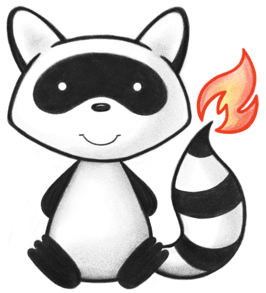
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum AdverseEventCategory { 037 038 /** 039 * The adverse event pertains to a product problem. 040 */ 041 PRODUCTPROBLEM, 042 /** 043 * The adverse event pertains to product quality. 044 */ 045 PRODUCTQUALITY, 046 /** 047 * The adverse event pertains to a product use error. 048 */ 049 PRODUCTUSEERROR, 050 /** 051 * The adverse event pertains to a wrong dose. 052 */ 053 WRONGDOSE, 054 /** 055 * The adverse event pertains to incorrect perscribing information. 056 */ 057 INCORRECTPRESCRIBINGINFORMATION, 058 /** 059 * The adverse event pertains to a wrong technique. 060 */ 061 WRONGTECHNIQUE, 062 /** 063 * The adverse event pertains to a wrong route of administration. 064 */ 065 WRONGROUTEOFADMINISTRATION, 066 /** 067 * The adverse event pertains to a wrong rate. 068 */ 069 WRONGRATE, 070 /** 071 * The adverse event pertains to a wrong duration. 072 */ 073 WRONGDURATION, 074 /** 075 * The adverse event pertains to a wrong time. 076 */ 077 WRONGTIME, 078 /** 079 * The adverse event pertains to an expired drug. 080 */ 081 EXPIREDDRUG, 082 /** 083 * The adverse event pertains to a medical device use error. 084 */ 085 MEDICALDEVICEUSEERROR, 086 /** 087 * The adverse event pertains to a problem with a different manufacturer of the 088 * same medication. 089 */ 090 PROBLEMDIFFERENTMANUFACTURER, 091 /** 092 * The adverse event pertains to an unsafe physical environment. 093 */ 094 UNSAFEPHYSICALENVIRONMENT, 095 /** 096 * added to help the parsers 097 */ 098 NULL; 099 100 public static AdverseEventCategory fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("product-problem".equals(codeString)) 104 return PRODUCTPROBLEM; 105 if ("product-quality".equals(codeString)) 106 return PRODUCTQUALITY; 107 if ("product-use-error".equals(codeString)) 108 return PRODUCTUSEERROR; 109 if ("wrong-dose".equals(codeString)) 110 return WRONGDOSE; 111 if ("incorrect-prescribing-information".equals(codeString)) 112 return INCORRECTPRESCRIBINGINFORMATION; 113 if ("wrong-technique".equals(codeString)) 114 return WRONGTECHNIQUE; 115 if ("wrong-route-of-administration".equals(codeString)) 116 return WRONGROUTEOFADMINISTRATION; 117 if ("wrong-rate".equals(codeString)) 118 return WRONGRATE; 119 if ("wrong-duration".equals(codeString)) 120 return WRONGDURATION; 121 if ("wrong-time".equals(codeString)) 122 return WRONGTIME; 123 if ("expired-drug".equals(codeString)) 124 return EXPIREDDRUG; 125 if ("medical-device-use-error".equals(codeString)) 126 return MEDICALDEVICEUSEERROR; 127 if ("problem-different-manufacturer".equals(codeString)) 128 return PROBLEMDIFFERENTMANUFACTURER; 129 if ("unsafe-physical-environment".equals(codeString)) 130 return UNSAFEPHYSICALENVIRONMENT; 131 throw new FHIRException("Unknown AdverseEventCategory code '" + codeString + "'"); 132 } 133 134 public String toCode() { 135 switch (this) { 136 case PRODUCTPROBLEM: 137 return "product-problem"; 138 case PRODUCTQUALITY: 139 return "product-quality"; 140 case PRODUCTUSEERROR: 141 return "product-use-error"; 142 case WRONGDOSE: 143 return "wrong-dose"; 144 case INCORRECTPRESCRIBINGINFORMATION: 145 return "incorrect-prescribing-information"; 146 case WRONGTECHNIQUE: 147 return "wrong-technique"; 148 case WRONGROUTEOFADMINISTRATION: 149 return "wrong-route-of-administration"; 150 case WRONGRATE: 151 return "wrong-rate"; 152 case WRONGDURATION: 153 return "wrong-duration"; 154 case WRONGTIME: 155 return "wrong-time"; 156 case EXPIREDDRUG: 157 return "expired-drug"; 158 case MEDICALDEVICEUSEERROR: 159 return "medical-device-use-error"; 160 case PROBLEMDIFFERENTMANUFACTURER: 161 return "problem-different-manufacturer"; 162 case UNSAFEPHYSICALENVIRONMENT: 163 return "unsafe-physical-environment"; 164 case NULL: 165 return null; 166 default: 167 return "?"; 168 } 169 } 170 171 public String getSystem() { 172 return "http://terminology.hl7.org/CodeSystem/adverse-event-category"; 173 } 174 175 public String getDefinition() { 176 switch (this) { 177 case PRODUCTPROBLEM: 178 return "The adverse event pertains to a product problem."; 179 case PRODUCTQUALITY: 180 return "The adverse event pertains to product quality."; 181 case PRODUCTUSEERROR: 182 return "The adverse event pertains to a product use error."; 183 case WRONGDOSE: 184 return "The adverse event pertains to a wrong dose."; 185 case INCORRECTPRESCRIBINGINFORMATION: 186 return "The adverse event pertains to incorrect perscribing information."; 187 case WRONGTECHNIQUE: 188 return "The adverse event pertains to a wrong technique."; 189 case WRONGROUTEOFADMINISTRATION: 190 return "The adverse event pertains to a wrong route of administration."; 191 case WRONGRATE: 192 return "The adverse event pertains to a wrong rate."; 193 case WRONGDURATION: 194 return "The adverse event pertains to a wrong duration."; 195 case WRONGTIME: 196 return "The adverse event pertains to a wrong time."; 197 case EXPIREDDRUG: 198 return "The adverse event pertains to an expired drug."; 199 case MEDICALDEVICEUSEERROR: 200 return "The adverse event pertains to a medical device use error."; 201 case PROBLEMDIFFERENTMANUFACTURER: 202 return "The adverse event pertains to a problem with a different manufacturer of the same medication."; 203 case UNSAFEPHYSICALENVIRONMENT: 204 return "The adverse event pertains to an unsafe physical environment."; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212 public String getDisplay() { 213 switch (this) { 214 case PRODUCTPROBLEM: 215 return "Product Problem"; 216 case PRODUCTQUALITY: 217 return "Product Quality"; 218 case PRODUCTUSEERROR: 219 return "Product Use Error"; 220 case WRONGDOSE: 221 return "Wrong Dose"; 222 case INCORRECTPRESCRIBINGINFORMATION: 223 return "Incorrect Prescribing Information"; 224 case WRONGTECHNIQUE: 225 return "Wrong Technique"; 226 case WRONGROUTEOFADMINISTRATION: 227 return "Wrong Route of Administration"; 228 case WRONGRATE: 229 return "Wrong Rate"; 230 case WRONGDURATION: 231 return "Wrong Duration"; 232 case WRONGTIME: 233 return "Wrong Time"; 234 case EXPIREDDRUG: 235 return "Expired Drug"; 236 case MEDICALDEVICEUSEERROR: 237 return "Medical Device Use Error"; 238 case PROBLEMDIFFERENTMANUFACTURER: 239 return "Problem with Different Manufacturer of Same Medicine"; 240 case UNSAFEPHYSICALENVIRONMENT: 241 return "Unsafe Physical Environment"; 242 case NULL: 243 return null; 244 default: 245 return "?"; 246 } 247 } 248 249}