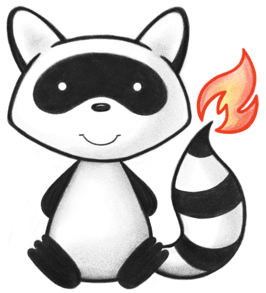
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum BundleType { 037 038 /** 039 * The bundle is a document. The first resource is a Composition. 040 */ 041 DOCUMENT, 042 /** 043 * The bundle is a message. The first resource is a MessageHeader. 044 */ 045 MESSAGE, 046 /** 047 * The bundle is a transaction - intended to be processed by a server as an 048 * atomic commit. 049 */ 050 TRANSACTION, 051 /** 052 * The bundle is a transaction response. Because the response is a transaction 053 * response, the transaction has succeeded, and all responses are error free. 054 */ 055 TRANSACTIONRESPONSE, 056 /** 057 * The bundle is a set of actions - intended to be processed by a server as a 058 * group of independent actions. 059 */ 060 BATCH, 061 /** 062 * The bundle is a batch response. Note that as a batch, some responses may 063 * indicate failure and others success. 064 */ 065 BATCHRESPONSE, 066 /** 067 * The bundle is a list of resources from a history interaction on a server. 068 */ 069 HISTORY, 070 /** 071 * The bundle is a list of resources returned as a result of a search/query 072 * interaction, operation, or message. 073 */ 074 SEARCHSET, 075 /** 076 * The bundle is a set of resources collected into a single package for ease of 077 * distribution that imposes no processing obligations or behavioral rules 078 * beyond persistence. 079 */ 080 COLLECTION, 081 /** 082 * added to help the parsers 083 */ 084 NULL; 085 086 public static BundleType fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("document".equals(codeString)) 090 return DOCUMENT; 091 if ("message".equals(codeString)) 092 return MESSAGE; 093 if ("transaction".equals(codeString)) 094 return TRANSACTION; 095 if ("transaction-response".equals(codeString)) 096 return TRANSACTIONRESPONSE; 097 if ("batch".equals(codeString)) 098 return BATCH; 099 if ("batch-response".equals(codeString)) 100 return BATCHRESPONSE; 101 if ("history".equals(codeString)) 102 return HISTORY; 103 if ("searchset".equals(codeString)) 104 return SEARCHSET; 105 if ("collection".equals(codeString)) 106 return COLLECTION; 107 throw new FHIRException("Unknown BundleType code '" + codeString + "'"); 108 } 109 110 public String toCode() { 111 switch (this) { 112 case DOCUMENT: 113 return "document"; 114 case MESSAGE: 115 return "message"; 116 case TRANSACTION: 117 return "transaction"; 118 case TRANSACTIONRESPONSE: 119 return "transaction-response"; 120 case BATCH: 121 return "batch"; 122 case BATCHRESPONSE: 123 return "batch-response"; 124 case HISTORY: 125 return "history"; 126 case SEARCHSET: 127 return "searchset"; 128 case COLLECTION: 129 return "collection"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getSystem() { 138 return "http://hl7.org/fhir/bundle-type"; 139 } 140 141 public String getDefinition() { 142 switch (this) { 143 case DOCUMENT: 144 return "The bundle is a document. The first resource is a Composition."; 145 case MESSAGE: 146 return "The bundle is a message. The first resource is a MessageHeader."; 147 case TRANSACTION: 148 return "The bundle is a transaction - intended to be processed by a server as an atomic commit."; 149 case TRANSACTIONRESPONSE: 150 return "The bundle is a transaction response. Because the response is a transaction response, the transaction has succeeded, and all responses are error free."; 151 case BATCH: 152 return "The bundle is a set of actions - intended to be processed by a server as a group of independent actions."; 153 case BATCHRESPONSE: 154 return "The bundle is a batch response. Note that as a batch, some responses may indicate failure and others success."; 155 case HISTORY: 156 return "The bundle is a list of resources from a history interaction on a server."; 157 case SEARCHSET: 158 return "The bundle is a list of resources returned as a result of a search/query interaction, operation, or message."; 159 case COLLECTION: 160 return "The bundle is a set of resources collected into a single package for ease of distribution that imposes no processing obligations or behavioral rules beyond persistence."; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDisplay() { 169 switch (this) { 170 case DOCUMENT: 171 return "Document"; 172 case MESSAGE: 173 return "Message"; 174 case TRANSACTION: 175 return "Transaction"; 176 case TRANSACTIONRESPONSE: 177 return "Transaction Response"; 178 case BATCH: 179 return "Batch"; 180 case BATCHRESPONSE: 181 return "Batch Response"; 182 case HISTORY: 183 return "History List"; 184 case SEARCHSET: 185 return "Search Results"; 186 case COLLECTION: 187 return "Collection"; 188 case NULL: 189 return null; 190 default: 191 return "?"; 192 } 193 } 194 195}