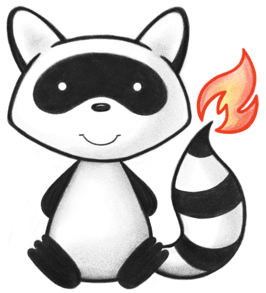
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum CarePlanActivityStatus { 037 038 /** 039 * Care plan activity is planned but no action has yet been taken. 040 */ 041 NOTSTARTED, 042 /** 043 * Appointment or other booking has occurred but activity has not yet begun. 044 */ 045 SCHEDULED, 046 /** 047 * Care plan activity has been started but is not yet complete. 048 */ 049 INPROGRESS, 050 /** 051 * Care plan activity was started but has temporarily ceased with an expectation 052 * of resumption at a future time. 053 */ 054 ONHOLD, 055 /** 056 * Care plan activity has been completed (more or less) as planned. 057 */ 058 COMPLETED, 059 /** 060 * The planned care plan activity has been withdrawn. 061 */ 062 CANCELLED, 063 /** 064 * The planned care plan activity has been ended prior to completion after the 065 * activity was started. 066 */ 067 STOPPED, 068 /** 069 * The current state of the care plan activity is not known. Note: This concept 070 * is not to be used for "other" - one of the listed statuses is presumed to 071 * apply, but the authoring/source system does not know which one. 072 */ 073 UNKNOWN, 074 /** 075 * Care plan activity was entered in error and voided. 076 */ 077 ENTEREDINERROR, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 083 public static CarePlanActivityStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("not-started".equals(codeString)) 087 return NOTSTARTED; 088 if ("scheduled".equals(codeString)) 089 return SCHEDULED; 090 if ("in-progress".equals(codeString)) 091 return INPROGRESS; 092 if ("on-hold".equals(codeString)) 093 return ONHOLD; 094 if ("completed".equals(codeString)) 095 return COMPLETED; 096 if ("cancelled".equals(codeString)) 097 return CANCELLED; 098 if ("stopped".equals(codeString)) 099 return STOPPED; 100 if ("unknown".equals(codeString)) 101 return UNKNOWN; 102 if ("entered-in-error".equals(codeString)) 103 return ENTEREDINERROR; 104 throw new FHIRException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 105 } 106 107 public String toCode() { 108 switch (this) { 109 case NOTSTARTED: 110 return "not-started"; 111 case SCHEDULED: 112 return "scheduled"; 113 case INPROGRESS: 114 return "in-progress"; 115 case ONHOLD: 116 return "on-hold"; 117 case COMPLETED: 118 return "completed"; 119 case CANCELLED: 120 return "cancelled"; 121 case STOPPED: 122 return "stopped"; 123 case UNKNOWN: 124 return "unknown"; 125 case ENTEREDINERROR: 126 return "entered-in-error"; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getSystem() { 135 return "http://hl7.org/fhir/care-plan-activity-status"; 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case NOTSTARTED: 141 return "Care plan activity is planned but no action has yet been taken."; 142 case SCHEDULED: 143 return "Appointment or other booking has occurred but activity has not yet begun."; 144 case INPROGRESS: 145 return "Care plan activity has been started but is not yet complete."; 146 case ONHOLD: 147 return "Care plan activity was started but has temporarily ceased with an expectation of resumption at a future time."; 148 case COMPLETED: 149 return "Care plan activity has been completed (more or less) as planned."; 150 case CANCELLED: 151 return "The planned care plan activity has been withdrawn."; 152 case STOPPED: 153 return "The planned care plan activity has been ended prior to completion after the activity was started."; 154 case UNKNOWN: 155 return "The current state of the care plan activity is not known. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which one."; 156 case ENTEREDINERROR: 157 return "Care plan activity was entered in error and voided."; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165 public String getDisplay() { 166 switch (this) { 167 case NOTSTARTED: 168 return "Not Started"; 169 case SCHEDULED: 170 return "Scheduled"; 171 case INPROGRESS: 172 return "In Progress"; 173 case ONHOLD: 174 return "On Hold"; 175 case COMPLETED: 176 return "Completed"; 177 case CANCELLED: 178 return "Cancelled"; 179 case STOPPED: 180 return "Stopped"; 181 case UNKNOWN: 182 return "Unknown"; 183 case ENTEREDINERROR: 184 return "Entered in Error"; 185 case NULL: 186 return null; 187 default: 188 return "?"; 189 } 190 } 191 192}