, but are 3957 * maintained by the code system for understanding legacy data. It might 3958 * not be known or specified whether an concept is inactive (and it may 3959 * depend on the context of use). 3960 */ 3961 public boolean getInactive() { 3962 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 3963 } 3964 3965 /** 3966 * @param value If the concept is inactive in the code system that defines it. 3967 * Inactive codes are those that are no longer to be used, but are 3968 * maintained by the code system for understanding legacy data. It 3969 * might not be known or specified whether an concept is inactive 3970 * (and it may depend on the context of use). 3971 */ 3972 public ValueSetExpansionContainsComponent setInactive(boolean value) { 3973 if (this.inactive == null) 3974 this.inactive = new BooleanType(); 3975 this.inactive.setValue(value); 3976 return this; 3977 } 3978 3979 /** 3980 * @return {@link #version} (The version of the code system from this code was 3981 * taken. Note that a well-maintained code system does not need the 3982 * version reported, because the meaning of codes is consistent across 3983 * versions. However this cannot consistently be assured, and when the 3984 * meaning is not guaranteed to be consistent, the version SHOULD be 3985 * exchanged.). This is the underlying object with id, value and 3986 * extensions. The accessor "getVersion" gives direct access to the 3987 * value 3988 */ 3989 public StringType getVersionElement() { 3990 if (this.version == null) 3991 if (Configuration.errorOnAutoCreate()) 3992 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.version"); 3993 else if (Configuration.doAutoCreate()) 3994 this.version = new StringType(); // bb 3995 return this.version; 3996 } 3997 3998 public boolean hasVersionElement() { 3999 return this.version != null && !this.version.isEmpty(); 4000 } 4001 4002 public boolean hasVersion() { 4003 return this.version != null && !this.version.isEmpty(); 4004 } 4005 4006 /** 4007 * @param value {@link #version} (The version of the code system from this code 4008 * was taken. Note that a well-maintained code system does not need 4009 * the version reported, because the meaning of codes is consistent 4010 * across versions. However this cannot consistently be assured, 4011 * and when the meaning is not guaranteed to be consistent, the 4012 * version SHOULD be exchanged.). This is the underlying object 4013 * with id, value and extensions. The accessor "getVersion" gives 4014 * direct access to the value 4015 */ 4016 public ValueSetExpansionContainsComponent setVersionElement(StringType value) { 4017 this.version = value; 4018 return this; 4019 } 4020 4021 /** 4022 * @return The version of the code system from this code was taken. Note that a 4023 * well-maintained code system does not need the version reported, 4024 * because the meaning of codes is consistent across versions. However 4025 * this cannot consistently be assured, and when the meaning is not 4026 * guaranteed to be consistent, the version SHOULD be exchanged. 4027 */ 4028 public String getVersion() { 4029 return this.version == null ? null : this.version.getValue(); 4030 } 4031 4032 /** 4033 * @param value The version of the code system from this code was taken. Note 4034 * that a well-maintained code system does not need the version 4035 * reported, because the meaning of codes is consistent across 4036 * versions. However this cannot consistently be assured, and when 4037 * the meaning is not guaranteed to be consistent, the version 4038 * SHOULD be exchanged. 4039 */ 4040 public ValueSetExpansionContainsComponent setVersion(String value) { 4041 if (Utilities.noString(value)) 4042 this.version = null; 4043 else { 4044 if (this.version == null) 4045 this.version = new StringType(); 4046 this.version.setValue(value); 4047 } 4048 return this; 4049 } 4050 4051 /** 4052 * @return {@link #code} (The code for this item in the expansion hierarchy. If 4053 * this code is missing the entry in the hierarchy is a place holder 4054 * (abstract) and does not represent a valid code in the value set.). 4055 * This is the underlying object with id, value and extensions. The 4056 * accessor "getCode" gives direct access to the value 4057 */ 4058 public CodeType getCodeElement() { 4059 if (this.code == null) 4060 if (Configuration.errorOnAutoCreate()) 4061 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.code"); 4062 else if (Configuration.doAutoCreate()) 4063 this.code = new CodeType(); // bb 4064 return this.code; 4065 } 4066 4067 public boolean hasCodeElement() { 4068 return this.code != null && !this.code.isEmpty(); 4069 } 4070 4071 public boolean hasCode() { 4072 return this.code != null && !this.code.isEmpty(); 4073 } 4074 4075 /** 4076 * @param value {@link #code} (The code for this item in the expansion 4077 * hierarchy. If this code is missing the entry in the hierarchy is 4078 * a place holder (abstract) and does not represent a valid code in 4079 * the value set.). This is the underlying object with id, value 4080 * and extensions. The accessor "getCode" gives direct access to 4081 * the value 4082 */ 4083 public ValueSetExpansionContainsComponent setCodeElement(CodeType value) { 4084 this.code = value; 4085 return this; 4086 } 4087 4088 /** 4089 * @return The code for this item in the expansion hierarchy. If this code is 4090 * missing the entry in the hierarchy is a place holder (abstract) and 4091 * does not represent a valid code in the value set. 4092 */ 4093 public String getCode() { 4094 return this.code == null ? null : this.code.getValue(); 4095 } 4096 4097 /** 4098 * @param value The code for this item in the expansion hierarchy. If this code 4099 * is missing the entry in the hierarchy is a place holder 4100 * (abstract) and does not represent a valid code in the value set. 4101 */ 4102 public ValueSetExpansionContainsComponent setCode(String value) { 4103 if (Utilities.noString(value)) 4104 this.code = null; 4105 else { 4106 if (this.code == null) 4107 this.code = new CodeType(); 4108 this.code.setValue(value); 4109 } 4110 return this; 4111 } 4112 4113 /** 4114 * @return {@link #display} (The recommended display for this item in the 4115 * expansion.). This is the underlying object with id, value and 4116 * extensions. The accessor "getDisplay" gives direct access to the 4117 * value 4118 */ 4119 public StringType getDisplayElement() { 4120 if (this.display == null) 4121 if (Configuration.errorOnAutoCreate()) 4122 throw new Error("Attempt to auto-create ValueSetExpansionContainsComponent.display"); 4123 else if (Configuration.doAutoCreate()) 4124 this.display = new StringType(); // bb 4125 return this.display; 4126 } 4127 4128 public boolean hasDisplayElement() { 4129 return this.display != null && !this.display.isEmpty(); 4130 } 4131 4132 public boolean hasDisplay() { 4133 return this.display != null && !this.display.isEmpty(); 4134 } 4135 4136 /** 4137 * @param value {@link #display} (The recommended display for this item in the 4138 * expansion.). This is the underlying object with id, value and 4139 * extensions. The accessor "getDisplay" gives direct access to the 4140 * value 4141 */ 4142 public ValueSetExpansionContainsComponent setDisplayElement(StringType value) { 4143 this.display = value; 4144 return this; 4145 } 4146 4147 /** 4148 * @return The recommended display for this item in the expansion. 4149 */ 4150 public String getDisplay() { 4151 return this.display == null ? null : this.display.getValue(); 4152 } 4153 4154 /** 4155 * @param value The recommended display for this item in the expansion. 4156 */ 4157 public ValueSetExpansionContainsComponent setDisplay(String value) { 4158 if (Utilities.noString(value)) 4159 this.display = null; 4160 else { 4161 if (this.display == null) 4162 this.display = new StringType(); 4163 this.display.setValue(value); 4164 } 4165 return this; 4166 } 4167 4168 /** 4169 * @return {@link #designation} (Additional representations for this item - 4170 * other languages, aliases, specialized purposes, used for particular 4171 * purposes, etc. These are relevant when the conditions of the 4172 * expansion do not fix to a single correct representation.) 4173 */ 4174 public List<ConceptReferenceDesignationComponent> getDesignation() { 4175 if (this.designation == null) 4176 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 4177 return this.designation; 4178 } 4179 4180 /** 4181 * @return Returns a reference to <code>this</code> for easy method chaining 4182 */ 4183 public ValueSetExpansionContainsComponent setDesignation( 4184 List<ConceptReferenceDesignationComponent> theDesignation) { 4185 this.designation = theDesignation; 4186 return this; 4187 } 4188 4189 public boolean hasDesignation() { 4190 if (this.designation == null) 4191 return false; 4192 for (ConceptReferenceDesignationComponent item : this.designation) 4193 if (!item.isEmpty()) 4194 return true; 4195 return false; 4196 } 4197 4198 public ConceptReferenceDesignationComponent addDesignation() { // 3 4199 ConceptReferenceDesignationComponent t = new ConceptReferenceDesignationComponent(); 4200 if (this.designation == null) 4201 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 4202 this.designation.add(t); 4203 return t; 4204 } 4205 4206 public ValueSetExpansionContainsComponent addDesignation(ConceptReferenceDesignationComponent t) { // 3 4207 if (t == null) 4208 return this; 4209 if (this.designation == null) 4210 this.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 4211 this.designation.add(t); 4212 return this; 4213 } 4214 4215 /** 4216 * @return The first repetition of repeating field {@link #designation}, 4217 * creating it if it does not already exist 4218 */ 4219 public ConceptReferenceDesignationComponent getDesignationFirstRep() { 4220 if (getDesignation().isEmpty()) { 4221 addDesignation(); 4222 } 4223 return getDesignation().get(0); 4224 } 4225 4226 /** 4227 * @return {@link #contains} (Other codes and entries contained under this entry 4228 * in the hierarchy.) 4229 */ 4230 public List<ValueSetExpansionContainsComponent> getContains() { 4231 if (this.contains == null) 4232 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 4233 return this.contains; 4234 } 4235 4236 /** 4237 * @return Returns a reference to <code>this</code> for easy method chaining 4238 */ 4239 public ValueSetExpansionContainsComponent setContains(List<ValueSetExpansionContainsComponent> theContains) { 4240 this.contains = theContains; 4241 return this; 4242 } 4243 4244 public boolean hasContains() { 4245 if (this.contains == null) 4246 return false; 4247 for (ValueSetExpansionContainsComponent item : this.contains) 4248 if (!item.isEmpty()) 4249 return true; 4250 return false; 4251 } 4252 4253 public ValueSetExpansionContainsComponent addContains() { // 3 4254 ValueSetExpansionContainsComponent t = new ValueSetExpansionContainsComponent(); 4255 if (this.contains == null) 4256 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 4257 this.contains.add(t); 4258 return t; 4259 } 4260 4261 public ValueSetExpansionContainsComponent addContains(ValueSetExpansionContainsComponent t) { // 3 4262 if (t == null) 4263 return this; 4264 if (this.contains == null) 4265 this.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 4266 this.contains.add(t); 4267 return this; 4268 } 4269 4270 /** 4271 * @return The first repetition of repeating field {@link #contains}, creating 4272 * it if it does not already exist 4273 */ 4274 public ValueSetExpansionContainsComponent getContainsFirstRep() { 4275 if (getContains().isEmpty()) { 4276 addContains(); 4277 } 4278 return getContains().get(0); 4279 } 4280 4281 protected void listChildren(List<Property> children) { 4282 super.listChildren(children); 4283 children.add(new Property("system", "uri", 4284 "An absolute URI which is the code system in which the code for this item in the expansion is defined.", 0, 1, 4285 system)); 4286 children.add(new Property("abstract", "boolean", 4287 "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.", 4288 0, 1, abstract_)); 4289 children.add(new Property("inactive", "boolean", 4290 "If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether an concept is inactive (and it may depend on the context of use).", 4291 0, 1, inactive)); 4292 children.add(new Property("version", "string", 4293 "The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 4294 0, 1, version)); 4295 children.add(new Property("code", "code", 4296 "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.", 4297 0, 1, code)); 4298 children.add( 4299 new Property("display", "string", "The recommended display for this item in the expansion.", 0, 1, display)); 4300 children.add(new Property("designation", "@ValueSet.compose.include.concept.designation", 4301 "Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.", 4302 0, java.lang.Integer.MAX_VALUE, designation)); 4303 children.add(new Property("contains", "@ValueSet.expansion.contains", 4304 "Other codes and entries contained under this entry in the hierarchy.", 0, java.lang.Integer.MAX_VALUE, 4305 contains)); 4306 } 4307 4308 @Override 4309 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4310 switch (_hash) { 4311 case -887328209: 4312 /* system */ return new Property("system", "uri", 4313 "An absolute URI which is the code system in which the code for this item in the expansion is defined.", 0, 4314 1, system); 4315 case 1732898850: 4316 /* abstract */ return new Property("abstract", "boolean", 4317 "If true, this entry is included in the expansion for navigational purposes, and the user cannot select the code directly as a proper value.", 4318 0, 1, abstract_); 4319 case 24665195: 4320 /* inactive */ return new Property("inactive", "boolean", 4321 "If the concept is inactive in the code system that defines it. Inactive codes are those that are no longer to be used, but are maintained by the code system for understanding legacy data. It might not be known or specified whether an concept is inactive (and it may depend on the context of use).", 4322 0, 1, inactive); 4323 case 351608024: 4324 /* version */ return new Property("version", "string", 4325 "The version of the code system from this code was taken. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 4326 0, 1, version); 4327 case 3059181: 4328 /* code */ return new Property("code", "code", 4329 "The code for this item in the expansion hierarchy. If this code is missing the entry in the hierarchy is a place holder (abstract) and does not represent a valid code in the value set.", 4330 0, 1, code); 4331 case 1671764162: 4332 /* display */ return new Property("display", "string", 4333 "The recommended display for this item in the expansion.", 0, 1, display); 4334 case -900931593: 4335 /* designation */ return new Property("designation", "@ValueSet.compose.include.concept.designation", 4336 "Additional representations for this item - other languages, aliases, specialized purposes, used for particular purposes, etc. These are relevant when the conditions of the expansion do not fix to a single correct representation.", 4337 0, java.lang.Integer.MAX_VALUE, designation); 4338 case -567445985: 4339 /* contains */ return new Property("contains", "@ValueSet.expansion.contains", 4340 "Other codes and entries contained under this entry in the hierarchy.", 0, java.lang.Integer.MAX_VALUE, 4341 contains); 4342 default: 4343 return super.getNamedProperty(_hash, _name, _checkValid); 4344 } 4345 4346 } 4347 4348 @Override 4349 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4350 switch (hash) { 4351 case -887328209: 4352 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // UriType 4353 case 1732898850: 4354 /* abstract */ return this.abstract_ == null ? new Base[0] : new Base[] { this.abstract_ }; // BooleanType 4355 case 24665195: 4356 /* inactive */ return this.inactive == null ? new Base[0] : new Base[] { this.inactive }; // BooleanType 4357 case 351608024: 4358 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4359 case 3059181: 4360 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 4361 case 1671764162: 4362 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 4363 case -900931593: 4364 /* designation */ return this.designation == null ? new Base[0] 4365 : this.designation.toArray(new Base[this.designation.size()]); // ConceptReferenceDesignationComponent 4366 case -567445985: 4367 /* contains */ return this.contains == null ? new Base[0] 4368 : this.contains.toArray(new Base[this.contains.size()]); // ValueSetExpansionContainsComponent 4369 default: 4370 return super.getProperty(hash, name, checkValid); 4371 } 4372 4373 } 4374 4375 @Override 4376 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4377 switch (hash) { 4378 case -887328209: // system 4379 this.system = castToUri(value); // UriType 4380 return value; 4381 case 1732898850: // abstract 4382 this.abstract_ = castToBoolean(value); // BooleanType 4383 return value; 4384 case 24665195: // inactive 4385 this.inactive = castToBoolean(value); // BooleanType 4386 return value; 4387 case 351608024: // version 4388 this.version = castToString(value); // StringType 4389 return value; 4390 case 3059181: // code 4391 this.code = castToCode(value); // CodeType 4392 return value; 4393 case 1671764162: // display 4394 this.display = castToString(value); // StringType 4395 return value; 4396 case -900931593: // designation 4397 this.getDesignation().add((ConceptReferenceDesignationComponent) value); // ConceptReferenceDesignationComponent 4398 return value; 4399 case -567445985: // contains 4400 this.getContains().add((ValueSetExpansionContainsComponent) value); // ValueSetExpansionContainsComponent 4401 return value; 4402 default: 4403 return super.setProperty(hash, name, value); 4404 } 4405 4406 } 4407 4408 @Override 4409 public Base setProperty(String name, Base value) throws FHIRException { 4410 if (name.equals("system")) { 4411 this.system = castToUri(value); // UriType 4412 } else if (name.equals("abstract")) { 4413 this.abstract_ = castToBoolean(value); // BooleanType 4414 } else if (name.equals("inactive")) { 4415 this.inactive = castToBoolean(value); // BooleanType 4416 } else if (name.equals("version")) { 4417 this.version = castToString(value); // StringType 4418 } else if (name.equals("code")) { 4419 this.code = castToCode(value); // CodeType 4420 } else if (name.equals("display")) { 4421 this.display = castToString(value); // StringType 4422 } else if (name.equals("designation")) { 4423 this.getDesignation().add((ConceptReferenceDesignationComponent) value); 4424 } else if (name.equals("contains")) { 4425 this.getContains().add((ValueSetExpansionContainsComponent) value); 4426 } else 4427 return super.setProperty(name, value); 4428 return value; 4429 } 4430 4431 @Override 4432 public void removeChild(String name, Base value) throws FHIRException { 4433 if (name.equals("system")) { 4434 this.system = null; 4435 } else if (name.equals("abstract")) { 4436 this.abstract_ = null; 4437 } else if (name.equals("inactive")) { 4438 this.inactive = null; 4439 } else if (name.equals("version")) { 4440 this.version = null; 4441 } else if (name.equals("code")) { 4442 this.code = null; 4443 } else if (name.equals("display")) { 4444 this.display = null; 4445 } else if (name.equals("designation")) { 4446 this.getDesignation().remove((ConceptReferenceDesignationComponent) value); 4447 } else if (name.equals("contains")) { 4448 this.getContains().remove((ValueSetExpansionContainsComponent) value); 4449 } else 4450 super.removeChild(name, value); 4451 4452 } 4453 4454 @Override 4455 public Base makeProperty(int hash, String name) throws FHIRException { 4456 switch (hash) { 4457 case -887328209: 4458 return getSystemElement(); 4459 case 1732898850: 4460 return getAbstractElement(); 4461 case 24665195: 4462 return getInactiveElement(); 4463 case 351608024: 4464 return getVersionElement(); 4465 case 3059181: 4466 return getCodeElement(); 4467 case 1671764162: 4468 return getDisplayElement(); 4469 case -900931593: 4470 return addDesignation(); 4471 case -567445985: 4472 return addContains(); 4473 default: 4474 return super.makeProperty(hash, name); 4475 } 4476 4477 } 4478 4479 @Override 4480 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4481 switch (hash) { 4482 case -887328209: 4483 /* system */ return new String[] { "uri" }; 4484 case 1732898850: 4485 /* abstract */ return new String[] { "boolean" }; 4486 case 24665195: 4487 /* inactive */ return new String[] { "boolean" }; 4488 case 351608024: 4489 /* version */ return new String[] { "string" }; 4490 case 3059181: 4491 /* code */ return new String[] { "code" }; 4492 case 1671764162: 4493 /* display */ return new String[] { "string" }; 4494 case -900931593: 4495 /* designation */ return new String[] { "@ValueSet.compose.include.concept.designation" }; 4496 case -567445985: 4497 /* contains */ return new String[] { "@ValueSet.expansion.contains" }; 4498 default: 4499 return super.getTypesForProperty(hash, name); 4500 } 4501 4502 } 4503 4504 @Override 4505 public Base addChild(String name) throws FHIRException { 4506 if (name.equals("system")) { 4507 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.system"); 4508 } else if (name.equals("abstract")) { 4509 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.abstract"); 4510 } else if (name.equals("inactive")) { 4511 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.inactive"); 4512 } else if (name.equals("version")) { 4513 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 4514 } else if (name.equals("code")) { 4515 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.code"); 4516 } else if (name.equals("display")) { 4517 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.display"); 4518 } else if (name.equals("designation")) { 4519 return addDesignation(); 4520 } else if (name.equals("contains")) { 4521 return addContains(); 4522 } else 4523 return super.addChild(name); 4524 } 4525 4526 public ValueSetExpansionContainsComponent copy() { 4527 ValueSetExpansionContainsComponent dst = new ValueSetExpansionContainsComponent(); 4528 copyValues(dst); 4529 return dst; 4530 } 4531 4532 public void copyValues(ValueSetExpansionContainsComponent dst) { 4533 super.copyValues(dst); 4534 dst.system = system == null ? null : system.copy(); 4535 dst.abstract_ = abstract_ == null ? null : abstract_.copy(); 4536 dst.inactive = inactive == null ? null : inactive.copy(); 4537 dst.version = version == null ? null : version.copy(); 4538 dst.code = code == null ? null : code.copy(); 4539 dst.display = display == null ? null : display.copy(); 4540 if (designation != null) { 4541 dst.designation = new ArrayList<ConceptReferenceDesignationComponent>(); 4542 for (ConceptReferenceDesignationComponent i : designation) 4543 dst.designation.add(i.copy()); 4544 } 4545 ; 4546 if (contains != null) { 4547 dst.contains = new ArrayList<ValueSetExpansionContainsComponent>(); 4548 for (ValueSetExpansionContainsComponent i : contains) 4549 dst.contains.add(i.copy()); 4550 } 4551 ; 4552 } 4553 4554 @Override 4555 public boolean equalsDeep(Base other_) { 4556 if (!super.equalsDeep(other_)) 4557 return false; 4558 if (!(other_ instanceof ValueSetExpansionContainsComponent)) 4559 return false; 4560 ValueSetExpansionContainsComponent o = (ValueSetExpansionContainsComponent) other_; 4561 return compareDeep(system, o.system, true) && compareDeep(abstract_, o.abstract_, true) 4562 && compareDeep(inactive, o.inactive, true) && compareDeep(version, o.version, true) 4563 && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 4564 && compareDeep(designation, o.designation, true) && compareDeep(contains, o.contains, true); 4565 } 4566 4567 @Override 4568 public boolean equalsShallow(Base other_) { 4569 if (!super.equalsShallow(other_)) 4570 return false; 4571 if (!(other_ instanceof ValueSetExpansionContainsComponent)) 4572 return false; 4573 ValueSetExpansionContainsComponent o = (ValueSetExpansionContainsComponent) other_; 4574 return compareValues(system, o.system, true) && compareValues(abstract_, o.abstract_, true) 4575 && compareValues(inactive, o.inactive, true) && compareValues(version, o.version, true) 4576 && compareValues(code, o.code, true) && compareValues(display, o.display, true); 4577 } 4578 4579 public boolean isEmpty() { 4580 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, abstract_, inactive, version, code, 4581 display, designation, contains); 4582 } 4583 4584 public String fhirType() { 4585 return "ValueSet.expansion.contains"; 4586 4587 } 4588 4589 } 4590 4591 /** 4592 * A formal identifier that is used to identify this value set when it is 4593 * represented in other formats, or referenced in a specification, model, design 4594 * or an instance. 4595 */ 4596 @Child(name = "identifier", type = { 4597 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4598 @Description(shortDefinition = "Additional identifier for the value set (business identifier)", formalDefinition = "A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.") 4599 protected List<Identifier> identifier; 4600 4601 /** 4602 * If this is set to 'true', then no new versions of the content logical 4603 * definition can be created. Note: Other metadata might still change. 4604 */ 4605 @Child(name = "immutable", type = { 4606 BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 4607 @Description(shortDefinition = "Indicates whether or not any change to the content logical definition may occur", formalDefinition = "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.") 4608 protected BooleanType immutable; 4609 4610 /** 4611 * Explanation of why this value set is needed and why it has been designed as 4612 * it has. 4613 */ 4614 @Child(name = "purpose", type = { 4615 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4616 @Description(shortDefinition = "Why this value set is defined", formalDefinition = "Explanation of why this value set is needed and why it has been designed as it has.") 4617 protected MarkdownType purpose; 4618 4619 /** 4620 * A copyright statement relating to the value set and/or its contents. 4621 * Copyright statements are generally legal restrictions on the use and 4622 * publishing of the value set. 4623 */ 4624 @Child(name = "copyright", type = { 4625 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4626 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.") 4627 protected MarkdownType copyright; 4628 4629 /** 4630 * A set of criteria that define the contents of the value set by including or 4631 * excluding codes selected from the specified code system(s) that the value set 4632 * draws from. This is also known as the Content Logical Definition (CLD). 4633 */ 4634 @Child(name = "compose", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = false) 4635 @Description(shortDefinition = "Content logical definition of the value set (CLD)", formalDefinition = "A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD).") 4636 protected ValueSetComposeComponent compose; 4637 4638 /** 4639 * A value set can also be "expanded", where the value set is turned into a 4640 * simple collection of enumerated codes. This element holds the expansion, if 4641 * it has been performed. 4642 */ 4643 @Child(name = "expansion", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = false) 4644 @Description(shortDefinition = "Used when the value set is \"expanded\"", formalDefinition = "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.") 4645 protected ValueSetExpansionComponent expansion; 4646 4647 private static final long serialVersionUID = 786712949L; 4648 4649 /** 4650 * Constructor 4651 */ 4652 public ValueSet() { 4653 super(); 4654 } 4655 4656 /** 4657 * Constructor 4658 */ 4659 public ValueSet(Enumeration<PublicationStatus> status) { 4660 super(); 4661 this.status = status; 4662 } 4663 4664 /** 4665 * @return {@link #url} (An absolute URI that is used to identify this value set 4666 * when it is referenced in a specification, model, design or an 4667 * instance; also called its canonical identifier. This SHOULD be 4668 * globally unique and SHOULD be a literal address at which at which an 4669 * authoritative instance of this value set is (or will be) published. 4670 * This URL can be the target of a canonical reference. It SHALL remain 4671 * the same when the value set is stored on different servers.). This is 4672 * the underlying object with id, value and extensions. The accessor 4673 * "getUrl" gives direct access to the value 4674 */ 4675 public UriType getUrlElement() { 4676 if (this.url == null) 4677 if (Configuration.errorOnAutoCreate()) 4678 throw new Error("Attempt to auto-create ValueSet.url"); 4679 else if (Configuration.doAutoCreate()) 4680 this.url = new UriType(); // bb 4681 return this.url; 4682 } 4683 4684 public boolean hasUrlElement() { 4685 return this.url != null && !this.url.isEmpty(); 4686 } 4687 4688 public boolean hasUrl() { 4689 return this.url != null && !this.url.isEmpty(); 4690 } 4691 4692 /** 4693 * @param value {@link #url} (An absolute URI that is used to identify this 4694 * value set when it is referenced in a specification, model, 4695 * design or an instance; also called its canonical identifier. 4696 * This SHOULD be globally unique and SHOULD be a literal address 4697 * at which at which an authoritative instance of this value set is 4698 * (or will be) published. This URL can be the target of a 4699 * canonical reference. It SHALL remain the same when the value set 4700 * is stored on different servers.). This is the underlying object 4701 * with id, value and extensions. The accessor "getUrl" gives 4702 * direct access to the value 4703 */ 4704 public ValueSet setUrlElement(UriType value) { 4705 this.url = value; 4706 return this; 4707 } 4708 4709 /** 4710 * @return An absolute URI that is used to identify this value set when it is 4711 * referenced in a specification, model, design or an instance; also 4712 * called its canonical identifier. This SHOULD be globally unique and 4713 * SHOULD be a literal address at which at which an authoritative 4714 * instance of this value set is (or will be) published. This URL can be 4715 * the target of a canonical reference. It SHALL remain the same when 4716 * the value set is stored on different servers. 4717 */ 4718 public String getUrl() { 4719 return this.url == null ? null : this.url.getValue(); 4720 } 4721 4722 /** 4723 * @param value An absolute URI that is used to identify this value set when it 4724 * is referenced in a specification, model, design or an instance; 4725 * also called its canonical identifier. This SHOULD be globally 4726 * unique and SHOULD be a literal address at which at which an 4727 * authoritative instance of this value set is (or will be) 4728 * published. This URL can be the target of a canonical reference. 4729 * It SHALL remain the same when the value set is stored on 4730 * different servers. 4731 */ 4732 public ValueSet setUrl(String value) { 4733 if (Utilities.noString(value)) 4734 this.url = null; 4735 else { 4736 if (this.url == null) 4737 this.url = new UriType(); 4738 this.url.setValue(value); 4739 } 4740 return this; 4741 } 4742 4743 /** 4744 * @return {@link #identifier} (A formal identifier that is used to identify 4745 * this value set when it is represented in other formats, or referenced 4746 * in a specification, model, design or an instance.) 4747 */ 4748 public List<Identifier> getIdentifier() { 4749 if (this.identifier == null) 4750 this.identifier = new ArrayList<Identifier>(); 4751 return this.identifier; 4752 } 4753 4754 /** 4755 * @return Returns a reference to <code>this</code> for easy method chaining 4756 */ 4757 public ValueSet setIdentifier(List<Identifier> theIdentifier) { 4758 this.identifier = theIdentifier; 4759 return this; 4760 } 4761 4762 public boolean hasIdentifier() { 4763 if (this.identifier == null) 4764 return false; 4765 for (Identifier item : this.identifier) 4766 if (!item.isEmpty()) 4767 return true; 4768 return false; 4769 } 4770 4771 public Identifier addIdentifier() { // 3 4772 Identifier t = new Identifier(); 4773 if (this.identifier == null) 4774 this.identifier = new ArrayList<Identifier>(); 4775 this.identifier.add(t); 4776 return t; 4777 } 4778 4779 public ValueSet addIdentifier(Identifier t) { // 3 4780 if (t == null) 4781 return this; 4782 if (this.identifier == null) 4783 this.identifier = new ArrayList<Identifier>(); 4784 this.identifier.add(t); 4785 return this; 4786 } 4787 4788 /** 4789 * @return The first repetition of repeating field {@link #identifier}, creating 4790 * it if it does not already exist 4791 */ 4792 public Identifier getIdentifierFirstRep() { 4793 if (getIdentifier().isEmpty()) { 4794 addIdentifier(); 4795 } 4796 return getIdentifier().get(0); 4797 } 4798 4799 /** 4800 * @return {@link #version} (The identifier that is used to identify this 4801 * version of the value set when it is referenced in a specification, 4802 * model, design or instance. This is an arbitrary value managed by the 4803 * value set author and is not expected to be globally unique. For 4804 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 4805 * is not available. There is also no expectation that versions can be 4806 * placed in a lexicographical sequence.). This is the underlying object 4807 * with id, value and extensions. The accessor "getVersion" gives direct 4808 * access to the value 4809 */ 4810 public StringType getVersionElement() { 4811 if (this.version == null) 4812 if (Configuration.errorOnAutoCreate()) 4813 throw new Error("Attempt to auto-create ValueSet.version"); 4814 else if (Configuration.doAutoCreate()) 4815 this.version = new StringType(); // bb 4816 return this.version; 4817 } 4818 4819 public boolean hasVersionElement() { 4820 return this.version != null && !this.version.isEmpty(); 4821 } 4822 4823 public boolean hasVersion() { 4824 return this.version != null && !this.version.isEmpty(); 4825 } 4826 4827 /** 4828 * @param value {@link #version} (The identifier that is used to identify this 4829 * version of the value set when it is referenced in a 4830 * specification, model, design or instance. This is an arbitrary 4831 * value managed by the value set author and is not expected to be 4832 * globally unique. For example, it might be a timestamp (e.g. 4833 * yyyymmdd) if a managed version is not available. There is also 4834 * no expectation that versions can be placed in a lexicographical 4835 * sequence.). This is the underlying object with id, value and 4836 * extensions. The accessor "getVersion" gives direct access to the 4837 * value 4838 */ 4839 public ValueSet setVersionElement(StringType value) { 4840 this.version = value; 4841 return this; 4842 } 4843 4844 /** 4845 * @return The identifier that is used to identify this version of the value set 4846 * when it is referenced in a specification, model, design or instance. 4847 * This is an arbitrary value managed by the value set author and is not 4848 * expected to be globally unique. For example, it might be a timestamp 4849 * (e.g. yyyymmdd) if a managed version is not available. There is also 4850 * no expectation that versions can be placed in a lexicographical 4851 * sequence. 4852 */ 4853 public String getVersion() { 4854 return this.version == null ? null : this.version.getValue(); 4855 } 4856 4857 /** 4858 * @param value The identifier that is used to identify this version of the 4859 * value set when it is referenced in a specification, model, 4860 * design or instance. This is an arbitrary value managed by the 4861 * value set author and is not expected to be globally unique. For 4862 * example, it might be a timestamp (e.g. yyyymmdd) if a managed 4863 * version is not available. There is also no expectation that 4864 * versions can be placed in a lexicographical sequence. 4865 */ 4866 public ValueSet setVersion(String value) { 4867 if (Utilities.noString(value)) 4868 this.version = null; 4869 else { 4870 if (this.version == null) 4871 this.version = new StringType(); 4872 this.version.setValue(value); 4873 } 4874 return this; 4875 } 4876 4877 /** 4878 * @return {@link #name} (A natural language name identifying the value set. 4879 * This name should be usable as an identifier for the module by machine 4880 * processing applications such as code generation.). This is the 4881 * underlying object with id, value and extensions. The accessor 4882 * "getName" gives direct access to the value 4883 */ 4884 public StringType getNameElement() { 4885 if (this.name == null) 4886 if (Configuration.errorOnAutoCreate()) 4887 throw new Error("Attempt to auto-create ValueSet.name"); 4888 else if (Configuration.doAutoCreate()) 4889 this.name = new StringType(); // bb 4890 return this.name; 4891 } 4892 4893 public boolean hasNameElement() { 4894 return this.name != null && !this.name.isEmpty(); 4895 } 4896 4897 public boolean hasName() { 4898 return this.name != null && !this.name.isEmpty(); 4899 } 4900 4901 /** 4902 * @param value {@link #name} (A natural language name identifying the value 4903 * set. This name should be usable as an identifier for the module 4904 * by machine processing applications such as code generation.). 4905 * This is the underlying object with id, value and extensions. The 4906 * accessor "getName" gives direct access to the value 4907 */ 4908 public ValueSet setNameElement(StringType value) { 4909 this.name = value; 4910 return this; 4911 } 4912 4913 /** 4914 * @return A natural language name identifying the value set. This name should 4915 * be usable as an identifier for the module by machine processing 4916 * applications such as code generation. 4917 */ 4918 public String getName() { 4919 return this.name == null ? null : this.name.getValue(); 4920 } 4921 4922 /** 4923 * @param value A natural language name identifying the value set. This name 4924 * should be usable as an identifier for the module by machine 4925 * processing applications such as code generation. 4926 */ 4927 public ValueSet setName(String value) { 4928 if (Utilities.noString(value)) 4929 this.name = null; 4930 else { 4931 if (this.name == null) 4932 this.name = new StringType(); 4933 this.name.setValue(value); 4934 } 4935 return this; 4936 } 4937 4938 /** 4939 * @return {@link #title} (A short, descriptive, user-friendly title for the 4940 * value set.). This is the underlying object with id, value and 4941 * extensions. The accessor "getTitle" gives direct access to the value 4942 */ 4943 public StringType getTitleElement() { 4944 if (this.title == null) 4945 if (Configuration.errorOnAutoCreate()) 4946 throw new Error("Attempt to auto-create ValueSet.title"); 4947 else if (Configuration.doAutoCreate()) 4948 this.title = new StringType(); // bb 4949 return this.title; 4950 } 4951 4952 public boolean hasTitleElement() { 4953 return this.title != null && !this.title.isEmpty(); 4954 } 4955 4956 public boolean hasTitle() { 4957 return this.title != null && !this.title.isEmpty(); 4958 } 4959 4960 /** 4961 * @param value {@link #title} (A short, descriptive, user-friendly title for 4962 * the value set.). This is the underlying object with id, value 4963 * and extensions. The accessor "getTitle" gives direct access to 4964 * the value 4965 */ 4966 public ValueSet setTitleElement(StringType value) { 4967 this.title = value; 4968 return this; 4969 } 4970 4971 /** 4972 * @return A short, descriptive, user-friendly title for the value set. 4973 */ 4974 public String getTitle() { 4975 return this.title == null ? null : this.title.getValue(); 4976 } 4977 4978 /** 4979 * @param value A short, descriptive, user-friendly title for the value set. 4980 */ 4981 public ValueSet setTitle(String value) { 4982 if (Utilities.noString(value)) 4983 this.title = null; 4984 else { 4985 if (this.title == null) 4986 this.title = new StringType(); 4987 this.title.setValue(value); 4988 } 4989 return this; 4990 } 4991 4992 /** 4993 * @return {@link #status} (The status of this value set. Enables tracking the 4994 * life-cycle of the content. The status of the value set applies to the 4995 * value set definition (ValueSet.compose) and the associated ValueSet 4996 * metadata. Expansions do not have a state.). This is the underlying 4997 * object with id, value and extensions. The accessor "getStatus" gives 4998 * direct access to the value 4999 */ 5000 public Enumeration<PublicationStatus> getStatusElement() { 5001 if (this.status == null) 5002 if (Configuration.errorOnAutoCreate()) 5003 throw new Error("Attempt to auto-create ValueSet.status"); 5004 else if (Configuration.doAutoCreate()) 5005 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 5006 return this.status; 5007 } 5008 5009 public boolean hasStatusElement() { 5010 return this.status != null && !this.status.isEmpty(); 5011 } 5012 5013 public boolean hasStatus() { 5014 return this.status != null && !this.status.isEmpty(); 5015 } 5016 5017 /** 5018 * @param value {@link #status} (The status of this value set. Enables tracking 5019 * the life-cycle of the content. The status of the value set 5020 * applies to the value set definition (ValueSet.compose) and the 5021 * associated ValueSet metadata. Expansions do not have a state.). 5022 * This is the underlying object with id, value and extensions. The 5023 * accessor "getStatus" gives direct access to the value 5024 */ 5025 public ValueSet setStatusElement(Enumeration<PublicationStatus> value) { 5026 this.status = value; 5027 return this; 5028 } 5029 5030 /** 5031 * @return The status of this value set. Enables tracking the life-cycle of the 5032 * content. The status of the value set applies to the value set 5033 * definition (ValueSet.compose) and the associated ValueSet metadata. 5034 * Expansions do not have a state. 5035 */ 5036 public PublicationStatus getStatus() { 5037 return this.status == null ? null : this.status.getValue(); 5038 } 5039 5040 /** 5041 * @param value The status of this value set. Enables tracking the life-cycle of 5042 * the content. The status of the value set applies to the value 5043 * set definition (ValueSet.compose) and the associated ValueSet 5044 * metadata. Expansions do not have a state. 5045 */ 5046 public ValueSet setStatus(PublicationStatus value) { 5047 if (this.status == null) 5048 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 5049 this.status.setValue(value); 5050 return this; 5051 } 5052 5053 /** 5054 * @return {@link #experimental} (A Boolean value to indicate that this value 5055 * set is authored for testing purposes (or 5056 * education/evaluation/marketing) and is not intended to be used for 5057 * genuine usage.). This is the underlying object with id, value and 5058 * extensions. The accessor "getExperimental" gives direct access to the 5059 * value 5060 */ 5061 public BooleanType getExperimentalElement() { 5062 if (this.experimental == null) 5063 if (Configuration.errorOnAutoCreate()) 5064 throw new Error("Attempt to auto-create ValueSet.experimental"); 5065 else if (Configuration.doAutoCreate()) 5066 this.experimental = new BooleanType(); // bb 5067 return this.experimental; 5068 } 5069 5070 public boolean hasExperimentalElement() { 5071 return this.experimental != null && !this.experimental.isEmpty(); 5072 } 5073 5074 public boolean hasExperimental() { 5075 return this.experimental != null && !this.experimental.isEmpty(); 5076 } 5077 5078 /** 5079 * @param value {@link #experimental} (A Boolean value to indicate that this 5080 * value set is authored for testing purposes (or 5081 * education/evaluation/marketing) and is not intended to be used 5082 * for genuine usage.). This is the underlying object with id, 5083 * value and extensions. The accessor "getExperimental" gives 5084 * direct access to the value 5085 */ 5086 public ValueSet setExperimentalElement(BooleanType value) { 5087 this.experimental = value; 5088 return this; 5089 } 5090 5091 /** 5092 * @return A Boolean value to indicate that this value set is authored for 5093 * testing purposes (or education/evaluation/marketing) and is not 5094 * intended to be used for genuine usage. 5095 */ 5096 public boolean getExperimental() { 5097 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 5098 } 5099 5100 /** 5101 * @param value A Boolean value to indicate that this value set is authored for 5102 * testing purposes (or education/evaluation/marketing) and is not 5103 * intended to be used for genuine usage. 5104 */ 5105 public ValueSet setExperimental(boolean value) { 5106 if (this.experimental == null) 5107 this.experimental = new BooleanType(); 5108 this.experimental.setValue(value); 5109 return this; 5110 } 5111 5112 /** 5113 * @return {@link #date} (The date (and optionally time) when the value set was 5114 * created or revised (e.g. the 'content logical definition').). This is 5115 * the underlying object with id, value and extensions. The accessor 5116 * "getDate" gives direct access to the value 5117 */ 5118 public DateTimeType getDateElement() { 5119 if (this.date == null) 5120 if (Configuration.errorOnAutoCreate()) 5121 throw new Error("Attempt to auto-create ValueSet.date"); 5122 else if (Configuration.doAutoCreate()) 5123 this.date = new DateTimeType(); // bb 5124 return this.date; 5125 } 5126 5127 public boolean hasDateElement() { 5128 return this.date != null && !this.date.isEmpty(); 5129 } 5130 5131 public boolean hasDate() { 5132 return this.date != null && !this.date.isEmpty(); 5133 } 5134 5135 /** 5136 * @param value {@link #date} (The date (and optionally time) when the value set 5137 * was created or revised (e.g. the 'content logical 5138 * definition').). This is the underlying object with id, value and 5139 * extensions. The accessor "getDate" gives direct access to the 5140 * value 5141 */ 5142 public ValueSet setDateElement(DateTimeType value) { 5143 this.date = value; 5144 return this; 5145 } 5146 5147 /** 5148 * @return The date (and optionally time) when the value set was created or 5149 * revised (e.g. the 'content logical definition'). 5150 */ 5151 public Date getDate() { 5152 return this.date == null ? null : this.date.getValue(); 5153 } 5154 5155 /** 5156 * @param value The date (and optionally time) when the value set was created or 5157 * revised (e.g. the 'content logical definition'). 5158 */ 5159 public ValueSet setDate(Date value) { 5160 if (value == null) 5161 this.date = null; 5162 else { 5163 if (this.date == null) 5164 this.date = new DateTimeType(); 5165 this.date.setValue(value); 5166 } 5167 return this; 5168 } 5169 5170 /** 5171 * @return {@link #publisher} (The name of the organization or individual that 5172 * published the value set.). This is the underlying object with id, 5173 * value and extensions. The accessor "getPublisher" gives direct access 5174 * to the value 5175 */ 5176 public StringType getPublisherElement() { 5177 if (this.publisher == null) 5178 if (Configuration.errorOnAutoCreate()) 5179 throw new Error("Attempt to auto-create ValueSet.publisher"); 5180 else if (Configuration.doAutoCreate()) 5181 this.publisher = new StringType(); // bb 5182 return this.publisher; 5183 } 5184 5185 public boolean hasPublisherElement() { 5186 return this.publisher != null && !this.publisher.isEmpty(); 5187 } 5188 5189 public boolean hasPublisher() { 5190 return this.publisher != null && !this.publisher.isEmpty(); 5191 } 5192 5193 /** 5194 * @param value {@link #publisher} (The name of the organization or individual 5195 * that published the value set.). This is the underlying object 5196 * with id, value and extensions. The accessor "getPublisher" gives 5197 * direct access to the value 5198 */ 5199 public ValueSet setPublisherElement(StringType value) { 5200 this.publisher = value; 5201 return this; 5202 } 5203 5204 /** 5205 * @return The name of the organization or individual that published the value 5206 * set. 5207 */ 5208 public String getPublisher() { 5209 return this.publisher == null ? null : this.publisher.getValue(); 5210 } 5211 5212 /** 5213 * @param value The name of the organization or individual that published the 5214 * value set. 5215 */ 5216 public ValueSet setPublisher(String value) { 5217 if (Utilities.noString(value)) 5218 this.publisher = null; 5219 else { 5220 if (this.publisher == null) 5221 this.publisher = new StringType(); 5222 this.publisher.setValue(value); 5223 } 5224 return this; 5225 } 5226 5227 /** 5228 * @return {@link #contact} (Contact details to assist a user in finding and 5229 * communicating with the publisher.) 5230 */ 5231 public List<ContactDetail> getContact() { 5232 if (this.contact == null) 5233 this.contact = new ArrayList<ContactDetail>(); 5234 return this.contact; 5235 } 5236 5237 /** 5238 * @return Returns a reference to <code>this</code> for easy method chaining 5239 */ 5240 public ValueSet setContact(List<ContactDetail> theContact) { 5241 this.contact = theContact; 5242 return this; 5243 } 5244 5245 public boolean hasContact() { 5246 if (this.contact == null) 5247 return false; 5248 for (ContactDetail item : this.contact) 5249 if (!item.isEmpty()) 5250 return true; 5251 return false; 5252 } 5253 5254 public ContactDetail addContact() { // 3 5255 ContactDetail t = new ContactDetail(); 5256 if (this.contact == null) 5257 this.contact = new ArrayList<ContactDetail>(); 5258 this.contact.add(t); 5259 return t; 5260 } 5261 5262 public ValueSet addContact(ContactDetail t) { // 3 5263 if (t == null) 5264 return this; 5265 if (this.contact == null) 5266 this.contact = new ArrayList<ContactDetail>(); 5267 this.contact.add(t); 5268 return this; 5269 } 5270 5271 /** 5272 * @return The first repetition of repeating field {@link #contact}, creating it 5273 * if it does not already exist 5274 */ 5275 public ContactDetail getContactFirstRep() { 5276 if (getContact().isEmpty()) { 5277 addContact(); 5278 } 5279 return getContact().get(0); 5280 } 5281 5282 /** 5283 * @return {@link #description} (A free text natural language description of the 5284 * value set from a consumer's perspective. The textual description 5285 * specifies the span of meanings for concepts to be included within the 5286 * Value Set Expansion, and also may specify the intended use and 5287 * limitations of the Value Set.). This is the underlying object with 5288 * id, value and extensions. The accessor "getDescription" gives direct 5289 * access to the value 5290 */ 5291 public MarkdownType getDescriptionElement() { 5292 if (this.description == null) 5293 if (Configuration.errorOnAutoCreate()) 5294 throw new Error("Attempt to auto-create ValueSet.description"); 5295 else if (Configuration.doAutoCreate()) 5296 this.description = new MarkdownType(); // bb 5297 return this.description; 5298 } 5299 5300 public boolean hasDescriptionElement() { 5301 return this.description != null && !this.description.isEmpty(); 5302 } 5303 5304 public boolean hasDescription() { 5305 return this.description != null && !this.description.isEmpty(); 5306 } 5307 5308 /** 5309 * @param value {@link #description} (A free text natural language description 5310 * of the value set from a consumer's perspective. The textual 5311 * description specifies the span of meanings for concepts to be 5312 * included within the Value Set Expansion, and also may specify 5313 * the intended use and limitations of the Value Set.). This is the 5314 * underlying object with id, value and extensions. The accessor 5315 * "getDescription" gives direct access to the value 5316 */ 5317 public ValueSet setDescriptionElement(MarkdownType value) { 5318 this.description = value; 5319 return this; 5320 } 5321 5322 /** 5323 * @return A free text natural language description of the value set from a 5324 * consumer's perspective. The textual description specifies the span of 5325 * meanings for concepts to be included within the Value Set Expansion, 5326 * and also may specify the intended use and limitations of the Value 5327 * Set. 5328 */ 5329 public String getDescription() { 5330 return this.description == null ? null : this.description.getValue(); 5331 } 5332 5333 /** 5334 * @param value A free text natural language description of the value set from a 5335 * consumer's perspective. The textual description specifies the 5336 * span of meanings for concepts to be included within the Value 5337 * Set Expansion, and also may specify the intended use and 5338 * limitations of the Value Set. 5339 */ 5340 public ValueSet setDescription(String value) { 5341 if (value == null) 5342 this.description = null; 5343 else { 5344 if (this.description == null) 5345 this.description = new MarkdownType(); 5346 this.description.setValue(value); 5347 } 5348 return this; 5349 } 5350 5351 /** 5352 * @return {@link #useContext} (The content was developed with a focus and 5353 * intent of supporting the contexts that are listed. These contexts may 5354 * be general categories (gender, age, ...) or may be references to 5355 * specific programs (insurance plans, studies, ...) and may be used to 5356 * assist with indexing and searching for appropriate value set 5357 * instances.) 5358 */ 5359 public List<UsageContext> getUseContext() { 5360 if (this.useContext == null) 5361 this.useContext = new ArrayList<UsageContext>(); 5362 return this.useContext; 5363 } 5364 5365 /** 5366 * @return Returns a reference to <code>this</code> for easy method chaining 5367 */ 5368 public ValueSet setUseContext(List<UsageContext> theUseContext) { 5369 this.useContext = theUseContext; 5370 return this; 5371 } 5372 5373 public boolean hasUseContext() { 5374 if (this.useContext == null) 5375 return false; 5376 for (UsageContext item : this.useContext) 5377 if (!item.isEmpty()) 5378 return true; 5379 return false; 5380 } 5381 5382 public UsageContext addUseContext() { // 3 5383 UsageContext t = new UsageContext(); 5384 if (this.useContext == null) 5385 this.useContext = new ArrayList<UsageContext>(); 5386 this.useContext.add(t); 5387 return t; 5388 } 5389 5390 public ValueSet addUseContext(UsageContext t) { // 3 5391 if (t == null) 5392 return this; 5393 if (this.useContext == null) 5394 this.useContext = new ArrayList<UsageContext>(); 5395 this.useContext.add(t); 5396 return this; 5397 } 5398 5399 /** 5400 * @return The first repetition of repeating field {@link #useContext}, creating 5401 * it if it does not already exist 5402 */ 5403 public UsageContext getUseContextFirstRep() { 5404 if (getUseContext().isEmpty()) { 5405 addUseContext(); 5406 } 5407 return getUseContext().get(0); 5408 } 5409 5410 /** 5411 * @return {@link #jurisdiction} (A legal or geographic region in which the 5412 * value set is intended to be used.) 5413 */ 5414 public List<CodeableConcept> getJurisdiction() { 5415 if (this.jurisdiction == null) 5416 this.jurisdiction = new ArrayList<CodeableConcept>(); 5417 return this.jurisdiction; 5418 } 5419 5420 /** 5421 * @return Returns a reference to <code>this</code> for easy method chaining 5422 */ 5423 public ValueSet setJurisdiction(List<CodeableConcept> theJurisdiction) { 5424 this.jurisdiction = theJurisdiction; 5425 return this; 5426 } 5427 5428 public boolean hasJurisdiction() { 5429 if (this.jurisdiction == null) 5430 return false; 5431 for (CodeableConcept item : this.jurisdiction) 5432 if (!item.isEmpty()) 5433 return true; 5434 return false; 5435 } 5436 5437 public CodeableConcept addJurisdiction() { // 3 5438 CodeableConcept t = new CodeableConcept(); 5439 if (this.jurisdiction == null) 5440 this.jurisdiction = new ArrayList<CodeableConcept>(); 5441 this.jurisdiction.add(t); 5442 return t; 5443 } 5444 5445 public ValueSet addJurisdiction(CodeableConcept t) { // 3 5446 if (t == null) 5447 return this; 5448 if (this.jurisdiction == null) 5449 this.jurisdiction = new ArrayList<CodeableConcept>(); 5450 this.jurisdiction.add(t); 5451 return this; 5452 } 5453 5454 /** 5455 * @return The first repetition of repeating field {@link #jurisdiction}, 5456 * creating it if it does not already exist 5457 */ 5458 public CodeableConcept getJurisdictionFirstRep() { 5459 if (getJurisdiction().isEmpty()) { 5460 addJurisdiction(); 5461 } 5462 return getJurisdiction().get(0); 5463 } 5464 5465 /** 5466 * @return {@link #immutable} (If this is set to 'true', then no new versions of 5467 * the content logical definition can be created. Note: Other metadata 5468 * might still change.). This is the underlying object with id, value 5469 * and extensions. The accessor "getImmutable" gives direct access to 5470 * the value 5471 */ 5472 public BooleanType getImmutableElement() { 5473 if (this.immutable == null) 5474 if (Configuration.errorOnAutoCreate()) 5475 throw new Error("Attempt to auto-create ValueSet.immutable"); 5476 else if (Configuration.doAutoCreate()) 5477 this.immutable = new BooleanType(); // bb 5478 return this.immutable; 5479 } 5480 5481 public boolean hasImmutableElement() { 5482 return this.immutable != null && !this.immutable.isEmpty(); 5483 } 5484 5485 public boolean hasImmutable() { 5486 return this.immutable != null && !this.immutable.isEmpty(); 5487 } 5488 5489 /** 5490 * @param value {@link #immutable} (If this is set to 'true', then no new 5491 * versions of the content logical definition can be created. Note: 5492 * Other metadata might still change.). This is the underlying 5493 * object with id, value and extensions. The accessor 5494 * "getImmutable" gives direct access to the value 5495 */ 5496 public ValueSet setImmutableElement(BooleanType value) { 5497 this.immutable = value; 5498 return this; 5499 } 5500 5501 /** 5502 * @return If this is set to 'true', then no new versions of the content logical 5503 * definition can be created. Note: Other metadata might still change. 5504 */ 5505 public boolean getImmutable() { 5506 return this.immutable == null || this.immutable.isEmpty() ? false : this.immutable.getValue(); 5507 } 5508 5509 /** 5510 * @param value If this is set to 'true', then no new versions of the content 5511 * logical definition can be created. Note: Other metadata might 5512 * still change. 5513 */ 5514 public ValueSet setImmutable(boolean value) { 5515 if (this.immutable == null) 5516 this.immutable = new BooleanType(); 5517 this.immutable.setValue(value); 5518 return this; 5519 } 5520 5521 /** 5522 * @return {@link #purpose} (Explanation of why this value set is needed and why 5523 * it has been designed as it has.). This is the underlying object with 5524 * id, value and extensions. The accessor "getPurpose" gives direct 5525 * access to the value 5526 */ 5527 public MarkdownType getPurposeElement() { 5528 if (this.purpose == null) 5529 if (Configuration.errorOnAutoCreate()) 5530 throw new Error("Attempt to auto-create ValueSet.purpose"); 5531 else if (Configuration.doAutoCreate()) 5532 this.purpose = new MarkdownType(); // bb 5533 return this.purpose; 5534 } 5535 5536 public boolean hasPurposeElement() { 5537 return this.purpose != null && !this.purpose.isEmpty(); 5538 } 5539 5540 public boolean hasPurpose() { 5541 return this.purpose != null && !this.purpose.isEmpty(); 5542 } 5543 5544 /** 5545 * @param value {@link #purpose} (Explanation of why this value set is needed 5546 * and why it has been designed as it has.). This is the underlying 5547 * object with id, value and extensions. The accessor "getPurpose" 5548 * gives direct access to the value 5549 */ 5550 public ValueSet setPurposeElement(MarkdownType value) { 5551 this.purpose = value; 5552 return this; 5553 } 5554 5555 /** 5556 * @return Explanation of why this value set is needed and why it has been 5557 * designed as it has. 5558 */ 5559 public String getPurpose() { 5560 return this.purpose == null ? null : this.purpose.getValue(); 5561 } 5562 5563 /** 5564 * @param value Explanation of why this value set is needed and why it has been 5565 * designed as it has. 5566 */ 5567 public ValueSet setPurpose(String value) { 5568 if (value == null) 5569 this.purpose = null; 5570 else { 5571 if (this.purpose == null) 5572 this.purpose = new MarkdownType(); 5573 this.purpose.setValue(value); 5574 } 5575 return this; 5576 } 5577 5578 /** 5579 * @return {@link #copyright} (A copyright statement relating to the value set 5580 * and/or its contents. Copyright statements are generally legal 5581 * restrictions on the use and publishing of the value set.). This is 5582 * the underlying object with id, value and extensions. The accessor 5583 * "getCopyright" gives direct access to the value 5584 */ 5585 public MarkdownType getCopyrightElement() { 5586 if (this.copyright == null) 5587 if (Configuration.errorOnAutoCreate()) 5588 throw new Error("Attempt to auto-create ValueSet.copyright"); 5589 else if (Configuration.doAutoCreate()) 5590 this.copyright = new MarkdownType(); // bb 5591 return this.copyright; 5592 } 5593 5594 public boolean hasCopyrightElement() { 5595 return this.copyright != null && !this.copyright.isEmpty(); 5596 } 5597 5598 public boolean hasCopyright() { 5599 return this.copyright != null && !this.copyright.isEmpty(); 5600 } 5601 5602 /** 5603 * @param value {@link #copyright} (A copyright statement relating to the value 5604 * set and/or its contents. Copyright statements are generally 5605 * legal restrictions on the use and publishing of the value set.). 5606 * This is the underlying object with id, value and extensions. The 5607 * accessor "getCopyright" gives direct access to the value 5608 */ 5609 public ValueSet setCopyrightElement(MarkdownType value) { 5610 this.copyright = value; 5611 return this; 5612 } 5613 5614 /** 5615 * @return A copyright statement relating to the value set and/or its contents. 5616 * Copyright statements are generally legal restrictions on the use and 5617 * publishing of the value set. 5618 */ 5619 public String getCopyright() { 5620 return this.copyright == null ? null : this.copyright.getValue(); 5621 } 5622 5623 /** 5624 * @param value A copyright statement relating to the value set and/or its 5625 * contents. Copyright statements are generally legal restrictions 5626 * on the use and publishing of the value set. 5627 */ 5628 public ValueSet setCopyright(String value) { 5629 if (value == null) 5630 this.copyright = null; 5631 else { 5632 if (this.copyright == null) 5633 this.copyright = new MarkdownType(); 5634 this.copyright.setValue(value); 5635 } 5636 return this; 5637 } 5638 5639 /** 5640 * @return {@link #compose} (A set of criteria that define the contents of the 5641 * value set by including or excluding codes selected from the specified 5642 * code system(s) that the value set draws from. This is also known as 5643 * the Content Logical Definition (CLD).) 5644 */ 5645 public ValueSetComposeComponent getCompose() { 5646 if (this.compose == null) 5647 if (Configuration.errorOnAutoCreate()) 5648 throw new Error("Attempt to auto-create ValueSet.compose"); 5649 else if (Configuration.doAutoCreate()) 5650 this.compose = new ValueSetComposeComponent(); // cc 5651 return this.compose; 5652 } 5653 5654 public boolean hasCompose() { 5655 return this.compose != null && !this.compose.isEmpty(); 5656 } 5657 5658 /** 5659 * @param value {@link #compose} (A set of criteria that define the contents of 5660 * the value set by including or excluding codes selected from the 5661 * specified code system(s) that the value set draws from. This is 5662 * also known as the Content Logical Definition (CLD).) 5663 */ 5664 public ValueSet setCompose(ValueSetComposeComponent value) { 5665 this.compose = value; 5666 return this; 5667 } 5668 5669 /** 5670 * @return {@link #expansion} (A value set can also be "expanded", where the 5671 * value set is turned into a simple collection of enumerated codes. 5672 * This element holds the expansion, if it has been performed.) 5673 */ 5674 public ValueSetExpansionComponent getExpansion() { 5675 if (this.expansion == null) 5676 if (Configuration.errorOnAutoCreate()) 5677 throw new Error("Attempt to auto-create ValueSet.expansion"); 5678 else if (Configuration.doAutoCreate()) 5679 this.expansion = new ValueSetExpansionComponent(); // cc 5680 return this.expansion; 5681 } 5682 5683 public boolean hasExpansion() { 5684 return this.expansion != null && !this.expansion.isEmpty(); 5685 } 5686 5687 /** 5688 * @param value {@link #expansion} (A value set can also be "expanded", where 5689 * the value set is turned into a simple collection of enumerated 5690 * codes. This element holds the expansion, if it has been 5691 * performed.) 5692 */ 5693 public ValueSet setExpansion(ValueSetExpansionComponent value) { 5694 this.expansion = value; 5695 return this; 5696 } 5697 5698 protected void listChildren(List<Property> children) { 5699 super.listChildren(children); 5700 children.add(new Property("url", "uri", 5701 "An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers.", 5702 0, 1, url)); 5703 children.add(new Property("identifier", "Identifier", 5704 "A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.", 5705 0, java.lang.Integer.MAX_VALUE, identifier)); 5706 children.add(new Property("version", "string", 5707 "The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 5708 0, 1, version)); 5709 children.add(new Property("name", "string", 5710 "A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 5711 0, 1, name)); 5712 children.add( 5713 new Property("title", "string", "A short, descriptive, user-friendly title for the value set.", 0, 1, title)); 5714 children.add(new Property("status", "code", 5715 "The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state.", 5716 0, 1, status)); 5717 children.add(new Property("experimental", "boolean", 5718 "A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 5719 0, 1, experimental)); 5720 children.add(new Property("date", "dateTime", 5721 "The date (and optionally time) when the value set was created or revised (e.g. the 'content logical definition').", 5722 0, 1, date)); 5723 children.add(new Property("publisher", "string", 5724 "The name of the organization or individual that published the value set.", 0, 1, publisher)); 5725 children.add(new Property("contact", "ContactDetail", 5726 "Contact details to assist a user in finding and communicating with the publisher.", 0, 5727 java.lang.Integer.MAX_VALUE, contact)); 5728 children.add(new Property("description", "markdown", 5729 "A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set.", 5730 0, 1, description)); 5731 children.add(new Property("useContext", "UsageContext", 5732 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate value set instances.", 5733 0, java.lang.Integer.MAX_VALUE, useContext)); 5734 children.add(new Property("jurisdiction", "CodeableConcept", 5735 "A legal or geographic region in which the value set is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 5736 jurisdiction)); 5737 children.add(new Property("immutable", "boolean", 5738 "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.", 5739 0, 1, immutable)); 5740 children.add(new Property("purpose", "markdown", 5741 "Explanation of why this value set is needed and why it has been designed as it has.", 0, 1, purpose)); 5742 children.add(new Property("copyright", "markdown", 5743 "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.", 5744 0, 1, copyright)); 5745 children.add(new Property("compose", "", 5746 "A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD).", 5747 0, 1, compose)); 5748 children.add(new Property("expansion", "", 5749 "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.", 5750 0, 1, expansion)); 5751 } 5752 5753 @Override 5754 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5755 switch (_hash) { 5756 case 116079: 5757 /* url */ return new Property("url", "uri", 5758 "An absolute URI that is used to identify this value set when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this value set is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the value set is stored on different servers.", 5759 0, 1, url); 5760 case -1618432855: 5761 /* identifier */ return new Property("identifier", "Identifier", 5762 "A formal identifier that is used to identify this value set when it is represented in other formats, or referenced in a specification, model, design or an instance.", 5763 0, java.lang.Integer.MAX_VALUE, identifier); 5764 case 351608024: 5765 /* version */ return new Property("version", "string", 5766 "The identifier that is used to identify this version of the value set when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the value set author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 5767 0, 1, version); 5768 case 3373707: 5769 /* name */ return new Property("name", "string", 5770 "A natural language name identifying the value set. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 5771 0, 1, name); 5772 case 110371416: 5773 /* title */ return new Property("title", "string", "A short, descriptive, user-friendly title for the value set.", 5774 0, 1, title); 5775 case -892481550: 5776 /* status */ return new Property("status", "code", 5777 "The status of this value set. Enables tracking the life-cycle of the content. The status of the value set applies to the value set definition (ValueSet.compose) and the associated ValueSet metadata. Expansions do not have a state.", 5778 0, 1, status); 5779 case -404562712: 5780 /* experimental */ return new Property("experimental", "boolean", 5781 "A Boolean value to indicate that this value set is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 5782 0, 1, experimental); 5783 case 3076014: 5784 /* date */ return new Property("date", "dateTime", 5785 "The date (and optionally time) when the value set was created or revised (e.g. the 'content logical definition').", 5786 0, 1, date); 5787 case 1447404028: 5788 /* publisher */ return new Property("publisher", "string", 5789 "The name of the organization or individual that published the value set.", 0, 1, publisher); 5790 case 951526432: 5791 /* contact */ return new Property("contact", "ContactDetail", 5792 "Contact details to assist a user in finding and communicating with the publisher.", 0, 5793 java.lang.Integer.MAX_VALUE, contact); 5794 case -1724546052: 5795 /* description */ return new Property("description", "markdown", 5796 "A free text natural language description of the value set from a consumer's perspective. The textual description specifies the span of meanings for concepts to be included within the Value Set Expansion, and also may specify the intended use and limitations of the Value Set.", 5797 0, 1, description); 5798 case -669707736: 5799 /* useContext */ return new Property("useContext", "UsageContext", 5800 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate value set instances.", 5801 0, java.lang.Integer.MAX_VALUE, useContext); 5802 case -507075711: 5803 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 5804 "A legal or geographic region in which the value set is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 5805 jurisdiction); 5806 case 1596987778: 5807 /* immutable */ return new Property("immutable", "boolean", 5808 "If this is set to 'true', then no new versions of the content logical definition can be created. Note: Other metadata might still change.", 5809 0, 1, immutable); 5810 case -220463842: 5811 /* purpose */ return new Property("purpose", "markdown", 5812 "Explanation of why this value set is needed and why it has been designed as it has.", 0, 1, purpose); 5813 case 1522889671: 5814 /* copyright */ return new Property("copyright", "markdown", 5815 "A copyright statement relating to the value set and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the value set.", 5816 0, 1, copyright); 5817 case 950497682: 5818 /* compose */ return new Property("compose", "", 5819 "A set of criteria that define the contents of the value set by including or excluding codes selected from the specified code system(s) that the value set draws from. This is also known as the Content Logical Definition (CLD).", 5820 0, 1, compose); 5821 case 17878207: 5822 /* expansion */ return new Property("expansion", "", 5823 "A value set can also be \"expanded\", where the value set is turned into a simple collection of enumerated codes. This element holds the expansion, if it has been performed.", 5824 0, 1, expansion); 5825 default: 5826 return super.getNamedProperty(_hash, _name, _checkValid); 5827 } 5828 5829 } 5830 5831 @Override 5832 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5833 switch (hash) { 5834 case 116079: 5835 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5836 case -1618432855: 5837 /* identifier */ return this.identifier == null ? new Base[0] 5838 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5839 case 351608024: 5840 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 5841 case 3373707: 5842 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5843 case 110371416: 5844 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5845 case -892481550: 5846 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 5847 case -404562712: 5848 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 5849 case 3076014: 5850 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 5851 case 1447404028: 5852 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 5853 case 951526432: 5854 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5855 case -1724546052: 5856 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5857 case -669707736: 5858 /* useContext */ return this.useContext == null ? new Base[0] 5859 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5860 case -507075711: 5861 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5862 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5863 case 1596987778: 5864 /* immutable */ return this.immutable == null ? new Base[0] : new Base[] { this.immutable }; // BooleanType 5865 case -220463842: 5866 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 5867 case 1522889671: 5868 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5869 case 950497682: 5870 /* compose */ return this.compose == null ? new Base[0] : new Base[] { this.compose }; // ValueSetComposeComponent 5871 case 17878207: 5872 /* expansion */ return this.expansion == null ? new Base[0] : new Base[] { this.expansion }; // ValueSetExpansionComponent 5873 default: 5874 return super.getProperty(hash, name, checkValid); 5875 } 5876 5877 } 5878 5879 @Override 5880 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5881 switch (hash) { 5882 case 116079: // url 5883 this.url = castToUri(value); // UriType 5884 return value; 5885 case -1618432855: // identifier 5886 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5887 return value; 5888 case 351608024: // version 5889 this.version = castToString(value); // StringType 5890 return value; 5891 case 3373707: // name 5892 this.name = castToString(value); // StringType 5893 return value; 5894 case 110371416: // title 5895 this.title = castToString(value); // StringType 5896 return value; 5897 case -892481550: // status 5898 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5899 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5900 return value; 5901 case -404562712: // experimental 5902 this.experimental = castToBoolean(value); // BooleanType 5903 return value; 5904 case 3076014: // date 5905 this.date = castToDateTime(value); // DateTimeType 5906 return value; 5907 case 1447404028: // publisher 5908 this.publisher = castToString(value); // StringType 5909 return value; 5910 case 951526432: // contact 5911 this.getContact().add(castToContactDetail(value)); // ContactDetail 5912 return value; 5913 case -1724546052: // description 5914 this.description = castToMarkdown(value); // MarkdownType 5915 return value; 5916 case -669707736: // useContext 5917 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5918 return value; 5919 case -507075711: // jurisdiction 5920 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5921 return value; 5922 case 1596987778: // immutable 5923 this.immutable = castToBoolean(value); // BooleanType 5924 return value; 5925 case -220463842: // purpose 5926 this.purpose = castToMarkdown(value); // MarkdownType 5927 return value; 5928 case 1522889671: // copyright 5929 this.copyright = castToMarkdown(value); // MarkdownType 5930 return value; 5931 case 950497682: // compose 5932 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 5933 return value; 5934 case 17878207: // expansion 5935 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 5936 return value; 5937 default: 5938 return super.setProperty(hash, name, value); 5939 } 5940 5941 } 5942 5943 @Override 5944 public Base setProperty(String name, Base value) throws FHIRException { 5945 if (name.equals("url")) { 5946 this.url = castToUri(value); // UriType 5947 } else if (name.equals("identifier")) { 5948 this.getIdentifier().add(castToIdentifier(value)); 5949 } else if (name.equals("version")) { 5950 this.version = castToString(value); // StringType 5951 } else if (name.equals("name")) { 5952 this.name = castToString(value); // StringType 5953 } else if (name.equals("title")) { 5954 this.title = castToString(value); // StringType 5955 } else if (name.equals("status")) { 5956 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5957 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5958 } else if (name.equals("experimental")) { 5959 this.experimental = castToBoolean(value); // BooleanType 5960 } else if (name.equals("date")) { 5961 this.date = castToDateTime(value); // DateTimeType 5962 } else if (name.equals("publisher")) { 5963 this.publisher = castToString(value); // StringType 5964 } else if (name.equals("contact")) { 5965 this.getContact().add(castToContactDetail(value)); 5966 } else if (name.equals("description")) { 5967 this.description = castToMarkdown(value); // MarkdownType 5968 } else if (name.equals("useContext")) { 5969 this.getUseContext().add(castToUsageContext(value)); 5970 } else if (name.equals("jurisdiction")) { 5971 this.getJurisdiction().add(castToCodeableConcept(value)); 5972 } else if (name.equals("immutable")) { 5973 this.immutable = castToBoolean(value); // BooleanType 5974 } else if (name.equals("purpose")) { 5975 this.purpose = castToMarkdown(value); // MarkdownType 5976 } else if (name.equals("copyright")) { 5977 this.copyright = castToMarkdown(value); // MarkdownType 5978 } else if (name.equals("compose")) { 5979 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 5980 } else if (name.equals("expansion")) { 5981 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 5982 } else 5983 return super.setProperty(name, value); 5984 return value; 5985 } 5986 5987 @Override 5988 public void removeChild(String name, Base value) throws FHIRException { 5989 if (name.equals("url")) { 5990 this.url = null; 5991 } else if (name.equals("identifier")) { 5992 this.getIdentifier().remove(castToIdentifier(value)); 5993 } else if (name.equals("version")) { 5994 this.version = null; 5995 } else if (name.equals("name")) { 5996 this.name = null; 5997 } else if (name.equals("title")) { 5998 this.title = null; 5999 } else if (name.equals("status")) { 6000 this.status = null; 6001 } else if (name.equals("experimental")) { 6002 this.experimental = null; 6003 } else if (name.equals("date")) { 6004 this.date = null; 6005 } else if (name.equals("publisher")) { 6006 this.publisher = null; 6007 } else if (name.equals("contact")) { 6008 this.getContact().remove(castToContactDetail(value)); 6009 } else if (name.equals("description")) { 6010 this.description = null; 6011 } else if (name.equals("useContext")) { 6012 this.getUseContext().remove(castToUsageContext(value)); 6013 } else if (name.equals("jurisdiction")) { 6014 this.getJurisdiction().remove(castToCodeableConcept(value)); 6015 } else if (name.equals("immutable")) { 6016 this.immutable = null; 6017 } else if (name.equals("purpose")) { 6018 this.purpose = null; 6019 } else if (name.equals("copyright")) { 6020 this.copyright = null; 6021 } else if (name.equals("compose")) { 6022 this.compose = (ValueSetComposeComponent) value; // ValueSetComposeComponent 6023 } else if (name.equals("expansion")) { 6024 this.expansion = (ValueSetExpansionComponent) value; // ValueSetExpansionComponent 6025 } else 6026 super.removeChild(name, value); 6027 6028 } 6029 6030 @Override 6031 public Base makeProperty(int hash, String name) throws FHIRException { 6032 switch (hash) { 6033 case 116079: 6034 return getUrlElement(); 6035 case -1618432855: 6036 return addIdentifier(); 6037 case 351608024: 6038 return getVersionElement(); 6039 case 3373707: 6040 return getNameElement(); 6041 case 110371416: 6042 return getTitleElement(); 6043 case -892481550: 6044 return getStatusElement(); 6045 case -404562712: 6046 return getExperimentalElement(); 6047 case 3076014: 6048 return getDateElement(); 6049 case 1447404028: 6050 return getPublisherElement(); 6051 case 951526432: 6052 return addContact(); 6053 case -1724546052: 6054 return getDescriptionElement(); 6055 case -669707736: 6056 return addUseContext(); 6057 case -507075711: 6058 return addJurisdiction(); 6059 case 1596987778: 6060 return getImmutableElement(); 6061 case -220463842: 6062 return getPurposeElement(); 6063 case 1522889671: 6064 return getCopyrightElement(); 6065 case 950497682: 6066 return getCompose(); 6067 case 17878207: 6068 return getExpansion(); 6069 default: 6070 return super.makeProperty(hash, name); 6071 } 6072 6073 } 6074 6075 @Override 6076 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6077 switch (hash) { 6078 case 116079: 6079 /* url */ return new String[] { "uri" }; 6080 case -1618432855: 6081 /* identifier */ return new String[] { "Identifier" }; 6082 case 351608024: 6083 /* version */ return new String[] { "string" }; 6084 case 3373707: 6085 /* name */ return new String[] { "string" }; 6086 case 110371416: 6087 /* title */ return new String[] { "string" }; 6088 case -892481550: 6089 /* status */ return new String[] { "code" }; 6090 case -404562712: 6091 /* experimental */ return new String[] { "boolean" }; 6092 case 3076014: 6093 /* date */ return new String[] { "dateTime" }; 6094 case 1447404028: 6095 /* publisher */ return new String[] { "string" }; 6096 case 951526432: 6097 /* contact */ return new String[] { "ContactDetail" }; 6098 case -1724546052: 6099 /* description */ return new String[] { "markdown" }; 6100 case -669707736: 6101 /* useContext */ return new String[] { "UsageContext" }; 6102 case -507075711: 6103 /* jurisdiction */ return new String[] { "CodeableConcept" }; 6104 case 1596987778: 6105 /* immutable */ return new String[] { "boolean" }; 6106 case -220463842: 6107 /* purpose */ return new String[] { "markdown" }; 6108 case 1522889671: 6109 /* copyright */ return new String[] { "markdown" }; 6110 case 950497682: 6111 /* compose */ return new String[] {}; 6112 case 17878207: 6113 /* expansion */ return new String[] {}; 6114 default: 6115 return super.getTypesForProperty(hash, name); 6116 } 6117 6118 } 6119 6120 @Override 6121 public Base addChild(String name) throws FHIRException { 6122 if (name.equals("url")) { 6123 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.url"); 6124 } else if (name.equals("identifier")) { 6125 return addIdentifier(); 6126 } else if (name.equals("version")) { 6127 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.version"); 6128 } else if (name.equals("name")) { 6129 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.name"); 6130 } else if (name.equals("title")) { 6131 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.title"); 6132 } else if (name.equals("status")) { 6133 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.status"); 6134 } else if (name.equals("experimental")) { 6135 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.experimental"); 6136 } else if (name.equals("date")) { 6137 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.date"); 6138 } else if (name.equals("publisher")) { 6139 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.publisher"); 6140 } else if (name.equals("contact")) { 6141 return addContact(); 6142 } else if (name.equals("description")) { 6143 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.description"); 6144 } else if (name.equals("useContext")) { 6145 return addUseContext(); 6146 } else if (name.equals("jurisdiction")) { 6147 return addJurisdiction(); 6148 } else if (name.equals("immutable")) { 6149 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.immutable"); 6150 } else if (name.equals("purpose")) { 6151 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.purpose"); 6152 } else if (name.equals("copyright")) { 6153 throw new FHIRException("Cannot call addChild on a singleton property ValueSet.copyright"); 6154 } else if (name.equals("compose")) { 6155 this.compose = new ValueSetComposeComponent(); 6156 return this.compose; 6157 } else if (name.equals("expansion")) { 6158 this.expansion = new ValueSetExpansionComponent(); 6159 return this.expansion; 6160 } else 6161 return super.addChild(name); 6162 } 6163 6164 public String fhirType() { 6165 return "ValueSet"; 6166 6167 } 6168 6169 public ValueSet copy() { 6170 ValueSet dst = new ValueSet(); 6171 copyValues(dst); 6172 return dst; 6173 } 6174 6175 public void copyValues(ValueSet dst) { 6176 super.copyValues(dst); 6177 dst.url = url == null ? null : url.copy(); 6178 if (identifier != null) { 6179 dst.identifier = new ArrayList<Identifier>(); 6180 for (Identifier i : identifier) 6181 dst.identifier.add(i.copy()); 6182 } 6183 ; 6184 dst.version = version == null ? null : version.copy(); 6185 dst.name = name == null ? null : name.copy(); 6186 dst.title = title == null ? null : title.copy(); 6187 dst.status = status == null ? null : status.copy(); 6188 dst.experimental = experimental == null ? null : experimental.copy(); 6189 dst.date = date == null ? null : date.copy(); 6190 dst.publisher = publisher == null ? null : publisher.copy(); 6191 if (contact != null) { 6192 dst.contact = new ArrayList<ContactDetail>(); 6193 for (ContactDetail i : contact) 6194 dst.contact.add(i.copy()); 6195 } 6196 ; 6197 dst.description = description == null ? null : description.copy(); 6198 if (useContext != null) { 6199 dst.useContext = new ArrayList<UsageContext>(); 6200 for (UsageContext i : useContext) 6201 dst.useContext.add(i.copy()); 6202 } 6203 ; 6204 if (jurisdiction != null) { 6205 dst.jurisdiction = new ArrayList<CodeableConcept>(); 6206 for (CodeableConcept i : jurisdiction) 6207 dst.jurisdiction.add(i.copy()); 6208 } 6209 ; 6210 dst.immutable = immutable == null ? null : immutable.copy(); 6211 dst.purpose = purpose == null ? null : purpose.copy(); 6212 dst.copyright = copyright == null ? null : copyright.copy(); 6213 dst.compose = compose == null ? null : compose.copy(); 6214 dst.expansion = expansion == null ? null : expansion.copy(); 6215 } 6216 6217 protected ValueSet typedCopy() { 6218 return copy(); 6219 } 6220 6221 @Override 6222 public boolean equalsDeep(Base other_) { 6223 if (!super.equalsDeep(other_)) 6224 return false; 6225 if (!(other_ instanceof ValueSet)) 6226 return false; 6227 ValueSet o = (ValueSet) other_; 6228 return compareDeep(identifier, o.identifier, true) && compareDeep(immutable, o.immutable, true) 6229 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 6230 && compareDeep(compose, o.compose, true) && compareDeep(expansion, o.expansion, true); 6231 } 6232 6233 @Override 6234 public boolean equalsShallow(Base other_) { 6235 if (!super.equalsShallow(other_)) 6236 return false; 6237 if (!(other_ instanceof ValueSet)) 6238 return false; 6239 ValueSet o = (ValueSet) other_; 6240 return compareValues(immutable, o.immutable, true) && compareValues(purpose, o.purpose, true) 6241 && compareValues(copyright, o.copyright, true); 6242 } 6243 6244 public boolean isEmpty() { 6245 return super.isEmpty() 6246 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, immutable, purpose, copyright, compose, expansion); 6247 } 6248 6249 @Override 6250 public ResourceType getResourceType() { 6251 return ResourceType.ValueSet; 6252 } 6253 6254 6255 6256 /** 6257 * Search parameter: <b>date</b> 6258 * <p> 6259 * Description: <b>The value set publication date</b><br> 6260 * Type: <b>date</b><br> 6261 * Path: <b>ValueSet.date</b><br> 6262 * </p> 6263 */ 6264 @SearchParamDefinition(name = "date", path = "ValueSet.date", description = "The value set publication date", type = "date") 6265 public static final String SP_DATE = "date"; 6266 /** 6267 * <b>Fluent Client</b> search parameter constant for <b>date</b> 6268 * <p> 6269 * Description: <b>The value set publication date</b><br> 6270 * Type: <b>date</b><br> 6271 * Path: <b>ValueSet.date</b><br> 6272 * </p> 6273 */ 6274 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6275 SP_DATE); 6276 6277 /** 6278 * Search parameter: <b>identifier</b> 6279 * <p> 6280 * Description: <b>External identifier for the value set</b><br> 6281 * Type: <b>token</b><br> 6282 * Path: <b>ValueSet.identifier</b><br> 6283 * </p> 6284 */ 6285 @SearchParamDefinition(name = "identifier", path = "ValueSet.identifier", description = "External identifier for the value set", type = "token") 6286 public static final String SP_IDENTIFIER = "identifier"; 6287 /** 6288 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6289 * <p> 6290 * Description: <b>External identifier for the value set</b><br> 6291 * Type: <b>token</b><br> 6292 * Path: <b>ValueSet.identifier</b><br> 6293 * </p> 6294 */ 6295 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6296 SP_IDENTIFIER); 6297 6298 /** 6299 * Search parameter: <b>code</b> 6300 * <p> 6301 * Description: <b>This special parameter searches for codes in the value set. 6302 * See additional notes on the ValueSet resource</b><br> 6303 * Type: <b>token</b><br> 6304 * Path: <b>ValueSet.expansion.contains.code, 6305 * ValueSet.compose.include.concept.code</b><br> 6306 * </p> 6307 */ 6308 @SearchParamDefinition(name = "code", path = "ValueSet.expansion.contains.code | ValueSet.compose.include.concept.code", description = "This special parameter searches for codes in the value set. See additional notes on the ValueSet resource", type = "token") 6309 public static final String SP_CODE = "code"; 6310 /** 6311 * <b>Fluent Client</b> search parameter constant for <b>code</b> 6312 * <p> 6313 * Description: <b>This special parameter searches for codes in the value set. 6314 * See additional notes on the ValueSet resource</b><br> 6315 * Type: <b>token</b><br> 6316 * Path: <b>ValueSet.expansion.contains.code, 6317 * ValueSet.compose.include.concept.code</b><br> 6318 * </p> 6319 */ 6320 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6321 SP_CODE); 6322 6323 /** 6324 * Search parameter: <b>context-type-value</b> 6325 * <p> 6326 * Description: <b>A use context type and value assigned to the value 6327 * set</b><br> 6328 * Type: <b>composite</b><br> 6329 * Path: <b></b><br> 6330 * </p> 6331 */ 6332 @SearchParamDefinition(name = "context-type-value", path = "ValueSet.useContext", description = "A use context type and value assigned to the value set", type = "composite", compositeOf = { 6333 "context-type", "context" }) 6334 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 6335 /** 6336 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 6337 * <p> 6338 * Description: <b>A use context type and value assigned to the value 6339 * set</b><br> 6340 * Type: <b>composite</b><br> 6341 * Path: <b></b><br> 6342 * </p> 6343 */ 6344 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 6345 SP_CONTEXT_TYPE_VALUE); 6346 6347 /** 6348 * Search parameter: <b>jurisdiction</b> 6349 * <p> 6350 * Description: <b>Intended jurisdiction for the value set</b><br> 6351 * Type: <b>token</b><br> 6352 * Path: <b>ValueSet.jurisdiction</b><br> 6353 * </p> 6354 */ 6355 @SearchParamDefinition(name = "jurisdiction", path = "ValueSet.jurisdiction", description = "Intended jurisdiction for the value set", type = "token") 6356 public static final String SP_JURISDICTION = "jurisdiction"; 6357 /** 6358 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6359 * <p> 6360 * Description: <b>Intended jurisdiction for the value set</b><br> 6361 * Type: <b>token</b><br> 6362 * Path: <b>ValueSet.jurisdiction</b><br> 6363 * </p> 6364 */ 6365 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6366 SP_JURISDICTION); 6367 6368 /** 6369 * Search parameter: <b>description</b> 6370 * <p> 6371 * Description: <b>The description of the value set</b><br> 6372 * Type: <b>string</b><br> 6373 * Path: <b>ValueSet.description</b><br> 6374 * </p> 6375 */ 6376 @SearchParamDefinition(name = "description", path = "ValueSet.description", description = "The description of the value set", type = "string") 6377 public static final String SP_DESCRIPTION = "description"; 6378 /** 6379 * <b>Fluent Client</b> search parameter constant for <b>description</b> 6380 * <p> 6381 * Description: <b>The description of the value set</b><br> 6382 * Type: <b>string</b><br> 6383 * Path: <b>ValueSet.description</b><br> 6384 * </p> 6385 */ 6386 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 6387 SP_DESCRIPTION); 6388 6389 /** 6390 * Search parameter: <b>context-type</b> 6391 * <p> 6392 * Description: <b>A type of use context assigned to the value set</b><br> 6393 * Type: <b>token</b><br> 6394 * Path: <b>ValueSet.useContext.code</b><br> 6395 * </p> 6396 */ 6397 @SearchParamDefinition(name = "context-type", path = "ValueSet.useContext.code", description = "A type of use context assigned to the value set", type = "token") 6398 public static final String SP_CONTEXT_TYPE = "context-type"; 6399 /** 6400 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 6401 * <p> 6402 * Description: <b>A type of use context assigned to the value set</b><br> 6403 * Type: <b>token</b><br> 6404 * Path: <b>ValueSet.useContext.code</b><br> 6405 * </p> 6406 */ 6407 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6408 SP_CONTEXT_TYPE); 6409 6410 /** 6411 * Search parameter: <b>title</b> 6412 * <p> 6413 * Description: <b>The human-friendly name of the value set</b><br> 6414 * Type: <b>string</b><br> 6415 * Path: <b>ValueSet.title</b><br> 6416 * </p> 6417 */ 6418 @SearchParamDefinition(name = "title", path = "ValueSet.title", description = "The human-friendly name of the value set", type = "string") 6419 public static final String SP_TITLE = "title"; 6420 /** 6421 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6422 * <p> 6423 * Description: <b>The human-friendly name of the value set</b><br> 6424 * Type: <b>string</b><br> 6425 * Path: <b>ValueSet.title</b><br> 6426 * </p> 6427 */ 6428 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 6429 SP_TITLE); 6430 6431 /** 6432 * Search parameter: <b>version</b> 6433 * <p> 6434 * Description: <b>The business version of the value set</b><br> 6435 * Type: <b>token</b><br> 6436 * Path: <b>ValueSet.version</b><br> 6437 * </p> 6438 */ 6439 @SearchParamDefinition(name = "version", path = "ValueSet.version", description = "The business version of the value set", type = "token") 6440 public static final String SP_VERSION = "version"; 6441 /** 6442 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6443 * <p> 6444 * Description: <b>The business version of the value set</b><br> 6445 * Type: <b>token</b><br> 6446 * Path: <b>ValueSet.version</b><br> 6447 * </p> 6448 */ 6449 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6450 SP_VERSION); 6451 6452 /** 6453 * Search parameter: <b>url</b> 6454 * <p> 6455 * Description: <b>The uri that identifies the value set</b><br> 6456 * Type: <b>uri</b><br> 6457 * Path: <b>ValueSet.url</b><br> 6458 * </p> 6459 */ 6460 @SearchParamDefinition(name = "url", path = "ValueSet.url", description = "The uri that identifies the value set", type = "uri") 6461 public static final String SP_URL = "url"; 6462 /** 6463 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6464 * <p> 6465 * Description: <b>The uri that identifies the value set</b><br> 6466 * Type: <b>uri</b><br> 6467 * Path: <b>ValueSet.url</b><br> 6468 * </p> 6469 */ 6470 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6471 6472 /** 6473 * Search parameter: <b>expansion</b> 6474 * <p> 6475 * Description: <b>Identifies the value set expansion (business 6476 * identifier)</b><br> 6477 * Type: <b>uri</b><br> 6478 * Path: <b>ValueSet.expansion.identifier</b><br> 6479 * </p> 6480 */ 6481 @SearchParamDefinition(name = "expansion", path = "ValueSet.expansion.identifier", description = "Identifies the value set expansion (business identifier)", type = "uri") 6482 public static final String SP_EXPANSION = "expansion"; 6483 /** 6484 * <b>Fluent Client</b> search parameter constant for <b>expansion</b> 6485 * <p> 6486 * Description: <b>Identifies the value set expansion (business 6487 * identifier)</b><br> 6488 * Type: <b>uri</b><br> 6489 * Path: <b>ValueSet.expansion.identifier</b><br> 6490 * </p> 6491 */ 6492 public static final ca.uhn.fhir.rest.gclient.UriClientParam EXPANSION = new ca.uhn.fhir.rest.gclient.UriClientParam( 6493 SP_EXPANSION); 6494 6495 /** 6496 * Search parameter: <b>reference</b> 6497 * <p> 6498 * Description: <b>A code system included or excluded in the value set or an 6499 * imported value set</b><br> 6500 * Type: <b>uri</b><br> 6501 * Path: <b>ValueSet.compose.include.system</b><br> 6502 * </p> 6503 */ 6504 @SearchParamDefinition(name = "reference", path = "ValueSet.compose.include.system", description = "A code system included or excluded in the value set or an imported value set", type = "uri") 6505 public static final String SP_REFERENCE = "reference"; 6506 /** 6507 * <b>Fluent Client</b> search parameter constant for <b>reference</b> 6508 * <p> 6509 * Description: <b>A code system included or excluded in the value set or an 6510 * imported value set</b><br> 6511 * Type: <b>uri</b><br> 6512 * Path: <b>ValueSet.compose.include.system</b><br> 6513 * </p> 6514 */ 6515 public static final ca.uhn.fhir.rest.gclient.UriClientParam REFERENCE = new ca.uhn.fhir.rest.gclient.UriClientParam( 6516 SP_REFERENCE); 6517 6518 /** 6519 * Search parameter: <b>context-quantity</b> 6520 * <p> 6521 * Description: <b>A quantity- or range-valued use context assigned to the value 6522 * set</b><br> 6523 * Type: <b>quantity</b><br> 6524 * Path: <b>ValueSet.useContext.valueQuantity, 6525 * ValueSet.useContext.valueRange</b><br> 6526 * </p> 6527 */ 6528 @SearchParamDefinition(name = "context-quantity", path = "(ValueSet.useContext.value as Quantity) | (ValueSet.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the value set", type = "quantity") 6529 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6530 /** 6531 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6532 * <p> 6533 * Description: <b>A quantity- or range-valued use context assigned to the value 6534 * set</b><br> 6535 * Type: <b>quantity</b><br> 6536 * Path: <b>ValueSet.useContext.valueQuantity, 6537 * ValueSet.useContext.valueRange</b><br> 6538 * </p> 6539 */ 6540 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 6541 SP_CONTEXT_QUANTITY); 6542 6543 /** 6544 * Search parameter: <b>name</b> 6545 * <p> 6546 * Description: <b>Computationally friendly name of the value set</b><br> 6547 * Type: <b>string</b><br> 6548 * Path: <b>ValueSet.name</b><br> 6549 * </p> 6550 */ 6551 @SearchParamDefinition(name = "name", path = "ValueSet.name", description = "Computationally friendly name of the value set", type = "string") 6552 public static final String SP_NAME = "name"; 6553 /** 6554 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6555 * <p> 6556 * Description: <b>Computationally friendly name of the value set</b><br> 6557 * Type: <b>string</b><br> 6558 * Path: <b>ValueSet.name</b><br> 6559 * </p> 6560 */ 6561 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 6562 SP_NAME); 6563 6564 /** 6565 * Search parameter: <b>context</b> 6566 * <p> 6567 * Description: <b>A use context assigned to the value set</b><br> 6568 * Type: <b>token</b><br> 6569 * Path: <b>ValueSet.useContext.valueCodeableConcept</b><br> 6570 * </p> 6571 */ 6572 @SearchParamDefinition(name = "context", path = "(ValueSet.useContext.value as CodeableConcept)", description = "A use context assigned to the value set", type = "token") 6573 public static final String SP_CONTEXT = "context"; 6574 /** 6575 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6576 * <p> 6577 * Description: <b>A use context assigned to the value set</b><br> 6578 * Type: <b>token</b><br> 6579 * Path: <b>ValueSet.useContext.valueCodeableConcept</b><br> 6580 * </p> 6581 */ 6582 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6583 SP_CONTEXT); 6584 6585 /** 6586 * Search parameter: <b>publisher</b> 6587 * <p> 6588 * Description: <b>Name of the publisher of the value set</b><br> 6589 * Type: <b>string</b><br> 6590 * Path: <b>ValueSet.publisher</b><br> 6591 * </p> 6592 */ 6593 @SearchParamDefinition(name = "publisher", path = "ValueSet.publisher", description = "Name of the publisher of the value set", type = "string") 6594 public static final String SP_PUBLISHER = "publisher"; 6595 /** 6596 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6597 * <p> 6598 * Description: <b>Name of the publisher of the value set</b><br> 6599 * Type: <b>string</b><br> 6600 * Path: <b>ValueSet.publisher</b><br> 6601 * </p> 6602 */ 6603 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 6604 SP_PUBLISHER); 6605 6606 /** 6607 * Search parameter: <b>context-type-quantity</b> 6608 * <p> 6609 * Description: <b>A use context type and quantity- or range-based value 6610 * assigned to the value set</b><br> 6611 * Type: <b>composite</b><br> 6612 * Path: <b></b><br> 6613 * </p> 6614 */ 6615 @SearchParamDefinition(name = "context-type-quantity", path = "ValueSet.useContext", description = "A use context type and quantity- or range-based value assigned to the value set", type = "composite", compositeOf = { 6616 "context-type", "context-quantity" }) 6617 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6618 /** 6619 * <b>Fluent Client</b> search parameter constant for 6620 * <b>context-type-quantity</b> 6621 * <p> 6622 * Description: <b>A use context type and quantity- or range-based value 6623 * assigned to the value set</b><br> 6624 * Type: <b>composite</b><br> 6625 * Path: <b></b><br> 6626 * </p> 6627 */ 6628 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 6629 SP_CONTEXT_TYPE_QUANTITY); 6630 6631 /** 6632 * Search parameter: <b>status</b> 6633 * <p> 6634 * Description: <b>The current status of the value set</b><br> 6635 * Type: <b>token</b><br> 6636 * Path: <b>ValueSet.status</b><br> 6637 * </p> 6638 */ 6639 @SearchParamDefinition(name = "status", path = "ValueSet.status", description = "The current status of the value set", type = "token") 6640 public static final String SP_STATUS = "status"; 6641 /** 6642 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6643 * <p> 6644 * Description: <b>The current status of the value set</b><br> 6645 * Type: <b>token</b><br> 6646 * Path: <b>ValueSet.status</b><br> 6647 * </p> 6648 */ 6649 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6650 SP_STATUS); 6651 6652}