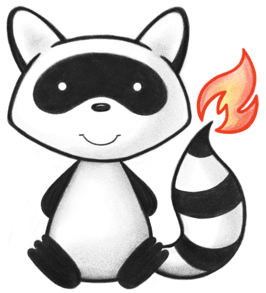
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Describes validation requirements, source(s), status and dates for one or 049 * more elements. 050 */ 051@ResourceDef(name = "VerificationResult", profile = "http://hl7.org/fhir/StructureDefinition/VerificationResult") 052public class VerificationResult extends DomainResource { 053 054 public enum Status { 055 /** 056 * ***TODO*** 057 */ 058 ATTESTED, 059 /** 060 * ***TODO*** 061 */ 062 VALIDATED, 063 /** 064 * ***TODO*** 065 */ 066 INPROCESS, 067 /** 068 * ***TODO*** 069 */ 070 REQREVALID, 071 /** 072 * ***TODO*** 073 */ 074 VALFAIL, 075 /** 076 * ***TODO*** 077 */ 078 REVALFAIL, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 084 public static Status fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("attested".equals(codeString)) 088 return ATTESTED; 089 if ("validated".equals(codeString)) 090 return VALIDATED; 091 if ("in-process".equals(codeString)) 092 return INPROCESS; 093 if ("req-revalid".equals(codeString)) 094 return REQREVALID; 095 if ("val-fail".equals(codeString)) 096 return VALFAIL; 097 if ("reval-fail".equals(codeString)) 098 return REVALFAIL; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown Status code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case ATTESTED: 108 return "attested"; 109 case VALIDATED: 110 return "validated"; 111 case INPROCESS: 112 return "in-process"; 113 case REQREVALID: 114 return "req-revalid"; 115 case VALFAIL: 116 return "val-fail"; 117 case REVALFAIL: 118 return "reval-fail"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getSystem() { 127 switch (this) { 128 case ATTESTED: 129 return "http://hl7.org/fhir/CodeSystem/status"; 130 case VALIDATED: 131 return "http://hl7.org/fhir/CodeSystem/status"; 132 case INPROCESS: 133 return "http://hl7.org/fhir/CodeSystem/status"; 134 case REQREVALID: 135 return "http://hl7.org/fhir/CodeSystem/status"; 136 case VALFAIL: 137 return "http://hl7.org/fhir/CodeSystem/status"; 138 case REVALFAIL: 139 return "http://hl7.org/fhir/CodeSystem/status"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case ATTESTED: 150 return "***TODO***"; 151 case VALIDATED: 152 return "***TODO***"; 153 case INPROCESS: 154 return "***TODO***"; 155 case REQREVALID: 156 return "***TODO***"; 157 case VALFAIL: 158 return "***TODO***"; 159 case REVALFAIL: 160 return "***TODO***"; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDisplay() { 169 switch (this) { 170 case ATTESTED: 171 return "Attested"; 172 case VALIDATED: 173 return "Validated"; 174 case INPROCESS: 175 return "In process"; 176 case REQREVALID: 177 return "Requires revalidation"; 178 case VALFAIL: 179 return "Validation failed"; 180 case REVALFAIL: 181 return "Re-Validation failed"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 } 189 190 public static class StatusEnumFactory implements EnumFactory<Status> { 191 public Status fromCode(String codeString) throws IllegalArgumentException { 192 if (codeString == null || "".equals(codeString)) 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("attested".equals(codeString)) 196 return Status.ATTESTED; 197 if ("validated".equals(codeString)) 198 return Status.VALIDATED; 199 if ("in-process".equals(codeString)) 200 return Status.INPROCESS; 201 if ("req-revalid".equals(codeString)) 202 return Status.REQREVALID; 203 if ("val-fail".equals(codeString)) 204 return Status.VALFAIL; 205 if ("reval-fail".equals(codeString)) 206 return Status.REVALFAIL; 207 throw new IllegalArgumentException("Unknown Status code '" + codeString + "'"); 208 } 209 210 public Enumeration<Status> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<Status>(this, Status.NULL, code); 215 String codeString = code.asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<Status>(this, Status.NULL, code); 218 if ("attested".equals(codeString)) 219 return new Enumeration<Status>(this, Status.ATTESTED, code); 220 if ("validated".equals(codeString)) 221 return new Enumeration<Status>(this, Status.VALIDATED, code); 222 if ("in-process".equals(codeString)) 223 return new Enumeration<Status>(this, Status.INPROCESS, code); 224 if ("req-revalid".equals(codeString)) 225 return new Enumeration<Status>(this, Status.REQREVALID, code); 226 if ("val-fail".equals(codeString)) 227 return new Enumeration<Status>(this, Status.VALFAIL, code); 228 if ("reval-fail".equals(codeString)) 229 return new Enumeration<Status>(this, Status.REVALFAIL, code); 230 throw new FHIRException("Unknown Status code '" + codeString + "'"); 231 } 232 233 public String toCode(Status code) { 234 if (code == Status.ATTESTED) 235 return "attested"; 236 if (code == Status.VALIDATED) 237 return "validated"; 238 if (code == Status.INPROCESS) 239 return "in-process"; 240 if (code == Status.REQREVALID) 241 return "req-revalid"; 242 if (code == Status.VALFAIL) 243 return "val-fail"; 244 if (code == Status.REVALFAIL) 245 return "reval-fail"; 246 return "?"; 247 } 248 249 public String toSystem(Status code) { 250 return code.getSystem(); 251 } 252 } 253 254 @Block() 255 public static class VerificationResultPrimarySourceComponent extends BackboneElement implements IBaseBackboneElement { 256 /** 257 * Reference to the primary source. 258 */ 259 @Child(name = "who", type = { Organization.class, Practitioner.class, 260 PractitionerRole.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 261 @Description(shortDefinition = "Reference to the primary source", formalDefinition = "Reference to the primary source.") 262 protected Reference who; 263 264 /** 265 * The actual object that is the target of the reference (Reference to the 266 * primary source.) 267 */ 268 protected Resource whoTarget; 269 270 /** 271 * Type of primary source (License Board; Primary Education; Continuing 272 * Education; Postal Service; Relationship owner; Registration Authority; legal 273 * source; issuing source; authoritative source). 274 */ 275 @Child(name = "type", type = { 276 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 277 @Description(shortDefinition = "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source)", formalDefinition = "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).") 278 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-primary-source-type") 279 protected List<CodeableConcept> type; 280 281 /** 282 * Method for communicating with the primary source (manual; API; Push). 283 */ 284 @Child(name = "communicationMethod", type = { 285 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 286 @Description(shortDefinition = "Method for exchanging information with the primary source", formalDefinition = "Method for communicating with the primary source (manual; API; Push).") 287 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-communication-method") 288 protected List<CodeableConcept> communicationMethod; 289 290 /** 291 * Status of the validation of the target against the primary source 292 * (successful; failed; unknown). 293 */ 294 @Child(name = "validationStatus", type = { 295 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 296 @Description(shortDefinition = "successful | failed | unknown", formalDefinition = "Status of the validation of the target against the primary source (successful; failed; unknown).") 297 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-validation-status") 298 protected CodeableConcept validationStatus; 299 300 /** 301 * When the target was validated against the primary source. 302 */ 303 @Child(name = "validationDate", type = { 304 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 305 @Description(shortDefinition = "When the target was validated against the primary source", formalDefinition = "When the target was validated against the primary source.") 306 protected DateTimeType validationDate; 307 308 /** 309 * Ability of the primary source to push updates/alerts (yes; no; undetermined). 310 */ 311 @Child(name = "canPushUpdates", type = { 312 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 313 @Description(shortDefinition = "yes | no | undetermined", formalDefinition = "Ability of the primary source to push updates/alerts (yes; no; undetermined).") 314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-can-push-updates") 315 protected CodeableConcept canPushUpdates; 316 317 /** 318 * Type of alerts/updates the primary source can send (specific requested 319 * changes; any changes; as defined by source). 320 */ 321 @Child(name = "pushTypeAvailable", type = { 322 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 323 @Description(shortDefinition = "specific | any | source", formalDefinition = "Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).") 324 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-push-type-available") 325 protected List<CodeableConcept> pushTypeAvailable; 326 327 private static final long serialVersionUID = -928858332L; 328 329 /** 330 * Constructor 331 */ 332 public VerificationResultPrimarySourceComponent() { 333 super(); 334 } 335 336 /** 337 * @return {@link #who} (Reference to the primary source.) 338 */ 339 public Reference getWho() { 340 if (this.who == null) 341 if (Configuration.errorOnAutoCreate()) 342 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.who"); 343 else if (Configuration.doAutoCreate()) 344 this.who = new Reference(); // cc 345 return this.who; 346 } 347 348 public boolean hasWho() { 349 return this.who != null && !this.who.isEmpty(); 350 } 351 352 /** 353 * @param value {@link #who} (Reference to the primary source.) 354 */ 355 public VerificationResultPrimarySourceComponent setWho(Reference value) { 356 this.who = value; 357 return this; 358 } 359 360 /** 361 * @return {@link #who} The actual object that is the target of the reference. 362 * The reference library doesn't populate this, but you can use it to 363 * hold the resource if you resolve it. (Reference to the primary 364 * source.) 365 */ 366 public Resource getWhoTarget() { 367 return this.whoTarget; 368 } 369 370 /** 371 * @param value {@link #who} The actual object that is the target of the 372 * reference. The reference library doesn't use these, but you can 373 * use it to hold the resource if you resolve it. (Reference to the 374 * primary source.) 375 */ 376 public VerificationResultPrimarySourceComponent setWhoTarget(Resource value) { 377 this.whoTarget = value; 378 return this; 379 } 380 381 /** 382 * @return {@link #type} (Type of primary source (License Board; Primary 383 * Education; Continuing Education; Postal Service; Relationship owner; 384 * Registration Authority; legal source; issuing source; authoritative 385 * source).) 386 */ 387 public List<CodeableConcept> getType() { 388 if (this.type == null) 389 this.type = new ArrayList<CodeableConcept>(); 390 return this.type; 391 } 392 393 /** 394 * @return Returns a reference to <code>this</code> for easy method chaining 395 */ 396 public VerificationResultPrimarySourceComponent setType(List<CodeableConcept> theType) { 397 this.type = theType; 398 return this; 399 } 400 401 public boolean hasType() { 402 if (this.type == null) 403 return false; 404 for (CodeableConcept item : this.type) 405 if (!item.isEmpty()) 406 return true; 407 return false; 408 } 409 410 public CodeableConcept addType() { // 3 411 CodeableConcept t = new CodeableConcept(); 412 if (this.type == null) 413 this.type = new ArrayList<CodeableConcept>(); 414 this.type.add(t); 415 return t; 416 } 417 418 public VerificationResultPrimarySourceComponent addType(CodeableConcept t) { // 3 419 if (t == null) 420 return this; 421 if (this.type == null) 422 this.type = new ArrayList<CodeableConcept>(); 423 this.type.add(t); 424 return this; 425 } 426 427 /** 428 * @return The first repetition of repeating field {@link #type}, creating it if 429 * it does not already exist 430 */ 431 public CodeableConcept getTypeFirstRep() { 432 if (getType().isEmpty()) { 433 addType(); 434 } 435 return getType().get(0); 436 } 437 438 /** 439 * @return {@link #communicationMethod} (Method for communicating with the 440 * primary source (manual; API; Push).) 441 */ 442 public List<CodeableConcept> getCommunicationMethod() { 443 if (this.communicationMethod == null) 444 this.communicationMethod = new ArrayList<CodeableConcept>(); 445 return this.communicationMethod; 446 } 447 448 /** 449 * @return Returns a reference to <code>this</code> for easy method chaining 450 */ 451 public VerificationResultPrimarySourceComponent setCommunicationMethod( 452 List<CodeableConcept> theCommunicationMethod) { 453 this.communicationMethod = theCommunicationMethod; 454 return this; 455 } 456 457 public boolean hasCommunicationMethod() { 458 if (this.communicationMethod == null) 459 return false; 460 for (CodeableConcept item : this.communicationMethod) 461 if (!item.isEmpty()) 462 return true; 463 return false; 464 } 465 466 public CodeableConcept addCommunicationMethod() { // 3 467 CodeableConcept t = new CodeableConcept(); 468 if (this.communicationMethod == null) 469 this.communicationMethod = new ArrayList<CodeableConcept>(); 470 this.communicationMethod.add(t); 471 return t; 472 } 473 474 public VerificationResultPrimarySourceComponent addCommunicationMethod(CodeableConcept t) { // 3 475 if (t == null) 476 return this; 477 if (this.communicationMethod == null) 478 this.communicationMethod = new ArrayList<CodeableConcept>(); 479 this.communicationMethod.add(t); 480 return this; 481 } 482 483 /** 484 * @return The first repetition of repeating field {@link #communicationMethod}, 485 * creating it if it does not already exist 486 */ 487 public CodeableConcept getCommunicationMethodFirstRep() { 488 if (getCommunicationMethod().isEmpty()) { 489 addCommunicationMethod(); 490 } 491 return getCommunicationMethod().get(0); 492 } 493 494 /** 495 * @return {@link #validationStatus} (Status of the validation of the target 496 * against the primary source (successful; failed; unknown).) 497 */ 498 public CodeableConcept getValidationStatus() { 499 if (this.validationStatus == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.validationStatus"); 502 else if (Configuration.doAutoCreate()) 503 this.validationStatus = new CodeableConcept(); // cc 504 return this.validationStatus; 505 } 506 507 public boolean hasValidationStatus() { 508 return this.validationStatus != null && !this.validationStatus.isEmpty(); 509 } 510 511 /** 512 * @param value {@link #validationStatus} (Status of the validation of the 513 * target against the primary source (successful; failed; 514 * unknown).) 515 */ 516 public VerificationResultPrimarySourceComponent setValidationStatus(CodeableConcept value) { 517 this.validationStatus = value; 518 return this; 519 } 520 521 /** 522 * @return {@link #validationDate} (When the target was validated against the 523 * primary source.). This is the underlying object with id, value and 524 * extensions. The accessor "getValidationDate" gives direct access to 525 * the value 526 */ 527 public DateTimeType getValidationDateElement() { 528 if (this.validationDate == null) 529 if (Configuration.errorOnAutoCreate()) 530 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.validationDate"); 531 else if (Configuration.doAutoCreate()) 532 this.validationDate = new DateTimeType(); // bb 533 return this.validationDate; 534 } 535 536 public boolean hasValidationDateElement() { 537 return this.validationDate != null && !this.validationDate.isEmpty(); 538 } 539 540 public boolean hasValidationDate() { 541 return this.validationDate != null && !this.validationDate.isEmpty(); 542 } 543 544 /** 545 * @param value {@link #validationDate} (When the target was validated against 546 * the primary source.). This is the underlying object with id, 547 * value and extensions. The accessor "getValidationDate" gives 548 * direct access to the value 549 */ 550 public VerificationResultPrimarySourceComponent setValidationDateElement(DateTimeType value) { 551 this.validationDate = value; 552 return this; 553 } 554 555 /** 556 * @return When the target was validated against the primary source. 557 */ 558 public Date getValidationDate() { 559 return this.validationDate == null ? null : this.validationDate.getValue(); 560 } 561 562 /** 563 * @param value When the target was validated against the primary source. 564 */ 565 public VerificationResultPrimarySourceComponent setValidationDate(Date value) { 566 if (value == null) 567 this.validationDate = null; 568 else { 569 if (this.validationDate == null) 570 this.validationDate = new DateTimeType(); 571 this.validationDate.setValue(value); 572 } 573 return this; 574 } 575 576 /** 577 * @return {@link #canPushUpdates} (Ability of the primary source to push 578 * updates/alerts (yes; no; undetermined).) 579 */ 580 public CodeableConcept getCanPushUpdates() { 581 if (this.canPushUpdates == null) 582 if (Configuration.errorOnAutoCreate()) 583 throw new Error("Attempt to auto-create VerificationResultPrimarySourceComponent.canPushUpdates"); 584 else if (Configuration.doAutoCreate()) 585 this.canPushUpdates = new CodeableConcept(); // cc 586 return this.canPushUpdates; 587 } 588 589 public boolean hasCanPushUpdates() { 590 return this.canPushUpdates != null && !this.canPushUpdates.isEmpty(); 591 } 592 593 /** 594 * @param value {@link #canPushUpdates} (Ability of the primary source to push 595 * updates/alerts (yes; no; undetermined).) 596 */ 597 public VerificationResultPrimarySourceComponent setCanPushUpdates(CodeableConcept value) { 598 this.canPushUpdates = value; 599 return this; 600 } 601 602 /** 603 * @return {@link #pushTypeAvailable} (Type of alerts/updates the primary source 604 * can send (specific requested changes; any changes; as defined by 605 * source).) 606 */ 607 public List<CodeableConcept> getPushTypeAvailable() { 608 if (this.pushTypeAvailable == null) 609 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 610 return this.pushTypeAvailable; 611 } 612 613 /** 614 * @return Returns a reference to <code>this</code> for easy method chaining 615 */ 616 public VerificationResultPrimarySourceComponent setPushTypeAvailable(List<CodeableConcept> thePushTypeAvailable) { 617 this.pushTypeAvailable = thePushTypeAvailable; 618 return this; 619 } 620 621 public boolean hasPushTypeAvailable() { 622 if (this.pushTypeAvailable == null) 623 return false; 624 for (CodeableConcept item : this.pushTypeAvailable) 625 if (!item.isEmpty()) 626 return true; 627 return false; 628 } 629 630 public CodeableConcept addPushTypeAvailable() { // 3 631 CodeableConcept t = new CodeableConcept(); 632 if (this.pushTypeAvailable == null) 633 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 634 this.pushTypeAvailable.add(t); 635 return t; 636 } 637 638 public VerificationResultPrimarySourceComponent addPushTypeAvailable(CodeableConcept t) { // 3 639 if (t == null) 640 return this; 641 if (this.pushTypeAvailable == null) 642 this.pushTypeAvailable = new ArrayList<CodeableConcept>(); 643 this.pushTypeAvailable.add(t); 644 return this; 645 } 646 647 /** 648 * @return The first repetition of repeating field {@link #pushTypeAvailable}, 649 * creating it if it does not already exist 650 */ 651 public CodeableConcept getPushTypeAvailableFirstRep() { 652 if (getPushTypeAvailable().isEmpty()) { 653 addPushTypeAvailable(); 654 } 655 return getPushTypeAvailable().get(0); 656 } 657 658 protected void listChildren(List<Property> children) { 659 super.listChildren(children); 660 children.add(new Property("who", "Reference(Organization|Practitioner|PractitionerRole)", 661 "Reference to the primary source.", 0, 1, who)); 662 children.add(new Property("type", "CodeableConcept", 663 "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).", 664 0, java.lang.Integer.MAX_VALUE, type)); 665 children.add(new Property("communicationMethod", "CodeableConcept", 666 "Method for communicating with the primary source (manual; API; Push).", 0, java.lang.Integer.MAX_VALUE, 667 communicationMethod)); 668 children.add(new Property("validationStatus", "CodeableConcept", 669 "Status of the validation of the target against the primary source (successful; failed; unknown).", 0, 1, 670 validationStatus)); 671 children.add(new Property("validationDate", "dateTime", 672 "When the target was validated against the primary source.", 0, 1, validationDate)); 673 children.add(new Property("canPushUpdates", "CodeableConcept", 674 "Ability of the primary source to push updates/alerts (yes; no; undetermined).", 0, 1, canPushUpdates)); 675 children.add(new Property("pushTypeAvailable", "CodeableConcept", 676 "Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).", 677 0, java.lang.Integer.MAX_VALUE, pushTypeAvailable)); 678 } 679 680 @Override 681 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 682 switch (_hash) { 683 case 117694: 684 /* who */ return new Property("who", "Reference(Organization|Practitioner|PractitionerRole)", 685 "Reference to the primary source.", 0, 1, who); 686 case 3575610: 687 /* type */ return new Property("type", "CodeableConcept", 688 "Type of primary source (License Board; Primary Education; Continuing Education; Postal Service; Relationship owner; Registration Authority; legal source; issuing source; authoritative source).", 689 0, java.lang.Integer.MAX_VALUE, type); 690 case 1314116695: 691 /* communicationMethod */ return new Property("communicationMethod", "CodeableConcept", 692 "Method for communicating with the primary source (manual; API; Push).", 0, java.lang.Integer.MAX_VALUE, 693 communicationMethod); 694 case 1775633867: 695 /* validationStatus */ return new Property("validationStatus", "CodeableConcept", 696 "Status of the validation of the target against the primary source (successful; failed; unknown).", 0, 1, 697 validationStatus); 698 case -280180793: 699 /* validationDate */ return new Property("validationDate", "dateTime", 700 "When the target was validated against the primary source.", 0, 1, validationDate); 701 case 1463787104: 702 /* canPushUpdates */ return new Property("canPushUpdates", "CodeableConcept", 703 "Ability of the primary source to push updates/alerts (yes; no; undetermined).", 0, 1, canPushUpdates); 704 case 945223605: 705 /* pushTypeAvailable */ return new Property("pushTypeAvailable", "CodeableConcept", 706 "Type of alerts/updates the primary source can send (specific requested changes; any changes; as defined by source).", 707 0, java.lang.Integer.MAX_VALUE, pushTypeAvailable); 708 default: 709 return super.getNamedProperty(_hash, _name, _checkValid); 710 } 711 712 } 713 714 @Override 715 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 716 switch (hash) { 717 case 117694: 718 /* who */ return this.who == null ? new Base[0] : new Base[] { this.who }; // Reference 719 case 3575610: 720 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 721 case 1314116695: 722 /* communicationMethod */ return this.communicationMethod == null ? new Base[0] 723 : this.communicationMethod.toArray(new Base[this.communicationMethod.size()]); // CodeableConcept 724 case 1775633867: 725 /* validationStatus */ return this.validationStatus == null ? new Base[0] 726 : new Base[] { this.validationStatus }; // CodeableConcept 727 case -280180793: 728 /* validationDate */ return this.validationDate == null ? new Base[0] : new Base[] { this.validationDate }; // DateTimeType 729 case 1463787104: 730 /* canPushUpdates */ return this.canPushUpdates == null ? new Base[0] : new Base[] { this.canPushUpdates }; // CodeableConcept 731 case 945223605: 732 /* pushTypeAvailable */ return this.pushTypeAvailable == null ? new Base[0] 733 : this.pushTypeAvailable.toArray(new Base[this.pushTypeAvailable.size()]); // CodeableConcept 734 default: 735 return super.getProperty(hash, name, checkValid); 736 } 737 738 } 739 740 @Override 741 public Base setProperty(int hash, String name, Base value) throws FHIRException { 742 switch (hash) { 743 case 117694: // who 744 this.who = castToReference(value); // Reference 745 return value; 746 case 3575610: // type 747 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 748 return value; 749 case 1314116695: // communicationMethod 750 this.getCommunicationMethod().add(castToCodeableConcept(value)); // CodeableConcept 751 return value; 752 case 1775633867: // validationStatus 753 this.validationStatus = castToCodeableConcept(value); // CodeableConcept 754 return value; 755 case -280180793: // validationDate 756 this.validationDate = castToDateTime(value); // DateTimeType 757 return value; 758 case 1463787104: // canPushUpdates 759 this.canPushUpdates = castToCodeableConcept(value); // CodeableConcept 760 return value; 761 case 945223605: // pushTypeAvailable 762 this.getPushTypeAvailable().add(castToCodeableConcept(value)); // CodeableConcept 763 return value; 764 default: 765 return super.setProperty(hash, name, value); 766 } 767 768 } 769 770 @Override 771 public Base setProperty(String name, Base value) throws FHIRException { 772 if (name.equals("who")) { 773 this.who = castToReference(value); // Reference 774 } else if (name.equals("type")) { 775 this.getType().add(castToCodeableConcept(value)); 776 } else if (name.equals("communicationMethod")) { 777 this.getCommunicationMethod().add(castToCodeableConcept(value)); 778 } else if (name.equals("validationStatus")) { 779 this.validationStatus = castToCodeableConcept(value); // CodeableConcept 780 } else if (name.equals("validationDate")) { 781 this.validationDate = castToDateTime(value); // DateTimeType 782 } else if (name.equals("canPushUpdates")) { 783 this.canPushUpdates = castToCodeableConcept(value); // CodeableConcept 784 } else if (name.equals("pushTypeAvailable")) { 785 this.getPushTypeAvailable().add(castToCodeableConcept(value)); 786 } else 787 return super.setProperty(name, value); 788 return value; 789 } 790 791 @Override 792 public void removeChild(String name, Base value) throws FHIRException { 793 if (name.equals("who")) { 794 this.who = null; 795 } else if (name.equals("type")) { 796 this.getType().remove(castToCodeableConcept(value)); 797 } else if (name.equals("communicationMethod")) { 798 this.getCommunicationMethod().remove(castToCodeableConcept(value)); 799 } else if (name.equals("validationStatus")) { 800 this.validationStatus = null; 801 } else if (name.equals("validationDate")) { 802 this.validationDate = null; 803 } else if (name.equals("canPushUpdates")) { 804 this.canPushUpdates = null; 805 } else if (name.equals("pushTypeAvailable")) { 806 this.getPushTypeAvailable().remove(castToCodeableConcept(value)); 807 } else 808 super.removeChild(name, value); 809 810 } 811 812 @Override 813 public Base makeProperty(int hash, String name) throws FHIRException { 814 switch (hash) { 815 case 117694: 816 return getWho(); 817 case 3575610: 818 return addType(); 819 case 1314116695: 820 return addCommunicationMethod(); 821 case 1775633867: 822 return getValidationStatus(); 823 case -280180793: 824 return getValidationDateElement(); 825 case 1463787104: 826 return getCanPushUpdates(); 827 case 945223605: 828 return addPushTypeAvailable(); 829 default: 830 return super.makeProperty(hash, name); 831 } 832 833 } 834 835 @Override 836 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 837 switch (hash) { 838 case 117694: 839 /* who */ return new String[] { "Reference" }; 840 case 3575610: 841 /* type */ return new String[] { "CodeableConcept" }; 842 case 1314116695: 843 /* communicationMethod */ return new String[] { "CodeableConcept" }; 844 case 1775633867: 845 /* validationStatus */ return new String[] { "CodeableConcept" }; 846 case -280180793: 847 /* validationDate */ return new String[] { "dateTime" }; 848 case 1463787104: 849 /* canPushUpdates */ return new String[] { "CodeableConcept" }; 850 case 945223605: 851 /* pushTypeAvailable */ return new String[] { "CodeableConcept" }; 852 default: 853 return super.getTypesForProperty(hash, name); 854 } 855 856 } 857 858 @Override 859 public Base addChild(String name) throws FHIRException { 860 if (name.equals("who")) { 861 this.who = new Reference(); 862 return this.who; 863 } else if (name.equals("type")) { 864 return addType(); 865 } else if (name.equals("communicationMethod")) { 866 return addCommunicationMethod(); 867 } else if (name.equals("validationStatus")) { 868 this.validationStatus = new CodeableConcept(); 869 return this.validationStatus; 870 } else if (name.equals("validationDate")) { 871 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.validationDate"); 872 } else if (name.equals("canPushUpdates")) { 873 this.canPushUpdates = new CodeableConcept(); 874 return this.canPushUpdates; 875 } else if (name.equals("pushTypeAvailable")) { 876 return addPushTypeAvailable(); 877 } else 878 return super.addChild(name); 879 } 880 881 public VerificationResultPrimarySourceComponent copy() { 882 VerificationResultPrimarySourceComponent dst = new VerificationResultPrimarySourceComponent(); 883 copyValues(dst); 884 return dst; 885 } 886 887 public void copyValues(VerificationResultPrimarySourceComponent dst) { 888 super.copyValues(dst); 889 dst.who = who == null ? null : who.copy(); 890 if (type != null) { 891 dst.type = new ArrayList<CodeableConcept>(); 892 for (CodeableConcept i : type) 893 dst.type.add(i.copy()); 894 } 895 ; 896 if (communicationMethod != null) { 897 dst.communicationMethod = new ArrayList<CodeableConcept>(); 898 for (CodeableConcept i : communicationMethod) 899 dst.communicationMethod.add(i.copy()); 900 } 901 ; 902 dst.validationStatus = validationStatus == null ? null : validationStatus.copy(); 903 dst.validationDate = validationDate == null ? null : validationDate.copy(); 904 dst.canPushUpdates = canPushUpdates == null ? null : canPushUpdates.copy(); 905 if (pushTypeAvailable != null) { 906 dst.pushTypeAvailable = new ArrayList<CodeableConcept>(); 907 for (CodeableConcept i : pushTypeAvailable) 908 dst.pushTypeAvailable.add(i.copy()); 909 } 910 ; 911 } 912 913 @Override 914 public boolean equalsDeep(Base other_) { 915 if (!super.equalsDeep(other_)) 916 return false; 917 if (!(other_ instanceof VerificationResultPrimarySourceComponent)) 918 return false; 919 VerificationResultPrimarySourceComponent o = (VerificationResultPrimarySourceComponent) other_; 920 return compareDeep(who, o.who, true) && compareDeep(type, o.type, true) 921 && compareDeep(communicationMethod, o.communicationMethod, true) 922 && compareDeep(validationStatus, o.validationStatus, true) 923 && compareDeep(validationDate, o.validationDate, true) && compareDeep(canPushUpdates, o.canPushUpdates, true) 924 && compareDeep(pushTypeAvailable, o.pushTypeAvailable, true); 925 } 926 927 @Override 928 public boolean equalsShallow(Base other_) { 929 if (!super.equalsShallow(other_)) 930 return false; 931 if (!(other_ instanceof VerificationResultPrimarySourceComponent)) 932 return false; 933 VerificationResultPrimarySourceComponent o = (VerificationResultPrimarySourceComponent) other_; 934 return compareValues(validationDate, o.validationDate, true); 935 } 936 937 public boolean isEmpty() { 938 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(who, type, communicationMethod, validationStatus, 939 validationDate, canPushUpdates, pushTypeAvailable); 940 } 941 942 public String fhirType() { 943 return "VerificationResult.primarySource"; 944 945 } 946 947 } 948 949 @Block() 950 public static class VerificationResultAttestationComponent extends BackboneElement implements IBaseBackboneElement { 951 /** 952 * The individual or organization attesting to information. 953 */ 954 @Child(name = "who", type = { Practitioner.class, PractitionerRole.class, 955 Organization.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 956 @Description(shortDefinition = "The individual or organization attesting to information", formalDefinition = "The individual or organization attesting to information.") 957 protected Reference who; 958 959 /** 960 * The actual object that is the target of the reference (The individual or 961 * organization attesting to information.) 962 */ 963 protected Resource whoTarget; 964 965 /** 966 * When the who is asserting on behalf of another (organization or individual). 967 */ 968 @Child(name = "onBehalfOf", type = { Organization.class, Practitioner.class, 969 PractitionerRole.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 970 @Description(shortDefinition = "When the who is asserting on behalf of another (organization or individual)", formalDefinition = "When the who is asserting on behalf of another (organization or individual).") 971 protected Reference onBehalfOf; 972 973 /** 974 * The actual object that is the target of the reference (When the who is 975 * asserting on behalf of another (organization or individual).) 976 */ 977 protected Resource onBehalfOfTarget; 978 979 /** 980 * The method by which attested information was submitted/retrieved (manual; 981 * API; Push). 982 */ 983 @Child(name = "communicationMethod", type = { 984 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 985 @Description(shortDefinition = "The method by which attested information was submitted/retrieved", formalDefinition = "The method by which attested information was submitted/retrieved (manual; API; Push).") 986 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-communication-method") 987 protected CodeableConcept communicationMethod; 988 989 /** 990 * The date the information was attested to. 991 */ 992 @Child(name = "date", type = { DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 993 @Description(shortDefinition = "The date the information was attested to", formalDefinition = "The date the information was attested to.") 994 protected DateType date; 995 996 /** 997 * A digital identity certificate associated with the attestation source. 998 */ 999 @Child(name = "sourceIdentityCertificate", type = { 1000 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1001 @Description(shortDefinition = "A digital identity certificate associated with the attestation source", formalDefinition = "A digital identity certificate associated with the attestation source.") 1002 protected StringType sourceIdentityCertificate; 1003 1004 /** 1005 * A digital identity certificate associated with the proxy entity submitting 1006 * attested information on behalf of the attestation source. 1007 */ 1008 @Child(name = "proxyIdentityCertificate", type = { 1009 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1010 @Description(shortDefinition = "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source", formalDefinition = "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.") 1011 protected StringType proxyIdentityCertificate; 1012 1013 /** 1014 * Signed assertion by the proxy entity indicating that they have the right to 1015 * submit attested information on behalf of the attestation source. 1016 */ 1017 @Child(name = "proxySignature", type = { 1018 Signature.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1019 @Description(shortDefinition = "Proxy signature", formalDefinition = "Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.") 1020 protected Signature proxySignature; 1021 1022 /** 1023 * Signed assertion by the attestation source that they have attested to the 1024 * information. 1025 */ 1026 @Child(name = "sourceSignature", type = { 1027 Signature.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1028 @Description(shortDefinition = "Attester signature", formalDefinition = "Signed assertion by the attestation source that they have attested to the information.") 1029 protected Signature sourceSignature; 1030 1031 private static final long serialVersionUID = -900018800L; 1032 1033 /** 1034 * Constructor 1035 */ 1036 public VerificationResultAttestationComponent() { 1037 super(); 1038 } 1039 1040 /** 1041 * @return {@link #who} (The individual or organization attesting to 1042 * information.) 1043 */ 1044 public Reference getWho() { 1045 if (this.who == null) 1046 if (Configuration.errorOnAutoCreate()) 1047 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.who"); 1048 else if (Configuration.doAutoCreate()) 1049 this.who = new Reference(); // cc 1050 return this.who; 1051 } 1052 1053 public boolean hasWho() { 1054 return this.who != null && !this.who.isEmpty(); 1055 } 1056 1057 /** 1058 * @param value {@link #who} (The individual or organization attesting to 1059 * information.) 1060 */ 1061 public VerificationResultAttestationComponent setWho(Reference value) { 1062 this.who = value; 1063 return this; 1064 } 1065 1066 /** 1067 * @return {@link #who} The actual object that is the target of the reference. 1068 * The reference library doesn't populate this, but you can use it to 1069 * hold the resource if you resolve it. (The individual or organization 1070 * attesting to information.) 1071 */ 1072 public Resource getWhoTarget() { 1073 return this.whoTarget; 1074 } 1075 1076 /** 1077 * @param value {@link #who} The actual object that is the target of the 1078 * reference. The reference library doesn't use these, but you can 1079 * use it to hold the resource if you resolve it. (The individual 1080 * or organization attesting to information.) 1081 */ 1082 public VerificationResultAttestationComponent setWhoTarget(Resource value) { 1083 this.whoTarget = value; 1084 return this; 1085 } 1086 1087 /** 1088 * @return {@link #onBehalfOf} (When the who is asserting on behalf of another 1089 * (organization or individual).) 1090 */ 1091 public Reference getOnBehalfOf() { 1092 if (this.onBehalfOf == null) 1093 if (Configuration.errorOnAutoCreate()) 1094 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.onBehalfOf"); 1095 else if (Configuration.doAutoCreate()) 1096 this.onBehalfOf = new Reference(); // cc 1097 return this.onBehalfOf; 1098 } 1099 1100 public boolean hasOnBehalfOf() { 1101 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 1102 } 1103 1104 /** 1105 * @param value {@link #onBehalfOf} (When the who is asserting on behalf of 1106 * another (organization or individual).) 1107 */ 1108 public VerificationResultAttestationComponent setOnBehalfOf(Reference value) { 1109 this.onBehalfOf = value; 1110 return this; 1111 } 1112 1113 /** 1114 * @return {@link #onBehalfOf} The actual object that is the target of the 1115 * reference. The reference library doesn't populate this, but you can 1116 * use it to hold the resource if you resolve it. (When the who is 1117 * asserting on behalf of another (organization or individual).) 1118 */ 1119 public Resource getOnBehalfOfTarget() { 1120 return this.onBehalfOfTarget; 1121 } 1122 1123 /** 1124 * @param value {@link #onBehalfOf} The actual object that is the target of the 1125 * reference. The reference library doesn't use these, but you can 1126 * use it to hold the resource if you resolve it. (When the who is 1127 * asserting on behalf of another (organization or individual).) 1128 */ 1129 public VerificationResultAttestationComponent setOnBehalfOfTarget(Resource value) { 1130 this.onBehalfOfTarget = value; 1131 return this; 1132 } 1133 1134 /** 1135 * @return {@link #communicationMethod} (The method by which attested 1136 * information was submitted/retrieved (manual; API; Push).) 1137 */ 1138 public CodeableConcept getCommunicationMethod() { 1139 if (this.communicationMethod == null) 1140 if (Configuration.errorOnAutoCreate()) 1141 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.communicationMethod"); 1142 else if (Configuration.doAutoCreate()) 1143 this.communicationMethod = new CodeableConcept(); // cc 1144 return this.communicationMethod; 1145 } 1146 1147 public boolean hasCommunicationMethod() { 1148 return this.communicationMethod != null && !this.communicationMethod.isEmpty(); 1149 } 1150 1151 /** 1152 * @param value {@link #communicationMethod} (The method by which attested 1153 * information was submitted/retrieved (manual; API; Push).) 1154 */ 1155 public VerificationResultAttestationComponent setCommunicationMethod(CodeableConcept value) { 1156 this.communicationMethod = value; 1157 return this; 1158 } 1159 1160 /** 1161 * @return {@link #date} (The date the information was attested to.). This is 1162 * the underlying object with id, value and extensions. The accessor 1163 * "getDate" gives direct access to the value 1164 */ 1165 public DateType getDateElement() { 1166 if (this.date == null) 1167 if (Configuration.errorOnAutoCreate()) 1168 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.date"); 1169 else if (Configuration.doAutoCreate()) 1170 this.date = new DateType(); // bb 1171 return this.date; 1172 } 1173 1174 public boolean hasDateElement() { 1175 return this.date != null && !this.date.isEmpty(); 1176 } 1177 1178 public boolean hasDate() { 1179 return this.date != null && !this.date.isEmpty(); 1180 } 1181 1182 /** 1183 * @param value {@link #date} (The date the information was attested to.). This 1184 * is the underlying object with id, value and extensions. The 1185 * accessor "getDate" gives direct access to the value 1186 */ 1187 public VerificationResultAttestationComponent setDateElement(DateType value) { 1188 this.date = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return The date the information was attested to. 1194 */ 1195 public Date getDate() { 1196 return this.date == null ? null : this.date.getValue(); 1197 } 1198 1199 /** 1200 * @param value The date the information was attested to. 1201 */ 1202 public VerificationResultAttestationComponent setDate(Date value) { 1203 if (value == null) 1204 this.date = null; 1205 else { 1206 if (this.date == null) 1207 this.date = new DateType(); 1208 this.date.setValue(value); 1209 } 1210 return this; 1211 } 1212 1213 /** 1214 * @return {@link #sourceIdentityCertificate} (A digital identity certificate 1215 * associated with the attestation source.). This is the underlying 1216 * object with id, value and extensions. The accessor 1217 * "getSourceIdentityCertificate" gives direct access to the value 1218 */ 1219 public StringType getSourceIdentityCertificateElement() { 1220 if (this.sourceIdentityCertificate == null) 1221 if (Configuration.errorOnAutoCreate()) 1222 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.sourceIdentityCertificate"); 1223 else if (Configuration.doAutoCreate()) 1224 this.sourceIdentityCertificate = new StringType(); // bb 1225 return this.sourceIdentityCertificate; 1226 } 1227 1228 public boolean hasSourceIdentityCertificateElement() { 1229 return this.sourceIdentityCertificate != null && !this.sourceIdentityCertificate.isEmpty(); 1230 } 1231 1232 public boolean hasSourceIdentityCertificate() { 1233 return this.sourceIdentityCertificate != null && !this.sourceIdentityCertificate.isEmpty(); 1234 } 1235 1236 /** 1237 * @param value {@link #sourceIdentityCertificate} (A digital identity 1238 * certificate associated with the attestation source.). This is 1239 * the underlying object with id, value and extensions. The 1240 * accessor "getSourceIdentityCertificate" gives direct access to 1241 * the value 1242 */ 1243 public VerificationResultAttestationComponent setSourceIdentityCertificateElement(StringType value) { 1244 this.sourceIdentityCertificate = value; 1245 return this; 1246 } 1247 1248 /** 1249 * @return A digital identity certificate associated with the attestation 1250 * source. 1251 */ 1252 public String getSourceIdentityCertificate() { 1253 return this.sourceIdentityCertificate == null ? null : this.sourceIdentityCertificate.getValue(); 1254 } 1255 1256 /** 1257 * @param value A digital identity certificate associated with the attestation 1258 * source. 1259 */ 1260 public VerificationResultAttestationComponent setSourceIdentityCertificate(String value) { 1261 if (Utilities.noString(value)) 1262 this.sourceIdentityCertificate = null; 1263 else { 1264 if (this.sourceIdentityCertificate == null) 1265 this.sourceIdentityCertificate = new StringType(); 1266 this.sourceIdentityCertificate.setValue(value); 1267 } 1268 return this; 1269 } 1270 1271 /** 1272 * @return {@link #proxyIdentityCertificate} (A digital identity certificate 1273 * associated with the proxy entity submitting attested information on 1274 * behalf of the attestation source.). This is the underlying object 1275 * with id, value and extensions. The accessor 1276 * "getProxyIdentityCertificate" gives direct access to the value 1277 */ 1278 public StringType getProxyIdentityCertificateElement() { 1279 if (this.proxyIdentityCertificate == null) 1280 if (Configuration.errorOnAutoCreate()) 1281 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.proxyIdentityCertificate"); 1282 else if (Configuration.doAutoCreate()) 1283 this.proxyIdentityCertificate = new StringType(); // bb 1284 return this.proxyIdentityCertificate; 1285 } 1286 1287 public boolean hasProxyIdentityCertificateElement() { 1288 return this.proxyIdentityCertificate != null && !this.proxyIdentityCertificate.isEmpty(); 1289 } 1290 1291 public boolean hasProxyIdentityCertificate() { 1292 return this.proxyIdentityCertificate != null && !this.proxyIdentityCertificate.isEmpty(); 1293 } 1294 1295 /** 1296 * @param value {@link #proxyIdentityCertificate} (A digital identity 1297 * certificate associated with the proxy entity submitting attested 1298 * information on behalf of the attestation source.). This is the 1299 * underlying object with id, value and extensions. The accessor 1300 * "getProxyIdentityCertificate" gives direct access to the value 1301 */ 1302 public VerificationResultAttestationComponent setProxyIdentityCertificateElement(StringType value) { 1303 this.proxyIdentityCertificate = value; 1304 return this; 1305 } 1306 1307 /** 1308 * @return A digital identity certificate associated with the proxy entity 1309 * submitting attested information on behalf of the attestation source. 1310 */ 1311 public String getProxyIdentityCertificate() { 1312 return this.proxyIdentityCertificate == null ? null : this.proxyIdentityCertificate.getValue(); 1313 } 1314 1315 /** 1316 * @param value A digital identity certificate associated with the proxy entity 1317 * submitting attested information on behalf of the attestation 1318 * source. 1319 */ 1320 public VerificationResultAttestationComponent setProxyIdentityCertificate(String value) { 1321 if (Utilities.noString(value)) 1322 this.proxyIdentityCertificate = null; 1323 else { 1324 if (this.proxyIdentityCertificate == null) 1325 this.proxyIdentityCertificate = new StringType(); 1326 this.proxyIdentityCertificate.setValue(value); 1327 } 1328 return this; 1329 } 1330 1331 /** 1332 * @return {@link #proxySignature} (Signed assertion by the proxy entity 1333 * indicating that they have the right to submit attested information on 1334 * behalf of the attestation source.) 1335 */ 1336 public Signature getProxySignature() { 1337 if (this.proxySignature == null) 1338 if (Configuration.errorOnAutoCreate()) 1339 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.proxySignature"); 1340 else if (Configuration.doAutoCreate()) 1341 this.proxySignature = new Signature(); // cc 1342 return this.proxySignature; 1343 } 1344 1345 public boolean hasProxySignature() { 1346 return this.proxySignature != null && !this.proxySignature.isEmpty(); 1347 } 1348 1349 /** 1350 * @param value {@link #proxySignature} (Signed assertion by the proxy entity 1351 * indicating that they have the right to submit attested 1352 * information on behalf of the attestation source.) 1353 */ 1354 public VerificationResultAttestationComponent setProxySignature(Signature value) { 1355 this.proxySignature = value; 1356 return this; 1357 } 1358 1359 /** 1360 * @return {@link #sourceSignature} (Signed assertion by the attestation source 1361 * that they have attested to the information.) 1362 */ 1363 public Signature getSourceSignature() { 1364 if (this.sourceSignature == null) 1365 if (Configuration.errorOnAutoCreate()) 1366 throw new Error("Attempt to auto-create VerificationResultAttestationComponent.sourceSignature"); 1367 else if (Configuration.doAutoCreate()) 1368 this.sourceSignature = new Signature(); // cc 1369 return this.sourceSignature; 1370 } 1371 1372 public boolean hasSourceSignature() { 1373 return this.sourceSignature != null && !this.sourceSignature.isEmpty(); 1374 } 1375 1376 /** 1377 * @param value {@link #sourceSignature} (Signed assertion by the attestation 1378 * source that they have attested to the information.) 1379 */ 1380 public VerificationResultAttestationComponent setSourceSignature(Signature value) { 1381 this.sourceSignature = value; 1382 return this; 1383 } 1384 1385 protected void listChildren(List<Property> children) { 1386 super.listChildren(children); 1387 children.add(new Property("who", "Reference(Practitioner|PractitionerRole|Organization)", 1388 "The individual or organization attesting to information.", 0, 1, who)); 1389 children.add(new Property("onBehalfOf", "Reference(Organization|Practitioner|PractitionerRole)", 1390 "When the who is asserting on behalf of another (organization or individual).", 0, 1, onBehalfOf)); 1391 children.add(new Property("communicationMethod", "CodeableConcept", 1392 "The method by which attested information was submitted/retrieved (manual; API; Push).", 0, 1, 1393 communicationMethod)); 1394 children.add(new Property("date", "date", "The date the information was attested to.", 0, 1, date)); 1395 children.add(new Property("sourceIdentityCertificate", "string", 1396 "A digital identity certificate associated with the attestation source.", 0, 1, sourceIdentityCertificate)); 1397 children.add(new Property("proxyIdentityCertificate", "string", 1398 "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.", 1399 0, 1, proxyIdentityCertificate)); 1400 children.add(new Property("proxySignature", "Signature", 1401 "Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.", 1402 0, 1, proxySignature)); 1403 children.add(new Property("sourceSignature", "Signature", 1404 "Signed assertion by the attestation source that they have attested to the information.", 0, 1, 1405 sourceSignature)); 1406 } 1407 1408 @Override 1409 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1410 switch (_hash) { 1411 case 117694: 1412 /* who */ return new Property("who", "Reference(Practitioner|PractitionerRole|Organization)", 1413 "The individual or organization attesting to information.", 0, 1, who); 1414 case -14402964: 1415 /* onBehalfOf */ return new Property("onBehalfOf", "Reference(Organization|Practitioner|PractitionerRole)", 1416 "When the who is asserting on behalf of another (organization or individual).", 0, 1, onBehalfOf); 1417 case 1314116695: 1418 /* communicationMethod */ return new Property("communicationMethod", "CodeableConcept", 1419 "The method by which attested information was submitted/retrieved (manual; API; Push).", 0, 1, 1420 communicationMethod); 1421 case 3076014: 1422 /* date */ return new Property("date", "date", "The date the information was attested to.", 0, 1, date); 1423 case -799067682: 1424 /* sourceIdentityCertificate */ return new Property("sourceIdentityCertificate", "string", 1425 "A digital identity certificate associated with the attestation source.", 0, 1, sourceIdentityCertificate); 1426 case 431558827: 1427 /* proxyIdentityCertificate */ return new Property("proxyIdentityCertificate", "string", 1428 "A digital identity certificate associated with the proxy entity submitting attested information on behalf of the attestation source.", 1429 0, 1, proxyIdentityCertificate); 1430 case 1455540714: 1431 /* proxySignature */ return new Property("proxySignature", "Signature", 1432 "Signed assertion by the proxy entity indicating that they have the right to submit attested information on behalf of the attestation source.", 1433 0, 1, proxySignature); 1434 case 1754480349: 1435 /* sourceSignature */ return new Property("sourceSignature", "Signature", 1436 "Signed assertion by the attestation source that they have attested to the information.", 0, 1, 1437 sourceSignature); 1438 default: 1439 return super.getNamedProperty(_hash, _name, _checkValid); 1440 } 1441 1442 } 1443 1444 @Override 1445 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1446 switch (hash) { 1447 case 117694: 1448 /* who */ return this.who == null ? new Base[0] : new Base[] { this.who }; // Reference 1449 case -14402964: 1450 /* onBehalfOf */ return this.onBehalfOf == null ? new Base[0] : new Base[] { this.onBehalfOf }; // Reference 1451 case 1314116695: 1452 /* communicationMethod */ return this.communicationMethod == null ? new Base[0] 1453 : new Base[] { this.communicationMethod }; // CodeableConcept 1454 case 3076014: 1455 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateType 1456 case -799067682: 1457 /* sourceIdentityCertificate */ return this.sourceIdentityCertificate == null ? new Base[0] 1458 : new Base[] { this.sourceIdentityCertificate }; // StringType 1459 case 431558827: 1460 /* proxyIdentityCertificate */ return this.proxyIdentityCertificate == null ? new Base[0] 1461 : new Base[] { this.proxyIdentityCertificate }; // StringType 1462 case 1455540714: 1463 /* proxySignature */ return this.proxySignature == null ? new Base[0] : new Base[] { this.proxySignature }; // Signature 1464 case 1754480349: 1465 /* sourceSignature */ return this.sourceSignature == null ? new Base[0] : new Base[] { this.sourceSignature }; // Signature 1466 default: 1467 return super.getProperty(hash, name, checkValid); 1468 } 1469 1470 } 1471 1472 @Override 1473 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1474 switch (hash) { 1475 case 117694: // who 1476 this.who = castToReference(value); // Reference 1477 return value; 1478 case -14402964: // onBehalfOf 1479 this.onBehalfOf = castToReference(value); // Reference 1480 return value; 1481 case 1314116695: // communicationMethod 1482 this.communicationMethod = castToCodeableConcept(value); // CodeableConcept 1483 return value; 1484 case 3076014: // date 1485 this.date = castToDate(value); // DateType 1486 return value; 1487 case -799067682: // sourceIdentityCertificate 1488 this.sourceIdentityCertificate = castToString(value); // StringType 1489 return value; 1490 case 431558827: // proxyIdentityCertificate 1491 this.proxyIdentityCertificate = castToString(value); // StringType 1492 return value; 1493 case 1455540714: // proxySignature 1494 this.proxySignature = castToSignature(value); // Signature 1495 return value; 1496 case 1754480349: // sourceSignature 1497 this.sourceSignature = castToSignature(value); // Signature 1498 return value; 1499 default: 1500 return super.setProperty(hash, name, value); 1501 } 1502 1503 } 1504 1505 @Override 1506 public Base setProperty(String name, Base value) throws FHIRException { 1507 if (name.equals("who")) { 1508 this.who = castToReference(value); // Reference 1509 } else if (name.equals("onBehalfOf")) { 1510 this.onBehalfOf = castToReference(value); // Reference 1511 } else if (name.equals("communicationMethod")) { 1512 this.communicationMethod = castToCodeableConcept(value); // CodeableConcept 1513 } else if (name.equals("date")) { 1514 this.date = castToDate(value); // DateType 1515 } else if (name.equals("sourceIdentityCertificate")) { 1516 this.sourceIdentityCertificate = castToString(value); // StringType 1517 } else if (name.equals("proxyIdentityCertificate")) { 1518 this.proxyIdentityCertificate = castToString(value); // StringType 1519 } else if (name.equals("proxySignature")) { 1520 this.proxySignature = castToSignature(value); // Signature 1521 } else if (name.equals("sourceSignature")) { 1522 this.sourceSignature = castToSignature(value); // Signature 1523 } else 1524 return super.setProperty(name, value); 1525 return value; 1526 } 1527 1528 @Override 1529 public void removeChild(String name, Base value) throws FHIRException { 1530 if (name.equals("who")) { 1531 this.who = null; 1532 } else if (name.equals("onBehalfOf")) { 1533 this.onBehalfOf = null; 1534 } else if (name.equals("communicationMethod")) { 1535 this.communicationMethod = null; 1536 } else if (name.equals("date")) { 1537 this.date = null; 1538 } else if (name.equals("sourceIdentityCertificate")) { 1539 this.sourceIdentityCertificate = null; 1540 } else if (name.equals("proxyIdentityCertificate")) { 1541 this.proxyIdentityCertificate = null; 1542 } else if (name.equals("proxySignature")) { 1543 this.proxySignature = null; 1544 } else if (name.equals("sourceSignature")) { 1545 this.sourceSignature = null; 1546 } else 1547 super.removeChild(name, value); 1548 1549 } 1550 1551 @Override 1552 public Base makeProperty(int hash, String name) throws FHIRException { 1553 switch (hash) { 1554 case 117694: 1555 return getWho(); 1556 case -14402964: 1557 return getOnBehalfOf(); 1558 case 1314116695: 1559 return getCommunicationMethod(); 1560 case 3076014: 1561 return getDateElement(); 1562 case -799067682: 1563 return getSourceIdentityCertificateElement(); 1564 case 431558827: 1565 return getProxyIdentityCertificateElement(); 1566 case 1455540714: 1567 return getProxySignature(); 1568 case 1754480349: 1569 return getSourceSignature(); 1570 default: 1571 return super.makeProperty(hash, name); 1572 } 1573 1574 } 1575 1576 @Override 1577 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1578 switch (hash) { 1579 case 117694: 1580 /* who */ return new String[] { "Reference" }; 1581 case -14402964: 1582 /* onBehalfOf */ return new String[] { "Reference" }; 1583 case 1314116695: 1584 /* communicationMethod */ return new String[] { "CodeableConcept" }; 1585 case 3076014: 1586 /* date */ return new String[] { "date" }; 1587 case -799067682: 1588 /* sourceIdentityCertificate */ return new String[] { "string" }; 1589 case 431558827: 1590 /* proxyIdentityCertificate */ return new String[] { "string" }; 1591 case 1455540714: 1592 /* proxySignature */ return new String[] { "Signature" }; 1593 case 1754480349: 1594 /* sourceSignature */ return new String[] { "Signature" }; 1595 default: 1596 return super.getTypesForProperty(hash, name); 1597 } 1598 1599 } 1600 1601 @Override 1602 public Base addChild(String name) throws FHIRException { 1603 if (name.equals("who")) { 1604 this.who = new Reference(); 1605 return this.who; 1606 } else if (name.equals("onBehalfOf")) { 1607 this.onBehalfOf = new Reference(); 1608 return this.onBehalfOf; 1609 } else if (name.equals("communicationMethod")) { 1610 this.communicationMethod = new CodeableConcept(); 1611 return this.communicationMethod; 1612 } else if (name.equals("date")) { 1613 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.date"); 1614 } else if (name.equals("sourceIdentityCertificate")) { 1615 throw new FHIRException( 1616 "Cannot call addChild on a singleton property VerificationResult.sourceIdentityCertificate"); 1617 } else if (name.equals("proxyIdentityCertificate")) { 1618 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.proxyIdentityCertificate"); 1619 } else if (name.equals("proxySignature")) { 1620 this.proxySignature = new Signature(); 1621 return this.proxySignature; 1622 } else if (name.equals("sourceSignature")) { 1623 this.sourceSignature = new Signature(); 1624 return this.sourceSignature; 1625 } else 1626 return super.addChild(name); 1627 } 1628 1629 public VerificationResultAttestationComponent copy() { 1630 VerificationResultAttestationComponent dst = new VerificationResultAttestationComponent(); 1631 copyValues(dst); 1632 return dst; 1633 } 1634 1635 public void copyValues(VerificationResultAttestationComponent dst) { 1636 super.copyValues(dst); 1637 dst.who = who == null ? null : who.copy(); 1638 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 1639 dst.communicationMethod = communicationMethod == null ? null : communicationMethod.copy(); 1640 dst.date = date == null ? null : date.copy(); 1641 dst.sourceIdentityCertificate = sourceIdentityCertificate == null ? null : sourceIdentityCertificate.copy(); 1642 dst.proxyIdentityCertificate = proxyIdentityCertificate == null ? null : proxyIdentityCertificate.copy(); 1643 dst.proxySignature = proxySignature == null ? null : proxySignature.copy(); 1644 dst.sourceSignature = sourceSignature == null ? null : sourceSignature.copy(); 1645 } 1646 1647 @Override 1648 public boolean equalsDeep(Base other_) { 1649 if (!super.equalsDeep(other_)) 1650 return false; 1651 if (!(other_ instanceof VerificationResultAttestationComponent)) 1652 return false; 1653 VerificationResultAttestationComponent o = (VerificationResultAttestationComponent) other_; 1654 return compareDeep(who, o.who, true) && compareDeep(onBehalfOf, o.onBehalfOf, true) 1655 && compareDeep(communicationMethod, o.communicationMethod, true) && compareDeep(date, o.date, true) 1656 && compareDeep(sourceIdentityCertificate, o.sourceIdentityCertificate, true) 1657 && compareDeep(proxyIdentityCertificate, o.proxyIdentityCertificate, true) 1658 && compareDeep(proxySignature, o.proxySignature, true) 1659 && compareDeep(sourceSignature, o.sourceSignature, true); 1660 } 1661 1662 @Override 1663 public boolean equalsShallow(Base other_) { 1664 if (!super.equalsShallow(other_)) 1665 return false; 1666 if (!(other_ instanceof VerificationResultAttestationComponent)) 1667 return false; 1668 VerificationResultAttestationComponent o = (VerificationResultAttestationComponent) other_; 1669 return compareValues(date, o.date, true) 1670 && compareValues(sourceIdentityCertificate, o.sourceIdentityCertificate, true) 1671 && compareValues(proxyIdentityCertificate, o.proxyIdentityCertificate, true); 1672 } 1673 1674 public boolean isEmpty() { 1675 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(who, onBehalfOf, communicationMethod, date, 1676 sourceIdentityCertificate, proxyIdentityCertificate, proxySignature, sourceSignature); 1677 } 1678 1679 public String fhirType() { 1680 return "VerificationResult.attestation"; 1681 1682 } 1683 1684 } 1685 1686 @Block() 1687 public static class VerificationResultValidatorComponent extends BackboneElement implements IBaseBackboneElement { 1688 /** 1689 * Reference to the organization validating information. 1690 */ 1691 @Child(name = "organization", type = { 1692 Organization.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1693 @Description(shortDefinition = "Reference to the organization validating information", formalDefinition = "Reference to the organization validating information.") 1694 protected Reference organization; 1695 1696 /** 1697 * The actual object that is the target of the reference (Reference to the 1698 * organization validating information.) 1699 */ 1700 protected Organization organizationTarget; 1701 1702 /** 1703 * A digital identity certificate associated with the validator. 1704 */ 1705 @Child(name = "identityCertificate", type = { 1706 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1707 @Description(shortDefinition = "A digital identity certificate associated with the validator", formalDefinition = "A digital identity certificate associated with the validator.") 1708 protected StringType identityCertificate; 1709 1710 /** 1711 * Signed assertion by the validator that they have validated the information. 1712 */ 1713 @Child(name = "attestationSignature", type = { 1714 Signature.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1715 @Description(shortDefinition = "Validator signature", formalDefinition = "Signed assertion by the validator that they have validated the information.") 1716 protected Signature attestationSignature; 1717 1718 private static final long serialVersionUID = 35580619L; 1719 1720 /** 1721 * Constructor 1722 */ 1723 public VerificationResultValidatorComponent() { 1724 super(); 1725 } 1726 1727 /** 1728 * Constructor 1729 */ 1730 public VerificationResultValidatorComponent(Reference organization) { 1731 super(); 1732 this.organization = organization; 1733 } 1734 1735 /** 1736 * @return {@link #organization} (Reference to the organization validating 1737 * information.) 1738 */ 1739 public Reference getOrganization() { 1740 if (this.organization == null) 1741 if (Configuration.errorOnAutoCreate()) 1742 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.organization"); 1743 else if (Configuration.doAutoCreate()) 1744 this.organization = new Reference(); // cc 1745 return this.organization; 1746 } 1747 1748 public boolean hasOrganization() { 1749 return this.organization != null && !this.organization.isEmpty(); 1750 } 1751 1752 /** 1753 * @param value {@link #organization} (Reference to the organization validating 1754 * information.) 1755 */ 1756 public VerificationResultValidatorComponent setOrganization(Reference value) { 1757 this.organization = value; 1758 return this; 1759 } 1760 1761 /** 1762 * @return {@link #organization} The actual object that is the target of the 1763 * reference. The reference library doesn't populate this, but you can 1764 * use it to hold the resource if you resolve it. (Reference to the 1765 * organization validating information.) 1766 */ 1767 public Organization getOrganizationTarget() { 1768 if (this.organizationTarget == null) 1769 if (Configuration.errorOnAutoCreate()) 1770 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.organization"); 1771 else if (Configuration.doAutoCreate()) 1772 this.organizationTarget = new Organization(); // aa 1773 return this.organizationTarget; 1774 } 1775 1776 /** 1777 * @param value {@link #organization} The actual object that is the target of 1778 * the reference. The reference library doesn't use these, but you 1779 * can use it to hold the resource if you resolve it. (Reference to 1780 * the organization validating information.) 1781 */ 1782 public VerificationResultValidatorComponent setOrganizationTarget(Organization value) { 1783 this.organizationTarget = value; 1784 return this; 1785 } 1786 1787 /** 1788 * @return {@link #identityCertificate} (A digital identity certificate 1789 * associated with the validator.). This is the underlying object with 1790 * id, value and extensions. The accessor "getIdentityCertificate" gives 1791 * direct access to the value 1792 */ 1793 public StringType getIdentityCertificateElement() { 1794 if (this.identityCertificate == null) 1795 if (Configuration.errorOnAutoCreate()) 1796 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.identityCertificate"); 1797 else if (Configuration.doAutoCreate()) 1798 this.identityCertificate = new StringType(); // bb 1799 return this.identityCertificate; 1800 } 1801 1802 public boolean hasIdentityCertificateElement() { 1803 return this.identityCertificate != null && !this.identityCertificate.isEmpty(); 1804 } 1805 1806 public boolean hasIdentityCertificate() { 1807 return this.identityCertificate != null && !this.identityCertificate.isEmpty(); 1808 } 1809 1810 /** 1811 * @param value {@link #identityCertificate} (A digital identity certificate 1812 * associated with the validator.). This is the underlying object 1813 * with id, value and extensions. The accessor 1814 * "getIdentityCertificate" gives direct access to the value 1815 */ 1816 public VerificationResultValidatorComponent setIdentityCertificateElement(StringType value) { 1817 this.identityCertificate = value; 1818 return this; 1819 } 1820 1821 /** 1822 * @return A digital identity certificate associated with the validator. 1823 */ 1824 public String getIdentityCertificate() { 1825 return this.identityCertificate == null ? null : this.identityCertificate.getValue(); 1826 } 1827 1828 /** 1829 * @param value A digital identity certificate associated with the validator. 1830 */ 1831 public VerificationResultValidatorComponent setIdentityCertificate(String value) { 1832 if (Utilities.noString(value)) 1833 this.identityCertificate = null; 1834 else { 1835 if (this.identityCertificate == null) 1836 this.identityCertificate = new StringType(); 1837 this.identityCertificate.setValue(value); 1838 } 1839 return this; 1840 } 1841 1842 /** 1843 * @return {@link #attestationSignature} (Signed assertion by the validator that 1844 * they have validated the information.) 1845 */ 1846 public Signature getAttestationSignature() { 1847 if (this.attestationSignature == null) 1848 if (Configuration.errorOnAutoCreate()) 1849 throw new Error("Attempt to auto-create VerificationResultValidatorComponent.attestationSignature"); 1850 else if (Configuration.doAutoCreate()) 1851 this.attestationSignature = new Signature(); // cc 1852 return this.attestationSignature; 1853 } 1854 1855 public boolean hasAttestationSignature() { 1856 return this.attestationSignature != null && !this.attestationSignature.isEmpty(); 1857 } 1858 1859 /** 1860 * @param value {@link #attestationSignature} (Signed assertion by the validator 1861 * that they have validated the information.) 1862 */ 1863 public VerificationResultValidatorComponent setAttestationSignature(Signature value) { 1864 this.attestationSignature = value; 1865 return this; 1866 } 1867 1868 protected void listChildren(List<Property> children) { 1869 super.listChildren(children); 1870 children.add(new Property("organization", "Reference(Organization)", 1871 "Reference to the organization validating information.", 0, 1, organization)); 1872 children.add(new Property("identityCertificate", "string", 1873 "A digital identity certificate associated with the validator.", 0, 1, identityCertificate)); 1874 children.add(new Property("attestationSignature", "Signature", 1875 "Signed assertion by the validator that they have validated the information.", 0, 1, attestationSignature)); 1876 } 1877 1878 @Override 1879 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1880 switch (_hash) { 1881 case 1178922291: 1882 /* organization */ return new Property("organization", "Reference(Organization)", 1883 "Reference to the organization validating information.", 0, 1, organization); 1884 case -854379015: 1885 /* identityCertificate */ return new Property("identityCertificate", "string", 1886 "A digital identity certificate associated with the validator.", 0, 1, identityCertificate); 1887 case -184196152: 1888 /* attestationSignature */ return new Property("attestationSignature", "Signature", 1889 "Signed assertion by the validator that they have validated the information.", 0, 1, attestationSignature); 1890 default: 1891 return super.getNamedProperty(_hash, _name, _checkValid); 1892 } 1893 1894 } 1895 1896 @Override 1897 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1898 switch (hash) { 1899 case 1178922291: 1900 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 1901 case -854379015: 1902 /* identityCertificate */ return this.identityCertificate == null ? new Base[0] 1903 : new Base[] { this.identityCertificate }; // StringType 1904 case -184196152: 1905 /* attestationSignature */ return this.attestationSignature == null ? new Base[0] 1906 : new Base[] { this.attestationSignature }; // Signature 1907 default: 1908 return super.getProperty(hash, name, checkValid); 1909 } 1910 1911 } 1912 1913 @Override 1914 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1915 switch (hash) { 1916 case 1178922291: // organization 1917 this.organization = castToReference(value); // Reference 1918 return value; 1919 case -854379015: // identityCertificate 1920 this.identityCertificate = castToString(value); // StringType 1921 return value; 1922 case -184196152: // attestationSignature 1923 this.attestationSignature = castToSignature(value); // Signature 1924 return value; 1925 default: 1926 return super.setProperty(hash, name, value); 1927 } 1928 1929 } 1930 1931 @Override 1932 public Base setProperty(String name, Base value) throws FHIRException { 1933 if (name.equals("organization")) { 1934 this.organization = castToReference(value); // Reference 1935 } else if (name.equals("identityCertificate")) { 1936 this.identityCertificate = castToString(value); // StringType 1937 } else if (name.equals("attestationSignature")) { 1938 this.attestationSignature = castToSignature(value); // Signature 1939 } else 1940 return super.setProperty(name, value); 1941 return value; 1942 } 1943 1944 @Override 1945 public void removeChild(String name, Base value) throws FHIRException { 1946 if (name.equals("organization")) { 1947 this.organization = null; 1948 } else if (name.equals("identityCertificate")) { 1949 this.identityCertificate = null; 1950 } else if (name.equals("attestationSignature")) { 1951 this.attestationSignature = null; 1952 } else 1953 super.removeChild(name, value); 1954 1955 } 1956 1957 @Override 1958 public Base makeProperty(int hash, String name) throws FHIRException { 1959 switch (hash) { 1960 case 1178922291: 1961 return getOrganization(); 1962 case -854379015: 1963 return getIdentityCertificateElement(); 1964 case -184196152: 1965 return getAttestationSignature(); 1966 default: 1967 return super.makeProperty(hash, name); 1968 } 1969 1970 } 1971 1972 @Override 1973 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1974 switch (hash) { 1975 case 1178922291: 1976 /* organization */ return new String[] { "Reference" }; 1977 case -854379015: 1978 /* identityCertificate */ return new String[] { "string" }; 1979 case -184196152: 1980 /* attestationSignature */ return new String[] { "Signature" }; 1981 default: 1982 return super.getTypesForProperty(hash, name); 1983 } 1984 1985 } 1986 1987 @Override 1988 public Base addChild(String name) throws FHIRException { 1989 if (name.equals("organization")) { 1990 this.organization = new Reference(); 1991 return this.organization; 1992 } else if (name.equals("identityCertificate")) { 1993 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.identityCertificate"); 1994 } else if (name.equals("attestationSignature")) { 1995 this.attestationSignature = new Signature(); 1996 return this.attestationSignature; 1997 } else 1998 return super.addChild(name); 1999 } 2000 2001 public VerificationResultValidatorComponent copy() { 2002 VerificationResultValidatorComponent dst = new VerificationResultValidatorComponent(); 2003 copyValues(dst); 2004 return dst; 2005 } 2006 2007 public void copyValues(VerificationResultValidatorComponent dst) { 2008 super.copyValues(dst); 2009 dst.organization = organization == null ? null : organization.copy(); 2010 dst.identityCertificate = identityCertificate == null ? null : identityCertificate.copy(); 2011 dst.attestationSignature = attestationSignature == null ? null : attestationSignature.copy(); 2012 } 2013 2014 @Override 2015 public boolean equalsDeep(Base other_) { 2016 if (!super.equalsDeep(other_)) 2017 return false; 2018 if (!(other_ instanceof VerificationResultValidatorComponent)) 2019 return false; 2020 VerificationResultValidatorComponent o = (VerificationResultValidatorComponent) other_; 2021 return compareDeep(organization, o.organization, true) 2022 && compareDeep(identityCertificate, o.identityCertificate, true) 2023 && compareDeep(attestationSignature, o.attestationSignature, true); 2024 } 2025 2026 @Override 2027 public boolean equalsShallow(Base other_) { 2028 if (!super.equalsShallow(other_)) 2029 return false; 2030 if (!(other_ instanceof VerificationResultValidatorComponent)) 2031 return false; 2032 VerificationResultValidatorComponent o = (VerificationResultValidatorComponent) other_; 2033 return compareValues(identityCertificate, o.identityCertificate, true); 2034 } 2035 2036 public boolean isEmpty() { 2037 return super.isEmpty() 2038 && ca.uhn.fhir.util.ElementUtil.isEmpty(organization, identityCertificate, attestationSignature); 2039 } 2040 2041 public String fhirType() { 2042 return "VerificationResult.validator"; 2043 2044 } 2045 2046 } 2047 2048 /** 2049 * A resource that was validated. 2050 */ 2051 @Child(name = "target", type = { 2052 Reference.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2053 @Description(shortDefinition = "A resource that was validated", formalDefinition = "A resource that was validated.") 2054 protected List<Reference> target; 2055 /** 2056 * The actual objects that are the target of the reference (A resource that was 2057 * validated.) 2058 */ 2059 protected List<Resource> targetTarget; 2060 2061 /** 2062 * The fhirpath location(s) within the resource that was validated. 2063 */ 2064 @Child(name = "targetLocation", type = { 2065 StringType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2066 @Description(shortDefinition = "The fhirpath location(s) within the resource that was validated", formalDefinition = "The fhirpath location(s) within the resource that was validated.") 2067 protected List<StringType> targetLocation; 2068 2069 /** 2070 * The frequency with which the target must be validated (none; initial; 2071 * periodic). 2072 */ 2073 @Child(name = "need", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2074 @Description(shortDefinition = "none | initial | periodic", formalDefinition = "The frequency with which the target must be validated (none; initial; periodic).") 2075 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-need") 2076 protected CodeableConcept need; 2077 2078 /** 2079 * The validation status of the target (attested; validated; in process; 2080 * requires revalidation; validation failed; revalidation failed). 2081 */ 2082 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2083 @Description(shortDefinition = "attested | validated | in-process | req-revalid | val-fail | reval-fail", formalDefinition = "The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).") 2084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-status") 2085 protected Enumeration<Status> status; 2086 2087 /** 2088 * When the validation status was updated. 2089 */ 2090 @Child(name = "statusDate", type = { 2091 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2092 @Description(shortDefinition = "When the validation status was updated", formalDefinition = "When the validation status was updated.") 2093 protected DateTimeType statusDate; 2094 2095 /** 2096 * What the target is validated against (nothing; primary source; multiple 2097 * sources). 2098 */ 2099 @Child(name = "validationType", type = { 2100 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2101 @Description(shortDefinition = "nothing | primary | multiple", formalDefinition = "What the target is validated against (nothing; primary source; multiple sources).") 2102 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-validation-type") 2103 protected CodeableConcept validationType; 2104 2105 /** 2106 * The primary process by which the target is validated (edit check; value set; 2107 * primary source; multiple sources; standalone; in context). 2108 */ 2109 @Child(name = "validationProcess", type = { 2110 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2111 @Description(shortDefinition = "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context)", formalDefinition = "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).") 2112 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-validation-process") 2113 protected List<CodeableConcept> validationProcess; 2114 2115 /** 2116 * Frequency of revalidation. 2117 */ 2118 @Child(name = "frequency", type = { Timing.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2119 @Description(shortDefinition = "Frequency of revalidation", formalDefinition = "Frequency of revalidation.") 2120 protected Timing frequency; 2121 2122 /** 2123 * The date/time validation was last completed (including failed validations). 2124 */ 2125 @Child(name = "lastPerformed", type = { 2126 DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2127 @Description(shortDefinition = "The date/time validation was last completed (including failed validations)", formalDefinition = "The date/time validation was last completed (including failed validations).") 2128 protected DateTimeType lastPerformed; 2129 2130 /** 2131 * The date when target is next validated, if appropriate. 2132 */ 2133 @Child(name = "nextScheduled", type = { 2134 DateType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 2135 @Description(shortDefinition = "The date when target is next validated, if appropriate", formalDefinition = "The date when target is next validated, if appropriate.") 2136 protected DateType nextScheduled; 2137 2138 /** 2139 * The result if validation fails (fatal; warning; record only; none). 2140 */ 2141 @Child(name = "failureAction", type = { 2142 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 2143 @Description(shortDefinition = "fatal | warn | rec-only | none", formalDefinition = "The result if validation fails (fatal; warning; record only; none).") 2144 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/verificationresult-failure-action") 2145 protected CodeableConcept failureAction; 2146 2147 /** 2148 * Information about the primary source(s) involved in validation. 2149 */ 2150 @Child(name = "primarySource", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2151 @Description(shortDefinition = "Information about the primary source(s) involved in validation", formalDefinition = "Information about the primary source(s) involved in validation.") 2152 protected List<VerificationResultPrimarySourceComponent> primarySource; 2153 2154 /** 2155 * Information about the entity attesting to information. 2156 */ 2157 @Child(name = "attestation", type = {}, order = 12, min = 0, max = 1, modifier = false, summary = false) 2158 @Description(shortDefinition = "Information about the entity attesting to information", formalDefinition = "Information about the entity attesting to information.") 2159 protected VerificationResultAttestationComponent attestation; 2160 2161 /** 2162 * Information about the entity validating information. 2163 */ 2164 @Child(name = "validator", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2165 @Description(shortDefinition = "Information about the entity validating information", formalDefinition = "Information about the entity validating information.") 2166 protected List<VerificationResultValidatorComponent> validator; 2167 2168 private static final long serialVersionUID = -284059204L; 2169 2170 /** 2171 * Constructor 2172 */ 2173 public VerificationResult() { 2174 super(); 2175 } 2176 2177 /** 2178 * Constructor 2179 */ 2180 public VerificationResult(Enumeration<Status> status) { 2181 super(); 2182 this.status = status; 2183 } 2184 2185 /** 2186 * @return {@link #target} (A resource that was validated.) 2187 */ 2188 public List<Reference> getTarget() { 2189 if (this.target == null) 2190 this.target = new ArrayList<Reference>(); 2191 return this.target; 2192 } 2193 2194 /** 2195 * @return Returns a reference to <code>this</code> for easy method chaining 2196 */ 2197 public VerificationResult setTarget(List<Reference> theTarget) { 2198 this.target = theTarget; 2199 return this; 2200 } 2201 2202 public boolean hasTarget() { 2203 if (this.target == null) 2204 return false; 2205 for (Reference item : this.target) 2206 if (!item.isEmpty()) 2207 return true; 2208 return false; 2209 } 2210 2211 public Reference addTarget() { // 3 2212 Reference t = new Reference(); 2213 if (this.target == null) 2214 this.target = new ArrayList<Reference>(); 2215 this.target.add(t); 2216 return t; 2217 } 2218 2219 public VerificationResult addTarget(Reference t) { // 3 2220 if (t == null) 2221 return this; 2222 if (this.target == null) 2223 this.target = new ArrayList<Reference>(); 2224 this.target.add(t); 2225 return this; 2226 } 2227 2228 /** 2229 * @return The first repetition of repeating field {@link #target}, creating it 2230 * if it does not already exist 2231 */ 2232 public Reference getTargetFirstRep() { 2233 if (getTarget().isEmpty()) { 2234 addTarget(); 2235 } 2236 return getTarget().get(0); 2237 } 2238 2239 /** 2240 * @deprecated Use Reference#setResource(IBaseResource) instead 2241 */ 2242 @Deprecated 2243 public List<Resource> getTargetTarget() { 2244 if (this.targetTarget == null) 2245 this.targetTarget = new ArrayList<Resource>(); 2246 return this.targetTarget; 2247 } 2248 2249 /** 2250 * @return {@link #targetLocation} (The fhirpath location(s) within the resource 2251 * that was validated.) 2252 */ 2253 public List<StringType> getTargetLocation() { 2254 if (this.targetLocation == null) 2255 this.targetLocation = new ArrayList<StringType>(); 2256 return this.targetLocation; 2257 } 2258 2259 /** 2260 * @return Returns a reference to <code>this</code> for easy method chaining 2261 */ 2262 public VerificationResult setTargetLocation(List<StringType> theTargetLocation) { 2263 this.targetLocation = theTargetLocation; 2264 return this; 2265 } 2266 2267 public boolean hasTargetLocation() { 2268 if (this.targetLocation == null) 2269 return false; 2270 for (StringType item : this.targetLocation) 2271 if (!item.isEmpty()) 2272 return true; 2273 return false; 2274 } 2275 2276 /** 2277 * @return {@link #targetLocation} (The fhirpath location(s) within the resource 2278 * that was validated.) 2279 */ 2280 public StringType addTargetLocationElement() {// 2 2281 StringType t = new StringType(); 2282 if (this.targetLocation == null) 2283 this.targetLocation = new ArrayList<StringType>(); 2284 this.targetLocation.add(t); 2285 return t; 2286 } 2287 2288 /** 2289 * @param value {@link #targetLocation} (The fhirpath location(s) within the 2290 * resource that was validated.) 2291 */ 2292 public VerificationResult addTargetLocation(String value) { // 1 2293 StringType t = new StringType(); 2294 t.setValue(value); 2295 if (this.targetLocation == null) 2296 this.targetLocation = new ArrayList<StringType>(); 2297 this.targetLocation.add(t); 2298 return this; 2299 } 2300 2301 /** 2302 * @param value {@link #targetLocation} (The fhirpath location(s) within the 2303 * resource that was validated.) 2304 */ 2305 public boolean hasTargetLocation(String value) { 2306 if (this.targetLocation == null) 2307 return false; 2308 for (StringType v : this.targetLocation) 2309 if (v.getValue().equals(value)) // string 2310 return true; 2311 return false; 2312 } 2313 2314 /** 2315 * @return {@link #need} (The frequency with which the target must be validated 2316 * (none; initial; periodic).) 2317 */ 2318 public CodeableConcept getNeed() { 2319 if (this.need == null) 2320 if (Configuration.errorOnAutoCreate()) 2321 throw new Error("Attempt to auto-create VerificationResult.need"); 2322 else if (Configuration.doAutoCreate()) 2323 this.need = new CodeableConcept(); // cc 2324 return this.need; 2325 } 2326 2327 public boolean hasNeed() { 2328 return this.need != null && !this.need.isEmpty(); 2329 } 2330 2331 /** 2332 * @param value {@link #need} (The frequency with which the target must be 2333 * validated (none; initial; periodic).) 2334 */ 2335 public VerificationResult setNeed(CodeableConcept value) { 2336 this.need = value; 2337 return this; 2338 } 2339 2340 /** 2341 * @return {@link #status} (The validation status of the target (attested; 2342 * validated; in process; requires revalidation; validation failed; 2343 * revalidation failed).). This is the underlying object with id, value 2344 * and extensions. The accessor "getStatus" gives direct access to the 2345 * value 2346 */ 2347 public Enumeration<Status> getStatusElement() { 2348 if (this.status == null) 2349 if (Configuration.errorOnAutoCreate()) 2350 throw new Error("Attempt to auto-create VerificationResult.status"); 2351 else if (Configuration.doAutoCreate()) 2352 this.status = new Enumeration<Status>(new StatusEnumFactory()); // bb 2353 return this.status; 2354 } 2355 2356 public boolean hasStatusElement() { 2357 return this.status != null && !this.status.isEmpty(); 2358 } 2359 2360 public boolean hasStatus() { 2361 return this.status != null && !this.status.isEmpty(); 2362 } 2363 2364 /** 2365 * @param value {@link #status} (The validation status of the target (attested; 2366 * validated; in process; requires revalidation; validation failed; 2367 * revalidation failed).). This is the underlying object with id, 2368 * value and extensions. The accessor "getStatus" gives direct 2369 * access to the value 2370 */ 2371 public VerificationResult setStatusElement(Enumeration<Status> value) { 2372 this.status = value; 2373 return this; 2374 } 2375 2376 /** 2377 * @return The validation status of the target (attested; validated; in process; 2378 * requires revalidation; validation failed; revalidation failed). 2379 */ 2380 public Status getStatus() { 2381 return this.status == null ? null : this.status.getValue(); 2382 } 2383 2384 /** 2385 * @param value The validation status of the target (attested; validated; in 2386 * process; requires revalidation; validation failed; revalidation 2387 * failed). 2388 */ 2389 public VerificationResult setStatus(Status value) { 2390 if (this.status == null) 2391 this.status = new Enumeration<Status>(new StatusEnumFactory()); 2392 this.status.setValue(value); 2393 return this; 2394 } 2395 2396 /** 2397 * @return {@link #statusDate} (When the validation status was updated.). This 2398 * is the underlying object with id, value and extensions. The accessor 2399 * "getStatusDate" gives direct access to the value 2400 */ 2401 public DateTimeType getStatusDateElement() { 2402 if (this.statusDate == null) 2403 if (Configuration.errorOnAutoCreate()) 2404 throw new Error("Attempt to auto-create VerificationResult.statusDate"); 2405 else if (Configuration.doAutoCreate()) 2406 this.statusDate = new DateTimeType(); // bb 2407 return this.statusDate; 2408 } 2409 2410 public boolean hasStatusDateElement() { 2411 return this.statusDate != null && !this.statusDate.isEmpty(); 2412 } 2413 2414 public boolean hasStatusDate() { 2415 return this.statusDate != null && !this.statusDate.isEmpty(); 2416 } 2417 2418 /** 2419 * @param value {@link #statusDate} (When the validation status was updated.). 2420 * This is the underlying object with id, value and extensions. The 2421 * accessor "getStatusDate" gives direct access to the value 2422 */ 2423 public VerificationResult setStatusDateElement(DateTimeType value) { 2424 this.statusDate = value; 2425 return this; 2426 } 2427 2428 /** 2429 * @return When the validation status was updated. 2430 */ 2431 public Date getStatusDate() { 2432 return this.statusDate == null ? null : this.statusDate.getValue(); 2433 } 2434 2435 /** 2436 * @param value When the validation status was updated. 2437 */ 2438 public VerificationResult setStatusDate(Date value) { 2439 if (value == null) 2440 this.statusDate = null; 2441 else { 2442 if (this.statusDate == null) 2443 this.statusDate = new DateTimeType(); 2444 this.statusDate.setValue(value); 2445 } 2446 return this; 2447 } 2448 2449 /** 2450 * @return {@link #validationType} (What the target is validated against 2451 * (nothing; primary source; multiple sources).) 2452 */ 2453 public CodeableConcept getValidationType() { 2454 if (this.validationType == null) 2455 if (Configuration.errorOnAutoCreate()) 2456 throw new Error("Attempt to auto-create VerificationResult.validationType"); 2457 else if (Configuration.doAutoCreate()) 2458 this.validationType = new CodeableConcept(); // cc 2459 return this.validationType; 2460 } 2461 2462 public boolean hasValidationType() { 2463 return this.validationType != null && !this.validationType.isEmpty(); 2464 } 2465 2466 /** 2467 * @param value {@link #validationType} (What the target is validated against 2468 * (nothing; primary source; multiple sources).) 2469 */ 2470 public VerificationResult setValidationType(CodeableConcept value) { 2471 this.validationType = value; 2472 return this; 2473 } 2474 2475 /** 2476 * @return {@link #validationProcess} (The primary process by which the target 2477 * is validated (edit check; value set; primary source; multiple 2478 * sources; standalone; in context).) 2479 */ 2480 public List<CodeableConcept> getValidationProcess() { 2481 if (this.validationProcess == null) 2482 this.validationProcess = new ArrayList<CodeableConcept>(); 2483 return this.validationProcess; 2484 } 2485 2486 /** 2487 * @return Returns a reference to <code>this</code> for easy method chaining 2488 */ 2489 public VerificationResult setValidationProcess(List<CodeableConcept> theValidationProcess) { 2490 this.validationProcess = theValidationProcess; 2491 return this; 2492 } 2493 2494 public boolean hasValidationProcess() { 2495 if (this.validationProcess == null) 2496 return false; 2497 for (CodeableConcept item : this.validationProcess) 2498 if (!item.isEmpty()) 2499 return true; 2500 return false; 2501 } 2502 2503 public CodeableConcept addValidationProcess() { // 3 2504 CodeableConcept t = new CodeableConcept(); 2505 if (this.validationProcess == null) 2506 this.validationProcess = new ArrayList<CodeableConcept>(); 2507 this.validationProcess.add(t); 2508 return t; 2509 } 2510 2511 public VerificationResult addValidationProcess(CodeableConcept t) { // 3 2512 if (t == null) 2513 return this; 2514 if (this.validationProcess == null) 2515 this.validationProcess = new ArrayList<CodeableConcept>(); 2516 this.validationProcess.add(t); 2517 return this; 2518 } 2519 2520 /** 2521 * @return The first repetition of repeating field {@link #validationProcess}, 2522 * creating it if it does not already exist 2523 */ 2524 public CodeableConcept getValidationProcessFirstRep() { 2525 if (getValidationProcess().isEmpty()) { 2526 addValidationProcess(); 2527 } 2528 return getValidationProcess().get(0); 2529 } 2530 2531 /** 2532 * @return {@link #frequency} (Frequency of revalidation.) 2533 */ 2534 public Timing getFrequency() { 2535 if (this.frequency == null) 2536 if (Configuration.errorOnAutoCreate()) 2537 throw new Error("Attempt to auto-create VerificationResult.frequency"); 2538 else if (Configuration.doAutoCreate()) 2539 this.frequency = new Timing(); // cc 2540 return this.frequency; 2541 } 2542 2543 public boolean hasFrequency() { 2544 return this.frequency != null && !this.frequency.isEmpty(); 2545 } 2546 2547 /** 2548 * @param value {@link #frequency} (Frequency of revalidation.) 2549 */ 2550 public VerificationResult setFrequency(Timing value) { 2551 this.frequency = value; 2552 return this; 2553 } 2554 2555 /** 2556 * @return {@link #lastPerformed} (The date/time validation was last completed 2557 * (including failed validations).). This is the underlying object with 2558 * id, value and extensions. The accessor "getLastPerformed" gives 2559 * direct access to the value 2560 */ 2561 public DateTimeType getLastPerformedElement() { 2562 if (this.lastPerformed == null) 2563 if (Configuration.errorOnAutoCreate()) 2564 throw new Error("Attempt to auto-create VerificationResult.lastPerformed"); 2565 else if (Configuration.doAutoCreate()) 2566 this.lastPerformed = new DateTimeType(); // bb 2567 return this.lastPerformed; 2568 } 2569 2570 public boolean hasLastPerformedElement() { 2571 return this.lastPerformed != null && !this.lastPerformed.isEmpty(); 2572 } 2573 2574 public boolean hasLastPerformed() { 2575 return this.lastPerformed != null && !this.lastPerformed.isEmpty(); 2576 } 2577 2578 /** 2579 * @param value {@link #lastPerformed} (The date/time validation was last 2580 * completed (including failed validations).). This is the 2581 * underlying object with id, value and extensions. The accessor 2582 * "getLastPerformed" gives direct access to the value 2583 */ 2584 public VerificationResult setLastPerformedElement(DateTimeType value) { 2585 this.lastPerformed = value; 2586 return this; 2587 } 2588 2589 /** 2590 * @return The date/time validation was last completed (including failed 2591 * validations). 2592 */ 2593 public Date getLastPerformed() { 2594 return this.lastPerformed == null ? null : this.lastPerformed.getValue(); 2595 } 2596 2597 /** 2598 * @param value The date/time validation was last completed (including failed 2599 * validations). 2600 */ 2601 public VerificationResult setLastPerformed(Date value) { 2602 if (value == null) 2603 this.lastPerformed = null; 2604 else { 2605 if (this.lastPerformed == null) 2606 this.lastPerformed = new DateTimeType(); 2607 this.lastPerformed.setValue(value); 2608 } 2609 return this; 2610 } 2611 2612 /** 2613 * @return {@link #nextScheduled} (The date when target is next validated, if 2614 * appropriate.). This is the underlying object with id, value and 2615 * extensions. The accessor "getNextScheduled" gives direct access to 2616 * the value 2617 */ 2618 public DateType getNextScheduledElement() { 2619 if (this.nextScheduled == null) 2620 if (Configuration.errorOnAutoCreate()) 2621 throw new Error("Attempt to auto-create VerificationResult.nextScheduled"); 2622 else if (Configuration.doAutoCreate()) 2623 this.nextScheduled = new DateType(); // bb 2624 return this.nextScheduled; 2625 } 2626 2627 public boolean hasNextScheduledElement() { 2628 return this.nextScheduled != null && !this.nextScheduled.isEmpty(); 2629 } 2630 2631 public boolean hasNextScheduled() { 2632 return this.nextScheduled != null && !this.nextScheduled.isEmpty(); 2633 } 2634 2635 /** 2636 * @param value {@link #nextScheduled} (The date when target is next validated, 2637 * if appropriate.). This is the underlying object with id, value 2638 * and extensions. The accessor "getNextScheduled" gives direct 2639 * access to the value 2640 */ 2641 public VerificationResult setNextScheduledElement(DateType value) { 2642 this.nextScheduled = value; 2643 return this; 2644 } 2645 2646 /** 2647 * @return The date when target is next validated, if appropriate. 2648 */ 2649 public Date getNextScheduled() { 2650 return this.nextScheduled == null ? null : this.nextScheduled.getValue(); 2651 } 2652 2653 /** 2654 * @param value The date when target is next validated, if appropriate. 2655 */ 2656 public VerificationResult setNextScheduled(Date value) { 2657 if (value == null) 2658 this.nextScheduled = null; 2659 else { 2660 if (this.nextScheduled == null) 2661 this.nextScheduled = new DateType(); 2662 this.nextScheduled.setValue(value); 2663 } 2664 return this; 2665 } 2666 2667 /** 2668 * @return {@link #failureAction} (The result if validation fails (fatal; 2669 * warning; record only; none).) 2670 */ 2671 public CodeableConcept getFailureAction() { 2672 if (this.failureAction == null) 2673 if (Configuration.errorOnAutoCreate()) 2674 throw new Error("Attempt to auto-create VerificationResult.failureAction"); 2675 else if (Configuration.doAutoCreate()) 2676 this.failureAction = new CodeableConcept(); // cc 2677 return this.failureAction; 2678 } 2679 2680 public boolean hasFailureAction() { 2681 return this.failureAction != null && !this.failureAction.isEmpty(); 2682 } 2683 2684 /** 2685 * @param value {@link #failureAction} (The result if validation fails (fatal; 2686 * warning; record only; none).) 2687 */ 2688 public VerificationResult setFailureAction(CodeableConcept value) { 2689 this.failureAction = value; 2690 return this; 2691 } 2692 2693 /** 2694 * @return {@link #primarySource} (Information about the primary source(s) 2695 * involved in validation.) 2696 */ 2697 public List<VerificationResultPrimarySourceComponent> getPrimarySource() { 2698 if (this.primarySource == null) 2699 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2700 return this.primarySource; 2701 } 2702 2703 /** 2704 * @return Returns a reference to <code>this</code> for easy method chaining 2705 */ 2706 public VerificationResult setPrimarySource(List<VerificationResultPrimarySourceComponent> thePrimarySource) { 2707 this.primarySource = thePrimarySource; 2708 return this; 2709 } 2710 2711 public boolean hasPrimarySource() { 2712 if (this.primarySource == null) 2713 return false; 2714 for (VerificationResultPrimarySourceComponent item : this.primarySource) 2715 if (!item.isEmpty()) 2716 return true; 2717 return false; 2718 } 2719 2720 public VerificationResultPrimarySourceComponent addPrimarySource() { // 3 2721 VerificationResultPrimarySourceComponent t = new VerificationResultPrimarySourceComponent(); 2722 if (this.primarySource == null) 2723 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2724 this.primarySource.add(t); 2725 return t; 2726 } 2727 2728 public VerificationResult addPrimarySource(VerificationResultPrimarySourceComponent t) { // 3 2729 if (t == null) 2730 return this; 2731 if (this.primarySource == null) 2732 this.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 2733 this.primarySource.add(t); 2734 return this; 2735 } 2736 2737 /** 2738 * @return The first repetition of repeating field {@link #primarySource}, 2739 * creating it if it does not already exist 2740 */ 2741 public VerificationResultPrimarySourceComponent getPrimarySourceFirstRep() { 2742 if (getPrimarySource().isEmpty()) { 2743 addPrimarySource(); 2744 } 2745 return getPrimarySource().get(0); 2746 } 2747 2748 /** 2749 * @return {@link #attestation} (Information about the entity attesting to 2750 * information.) 2751 */ 2752 public VerificationResultAttestationComponent getAttestation() { 2753 if (this.attestation == null) 2754 if (Configuration.errorOnAutoCreate()) 2755 throw new Error("Attempt to auto-create VerificationResult.attestation"); 2756 else if (Configuration.doAutoCreate()) 2757 this.attestation = new VerificationResultAttestationComponent(); // cc 2758 return this.attestation; 2759 } 2760 2761 public boolean hasAttestation() { 2762 return this.attestation != null && !this.attestation.isEmpty(); 2763 } 2764 2765 /** 2766 * @param value {@link #attestation} (Information about the entity attesting to 2767 * information.) 2768 */ 2769 public VerificationResult setAttestation(VerificationResultAttestationComponent value) { 2770 this.attestation = value; 2771 return this; 2772 } 2773 2774 /** 2775 * @return {@link #validator} (Information about the entity validating 2776 * information.) 2777 */ 2778 public List<VerificationResultValidatorComponent> getValidator() { 2779 if (this.validator == null) 2780 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2781 return this.validator; 2782 } 2783 2784 /** 2785 * @return Returns a reference to <code>this</code> for easy method chaining 2786 */ 2787 public VerificationResult setValidator(List<VerificationResultValidatorComponent> theValidator) { 2788 this.validator = theValidator; 2789 return this; 2790 } 2791 2792 public boolean hasValidator() { 2793 if (this.validator == null) 2794 return false; 2795 for (VerificationResultValidatorComponent item : this.validator) 2796 if (!item.isEmpty()) 2797 return true; 2798 return false; 2799 } 2800 2801 public VerificationResultValidatorComponent addValidator() { // 3 2802 VerificationResultValidatorComponent t = new VerificationResultValidatorComponent(); 2803 if (this.validator == null) 2804 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2805 this.validator.add(t); 2806 return t; 2807 } 2808 2809 public VerificationResult addValidator(VerificationResultValidatorComponent t) { // 3 2810 if (t == null) 2811 return this; 2812 if (this.validator == null) 2813 this.validator = new ArrayList<VerificationResultValidatorComponent>(); 2814 this.validator.add(t); 2815 return this; 2816 } 2817 2818 /** 2819 * @return The first repetition of repeating field {@link #validator}, creating 2820 * it if it does not already exist 2821 */ 2822 public VerificationResultValidatorComponent getValidatorFirstRep() { 2823 if (getValidator().isEmpty()) { 2824 addValidator(); 2825 } 2826 return getValidator().get(0); 2827 } 2828 2829 protected void listChildren(List<Property> children) { 2830 super.listChildren(children); 2831 children.add(new Property("target", "Reference(Any)", "A resource that was validated.", 0, 2832 java.lang.Integer.MAX_VALUE, target)); 2833 children.add( 2834 new Property("targetLocation", "string", "The fhirpath location(s) within the resource that was validated.", 0, 2835 java.lang.Integer.MAX_VALUE, targetLocation)); 2836 children.add(new Property("need", "CodeableConcept", 2837 "The frequency with which the target must be validated (none; initial; periodic).", 0, 1, need)); 2838 children.add(new Property("status", "code", 2839 "The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).", 2840 0, 1, status)); 2841 children.add(new Property("statusDate", "dateTime", "When the validation status was updated.", 0, 1, statusDate)); 2842 children.add(new Property("validationType", "CodeableConcept", 2843 "What the target is validated against (nothing; primary source; multiple sources).", 0, 1, validationType)); 2844 children.add(new Property("validationProcess", "CodeableConcept", 2845 "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).", 2846 0, java.lang.Integer.MAX_VALUE, validationProcess)); 2847 children.add(new Property("frequency", "Timing", "Frequency of revalidation.", 0, 1, frequency)); 2848 children.add(new Property("lastPerformed", "dateTime", 2849 "The date/time validation was last completed (including failed validations).", 0, 1, lastPerformed)); 2850 children.add(new Property("nextScheduled", "date", "The date when target is next validated, if appropriate.", 0, 1, 2851 nextScheduled)); 2852 children.add(new Property("failureAction", "CodeableConcept", 2853 "The result if validation fails (fatal; warning; record only; none).", 0, 1, failureAction)); 2854 children.add(new Property("primarySource", "", "Information about the primary source(s) involved in validation.", 0, 2855 java.lang.Integer.MAX_VALUE, primarySource)); 2856 children.add( 2857 new Property("attestation", "", "Information about the entity attesting to information.", 0, 1, attestation)); 2858 children.add(new Property("validator", "", "Information about the entity validating information.", 0, 2859 java.lang.Integer.MAX_VALUE, validator)); 2860 } 2861 2862 @Override 2863 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2864 switch (_hash) { 2865 case -880905839: 2866 /* target */ return new Property("target", "Reference(Any)", "A resource that was validated.", 0, 2867 java.lang.Integer.MAX_VALUE, target); 2868 case 308958310: 2869 /* targetLocation */ return new Property("targetLocation", "string", 2870 "The fhirpath location(s) within the resource that was validated.", 0, java.lang.Integer.MAX_VALUE, 2871 targetLocation); 2872 case 3377302: 2873 /* need */ return new Property("need", "CodeableConcept", 2874 "The frequency with which the target must be validated (none; initial; periodic).", 0, 1, need); 2875 case -892481550: 2876 /* status */ return new Property("status", "code", 2877 "The validation status of the target (attested; validated; in process; requires revalidation; validation failed; revalidation failed).", 2878 0, 1, status); 2879 case 247524032: 2880 /* statusDate */ return new Property("statusDate", "dateTime", "When the validation status was updated.", 0, 1, 2881 statusDate); 2882 case -279681197: 2883 /* validationType */ return new Property("validationType", "CodeableConcept", 2884 "What the target is validated against (nothing; primary source; multiple sources).", 0, 1, validationType); 2885 case 797680566: 2886 /* validationProcess */ return new Property("validationProcess", "CodeableConcept", 2887 "The primary process by which the target is validated (edit check; value set; primary source; multiple sources; standalone; in context).", 2888 0, java.lang.Integer.MAX_VALUE, validationProcess); 2889 case -70023844: 2890 /* frequency */ return new Property("frequency", "Timing", "Frequency of revalidation.", 0, 1, frequency); 2891 case -1313229366: 2892 /* lastPerformed */ return new Property("lastPerformed", "dateTime", 2893 "The date/time validation was last completed (including failed validations).", 0, 1, lastPerformed); 2894 case 1874589434: 2895 /* nextScheduled */ return new Property("nextScheduled", "date", 2896 "The date when target is next validated, if appropriate.", 0, 1, nextScheduled); 2897 case 1816382560: 2898 /* failureAction */ return new Property("failureAction", "CodeableConcept", 2899 "The result if validation fails (fatal; warning; record only; none).", 0, 1, failureAction); 2900 case -528721731: 2901 /* primarySource */ return new Property("primarySource", "", 2902 "Information about the primary source(s) involved in validation.", 0, java.lang.Integer.MAX_VALUE, 2903 primarySource); 2904 case -709624112: 2905 /* attestation */ return new Property("attestation", "", "Information about the entity attesting to information.", 2906 0, 1, attestation); 2907 case -1109783726: 2908 /* validator */ return new Property("validator", "", "Information about the entity validating information.", 0, 2909 java.lang.Integer.MAX_VALUE, validator); 2910 default: 2911 return super.getNamedProperty(_hash, _name, _checkValid); 2912 } 2913 2914 } 2915 2916 @Override 2917 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2918 switch (hash) { 2919 case -880905839: 2920 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // Reference 2921 case 308958310: 2922 /* targetLocation */ return this.targetLocation == null ? new Base[0] 2923 : this.targetLocation.toArray(new Base[this.targetLocation.size()]); // StringType 2924 case 3377302: 2925 /* need */ return this.need == null ? new Base[0] : new Base[] { this.need }; // CodeableConcept 2926 case -892481550: 2927 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<Status> 2928 case 247524032: 2929 /* statusDate */ return this.statusDate == null ? new Base[0] : new Base[] { this.statusDate }; // DateTimeType 2930 case -279681197: 2931 /* validationType */ return this.validationType == null ? new Base[0] : new Base[] { this.validationType }; // CodeableConcept 2932 case 797680566: 2933 /* validationProcess */ return this.validationProcess == null ? new Base[0] 2934 : this.validationProcess.toArray(new Base[this.validationProcess.size()]); // CodeableConcept 2935 case -70023844: 2936 /* frequency */ return this.frequency == null ? new Base[0] : new Base[] { this.frequency }; // Timing 2937 case -1313229366: 2938 /* lastPerformed */ return this.lastPerformed == null ? new Base[0] : new Base[] { this.lastPerformed }; // DateTimeType 2939 case 1874589434: 2940 /* nextScheduled */ return this.nextScheduled == null ? new Base[0] : new Base[] { this.nextScheduled }; // DateType 2941 case 1816382560: 2942 /* failureAction */ return this.failureAction == null ? new Base[0] : new Base[] { this.failureAction }; // CodeableConcept 2943 case -528721731: 2944 /* primarySource */ return this.primarySource == null ? new Base[0] 2945 : this.primarySource.toArray(new Base[this.primarySource.size()]); // VerificationResultPrimarySourceComponent 2946 case -709624112: 2947 /* attestation */ return this.attestation == null ? new Base[0] : new Base[] { this.attestation }; // VerificationResultAttestationComponent 2948 case -1109783726: 2949 /* validator */ return this.validator == null ? new Base[0] 2950 : this.validator.toArray(new Base[this.validator.size()]); // VerificationResultValidatorComponent 2951 default: 2952 return super.getProperty(hash, name, checkValid); 2953 } 2954 2955 } 2956 2957 @Override 2958 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2959 switch (hash) { 2960 case -880905839: // target 2961 this.getTarget().add(castToReference(value)); // Reference 2962 return value; 2963 case 308958310: // targetLocation 2964 this.getTargetLocation().add(castToString(value)); // StringType 2965 return value; 2966 case 3377302: // need 2967 this.need = castToCodeableConcept(value); // CodeableConcept 2968 return value; 2969 case -892481550: // status 2970 value = new StatusEnumFactory().fromType(castToCode(value)); 2971 this.status = (Enumeration) value; // Enumeration<Status> 2972 return value; 2973 case 247524032: // statusDate 2974 this.statusDate = castToDateTime(value); // DateTimeType 2975 return value; 2976 case -279681197: // validationType 2977 this.validationType = castToCodeableConcept(value); // CodeableConcept 2978 return value; 2979 case 797680566: // validationProcess 2980 this.getValidationProcess().add(castToCodeableConcept(value)); // CodeableConcept 2981 return value; 2982 case -70023844: // frequency 2983 this.frequency = castToTiming(value); // Timing 2984 return value; 2985 case -1313229366: // lastPerformed 2986 this.lastPerformed = castToDateTime(value); // DateTimeType 2987 return value; 2988 case 1874589434: // nextScheduled 2989 this.nextScheduled = castToDate(value); // DateType 2990 return value; 2991 case 1816382560: // failureAction 2992 this.failureAction = castToCodeableConcept(value); // CodeableConcept 2993 return value; 2994 case -528721731: // primarySource 2995 this.getPrimarySource().add((VerificationResultPrimarySourceComponent) value); // VerificationResultPrimarySourceComponent 2996 return value; 2997 case -709624112: // attestation 2998 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 2999 return value; 3000 case -1109783726: // validator 3001 this.getValidator().add((VerificationResultValidatorComponent) value); // VerificationResultValidatorComponent 3002 return value; 3003 default: 3004 return super.setProperty(hash, name, value); 3005 } 3006 3007 } 3008 3009 @Override 3010 public Base setProperty(String name, Base value) throws FHIRException { 3011 if (name.equals("target")) { 3012 this.getTarget().add(castToReference(value)); 3013 } else if (name.equals("targetLocation")) { 3014 this.getTargetLocation().add(castToString(value)); 3015 } else if (name.equals("need")) { 3016 this.need = castToCodeableConcept(value); // CodeableConcept 3017 } else if (name.equals("status")) { 3018 value = new StatusEnumFactory().fromType(castToCode(value)); 3019 this.status = (Enumeration) value; // Enumeration<Status> 3020 } else if (name.equals("statusDate")) { 3021 this.statusDate = castToDateTime(value); // DateTimeType 3022 } else if (name.equals("validationType")) { 3023 this.validationType = castToCodeableConcept(value); // CodeableConcept 3024 } else if (name.equals("validationProcess")) { 3025 this.getValidationProcess().add(castToCodeableConcept(value)); 3026 } else if (name.equals("frequency")) { 3027 this.frequency = castToTiming(value); // Timing 3028 } else if (name.equals("lastPerformed")) { 3029 this.lastPerformed = castToDateTime(value); // DateTimeType 3030 } else if (name.equals("nextScheduled")) { 3031 this.nextScheduled = castToDate(value); // DateType 3032 } else if (name.equals("failureAction")) { 3033 this.failureAction = castToCodeableConcept(value); // CodeableConcept 3034 } else if (name.equals("primarySource")) { 3035 this.getPrimarySource().add((VerificationResultPrimarySourceComponent) value); 3036 } else if (name.equals("attestation")) { 3037 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 3038 } else if (name.equals("validator")) { 3039 this.getValidator().add((VerificationResultValidatorComponent) value); 3040 } else 3041 return super.setProperty(name, value); 3042 return value; 3043 } 3044 3045 @Override 3046 public void removeChild(String name, Base value) throws FHIRException { 3047 if (name.equals("target")) { 3048 this.getTarget().remove(castToReference(value)); 3049 } else if (name.equals("targetLocation")) { 3050 this.getTargetLocation().remove(castToString(value)); 3051 } else if (name.equals("need")) { 3052 this.need = null; 3053 } else if (name.equals("status")) { 3054 this.status = null; 3055 } else if (name.equals("statusDate")) { 3056 this.statusDate = null; 3057 } else if (name.equals("validationType")) { 3058 this.validationType = null; 3059 } else if (name.equals("validationProcess")) { 3060 this.getValidationProcess().remove(castToCodeableConcept(value)); 3061 } else if (name.equals("frequency")) { 3062 this.frequency = null; 3063 } else if (name.equals("lastPerformed")) { 3064 this.lastPerformed = null; 3065 } else if (name.equals("nextScheduled")) { 3066 this.nextScheduled = null; 3067 } else if (name.equals("failureAction")) { 3068 this.failureAction = null; 3069 } else if (name.equals("primarySource")) { 3070 this.getPrimarySource().remove((VerificationResultPrimarySourceComponent) value); 3071 } else if (name.equals("attestation")) { 3072 this.attestation = (VerificationResultAttestationComponent) value; // VerificationResultAttestationComponent 3073 } else if (name.equals("validator")) { 3074 this.getValidator().remove((VerificationResultValidatorComponent) value); 3075 } else 3076 super.removeChild(name, value); 3077 3078 } 3079 3080 @Override 3081 public Base makeProperty(int hash, String name) throws FHIRException { 3082 switch (hash) { 3083 case -880905839: 3084 return addTarget(); 3085 case 308958310: 3086 return addTargetLocationElement(); 3087 case 3377302: 3088 return getNeed(); 3089 case -892481550: 3090 return getStatusElement(); 3091 case 247524032: 3092 return getStatusDateElement(); 3093 case -279681197: 3094 return getValidationType(); 3095 case 797680566: 3096 return addValidationProcess(); 3097 case -70023844: 3098 return getFrequency(); 3099 case -1313229366: 3100 return getLastPerformedElement(); 3101 case 1874589434: 3102 return getNextScheduledElement(); 3103 case 1816382560: 3104 return getFailureAction(); 3105 case -528721731: 3106 return addPrimarySource(); 3107 case -709624112: 3108 return getAttestation(); 3109 case -1109783726: 3110 return addValidator(); 3111 default: 3112 return super.makeProperty(hash, name); 3113 } 3114 3115 } 3116 3117 @Override 3118 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3119 switch (hash) { 3120 case -880905839: 3121 /* target */ return new String[] { "Reference" }; 3122 case 308958310: 3123 /* targetLocation */ return new String[] { "string" }; 3124 case 3377302: 3125 /* need */ return new String[] { "CodeableConcept" }; 3126 case -892481550: 3127 /* status */ return new String[] { "code" }; 3128 case 247524032: 3129 /* statusDate */ return new String[] { "dateTime" }; 3130 case -279681197: 3131 /* validationType */ return new String[] { "CodeableConcept" }; 3132 case 797680566: 3133 /* validationProcess */ return new String[] { "CodeableConcept" }; 3134 case -70023844: 3135 /* frequency */ return new String[] { "Timing" }; 3136 case -1313229366: 3137 /* lastPerformed */ return new String[] { "dateTime" }; 3138 case 1874589434: 3139 /* nextScheduled */ return new String[] { "date" }; 3140 case 1816382560: 3141 /* failureAction */ return new String[] { "CodeableConcept" }; 3142 case -528721731: 3143 /* primarySource */ return new String[] {}; 3144 case -709624112: 3145 /* attestation */ return new String[] {}; 3146 case -1109783726: 3147 /* validator */ return new String[] {}; 3148 default: 3149 return super.getTypesForProperty(hash, name); 3150 } 3151 3152 } 3153 3154 @Override 3155 public Base addChild(String name) throws FHIRException { 3156 if (name.equals("target")) { 3157 return addTarget(); 3158 } else if (name.equals("targetLocation")) { 3159 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.targetLocation"); 3160 } else if (name.equals("need")) { 3161 this.need = new CodeableConcept(); 3162 return this.need; 3163 } else if (name.equals("status")) { 3164 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.status"); 3165 } else if (name.equals("statusDate")) { 3166 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.statusDate"); 3167 } else if (name.equals("validationType")) { 3168 this.validationType = new CodeableConcept(); 3169 return this.validationType; 3170 } else if (name.equals("validationProcess")) { 3171 return addValidationProcess(); 3172 } else if (name.equals("frequency")) { 3173 this.frequency = new Timing(); 3174 return this.frequency; 3175 } else if (name.equals("lastPerformed")) { 3176 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.lastPerformed"); 3177 } else if (name.equals("nextScheduled")) { 3178 throw new FHIRException("Cannot call addChild on a singleton property VerificationResult.nextScheduled"); 3179 } else if (name.equals("failureAction")) { 3180 this.failureAction = new CodeableConcept(); 3181 return this.failureAction; 3182 } else if (name.equals("primarySource")) { 3183 return addPrimarySource(); 3184 } else if (name.equals("attestation")) { 3185 this.attestation = new VerificationResultAttestationComponent(); 3186 return this.attestation; 3187 } else if (name.equals("validator")) { 3188 return addValidator(); 3189 } else 3190 return super.addChild(name); 3191 } 3192 3193 public String fhirType() { 3194 return "VerificationResult"; 3195 3196 } 3197 3198 public VerificationResult copy() { 3199 VerificationResult dst = new VerificationResult(); 3200 copyValues(dst); 3201 return dst; 3202 } 3203 3204 public void copyValues(VerificationResult dst) { 3205 super.copyValues(dst); 3206 if (target != null) { 3207 dst.target = new ArrayList<Reference>(); 3208 for (Reference i : target) 3209 dst.target.add(i.copy()); 3210 } 3211 ; 3212 if (targetLocation != null) { 3213 dst.targetLocation = new ArrayList<StringType>(); 3214 for (StringType i : targetLocation) 3215 dst.targetLocation.add(i.copy()); 3216 } 3217 ; 3218 dst.need = need == null ? null : need.copy(); 3219 dst.status = status == null ? null : status.copy(); 3220 dst.statusDate = statusDate == null ? null : statusDate.copy(); 3221 dst.validationType = validationType == null ? null : validationType.copy(); 3222 if (validationProcess != null) { 3223 dst.validationProcess = new ArrayList<CodeableConcept>(); 3224 for (CodeableConcept i : validationProcess) 3225 dst.validationProcess.add(i.copy()); 3226 } 3227 ; 3228 dst.frequency = frequency == null ? null : frequency.copy(); 3229 dst.lastPerformed = lastPerformed == null ? null : lastPerformed.copy(); 3230 dst.nextScheduled = nextScheduled == null ? null : nextScheduled.copy(); 3231 dst.failureAction = failureAction == null ? null : failureAction.copy(); 3232 if (primarySource != null) { 3233 dst.primarySource = new ArrayList<VerificationResultPrimarySourceComponent>(); 3234 for (VerificationResultPrimarySourceComponent i : primarySource) 3235 dst.primarySource.add(i.copy()); 3236 } 3237 ; 3238 dst.attestation = attestation == null ? null : attestation.copy(); 3239 if (validator != null) { 3240 dst.validator = new ArrayList<VerificationResultValidatorComponent>(); 3241 for (VerificationResultValidatorComponent i : validator) 3242 dst.validator.add(i.copy()); 3243 } 3244 ; 3245 } 3246 3247 protected VerificationResult typedCopy() { 3248 return copy(); 3249 } 3250 3251 @Override 3252 public boolean equalsDeep(Base other_) { 3253 if (!super.equalsDeep(other_)) 3254 return false; 3255 if (!(other_ instanceof VerificationResult)) 3256 return false; 3257 VerificationResult o = (VerificationResult) other_; 3258 return compareDeep(target, o.target, true) && compareDeep(targetLocation, o.targetLocation, true) 3259 && compareDeep(need, o.need, true) && compareDeep(status, o.status, true) 3260 && compareDeep(statusDate, o.statusDate, true) && compareDeep(validationType, o.validationType, true) 3261 && compareDeep(validationProcess, o.validationProcess, true) && compareDeep(frequency, o.frequency, true) 3262 && compareDeep(lastPerformed, o.lastPerformed, true) && compareDeep(nextScheduled, o.nextScheduled, true) 3263 && compareDeep(failureAction, o.failureAction, true) && compareDeep(primarySource, o.primarySource, true) 3264 && compareDeep(attestation, o.attestation, true) && compareDeep(validator, o.validator, true); 3265 } 3266 3267 @Override 3268 public boolean equalsShallow(Base other_) { 3269 if (!super.equalsShallow(other_)) 3270 return false; 3271 if (!(other_ instanceof VerificationResult)) 3272 return false; 3273 VerificationResult o = (VerificationResult) other_; 3274 return compareValues(targetLocation, o.targetLocation, true) && compareValues(status, o.status, true) 3275 && compareValues(statusDate, o.statusDate, true) && compareValues(lastPerformed, o.lastPerformed, true) 3276 && compareValues(nextScheduled, o.nextScheduled, true); 3277 } 3278 3279 public boolean isEmpty() { 3280 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, targetLocation, need, status, statusDate, 3281 validationType, validationProcess, frequency, lastPerformed, nextScheduled, failureAction, primarySource, 3282 attestation, validator); 3283 } 3284 3285 @Override 3286 public ResourceType getResourceType() { 3287 return ResourceType.VerificationResult; 3288 } 3289 3290 /** 3291 * Search parameter: <b>target</b> 3292 * <p> 3293 * Description: <b>A resource that was validated</b><br> 3294 * Type: <b>reference</b><br> 3295 * Path: <b>VerificationResult.target</b><br> 3296 * </p> 3297 */ 3298 @SearchParamDefinition(name = "target", path = "VerificationResult.target", description = "A resource that was validated", type = "reference") 3299 public static final String SP_TARGET = "target"; 3300 /** 3301 * <b>Fluent Client</b> search parameter constant for <b>target</b> 3302 * <p> 3303 * Description: <b>A resource that was validated</b><br> 3304 * Type: <b>reference</b><br> 3305 * Path: <b>VerificationResult.target</b><br> 3306 * </p> 3307 */ 3308 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3309 SP_TARGET); 3310 3311 /** 3312 * Constant for fluent queries to be used to add include statements. Specifies 3313 * the path value of "<b>VerificationResult:target</b>". 3314 */ 3315 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include( 3316 "VerificationResult:target").toLocked(); 3317 3318}