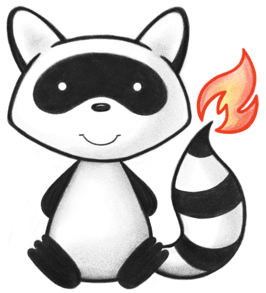
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum CompositionAltcodeKind { 037 038 /** 039 * The code is an alternative code that can be used in any of the circumstances 040 * that the primary code can be used. 041 */ 042 ALTERNATE, 043 /** 044 * The code should no longer be used, but was used in the past. 045 */ 046 DEPRECATED, 047 /** 048 * The code is an alternative to be used when a case insensitive code is 049 * required (when the primary codes are case sensitive). 050 */ 051 CASEINSENSITIVE, 052 /** 053 * The code is an alternative to be used when a case sensitive code is required 054 * (when the primary codes are case insensitive). 055 */ 056 CASESENSITIVE, 057 /** 058 * The code is an alternative for the primary code that is built using the 059 * expression grammar defined by the code system. 060 */ 061 EXPRESSION, 062 /** 063 * added to help the parsers 064 */ 065 NULL; 066 067 public static CompositionAltcodeKind fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("alternate".equals(codeString)) 071 return ALTERNATE; 072 if ("deprecated".equals(codeString)) 073 return DEPRECATED; 074 if ("case-insensitive".equals(codeString)) 075 return CASEINSENSITIVE; 076 if ("case-sensitive".equals(codeString)) 077 return CASESENSITIVE; 078 if ("expression".equals(codeString)) 079 return EXPRESSION; 080 throw new FHIRException("Unknown CompositionAltcodeKind code '" + codeString + "'"); 081 } 082 083 public String toCode() { 084 switch (this) { 085 case ALTERNATE: 086 return "alternate"; 087 case DEPRECATED: 088 return "deprecated"; 089 case CASEINSENSITIVE: 090 return "case-insensitive"; 091 case CASESENSITIVE: 092 return "case-sensitive"; 093 case EXPRESSION: 094 return "expression"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 return "http://terminology.hl7.org/CodeSystem/composition-altcode-kind"; 104 } 105 106 public String getDefinition() { 107 switch (this) { 108 case ALTERNATE: 109 return "The code is an alternative code that can be used in any of the circumstances that the primary code can be used."; 110 case DEPRECATED: 111 return "The code should no longer be used, but was used in the past."; 112 case CASEINSENSITIVE: 113 return "The code is an alternative to be used when a case insensitive code is required (when the primary codes are case sensitive)."; 114 case CASESENSITIVE: 115 return "The code is an alternative to be used when a case sensitive code is required (when the primary codes are case insensitive)."; 116 case EXPRESSION: 117 return "The code is an alternative for the primary code that is built using the expression grammar defined by the code system."; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getDisplay() { 126 switch (this) { 127 case ALTERNATE: 128 return "Alternate Code"; 129 case DEPRECATED: 130 return "Deprecated"; 131 case CASEINSENSITIVE: 132 return "Case Insensitive"; 133 case CASESENSITIVE: 134 return "Case Sensitive"; 135 case EXPRESSION: 136 return "Expression"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144}