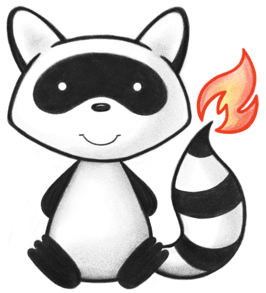
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * An authorization for the provision of glasses and/or contact lenses to a 051 * patient. 052 */ 053@ResourceDef(name = "VisionPrescription", profile = "http://hl7.org/fhir/StructureDefinition/VisionPrescription") 054public class VisionPrescription extends DomainResource { 055 056 public enum VisionStatus { 057 /** 058 * The instance is currently in-force. 059 */ 060 ACTIVE, 061 /** 062 * The instance is withdrawn, rescinded or reversed. 063 */ 064 CANCELLED, 065 /** 066 * A new instance the contents of which is not complete. 067 */ 068 DRAFT, 069 /** 070 * The instance was entered in error. 071 */ 072 ENTEREDINERROR, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 078 public static VisionStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("active".equals(codeString)) 082 return ACTIVE; 083 if ("cancelled".equals(codeString)) 084 return CANCELLED; 085 if ("draft".equals(codeString)) 086 return DRAFT; 087 if ("entered-in-error".equals(codeString)) 088 return ENTEREDINERROR; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown VisionStatus code '" + codeString + "'"); 093 } 094 095 public String toCode() { 096 switch (this) { 097 case ACTIVE: 098 return "active"; 099 case CANCELLED: 100 return "cancelled"; 101 case DRAFT: 102 return "draft"; 103 case ENTEREDINERROR: 104 return "entered-in-error"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getSystem() { 113 switch (this) { 114 case ACTIVE: 115 return "http://hl7.org/fhir/fm-status"; 116 case CANCELLED: 117 return "http://hl7.org/fhir/fm-status"; 118 case DRAFT: 119 return "http://hl7.org/fhir/fm-status"; 120 case ENTEREDINERROR: 121 return "http://hl7.org/fhir/fm-status"; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getDefinition() { 130 switch (this) { 131 case ACTIVE: 132 return "The instance is currently in-force."; 133 case CANCELLED: 134 return "The instance is withdrawn, rescinded or reversed."; 135 case DRAFT: 136 return "A new instance the contents of which is not complete."; 137 case ENTEREDINERROR: 138 return "The instance was entered in error."; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDisplay() { 147 switch (this) { 148 case ACTIVE: 149 return "Active"; 150 case CANCELLED: 151 return "Cancelled"; 152 case DRAFT: 153 return "Draft"; 154 case ENTEREDINERROR: 155 return "Entered in Error"; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 } 163 164 public static class VisionStatusEnumFactory implements EnumFactory<VisionStatus> { 165 public VisionStatus fromCode(String codeString) throws IllegalArgumentException { 166 if (codeString == null || "".equals(codeString)) 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("active".equals(codeString)) 170 return VisionStatus.ACTIVE; 171 if ("cancelled".equals(codeString)) 172 return VisionStatus.CANCELLED; 173 if ("draft".equals(codeString)) 174 return VisionStatus.DRAFT; 175 if ("entered-in-error".equals(codeString)) 176 return VisionStatus.ENTEREDINERROR; 177 throw new IllegalArgumentException("Unknown VisionStatus code '" + codeString + "'"); 178 } 179 180 public Enumeration<VisionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 181 if (code == null) 182 return null; 183 if (code.isEmpty()) 184 return new Enumeration<VisionStatus>(this, VisionStatus.NULL, code); 185 String codeString = code.asStringValue(); 186 if (codeString == null || "".equals(codeString)) 187 return new Enumeration<VisionStatus>(this, VisionStatus.NULL, code); 188 if ("active".equals(codeString)) 189 return new Enumeration<VisionStatus>(this, VisionStatus.ACTIVE, code); 190 if ("cancelled".equals(codeString)) 191 return new Enumeration<VisionStatus>(this, VisionStatus.CANCELLED, code); 192 if ("draft".equals(codeString)) 193 return new Enumeration<VisionStatus>(this, VisionStatus.DRAFT, code); 194 if ("entered-in-error".equals(codeString)) 195 return new Enumeration<VisionStatus>(this, VisionStatus.ENTEREDINERROR, code); 196 throw new FHIRException("Unknown VisionStatus code '" + codeString + "'"); 197 } 198 199 public String toCode(VisionStatus code) { 200 if (code == VisionStatus.NULL) 201 return null; 202 if (code == VisionStatus.ACTIVE) 203 return "active"; 204 if (code == VisionStatus.CANCELLED) 205 return "cancelled"; 206 if (code == VisionStatus.DRAFT) 207 return "draft"; 208 if (code == VisionStatus.ENTEREDINERROR) 209 return "entered-in-error"; 210 return "?"; 211 } 212 213 public String toSystem(VisionStatus code) { 214 return code.getSystem(); 215 } 216 } 217 218 public enum VisionEyes { 219 /** 220 * Right Eye. 221 */ 222 RIGHT, 223 /** 224 * Left Eye. 225 */ 226 LEFT, 227 /** 228 * added to help the parsers with the generic types 229 */ 230 NULL; 231 232 public static VisionEyes fromCode(String codeString) throws FHIRException { 233 if (codeString == null || "".equals(codeString)) 234 return null; 235 if ("right".equals(codeString)) 236 return RIGHT; 237 if ("left".equals(codeString)) 238 return LEFT; 239 if (Configuration.isAcceptInvalidEnums()) 240 return null; 241 else 242 throw new FHIRException("Unknown VisionEyes code '" + codeString + "'"); 243 } 244 245 public String toCode() { 246 switch (this) { 247 case RIGHT: 248 return "right"; 249 case LEFT: 250 return "left"; 251 case NULL: 252 return null; 253 default: 254 return "?"; 255 } 256 } 257 258 public String getSystem() { 259 switch (this) { 260 case RIGHT: 261 return "http://hl7.org/fhir/vision-eye-codes"; 262 case LEFT: 263 return "http://hl7.org/fhir/vision-eye-codes"; 264 case NULL: 265 return null; 266 default: 267 return "?"; 268 } 269 } 270 271 public String getDefinition() { 272 switch (this) { 273 case RIGHT: 274 return "Right Eye."; 275 case LEFT: 276 return "Left Eye."; 277 case NULL: 278 return null; 279 default: 280 return "?"; 281 } 282 } 283 284 public String getDisplay() { 285 switch (this) { 286 case RIGHT: 287 return "Right Eye"; 288 case LEFT: 289 return "Left Eye"; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 } 297 298 public static class VisionEyesEnumFactory implements EnumFactory<VisionEyes> { 299 public VisionEyes fromCode(String codeString) throws IllegalArgumentException { 300 if (codeString == null || "".equals(codeString)) 301 if (codeString == null || "".equals(codeString)) 302 return null; 303 if ("right".equals(codeString)) 304 return VisionEyes.RIGHT; 305 if ("left".equals(codeString)) 306 return VisionEyes.LEFT; 307 throw new IllegalArgumentException("Unknown VisionEyes code '" + codeString + "'"); 308 } 309 310 public Enumeration<VisionEyes> fromType(PrimitiveType<?> code) throws FHIRException { 311 if (code == null) 312 return null; 313 if (code.isEmpty()) 314 return new Enumeration<VisionEyes>(this, VisionEyes.NULL, code); 315 String codeString = code.asStringValue(); 316 if (codeString == null || "".equals(codeString)) 317 return new Enumeration<VisionEyes>(this, VisionEyes.NULL, code); 318 if ("right".equals(codeString)) 319 return new Enumeration<VisionEyes>(this, VisionEyes.RIGHT, code); 320 if ("left".equals(codeString)) 321 return new Enumeration<VisionEyes>(this, VisionEyes.LEFT, code); 322 throw new FHIRException("Unknown VisionEyes code '" + codeString + "'"); 323 } 324 325 public String toCode(VisionEyes code) { 326 if (code == VisionEyes.NULL) 327 return null; 328 if (code == VisionEyes.RIGHT) 329 return "right"; 330 if (code == VisionEyes.LEFT) 331 return "left"; 332 return "?"; 333 } 334 335 public String toSystem(VisionEyes code) { 336 return code.getSystem(); 337 } 338 } 339 340 public enum VisionBase { 341 /** 342 * top. 343 */ 344 UP, 345 /** 346 * bottom. 347 */ 348 DOWN, 349 /** 350 * inner edge. 351 */ 352 IN, 353 /** 354 * outer edge. 355 */ 356 OUT, 357 /** 358 * added to help the parsers with the generic types 359 */ 360 NULL; 361 362 public static VisionBase fromCode(String codeString) throws FHIRException { 363 if (codeString == null || "".equals(codeString)) 364 return null; 365 if ("up".equals(codeString)) 366 return UP; 367 if ("down".equals(codeString)) 368 return DOWN; 369 if ("in".equals(codeString)) 370 return IN; 371 if ("out".equals(codeString)) 372 return OUT; 373 if (Configuration.isAcceptInvalidEnums()) 374 return null; 375 else 376 throw new FHIRException("Unknown VisionBase code '" + codeString + "'"); 377 } 378 379 public String toCode() { 380 switch (this) { 381 case UP: 382 return "up"; 383 case DOWN: 384 return "down"; 385 case IN: 386 return "in"; 387 case OUT: 388 return "out"; 389 case NULL: 390 return null; 391 default: 392 return "?"; 393 } 394 } 395 396 public String getSystem() { 397 switch (this) { 398 case UP: 399 return "http://hl7.org/fhir/vision-base-codes"; 400 case DOWN: 401 return "http://hl7.org/fhir/vision-base-codes"; 402 case IN: 403 return "http://hl7.org/fhir/vision-base-codes"; 404 case OUT: 405 return "http://hl7.org/fhir/vision-base-codes"; 406 case NULL: 407 return null; 408 default: 409 return "?"; 410 } 411 } 412 413 public String getDefinition() { 414 switch (this) { 415 case UP: 416 return "top."; 417 case DOWN: 418 return "bottom."; 419 case IN: 420 return "inner edge."; 421 case OUT: 422 return "outer edge."; 423 case NULL: 424 return null; 425 default: 426 return "?"; 427 } 428 } 429 430 public String getDisplay() { 431 switch (this) { 432 case UP: 433 return "Up"; 434 case DOWN: 435 return "Down"; 436 case IN: 437 return "In"; 438 case OUT: 439 return "Out"; 440 case NULL: 441 return null; 442 default: 443 return "?"; 444 } 445 } 446 } 447 448 public static class VisionBaseEnumFactory implements EnumFactory<VisionBase> { 449 public VisionBase fromCode(String codeString) throws IllegalArgumentException { 450 if (codeString == null || "".equals(codeString)) 451 if (codeString == null || "".equals(codeString)) 452 return null; 453 if ("up".equals(codeString)) 454 return VisionBase.UP; 455 if ("down".equals(codeString)) 456 return VisionBase.DOWN; 457 if ("in".equals(codeString)) 458 return VisionBase.IN; 459 if ("out".equals(codeString)) 460 return VisionBase.OUT; 461 throw new IllegalArgumentException("Unknown VisionBase code '" + codeString + "'"); 462 } 463 464 public Enumeration<VisionBase> fromType(PrimitiveType<?> code) throws FHIRException { 465 if (code == null) 466 return null; 467 if (code.isEmpty()) 468 return new Enumeration<VisionBase>(this, VisionBase.NULL, code); 469 String codeString = code.asStringValue(); 470 if (codeString == null || "".equals(codeString)) 471 return new Enumeration<VisionBase>(this, VisionBase.NULL, code); 472 if ("up".equals(codeString)) 473 return new Enumeration<VisionBase>(this, VisionBase.UP, code); 474 if ("down".equals(codeString)) 475 return new Enumeration<VisionBase>(this, VisionBase.DOWN, code); 476 if ("in".equals(codeString)) 477 return new Enumeration<VisionBase>(this, VisionBase.IN, code); 478 if ("out".equals(codeString)) 479 return new Enumeration<VisionBase>(this, VisionBase.OUT, code); 480 throw new FHIRException("Unknown VisionBase code '" + codeString + "'"); 481 } 482 483 public String toCode(VisionBase code) { 484 if (code == VisionBase.NULL) 485 return null; 486 if (code == VisionBase.UP) 487 return "up"; 488 if (code == VisionBase.DOWN) 489 return "down"; 490 if (code == VisionBase.IN) 491 return "in"; 492 if (code == VisionBase.OUT) 493 return "out"; 494 return "?"; 495 } 496 497 public String toSystem(VisionBase code) { 498 return code.getSystem(); 499 } 500 } 501 502 @Block() 503 public static class VisionPrescriptionLensSpecificationComponent extends BackboneElement 504 implements IBaseBackboneElement { 505 /** 506 * Identifies the type of vision correction product which is required for the 507 * patient. 508 */ 509 @Child(name = "product", type = { 510 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 511 @Description(shortDefinition = "Product to be supplied", formalDefinition = "Identifies the type of vision correction product which is required for the patient.") 512 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vision-product") 513 protected CodeableConcept product; 514 515 /** 516 * The eye for which the lens specification applies. 517 */ 518 @Child(name = "eye", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 519 @Description(shortDefinition = "right | left", formalDefinition = "The eye for which the lens specification applies.") 520 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vision-eye-codes") 521 protected Enumeration<VisionEyes> eye; 522 523 /** 524 * Lens power measured in dioptres (0.25 units). 525 */ 526 @Child(name = "sphere", type = { 527 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 528 @Description(shortDefinition = "Power of the lens", formalDefinition = "Lens power measured in dioptres (0.25 units).") 529 protected DecimalType sphere; 530 531 /** 532 * Power adjustment for astigmatism measured in dioptres (0.25 units). 533 */ 534 @Child(name = "cylinder", type = { 535 DecimalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 536 @Description(shortDefinition = "Lens power for astigmatism", formalDefinition = "Power adjustment for astigmatism measured in dioptres (0.25 units).") 537 protected DecimalType cylinder; 538 539 /** 540 * Adjustment for astigmatism measured in integer degrees. 541 */ 542 @Child(name = "axis", type = { IntegerType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 543 @Description(shortDefinition = "Lens meridian which contain no power for astigmatism", formalDefinition = "Adjustment for astigmatism measured in integer degrees.") 544 protected IntegerType axis; 545 546 /** 547 * Allows for adjustment on two axis. 548 */ 549 @Child(name = "prism", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 550 @Description(shortDefinition = "Eye alignment compensation", formalDefinition = "Allows for adjustment on two axis.") 551 protected List<PrismComponent> prism; 552 553 /** 554 * Power adjustment for multifocal lenses measured in dioptres (0.25 units). 555 */ 556 @Child(name = "add", type = { DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 557 @Description(shortDefinition = "Added power for multifocal levels", formalDefinition = "Power adjustment for multifocal lenses measured in dioptres (0.25 units).") 558 protected DecimalType add; 559 560 /** 561 * Contact lens power measured in dioptres (0.25 units). 562 */ 563 @Child(name = "power", type = { DecimalType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 564 @Description(shortDefinition = "Contact lens power", formalDefinition = "Contact lens power measured in dioptres (0.25 units).") 565 protected DecimalType power; 566 567 /** 568 * Back curvature measured in millimetres. 569 */ 570 @Child(name = "backCurve", type = { 571 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 572 @Description(shortDefinition = "Contact lens back curvature", formalDefinition = "Back curvature measured in millimetres.") 573 protected DecimalType backCurve; 574 575 /** 576 * Contact lens diameter measured in millimetres. 577 */ 578 @Child(name = "diameter", type = { 579 DecimalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 580 @Description(shortDefinition = "Contact lens diameter", formalDefinition = "Contact lens diameter measured in millimetres.") 581 protected DecimalType diameter; 582 583 /** 584 * The recommended maximum wear period for the lens. 585 */ 586 @Child(name = "duration", type = { 587 Quantity.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 588 @Description(shortDefinition = "Lens wear duration", formalDefinition = "The recommended maximum wear period for the lens.") 589 protected Quantity duration; 590 591 /** 592 * Special color or pattern. 593 */ 594 @Child(name = "color", type = { StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 595 @Description(shortDefinition = "Color required", formalDefinition = "Special color or pattern.") 596 protected StringType color; 597 598 /** 599 * Brand recommendations or restrictions. 600 */ 601 @Child(name = "brand", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 602 @Description(shortDefinition = "Brand required", formalDefinition = "Brand recommendations or restrictions.") 603 protected StringType brand; 604 605 /** 606 * Notes for special requirements such as coatings and lens materials. 607 */ 608 @Child(name = "note", type = { 609 Annotation.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 610 @Description(shortDefinition = "Notes for coatings", formalDefinition = "Notes for special requirements such as coatings and lens materials.") 611 protected List<Annotation> note; 612 613 private static final long serialVersionUID = 688924460L; 614 615 /** 616 * Constructor 617 */ 618 public VisionPrescriptionLensSpecificationComponent() { 619 super(); 620 } 621 622 /** 623 * Constructor 624 */ 625 public VisionPrescriptionLensSpecificationComponent(CodeableConcept product, Enumeration<VisionEyes> eye) { 626 super(); 627 this.product = product; 628 this.eye = eye; 629 } 630 631 /** 632 * @return {@link #product} (Identifies the type of vision correction product 633 * which is required for the patient.) 634 */ 635 public CodeableConcept getProduct() { 636 if (this.product == null) 637 if (Configuration.errorOnAutoCreate()) 638 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.product"); 639 else if (Configuration.doAutoCreate()) 640 this.product = new CodeableConcept(); // cc 641 return this.product; 642 } 643 644 public boolean hasProduct() { 645 return this.product != null && !this.product.isEmpty(); 646 } 647 648 /** 649 * @param value {@link #product} (Identifies the type of vision correction 650 * product which is required for the patient.) 651 */ 652 public VisionPrescriptionLensSpecificationComponent setProduct(CodeableConcept value) { 653 this.product = value; 654 return this; 655 } 656 657 /** 658 * @return {@link #eye} (The eye for which the lens specification applies.). 659 * This is the underlying object with id, value and extensions. The 660 * accessor "getEye" gives direct access to the value 661 */ 662 public Enumeration<VisionEyes> getEyeElement() { 663 if (this.eye == null) 664 if (Configuration.errorOnAutoCreate()) 665 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.eye"); 666 else if (Configuration.doAutoCreate()) 667 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); // bb 668 return this.eye; 669 } 670 671 public boolean hasEyeElement() { 672 return this.eye != null && !this.eye.isEmpty(); 673 } 674 675 public boolean hasEye() { 676 return this.eye != null && !this.eye.isEmpty(); 677 } 678 679 /** 680 * @param value {@link #eye} (The eye for which the lens specification 681 * applies.). This is the underlying object with id, value and 682 * extensions. The accessor "getEye" gives direct access to the 683 * value 684 */ 685 public VisionPrescriptionLensSpecificationComponent setEyeElement(Enumeration<VisionEyes> value) { 686 this.eye = value; 687 return this; 688 } 689 690 /** 691 * @return The eye for which the lens specification applies. 692 */ 693 public VisionEyes getEye() { 694 return this.eye == null ? null : this.eye.getValue(); 695 } 696 697 /** 698 * @param value The eye for which the lens specification applies. 699 */ 700 public VisionPrescriptionLensSpecificationComponent setEye(VisionEyes value) { 701 if (this.eye == null) 702 this.eye = new Enumeration<VisionEyes>(new VisionEyesEnumFactory()); 703 this.eye.setValue(value); 704 return this; 705 } 706 707 /** 708 * @return {@link #sphere} (Lens power measured in dioptres (0.25 units).). This 709 * is the underlying object with id, value and extensions. The accessor 710 * "getSphere" gives direct access to the value 711 */ 712 public DecimalType getSphereElement() { 713 if (this.sphere == null) 714 if (Configuration.errorOnAutoCreate()) 715 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.sphere"); 716 else if (Configuration.doAutoCreate()) 717 this.sphere = new DecimalType(); // bb 718 return this.sphere; 719 } 720 721 public boolean hasSphereElement() { 722 return this.sphere != null && !this.sphere.isEmpty(); 723 } 724 725 public boolean hasSphere() { 726 return this.sphere != null && !this.sphere.isEmpty(); 727 } 728 729 /** 730 * @param value {@link #sphere} (Lens power measured in dioptres (0.25 units).). 731 * This is the underlying object with id, value and extensions. The 732 * accessor "getSphere" gives direct access to the value 733 */ 734 public VisionPrescriptionLensSpecificationComponent setSphereElement(DecimalType value) { 735 this.sphere = value; 736 return this; 737 } 738 739 /** 740 * @return Lens power measured in dioptres (0.25 units). 741 */ 742 public BigDecimal getSphere() { 743 return this.sphere == null ? null : this.sphere.getValue(); 744 } 745 746 /** 747 * @param value Lens power measured in dioptres (0.25 units). 748 */ 749 public VisionPrescriptionLensSpecificationComponent setSphere(BigDecimal value) { 750 if (value == null) 751 this.sphere = null; 752 else { 753 if (this.sphere == null) 754 this.sphere = new DecimalType(); 755 this.sphere.setValue(value); 756 } 757 return this; 758 } 759 760 /** 761 * @param value Lens power measured in dioptres (0.25 units). 762 */ 763 public VisionPrescriptionLensSpecificationComponent setSphere(long value) { 764 this.sphere = new DecimalType(); 765 this.sphere.setValue(value); 766 return this; 767 } 768 769 /** 770 * @param value Lens power measured in dioptres (0.25 units). 771 */ 772 public VisionPrescriptionLensSpecificationComponent setSphere(double value) { 773 this.sphere = new DecimalType(); 774 this.sphere.setValue(value); 775 return this; 776 } 777 778 /** 779 * @return {@link #cylinder} (Power adjustment for astigmatism measured in 780 * dioptres (0.25 units).). This is the underlying object with id, value 781 * and extensions. The accessor "getCylinder" gives direct access to the 782 * value 783 */ 784 public DecimalType getCylinderElement() { 785 if (this.cylinder == null) 786 if (Configuration.errorOnAutoCreate()) 787 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.cylinder"); 788 else if (Configuration.doAutoCreate()) 789 this.cylinder = new DecimalType(); // bb 790 return this.cylinder; 791 } 792 793 public boolean hasCylinderElement() { 794 return this.cylinder != null && !this.cylinder.isEmpty(); 795 } 796 797 public boolean hasCylinder() { 798 return this.cylinder != null && !this.cylinder.isEmpty(); 799 } 800 801 /** 802 * @param value {@link #cylinder} (Power adjustment for astigmatism measured in 803 * dioptres (0.25 units).). This is the underlying object with id, 804 * value and extensions. The accessor "getCylinder" gives direct 805 * access to the value 806 */ 807 public VisionPrescriptionLensSpecificationComponent setCylinderElement(DecimalType value) { 808 this.cylinder = value; 809 return this; 810 } 811 812 /** 813 * @return Power adjustment for astigmatism measured in dioptres (0.25 units). 814 */ 815 public BigDecimal getCylinder() { 816 return this.cylinder == null ? null : this.cylinder.getValue(); 817 } 818 819 /** 820 * @param value Power adjustment for astigmatism measured in dioptres (0.25 821 * units). 822 */ 823 public VisionPrescriptionLensSpecificationComponent setCylinder(BigDecimal value) { 824 if (value == null) 825 this.cylinder = null; 826 else { 827 if (this.cylinder == null) 828 this.cylinder = new DecimalType(); 829 this.cylinder.setValue(value); 830 } 831 return this; 832 } 833 834 /** 835 * @param value Power adjustment for astigmatism measured in dioptres (0.25 836 * units). 837 */ 838 public VisionPrescriptionLensSpecificationComponent setCylinder(long value) { 839 this.cylinder = new DecimalType(); 840 this.cylinder.setValue(value); 841 return this; 842 } 843 844 /** 845 * @param value Power adjustment for astigmatism measured in dioptres (0.25 846 * units). 847 */ 848 public VisionPrescriptionLensSpecificationComponent setCylinder(double value) { 849 this.cylinder = new DecimalType(); 850 this.cylinder.setValue(value); 851 return this; 852 } 853 854 /** 855 * @return {@link #axis} (Adjustment for astigmatism measured in integer 856 * degrees.). This is the underlying object with id, value and 857 * extensions. The accessor "getAxis" gives direct access to the value 858 */ 859 public IntegerType getAxisElement() { 860 if (this.axis == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.axis"); 863 else if (Configuration.doAutoCreate()) 864 this.axis = new IntegerType(); // bb 865 return this.axis; 866 } 867 868 public boolean hasAxisElement() { 869 return this.axis != null && !this.axis.isEmpty(); 870 } 871 872 public boolean hasAxis() { 873 return this.axis != null && !this.axis.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #axis} (Adjustment for astigmatism measured in integer 878 * degrees.). This is the underlying object with id, value and 879 * extensions. The accessor "getAxis" gives direct access to the 880 * value 881 */ 882 public VisionPrescriptionLensSpecificationComponent setAxisElement(IntegerType value) { 883 this.axis = value; 884 return this; 885 } 886 887 /** 888 * @return Adjustment for astigmatism measured in integer degrees. 889 */ 890 public int getAxis() { 891 return this.axis == null || this.axis.isEmpty() ? 0 : this.axis.getValue(); 892 } 893 894 /** 895 * @param value Adjustment for astigmatism measured in integer degrees. 896 */ 897 public VisionPrescriptionLensSpecificationComponent setAxis(int value) { 898 if (this.axis == null) 899 this.axis = new IntegerType(); 900 this.axis.setValue(value); 901 return this; 902 } 903 904 /** 905 * @return {@link #prism} (Allows for adjustment on two axis.) 906 */ 907 public List<PrismComponent> getPrism() { 908 if (this.prism == null) 909 this.prism = new ArrayList<PrismComponent>(); 910 return this.prism; 911 } 912 913 /** 914 * @return Returns a reference to <code>this</code> for easy method chaining 915 */ 916 public VisionPrescriptionLensSpecificationComponent setPrism(List<PrismComponent> thePrism) { 917 this.prism = thePrism; 918 return this; 919 } 920 921 public boolean hasPrism() { 922 if (this.prism == null) 923 return false; 924 for (PrismComponent item : this.prism) 925 if (!item.isEmpty()) 926 return true; 927 return false; 928 } 929 930 public PrismComponent addPrism() { // 3 931 PrismComponent t = new PrismComponent(); 932 if (this.prism == null) 933 this.prism = new ArrayList<PrismComponent>(); 934 this.prism.add(t); 935 return t; 936 } 937 938 public VisionPrescriptionLensSpecificationComponent addPrism(PrismComponent t) { // 3 939 if (t == null) 940 return this; 941 if (this.prism == null) 942 this.prism = new ArrayList<PrismComponent>(); 943 this.prism.add(t); 944 return this; 945 } 946 947 /** 948 * @return The first repetition of repeating field {@link #prism}, creating it 949 * if it does not already exist 950 */ 951 public PrismComponent getPrismFirstRep() { 952 if (getPrism().isEmpty()) { 953 addPrism(); 954 } 955 return getPrism().get(0); 956 } 957 958 /** 959 * @return {@link #add} (Power adjustment for multifocal lenses measured in 960 * dioptres (0.25 units).). This is the underlying object with id, value 961 * and extensions. The accessor "getAdd" gives direct access to the 962 * value 963 */ 964 public DecimalType getAddElement() { 965 if (this.add == null) 966 if (Configuration.errorOnAutoCreate()) 967 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.add"); 968 else if (Configuration.doAutoCreate()) 969 this.add = new DecimalType(); // bb 970 return this.add; 971 } 972 973 public boolean hasAddElement() { 974 return this.add != null && !this.add.isEmpty(); 975 } 976 977 public boolean hasAdd() { 978 return this.add != null && !this.add.isEmpty(); 979 } 980 981 /** 982 * @param value {@link #add} (Power adjustment for multifocal lenses measured in 983 * dioptres (0.25 units).). This is the underlying object with id, 984 * value and extensions. The accessor "getAdd" gives direct access 985 * to the value 986 */ 987 public VisionPrescriptionLensSpecificationComponent setAddElement(DecimalType value) { 988 this.add = value; 989 return this; 990 } 991 992 /** 993 * @return Power adjustment for multifocal lenses measured in dioptres (0.25 994 * units). 995 */ 996 public BigDecimal getAdd() { 997 return this.add == null ? null : this.add.getValue(); 998 } 999 1000 /** 1001 * @param value Power adjustment for multifocal lenses measured in dioptres 1002 * (0.25 units). 1003 */ 1004 public VisionPrescriptionLensSpecificationComponent setAdd(BigDecimal value) { 1005 if (value == null) 1006 this.add = null; 1007 else { 1008 if (this.add == null) 1009 this.add = new DecimalType(); 1010 this.add.setValue(value); 1011 } 1012 return this; 1013 } 1014 1015 /** 1016 * @param value Power adjustment for multifocal lenses measured in dioptres 1017 * (0.25 units). 1018 */ 1019 public VisionPrescriptionLensSpecificationComponent setAdd(long value) { 1020 this.add = new DecimalType(); 1021 this.add.setValue(value); 1022 return this; 1023 } 1024 1025 /** 1026 * @param value Power adjustment for multifocal lenses measured in dioptres 1027 * (0.25 units). 1028 */ 1029 public VisionPrescriptionLensSpecificationComponent setAdd(double value) { 1030 this.add = new DecimalType(); 1031 this.add.setValue(value); 1032 return this; 1033 } 1034 1035 /** 1036 * @return {@link #power} (Contact lens power measured in dioptres (0.25 1037 * units).). This is the underlying object with id, value and 1038 * extensions. The accessor "getPower" gives direct access to the value 1039 */ 1040 public DecimalType getPowerElement() { 1041 if (this.power == null) 1042 if (Configuration.errorOnAutoCreate()) 1043 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.power"); 1044 else if (Configuration.doAutoCreate()) 1045 this.power = new DecimalType(); // bb 1046 return this.power; 1047 } 1048 1049 public boolean hasPowerElement() { 1050 return this.power != null && !this.power.isEmpty(); 1051 } 1052 1053 public boolean hasPower() { 1054 return this.power != null && !this.power.isEmpty(); 1055 } 1056 1057 /** 1058 * @param value {@link #power} (Contact lens power measured in dioptres (0.25 1059 * units).). This is the underlying object with id, value and 1060 * extensions. The accessor "getPower" gives direct access to the 1061 * value 1062 */ 1063 public VisionPrescriptionLensSpecificationComponent setPowerElement(DecimalType value) { 1064 this.power = value; 1065 return this; 1066 } 1067 1068 /** 1069 * @return Contact lens power measured in dioptres (0.25 units). 1070 */ 1071 public BigDecimal getPower() { 1072 return this.power == null ? null : this.power.getValue(); 1073 } 1074 1075 /** 1076 * @param value Contact lens power measured in dioptres (0.25 units). 1077 */ 1078 public VisionPrescriptionLensSpecificationComponent setPower(BigDecimal value) { 1079 if (value == null) 1080 this.power = null; 1081 else { 1082 if (this.power == null) 1083 this.power = new DecimalType(); 1084 this.power.setValue(value); 1085 } 1086 return this; 1087 } 1088 1089 /** 1090 * @param value Contact lens power measured in dioptres (0.25 units). 1091 */ 1092 public VisionPrescriptionLensSpecificationComponent setPower(long value) { 1093 this.power = new DecimalType(); 1094 this.power.setValue(value); 1095 return this; 1096 } 1097 1098 /** 1099 * @param value Contact lens power measured in dioptres (0.25 units). 1100 */ 1101 public VisionPrescriptionLensSpecificationComponent setPower(double value) { 1102 this.power = new DecimalType(); 1103 this.power.setValue(value); 1104 return this; 1105 } 1106 1107 /** 1108 * @return {@link #backCurve} (Back curvature measured in millimetres.). This is 1109 * the underlying object with id, value and extensions. The accessor 1110 * "getBackCurve" gives direct access to the value 1111 */ 1112 public DecimalType getBackCurveElement() { 1113 if (this.backCurve == null) 1114 if (Configuration.errorOnAutoCreate()) 1115 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.backCurve"); 1116 else if (Configuration.doAutoCreate()) 1117 this.backCurve = new DecimalType(); // bb 1118 return this.backCurve; 1119 } 1120 1121 public boolean hasBackCurveElement() { 1122 return this.backCurve != null && !this.backCurve.isEmpty(); 1123 } 1124 1125 public boolean hasBackCurve() { 1126 return this.backCurve != null && !this.backCurve.isEmpty(); 1127 } 1128 1129 /** 1130 * @param value {@link #backCurve} (Back curvature measured in millimetres.). 1131 * This is the underlying object with id, value and extensions. The 1132 * accessor "getBackCurve" gives direct access to the value 1133 */ 1134 public VisionPrescriptionLensSpecificationComponent setBackCurveElement(DecimalType value) { 1135 this.backCurve = value; 1136 return this; 1137 } 1138 1139 /** 1140 * @return Back curvature measured in millimetres. 1141 */ 1142 public BigDecimal getBackCurve() { 1143 return this.backCurve == null ? null : this.backCurve.getValue(); 1144 } 1145 1146 /** 1147 * @param value Back curvature measured in millimetres. 1148 */ 1149 public VisionPrescriptionLensSpecificationComponent setBackCurve(BigDecimal value) { 1150 if (value == null) 1151 this.backCurve = null; 1152 else { 1153 if (this.backCurve == null) 1154 this.backCurve = new DecimalType(); 1155 this.backCurve.setValue(value); 1156 } 1157 return this; 1158 } 1159 1160 /** 1161 * @param value Back curvature measured in millimetres. 1162 */ 1163 public VisionPrescriptionLensSpecificationComponent setBackCurve(long value) { 1164 this.backCurve = new DecimalType(); 1165 this.backCurve.setValue(value); 1166 return this; 1167 } 1168 1169 /** 1170 * @param value Back curvature measured in millimetres. 1171 */ 1172 public VisionPrescriptionLensSpecificationComponent setBackCurve(double value) { 1173 this.backCurve = new DecimalType(); 1174 this.backCurve.setValue(value); 1175 return this; 1176 } 1177 1178 /** 1179 * @return {@link #diameter} (Contact lens diameter measured in millimetres.). 1180 * This is the underlying object with id, value and extensions. The 1181 * accessor "getDiameter" gives direct access to the value 1182 */ 1183 public DecimalType getDiameterElement() { 1184 if (this.diameter == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.diameter"); 1187 else if (Configuration.doAutoCreate()) 1188 this.diameter = new DecimalType(); // bb 1189 return this.diameter; 1190 } 1191 1192 public boolean hasDiameterElement() { 1193 return this.diameter != null && !this.diameter.isEmpty(); 1194 } 1195 1196 public boolean hasDiameter() { 1197 return this.diameter != null && !this.diameter.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #diameter} (Contact lens diameter measured in 1202 * millimetres.). This is the underlying object with id, value and 1203 * extensions. The accessor "getDiameter" gives direct access to 1204 * the value 1205 */ 1206 public VisionPrescriptionLensSpecificationComponent setDiameterElement(DecimalType value) { 1207 this.diameter = value; 1208 return this; 1209 } 1210 1211 /** 1212 * @return Contact lens diameter measured in millimetres. 1213 */ 1214 public BigDecimal getDiameter() { 1215 return this.diameter == null ? null : this.diameter.getValue(); 1216 } 1217 1218 /** 1219 * @param value Contact lens diameter measured in millimetres. 1220 */ 1221 public VisionPrescriptionLensSpecificationComponent setDiameter(BigDecimal value) { 1222 if (value == null) 1223 this.diameter = null; 1224 else { 1225 if (this.diameter == null) 1226 this.diameter = new DecimalType(); 1227 this.diameter.setValue(value); 1228 } 1229 return this; 1230 } 1231 1232 /** 1233 * @param value Contact lens diameter measured in millimetres. 1234 */ 1235 public VisionPrescriptionLensSpecificationComponent setDiameter(long value) { 1236 this.diameter = new DecimalType(); 1237 this.diameter.setValue(value); 1238 return this; 1239 } 1240 1241 /** 1242 * @param value Contact lens diameter measured in millimetres. 1243 */ 1244 public VisionPrescriptionLensSpecificationComponent setDiameter(double value) { 1245 this.diameter = new DecimalType(); 1246 this.diameter.setValue(value); 1247 return this; 1248 } 1249 1250 /** 1251 * @return {@link #duration} (The recommended maximum wear period for the lens.) 1252 */ 1253 public Quantity getDuration() { 1254 if (this.duration == null) 1255 if (Configuration.errorOnAutoCreate()) 1256 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.duration"); 1257 else if (Configuration.doAutoCreate()) 1258 this.duration = new Quantity(); // cc 1259 return this.duration; 1260 } 1261 1262 public boolean hasDuration() { 1263 return this.duration != null && !this.duration.isEmpty(); 1264 } 1265 1266 /** 1267 * @param value {@link #duration} (The recommended maximum wear period for the 1268 * lens.) 1269 */ 1270 public VisionPrescriptionLensSpecificationComponent setDuration(Quantity value) { 1271 this.duration = value; 1272 return this; 1273 } 1274 1275 /** 1276 * @return {@link #color} (Special color or pattern.). This is the underlying 1277 * object with id, value and extensions. The accessor "getColor" gives 1278 * direct access to the value 1279 */ 1280 public StringType getColorElement() { 1281 if (this.color == null) 1282 if (Configuration.errorOnAutoCreate()) 1283 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.color"); 1284 else if (Configuration.doAutoCreate()) 1285 this.color = new StringType(); // bb 1286 return this.color; 1287 } 1288 1289 public boolean hasColorElement() { 1290 return this.color != null && !this.color.isEmpty(); 1291 } 1292 1293 public boolean hasColor() { 1294 return this.color != null && !this.color.isEmpty(); 1295 } 1296 1297 /** 1298 * @param value {@link #color} (Special color or pattern.). This is the 1299 * underlying object with id, value and extensions. The accessor 1300 * "getColor" gives direct access to the value 1301 */ 1302 public VisionPrescriptionLensSpecificationComponent setColorElement(StringType value) { 1303 this.color = value; 1304 return this; 1305 } 1306 1307 /** 1308 * @return Special color or pattern. 1309 */ 1310 public String getColor() { 1311 return this.color == null ? null : this.color.getValue(); 1312 } 1313 1314 /** 1315 * @param value Special color or pattern. 1316 */ 1317 public VisionPrescriptionLensSpecificationComponent setColor(String value) { 1318 if (Utilities.noString(value)) 1319 this.color = null; 1320 else { 1321 if (this.color == null) 1322 this.color = new StringType(); 1323 this.color.setValue(value); 1324 } 1325 return this; 1326 } 1327 1328 /** 1329 * @return {@link #brand} (Brand recommendations or restrictions.). This is the 1330 * underlying object with id, value and extensions. The accessor 1331 * "getBrand" gives direct access to the value 1332 */ 1333 public StringType getBrandElement() { 1334 if (this.brand == null) 1335 if (Configuration.errorOnAutoCreate()) 1336 throw new Error("Attempt to auto-create VisionPrescriptionLensSpecificationComponent.brand"); 1337 else if (Configuration.doAutoCreate()) 1338 this.brand = new StringType(); // bb 1339 return this.brand; 1340 } 1341 1342 public boolean hasBrandElement() { 1343 return this.brand != null && !this.brand.isEmpty(); 1344 } 1345 1346 public boolean hasBrand() { 1347 return this.brand != null && !this.brand.isEmpty(); 1348 } 1349 1350 /** 1351 * @param value {@link #brand} (Brand recommendations or restrictions.). This is 1352 * the underlying object with id, value and extensions. The 1353 * accessor "getBrand" gives direct access to the value 1354 */ 1355 public VisionPrescriptionLensSpecificationComponent setBrandElement(StringType value) { 1356 this.brand = value; 1357 return this; 1358 } 1359 1360 /** 1361 * @return Brand recommendations or restrictions. 1362 */ 1363 public String getBrand() { 1364 return this.brand == null ? null : this.brand.getValue(); 1365 } 1366 1367 /** 1368 * @param value Brand recommendations or restrictions. 1369 */ 1370 public VisionPrescriptionLensSpecificationComponent setBrand(String value) { 1371 if (Utilities.noString(value)) 1372 this.brand = null; 1373 else { 1374 if (this.brand == null) 1375 this.brand = new StringType(); 1376 this.brand.setValue(value); 1377 } 1378 return this; 1379 } 1380 1381 /** 1382 * @return {@link #note} (Notes for special requirements such as coatings and 1383 * lens materials.) 1384 */ 1385 public List<Annotation> getNote() { 1386 if (this.note == null) 1387 this.note = new ArrayList<Annotation>(); 1388 return this.note; 1389 } 1390 1391 /** 1392 * @return Returns a reference to <code>this</code> for easy method chaining 1393 */ 1394 public VisionPrescriptionLensSpecificationComponent setNote(List<Annotation> theNote) { 1395 this.note = theNote; 1396 return this; 1397 } 1398 1399 public boolean hasNote() { 1400 if (this.note == null) 1401 return false; 1402 for (Annotation item : this.note) 1403 if (!item.isEmpty()) 1404 return true; 1405 return false; 1406 } 1407 1408 public Annotation addNote() { // 3 1409 Annotation t = new Annotation(); 1410 if (this.note == null) 1411 this.note = new ArrayList<Annotation>(); 1412 this.note.add(t); 1413 return t; 1414 } 1415 1416 public VisionPrescriptionLensSpecificationComponent addNote(Annotation t) { // 3 1417 if (t == null) 1418 return this; 1419 if (this.note == null) 1420 this.note = new ArrayList<Annotation>(); 1421 this.note.add(t); 1422 return this; 1423 } 1424 1425 /** 1426 * @return The first repetition of repeating field {@link #note}, creating it if 1427 * it does not already exist 1428 */ 1429 public Annotation getNoteFirstRep() { 1430 if (getNote().isEmpty()) { 1431 addNote(); 1432 } 1433 return getNote().get(0); 1434 } 1435 1436 protected void listChildren(List<Property> children) { 1437 super.listChildren(children); 1438 children.add(new Property("product", "CodeableConcept", 1439 "Identifies the type of vision correction product which is required for the patient.", 0, 1, product)); 1440 children.add(new Property("eye", "code", "The eye for which the lens specification applies.", 0, 1, eye)); 1441 children.add(new Property("sphere", "decimal", "Lens power measured in dioptres (0.25 units).", 0, 1, sphere)); 1442 children.add(new Property("cylinder", "decimal", 1443 "Power adjustment for astigmatism measured in dioptres (0.25 units).", 0, 1, cylinder)); 1444 children 1445 .add(new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1, axis)); 1446 children 1447 .add(new Property("prism", "", "Allows for adjustment on two axis.", 0, java.lang.Integer.MAX_VALUE, prism)); 1448 children.add(new Property("add", "decimal", 1449 "Power adjustment for multifocal lenses measured in dioptres (0.25 units).", 0, 1, add)); 1450 children 1451 .add(new Property("power", "decimal", "Contact lens power measured in dioptres (0.25 units).", 0, 1, power)); 1452 children.add(new Property("backCurve", "decimal", "Back curvature measured in millimetres.", 0, 1, backCurve)); 1453 children 1454 .add(new Property("diameter", "decimal", "Contact lens diameter measured in millimetres.", 0, 1, diameter)); 1455 children.add(new Property("duration", "SimpleQuantity", "The recommended maximum wear period for the lens.", 0, 1, 1456 duration)); 1457 children.add(new Property("color", "string", "Special color or pattern.", 0, 1, color)); 1458 children.add(new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1, brand)); 1459 children.add(new Property("note", "Annotation", 1460 "Notes for special requirements such as coatings and lens materials.", 0, java.lang.Integer.MAX_VALUE, note)); 1461 } 1462 1463 @Override 1464 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1465 switch (_hash) { 1466 case -309474065: 1467 /* product */ return new Property("product", "CodeableConcept", 1468 "Identifies the type of vision correction product which is required for the patient.", 0, 1, product); 1469 case 100913: 1470 /* eye */ return new Property("eye", "code", "The eye for which the lens specification applies.", 0, 1, eye); 1471 case -895981619: 1472 /* sphere */ return new Property("sphere", "decimal", "Lens power measured in dioptres (0.25 units).", 0, 1, 1473 sphere); 1474 case -349378602: 1475 /* cylinder */ return new Property("cylinder", "decimal", 1476 "Power adjustment for astigmatism measured in dioptres (0.25 units).", 0, 1, cylinder); 1477 case 3008417: 1478 /* axis */ return new Property("axis", "integer", "Adjustment for astigmatism measured in integer degrees.", 0, 1479 1, axis); 1480 case 106935105: 1481 /* prism */ return new Property("prism", "", "Allows for adjustment on two axis.", 0, 1482 java.lang.Integer.MAX_VALUE, prism); 1483 case 96417: 1484 /* add */ return new Property("add", "decimal", 1485 "Power adjustment for multifocal lenses measured in dioptres (0.25 units).", 0, 1, add); 1486 case 106858757: 1487 /* power */ return new Property("power", "decimal", "Contact lens power measured in dioptres (0.25 units).", 0, 1488 1, power); 1489 case 1309344840: 1490 /* backCurve */ return new Property("backCurve", "decimal", "Back curvature measured in millimetres.", 0, 1, 1491 backCurve); 1492 case -233204595: 1493 /* diameter */ return new Property("diameter", "decimal", "Contact lens diameter measured in millimetres.", 0, 1494 1, diameter); 1495 case -1992012396: 1496 /* duration */ return new Property("duration", "SimpleQuantity", 1497 "The recommended maximum wear period for the lens.", 0, 1, duration); 1498 case 94842723: 1499 /* color */ return new Property("color", "string", "Special color or pattern.", 0, 1, color); 1500 case 93997959: 1501 /* brand */ return new Property("brand", "string", "Brand recommendations or restrictions.", 0, 1, brand); 1502 case 3387378: 1503 /* note */ return new Property("note", "Annotation", 1504 "Notes for special requirements such as coatings and lens materials.", 0, java.lang.Integer.MAX_VALUE, 1505 note); 1506 default: 1507 return super.getNamedProperty(_hash, _name, _checkValid); 1508 } 1509 1510 } 1511 1512 @Override 1513 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1514 switch (hash) { 1515 case -309474065: 1516 /* product */ return this.product == null ? new Base[0] : new Base[] { this.product }; // CodeableConcept 1517 case 100913: 1518 /* eye */ return this.eye == null ? new Base[0] : new Base[] { this.eye }; // Enumeration<VisionEyes> 1519 case -895981619: 1520 /* sphere */ return this.sphere == null ? new Base[0] : new Base[] { this.sphere }; // DecimalType 1521 case -349378602: 1522 /* cylinder */ return this.cylinder == null ? new Base[0] : new Base[] { this.cylinder }; // DecimalType 1523 case 3008417: 1524 /* axis */ return this.axis == null ? new Base[0] : new Base[] { this.axis }; // IntegerType 1525 case 106935105: 1526 /* prism */ return this.prism == null ? new Base[0] : this.prism.toArray(new Base[this.prism.size()]); // PrismComponent 1527 case 96417: 1528 /* add */ return this.add == null ? new Base[0] : new Base[] { this.add }; // DecimalType 1529 case 106858757: 1530 /* power */ return this.power == null ? new Base[0] : new Base[] { this.power }; // DecimalType 1531 case 1309344840: 1532 /* backCurve */ return this.backCurve == null ? new Base[0] : new Base[] { this.backCurve }; // DecimalType 1533 case -233204595: 1534 /* diameter */ return this.diameter == null ? new Base[0] : new Base[] { this.diameter }; // DecimalType 1535 case -1992012396: 1536 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Quantity 1537 case 94842723: 1538 /* color */ return this.color == null ? new Base[0] : new Base[] { this.color }; // StringType 1539 case 93997959: 1540 /* brand */ return this.brand == null ? new Base[0] : new Base[] { this.brand }; // StringType 1541 case 3387378: 1542 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1543 default: 1544 return super.getProperty(hash, name, checkValid); 1545 } 1546 1547 } 1548 1549 @Override 1550 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1551 switch (hash) { 1552 case -309474065: // product 1553 this.product = castToCodeableConcept(value); // CodeableConcept 1554 return value; 1555 case 100913: // eye 1556 value = new VisionEyesEnumFactory().fromType(castToCode(value)); 1557 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1558 return value; 1559 case -895981619: // sphere 1560 this.sphere = castToDecimal(value); // DecimalType 1561 return value; 1562 case -349378602: // cylinder 1563 this.cylinder = castToDecimal(value); // DecimalType 1564 return value; 1565 case 3008417: // axis 1566 this.axis = castToInteger(value); // IntegerType 1567 return value; 1568 case 106935105: // prism 1569 this.getPrism().add((PrismComponent) value); // PrismComponent 1570 return value; 1571 case 96417: // add 1572 this.add = castToDecimal(value); // DecimalType 1573 return value; 1574 case 106858757: // power 1575 this.power = castToDecimal(value); // DecimalType 1576 return value; 1577 case 1309344840: // backCurve 1578 this.backCurve = castToDecimal(value); // DecimalType 1579 return value; 1580 case -233204595: // diameter 1581 this.diameter = castToDecimal(value); // DecimalType 1582 return value; 1583 case -1992012396: // duration 1584 this.duration = castToQuantity(value); // Quantity 1585 return value; 1586 case 94842723: // color 1587 this.color = castToString(value); // StringType 1588 return value; 1589 case 93997959: // brand 1590 this.brand = castToString(value); // StringType 1591 return value; 1592 case 3387378: // note 1593 this.getNote().add(castToAnnotation(value)); // Annotation 1594 return value; 1595 default: 1596 return super.setProperty(hash, name, value); 1597 } 1598 1599 } 1600 1601 @Override 1602 public Base setProperty(String name, Base value) throws FHIRException { 1603 if (name.equals("product")) { 1604 this.product = castToCodeableConcept(value); // CodeableConcept 1605 } else if (name.equals("eye")) { 1606 value = new VisionEyesEnumFactory().fromType(castToCode(value)); 1607 this.eye = (Enumeration) value; // Enumeration<VisionEyes> 1608 } else if (name.equals("sphere")) { 1609 this.sphere = castToDecimal(value); // DecimalType 1610 } else if (name.equals("cylinder")) { 1611 this.cylinder = castToDecimal(value); // DecimalType 1612 } else if (name.equals("axis")) { 1613 this.axis = castToInteger(value); // IntegerType 1614 } else if (name.equals("prism")) { 1615 this.getPrism().add((PrismComponent) value); 1616 } else if (name.equals("add")) { 1617 this.add = castToDecimal(value); // DecimalType 1618 } else if (name.equals("power")) { 1619 this.power = castToDecimal(value); // DecimalType 1620 } else if (name.equals("backCurve")) { 1621 this.backCurve = castToDecimal(value); // DecimalType 1622 } else if (name.equals("diameter")) { 1623 this.diameter = castToDecimal(value); // DecimalType 1624 } else if (name.equals("duration")) { 1625 this.duration = castToQuantity(value); // Quantity 1626 } else if (name.equals("color")) { 1627 this.color = castToString(value); // StringType 1628 } else if (name.equals("brand")) { 1629 this.brand = castToString(value); // StringType 1630 } else if (name.equals("note")) { 1631 this.getNote().add(castToAnnotation(value)); 1632 } else 1633 return super.setProperty(name, value); 1634 return value; 1635 } 1636 1637 @Override 1638 public void removeChild(String name, Base value) throws FHIRException { 1639 if (name.equals("product")) { 1640 this.product = null; 1641 } else if (name.equals("eye")) { 1642 this.eye = null; 1643 } else if (name.equals("sphere")) { 1644 this.sphere = null; 1645 } else if (name.equals("cylinder")) { 1646 this.cylinder = null; 1647 } else if (name.equals("axis")) { 1648 this.axis = null; 1649 } else if (name.equals("prism")) { 1650 this.getPrism().remove((PrismComponent) value); 1651 } else if (name.equals("add")) { 1652 this.add = null; 1653 } else if (name.equals("power")) { 1654 this.power = null; 1655 } else if (name.equals("backCurve")) { 1656 this.backCurve = null; 1657 } else if (name.equals("diameter")) { 1658 this.diameter = null; 1659 } else if (name.equals("duration")) { 1660 this.duration = null; 1661 } else if (name.equals("color")) { 1662 this.color = null; 1663 } else if (name.equals("brand")) { 1664 this.brand = null; 1665 } else if (name.equals("note")) { 1666 this.getNote().remove(castToAnnotation(value)); 1667 } else 1668 super.removeChild(name, value); 1669 1670 } 1671 1672 @Override 1673 public Base makeProperty(int hash, String name) throws FHIRException { 1674 switch (hash) { 1675 case -309474065: 1676 return getProduct(); 1677 case 100913: 1678 return getEyeElement(); 1679 case -895981619: 1680 return getSphereElement(); 1681 case -349378602: 1682 return getCylinderElement(); 1683 case 3008417: 1684 return getAxisElement(); 1685 case 106935105: 1686 return addPrism(); 1687 case 96417: 1688 return getAddElement(); 1689 case 106858757: 1690 return getPowerElement(); 1691 case 1309344840: 1692 return getBackCurveElement(); 1693 case -233204595: 1694 return getDiameterElement(); 1695 case -1992012396: 1696 return getDuration(); 1697 case 94842723: 1698 return getColorElement(); 1699 case 93997959: 1700 return getBrandElement(); 1701 case 3387378: 1702 return addNote(); 1703 default: 1704 return super.makeProperty(hash, name); 1705 } 1706 1707 } 1708 1709 @Override 1710 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1711 switch (hash) { 1712 case -309474065: 1713 /* product */ return new String[] { "CodeableConcept" }; 1714 case 100913: 1715 /* eye */ return new String[] { "code" }; 1716 case -895981619: 1717 /* sphere */ return new String[] { "decimal" }; 1718 case -349378602: 1719 /* cylinder */ return new String[] { "decimal" }; 1720 case 3008417: 1721 /* axis */ return new String[] { "integer" }; 1722 case 106935105: 1723 /* prism */ return new String[] {}; 1724 case 96417: 1725 /* add */ return new String[] { "decimal" }; 1726 case 106858757: 1727 /* power */ return new String[] { "decimal" }; 1728 case 1309344840: 1729 /* backCurve */ return new String[] { "decimal" }; 1730 case -233204595: 1731 /* diameter */ return new String[] { "decimal" }; 1732 case -1992012396: 1733 /* duration */ return new String[] { "SimpleQuantity" }; 1734 case 94842723: 1735 /* color */ return new String[] { "string" }; 1736 case 93997959: 1737 /* brand */ return new String[] { "string" }; 1738 case 3387378: 1739 /* note */ return new String[] { "Annotation" }; 1740 default: 1741 return super.getTypesForProperty(hash, name); 1742 } 1743 1744 } 1745 1746 @Override 1747 public Base addChild(String name) throws FHIRException { 1748 if (name.equals("product")) { 1749 this.product = new CodeableConcept(); 1750 return this.product; 1751 } else if (name.equals("eye")) { 1752 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.eye"); 1753 } else if (name.equals("sphere")) { 1754 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.sphere"); 1755 } else if (name.equals("cylinder")) { 1756 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.cylinder"); 1757 } else if (name.equals("axis")) { 1758 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.axis"); 1759 } else if (name.equals("prism")) { 1760 return addPrism(); 1761 } else if (name.equals("add")) { 1762 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.add"); 1763 } else if (name.equals("power")) { 1764 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.power"); 1765 } else if (name.equals("backCurve")) { 1766 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.backCurve"); 1767 } else if (name.equals("diameter")) { 1768 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.diameter"); 1769 } else if (name.equals("duration")) { 1770 this.duration = new Quantity(); 1771 return this.duration; 1772 } else if (name.equals("color")) { 1773 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.color"); 1774 } else if (name.equals("brand")) { 1775 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.brand"); 1776 } else if (name.equals("note")) { 1777 return addNote(); 1778 } else 1779 return super.addChild(name); 1780 } 1781 1782 public VisionPrescriptionLensSpecificationComponent copy() { 1783 VisionPrescriptionLensSpecificationComponent dst = new VisionPrescriptionLensSpecificationComponent(); 1784 copyValues(dst); 1785 return dst; 1786 } 1787 1788 public void copyValues(VisionPrescriptionLensSpecificationComponent dst) { 1789 super.copyValues(dst); 1790 dst.product = product == null ? null : product.copy(); 1791 dst.eye = eye == null ? null : eye.copy(); 1792 dst.sphere = sphere == null ? null : sphere.copy(); 1793 dst.cylinder = cylinder == null ? null : cylinder.copy(); 1794 dst.axis = axis == null ? null : axis.copy(); 1795 if (prism != null) { 1796 dst.prism = new ArrayList<PrismComponent>(); 1797 for (PrismComponent i : prism) 1798 dst.prism.add(i.copy()); 1799 } 1800 ; 1801 dst.add = add == null ? null : add.copy(); 1802 dst.power = power == null ? null : power.copy(); 1803 dst.backCurve = backCurve == null ? null : backCurve.copy(); 1804 dst.diameter = diameter == null ? null : diameter.copy(); 1805 dst.duration = duration == null ? null : duration.copy(); 1806 dst.color = color == null ? null : color.copy(); 1807 dst.brand = brand == null ? null : brand.copy(); 1808 if (note != null) { 1809 dst.note = new ArrayList<Annotation>(); 1810 for (Annotation i : note) 1811 dst.note.add(i.copy()); 1812 } 1813 ; 1814 } 1815 1816 @Override 1817 public boolean equalsDeep(Base other_) { 1818 if (!super.equalsDeep(other_)) 1819 return false; 1820 if (!(other_ instanceof VisionPrescriptionLensSpecificationComponent)) 1821 return false; 1822 VisionPrescriptionLensSpecificationComponent o = (VisionPrescriptionLensSpecificationComponent) other_; 1823 return compareDeep(product, o.product, true) && compareDeep(eye, o.eye, true) 1824 && compareDeep(sphere, o.sphere, true) && compareDeep(cylinder, o.cylinder, true) 1825 && compareDeep(axis, o.axis, true) && compareDeep(prism, o.prism, true) && compareDeep(add, o.add, true) 1826 && compareDeep(power, o.power, true) && compareDeep(backCurve, o.backCurve, true) 1827 && compareDeep(diameter, o.diameter, true) && compareDeep(duration, o.duration, true) 1828 && compareDeep(color, o.color, true) && compareDeep(brand, o.brand, true) && compareDeep(note, o.note, true); 1829 } 1830 1831 @Override 1832 public boolean equalsShallow(Base other_) { 1833 if (!super.equalsShallow(other_)) 1834 return false; 1835 if (!(other_ instanceof VisionPrescriptionLensSpecificationComponent)) 1836 return false; 1837 VisionPrescriptionLensSpecificationComponent o = (VisionPrescriptionLensSpecificationComponent) other_; 1838 return compareValues(eye, o.eye, true) && compareValues(sphere, o.sphere, true) 1839 && compareValues(cylinder, o.cylinder, true) && compareValues(axis, o.axis, true) 1840 && compareValues(add, o.add, true) && compareValues(power, o.power, true) 1841 && compareValues(backCurve, o.backCurve, true) && compareValues(diameter, o.diameter, true) 1842 && compareValues(color, o.color, true) && compareValues(brand, o.brand, true); 1843 } 1844 1845 public boolean isEmpty() { 1846 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(product, eye, sphere, cylinder, axis, prism, add, 1847 power, backCurve, diameter, duration, color, brand, note); 1848 } 1849 1850 public String fhirType() { 1851 return "VisionPrescription.lensSpecification"; 1852 1853 } 1854 1855 } 1856 1857 @Block() 1858 public static class PrismComponent extends BackboneElement implements IBaseBackboneElement { 1859 /** 1860 * Amount of prism to compensate for eye alignment in fractional units. 1861 */ 1862 @Child(name = "amount", type = { 1863 DecimalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1864 @Description(shortDefinition = "Amount of adjustment", formalDefinition = "Amount of prism to compensate for eye alignment in fractional units.") 1865 protected DecimalType amount; 1866 1867 /** 1868 * The relative base, or reference lens edge, for the prism. 1869 */ 1870 @Child(name = "base", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1871 @Description(shortDefinition = "up | down | in | out", formalDefinition = "The relative base, or reference lens edge, for the prism.") 1872 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vision-base-codes") 1873 protected Enumeration<VisionBase> base; 1874 1875 private static final long serialVersionUID = 1677247628L; 1876 1877 /** 1878 * Constructor 1879 */ 1880 public PrismComponent() { 1881 super(); 1882 } 1883 1884 /** 1885 * Constructor 1886 */ 1887 public PrismComponent(DecimalType amount, Enumeration<VisionBase> base) { 1888 super(); 1889 this.amount = amount; 1890 this.base = base; 1891 } 1892 1893 /** 1894 * @return {@link #amount} (Amount of prism to compensate for eye alignment in 1895 * fractional units.). This is the underlying object with id, value and 1896 * extensions. The accessor "getAmount" gives direct access to the value 1897 */ 1898 public DecimalType getAmountElement() { 1899 if (this.amount == null) 1900 if (Configuration.errorOnAutoCreate()) 1901 throw new Error("Attempt to auto-create PrismComponent.amount"); 1902 else if (Configuration.doAutoCreate()) 1903 this.amount = new DecimalType(); // bb 1904 return this.amount; 1905 } 1906 1907 public boolean hasAmountElement() { 1908 return this.amount != null && !this.amount.isEmpty(); 1909 } 1910 1911 public boolean hasAmount() { 1912 return this.amount != null && !this.amount.isEmpty(); 1913 } 1914 1915 /** 1916 * @param value {@link #amount} (Amount of prism to compensate for eye alignment 1917 * in fractional units.). This is the underlying object with id, 1918 * value and extensions. The accessor "getAmount" gives direct 1919 * access to the value 1920 */ 1921 public PrismComponent setAmountElement(DecimalType value) { 1922 this.amount = value; 1923 return this; 1924 } 1925 1926 /** 1927 * @return Amount of prism to compensate for eye alignment in fractional units. 1928 */ 1929 public BigDecimal getAmount() { 1930 return this.amount == null ? null : this.amount.getValue(); 1931 } 1932 1933 /** 1934 * @param value Amount of prism to compensate for eye alignment in fractional 1935 * units. 1936 */ 1937 public PrismComponent setAmount(BigDecimal value) { 1938 if (this.amount == null) 1939 this.amount = new DecimalType(); 1940 this.amount.setValue(value); 1941 return this; 1942 } 1943 1944 /** 1945 * @param value Amount of prism to compensate for eye alignment in fractional 1946 * units. 1947 */ 1948 public PrismComponent setAmount(long value) { 1949 this.amount = new DecimalType(); 1950 this.amount.setValue(value); 1951 return this; 1952 } 1953 1954 /** 1955 * @param value Amount of prism to compensate for eye alignment in fractional 1956 * units. 1957 */ 1958 public PrismComponent setAmount(double value) { 1959 this.amount = new DecimalType(); 1960 this.amount.setValue(value); 1961 return this; 1962 } 1963 1964 /** 1965 * @return {@link #base} (The relative base, or reference lens edge, for the 1966 * prism.). This is the underlying object with id, value and extensions. 1967 * The accessor "getBase" gives direct access to the value 1968 */ 1969 public Enumeration<VisionBase> getBaseElement() { 1970 if (this.base == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create PrismComponent.base"); 1973 else if (Configuration.doAutoCreate()) 1974 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); // bb 1975 return this.base; 1976 } 1977 1978 public boolean hasBaseElement() { 1979 return this.base != null && !this.base.isEmpty(); 1980 } 1981 1982 public boolean hasBase() { 1983 return this.base != null && !this.base.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #base} (The relative base, or reference lens edge, for 1988 * the prism.). This is the underlying object with id, value and 1989 * extensions. The accessor "getBase" gives direct access to the 1990 * value 1991 */ 1992 public PrismComponent setBaseElement(Enumeration<VisionBase> value) { 1993 this.base = value; 1994 return this; 1995 } 1996 1997 /** 1998 * @return The relative base, or reference lens edge, for the prism. 1999 */ 2000 public VisionBase getBase() { 2001 return this.base == null ? null : this.base.getValue(); 2002 } 2003 2004 /** 2005 * @param value The relative base, or reference lens edge, for the prism. 2006 */ 2007 public PrismComponent setBase(VisionBase value) { 2008 if (this.base == null) 2009 this.base = new Enumeration<VisionBase>(new VisionBaseEnumFactory()); 2010 this.base.setValue(value); 2011 return this; 2012 } 2013 2014 protected void listChildren(List<Property> children) { 2015 super.listChildren(children); 2016 children.add(new Property("amount", "decimal", 2017 "Amount of prism to compensate for eye alignment in fractional units.", 0, 1, amount)); 2018 children 2019 .add(new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 1, base)); 2020 } 2021 2022 @Override 2023 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2024 switch (_hash) { 2025 case -1413853096: 2026 /* amount */ return new Property("amount", "decimal", 2027 "Amount of prism to compensate for eye alignment in fractional units.", 0, 1, amount); 2028 case 3016401: 2029 /* base */ return new Property("base", "code", "The relative base, or reference lens edge, for the prism.", 0, 2030 1, base); 2031 default: 2032 return super.getNamedProperty(_hash, _name, _checkValid); 2033 } 2034 2035 } 2036 2037 @Override 2038 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2039 switch (hash) { 2040 case -1413853096: 2041 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // DecimalType 2042 case 3016401: 2043 /* base */ return this.base == null ? new Base[0] : new Base[] { this.base }; // Enumeration<VisionBase> 2044 default: 2045 return super.getProperty(hash, name, checkValid); 2046 } 2047 2048 } 2049 2050 @Override 2051 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2052 switch (hash) { 2053 case -1413853096: // amount 2054 this.amount = castToDecimal(value); // DecimalType 2055 return value; 2056 case 3016401: // base 2057 value = new VisionBaseEnumFactory().fromType(castToCode(value)); 2058 this.base = (Enumeration) value; // Enumeration<VisionBase> 2059 return value; 2060 default: 2061 return super.setProperty(hash, name, value); 2062 } 2063 2064 } 2065 2066 @Override 2067 public Base setProperty(String name, Base value) throws FHIRException { 2068 if (name.equals("amount")) { 2069 this.amount = castToDecimal(value); // DecimalType 2070 } else if (name.equals("base")) { 2071 value = new VisionBaseEnumFactory().fromType(castToCode(value)); 2072 this.base = (Enumeration) value; // Enumeration<VisionBase> 2073 } else 2074 return super.setProperty(name, value); 2075 return value; 2076 } 2077 2078 @Override 2079 public void removeChild(String name, Base value) throws FHIRException { 2080 if (name.equals("amount")) { 2081 this.amount = null; 2082 } else if (name.equals("base")) { 2083 this.base = null; 2084 } else 2085 super.removeChild(name, value); 2086 2087 } 2088 2089 @Override 2090 public Base makeProperty(int hash, String name) throws FHIRException { 2091 switch (hash) { 2092 case -1413853096: 2093 return getAmountElement(); 2094 case 3016401: 2095 return getBaseElement(); 2096 default: 2097 return super.makeProperty(hash, name); 2098 } 2099 2100 } 2101 2102 @Override 2103 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2104 switch (hash) { 2105 case -1413853096: 2106 /* amount */ return new String[] { "decimal" }; 2107 case 3016401: 2108 /* base */ return new String[] { "code" }; 2109 default: 2110 return super.getTypesForProperty(hash, name); 2111 } 2112 2113 } 2114 2115 @Override 2116 public Base addChild(String name) throws FHIRException { 2117 if (name.equals("amount")) { 2118 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.amount"); 2119 } else if (name.equals("base")) { 2120 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.base"); 2121 } else 2122 return super.addChild(name); 2123 } 2124 2125 public PrismComponent copy() { 2126 PrismComponent dst = new PrismComponent(); 2127 copyValues(dst); 2128 return dst; 2129 } 2130 2131 public void copyValues(PrismComponent dst) { 2132 super.copyValues(dst); 2133 dst.amount = amount == null ? null : amount.copy(); 2134 dst.base = base == null ? null : base.copy(); 2135 } 2136 2137 @Override 2138 public boolean equalsDeep(Base other_) { 2139 if (!super.equalsDeep(other_)) 2140 return false; 2141 if (!(other_ instanceof PrismComponent)) 2142 return false; 2143 PrismComponent o = (PrismComponent) other_; 2144 return compareDeep(amount, o.amount, true) && compareDeep(base, o.base, true); 2145 } 2146 2147 @Override 2148 public boolean equalsShallow(Base other_) { 2149 if (!super.equalsShallow(other_)) 2150 return false; 2151 if (!(other_ instanceof PrismComponent)) 2152 return false; 2153 PrismComponent o = (PrismComponent) other_; 2154 return compareValues(amount, o.amount, true) && compareValues(base, o.base, true); 2155 } 2156 2157 public boolean isEmpty() { 2158 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(amount, base); 2159 } 2160 2161 public String fhirType() { 2162 return "VisionPrescription.lensSpecification.prism"; 2163 2164 } 2165 2166 } 2167 2168 /** 2169 * A unique identifier assigned to this vision prescription. 2170 */ 2171 @Child(name = "identifier", type = { 2172 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2173 @Description(shortDefinition = "Business Identifier for vision prescription", formalDefinition = "A unique identifier assigned to this vision prescription.") 2174 protected List<Identifier> identifier; 2175 2176 /** 2177 * The status of the resource instance. 2178 */ 2179 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2180 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 2181 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 2182 protected Enumeration<VisionStatus> status; 2183 2184 /** 2185 * The date this resource was created. 2186 */ 2187 @Child(name = "created", type = { DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2188 @Description(shortDefinition = "Response creation date", formalDefinition = "The date this resource was created.") 2189 protected DateTimeType created; 2190 2191 /** 2192 * A resource reference to the person to whom the vision prescription applies. 2193 */ 2194 @Child(name = "patient", type = { Patient.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2195 @Description(shortDefinition = "Who prescription is for", formalDefinition = "A resource reference to the person to whom the vision prescription applies.") 2196 protected Reference patient; 2197 2198 /** 2199 * The actual object that is the target of the reference (A resource reference 2200 * to the person to whom the vision prescription applies.) 2201 */ 2202 protected Patient patientTarget; 2203 2204 /** 2205 * A reference to a resource that identifies the particular occurrence of 2206 * contact between patient and health care provider during which the 2207 * prescription was issued. 2208 */ 2209 @Child(name = "encounter", type = { Encounter.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2210 @Description(shortDefinition = "Created during encounter / admission / stay", formalDefinition = "A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.") 2211 protected Reference encounter; 2212 2213 /** 2214 * The actual object that is the target of the reference (A reference to a 2215 * resource that identifies the particular occurrence of contact between patient 2216 * and health care provider during which the prescription was issued.) 2217 */ 2218 protected Encounter encounterTarget; 2219 2220 /** 2221 * The date (and perhaps time) when the prescription was written. 2222 */ 2223 @Child(name = "dateWritten", type = { 2224 DateTimeType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 2225 @Description(shortDefinition = "When prescription was authorized", formalDefinition = "The date (and perhaps time) when the prescription was written.") 2226 protected DateTimeType dateWritten; 2227 2228 /** 2229 * The healthcare professional responsible for authorizing the prescription. 2230 */ 2231 @Child(name = "prescriber", type = { Practitioner.class, 2232 PractitionerRole.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 2233 @Description(shortDefinition = "Who authorized the vision prescription", formalDefinition = "The healthcare professional responsible for authorizing the prescription.") 2234 protected Reference prescriber; 2235 2236 /** 2237 * The actual object that is the target of the reference (The healthcare 2238 * professional responsible for authorizing the prescription.) 2239 */ 2240 protected Resource prescriberTarget; 2241 2242 /** 2243 * Contain the details of the individual lens specifications and serves as the 2244 * authorization for the fullfillment by certified professionals. 2245 */ 2246 @Child(name = "lensSpecification", type = {}, order = 7, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2247 @Description(shortDefinition = "Vision lens authorization", formalDefinition = "Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.") 2248 protected List<VisionPrescriptionLensSpecificationComponent> lensSpecification; 2249 2250 private static final long serialVersionUID = 988021071L; 2251 2252 /** 2253 * Constructor 2254 */ 2255 public VisionPrescription() { 2256 super(); 2257 } 2258 2259 /** 2260 * Constructor 2261 */ 2262 public VisionPrescription(Enumeration<VisionStatus> status, DateTimeType created, Reference patient, 2263 DateTimeType dateWritten, Reference prescriber) { 2264 super(); 2265 this.status = status; 2266 this.created = created; 2267 this.patient = patient; 2268 this.dateWritten = dateWritten; 2269 this.prescriber = prescriber; 2270 } 2271 2272 /** 2273 * @return {@link #identifier} (A unique identifier assigned to this vision 2274 * prescription.) 2275 */ 2276 public List<Identifier> getIdentifier() { 2277 if (this.identifier == null) 2278 this.identifier = new ArrayList<Identifier>(); 2279 return this.identifier; 2280 } 2281 2282 /** 2283 * @return Returns a reference to <code>this</code> for easy method chaining 2284 */ 2285 public VisionPrescription setIdentifier(List<Identifier> theIdentifier) { 2286 this.identifier = theIdentifier; 2287 return this; 2288 } 2289 2290 public boolean hasIdentifier() { 2291 if (this.identifier == null) 2292 return false; 2293 for (Identifier item : this.identifier) 2294 if (!item.isEmpty()) 2295 return true; 2296 return false; 2297 } 2298 2299 public Identifier addIdentifier() { // 3 2300 Identifier t = new Identifier(); 2301 if (this.identifier == null) 2302 this.identifier = new ArrayList<Identifier>(); 2303 this.identifier.add(t); 2304 return t; 2305 } 2306 2307 public VisionPrescription addIdentifier(Identifier t) { // 3 2308 if (t == null) 2309 return this; 2310 if (this.identifier == null) 2311 this.identifier = new ArrayList<Identifier>(); 2312 this.identifier.add(t); 2313 return this; 2314 } 2315 2316 /** 2317 * @return The first repetition of repeating field {@link #identifier}, creating 2318 * it if it does not already exist 2319 */ 2320 public Identifier getIdentifierFirstRep() { 2321 if (getIdentifier().isEmpty()) { 2322 addIdentifier(); 2323 } 2324 return getIdentifier().get(0); 2325 } 2326 2327 /** 2328 * @return {@link #status} (The status of the resource instance.). This is the 2329 * underlying object with id, value and extensions. The accessor 2330 * "getStatus" gives direct access to the value 2331 */ 2332 public Enumeration<VisionStatus> getStatusElement() { 2333 if (this.status == null) 2334 if (Configuration.errorOnAutoCreate()) 2335 throw new Error("Attempt to auto-create VisionPrescription.status"); 2336 else if (Configuration.doAutoCreate()) 2337 this.status = new Enumeration<VisionStatus>(new VisionStatusEnumFactory()); // bb 2338 return this.status; 2339 } 2340 2341 public boolean hasStatusElement() { 2342 return this.status != null && !this.status.isEmpty(); 2343 } 2344 2345 public boolean hasStatus() { 2346 return this.status != null && !this.status.isEmpty(); 2347 } 2348 2349 /** 2350 * @param value {@link #status} (The status of the resource instance.). This is 2351 * the underlying object with id, value and extensions. The 2352 * accessor "getStatus" gives direct access to the value 2353 */ 2354 public VisionPrescription setStatusElement(Enumeration<VisionStatus> value) { 2355 this.status = value; 2356 return this; 2357 } 2358 2359 /** 2360 * @return The status of the resource instance. 2361 */ 2362 public VisionStatus getStatus() { 2363 return this.status == null ? null : this.status.getValue(); 2364 } 2365 2366 /** 2367 * @param value The status of the resource instance. 2368 */ 2369 public VisionPrescription setStatus(VisionStatus value) { 2370 if (this.status == null) 2371 this.status = new Enumeration<VisionStatus>(new VisionStatusEnumFactory()); 2372 this.status.setValue(value); 2373 return this; 2374 } 2375 2376 /** 2377 * @return {@link #created} (The date this resource was created.). This is the 2378 * underlying object with id, value and extensions. The accessor 2379 * "getCreated" gives direct access to the value 2380 */ 2381 public DateTimeType getCreatedElement() { 2382 if (this.created == null) 2383 if (Configuration.errorOnAutoCreate()) 2384 throw new Error("Attempt to auto-create VisionPrescription.created"); 2385 else if (Configuration.doAutoCreate()) 2386 this.created = new DateTimeType(); // bb 2387 return this.created; 2388 } 2389 2390 public boolean hasCreatedElement() { 2391 return this.created != null && !this.created.isEmpty(); 2392 } 2393 2394 public boolean hasCreated() { 2395 return this.created != null && !this.created.isEmpty(); 2396 } 2397 2398 /** 2399 * @param value {@link #created} (The date this resource was created.). This is 2400 * the underlying object with id, value and extensions. The 2401 * accessor "getCreated" gives direct access to the value 2402 */ 2403 public VisionPrescription setCreatedElement(DateTimeType value) { 2404 this.created = value; 2405 return this; 2406 } 2407 2408 /** 2409 * @return The date this resource was created. 2410 */ 2411 public Date getCreated() { 2412 return this.created == null ? null : this.created.getValue(); 2413 } 2414 2415 /** 2416 * @param value The date this resource was created. 2417 */ 2418 public VisionPrescription setCreated(Date value) { 2419 if (this.created == null) 2420 this.created = new DateTimeType(); 2421 this.created.setValue(value); 2422 return this; 2423 } 2424 2425 /** 2426 * @return {@link #patient} (A resource reference to the person to whom the 2427 * vision prescription applies.) 2428 */ 2429 public Reference getPatient() { 2430 if (this.patient == null) 2431 if (Configuration.errorOnAutoCreate()) 2432 throw new Error("Attempt to auto-create VisionPrescription.patient"); 2433 else if (Configuration.doAutoCreate()) 2434 this.patient = new Reference(); // cc 2435 return this.patient; 2436 } 2437 2438 public boolean hasPatient() { 2439 return this.patient != null && !this.patient.isEmpty(); 2440 } 2441 2442 /** 2443 * @param value {@link #patient} (A resource reference to the person to whom the 2444 * vision prescription applies.) 2445 */ 2446 public VisionPrescription setPatient(Reference value) { 2447 this.patient = value; 2448 return this; 2449 } 2450 2451 /** 2452 * @return {@link #patient} The actual object that is the target of the 2453 * reference. The reference library doesn't populate this, but you can 2454 * use it to hold the resource if you resolve it. (A resource reference 2455 * to the person to whom the vision prescription applies.) 2456 */ 2457 public Patient getPatientTarget() { 2458 if (this.patientTarget == null) 2459 if (Configuration.errorOnAutoCreate()) 2460 throw new Error("Attempt to auto-create VisionPrescription.patient"); 2461 else if (Configuration.doAutoCreate()) 2462 this.patientTarget = new Patient(); // aa 2463 return this.patientTarget; 2464 } 2465 2466 /** 2467 * @param value {@link #patient} The actual object that is the target of the 2468 * reference. The reference library doesn't use these, but you can 2469 * use it to hold the resource if you resolve it. (A resource 2470 * reference to the person to whom the vision prescription 2471 * applies.) 2472 */ 2473 public VisionPrescription setPatientTarget(Patient value) { 2474 this.patientTarget = value; 2475 return this; 2476 } 2477 2478 /** 2479 * @return {@link #encounter} (A reference to a resource that identifies the 2480 * particular occurrence of contact between patient and health care 2481 * provider during which the prescription was issued.) 2482 */ 2483 public Reference getEncounter() { 2484 if (this.encounter == null) 2485 if (Configuration.errorOnAutoCreate()) 2486 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 2487 else if (Configuration.doAutoCreate()) 2488 this.encounter = new Reference(); // cc 2489 return this.encounter; 2490 } 2491 2492 public boolean hasEncounter() { 2493 return this.encounter != null && !this.encounter.isEmpty(); 2494 } 2495 2496 /** 2497 * @param value {@link #encounter} (A reference to a resource that identifies 2498 * the particular occurrence of contact between patient and health 2499 * care provider during which the prescription was issued.) 2500 */ 2501 public VisionPrescription setEncounter(Reference value) { 2502 this.encounter = value; 2503 return this; 2504 } 2505 2506 /** 2507 * @return {@link #encounter} The actual object that is the target of the 2508 * reference. The reference library doesn't populate this, but you can 2509 * use it to hold the resource if you resolve it. (A reference to a 2510 * resource that identifies the particular occurrence of contact between 2511 * patient and health care provider during which the prescription was 2512 * issued.) 2513 */ 2514 public Encounter getEncounterTarget() { 2515 if (this.encounterTarget == null) 2516 if (Configuration.errorOnAutoCreate()) 2517 throw new Error("Attempt to auto-create VisionPrescription.encounter"); 2518 else if (Configuration.doAutoCreate()) 2519 this.encounterTarget = new Encounter(); // aa 2520 return this.encounterTarget; 2521 } 2522 2523 /** 2524 * @param value {@link #encounter} The actual object that is the target of the 2525 * reference. The reference library doesn't use these, but you can 2526 * use it to hold the resource if you resolve it. (A reference to a 2527 * resource that identifies the particular occurrence of contact 2528 * between patient and health care provider during which the 2529 * prescription was issued.) 2530 */ 2531 public VisionPrescription setEncounterTarget(Encounter value) { 2532 this.encounterTarget = value; 2533 return this; 2534 } 2535 2536 /** 2537 * @return {@link #dateWritten} (The date (and perhaps time) when the 2538 * prescription was written.). This is the underlying object with id, 2539 * value and extensions. The accessor "getDateWritten" gives direct 2540 * access to the value 2541 */ 2542 public DateTimeType getDateWrittenElement() { 2543 if (this.dateWritten == null) 2544 if (Configuration.errorOnAutoCreate()) 2545 throw new Error("Attempt to auto-create VisionPrescription.dateWritten"); 2546 else if (Configuration.doAutoCreate()) 2547 this.dateWritten = new DateTimeType(); // bb 2548 return this.dateWritten; 2549 } 2550 2551 public boolean hasDateWrittenElement() { 2552 return this.dateWritten != null && !this.dateWritten.isEmpty(); 2553 } 2554 2555 public boolean hasDateWritten() { 2556 return this.dateWritten != null && !this.dateWritten.isEmpty(); 2557 } 2558 2559 /** 2560 * @param value {@link #dateWritten} (The date (and perhaps time) when the 2561 * prescription was written.). This is the underlying object with 2562 * id, value and extensions. The accessor "getDateWritten" gives 2563 * direct access to the value 2564 */ 2565 public VisionPrescription setDateWrittenElement(DateTimeType value) { 2566 this.dateWritten = value; 2567 return this; 2568 } 2569 2570 /** 2571 * @return The date (and perhaps time) when the prescription was written. 2572 */ 2573 public Date getDateWritten() { 2574 return this.dateWritten == null ? null : this.dateWritten.getValue(); 2575 } 2576 2577 /** 2578 * @param value The date (and perhaps time) when the prescription was written. 2579 */ 2580 public VisionPrescription setDateWritten(Date value) { 2581 if (this.dateWritten == null) 2582 this.dateWritten = new DateTimeType(); 2583 this.dateWritten.setValue(value); 2584 return this; 2585 } 2586 2587 /** 2588 * @return {@link #prescriber} (The healthcare professional responsible for 2589 * authorizing the prescription.) 2590 */ 2591 public Reference getPrescriber() { 2592 if (this.prescriber == null) 2593 if (Configuration.errorOnAutoCreate()) 2594 throw new Error("Attempt to auto-create VisionPrescription.prescriber"); 2595 else if (Configuration.doAutoCreate()) 2596 this.prescriber = new Reference(); // cc 2597 return this.prescriber; 2598 } 2599 2600 public boolean hasPrescriber() { 2601 return this.prescriber != null && !this.prescriber.isEmpty(); 2602 } 2603 2604 /** 2605 * @param value {@link #prescriber} (The healthcare professional responsible for 2606 * authorizing the prescription.) 2607 */ 2608 public VisionPrescription setPrescriber(Reference value) { 2609 this.prescriber = value; 2610 return this; 2611 } 2612 2613 /** 2614 * @return {@link #prescriber} The actual object that is the target of the 2615 * reference. The reference library doesn't populate this, but you can 2616 * use it to hold the resource if you resolve it. (The healthcare 2617 * professional responsible for authorizing the prescription.) 2618 */ 2619 public Resource getPrescriberTarget() { 2620 return this.prescriberTarget; 2621 } 2622 2623 /** 2624 * @param value {@link #prescriber} The actual object that is the target of the 2625 * reference. The reference library doesn't use these, but you can 2626 * use it to hold the resource if you resolve it. (The healthcare 2627 * professional responsible for authorizing the prescription.) 2628 */ 2629 public VisionPrescription setPrescriberTarget(Resource value) { 2630 this.prescriberTarget = value; 2631 return this; 2632 } 2633 2634 /** 2635 * @return {@link #lensSpecification} (Contain the details of the individual 2636 * lens specifications and serves as the authorization for the 2637 * fullfillment by certified professionals.) 2638 */ 2639 public List<VisionPrescriptionLensSpecificationComponent> getLensSpecification() { 2640 if (this.lensSpecification == null) 2641 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2642 return this.lensSpecification; 2643 } 2644 2645 /** 2646 * @return Returns a reference to <code>this</code> for easy method chaining 2647 */ 2648 public VisionPrescription setLensSpecification( 2649 List<VisionPrescriptionLensSpecificationComponent> theLensSpecification) { 2650 this.lensSpecification = theLensSpecification; 2651 return this; 2652 } 2653 2654 public boolean hasLensSpecification() { 2655 if (this.lensSpecification == null) 2656 return false; 2657 for (VisionPrescriptionLensSpecificationComponent item : this.lensSpecification) 2658 if (!item.isEmpty()) 2659 return true; 2660 return false; 2661 } 2662 2663 public VisionPrescriptionLensSpecificationComponent addLensSpecification() { // 3 2664 VisionPrescriptionLensSpecificationComponent t = new VisionPrescriptionLensSpecificationComponent(); 2665 if (this.lensSpecification == null) 2666 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2667 this.lensSpecification.add(t); 2668 return t; 2669 } 2670 2671 public VisionPrescription addLensSpecification(VisionPrescriptionLensSpecificationComponent t) { // 3 2672 if (t == null) 2673 return this; 2674 if (this.lensSpecification == null) 2675 this.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2676 this.lensSpecification.add(t); 2677 return this; 2678 } 2679 2680 /** 2681 * @return The first repetition of repeating field {@link #lensSpecification}, 2682 * creating it if it does not already exist 2683 */ 2684 public VisionPrescriptionLensSpecificationComponent getLensSpecificationFirstRep() { 2685 if (getLensSpecification().isEmpty()) { 2686 addLensSpecification(); 2687 } 2688 return getLensSpecification().get(0); 2689 } 2690 2691 protected void listChildren(List<Property> children) { 2692 super.listChildren(children); 2693 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this vision prescription.", 2694 0, java.lang.Integer.MAX_VALUE, identifier)); 2695 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2696 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 2697 children.add(new Property("patient", "Reference(Patient)", 2698 "A resource reference to the person to whom the vision prescription applies.", 0, 1, patient)); 2699 children.add(new Property("encounter", "Reference(Encounter)", 2700 "A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.", 2701 0, 1, encounter)); 2702 children.add(new Property("dateWritten", "dateTime", 2703 "The date (and perhaps time) when the prescription was written.", 0, 1, dateWritten)); 2704 children.add(new Property("prescriber", "Reference(Practitioner|PractitionerRole)", 2705 "The healthcare professional responsible for authorizing the prescription.", 0, 1, prescriber)); 2706 children.add(new Property("lensSpecification", "", 2707 "Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.", 2708 0, java.lang.Integer.MAX_VALUE, lensSpecification)); 2709 } 2710 2711 @Override 2712 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2713 switch (_hash) { 2714 case -1618432855: 2715 /* identifier */ return new Property("identifier", "Identifier", 2716 "A unique identifier assigned to this vision prescription.", 0, java.lang.Integer.MAX_VALUE, identifier); 2717 case -892481550: 2718 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2719 case 1028554472: 2720 /* created */ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 2721 case -791418107: 2722 /* patient */ return new Property("patient", "Reference(Patient)", 2723 "A resource reference to the person to whom the vision prescription applies.", 0, 1, patient); 2724 case 1524132147: 2725 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2726 "A reference to a resource that identifies the particular occurrence of contact between patient and health care provider during which the prescription was issued.", 2727 0, 1, encounter); 2728 case -1496880759: 2729 /* dateWritten */ return new Property("dateWritten", "dateTime", 2730 "The date (and perhaps time) when the prescription was written.", 0, 1, dateWritten); 2731 case 1430631077: 2732 /* prescriber */ return new Property("prescriber", "Reference(Practitioner|PractitionerRole)", 2733 "The healthcare professional responsible for authorizing the prescription.", 0, 1, prescriber); 2734 case -1767318363: 2735 /* lensSpecification */ return new Property("lensSpecification", "", 2736 "Contain the details of the individual lens specifications and serves as the authorization for the fullfillment by certified professionals.", 2737 0, java.lang.Integer.MAX_VALUE, lensSpecification); 2738 default: 2739 return super.getNamedProperty(_hash, _name, _checkValid); 2740 } 2741 2742 } 2743 2744 @Override 2745 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2746 switch (hash) { 2747 case -1618432855: 2748 /* identifier */ return this.identifier == null ? new Base[0] 2749 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2750 case -892481550: 2751 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<VisionStatus> 2752 case 1028554472: 2753 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 2754 case -791418107: 2755 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 2756 case 1524132147: 2757 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2758 case -1496880759: 2759 /* dateWritten */ return this.dateWritten == null ? new Base[0] : new Base[] { this.dateWritten }; // DateTimeType 2760 case 1430631077: 2761 /* prescriber */ return this.prescriber == null ? new Base[0] : new Base[] { this.prescriber }; // Reference 2762 case -1767318363: 2763 /* lensSpecification */ return this.lensSpecification == null ? new Base[0] 2764 : this.lensSpecification.toArray(new Base[this.lensSpecification.size()]); // VisionPrescriptionLensSpecificationComponent 2765 default: 2766 return super.getProperty(hash, name, checkValid); 2767 } 2768 2769 } 2770 2771 @Override 2772 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2773 switch (hash) { 2774 case -1618432855: // identifier 2775 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2776 return value; 2777 case -892481550: // status 2778 value = new VisionStatusEnumFactory().fromType(castToCode(value)); 2779 this.status = (Enumeration) value; // Enumeration<VisionStatus> 2780 return value; 2781 case 1028554472: // created 2782 this.created = castToDateTime(value); // DateTimeType 2783 return value; 2784 case -791418107: // patient 2785 this.patient = castToReference(value); // Reference 2786 return value; 2787 case 1524132147: // encounter 2788 this.encounter = castToReference(value); // Reference 2789 return value; 2790 case -1496880759: // dateWritten 2791 this.dateWritten = castToDateTime(value); // DateTimeType 2792 return value; 2793 case 1430631077: // prescriber 2794 this.prescriber = castToReference(value); // Reference 2795 return value; 2796 case -1767318363: // lensSpecification 2797 this.getLensSpecification().add((VisionPrescriptionLensSpecificationComponent) value); // VisionPrescriptionLensSpecificationComponent 2798 return value; 2799 default: 2800 return super.setProperty(hash, name, value); 2801 } 2802 2803 } 2804 2805 @Override 2806 public Base setProperty(String name, Base value) throws FHIRException { 2807 if (name.equals("identifier")) { 2808 this.getIdentifier().add(castToIdentifier(value)); 2809 } else if (name.equals("status")) { 2810 value = new VisionStatusEnumFactory().fromType(castToCode(value)); 2811 this.status = (Enumeration) value; // Enumeration<VisionStatus> 2812 } else if (name.equals("created")) { 2813 this.created = castToDateTime(value); // DateTimeType 2814 } else if (name.equals("patient")) { 2815 this.patient = castToReference(value); // Reference 2816 } else if (name.equals("encounter")) { 2817 this.encounter = castToReference(value); // Reference 2818 } else if (name.equals("dateWritten")) { 2819 this.dateWritten = castToDateTime(value); // DateTimeType 2820 } else if (name.equals("prescriber")) { 2821 this.prescriber = castToReference(value); // Reference 2822 } else if (name.equals("lensSpecification")) { 2823 this.getLensSpecification().add((VisionPrescriptionLensSpecificationComponent) value); 2824 } else 2825 return super.setProperty(name, value); 2826 return value; 2827 } 2828 2829 @Override 2830 public void removeChild(String name, Base value) throws FHIRException { 2831 if (name.equals("identifier")) { 2832 this.getIdentifier().remove(castToIdentifier(value)); 2833 } else if (name.equals("status")) { 2834 this.status = null; 2835 } else if (name.equals("created")) { 2836 this.created = null; 2837 } else if (name.equals("patient")) { 2838 this.patient = null; 2839 } else if (name.equals("encounter")) { 2840 this.encounter = null; 2841 } else if (name.equals("dateWritten")) { 2842 this.dateWritten = null; 2843 } else if (name.equals("prescriber")) { 2844 this.prescriber = null; 2845 } else if (name.equals("lensSpecification")) { 2846 this.getLensSpecification().remove((VisionPrescriptionLensSpecificationComponent) value); 2847 } else 2848 super.removeChild(name, value); 2849 2850 } 2851 2852 @Override 2853 public Base makeProperty(int hash, String name) throws FHIRException { 2854 switch (hash) { 2855 case -1618432855: 2856 return addIdentifier(); 2857 case -892481550: 2858 return getStatusElement(); 2859 case 1028554472: 2860 return getCreatedElement(); 2861 case -791418107: 2862 return getPatient(); 2863 case 1524132147: 2864 return getEncounter(); 2865 case -1496880759: 2866 return getDateWrittenElement(); 2867 case 1430631077: 2868 return getPrescriber(); 2869 case -1767318363: 2870 return addLensSpecification(); 2871 default: 2872 return super.makeProperty(hash, name); 2873 } 2874 2875 } 2876 2877 @Override 2878 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2879 switch (hash) { 2880 case -1618432855: 2881 /* identifier */ return new String[] { "Identifier" }; 2882 case -892481550: 2883 /* status */ return new String[] { "code" }; 2884 case 1028554472: 2885 /* created */ return new String[] { "dateTime" }; 2886 case -791418107: 2887 /* patient */ return new String[] { "Reference" }; 2888 case 1524132147: 2889 /* encounter */ return new String[] { "Reference" }; 2890 case -1496880759: 2891 /* dateWritten */ return new String[] { "dateTime" }; 2892 case 1430631077: 2893 /* prescriber */ return new String[] { "Reference" }; 2894 case -1767318363: 2895 /* lensSpecification */ return new String[] {}; 2896 default: 2897 return super.getTypesForProperty(hash, name); 2898 } 2899 2900 } 2901 2902 @Override 2903 public Base addChild(String name) throws FHIRException { 2904 if (name.equals("identifier")) { 2905 return addIdentifier(); 2906 } else if (name.equals("status")) { 2907 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.status"); 2908 } else if (name.equals("created")) { 2909 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.created"); 2910 } else if (name.equals("patient")) { 2911 this.patient = new Reference(); 2912 return this.patient; 2913 } else if (name.equals("encounter")) { 2914 this.encounter = new Reference(); 2915 return this.encounter; 2916 } else if (name.equals("dateWritten")) { 2917 throw new FHIRException("Cannot call addChild on a singleton property VisionPrescription.dateWritten"); 2918 } else if (name.equals("prescriber")) { 2919 this.prescriber = new Reference(); 2920 return this.prescriber; 2921 } else if (name.equals("lensSpecification")) { 2922 return addLensSpecification(); 2923 } else 2924 return super.addChild(name); 2925 } 2926 2927 public String fhirType() { 2928 return "VisionPrescription"; 2929 2930 } 2931 2932 public VisionPrescription copy() { 2933 VisionPrescription dst = new VisionPrescription(); 2934 copyValues(dst); 2935 return dst; 2936 } 2937 2938 public void copyValues(VisionPrescription dst) { 2939 super.copyValues(dst); 2940 if (identifier != null) { 2941 dst.identifier = new ArrayList<Identifier>(); 2942 for (Identifier i : identifier) 2943 dst.identifier.add(i.copy()); 2944 } 2945 ; 2946 dst.status = status == null ? null : status.copy(); 2947 dst.created = created == null ? null : created.copy(); 2948 dst.patient = patient == null ? null : patient.copy(); 2949 dst.encounter = encounter == null ? null : encounter.copy(); 2950 dst.dateWritten = dateWritten == null ? null : dateWritten.copy(); 2951 dst.prescriber = prescriber == null ? null : prescriber.copy(); 2952 if (lensSpecification != null) { 2953 dst.lensSpecification = new ArrayList<VisionPrescriptionLensSpecificationComponent>(); 2954 for (VisionPrescriptionLensSpecificationComponent i : lensSpecification) 2955 dst.lensSpecification.add(i.copy()); 2956 } 2957 ; 2958 } 2959 2960 protected VisionPrescription typedCopy() { 2961 return copy(); 2962 } 2963 2964 @Override 2965 public boolean equalsDeep(Base other_) { 2966 if (!super.equalsDeep(other_)) 2967 return false; 2968 if (!(other_ instanceof VisionPrescription)) 2969 return false; 2970 VisionPrescription o = (VisionPrescription) other_; 2971 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2972 && compareDeep(created, o.created, true) && compareDeep(patient, o.patient, true) 2973 && compareDeep(encounter, o.encounter, true) && compareDeep(dateWritten, o.dateWritten, true) 2974 && compareDeep(prescriber, o.prescriber, true) && compareDeep(lensSpecification, o.lensSpecification, true); 2975 } 2976 2977 @Override 2978 public boolean equalsShallow(Base other_) { 2979 if (!super.equalsShallow(other_)) 2980 return false; 2981 if (!(other_ instanceof VisionPrescription)) 2982 return false; 2983 VisionPrescription o = (VisionPrescription) other_; 2984 return compareValues(status, o.status, true) && compareValues(created, o.created, true) 2985 && compareValues(dateWritten, o.dateWritten, true); 2986 } 2987 2988 public boolean isEmpty() { 2989 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, created, patient, encounter, 2990 dateWritten, prescriber, lensSpecification); 2991 } 2992 2993 @Override 2994 public ResourceType getResourceType() { 2995 return ResourceType.VisionPrescription; 2996 } 2997 2998 /** 2999 * Search parameter: <b>prescriber</b> 3000 * <p> 3001 * Description: <b>Who authorized the vision prescription</b><br> 3002 * Type: <b>reference</b><br> 3003 * Path: <b>VisionPrescription.prescriber</b><br> 3004 * </p> 3005 */ 3006 @SearchParamDefinition(name = "prescriber", path = "VisionPrescription.prescriber", description = "Who authorized the vision prescription", type = "reference", providesMembershipIn = { 3007 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 3008 PractitionerRole.class }) 3009 public static final String SP_PRESCRIBER = "prescriber"; 3010 /** 3011 * <b>Fluent Client</b> search parameter constant for <b>prescriber</b> 3012 * <p> 3013 * Description: <b>Who authorized the vision prescription</b><br> 3014 * Type: <b>reference</b><br> 3015 * Path: <b>VisionPrescription.prescriber</b><br> 3016 * </p> 3017 */ 3018 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRESCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3019 SP_PRESCRIBER); 3020 3021 /** 3022 * Constant for fluent queries to be used to add include statements. Specifies 3023 * the path value of "<b>VisionPrescription:prescriber</b>". 3024 */ 3025 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRESCRIBER = new ca.uhn.fhir.model.api.Include( 3026 "VisionPrescription:prescriber").toLocked(); 3027 3028 /** 3029 * Search parameter: <b>identifier</b> 3030 * <p> 3031 * Description: <b>Return prescriptions with this external identifier</b><br> 3032 * Type: <b>token</b><br> 3033 * Path: <b>VisionPrescription.identifier</b><br> 3034 * </p> 3035 */ 3036 @SearchParamDefinition(name = "identifier", path = "VisionPrescription.identifier", description = "Return prescriptions with this external identifier", type = "token") 3037 public static final String SP_IDENTIFIER = "identifier"; 3038 /** 3039 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3040 * <p> 3041 * Description: <b>Return prescriptions with this external identifier</b><br> 3042 * Type: <b>token</b><br> 3043 * Path: <b>VisionPrescription.identifier</b><br> 3044 * </p> 3045 */ 3046 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3047 SP_IDENTIFIER); 3048 3049 /** 3050 * Search parameter: <b>patient</b> 3051 * <p> 3052 * Description: <b>The identity of a patient to list dispenses for</b><br> 3053 * Type: <b>reference</b><br> 3054 * Path: <b>VisionPrescription.patient</b><br> 3055 * </p> 3056 */ 3057 @SearchParamDefinition(name = "patient", path = "VisionPrescription.patient", description = "The identity of a patient to list dispenses for", type = "reference", providesMembershipIn = { 3058 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3059 public static final String SP_PATIENT = "patient"; 3060 /** 3061 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3062 * <p> 3063 * Description: <b>The identity of a patient to list dispenses for</b><br> 3064 * Type: <b>reference</b><br> 3065 * Path: <b>VisionPrescription.patient</b><br> 3066 * </p> 3067 */ 3068 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3069 SP_PATIENT); 3070 3071 /** 3072 * Constant for fluent queries to be used to add include statements. Specifies 3073 * the path value of "<b>VisionPrescription:patient</b>". 3074 */ 3075 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3076 "VisionPrescription:patient").toLocked(); 3077 3078 /** 3079 * Search parameter: <b>datewritten</b> 3080 * <p> 3081 * Description: <b>Return prescriptions written on this date</b><br> 3082 * Type: <b>date</b><br> 3083 * Path: <b>VisionPrescription.dateWritten</b><br> 3084 * </p> 3085 */ 3086 @SearchParamDefinition(name = "datewritten", path = "VisionPrescription.dateWritten", description = "Return prescriptions written on this date", type = "date") 3087 public static final String SP_DATEWRITTEN = "datewritten"; 3088 /** 3089 * <b>Fluent Client</b> search parameter constant for <b>datewritten</b> 3090 * <p> 3091 * Description: <b>Return prescriptions written on this date</b><br> 3092 * Type: <b>date</b><br> 3093 * Path: <b>VisionPrescription.dateWritten</b><br> 3094 * </p> 3095 */ 3096 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATEWRITTEN = new ca.uhn.fhir.rest.gclient.DateClientParam( 3097 SP_DATEWRITTEN); 3098 3099 /** 3100 * Search parameter: <b>encounter</b> 3101 * <p> 3102 * Description: <b>Return prescriptions with this encounter identifier</b><br> 3103 * Type: <b>reference</b><br> 3104 * Path: <b>VisionPrescription.encounter</b><br> 3105 * </p> 3106 */ 3107 @SearchParamDefinition(name = "encounter", path = "VisionPrescription.encounter", description = "Return prescriptions with this encounter identifier", type = "reference", providesMembershipIn = { 3108 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 3109 public static final String SP_ENCOUNTER = "encounter"; 3110 /** 3111 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3112 * <p> 3113 * Description: <b>Return prescriptions with this encounter identifier</b><br> 3114 * Type: <b>reference</b><br> 3115 * Path: <b>VisionPrescription.encounter</b><br> 3116 * </p> 3117 */ 3118 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3119 SP_ENCOUNTER); 3120 3121 /** 3122 * Constant for fluent queries to be used to add include statements. Specifies 3123 * the path value of "<b>VisionPrescription:encounter</b>". 3124 */ 3125 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3126 "VisionPrescription:encounter").toLocked(); 3127 3128 /** 3129 * Search parameter: <b>status</b> 3130 * <p> 3131 * Description: <b>The status of the vision prescription</b><br> 3132 * Type: <b>token</b><br> 3133 * Path: <b>VisionPrescription.status</b><br> 3134 * </p> 3135 */ 3136 @SearchParamDefinition(name = "status", path = "VisionPrescription.status", description = "The status of the vision prescription", type = "token") 3137 public static final String SP_STATUS = "status"; 3138 /** 3139 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3140 * <p> 3141 * Description: <b>The status of the vision prescription</b><br> 3142 * Type: <b>token</b><br> 3143 * Path: <b>VisionPrescription.status</b><br> 3144 * </p> 3145 */ 3146 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3147 SP_STATUS); 3148 3149}