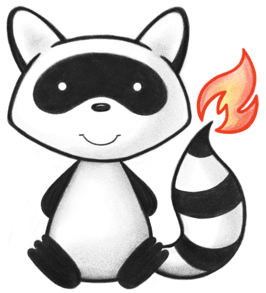
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum AllergyIntoleranceCriticality { 037 038 /** 039 * Worst case result of a future exposure is not assessed to be life-threatening 040 * or having high potential for organ system failure. 041 */ 042 LOW, 043 /** 044 * Worst case result of a future exposure is assessed to be life-threatening or 045 * having high potential for organ system failure. 046 */ 047 HIGH, 048 /** 049 * Unable to assess the worst case result of a future exposure. 050 */ 051 UNABLETOASSESS, 052 /** 053 * added to help the parsers 054 */ 055 NULL; 056 057 public static AllergyIntoleranceCriticality fromCode(String codeString) throws FHIRException { 058 if (codeString == null || "".equals(codeString)) 059 return null; 060 if ("low".equals(codeString)) 061 return LOW; 062 if ("high".equals(codeString)) 063 return HIGH; 064 if ("unable-to-assess".equals(codeString)) 065 return UNABLETOASSESS; 066 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 067 } 068 069 public String toCode() { 070 switch (this) { 071 case LOW: 072 return "low"; 073 case HIGH: 074 return "high"; 075 case UNABLETOASSESS: 076 return "unable-to-assess"; 077 case NULL: 078 return null; 079 default: 080 return "?"; 081 } 082 } 083 084 public String getSystem() { 085 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 086 } 087 088 public String getDefinition() { 089 switch (this) { 090 case LOW: 091 return "Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure."; 092 case HIGH: 093 return "Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure."; 094 case UNABLETOASSESS: 095 return "Unable to assess the worst case result of a future exposure."; 096 case NULL: 097 return null; 098 default: 099 return "?"; 100 } 101 } 102 103 public String getDisplay() { 104 switch (this) { 105 case LOW: 106 return "Low Risk"; 107 case HIGH: 108 return "High Risk"; 109 case UNABLETOASSESS: 110 return "Unable to Assess Risk"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118}