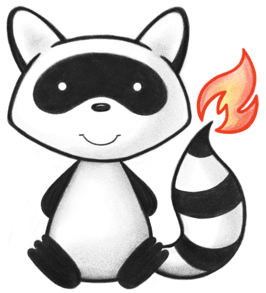
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum AppointmentCancellationReason { 037 038 /** 039 * null 040 */ 041 PAT, 042 /** 043 * null 044 */ 045 PATCRS, 046 /** 047 * null 048 */ 049 PATCPP, 050 /** 051 * null 052 */ 053 PATDEC, 054 /** 055 * null 056 */ 057 PATFB, 058 /** 059 * null 060 */ 061 PATLT, 062 /** 063 * null 064 */ 065 PATMT, 066 /** 067 * null 068 */ 069 PATMV, 070 /** 071 * null 072 */ 073 PATPREG, 074 /** 075 * null 076 */ 077 PATSWL, 078 /** 079 * null 080 */ 081 PATUCP, 082 /** 083 * null 084 */ 085 PROV, 086 /** 087 * null 088 */ 089 PROVPERS, 090 /** 091 * null 092 */ 093 PROVDCH, 094 /** 095 * null 096 */ 097 PROVEDU, 098 /** 099 * null 100 */ 101 PROVHOSP, 102 /** 103 * null 104 */ 105 PROVLABS, 106 /** 107 * null 108 */ 109 PROVMRI, 110 /** 111 * null 112 */ 113 PROVONC, 114 /** 115 * null 116 */ 117 MAINT, 118 /** 119 * null 120 */ 121 MEDSINC, 122 /** 123 * null 124 */ 125 OTHER, 126 /** 127 * null 128 */ 129 OTHCMS, 130 /** 131 * null 132 */ 133 OTHERR, 134 /** 135 * null 136 */ 137 OTHFIN, 138 /** 139 * null 140 */ 141 OTHIV, 142 /** 143 * null 144 */ 145 OTHINT, 146 /** 147 * null 148 */ 149 OTHMU, 150 /** 151 * null 152 */ 153 OTHROOM, 154 /** 155 * null 156 */ 157 OTHOERR, 158 /** 159 * null 160 */ 161 OTHSWIE, 162 /** 163 * null 164 */ 165 OTHWEATH, 166 /** 167 * added to help the parsers 168 */ 169 NULL; 170 171 public static AppointmentCancellationReason fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("pat".equals(codeString)) 175 return PAT; 176 if ("pat-crs".equals(codeString)) 177 return PATCRS; 178 if ("pat-cpp".equals(codeString)) 179 return PATCPP; 180 if ("pat-dec".equals(codeString)) 181 return PATDEC; 182 if ("pat-fb".equals(codeString)) 183 return PATFB; 184 if ("pat-lt".equals(codeString)) 185 return PATLT; 186 if ("pat-mt".equals(codeString)) 187 return PATMT; 188 if ("pat-mv".equals(codeString)) 189 return PATMV; 190 if ("pat-preg".equals(codeString)) 191 return PATPREG; 192 if ("pat-swl".equals(codeString)) 193 return PATSWL; 194 if ("pat-ucp".equals(codeString)) 195 return PATUCP; 196 if ("prov".equals(codeString)) 197 return PROV; 198 if ("prov-pers".equals(codeString)) 199 return PROVPERS; 200 if ("prov-dch".equals(codeString)) 201 return PROVDCH; 202 if ("prov-edu".equals(codeString)) 203 return PROVEDU; 204 if ("prov-hosp".equals(codeString)) 205 return PROVHOSP; 206 if ("prov-labs".equals(codeString)) 207 return PROVLABS; 208 if ("prov-mri".equals(codeString)) 209 return PROVMRI; 210 if ("prov-onc".equals(codeString)) 211 return PROVONC; 212 if ("maint".equals(codeString)) 213 return MAINT; 214 if ("meds-inc".equals(codeString)) 215 return MEDSINC; 216 if ("other".equals(codeString)) 217 return OTHER; 218 if ("oth-cms".equals(codeString)) 219 return OTHCMS; 220 if ("oth-err".equals(codeString)) 221 return OTHERR; 222 if ("oth-fin".equals(codeString)) 223 return OTHFIN; 224 if ("oth-iv".equals(codeString)) 225 return OTHIV; 226 if ("oth-int".equals(codeString)) 227 return OTHINT; 228 if ("oth-mu".equals(codeString)) 229 return OTHMU; 230 if ("oth-room".equals(codeString)) 231 return OTHROOM; 232 if ("oth-oerr".equals(codeString)) 233 return OTHOERR; 234 if ("oth-swie".equals(codeString)) 235 return OTHSWIE; 236 if ("oth-weath".equals(codeString)) 237 return OTHWEATH; 238 throw new FHIRException("Unknown AppointmentCancellationReason code '" + codeString + "'"); 239 } 240 241 public String toCode() { 242 switch (this) { 243 case PAT: 244 return "pat"; 245 case PATCRS: 246 return "pat-crs"; 247 case PATCPP: 248 return "pat-cpp"; 249 case PATDEC: 250 return "pat-dec"; 251 case PATFB: 252 return "pat-fb"; 253 case PATLT: 254 return "pat-lt"; 255 case PATMT: 256 return "pat-mt"; 257 case PATMV: 258 return "pat-mv"; 259 case PATPREG: 260 return "pat-preg"; 261 case PATSWL: 262 return "pat-swl"; 263 case PATUCP: 264 return "pat-ucp"; 265 case PROV: 266 return "prov"; 267 case PROVPERS: 268 return "prov-pers"; 269 case PROVDCH: 270 return "prov-dch"; 271 case PROVEDU: 272 return "prov-edu"; 273 case PROVHOSP: 274 return "prov-hosp"; 275 case PROVLABS: 276 return "prov-labs"; 277 case PROVMRI: 278 return "prov-mri"; 279 case PROVONC: 280 return "prov-onc"; 281 case MAINT: 282 return "maint"; 283 case MEDSINC: 284 return "meds-inc"; 285 case OTHER: 286 return "other"; 287 case OTHCMS: 288 return "oth-cms"; 289 case OTHERR: 290 return "oth-err"; 291 case OTHFIN: 292 return "oth-fin"; 293 case OTHIV: 294 return "oth-iv"; 295 case OTHINT: 296 return "oth-int"; 297 case OTHMU: 298 return "oth-mu"; 299 case OTHROOM: 300 return "oth-room"; 301 case OTHOERR: 302 return "oth-oerr"; 303 case OTHSWIE: 304 return "oth-swie"; 305 case OTHWEATH: 306 return "oth-weath"; 307 case NULL: 308 return null; 309 default: 310 return "?"; 311 } 312 } 313 314 public String getSystem() { 315 return "http://terminology.hl7.org/CodeSystem/appointment-cancellation-reason"; 316 } 317 318 public String getDefinition() { 319 switch (this) { 320 case PAT: 321 return ""; 322 case PATCRS: 323 return ""; 324 case PATCPP: 325 return ""; 326 case PATDEC: 327 return ""; 328 case PATFB: 329 return ""; 330 case PATLT: 331 return ""; 332 case PATMT: 333 return ""; 334 case PATMV: 335 return ""; 336 case PATPREG: 337 return ""; 338 case PATSWL: 339 return ""; 340 case PATUCP: 341 return ""; 342 case PROV: 343 return ""; 344 case PROVPERS: 345 return ""; 346 case PROVDCH: 347 return ""; 348 case PROVEDU: 349 return ""; 350 case PROVHOSP: 351 return ""; 352 case PROVLABS: 353 return ""; 354 case PROVMRI: 355 return ""; 356 case PROVONC: 357 return ""; 358 case MAINT: 359 return ""; 360 case MEDSINC: 361 return ""; 362 case OTHER: 363 return ""; 364 case OTHCMS: 365 return ""; 366 case OTHERR: 367 return ""; 368 case OTHFIN: 369 return ""; 370 case OTHIV: 371 return ""; 372 case OTHINT: 373 return ""; 374 case OTHMU: 375 return ""; 376 case OTHROOM: 377 return ""; 378 case OTHOERR: 379 return ""; 380 case OTHSWIE: 381 return ""; 382 case OTHWEATH: 383 return ""; 384 case NULL: 385 return null; 386 default: 387 return "?"; 388 } 389 } 390 391 public String getDisplay() { 392 switch (this) { 393 case PAT: 394 return "Patient"; 395 case PATCRS: 396 return "Patient: Canceled via automated reminder system"; 397 case PATCPP: 398 return "Patient: Canceled via Patient Portal"; 399 case PATDEC: 400 return "Patient: Deceased"; 401 case PATFB: 402 return "Patient: Feeling Better"; 403 case PATLT: 404 return "Patient: Lack of Transportation"; 405 case PATMT: 406 return "Patient: Member Terminated"; 407 case PATMV: 408 return "Patient: Moved"; 409 case PATPREG: 410 return "Patient: Pregnant"; 411 case PATSWL: 412 return "Patient: Scheduled from Wait List"; 413 case PATUCP: 414 return "Patient: Unhappy/Changed Provider"; 415 case PROV: 416 return "Provider"; 417 case PROVPERS: 418 return "Provider: Personal"; 419 case PROVDCH: 420 return "Provider: Discharged"; 421 case PROVEDU: 422 return "Provider: Edu/Meeting"; 423 case PROVHOSP: 424 return "Provider: Hospitalized"; 425 case PROVLABS: 426 return "Provider: Labs Out of Acceptable Range"; 427 case PROVMRI: 428 return "Provider: MRI Screening Form Marked Do Not Proceed"; 429 case PROVONC: 430 return "Provider: Oncology Treatment Plan Changes"; 431 case MAINT: 432 return "Equipment Maintenance/Repair"; 433 case MEDSINC: 434 return "Prep/Med Incomplete"; 435 case OTHER: 436 return "Other"; 437 case OTHCMS: 438 return "Other: CMS Therapy Cap Service Not Authorized"; 439 case OTHERR: 440 return "Other: Error"; 441 case OTHFIN: 442 return "Other: Financial"; 443 case OTHIV: 444 return "Other: Improper IV Access/Infiltrate IV"; 445 case OTHINT: 446 return "Other: No Interpreter Available"; 447 case OTHMU: 448 return "Other: Prep/Med/Results Unavailable"; 449 case OTHROOM: 450 return "Other: Room/Resource Maintenance"; 451 case OTHOERR: 452 return "Other: Schedule Order Error"; 453 case OTHSWIE: 454 return "Other: Silent Walk In Error"; 455 case OTHWEATH: 456 return "Other: Weather"; 457 case NULL: 458 return null; 459 default: 460 return "?"; 461 } 462 } 463 464}