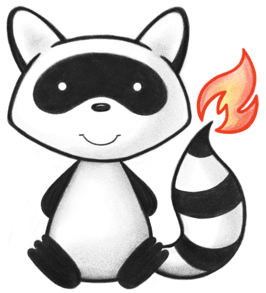
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum CertaintySubcomponentRating { 037 038 /** 039 * no change to quality rating. 040 */ 041 NOCHANGE, 042 /** 043 * reduce quality rating by 1. 044 */ 045 DOWNCODE1, 046 /** 047 * reduce quality rating by 2. 048 */ 049 DOWNCODE2, 050 /** 051 * reduce quality rating by 3. 052 */ 053 DOWNCODE3, 054 /** 055 * increase quality rating by 1. 056 */ 057 UPCODE1, 058 /** 059 * increase quality rating by 2. 060 */ 061 UPCODE2, 062 /** 063 * no serious concern. 064 */ 065 NOCONCERN, 066 /** 067 * serious concern. 068 */ 069 SERIOUSCONCERN, 070 /** 071 * critical concern. 072 */ 073 CRITICALCONCERN, 074 /** 075 * possible reason for increasing quality rating was checked and found to 076 * bepresent. 077 */ 078 PRESENT, 079 /** 080 * possible reason for increasing quality rating was checked and found to be 081 * absent. 082 */ 083 ABSENT, 084 /** 085 * added to help the parsers 086 */ 087 NULL; 088 089 public static CertaintySubcomponentRating fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("no-change".equals(codeString)) 093 return NOCHANGE; 094 if ("downcode1".equals(codeString)) 095 return DOWNCODE1; 096 if ("downcode2".equals(codeString)) 097 return DOWNCODE2; 098 if ("downcode3".equals(codeString)) 099 return DOWNCODE3; 100 if ("upcode1".equals(codeString)) 101 return UPCODE1; 102 if ("upcode2".equals(codeString)) 103 return UPCODE2; 104 if ("no-concern".equals(codeString)) 105 return NOCONCERN; 106 if ("serious-concern".equals(codeString)) 107 return SERIOUSCONCERN; 108 if ("critical-concern".equals(codeString)) 109 return CRITICALCONCERN; 110 if ("present".equals(codeString)) 111 return PRESENT; 112 if ("absent".equals(codeString)) 113 return ABSENT; 114 throw new FHIRException("Unknown CertaintySubcomponentRating code '" + codeString + "'"); 115 } 116 117 public String toCode() { 118 switch (this) { 119 case NOCHANGE: 120 return "no-change"; 121 case DOWNCODE1: 122 return "downcode1"; 123 case DOWNCODE2: 124 return "downcode2"; 125 case DOWNCODE3: 126 return "downcode3"; 127 case UPCODE1: 128 return "upcode1"; 129 case UPCODE2: 130 return "upcode2"; 131 case NOCONCERN: 132 return "no-concern"; 133 case SERIOUSCONCERN: 134 return "serious-concern"; 135 case CRITICALCONCERN: 136 return "critical-concern"; 137 case PRESENT: 138 return "present"; 139 case ABSENT: 140 return "absent"; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 148 public String getSystem() { 149 return "http://terminology.hl7.org/CodeSystem/certainty-subcomponent-rating"; 150 } 151 152 public String getDefinition() { 153 switch (this) { 154 case NOCHANGE: 155 return "no change to quality rating."; 156 case DOWNCODE1: 157 return "reduce quality rating by 1."; 158 case DOWNCODE2: 159 return "reduce quality rating by 2."; 160 case DOWNCODE3: 161 return "reduce quality rating by 3."; 162 case UPCODE1: 163 return "increase quality rating by 1."; 164 case UPCODE2: 165 return "increase quality rating by 2."; 166 case NOCONCERN: 167 return "no serious concern."; 168 case SERIOUSCONCERN: 169 return "serious concern."; 170 case CRITICALCONCERN: 171 return "critical concern."; 172 case PRESENT: 173 return "possible reason for increasing quality rating was checked and found to bepresent."; 174 case ABSENT: 175 return "possible reason for increasing quality rating was checked and found to be absent."; 176 case NULL: 177 return null; 178 default: 179 return "?"; 180 } 181 } 182 183 public String getDisplay() { 184 switch (this) { 185 case NOCHANGE: 186 return "no change to rating"; 187 case DOWNCODE1: 188 return "reduce rating: -1"; 189 case DOWNCODE2: 190 return "reduce rating: -2"; 191 case DOWNCODE3: 192 return "reduce rating: -3"; 193 case UPCODE1: 194 return "increase rating: +1"; 195 case UPCODE2: 196 return "increase rating: +2"; 197 case NOCONCERN: 198 return "no serious concern"; 199 case SERIOUSCONCERN: 200 return "serious concern"; 201 case CRITICALCONCERN: 202 return "critical concern"; 203 case PRESENT: 204 return "present"; 205 case ABSENT: 206 return "absent"; 207 case NULL: 208 return null; 209 default: 210 return "?"; 211 } 212 } 213 214}