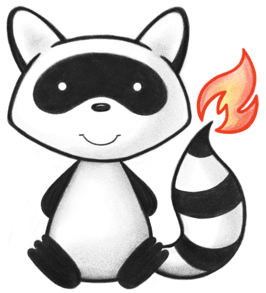
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Describes validation requirements, source(s), status and dates for one or 049 * more elements. 050 */ 051@ResourceDef(name = "VerificationResult", profile = "http://hl7.org/fhir/StructureDefinition/VerificationResult") 052public class VerificationResult extends DomainResource { 053 054 public enum Status { 055 /** 056 * ***TODO*** 057 */ 058 ATTESTED, 059 /** 060 * ***TODO*** 061 */ 062 VALIDATED, 063 /** 064 * ***TODO*** 065 */ 066 INPROCESS, 067 /** 068 * ***TODO*** 069 */ 070 REQREVALID, 071 /** 072 * ***TODO*** 073 */ 074 VALFAIL, 075 /** 076 * ***TODO*** 077 */ 078 REVALFAIL, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 084 public static Status fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("attested".equals(codeString)) 088 return ATTESTED; 089 if ("validated".equals(codeString)) 090 return VALIDATED; 091 if ("in-process".equals(codeString)) 092 return INPROCESS; 093 if ("req-revalid".equals(codeString)) 094 return REQREVALID; 095 if ("val-fail".equals(codeString)) 096 return VALFAIL; 097 if ("reval-fail".equals(codeString)) 098 return REVALFAIL; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown Status code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case ATTESTED: 108 return "attested"; 109 case VALIDATED: 110 return "validated"; 111 case INPROCESS: 112 return "in-process"; 113 case REQREVALID: 114 return "req-revalid"; 115 case VALFAIL: 116 return "val-fail"; 117 case REVALFAIL: 118 return "reval-fail"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getSystem() { 127 switch (this) { 128 case ATTESTED: 129 return "http://hl7.org/fhir/CodeSystem/status"; 130 case VALIDATED: 131 return "http://hl7.org/fhir/CodeSystem/status"; 132 case INPROCESS: 133 return "http://hl7.org/fhir/CodeSystem/status"; 134 case REQREVALID: 135 return "http://hl7.org/fhir/CodeSystem/status"; 136 case VALFAIL: 137 return "http://hl7.org/fhir/CodeSystem/status"; 138 case REVALFAIL: 139 return "http://hl7.org/fhir/CodeSystem/status"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case ATTESTED: 150 return "***TODO***"; 151 case VALIDATED: 152 return "***TODO***"; 153 case INPROCESS: 154 return "***TODO***"; 155 case REQREVALID: 156 return "***TODO***"; 157 case VALFAIL: 158 return "***TODO***"; 159 case REVALFAIL: 160 return "***TODO***"; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDisplay() { 169 switch (this) { 170 case ATTESTED: 171 return "Attested"; 172 case VALIDATED: 173 return "Validated"; 174 case INPROCESS: 175 return "In process"; 176 case REQREVALID: 177 return "Requires revalidation"; 178 case VALFAIL: 179 return "Validation failed"; 180 case REVALFAIL: 181 return "Re-Validation failed"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 } 189 190 public static class StatusEnumFactory implements EnumFactory<Status> { 191 public Status fromCode(String codeString) throws IllegalArgumentException { 192 if (codeString == null || "".equals(codeString)) 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("attested".equals(codeString)) 196 return Status.ATTESTED; 197 if ("validated".equals(codeString)) 198 return Status.VALIDATED; 199 if ("in-process".equals(codeString)) 200 return Status.INPROCESS; 201 if ("req-revalid".equals(codeString)) 202 return Status.REQREVALID; 203 if ("val-fail".equals(codeString)) 204 return Status.VALFAIL; 205 if ("reval-fail".equals(codeString)) 206 return Status.REVALFAIL; 207 throw new IllegalArgumentException("Unknown Status code '" + codeString + "'"); 208 } 209 210 public Enumeration<Status> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<Status>(this, Status.NULL, code); 215 String codeString = code.asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<Status>(this, Status.NULL, code); 218 if ("attested".equals(codeString)) 219 return new Enumeration<Status>(this, Status.ATTESTED, code); 220 if ("validated".equals(codeString)) 221 return new Enumeration<Status>(this, Status.VALIDATED, code); 222 if ("in-process".equals(codeString)) 223 return new Enumeration<Status>(this, Status.INPROCESS, code); 224 if ("req-revalid".equals(codeString)) 225 return new Enumeration<Status>(this, Status.REQREVALID, code); 226 if ("val-fail".equals(codeString)) 227 return new Enumeration<Status>(this, Status.VALFAIL, code); 228 if ("reval-fail".equals(codeString)) 229 return new Enumeration<Status>(this, Status.REVALFAIL, code); 230 throw new FHIRException("Unknown Status code '" + codeString + "'"); 231 } 232 233 public String toCode(Status code) { 234 if (code == Status.NULL) 235 return null; 236 if (code == Status.ATTESTED) 237 return "attested"; 238 if (code == Status.VALIDATED) 239 return "validated"; 240 if (code == Status.INPROCESS) 241 return "in-process"; 242 if (code == Status.REQREVALID) 243 return "req-revalid"; 244 if (code == Status.VALFAIL) 245 return "val-fail"; 246 if (code == Status.REVALFAIL) 247 return "reval-fail"; 248 return "?"; 249 } 250 251 public String toSystem(Status code) { 252 return code.getSystem(); 253 } 254 } 255 256 @Block() 257 public static class VerificationResultPrimarySourceComponent extends BackboneElement implements IBaseBackboneElement { 258 /** 259 * Reference to the primary source. 260 */ 261 @Child(name = "who", type = { Organization.class, Practitioner.class, 262 PractitionerRole.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 263 @Description(shortDefinition = "Reference to the primary source", formalDefinition = "Reference to the primary source.") 264 protected Reference who; 265 266 /** 267 * The actual object that is the target of the reference (Reference to the 268 * primary source.) 269 */ 270 protected Resource whoTarget; 271 272 /** 273 * Type of primary source (License Board; Primary Education; Continuing 274 * Education; Postal Service; Relationship owner; Registration Authority; legal 275 * source; issuing source; authoritative source). 276 */ 277 @Child(name = "type", type = { 278 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 279 @Description(shortDefinition = "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source)", formalDefinition = "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).") 280 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-primary-source-type") 281 protected List<CodeableConcept> type; 282 283 /** 284 * Method for communicating with the primary source (manual; API; Push). 285 */ 286 @Child(name = "communicationMethod", type = { 287 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 288 @Description(shortDefinition = "Method for exchanging information with the primary source", formalDefinition = "Method for communicating with the primary source (manual; API; Push).") 289 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-communication-method") 290 protected List<CodeableConcept> communicationMethod; 291 292 /** 293 * Status of the validation of the target against the primary source 294 * (successful; failed; unknown). 295 */ 296 @Child(name = "validationStatus", type = { 297 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 298 @Description(shortDefinition = "successful | failed | unknown", formalDefinition = "Status of the validation of the target against the primary source (successful; failed; unknown).") 299 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-validation-status") 300 protected CodeableConcept validationStatus; 301 302 /** 303 * When the target was validated against the primary source. 304 */ 305 @Child(name = "validationDate", type = { 306 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 307 @Description(shortDefinition = "When the target was validated against the primary source", formalDefinition = "When the target was validated against the primary source.") 308 protected DateTimeType validationDate; 309 310 /** 311 * Ability of the primary source to push updates/alerts (yes; no; undetermined). 312 */ 313 @Child(name = "canPushUpdates", type = { 314 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 315 @Description(shortDefinition = "yes | no | undetermined", formalDefinition = "Ability of the primary source to push updates/alerts (yes; no; undetermined).") 316 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-can-push-updates") 317 protected CodeableConcept canPushUpdates; 318 319 /** 320 * Type of alerts/updates the primary source can send (specific requested 321 * changes; any changes; as defined by source). 322 */ 323 @Child(name = "pushTypeAvailable", type = { 324 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 325 @Description(shortDefinition = "specific | any | source", formalDefinition = "Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).") 326 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-push-type-available") 327 protected List<CodeableConcept> pushTypeAvailable; 328 329 private static final long serialVersionUID = -928858332L; 330 331 /** 332 * Constructor 333 */ 334 public VerificationResultPrimarySourceComponent() { 335 super(); 336 } 337 338 /** 339 * @return {@link #who} (Reference to the primary source.) 340 */ 341 public Reference getWho() { 342 if (this.who == null) 343 if (Configuration.errorOnAutoCreate()) 344 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.who"); 345 else if (Configuration.doAutoCreate()) 346 this.who = new Reference(); // cc 347 return this.who; 348 } 349 350 public boolean hasWho() { 351 return this.who != null && !this.who.isEmpty(); 352 } 353 354 /** 355 * @param value {@link #who} (Reference to the primary source.) 356 */ 357 public VerificationResultPrimarySourceComponent setWho(Reference value) { 358 this.who = value; 359 return this; 360 } 361 362 /** 363 * @return {@link #who} The actual object that is the target of the reference. 364 * The reference library doesn't populate this, but you can use it to 365 * hold the resource if you resolve it. (Reference to the primary 366 * source.) 367 */ 368 public Resource getWhoTarget() { 369 return this.whoTarget; 370 } 371 372 /** 373 * @param value {@link #who} The actual object that is the target of the 374 * reference. The reference library doesn't use these, but you can 375 * use it to hold the resource if you resolve it. (Reference to the 376 * primary source.) 377 */ 378 public VerificationResultPrimarySourceComponent setWhoTarget(Resource value) { 379 this.whoTarget = value; 380 return this; 381 } 382 383 /** 384 * @return {@link #type} (Type of primary source (License Board; Primary 385 * Education; Continuing Education; Postal Service; Relationship owner; 386 * Registration Authority; legal source; issuing source; authoritative 387 * source).) 388 */ 389 public List<CodeableConcept> getType() { 390 if (this.type == null) 391 this.type = new ArrayList<CodeableConcept>(); 392 return this.type; 393 } 394 395 /** 396 * @return Returns a reference to <code>this</code> for easy method chaining 397 */ 398 public VerificationResultPrimarySourceComponent setType(List<CodeableConcept> theType) { 399 this.type = theType; 400 return this; 401 } 402 403 public boolean hasType() { 404 if (this.type == null) 405 return false; 406 for (CodeableConcept item : this.type) 407 if (!item.isEmpty()) 408 return true; 409 return false; 410 } 411 412 public CodeableConcept addType() { // 3 413 CodeableConcept t = new CodeableConcept(); 414 if (this.type == null) 415 this.type = new ArrayList<CodeableConcept>(); 416 this.type.add(t); 417 return t; 418 } 419 420 public VerificationResultPrimarySourceComponent addType(CodeableConcept t) { // 3 421 if (t == null) 422 return this; 423 if (this.type == null) 424 this.type = new ArrayList<CodeableConcept>(); 425 this.type.add(t); 426 return this; 427 } 428 429 /** 430 * @return The first repetition of repeating field {@link #type}, creating it if 431 * it does not already exist 432 */ 433 public CodeableConcept getTypeFirstRep() { 434 if (getType().isEmpty()) { 435 addType(); 436 } 437 return getType().get(0); 438 } 439 440 /** 441 * @return {@link #communicationMethod} (Method for communicating with the 442 * primary source (manual; API; Push).) 443 */ 444 public List<CodeableConcept> getCommunicationMethod() { 445 if (this.communicationMethod == null) 446 this.communicationMethod = new ArrayList<CodeableConcept>(); 447 return this.communicationMethod; 448 } 449 450 /** 451 * @return Returns a reference to <code>this</code> for easy method chaining 452 */ 453 public VerificationResultPrimarySourceComponent setCommunicationMethod( 454 List<CodeableConcept> theCommunicationMethod) { 455 this.communicationMethod = theCommunicationMethod; 456 return this; 457 } 458 459 public boolean hasCommunicationMethod() { 460 if (this.communicationMethod == null) 461 return false; 462 for (CodeableConcept item : this.communicationMethod) 463 if (!item.isEmpty()) 464 return true; 465 return false; 466 } 467 468 public CodeableConcept addCommunicationMethod() { // 3 469 CodeableConcept t = new CodeableConcept(); 470 if (this.communicationMethod == null) 471 this.communicationMethod = new ArrayList<CodeableConcept>(); 472 this.communicationMethod.add(t); 473 return t; 474 } 475 476 public VerificationResultPrimarySourceComponent addCommunicationMethod(CodeableConcept t) { // 3 477 if (t == null) 478 return this; 479 if (this.communicationMethod == null) 480 this.communicationMethod = new ArrayList<CodeableConcept>(); 481 this.communicationMethod.add(t); 482 return this; 483 } 484 485 /** 486 * @return The first repetition of repeating field {@link #communicationMethod}, 487 * creating it if it does not already exist 488 */ 489 public CodeableConcept getCommunicationMethodFirstRep() { 490 if (getCommunicationMethod().isEmpty()) { 491 addCommunicationMethod(); 492 } 493 return getCommunicationMethod().get(0); 494 } 495 496 /** 497 * @return {@link #validationStatus} (Status of the validation of the target 498 * against the primary source (successful; failed; unknown).) 499 */ 500 public CodeableConcept getValidationStatus() { 501 if (this.validationStatus == null) 502 if (Configuration.errorOnAutoCreate()) 503 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.validationStatus"); 504 else if (Configuration.doAutoCreate()) 505 this.validationStatus = new CodeableConcept(); // cc 506 return this.validationStatus; 507 } 508 509 public boolean hasValidationStatus() { 510 return this.validationStatus != null && !this.validationStatus.isEmpty(); 511 } 512 513 /** 514 * @param value {@link #validationStatus} (Status of the validation of the 515 * target against the primary source (successful; failed; 516 * unknown).) 517 */ 518 public VerificationResultPrimarySourceComponent setValidationStatus(CodeableConcept value) { 519 this.validationStatus = value; 520 return this; 521 } 522 523 /** 524 * @return {@link #validationDate} (When the target was validated against the 525 * primary source.). This is the underlying object with id, value and 526 * extensions. The accessor "getValidationDate" gives direct access to 527 * the value 528 */ 529 public DateTimeType getValidationDateElement() { 530 if (this.validationDate == null) 531 if (Configuration.errorOnAutoCreate()) 532 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.validationDate"); 533 else if (Configuration.doAutoCreate()) 534 this.validationDate = new DateTimeType(); // bb 535 return this.validationDate; 536 } 537 538 public boolean hasValidationDateElement() { 539 return this.validationDate != null && !this.validationDate.isEmpty(); 540 } 541 542 public boolean hasValidationDate() { 543 return this.validationDate != null && !this.validationDate.isEmpty(); 544 } 545 546 /** 547 * @param value {@link #validationDate} (When the target was validated against 548 * the primary source.). This is the underlying object with id, 549 * value and extensions. The accessor "getValidationDate" gives 550 * direct access to the value 551 */ 552 public VerificationResultPrimarySourceComponent setValidationDateElement(DateTimeType value) { 553 this.validationDate = value; 554 return this; 555 } 556 557 /** 558 * @return When the target was validated against the primary source. 559 */ 560 public Date getValidationDate() { 561 return this.validationDate == null ? null : this.validationDate.getValue(); 562 } 563 564 /** 565 * @param value When the target was validated against the primary source. 566 */ 567 public VerificationResultPrimarySourceComponent setValidationDate(Date value) { 568 if (value == null) 569 this.validationDate = null; 570 else { 571 if (this.validationDate == null) 572 this.validationDate = new DateTimeType(); 573 this.validationDate.setValue(value); 574 } 575 return this; 576 } 577 578 /** 579 * @return {@link #canPushUpdates} (Ability of the primary source to push 580 * updates/alerts (yes; no; undetermined).) 581 */ 582 public CodeableConcept getCanPushUpdates() { 583 if (this.canPushUpdates == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.canPushUpdates"); 586 else if (Configuration.doAutoCreate()) 587 this.canPushUpdates = new CodeableConcept(); // cc 588 return this.canPushUpdates; 589 } 590 591 public boolean hasCanPushUpdates() { 592 return this.canPushUpdates != null && !this.canPushUpdates.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #canPushUpdates} (Ability of the primary source to push 597 * updates/alerts (yes; no; undetermined).) 598 */ 599 public VerificationResultPrimarySourceComponent setCanPushUpdates(CodeableConcept value) { 600 this.canPushUpdates = value; 601 return this; 602 } 603 604 /** 605 * @return {@link #pushTypeAvailable} (Type of alerts/updates the primary source 606 * can send (specific requested changes; any changes; as defined by 607 * source).) 608 */ 609 public List<CodeableConcept> getPushTypeAvailable() { 610 if (this.pushTypeAvailable == null) 611 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 612 return this.pushTypeAvailable; 613 } 614 615 /** 616 * @return Returns a reference to <code>this</code> for easy method chaining 617 */ 618 public VerificationResultPrimarySourceComponent setPushTypeAvailable(List<CodeableConcept> thePushTypeAvailable) { 619 this.pushTypeAvailable = thePushTypeAvailable; 620 return this; 621 } 622 623 public boolean hasPushTypeAvailable() { 624 if (this.pushTypeAvailable == null) 625 return false; 626 for (CodeableConcept item : this.pushTypeAvailable) 627 if (!item.isEmpty()) 628 return true; 629 return false; 630 } 631 632 public CodeableConcept addPushTypeAvailable() { // 3 633 CodeableConcept t = new CodeableConcept(); 634 if (this.pushTypeAvailable == null) 635 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 636 this.pushTypeAvailable.add(t); 637 return t; 638 } 639 640 public VerificationResultPrimarySourceComponent addPushTypeAvailable(CodeableConcept t) { // 3 641 if (t == null) 642 return this; 643 if (this.pushTypeAvailable == null) 644 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 645 this.pushTypeAvailable.add(t); 646 return this; 647 } 648 649 /** 650 * @return The first repetition of repeating field {@link #pushTypeAvailable}, 651 * creating it if it does not already exist 652 */ 653 public CodeableConcept getPushTypeAvailableFirstRep() { 654 if (getPushTypeAvailable().isEmpty()) { 655 addPushTypeAvailable(); 656 } 657 return getPushTypeAvailable().get(0); 658 } 659 660 protected void listChildren(List<Property> children) { 661 super.listChildren(children); 662 children.add(new Property("who", "Reference(Organization|Practitioner|PractitionerRole)", 663 "Reference to the primary source.", 0, 1, who)); 664 children.add(new Property("type", "CodeableConcept", 665 "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).", 666 0, java.lang.Integer.MAX_VALUE, type)); 667 children.add(new Property("communicationMethod", "CodeableConcept", 668 "Method for communicating with the primary source (manual; API; Push).", 0, java.lang.Integer.MAX_VALUE, 669 communicationMethod)); 670 children.add(new Property("validationStatus", "CodeableConcept", 671 "Status of the validation of the target against the primary source (successful; failed; unknown).", 0, 1, 672 validationStatus)); 673 children.add(new Property("validationDate", "dateTime", 674 "When the target was validated against the primary source.", 0, 1, validationDate)); 675 children.add(new Property("canPushUpdates", "CodeableConcept", 676 "Ability of the primary source to push updates/alerts (yes; no; undetermined).", 0, 1, canPushUpdates)); 677 children.add(new Property("pushTypeAvailable", "CodeableConcept", 678 "Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).", 679 0, java.lang.Integer.MAX_VALUE, pushTypeAvailable)); 680 } 681 682 @Override 683 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 684 switch (_hash) { 685 case 117694: 686 /* who */ return new Property("who", "Reference(Organization|Practitioner|PractitionerRole)", 687 "Reference to the primary source.", 0, 1, who); 688 case 3575610: 689 /* type */ return new Property("type", "CodeableConcept", 690 "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).", 691 0, java.lang.Integer.MAX_VALUE, type); 692 case 1314116695: 693 /* communicationMethod */ return new Property("communicationMethod", "CodeableConcept", 694 "Method for communicating with the primary source (manual; API; Push).", 0, java.lang.Integer.MAX_VALUE, 695 communicationMethod); 696 case 1775633867: 697 /* validationStatus */ return new Property("validationStatus", "CodeableConcept", 698 "Status of the validation of the target against the primary source (successful; failed; unknown).", 0, 1, 699 validationStatus); 700 case -280180793: 701 /* validationDate */ return new Property("validationDate", "dateTime", 702 "When the target was validated against the primary source.", 0, 1, validationDate); 703 case 1463787104: 704 /* canPushUpdates */ return new Property("canPushUpdates", "CodeableConcept", 705 "Ability of the primary source to push updates/alerts (yes; no; undetermined).", 0, 1, canPushUpdates); 706 case 945223605: 707 /* pushTypeAvailable */ return new Property("pushTypeAvailable", "CodeableConcept", 708 "Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).", 709 0, java.lang.Integer.MAX_VALUE, pushTypeAvailable); 710 default: 711 return super.getNamedProperty(_hash, _name, _checkValid); 712 } 713 714 } 715 716 @Override 717 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 718 switch (hash) { 719 case 117694: 720 /* who */ return this.who == null ? new Base[0] : new Base[] { this.who }; // Reference 721 case 3575610: 722 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 723 case 1314116695: 724 /* communicationMethod */ return this.communicationMethod == null ? new Base[0] 725 : this.communicationMethod.toArray(new Base[this.communicationMethod.size()]); // CodeableConcept 726 case 1775633867: 727 /* validationStatus */ return this.validationStatus == null ? new Base[0] 728 : new Base[] { this.validationStatus }; // CodeableConcept 729 case -280180793: 730 /* validationDate */ return this.validationDate == null ? new Base[0] : new Base[] { this.validationDate }; // DateTimeType 731 case 1463787104: 732 /* canPushUpdates */ return this.canPushUpdates == null ? new Base[0] : new Base[] { this.canPushUpdates }; // CodeableConcept 733 case 945223605: 734 /* pushTypeAvailable */ return this.pushTypeAvailable == null ? new Base[0] 735 : this.pushTypeAvailable.toArray(new Base[this.pushTypeAvailable.size()]); // CodeableConcept 736 default: 737 return super.getProperty(hash, name, checkValid); 738 } 739 740 } 741 742 @Override 743 public Base setProperty(int hash, String name, Base value) throws FHIRException { 744 switch (hash) { 745 case 117694: // who 746 this.who = castToReference(value); // Reference 747 return value; 748 case 3575610: // type 749 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 750 return value; 751 case 1314116695: // communicationMethod 752 this.getCommunicationMethod().add(castToCodeableConcept(value)); // CodeableConcept 753 return value; 754 case 1775633867: // validationStatus 755 this.validationStatus = castToCodeableConcept(value); // CodeableConcept 756 return value; 757 case -280180793: // validationDate 758 this.validationDate = castToDateTime(value); // DateTimeType 759 return value; 760 case 1463787104: // canPushUpdates 761 this.canPushUpdates = castToCodeableConcept(value); // CodeableConcept 762 return value; 763 case 945223605: // pushTypeAvailable 764 this.getPushTypeAvailable().add(castToCodeableConcept(value)); // CodeableConcept 765 return value; 766 default: 767 return super.setProperty(hash, name, value); 768 } 769 770 } 771 772 @Override 773 public Base setProperty(String name, Base value) throws FHIRException { 774 if (name.equals("who")) { 775 this.who = castToReference(value); // Reference 776 } else if (name.equals("type")) { 777 this.getType().add(castToCodeableConcept(value)); 778 } else if (name.equals("communicationMethod")) { 779 this.getCommunicationMethod().add(castToCodeableConcept(value)); 780 } else if (name.equals("validationStatus")) { 781 this.validationStatus = castToCodeableConcept(value); // CodeableConcept 782 } else if (name.equals("validationDate")) { 783 this.validationDate = castToDateTime(value); // DateTimeType 784 } else if (name.equals("canPushUpdates")) { 785 this.canPushUpdates = castToCodeableConcept(value); // CodeableConcept 786 } else if (name.equals("pushTypeAvailable")) { 787 this.getPushTypeAvailable().add(castToCodeableConcept(value)); 788 } else 789 return super.setProperty(name, value); 790 return value; 791 } 792 793 @Override 794 public void removeChild(String name, Base value) throws FHIRException { 795 if (name.equals("who")) { 796 this.who = null; 797 } else if (name.equals("type")) { 798 this.getType().remove(castToCodeableConcept(value)); 799 } else if (name.equals("communicationMethod")) { 800 this.getCommunicationMethod().remove(castToCodeableConcept(value)); 801 } else if (name.equals("validationStatus")) { 802 this.validationStatus = null; 803 } else if (name.equals("validationDate")) { 804 this.validationDate = null; 805 } else if (name.equals("canPushUpdates")) { 806 this.canPushUpdates = null; 807 } else if (name.equals("pushTypeAvailable")) { 808 this.getPushTypeAvailable().remove(castToCodeableConcept(value)); 809 } else 810 super.removeChild(name, value); 811 812 } 813 814 @Override 815 public Base makeProperty(int hash, String name) throws FHIRException { 816 switch (hash) { 817 case 117694: 818 return getWho(); 819 case 3575610: 820 return addType(); 821 case 1314116695: 822 return addCommunicationMethod(); 823 case 1775633867: 824 return getValidationStatus(); 825 case -280180793: 826 return getValidationDateElement(); 827 case 1463787104: 828 return getCanPushUpdates(); 829 case 945223605: 830 return addPushTypeAvailable(); 831 default: 832 return super.makeProperty(hash, name); 833 } 834 835 } 836 837 @Override 838 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 839 switch (hash) { 840 case 117694: 841 /* who */ return new String[] { "Reference" }; 842 case 3575610: 843 /* type */ return new String[] { "CodeableConcept" }; 844 case 1314116695: 845 /* communicationMethod */ return new String[] { "CodeableConcept" }; 846 case 1775633867: 847 /* validationStatus */ return new String[] { "CodeableConcept" }; 848 case -280180793: 849 /* validationDate */ return new String[] { "dateTime" }; 850 case 1463787104: 851 /* canPushUpdates */ return new String[] { "CodeableConcept" }; 852 case 945223605: 853 /* pushTypeAvailable */ return new String[] { "CodeableConcept" }; 854 default: 855 return super.getTypesForProperty(hash, name); 856 } 857 858 } 859 860 @Override 861 public Base addChild(String name) throws FHIRException { 862 if (name.equals("who")) { 863 this.who = new Reference(); 864 return this.who; 865 } else if (name.equals("type")) { 866 return addType(); 867 } else if (name.equals("communicationMethod")) { 868 return addCommunicationMethod(); 869 } else if (name.equals("validationStatus")) { 870 this.validationStatus = new CodeableConcept(); 871 return this.validationStatus; 872 } else if (name.equals("validationDate")) { 873 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.validationDate"); 874 } else if (name.equals("canPushUpdates")) { 875 this.canPushUpdates = new CodeableConcept(); 876 return this.canPushUpdates; 877 } else if (name.equals("pushTypeAvailable")) { 878 return addPushTypeAvailable(); 879 } else 880 return super.addChild(name); 881 } 882 883 public VerificationResultPrimarySourceComponent copy() { 884 VerificationResultPrimarySourceComponent dst = new VerificationResultPrimarySourceComponent(); 885 copyValues(dst); 886 return dst; 887 } 888 889 public void copyValues(VerificationResultPrimarySourceComponent dst) { 890 super.copyValues(dst); 891 dst.who = who == null ? null : who.copy(); 892 if (type != null) { 893 dst.type = new ArrayList<CodeableConcept>(); 894 for (CodeableConcept i : type) 895 dst.type.add(i.copy()); 896 } 897 ; 898 if (communicationMethod != null) { 899 dst.communicationMethod = new ArrayList<CodeableConcept>(); 900 for (CodeableConcept i : communicationMethod) 901 dst.communicationMethod.add(i.copy()); 902 } 903 ; 904 dst.validationStatus = validationStatus == null ? null : validationStatus.copy(); 905 dst.validationDate = validationDate == null ? null : validationDate.copy(); 906 dst.canPushUpdates = canPushUpdates == null ? null : canPushUpdates.copy(); 907 if (pushTypeAvailable != null) { 908 dst.pushTypeAvailable = new ArrayList<CodeableConcept>(); 909 for (CodeableConcept i : pushTypeAvailable) 910 dst.pushTypeAvailable.add(i.copy()); 911 } 912 ; 913 } 914 915 @Override 916 public boolean equalsDeep(Base other_) { 917 if (!super.equalsDeep(other_)) 918 return false; 919 if (!(other_ instanceof VerificationResultPrimarySourceComponent)) 920 return false; 921 VerificationResultPrimarySourceComponent o = (VerificationResultPrimarySourceComponent) other_; 922 return compareDeep(who, o.who, true) && compareDeep(type, o.type, true) 923 && compareDeep(communicationMethod, o.communicationMethod, true) 924 && compareDeep(validationStatus, o.validationStatus, true) 925 && compareDeep(validationDate, o.validationDate, true) && compareDeep(canPushUpdates, o.canPushUpdates, true) 926 && compareDeep(pushTypeAvailable, o.pushTypeAvailable, true); 927 } 928 929 @Override 930 public boolean equalsShallow(Base other_) { 931 if (!super.equalsShallow(other_)) 932 return false; 933 if (!(other_ instanceof VerificationResultPrimarySourceComponent)) 934 return false; 935 VerificationResultPrimarySourceComponent o = (VerificationResultPrimarySourceComponent) other_; 936 return compareValues(validationDate, o.validationDate, true); 937 } 938 939 public boolean isEmpty() { 940 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(who, type, communicationMethod, validationStatus, 941 validationDate, canPushUpdates, pushTypeAvailable); 942 } 943 944 public String fhirType() { 945 return "VerificationResult.primarySource"; 946 947 } 948 949 } 950 951 @Block() 952 public static class VerificationResultAttestationComponent extends BackboneElement implements IBaseBackboneElement { 953 /** 954 * The individual or organization attesting to information. 955 */ 956 @Child(name = "who", type = { Practitioner.class, PractitionerRole.class, 957 Organization.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 958 @Description(shortDefinition = "The individual or organization attesting to information", formalDefinition = "The individual or organization attesting to information.") 959 protected Reference who; 960 961 /** 962 * The actual object that is the target of the reference (The individual or 963 * organization attesting to information.) 964 */ 965 protected Resource whoTarget; 966 967 /** 968 * When the who is asserting on behalf of another (organization or individual). 969 */ 970 @Child(name = "onBehalfOf", type = { Organization.class, Practitioner.class, 971 PractitionerRole.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 972 @Description(shortDefinition = "When the who is asserting on behalf of another (organization or individual)", formalDefinition = "When the who is asserting on behalf of another (organization or individual).") 973 protected Reference onBehalfOf; 974 975 /** 976 * The actual object that is the target of the reference (When the who is 977 * asserting on behalf of another (organization or individual).) 978 */ 979 protected Resource onBehalfOfTarget; 980 981 /** 982 * The method by which attested information was submitted/retrieved (manual; 983 * API; Push). 984 */ 985 @Child(name = "communicationMethod", type = { 986 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 987 @Description(shortDefinition = "The method by which attested information was submitted/retrieved", formalDefinition = "The method by which attested information was submitted/retrieved (manual; API; Push).") 988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-communication-method") 989 protected CodeableConcept communicationMethod; 990 991 /** 992 * The date the information was attested to. 993 */ 994 @Child(name = "date", type = { DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 995 @Description(shortDefinition = "The date the information was attested to", formalDefinition = "The date the information was attested to.") 996 protected DateType date; 997 998 /** 999 * A digital identity certificate associated with the attestation source. 1000 */ 1001 @Child(name = "sourceIdentityCertificate", type = { 1002 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1003 @Description(shortDefinition = "A digital identity certificate associated with the attestation source", formalDefinition = "A digital identity certificate associated with the attestation source.") 1004 protected StringType sourceIdentityCertificate; 1005 1006 /** 1007 * A digital identity certificate associated with the proxy entity submitting 1008 * attested information on behalf of the attestation source. 1009 */ 1010 @Child(name = "proxyIdentityCertificate", type = { 1011 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1012 @Description(shortDefinition = "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source", formalDefinition = "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.") 1013 protected StringType proxyIdentityCertificate; 1014 1015 /** 1016 * Signed assertion by the proxy entity indicating that they have the right to 1017 * submit attested information on behalf of the attestation source. 1018 */ 1019 @Child(name = "proxySignature", type = { 1020 Signature.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1021 @Description(shortDefinition = "Proxy signature", formalDefinition = "Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.") 1022 protected Signature proxySignature; 1023 1024 /** 1025 * Signed assertion by the attestation source that they have attested to the 1026 * information. 1027 */ 1028 @Child(name = "sourceSignature", type = { 1029 Signature.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1030 @Description(shortDefinition = "Attester signature", formalDefinition = "Signed assertion by the attestation source that they have attested to the information.") 1031 protected Signature sourceSignature; 1032 1033 private static final long serialVersionUID = -900018800L; 1034 1035 /** 1036 * Constructor 1037 */ 1038 public VerificationResultAttestationComponent() { 1039 super(); 1040 } 1041 1042 /** 1043 * @return {@link #who} (The individual or organization attesting to 1044 * information.) 1045 */ 1046 public Reference getWho() { 1047 if (this.who == null) 1048 if (Configuration.errorOnAutoCreate()) 1049 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.who"); 1050 else if (Configuration.doAutoCreate()) 1051 this.who = new Reference(); // cc 1052 return this.who; 1053 } 1054 1055 public boolean hasWho() { 1056 return this.who != null && !this.who.isEmpty(); 1057 } 1058 1059 /** 1060 * @param value {@link #who} (The individual or organization attesting to 1061 * information.) 1062 */ 1063 public VerificationResultAttestationComponent setWho(Reference value) { 1064 this.who = value; 1065 return this; 1066 } 1067 1068 /** 1069 * @return {@link #who} The actual object that is the target of the reference. 1070 * The reference library doesn't populate this, but you can use it to 1071 * hold the resource if you resolve it. (The individual or organization 1072 * attesting to information.) 1073 */ 1074 public Resource getWhoTarget() { 1075 return this.whoTarget; 1076 } 1077 1078 /** 1079 * @param value {@link #who} The actual object that is the target of the 1080 * reference. The reference library doesn't use these, but you can 1081 * use it to hold the resource if you resolve it. (The individual 1082 * or organization attesting to information.) 1083 */ 1084 public VerificationResultAttestationComponent setWhoTarget(Resource value) { 1085 this.whoTarget = value; 1086 return this; 1087 } 1088 1089 /** 1090 * @return {@link #onBehalfOf} (When the who is asserting on behalf of another 1091 * (organization or individual).) 1092 */ 1093 public Reference getOnBehalfOf() { 1094 if (this.onBehalfOf == null) 1095 if (Configuration.errorOnAutoCreate()) 1096 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.onBehalfOf"); 1097 else if (Configuration.doAutoCreate()) 1098 this.onBehalfOf = new Reference(); // cc 1099 return this.onBehalfOf; 1100 } 1101 1102 public boolean hasOnBehalfOf() { 1103 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 1104 } 1105 1106 /** 1107 * @param value {@link #onBehalfOf} (When the who is asserting on behalf of 1108 * another (organization or individual).) 1109 */ 1110 public VerificationResultAttestationComponent setOnBehalfOf(Reference value) { 1111 this.onBehalfOf = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return {@link #onBehalfOf} The actual object that is the target of the 1117 * reference. The reference library doesn't populate this, but you can 1118 * use it to hold the resource if you resolve it. (When the who is 1119 * asserting on behalf of another (organization or individual).) 1120 */ 1121 public Resource getOnBehalfOfTarget() { 1122 return this.onBehalfOfTarget; 1123 } 1124 1125 /** 1126 * @param value {@link #onBehalfOf} The actual object that is the target of the 1127 * reference. The reference library doesn't use these, but you can 1128 * use it to hold the resource if you resolve it. (When the who is 1129 * asserting on behalf of another (organization or individual).) 1130 */ 1131 public VerificationResultAttestationComponent setOnBehalfOfTarget(Resource value) { 1132 this.onBehalfOfTarget = value; 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #communicationMethod} (The method by which attested 1138 * information was submitted/retrieved (manual; API; Push).) 1139 */ 1140 public CodeableConcept getCommunicationMethod() { 1141 if (this.communicationMethod == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.communicationMethod"); 1144 else if (Configuration.doAutoCreate()) 1145 this.communicationMethod = new CodeableConcept(); // cc 1146 return this.communicationMethod; 1147 } 1148 1149 public boolean hasCommunicationMethod() { 1150 return this.communicationMethod != null && !this.communicationMethod.isEmpty(); 1151 } 1152 1153 /** 1154 * @param value {@link #communicationMethod} (The method by which attested 1155 * information was submitted/retrieved (manual; API; Push).) 1156 */ 1157 public VerificationResultAttestationComponent setCommunicationMethod(CodeableConcept value) { 1158 this.communicationMethod = value; 1159 return this; 1160 } 1161 1162 /** 1163 * @return {@link #date} (The date the information was attested to.). This is 1164 * the underlying object with id, value and extensions. The accessor 1165 * "getDate" gives direct access to the value 1166 */ 1167 public DateType getDateElement() { 1168 if (this.date == null) 1169 if (Configuration.errorOnAutoCreate()) 1170 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.date"); 1171 else if (Configuration.doAutoCreate()) 1172 this.date = new DateType(); // bb 1173 return this.date; 1174 } 1175 1176 public boolean hasDateElement() { 1177 return this.date != null && !this.date.isEmpty(); 1178 } 1179 1180 public boolean hasDate() { 1181 return this.date != null && !this.date.isEmpty(); 1182 } 1183 1184 /** 1185 * @param value {@link #date} (The date the information was attested to.). This 1186 * is the underlying object with id, value and extensions. The 1187 * accessor "getDate" gives direct access to the value 1188 */ 1189 public VerificationResultAttestationComponent setDateElement(DateType value) { 1190 this.date = value; 1191 return this; 1192 } 1193 1194 /** 1195 * @return The date the information was attested to. 1196 */ 1197 public Date getDate() { 1198 return this.date == null ? null : this.date.getValue(); 1199 } 1200 1201 /** 1202 * @param value The date the information was attested to. 1203 */ 1204 public VerificationResultAttestationComponent setDate(Date value) { 1205 if (value == null) 1206 this.date = null; 1207 else { 1208 if (this.date == null) 1209 this.date = new DateType(); 1210 this.date.setValue(value); 1211 } 1212 return this; 1213 } 1214 1215 /** 1216 * @return {@link #sourceIdentityCertificate} (A digital identity certificate 1217 * associated with the attestation source.). This is the underlying 1218 * object with id, value and extensions. The accessor 1219 * "getSourceIdentityCertificate" gives direct access to the value 1220 */ 1221 public StringType getSourceIdentityCertificateElement() { 1222 if (this.sourceIdentityCertificate == null) 1223 if (Configuration.errorOnAutoCreate()) 1224 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.sourceIdentityCertificate"); 1225 else if (Configuration.doAutoCreate()) 1226 this.sourceIdentityCertificate = new StringType(); // bb 1227 return this.sourceIdentityCertificate; 1228 } 1229 1230 public boolean hasSourceIdentityCertificateElement() { 1231 return this.sourceIdentityCertificate != null && !this.sourceIdentityCertificate.isEmpty(); 1232 } 1233 1234 public boolean hasSourceIdentityCertificate() { 1235 return this.sourceIdentityCertificate != null && !this.sourceIdentityCertificate.isEmpty(); 1236 } 1237 1238 /** 1239 * @param value {@link #sourceIdentityCertificate} (A digital identity 1240 * certificate associated with the attestation source.). This is 1241 * the underlying object with id, value and extensions. The 1242 * accessor "getSourceIdentityCertificate" gives direct access to 1243 * the value 1244 */ 1245 public VerificationResultAttestationComponent setSourceIdentityCertificateElement(StringType value) { 1246 this.sourceIdentityCertificate = value; 1247 return this; 1248 } 1249 1250 /** 1251 * @return A digital identity certificate associated with the attestation 1252 * source. 1253 */ 1254 public String getSourceIdentityCertificate() { 1255 return this.sourceIdentityCertificate == null ? null : this.sourceIdentityCertificate.getValue(); 1256 } 1257 1258 /** 1259 * @param value A digital identity certificate associated with the attestation 1260 * source. 1261 */ 1262 public VerificationResultAttestationComponent setSourceIdentityCertificate(String value) { 1263 if (Utilities.noString(value)) 1264 this.sourceIdentityCertificate = null; 1265 else { 1266 if (this.sourceIdentityCertificate == null) 1267 this.sourceIdentityCertificate = new StringType(); 1268 this.sourceIdentityCertificate.setValue(value); 1269 } 1270 return this; 1271 } 1272 1273 /** 1274 * @return {@link #proxyIdentityCertificate} (A digital identity certificate 1275 * associated with the proxy entity submitting attested information on 1276 * behalf of the attestation source.). This is the underlying object 1277 * with id, value and extensions. The accessor 1278 * "getProxyIdentityCertificate" gives direct access to the value 1279 */ 1280 public StringType getProxyIdentityCertificateElement() { 1281 if (this.proxyIdentityCertificate == null) 1282 if (Configuration.errorOnAutoCreate()) 1283 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.proxyIdentityCertificate"); 1284 else if (Configuration.doAutoCreate()) 1285 this.proxyIdentityCertificate = new StringType(); // bb 1286 return this.proxyIdentityCertificate; 1287 } 1288 1289 public boolean hasProxyIdentityCertificateElement() { 1290 return this.proxyIdentityCertificate != null && !this.proxyIdentityCertificate.isEmpty(); 1291 } 1292 1293 public boolean hasProxyIdentityCertificate() { 1294 return this.proxyIdentityCertificate != null && !this.proxyIdentityCertificate.isEmpty(); 1295 } 1296 1297 /** 1298 * @param value {@link #proxyIdentityCertificate} (A digital identity 1299 * certificate associated with the proxy entity submitting attested 1300 * information on behalf of the attestation source.). This is the 1301 * underlying object with id, value and extensions. The accessor 1302 * "getProxyIdentityCertificate" gives direct access to the value 1303 */ 1304 public VerificationResultAttestationComponent setProxyIdentityCertificateElement(StringType value) { 1305 this.proxyIdentityCertificate = value; 1306 return this; 1307 } 1308 1309 /** 1310 * @return A digital identity certificate associated with the proxy entity 1311 * submitting attested information on behalf of the attestation source. 1312 */ 1313 public String getProxyIdentityCertificate() { 1314 return this.proxyIdentityCertificate == null ? null : this.proxyIdentityCertificate.getValue(); 1315 } 1316 1317 /** 1318 * @param value A digital identity certificate associated with the proxy entity 1319 * submitting attested information on behalf of the attestation 1320 * source. 1321 */ 1322 public VerificationResultAttestationComponent setProxyIdentityCertificate(String value) { 1323 if (Utilities.noString(value)) 1324 this.proxyIdentityCertificate = null; 1325 else { 1326 if (this.proxyIdentityCertificate == null) 1327 this.proxyIdentityCertificate = new StringType(); 1328 this.proxyIdentityCertificate.setValue(value); 1329 } 1330 return this; 1331 } 1332 1333 /** 1334 * @return {@link #proxySignature} (Signed assertion by the proxy entity 1335 * indicating that they have the right to submit attested information on 1336 * behalf of the attestation source.) 1337 */ 1338 public Signature getProxySignature() { 1339 if (this.proxySignature == null) 1340 if (Configuration.errorOnAutoCreate()) 1341 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.proxySignature"); 1342 else if (Configuration.doAutoCreate()) 1343 this.proxySignature = new Signature(); // cc 1344 return this.proxySignature; 1345 } 1346 1347 public boolean hasProxySignature() { 1348 return this.proxySignature != null && !this.proxySignature.isEmpty(); 1349 } 1350 1351 /** 1352 * @param value {@link #proxySignature} (Signed assertion by the proxy entity 1353 * indicating that they have the right to submit attested 1354 * information on behalf of the attestation source.) 1355 */ 1356 public VerificationResultAttestationComponent setProxySignature(Signature value) { 1357 this.proxySignature = value; 1358 return this; 1359 } 1360 1361 /** 1362 * @return {@link #sourceSignature} (Signed assertion by the attestation source 1363 * that they have attested to the information.) 1364 */ 1365 public Signature getSourceSignature() { 1366 if (this.sourceSignature == null) 1367 if (Configuration.errorOnAutoCreate()) 1368 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.sourceSignature"); 1369 else if (Configuration.doAutoCreate()) 1370 this.sourceSignature = new Signature(); // cc 1371 return this.sourceSignature; 1372 } 1373 1374 public boolean hasSourceSignature() { 1375 return this.sourceSignature != null && !this.sourceSignature.isEmpty(); 1376 } 1377 1378 /** 1379 * @param value {@link #sourceSignature} (Signed assertion by the attestation 1380 * source that they have attested to the information.) 1381 */ 1382 public VerificationResultAttestationComponent setSourceSignature(Signature value) { 1383 this.sourceSignature = value; 1384 return this; 1385 } 1386 1387 protected void listChildren(List<Property> children) { 1388 super.listChildren(children); 1389 children.add(new Property("who", "Reference(Practitioner|PractitionerRole|Organization)", 1390 "The individual or organization attesting to information.", 0, 1, who)); 1391 children.add(new Property("onBehalfOf", "Reference(Organization|Practitioner|PractitionerRole)", 1392 "When the who is asserting on behalf of another (organization or individual).", 0, 1, onBehalfOf)); 1393 children.add(new Property("communicationMethod", "CodeableConcept", 1394 "The method by which attested information was submitted/retrieved (manual; API; Push).", 0, 1, 1395 communicationMethod)); 1396 children.add(new Property("date", "date", "The date the information was attested to.", 0, 1, date)); 1397 children.add(new Property("sourceIdentityCertificate", "string", 1398 "A digital identity certificate associated with the attestation source.", 0, 1, sourceIdentityCertificate)); 1399 children.add(new Property("proxyIdentityCertificate", "string", 1400 "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.", 1401 0, 1, proxyIdentityCertificate)); 1402 children.add(new Property("proxySignature", "Signature", 1403 "Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.", 1404 0, 1, proxySignature)); 1405 children.add(new Property("sourceSignature", "Signature", 1406 "Signed assertion by the attestation source that they have attested to the information.", 0, 1, 1407 sourceSignature)); 1408 } 1409 1410 @Override 1411 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1412 switch (_hash) { 1413 case 117694: 1414 /* who */ return new Property("who", "Reference(Practitioner|PractitionerRole|Organization)", 1415 "The individual or organization attesting to information.", 0, 1, who); 1416 case -14402964: 1417 /* onBehalfOf */ return new Property("onBehalfOf", "Reference(Organization|Practitioner|PractitionerRole)", 1418 "When the who is asserting on behalf of another (organization or individual).", 0, 1, onBehalfOf); 1419 case 1314116695: 1420 /* communicationMethod */ return new Property("communicationMethod", "CodeableConcept", 1421 "The method by which attested information was submitted/retrieved (manual; API; Push).", 0, 1, 1422 communicationMethod); 1423 case 3076014: 1424 /* date */ return new Property("date", "date", "The date the information was attested to.", 0, 1, date); 1425 case -799067682: 1426 /* sourceIdentityCertificate */ return new Property("sourceIdentityCertificate", "string", 1427 "A digital identity certificate associated with the attestation source.", 0, 1, sourceIdentityCertificate); 1428 case 431558827: 1429 /* proxyIdentityCertificate */ return new Property("proxyIdentityCertificate", "string", 1430 "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.", 1431 0, 1, proxyIdentityCertificate); 1432 case 1455540714: 1433 /* proxySignature */ return new Property("proxySignature", "Signature", 1434 "Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.", 1435 0, 1, proxySignature); 1436 case 1754480349: 1437 /* sourceSignature */ return new Property("sourceSignature", "Signature", 1438 "Signed assertion by the attestation source that they have attested to the information.", 0, 1, 1439 sourceSignature); 1440 default: 1441 return super.getNamedProperty(_hash, _name, _checkValid); 1442 } 1443 1444 } 1445 1446 @Override 1447 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1448 switch (hash) { 1449 case 117694: 1450 /* who */ return this.who == null ? new Base[0] : new Base[] { this.who }; // Reference 1451 case -14402964: 1452 /* onBehalfOf */ return this.onBehalfOf == null ? new Base[0] : new Base[] { this.onBehalfOf }; // Reference 1453 case 1314116695: 1454 /* communicationMethod */ return this.communicationMethod == null ? new Base[0] 1455 : new Base[] { this.communicationMethod }; // CodeableConcept 1456 case 3076014: 1457 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 1458 case -799067682: 1459 /* sourceIdentityCertificate */ return this.sourceIdentityCertificate == null ? new Base[0] 1460 : new Base[] { this.sourceIdentityCertificate }; // StringType 1461 case 431558827: 1462 /* proxyIdentityCertificate */ return this.proxyIdentityCertificate == null ? new Base[0] 1463 : new Base[] { this.proxyIdentityCertificate }; // StringType 1464 case 1455540714: 1465 /* proxySignature */ return this.proxySignature == null ? new Base[0] : new Base[] { this.proxySignature }; // Signature 1466 case 1754480349: 1467 /* sourceSignature */ return this.sourceSignature == null ? new Base[0] : new Base[] { this.sourceSignature }; // Signature 1468 default: 1469 return super.getProperty(hash, name, checkValid); 1470 } 1471 1472 } 1473 1474 @Override 1475 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1476 switch (hash) { 1477 case 117694: // who 1478 this.who = castToReference(value); // Reference 1479 return value; 1480 case -14402964: // onBehalfOf 1481 this.onBehalfOf = castToReference(value); // Reference 1482 return value; 1483 case 1314116695: // communicationMethod 1484 this.communicationMethod = castToCodeableConcept(value); // CodeableConcept 1485 return value; 1486 case 3076014: // date 1487 this.date = castToDate(value); // DateType 1488 return value; 1489 case -799067682: // sourceIdentityCertificate 1490 this.sourceIdentityCertificate = castToString(value); // StringType 1491 return value; 1492 case 431558827: // proxyIdentityCertificate 1493 this.proxyIdentityCertificate = castToString(value); // StringType 1494 return value; 1495 case 1455540714: // proxySignature 1496 this.proxySignature = castToSignature(value); // Signature 1497 return value; 1498 case 1754480349: // sourceSignature 1499 this.sourceSignature = castToSignature(value); // Signature 1500 return value; 1501 default: 1502 return super.setProperty(hash, name, value); 1503 } 1504 1505 } 1506 1507 @Override 1508 public Base setProperty(String name, Base value) throws FHIRException { 1509 if (name.equals("who")) { 1510 this.who = castToReference(value); // Reference 1511 } else if (name.equals("onBehalfOf")) { 1512 this.onBehalfOf = castToReference(value); // Reference 1513 } else if (name.equals("communicationMethod")) { 1514 this.communicationMethod = castToCodeableConcept(value); // CodeableConcept 1515 } else if (name.equals("date")) { 1516 this.date = castToDate(value); // DateType 1517 } else if (name.equals("sourceIdentityCertificate")) { 1518 this.sourceIdentityCertificate = castToString(value); // StringType 1519 } else if (name.equals("proxyIdentityCertificate")) { 1520 this.proxyIdentityCertificate = castToString(value); // StringType 1521 } else if (name.equals("proxySignature")) { 1522 this.proxySignature = castToSignature(value); // Signature 1523 } else if (name.equals("sourceSignature")) { 1524 this.sourceSignature = castToSignature(value); // Signature 1525 } else 1526 return super.setProperty(name, value); 1527 return value; 1528 } 1529 1530 @Override 1531 public void removeChild(String name, Base value) throws FHIRException { 1532 if (name.equals("who")) { 1533 this.who = null; 1534 } else if (name.equals("onBehalfOf")) { 1535 this.onBehalfOf = null; 1536 } else if (name.equals("communicationMethod")) { 1537 this.communicationMethod = null; 1538 } else if (name.equals("date")) { 1539 this.date = null; 1540 } else if (name.equals("sourceIdentityCertificate")) { 1541 this.sourceIdentityCertificate = null; 1542 } else if (name.equals("proxyIdentityCertificate")) { 1543 this.proxyIdentityCertificate = null; 1544 } else if (name.equals("proxySignature")) { 1545 this.proxySignature = null; 1546 } else if (name.equals("sourceSignature")) { 1547 this.sourceSignature = null; 1548 } else 1549 super.removeChild(name, value); 1550 1551 } 1552 1553 @Override 1554 public Base makeProperty(int hash, String name) throws FHIRException { 1555 switch (hash) { 1556 case 117694: 1557 return getWho(); 1558 case -14402964: 1559 return getOnBehalfOf(); 1560 case 1314116695: 1561 return getCommunicationMethod(); 1562 case 3076014: 1563 return getDateElement(); 1564 case -799067682: 1565 return getSourceIdentityCertificateElement(); 1566 case 431558827: 1567 return getProxyIdentityCertificateElement(); 1568 case 1455540714: 1569 return getProxySignature(); 1570 case 1754480349: 1571 return getSourceSignature(); 1572 default: 1573 return super.makeProperty(hash, name); 1574 } 1575 1576 } 1577 1578 @Override 1579 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1580 switch (hash) { 1581 case 117694: 1582 /* who */ return new String[] { "Reference" }; 1583 case -14402964: 1584 /* onBehalfOf */ return new String[] { "Reference" }; 1585 case 1314116695: 1586 /* communicationMethod */ return new String[] { "CodeableConcept" }; 1587 case 3076014: 1588 /* date */ return new String[] { "date" }; 1589 case -799067682: 1590 /* sourceIdentityCertificate */ return new String[] { "string" }; 1591 case 431558827: 1592 /* proxyIdentityCertificate */ return new String[] { "string" }; 1593 case 1455540714: 1594 /* proxySignature */ return new String[] { "Signature" }; 1595 case 1754480349: 1596 /* sourceSignature */ return new String[] { "Signature" }; 1597 default: 1598 return super.getTypesForProperty(hash, name); 1599 } 1600 1601 } 1602 1603 @Override 1604 public Base addChild(String name) throws FHIRException { 1605 if (name.equals("who")) { 1606 this.who = new Reference(); 1607 return this.who; 1608 } else if (name.equals("onBehalfOf")) { 1609 this.onBehalfOf = new Reference(); 1610 return this.onBehalfOf; 1611 } else if (name.equals("communicationMethod")) { 1612 this.communicationMethod = new CodeableConcept(); 1613 return this.communicationMethod; 1614 } else if (name.equals("date")) { 1615 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.date"); 1616 } else if (name.equals("sourceIdentityCertificate")) { 1617 throw new FHIRException( 1618 "Cannot call addChild on a singleton property VerificationResult.sourceIdentityCertificate"); 1619 } else if (name.equals("proxyIdentityCertificate")) { 1620 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.proxyIdentityCertificate"); 1621 } else if (name.equals("proxySignature")) { 1622 this.proxySignature = new Signature(); 1623 return this.proxySignature; 1624 } else if (name.equals("sourceSignature")) { 1625 this.sourceSignature = new Signature(); 1626 return this.sourceSignature; 1627 } else 1628 return super.addChild(name); 1629 } 1630 1631 public VerificationResultAttestationComponent copy() { 1632 VerificationResultAttestationComponent dst = new VerificationResultAttestationComponent(); 1633 copyValues(dst); 1634 return dst; 1635 } 1636 1637 public void copyValues(VerificationResultAttestationComponent dst) { 1638 super.copyValues(dst); 1639 dst.who = who == null ? null : who.copy(); 1640 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 1641 dst.communicationMethod = communicationMethod == null ? null : communicationMethod.copy(); 1642 dst.date = date == null ? null : date.copy(); 1643 dst.sourceIdentityCertificate = sourceIdentityCertificate == null ? null : sourceIdentityCertificate.copy(); 1644 dst.proxyIdentityCertificate = proxyIdentityCertificate == null ? null : proxyIdentityCertificate.copy(); 1645 dst.proxySignature = proxySignature == null ? null : proxySignature.copy(); 1646 dst.sourceSignature = sourceSignature == null ? null : sourceSignature.copy(); 1647 } 1648 1649 @Override 1650 public boolean equalsDeep(Base other_) { 1651 if (!super.equalsDeep(other_)) 1652 return false; 1653 if (!(other_ instanceof VerificationResultAttestationComponent)) 1654 return false; 1655 VerificationResultAttestationComponent o = (VerificationResultAttestationComponent) other_; 1656 return compareDeep(who, o.who, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) 1657 && compareDeep(communicationMethod, o.communicationMethod, true) && compareDeep(date, o.date, true) 1658 && compareDeep(sourceIdentityCertificate, o.sourceIdentityCertificate, true) 1659 && compareDeep(proxyIdentityCertificate, o.proxyIdentityCertificate, true) 1660 && compareDeep(proxySignature, o.proxySignature, true) 1661 && compareDeep(sourceSignature, o.sourceSignature, true); 1662 } 1663 1664 @Override 1665 public boolean equalsShallow(Base other_) { 1666 if (!super.equalsShallow(other_)) 1667 return false; 1668 if (!(other_ instanceof VerificationResultAttestationComponent)) 1669 return false; 1670 VerificationResultAttestationComponent o = (VerificationResultAttestationComponent) other_; 1671 return compareValues(date, o.date, true) 1672 && compareValues(sourceIdentityCertificate, o.sourceIdentityCertificate, true) 1673 && compareValues(proxyIdentityCertificate, o.proxyIdentityCertificate, true); 1674 } 1675 1676 public boolean isEmpty() { 1677 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(who, onBehalfOf, communicationMethod, date, 1678 sourceIdentityCertificate, proxyIdentityCertificate, proxySignature, sourceSignature); 1679 } 1680 1681 public String fhirType() { 1682 return "VerificationResult.attestation"; 1683 1684 } 1685 1686 } 1687 1688 @Block() 1689 public static class VerificationResultValidatorComponent extends BackboneElement implements IBaseBackboneElement { 1690 /** 1691 * Reference to the organization validating information. 1692 */ 1693 @Child(name = "organization", type = { 1694 Organization.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1695 @Description(shortDefinition = "Reference to the organization validating information", formalDefinition = "Reference to the organization validating information.") 1696 protected Reference organization; 1697 1698 /** 1699 * The actual object that is the target of the reference (Reference to the 1700 * organization validating information.) 1701 */ 1702 protected Organization organizationTarget; 1703 1704 /** 1705 * A digital identity certificate associated with the validator. 1706 */ 1707 @Child(name = "identityCertificate", type = { 1708 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1709 @Description(shortDefinition = "A digital identity certificate associated with the validator", formalDefinition = "A digital identity certificate associated with the validator.") 1710 protected StringType identityCertificate; 1711 1712 /** 1713 * Signed assertion by the validator that they have validated the information. 1714 */ 1715 @Child(name = "attestationSignature", type = { 1716 Signature.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1717 @Description(shortDefinition = "Validator signature", formalDefinition = "Signed assertion by the validator that they have validated the information.") 1718 protected Signature attestationSignature; 1719 1720 private static final long serialVersionUID = 35580619L; 1721 1722 /** 1723 * Constructor 1724 */ 1725 public VerificationResultValidatorComponent() { 1726 super(); 1727 } 1728 1729 /** 1730 * Constructor 1731 */ 1732 public VerificationResultValidatorComponent(Reference organization) { 1733 super(); 1734 this.organization = organization; 1735 } 1736 1737 /** 1738 * @return {@link #organization} (Reference to the organization validating 1739 * information.) 1740 */ 1741 public Reference getOrganization() { 1742 if (this.organization == null) 1743 if (Configuration.errorOnAutoCreate()) 1744 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.organization"); 1745 else if (Configuration.doAutoCreate()) 1746 this.organization = new Reference(); // cc 1747 return this.organization; 1748 } 1749 1750 public boolean hasOrganization() { 1751 return this.organization != null && !this.organization.isEmpty(); 1752 } 1753 1754 /** 1755 * @param value {@link #organization} (Reference to the organization validating 1756 * information.) 1757 */ 1758 public VerificationResultValidatorComponent setOrganization(Reference value) { 1759 this.organization = value; 1760 return this; 1761 } 1762 1763 /** 1764 * @return {@link #organization} The actual object that is the target of the 1765 * reference. The reference library doesn't populate this, but you can 1766 * use it to hold the resource if you resolve it. (Reference to the 1767 * organization validating information.) 1768 */ 1769 public Organization getOrganizationTarget() { 1770 if (this.organizationTarget == null) 1771 if (Configuration.errorOnAutoCreate()) 1772 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.organization"); 1773 else if (Configuration.doAutoCreate()) 1774 this.organizationTarget = new Organization(); // aa 1775 return this.organizationTarget; 1776 } 1777 1778 /** 1779 * @param value {@link #organization} The actual object that is the target of 1780 * the reference. The reference library doesn't use these, but you 1781 * can use it to hold the resource if you resolve it. (Reference to 1782 * the organization validating information.) 1783 */ 1784 public VerificationResultValidatorComponent setOrganizationTarget(Organization value) { 1785 this.organizationTarget = value; 1786 return this; 1787 } 1788 1789 /** 1790 * @return {@link #identityCertificate} (A digital identity certificate 1791 * associated with the validator.). This is the underlying object with 1792 * id, value and extensions. The accessor "getIdentityCertificate" gives 1793 * direct access to the value 1794 */ 1795 public StringType getIdentityCertificateElement() { 1796 if (this.identityCertificate == null) 1797 if (Configuration.errorOnAutoCreate()) 1798 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.identityCertificate"); 1799 else if (Configuration.doAutoCreate()) 1800 this.identityCertificate = new StringType(); // bb 1801 return this.identityCertificate; 1802 } 1803 1804 public boolean hasIdentityCertificateElement() { 1805 return this.identityCertificate != null && !this.identityCertificate.isEmpty(); 1806 } 1807 1808 public boolean hasIdentityCertificate() { 1809 return this.identityCertificate != null && !this.identityCertificate.isEmpty(); 1810 } 1811 1812 /** 1813 * @param value {@link #identityCertificate} (A digital identity certificate 1814 * associated with the validator.). This is the underlying object 1815 * with id, value and extensions. The accessor 1816 * "getIdentityCertificate" gives direct access to the value 1817 */ 1818 public VerificationResultValidatorComponent setIdentityCertificateElement(StringType value) { 1819 this.identityCertificate = value; 1820 return this; 1821 } 1822 1823 /** 1824 * @return A digital identity certificate associated with the validator. 1825 */ 1826 public String getIdentityCertificate() { 1827 return this.identityCertificate == null ? null : this.identityCertificate.getValue(); 1828 } 1829 1830 /** 1831 * @param value A digital identity certificate associated with the validator. 1832 */ 1833 public VerificationResultValidatorComponent setIdentityCertificate(String value) { 1834 if (Utilities.noString(value)) 1835 this.identityCertificate = null; 1836 else { 1837 if (this.identityCertificate == null) 1838 this.identityCertificate = new StringType(); 1839 this.identityCertificate.setValue(value); 1840 } 1841 return this; 1842 } 1843 1844 /** 1845 * @return {@link #attestationSignature} (Signed assertion by the validator that 1846 * they have validated the information.) 1847 */ 1848 public Signature getAttestationSignature() { 1849 if (this.attestationSignature == null) 1850 if (Configuration.errorOnAutoCreate()) 1851 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.attestationSignature"); 1852 else if (Configuration.doAutoCreate()) 1853 this.attestationSignature = new Signature(); // cc 1854 return this.attestationSignature; 1855 } 1856 1857 public boolean hasAttestationSignature() { 1858 return this.attestationSignature != null && !this.attestationSignature.isEmpty(); 1859 } 1860 1861 /** 1862 * @param value {@link #attestationSignature} (Signed assertion by the validator 1863 * that they have validated the information.) 1864 */ 1865 public VerificationResultValidatorComponent setAttestationSignature(Signature value) { 1866 this.attestationSignature = value; 1867 return this; 1868 } 1869 1870 protected void listChildren(List<Property> children) { 1871 super.listChildren(children); 1872 children.add(new Property("organization", "Reference(Organization)", 1873 "Reference to the organization validating information.", 0, 1, organization)); 1874 children.add(new Property("identityCertificate", "string", 1875 "A digital identity certificate associated with the validator.", 0, 1, identityCertificate)); 1876 children.add(new Property("attestationSignature", "Signature", 1877 "Signed assertion by the validator that they have validated the information.", 0, 1, attestationSignature)); 1878 } 1879 1880 @Override 1881 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1882 switch (_hash) { 1883 case 1178922291: 1884 /* organization */ return new Property("organization", "Reference(Organization)", 1885 "Reference to the organization validating information.", 0, 1, organization); 1886 case -854379015: 1887 /* identityCertificate */ return new Property("identityCertificate", "string", 1888 "A digital identity certificate associated with the validator.", 0, 1, identityCertificate); 1889 case -184196152: 1890 /* attestationSignature */ return new Property("attestationSignature", "Signature", 1891 "Signed assertion by the validator that they have validated the information.", 0, 1, attestationSignature); 1892 default: 1893 return super.getNamedProperty(_hash, _name, _checkValid); 1894 } 1895 1896 } 1897 1898 @Override 1899 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1900 switch (hash) { 1901 case 1178922291: 1902 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 1903 case -854379015: 1904 /* identityCertificate */ return this.identityCertificate == null ? new Base[0] 1905 : new Base[] { this.identityCertificate }; // StringType 1906 case -184196152: 1907 /* attestationSignature */ return this.attestationSignature == null ? new Base[0] 1908 : new Base[] { this.attestationSignature }; // Signature 1909 default: 1910 return super.getProperty(hash, name, checkValid); 1911 } 1912 1913 } 1914 1915 @Override 1916 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1917 switch (hash) { 1918 case 1178922291: // organization 1919 this.organization = castToReference(value); // Reference 1920 return value; 1921 case -854379015: // identityCertificate 1922 this.identityCertificate = castToString(value); // StringType 1923 return value; 1924 case -184196152: // attestationSignature 1925 this.attestationSignature = castToSignature(value); // Signature 1926 return value; 1927 default: 1928 return super.setProperty(hash, name, value); 1929 } 1930 1931 } 1932 1933 @Override 1934 public Base setProperty(String name, Base value) throws FHIRException { 1935 if (name.equals("organization")) { 1936 this.organization = castToReference(value); // Reference 1937 } else if (name.equals("identityCertificate")) { 1938 this.identityCertificate = castToString(value); // StringType 1939 } else if (name.equals("attestationSignature")) { 1940 this.attestationSignature = castToSignature(value); // Signature 1941 } else 1942 return super.setProperty(name, value); 1943 return value; 1944 } 1945 1946 @Override 1947 public void removeChild(String name, Base value) throws FHIRException { 1948 if (name.equals("organization")) { 1949 this.organization = null; 1950 } else if (name.equals("identityCertificate")) { 1951 this.identityCertificate = null; 1952 } else if (name.equals("attestationSignature")) { 1953 this.attestationSignature = null; 1954 } else 1955 super.removeChild(name, value); 1956 1957 } 1958 1959 @Override 1960 public Base makeProperty(int hash, String name) throws FHIRException { 1961 switch (hash) { 1962 case 1178922291: 1963 return getOrganization(); 1964 case -854379015: 1965 return getIdentityCertificateElement(); 1966 case -184196152: 1967 return getAttestationSignature(); 1968 default: 1969 return super.makeProperty(hash, name); 1970 } 1971 1972 } 1973 1974 @Override 1975 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1976 switch (hash) { 1977 case 1178922291: 1978 /* organization */ return new String[] { "Reference" }; 1979 case -854379015: 1980 /* identityCertificate */ return new String[] { "string" }; 1981 case -184196152: 1982 /* attestationSignature */ return new String[] { "Signature" }; 1983 default: 1984 return super.getTypesForProperty(hash, name); 1985 } 1986 1987 } 1988 1989 @Override 1990 public Base addChild(String name) throws FHIRException { 1991 if (name.equals("organization")) { 1992 this.organization = new Reference(); 1993 return this.organization; 1994 } else if (name.equals("identityCertificate")) { 1995 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.identityCertificate"); 1996 } else if (name.equals("attestationSignature")) { 1997 this.attestationSignature = new Signature(); 1998 return this.attestationSignature; 1999 } else 2000 return super.addChild(name); 2001 } 2002 2003 public VerificationResultValidatorComponent copy() { 2004 VerificationResultValidatorComponent dst = new VerificationResultValidatorComponent(); 2005 copyValues(dst); 2006 return dst; 2007 } 2008 2009 public void copyValues(VerificationResultValidatorComponent dst) { 2010 super.copyValues(dst); 2011 dst.organization = organization == null ? null : organization.copy(); 2012 dst.identityCertificate = identityCertificate == null ? null : identityCertificate.copy(); 2013 dst.attestationSignature = attestationSignature == null ? null : attestationSignature.copy(); 2014 } 2015 2016 @Override 2017 public boolean equalsDeep(Base other_) { 2018 if (!super.equalsDeep(other_)) 2019 return false; 2020 if (!(other_ instanceof VerificationResultValidatorComponent)) 2021 return false; 2022 VerificationResultValidatorComponent o = (VerificationResultValidatorComponent) other_; 2023 return compareDeep(organization, o.organization, true) 2024 && compareDeep(identityCertificate, o.identityCertificate, true) 2025 && compareDeep(attestationSignature, o.attestationSignature, true); 2026 } 2027 2028 @Override 2029 public boolean equalsShallow(Base other_) { 2030 if (!super.equalsShallow(other_)) 2031 return false; 2032 if (!(other_ instanceof VerificationResultValidatorComponent)) 2033 return false; 2034 VerificationResultValidatorComponent o = (VerificationResultValidatorComponent) other_; 2035 return compareValues(identityCertificate, o.identityCertificate, true); 2036 } 2037 2038 public boolean isEmpty() { 2039 return super.isEmpty() 2040 && ca.uhn.fhir.util.ElementUtil.isEmpty(organization, identityCertificate, attestationSignature); 2041 } 2042 2043 public String fhirType() { 2044 return "VerificationResult.validator"; 2045 2046 } 2047 2048 } 2049 2050 /** 2051 * A resource that was validated. 2052 */ 2053 @Child(name = "target", type = { 2054 Reference.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2055 @Description(shortDefinition = "A resource that was validated", formalDefinition = "A resource that was validated.") 2056 protected List<Reference> target; 2057 /** 2058 * The actual objects that are the target of the reference (A resource that was 2059 * validated.) 2060 */ 2061 protected List<Resource> targetTarget; 2062 2063 /** 2064 * The fhirpath location(s) within the resource that was validated. 2065 */ 2066 @Child(name = "targetLocation", type = { 2067 StringType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2068 @Description(shortDefinition = "The fhirpath location(s) within the resource that was validated", formalDefinition = "The fhirpath location(s) within the resource that was validated.") 2069 protected List<StringType> targetLocation; 2070 2071 /** 2072 * The frequency with which the target must be validated (none; initial; 2073 * periodic). 2074 */ 2075 @Child(name = "need", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2076 @Description(shortDefinition = "none | initial | periodic", formalDefinition = "The frequency with which the target must be validated (none; initial; periodic).") 2077 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-need") 2078 protected CodeableConcept need; 2079 2080 /** 2081 * The validation status of the target (attested; validated; in process; 2082 * requires revalidation; validation failed; revalidation failed). 2083 */ 2084 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2085 @Description(shortDefinition = "attested | validated | in-process | req-revalid | val-fail | reval-fail", formalDefinition = "The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).") 2086 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-status") 2087 protected Enumeration<Status> status; 2088 2089 /** 2090 * When the validation status was updated. 2091 */ 2092 @Child(name = "statusDate", type = { 2093 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2094 @Description(shortDefinition = "When the validation status was updated", formalDefinition = "When the validation status was updated.") 2095 protected DateTimeType statusDate; 2096 2097 /** 2098 * What the target is validated against (nothing; primary source; multiple 2099 * sources). 2100 */ 2101 @Child(name = "validationType", type = { 2102 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2103 @Description(shortDefinition = "nothing | primary | multiple", formalDefinition = "What the target is validated against (nothing; primary source; multiple sources).") 2104 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-validation-type") 2105 protected CodeableConcept validationType; 2106 2107 /** 2108 * The primary process by which the target is validated (edit check; value set; 2109 * primary source; multiple sources; standalone; in context). 2110 */ 2111 @Child(name = "validationProcess", type = { 2112 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2113 @Description(shortDefinition = "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context)", formalDefinition = "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).") 2114 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-validation-process") 2115 protected List<CodeableConcept> validationProcess; 2116 2117 /** 2118 * Frequency of revalidation. 2119 */ 2120 @Child(name = "frequency", type = { Timing.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2121 @Description(shortDefinition = "Frequency of revalidation", formalDefinition = "Frequency of revalidation.") 2122 protected Timing frequency; 2123 2124 /** 2125 * The date/time validation was last completed (including failed validations). 2126 */ 2127 @Child(name = "lastPerformed", type = { 2128 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2129 @Description(shortDefinition = "The date/time validation was last completed (including failed validations)", formalDefinition = "The date/time validation was last completed (including failed validations).") 2130 protected DateTimeType lastPerformed; 2131 2132 /** 2133 * The date when target is next validated, if appropriate. 2134 */ 2135 @Child(name = "nextScheduled", type = { 2136 DateType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 2137 @Description(shortDefinition = "The date when target is next validated, if appropriate", formalDefinition = "The date when target is next validated, if appropriate.") 2138 protected DateType nextScheduled; 2139 2140 /** 2141 * The result if validation fails (fatal; warning; record only; none). 2142 */ 2143 @Child(name = "failureAction", type = { 2144 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 2145 @Description(shortDefinition = "fatal | warn | rec-only | none", formalDefinition = "The result if validation fails (fatal; warning; record only; none).") 2146 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-failure-action") 2147 protected CodeableConcept failureAction; 2148 2149 /** 2150 * Information about the primary source(s) involved in validation. 2151 */ 2152 @Child(name = "primarySource", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2153 @Description(shortDefinition = "Information about the primary source(s) involved in validation", formalDefinition = "Information about the primary source(s) involved in validation.") 2154 protected List<VerificationResultPrimarySourceComponent> primarySource; 2155 2156 /** 2157 * Information about the entity attesting to information. 2158 */ 2159 @Child(name = "attestation", type = {}, order = 12, min = 0, max = 1, modifier = false, summary = false) 2160 @Description(shortDefinition = "Information about the entity attesting to information", formalDefinition = "Information about the entity attesting to information.") 2161 protected VerificationResultAttestationComponent attestation; 2162 2163 /** 2164 * Information about the entity validating information. 2165 */ 2166 @Child(name = "validator", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2167 @Description(shortDefinition = "Information about the entity validating information", formalDefinition = "Information about the entity validating information.") 2168 protected List<VerificationResultValidatorComponent> validator; 2169 2170 private static final long serialVersionUID = -284059204L; 2171 2172 /** 2173 * Constructor 2174 */ 2175 public VerificationResult() { 2176 super(); 2177 } 2178 2179 /** 2180 * Constructor 2181 */ 2182 public VerificationResult(Enumeration<Status> status) { 2183 super(); 2184 this.status = status; 2185 } 2186 2187 /** 2188 * @return {@link #target} (A resource that was validated.) 2189 */ 2190 public List<Reference> getTarget() { 2191 if (this.target == null) 2192 this.target = new ArrayList<Reference>(); 2193 return this.target; 2194 } 2195 2196 /** 2197 * @return Returns a reference to <code>this</code> for easy method chaining 2198 */ 2199 public VerificationResult setTarget(List<Reference> theTarget) { 2200 this.target = theTarget; 2201 return this; 2202 } 2203 2204 public boolean hasTarget() { 2205 if (this.target == null) 2206 return false; 2207 for (Reference item : this.target) 2208 if (!item.isEmpty()) 2209 return true; 2210 return false; 2211 } 2212 2213 public Reference addTarget() { // 3 2214 Reference t = new Reference(); 2215 if (this.target == null) 2216 this.target = new ArrayList<Reference>(); 2217 this.target.add(t); 2218 return t; 2219 } 2220 2221 public VerificationResult addTarget(Reference t) { // 3 2222 if (t == null) 2223 return this; 2224 if (this.target == null) 2225 this.target = new ArrayList<Reference>(); 2226 this.target.add(t); 2227 return this; 2228 } 2229 2230 /** 2231 * @return The first repetition of repeating field {@link #target}, creating it 2232 * if it does not already exist 2233 */ 2234 public Reference getTargetFirstRep() { 2235 if (getTarget().isEmpty()) { 2236 addTarget(); 2237 } 2238 return getTarget().get(0); 2239 } 2240 2241 /** 2242 * @deprecated Use Reference#setResource(IBaseResource) instead 2243 */ 2244 @Deprecated 2245 public List<Resource> getTargetTarget() { 2246 if (this.targetTarget == null) 2247 this.targetTarget = new ArrayList<Resource>(); 2248 return this.targetTarget; 2249 } 2250 2251 /** 2252 * @return {@link #targetLocation} (The fhirpath location(s) within the resource 2253 * that was validated.) 2254 */ 2255 public List<StringType> getTargetLocation() { 2256 if (this.targetLocation == null) 2257 this.targetLocation = new ArrayList<StringType>(); 2258 return this.targetLocation; 2259 } 2260 2261 /** 2262 * @return Returns a reference to <code>this</code> for easy method chaining 2263 */ 2264 public VerificationResult setTargetLocation(List<StringType> theTargetLocation) { 2265 this.targetLocation = theTargetLocation; 2266 return this; 2267 } 2268 2269 public boolean hasTargetLocation() { 2270 if (this.targetLocation == null) 2271 return false; 2272 for (StringType item : this.targetLocation) 2273 if (!item.isEmpty()) 2274 return true; 2275 return false; 2276 } 2277 2278 /** 2279 * @return {@link #targetLocation} (The fhirpath location(s) within the resource 2280 * that was validated.) 2281 */ 2282 public StringType addTargetLocationElement() {// 2 2283 StringType t = new StringType(); 2284 if (this.targetLocation == null) 2285 this.targetLocation = new ArrayList<StringType>(); 2286 this.targetLocation.add(t); 2287 return t; 2288 } 2289 2290 /** 2291 * @param value {@link #targetLocation} (The fhirpath location(s) within the 2292 * resource that was validated.) 2293 */ 2294 public VerificationResult addTargetLocation(String value) { // 1 2295 StringType t = new StringType(); 2296 t.setValue(value); 2297 if (this.targetLocation == null) 2298 this.targetLocation = new ArrayList<StringType>(); 2299 this.targetLocation.add(t); 2300 return this; 2301 } 2302 2303 /** 2304 * @param value {@link #targetLocation} (The fhirpath location(s) within the 2305 * resource that was validated.) 2306 */ 2307 public boolean hasTargetLocation(String value) { 2308 if (this.targetLocation == null) 2309 return false; 2310 for (StringType v : this.targetLocation) 2311 if (v.getValue().equals(value)) // string 2312 return true; 2313 return false; 2314 } 2315 2316 /** 2317 * @return {@link #need} (The frequency with which the target must be validated 2318 * (none; initial; periodic).) 2319 */ 2320 public CodeableConcept getNeed() { 2321 if (this.need == null) 2322 if (Configuration.errorOnAutoCreate()) 2323 throw new Error("Attempt to auto-create VerificationResult.need"); 2324 else if (Configuration.doAutoCreate()) 2325 this.need = new CodeableConcept(); // cc 2326 return this.need; 2327 } 2328 2329 public boolean hasNeed() { 2330 return this.need != null && !this.need.isEmpty(); 2331 } 2332 2333 /** 2334 * @param value {@link #need} (The frequency with which the target must be 2335 * validated (none; initial; periodic).) 2336 */ 2337 public VerificationResult setNeed(CodeableConcept value) { 2338 this.need = value; 2339 return this; 2340 } 2341 2342 /** 2343 * @return {@link #status} (The validation status of the target (attested; 2344 * validated; in process; requires revalidation; validation failed; 2345 * revalidation failed).). This is the underlying object with id, value 2346 * and extensions. The accessor "getStatus" gives direct access to the 2347 * value 2348 */ 2349 public Enumeration<Status> getStatusElement() { 2350 if (this.status == null) 2351 if (Configuration.errorOnAutoCreate()) 2352 throw new Error("Attempt to auto-create VerificationResult.status"); 2353 else if (Configuration.doAutoCreate()) 2354 this.status = new Enumeration<Status>(new StatusEnumFactory()); // bb 2355 return this.status; 2356 } 2357 2358 public boolean hasStatusElement() { 2359 return this.status != null && !this.status.isEmpty(); 2360 } 2361 2362 public boolean hasStatus() { 2363 return this.status != null && !this.status.isEmpty(); 2364 } 2365 2366 /** 2367 * @param value {@link #status} (The validation status of the target (attested; 2368 * validated; in process; requires revalidation; validation failed; 2369 * revalidation failed).). This is the underlying object with id, 2370 * value and extensions. The accessor "getStatus" gives direct 2371 * access to the value 2372 */ 2373 public VerificationResult setStatusElement(Enumeration<Status> value) { 2374 this.status = value; 2375 return this; 2376 } 2377 2378 /** 2379 * @return The validation status of the target (attested; validated; in process; 2380 * requires revalidation; validation failed; revalidation failed). 2381 */ 2382 public Status getStatus() { 2383 return this.status == null ? null : this.status.getValue(); 2384 } 2385 2386 /** 2387 * @param value The validation status of the target (attested; validated; in 2388 * process; requires revalidation; validation failed; revalidation 2389 * failed). 2390 */ 2391 public VerificationResult setStatus(Status value) { 2392 if (this.status == null) 2393 this.status = new Enumeration<Status>(new StatusEnumFactory()); 2394 this.status.setValue(value); 2395 return this; 2396 } 2397 2398 /** 2399 * @return {@link #statusDate} (When the validation status was updated.). This 2400 * is the underlying object with id, value and extensions. The accessor 2401 * "getStatusDate" gives direct access to the value 2402 */ 2403 public DateTimeType getStatusDateElement() { 2404 if (this.statusDate == null) 2405 if (Configuration.errorOnAutoCreate()) 2406 throw new Error("Attempt to auto-create VerificationResult.statusDate"); 2407 else if (Configuration.doAutoCreate()) 2408 this.statusDate = new DateTimeType(); // bb 2409 return this.statusDate; 2410 } 2411 2412 public boolean hasStatusDateElement() { 2413 return this.statusDate != null && !this.statusDate.isEmpty(); 2414 } 2415 2416 public boolean hasStatusDate() { 2417 return this.statusDate != null && !this.statusDate.isEmpty(); 2418 } 2419 2420 /** 2421 * @param value {@link #statusDate} (When the validation status was updated.). 2422 * This is the underlying object with id, value and extensions. The 2423 * accessor "getStatusDate" gives direct access to the value 2424 */ 2425 public VerificationResult setStatusDateElement(DateTimeType value) { 2426 this.statusDate = value; 2427 return this; 2428 } 2429 2430 /** 2431 * @return When the validation status was updated. 2432 */ 2433 public Date getStatusDate() { 2434 return this.statusDate == null ? null : this.statusDate.getValue(); 2435 } 2436 2437 /** 2438 * @param value When the validation status was updated. 2439 */ 2440 public VerificationResult setStatusDate(Date value) { 2441 if (value == null) 2442 this.statusDate = null; 2443 else { 2444 if (this.statusDate == null) 2445 this.statusDate = new DateTimeType(); 2446 this.statusDate.setValue(value); 2447 } 2448 return this; 2449 } 2450 2451 /** 2452 * @return {@link #validationType} (What the target is validated against 2453 * (nothing; primary source; multiple sources).) 2454 */ 2455 public CodeableConcept getValidationType() { 2456 if (this.validationType == null) 2457 if (Configuration.errorOnAutoCreate()) 2458 throw new Error("Attempt to auto-create VerificationResult.validationType"); 2459 else if (Configuration.doAutoCreate()) 2460 this.validationType = new CodeableConcept(); // cc 2461 return this.validationType; 2462 } 2463 2464 public boolean hasValidationType() { 2465 return this.validationType != null && !this.validationType.isEmpty(); 2466 } 2467 2468 /** 2469 * @param value {@link #validationType} (What the target is validated against 2470 * (nothing; primary source; multiple sources).) 2471 */ 2472 public VerificationResult setValidationType(CodeableConcept value) { 2473 this.validationType = value; 2474 return this; 2475 } 2476 2477 /** 2478 * @return {@link #validationProcess} (The primary process by which the target 2479 * is validated (edit check; value set; primary source; multiple 2480 * sources; standalone; in context).) 2481 */ 2482 public List<CodeableConcept> getValidationProcess() { 2483 if (this.validationProcess == null) 2484 this.validationProcess = new ArrayList<CodeableConcept>(); 2485 return this.validationProcess; 2486 } 2487 2488 /** 2489 * @return Returns a reference to <code>this</code> for easy method chaining 2490 */ 2491 public VerificationResult setValidationProcess(List<CodeableConcept> theValidationProcess) { 2492 this.validationProcess = theValidationProcess; 2493 return this; 2494 } 2495 2496 public boolean hasValidationProcess() { 2497 if (this.validationProcess == null) 2498 return false; 2499 for (CodeableConcept item : this.validationProcess) 2500 if (!item.isEmpty()) 2501 return true; 2502 return false; 2503 } 2504 2505 public CodeableConcept addValidationProcess() { // 3 2506 CodeableConcept t = new CodeableConcept(); 2507 if (this.validationProcess == null) 2508 this.validationProcess = new ArrayList<CodeableConcept>(); 2509 this.validationProcess.add(t); 2510 return t; 2511 } 2512 2513 public VerificationResult addValidationProcess(CodeableConcept t) { // 3 2514 if (t == null) 2515 return this; 2516 if (this.validationProcess == null) 2517 this.validationProcess = new ArrayList<CodeableConcept>(); 2518 this.validationProcess.add(t); 2519 return this; 2520 } 2521 2522 /** 2523 * @return The first repetition of repeating field {@link #validationProcess}, 2524 * creating it if it does not already exist 2525 */ 2526 public CodeableConcept getValidationProcessFirstRep() { 2527 if (getValidationProcess().isEmpty()) { 2528 addValidationProcess(); 2529 } 2530 return getValidationProcess().get(0); 2531 } 2532 2533 /** 2534 * @return {@link #frequency} (Frequency of revalidation.) 2535 */ 2536 public Timing getFrequency() { 2537 if (this.frequency == null) 2538 if (Configuration.errorOnAutoCreate()) 2539 throw new Error("Attempt to auto-create VerificationResult.frequency"); 2540 else if (Configuration.doAutoCreate()) 2541 this.frequency = new Timing(); // cc 2542 return this.frequency; 2543 } 2544 2545 public boolean hasFrequency() { 2546 return this.frequency != null && !this.frequency.isEmpty(); 2547 } 2548 2549 /** 2550 * @param value {@link #frequency} (Frequency of revalidation.) 2551 */ 2552 public VerificationResult setFrequency(Timing value) { 2553 this.frequency = value; 2554 return this; 2555 } 2556 2557 /** 2558 * @return {@link #lastPerformed} (The date/time validation was last completed 2559 * (including failed validations).). This is the underlying object with 2560 * id, value and extensions. The accessor "getLastPerformed" gives 2561 * direct access to the value 2562 */ 2563 public DateTimeType getLastPerformedElement() { 2564 if (this.lastPerformed == null) 2565 if (Configuration.errorOnAutoCreate()) 2566 throw new Error("Attempt to auto-create VerificationResult.lastPerformed"); 2567 else if (Configuration.doAutoCreate()) 2568 this.lastPerformed = new DateTimeType(); // bb 2569 return this.lastPerformed; 2570 } 2571 2572 public boolean hasLastPerformedElement() { 2573 return this.lastPerformed != null && !this.lastPerformed.isEmpty(); 2574 } 2575 2576 public boolean hasLastPerformed() { 2577 return this.lastPerformed != null && !this.lastPerformed.isEmpty(); 2578 } 2579 2580 /** 2581 * @param value {@link #lastPerformed} (The date/time validation was last 2582 * completed (including failed validations).). This is the 2583 * underlying object with id, value and extensions. The accessor 2584 * "getLastPerformed" gives direct access to the value 2585 */ 2586 public VerificationResult setLastPerformedElement(DateTimeType value) { 2587 this.lastPerformed = value; 2588 return this; 2589 } 2590 2591 /** 2592 * @return The date/time validation was last completed (including failed 2593 * validations). 2594 */ 2595 public Date getLastPerformed() { 2596 return this.lastPerformed == null ? null : this.lastPerformed.getValue(); 2597 } 2598 2599 /** 2600 * @param value The date/time validation was last completed (including failed 2601 * validations). 2602 */ 2603 public VerificationResult setLastPerformed(Date value) { 2604 if (value == null) 2605 this.lastPerformed = null; 2606 else { 2607 if (this.lastPerformed == null) 2608 this.lastPerformed = new DateTimeType(); 2609 this.lastPerformed.setValue(value); 2610 } 2611 return this; 2612 } 2613 2614 /** 2615 * @return {@link #nextScheduled} (The date when target is next validated, if 2616 * appropriate.). This is the underlying object with id, value and 2617 * extensions. The accessor "getNextScheduled" gives direct access to 2618 * the value 2619 */ 2620 public DateType getNextScheduledElement() { 2621 if (this.nextScheduled == null) 2622 if (Configuration.errorOnAutoCreate()) 2623 throw new Error("Attempt to auto-create VerificationResult.nextScheduled"); 2624 else if (Configuration.doAutoCreate()) 2625 this.nextScheduled = new DateType(); // bb 2626 return this.nextScheduled; 2627 } 2628 2629 public boolean hasNextScheduledElement() { 2630 return this.nextScheduled != null && !this.nextScheduled.isEmpty(); 2631 } 2632 2633 public boolean hasNextScheduled() { 2634 return this.nextScheduled != null && !this.nextScheduled.isEmpty(); 2635 } 2636 2637 /** 2638 * @param value {@link #nextScheduled} (The date when target is next validated, 2639 * if appropriate.). This is the underlying object with id, value 2640 * and extensions. The accessor "getNextScheduled" gives direct 2641 * access to the value 2642 */ 2643 public VerificationResult setNextScheduledElement(DateType value) { 2644 this.nextScheduled = value; 2645 return this; 2646 } 2647 2648 /** 2649 * @return The date when target is next validated, if appropriate. 2650 */ 2651 public Date getNextScheduled() { 2652 return this.nextScheduled == null ? null : this.nextScheduled.getValue(); 2653 } 2654 2655 /** 2656 * @param value The date when target is next validated, if appropriate. 2657 */ 2658 public VerificationResult setNextScheduled(Date value) { 2659 if (value == null) 2660 this.nextScheduled = null; 2661 else { 2662 if (this.nextScheduled == null) 2663 this.nextScheduled = new DateType(); 2664 this.nextScheduled.setValue(value); 2665 } 2666 return this; 2667 } 2668 2669 /** 2670 * @return {@link #failureAction} (The result if validation fails (fatal; 2671 * warning; record only; none).) 2672 */ 2673 public CodeableConcept getFailureAction() { 2674 if (this.failureAction == null) 2675 if (Configuration.errorOnAutoCreate()) 2676 throw new Error("Attempt to auto-create VerificationResult.failureAction"); 2677 else if (Configuration.doAutoCreate()) 2678 this.failureAction = new CodeableConcept(); // cc 2679 return this.failureAction; 2680 } 2681 2682 public boolean hasFailureAction() { 2683 return this.failureAction != null && !this.failureAction.isEmpty(); 2684 } 2685 2686 /** 2687 * @param value {@link #failureAction} (The result if validation fails (fatal; 2688 * warning; record only; none).) 2689 */ 2690 public VerificationResult setFailureAction(CodeableConcept value) { 2691 this.failureAction = value; 2692 return this; 2693 } 2694 2695 /** 2696 * @return {@link #primarySource} (Information about the primary source(s) 2697 * involved in validation.) 2698 */ 2699 public List<VerificationResultPrimarySourceComponent> getPrimarySource() { 2700 if (this.primarySource == null) 2701 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2702 return this.primarySource; 2703 } 2704 2705 /** 2706 * @return Returns a reference to <code>this</code> for easy method chaining 2707 */ 2708 public VerificationResult setPrimarySource(List<VerificationResultPrimarySourceComponent> thePrimarySource) { 2709 this.primarySource = thePrimarySource; 2710 return this; 2711 } 2712 2713 public boolean hasPrimarySource() { 2714 if (this.primarySource == null) 2715 return false; 2716 for (VerificationResultPrimarySourceComponent item : this.primarySource) 2717 if (!item.isEmpty()) 2718 return true; 2719 return false; 2720 } 2721 2722 public VerificationResultPrimarySourceComponent addPrimarySource() { // 3 2723 VerificationResultPrimarySourceComponent t = new VerificationResultPrimarySourceComponent(); 2724 if (this.primarySource == null) 2725 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2726 this.primarySource.add(t); 2727 return t; 2728 } 2729 2730 public VerificationResult addPrimarySource(VerificationResultPrimarySourceComponent t) { // 3 2731 if (t == null) 2732 return this; 2733 if (this.primarySource == null) 2734 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2735 this.primarySource.add(t); 2736 return this; 2737 } 2738 2739 /** 2740 * @return The first repetition of repeating field {@link #primarySource}, 2741 * creating it if it does not already exist 2742 */ 2743 public VerificationResultPrimarySourceComponent getPrimarySourceFirstRep() { 2744 if (getPrimarySource().isEmpty()) { 2745 addPrimarySource(); 2746 } 2747 return getPrimarySource().get(0); 2748 } 2749 2750 /** 2751 * @return {@link #attestation} (Information about the entity attesting to 2752 * information.) 2753 */ 2754 public VerificationResultAttestationComponent getAttestation() { 2755 if (this.attestation == null) 2756 if (Configuration.errorOnAutoCreate()) 2757 throw new Error("Attempt to auto-create VerificationResult.attestation"); 2758 else if (Configuration.doAutoCreate()) 2759 this.attestation = new VerificationResultAttestationComponent(); // cc 2760 return this.attestation; 2761 } 2762 2763 public boolean hasAttestation() { 2764 return this.attestation != null && !this.attestation.isEmpty(); 2765 } 2766 2767 /** 2768 * @param value {@link #attestation} (Information about the entity attesting to 2769 * information.) 2770 */ 2771 public VerificationResult setAttestation(VerificationResultAttestationComponent value) { 2772 this.attestation = value; 2773 return this; 2774 } 2775 2776 /** 2777 * @return {@link #validator} (Information about the entity validating 2778 * information.) 2779 */ 2780 public List<VerificationResultValidatorComponent> getValidator() { 2781 if (this.validator == null) 2782 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2783 return this.validator; 2784 } 2785 2786 /** 2787 * @return Returns a reference to <code>this</code> for easy method chaining 2788 */ 2789 public VerificationResult setValidator(List<VerificationResultValidatorComponent> theValidator) { 2790 this.validator = theValidator; 2791 return this; 2792 } 2793 2794 public boolean hasValidator() { 2795 if (this.validator == null) 2796 return false; 2797 for (VerificationResultValidatorComponent item : this.validator) 2798 if (!item.isEmpty()) 2799 return true; 2800 return false; 2801 } 2802 2803 public VerificationResultValidatorComponent addValidator() { // 3 2804 VerificationResultValidatorComponent t = new VerificationResultValidatorComponent(); 2805 if (this.validator == null) 2806 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2807 this.validator.add(t); 2808 return t; 2809 } 2810 2811 public VerificationResult addValidator(VerificationResultValidatorComponent t) { // 3 2812 if (t == null) 2813 return this; 2814 if (this.validator == null) 2815 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2816 this.validator.add(t); 2817 return this; 2818 } 2819 2820 /** 2821 * @return The first repetition of repeating field {@link #validator}, creating 2822 * it if it does not already exist 2823 */ 2824 public VerificationResultValidatorComponent getValidatorFirstRep() { 2825 if (getValidator().isEmpty()) { 2826 addValidator(); 2827 } 2828 return getValidator().get(0); 2829 } 2830 2831 protected void listChildren(List<Property> children) { 2832 super.listChildren(children); 2833 children.add(new Property("target", "Reference(Any)", "A resource that was validated.", 0, 2834 java.lang.Integer.MAX_VALUE, target)); 2835 children.add( 2836 new Property("targetLocation", "string", "The fhirpath location(s) within the resource that was validated.", 0, 2837 java.lang.Integer.MAX_VALUE, targetLocation)); 2838 children.add(new Property("need", "CodeableConcept", 2839 "The frequency with which the target must be validated (none; initial; periodic).", 0, 1, need)); 2840 children.add(new Property("status", "code", 2841 "The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).", 2842 0, 1, status)); 2843 children.add(new Property("statusDate", "dateTime", "When the validation status was updated.", 0, 1, statusDate)); 2844 children.add(new Property("validationType", "CodeableConcept", 2845 "What the target is validated against (nothing; primary source; multiple sources).", 0, 1, validationType)); 2846 children.add(new Property("validationProcess", "CodeableConcept", 2847 "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).", 2848 0, java.lang.Integer.MAX_VALUE, validationProcess)); 2849 children.add(new Property("frequency", "Timing", "Frequency of revalidation.", 0, 1, frequency)); 2850 children.add(new Property("lastPerformed", "dateTime", 2851 "The date/time validation was last completed (including failed validations).", 0, 1, lastPerformed)); 2852 children.add(new Property("nextScheduled", "date", "The date when target is next validated, if appropriate.", 0, 1, 2853 nextScheduled)); 2854 children.add(new Property("failureAction", "CodeableConcept", 2855 "The result if validation fails (fatal; warning; record only; none).", 0, 1, failureAction)); 2856 children.add(new Property("primarySource", "", "Information about the primary source(s) involved in validation.", 0, 2857 java.lang.Integer.MAX_VALUE, primarySource)); 2858 children.add( 2859 new Property("attestation", "", "Information about the entity attesting to information.", 0, 1, attestation)); 2860 children.add(new Property("validator", "", "Information about the entity validating information.", 0, 2861 java.lang.Integer.MAX_VALUE, validator)); 2862 } 2863 2864 @Override 2865 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2866 switch (_hash) { 2867 case -880905839: 2868 /* target */ return new Property("target", "Reference(Any)", "A resource that was validated.", 0, 2869 java.lang.Integer.MAX_VALUE, target); 2870 case 308958310: 2871 /* targetLocation */ return new Property("targetLocation", "string", 2872 "The fhirpath location(s) within the resource that was validated.", 0, java.lang.Integer.MAX_VALUE, 2873 targetLocation); 2874 case 3377302: 2875 /* need */ return new Property("need", "CodeableConcept", 2876 "The frequency with which the target must be validated (none; initial; periodic).", 0, 1, need); 2877 case -892481550: 2878 /* status */ return new Property("status", "code", 2879 "The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).", 2880 0, 1, status); 2881 case 247524032: 2882 /* statusDate */ return new Property("statusDate", "dateTime", "When the validation status was updated.", 0, 1, 2883 statusDate); 2884 case -279681197: 2885 /* validationType */ return new Property("validationType", "CodeableConcept", 2886 "What the target is validated against (nothing; primary source; multiple sources).", 0, 1, validationType); 2887 case 797680566: 2888 /* validationProcess */ return new Property("validationProcess", "CodeableConcept", 2889 "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).", 2890 0, java.lang.Integer.MAX_VALUE, validationProcess); 2891 case -70023844: 2892 /* frequency */ return new Property("frequency", "Timing", "Frequency of revalidation.", 0, 1, frequency); 2893 case -1313229366: 2894 /* lastPerformed */ return new Property("lastPerformed", "dateTime", 2895 "The date/time validation was last completed (including failed validations).", 0, 1, lastPerformed); 2896 case 1874589434: 2897 /* nextScheduled */ return new Property("nextScheduled", "date", 2898 "The date when target is next validated, if appropriate.", 0, 1, nextScheduled); 2899 case 1816382560: 2900 /* failureAction */ return new Property("failureAction", "CodeableConcept", 2901 "The result if validation fails (fatal; warning; record only; none).", 0, 1, failureAction); 2902 case -528721731: 2903 /* primarySource */ return new Property("primarySource", "", 2904 "Information about the primary source(s) involved in validation.", 0, java.lang.Integer.MAX_VALUE, 2905 primarySource); 2906 case -709624112: 2907 /* attestation */ return new Property("attestation", "", "Information about the entity attesting to information.", 2908 0, 1, attestation); 2909 case -1109783726: 2910 /* validator */ return new Property("validator", "", "Information about the entity validating information.", 0, 2911 java.lang.Integer.MAX_VALUE, validator); 2912 default: 2913 return super.getNamedProperty(_hash, _name, _checkValid); 2914 } 2915 2916 } 2917 2918 @Override 2919 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2920 switch (hash) { 2921 case -880905839: 2922 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // Reference 2923 case 308958310: 2924 /* targetLocation */ return this.targetLocation == null ? new Base[0] 2925 : this.targetLocation.toArray(new Base[this.targetLocation.size()]); // StringType 2926 case 3377302: 2927 /* need */ return this.need == null ? new Base[0] : new Base[] { this.need }; // CodeableConcept 2928 case -892481550: 2929 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<Status> 2930 case 247524032: 2931 /* statusDate */ return this.statusDate == null ? new Base[0] : new Base[] { this.statusDate }; // DateTimeType 2932 case -279681197: 2933 /* validationType */ return this.validationType == null ? new Base[0] : new Base[] { this.validationType }; // CodeableConcept 2934 case 797680566: 2935 /* validationProcess */ return this.validationProcess == null ? new Base[0] 2936 : this.validationProcess.toArray(new Base[this.validationProcess.size()]); // CodeableConcept 2937 case -70023844: 2938 /* frequency */ return this.frequency == null ? new Base[0] : new Base[] { this.frequency }; // Timing 2939 case -1313229366: 2940 /* lastPerformed */ return this.lastPerformed == null ? new Base[0] : new Base[] { this.lastPerformed }; // DateTimeType 2941 case 1874589434: 2942 /* nextScheduled */ return this.nextScheduled == null ? new Base[0] : new Base[] { this.nextScheduled }; // DateType 2943 case 1816382560: 2944 /* failureAction */ return this.failureAction == null ? new Base[0] : new Base[] { this.failureAction }; // CodeableConcept 2945 case -528721731: 2946 /* primarySource */ return this.primarySource == null ? new Base[0] 2947 : this.primarySource.toArray(new Base[this.primarySource.size()]); // VerificationResultPrimarySourceComponent 2948 case -709624112: 2949 /* attestation */ return this.attestation == null ? new Base[0] : new Base[] { this.attestation }; // VerificationResultAttestationComponent 2950 case -1109783726: 2951 /* validator */ return this.validator == null ? new Base[0] 2952 : this.validator.toArray(new Base[this.validator.size()]); // VerificationResultValidatorComponent 2953 default: 2954 return super.getProperty(hash, name, checkValid); 2955 } 2956 2957 } 2958 2959 @Override 2960 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2961 switch (hash) { 2962 case -880905839: // target 2963 this.getTarget().add(castToReference(value)); // Reference 2964 return value; 2965 case 308958310: // targetLocation 2966 this.getTargetLocation().add(castToString(value)); // StringType 2967 return value; 2968 case 3377302: // need 2969 this.need = castToCodeableConcept(value); // CodeableConcept 2970 return value; 2971 case -892481550: // status 2972 value = new StatusEnumFactory().fromType(castToCode(value)); 2973 this.status = (Enumeration) value; // Enumeration<Status> 2974 return value; 2975 case 247524032: // statusDate 2976 this.statusDate = castToDateTime(value); // DateTimeType 2977 return value; 2978 case -279681197: // validationType 2979 this.validationType = castToCodeableConcept(value); // CodeableConcept 2980 return value; 2981 case 797680566: // validationProcess 2982 this.getValidationProcess().add(castToCodeableConcept(value)); // CodeableConcept 2983 return value; 2984 case -70023844: // frequency 2985 this.frequency = castToTiming(value); // Timing 2986 return value; 2987 case -1313229366: // lastPerformed 2988 this.lastPerformed = castToDateTime(value); // DateTimeType 2989 return value; 2990 case 1874589434: // nextScheduled 2991 this.nextScheduled = castToDate(value); // DateType 2992 return value; 2993 case 1816382560: // failureAction 2994 this.failureAction = castToCodeableConcept(value); // CodeableConcept 2995 return value; 2996 case -528721731: // primarySource 2997 this.getPrimarySource().add((VerificationResultPrimarySourceComponent) value); // VerificationResultPrimarySourceComponent 2998 return value; 2999 case -709624112: // attestation 3000 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 3001 return value; 3002 case -1109783726: // validator 3003 this.getValidator().add((VerificationResultValidatorComponent) value); // VerificationResultValidatorComponent 3004 return value; 3005 default: 3006 return super.setProperty(hash, name, value); 3007 } 3008 3009 } 3010 3011 @Override 3012 public Base setProperty(String name, Base value) throws FHIRException { 3013 if (name.equals("target")) { 3014 this.getTarget().add(castToReference(value)); 3015 } else if (name.equals("targetLocation")) { 3016 this.getTargetLocation().add(castToString(value)); 3017 } else if (name.equals("need")) { 3018 this.need = castToCodeableConcept(value); // CodeableConcept 3019 } else if (name.equals("status")) { 3020 value = new StatusEnumFactory().fromType(castToCode(value)); 3021 this.status = (Enumeration) value; // Enumeration<Status> 3022 } else if (name.equals("statusDate")) { 3023 this.statusDate = castToDateTime(value); // DateTimeType 3024 } else if (name.equals("validationType")) { 3025 this.validationType = castToCodeableConcept(value); // CodeableConcept 3026 } else if (name.equals("validationProcess")) { 3027 this.getValidationProcess().add(castToCodeableConcept(value)); 3028 } else if (name.equals("frequency")) { 3029 this.frequency = castToTiming(value); // Timing 3030 } else if (name.equals("lastPerformed")) { 3031 this.lastPerformed = castToDateTime(value); // DateTimeType 3032 } else if (name.equals("nextScheduled")) { 3033 this.nextScheduled = castToDate(value); // DateType 3034 } else if (name.equals("failureAction")) { 3035 this.failureAction = castToCodeableConcept(value); // CodeableConcept 3036 } else if (name.equals("primarySource")) { 3037 this.getPrimarySource().add((VerificationResultPrimarySourceComponent) value); 3038 } else if (name.equals("attestation")) { 3039 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 3040 } else if (name.equals("validator")) { 3041 this.getValidator().add((VerificationResultValidatorComponent) value); 3042 } else 3043 return super.setProperty(name, value); 3044 return value; 3045 } 3046 3047 @Override 3048 public void removeChild(String name, Base value) throws FHIRException { 3049 if (name.equals("target")) { 3050 this.getTarget().remove(castToReference(value)); 3051 } else if (name.equals("targetLocation")) { 3052 this.getTargetLocation().remove(castToString(value)); 3053 } else if (name.equals("need")) { 3054 this.need = null; 3055 } else if (name.equals("status")) { 3056 this.status = null; 3057 } else if (name.equals("statusDate")) { 3058 this.statusDate = null; 3059 } else if (name.equals("validationType")) { 3060 this.validationType = null; 3061 } else if (name.equals("validationProcess")) { 3062 this.getValidationProcess().remove(castToCodeableConcept(value)); 3063 } else if (name.equals("frequency")) { 3064 this.frequency = null; 3065 } else if (name.equals("lastPerformed")) { 3066 this.lastPerformed = null; 3067 } else if (name.equals("nextScheduled")) { 3068 this.nextScheduled = null; 3069 } else if (name.equals("failureAction")) { 3070 this.failureAction = null; 3071 } else if (name.equals("primarySource")) { 3072 this.getPrimarySource().remove((VerificationResultPrimarySourceComponent) value); 3073 } else if (name.equals("attestation")) { 3074 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 3075 } else if (name.equals("validator")) { 3076 this.getValidator().remove((VerificationResultValidatorComponent) value); 3077 } else 3078 super.removeChild(name, value); 3079 3080 } 3081 3082 @Override 3083 public Base makeProperty(int hash, String name) throws FHIRException { 3084 switch (hash) { 3085 case -880905839: 3086 return addTarget(); 3087 case 308958310: 3088 return addTargetLocationElement(); 3089 case 3377302: 3090 return getNeed(); 3091 case -892481550: 3092 return getStatusElement(); 3093 case 247524032: 3094 return getStatusDateElement(); 3095 case -279681197: 3096 return getValidationType(); 3097 case 797680566: 3098 return addValidationProcess(); 3099 case -70023844: 3100 return getFrequency(); 3101 case -1313229366: 3102 return getLastPerformedElement(); 3103 case 1874589434: 3104 return getNextScheduledElement(); 3105 case 1816382560: 3106 return getFailureAction(); 3107 case -528721731: 3108 return addPrimarySource(); 3109 case -709624112: 3110 return getAttestation(); 3111 case -1109783726: 3112 return addValidator(); 3113 default: 3114 return super.makeProperty(hash, name); 3115 } 3116 3117 } 3118 3119 @Override 3120 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3121 switch (hash) { 3122 case -880905839: 3123 /* target */ return new String[] { "Reference" }; 3124 case 308958310: 3125 /* targetLocation */ return new String[] { "string" }; 3126 case 3377302: 3127 /* need */ return new String[] { "CodeableConcept" }; 3128 case -892481550: 3129 /* status */ return new String[] { "code" }; 3130 case 247524032: 3131 /* statusDate */ return new String[] { "dateTime" }; 3132 case -279681197: 3133 /* validationType */ return new String[] { "CodeableConcept" }; 3134 case 797680566: 3135 /* validationProcess */ return new String[] { "CodeableConcept" }; 3136 case -70023844: 3137 /* frequency */ return new String[] { "Timing" }; 3138 case -1313229366: 3139 /* lastPerformed */ return new String[] { "dateTime" }; 3140 case 1874589434: 3141 /* nextScheduled */ return new String[] { "date" }; 3142 case 1816382560: 3143 /* failureAction */ return new String[] { "CodeableConcept" }; 3144 case -528721731: 3145 /* primarySource */ return new String[] {}; 3146 case -709624112: 3147 /* attestation */ return new String[] {}; 3148 case -1109783726: 3149 /* validator */ return new String[] {}; 3150 default: 3151 return super.getTypesForProperty(hash, name); 3152 } 3153 3154 } 3155 3156 @Override 3157 public Base addChild(String name) throws FHIRException { 3158 if (name.equals("target")) { 3159 return addTarget(); 3160 } else if (name.equals("targetLocation")) { 3161 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.targetLocation"); 3162 } else if (name.equals("need")) { 3163 this.need = new CodeableConcept(); 3164 return this.need; 3165 } else if (name.equals("status")) { 3166 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.status"); 3167 } else if (name.equals("statusDate")) { 3168 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.statusDate"); 3169 } else if (name.equals("validationType")) { 3170 this.validationType = new CodeableConcept(); 3171 return this.validationType; 3172 } else if (name.equals("validationProcess")) { 3173 return addValidationProcess(); 3174 } else if (name.equals("frequency")) { 3175 this.frequency = new Timing(); 3176 return this.frequency; 3177 } else if (name.equals("lastPerformed")) { 3178 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.lastPerformed"); 3179 } else if (name.equals("nextScheduled")) { 3180 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.nextScheduled"); 3181 } else if (name.equals("failureAction")) { 3182 this.failureAction = new CodeableConcept(); 3183 return this.failureAction; 3184 } else if (name.equals("primarySource")) { 3185 return addPrimarySource(); 3186 } else if (name.equals("attestation")) { 3187 this.attestation = new VerificationResultAttestationComponent(); 3188 return this.attestation; 3189 } else if (name.equals("validator")) { 3190 return addValidator(); 3191 } else 3192 return super.addChild(name); 3193 } 3194 3195 public String fhirType() { 3196 return "VerificationResult"; 3197 3198 } 3199 3200 public VerificationResult copy() { 3201 VerificationResult dst = new VerificationResult(); 3202 copyValues(dst); 3203 return dst; 3204 } 3205 3206 public void copyValues(VerificationResult dst) { 3207 super.copyValues(dst); 3208 if (target != null) { 3209 dst.target = new ArrayList<Reference>(); 3210 for (Reference i : target) 3211 dst.target.add(i.copy()); 3212 } 3213 ; 3214 if (targetLocation != null) { 3215 dst.targetLocation = new ArrayList<StringType>(); 3216 for (StringType i : targetLocation) 3217 dst.targetLocation.add(i.copy()); 3218 } 3219 ; 3220 dst.need = need == null ? null : need.copy(); 3221 dst.status = status == null ? null : status.copy(); 3222 dst.statusDate = statusDate == null ? null : statusDate.copy(); 3223 dst.validationType = validationType == null ? null : validationType.copy(); 3224 if (validationProcess != null) { 3225 dst.validationProcess = new ArrayList<CodeableConcept>(); 3226 for (CodeableConcept i : validationProcess) 3227 dst.validationProcess.add(i.copy()); 3228 } 3229 ; 3230 dst.frequency = frequency == null ? null : frequency.copy(); 3231 dst.lastPerformed = lastPerformed == null ? null : lastPerformed.copy(); 3232 dst.nextScheduled = nextScheduled == null ? null : nextScheduled.copy(); 3233 dst.failureAction = failureAction == null ? null : failureAction.copy(); 3234 if (primarySource != null) { 3235 dst.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 3236 for (VerificationResultPrimarySourceComponent i : primarySource) 3237 dst.primarySource.add(i.copy()); 3238 } 3239 ; 3240 dst.attestation = attestation == null ? null : attestation.copy(); 3241 if (validator != null) { 3242 dst.validator = new ArrayList<VerificationResultValidatorComponent>(); 3243 for (VerificationResultValidatorComponent i : validator) 3244 dst.validator.add(i.copy()); 3245 } 3246 ; 3247 } 3248 3249 protected VerificationResult typedCopy() { 3250 return copy(); 3251 } 3252 3253 @Override 3254 public boolean equalsDeep(Base other_) { 3255 if (!super.equalsDeep(other_)) 3256 return false; 3257 if (!(other_ instanceof VerificationResult)) 3258 return false; 3259 VerificationResult o = (VerificationResult) other_; 3260 return compareDeep(target, o.target, true) && compareDeep(targetLocation, o.targetLocation, true) 3261 && compareDeep(need, o.need, true) && compareDeep(status, o.status, true) 3262 && compareDeep(statusDate, o.statusDate, true) && compareDeep(validationType, o.validationType, true) 3263 && compareDeep(validationProcess, o.validationProcess, true) && compareDeep(frequency, o.frequency, true) 3264 && compareDeep(lastPerformed, o.lastPerformed, true) && compareDeep(nextScheduled, o.nextScheduled, true) 3265 && compareDeep(failureAction, o.failureAction, true) && compareDeep(primarySource, o.primarySource, true) 3266 && compareDeep(attestation, o.attestation, true) && compareDeep(validator, o.validator, true); 3267 } 3268 3269 @Override 3270 public boolean equalsShallow(Base other_) { 3271 if (!super.equalsShallow(other_)) 3272 return false; 3273 if (!(other_ instanceof VerificationResult)) 3274 return false; 3275 VerificationResult o = (VerificationResult) other_; 3276 return compareValues(targetLocation, o.targetLocation, true) && compareValues(status, o.status, true) 3277 && compareValues(statusDate, o.statusDate, true) && compareValues(lastPerformed, o.lastPerformed, true) 3278 && compareValues(nextScheduled, o.nextScheduled, true); 3279 } 3280 3281 public boolean isEmpty() { 3282 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, targetLocation, need, status, statusDate, 3283 validationType, validationProcess, frequency, lastPerformed, nextScheduled, failureAction, primarySource, 3284 attestation, validator); 3285 } 3286 3287 @Override 3288 public ResourceType getResourceType() { 3289 return ResourceType.VerificationResult; 3290 } 3291 3292 /** 3293 * Search parameter: <b>target</b> 3294 * <p> 3295 * Description: <b>A resource that was validated</b><br> 3296 * Type: <b>reference</b><br> 3297 * Path: <b>VerificationResult.target</b><br> 3298 * </p> 3299 */ 3300 @SearchParamDefinition(name = "target", path = "VerificationResult.target", description = "A resource that was validated", type = "reference") 3301 public static final String SP_TARGET = "target"; 3302 /** 3303 * <b>Fluent Client</b> search parameter constant for <b>target</b> 3304 * <p> 3305 * Description: <b>A resource that was validated</b><br> 3306 * Type: <b>reference</b><br> 3307 * Path: <b>VerificationResult.target</b><br> 3308 * </p> 3309 */ 3310 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3311 SP_TARGET); 3312 3313 /** 3314 * Constant for fluent queries to be used to add include statements. Specifies 3315 * the path value of "<b>VerificationResult:target</b>". 3316 */ 3317 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include( 3318 "VerificationResult:target").toLocked(); 3319 3320}