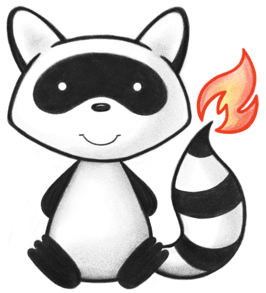
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ChargeitemStatus { 037 038 /** 039 * The charge item has been entered, but the charged service is not yet 040 * complete, so it shall not be billed yet but might be used in the context of 041 * pre-authorization. 042 */ 043 PLANNED, 044 /** 045 * The charge item is ready for billing. 046 */ 047 BILLABLE, 048 /** 049 * The charge item has been determined to be not billable (e.g. due to rules 050 * associated with the billing code). 051 */ 052 NOTBILLABLE, 053 /** 054 * The processing of the charge was aborted. 055 */ 056 ABORTED, 057 /** 058 * The charge item has been billed (e.g. a billing engine has generated 059 * financial transactions by applying the associated ruled for the charge item 060 * to the context of the Encounter, and placed them into Claims/Invoices. 061 */ 062 BILLED, 063 /** 064 * The charge item has been entered in error and should not be processed for 065 * billing. 066 */ 067 ENTEREDINERROR, 068 /** 069 * The authoring system does not know which of the status values currently 070 * applies for this charge item Note: This concept is not to be used for "other" 071 * - one of the listed statuses is presumed to apply, it's just not known which 072 * one. 073 */ 074 UNKNOWN, 075 /** 076 * added to help the parsers 077 */ 078 NULL; 079 080 public static ChargeitemStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("planned".equals(codeString)) 084 return PLANNED; 085 if ("billable".equals(codeString)) 086 return BILLABLE; 087 if ("not-billable".equals(codeString)) 088 return NOTBILLABLE; 089 if ("aborted".equals(codeString)) 090 return ABORTED; 091 if ("billed".equals(codeString)) 092 return BILLED; 093 if ("entered-in-error".equals(codeString)) 094 return ENTEREDINERROR; 095 if ("unknown".equals(codeString)) 096 return UNKNOWN; 097 throw new FHIRException("Unknown ChargeitemStatus code '" + codeString + "'"); 098 } 099 100 public String toCode() { 101 switch (this) { 102 case PLANNED: 103 return "planned"; 104 case BILLABLE: 105 return "billable"; 106 case NOTBILLABLE: 107 return "not-billable"; 108 case ABORTED: 109 return "aborted"; 110 case BILLED: 111 return "billed"; 112 case ENTEREDINERROR: 113 return "entered-in-error"; 114 case UNKNOWN: 115 return "unknown"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 return "http://hl7.org/fhir/chargeitem-status"; 125 } 126 127 public String getDefinition() { 128 switch (this) { 129 case PLANNED: 130 return "The charge item has been entered, but the charged service is not yet complete, so it shall not be billed yet but might be used in the context of pre-authorization."; 131 case BILLABLE: 132 return "The charge item is ready for billing."; 133 case NOTBILLABLE: 134 return "The charge item has been determined to be not billable (e.g. due to rules associated with the billing code)."; 135 case ABORTED: 136 return "The processing of the charge was aborted."; 137 case BILLED: 138 return "The charge item has been billed (e.g. a billing engine has generated financial transactions by applying the associated ruled for the charge item to the context of the Encounter, and placed them into Claims/Invoices."; 139 case ENTEREDINERROR: 140 return "The charge item has been entered in error and should not be processed for billing."; 141 case UNKNOWN: 142 return "The authoring system does not know which of the status values currently applies for this charge item Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 143 case NULL: 144 return null; 145 default: 146 return "?"; 147 } 148 } 149 150 public String getDisplay() { 151 switch (this) { 152 case PLANNED: 153 return "Planned"; 154 case BILLABLE: 155 return "Billable"; 156 case NOTBILLABLE: 157 return "Not billable"; 158 case ABORTED: 159 return "Aborted"; 160 case BILLED: 161 return "Billed"; 162 case ENTEREDINERROR: 163 return "Entered in Error"; 164 case UNKNOWN: 165 return "Unknown"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 173}