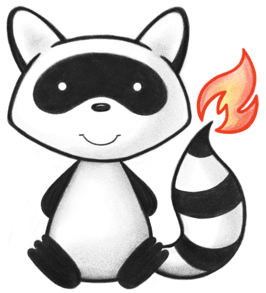
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ActionSelectionBehavior { 037 038 /** 039 * Any number of the actions in the group may be chosen, from zero to all. 040 */ 041 ANY, 042 /** 043 * All the actions in the group must be selected as a single unit. 044 */ 045 ALL, 046 /** 047 * All the actions in the group are meant to be chosen as a single unit: either 048 * all must be selected by the end user, or none may be selected. 049 */ 050 ALLORNONE, 051 /** 052 * The end user must choose one and only one of the selectable actions in the 053 * group. The user SHALL NOT choose none of the actions in the group. 054 */ 055 EXACTLYONE, 056 /** 057 * The end user may choose zero or at most one of the actions in the group. 058 */ 059 ATMOSTONE, 060 /** 061 * The end user must choose a minimum of one, and as many additional as desired. 062 */ 063 ONEORMORE, 064 /** 065 * added to help the parsers 066 */ 067 NULL; 068 069 public static ActionSelectionBehavior fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("any".equals(codeString)) 073 return ANY; 074 if ("all".equals(codeString)) 075 return ALL; 076 if ("all-or-none".equals(codeString)) 077 return ALLORNONE; 078 if ("exactly-one".equals(codeString)) 079 return EXACTLYONE; 080 if ("at-most-one".equals(codeString)) 081 return ATMOSTONE; 082 if ("one-or-more".equals(codeString)) 083 return ONEORMORE; 084 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case ANY: 090 return "any"; 091 case ALL: 092 return "all"; 093 case ALLORNONE: 094 return "all-or-none"; 095 case EXACTLYONE: 096 return "exactly-one"; 097 case ATMOSTONE: 098 return "at-most-one"; 099 case ONEORMORE: 100 return "one-or-more"; 101 case NULL: 102 return null; 103 default: 104 return "?"; 105 } 106 } 107 108 public String getSystem() { 109 return "http://hl7.org/fhir/action-selection-behavior"; 110 } 111 112 public String getDefinition() { 113 switch (this) { 114 case ANY: 115 return "Any number of the actions in the group may be chosen, from zero to all."; 116 case ALL: 117 return "All the actions in the group must be selected as a single unit."; 118 case ALLORNONE: 119 return "All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected."; 120 case EXACTLYONE: 121 return "The end user must choose one and only one of the selectable actions in the group. The user SHALL NOT choose none of the actions in the group."; 122 case ATMOSTONE: 123 return "The end user may choose zero or at most one of the actions in the group."; 124 case ONEORMORE: 125 return "The end user must choose a minimum of one, and as many additional as desired."; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getDisplay() { 134 switch (this) { 135 case ANY: 136 return "Any"; 137 case ALL: 138 return "All"; 139 case ALLORNONE: 140 return "All Or None"; 141 case EXACTLYONE: 142 return "Exactly One"; 143 case ATMOSTONE: 144 return "At Most One"; 145 case ONEORMORE: 146 return "One Or More"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154}