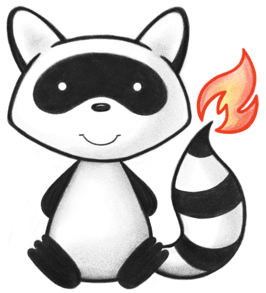
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum AssertResponseCodeTypes { 037 038 /** 039 * Response code is 200. 040 */ 041 OKAY, 042 /** 043 * Response code is 201. 044 */ 045 CREATED, 046 /** 047 * Response code is 204. 048 */ 049 NOCONTENT, 050 /** 051 * Response code is 304. 052 */ 053 NOTMODIFIED, 054 /** 055 * Response code is 400. 056 */ 057 BAD, 058 /** 059 * Response code is 403. 060 */ 061 FORBIDDEN, 062 /** 063 * Response code is 404. 064 */ 065 NOTFOUND, 066 /** 067 * Response code is 405. 068 */ 069 METHODNOTALLOWED, 070 /** 071 * Response code is 409. 072 */ 073 CONFLICT, 074 /** 075 * Response code is 410. 076 */ 077 GONE, 078 /** 079 * Response code is 412. 080 */ 081 PRECONDITIONFAILED, 082 /** 083 * Response code is 422. 084 */ 085 UNPROCESSABLE, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 091 public static AssertResponseCodeTypes fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("okay".equals(codeString)) 095 return OKAY; 096 if ("created".equals(codeString)) 097 return CREATED; 098 if ("noContent".equals(codeString)) 099 return NOCONTENT; 100 if ("notModified".equals(codeString)) 101 return NOTMODIFIED; 102 if ("bad".equals(codeString)) 103 return BAD; 104 if ("forbidden".equals(codeString)) 105 return FORBIDDEN; 106 if ("notFound".equals(codeString)) 107 return NOTFOUND; 108 if ("methodNotAllowed".equals(codeString)) 109 return METHODNOTALLOWED; 110 if ("conflict".equals(codeString)) 111 return CONFLICT; 112 if ("gone".equals(codeString)) 113 return GONE; 114 if ("preconditionFailed".equals(codeString)) 115 return PRECONDITIONFAILED; 116 if ("unprocessable".equals(codeString)) 117 return UNPROCESSABLE; 118 throw new FHIRException("Unknown AssertResponseCodeTypes code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case OKAY: 124 return "okay"; 125 case CREATED: 126 return "created"; 127 case NOCONTENT: 128 return "noContent"; 129 case NOTMODIFIED: 130 return "notModified"; 131 case BAD: 132 return "bad"; 133 case FORBIDDEN: 134 return "forbidden"; 135 case NOTFOUND: 136 return "notFound"; 137 case METHODNOTALLOWED: 138 return "methodNotAllowed"; 139 case CONFLICT: 140 return "conflict"; 141 case GONE: 142 return "gone"; 143 case PRECONDITIONFAILED: 144 return "preconditionFailed"; 145 case UNPROCESSABLE: 146 return "unprocessable"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 return "http://hl7.org/fhir/assert-response-code-types"; 156 } 157 158 public String getDefinition() { 159 switch (this) { 160 case OKAY: 161 return "Response code is 200."; 162 case CREATED: 163 return "Response code is 201."; 164 case NOCONTENT: 165 return "Response code is 204."; 166 case NOTMODIFIED: 167 return "Response code is 304."; 168 case BAD: 169 return "Response code is 400."; 170 case FORBIDDEN: 171 return "Response code is 403."; 172 case NOTFOUND: 173 return "Response code is 404."; 174 case METHODNOTALLOWED: 175 return "Response code is 405."; 176 case CONFLICT: 177 return "Response code is 409."; 178 case GONE: 179 return "Response code is 410."; 180 case PRECONDITIONFAILED: 181 return "Response code is 412."; 182 case UNPROCESSABLE: 183 return "Response code is 422."; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191 public String getDisplay() { 192 switch (this) { 193 case OKAY: 194 return "okay"; 195 case CREATED: 196 return "created"; 197 case NOCONTENT: 198 return "noContent"; 199 case NOTMODIFIED: 200 return "notModified"; 201 case BAD: 202 return "bad"; 203 case FORBIDDEN: 204 return "forbidden"; 205 case NOTFOUND: 206 return "notFound"; 207 case METHODNOTALLOWED: 208 return "methodNotAllowed"; 209 case CONFLICT: 210 return "conflict"; 211 case GONE: 212 return "gone"; 213 case PRECONDITIONFAILED: 214 return "preconditionFailed"; 215 case UNPROCESSABLE: 216 return "unprocessable"; 217 case NULL: 218 return null; 219 default: 220 return "?"; 221 } 222 } 223 224}